You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@cordova.apache.org by za...@apache.org on 2015/02/20 20:37:56 UTC
[02/20] cordova-firefoxos git commit: CB-7567 Don't use adm-zip
because it creates invalid zip files
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/README.md
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/bl/README.md b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/README.md
new file mode 100644
index 0000000..6b7fb6d
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/README.md
@@ -0,0 +1,198 @@
+# bl *(BufferList)*
+
+**A Node.js Buffer list collector, reader and streamer thingy.**
+
+[](https://nodei.co/npm/bl/)
+[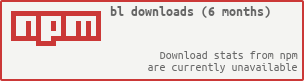](https://nodei.co/npm/bl/)
+
+**bl** is a storage object for collections of Node Buffers, exposing them with the main Buffer readable API. Also works as a duplex stream so you can collect buffers from a stream that emits them and emit buffers to a stream that consumes them!
+
+The original buffers are kept intact and copies are only done as necessary. Any reads that require the use of a single original buffer will return a slice of that buffer only (which references the same memory as the original buffer). Reads that span buffers perform concatenation as required and return the results transparently.
+
+```js
+const BufferList = require('bl')
+
+var bl = new BufferList()
+bl.append(new Buffer('abcd'))
+bl.append(new Buffer('efg'))
+bl.append('hi') // bl will also accept & convert Strings
+bl.append(new Buffer('j'))
+bl.append(new Buffer([ 0x3, 0x4 ]))
+
+console.log(bl.length) // 12
+
+console.log(bl.slice(0, 10).toString('ascii')) // 'abcdefghij'
+console.log(bl.slice(3, 10).toString('ascii')) // 'defghij'
+console.log(bl.slice(3, 6).toString('ascii')) // 'def'
+console.log(bl.slice(3, 8).toString('ascii')) // 'defgh'
+console.log(bl.slice(5, 10).toString('ascii')) // 'fghij'
+
+// or just use toString!
+console.log(bl.toString()) // 'abcdefghij\u0003\u0004'
+console.log(bl.toString('ascii', 3, 8)) // 'defgh'
+console.log(bl.toString('ascii', 5, 10)) // 'fghij'
+
+// other standard Buffer readables
+console.log(bl.readUInt16BE(10)) // 0x0304
+console.log(bl.readUInt16LE(10)) // 0x0403
+```
+
+Give it a callback in the constructor and use it just like **[concat-stream](https://github.com/maxogden/node-concat-stream)**:
+
+```js
+const bl = require('bl')
+ , fs = require('fs')
+
+fs.createReadStream('README.md')
+ .pipe(bl(function (err, data) { // note 'new' isn't strictly required
+ // `data` is a complete Buffer object containing the full data
+ console.log(data.toString())
+ }))
+```
+
+Note that when you use the *callback* method like this, the resulting `data` parameter is a concatenation of all `Buffer` objects in the list. If you want to avoid the overhead of this concatenation (in cases of extreme performance consciousness), then avoid the *callback* method and just listen to `'end'` instead, like a standard Stream.
+
+Or to fetch a URL using [hyperquest](https://github.com/substack/hyperquest) (should work with [request](http://github.com/mikeal/request) and even plain Node http too!):
+```js
+const hyperquest = require('hyperquest')
+ , bl = require('bl')
+ , url = 'https://raw.github.com/rvagg/bl/master/README.md'
+
+hyperquest(url).pipe(bl(function (err, data) {
+ console.log(data.toString())
+}))
+```
+
+Or, use it as a readable stream to recompose a list of Buffers to an output source:
+
+```js
+const BufferList = require('bl')
+ , fs = require('fs')
+
+var bl = new BufferList()
+bl.append(new Buffer('abcd'))
+bl.append(new Buffer('efg'))
+bl.append(new Buffer('hi'))
+bl.append(new Buffer('j'))
+
+bl.pipe(fs.createWriteStream('gibberish.txt'))
+```
+
+## API
+
+ * <a href="#ctor"><code><b>new BufferList([ callback ])</b></code></a>
+ * <a href="#length"><code>bl.<b>length</b></code></a>
+ * <a href="#append"><code>bl.<b>append(buffer)</b></code></a>
+ * <a href="#get"><code>bl.<b>get(index)</b></code></a>
+ * <a href="#slice"><code>bl.<b>slice([ start[, end ] ])</b></code></a>
+ * <a href="#copy"><code>bl.<b>copy(dest, [ destStart, [ srcStart [, srcEnd ] ] ])</b></code></a>
+ * <a href="#duplicate"><code>bl.<b>duplicate()</b></code></a>
+ * <a href="#consume"><code>bl.<b>consume(bytes)</b></code></a>
+ * <a href="#toString"><code>bl.<b>toString([encoding, [ start, [ end ]]])</b></code></a>
+ * <a href="#readXX"><code>bl.<b>readDoubleBE()</b></code>, <code>bl.<b>readDoubleLE()</b></code>, <code>bl.<b>readFloatBE()</b></code>, <code>bl.<b>readFloatLE()</b></code>, <code>bl.<b>readInt32BE()</b></code>, <code>bl.<b>readInt32LE()</b></code>, <code>bl.<b>readUInt32BE()</b></code>, <code>bl.<b>readUInt32LE()</b></code>, <code>bl.<b>readInt16BE()</b></code>, <code>bl.<b>readInt16LE()</b></code>, <code>bl.<b>readUInt16BE()</b></code>, <code>bl.<b>readUInt16LE()</b></code>, <code>bl.<b>readInt8()</b></code>, <code>bl.<b>readUInt8()</b></code></a>
+ * <a href="#streams">Streams</a>
+
+--------------------------------------------------------
+<a name="ctor"></a>
+### new BufferList([ callback | buffer | buffer array ])
+The constructor takes an optional callback, if supplied, the callback will be called with an error argument followed by a reference to the **bl** instance, when `bl.end()` is called (i.e. from a piped stream). This is a convenient method of collecting the entire contents of a stream, particularly when the stream is *chunky*, such as a network stream.
+
+Normally, no arguments are required for the constructor, but you can initialise the list by passing in a single `Buffer` object or an array of `Buffer` object.
+
+`new` is not strictly required, if you don't instantiate a new object, it will be done automatically for you so you can create a new instance simply with:
+
+```js
+var bl = require('bl')
+var myinstance = bl()
+
+// equivilant to:
+
+var BufferList = require('bl')
+var myinstance = new BufferList()
+```
+
+--------------------------------------------------------
+<a name="length"></a>
+### bl.length
+Get the length of the list in bytes. This is the sum of the lengths of all of the buffers contained in the list, minus any initial offset for a semi-consumed buffer at the beginning. Should accurately represent the total number of bytes that can be read from the list.
+
+--------------------------------------------------------
+<a name="append"></a>
+### bl.append(buffer)
+`append(buffer)` adds an additional buffer or BufferList to the internal list.
+
+--------------------------------------------------------
+<a name="get"></a>
+### bl.get(index)
+`get()` will return the byte at the specified index.
+
+--------------------------------------------------------
+<a name="slice"></a>
+### bl.slice([ start, [ end ] ])
+`slice()` returns a new `Buffer` object containing the bytes within the range specified. Both `start` and `end` are optional and will default to the beginning and end of the list respectively.
+
+If the requested range spans a single internal buffer then a slice of that buffer will be returned which shares the original memory range of that Buffer. If the range spans multiple buffers then copy operations will likely occur to give you a uniform Buffer.
+
+--------------------------------------------------------
+<a name="copy"></a>
+### bl.copy(dest, [ destStart, [ srcStart [, srcEnd ] ] ])
+`copy()` copies the content of the list in the `dest` buffer, starting from `destStart` and containing the bytes within the range specified with `srcStart` to `srcEnd`. `destStart`, `start` and `end` are optional and will default to the beginning of the `dest` buffer, and the beginning and end of the list respectively.
+
+--------------------------------------------------------
+<a name="duplicate"></a>
+### bl.duplicate()
+`duplicate()` performs a **shallow-copy** of the list. The internal Buffers remains the same, so if you change the underlying Buffers, the change will be reflected in both the original and the duplicate. This method is needed if you want to call `consume()` or `pipe()` and still keep the original list.Example:
+
+```js
+var bl = new BufferList()
+
+bl.append('hello')
+bl.append(' world')
+bl.append('\n')
+
+bl.duplicate().pipe(process.stdout, { end: false })
+
+console.log(bl.toString())
+```
+
+--------------------------------------------------------
+<a name="consume"></a>
+### bl.consume(bytes)
+`consume()` will shift bytes *off the start of the list*. The number of bytes consumed don't need to line up with the sizes of the internal Buffers—initial offsets will be calculated accordingly in order to give you a consistent view of the data.
+
+--------------------------------------------------------
+<a name="toString"></a>
+### bl.toString([encoding, [ start, [ end ]]])
+`toString()` will return a string representation of the buffer. The optional `start` and `end` arguments are passed on to `slice()`, while the `encoding` is passed on to `toString()` of the resulting Buffer. See the [Buffer#toString()](http://nodejs.org/docs/latest/api/buffer.html#buffer_buf_tostring_encoding_start_end) documentation for more information.
+
+--------------------------------------------------------
+<a name="readXX"></a>
+### bl.readDoubleBE(), bl.readDoubleLE(), bl.readFloatBE(), bl.readFloatLE(), bl.readInt32BE(), bl.readInt32LE(), bl.readUInt32BE(), bl.readUInt32LE(), bl.readInt16BE(), bl.readInt16LE(), bl.readUInt16BE(), bl.readUInt16LE(), bl.readInt8(), bl.readUInt8()
+
+All of the standard byte-reading methods of the `Buffer` interface are implemented and will operate across internal Buffer boundaries transparently.
+
+See the <b><code>[Buffer](http://nodejs.org/docs/latest/api/buffer.html)</code></b> documentation for how these work.
+
+--------------------------------------------------------
+<a name="streams"></a>
+### Streams
+**bl** is a Node **[Duplex Stream](http://nodejs.org/docs/latest/api/stream.html#stream_class_stream_duplex)**, so it can be read from and written to like a standard Node stream. You can also `pipe()` to and from a **bl** instance.
+
+--------------------------------------------------------
+
+## Contributors
+
+**bl** is brought to you by the following hackers:
+
+ * [Rod Vagg](https://github.com/rvagg)
+ * [Matteo Collina](https://github.com/mcollina)
+ * [Jarett Cruger](https://github.com/jcrugzz)
+
+=======
+
+<a name="license"></a>
+## License & copyright
+
+Copyright (c) 2013-2014 bl contributors (listed above).
+
+bl is licensed under the MIT license. All rights not explicitly granted in the MIT license are reserved. See the included LICENSE.md file for more details.
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/bl.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/bl/bl.js b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/bl.js
new file mode 100644
index 0000000..7a2f997
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/bl.js
@@ -0,0 +1,216 @@
+var DuplexStream = require('readable-stream/duplex')
+ , util = require('util')
+
+function BufferList (callback) {
+ if (!(this instanceof BufferList))
+ return new BufferList(callback)
+
+ this._bufs = []
+ this.length = 0
+
+ if (typeof callback == 'function') {
+ this._callback = callback
+
+ var piper = function (err) {
+ if (this._callback) {
+ this._callback(err)
+ this._callback = null
+ }
+ }.bind(this)
+
+ this.on('pipe', function (src) {
+ src.on('error', piper)
+ })
+ this.on('unpipe', function (src) {
+ src.removeListener('error', piper)
+ })
+ }
+ else if (Buffer.isBuffer(callback))
+ this.append(callback)
+ else if (Array.isArray(callback)) {
+ callback.forEach(function (b) {
+ Buffer.isBuffer(b) && this.append(b)
+ }.bind(this))
+ }
+
+ DuplexStream.call(this)
+}
+
+util.inherits(BufferList, DuplexStream)
+
+BufferList.prototype._offset = function (offset) {
+ var tot = 0, i = 0, _t
+ for (; i < this._bufs.length; i++) {
+ _t = tot + this._bufs[i].length
+ if (offset < _t)
+ return [ i, offset - tot ]
+ tot = _t
+ }
+}
+
+BufferList.prototype.append = function (buf) {
+ var isBuffer = Buffer.isBuffer(buf) ||
+ buf instanceof BufferList
+
+ this._bufs.push(isBuffer ? buf : new Buffer(buf))
+ this.length += buf.length
+ return this
+}
+
+BufferList.prototype._write = function (buf, encoding, callback) {
+ this.append(buf)
+ if (callback)
+ callback()
+}
+
+BufferList.prototype._read = function (size) {
+ if (!this.length)
+ return this.push(null)
+ size = Math.min(size, this.length)
+ this.push(this.slice(0, size))
+ this.consume(size)
+}
+
+BufferList.prototype.end = function (chunk) {
+ DuplexStream.prototype.end.call(this, chunk)
+
+ if (this._callback) {
+ this._callback(null, this.slice())
+ this._callback = null
+ }
+}
+
+BufferList.prototype.get = function (index) {
+ return this.slice(index, index + 1)[0]
+}
+
+BufferList.prototype.slice = function (start, end) {
+ return this.copy(null, 0, start, end)
+}
+
+BufferList.prototype.copy = function (dst, dstStart, srcStart, srcEnd) {
+ if (typeof srcStart != 'number' || srcStart < 0)
+ srcStart = 0
+ if (typeof srcEnd != 'number' || srcEnd > this.length)
+ srcEnd = this.length
+ if (srcStart >= this.length)
+ return dst || new Buffer(0)
+ if (srcEnd <= 0)
+ return dst || new Buffer(0)
+
+ var copy = !!dst
+ , off = this._offset(srcStart)
+ , len = srcEnd - srcStart
+ , bytes = len
+ , bufoff = (copy && dstStart) || 0
+ , start = off[1]
+ , l
+ , i
+
+ // copy/slice everything
+ if (srcStart === 0 && srcEnd == this.length) {
+ if (!copy) // slice, just return a full concat
+ return Buffer.concat(this._bufs)
+
+ // copy, need to copy individual buffers
+ for (i = 0; i < this._bufs.length; i++) {
+ this._bufs[i].copy(dst, bufoff)
+ bufoff += this._bufs[i].length
+ }
+
+ return dst
+ }
+
+ // easy, cheap case where it's a subset of one of the buffers
+ if (bytes <= this._bufs[off[0]].length - start) {
+ return copy
+ ? this._bufs[off[0]].copy(dst, dstStart, start, start + bytes)
+ : this._bufs[off[0]].slice(start, start + bytes)
+ }
+
+ if (!copy) // a slice, we need something to copy in to
+ dst = new Buffer(len)
+
+ for (i = off[0]; i < this._bufs.length; i++) {
+ l = this._bufs[i].length - start
+
+ if (bytes > l) {
+ this._bufs[i].copy(dst, bufoff, start)
+ } else {
+ this._bufs[i].copy(dst, bufoff, start, start + bytes)
+ break
+ }
+
+ bufoff += l
+ bytes -= l
+
+ if (start)
+ start = 0
+ }
+
+ return dst
+}
+
+BufferList.prototype.toString = function (encoding, start, end) {
+ return this.slice(start, end).toString(encoding)
+}
+
+BufferList.prototype.consume = function (bytes) {
+ while (this._bufs.length) {
+ if (bytes > this._bufs[0].length) {
+ bytes -= this._bufs[0].length
+ this.length -= this._bufs[0].length
+ this._bufs.shift()
+ } else {
+ this._bufs[0] = this._bufs[0].slice(bytes)
+ this.length -= bytes
+ break
+ }
+ }
+ return this
+}
+
+BufferList.prototype.duplicate = function () {
+ var i = 0
+ , copy = new BufferList()
+
+ for (; i < this._bufs.length; i++)
+ copy.append(this._bufs[i])
+
+ return copy
+}
+
+BufferList.prototype.destroy = function () {
+ this._bufs.length = 0;
+ this.length = 0;
+ this.push(null);
+}
+
+;(function () {
+ var methods = {
+ 'readDoubleBE' : 8
+ , 'readDoubleLE' : 8
+ , 'readFloatBE' : 4
+ , 'readFloatLE' : 4
+ , 'readInt32BE' : 4
+ , 'readInt32LE' : 4
+ , 'readUInt32BE' : 4
+ , 'readUInt32LE' : 4
+ , 'readInt16BE' : 2
+ , 'readInt16LE' : 2
+ , 'readUInt16BE' : 2
+ , 'readUInt16LE' : 2
+ , 'readInt8' : 1
+ , 'readUInt8' : 1
+ }
+
+ for (var m in methods) {
+ (function (m) {
+ BufferList.prototype[m] = function (offset) {
+ return this.slice(offset, offset + methods[m])[m](0)
+ }
+ }(m))
+ }
+}())
+
+module.exports = BufferList
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/package.json
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/bl/package.json b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/package.json
new file mode 100644
index 0000000..3ffbd6a
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/package.json
@@ -0,0 +1,62 @@
+{
+ "name": "bl",
+ "version": "0.9.4",
+ "description": "Buffer List: collect buffers and access with a standard readable Buffer interface, streamable too!",
+ "main": "bl.js",
+ "scripts": {
+ "test": "node test/test.js | faucet",
+ "test-local": "brtapsauce-local test/basic-test.js"
+ },
+ "repository": {
+ "type": "git",
+ "url": "https://github.com/rvagg/bl.git"
+ },
+ "homepage": "https://github.com/rvagg/bl",
+ "authors": [
+ "Rod Vagg <ro...@vagg.org> (https://github.com/rvagg)",
+ "Matteo Collina <ma...@gmail.com> (https://github.com/mcollina)",
+ "Jarett Cruger <jc...@gmail.com> (https://github.com/jcrugzz)"
+ ],
+ "keywords": [
+ "buffer",
+ "buffers",
+ "stream",
+ "awesomesauce"
+ ],
+ "license": "MIT",
+ "dependencies": {
+ "readable-stream": "~1.0.26"
+ },
+ "devDependencies": {
+ "tape": "~2.12.3",
+ "hash_file": "~0.1.1",
+ "faucet": "~0.0.1",
+ "brtapsauce": "~0.3.0"
+ },
+ "gitHead": "e7f90703c5f90ca26f60455ea6ad0b6be4a9feee",
+ "bugs": {
+ "url": "https://github.com/rvagg/bl/issues"
+ },
+ "_id": "bl@0.9.4",
+ "_shasum": "4702ddf72fbe0ecd82787c00c113aea1935ad0e7",
+ "_from": "bl@>=0.9.0 <0.10.0",
+ "_npmVersion": "2.1.18",
+ "_nodeVersion": "1.0.3",
+ "_npmUser": {
+ "name": "rvagg",
+ "email": "rod@vagg.org"
+ },
+ "maintainers": [
+ {
+ "name": "rvagg",
+ "email": "rod@vagg.org"
+ }
+ ],
+ "dist": {
+ "shasum": "4702ddf72fbe0ecd82787c00c113aea1935ad0e7",
+ "tarball": "http://registry.npmjs.org/bl/-/bl-0.9.4.tgz"
+ },
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/bl/-/bl-0.9.4.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/basic-test.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/basic-test.js b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/basic-test.js
new file mode 100644
index 0000000..75116a3
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/basic-test.js
@@ -0,0 +1,541 @@
+var tape = require('tape')
+ , crypto = require('crypto')
+ , fs = require('fs')
+ , hash = require('hash_file')
+ , BufferList = require('../')
+
+ , encodings =
+ ('hex utf8 utf-8 ascii binary base64'
+ + (process.browser ? '' : ' ucs2 ucs-2 utf16le utf-16le')).split(' ')
+
+tape('single bytes from single buffer', function (t) {
+ var bl = new BufferList()
+ bl.append(new Buffer('abcd'))
+
+ t.equal(bl.length, 4)
+
+ t.equal(bl.get(0), 97)
+ t.equal(bl.get(1), 98)
+ t.equal(bl.get(2), 99)
+ t.equal(bl.get(3), 100)
+
+ t.end()
+})
+
+tape('single bytes from multiple buffers', function (t) {
+ var bl = new BufferList()
+ bl.append(new Buffer('abcd'))
+ bl.append(new Buffer('efg'))
+ bl.append(new Buffer('hi'))
+ bl.append(new Buffer('j'))
+
+ t.equal(bl.length, 10)
+
+ t.equal(bl.get(0), 97)
+ t.equal(bl.get(1), 98)
+ t.equal(bl.get(2), 99)
+ t.equal(bl.get(3), 100)
+ t.equal(bl.get(4), 101)
+ t.equal(bl.get(5), 102)
+ t.equal(bl.get(6), 103)
+ t.equal(bl.get(7), 104)
+ t.equal(bl.get(8), 105)
+ t.equal(bl.get(9), 106)
+ t.end()
+})
+
+tape('multi bytes from single buffer', function (t) {
+ var bl = new BufferList()
+ bl.append(new Buffer('abcd'))
+
+ t.equal(bl.length, 4)
+
+ t.equal(bl.slice(0, 4).toString('ascii'), 'abcd')
+ t.equal(bl.slice(0, 3).toString('ascii'), 'abc')
+ t.equal(bl.slice(1, 4).toString('ascii'), 'bcd')
+
+ t.end()
+})
+
+tape('multiple bytes from multiple buffers', function (t) {
+ var bl = new BufferList()
+
+ bl.append(new Buffer('abcd'))
+ bl.append(new Buffer('efg'))
+ bl.append(new Buffer('hi'))
+ bl.append(new Buffer('j'))
+
+ t.equal(bl.length, 10)
+
+ t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
+ t.equal(bl.slice(3, 10).toString('ascii'), 'defghij')
+ t.equal(bl.slice(3, 6).toString('ascii'), 'def')
+ t.equal(bl.slice(3, 8).toString('ascii'), 'defgh')
+ t.equal(bl.slice(5, 10).toString('ascii'), 'fghij')
+
+ t.end()
+})
+
+tape('multiple bytes from multiple buffer lists', function (t) {
+ var bl = new BufferList()
+
+ bl.append(new BufferList([new Buffer('abcd'), new Buffer('efg')]))
+ bl.append(new BufferList([new Buffer('hi'), new Buffer('j')]))
+
+ t.equal(bl.length, 10)
+
+ t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
+ t.equal(bl.slice(3, 10).toString('ascii'), 'defghij')
+ t.equal(bl.slice(3, 6).toString('ascii'), 'def')
+ t.equal(bl.slice(3, 8).toString('ascii'), 'defgh')
+ t.equal(bl.slice(5, 10).toString('ascii'), 'fghij')
+
+ t.end()
+})
+
+tape('consuming from multiple buffers', function (t) {
+ var bl = new BufferList()
+
+ bl.append(new Buffer('abcd'))
+ bl.append(new Buffer('efg'))
+ bl.append(new Buffer('hi'))
+ bl.append(new Buffer('j'))
+
+ t.equal(bl.length, 10)
+
+ t.equal(bl.slice(0, 10).toString('ascii'), 'abcdefghij')
+
+ bl.consume(3)
+ t.equal(bl.length, 7)
+ t.equal(bl.slice(0, 7).toString('ascii'), 'defghij')
+
+ bl.consume(2)
+ t.equal(bl.length, 5)
+ t.equal(bl.slice(0, 5).toString('ascii'), 'fghij')
+
+ bl.consume(1)
+ t.equal(bl.length, 4)
+ t.equal(bl.slice(0, 4).toString('ascii'), 'ghij')
+
+ bl.consume(1)
+ t.equal(bl.length, 3)
+ t.equal(bl.slice(0, 3).toString('ascii'), 'hij')
+
+ bl.consume(2)
+ t.equal(bl.length, 1)
+ t.equal(bl.slice(0, 1).toString('ascii'), 'j')
+
+ t.end()
+})
+
+tape('test readUInt8 / readInt8', function (t) {
+ var buf1 = new Buffer(1)
+ , buf2 = new Buffer(3)
+ , buf3 = new Buffer(3)
+ , bl = new BufferList()
+
+ buf2[1] = 0x3
+ buf2[2] = 0x4
+ buf3[0] = 0x23
+ buf3[1] = 0x42
+
+ bl.append(buf1)
+ bl.append(buf2)
+ bl.append(buf3)
+
+ t.equal(bl.readUInt8(2), 0x3)
+ t.equal(bl.readInt8(2), 0x3)
+ t.equal(bl.readUInt8(3), 0x4)
+ t.equal(bl.readInt8(3), 0x4)
+ t.equal(bl.readUInt8(4), 0x23)
+ t.equal(bl.readInt8(4), 0x23)
+ t.equal(bl.readUInt8(5), 0x42)
+ t.equal(bl.readInt8(5), 0x42)
+ t.end()
+})
+
+tape('test readUInt16LE / readUInt16BE / readInt16LE / readInt16BE', function (t) {
+ var buf1 = new Buffer(1)
+ , buf2 = new Buffer(3)
+ , buf3 = new Buffer(3)
+ , bl = new BufferList()
+
+ buf2[1] = 0x3
+ buf2[2] = 0x4
+ buf3[0] = 0x23
+ buf3[1] = 0x42
+
+ bl.append(buf1)
+ bl.append(buf2)
+ bl.append(buf3)
+
+ t.equal(bl.readUInt16BE(2), 0x0304)
+ t.equal(bl.readUInt16LE(2), 0x0403)
+ t.equal(bl.readInt16BE(2), 0x0304)
+ t.equal(bl.readInt16LE(2), 0x0403)
+ t.equal(bl.readUInt16BE(3), 0x0423)
+ t.equal(bl.readUInt16LE(3), 0x2304)
+ t.equal(bl.readInt16BE(3), 0x0423)
+ t.equal(bl.readInt16LE(3), 0x2304)
+ t.equal(bl.readUInt16BE(4), 0x2342)
+ t.equal(bl.readUInt16LE(4), 0x4223)
+ t.equal(bl.readInt16BE(4), 0x2342)
+ t.equal(bl.readInt16LE(4), 0x4223)
+ t.end()
+})
+
+tape('test readUInt32LE / readUInt32BE / readInt32LE / readInt32BE', function (t) {
+ var buf1 = new Buffer(1)
+ , buf2 = new Buffer(3)
+ , buf3 = new Buffer(3)
+ , bl = new BufferList()
+
+ buf2[1] = 0x3
+ buf2[2] = 0x4
+ buf3[0] = 0x23
+ buf3[1] = 0x42
+
+ bl.append(buf1)
+ bl.append(buf2)
+ bl.append(buf3)
+
+ t.equal(bl.readUInt32BE(2), 0x03042342)
+ t.equal(bl.readUInt32LE(2), 0x42230403)
+ t.equal(bl.readInt32BE(2), 0x03042342)
+ t.equal(bl.readInt32LE(2), 0x42230403)
+ t.end()
+})
+
+tape('test readFloatLE / readFloatBE', function (t) {
+ var buf1 = new Buffer(1)
+ , buf2 = new Buffer(3)
+ , buf3 = new Buffer(3)
+ , bl = new BufferList()
+
+ buf2[1] = 0x00
+ buf2[2] = 0x00
+ buf3[0] = 0x80
+ buf3[1] = 0x3f
+
+ bl.append(buf1)
+ bl.append(buf2)
+ bl.append(buf3)
+
+ t.equal(bl.readFloatLE(2), 0x01)
+ t.end()
+})
+
+tape('test readDoubleLE / readDoubleBE', function (t) {
+ var buf1 = new Buffer(1)
+ , buf2 = new Buffer(3)
+ , buf3 = new Buffer(10)
+ , bl = new BufferList()
+
+ buf2[1] = 0x55
+ buf2[2] = 0x55
+ buf3[0] = 0x55
+ buf3[1] = 0x55
+ buf3[2] = 0x55
+ buf3[3] = 0x55
+ buf3[4] = 0xd5
+ buf3[5] = 0x3f
+
+ bl.append(buf1)
+ bl.append(buf2)
+ bl.append(buf3)
+
+ t.equal(bl.readDoubleLE(2), 0.3333333333333333)
+ t.end()
+})
+
+tape('test toString', function (t) {
+ var bl = new BufferList()
+
+ bl.append(new Buffer('abcd'))
+ bl.append(new Buffer('efg'))
+ bl.append(new Buffer('hi'))
+ bl.append(new Buffer('j'))
+
+ t.equal(bl.toString('ascii', 0, 10), 'abcdefghij')
+ t.equal(bl.toString('ascii', 3, 10), 'defghij')
+ t.equal(bl.toString('ascii', 3, 6), 'def')
+ t.equal(bl.toString('ascii', 3, 8), 'defgh')
+ t.equal(bl.toString('ascii', 5, 10), 'fghij')
+
+ t.end()
+})
+
+tape('test toString encoding', function (t) {
+ var bl = new BufferList()
+ , b = new Buffer('abcdefghij\xff\x00')
+
+ bl.append(new Buffer('abcd'))
+ bl.append(new Buffer('efg'))
+ bl.append(new Buffer('hi'))
+ bl.append(new Buffer('j'))
+ bl.append(new Buffer('\xff\x00'))
+
+ encodings.forEach(function (enc) {
+ t.equal(bl.toString(enc), b.toString(enc), enc)
+ })
+
+ t.end()
+})
+
+!process.browser && tape('test stream', function (t) {
+ var random = crypto.randomBytes(65534)
+ , rndhash = hash(random, 'md5')
+ , md5sum = crypto.createHash('md5')
+ , bl = new BufferList(function (err, buf) {
+ t.ok(Buffer.isBuffer(buf))
+ t.ok(err === null)
+ t.equal(rndhash, hash(bl.slice(), 'md5'))
+ t.equal(rndhash, hash(buf, 'md5'))
+
+ bl.pipe(fs.createWriteStream('/tmp/bl_test_rnd_out.dat'))
+ .on('close', function () {
+ var s = fs.createReadStream('/tmp/bl_test_rnd_out.dat')
+ s.on('data', md5sum.update.bind(md5sum))
+ s.on('end', function() {
+ t.equal(rndhash, md5sum.digest('hex'), 'woohoo! correct hash!')
+ t.end()
+ })
+ })
+
+ })
+
+ fs.writeFileSync('/tmp/bl_test_rnd.dat', random)
+ fs.createReadStream('/tmp/bl_test_rnd.dat').pipe(bl)
+})
+
+tape('instantiation with Buffer', function (t) {
+ var buf = crypto.randomBytes(1024)
+ , buf2 = crypto.randomBytes(1024)
+ , b = BufferList(buf)
+
+ t.equal(buf.toString('hex'), b.slice().toString('hex'), 'same buffer')
+ b = BufferList([ buf, buf2 ])
+ t.equal(b.slice().toString('hex'), Buffer.concat([ buf, buf2 ]).toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('test String appendage', function (t) {
+ var bl = new BufferList()
+ , b = new Buffer('abcdefghij\xff\x00')
+
+ bl.append('abcd')
+ bl.append('efg')
+ bl.append('hi')
+ bl.append('j')
+ bl.append('\xff\x00')
+
+ encodings.forEach(function (enc) {
+ t.equal(bl.toString(enc), b.toString(enc))
+ })
+
+ t.end()
+})
+
+tape('write nothing, should get empty buffer', function (t) {
+ t.plan(3)
+ BufferList(function (err, data) {
+ t.notOk(err, 'no error')
+ t.ok(Buffer.isBuffer(data), 'got a buffer')
+ t.equal(0, data.length, 'got a zero-length buffer')
+ t.end()
+ }).end()
+})
+
+tape('unicode string', function (t) {
+ t.plan(2)
+ var inp1 = '\u2600'
+ , inp2 = '\u2603'
+ , exp = inp1 + ' and ' + inp2
+ , bl = BufferList()
+ bl.write(inp1)
+ bl.write(' and ')
+ bl.write(inp2)
+ t.equal(exp, bl.toString())
+ t.equal(new Buffer(exp).toString('hex'), bl.toString('hex'))
+})
+
+tape('should emit finish', function (t) {
+ var source = BufferList()
+ , dest = BufferList()
+
+ source.write('hello')
+ source.pipe(dest)
+
+ dest.on('finish', function () {
+ t.equal(dest.toString('utf8'), 'hello')
+ t.end()
+ })
+})
+
+tape('basic copy', function (t) {
+ var buf = crypto.randomBytes(1024)
+ , buf2 = new Buffer(1024)
+ , b = BufferList(buf)
+
+ b.copy(buf2)
+ t.equal(b.slice().toString('hex'), buf2.toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('copy after many appends', function (t) {
+ var buf = crypto.randomBytes(512)
+ , buf2 = new Buffer(1024)
+ , b = BufferList(buf)
+
+ b.append(buf)
+ b.copy(buf2)
+ t.equal(b.slice().toString('hex'), buf2.toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('copy at a precise position', function (t) {
+ var buf = crypto.randomBytes(1004)
+ , buf2 = new Buffer(1024)
+ , b = BufferList(buf)
+
+ b.copy(buf2, 20)
+ t.equal(b.slice().toString('hex'), buf2.slice(20).toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('copy starting from a precise location', function (t) {
+ var buf = crypto.randomBytes(10)
+ , buf2 = new Buffer(5)
+ , b = BufferList(buf)
+
+ b.copy(buf2, 0, 5)
+ t.equal(b.slice(5).toString('hex'), buf2.toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('copy in an interval', function (t) {
+ var rnd = crypto.randomBytes(10)
+ , b = BufferList(rnd) // put the random bytes there
+ , actual = new Buffer(3)
+ , expected = new Buffer(3)
+
+ rnd.copy(expected, 0, 5, 8)
+ b.copy(actual, 0, 5, 8)
+
+ t.equal(actual.toString('hex'), expected.toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('copy an interval between two buffers', function (t) {
+ var buf = crypto.randomBytes(10)
+ , buf2 = new Buffer(10)
+ , b = BufferList(buf)
+
+ b.append(buf)
+ b.copy(buf2, 0, 5, 15)
+
+ t.equal(b.slice(5, 15).toString('hex'), buf2.toString('hex'), 'same buffer')
+ t.end()
+})
+
+tape('duplicate', function (t) {
+ t.plan(2)
+
+ var bl = new BufferList('abcdefghij\xff\x00')
+ , dup = bl.duplicate()
+
+ t.equal(bl.prototype, dup.prototype)
+ t.equal(bl.toString('hex'), dup.toString('hex'))
+})
+
+tape('destroy no pipe', function (t) {
+ t.plan(2)
+
+ var bl = new BufferList('alsdkfja;lsdkfja;lsdk')
+ bl.destroy()
+
+ t.equal(bl._bufs.length, 0)
+ t.equal(bl.length, 0)
+})
+
+!process.browser && tape('destroy with pipe before read end', function (t) {
+ t.plan(2)
+
+ var bl = new BufferList()
+ fs.createReadStream(__dirname + '/sauce.js')
+ .pipe(bl)
+
+ bl.destroy()
+
+ t.equal(bl._bufs.length, 0)
+ t.equal(bl.length, 0)
+
+})
+
+!process.browser && tape('destroy with pipe before read end with race', function (t) {
+ t.plan(2)
+
+ var bl = new BufferList()
+ fs.createReadStream(__dirname + '/sauce.js')
+ .pipe(bl)
+
+ setTimeout(function () {
+ bl.destroy()
+ setTimeout(function () {
+ t.equal(bl._bufs.length, 0)
+ t.equal(bl.length, 0)
+ }, 500)
+ }, 500)
+})
+
+!process.browser && tape('destroy with pipe after read end', function (t) {
+ t.plan(2)
+
+ var bl = new BufferList()
+ fs.createReadStream(__dirname + '/sauce.js')
+ .on('end', onEnd)
+ .pipe(bl)
+
+ function onEnd () {
+ bl.destroy()
+
+ t.equal(bl._bufs.length, 0)
+ t.equal(bl.length, 0)
+ }
+})
+
+!process.browser && tape('destroy with pipe while writing to a destination', function (t) {
+ t.plan(4)
+
+ var bl = new BufferList()
+ , ds = new BufferList()
+
+ fs.createReadStream(__dirname + '/sauce.js')
+ .on('end', onEnd)
+ .pipe(bl)
+
+ function onEnd () {
+ bl.pipe(ds)
+
+ setTimeout(function () {
+ bl.destroy()
+
+ t.equals(bl._bufs.length, 0)
+ t.equals(bl.length, 0)
+
+ ds.destroy()
+
+ t.equals(bl._bufs.length, 0)
+ t.equals(bl.length, 0)
+
+ }, 100)
+ }
+})
+
+!process.browser && tape('handle error', function (t) {
+ t.plan(2)
+ fs.createReadStream('/does/not/exist').pipe(BufferList(function (err, data) {
+ t.ok(err instanceof Error, 'has error')
+ t.notOk(data, 'no data')
+ }))
+})
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/sauce.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/sauce.js b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/sauce.js
new file mode 100644
index 0000000..a6d2862
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/sauce.js
@@ -0,0 +1,38 @@
+#!/usr/bin/env node
+
+const user = process.env.SAUCE_USER
+ , key = process.env.SAUCE_KEY
+ , path = require('path')
+ , brtapsauce = require('brtapsauce')
+ , testFile = path.join(__dirname, 'basic-test.js')
+
+ , capabilities = [
+ { browserName: 'chrome' , platform: 'Windows XP', version: '' }
+ , { browserName: 'firefox' , platform: 'Windows 8' , version: '' }
+ , { browserName: 'firefox' , platform: 'Windows XP', version: '4' }
+ , { browserName: 'internet explorer' , platform: 'Windows 8' , version: '10' }
+ , { browserName: 'internet explorer' , platform: 'Windows 7' , version: '9' }
+ , { browserName: 'internet explorer' , platform: 'Windows 7' , version: '8' }
+ , { browserName: 'internet explorer' , platform: 'Windows XP', version: '7' }
+ , { browserName: 'internet explorer' , platform: 'Windows XP', version: '6' }
+ , { browserName: 'safari' , platform: 'Windows 7' , version: '5' }
+ , { browserName: 'safari' , platform: 'OS X 10.8' , version: '6' }
+ , { browserName: 'opera' , platform: 'Windows 7' , version: '' }
+ , { browserName: 'opera' , platform: 'Windows 7' , version: '11' }
+ , { browserName: 'ipad' , platform: 'OS X 10.8' , version: '6' }
+ , { browserName: 'android' , platform: 'Linux' , version: '4.0', 'device-type': 'tablet' }
+ ]
+
+if (!user)
+ throw new Error('Must set a SAUCE_USER env var')
+if (!key)
+ throw new Error('Must set a SAUCE_KEY env var')
+
+brtapsauce({
+ name : 'Traversty'
+ , user : user
+ , key : key
+ , brsrc : testFile
+ , capabilities : capabilities
+ , options : { timeout: 60 * 6 }
+})
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/test.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/test.js b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/test.js
new file mode 100644
index 0000000..aa9b487
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/bl/test/test.js
@@ -0,0 +1,9 @@
+require('./basic-test')
+
+if (!process.env.SAUCE_KEY || !process.env.SAUCE_USER)
+ return console.log('SAUCE_KEY and/or SAUCE_USER not set, not running sauce tests')
+
+if (!/v0\.10/.test(process.version))
+ return console.log('Not Node v0.10.x, not running sauce tests')
+
+require('./sauce.js')
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/.npmignore b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/.npmignore
@@ -0,0 +1 @@
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/LICENSE b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/LICENSE
new file mode 100644
index 0000000..757562e
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2014 Mathias Buus
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/README.md
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/README.md b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/README.md
new file mode 100644
index 0000000..df800c1
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/README.md
@@ -0,0 +1,47 @@
+# end-of-stream
+
+A node module that calls a callback when a readable/writable/duplex stream has completed or failed.
+
+ npm install end-of-stream
+
+## Usage
+
+Simply pass a stream and a callback to the `eos`.
+Both legacy streams and streams2 are supported.
+
+``` js
+var eos = require('end-of-stream');
+
+eos(readableStream, function(err) {
+ if (err) return console.log('stream had an error or closed early');
+ console.log('stream has ended');
+});
+
+eos(writableStream, function(err) {
+ if (err) return console.log('stream had an error or closed early');
+ console.log('stream has finished');
+});
+
+eos(duplexStream, function(err) {
+ if (err) return console.log('stream had an error or closed early');
+ console.log('stream has ended and finished');
+});
+
+eos(duplexStream, {readable:false}, function(err) {
+ if (err) return console.log('stream had an error or closed early');
+ console.log('stream has ended but might still be writable');
+});
+
+eos(duplexStream, {writable:false}, function(err) {
+ if (err) return console.log('stream had an error or closed early');
+ console.log('stream has ended but might still be readable');
+});
+
+eos(readableStream, {error:false}, function(err) {
+ // do not treat emit('error', err) as a end-of-stream
+});
+```
+
+## License
+
+MIT
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/index.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/index.js b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/index.js
new file mode 100644
index 0000000..f92fc19
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/index.js
@@ -0,0 +1,83 @@
+var once = require('once');
+
+var noop = function() {};
+
+var isRequest = function(stream) {
+ return stream.setHeader && typeof stream.abort === 'function';
+};
+
+var isChildProcess = function(stream) {
+ return stream.stdio && Array.isArray(stream.stdio) && stream.stdio.length === 3
+};
+
+var eos = function(stream, opts, callback) {
+ if (typeof opts === 'function') return eos(stream, null, opts);
+ if (!opts) opts = {};
+
+ callback = once(callback || noop);
+
+ var ws = stream._writableState;
+ var rs = stream._readableState;
+ var readable = opts.readable || (opts.readable !== false && stream.readable);
+ var writable = opts.writable || (opts.writable !== false && stream.writable);
+
+ var onlegacyfinish = function() {
+ if (!stream.writable) onfinish();
+ };
+
+ var onfinish = function() {
+ writable = false;
+ if (!readable) callback();
+ };
+
+ var onend = function() {
+ readable = false;
+ if (!writable) callback();
+ };
+
+ var onexit = function(exitCode) {
+ callback(exitCode ? new Error('exited with error code: ' + exitCode) : null);
+ };
+
+ var onclose = function() {
+ if (readable && !(rs && rs.ended)) return callback(new Error('premature close'));
+ if (writable && !(ws && ws.ended)) return callback(new Error('premature close'));
+ };
+
+ var onrequest = function() {
+ stream.req.on('finish', onfinish);
+ };
+
+ if (isRequest(stream)) {
+ stream.on('complete', onfinish);
+ stream.on('abort', onclose);
+ if (stream.req) onrequest();
+ else stream.on('request', onrequest);
+ } else if (writable && !ws) { // legacy streams
+ stream.on('end', onlegacyfinish);
+ stream.on('close', onlegacyfinish);
+ }
+
+ if (isChildProcess(stream)) stream.on('exit', onexit);
+
+ stream.on('end', onend);
+ stream.on('finish', onfinish);
+ if (opts.error !== false) stream.on('error', callback);
+ stream.on('close', onclose);
+
+ return function() {
+ stream.removeListener('complete', onfinish);
+ stream.removeListener('abort', onclose);
+ stream.removeListener('request', onrequest);
+ if (stream.req) stream.req.removeListener('finish', onfinish);
+ stream.removeListener('end', onlegacyfinish);
+ stream.removeListener('close', onlegacyfinish);
+ stream.removeListener('finish', onfinish);
+ stream.removeListener('exit', onexit);
+ stream.removeListener('end', onend);
+ stream.removeListener('error', callback);
+ stream.removeListener('close', onclose);
+ };
+};
+
+module.exports = eos;
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/package.json
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/package.json b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/package.json
new file mode 100644
index 0000000..3696300
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/package.json
@@ -0,0 +1,56 @@
+{
+ "name": "end-of-stream",
+ "version": "1.1.0",
+ "description": "Call a callback when a readable/writable/duplex stream has completed or failed.",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/mafintosh/end-of-stream.git"
+ },
+ "dependencies": {
+ "once": "~1.3.0"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "keywords": [
+ "stream",
+ "streams",
+ "callback",
+ "finish",
+ "close",
+ "end",
+ "wait"
+ ],
+ "bugs": {
+ "url": "https://github.com/mafintosh/end-of-stream/issues"
+ },
+ "homepage": "https://github.com/mafintosh/end-of-stream",
+ "main": "index.js",
+ "author": {
+ "name": "Mathias Buus",
+ "email": "mathiasbuus@gmail.com"
+ },
+ "license": "MIT",
+ "gitHead": "16120f1529961ffd6e48118d8d978c97444633d4",
+ "_id": "end-of-stream@1.1.0",
+ "_shasum": "e9353258baa9108965efc41cb0ef8ade2f3cfb07",
+ "_from": "end-of-stream@>=1.0.0 <2.0.0",
+ "_npmVersion": "1.4.23",
+ "_npmUser": {
+ "name": "mafintosh",
+ "email": "mathiasbuus@gmail.com"
+ },
+ "maintainers": [
+ {
+ "name": "mafintosh",
+ "email": "mathiasbuus@gmail.com"
+ }
+ ],
+ "dist": {
+ "shasum": "e9353258baa9108965efc41cb0ef8ade2f3cfb07",
+ "tarball": "http://registry.npmjs.org/end-of-stream/-/end-of-stream-1.1.0.tgz"
+ },
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/end-of-stream/-/end-of-stream-1.1.0.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/test.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/test.js b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/test.js
new file mode 100644
index 0000000..03cb93e
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/end-of-stream/test.js
@@ -0,0 +1,77 @@
+var assert = require('assert');
+var eos = require('./index');
+
+var expected = 8;
+var fs = require('fs');
+var cp = require('child_process');
+var net = require('net');
+
+var ws = fs.createWriteStream('/dev/null');
+eos(ws, function(err) {
+ expected--;
+ assert(!!err);
+ if (!expected) process.exit(0);
+});
+ws.close();
+
+var rs = fs.createReadStream('/dev/random');
+eos(rs, function(err) {
+ expected--;
+ assert(!!err);
+ if (!expected) process.exit(0);
+});
+rs.close();
+
+var rs = fs.createReadStream(__filename);
+eos(rs, function(err) {
+ expected--;
+ assert(!err);
+ if (!expected) process.exit(0);
+});
+rs.pipe(fs.createWriteStream('/dev/null'));
+
+var rs = fs.createReadStream(__filename);
+eos(rs, function(err) {
+ throw new Error('no go')
+})();
+rs.pipe(fs.createWriteStream('/dev/null'));
+
+var exec = cp.exec('echo hello world');
+eos(exec, function(err) {
+ expected--;
+ assert(!err);
+ if (!expected) process.exit(0);
+});
+
+var spawn = cp.spawn('echo', ['hello world']);
+eos(spawn, function(err) {
+ expected--;
+ assert(!err);
+ if (!expected) process.exit(0);
+});
+
+var socket = net.connect(50000);
+eos(socket, function(err) {
+ expected--;
+ assert(!!err);
+ if (!expected) process.exit(0);
+});
+
+var server = net.createServer(function(socket) {
+ eos(socket, function() {
+ expected--;
+ if (!expected) process.exit(0);
+ });
+ socket.destroy();
+}).listen(30000, function() {
+ var socket = net.connect(30000);
+ eos(socket, function() {
+ expected--;
+ if (!expected) process.exit(0);
+ });
+});
+
+setTimeout(function() {
+ assert(expected === 0);
+ process.exit(0);
+}, 1000);
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.jshintrc b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.jshintrc
new file mode 100644
index 0000000..77887b5
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.jshintrc
@@ -0,0 +1,30 @@
+{
+ "maxdepth": 4,
+ "maxstatements": 200,
+ "maxcomplexity": 12,
+ "maxlen": 80,
+ "maxparams": 5,
+
+ "curly": true,
+ "eqeqeq": true,
+ "immed": true,
+ "latedef": false,
+ "noarg": true,
+ "noempty": true,
+ "nonew": true,
+ "undef": true,
+ "unused": "vars",
+ "trailing": true,
+
+ "quotmark": true,
+ "expr": true,
+ "asi": true,
+
+ "browser": false,
+ "esnext": true,
+ "devel": false,
+ "node": false,
+ "nonstandard": false,
+
+ "predef": ["require", "module", "__dirname", "__filename"]
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.npmignore b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/.npmignore
@@ -0,0 +1 @@
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/LICENCE
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/LICENCE b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/LICENCE
new file mode 100644
index 0000000..1a14b43
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/LICENCE
@@ -0,0 +1,19 @@
+Copyright (c) 2012-2014 Raynos.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/Makefile
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/Makefile b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/Makefile
new file mode 100644
index 0000000..d583fcf
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/Makefile
@@ -0,0 +1,4 @@
+browser:
+ node ./support/compile
+
+.PHONY: browser
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/README.md
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/README.md b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/README.md
new file mode 100644
index 0000000..093cb29
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/README.md
@@ -0,0 +1,32 @@
+# xtend
+
+[![browser support][3]][4]
+
+[](http://github.com/badges/stability-badges)
+
+Extend like a boss
+
+xtend is a basic utility library which allows you to extend an object by appending all of the properties from each object in a list. When there are identical properties, the right-most property takes precedence.
+
+## Examples
+
+```js
+var extend = require("xtend")
+
+// extend returns a new object. Does not mutate arguments
+var combination = extend({
+ a: "a",
+ b: 'c'
+}, {
+ b: "b"
+})
+// { a: "a", b: "b" }
+```
+
+## Stability status: Locked
+
+## MIT Licenced
+
+
+ [3]: http://ci.testling.com/Raynos/xtend.png
+ [4]: http://ci.testling.com/Raynos/xtend
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/immutable.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/immutable.js b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/immutable.js
new file mode 100644
index 0000000..5b76015
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/immutable.js
@@ -0,0 +1,17 @@
+module.exports = extend
+
+function extend() {
+ var target = {}
+
+ for (var i = 0; i < arguments.length; i++) {
+ var source = arguments[i]
+
+ for (var key in source) {
+ if (source.hasOwnProperty(key)) {
+ target[key] = source[key]
+ }
+ }
+ }
+
+ return target
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/mutable.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/mutable.js b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/mutable.js
new file mode 100644
index 0000000..a34475e
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/mutable.js
@@ -0,0 +1,15 @@
+module.exports = extend
+
+function extend(target) {
+ for (var i = 1; i < arguments.length; i++) {
+ var source = arguments[i]
+
+ for (var key in source) {
+ if (source.hasOwnProperty(key)) {
+ target[key] = source[key]
+ }
+ }
+ }
+
+ return target
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/package.json
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/package.json b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/package.json
new file mode 100644
index 0000000..907a720
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/package.json
@@ -0,0 +1,88 @@
+{
+ "name": "xtend",
+ "version": "4.0.0",
+ "description": "extend like a boss",
+ "keywords": [
+ "extend",
+ "merge",
+ "options",
+ "opts",
+ "object",
+ "array"
+ ],
+ "author": {
+ "name": "Raynos",
+ "email": "raynos2@gmail.com"
+ },
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/Raynos/xtend.git"
+ },
+ "main": "immutable",
+ "scripts": {
+ "test": "node test"
+ },
+ "dependencies": {},
+ "devDependencies": {
+ "tape": "~1.1.0"
+ },
+ "homepage": "https://github.com/Raynos/xtend",
+ "contributors": [
+ {
+ "name": "Jake Verbaten"
+ },
+ {
+ "name": "Matt Esch"
+ }
+ ],
+ "bugs": {
+ "url": "https://github.com/Raynos/xtend/issues",
+ "email": "raynos2@gmail.com"
+ },
+ "licenses": [
+ {
+ "type": "MIT",
+ "url": "http://github.com/raynos/xtend/raw/master/LICENSE"
+ }
+ ],
+ "testling": {
+ "files": "test.js",
+ "browsers": [
+ "ie/7..latest",
+ "firefox/16..latest",
+ "firefox/nightly",
+ "chrome/22..latest",
+ "chrome/canary",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest"
+ ]
+ },
+ "engines": {
+ "node": ">=0.4"
+ },
+ "gitHead": "94a95d76154103290533b2c55ffa0fe4be16bfef",
+ "_id": "xtend@4.0.0",
+ "_shasum": "8bc36ff87aedbe7ce9eaf0bca36b2354a743840f",
+ "_from": "xtend@>=4.0.0 <5.0.0",
+ "_npmVersion": "1.4.15",
+ "_npmUser": {
+ "name": "raynos",
+ "email": "raynos2@gmail.com"
+ },
+ "maintainers": [
+ {
+ "name": "raynos",
+ "email": "raynos2@gmail.com"
+ }
+ ],
+ "dist": {
+ "shasum": "8bc36ff87aedbe7ce9eaf0bca36b2354a743840f",
+ "tarball": "http://registry.npmjs.org/xtend/-/xtend-4.0.0.tgz"
+ },
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/xtend/-/xtend-4.0.0.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/test.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/test.js b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/test.js
new file mode 100644
index 0000000..3369d79
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/node_modules/xtend/test.js
@@ -0,0 +1,63 @@
+var test = require("tape")
+var extend = require("./")
+var mutableExtend = require("./mutable")
+
+test("merge", function(assert) {
+ var a = { a: "foo" }
+ var b = { b: "bar" }
+
+ assert.deepEqual(extend(a, b), { a: "foo", b: "bar" })
+ assert.end()
+})
+
+test("replace", function(assert) {
+ var a = { a: "foo" }
+ var b = { a: "bar" }
+
+ assert.deepEqual(extend(a, b), { a: "bar" })
+ assert.end()
+})
+
+test("undefined", function(assert) {
+ var a = { a: undefined }
+ var b = { b: "foo" }
+
+ assert.deepEqual(extend(a, b), { a: undefined, b: "foo" })
+ assert.deepEqual(extend(b, a), { a: undefined, b: "foo" })
+ assert.end()
+})
+
+test("handle 0", function(assert) {
+ var a = { a: "default" }
+ var b = { a: 0 }
+
+ assert.deepEqual(extend(a, b), { a: 0 })
+ assert.deepEqual(extend(b, a), { a: "default" })
+ assert.end()
+})
+
+test("is immutable", function (assert) {
+ var record = {}
+
+ extend(record, { foo: "bar" })
+ assert.equal(record.foo, undefined)
+ assert.end()
+})
+
+test("null as argument", function (assert) {
+ var a = { foo: "bar" }
+ var b = null
+ var c = void 0
+
+ assert.deepEqual(extend(b, a, c), { foo: "bar" })
+ assert.end()
+})
+
+test("mutable", function (assert) {
+ var a = { foo: "bar" }
+
+ mutableExtend(a, { bar: "baz" })
+
+ assert.equal(a.bar, "baz")
+ assert.end()
+})
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/pack.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/pack.js b/node_modules/archiver/node_modules/tar-stream/pack.js
new file mode 100644
index 0000000..eec3496
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/pack.js
@@ -0,0 +1,194 @@
+var util = require('util')
+var eos = require('end-of-stream')
+var headers = require('./headers')
+
+var Readable = require('readable-stream').Readable
+var Writable = require('readable-stream').Writable
+var PassThrough = require('readable-stream').PassThrough
+
+var END_OF_TAR = new Buffer(1024)
+END_OF_TAR.fill(0)
+
+var noop = function() {}
+
+var overflow = function(self, size) {
+ size &= 511
+ if (size) self.push(END_OF_TAR.slice(0, 512 - size))
+}
+
+var Sink = function(to) {
+ Writable.call(this)
+ this.written = 0
+ this._to = to
+ this._destroyed = false
+}
+
+util.inherits(Sink, Writable)
+
+Sink.prototype._write = function(data, enc, cb) {
+ this.written += data.length
+ if (this._to.push(data)) return cb()
+ this._to._drain = cb
+}
+
+Sink.prototype.destroy = function() {
+ if (this._destroyed) return
+ this._destroyed = true
+ this.emit('close')
+}
+
+var Void = function() {
+ Writable.call(this)
+ this._destroyed = false
+}
+
+util.inherits(Void, Writable)
+
+Void.prototype._write = function(data, enc, cb) {
+ cb(new Error('No body allowed for this entry'))
+}
+
+Void.prototype.destroy = function() {
+ if (this._destroyed) return
+ this._destroyed = true
+ this.emit('close')
+}
+
+var Pack = function(opts) {
+ if (!(this instanceof Pack)) return new Pack(opts)
+ Readable.call(this, opts)
+
+ this._drain = noop
+ this._finalized = false
+ this._finalizing = false
+ this._destroyed = false
+ this._stream = null
+}
+
+util.inherits(Pack, Readable)
+
+Pack.prototype.entry = function(header, buffer, callback) {
+ if (this._stream) throw new Error('already piping an entry')
+ if (this._finalized || this._destroyed) return
+
+ if (typeof buffer === 'function') {
+ callback = buffer
+ buffer = null
+ }
+
+ if (!callback) callback = noop
+
+ var self = this
+
+ if (!header.size) header.size = 0
+ if (!header.type) header.type = 'file'
+ if (!header.mode) header.mode = header.type === 'directory' ? 0755 : 0644
+ if (!header.uid) header.uid = 0
+ if (!header.gid) header.gid = 0
+ if (!header.mtime) header.mtime = new Date()
+
+ if (typeof buffer === 'string') buffer = new Buffer(buffer)
+ if (Buffer.isBuffer(buffer)) {
+ header.size = buffer.length
+ this._encode(header)
+ this.push(buffer)
+ overflow(self, header.size)
+ process.nextTick(callback)
+ return new Void()
+ }
+ if (header.type !== 'file' && header.type !== 'contigious-file') {
+ this._encode(header)
+ process.nextTick(callback)
+ return new Void()
+ }
+
+ var sink = new Sink(this)
+
+ this._encode(header)
+ this._stream = sink
+
+ eos(sink, function(err) {
+ self._stream = null
+
+ if (err) { // stream was closed
+ self.destroy()
+ return callback(err)
+ }
+
+ if (sink.written !== header.size) { // corrupting tar
+ self.destroy()
+ return callback(new Error('size mismatch'))
+ }
+
+ overflow(self, header.size)
+ if (self._finalizing) self.finalize()
+ callback()
+ })
+
+ return sink
+}
+
+Pack.prototype.finalize = function() {
+ if (this._stream) {
+ this._finalizing = true
+ return
+ }
+
+ if (this._finalized) return
+ this._finalized = true
+ this.push(END_OF_TAR)
+ this.push(null)
+}
+
+Pack.prototype.destroy = function(err) {
+ if (this._destroyed) return
+ this._destroyed = true
+
+ if (err) this.emit('error', err)
+ this.emit('close')
+ if (this._stream && this._stream.destroy) this._stream.destroy()
+}
+
+Pack.prototype._encode = function(header) {
+ var buf = headers.encode(header)
+ if (buf) this.push(buf)
+ else this._encodePax(header)
+}
+
+Pack.prototype._encodePax = function(header) {
+ var paxHeader = headers.encodePax({
+ name: header.name,
+ linkname: header.linkname
+ })
+
+ var newHeader = {
+ name: 'PaxHeader',
+ mode: header.mode,
+ uid: header.uid,
+ gid: header.gid,
+ size: paxHeader.length,
+ mtime: header.mtime,
+ type: 'pax-header',
+ linkname: header.linkname && 'PaxHeader',
+ uname: header.uname,
+ gname: header.gname,
+ devmajor: header.devmajor,
+ devminor: header.devminor
+ }
+
+ this.push(headers.encode(newHeader))
+ this.push(paxHeader)
+ overflow(this, paxHeader.length)
+
+ newHeader.size = header.size
+ newHeader.type = header.type
+ this.push(headers.encode(newHeader))
+}
+
+Pack.prototype._read = function(n) {
+ var drain = this._drain
+ this._drain = noop
+ drain()
+}
+
+module.exports = Pack
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/tar-stream/package.json
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/tar-stream/package.json b/node_modules/archiver/node_modules/tar-stream/package.json
new file mode 100644
index 0000000..f84366a
--- /dev/null
+++ b/node_modules/archiver/node_modules/tar-stream/package.json
@@ -0,0 +1,80 @@
+{
+ "name": "tar-stream",
+ "version": "1.1.2",
+ "description": "tar-stream is a streaming tar parser and generator and nothing else. It is streams2 and operates purely using streams which means you can easily extract/parse tarballs without ever hitting the file system.",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com:mafintosh/tar-stream.git"
+ },
+ "author": {
+ "name": "Mathias Buus",
+ "email": "mathiasbuus@gmail.com"
+ },
+ "engines": {
+ "node": ">= 0.8.0"
+ },
+ "dependencies": {
+ "bl": "^0.9.0",
+ "end-of-stream": "^1.0.0",
+ "readable-stream": "^1.0.33",
+ "xtend": "^4.0.0"
+ },
+ "devDependencies": {
+ "concat-stream": "^1.4.6",
+ "tape": "^3.0.3"
+ },
+ "scripts": {
+ "test": "tape test/*.js"
+ },
+ "keywords": [
+ "tar",
+ "tarball",
+ "parse",
+ "parser",
+ "generate",
+ "generator",
+ "stream",
+ "stream2",
+ "streams",
+ "streams2",
+ "streaming",
+ "pack",
+ "extract",
+ "modify"
+ ],
+ "bugs": {
+ "url": "https://github.com/mafintosh/tar-stream/issues"
+ },
+ "homepage": "https://github.com/mafintosh/tar-stream",
+ "main": "index.js",
+ "files": [
+ "*.js",
+ "LICENSE"
+ ],
+ "directories": {
+ "test": "test"
+ },
+ "license": "MIT",
+ "gitHead": "d1b85db2af5ad57591bc255739ace5c6ad513e25",
+ "_id": "tar-stream@1.1.2",
+ "_shasum": "14652d7bdb5a557ef58e55ccee68a4d642963d6e",
+ "_from": "tar-stream@>=1.1.0 <1.2.0",
+ "_npmVersion": "2.1.17",
+ "_nodeVersion": "0.10.35",
+ "_npmUser": {
+ "name": "mafintosh",
+ "email": "mathiasbuus@gmail.com"
+ },
+ "maintainers": [
+ {
+ "name": "mafintosh",
+ "email": "mathiasbuus@gmail.com"
+ }
+ ],
+ "dist": {
+ "shasum": "14652d7bdb5a557ef58e55ccee68a4d642963d6e",
+ "tarball": "http://registry.npmjs.org/tar-stream/-/tar-stream-1.1.2.tgz"
+ },
+ "_resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-1.1.2.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/wrappy/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/wrappy/LICENSE b/node_modules/archiver/node_modules/wrappy/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/archiver/node_modules/wrappy/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/wrappy/README.md
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/wrappy/README.md b/node_modules/archiver/node_modules/wrappy/README.md
new file mode 100644
index 0000000..98eab25
--- /dev/null
+++ b/node_modules/archiver/node_modules/wrappy/README.md
@@ -0,0 +1,36 @@
+# wrappy
+
+Callback wrapping utility
+
+## USAGE
+
+```javascript
+var wrappy = require("wrappy")
+
+// var wrapper = wrappy(wrapperFunction)
+
+// make sure a cb is called only once
+// See also: http://npm.im/once for this specific use case
+var once = wrappy(function (cb) {
+ var called = false
+ return function () {
+ if (called) return
+ called = true
+ return cb.apply(this, arguments)
+ }
+})
+
+function printBoo () {
+ console.log('boo')
+}
+// has some rando property
+printBoo.iAmBooPrinter = true
+
+var onlyPrintOnce = once(printBoo)
+
+onlyPrintOnce() // prints 'boo'
+onlyPrintOnce() // does nothing
+
+// random property is retained!
+assert.equal(onlyPrintOnce.iAmBooPrinter, true)
+```
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/wrappy/package.json
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/wrappy/package.json b/node_modules/archiver/node_modules/wrappy/package.json
new file mode 100644
index 0000000..b88e662
--- /dev/null
+++ b/node_modules/archiver/node_modules/wrappy/package.json
@@ -0,0 +1,52 @@
+{
+ "name": "wrappy",
+ "version": "1.0.1",
+ "description": "Callback wrapping utility",
+ "main": "wrappy.js",
+ "directories": {
+ "test": "test"
+ },
+ "dependencies": {},
+ "devDependencies": {
+ "tap": "^0.4.12"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "repository": {
+ "type": "git",
+ "url": "https://github.com/npm/wrappy"
+ },
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me/"
+ },
+ "license": "ISC",
+ "bugs": {
+ "url": "https://github.com/npm/wrappy/issues"
+ },
+ "homepage": "https://github.com/npm/wrappy",
+ "gitHead": "006a8cbac6b99988315834c207896eed71fd069a",
+ "_id": "wrappy@1.0.1",
+ "_shasum": "1e65969965ccbc2db4548c6b84a6f2c5aedd4739",
+ "_from": "wrappy@1.0.1",
+ "_npmVersion": "2.0.0",
+ "_nodeVersion": "0.10.31",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "dist": {
+ "shasum": "1e65969965ccbc2db4548c6b84a6f2c5aedd4739",
+ "tarball": "http://registry.npmjs.org/wrappy/-/wrappy-1.0.1.tgz"
+ },
+ "_resolved": "https://registry.npmjs.org/wrappy/-/wrappy-1.0.1.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/wrappy/test/basic.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/wrappy/test/basic.js b/node_modules/archiver/node_modules/wrappy/test/basic.js
new file mode 100644
index 0000000..5ed0fcd
--- /dev/null
+++ b/node_modules/archiver/node_modules/wrappy/test/basic.js
@@ -0,0 +1,51 @@
+var test = require('tap').test
+var wrappy = require('../wrappy.js')
+
+test('basic', function (t) {
+ function onceifier (cb) {
+ var called = false
+ return function () {
+ if (called) return
+ called = true
+ return cb.apply(this, arguments)
+ }
+ }
+ onceifier.iAmOnce = {}
+ var once = wrappy(onceifier)
+ t.equal(once.iAmOnce, onceifier.iAmOnce)
+
+ var called = 0
+ function boo () {
+ t.equal(called, 0)
+ called++
+ }
+ // has some rando property
+ boo.iAmBoo = true
+
+ var onlyPrintOnce = once(boo)
+
+ onlyPrintOnce() // prints 'boo'
+ onlyPrintOnce() // does nothing
+ t.equal(called, 1)
+
+ // random property is retained!
+ t.equal(onlyPrintOnce.iAmBoo, true)
+
+ var logs = []
+ var logwrap = wrappy(function (msg, cb) {
+ logs.push(msg + ' wrapping cb')
+ return function () {
+ logs.push(msg + ' before cb')
+ var ret = cb.apply(this, arguments)
+ logs.push(msg + ' after cb')
+ }
+ })
+
+ var c = logwrap('foo', function () {
+ t.same(logs, [ 'foo wrapping cb', 'foo before cb' ])
+ })
+ c()
+ t.same(logs, [ 'foo wrapping cb', 'foo before cb', 'foo after cb' ])
+
+ t.end()
+})
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/wrappy/wrappy.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/wrappy/wrappy.js b/node_modules/archiver/node_modules/wrappy/wrappy.js
new file mode 100644
index 0000000..bb7e7d6
--- /dev/null
+++ b/node_modules/archiver/node_modules/wrappy/wrappy.js
@@ -0,0 +1,33 @@
+// Returns a wrapper function that returns a wrapped callback
+// The wrapper function should do some stuff, and return a
+// presumably different callback function.
+// This makes sure that own properties are retained, so that
+// decorations and such are not lost along the way.
+module.exports = wrappy
+function wrappy (fn, cb) {
+ if (fn && cb) return wrappy(fn)(cb)
+
+ if (typeof fn !== 'function')
+ throw new TypeError('need wrapper function')
+
+ Object.keys(fn).forEach(function (k) {
+ wrapper[k] = fn[k]
+ })
+
+ return wrapper
+
+ function wrapper() {
+ var args = new Array(arguments.length)
+ for (var i = 0; i < args.length; i++) {
+ args[i] = arguments[i]
+ }
+ var ret = fn.apply(this, args)
+ var cb = args[args.length-1]
+ if (typeof ret === 'function' && ret !== cb) {
+ Object.keys(cb).forEach(function (k) {
+ ret[k] = cb[k]
+ })
+ }
+ return ret
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/zip-stream/LICENSE-MIT
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/zip-stream/LICENSE-MIT b/node_modules/archiver/node_modules/zip-stream/LICENSE-MIT
new file mode 100644
index 0000000..819b403
--- /dev/null
+++ b/node_modules/archiver/node_modules/zip-stream/LICENSE-MIT
@@ -0,0 +1,22 @@
+Copyright (c) 2014 Chris Talkington, contributors.
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/zip-stream/README.md
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/zip-stream/README.md b/node_modules/archiver/node_modules/zip-stream/README.md
new file mode 100644
index 0000000..f12fdcf
--- /dev/null
+++ b/node_modules/archiver/node_modules/zip-stream/README.md
@@ -0,0 +1,105 @@
+# zip-stream v0.5.1 [](https://travis-ci.org/archiverjs/node-zip-stream)
+
+zip-stream is a streaming zip archive generator based on the `ZipArchiveOutputStream` prototype found in the [compress-commons](https://www.npmjs.org/package/compress-commons) project.
+
+It was originally created to be a successor to [zipstream](https://npmjs.org/package/zipstream).
+
+[](https://nodei.co/npm/zip-stream/)
+
+### Install
+
+```bash
+npm install zip-stream --save
+```
+
+You can also use `npm install https://github.com/archiverjs/node-zip-stream/archive/master.tar.gz` to test upcoming versions.
+
+### Usage
+
+This module is meant to be wrapped internally by other modules and therefore lacks any queue management. This means you have to wait until the previous entry has been fully consumed to add another. Nested callbacks should be used to add multiple entries. There are modules like [async](https://npmjs.org/package/async) that ease the so called "callback hell".
+
+If you want a module that handles entry queueing and much more, you should check out [archiver](https://npmjs.org/package/archiver) which uses this module internally.
+
+```js
+var packer = require('zip-stream');
+var archive = new packer(); // OR new packer(options)
+
+archive.on('error', function(err) {
+ throw err;
+});
+
+// pipe archive where you want it (ie fs, http, etc)
+// listen to the destination's end, close, or finish event
+
+archive.entry('string contents', { name: 'string.txt' }, function(err, entry) {
+ if (err) throw err;
+ archive.entry(null, { name: 'directory/' }, function(err, entry) {
+ if (err) throw err;
+ archive.finish();
+ });
+});
+```
+
+### Instance API
+
+#### getBytesWritten()
+
+Returns the current number of bytes written to this stream.
+
+#### entry(input, data, callback(err, data))
+
+Appends an input source (text string, buffer, or stream) to the instance. When the instance has received, processed, and emitted the input, the callback is fired.
+
+#### finish()
+
+Finalizes the instance. You should listen to the destination stream's `end`/`close`/`finish` event to know when all output has been safely consumed. (`finalize` is aliased for back-compat)
+
+### Instance Options
+
+#### comment `string`
+
+Sets the zip comment.
+
+#### store `boolean`
+
+If true, all entry contents will be archived without compression by default.
+
+#### zlib `object`
+
+Passed to node's [zlib](http://nodejs.org/api/zlib.html#zlib_options) module to control compression. Options may vary by node version.
+
+### Entry Data
+
+#### name `string` `required`
+
+Sets the entry name including internal path.
+
+#### type `string`
+
+Sets the entry type. Defaults to `file` or `directory` if name ends with trailing slash.
+
+#### date `string|Date`
+
+Sets the entry date. This can be any valid date string or instance. Defaults to current time in locale.
+
+#### store `boolean`
+
+If true, entry contents will be archived without compression.
+
+#### comment `string`
+
+Sets the entry comment.
+
+#### mode `number`
+
+Sets the entry permissions.
+
+## Things of Interest
+
+- [Releases](https://github.com/archiverjs/node-zip-stream/releases)
+- [Contributing](https://github.com/archiverjs/node-zip-stream/blob/master/CONTRIBUTING.md)
+- [MIT License](https://github.com/archiverjs/node-zip-stream/blob/master/LICENSE-MIT)
+
+## Credits
+
+Concept inspired by Antoine van Wel's [zipstream](https://npmjs.org/package/zipstream) module, which is no longer being updated.
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/zip-stream/lib/util/index.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/zip-stream/lib/util/index.js b/node_modules/archiver/node_modules/zip-stream/lib/util/index.js
new file mode 100644
index 0000000..35aedfd
--- /dev/null
+++ b/node_modules/archiver/node_modules/zip-stream/lib/util/index.js
@@ -0,0 +1,103 @@
+/**
+ * node-zip-stream
+ *
+ * Copyright (c) 2014 Chris Talkington, contributors.
+ * Licensed under the MIT license.
+ * https://github.com/archiverjs/node-zip-stream/blob/master/LICENSE-MIT
+ */
+var fs = require('fs');
+var path = require('path');
+
+var Stream = require('stream').Stream;
+var PassThrough = require('readable-stream').PassThrough;
+
+var _ = require('lodash');
+
+var util = module.exports = {};
+
+util.convertDateTimeDos = function(input) {
+ return new Date(
+ ((input >> 25) & 0x7f) + 1980,
+ ((input >> 21) & 0x0f) - 1,
+ (input >> 16) & 0x1f,
+ (input >> 11) & 0x1f,
+ (input >> 5) & 0x3f,
+ (input & 0x1f) << 1
+ );
+};
+
+util.dateify = function(dateish) {
+ dateish = dateish || new Date();
+
+ if (dateish instanceof Date) {
+ dateish = dateish;
+ } else if (typeof dateish === 'string') {
+ dateish = new Date(dateish);
+ } else {
+ dateish = new Date();
+ }
+
+ return dateish;
+};
+
+// this is slightly different from lodash version
+util.defaults = function(object, source, guard) {
+ var args = arguments;
+ args[0] = args[0] || {};
+
+ return _.defaults.apply(_, args);
+};
+
+util.dosDateTime = function(d, utc) {
+ d = (d instanceof Date) ? d : util.dateify(d);
+ utc = utc || false;
+
+ var year = utc ? d.getUTCFullYear() : d.getFullYear();
+
+ if (year < 1980) {
+ return 2162688; // 1980-1-1 00:00:00
+ } else if (year >= 2044) {
+ return 2141175677; // 2043-12-31 23:59:58
+ }
+
+ var val = {
+ year: year,
+ month: utc ? d.getUTCMonth() : d.getMonth(),
+ date: utc ? d.getUTCDate() : d.getDate(),
+ hours: utc ? d.getUTCHours() : d.getHours(),
+ minutes: utc ? d.getUTCMinutes() : d.getMinutes(),
+ seconds: utc ? d.getUTCSeconds() : d.getSeconds()
+ };
+
+ return ((val.year-1980) << 25) | ((val.month+1) << 21) | (val.date << 16) |
+ (val.hours << 11) | (val.minutes << 5) | (val.seconds / 2);
+};
+
+util.isStream = function(source) {
+ return source instanceof Stream;
+};
+
+util.normalizeInputSource = function(source) {
+ if (source === null) {
+ return new Buffer(0);
+ } else if (typeof source === 'string') {
+ return new Buffer(source);
+ } else if (util.isStream(source) && !source._readableState) {
+ var normalized = new PassThrough();
+ source.pipe(normalized);
+
+ return normalized;
+ }
+
+ return source;
+};
+
+util.sanitizePath = function() {
+ var filepath = path.join.apply(path, arguments);
+ return filepath.replace(/\\/g, '/').replace(/:/g, '').replace(/^\/+/, '');
+};
+
+util.unixifyPath = function() {
+ var filepath = path.join.apply(path, arguments);
+ return filepath.replace(/\\/g, '/');
+};
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/zip-stream/lib/zip-stream.js
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/zip-stream/lib/zip-stream.js b/node_modules/archiver/node_modules/zip-stream/lib/zip-stream.js
new file mode 100644
index 0000000..cd64d8f
--- /dev/null
+++ b/node_modules/archiver/node_modules/zip-stream/lib/zip-stream.js
@@ -0,0 +1,110 @@
+/**
+ * node-zip-stream
+ *
+ * Copyright (c) 2014 Chris Talkington, contributors.
+ * Licensed under the MIT license.
+ * https://github.com/archiverjs/node-zip-stream/blob/master/LICENSE-MIT
+ */
+var inherits = require('util').inherits;
+
+var ZipArchiveOutputStream = require('compress-commons').ZipArchiveOutputStream;
+var ZipArchiveEntry = require('compress-commons').ZipArchiveEntry;
+
+var util = require('./util');
+
+var ZipStream = module.exports = function(options) {
+ if (!(this instanceof ZipStream)) {
+ return new ZipStream(options);
+ }
+
+ options = this.options = options || {};
+ options.zlib = options.zlib || {};
+
+ ZipArchiveOutputStream.call(this, options);
+
+ if (typeof options.level === 'number' && options.level >= 0) {
+ options.zlib.level = options.level;
+ delete options.level;
+ }
+
+ if (options.zlib.level && options.zlib.level === 0) {
+ options.store = true;
+ }
+
+ if (options.comment && options.comment.length > 0) {
+ this.setComment(options.comment);
+ }
+};
+
+inherits(ZipStream, ZipArchiveOutputStream);
+
+ZipStream.prototype._normalizeFileData = function(data) {
+ data = util.defaults(data, {
+ type: 'file',
+ name: null,
+ date: null,
+ mode: null,
+ store: this.options.store,
+ comment: ''
+ });
+
+ var isDir = data.type === 'directory';
+
+ if (data.name) {
+ data.name = util.sanitizePath(data.name);
+
+ if (data.name.slice(-1) === '/') {
+ isDir = true;
+ data.type = 'directory';
+ } else if (isDir) {
+ data.name += '/';
+ }
+ }
+
+ if (isDir) {
+ data.store = true;
+ }
+
+ data.date = util.dateify(data.date);
+
+ return data;
+};
+
+ZipStream.prototype.entry = function(source, data, callback) {
+ if (typeof callback !== 'function') {
+ callback = this._emitErrorCallback.bind(this);
+ }
+
+ data = this._normalizeFileData(data);
+
+ if (data.type !== 'file' && data.type !== 'directory') {
+ callback(new Error(data.type + ' entries not currently supported'));
+ return;
+ }
+
+ if (typeof data.name !== 'string' || data.name.length === 0) {
+ callback(new Error('entry name must be a non-empty string value'));
+ return;
+ }
+
+ var entry = new ZipArchiveEntry(data.name);
+ entry.setTime(data.date);
+
+ if (data.store) {
+ entry.setMethod(0);
+ }
+
+ if (data.comment.length > 0) {
+ entry.setComment(data.comment);
+ }
+
+ if (typeof data.mode === 'number') {
+ entry.setUnixMode(data.mode);
+ }
+
+ return ZipArchiveOutputStream.prototype.entry.call(this, entry, source, callback);
+};
+
+ZipStream.prototype.finalize = function() {
+ this.finish();
+};
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/LICENSE-MIT
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/LICENSE-MIT b/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/LICENSE-MIT
new file mode 100644
index 0000000..819b403
--- /dev/null
+++ b/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/LICENSE-MIT
@@ -0,0 +1,22 @@
+Copyright (c) 2014 Chris Talkington, contributors.
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-firefoxos/blob/bd21ce3b/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/README.md
----------------------------------------------------------------------
diff --git a/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/README.md b/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/README.md
new file mode 100644
index 0000000..0124a0e
--- /dev/null
+++ b/node_modules/archiver/node_modules/zip-stream/node_modules/compress-commons/README.md
@@ -0,0 +1,25 @@
+# Compress Commons v0.2.7 [](https://travis-ci.org/archiverjs/node-compress-commons)
+
+Compress Commons is a library that defines a common interface for working with archive formats within node.
+
+[](https://nodei.co/npm/compress-commons/)
+
+## Install
+
+```bash
+npm install compress-commons --save
+```
+
+You can also use `npm install https://github.com/archiverjs/node-compress-commons/archive/master.tar.gz` to test upcoming versions.
+
+## Things of Interest
+
+- [Changelog](https://github.com/archiverjs/node-compress-commons/releases)
+- [Contributing](https://github.com/archiverjs/node-compress-commons/blob/master/CONTRIBUTING.md)
+- [MIT License](https://github.com/archiverjs/node-compress-commons/blob/master/LICENSE-MIT)
+
+## Credits
+
+Concept inspired by [Apache Commons Compress](http://commons.apache.org/proper/commons-compress/)™.
+
+Some logic derived from [Apache Commons Compress](http://commons.apache.org/proper/commons-compress/)™ and [OpenJDK 7](http://openjdk.java.net/).
\ No newline at end of file
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org