You are viewing a plain text version of this content. The canonical link for it is here.
Posted to notifications@teaclave.apache.org by GitBox <gi...@apache.org> on 2020/01/14 06:40:30 UTC
[GitHub] [incubator-teaclave-sgx-sdk] yangji12138 opened a new issue #195:
Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
yangji12138 opened a new issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195
I'm a newbee in rust and sgx. When I'm writing some toy examples for sgx_get_quote() in the simulation mode, I meet some problems that I can't solve.
In my main.rs file, I define some extern C function:
```
extern "C" {
pub fn sgx_init_quote(p_target_info: *mut sgx_target_info_t, p_gid: *mut sgx_epid_group_id_t) -> sgx_status_t;
pub fn sgx_calc_quote_size(p_sig_rl: *const uint8_t, sig_rl_size: uint32_t, p_quote_size: *mut uint32_t) -> sgx_status_t;
pub fn sgx_get_quote(p_report: *const sgx_report_t, quote_type: sgx_quote_sign_type_t,
p_spid: *const sgx_spid_t, p_nonce: *const sgx_quote_nonce_t, p_sig_rl: *const uint8_t,
sig_rl_size: uint32_t, p_qe_report: *mut sgx_report_t, p_quote: *mut sgx_quote_t,
quote_size: uint32_t) -> sgx_status_t;
}
```
Then I initialize some paramters to call these functions:
```
let mut target_info = sgx_target_info_t::default();
let mut gid = sgx_epid_group_id_t::default();
let status = unsafe { sgx_init_quote(&mut target_info, &mut gid) };
if status != sgx_status_t::SGX_SUCCESS {
println!("[-] Get error when get_init_quote, {}", status.as_str());
return;
}
println!("SGX_INIT_QUOTE SUCCESS!");
let mut quote_size: u32 = 0;
let status = unsafe { sgx_calc_quote_size(std::ptr::null(), 0, &mut quote_size) };
if status != sgx_status_t::SGX_SUCCESS {
println!("[-] Get error when sgx_calc_quote_size, {}", status.as_str());
return;
}
println!("SGX_calc_quote_size SUCCESS!");
let spid = sgx_spid_t::default();
let mut report = sgx_report_t::default();
let mut the_quote = vec![0u8; quote_size as usize].into_boxed_slice();
let status = unsafe {
sgx_get_quote(&report,
sgx_quote_sign_type_t::SGX_UNLINKABLE_SIGNATURE,
&spid,
std::ptr::null(),
std::ptr::null(),
0,
std::ptr::null_mut(),
the_quote.as_mut_ptr() as *mut sgx_quote_t,
quote_size,
)
};
if status != sgx_status_t::SGX_SUCCESS {
println!("[-] Get error when sgx_get_quote, {}", status.as_str());
return;
}
println!("SGX_GET_QUOTE SUCCESS!");
```
The results show that I can call sgx_init_quote() and sgx_calc_quote_size() successfully. But when I call sgx_get_quote(), it returns internal error "SGX_ERROR_INVALID_PARAMETER". I'm really confused of it because I set it as default value.
The following is the result:
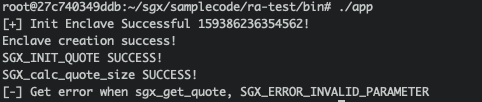
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org
[GitHub] [incubator-teaclave-sgx-sdk] yangji12138 commented on issue #195:
Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
Posted by GitBox <gi...@apache.org>.
yangji12138 commented on issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195#issuecomment-574520277
Thanks for your help. According to the official document, I implement get_quote_func() just in the untrusted app. I try many different ways to initialize the paramter, but none of them work..
Following the sample code provided by ue-ra, I wrote a version. But it has the same problem:
```
// Init Enclave parameter
let quote_type = sgx_quote_sign_type_t::SGX_LINKABLE_SIGNATURE;
let spid = sgx_spid_t::default();
let p_spid = &spid as *const sgx_spid_t;
// Optional Output: qe_report could be null
let mut qe_report = sgx_report_t::default();
const RET_QUOTE_BUF_LEN : u32 = 2048;
let mut return_quote_buf : [u8; RET_QUOTE_BUF_LEN as usize] = [0;RET_QUOTE_BUF_LEN as usize];
let p_quote = return_quote_buf.as_mut_ptr();
let max_len = RET_QUOTE_BUF_LEN;
let mut quote_len : u32 = 0;
let p_quote_len = &mut quote_len as *mut u32;
// NULL Pointer
let (p_sigrl, sigrl_len) = (ptr::null() as *const u8, 0);
let quote_nonce = sgx_quote_nonce_t::default();
let p_nonce = "e_nonce as * const sgx_quote_nonce_t;
// Init Enclave
let mut ti : sgx_target_info_t = sgx_target_info_t::default();
let mut eg : sgx_epid_group_id_t = sgx_epid_group_id_t::default();
let mut rt : sgx_status_t = sgx_status_t::SGX_ERROR_UNEXPECTED;
let res = unsafe {
rust_init_quote(&mut ti as *mut sgx_target_info_t,
&mut eg as *mut sgx_epid_group_id_t)
};
println!("eg = {:?}", eg);
if res != sgx_status_t::SGX_SUCCESS {
println!("Get error when init quote");
return;
}
let result = unsafe {
rust_get_quote(p_sigrl,
sigrl_len,
p_report,
quote_type,
p_spid,
p_nonce,
&mut qe_report as *mut sgx_report_t,
p_quote,
max_len,
p_quote_len)
};
```
Really confused. I was wondering whether it is because that I use a simulation mode.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org
[GitHub] [incubator-teaclave-sgx-sdk] dingelish edited a comment on issue
#195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
Posted by GitBox <gi...@apache.org>.
dingelish edited a comment on issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195#issuecomment-574040669
Please look closer into Intel's manual:
https://download.01.org/intel-sgx/sgx-linux/2.7.1/docs/Intel_SGX_Developer_Reference_Linux_2.7.1_Open_Source.pdf
sgx_get_quote is on page 163-165.
at the bottom of page 165, intel writes clearly:
> Library: Requirements - libsgx_epid.so or libsgx_uae_service_sim.so (simulation)
these two requirements (two general linux shared object), as well as the name of "uae_service", both indicate that this function can only be called in the untrusted app. you cannot call it in the enclave.
you can look into the mutual-ra enclave sample to see how to generate a quote:
https://github.com/apache/incubator-teaclave-sgx-sdk/blob/master/samplecode/mutual-ra/enclave/src/lib.rs#L373
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org
[GitHub] [incubator-teaclave-sgx-sdk] uraj commented on issue #195: Get
SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
Posted by GitBox <gi...@apache.org>.
uraj commented on issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195#issuecomment-574552201
You need to initialize the report using `sgx_create_report`
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org
[GitHub] [incubator-teaclave-sgx-sdk] dingelish commented on issue #195: Get
SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
Posted by GitBox <gi...@apache.org>.
dingelish commented on issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195#issuecomment-574040669
Please look closer into Intel's manual:
https://download.01.org/intel-sgx/sgx-linux/2.7.1/docs/Intel_SGX_Developer_Reference_Linux_2.7.1_Open_Source.pdf
sgx_get_quote is on page 163-165.
at the bottom of page 165, intel writes clearly:
> Library: Requirements - libsgx_epid.so or libsgx_uae_service_sim.so (simulation)
these two requirements (two general linux shared object), as well as the name of "uae_service) both indicate that this function can only be called in the untrusted app. you cannot call it in the enclave.
you can look into the mutual-ra enclave sample to see how to generate a quote:
https://github.com/apache/incubator-teaclave-sgx-sdk/blob/master/samplecode/mutual-ra/enclave/src/lib.rs#L373
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org
[GitHub] [incubator-teaclave-sgx-sdk] yangji12138 closed issue #195: Get
SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
Posted by GitBox <gi...@apache.org>.
yangji12138 closed issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org
[GitHub] [incubator-teaclave-sgx-sdk] yangji12138 commented on issue #195:
Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
Posted by GitBox <gi...@apache.org>.
yangji12138 commented on issue #195: Get SGX_ERROR_INVALID_PARAMETER when Calling sgx_get_quote()
URL: https://github.com/apache/incubator-teaclave-sgx-sdk/issues/195#issuecomment-574695618
> You need to initialize the report using `sgx_create_report`
Problem Sloved. Many Thanks!
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@teaclave.apache.org
For additional commands, e-mail: notifications-help@teaclave.apache.org