You are viewing a plain text version of this content. The canonical link for it is here.
Posted to notifications@apisix.apache.org by GitBox <gi...@apache.org> on 2020/12/07 18:15:47 UTC
[GitHub] [apisix-dashboard] liuxiran opened a new pull request #979: Refactor online debug be
liuxiran opened a new pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979
Please answer these questions before submitting a pull request
- Why submit this pull request?
- [ ] New feature provided
- Related issues
split from https://github.com/apache/apisix-dashboard/pull/964
Be of #835
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-747911481
Thanks for @nic-chen 's help, CI passed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] nic-chen commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
nic-chen commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r542083176
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +401,61 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ BodyParams map[string]string `json:"bodyParams,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"headerParams,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer func() {
+ if resp != nil {
+ _ = resp.Body.Close()
+ }
+ }()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
Review comment:
We should abstract this so that we could support other protocols such as WebSocket and gRPC in the future.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] juzhiyuan commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
juzhiyuan commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r546176364
##########
File path: api/internal/handler/route_online_debug/route_online_debug.go
##########
@@ -0,0 +1,129 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package route_online_debug
+
+import (
+ "encoding/json"
+ "fmt"
+ "io/ioutil"
+ "net/http"
+ "reflect"
+ "strings"
+ "time"
+
+ "github.com/apisix/manager-api/internal/handler"
+ "github.com/gin-gonic/gin"
+ "github.com/shiningrush/droplet"
+ "github.com/shiningrush/droplet/data"
+ "github.com/shiningrush/droplet/wrapper"
+ wgin "github.com/shiningrush/droplet/wrapper/gin"
+)
+
+type Handler struct {
+}
+
+func NewHandler() (handler.RouteRegister, error) {
+ return &Handler{}, nil
+}
+
+type ProtocolSupport interface {
+ RequestForwarding(c droplet.Context) (interface{}, error)
+}
+
+var protocolMap map[string]ProtocolSupport
+
+func init() {
+ protocolMap = make(map[string]ProtocolSupport)
+ protocolMap["http"] = &HTTPProtocolSupport{}
+}
+
+func (h *Handler) ApplyRoute(r *gin.Engine) {
+ r.POST("/apisix/admin/debug-request-forwarding", wgin.Wraps(DebugRequestForwarding,
+ wrapper.InputType(reflect.TypeOf(ParamsInput{}))))
+}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
Review comment:
May refer a issue here, instead of commenting :)
##########
File path: api/internal/handler/route_online_debug/route_online_debug.go
##########
@@ -0,0 +1,129 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package route_online_debug
+
+import (
+ "encoding/json"
+ "fmt"
+ "io/ioutil"
+ "net/http"
+ "reflect"
+ "strings"
+ "time"
+
+ "github.com/apisix/manager-api/internal/handler"
+ "github.com/gin-gonic/gin"
+ "github.com/shiningrush/droplet"
+ "github.com/shiningrush/droplet/data"
+ "github.com/shiningrush/droplet/wrapper"
+ wgin "github.com/shiningrush/droplet/wrapper/gin"
+)
+
+type Handler struct {
+}
+
+func NewHandler() (handler.RouteRegister, error) {
+ return &Handler{}, nil
+}
+
+type ProtocolSupport interface {
+ RequestForwarding(c droplet.Context) (interface{}, error)
+}
+
+var protocolMap map[string]ProtocolSupport
+
+func init() {
+ protocolMap = make(map[string]ProtocolSupport)
+ protocolMap["http"] = &HTTPProtocolSupport{}
+}
+
+func (h *Handler) ApplyRoute(r *gin.Engine) {
+ r.POST("/apisix/admin/debug-request-forwarding", wgin.Wraps(DebugRequestForwarding,
+ wrapper.InputType(reflect.TypeOf(ParamsInput{}))))
+}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
Review comment:
could we combine into the following style?
```go
if err != nil {
result.Data = string(body)
} else {
result.Data = returnData
}
result.Code = resp.StatusCode
result.Message = resp.Status
```
##########
File path: api/internal/handler/route_online_debug/route_online_debug.go
##########
@@ -0,0 +1,129 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package route_online_debug
+
+import (
+ "encoding/json"
+ "fmt"
+ "io/ioutil"
+ "net/http"
+ "reflect"
+ "strings"
+ "time"
+
+ "github.com/apisix/manager-api/internal/handler"
+ "github.com/gin-gonic/gin"
+ "github.com/shiningrush/droplet"
+ "github.com/shiningrush/droplet/data"
+ "github.com/shiningrush/droplet/wrapper"
+ wgin "github.com/shiningrush/droplet/wrapper/gin"
+)
+
+type Handler struct {
+}
+
+func NewHandler() (handler.RouteRegister, error) {
+ return &Handler{}, nil
+}
+
+type ProtocolSupport interface {
+ RequestForwarding(c droplet.Context) (interface{}, error)
+}
+
+var protocolMap map[string]ProtocolSupport
+
+func init() {
+ protocolMap = make(map[string]ProtocolSupport)
+ protocolMap["http"] = &HTTPProtocolSupport{}
+}
+
+func (h *Handler) ApplyRoute(r *gin.Engine) {
+ r.POST("/apisix/admin/debug-request-forwarding", wgin.Wraps(DebugRequestForwarding,
+ wrapper.InputType(reflect.TypeOf(ParamsInput{}))))
+}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
Review comment:
extra empty line
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -0,0 +1,852 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package e2e
+
+import (
+ "io/ioutil"
+ "net/http"
+ "strings"
+ "testing"
+ "time"
+
+ "github.com/stretchr/testify/assert"
+ "github.com/tidwall/gjson"
+)
+
+func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
+ tests := []HttpTestCase{
+ {
+ caseDesc: "hit route that not exist",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello_",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: "{\"error_msg\":\"404 Route Not Found\"}\n",
+ },
+ }
+ for _, tc := range tests {
+ testCaseCheck(tc)
+ }
+ basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "`+APISIXInternalUrl+`/hello_","method": "GET","request_protocol": "http"}`))
+ request.Header.Add("Authorization", token)
+ resp, err := http.DefaultClient.Do(request)
+ if err != nil {
+ return
+ }
+ defer resp.Body.Close()
+ respBody, _ := ioutil.ReadAll(resp.Body)
+ realBody := gjson.Get(string(respBody), "data")
+ assert.Equal(t, `{"code":404,"message":"404 Not Found","data":{"error_msg":"404 Route Not Found"}}`, realBody.String())
+}
+
+func TestRoute_Online_Debug_Route_With_Query_Params(t *testing.T) {
+ tests := []HttpTestCase{
+ {
+ caseDesc: "hit route that not exist",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: "{\"error_msg\":\"404 Route Not Found\"}\n",
+ },
+ {
+ caseDesc: "create route with query params",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPut,
+ Path: "/apisix/admin/routes/r1",
+ Body: `{
+ "uri": "/hello",
+ "methods": ["GET"],
+ "vars": [
+ ["arg_name","==","aaa"]
+ ],
+ "upstream": {
+ "type": "roundrobin",
+ "nodes": [{
+ "host": "172.16.238.20",
+ "port": 1980,
+ "weight": 1
+ }]
+ }
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "online debug route with query params",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPost,
+ Path: "/apisix/admin/debug-request-forwarding",
+ Body: `{
+ "url": "` + APISIXInternalUrl + `/hello?name=aaa",
+ "request_protocol": "http",
+ "method": "GET"
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ },
+ {
+ caseDesc: "delete route",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodDelete,
+ Path: "/apisix/admin/routes/r1",
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ },
+ {
+ caseDesc: "verify the deleted route ",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: `{"error_msg":"404 Route Not Found"}`,
+ Sleep: sleepTime,
+ },
+ }
+
+ for _, tc := range tests {
+ testCaseCheck(tc)
+ }
+}
+
+func TestRoute_Online_Debug_Route_With_Header_Params(t *testing.T) {
+ tests := []HttpTestCase{
+ {
+ caseDesc: "make sure the route is not created ",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: `{"error_msg":"404 Route Not Found"}`,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "create route with header params",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPut,
+ Path: "/apisix/admin/routes/r1",
+ Body: `{
+ "uri": "/hello",
+ "methods": ["GET"],
+ "vars": [
+ ["http_version","==","v2"]
+ ],
+ "upstream": {
+ "type": "roundrobin",
+ "nodes": [{
+ "host": "172.16.238.20",
+ "port": 1980,
+ "weight": 1
+ }]
+ }
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "online debug route with header params",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPost,
+ Path: "/apisix/admin/debug-request-forwarding",
+ Body: `{
+ "url": "` + APISIXInternalUrl + `/hello",
+ "request_protocol": "http",
+ "method": "GET",
+ "header_params": {
+ "version": ["v2"]
+ }
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ },
+ {
+ caseDesc: "delete route",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodDelete,
+ Path: "/apisix/admin/routes/r1",
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ },
+ {
+ caseDesc: "verify the deleted route ",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: `{"error_msg":"404 Route Not Found"}`,
+ Sleep: sleepTime,
+ },
+ }
+
+ for _, tc := range tests {
+ testCaseCheck(tc)
+ }
+}
+
+func TestRoute_Online_Debug_Route_With_Body_Params(t *testing.T) {
+ tests := []HttpTestCase{
+ {
+ caseDesc: "make sure the route is not created ",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: `{"error_msg":"404 Route Not Found"}`,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "create route with method POST",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPut,
+ Path: "/apisix/admin/routes/r1",
+ Body: `{
+ "uri": "/hello",
+ "methods": ["POST"],
+ "upstream": {
+ "type": "roundrobin",
+ "nodes": [{
+ "host": "172.16.238.20",
+ "port": 1980,
+ "weight": 1
+ }]
+ }
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "online debug route with body params",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPost,
+ Path: "/apisix/admin/debug-request-forwarding",
+ Body: `{
+ "url": "` + APISIXInternalUrl + `/hello",
+ "request_protocol": "http",
+ "method": "POST",
+ "body_params": {
+ "name": "test",
+ "desc": "online debug route with body params"
+ }
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ },
+ {
+ caseDesc: "delete route",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodDelete,
+ Path: "/apisix/admin/routes/r1",
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ },
+ {
+ caseDesc: "verify the deleted route ",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: `{"error_msg":"404 Route Not Found"}`,
+ Sleep: sleepTime,
+ },
+ }
+
+ for _, tc := range tests {
+ testCaseCheck(tc)
+ }
+}
+
+func TestRoute_Online_Debug_Route_With_Basic_Auth(t *testing.T) {
+ tests := []HttpTestCase{
+ {
+ caseDesc: "make sure the route is not created ",
+ Object: APISIXExpect(t),
+ Method: http.MethodGet,
+ Path: "/hello",
+ ExpectStatus: http.StatusNotFound,
+ ExpectBody: `{"error_msg":"404 Route Not Found"}`,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "create route enable basic-auth plugin",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPut,
+ Path: "/apisix/admin/routes/r1",
+ Body: `{
+ "uri": "/hello",
+ "methods": ["GET"],
+ "plugins": {
+ "basic-auth": {}
+ },
+ "upstream": {
+ "type": "roundrobin",
+ "nodes": [{
+ "host": "172.16.238.20",
+ "port": 1980,
+ "weight": 1
+ }]
+ }
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "make sure the consumer is not created",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodGet,
+ Path: "/apisix/admin/consumers/jack",
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusNotFound,
+ },
+ {
+ caseDesc: "create consumer",
+ Object: ManagerApiExpect(t),
+ Path: "/apisix/admin/consumers",
+ Method: http.MethodPost,
+ Body: `{
+ "username": "jack",
+ "plugins": {
+ "basic-auth": {
+ "disable": false,
+ "username": "jack",
+ "password": "123456"
+ }
+ },
+ "desc": "test description"
+ }`,
+ Headers: map[string]string{"Authorization": token},
+ ExpectStatus: http.StatusOK,
+ Sleep: sleepTime,
+ },
+ {
+ caseDesc: "online debug route with username and password",
+ Object: ManagerApiExpect(t),
+ Method: http.MethodPost,
+ Path: "/apisix/admin/debug-request-forwarding",
+ Body: `{
+ "url": "` + APISIXInternalUrl + `/hello",
+ "request_protocol": "http",
+ "method": "GET",
+ "header_params": {
+ "Authorization": ["Basic amFjazoxMjM0NTYKIA=="]
Review comment:
Fixed token?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-740415708
Request help:
I added some e2e test cases for the new API `POST /apisix/admin/debug-request-forwarding`, which is used to forward the online debug request from FE. As we can see now the CI for BE e2e test are failed , but the same cases were passed when I called the API from FE.
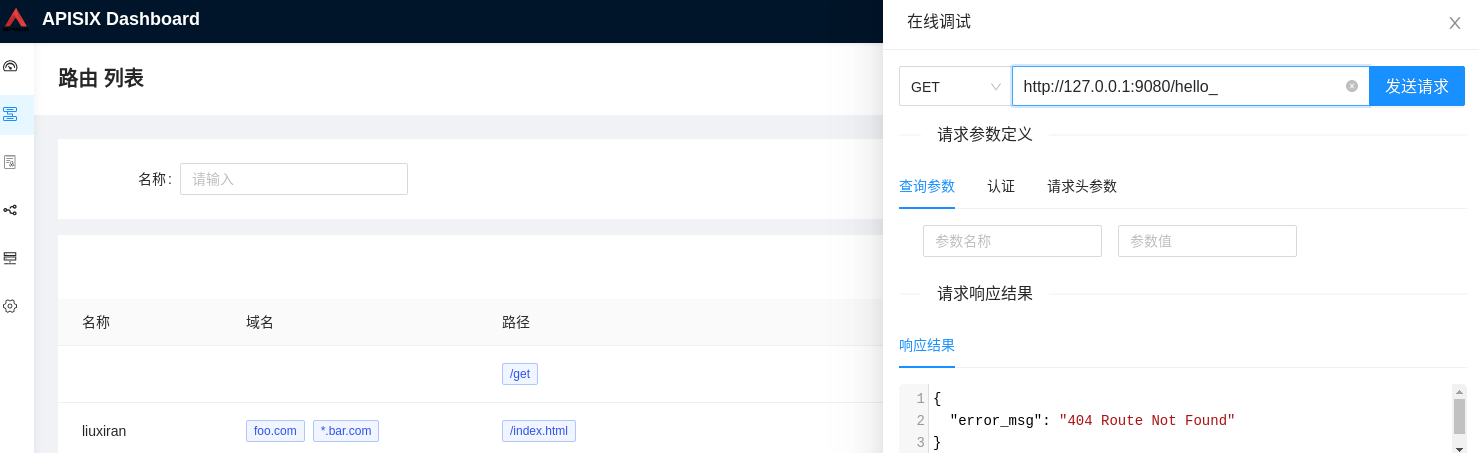
After attempted again and again, it is seems that It has nothing to do with code logic. I still can not find the clue. Ask for help.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] membphis commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
membphis commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r543388169
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -42,7 +42,7 @@ func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
testCaseCheck(tc)
}
basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
- request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET"}`))
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET","protocol": "http"}`))
Review comment:
can we use `http://172.16.238.30` as a global variable?
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -42,7 +42,7 @@ func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
testCaseCheck(tc)
}
basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
- request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET"}`))
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET","protocol": "http"}`))
Review comment:
hard code is not a good way
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (8d7195e) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `1.16%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 40.91% -1.17%
==========================================
Files 23 23
Lines 1414 1459 +45
==========================================
+ Hits 595 597 +2
- Misses 730 771 +41
- Partials 89 91 +2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/handler/consumer/consumer.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvY29uc3VtZXIvY29uc3VtZXIuZ28=) | `34.92% <0.00%> (-5.90%)` | :arrow_down: |
| [api/internal/handler/ssl/ssl.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc3NsL3NzbC5nbw==) | `28.17% <0.00%> (ø)` | |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...8d7195e](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (48e607b) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/c2e0e6fe511e534c071cb460f49727427634126f?el=desc) (c2e0e6f) will **not change** coverage.
> The diff coverage is `n/a`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
=======================================
Coverage 40.51% 40.51%
=======================================
Files 26 26
Lines 1597 1597
=======================================
Hits 647 647
Misses 856 856
Partials 94 94
```
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [c2e0e6f...48e607b](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r546146872
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +412,75 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
+ return result, nil
+}
Review comment:
done
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] nic-chen commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
nic-chen commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-748010232
ping @membphis @tokers
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-743182247
Thanks for @nic-chen @juzhiyuan @LiteSun ‘s help~ all the e2e test cases passed, this pr is ready for review.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r545629595
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -42,7 +42,7 @@ func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
testCaseCheck(tc)
}
basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
- request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET"}`))
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET","protocol": "http"}`))
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (b820402) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/29e6dd51b3b1d40ebcc61cc37275e5a7fda09b31?el=desc) (29e6dd5) will **decrease** coverage by `0.88%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 41.80% 40.91% -0.89%
==========================================
Files 23 23
Lines 1428 1459 +31
==========================================
Hits 597 597
- Misses 740 771 +31
Partials 91 91
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [29e6dd5...b820402](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (7433f52) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/c2e0e6fe511e534c071cb460f49727427634126f?el=desc) (c2e0e6f) will **not change** coverage.
> The diff coverage is `n/a`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
=======================================
Coverage 40.51% 40.51%
=======================================
Files 26 26
Lines 1597 1597
=======================================
Hits 647 647
Misses 856 856
Partials 94 94
```
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [c2e0e6f...7433f52](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r545624500
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +412,75 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
+ return result, nil
+}
Review comment:
> @liuxiran FYI, since the test file is `route_online_debug_test.go`, the recommended name for this separate file is `route_online_debug.go`. Thanks.
got it~
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] imjoey commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
imjoey commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r543860366
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -42,7 +42,7 @@ func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
testCaseCheck(tc)
}
basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
- request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET"}`))
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET","protocol": "http"}`))
Review comment:
@liuxiran maybe define `http://172.16.238.30` as a global constant or variable is the first step. Then we could get to see why `127.0.0.0:9080` is not working. Thanks.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r542274106
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +401,61 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ BodyParams map[string]string `json:"bodyParams,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"headerParams,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer func() {
+ if resp != nil {
+ _ = resp.Body.Close()
+ }
+ }()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
Review comment:
Add a new field `request_protocol ` to distinguish different protocols, and now we only support `http` protocol
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r543415884
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -42,7 +42,7 @@ func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
testCaseCheck(tc)
}
basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
- request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET"}`))
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET","protocol": "http"}`))
Review comment:
> can we use `http://172.16.238.30` as a global variable?
I tried `127.0.0.1:9080` at first, but test case Run failed
until I tried this internal IP `172.16.238.30`of Docker, the debug forwarding request test cases were passed.
I didn't know why actually, maybe it has something to do with our Docker test environment
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (d92dc28) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/29e6dd51b3b1d40ebcc61cc37275e5a7fda09b31?el=desc) (29e6dd5) will **decrease** coverage by `0.63%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 41.80% 41.17% -0.64%
==========================================
Files 23 23
Lines 1428 1445 +17
==========================================
- Hits 597 595 -2
- Misses 740 761 +21
+ Partials 91 89 -2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/handler/consumer/consumer.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvY29uc3VtZXIvY29uc3VtZXIuZ28=) | `40.81% <0.00%> (+5.89%)` | :arrow_up: |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [29e6dd5...d92dc28](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] juzhiyuan commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
juzhiyuan commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-740504841
cc @nic-chen @idbeta
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (9d64dcc) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `2.78%`.
> The diff coverage is `7.40%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 39.29% -2.79%
==========================================
Files 23 26 +3
Lines 1414 1611 +197
==========================================
+ Hits 595 633 +38
- Misses 730 887 +157
- Partials 89 91 +2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `39.47% <7.40%> (-7.09%)` | :arrow_down: |
| [api/internal/core/store/validate.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvdmFsaWRhdGUuZ28=) | `59.88% <0.00%> (-1.41%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/utils/json\_patch.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL3V0aWxzL2pzb25fcGF0Y2guZ28=) | `34.48% <0.00%> (ø)` | |
| [api/internal/core/store/store\_mock.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmVfbW9jay5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/filter/schema.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ZpbHRlci9zY2hlbWEuZ28=) | `0.00% <0.00%> (ø)` | |
| ... and [2 more](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree-more) | |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...9d64dcc](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] nic-chen commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
nic-chen commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r544894439
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +412,75 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
+ return result, nil
+}
Review comment:
we should put these codes in a separate file.
what do you think?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (fbedad2) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `2.99%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 39.08% -3.00%
==========================================
Files 23 26 +3
Lines 1414 1612 +198
==========================================
+ Hits 595 630 +35
- Misses 730 891 +161
- Partials 89 91 +2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `38.42% <0.00%> (-8.14%)` | :arrow_down: |
| [api/internal/core/store/validate.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvdmFsaWRhdGUuZ28=) | `59.88% <0.00%> (-1.41%)` | :arrow_down: |
| [api/internal/core/store/store.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmUuZ28=) | `78.57% <0.00%> (-0.65%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/core/store/store\_mock.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmVfbW9jay5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/filter/schema.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ZpbHRlci9zY2hlbWEuZ28=) | `0.00% <0.00%> (ø)` | |
| ... and [3 more](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree-more) | |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...fbedad2](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (8b7bdcf) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/29e6dd51b3b1d40ebcc61cc37275e5a7fda09b31?el=desc) (29e6dd5) will **decrease** coverage by `0.88%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 41.80% 40.91% -0.89%
==========================================
Files 23 23
Lines 1428 1459 +31
==========================================
Hits 597 597
- Misses 740 771 +31
Partials 91 91
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [29e6dd5...8b7bdcf](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] nic-chen commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
nic-chen commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r543381385
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +401,61 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ BodyParams map[string]string `json:"bodyParams,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"headerParams,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer func() {
+ if resp != nil {
+ _ = resp.Body.Close()
+ }
+ }()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
Review comment:
@liuxiran
Should define an interface and implement `http`.
You could refer:
https://github.com/apache/apisix-dashboard/blob/master/api/internal/core/storage/storage.go
and
https://github.com/apache/apisix-dashboard/blob/master/api/internal/core/storage/etcd.go
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (4c729fc) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `2.77%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 39.30% -2.78%
==========================================
Files 23 26 +3
Lines 1414 1603 +189
==========================================
+ Hits 595 630 +35
- Misses 730 882 +152
- Partials 89 91 +2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/core/store/validate.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvdmFsaWRhdGUuZ28=) | `59.88% <0.00%> (-1.41%)` | :arrow_down: |
| [api/internal/core/store/store.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmUuZ28=) | `78.57% <0.00%> (-0.65%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/utils/json\_patch.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL3V0aWxzL2pzb25fcGF0Y2guZ28=) | `34.48% <0.00%> (ø)` | |
| [api/filter/schema.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ZpbHRlci9zY2hlbWEuZ28=) | `0.00% <0.00%> (ø)` | |
| ... and [3 more](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree-more) | |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...4c729fc](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (d26100f) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `2.71%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 39.36% -2.72%
==========================================
Files 23 26 +3
Lines 1414 1603 +189
==========================================
+ Hits 595 631 +36
- Misses 730 881 +151
- Partials 89 91 +2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/core/store/validate.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvdmFsaWRhdGUuZ28=) | `59.88% <0.00%> (-1.41%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/utils/json\_patch.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL3V0aWxzL2pzb25fcGF0Y2guZ28=) | `34.48% <0.00%> (ø)` | |
| [api/filter/schema.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ZpbHRlci9zY2hlbWEuZ28=) | `0.00% <0.00%> (ø)` | |
| [api/internal/core/store/store\_mock.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmVfbW9jay5nbw==) | `0.00% <0.00%> (ø)` | |
| ... and [2 more](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree-more) | |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...d26100f](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] juzhiyuan merged pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
juzhiyuan merged pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] membphis commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
membphis commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-747459001
@liuxiran I think you can fix the comment soon, then we can merge this PR soon ^_^
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] imjoey commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
imjoey commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-748076378
@liuxiran pls rebase the codes at your convenience. Thanks.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] membphis commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
membphis commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r544886384
##########
File path: api/test/e2e/route_online_debug_test.go
##########
@@ -42,7 +42,7 @@ func TestRoute_Online_Debug_Route_Not_Exist(t *testing.T) {
testCaseCheck(tc)
}
basepath := "http://127.0.0.1:9000/apisix/admin/debug-request-forwarding"
- request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET"}`))
+ request, _ := http.NewRequest("POST", basepath, strings.NewReader(`{"url": "http://172.16.238.30:9080/hello_","method": "GET","protocol": "http"}`))
Review comment:
> maybe define `http://172.16.238.30` as a global constant or variable is the first step.
yes, that is a better way
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] imjoey commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
imjoey commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r545622926
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +412,75 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
+ return result, nil
+}
Review comment:
@liuxiran Just a reminder, since the test file is `route_online_debug_test.go`, the recommended name for this separate file is `route_online_debug.go`. Thanks.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran removed a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran removed a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-747911481
Thanks for @nic-chen 's help, CI passed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (86f5734) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `2.71%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 39.36% -2.72%
==========================================
Files 23 26 +3
Lines 1414 1603 +189
==========================================
+ Hits 595 631 +36
- Misses 730 881 +151
- Partials 89 91 +2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/core/store/validate.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvdmFsaWRhdGUuZ28=) | `59.88% <0.00%> (-1.41%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/utils/json\_patch.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL3V0aWxzL2pzb25fcGF0Y2guZ28=) | `34.48% <0.00%> (ø)` | |
| [api/internal/core/store/store\_mock.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmVfbW9jay5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/filter/schema.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ZpbHRlci9zY2hlbWEuZ28=) | `0.00% <0.00%> (ø)` | |
| ... and [2 more](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree-more) | |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...86f5734](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r545549317
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +412,75 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
+ return result, nil
+}
Review comment:
> we should put these codes in a separate file.
> what do you think?
ok, tring to fix~
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r542274106
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +401,61 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ BodyParams map[string]string `json:"bodyParams,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"headerParams,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer func() {
+ if resp != nil {
+ _ = resp.Body.Close()
+ }
+ }()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
Review comment:
Add a new field `protocol` to distinguish different protocols, and now we only support `http` protocol
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r544826857
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +401,61 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ BodyParams map[string]string `json:"bodyParams,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"headerParams,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer func() {
+ if resp != nil {
+ _ = resp.Body.Close()
+ }
+ }()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
Review comment:
updated, please recheck when you have time @nic-chen ^_^
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] liuxiran commented on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
liuxiran commented on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-748375189
> @liuxiran I think you can fix the comment soon, then we can merge this PR soon ^_^
Thanks for all of your advices and guidances, IMHO this pr is ready for merge, any feedback is appreciated ^_^
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (d0c9275) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `1.06%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 41.01% -1.07%
==========================================
Files 23 24 +1
Lines 1414 1480 +66
==========================================
+ Hits 595 607 +12
- Misses 730 778 +48
- Partials 89 95 +6
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/handler/consumer/consumer.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvY29uc3VtZXIvY29uc3VtZXIuZ28=) | `34.92% <0.00%> (-5.90%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/utils/json\_patch.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL3V0aWxzL2pzb25fcGF0Y2guZ28=) | `34.48% <0.00%> (ø)` | |
| [api/internal/handler/ssl/ssl.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc3NsL3NzbC5nbw==) | `29.47% <0.00%> (+1.30%)` | :arrow_up: |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...d0c9275](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] imjoey commented on a change in pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
imjoey commented on a change in pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#discussion_r545622926
##########
File path: api/internal/handler/route/route.go
##########
@@ -397,3 +412,75 @@ func Exist(c *gin.Context) (interface{}, error) {
return nil, nil
}
+
+type ParamsInput struct {
+ URL string `json:"url,omitempty"`
+ RequestProtocol string `json:"request_protocol,omitempty"`
+ BodyParams map[string]string `json:"body_params,omitempty"`
+ Method string `json:"method,omitempty"`
+ HeaderParams map[string][]string `json:"header_params,omitempty"`
+}
+
+type Result struct {
+ Code int `json:"code,omitempty"`
+ Message string `json:"message,omitempty"`
+ Data interface{} `json:"data,omitempty"`
+}
+
+func (h *Handler) DebugRequestForwarding(c droplet.Context) (interface{}, error) {
+ //TODO: other Protocols, e.g: grpc, websocket
+ paramsInput := c.Input().(*ParamsInput)
+ requestProtocol := paramsInput.RequestProtocol
+ if requestProtocol == "" {
+ requestProtocol = "http"
+ }
+ if v, ok := protocolMap[requestProtocol]; ok {
+ return v.RequestForwarding(c)
+ } else {
+ return &data.SpecCodeResponse{StatusCode: http.StatusBadRequest}, fmt.Errorf("protocol unspported %s", paramsInput.RequestProtocol)
+ }
+}
+
+type HTTPProtocolSupport struct {
+}
+
+func (h *HTTPProtocolSupport) RequestForwarding(c droplet.Context) (interface{}, error) {
+ paramsInput := c.Input().(*ParamsInput)
+ bodyParams, _ := json.Marshal(paramsInput.BodyParams)
+ client := &http.Client{}
+
+ client.Timeout = 5 * time.Second
+ req, err := http.NewRequest(strings.ToUpper(paramsInput.Method), paramsInput.URL, strings.NewReader(string(bodyParams)))
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ for k, v := range paramsInput.HeaderParams {
+ for _, v1 := range v {
+ req.Header.Add(k, v1)
+ }
+ }
+ resp, err := client.Do(req)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ defer resp.Body.Close()
+
+ body, err := ioutil.ReadAll(resp.Body)
+ if err != nil {
+ return &data.SpecCodeResponse{StatusCode: http.StatusInternalServerError}, err
+ }
+ returnData := make(map[string]interface{})
+ result := &Result{}
+ err = json.Unmarshal(body, &returnData)
+ if err != nil {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = string(body)
+ } else {
+ result.Code = resp.StatusCode
+ result.Message = resp.Status
+ result.Data = returnData
+
+ }
+ return result, nil
+}
Review comment:
@liuxiran FYI, since the test file is `route_online_debug_test.go`, the recommended name for this separate file is `route_online_debug.go`. Thanks.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix-dashboard] codecov-io edited a comment on pull request #979: Refactor online debug be
Posted by GitBox <gi...@apache.org>.
codecov-io edited a comment on pull request #979:
URL: https://github.com/apache/apisix-dashboard/pull/979#issuecomment-742940185
# [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=h1) Report
> Merging [#979](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=desc) (72e5368) into [master](https://codecov.io/gh/apache/apisix-dashboard/commit/78db532402a86cbdc4e8d3a58396f126bf313695?el=desc) (78db532) will **decrease** coverage by `0.38%`.
> The diff coverage is `0.00%`.
[](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree)
```diff
@@ Coverage Diff @@
## master #979 +/- ##
==========================================
- Coverage 42.07% 41.69% -0.39%
==========================================
Files 23 25 +2
Lines 1414 1487 +73
==========================================
+ Hits 595 620 +25
- Misses 730 780 +50
+ Partials 89 87 -2
```
| [Impacted Files](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=tree) | Coverage Δ | |
|---|---|---|
| [api/internal/handler/route/route.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvcm91dGUvcm91dGUuZ28=) | `40.00% <0.00%> (-6.57%)` | :arrow_down: |
| [api/internal/core/entity/entity.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvZW50aXR5L2VudGl0eS5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/handler/service/service.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc2VydmljZS9zZXJ2aWNlLmdv) | `27.65% <0.00%> (ø)` | |
| [api/internal/handler/upstream/upstream.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvdXBzdHJlYW0vdXBzdHJlYW0uZ28=) | `19.82% <0.00%> (ø)` | |
| [.../internal/handler/authentication/authentication.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvYXV0aGVudGljYXRpb24vYXV0aGVudGljYXRpb24uZ28=) | `82.35% <0.00%> (ø)` | |
| [api/internal/core/store/store\_mock.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2NvcmUvc3RvcmUvc3RvcmVfbW9jay5nbw==) | `0.00% <0.00%> (ø)` | |
| [api/internal/utils/json\_patch.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL3V0aWxzL2pzb25fcGF0Y2guZ28=) | `34.48% <0.00%> (ø)` | |
| [api/internal/handler/ssl/ssl.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvc3NsL3NzbC5nbw==) | `29.47% <0.00%> (+1.30%)` | :arrow_up: |
| [api/internal/handler/consumer/consumer.go](https://codecov.io/gh/apache/apisix-dashboard/pull/979/diff?src=pr&el=tree#diff-YXBpL2ludGVybmFsL2hhbmRsZXIvY29uc3VtZXIvY29uc3VtZXIuZ28=) | `68.62% <0.00%> (+27.81%)` | :arrow_up: |
------
[Continue to review full report at Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=continue).
> **Legend** - [Click here to learn more](https://docs.codecov.io/docs/codecov-delta)
> `Δ = absolute <relative> (impact)`, `ø = not affected`, `? = missing data`
> Powered by [Codecov](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=footer). Last update [78db532...72e5368](https://codecov.io/gh/apache/apisix-dashboard/pull/979?src=pr&el=lastupdated). Read the [comment docs](https://docs.codecov.io/docs/pull-request-comments).
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org