You are viewing a plain text version of this content. The canonical link for it is here.
Posted to dev@wicket.apache.org by GitBox <gi...@apache.org> on 2021/04/08 10:43:27 UTC
[GitHub] [wicket] reiern70 opened a new pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
reiern70 opened a new pull request #467:
URL: https://github.com/apache/wicket/pull/467
[WICKET-6876] add an AJAX behavior to transfer client side file information to server side (when a file is selected for upload at client side).
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817954505
@martin-g Many thanks for review and feedback. I have addressed your comments.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-818522077
@svenmeier and @martin-g Many thanks for reviews. Rebased and squashed commits. Merging now.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611442833
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
+ super.renderHead(component, response);
+ }
+
+ /**
+ * Creates an {@link OnFilesSelectedBehavior} based on lambda expressions
+ *
+ * @param select {@link SerializableBiConsumer}
+ *
+ * @return the {@link org.apache.wicket.markup.html.form.upload.OnFilesSelectedBehavior} behavior
+ */
+ public static OnFilesSelectedBehavior select(
+ SerializableBiConsumer<AjaxRequestTarget, List<FileDescription>> select)
Review comment:
Here, I think, he meant the class name, not the method name,
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611418517
##########
File path: wicket-examples/src/main/java/org/apache/wicket/examples/ajax/builtin/EffectsPage.html
##########
@@ -1,5 +1,14 @@
<?xml version="1.0" encoding="UTF-8" ?>
<html xmlns:wicket="http://wicket.apache.org">
+<head>
Review comment:
Hm... no idea. Reverting back. Thanks for spotting this.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611783871
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
Review comment:
Fixed this too
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817105914
My IDE suggest me to use let instead of var
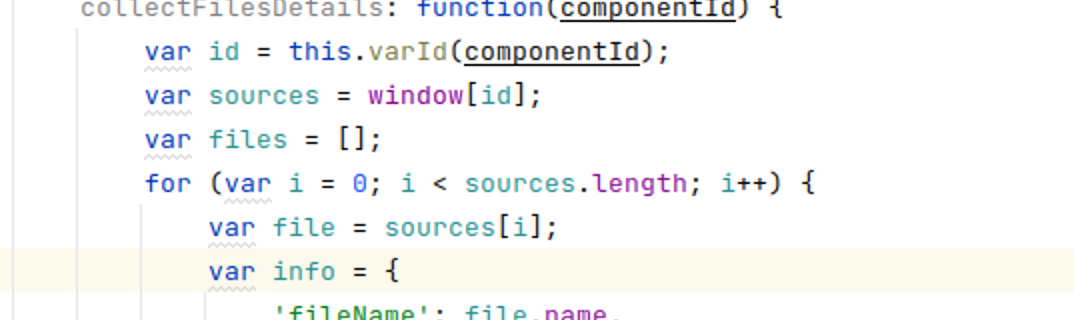
I was using let, reverting to use var otherwise build fails.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 merged pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 merged pull request #467:
URL: https://github.com/apache/wicket/pull/467
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-816488695
> IMHO OnFileSelectedBehavior deserves to be a top-level class. Maybe with its own JS resource, since the script to collect dynamicExtraParameters is getting very long now.
>
> With a static factory method users could use a lambda expression (like we have for several other behaviors, e.g. AjaxFormSubmitBehavior#onSubmit):
>
> ```
> file.add(OnFileSelectedBehavior.onSelected((AjaxRequestTarget target, List<OnFileSelectedBehavior.FileDescription> fileDescriptions) -> {
> // ...
> }));
> ```
>
> Maybe drop the "On"-prefix too, since almost all current behaviors don't have it - are you doing Android development? ;)
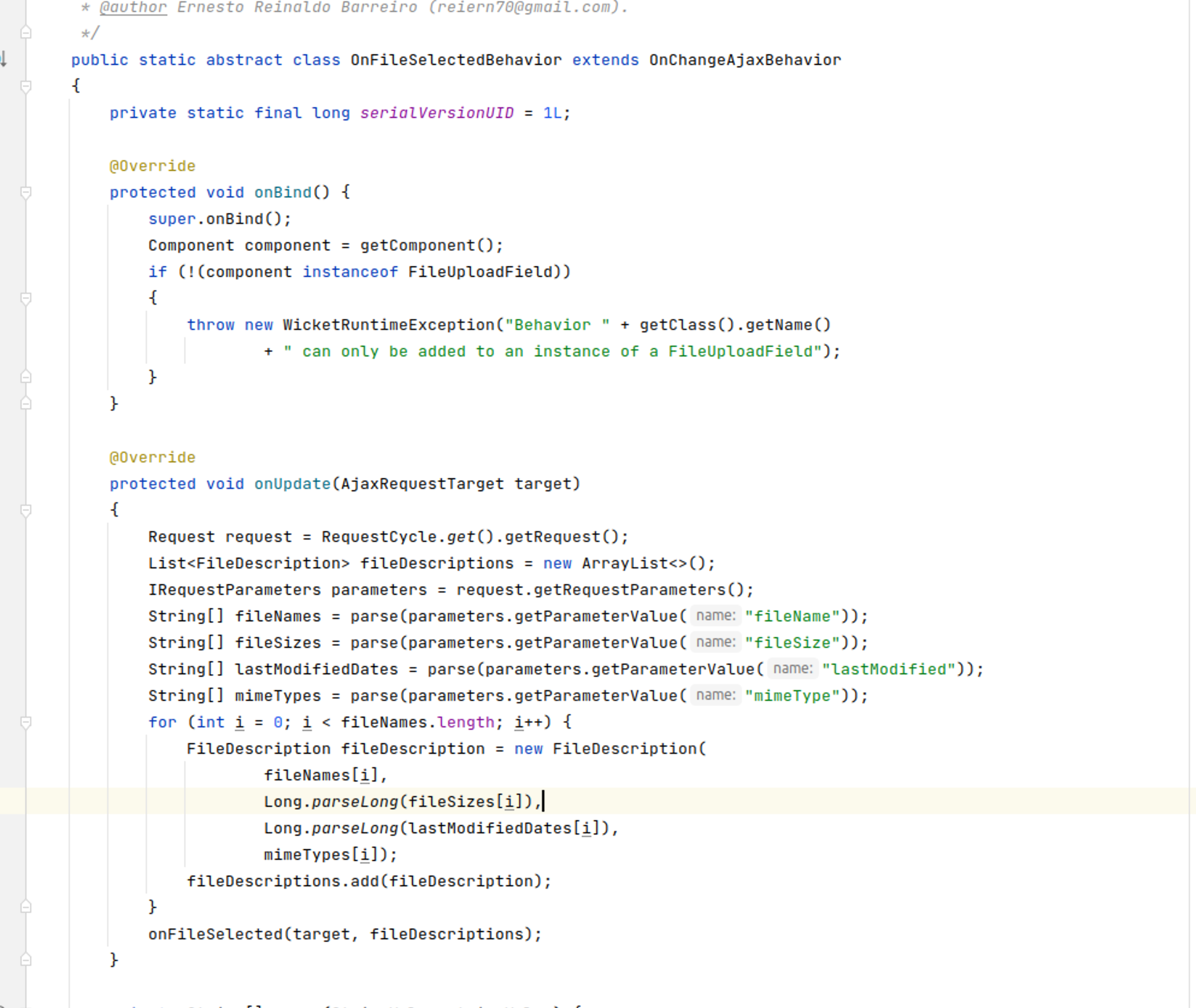
I see a lot of 'On' hanging around. Thus unless there is a very strong opinion about it from most of the developers I will keep it.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-816443335
@svenmeier and @martin-g
Thanks for your comments:
> IMHO OnFileSelectedBehavior deserves to be a top-level class.
My rationale was the following: this class will only be used in relation with FileUploadField, why then pollute package? Of course, this is a matter of taste and consistency with project practices/conventions. Moving it top-level class.
> Maybe with its own JS resource, since the script to collect dynamicExtraParameters is getting very long now.
True.
> With a static factory method users could use a lambda expression (like we have for several other behaviors, e.g. AjaxFormSubmitBehavior#onSubmit):
>
> ```
> file.add(OnFileSelectedBehavior.onSelected((AjaxRequestTarget target, List<OnFileSelectedBehavior.FileDescription> fileDescriptions) -> {
> // ...
> }));
> ```
>
> Maybe drop the "On"-prefix too, since almost all current behaviors don't have it - are you doing Android development? ;)
Never did Android development in my life. If you both agree this should be done I will gladly do it ;-)
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612185917
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
+ console.log(id)
Review comment:
debug leftover
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
Review comment:
you can move this line inside the `if` below
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.java
##########
@@ -0,0 +1,130 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file(s) (at client side), before uploading it (them).
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class FilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(FilesSelectedBehavior.class, "FilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++)
+ {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.FilesSelected.precondition(this);";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.FilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
Review comment:
For simplification you can use again JavaScript's `this` as in the precondition. And in the JS code : `inputField.id` to get the id.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817962998
> > **Note:** A just thought that a possible addition in case of multiple file uploads is a component with a table displaying all the selected files. WDYT?
>
> I don't think we should add such component. Every application may want to display it in a different way.
True. I have added some example on how to do that on ajax-upload page
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611442808
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
Review comment:
fixed.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817105190
> @svenmeier and @martin-g
>
> Thanks for your comments:
>
> > IMHO OnFileSelectedBehavior deserves to be a top-level class.
>
> My rationale was the following: this class will only be used in relation with FileUploadField, why then pollute package? Of course, this is a matter of taste and consistency with project practices/conventions. Moving it top-level class.
>
> > Maybe with its own JS resource, since the script to collect dynamicExtraParameters is getting very long now.
>
> True.
>
> > With a static factory method users could use a lambda expression (like we have for several other behaviors, e.g. AjaxFormSubmitBehavior#onSubmit):
> > ```
> > file.add(OnFileSelectedBehavior.onSelected((AjaxRequestTarget target, List<OnFileSelectedBehavior.FileDescription> fileDescriptions) -> {
> > // ...
> > }));
> > ```
> >
> >
> > Maybe drop the "On"-prefix too, since almost all current behaviors don't have it - are you doing Android development? ;)
>
> Never did Android development in my life. If you both agree this should be done I will gladly do it ;-)
@svenmeier My latest commit introduces factory method as what you suggested
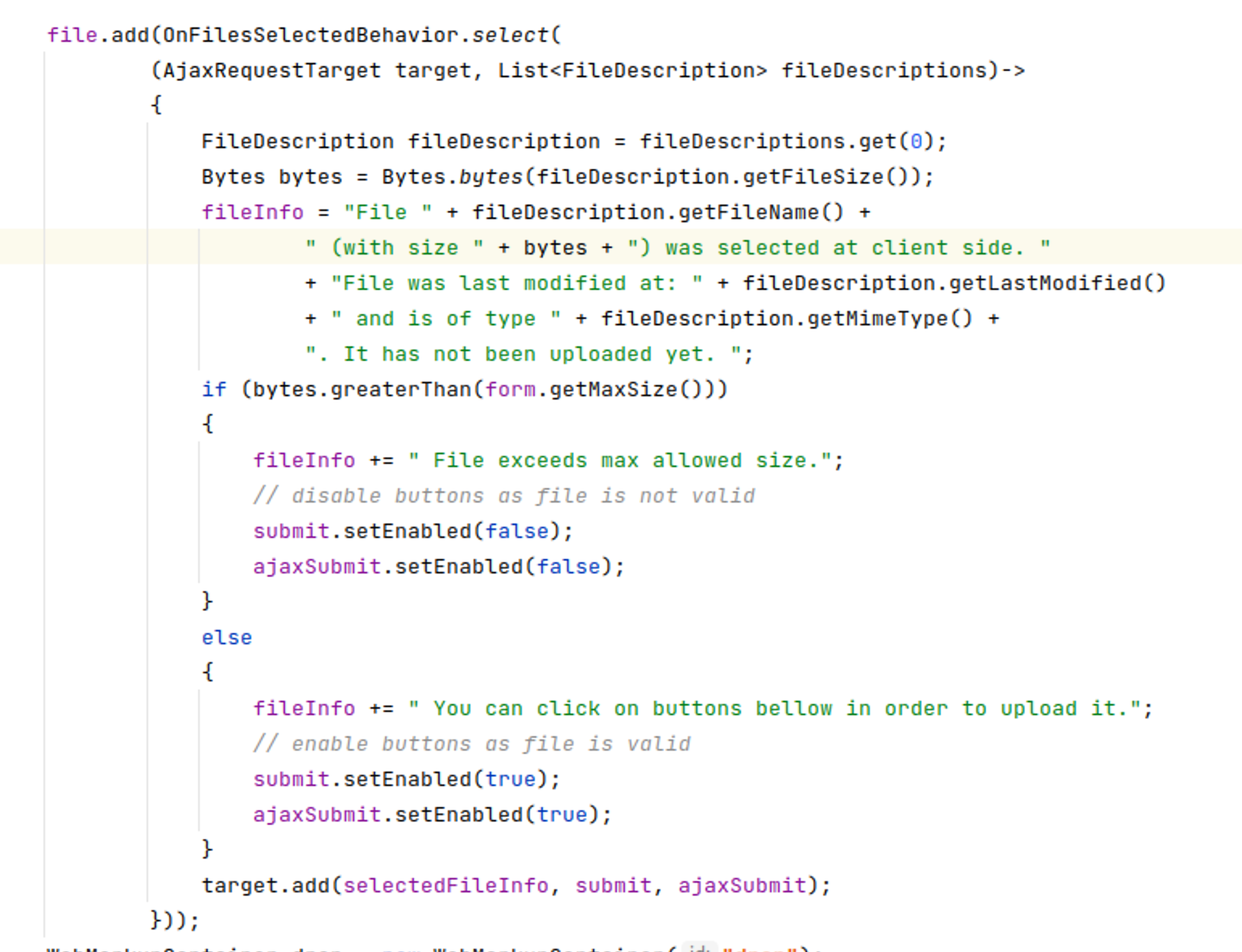
thanks for pointing this out.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611435810
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
Review comment:
Thanks.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612213482
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
+ console.log(id)
Review comment:
I see... Thanks for info. I see you can maybe also type Wicket.Log.enable=true; in console to enable it for some page.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611785063
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
+ super.renderHead(component, response);
+ }
+
+ /**
+ * Creates an {@link OnFilesSelectedBehavior} based on lambda expressions
+ *
+ * @param select {@link SerializableBiConsumer}
+ *
+ * @return the {@link org.apache.wicket.markup.html.form.upload.OnFilesSelectedBehavior} behavior
+ */
+ public static OnFilesSelectedBehavior select(
+ SerializableBiConsumer<AjaxRequestTarget, List<FileDescription>> select)
Review comment:
renamed behavior to FilesSelectedBehavior and factory method to onSelected.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612196391
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
+ console.log(id)
Review comment:
Do we have a Wicket.log? On our private project I have added a log function what can be enabled via some flag you can change at browser console (to switch on debugging).
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r610424557
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
Review comment:
To myself: can we use getParameterValues instead?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817473232
rebased on top oof latest master + squashed commits. Waiting for ok to merge )or do more changes if asked)
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611791702
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -21,12 +21,21 @@
import java.util.List;
import org.apache.commons.fileupload.FileItem;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
import org.apache.wicket.markup.ComponentTag;
import org.apache.wicket.markup.html.form.FormComponent;
import org.apache.wicket.model.IModel;
import org.apache.wicket.protocol.http.IMultipartWebRequest;
+import org.apache.wicket.request.IRequestParameters;
import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
import org.apache.wicket.util.convert.ConversionException;
+import org.apache.wicket.util.string.StringValue;
Review comment:
Fixed too.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r610422618
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
+ Long.parseLong(lastModifiedDates[i]),
+ mimeTypes[i]);
+ fileDescriptions.add(fileDescription);
+ }
+ onFileSelected(target, fileDescriptions);
+ }
+
+ private String[] parse(StringValue stringValue) {
+ return stringValue.toString().split(",");
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
Review comment:
ok.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612204146
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
+ console.log(id)
Review comment:
Yes - https://github.com/apache/wicket/blob/048e5681df960018722237ee6254273e839b5fd2/wicket-core/src/main/java/org/apache/wicket/ajax/res/js/wicket-ajax-jquery.js#L189-L222
It could be enabled/disabled via DebugSettings#ajaxDebugModeEnabled
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] svenmeier commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
svenmeier commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-816178510
IMHO OnFileSelectedBehavior deserves to be a top-level class. Maybe with its own JS resource, since the script to collect dynamicExtraParameters is getting very long now.
With a static factory method users could use a lambda expression (like we have for several other behaviors, e.g. AjaxFormSubmitBehavior#onSubmit):
file.add(OnFileSelectedBehavior.onSelected((AjaxRequestTarget target, List<OnFileSelectedBehavior.FileDescription> fileDescriptions) -> {
// ...
}));
Maybe drop the "On"-prefix too, since almost all current behaviors don't have it - are you doing Android development? ;)
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611606297
##########
File path: wicket-examples/src/main/java/org/apache/wicket/examples/ajax/builtin/FileUploadPage$MultipleFileUploadsSamplePanel.html
##########
@@ -0,0 +1,37 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<html xmlns="http://www.w3.org/1999/xhtml" xmlns:wicket="http://wicket.apache.org">
+<head>
+ <wicket:head>
+ <script type="text/css">
+ table.files {
+ width: 1000px;
+ display: table;
+ }
+ table.files tbody {
+ width: 100%;
+ }
+ table.files td {
+ width: 25%;
+ }
+ </script>s
Review comment:
Fixed.
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
+ * @param componentId
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (component, componentId) {
+ var id = this.varId(componentId);
+ if (component.files && component.files.length > 0) {
+ window[id] = component.files;
+ return true;
+ }
+ return false;
+ },
+
+ /**
+ * Collects selected files IDs
Review comment:
Fixed.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612204956
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.java
##########
@@ -0,0 +1,130 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file(s) (at client side), before uploading it (them).
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class FilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(FilesSelectedBehavior.class, "FilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++)
+ {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.FilesSelected.precondition(this);";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.FilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
Review comment:
I might be wrong but I liked how you did it in `precondition()`!
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-816784650
The new section on examples looks like
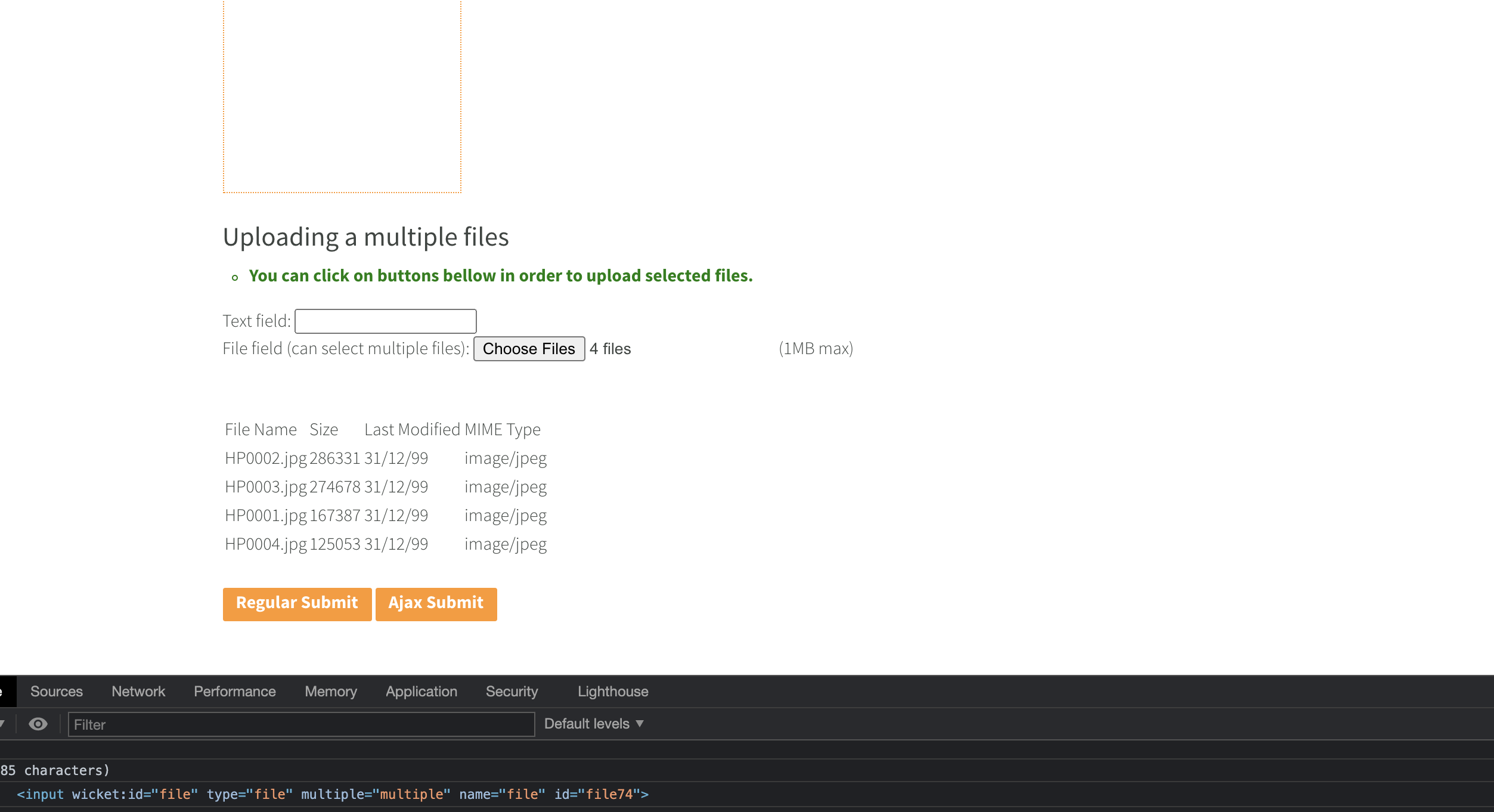
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612194043
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.java
##########
@@ -0,0 +1,130 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file(s) (at client side), before uploading it (them).
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class FilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(FilesSelectedBehavior.class, "FilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++)
+ {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.FilesSelected.precondition(this);";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.FilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
Review comment:
Hum... I was not sure this would work: i.e. that in this case this will point to inputField, this is the reason I didn't try that. Giving it a try
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611443395
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
Review comment:
done.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611435382
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
Review comment:
No particular reason I think. I will fix.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612205551
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.java
##########
@@ -0,0 +1,130 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file(s) (at client side), before uploading it (them).
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class FilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(FilesSelectedBehavior.class, "FilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++)
+ {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.FilesSelected.precondition(this);";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.FilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
Review comment:
This does not seems to refer to inputField in this case. I could use attrs.c as it is the ID but I do not see and extra benefit in this. Keeping as it is.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611441866
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
Review comment:
Fixed.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611417326
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
+ super.renderHead(component, response);
+ }
+
+ /**
+ * Creates an {@link OnFilesSelectedBehavior} based on lambda expressions
+ *
+ * @param select {@link SerializableBiConsumer}
+ *
+ * @return the {@link org.apache.wicket.markup.html.form.upload.OnFilesSelectedBehavior} behavior
+ */
+ public static OnFilesSelectedBehavior select(
+ SerializableBiConsumer<AjaxRequestTarget, List<FileDescription>> select)
Review comment:
Hum... Maybe I understood not correctly what @svenmeier was asking?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-816784007
@svenmeier and @martin-g
Thanks for your feedback and useful comments. I have reworked PR taking into account your comments. Can you please review again?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611431050
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
+ super.renderHead(component, response);
+ }
+
+ /**
+ * Creates an {@link OnFilesSelectedBehavior} based on lambda expressions
+ *
+ * @param select {@link SerializableBiConsumer}
+ *
+ * @return the {@link org.apache.wicket.markup.html.form.upload.OnFilesSelectedBehavior} behavior
+ */
+ public static OnFilesSelectedBehavior select(
+ SerializableBiConsumer<AjaxRequestTarget, List<FileDescription>> select)
Review comment:
@svenmeier said "file.add(OnFileSelectedBehavior.onSelected"
I am fine with either `onSelect` and `onSelected` but I like `onSelect` better, it is more consistent with the rest similar methods.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612216948
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
+ console.log(id)
Review comment:
Yes! It will survive until the next page reload.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r612196582
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.FilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for FilesSelectedBehavior.
+ */
+ Wicket.FilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (or not) server round-trip.
+ *
+ * @param inputField the file input field
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (inputField) {
+ var id = this.varId(inputField.id);
Review comment:
done.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611436687
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
+ super.renderHead(component, response);
+ }
+
+ /**
+ * Creates an {@link OnFilesSelectedBehavior} based on lambda expressions
+ *
+ * @param select {@link SerializableBiConsumer}
+ *
+ * @return the {@link org.apache.wicket.markup.html.form.upload.OnFilesSelectedBehavior} behavior
+ */
+ public static OnFilesSelectedBehavior select(
+ SerializableBiConsumer<AjaxRequestTarget, List<FileDescription>> select)
Review comment:

?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-816444615
**Note:** A just thought that a possible addition in case of multiple file uploads is a component with a table displaying all the selected files. WDYT?
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r609576034
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +57,74 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ IRequestParameters parameters = request.getRequestParameters();
+ String fileName = parameters.getParameterValue("fileName").toString();
+ long fileSize = parameters.getParameterValue("fileSize").toLong(0);
+ Date lastModified = new Date(parameters.getParameterValue("lastModified").toLong(0));
+ String mimeType = parameters.getParameterValue("mimeType").toString();
+ onFileSelected(target, fileName, fileSize, lastModified, mimeType);
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileName The client file name
+ * @param fileSize The client file size
+ * @param lastModified The {@link java.util.Date} when file was last modified.
+ * @param mimeType The MIME type of file.
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, String fileName, long fileSize,
Review comment:
I think we should think about the `multiple` support now, because later this method signature may need to be changed to something like `protected abstract void onFilesSelected(AjaxRequestTarget target, List<FileDescription>)`
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611600218
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
+ * @param componentId
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (component, componentId) {
+ var id = this.varId(componentId);
+ if (component.files && component.files.length > 0) {
+ window[id] = component.files;
Review comment:
Yes. I think we can.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r610423763
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
+ Long.parseLong(lastModifiedDates[i]),
+ mimeTypes[i]);
+ fileDescriptions.add(fileDescription);
+ }
+ onFileSelected(target, fileDescriptions);
+ }
+
+ private String[] parse(StringValue stringValue) {
+ return stringValue.toString().split(",");
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes) {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "if (this.files) { window." + component.getMarkupId() + "_files = this.files; return true; } " +
Review comment:
Also I though about cleaning garbage after use it: this only needs to be kept in context of this AJAX request. Maybe we can even use attrs object as a holder. I will check.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611363797
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -21,12 +21,21 @@
import java.util.List;
import org.apache.commons.fileupload.FileItem;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
import org.apache.wicket.markup.ComponentTag;
import org.apache.wicket.markup.html.form.FormComponent;
import org.apache.wicket.model.IModel;
import org.apache.wicket.protocol.http.IMultipartWebRequest;
+import org.apache.wicket.request.IRequestParameters;
import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
import org.apache.wicket.util.convert.ConversionException;
+import org.apache.wicket.util.string.StringValue;
Review comment:
It seems these imports are no more used.
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
Review comment:
this line should be removed
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
Review comment:
what is the type of `component` here ?
This parameter name is a little confusing. It sounds like a Wicket Component, but it is something else.
##########
File path: wicket-examples/src/main/java/org/apache/wicket/examples/ajax/builtin/EffectsPage.html
##########
@@ -1,5 +1,14 @@
<?xml version="1.0" encoding="UTF-8" ?>
<html xmlns:wicket="http://wicket.apache.org">
+<head>
Review comment:
Why EffectsPage.html is modified ?
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
Review comment:
Any reason `super.**` to be called after ? I don't think there is any difference which one is first in this case. It just looks suspicious this way.
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
Review comment:
... selected file`(s)` before uploading it/them.
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.java
##########
@@ -0,0 +1,134 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+package org.apache.wicket.markup.html.form.upload;
+
+import java.util.ArrayList;
+import java.util.List;
+import javax.servlet.http.HttpServletRequest;
+import org.apache.wicket.Component;
+import org.apache.wicket.WicketRuntimeException;
+import org.apache.wicket.ajax.AjaxRequestTarget;
+import org.apache.wicket.ajax.attributes.AjaxRequestAttributes;
+import org.apache.wicket.ajax.attributes.IAjaxCallListener;
+import org.apache.wicket.ajax.form.AjaxFormSubmitBehavior;
+import org.apache.wicket.ajax.form.OnChangeAjaxBehavior;
+import org.apache.wicket.markup.head.IHeaderResponse;
+import org.apache.wicket.markup.head.JavaScriptHeaderItem;
+import org.apache.wicket.request.IRequestParameters;
+import org.apache.wicket.request.Request;
+import org.apache.wicket.request.cycle.RequestCycle;
+import org.apache.wicket.request.resource.JavaScriptResourceReference;
+import org.apache.wicket.request.resource.ResourceReference;
+import org.apache.wicket.util.lang.Args;
+import org.apache.wicket.util.string.StringValue;
+import org.danekja.java.util.function.serializable.SerializableBiConsumer;
+import org.danekja.java.util.function.serializable.SerializableConsumer;
+import com.github.openjson.JSONArray;
+import com.github.openjson.JSONObject;
+import com.github.openjson.JSONTokener;
+
+/**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+ * s *
+ *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+public abstract class OnFilesSelectedBehavior extends OnChangeAjaxBehavior {
+
+ private static final long serialVersionUID = 1L;
+
+ private static final ResourceReference JS = new JavaScriptResourceReference(OnFilesSelectedBehavior.class, "OnFilesSelectedBehavior.js");
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField)) {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target) {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ // data is streamed as JSON.
+ StringValue fileInfos = parameters.getParameterValue("fileInfos");
+ JSONArray jsonArray = new JSONArray(fileInfos.toString());
+ for (int i = 0; i < jsonArray.length(); i++) {
+ fileDescriptions.add(new FileDescription((JSONObject)jsonArray.get(i)));
+ }
+ onSelected(target, fileDescriptions);
+ }
+
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes)
+ {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "return Wicket.OnFilesSelected.precondition(this, '" + component.getMarkupId() + "');";
+ }
+ });
+ attributes.getDynamicExtraParameters().add("return Wicket.OnFilesSelected.collectFilesDetails('" + getComponent().getMarkupId() + "');");
+ }
+
+ @Override
+ public void renderHead(Component component, IHeaderResponse response)
+ {
+ response.render(JavaScriptHeaderItem.forReference(JS));
+ super.renderHead(component, response);
+ }
+
+ /**
+ * Creates an {@link OnFilesSelectedBehavior} based on lambda expressions
+ *
+ * @param select {@link SerializableBiConsumer}
+ *
+ * @return the {@link org.apache.wicket.markup.html.form.upload.OnFilesSelectedBehavior} behavior
+ */
+ public static OnFilesSelectedBehavior select(
+ SerializableBiConsumer<AjaxRequestTarget, List<FileDescription>> select)
Review comment:
For consistency with the other similar methods the method name should be `onSelect`
https://github.com/apache/wicket/blob/048e5681df960018722237ee6254273e839b5fd2/wicket-core/src/main/java/org/apache/wicket/ajax/AjaxEventBehavior.java#L166
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
Review comment:
s/oor/or/
##########
File path: wicket-examples/src/main/java/org/apache/wicket/examples/ajax/builtin/FileUploadPage$MultipleFileUploadsSamplePanel.html
##########
@@ -0,0 +1,37 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<html xmlns="http://www.w3.org/1999/xhtml" xmlns:wicket="http://wicket.apache.org">
+<head>
+ <wicket:head>
+ <script type="text/css">
+ table.files {
+ width: 1000px;
+ display: table;
+ }
+ table.files tbody {
+ width: 100%;
+ }
+ table.files td {
+ width: 25%;
+ }
+ </script>s
Review comment:
dangling `s` at the end of the line
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
+ * @param componentId
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (component, componentId) {
+ var id = this.varId(componentId);
+ if (component.files && component.files.length > 0) {
+ window[id] = component.files;
+ return true;
+ }
+ return false;
+ },
+
+ /**
+ * Collects selected files IDs
Review comment:
s/IDs/details/ ?
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
+ * @param componentId
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (component, componentId) {
+ var id = this.varId(componentId);
+ if (component.files && component.files.length > 0) {
+ window[id] = component.files;
Review comment:
Can we use `Wicket.OnFilesSelected[id]` instead of `window[id]` ?
We should try to not pollute the global namespace, even temporarily.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611606118
##########
File path: wicket-examples/src/main/java/org/apache/wicket/examples/ajax/builtin/EffectsPage.html
##########
@@ -1,5 +1,14 @@
<?xml version="1.0" encoding="UTF-8" ?>
<html xmlns:wicket="http://wicket.apache.org">
+<head>
Review comment:
Fixed.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r611617845
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/OnFilesSelectedBehavior.js
##########
@@ -0,0 +1,79 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+;(function (undefined) {
+
+ 'use strict';
+
+ if (typeof(Wicket.OnFilesSelected) === 'object') {
+ return;
+ }
+
+ /**
+ * Contains JS code for OnFilesSelectedBehavior.
+ */
+ Wicket.OnFilesSelected = {
+
+ // the id of temporary object holding files.
+ varId: function (componentId) {
+ return componentId + "_files";
+ },
+
+ /**
+ * Precondition to trigger (oor not) server round-trip.
+ *
+ * @param component
+ * @param componentId
+ * @returns {boolean} true if some files (file) were (was) selected,
+ */
+ precondition : function (component, componentId) {
+ var id = this.varId(componentId);
+ if (component.files && component.files.length > 0) {
+ window[id] = component.files;
Review comment:
fixed
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r610330790
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
Review comment:
Prefer `Long.parseLong(String s, int radix)` instead
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
+ Long.parseLong(lastModifiedDates[i]),
+ mimeTypes[i]);
+ fileDescriptions.add(fileDescription);
+ }
+ onFileSelected(target, fileDescriptions);
+ }
+
+ private String[] parse(StringValue stringValue) {
+ return stringValue.toString().split(",");
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
Review comment:
s/onFileSelected/onSelect/ ? I think @svenmeier also suggested this name
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
+ Long.parseLong(lastModifiedDates[i]),
+ mimeTypes[i]);
+ fileDescriptions.add(fileDescription);
+ }
+ onFileSelected(target, fileDescriptions);
+ }
+
+ private String[] parse(StringValue stringValue) {
+ return stringValue.toString().split(",");
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes) {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "if (this.files) { window." + component.getMarkupId() + "_files = this.files; return true; } " +
+ "else return false;";
+ }
+ });
+
+ String varName = "window."+getComponent().getMarkupId()+"_files";
+ attributes.getDynamicExtraParameters().add("var files = {};" +
+ "var fileNames = [];" +
+ "var fileSizes = [];" +
+ "var lastModifiedDates = [];" +
+ "var mimeTypes = [];" +
+ "for (var i = 0; i < "+varName+".length; i++) {" +
+ " f = " + varName + "[i];" +
Review comment:
missing `var` for `f`
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
+ Long.parseLong(lastModifiedDates[i]),
+ mimeTypes[i]);
+ fileDescriptions.add(fileDescription);
+ }
+ onFileSelected(target, fileDescriptions);
+ }
+
+ private String[] parse(StringValue stringValue) {
+ return stringValue.toString().split(",");
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes) {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "if (this.files) { window." + component.getMarkupId() + "_files = this.files; return true; } " +
Review comment:
Let's put the new property to `window.Wicket` namespace instead of to the global `window` namespace.
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +59,146 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * Description of file properties as in browser client side.
+ */
+ public static class FileDescription implements Serializable {
+
+ private static final long serialVersionUID = 1L;
+
+ private final String fileName;
+ private final long fileSize;
+ private final Date lastModified;
+ private final String mimeType;
+
+ public FileDescription(String fileName, long fileSize, long lastModified, String mimeType) {
+ this.fileName = fileName;
+ this.fileSize = fileSize;
+ this.lastModified = new Date(lastModified);
+ this.mimeType = mimeType;
+ }
+
+ public String getFileName() {
+ return fileName;
+ }
+
+ public long getFileSize() {
+ return fileSize;
+ }
+
+ public Date getLastModified() {
+ return lastModified;
+ }
+
+ public String getMimeType() {
+ return mimeType;
+ }
+
+ @Override
+ public boolean equals(Object o) {
+ if (this == o) return true;
+ if (o == null || getClass() != o.getClass()) return false;
+ FileDescription that = (FileDescription) o;
+ return fileName.equals(that.fileName);
+ }
+
+ @Override
+ public int hashCode() {
+ return Objects.hash(fileName);
+ }
+ }
+
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ List<FileDescription> fileDescriptions = new ArrayList<>();
+ IRequestParameters parameters = request.getRequestParameters();
+ String[] fileNames = parse(parameters.getParameterValue("fileName"));
+ String[] fileSizes = parse(parameters.getParameterValue("fileSize"));
+ String[] lastModifiedDates = parse(parameters.getParameterValue("lastModified"));
+ String[] mimeTypes = parse(parameters.getParameterValue("mimeType"));
+ for (int i = 0; i < fileNames.length; i++) {
+ FileDescription fileDescription = new FileDescription(
+ fileNames[i],
+ Long.parseLong(fileSizes[i]),
+ Long.parseLong(lastModifiedDates[i]),
+ mimeTypes[i]);
+ fileDescriptions.add(fileDescription);
+ }
+ onFileSelected(target, fileDescriptions);
+ }
+
+ private String[] parse(StringValue stringValue) {
+ return stringValue.toString().split(",");
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileDescriptions A list of FileDescription
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, List<FileDescription> fileDescriptions);
+
+ @Override
+ protected void updateAjaxAttributes(AjaxRequestAttributes attributes) {
+ super.updateAjaxAttributes(attributes);
+ attributes.getAjaxCallListeners().add(new IAjaxCallListener()
+ {
+ @Override
+ public CharSequence getPrecondition(Component component)
+ {
+ return "if (this.files) { window." + component.getMarkupId() + "_files = this.files; return true; } " +
+ "else return false;";
+ }
+ });
+
+ String varName = "window."+getComponent().getMarkupId()+"_files";
+ attributes.getDynamicExtraParameters().add("var files = {};" +
+ "var fileNames = [];" +
+ "var fileSizes = [];" +
+ "var lastModifiedDates = [];" +
+ "var mimeTypes = [];" +
+ "for (var i = 0; i < "+varName+".length; i++) {" +
+ " f = " + varName + "[i];" +
+ "fileNames.push(f.name);" +
+ "fileSizes.push(f.size);" +
+ "lastModifiedDates.push(f.lastModified);" +
+ "mimeTypes.push(f.type);" +
+ "};" +
+ "var info = {" +
+ "'fileName': fileNames, " +
+ "'fileSize': fileSizes, " +
+ "'lastModified': lastModifiedDates, " +
+ "'mimeType': mimeTypes, " +
+ "};"
+ + "console.log(info);"
Review comment:
Debug leftover
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r609600306
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +57,74 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ IRequestParameters parameters = request.getRequestParameters();
+ String fileName = parameters.getParameterValue("fileName").toString();
+ long fileSize = parameters.getParameterValue("fileSize").toLong(0);
+ Date lastModified = new Date(parameters.getParameterValue("lastModified").toLong(0));
+ String mimeType = parameters.getParameterValue("mimeType").toString();
+ onFileSelected(target, fileName, fileSize, lastModified, mimeType);
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileName The client file name
+ * @param fileSize The client file size
+ * @param lastModified The {@link java.util.Date} when file was last modified.
+ * @param mimeType The MIME type of file.
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, String fileName, long fileSize,
Review comment:
Oh. I was thinking to roll a different behavior. But you are probably right. I will add support for multiple values ASAP
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] martin-g commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
martin-g commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817529651
> **Note:** A just thought that a possible addition in case of multiple file uploads is a component with a table displaying all the selected files. WDYT?
I don't think we should add such component. Every application may want to display it in a different way.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on pull request #467:
URL: https://github.com/apache/wicket/pull/467#issuecomment-817106343
once reviewed and approved I will rebase on top of master and squash commits.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [wicket] reiern70 commented on a change in pull request #467: [WICKET-6876] add an AJAX behavior to transfer client side file infor…
Posted by GitBox <gi...@apache.org>.
reiern70 commented on a change in pull request #467:
URL: https://github.com/apache/wicket/pull/467#discussion_r609988047
##########
File path: wicket-core/src/main/java/org/apache/wicket/markup/html/form/upload/FileUploadField.java
##########
@@ -47,6 +57,74 @@
{
private static final long serialVersionUID = 1L;
+ /**
+ * {@link org.apache.wicket.ajax.form.OnChangeAjaxBehavior} that streams back to server properties
+ * of the selected file (at client side), even when file has not yet being uploaded.
+s *
+ * @author Ernesto Reinaldo Barreiro (reiern70@gmail.com).
+ */
+ public static abstract class OnFileSelectedBehavior extends OnChangeAjaxBehavior
+ {
+ private static final long serialVersionUID = 1L;
+
+ @Override
+ protected void onBind() {
+ super.onBind();
+ Component component = getComponent();
+ if (!(component instanceof FileUploadField))
+ {
+ throw new WicketRuntimeException("Behavior " + getClass().getName()
+ + " can only be added to an instance of a FileUploadField");
+ }
+ }
+
+ @Override
+ protected void onUpdate(AjaxRequestTarget target)
+ {
+ Request request = RequestCycle.get().getRequest();
+ IRequestParameters parameters = request.getRequestParameters();
+ String fileName = parameters.getParameterValue("fileName").toString();
+ long fileSize = parameters.getParameterValue("fileSize").toLong(0);
+ Date lastModified = new Date(parameters.getParameterValue("lastModified").toLong(0));
+ String mimeType = parameters.getParameterValue("mimeType").toString();
+ onFileSelected(target, fileName, fileSize, lastModified, mimeType);
+ }
+
+ /**
+ * Called when a file, at client side is selected.
+ *
+ * @param target The {@link org.apache.wicket.ajax.AjaxRequestTarget}
+ * @param fileName The client file name
+ * @param fileSize The client file size
+ * @param lastModified The {@link java.util.Date} when file was last modified.
+ * @param mimeType The MIME type of file.
+ */
+ protected abstract void onFileSelected(AjaxRequestTarget target, String fileName, long fileSize,
Review comment:
My latest commit adds support for multiple files. I have tested it locally and it seems to work (with multiple files). Not sure if the way I did it is the best/correct one.
--
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org