You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@nuttx.apache.org by GitBox <gi...@apache.org> on 2022/08/23 06:20:58 UTC
[GitHub] [incubator-nuttx] anchao opened a new pull request, #6897: arch/sim: add windows host simulate support
anchao opened a new pull request, #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897
## Summary
arch/sim: add windows host simulate support
sim/host: move host implement to posix directory
This PR is depends on cmake support:
https://github.com/apache/incubator-nuttx/pull/6718
Preview version is on private project, will be contributed to the community asap:
```
git clone git@github.com:anchao/incubator-nuttx.git -b windows
git clone git@github.com:anchao/incubator-nuttx-apps.git -b windows
mv incubator-nuttx-apps apps
cd incubator-nuttx
```
Compile with cmake:
```
cmake -B vs2022 -DBOARD_CONFIG=sim/windows -G"Visual Studio 17 2022" -A Win32
cmake --build vs2022
```
If you want to use Visual Studio, you can import the solution directly from the IDE after CMake generates the solution:
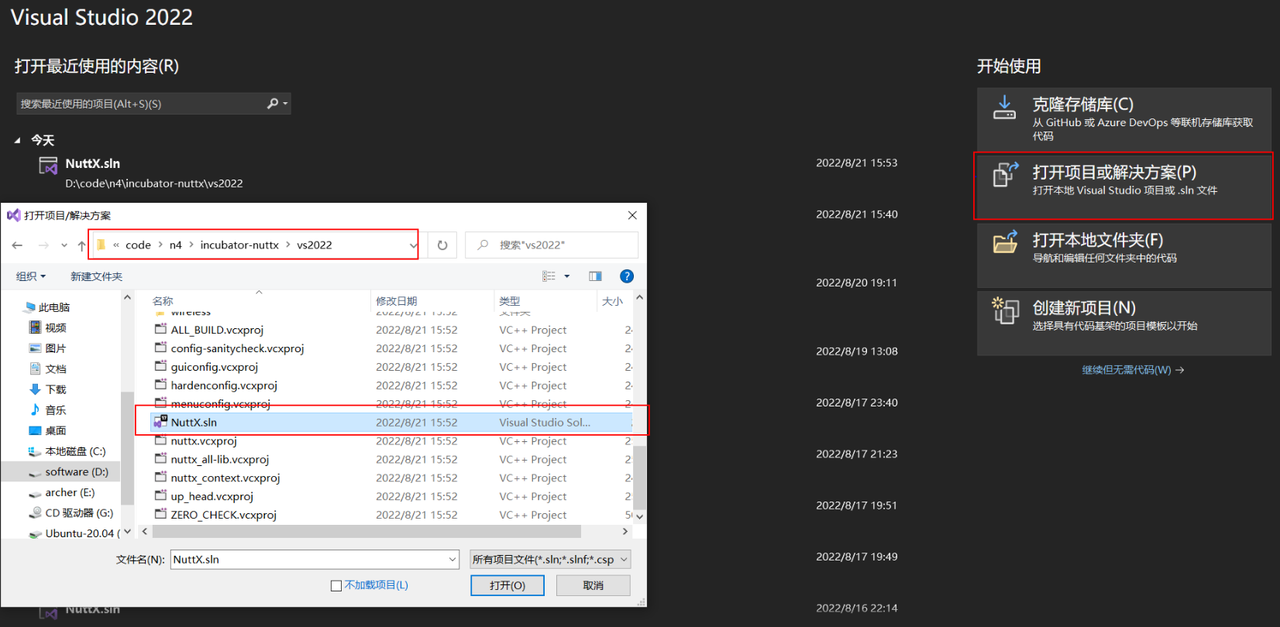
After the compilation is completed, Debug\nuttx.exe will be generated:
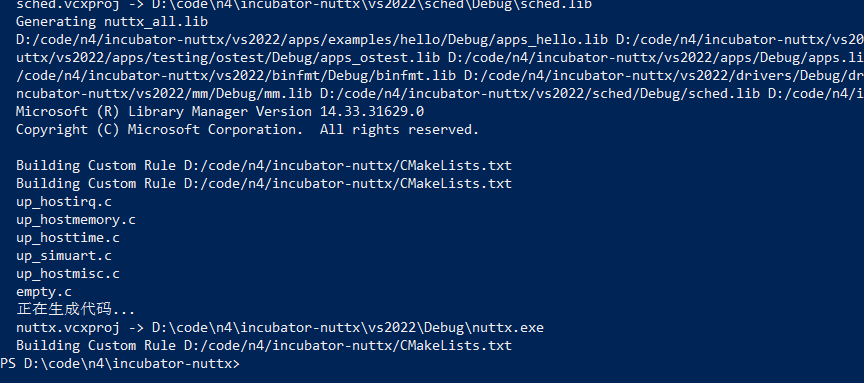
Run the simulator:
PowerShell:
(The default terminal operation has encoding issues, but it does not affect normal use, all commands can be executed normally)
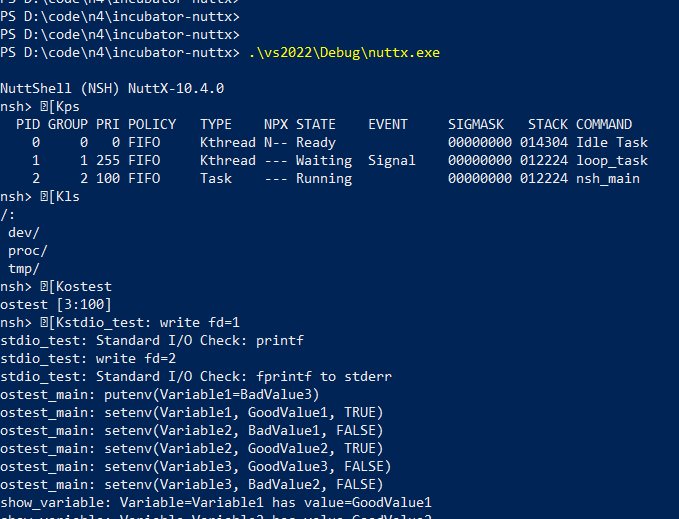
Visual Studio
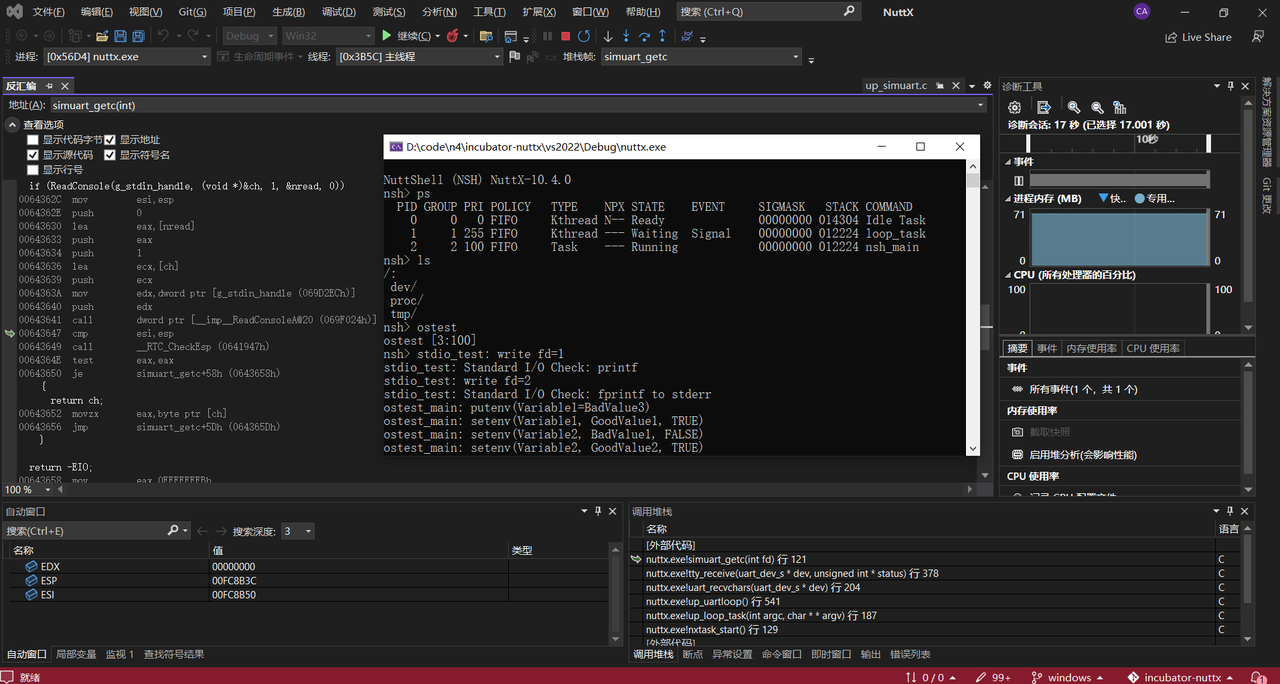
## Impact
N/A
## Testing
visual studio 2022 + cmake
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952409894
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,145 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -ENOSYS;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (WriteConsole(g_stdout_handle, &ch, 1, &nwritten, NULL))
Review Comment:
check fd like simuart_checkc
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,145 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -ENOSYS;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (WriteConsole(g_stdout_handle, &ch, 1, &nwritten, NULL))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getc
+ ****************************************************************************/
+
+int simuart_getc(int fd)
+{
+ unsigned char ch;
+ DWORD nread;
+
+ if (ReadConsole(g_stdin_handle, &ch, 1, &nread, 0))
Review Comment:
check fd like simuart_checkc
##########
arch/sim/src/sim/up_internal.h:
##########
@@ -32,7 +32,9 @@
#ifndef __ASSEMBLY__
# include <sys/types.h>
# include <stdbool.h>
-# include <netinet/in.h>
+# if defined(CONFIG_SIM_NETDEV_TAP) || defined(CONFIG_SIM_NETDEV_VPNKIT)
Review Comment:
let's remove the empty vpnkit_ifup and vpnkit_ifdown, adn remove CONFIG_SIM_NETDEV_VPNKIT
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952377494
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
Review Comment:
LL->ull
##########
arch/sim/src/Makefile:
##########
@@ -75,7 +75,12 @@ CSRCS += up_vfork.c
endif
endif
-VPATH = sim
+VPATH = :sim
+ifeq ($(CONFIG_HOST_WINDOWS),y)
Review Comment:
CONFIG_WINDOWS_NATIVE
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
+ FILETIME ftime;
+
+ if (rtc)
+ {
+ GetSystemTimeAsFileTime(&ftime);
+
+ counter.QuadPart = (((uint64_t)ftime.dwHighDateTime << 32 |
+ ftime.dwLowDateTime) -
+ DELTA_EPOCH_IN_100NS) * 100;
+ }
+ else
+ {
+ QueryPerformanceFrequency(&freq);
+ QueryPerformanceCounter(&counter);
+
+ counter.QuadPart = counter.QuadPart * POW10_9 / freq.QuadPart;
+ }
+
+ if (rtc)
+ {
+ return counter.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = counter.QuadPart;
+ }
+
+ return counter.QuadPart - start;
Review Comment:
move after line 68
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
+ FILETIME ftime;
+
+ if (rtc)
+ {
+ GetSystemTimeAsFileTime(&ftime);
+
+ counter.QuadPart = (((uint64_t)ftime.dwHighDateTime << 32 |
+ ftime.dwLowDateTime) -
+ DELTA_EPOCH_IN_100NS) * 100;
+ }
+ else
+ {
+ QueryPerformanceFrequency(&freq);
+ QueryPerformanceCounter(&counter);
+
+ counter.QuadPart = counter.QuadPart * POW10_9 / freq.QuadPart;
+ }
+
+ if (rtc)
+ {
+ return counter.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = counter.QuadPart;
+ }
+
+ return counter.QuadPart - start;
+}
+
+/****************************************************************************
+ * Name: host_sleep
+ ****************************************************************************/
+
+void host_sleep(uint64_t nsec)
+{
+ LARGE_INTEGER due;
+ HANDLE timer;
+
+ /* Convert to 100 nanosecond interval,
+ * negative value indicates relative time
+ */
+
+ due.QuadPart = -(10 * ((nsec + 999) / 1000));
Review Comment:
```suggestion
due.QuadPart = -((nsec + 99) / 100);
```
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
Review Comment:
split to two line
##########
tools/ci/testlist/sim-02.dat:
##########
@@ -12,3 +12,6 @@
# macOS doesn't have X11
-Darwin,sim:touchscreen
+
+# Do not build Windows configs
+-Darwin,sim:windows
Review Comment:
need disable linux too
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
Review Comment:
uint64_t to LARGE_INTEGER
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
+ FILETIME ftime;
+
+ if (rtc)
+ {
+ GetSystemTimeAsFileTime(&ftime);
+
+ counter.QuadPart = (((uint64_t)ftime.dwHighDateTime << 32 |
+ ftime.dwLowDateTime) -
+ DELTA_EPOCH_IN_100NS) * 100;
+ }
+ else
+ {
+ QueryPerformanceFrequency(&freq);
+ QueryPerformanceCounter(&counter);
+
+ counter.QuadPart = counter.QuadPart * POW10_9 / freq.QuadPart;
+ }
+
+ if (rtc)
+ {
+ return counter.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = counter.QuadPart;
+ }
+
+ return counter.QuadPart - start;
+}
+
+/****************************************************************************
+ * Name: host_sleep
+ ****************************************************************************/
+
+void host_sleep(uint64_t nsec)
+{
+ LARGE_INTEGER due;
+ HANDLE timer;
+
+ /* Convert to 100 nanosecond interval,
+ * negative value indicates relative time
+ */
+
+ due.QuadPart = -(10 * ((nsec + 999) / 1000));
+
+ timer = CreateWaitableTimer(NULL, TRUE, NULL);
+ if (timer != NULL)
+ {
+ SetWaitableTimer(timer, &due, 0, NULL, NULL, 0);
+ WaitForSingleObject(timer, INFINITE);
+ CloseHandle(timer);
+ }
+}
+
+/****************************************************************************
+ * Name: host_sleepuntil
+ ****************************************************************************/
+
+void host_sleepuntil(uint64_t nsec)
+{
+ uint64_t now;
+
+ now = host_gettime(false);
+ if (nsec > now + 1000)
Review Comment:
if (nsec > now)
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
+ FILETIME ftime;
+
+ if (rtc)
+ {
+ GetSystemTimeAsFileTime(&ftime);
+
+ counter.QuadPart = (((uint64_t)ftime.dwHighDateTime << 32 |
+ ftime.dwLowDateTime) -
+ DELTA_EPOCH_IN_100NS) * 100;
Review Comment:
merge to previous linke
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
+ FILETIME ftime;
+
+ if (rtc)
+ {
+ GetSystemTimeAsFileTime(&ftime);
+
+ counter.QuadPart = (((uint64_t)ftime.dwHighDateTime << 32 |
+ ftime.dwLowDateTime) -
+ DELTA_EPOCH_IN_100NS) * 100;
+ }
+ else
+ {
+ QueryPerformanceFrequency(&freq);
+ QueryPerformanceCounter(&counter);
+
+ counter.QuadPart = counter.QuadPart * POW10_9 / freq.QuadPart;
+ }
+
+ if (rtc)
+ {
+ return counter.QuadPart;
Review Comment:
move after line 61
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952389320
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,138 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define POW10_9 (1000000000ull)
+
+/* Number of 100ns-seconds between the beginning of the Windows epoch
+ * (Jan. 1, 1601) and the Unix epoch (Jan. 1, 1970)
+ */
+
+#define DELTA_EPOCH_IN_100NS (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static uint64_t start;
+ LARGE_INTEGER freq, counter;
+ FILETIME ftime;
+
+ if (rtc)
+ {
+ GetSystemTimeAsFileTime(&ftime);
+
+ counter.QuadPart = (((uint64_t)ftime.dwHighDateTime << 32 |
+ ftime.dwLowDateTime) -
+ DELTA_EPOCH_IN_100NS) * 100;
+ }
+ else
+ {
+ QueryPerformanceFrequency(&freq);
+ QueryPerformanceCounter(&counter);
+
+ counter.QuadPart = counter.QuadPart * POW10_9 / freq.QuadPart;
+ }
+
+ if (rtc)
+ {
+ return counter.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = counter.QuadPart;
+ }
+
+ return counter.QuadPart - start;
+}
+
+/****************************************************************************
+ * Name: host_sleep
+ ****************************************************************************/
+
+void host_sleep(uint64_t nsec)
+{
+ LARGE_INTEGER due;
+ HANDLE timer;
+
+ /* Convert to 100 nanosecond interval,
+ * negative value indicates relative time
+ */
+
+ due.QuadPart = -(10 * ((nsec + 999) / 1000));
Review Comment:
let's add macro NSTO100NS?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952206431
##########
libs/libc/machine/sim/arch_setjmp_x86.asm:
##########
@@ -0,0 +1,73 @@
+;***************************************************************************
+; libs/libc/machine/sim/arch_setjmp_x86.asm
+;
+; Licensed to the Apache Software Foundation (ASF) under one or more
+; contributor license agreements. See the NOTICE file distributed with
+; this work for additional information regarding copyright ownership. The
+; ASF licenses this file to you under the Apache License, Version 2.0 (the
+; "License"); you may not use this file except in compliance with the
+; License. You may obtain a copy of the License at
+;
+; http://www.apache.org/licenses/LICENSE-2.0
+;
+; Unless required by applicable law or agreed to in writing, software
+; distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+; WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+; License for the specific language governing permissions and limitations
+; under the License.
+;
+;***************************************************************************
+
+;***************************************************************************
+; Public Functions
+;***************************************************************************
+
+.model flat, c
+.code
+
+public setjmp
+public longjmp
+
+setjmp:
+ mov eax,dword ptr [esp+4]
+ mov dword ptr [eax],ebx
+ mov dword ptr [eax+4],esi
+ mov dword ptr [eax+8],edi
+
+ ; Save the value of SP as will be after we return
+
+ lea ecx, dword ptr [esp+4]
+ mov dword ptr [eax+10h],ecx
+
+ ; Save the return PC
+
+ mov ecx, dword ptr [esp]
+ mov dword ptr [eax+14h],ecx
+
+ ; Save the framepointer
+
+ mov dword ptr [eax+0Ch],ebp
Review Comment:
0Ch->0ch
##########
arch/sim/src/Makefile:
##########
@@ -24,6 +24,7 @@ ARCH_SRCDIR = $(TOPDIR)$(DELIM)arch$(DELIM)$(CONFIG_ARCH)$(DELIM)src
INCLUDES += ${shell $(INCDIR) "$(CC)" $(ARCH_SRCDIR)}
INCLUDES += ${shell $(INCDIR) "$(CC)" $(ARCH_SRCDIR)$(DELIM)chip}
+INCLUDES += ${shell $(INCDIR) "$(CC)" $(ARCH_SRCDIR)$(DELIM)chip$(DELIM)posix}
Review Comment:
don't need
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
Review Comment:
remove the check
##########
libs/libc/machine/sim/arch_setjmp_x86.asm:
##########
@@ -0,0 +1,73 @@
+;***************************************************************************
+; libs/libc/machine/sim/arch_setjmp_x86.asm
+;
+; Licensed to the Apache Software Foundation (ASF) under one or more
+; contributor license agreements. See the NOTICE file distributed with
+; this work for additional information regarding copyright ownership. The
+; ASF licenses this file to you under the Apache License, Version 2.0 (the
+; "License"); you may not use this file except in compliance with the
+; License. You may obtain a copy of the License at
+;
+; http://www.apache.org/licenses/LICENSE-2.0
+;
+; Unless required by applicable law or agreed to in writing, software
+; distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+; WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+; License for the specific language governing permissions and limitations
+; under the License.
+;
+;***************************************************************************
+
+;***************************************************************************
+; Public Functions
+;***************************************************************************
+
+.model flat, c
+.code
+
+public setjmp
+public longjmp
+
+setjmp:
+ mov eax,dword ptr [esp+4]
Review Comment:
add space after ALL ,
##########
libs/libc/machine/sim/arch_setjmp_x86.asm:
##########
@@ -0,0 +1,73 @@
+;***************************************************************************
+; libs/libc/machine/sim/arch_setjmp_x86.asm
+;
+; Licensed to the Apache Software Foundation (ASF) under one or more
+; contributor license agreements. See the NOTICE file distributed with
+; this work for additional information regarding copyright ownership. The
+; ASF licenses this file to you under the Apache License, Version 2.0 (the
+; "License"); you may not use this file except in compliance with the
+; License. You may obtain a copy of the License at
+;
+; http://www.apache.org/licenses/LICENSE-2.0
+;
+; Unless required by applicable law or agreed to in writing, software
+; distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+; WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+; License for the specific language governing permissions and limitations
+; under the License.
+;
+;***************************************************************************
+
+;***************************************************************************
+; Public Functions
+;***************************************************************************
+
+.model flat, c
+.code
+
+public setjmp
+public longjmp
+
+setjmp:
+ mov eax,dword ptr [esp+4]
+ mov dword ptr [eax],ebx
+ mov dword ptr [eax+4],esi
+ mov dword ptr [eax+8],edi
+
+ ; Save the value of SP as will be after we return
+
+ lea ecx, dword ptr [esp+4]
+ mov dword ptr [eax+10h],ecx
+
+ ; Save the return PC
+
+ mov ecx, dword ptr [esp]
+ mov dword ptr [eax+14h],ecx
+
+ ; Save the framepointer
+
+ mov dword ptr [eax+0Ch],ebp
+
+ ; And return 0
+
+ xor eax, eax
+ ret
+
+longjmp:
+ mov ecx,dword ptr [esp+4] ; jmpbuf in ecx.
+ mov eax,dword ptr [esp+8] ; Second argument is return value
+
+ ; Save the return address now
+
+ mov edx,dword ptr [ecx+14h]
+
+ ; Restore registers
+
+ mov ebx,dword ptr [ecx]
+ mov esi,dword ptr [ecx+4]
+ mov edi,dword ptr [ecx+8]
+ mov ebp,dword ptr [ecx+0Ch]
Review Comment:
0Ch->0ch
##########
boards/sim/sim/sim/configs/windows/defconfig:
##########
@@ -0,0 +1,30 @@
+#
+# This file is autogenerated: PLEASE DO NOT EDIT IT.
+#
+# You can use "make menuconfig" to make any modifications to the installed .config file.
+# You can then do "make savedefconfig" to generate a new defconfig file that includes your
+# modifications.
+#
+CONFIG_ARCH="sim"
+CONFIG_ARCH_BOARD="sim"
+CONFIG_ARCH_BOARD_SIM=y
+CONFIG_ARCH_CHIP="sim"
+CONFIG_ARCH_FLOAT_H=y
+CONFIG_ARCH_SIM=y
Review Comment:
should we add CONFIG_SIM_M32=y
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (g_stdout_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (WriteConsole(g_stdout_handle,
+ (const void *)&ch, 1, &nwritten, NULL))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getc
+ ****************************************************************************/
+
+int simuart_getc(int fd)
+{
+ unsigned char ch;
+ DWORD nread;
+
+ if (g_stdin_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (ReadConsole(g_stdin_handle, (void *)&ch, 1, &nread, 0))
Review Comment:
remove (void *)
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (g_stdout_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (WriteConsole(g_stdout_handle,
+ (const void *)&ch, 1, &nwritten, NULL))
Review Comment:
remove (const void *)
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (g_stdout_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (WriteConsole(g_stdout_handle,
+ (const void *)&ch, 1, &nwritten, NULL))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getc
+ ****************************************************************************/
+
+int simuart_getc(int fd)
+{
+ unsigned char ch;
+ DWORD nread;
+
+ if (g_stdin_handle == NULL)
Review Comment:
remove, let WriteConsole check it
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (g_stdout_handle == NULL)
Review Comment:
remove, let WriteConsole check it
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
Review Comment:
ENOSYS
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (g_stdout_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (WriteConsole(g_stdout_handle,
+ (const void *)&ch, 1, &nwritten, NULL))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getc
+ ****************************************************************************/
+
+int simuart_getc(int fd)
+{
+ unsigned char ch;
+ DWORD nread;
+
+ if (g_stdin_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (ReadConsole(g_stdin_handle, (void *)&ch, 1, &nread, 0))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getcflag
+ ****************************************************************************/
+
+int simuart_getcflag(int fd, unsigned int *cflag)
+{
+ return 0;
Review Comment:
-ENOSYS
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,122 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+/* 100ns difference between Windows and UNIX timebase. */
+
+#define FACTOR (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static LONGLONG start;
+ LARGE_INTEGER now;
+
+ GetSystemTimeAsFileTime((LPFILETIME)&now);
+
+ now.QuadPart -= FACTOR;
+ now.QuadPart *= 100;
+
+ if (rtc)
+ {
+ return now.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = now.QuadPart;
+ }
+
+ return now.QuadPart - start;
+}
+
+/****************************************************************************
+ * Name: host_sleep
+ ****************************************************************************/
+
+void host_sleep(uint64_t nsec)
+{
+ LARGE_INTEGER due;
+ HANDLE timer;
+
+ /* Convert to 100 nanosecond interval,
+ * negative value indicates relative time
+ */
+
+ due.QuadPart = -(10 * ((nsec + 999) / 1000));
+
+ timer = CreateWaitableTimer(NULL, TRUE, NULL);
+ if (timer != NULL)
+ {
+ SetWaitableTimer(timer, &due, 0, NULL, NULL, 0);
+ WaitForSingleObject(timer, INFINITE);
+ CloseHandle(timer);
+ }
+}
+
+/****************************************************************************
+ * Name: host_sleepuntil
+ ****************************************************************************/
+
+void host_sleepuntil(uint64_t nsec)
+{
+ uint64_t now;
+
+ now = host_gettime(false);
+ if (nsec > now + 1000)
+ host_sleep(nsec - now);
+}
+
+/****************************************************************************
+ * Name: host_settimer
+ *
+ * Description:
+ * Set up a timer to send periodic signals.
+ *
+ * Input Parameters:
+ * irq - a pointer where we save the host signal number for SIGALRM
+ *
+ * Returned Value:
+ * On success, (0) zero value is returned, otherwise a negative value.
+ *
+ ****************************************************************************/
+
+int host_settimer(int *irq)
+{
+ return 0;
Review Comment:
-ENOSYS
##########
arch/sim/src/sim/win/up_hostmisc.c:
##########
@@ -0,0 +1,49 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmisc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_abort
+ *
+ * Description:
+ * Abort the simulation
+ *
+ * Input Parameters:
+ * status - Exit status to set
+ ****************************************************************************/
+
+void host_abort(int status)
+{
+ PostQuitMessage(status);
Review Comment:
ExitProcess
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
Review Comment:
ditto
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
Review Comment:
CreateFileMapping/MapViewOfFile
https://docs.microsoft.com/en-us/windows/win32/memory/creating-named-shared-memory
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
+}
+
+void host_free_shmem(void *mem)
+{
+ _aligned_free(mem);
+}
+
+size_t host_malloc_size(void *mem)
+{
+ return _msize(mem);
+}
+
+void *host_memalign(size_t alignment, size_t size)
+{
+ return _aligned_malloc(size, alignment);
+}
+
+void host_free(void *mem)
+{
+ _aligned_free(mem);
Review Comment:
HeapFree
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,122 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+/* 100ns difference between Windows and UNIX timebase. */
+
+#define FACTOR (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static LONGLONG start;
+ LARGE_INTEGER now;
+
+ GetSystemTimeAsFileTime((LPFILETIME)&now);
Review Comment:
let's use to void the time jump:
https://github.com/Alexpux/mingw-w64/blob/master/mingw-w64-libraries/winpthreads/src/clock.c
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
+}
+
+void host_free_shmem(void *mem)
+{
+ _aligned_free(mem);
Review Comment:
UnmapViewOfFile
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
+}
+
+void host_free_shmem(void *mem)
+{
+ _aligned_free(mem);
+}
+
+size_t host_malloc_size(void *mem)
+{
+ return _msize(mem);
+}
+
+void *host_memalign(size_t alignment, size_t size)
+{
+ return _aligned_malloc(size, alignment);
+}
+
+void host_free(void *mem)
+{
+ _aligned_free(mem);
+}
+
+void *host_realloc(void *oldmem, size_t size)
+{
+ return _aligned_realloc(oldmem, size, 8);
Review Comment:
HeapReAlloc
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,122 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+/* 100ns difference between Windows and UNIX timebase. */
+
+#define FACTOR (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static LONGLONG start;
+ LARGE_INTEGER now;
Review Comment:
FILETIME and remove the cast at line 50
##########
arch/sim/src/sim/win/up_hostirq.c:
##########
@@ -0,0 +1,97 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostirq.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <windows.h>
Review Comment:
add blank line
##########
arch/sim/src/sim/win/up_simuart.c:
##########
@@ -0,0 +1,162 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_simuart.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static HANDLE g_stdin_handle;
+static HANDLE g_stdout_handle;
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: simuart_start
+ ****************************************************************************/
+
+void simuart_start(void)
+{
+ DWORD mode;
+
+ if (g_stdin_handle == NULL)
+ {
+ g_stdin_handle = GetStdHandle(STD_INPUT_HANDLE);
+ if (GetConsoleMode(g_stdin_handle, &mode))
+ {
+ SetConsoleMode(g_stdin_handle, mode & ~(ENABLE_MOUSE_INPUT |
+ ENABLE_WINDOW_INPUT |
+ ENABLE_ECHO_INPUT |
+ ENABLE_LINE_INPUT));
+ FlushConsoleInputBuffer(g_stdin_handle);
+ }
+ }
+
+ if (g_stdout_handle == NULL)
+ {
+ g_stdout_handle = GetStdHandle(STD_OUTPUT_HANDLE);
+ }
+}
+
+/****************************************************************************
+ * Name: simuart_open
+ ****************************************************************************/
+
+int simuart_open(const char *pathname)
+{
+ return -EPERM;
+}
+
+/****************************************************************************
+ * Name: simuart_close
+ ****************************************************************************/
+
+void simuart_close(int fd)
+{
+}
+
+/****************************************************************************
+ * Name: simuart_putc
+ ****************************************************************************/
+
+int simuart_putc(int fd, int ch)
+{
+ DWORD nwritten;
+
+ if (g_stdout_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (WriteConsole(g_stdout_handle,
+ (const void *)&ch, 1, &nwritten, NULL))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getc
+ ****************************************************************************/
+
+int simuart_getc(int fd)
+{
+ unsigned char ch;
+ DWORD nread;
+
+ if (g_stdin_handle == NULL)
+ {
+ return -EPERM;
+ }
+
+ if (ReadConsole(g_stdin_handle, (void *)&ch, 1, &nread, 0))
+ {
+ return ch;
+ }
+
+ return -EIO;
+}
+
+/****************************************************************************
+ * Name: simuart_getcflag
+ ****************************************************************************/
+
+int simuart_getcflag(int fd, unsigned int *cflag)
+{
+ return 0;
+}
+
+/****************************************************************************
+ * Name: simuart_setcflag
+ ****************************************************************************/
+
+int simuart_setcflag(int fd, unsigned int cflag)
+{
+ return 0;
Review Comment:
-ENOSYS
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
Review Comment:
HeapAlloc
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
+}
+
+void host_free_shmem(void *mem)
+{
+ _aligned_free(mem);
+}
+
+size_t host_malloc_size(void *mem)
+{
+ return _msize(mem);
Review Comment:
HeapSize
##########
arch/sim/src/sim/win/up_hostmisc.c:
##########
@@ -0,0 +1,49 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmisc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_abort
+ *
+ * Description:
+ * Abort the simulation
+ *
+ * Input Parameters:
+ * status - Exit status to set
+ ****************************************************************************/
+
+void host_abort(int status)
+{
+ PostQuitMessage(status);
+}
+
+int host_backtrace(void** array, int size)
+{
+ return 0;
Review Comment:
CaptureStackBackTrace
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,122 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+/* 100ns difference between Windows and UNIX timebase. */
+
+#define FACTOR (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static LONGLONG start;
Review Comment:
uint64_t
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,122 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+/* 100ns difference between Windows and UNIX timebase. */
+
+#define FACTOR (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static LONGLONG start;
+ LARGE_INTEGER now;
+
+ GetSystemTimeAsFileTime((LPFILETIME)&now);
+
+ now.QuadPart -= FACTOR;
+ now.QuadPart *= 100;
+
+ if (rtc)
+ {
+ return now.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = now.QuadPart;
+ }
+
+ return now.QuadPart - start;
+}
+
+/****************************************************************************
+ * Name: host_sleep
+ ****************************************************************************/
+
+void host_sleep(uint64_t nsec)
+{
+ LARGE_INTEGER due;
+ HANDLE timer;
+
+ /* Convert to 100 nanosecond interval,
+ * negative value indicates relative time
+ */
+
+ due.QuadPart = -(10 * ((nsec + 999) / 1000));
+
+ timer = CreateWaitableTimer(NULL, TRUE, NULL);
Review Comment:
call Sleep instead
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] anchao commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
anchao commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952264286
##########
boards/sim/sim/sim/configs/windows/defconfig:
##########
@@ -0,0 +1,30 @@
+#
+# This file is autogenerated: PLEASE DO NOT EDIT IT.
+#
+# You can use "make menuconfig" to make any modifications to the installed .config file.
+# You can then do "make savedefconfig" to generate a new defconfig file that includes your
+# modifications.
+#
+CONFIG_ARCH="sim"
+CONFIG_ARCH_BOARD="sim"
+CONFIG_ARCH_BOARD_SIM=y
+CONFIG_ARCH_CHIP="sim"
+CONFIG_ARCH_FLOAT_H=y
+CONFIG_ARCH_SIM=y
Review Comment:
already define CONFIG_HOST_X86 in this config
##########
arch/sim/src/Makefile:
##########
@@ -24,6 +24,7 @@ ARCH_SRCDIR = $(TOPDIR)$(DELIM)arch$(DELIM)$(CONFIG_ARCH)$(DELIM)src
INCLUDES += ${shell $(INCDIR) "$(CC)" $(ARCH_SRCDIR)}
INCLUDES += ${shell $(INCDIR) "$(CC)" $(ARCH_SRCDIR)$(DELIM)chip}
+INCLUDES += ${shell $(INCDIR) "$(CC)" $(ARCH_SRCDIR)$(DELIM)chip$(DELIM)posix}
Review Comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] anchao commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
anchao commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952354194
##########
arch/sim/src/sim/win/up_hosttime.c:
##########
@@ -0,0 +1,122 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hosttime.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <stdint.h>
+#include <stdbool.h>
+#include <windows.h>
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+/* 100ns difference between Windows and UNIX timebase. */
+
+#define FACTOR (0x19db1ded53e8000LL)
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_gettime
+ ****************************************************************************/
+
+uint64_t host_gettime(bool rtc)
+{
+ static LONGLONG start;
+ LARGE_INTEGER now;
+
+ GetSystemTimeAsFileTime((LPFILETIME)&now);
+
+ now.QuadPart -= FACTOR;
+ now.QuadPart *= 100;
+
+ if (rtc)
+ {
+ return now.QuadPart;
+ }
+
+ if (start == 0)
+ {
+ start = now.QuadPart;
+ }
+
+ return now.QuadPart - start;
+}
+
+/****************************************************************************
+ * Name: host_sleep
+ ****************************************************************************/
+
+void host_sleep(uint64_t nsec)
+{
+ LARGE_INTEGER due;
+ HANDLE timer;
+
+ /* Convert to 100 nanosecond interval,
+ * negative value indicates relative time
+ */
+
+ due.QuadPart = -(10 * ((nsec + 999) / 1000));
+
+ timer = CreateWaitableTimer(NULL, TRUE, NULL);
Review Comment:
Precision of Sleep() does not match with host api
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] xiaoxiang781216 merged pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 merged PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] anchao commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
anchao commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952272796
##########
arch/sim/src/sim/win/up_hostmisc.c:
##########
@@ -0,0 +1,49 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmisc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_abort
+ *
+ * Description:
+ * Abort the simulation
+ *
+ * Input Parameters:
+ * status - Exit status to set
+ ****************************************************************************/
+
+void host_abort(int status)
+{
+ PostQuitMessage(status);
+}
+
+int host_backtrace(void** array, int size)
+{
+ return 0;
Review Comment:
GOT!
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] anchao commented on a diff in pull request #6897: arch/sim: add windows host simulate support
Posted by GitBox <gi...@apache.org>.
anchao commented on code in PR #6897:
URL: https://github.com/apache/incubator-nuttx/pull/6897#discussion_r952293869
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
+}
+
+void host_free_shmem(void *mem)
+{
+ _aligned_free(mem);
+}
+
+size_t host_malloc_size(void *mem)
+{
+ return _msize(mem);
Review Comment:
windows heap api does not provide the memalign version, let us keep current implement
##########
arch/sim/src/sim/win/up_hostmemory.c:
##########
@@ -0,0 +1,76 @@
+/****************************************************************************
+ * arch/sim/src/sim/win/up_hostmemory.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <windows.h>
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: host_alloc_heap
+ *
+ * Description:
+ * Allocate executable memory for heap.
+ *
+ ****************************************************************************/
+
+void *host_alloc_heap(size_t sz)
+{
+ return _aligned_malloc(sz, 8);
+}
+
+void *host_alloc_shmem(const char *name, size_t size, int master)
+{
+ return _aligned_malloc(size, 8);
+}
+
+void host_free_shmem(void *mem)
+{
+ _aligned_free(mem);
Review Comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
To unsubscribe, e-mail: commits-unsubscribe@nuttx.apache.org
For queries about this service, please contact Infrastructure at:
users@infra.apache.org