You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@nuttx.apache.org by GitBox <gi...@apache.org> on 2021/05/27 10:00:43 UTC
[GitHub] [incubator-nuttx] cwespressif opened a new pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
cwespressif opened a new pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794
## Summary
riscv/esp32c3: Support ESP32-C3 RTC driver
## Impact
## Testing
The rtc driver was successfully tested in esp32c3-devkitc using the alarm example.
- config:
`./tools/configure esp32c3-devkit:rtc`
- test results
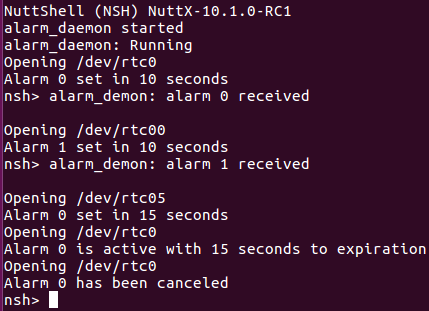
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642432397
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
Review comment:
@cwespressif can you please add some comment here to explain the difference of one flow to another in favor of good documentation of this code?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646252426
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646255316
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
Review comment:
Multiple time sources may not be easy to manage, especially in sleep mode, anyway, I will consider it later.
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
+ {
+ rtcerr("ERROR: Failed to create rt_timer error=%d\n", ret);
+ spin_unlock_irqrestore(NULL, flags);
+ return ret;
+ }
+ }
+
+ cbinfo->ac_cb = alminfo->as_cb;
+ cbinfo->ac_arg = alminfo->as_arg;
+ cbinfo->deadline_us = alminfo->as_time.tv_sec * USEC_PER_SEC +
+ alminfo->as_time.tv_nsec / NSEC_PER_USEC;
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ rtcerr("ERROR: failed to creat alarm timer\n");
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644070922
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
Review comment:
```suggestion
/* Now set the time (with a accuracy of seconds) */
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646252095
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -349,6 +361,49 @@ struct esp32c3_rtc_sleep_config_s
uint32_t light_slp_reject : 1;
};
+#ifdef CONFIG_RTC_DRIVER
+
+#ifdef CONFIG_RTC_ALARM
+struct alm_cbinfo_s
+{
+ struct rt_timer_s *alarm_hdl; /* timer id point to here */
+ volatile alm_callback_t ac_cb; /* Client callback function */
+ volatile FAR void *ac_arg; /* Argument to pass with the callback function */
+ uint64_t deadline_us;
+ uint8_t index;
+};
+#endif
+
+struct esp32c3_rtc_backup_s
+{
+ uint64_t magic;
+ int64_t offset; /* offset time from RTC HW value */
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646257216
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
Review comment:
Regarding the issue of prefixes, I have discussed with @gustavonihei , adding `esp32c3_ ` in to a function name is redundant, and using the prefix of `rtc` to describe the function module more accurately, maybe we can discuss it again.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r641102819
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -210,18 +214,22 @@
*/
#define RTC_CONFIG_DEFAULT() {\
- .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
- .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
- .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
- .clkctl_init = 1, \
- .pwrctl_init = 1, \
- .rtc_dboost_fpd = 1, \
- .xtal_fpu = 0, \
- .bbpll_fpu = 0, \
- .cpu_waiti_clk_gate = 1, \
- .cali_ocode = 0\
+ .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
+ .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
+ .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
+ .clkctl_init = 1, \
+ .pwrctl_init = 1, \
+ .rtc_dboost_fpd = 1, \
+ .xtal_fpu = 0, \
+ .bbpll_fpu = 0, \
+ .cpu_waiti_clk_gate = 1, \
+ .cali_ocode = 0\
}
+#ifdef CONFIG_RTC_DRIVER
+# define MAGIC_RTC_SAVE (0x11223344556677ull)
Review comment:
Done, thanks for the suggestion.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646252154
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -1263,6 +1330,50 @@ static void IRAM_ATTR esp32c3_rtc_clk_cpu_freq_to_pll_mhz(
ets_update_cpu_frequency(cpu_freq_mhz);
}
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: esp32c3_rt_cb_handler
+ *
+ * Description:
+ * RT-Timer service routine
+ *
+ * Input Parameters:
+ * arg - Information about the RT-Timer configuration.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+static void IRAM_ATTR esp32c3_rt_cb_handler(FAR void *arg)
+{
+ FAR struct alm_cbinfo_s *cbinfo = (struct alm_cbinfo_s *)arg;
+ alm_callback_t cb;
+ FAR void *cb_arg;
+ int alminfo_id;
+
+ DEBUGASSERT(cbinfo != NULL);
+ alminfo_id = cbinfo->index;
+ DEBUGASSERT((RTC_ALARM0 <= alminfo_id) &&
+ (alminfo_id < RTC_ALARM_LAST));
+
+ if (cbinfo->ac_cb != NULL)
+ {
+ /* Alarm callback */
+
+ cb = cbinfo->ac_cb;
+ cb_arg = (FAR void *)cbinfo->ac_arg;
+
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642439500
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
+ {
+ rtcerr("ERROR: Failed to create rt_timer error=%d\n", ret);
+ spin_unlock_irqrestore(NULL, flags);
+ return ret;
+ }
+ }
+
+ cbinfo->ac_cb = alminfo->as_cb;
+ cbinfo->ac_arg = alminfo->as_arg;
+ cbinfo->deadline_us = alminfo->as_time.tv_sec * USEC_PER_SEC +
+ alminfo->as_time.tv_nsec / NSEC_PER_USEC;
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ rtcerr("ERROR: failed to creat alarm timer\n");
Review comment:
```suggestion
rtcerr("ERROR: failed to create alarm timer\n");
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642442944
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
+ {
+ rtcerr("ERROR: Failed to create rt_timer error=%d\n", ret);
+ spin_unlock_irqrestore(NULL, flags);
+ return ret;
+ }
+ }
+
+ cbinfo->ac_cb = alminfo->as_cb;
+ cbinfo->ac_arg = alminfo->as_arg;
+ cbinfo->deadline_us = alminfo->as_time.tv_sec * USEC_PER_SEC +
+ alminfo->as_time.tv_nsec / NSEC_PER_USEC;
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ rtcerr("ERROR: failed to creat alarm timer\n");
+ }
+ else
+ {
+ rtcinfo("Start RTC alarm.\n");
+ rt_timer_start(cbinfo->alarm_hdl, cbinfo->deadline_us, false);
+ ret = OK;
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: up_rtc_cancelalarm
+ *
+ * Description:
+ * Cancel an alarm.
+ *
+ * Input Parameters:
+ * alarmid - Identifies the alarm to be cancelled
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_cancelalarm(enum alm_id_e alarmid)
+{
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -ENODATA;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) &&
+ (alarmid < RTC_ALARM_LAST));
+
+ /* Set the alarm in hardware and enable interrupts */
+
+ cbinfo = &g_alarmcb[alarmid];
+
+ if (cbinfo->ac_cb != NULL)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ /* Stop and delete the alarm */
+
+ rtcinfo("Cancel RTC alarm.\n");
+ rt_timer_stop(cbinfo->alarm_hdl);
+ rt_timer_delete(cbinfo->alarm_hdl);
+ cbinfo->ac_cb = NULL;
+ cbinfo->deadline_us = 0;
+ cbinfo->alarm_hdl = NULL;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: up_rtc_rdalarm
+ *
+ * Description:
+ * Query an alarm configured in hardware.
+ *
+ * Input Parameters:
+ * tp - Location to return the timer match register.
+ * alarmid - Identifies the alarm to be cancelled
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_rdalarm(FAR struct timespec *tp, uint32_t alarmid)
+{
+ irqstate_t flags;
+ FAR struct alm_cbinfo_s *cbinfo;
+ DEBUGASSERT(tp != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) &&
+ (alarmid < RTC_ALARM_LAST));
+
+ flags = spin_lock_irqsave(NULL);
+
+ /* Set the alarm in hardware and enable interrupts */
Review comment:
This comment seem to be out of context.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642433324
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
Review comment:
```suggestion
* ts - the time to use
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644079921
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
+
+ return up_rtc_settime(&ts);
+}
+
+/****************************************************************************
+ * Name: rtc_havesettime
+ *
+ * Description:
+ * Implements the havesettime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ *
+ * Returned Value:
+ * Returns true if RTC date-time have been previously set.
+ *
+ ****************************************************************************/
+
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower)
+{
+ if (esp32c3_rtc_get_boot_time() == 0)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+/****************************************************************************
+ * Name: rtc_setalarm
+ *
+ * Description:
+ * Set a new alarm. This function implements the setalarm() method of the
+ * RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ struct alm_setalarm_s lowerinfo;
+ int ret = -EINVAL;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ priv = (FAR struct esp32c3_lowerhalf_s *)lower;
+
+ /* Remember the callback information */
+
+ cbinfo = &priv->cbinfo[alarminfo->id];
+ cbinfo->cb = alarminfo->cb;
+ cbinfo->priv = alarminfo->priv;
+
+ /* Set the alarm */
+
+ lowerinfo.as_id = alarminfo->id;
+ lowerinfo.as_cb = rtc_alarm_callback;
+ lowerinfo.as_arg = priv;
+
+ /* Convert the RTC time to a timespec (1 second accuracy) */
+
+ lowerinfo.as_time.tv_sec = mktime((FAR struct tm *)&alarminfo->time);
+ lowerinfo.as_time.tv_nsec = 0;
+
+ /* And set the alarm */
+
+ ret = up_rtc_setalarm(&lowerinfo);
+ if (ret < 0)
+ {
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_setrelative
+ *
+ * Description:
+ * Set a new alarm relative to the current time. This function implements
+ * the setrelative() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo)
+{
+ struct lower_setalarm_s setalarm;
+ time_t seconds;
+ int ret = -EINVAL;
+ irqstate_t flags;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ if (alarminfo->reltime > 0)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ seconds = alarminfo->reltime;
+ gmtime_r(&seconds, (FAR struct tm *)&setalarm.time);
+
+ /* The set the alarm using this absolute time */
+
+ setalarm.id = alarminfo->id;
+ setalarm.cb = alarminfo->cb;
+ setalarm.priv = alarminfo->priv;
+ ret = rtc_setalarm(lower, &setalarm);
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_cancelalarm
+ *
+ * Description:
+ * Cancel the current alarm. This function implements the cancelalarm()
+ * method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarmid - the alarm id
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid)
Review comment:
Place it in the line before or fix the alignment
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644078106
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
+
+ return up_rtc_settime(&ts);
+}
+
+/****************************************************************************
+ * Name: rtc_havesettime
+ *
+ * Description:
+ * Implements the havesettime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ *
+ * Returned Value:
+ * Returns true if RTC date-time have been previously set.
+ *
+ ****************************************************************************/
+
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower)
+{
+ if (esp32c3_rtc_get_boot_time() == 0)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+/****************************************************************************
+ * Name: rtc_setalarm
+ *
+ * Description:
+ * Set a new alarm. This function implements the setalarm() method of the
+ * RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ struct alm_setalarm_s lowerinfo;
+ int ret = -EINVAL;
Review comment:
No need to initialize.
It will be overwritten in line 364
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r647922914
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644084619
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.h
##########
@@ -0,0 +1,44 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __ARCH_RISCV_SRC_ESP32C3_ESP32C3_RTC_LOWERHALF_H
+#define __ARCH_RISCV_SRC_ESP32C3_ESP32C3_RTC_LOWERHALF_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: esp32c3_rtc_driverinit
+ ****************************************************************************/
Review comment:
Complete the function header
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646258077
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
+
+ return up_rtc_settime(&ts);
+}
+
+/****************************************************************************
+ * Name: rtc_havesettime
+ *
+ * Description:
+ * Implements the havesettime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ *
+ * Returned Value:
+ * Returns true if RTC date-time have been previously set.
+ *
+ ****************************************************************************/
+
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower)
+{
+ if (esp32c3_rtc_get_boot_time() == 0)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+/****************************************************************************
+ * Name: rtc_setalarm
+ *
+ * Description:
+ * Set a new alarm. This function implements the setalarm() method of the
+ * RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ struct alm_setalarm_s lowerinfo;
+ int ret = -EINVAL;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ priv = (FAR struct esp32c3_lowerhalf_s *)lower;
+
+ /* Remember the callback information */
+
+ cbinfo = &priv->cbinfo[alarminfo->id];
+ cbinfo->cb = alarminfo->cb;
+ cbinfo->priv = alarminfo->priv;
+
+ /* Set the alarm */
+
+ lowerinfo.as_id = alarminfo->id;
+ lowerinfo.as_cb = rtc_alarm_callback;
+ lowerinfo.as_arg = priv;
+
+ /* Convert the RTC time to a timespec (1 second accuracy) */
+
+ lowerinfo.as_time.tv_sec = mktime((FAR struct tm *)&alarminfo->time);
+ lowerinfo.as_time.tv_nsec = 0;
+
+ /* And set the alarm */
+
+ ret = up_rtc_setalarm(&lowerinfo);
+ if (ret < 0)
+ {
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_setrelative
+ *
+ * Description:
+ * Set a new alarm relative to the current time. This function implements
+ * the setrelative() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo)
+{
+ struct lower_setalarm_s setalarm;
+ time_t seconds;
+ int ret = -EINVAL;
+ irqstate_t flags;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ if (alarminfo->reltime > 0)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ seconds = alarminfo->reltime;
+ gmtime_r(&seconds, (FAR struct tm *)&setalarm.time);
+
+ /* The set the alarm using this absolute time */
+
+ setalarm.id = alarminfo->id;
+ setalarm.cb = alarminfo->cb;
+ setalarm.priv = alarminfo->priv;
+ ret = rtc_setalarm(lower, &setalarm);
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_cancelalarm
+ *
+ * Description:
+ * Cancel the current alarm. This function implements the cancelalarm()
+ * method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarmid - the alarm id
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+
+ DEBUGASSERT(lower != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ priv = (FAR struct esp32c3_lowerhalf_s *)lower;
+
+ /* Nullify callback information to reduce window for race conditions */
+
+ cbinfo = &priv->cbinfo[alarmid];
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Then cancel the alarm */
+
+ return up_rtc_cancelalarm((enum alm_id_e)alarmid);
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdalarm
+ *
+ * Description:
+ * Query the RTC alarm.
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to query the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo)
+{
+ struct timespec ts;
+ int ret = -EINVAL;
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] gustavonihei commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
gustavonihei commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644282367
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -210,18 +214,26 @@
*/
#define RTC_CONFIG_DEFAULT() {\
- .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
- .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
- .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
- .clkctl_init = 1, \
- .pwrctl_init = 1, \
- .rtc_dboost_fpd = 1, \
- .xtal_fpu = 0, \
- .bbpll_fpu = 0, \
- .cpu_waiti_clk_gate = 1, \
- .cali_ocode = 0\
+ .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
+ .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
+ .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
+ .clkctl_init = 1, \
+ .pwrctl_init = 1, \
+ .rtc_dboost_fpd = 1, \
+ .xtal_fpu = 0, \
+ .bbpll_fpu = 0, \
+ .cpu_waiti_clk_gate = 1, \
+ .cali_ocode = 0\
}
+#ifdef CONFIG_RTC_DRIVER
+/* The magic data for the struct esp32c3_rtc_backup_s that is in RTC slow
+ * memory.
+ */
+
+# define MAGIC_RTC_SAVE (0x11223344556677ull)
Review comment:
```suggestion
# define MAGIC_RTC_SAVE (UINT64_C(0x11223344556677))
```
nit: Use the definitions from `inttypes.h` for applying the suffix to integer constants in a portable and abstract way.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646253918
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
Review comment:
Already added, please take a look, thank you.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646252347
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642428813
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
Review comment:
```suggestion
* RTC/counter hardware implementation is selected. It is only used by the
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646257456
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
Review comment:
Done
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
+
+ return up_rtc_settime(&ts);
+}
+
+/****************************************************************************
+ * Name: rtc_havesettime
+ *
+ * Description:
+ * Implements the havesettime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ *
+ * Returned Value:
+ * Returns true if RTC date-time have been previously set.
+ *
+ ****************************************************************************/
+
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower)
+{
+ if (esp32c3_rtc_get_boot_time() == 0)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+/****************************************************************************
+ * Name: rtc_setalarm
+ *
+ * Description:
+ * Set a new alarm. This function implements the setalarm() method of the
+ * RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ struct alm_setalarm_s lowerinfo;
+ int ret = -EINVAL;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ priv = (FAR struct esp32c3_lowerhalf_s *)lower;
+
+ /* Remember the callback information */
+
+ cbinfo = &priv->cbinfo[alarminfo->id];
+ cbinfo->cb = alarminfo->cb;
+ cbinfo->priv = alarminfo->priv;
+
+ /* Set the alarm */
+
+ lowerinfo.as_id = alarminfo->id;
+ lowerinfo.as_cb = rtc_alarm_callback;
+ lowerinfo.as_arg = priv;
+
+ /* Convert the RTC time to a timespec (1 second accuracy) */
+
+ lowerinfo.as_time.tv_sec = mktime((FAR struct tm *)&alarminfo->time);
+ lowerinfo.as_time.tv_nsec = 0;
+
+ /* And set the alarm */
+
+ ret = up_rtc_setalarm(&lowerinfo);
+ if (ret < 0)
+ {
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_setrelative
+ *
+ * Description:
+ * Set a new alarm relative to the current time. This function implements
+ * the setrelative() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo)
+{
+ struct lower_setalarm_s setalarm;
+ time_t seconds;
+ int ret = -EINVAL;
+ irqstate_t flags;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ if (alarminfo->reltime > 0)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ seconds = alarminfo->reltime;
+ gmtime_r(&seconds, (FAR struct tm *)&setalarm.time);
+
+ /* The set the alarm using this absolute time */
+
+ setalarm.id = alarminfo->id;
+ setalarm.cb = alarminfo->cb;
+ setalarm.priv = alarminfo->priv;
+ ret = rtc_setalarm(lower, &setalarm);
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_cancelalarm
+ *
+ * Description:
+ * Cancel the current alarm. This function implements the cancelalarm()
+ * method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarmid - the alarm id
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid)
Review comment:
Done
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.h
##########
@@ -0,0 +1,44 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __ARCH_RISCV_SRC_ESP32C3_ESP32C3_RTC_LOWERHALF_H
+#define __ARCH_RISCV_SRC_ESP32C3_ESP32C3_RTC_LOWERHALF_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: esp32c3_rtc_driverinit
+ ****************************************************************************/
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646528828
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
Review comment:
So, you need to fix the header description, because it says `up_rtc_time` will be used if `CONFIG_RTC_HIRES` and `CONFIG_RTC_DATETIME` are **not** set.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646258113
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
+
+ return up_rtc_settime(&ts);
+}
+
+/****************************************************************************
+ * Name: rtc_havesettime
+ *
+ * Description:
+ * Implements the havesettime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ *
+ * Returned Value:
+ * Returns true if RTC date-time have been previously set.
+ *
+ ****************************************************************************/
+
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower)
+{
+ if (esp32c3_rtc_get_boot_time() == 0)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+/****************************************************************************
+ * Name: rtc_setalarm
+ *
+ * Description:
+ * Set a new alarm. This function implements the setalarm() method of the
+ * RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ struct alm_setalarm_s lowerinfo;
+ int ret = -EINVAL;
Review comment:
Done
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
Review comment:
Done
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
+ {
+ rtcerr("ERROR: Failed to create rt_timer error=%d\n", ret);
+ spin_unlock_irqrestore(NULL, flags);
+ return ret;
+ }
+ }
+
+ cbinfo->ac_cb = alminfo->as_cb;
+ cbinfo->ac_arg = alminfo->as_arg;
+ cbinfo->deadline_us = alminfo->as_time.tv_sec * USEC_PER_SEC +
+ alminfo->as_time.tv_nsec / NSEC_PER_USEC;
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ rtcerr("ERROR: failed to creat alarm timer\n");
+ }
+ else
+ {
+ rtcinfo("Start RTC alarm.\n");
+ rt_timer_start(cbinfo->alarm_hdl, cbinfo->deadline_us, false);
+ ret = OK;
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: up_rtc_cancelalarm
+ *
+ * Description:
+ * Cancel an alarm.
+ *
+ * Input Parameters:
+ * alarmid - Identifies the alarm to be cancelled
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_cancelalarm(enum alm_id_e alarmid)
+{
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -ENODATA;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) &&
+ (alarmid < RTC_ALARM_LAST));
+
+ /* Set the alarm in hardware and enable interrupts */
+
+ cbinfo = &g_alarmcb[alarmid];
+
+ if (cbinfo->ac_cb != NULL)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ /* Stop and delete the alarm */
+
+ rtcinfo("Cancel RTC alarm.\n");
+ rt_timer_stop(cbinfo->alarm_hdl);
+ rt_timer_delete(cbinfo->alarm_hdl);
+ cbinfo->ac_cb = NULL;
+ cbinfo->deadline_us = 0;
+ cbinfo->alarm_hdl = NULL;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: up_rtc_rdalarm
+ *
+ * Description:
+ * Query an alarm configured in hardware.
+ *
+ * Input Parameters:
+ * tp - Location to return the timer match register.
+ * alarmid - Identifies the alarm to be cancelled
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_rdalarm(FAR struct timespec *tp, uint32_t alarmid)
+{
+ irqstate_t flags;
+ FAR struct alm_cbinfo_s *cbinfo;
+ DEBUGASSERT(tp != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) &&
+ (alarmid < RTC_ALARM_LAST));
+
+ flags = spin_lock_irqsave(NULL);
+
+ /* Set the alarm in hardware and enable interrupts */
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646255665
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
Review comment:
Agree, I have modified.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646530701
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
Review comment:
Ok
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644021151
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
Review comment:
I don't believe it's necessary
since Make.defs already ensures lh will only be included in case RTC driver is selected.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642419555
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -349,6 +361,49 @@ struct esp32c3_rtc_sleep_config_s
uint32_t light_slp_reject : 1;
};
+#ifdef CONFIG_RTC_DRIVER
+
+#ifdef CONFIG_RTC_ALARM
+struct alm_cbinfo_s
+{
+ struct rt_timer_s *alarm_hdl; /* timer id point to here */
Review comment:
```suggestion
struct rt_timer_s *alarm_hdl; /* Timer id point to here */
```
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -349,6 +361,49 @@ struct esp32c3_rtc_sleep_config_s
uint32_t light_slp_reject : 1;
};
+#ifdef CONFIG_RTC_DRIVER
+
+#ifdef CONFIG_RTC_ALARM
+struct alm_cbinfo_s
+{
+ struct rt_timer_s *alarm_hdl; /* timer id point to here */
+ volatile alm_callback_t ac_cb; /* Client callback function */
+ volatile FAR void *ac_arg; /* Argument to pass with the callback function */
+ uint64_t deadline_us;
+ uint8_t index;
+};
+#endif
+
+struct esp32c3_rtc_backup_s
+{
+ uint64_t magic;
+ int64_t offset; /* offset time from RTC HW value */
Review comment:
```suggestion
int64_t offset; /* Offset time from RTC HW value */
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642425522
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
Review comment:
```suggestion
putreg32((uint32_t)(time_us & UINT32_MAX), RTC_BOOT_TIME_LOW_REG);
```
PTAL at the helpers from `include/stdint.h`
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642441507
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
+ {
+ rtcerr("ERROR: Failed to create rt_timer error=%d\n", ret);
+ spin_unlock_irqrestore(NULL, flags);
+ return ret;
+ }
+ }
+
+ cbinfo->ac_cb = alminfo->as_cb;
+ cbinfo->ac_arg = alminfo->as_arg;
+ cbinfo->deadline_us = alminfo->as_time.tv_sec * USEC_PER_SEC +
+ alminfo->as_time.tv_nsec / NSEC_PER_USEC;
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ rtcerr("ERROR: failed to creat alarm timer\n");
Review comment:
BTW, if the alarm was failed to be created, wouldn't this code return in line 2517?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642442944
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
+ {
+ rtcerr("ERROR: Failed to create rt_timer error=%d\n", ret);
+ spin_unlock_irqrestore(NULL, flags);
+ return ret;
+ }
+ }
+
+ cbinfo->ac_cb = alminfo->as_cb;
+ cbinfo->ac_arg = alminfo->as_arg;
+ cbinfo->deadline_us = alminfo->as_time.tv_sec * USEC_PER_SEC +
+ alminfo->as_time.tv_nsec / NSEC_PER_USEC;
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ rtcerr("ERROR: failed to creat alarm timer\n");
+ }
+ else
+ {
+ rtcinfo("Start RTC alarm.\n");
+ rt_timer_start(cbinfo->alarm_hdl, cbinfo->deadline_us, false);
+ ret = OK;
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: up_rtc_cancelalarm
+ *
+ * Description:
+ * Cancel an alarm.
+ *
+ * Input Parameters:
+ * alarmid - Identifies the alarm to be cancelled
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_cancelalarm(enum alm_id_e alarmid)
+{
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -ENODATA;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) &&
+ (alarmid < RTC_ALARM_LAST));
+
+ /* Set the alarm in hardware and enable interrupts */
+
+ cbinfo = &g_alarmcb[alarmid];
+
+ if (cbinfo->ac_cb != NULL)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ /* Stop and delete the alarm */
+
+ rtcinfo("Cancel RTC alarm.\n");
+ rt_timer_stop(cbinfo->alarm_hdl);
+ rt_timer_delete(cbinfo->alarm_hdl);
+ cbinfo->ac_cb = NULL;
+ cbinfo->deadline_us = 0;
+ cbinfo->alarm_hdl = NULL;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: up_rtc_rdalarm
+ *
+ * Description:
+ * Query an alarm configured in hardware.
+ *
+ * Input Parameters:
+ * tp - Location to return the timer match register.
+ * alarmid - Identifies the alarm to be cancelled
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_rdalarm(FAR struct timespec *tp, uint32_t alarmid)
+{
+ irqstate_t flags;
+ FAR struct alm_cbinfo_s *cbinfo;
+ DEBUGASSERT(tp != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) &&
+ (alarmid < RTC_ALARM_LAST));
+
+ flags = spin_lock_irqsave(NULL);
+
+ /* Set the alarm in hardware and enable interrupts */
Review comment:
This comments seem to be out of context.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644050086
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
Review comment:
I suggest to use meaninfull descriptions here.
I often take them from the driver peripheral header file in the section "IOCTLS":
PTAL: https://github.com/apache/incubator-nuttx/blob/master/include/nuttx/timers/rtc.h#L109
For example:
```suggestion
* Returns the current RTC time.
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644036150
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
Review comment:
```suggestion
.ops = &g_rtc_ops,
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646254010
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644081147
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
+ return ret;
+
+#else
+ time_t timer;
+
+ /* The resolution of time is only 1 second */
+
+ timer = up_rtc_time();
+
+ /* Convert the one second epoch time to a struct tm */
+
+ if (gmtime_r(&timer, (FAR struct tm *)rtctime) == 0)
+ {
+ int errcode = get_errno();
+ DEBUGASSERT(errcode > 0);
+
+ rtcerr("ERROR: gmtime_r failed: %d\n", errcode);
+ return -errcode;
+ }
+
+ return OK;
+#endif
+}
+
+/****************************************************************************
+ * Name: rtc_settime
+ *
+ * Description:
+ * Implements the settime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The new time to set
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ struct timespec ts;
+
+ /* Convert the struct rtc_time to a time_t. Here we assume that struct
+ * rtc_time is cast compatible with struct tm.
+ */
+
+ ts.tv_sec = mktime((FAR struct tm *)rtctime);
+ ts.tv_nsec = 0;
+
+ /* Now set the time (to one second accuracy) */
+
+ return up_rtc_settime(&ts);
+}
+
+/****************************************************************************
+ * Name: rtc_havesettime
+ *
+ * Description:
+ * Implements the havesettime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ *
+ * Returned Value:
+ * Returns true if RTC date-time have been previously set.
+ *
+ ****************************************************************************/
+
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower)
+{
+ if (esp32c3_rtc_get_boot_time() == 0)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+/****************************************************************************
+ * Name: rtc_setalarm
+ *
+ * Description:
+ * Set a new alarm. This function implements the setalarm() method of the
+ * RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ struct alm_setalarm_s lowerinfo;
+ int ret = -EINVAL;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ priv = (FAR struct esp32c3_lowerhalf_s *)lower;
+
+ /* Remember the callback information */
+
+ cbinfo = &priv->cbinfo[alarminfo->id];
+ cbinfo->cb = alarminfo->cb;
+ cbinfo->priv = alarminfo->priv;
+
+ /* Set the alarm */
+
+ lowerinfo.as_id = alarminfo->id;
+ lowerinfo.as_cb = rtc_alarm_callback;
+ lowerinfo.as_arg = priv;
+
+ /* Convert the RTC time to a timespec (1 second accuracy) */
+
+ lowerinfo.as_time.tv_sec = mktime((FAR struct tm *)&alarminfo->time);
+ lowerinfo.as_time.tv_nsec = 0;
+
+ /* And set the alarm */
+
+ ret = up_rtc_setalarm(&lowerinfo);
+ if (ret < 0)
+ {
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_setrelative
+ *
+ * Description:
+ * Set a new alarm relative to the current time. This function implements
+ * the setrelative() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to set the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo)
+{
+ struct lower_setalarm_s setalarm;
+ time_t seconds;
+ int ret = -EINVAL;
+ irqstate_t flags;
+
+ DEBUGASSERT(lower != NULL && alarminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarminfo->id) &&
+ (alarminfo->id < RTC_ALARM_LAST));
+
+ if (alarminfo->reltime > 0)
+ {
+ flags = spin_lock_irqsave(NULL);
+
+ seconds = alarminfo->reltime;
+ gmtime_r(&seconds, (FAR struct tm *)&setalarm.time);
+
+ /* The set the alarm using this absolute time */
+
+ setalarm.id = alarminfo->id;
+ setalarm.cb = alarminfo->cb;
+ setalarm.priv = alarminfo->priv;
+ ret = rtc_setalarm(lower, &setalarm);
+
+ spin_unlock_irqrestore(NULL, flags);
+ }
+
+ return ret;
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_cancelalarm
+ *
+ * Description:
+ * Cancel the current alarm. This function implements the cancelalarm()
+ * method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarmid - the alarm id
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *priv;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+
+ DEBUGASSERT(lower != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ priv = (FAR struct esp32c3_lowerhalf_s *)lower;
+
+ /* Nullify callback information to reduce window for race conditions */
+
+ cbinfo = &priv->cbinfo[alarmid];
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Then cancel the alarm */
+
+ return up_rtc_cancelalarm((enum alm_id_e)alarmid);
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdalarm
+ *
+ * Description:
+ * Query the RTC alarm.
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * alarminfo - Provided information needed to query the alarm
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo)
+{
+ struct timespec ts;
+ int ret = -EINVAL;
Review comment:
again
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] gustavonihei commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
gustavonihei commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644282367
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -210,18 +214,26 @@
*/
#define RTC_CONFIG_DEFAULT() {\
- .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
- .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
- .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
- .clkctl_init = 1, \
- .pwrctl_init = 1, \
- .rtc_dboost_fpd = 1, \
- .xtal_fpu = 0, \
- .bbpll_fpu = 0, \
- .cpu_waiti_clk_gate = 1, \
- .cali_ocode = 0\
+ .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
+ .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
+ .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
+ .clkctl_init = 1, \
+ .pwrctl_init = 1, \
+ .rtc_dboost_fpd = 1, \
+ .xtal_fpu = 0, \
+ .bbpll_fpu = 0, \
+ .cpu_waiti_clk_gate = 1, \
+ .cali_ocode = 0\
}
+#ifdef CONFIG_RTC_DRIVER
+/* The magic data for the struct esp32c3_rtc_backup_s that is in RTC slow
+ * memory.
+ */
+
+# define MAGIC_RTC_SAVE (0x11223344556677ull)
Review comment:
```suggestion
# define MAGIC_RTC_SAVE (UINT64_C(0x11223344556677))
```
nit: Use the definitions from `inttypes.h` for applying the suffix to integer constants
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#issuecomment-858580628
LGTM
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642429782
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
Review comment:
Maybe you should consider make this snippet be conditioned also to `CONFIG_RTC_DATETIME`.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644034107
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
Review comment:
Could you please rename these operations to use `esp32c3_rtc_lh_` as the prefix, instead of `rtc_`?
Reason:
NuttX coding style determines the functions' name should be prefixed with the module it is.
PTAL: https://nuttx.apache.org/docs/latest/contributing/coding_style.html#function-naming
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642420514
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -1263,6 +1330,50 @@ static void IRAM_ATTR esp32c3_rtc_clk_cpu_freq_to_pll_mhz(
ets_update_cpu_frequency(cpu_freq_mhz);
}
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: esp32c3_rt_cb_handler
+ *
+ * Description:
+ * RT-Timer service routine
+ *
+ * Input Parameters:
+ * arg - Information about the RT-Timer configuration.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+static void IRAM_ATTR esp32c3_rt_cb_handler(FAR void *arg)
+{
+ FAR struct alm_cbinfo_s *cbinfo = (struct alm_cbinfo_s *)arg;
+ alm_callback_t cb;
+ FAR void *cb_arg;
+ int alminfo_id;
+
+ DEBUGASSERT(cbinfo != NULL);
+ alminfo_id = cbinfo->index;
+ DEBUGASSERT((RTC_ALARM0 <= alminfo_id) &&
+ (alminfo_id < RTC_ALARM_LAST));
+
+ if (cbinfo->ac_cb != NULL)
+ {
+ /* Alarm callback */
+
+ cb = cbinfo->ac_cb;
+ cb_arg = (FAR void *)cbinfo->ac_arg;
+
Review comment:
```suggestion
```
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646251997
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -349,6 +361,49 @@ struct esp32c3_rtc_sleep_config_s
uint32_t light_slp_reject : 1;
};
+#ifdef CONFIG_RTC_DRIVER
+
+#ifdef CONFIG_RTC_ALARM
+struct alm_cbinfo_s
+{
+ struct rt_timer_s *alarm_hdl; /* timer id point to here */
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646529460
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
Review comment:
good
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642432397
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
Review comment:
@cwespressif can you please add some comment here to explain the difference of one flow to another on behalf of good documentation of this code?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646257410
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r648842406
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
Review comment:
According to the discussion, I have changed the `rtc_` prefix to `rtc_lh_`
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646257255
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] acassis merged pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
acassis merged pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642440585
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
Review comment:
Is it really positive when fails?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r642438317
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
Review comment:
Only a comment: In the future maybe we can let `up_rtc_gettime` with the HW RTC and this one with the SYSTIMER.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646253609
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
Review comment:
If `CONFIG_RTC `or `CONFIG_RTC_DATETIME ` is set, we can get the time through `up_rtc_time`
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646255498
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -2135,3 +2246,415 @@ void IRAM_ATTR esp32c3_rtc_sleep_set_wakeup_time(uint64_t t)
modifyreg32(RTC_CNTL_INT_CLR_REG, 0, RTC_CNTL_MAIN_TIMER_INT_CLR_M);
modifyreg32(RTC_CNTL_SLP_TIMER1_REG, 0, RTC_CNTL_MAIN_TIMER_ALARM_EN_M);
}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_set_boot_time
+ *
+ * Description:
+ * Set time to RTC register to replace the original boot time.
+ *
+ * Input Parameters:
+ * time_us - set time in microseconds.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+void IRAM_ATTR esp32c3_rtc_set_boot_time(uint64_t time_us)
+{
+ putreg32((uint32_t)(time_us & 0xffffffff), RTC_BOOT_TIME_LOW_REG);
+ putreg32((uint32_t)(time_us >> 32), RTC_BOOT_TIME_HIGH_REG);
+}
+
+/****************************************************************************
+ * Name: esp32c3_rtc_get_boot_time
+ *
+ * Description:
+ * Get time of RTC register to indicate the original boot time.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * time_us - get time in microseconds.
+ *
+ ****************************************************************************/
+
+uint64_t IRAM_ATTR esp32c3_rtc_get_boot_time(void)
+{
+ return ((uint64_t)getreg32(RTC_BOOT_TIME_LOW_REG))
+ + (((uint64_t)getreg32(RTC_BOOT_TIME_HIGH_REG)) << 32);
+}
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Name: up_rtc_time
+ *
+ * Description:
+ * Get the current time in seconds. This is similar to the standard time()
+ * function. This interface is only required if the low-resolution
+ * RTC/counter hardware implementation selected. It is only used by the
+ * RTOS during initialization to set up the system time when CONFIG_RTC is
+ * set but neither CONFIG_RTC_HIRES nor CONFIG_RTC_DATETIME are set.
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * The current time in seconds
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_RTC_HIRES
+time_t up_rtc_time(void)
+{
+ uint64_t time_us;
+ irqstate_t flags;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return (time_t)(time_us / USEC_PER_SEC);
+}
+#endif /* CONFIG_RTC_HIRES */
+
+/****************************************************************************
+ * Name: up_rtc_settime
+ *
+ * Description:
+ * Set the RTC to the provided time. All RTC implementations must be
+ * able to set their time based on a standard timespec.
+ *
+ * Input Parameters:
+ * tp - the time to use
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_settime(FAR const struct timespec *ts)
+{
+ irqstate_t flags;
+ uint64_t now_us;
+ uint64_t rtc_offset_us;
+
+ DEBUGASSERT(ts != NULL && ts->tv_nsec < NSEC_PER_SEC);
+ flags = spin_lock_irqsave(NULL);
+
+ now_us = ((uint64_t) ts->tv_sec) * USEC_PER_SEC +
+ ts->tv_nsec / NSEC_PER_USEC;
+ if (g_rt_timer_enabled == true)
+ {
+ rtc_offset_us = now_us - rt_timer_time_us();
+ }
+ else
+ {
+ rtc_offset_us = now_us - esp32c3_rtc_get_time_us();
+ }
+
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(rtc_offset_us);
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_initialize
+ *
+ * Description:
+ * Initialize the hardware RTC per the selected configuration.
+ * This function is called once during the OS initialization sequence
+ *
+ * Input Parameters:
+ * None
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_initialize(void)
+{
+#ifndef CONFIG_PM
+ /* Initialize RTC controller parameters */
+
+ esp32c3_rtc_init();
+ esp32c3_rtc_clk_set();
+#endif
+
+ g_rtc_save = &rtc_saved_data;
+
+ /* If saved data is invalid, clear offset information */
+
+ if (g_rtc_save->magic != MAGIC_RTC_SAVE)
+ {
+ g_rtc_save->magic = MAGIC_RTC_SAVE;
+ g_rtc_save->offset = 0;
+ esp32c3_rtc_set_boot_time(0);
+ }
+
+#ifdef CONFIG_RTC_HIRES
+ /* Synchronize the base time to the RTC time */
+
+ up_rtc_gettime(&g_basetime);
+#endif
+
+ g_rtc_enabled = true;
+
+ return OK;
+}
+
+/****************************************************************************
+ * Name: up_rtc_gettime
+ *
+ * Description:
+ * Get the current time from the high resolution RTC time or RT-Timer. This
+ * interface is only supported by the high-resolution RTC/counter hardware
+ * implementation. It is used to replace the system timer.
+ *
+ * Input Parameters:
+ * tp - The location to return the RTC time or RT-Timer value.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_HIRES
+int up_rtc_gettime(FAR struct timespec *tp)
+{
+ irqstate_t flags;
+ uint64_t time_us;
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (g_rt_timer_enabled == true)
+ {
+ time_us = rt_timer_time_us() + g_rtc_save->offset +
+ esp32c3_rtc_get_boot_time();
+ }
+ else
+ {
+ time_us = = esp32c3_rtc_get_time_us() +
+ esp32c3_rtc_get_boot_time();
+ }
+
+ tp->tv_sec = time_us / USEC_PER_SEC;
+ tp->tv_nsec = (time_us % USEC_PER_SEC) * NSEC_PER_USEC;
+
+ spin_unlock_irqrestore(NULL, flags);
+
+ return OK;
+}
+#endif /* CONFIG_RTC_HIRES */
+
+#ifdef CONFIG_RTC_ALARM
+
+/****************************************************************************
+ * Name: up_rtc_setalarm
+ *
+ * Description:
+ * Set up an alarm.
+ *
+ * Input Parameters:
+ * alminfo - Information about the alarm configuration.
+ *
+ * Returned Value:
+ * Zero (OK) on success; a negated errno on failure
+ *
+ ****************************************************************************/
+
+int up_rtc_setalarm(FAR struct alm_setalarm_s *alminfo)
+{
+ struct rt_timer_args_s rt_timer_args;
+ FAR struct alm_cbinfo_s *cbinfo;
+ irqstate_t flags;
+ int ret = -EBUSY;
+ int id;
+
+ DEBUGASSERT(alminfo != NULL);
+ DEBUGASSERT((RTC_ALARM0 <= alminfo->as_id) &&
+ (alminfo->as_id < RTC_ALARM_LAST));
+
+ /* Set the alarm in RT-Timer */
+
+ id = alminfo->as_id;
+ cbinfo = &g_alarmcb[id];
+
+ if (cbinfo->ac_cb == NULL)
+ {
+ /* Create the RT-Timer alarm */
+
+ flags = spin_lock_irqsave(NULL);
+
+ if (cbinfo->alarm_hdl == NULL)
+ {
+ cbinfo->index = id;
+ rt_timer_args.arg = cbinfo;
+ rt_timer_args.callback = esp32c3_rt_cb_handler;
+ ret = rt_timer_create(&rt_timer_args, &cbinfo->alarm_hdl);
+ if (ret)
Review comment:
It has been modified.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r640595997
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -210,18 +214,22 @@
*/
#define RTC_CONFIG_DEFAULT() {\
- .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
- .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
- .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
- .clkctl_init = 1, \
- .pwrctl_init = 1, \
- .rtc_dboost_fpd = 1, \
- .xtal_fpu = 0, \
- .bbpll_fpu = 0, \
- .cpu_waiti_clk_gate = 1, \
- .cali_ocode = 0\
+ .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
+ .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
+ .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
+ .clkctl_init = 1, \
+ .pwrctl_init = 1, \
+ .rtc_dboost_fpd = 1, \
+ .xtal_fpu = 0, \
+ .bbpll_fpu = 0, \
+ .cpu_waiti_clk_gate = 1, \
+ .cali_ocode = 0\
}
+#ifdef CONFIG_RTC_DRIVER
+# define MAGIC_RTC_SAVE (0x11223344556677ull)
Review comment:
pls,
add a description for this number (a justification)
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] cwespressif commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
cwespressif commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r646257854
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc.c
##########
@@ -210,18 +214,26 @@
*/
#define RTC_CONFIG_DEFAULT() {\
- .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
- .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
- .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
- .clkctl_init = 1, \
- .pwrctl_init = 1, \
- .rtc_dboost_fpd = 1, \
- .xtal_fpu = 0, \
- .bbpll_fpu = 0, \
- .cpu_waiti_clk_gate = 1, \
- .cali_ocode = 0\
+ .ck8m_wait = RTC_CNTL_CK8M_WAIT_DEFAULT, \
+ .xtal_wait = RTC_CNTL_XTL_BUF_WAIT_DEFAULT, \
+ .pll_wait = RTC_CNTL_PLL_BUF_WAIT_DEFAULT, \
+ .clkctl_init = 1, \
+ .pwrctl_init = 1, \
+ .rtc_dboost_fpd = 1, \
+ .xtal_fpu = 0, \
+ .bbpll_fpu = 0, \
+ .cpu_waiti_clk_gate = 1, \
+ .cali_ocode = 0\
}
+#ifdef CONFIG_RTC_DRIVER
+/* The magic data for the struct esp32c3_rtc_backup_s that is in RTC slow
+ * memory.
+ */
+
+# define MAGIC_RTC_SAVE (0x11223344556677ull)
Review comment:
Done
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx] saramonteiro commented on a change in pull request #3794: risc-v/esp32c3: Support ESP32-C3 RTC driver
Posted by GitBox <gi...@apache.org>.
saramonteiro commented on a change in pull request #3794:
URL: https://github.com/apache/incubator-nuttx/pull/3794#discussion_r644067647
##########
File path: arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
##########
@@ -0,0 +1,575 @@
+/****************************************************************************
+ * arch/risc-v/src/esp32c3/esp32c3_rtc_lowerhalf.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+#include <nuttx/spinlock.h>
+
+#include <sys/types.h>
+#include <stdbool.h>
+#include <string.h>
+#include <errno.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+
+#include "esp32c3_rtc.h"
+#include "hardware/esp32c3_tim.h"
+
+#ifdef CONFIG_RTC_DRIVER
+
+/****************************************************************************
+ * Private Types
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+struct esp32c3_cbinfo_s
+{
+ volatile rtc_alarm_callback_t cb; /* Callback when the alarm expires */
+ volatile FAR void *priv; /* Private argurment to accompany callback */
+};
+#endif
+
+/* This is the private type for the RTC state. It must be cast compatible
+ * with struct rtc_lowerhalf_s.
+ */
+
+struct esp32c3_lowerhalf_s
+{
+ /* This is the contained reference to the read-only, lower-half
+ * operations vtable (which may lie in FLASH or ROM)
+ */
+
+ FAR const struct rtc_ops_s *ops;
+#ifdef CONFIG_RTC_ALARM
+ /* Alarm callback information */
+
+ struct esp32c3_cbinfo_s cbinfo[RTC_ALARM_LAST];
+#endif
+};
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+/* Prototypes for static methods in struct rtc_ops_s */
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid);
+static int rtc_setalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setalarm_s *alarminfo);
+static int rtc_setrelative(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct lower_setrelative_s *alarminfo);
+static int rtc_cancelalarm(FAR struct rtc_lowerhalf_s *lower,
+ int alarmid);
+static int rtc_rdalarm(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct lower_rdalarm_s *alarminfo);
+#endif
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+/* ESP32-C3 RTC driver operations */
+
+static const struct rtc_ops_s g_rtc_ops =
+{
+ .rdtime = rtc_rdtime,
+ .settime = rtc_settime,
+ .havesettime = rtc_havesettime,
+#ifdef CONFIG_RTC_ALARM
+ .setalarm = rtc_setalarm,
+ .setrelative = rtc_setrelative,
+ .cancelalarm = rtc_cancelalarm,
+ .rdalarm = rtc_rdalarm,
+#endif
+#ifdef CONFIG_RTC_PERIODIC
+ .setperiodic = NULL,
+ .cancelperiodic = NULL,
+#endif
+#ifdef CONFIG_RTC_IOCTL
+ .ioctl = NULL,
+#endif
+#ifndef CONFIG_DISABLE_PSEUDOFS_OPERATIONS
+ .destroy = NULL,
+#endif
+};
+
+/* ESP32-C3 RTC device state */
+
+static struct esp32c3_lowerhalf_s g_rtc_lowerhalf =
+{
+ .ops = &g_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: rtc_alarm_callback
+ *
+ * Description:
+ * This is the function that is called from the RTC driver when the alarm
+ * goes off. It just invokes the upper half drivers callback.
+ *
+ * Returned Value:
+ * None
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_RTC_ALARM
+static void rtc_alarm_callback(FAR void *arg, unsigned int alarmid)
+{
+ FAR struct esp32c3_lowerhalf_s *lower;
+ FAR struct esp32c3_cbinfo_s *cbinfo;
+ rtc_alarm_callback_t cb;
+ FAR void *priv;
+
+ DEBUGASSERT((RTC_ALARM0 <= alarmid) && (alarmid < RTC_ALARM_LAST));
+
+ lower = (struct esp32c3_lowerhalf_s *)arg;
+ cbinfo = &lower->cbinfo[alarmid];
+
+ /* Sample and clear the callback information to minimize the window in
+ * time in which race conditions can occur.
+ */
+
+ cb = (rtc_alarm_callback_t)cbinfo->cb;
+ priv = (FAR void *)cbinfo->priv;
+
+ cbinfo->cb = NULL;
+ cbinfo->priv = NULL;
+
+ /* Perform the callback */
+
+ if (cb != NULL)
+ {
+ cb(priv, alarmid);
+ }
+}
+#endif /* CONFIG_RTC_ALARM */
+
+/****************************************************************************
+ * Name: rtc_rdtime
+ *
+ * Description:
+ * Implements the rdtime() method of the RTC driver interface
+ *
+ * Input Parameters:
+ * lower - A reference to RTC lower half driver state structure
+ * rcttime - The location in which to return the current RTC time.
+ *
+ * Returned Value:
+ * Zero (OK) is returned on success; a negated errno value is returned
+ * on any failure.
+ *
+ ****************************************************************************/
+
+static int rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+#if defined(CONFIG_RTC_DATETIME)
+ /* This operation depends on the fact that struct rtc_time is cast
+ * compatible with struct tm.
+ */
+
+ return up_rtc_getdatetime((FAR struct tm *)rtctime);
+
+#elif defined(CONFIG_RTC_HIRES)
+ FAR struct timespec ts;
+ int ret;
+
+ /* Get the higher resolution time */
+
+ ret = up_rtc_gettime(&ts);
+ if (ret < 0)
+ {
+ goto errout;
+ }
+
+ /* Convert the one second epoch time to a struct tm. This operation
+ * depends on the fact that struct rtc_time and struct tm are cast
+ * compatible.
+ */
+
+ if (!gmtime_r(&ts.tv_sec, (FAR struct tm *)rtctime))
+ {
+ ret = -get_errno();
+ goto errout;
+ }
+
+ return OK;
+
+errout:
+ DEBUGASSERT(ret < 0);
Review comment:
I think it's redundant
This label will be used only in case ret < 0.
Consider removing it and use rtc error debug messages in case of error.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org