You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@nuttx.apache.org by GitBox <gi...@apache.org> on 2020/02/13 18:41:16 UTC
[GitHub] [incubator-nuttx] xiaoxiang781216 opened a new pull request #274:
Improve sim timer
xiaoxiang781216 opened a new pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274
The change include:
1.Implment tickless mode with common code in drivers/timer
2.Sleep with the absolute time to avoid the time drift
3.Add RTC driver to make the complete date/time simulation
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on a change in pull request
#274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379905384
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
Also, doesn't it require administrative privileges to set the host system time? When running with TAPDEV, that will probably always be true, but it is not generally true.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on a change in pull request
#274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379910545
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
I think you could also reimplement this change to avoid using clock_settime() altogether:
1. Add a time global g_clock_offset initialized to zero.
2. Add g_clock_offset to the value returned by host_gettime()
3. In host_settime(), don't actually set the time. Just update the offset based on the before and after setting time.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379289488
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
isn't host clock_settime failing for some reasons?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo merged pull request #274: Improve sim
timer
Posted by GitBox <gi...@apache.org>.
patacongo merged pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586551386
> Is this PR ready to be merged? I wait to merge PRs if there is open discussion.
Yes, I think that I have addressed @yamt 's concern.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379912272
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
@yamt @patacongo this patch should resolve the problem:
https://github.com/apache/incubator-nuttx/pull/288
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo edited a comment on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
patacongo edited a comment on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586723811
Yes, many parts of the simulator were designed assuming that there is no preemption. So in SMP mode (or if we were to implement preemptive behavior). It will uncover some of the shortcuts in the simulator that assume non-preemptive behavior.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379446718
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
If so, we just need to keep the sync option on, then the change just happened inside simulator process, other process don't see the real change.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586723584
> @xiaoxiang781216 "...timing in PC is still very different from the real hardware, so most people don't consider to measure the realtime or performance on simulator..."
>
> True, but real-time pre-emptyive beahvor is good for testing realtime concurrent behaviors, race conditions, etc. it is good for testing, but not performance measurement. It provides a higher level of fidelity in the simulation.
Yes, enable SMP can provide some real-time pre-emptive behavior. Actually, I found some concurrent issue in handle duplication I never hit before with a single CPU.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo edited a comment on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
patacongo edited a comment on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586588624
"In most case, the interrupt simulate through idle thread is same as through pthread/signal." Except with simulated asynchronous timer and interrupts we can have true pre-emptive behavior in the simulation. It is only useful for non-realtime testing now.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379331923
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
No, clock_settime return 0. After more testing, once the host disable internet time sync:
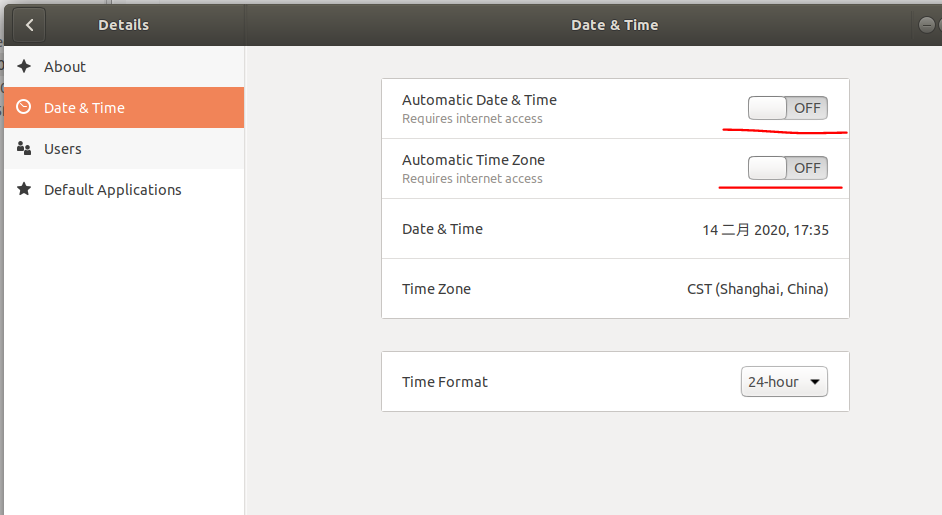
Simulator can change the host clock now.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379833656
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
while adjustnig time with ntp server is common,
* it usually does not prevent clock_settime
* it isn't always possible. eg. offline machines
i don't understand your use case to modify host os's clock. can you explain a bit?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379449390
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
i'm not sure what "the sync option" is. but i guess it isn't available for every host environments, isn't it?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379910187
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
> i'm fine to work on a patch by myself.
> but i want to understand why @xiaoxiang781216 wants this behavior.
Actually, I don't care whether clock_settime change the host wall clock, because I run Ubuntu in VM:). And then I select a simplest method(clock_settime) to implment rtc_ops, another approach is saving the changed value locally and adjust the return value from rdtime.
I will provide a patch to resolve your concern.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on issue #274: Improve sim
timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586588624
"In most case, the interrupt simulate through idle thread is same as through pthread/signal." Except with simulated asyncrhonous timer and interrupts we can have true pre-emptive behavior in the simulation.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on issue #274: Improve sim
timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586723811
Yes, many parts of the simulator were designed assume that there is no preemption. So in SMP more (or if we were to implement preemptive behavior). It will uncover some of the shortcuts in the simulator that assume non-preemptive behavior.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379840491
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
Here is the several reason:
1.rtc_ops_s define two callbacks(rdtime/settime), it's natual to map both call to host side(clock_getime/clock_settime with CLOCK_REALTIME) to make the sim RTC driver complete.
2.It's very useful to test NuttX NTP client logic in simulated environment.
3.My test show that simulator is hard to make the real modification to host wallclock.
4.RTC driver implment settime, but the modification is always activated by the user(date -s or CONFIG_NTP_xxx).
The side effect is totally controlled by user, and the user need take expicit action to make the change happen.
So, I don't think there is any reason why we skip settime to redue the simulator capability.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586552455
> Some time back, i attempted to implement a timer for the simulation using a host timer on a timer pthread . I ran into the same problems as I did when I tried to implement simulated interrupts: https://cwiki.apache.org/confluence/display/NUTTX/NuttX+Simulation
>
This patch has a smaller scope: utilize the host timer to implement RTC get/set without arlarm support. So the pthread sync or signal handler isn't an issue here.
> Basically, you cannot do a NuttX context switch from the context of a host signal handler or from host pthreads. I still have the host-based timer implementation and can send a tarball to anyone who is interested. It is from 2014 and so would need some minor environmental updates.
In most case, the interrupt simulate through idle thread is same as through pthread/signal. Only two behaviour difference from my daily use:
1.The bad busy loop block all work in idle thread and then the simulator stop response
2.Simulated interrupt always happen inside idle thread which make the cpu loading generate the wrong report
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379908746
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
i'm fine to work on a patch by myself.
but i want to understand why @xiaoxiang781216 wants this behavior.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on issue #274: Improve sim
timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586396148
Is this PR ready to be merged? I wait to merge PRs if there is open discussion.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on issue #274: Improve sim
timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586394703
Some time back, i attempted to implement a timer for the simulation using a host timer on a timer pthread . I ran into the same problems as I did when I tried to implement simulated interrupts: https://cwiki.apache.org/confluence/display/NUTTX/NuttX+Simulation
Basically, you cannot do a NuttX context switch from the context of a host signal handler or from host pthreads. I still have the host-based timer implementation and can send a tarball to anyone who is interested. It is from 2014 and so would need some minor environmental updates.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379265841
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
NuttShell (NSH) NuttX-8.2
nsh> date
Fri, Feb 14 06:05:26 2020
nsh> date -s "Feb 1 00:00:00 2020"
nsh> date
Sat, Feb 01 00:00:02 2020
nsh> date
Sat, Feb 01 00:00:14 2020
nsh> poweroff
xiaoxiang@xiaoxiang-VirtualBox:~/mirtos$ date
2020年 02月 14日 星期五 14:06:12 CST
From the above test, nuttx get eh changed value, but host doesn't change.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379905077
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
i don't understand why you need to adjust host os time to test nuttx ntp client logic.
i'm not sure about your host os. but as far as i know it's common for OSes not to prevent clock_settime from adjusting time even when network time sync stuff is enabled. at least macOS is in this category.
IMO it's enough reason not to make the sim call clock_settime.
wrt user experience, I, as a user, would be very surprised if modifying nuttx time in the sim modifies host OS time. i might be a minority though.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379482266
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
"The sync option" allow OS auto adjust date/time from NTP server, so it should exist on all modem OS and enabled by default. From my test, the change just happen inside simulator once this option turn on(out of box state), which is the behaviour what most people expect.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586721140
> "In most case, the interrupt simulate through idle thread is same as through pthread/signal." Except with simulated asynchronous timer and interrupts we can have true pre-emptive behavior in the simulation. It is only useful for non-realtime testing now.
But, even we use pthread/signal to simulate the interrupt, the timing in PC is still very different from the real hardware, so most people don't consider to measure the realtime or performance on simulator.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo edited a comment on issue #274: Improve
sim timer
Posted by GitBox <gi...@apache.org>.
patacongo edited a comment on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586721998
@xiaoxiang781216 "...timing in PC is still very different from the real hardware, so most people don't consider to measure the realtime or performance on simulator..."
True, but real-time pre-emptyive beahvor is good for testing realtime concurrent behaviors, race conditions, etc. it is good for testing, but not performance measurement. It provides a higher level of fidelity in the simulation.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on a change in pull request
#274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379905297
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
@yamt "I, as a user, would be very surprised if modifying nuttx time in the sim modifies host OS time."
I think most people would be surprised at that. I think this is a solution specific to Xiang's applications. This PR has been merged.
Why don't you consider submitting a new PR to make this behavior configurable? If you want the behavior, then having to set a configuration operation would eliminate any surprises.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379064231
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
does this mean the sim can modify the clock of the host os?
it's a surprising behavior for me.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] yamt commented on a change in pull request #274:
Improve sim timer
Posted by GitBox <gi...@apache.org>.
yamt commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379333291
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
i think it's better to keep a diff from host os clock, rather than changing the host clock. how do you think?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] xiaoxiang781216 commented on a change in pull
request #274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379910942
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
Yes, this is what I will provide in the couple minutes.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on issue #274: Improve sim
timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on issue #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#issuecomment-586721998
@xiaoxiang781216 "...timing in PC is still very different from the real hardware, so most people don't consider to measure the realtime or performance on simulator..."
True, but it is good for testing realtime concurrent behaviors, race conditions, etc. it is good for testing, but not performance measurement.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services
[GitHub] [incubator-nuttx] patacongo commented on a change in pull request
#274: Improve sim timer
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #274: Improve sim timer
URL: https://github.com/apache/incubator-nuttx/pull/274#discussion_r379909062
##########
File path: arch/sim/src/sim/up_rtc.c
##########
@@ -0,0 +1,127 @@
+/****************************************************************************
+ * arch/sim/src/sim/up_rtc.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <nuttx/arch.h>
+#include <nuttx/timers/rtc.h>
+#include <nuttx/timers/arch_rtc.h>
+
+#include "up_internal.h"
+
+/****************************************************************************
+ * Private Function Prototypes
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime);
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime);
+static bool sim_rtc_havesettime(FAR struct rtc_lowerhalf_s *lower);
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+static const struct rtc_ops_s g_sim_rtc_ops =
+{
+ .rdtime = sim_rtc_rdtime,
+ .settime = sim_rtc_settime,
+ .havesettime = sim_rtc_havesettime,
+};
+
+static struct rtc_lowerhalf_s g_sim_rtc =
+{
+ .ops = &g_sim_rtc_ops,
+};
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+static int sim_rtc_rdtime(FAR struct rtc_lowerhalf_s *lower,
+ FAR struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+ time_t sec;
+
+
+ nsec = host_gettime(true);
+ sec = nsec / NSEC_PER_SEC;
+ nsec -= sec * NSEC_PER_SEC;
+
+ gmtime_r(&sec, (FAR struct tm *)rtctime);
+ rtctime->tm_nsec = nsec;
+
+ return OK;
+}
+
+static int sim_rtc_settime(FAR struct rtc_lowerhalf_s *lower,
+ FAR const struct rtc_time *rtctime)
+{
+ uint64_t nsec;
+
+ nsec = mktime((FAR struct tm *)rtctime);
+ nsec *= NSEC_PER_SEC;
+ nsec += rtctime->tm_nsec;
+ host_settime(true, nsec);
Review comment:
We will have to wait for @xiaoxiang781216 reponse to know. But I imagine it is because he also used host_gettime(), for example in sim_rtc_rdtime().
I suppose that if you wanted to make setting the host system time automatically, you would also want to avoid useing the host system time as well.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
With regards,
Apache Git Services