You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@nuttx.apache.org by GitBox <gi...@apache.org> on 2020/10/03 22:36:07 UTC
[GitHub] [incubator-nuttx-apps] patacongo opened a new pull request #417: apps/fsutils/ipcfg: Add support for IPv6
patacongo opened a new pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417
## Summary
The IP Configuration File access library at apps/fsutils/ipcfg was originally merged with IPv4 support only. Only hooks were provided for IPv6. This PR adds that missing IPv6 support.
## Impact
This could potentially effect apps/netutils/netinit which is the only client of apps/fsutils/ipcfg.
## Testing
The changes were verified using apps/examples/ipcfg with the base configuration stm32f4discovery:netnsh
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499249885
##########
File path: fsutils/ipcfg/ipcfg_text.c
##########
@@ -0,0 +1,810 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_text.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <ctype.h>
+
+#include <arpa/inet.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifndef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static const char *g_ipv4proto_name[] =
+{
+ "none", /* IPv4PROTO_NONE */
+ "static", /* IPv4PROTO_STATIC */
+ "dhcp", /* IPv4PROTO_DHCP */
+ "fallback" /* IPv4PROTO_FALLBACK */
+};
+#endif
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static const char *g_ipv6proto_name[] =
+{
+ "none", /* IPv6PROTO_NONE */
+ "static", /* IPv6PROTO_STATIC */
+ "dhcp", /* IPv6PROTO_AUTOCONF */
+ "fallback" /* IPv4PROTO_FALLBACK */
+};
+#endif
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_trim
+ *
+ * Description:
+ * Skip over any whitespace.
+ *
+ * Input Parameters:
+ * line - Pointer to line buffer
+ * index - Current index into the line buffer
+ *
+ * Returned Value:
+ * New value of index.
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_IPCFG_BINARY
+static int ipcfg_trim(FAR char *line, int index)
+{
+ int ret;
+ while (line[index] != '\0' && isspace(line[index]))
+ {
+ index++;
+ }
+
+ ret = index;
+ while (line[index] != '\0')
+ {
+ if (!isprint(line[index]))
+ {
+ line[index] = '\0';
+ break;
+ }
+
+ index++;
+ }
+
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_put_ipv4addr
+ *
+ * Description:
+ * Write a <variable>=<address> value pair to the stream.
+ *
+ * Input Parameters:
+ * stream - The output stream
+ * variable - The variable namespace
+ * address - The IP address to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static int ipcfg_put_ipv4addr(FAR FILE *stream, FAR const char *variable,
+ in_addr_t address)
+{
+ if (address != 0)
+ {
+ struct in_addr saddr =
+ {
+ address
+ };
+
+ char converted[INET_ADDRSTRLEN];
+
+ /* Convert the address to ASCII text */
+
+ if (inet_ntop(AF_INET, &saddr, converted, INET_ADDRSTRLEN) == NULL)
+ {
+ int ret = -errno;
+ fprintf(stderr, "ERROR: inet_ntop() failed: %d\n", ret);
+ return ret;
+ }
+
+ fprintf(stream, "%s=%s\n", variable, converted);
+ }
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_check_ipv6addr
+ *
+ * Description:
+ * Check for a valid IPv6 address, i.e., not all zeroes.
+ *
+ * Input Parameters:
+ * address - A pointer to the address to check.
+ *
+ * Returned value:
+ * Zero (OK) is returned if the address is non-zero. -ENXIO is returned if
+ * the address is zero.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv6
+static int ipcfg_check_ipv6addr(FAR const struct in6_addr *address)
+{
+ int i;
+
+ for (i = 0; i < 4; i++)
+ {
+ if (address->s6_addr32[i] != 0)
+ {
+ return OK;
+ }
+ }
+
+ return -ENXIO;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_put_ipv6addr
+ *
+ * Description:
+ * Write a <variable>=<address> value pair to the stream.
+ *
+ * Input Parameters:
+ * stream - The output stream
+ * variable - The variable namespace
+ * address - The IP address to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static int ipcfg_put_ipv6addr(FAR FILE *stream, FAR const char *variable,
+ FAR const struct in6_addr *address)
+{
+ /* If the address is all zero, then omit it */
+
+ if (ipcfg_check_ipv6addr(address) == OK)
+ {
+ char converted[INET6_ADDRSTRLEN];
+
+ /* Convert the address to ASCII text */
+
+ if (inet_ntop(AF_INET6, address, converted, INET6_ADDRSTRLEN) == NULL)
+ {
+ int ret = -errno;
+ fprintf(stderr, "ERROR: inet_ntop() failed: %d\n", ret);
+ return ret;
+ }
+
+ fprintf(stream, "%s=%s\n", variable, converted);
+ }
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * stream - Stream of the open file to write to
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static int ipcfg_write_ipv4(FAR FILE *stream,
+ FAR const struct ipv4cfg_s *ipv4cfg)
+{
+ /* Format and write the file */
+
+ if ((unsigned)ipv4cfg->proto > MAX_IPv4PROTO)
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv4PROTO value: %d\n",
+ ipv4cfg->proto);
+ return -EINVAL;
+ }
+
+ fprintf(stream, "IPv4PROTO=%s\n", g_ipv4proto_name[ipv4cfg->proto]);
+
+ ipcfg_put_ipv4addr(stream, "IPv4IPADDR", ipv4cfg->ipaddr);
+ ipcfg_put_ipv4addr(stream, "IPv4NETMASK", ipv4cfg->netmask);
+ ipcfg_put_ipv4addr(stream, "IPv4ROUTER", ipv4cfg->router);
+ ipcfg_put_ipv4addr(stream, "IPv4DNS", ipv4cfg->dnsaddr);
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * stream - Stream of the open file to write to
+ * ipv6cfg - The IPv6 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static int ipcfg_write_ipv6(FAR FILE *stream,
+ FAR const struct ipv6cfg_s *ipv6cfg)
+{
+ /* Format and write the file */
+
+ if ((unsigned)ipv6cfg->proto > MAX_IPv6PROTO)
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv6PROTO value: %d\n",
+ ipv6cfg->proto);
+ return -EINVAL;
+ }
+
+ fprintf(stream, "IPv6PROTO=%s\n", g_ipv6proto_name[ipv6cfg->proto]);
+
+ ipcfg_put_ipv6addr(stream, "IPv6IPADDR", &ipv6cfg->ipaddr);
+ ipcfg_put_ipv6addr(stream, "IPv6NETMASK", &ipv6cfg->netmask);
+ ipcfg_put_ipv6addr(stream, "IPv6ROUTER", &ipv6cfg->router);
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv4cfg_s *ipv4cfg)
+{
+ char line[MAX_LINESIZE];
+ FAR FILE *stream;
+ int index;
+ int ret = -ENOENT;
+
+ DEBUGASSERT(stream != NULL && ipv4cfg != NULL);
+
+ /* Open the file for reading */
+
+ stream = fopen(path, "r");
+ if (stream == NULL)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+ /* Process each line in the file */
+
+ memset(ipv4cfg, 0, sizeof(FAR struct ipv4cfg_s));
+
+ while (fgets(line, MAX_LINESIZE, stream) != NULL)
+ {
+ /* Skip any leading whitespace */
+
+ index = ipcfg_trim(line, 0);
+
+ /* Check for a blank line or a comment */
+
+ if (line[index] != '\0' && line[index] != '#')
+ {
+ FAR char *variable = &line[index];
+ FAR char *value;
+
+ /* Expect <variable>=<value> pair */
+
+ value = strchr(variable, '=');
+ if (value == NULL)
+ {
+ fprintf(stderr, "ERROR: Skipping malformed line in file: %s\n",
+ line);
+ continue;
+ }
+
+ /* NUL-terminate the variable string */
+
+ *value++ = '\0';
+
+ /* Process the variable assignment */
+
+ if (strcmp(variable, "DEVICE") == 0)
+ {
+ /* Just assure that it matches the filename */
+
+ if (strcmp(value, netdev) != 0)
+ {
+ fprintf(stderr, "ERROR: Bad device in file: %s=%s\n",
+ variable, value);
+ }
+ }
+ else if (strcmp(variable, "IPv4PROTO") == 0)
+ {
+ if (strcmp(value, "none") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_NONE;
+ }
+ else if (strcmp(value, "static") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_STATIC;
+ }
+ else if (strcmp(value, "dhcp") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_DHCP;
+ }
+ else if (strcmp(value, "fallback") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_FALLBACK;
+ }
+ else
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv4PROTO: %s=%s\n",
+ variable, value);
+ }
+
+ /* Assume IPv4 settings are present if the IPv4BOOTPROTO
+ * setting is encountered.
+ */
+
+ ret = OK;
+ }
+ else if (strcmp(variable, "IPv4IPADDR") == 0)
+ {
+ ipv4cfg->ipaddr = inet_addr(value);
+ }
+ else if (strcmp(variable, "IPv4NETMASK") == 0)
+ {
+ ipv4cfg->netmask = inet_addr(value);
+ }
+ else if (strcmp(variable, "IPv4ROUTER") == 0)
+ {
+ ipv4cfg->router = inet_addr(value);
+ }
+ else if (strcmp(variable, "IPv4DNS") == 0)
+ {
+ ipv4cfg->dnsaddr = inet_addr(value);
+ }
+
+ /* Anything other than some IPv6 settings would be an error.
+ * This is a sloppy check because it does not detect invalid
+ * names variables that begin with "IPv6".
+ */
+
+ else if (strncmp(variable, "IPv6", 4) != 0)
+ {
+ fprintf(stderr, "ERROR: Unrecognized variable: %s=%s\n",
+ variable, value);
+ }
+ }
+ }
+
+ /* Close the file and return */
+
+ fclose(stream);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv6
+ *
+ * Description:
+ * Read IPv6 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv6cfg - Location to read IPv6 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv6
+int ipcfg_read_text_ipv6(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv6cfg_s *ipv6cfg)
+{
+ char line[MAX_LINESIZE];
+ FAR FILE *stream;
+ bool found = false;
+ int index;
+ int ret;
+
+ DEBUGASSERT(path != NULL && netdev != NULL && ipv6cfg != NULL);
+
+ /* Open the file for reading */
+
+ stream = fopen(path, "r");
+ if (stream == NULL)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+ /* Process each line in the file */
+
+ memset(ipv6cfg, 0, sizeof(FAR struct ipv6cfg_s));
+
+ while (fgets(line, MAX_LINESIZE, stream) != NULL)
+ {
+ /* Skip any leading whitespace */
+
+ index = ipcfg_trim(line, 0);
+
+ /* Check for a blank line or a comment */
+
+ if (line[index] != '\0' && line[index] != '#')
+ {
+ FAR char *variable = &line[index];
+ FAR char *value;
+
+ /* Expect <variable>=<value> pair */
+
+ value = strchr(variable, '=');
+ if (value == NULL)
+ {
+ fprintf(stderr, "ERROR: Skipping malformed line in file: %s\n",
+ line);
+ continue;
+ }
+
+ /* NUL-terminate the variable string */
+
+ *value++ = '\0';
+
+ /* Process the variable assignment */
+
+ if (strcmp(variable, "DEVICE") == 0)
+ {
+ /* Just assure that it matches the filename */
+
+ if (strcmp(value, netdev) != 0)
+ {
+ fprintf(stderr, "ERROR: Bad device in file: %s=%s\n",
+ variable, value);
+ }
+ }
+ else if (strcmp(variable, "IPv6PROTO") == 0)
+ {
+ if (strcmp(value, "none") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_NONE;
+ }
+ else if (strcmp(value, "static") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_STATIC;
+ }
+ else if (strcmp(value, "autoconf") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_AUTOCONF;
+ }
+ else if (strcmp(value, "fallback") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_FALLBACK;
+ }
+ else
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv6PROTO: %s=%s\n",
+ variable, value);
+ }
+
+ /* Assume IPv4 settings are present if the IPv6BOOTPROTO
+ * setting is encountered.
+ */
+
+ found = true;
+ }
+ else if (strcmp(variable, "IPv6IPADDR") == 0)
+ {
+ ret = inet_pton(AF_INET6, value, &ipv6cfg->ipaddr);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: inet_pton() failed: %d\n", ret);
+ return ret;
+ }
+ }
+ else if (strcmp(variable, "IPv6NETMASK") == 0)
+ {
+ ret = inet_pton(AF_INET6, value, &ipv6cfg->netmask);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: inet_pton() failed: %d\n", ret);
+ return ret;
+ }
+ }
+ else if (strcmp(variable, "IPv6ROUTER") == 0)
+ {
+ ret = inet_pton(AF_INET6, value, &ipv6cfg->router);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: inet_pton() failed: %d\n", ret);
+ return ret;
+ }
+ }
+
+ /* Anything other than some IPv4 settings would be an error.
+ * This is a sloppy check because it does not detect invalid
+ * names variables that begin with "IPv4".
+ */
+
+ else if (strncmp(variable, "IPv4", 4) != 0)
+ {
+ fprintf(stderr, "ERROR: Unrecognized variable: %s=%s\n",
+ variable, value);
+ }
+ }
+ }
+
+ /* Close the file and return */
+
+ fclose(stream);
+ return found ? OK : -ENOENT;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+int ipcfg_write_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR const struct ipv4cfg_s *ipv4cfg)
+{
+#ifdef CONFIG_NET_IPv6
+ struct ipv6cfg_s ipv6cfg;
+ bool ipv6 = false;
+#endif
+ FAR FILE *stream;
+ int ret;
+
+ DEBUGASSERT(stream != NULL && ipv4cfg != NULL);
+
+#ifdef CONFIG_NET_IPv6
+ /* Read any IPv6 data in the file */
+
+ ret = ipcfg_read_text_ipv6(path, netdev, &ipv6cfg);
+ if (ret < 0)
+ {
+ /* -ENOENT is not an error. It simply means that there is no IPv6
+ * configuration in the file.
+ */
+
+ if (ret != -ENOENT)
+ {
+ return ret;
+ }
+ }
+ else
+ {
+ ipv6 = true;
+ }
+#endif
+
+ /* Open the file for writing (truncates) */
+
+ stream = fopen(path, "w");
+ if (stream == NULL)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ return ret;
+ }
+
+ /* Save the device name */
+
+ fprintf(stream, "DEVICE=%s\n", netdev);
+
+ /* Write the IPv4 configuration */
+
+ ret = ipcfg_write_ipv4(stream, ipv4cfg);
+ if (ret < 0)
+ {
+ return ret;
+ }
+
+#ifdef CONFIG_NET_IPv6
+ /* Followed by any IPv6 data in the file */
+
+ if (ipv6)
+ {
+ ret = ipcfg_write_ipv6(stream, &ipv6cfg);
+ }
+#endif
+
+ fclose(stream);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a binary IP Configuration file.
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] davids5 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
davids5 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703575198
> > @xiaoxiang781216 @davids5 There is no impact to the release from this PR (because there is still no user of ipcfg in master) so this could be merged whenever you are comfortable. I have tested pretty thoroughly and I am confident in the state of the changes. I think David has become exasperated since he has closed PR #415 . After this is merged, I will submit a PR to get the IPv4 and IPv6 configuration from the configuration file. I will not implement David's full polling logic from PR #415 , only the logic that gets the IP addresses which I think is non-controversial. It would be nice if you two could resolve your differences and we could make forward progress on that.
@xiaoxiang781216 Per @patacongo request:
I do not "reject other people's comments." I do look for them to acknowledged the points and concern I have and respond to it. This does not happen with you and I. (I truly believe it is miscommunication) I take more responsibility in that failing as I can not speak Chinese. I wish I had the capability to speak Chinese as well as you speak English so that I could ensure you are acknowledging my points and concerns and have a counter argument that nullifies them. Our common languages is "C" this is why I ask you to do a PR.
>
> Sure, let's go ahead. Actually, all my suggestions are very simple:
>
> 1. Make NETINIT_ESTABLISH_POLL_RATE/NETINIT_LOSS_POLL_RATE available in both interrupt/poll case
Prior to my PR HW that could support network monitoring enabled the whole network monitor via ARCH_PHY_INTERRUPT.
This was safe. The state (edges) of the network were provided to the monitor ONLY on transitions.
On the UP edge a **DELAY** was used to the bring up networking. On a DOWN edge no delay was used to bring down the network it was the checked at **CONFIG_NETINIT_RETRYMSEC** . Once the network was up the PHY was ONLY queried ASYNCHRONOUSLY every 3600.
When I asked if it supported polled mode. Greg responded with no. AND a concern that HW may not support link management while active:
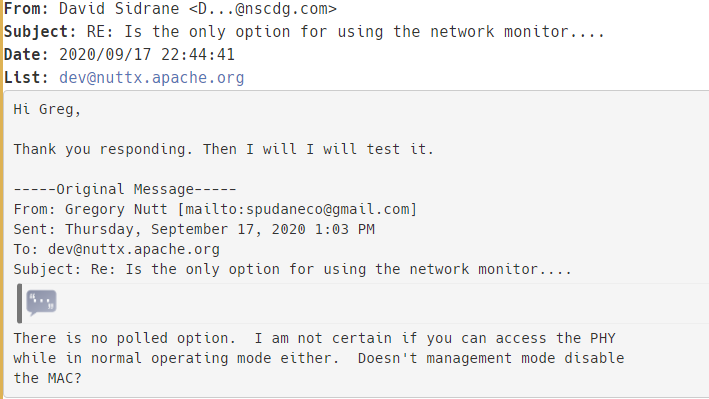
My first test was to do poling in the F7 driver using a LAN8742AI-CZ-TR and simulate the interrupts.
1) I PROVED that the specific combination of the STM32F7' MAC and LAN8742AI PHY do not disabled the MAC during management.
2) The polling logic in the driver would be a waste of resources to simulate the interrupt as it would be a duplicate of what the the client task could be modified to do polling with **only a semantic** change..
Since other SOC / PHY may have the limitation. I did not want to cause some poor user to have that heartache. It would waste their time and reflect badly on NuttX,
My reason for not accepting your change is the your change can case **user specified** _periodic_ networking issues with HW that disable the MAC in management mode. I can not imagine how hard that will be to trouble shoot. I feel strongly about this, I would not expose a user to that. I would not want it done to me.
Further more the word polled and interrupt to the 180% opposite. The time components in the monitor are DELAYS from edges, not POLLING rates. I introduced that notion by setting a set of DELAYS = to a set of POLLING RATES based on CONFIG_ARCH_PHY_POLLED.
> 2. Make NETINIT_DHCP_FALLBACK available in both interrupt/poll case
This may work. But is will be only based on the CONFIG_NETINIT_RETRYMSEC, not the polling rates.
It needs to be tested on HW that can support it.
> 3. Initialize g_netconf with the default if ipcfg_read return fail to avoid the code duplication
I like the idea of saving space and reducing complexity, My attempt at it gave me concerns that the combination of the CONFIG_* permutations with the run time choices made it very hard to follow and test. You may have better results with it. This is why I asked for a PR from you if you wanted the change in.
> The first two only involve the minor change in Kconfig, the 3rd one just need reorder the initialition code.
I did run out of time on this. I have products that needed all the functionality that I added. The concerns of binary compatibility will be an issue based on the host/network ordering.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499249762
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
+};
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv4)
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703259126
> Actually, all my suggestions are very simple:
>
> 1. Make NETINIT_ESTABLISH_POLL_RATE/NETINIT_LOSS_POLL_RATE available in both interrupt/poll case
>
> 2. Make NETINIT_DHCP_FALLBACK available in both interrupt/poll case
>
> 3. Initialize g_netconf with the default if ipcfg_read return fail to avoid the code duplication
>
>
> The first two only involve the minor change in Kconfig, the 3rd one just need reorder the initialition code.
Unfortunately, this will not happen. David and closed #415 and you can read his comments here: PX4/NuttX-apps#7
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] davids5 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
davids5 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-704259797
> > @patacongo based on the last comment above, can you please use the extra 16 bit in the header as a version number?
>
> No, but you are welcome to submit a PR.
>
> I will be integrating IPv6 support into netinit today. This may conflict with your plans, but I hope not.
PR is here. https://github.com/apache/incubator-nuttx-apps/pull/419
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499249996
##########
File path: fsutils/ipcfg/ipcfg_binary.c
##########
@@ -0,0 +1,567 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_binary.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <fcntl.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifdef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_open (for binary mode)
+ *
+ * Description:
+ * Form the complete path to the ipcfg file and open it.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * oflags - File open flags
+ * mode - File creation mode
+ *
+ * Returned Value:
+ * The open file descriptor is returned on success; a negated errno value
+ * is returned on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_BINARY
+static int ipcfg_open(FAR const char *path, int oflags, mode_t mode)
+{
+ int fd;
+ int ret;
+
+ /* Now open the file */
+
+ fd = open(path, oflags, mode);
+ if (fd < 0)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+#if defined(CONFIG_IPCFG_OFFSET) && CONFIG_IPCFG_OFFSET > 0
+ /* If the binary file is accessed on a character device as a binary
+ * file, then there is also an option to seek to a location on the
+ * media before reading or writing the file.
+ */
+
+ ret = lseek(fd, CONFIG_IPCFG_OFFSET, SEEK_SET);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to seek to $ld: %d\n",
+ (long)CONFIG_IPCFG_OFFSET, ret);
+
+ close(fd);
+ return ret;
+ }
+
+#endif
+
+ return fd;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary
+ *
+ * Description:
+ * Read from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * buffer - Location to read from
+ * nbytes - Number of bytes to read
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_read_binary(int fd, FAR void *buffer, size_t nbytes)
+{
+ ssize_t nread;
+ int ret;
+
+ /* Read from the file */
+
+ nread = read(fd, buffer, nbytes);
+ if (nread < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to read from file: %d\n", ret);
+ }
+ else if (nread != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad read size: %ld\n", (long)nread);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: ipcfg_write_binary
+ *
+ * Description:
+ * Write to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to write to
+ * buffer - Location to write to
+ * nbytes - Number of bytes to wrtie
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_WRITABLE
+static int ipcfg_write_binary(int fd, FAR const void *buffer, size_t nbytes)
+{
+ ssize_t nwritten;
+ int ret;
+
+ /* Read from the file */
+
+ nwritten = write(fd, buffer, nbytes);
+ if (nwritten < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to write to file: %d\n", ret);
+ }
+ else if (nwritten != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad write size: %ld\n", (long)nwritten);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_find_binary
+ *
+ * Description:
+ * Read the location of IPv4 data in a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * af - Identifies the address family whose IP configuration is
+ * requested. May be either AF_INET or AF_INET6.
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_find_binary(int fd, sa_family_t af)
+{
+ struct ipcfg_header_s hdr;
+ off_t pos;
+ int ret;
+
+ for (; ; )
+ {
+ /* Read the header (careful.. could be uninitialized in the case of a
+ * character driver).
+ */
+
+ ret = ipcfg_read_binary(fd, &hdr, sizeof(struct ipcfg_header_s));
+ if (ret < 0)
+ {
+ /* Return on any read error */
+
+ return (int)ret;
+ }
+ else if (hdr.type == af)
+ {
+ return OK;
+ }
+ else if (hdr.type != AF_INET && hdr.type != AF_INET6)
+ {
+ return -EINVAL;
+ }
+ else if (hdr.next == 0)
+ {
+ fprintf(stderr, "ERROR: IP configuration not found\n");
+ return -ENOENT;
+ }
+
+ /* Skip to the next IP configuration record */
+
+ pos = lseek(fd, hdr.next, SEEK_CUR);
+ if (pos < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: lseek failed: %d\n", ret);
+ return ret;
+ }
+ }
+}
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg)
+{
+ int fd;
+ int ret;
+
+ DEBUGASSERT(path != NULL && ipv4cfg != NULL);
+
+ /* Open the file for reading */
+
+ fd = ipcfg_open(path, O_RDONLY, 0666);
+ if (fd < 0)
+ {
+ return fd;
+ }
+
+ /* Find the IPv4 binary in the IP configuration file */
+
+ ret = ipcfg_find_binary(fd, AF_INET);
+ if (ret < 0)
+ {
+ goto errout_with_fd;
+ }
+
+ /* Read the IPv4 Configuration */
+
+ ret = ipcfg_read_binary(fd, ipv4cfg, sizeof(struct ipv4cfg_s));
+
+errout_with_fd:
+ close(fd);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo edited a comment on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo edited a comment on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703282339
@xiaoxiang781216 Thanks for the merge.
I have some concerns about byte ordering.
- apps/fsutils/ipcfg does not care about network vs. host byte ordering. It saves and returns addresses in the same order that they were obtained. Perhaps this should be specified as network order?
- But it does call inet_addr() which expects the string representation in host order, but returns the binary in network order.
- It also calls inet_pton() and inet_ntop(). inet_pton() expects the string representation in host order. The comments say that it returns the binary in network order, but I don't see that. It looks like it returns the data in host order (but I might be wrong; the code all works okay as it).
- inet_ntop() comments say that the input in network byte ordering. That is true, but it seems to be hard coded to work only on a little endian machine for the IPv4 conversion. The IPv6 conversion calls ntohs() for each 16-bit value. I think that is okay.
- apps/examples/provides addresses in network order. In testing, the final addresses are printed in the correct host byte order. So that seems to be fine.
- David's netinit change assumes that the IPv4 values returned by ipcfg are in host order and uses HTONL() to convert the addresses to network order.
This is confusing. I think the byte ordering needs to be clearly stated (network ordering), inet_pton() et al should be verified, and netinit.c should not call HTONL. I will look at this more when I incorporate the ipcfg changes in netinit. Perhaps there is no problem that a few comments cannot fix.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] xiaoxiang781216 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703261648
One more comment: can we replace all printf in fsutils/ipcfg with ferr? It will save a lot of code size.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] xiaoxiang781216 merged pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 merged pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] xiaoxiang781216 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703264587
@patacongo there are some nxstyle warnning need to resolve:
/home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:393:78: error: Long line found
/home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:416:82: error: Long line found
/home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:543:78: error: Long line found
/home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:566:82: error: Long line found
All looks fine, I will merge the change once it pass the precheck.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] xiaoxiang781216 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703289717
> @xiaoxiang781216 Thanks for the merge.
>
> I have some concerns about byte ordering.
>
> * apps/fsutils/ipcfg does not care about network vs. host byte ordering. It saves and returns addresses in the same order that they were obtained. Perhaps this should be specified as network order?
Could be, but it isn't so critifal except the user extract config file to other device. Otherwise, the real requirement is that the load/save use the same order(either little, big or native).
> * But it does call inet_addr() which expects the string representation in host order, but returns the binary in network order.
string don't have the order concept, only the binary bigger than one byte has.
> * It also calls inet_pton() and inet_ntop(). inet_pton() expects the string representation in host order. The comments say that it returns the binary in network order, but I don't see that. It looks like it returns the data in host order (but I might be wrong; the code all works okay as it).
The addres return in network order:
https://github.com/apache/incubator-nuttx/blob/master/libs/libc/net/lib_inetpton.c#L145
https://github.com/apache/incubator-nuttx/blob/master/libs/libc/net/lib_inetpton.c#L270
> * inet_ntop() comments say that the input in network byte ordering. That is true, but it seems to be hard coded to work only on a little endian machine for the IPv4 conversion. The IPv6 conversion calls ntohs() for each 16-bit value. I think that is okay.
IPv4 work for big endian machine too:
https://github.com/apache/incubator-nuttx/blob/master/libs/libc/net/lib_inetntop.c#L112
because the code access the address through char * from low to high.
> * apps/examples/provides addresses in network order. In testing, the final addresses are printed in the correct host byte order. So that seems to be fine.
> * David's netinit change assumes that the IPv4 values returned by ipcfg are in host order and uses HTONL() to convert the addresses to network order.
>
> This is confusing. I think the byte ordering needs to be clearly stated (network ordering), inet_pton() et al should be verified, and netinit.c should not call HTONL. I will look at this more when I incorporate the ipcfg changes in netinit. Perhaps there is no problem that a few comments cannot fix.
Since these routines don't validate the range of input and the conversion happen inside the implementation, the result of load is always good regardless what's order the caller use. But it's always good to appoint the order(network), so the user don't surprise when he/she cat the saved file and it also make the config file truely independent from device.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703179220
@xiaoxiang781216 @davids5 There is no impact to the release from this PR (because there is still no user of ipcfg in master) so this could be merged whenever you are comfortable. I have tested pretty thoroughly and I am confident in the state of the changes. I think David has become exasperated. After this is merged, I will submit a PR to get the IPv4 and IPv6 configuration from the configuration file. I will not implement David's full polling logic, only the logic that gets the IP addresses. It would be nice if you two could resolve your differences and we could make forward progress on that.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703264890
>
>
> @patacongo there are some nxstyle warnning need to resolve:
> /home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:393:78: error: Long line found
> /home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:416:82: error: Long line found
> /home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:543:78: error: Long line found
> /home/runner/work/incubator-nuttx-apps/incubator-nuttx-apps/apps/fsutils/ipcfg/ipcfg_text.c:566:82: error: Long line found
> All looks fine, I will merge the change once it pass the precheck.
Fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703678582
>
>
> @patacongo based on the last comment above, can you please use the extra 16 bit in the header as a version number?
No, but you are welcome to submit a PR.
I will be integrating IPv6 support into netinit today. This may conflict with your plans, but I hope not.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499249954
##########
File path: fsutils/ipcfg/ipcfg_binary.c
##########
@@ -0,0 +1,567 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_binary.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <fcntl.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifdef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_open (for binary mode)
+ *
+ * Description:
+ * Form the complete path to the ipcfg file and open it.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * oflags - File open flags
+ * mode - File creation mode
+ *
+ * Returned Value:
+ * The open file descriptor is returned on success; a negated errno value
+ * is returned on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_BINARY
+static int ipcfg_open(FAR const char *path, int oflags, mode_t mode)
+{
+ int fd;
+ int ret;
+
+ /* Now open the file */
+
+ fd = open(path, oflags, mode);
+ if (fd < 0)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+#if defined(CONFIG_IPCFG_OFFSET) && CONFIG_IPCFG_OFFSET > 0
+ /* If the binary file is accessed on a character device as a binary
+ * file, then there is also an option to seek to a location on the
+ * media before reading or writing the file.
+ */
+
+ ret = lseek(fd, CONFIG_IPCFG_OFFSET, SEEK_SET);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to seek to $ld: %d\n",
+ (long)CONFIG_IPCFG_OFFSET, ret);
+
+ close(fd);
+ return ret;
+ }
+
+#endif
+
+ return fd;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary
+ *
+ * Description:
+ * Read from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * buffer - Location to read from
+ * nbytes - Number of bytes to read
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_read_binary(int fd, FAR void *buffer, size_t nbytes)
+{
+ ssize_t nread;
+ int ret;
+
+ /* Read from the file */
+
+ nread = read(fd, buffer, nbytes);
+ if (nread < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to read from file: %d\n", ret);
+ }
+ else if (nread != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad read size: %ld\n", (long)nread);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: ipcfg_write_binary
+ *
+ * Description:
+ * Write to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to write to
+ * buffer - Location to write to
+ * nbytes - Number of bytes to wrtie
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_WRITABLE
+static int ipcfg_write_binary(int fd, FAR const void *buffer, size_t nbytes)
+{
+ ssize_t nwritten;
+ int ret;
+
+ /* Read from the file */
+
+ nwritten = write(fd, buffer, nbytes);
+ if (nwritten < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to write to file: %d\n", ret);
+ }
+ else if (nwritten != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad write size: %ld\n", (long)nwritten);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_find_binary
+ *
+ * Description:
+ * Read the location of IPv4 data in a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * af - Identifies the address family whose IP configuration is
+ * requested. May be either AF_INET or AF_INET6.
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_find_binary(int fd, sa_family_t af)
+{
+ struct ipcfg_header_s hdr;
+ off_t pos;
+ int ret;
+
+ for (; ; )
+ {
+ /* Read the header (careful.. could be uninitialized in the case of a
+ * character driver).
+ */
+
+ ret = ipcfg_read_binary(fd, &hdr, sizeof(struct ipcfg_header_s));
+ if (ret < 0)
+ {
+ /* Return on any read error */
+
+ return (int)ret;
+ }
+ else if (hdr.type == af)
+ {
+ return OK;
+ }
+ else if (hdr.type != AF_INET && hdr.type != AF_INET6)
+ {
+ return -EINVAL;
+ }
+ else if (hdr.next == 0)
+ {
+ fprintf(stderr, "ERROR: IP configuration not found\n");
+ return -ENOENT;
+ }
+
+ /* Skip to the next IP configuration record */
+
+ pos = lseek(fd, hdr.next, SEEK_CUR);
+ if (pos < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: lseek failed: %d\n", ret);
+ return ret;
+ }
+ }
+}
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg)
+{
+ int fd;
+ int ret;
+
+ DEBUGASSERT(path != NULL && ipv4cfg != NULL);
+
+ /* Open the file for reading */
+
+ fd = ipcfg_open(path, O_RDONLY, 0666);
+ if (fd < 0)
+ {
+ return fd;
+ }
+
+ /* Find the IPv4 binary in the IP configuration file */
+
+ ret = ipcfg_find_binary(fd, AF_INET);
+ if (ret < 0)
+ {
+ goto errout_with_fd;
+ }
+
+ /* Read the IPv4 Configuration */
+
+ ret = ipcfg_read_binary(fd, ipv4cfg, sizeof(struct ipv4cfg_s));
+
+errout_with_fd:
+ close(fd);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703288831
> * apps/fsutils/ipcfg does not care about network vs. host byte ordering. It saves and returns addresses in the same order that they were obtained. Perhaps this should be specified as network order?
It already is specified as little endian. In apps/fsutils/ipcfg.h:
All of the following addresses are in network order. The special value
zero is used to indicate that the address is not available:
> * inet_ntop() comments say that the input in network byte ordering. That is true, but it seems to be hard coded to work only on a little endian machine for the IPv4 conversion. The IPv6 conversion calls ntohs() for each 16-bit value. I think that is okay.
No, the inet_ntop() IPv4 logic is correct, but very confusing. I added a PR that adds comments (only) to clarify the endian-ness issues. See PR apache/incubator-nuttx#1935
> * David's netinit change assumes that the IPv4 values returned by ipcfg are in host order and uses HTONL() to convert the addresses to network order.
That would then be wrong. It does not matter since that PR was not merged.
> This is confusing. I think the byte ordering needs to be clearly stated (network ordering), inet_pton() et al should be verified, and netinit.c should not call HTONL.
I am thinking everything is OK, but very confusing. Endian-ness issues are always confusing.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499250124
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
+};
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv4)
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv6)
+int ipcfg_read_binary_ipv6(FAR const char *path,
+ FAR struct ipv6cfg_s *ipv6cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_binary_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_IPCFG_BINARY) && \
+ defined(CONFIG_NET_IPv4)
+int ipcfg_write_binary_ipv4(FAR const char *path,
+ FAR const struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_binary_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv6cfg - The IPv6 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_IPCFG_BINARY) && \
+ defined(CONFIG_NET_IPv6)
+int ipcfg_write_binary_ipv6(FAR const char *path,
+ FAR const struct ipv6cfg_s *ipv6cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv6
+ *
+ * Description:
+ * Read IPv6 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv6cfg - Location to read IPv6 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv6
+int ipcfg_read_text_ipv6(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv6cfg_s *ipv6cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+int ipcfg_write_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR const struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a binary IP Configuration file.
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703263879
>
>
> One more comment: can we replace all printf in fsutils/ipcfg with ferr? It will save a lot of code size.
Done
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo edited a comment on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo edited a comment on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703282339
@xiaoxiang781216 Thanks for the merge.
I have some concerns about byte ordering.
- apps/fsutils/ipcfg does not care about network vs. host byte ordering. It saves and returns addresses in the same order that they were obtained. Perhaps this should be specified as network order?
- But it does call inet_addr() which expects the string representation in host order, but returns the binary in network order.
- It also calls inet_pton() and inet_ntop(). inet_pton() expects the string representation in host order. The comments say that it returns the binary in network order, but I don't see that. It looks like it returns the data in host order (but I might be wrong).
- inet_ntop() comments say that the input in network byte ordering. That is true, but it seems to be hard coded to work only on a little endian machine for the IPv4 conversion. The IPv6 conversion calls ntohs() for each 16-bit value. I think that is okay.
- apps/examples/provides addresses in network order. In testing, the final addresses are printed in the correct host byte order. So that seems to be fine.
- David's netinit change assumes that the IPv4 values returned by ipcfg are in host order and uses HTONL() to convert the addresses to network order.
This is confusing. I think the byte ordering needs to be clearly stated (network ordering), inet_pton() et al should be verified, and netinit.c should not call HTONL. I will look at this more when I incorporate the ipcfg changes in netinit. Perhaps there is no problem that a few comments cannot fix.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499249687
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo edited a comment on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo edited a comment on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703179220
@xiaoxiang781216 @davids5 There is no impact to the release from this PR (because there is still no user of ipcfg in master) so this could be merged whenever you are comfortable. I have tested pretty thoroughly and I am confident in the state of the changes. I think David has become exasperated since he has closed PR #415 . After this is merged, I will submit a PR to get the IPv4 and IPv6 configuration from the configuration file. I will not implement David's full polling logic from PR #415 , only the logic that gets the IP addresses which I think is non-controversial. It would be nice if you two could resolve your differences and we could make forward progress on that.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] xiaoxiang781216 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703208419
> @xiaoxiang781216 @davids5 There is no impact to the release from this PR (because there is still no user of ipcfg in master) so this could be merged whenever you are comfortable. I have tested pretty thoroughly and I am confident in the state of the changes. I think David has become exasperated since he has closed PR #415 . After this is merged, I will submit a PR to get the IPv4 and IPv6 configuration from the configuration file. I will not implement David's full polling logic from PR #415 , only the logic that gets the IP addresses which I think is non-controversial. It would be nice if you two could resolve your differences and we could make forward progress on that.
Sure, let's go ahead. Actually, all my suggestions are very simple:
1. Make NETINIT_ESTABLISH_POLL_RATE/NETINIT_LOSS_POLL_RATE available in both interrupt/poll case
2. Make NETINIT_DHCP_FALLBACK available in both interrupt/poll case
3. Initialize g_netconf with the default if ipcfg_read return fail to avoid the code duplication
The first two only involve the minor change in Kconfig, the 3rd one just need reorder the initialition code.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499250212
##########
File path: fsutils/ipcfg/ipcfg_text.c
##########
@@ -0,0 +1,810 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_text.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <ctype.h>
+
+#include <arpa/inet.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifndef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static const char *g_ipv4proto_name[] =
+{
+ "none", /* IPv4PROTO_NONE */
+ "static", /* IPv4PROTO_STATIC */
+ "dhcp", /* IPv4PROTO_DHCP */
+ "fallback" /* IPv4PROTO_FALLBACK */
+};
+#endif
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static const char *g_ipv6proto_name[] =
+{
+ "none", /* IPv6PROTO_NONE */
+ "static", /* IPv6PROTO_STATIC */
+ "dhcp", /* IPv6PROTO_AUTOCONF */
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] xiaoxiang781216 commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
xiaoxiang781216 commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499205762
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
Review comment:
AF_INET, not true anymore?
##########
File path: fsutils/ipcfg/ipcfg_text.c
##########
@@ -0,0 +1,810 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_text.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <ctype.h>
+
+#include <arpa/inet.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifndef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static const char *g_ipv4proto_name[] =
+{
+ "none", /* IPv4PROTO_NONE */
+ "static", /* IPv4PROTO_STATIC */
+ "dhcp", /* IPv4PROTO_DHCP */
+ "fallback" /* IPv4PROTO_FALLBACK */
+};
+#endif
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static const char *g_ipv6proto_name[] =
+{
+ "none", /* IPv6PROTO_NONE */
+ "static", /* IPv6PROTO_STATIC */
+ "dhcp", /* IPv6PROTO_AUTOCONF */
Review comment:
dhcp->autoconf to match the read path
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
+};
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv4)
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
Review comment:
ipv4cfg->ipv6cfg, IPv4->IPv6
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
+};
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv4)
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
Review comment:
IPv4->IPv6
##########
File path: fsutils/ipcfg/ipcfg_binary.c
##########
@@ -0,0 +1,567 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_binary.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <fcntl.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifdef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_open (for binary mode)
+ *
+ * Description:
+ * Form the complete path to the ipcfg file and open it.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * oflags - File open flags
+ * mode - File creation mode
+ *
+ * Returned Value:
+ * The open file descriptor is returned on success; a negated errno value
+ * is returned on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_BINARY
+static int ipcfg_open(FAR const char *path, int oflags, mode_t mode)
+{
+ int fd;
+ int ret;
+
+ /* Now open the file */
+
+ fd = open(path, oflags, mode);
+ if (fd < 0)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+#if defined(CONFIG_IPCFG_OFFSET) && CONFIG_IPCFG_OFFSET > 0
+ /* If the binary file is accessed on a character device as a binary
+ * file, then there is also an option to seek to a location on the
+ * media before reading or writing the file.
+ */
+
+ ret = lseek(fd, CONFIG_IPCFG_OFFSET, SEEK_SET);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to seek to $ld: %d\n",
+ (long)CONFIG_IPCFG_OFFSET, ret);
+
+ close(fd);
+ return ret;
+ }
+
+#endif
+
+ return fd;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary
+ *
+ * Description:
+ * Read from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * buffer - Location to read from
+ * nbytes - Number of bytes to read
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_read_binary(int fd, FAR void *buffer, size_t nbytes)
+{
+ ssize_t nread;
+ int ret;
+
+ /* Read from the file */
+
+ nread = read(fd, buffer, nbytes);
+ if (nread < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to read from file: %d\n", ret);
+ }
+ else if (nread != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad read size: %ld\n", (long)nread);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: ipcfg_write_binary
+ *
+ * Description:
+ * Write to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to write to
+ * buffer - Location to write to
+ * nbytes - Number of bytes to wrtie
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_WRITABLE
+static int ipcfg_write_binary(int fd, FAR const void *buffer, size_t nbytes)
+{
+ ssize_t nwritten;
+ int ret;
+
+ /* Read from the file */
+
+ nwritten = write(fd, buffer, nbytes);
+ if (nwritten < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to write to file: %d\n", ret);
+ }
+ else if (nwritten != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad write size: %ld\n", (long)nwritten);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_find_binary
+ *
+ * Description:
+ * Read the location of IPv4 data in a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * af - Identifies the address family whose IP configuration is
+ * requested. May be either AF_INET or AF_INET6.
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_find_binary(int fd, sa_family_t af)
+{
+ struct ipcfg_header_s hdr;
+ off_t pos;
+ int ret;
+
+ for (; ; )
+ {
+ /* Read the header (careful.. could be uninitialized in the case of a
+ * character driver).
+ */
+
+ ret = ipcfg_read_binary(fd, &hdr, sizeof(struct ipcfg_header_s));
+ if (ret < 0)
+ {
+ /* Return on any read error */
+
+ return (int)ret;
+ }
+ else if (hdr.type == af)
+ {
+ return OK;
+ }
+ else if (hdr.type != AF_INET && hdr.type != AF_INET6)
+ {
+ return -EINVAL;
+ }
+ else if (hdr.next == 0)
+ {
+ fprintf(stderr, "ERROR: IP configuration not found\n");
+ return -ENOENT;
+ }
+
+ /* Skip to the next IP configuration record */
+
+ pos = lseek(fd, hdr.next, SEEK_CUR);
+ if (pos < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: lseek failed: %d\n", ret);
+ return ret;
+ }
+ }
+}
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg)
+{
+ int fd;
+ int ret;
+
+ DEBUGASSERT(path != NULL && ipv4cfg != NULL);
+
+ /* Open the file for reading */
+
+ fd = ipcfg_open(path, O_RDONLY, 0666);
+ if (fd < 0)
+ {
+ return fd;
+ }
+
+ /* Find the IPv4 binary in the IP configuration file */
+
+ ret = ipcfg_find_binary(fd, AF_INET);
+ if (ret < 0)
+ {
+ goto errout_with_fd;
+ }
+
+ /* Read the IPv4 Configuration */
+
+ ret = ipcfg_read_binary(fd, ipv4cfg, sizeof(struct ipv4cfg_s));
+
+errout_with_fd:
+ close(fd);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
Review comment:
IPv4->IPv6
##########
File path: fsutils/ipcfg/ipcfg_binary.c
##########
@@ -0,0 +1,567 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_binary.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <fcntl.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifdef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_open (for binary mode)
+ *
+ * Description:
+ * Form the complete path to the ipcfg file and open it.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * oflags - File open flags
+ * mode - File creation mode
+ *
+ * Returned Value:
+ * The open file descriptor is returned on success; a negated errno value
+ * is returned on any failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_BINARY
+static int ipcfg_open(FAR const char *path, int oflags, mode_t mode)
+{
+ int fd;
+ int ret;
+
+ /* Now open the file */
+
+ fd = open(path, oflags, mode);
+ if (fd < 0)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+#if defined(CONFIG_IPCFG_OFFSET) && CONFIG_IPCFG_OFFSET > 0
+ /* If the binary file is accessed on a character device as a binary
+ * file, then there is also an option to seek to a location on the
+ * media before reading or writing the file.
+ */
+
+ ret = lseek(fd, CONFIG_IPCFG_OFFSET, SEEK_SET);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to seek to $ld: %d\n",
+ (long)CONFIG_IPCFG_OFFSET, ret);
+
+ close(fd);
+ return ret;
+ }
+
+#endif
+
+ return fd;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary
+ *
+ * Description:
+ * Read from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * buffer - Location to read from
+ * nbytes - Number of bytes to read
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_read_binary(int fd, FAR void *buffer, size_t nbytes)
+{
+ ssize_t nread;
+ int ret;
+
+ /* Read from the file */
+
+ nread = read(fd, buffer, nbytes);
+ if (nread < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to read from file: %d\n", ret);
+ }
+ else if (nread != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad read size: %ld\n", (long)nread);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+
+/****************************************************************************
+ * Name: ipcfg_write_binary
+ *
+ * Description:
+ * Write to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to write to
+ * buffer - Location to write to
+ * nbytes - Number of bytes to wrtie
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_IPCFG_WRITABLE
+static int ipcfg_write_binary(int fd, FAR const void *buffer, size_t nbytes)
+{
+ ssize_t nwritten;
+ int ret;
+
+ /* Read from the file */
+
+ nwritten = write(fd, buffer, nbytes);
+ if (nwritten < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to write to file: %d\n", ret);
+ }
+ else if (nwritten != nbytes)
+ {
+ ret = -EIO;
+ fprintf(stderr, "ERROR: Bad write size: %ld\n", (long)nwritten);
+ }
+ else
+ {
+ ret = OK;
+ }
+
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_find_binary
+ *
+ * Description:
+ * Read the location of IPv4 data in a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * fd - File descriptor of the open file to read from
+ * af - Identifies the address family whose IP configuration is
+ * requested. May be either AF_INET or AF_INET6.
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+static int ipcfg_find_binary(int fd, sa_family_t af)
+{
+ struct ipcfg_header_s hdr;
+ off_t pos;
+ int ret;
+
+ for (; ; )
+ {
+ /* Read the header (careful.. could be uninitialized in the case of a
+ * character driver).
+ */
+
+ ret = ipcfg_read_binary(fd, &hdr, sizeof(struct ipcfg_header_s));
+ if (ret < 0)
+ {
+ /* Return on any read error */
+
+ return (int)ret;
+ }
+ else if (hdr.type == af)
+ {
+ return OK;
+ }
+ else if (hdr.type != AF_INET && hdr.type != AF_INET6)
+ {
+ return -EINVAL;
+ }
+ else if (hdr.next == 0)
+ {
+ fprintf(stderr, "ERROR: IP configuration not found\n");
+ return -ENOENT;
+ }
+
+ /* Skip to the next IP configuration record */
+
+ pos = lseek(fd, hdr.next, SEEK_CUR);
+ if (pos < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: lseek failed: %d\n", ret);
+ return ret;
+ }
+ }
+}
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg)
+{
+ int fd;
+ int ret;
+
+ DEBUGASSERT(path != NULL && ipv4cfg != NULL);
+
+ /* Open the file for reading */
+
+ fd = ipcfg_open(path, O_RDONLY, 0666);
+ if (fd < 0)
+ {
+ return fd;
+ }
+
+ /* Find the IPv4 binary in the IP configuration file */
+
+ ret = ipcfg_find_binary(fd, AF_INET);
+ if (ret < 0)
+ {
+ goto errout_with_fd;
+ }
+
+ /* Read the IPv4 Configuration */
+
+ ret = ipcfg_read_binary(fd, ipv4cfg, sizeof(struct ipv4cfg_s));
+
+errout_with_fd:
+ close(fd);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
Review comment:
ipv6cfg - Location to read IPv6 configration to
##########
File path: fsutils/ipcfg/ipcfg_text.c
##########
@@ -0,0 +1,810 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg_text.c
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdio.h>
+#include <stdbool.h>
+#include <unistd.h>
+#include <ctype.h>
+
+#include <arpa/inet.h>
+
+#include "fsutils/ipcfg.h"
+#include "ipcfg.h"
+
+#ifndef CONFIG_IPCFG_BINARY
+
+/****************************************************************************
+ * Private Data
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static const char *g_ipv4proto_name[] =
+{
+ "none", /* IPv4PROTO_NONE */
+ "static", /* IPv4PROTO_STATIC */
+ "dhcp", /* IPv4PROTO_DHCP */
+ "fallback" /* IPv4PROTO_FALLBACK */
+};
+#endif
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static const char *g_ipv6proto_name[] =
+{
+ "none", /* IPv6PROTO_NONE */
+ "static", /* IPv6PROTO_STATIC */
+ "dhcp", /* IPv6PROTO_AUTOCONF */
+ "fallback" /* IPv4PROTO_FALLBACK */
+};
+#endif
+
+/****************************************************************************
+ * Private Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_trim
+ *
+ * Description:
+ * Skip over any whitespace.
+ *
+ * Input Parameters:
+ * line - Pointer to line buffer
+ * index - Current index into the line buffer
+ *
+ * Returned Value:
+ * New value of index.
+ *
+ ****************************************************************************/
+
+#ifndef CONFIG_IPCFG_BINARY
+static int ipcfg_trim(FAR char *line, int index)
+{
+ int ret;
+ while (line[index] != '\0' && isspace(line[index]))
+ {
+ index++;
+ }
+
+ ret = index;
+ while (line[index] != '\0')
+ {
+ if (!isprint(line[index]))
+ {
+ line[index] = '\0';
+ break;
+ }
+
+ index++;
+ }
+
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_put_ipv4addr
+ *
+ * Description:
+ * Write a <variable>=<address> value pair to the stream.
+ *
+ * Input Parameters:
+ * stream - The output stream
+ * variable - The variable namespace
+ * address - The IP address to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static int ipcfg_put_ipv4addr(FAR FILE *stream, FAR const char *variable,
+ in_addr_t address)
+{
+ if (address != 0)
+ {
+ struct in_addr saddr =
+ {
+ address
+ };
+
+ char converted[INET_ADDRSTRLEN];
+
+ /* Convert the address to ASCII text */
+
+ if (inet_ntop(AF_INET, &saddr, converted, INET_ADDRSTRLEN) == NULL)
+ {
+ int ret = -errno;
+ fprintf(stderr, "ERROR: inet_ntop() failed: %d\n", ret);
+ return ret;
+ }
+
+ fprintf(stream, "%s=%s\n", variable, converted);
+ }
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_check_ipv6addr
+ *
+ * Description:
+ * Check for a valid IPv6 address, i.e., not all zeroes.
+ *
+ * Input Parameters:
+ * address - A pointer to the address to check.
+ *
+ * Returned value:
+ * Zero (OK) is returned if the address is non-zero. -ENXIO is returned if
+ * the address is zero.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv6
+static int ipcfg_check_ipv6addr(FAR const struct in6_addr *address)
+{
+ int i;
+
+ for (i = 0; i < 4; i++)
+ {
+ if (address->s6_addr32[i] != 0)
+ {
+ return OK;
+ }
+ }
+
+ return -ENXIO;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_put_ipv6addr
+ *
+ * Description:
+ * Write a <variable>=<address> value pair to the stream.
+ *
+ * Input Parameters:
+ * stream - The output stream
+ * variable - The variable namespace
+ * address - The IP address to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static int ipcfg_put_ipv6addr(FAR FILE *stream, FAR const char *variable,
+ FAR const struct in6_addr *address)
+{
+ /* If the address is all zero, then omit it */
+
+ if (ipcfg_check_ipv6addr(address) == OK)
+ {
+ char converted[INET6_ADDRSTRLEN];
+
+ /* Convert the address to ASCII text */
+
+ if (inet_ntop(AF_INET6, address, converted, INET6_ADDRSTRLEN) == NULL)
+ {
+ int ret = -errno;
+ fprintf(stderr, "ERROR: inet_ntop() failed: %d\n", ret);
+ return ret;
+ }
+
+ fprintf(stream, "%s=%s\n", variable, converted);
+ }
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * stream - Stream of the open file to write to
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+static int ipcfg_write_ipv4(FAR FILE *stream,
+ FAR const struct ipv4cfg_s *ipv4cfg)
+{
+ /* Format and write the file */
+
+ if ((unsigned)ipv4cfg->proto > MAX_IPv4PROTO)
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv4PROTO value: %d\n",
+ ipv4cfg->proto);
+ return -EINVAL;
+ }
+
+ fprintf(stream, "IPv4PROTO=%s\n", g_ipv4proto_name[ipv4cfg->proto]);
+
+ ipcfg_put_ipv4addr(stream, "IPv4IPADDR", ipv4cfg->ipaddr);
+ ipcfg_put_ipv4addr(stream, "IPv4NETMASK", ipv4cfg->netmask);
+ ipcfg_put_ipv4addr(stream, "IPv4ROUTER", ipv4cfg->router);
+ ipcfg_put_ipv4addr(stream, "IPv4DNS", ipv4cfg->dnsaddr);
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * stream - Stream of the open file to write to
+ * ipv6cfg - The IPv6 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv6)
+static int ipcfg_write_ipv6(FAR FILE *stream,
+ FAR const struct ipv6cfg_s *ipv6cfg)
+{
+ /* Format and write the file */
+
+ if ((unsigned)ipv6cfg->proto > MAX_IPv6PROTO)
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv6PROTO value: %d\n",
+ ipv6cfg->proto);
+ return -EINVAL;
+ }
+
+ fprintf(stream, "IPv6PROTO=%s\n", g_ipv6proto_name[ipv6cfg->proto]);
+
+ ipcfg_put_ipv6addr(stream, "IPv6IPADDR", &ipv6cfg->ipaddr);
+ ipcfg_put_ipv6addr(stream, "IPv6NETMASK", &ipv6cfg->netmask);
+ ipcfg_put_ipv6addr(stream, "IPv6ROUTER", &ipv6cfg->router);
+
+ return OK;
+}
+#endif
+
+/****************************************************************************
+ * Public Functions
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv4cfg_s *ipv4cfg)
+{
+ char line[MAX_LINESIZE];
+ FAR FILE *stream;
+ int index;
+ int ret = -ENOENT;
+
+ DEBUGASSERT(stream != NULL && ipv4cfg != NULL);
+
+ /* Open the file for reading */
+
+ stream = fopen(path, "r");
+ if (stream == NULL)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+ /* Process each line in the file */
+
+ memset(ipv4cfg, 0, sizeof(FAR struct ipv4cfg_s));
+
+ while (fgets(line, MAX_LINESIZE, stream) != NULL)
+ {
+ /* Skip any leading whitespace */
+
+ index = ipcfg_trim(line, 0);
+
+ /* Check for a blank line or a comment */
+
+ if (line[index] != '\0' && line[index] != '#')
+ {
+ FAR char *variable = &line[index];
+ FAR char *value;
+
+ /* Expect <variable>=<value> pair */
+
+ value = strchr(variable, '=');
+ if (value == NULL)
+ {
+ fprintf(stderr, "ERROR: Skipping malformed line in file: %s\n",
+ line);
+ continue;
+ }
+
+ /* NUL-terminate the variable string */
+
+ *value++ = '\0';
+
+ /* Process the variable assignment */
+
+ if (strcmp(variable, "DEVICE") == 0)
+ {
+ /* Just assure that it matches the filename */
+
+ if (strcmp(value, netdev) != 0)
+ {
+ fprintf(stderr, "ERROR: Bad device in file: %s=%s\n",
+ variable, value);
+ }
+ }
+ else if (strcmp(variable, "IPv4PROTO") == 0)
+ {
+ if (strcmp(value, "none") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_NONE;
+ }
+ else if (strcmp(value, "static") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_STATIC;
+ }
+ else if (strcmp(value, "dhcp") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_DHCP;
+ }
+ else if (strcmp(value, "fallback") == 0)
+ {
+ ipv4cfg->proto = IPv4PROTO_FALLBACK;
+ }
+ else
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv4PROTO: %s=%s\n",
+ variable, value);
+ }
+
+ /* Assume IPv4 settings are present if the IPv4BOOTPROTO
+ * setting is encountered.
+ */
+
+ ret = OK;
+ }
+ else if (strcmp(variable, "IPv4IPADDR") == 0)
+ {
+ ipv4cfg->ipaddr = inet_addr(value);
+ }
+ else if (strcmp(variable, "IPv4NETMASK") == 0)
+ {
+ ipv4cfg->netmask = inet_addr(value);
+ }
+ else if (strcmp(variable, "IPv4ROUTER") == 0)
+ {
+ ipv4cfg->router = inet_addr(value);
+ }
+ else if (strcmp(variable, "IPv4DNS") == 0)
+ {
+ ipv4cfg->dnsaddr = inet_addr(value);
+ }
+
+ /* Anything other than some IPv6 settings would be an error.
+ * This is a sloppy check because it does not detect invalid
+ * names variables that begin with "IPv6".
+ */
+
+ else if (strncmp(variable, "IPv6", 4) != 0)
+ {
+ fprintf(stderr, "ERROR: Unrecognized variable: %s=%s\n",
+ variable, value);
+ }
+ }
+ }
+
+ /* Close the file and return */
+
+ fclose(stream);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv6
+ *
+ * Description:
+ * Read IPv6 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv6cfg - Location to read IPv6 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv6
+int ipcfg_read_text_ipv6(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv6cfg_s *ipv6cfg)
+{
+ char line[MAX_LINESIZE];
+ FAR FILE *stream;
+ bool found = false;
+ int index;
+ int ret;
+
+ DEBUGASSERT(path != NULL && netdev != NULL && ipv6cfg != NULL);
+
+ /* Open the file for reading */
+
+ stream = fopen(path, "r");
+ if (stream == NULL)
+ {
+ ret = -errno;
+ if (ret != -ENOENT)
+ {
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ }
+
+ return ret;
+ }
+
+ /* Process each line in the file */
+
+ memset(ipv6cfg, 0, sizeof(FAR struct ipv6cfg_s));
+
+ while (fgets(line, MAX_LINESIZE, stream) != NULL)
+ {
+ /* Skip any leading whitespace */
+
+ index = ipcfg_trim(line, 0);
+
+ /* Check for a blank line or a comment */
+
+ if (line[index] != '\0' && line[index] != '#')
+ {
+ FAR char *variable = &line[index];
+ FAR char *value;
+
+ /* Expect <variable>=<value> pair */
+
+ value = strchr(variable, '=');
+ if (value == NULL)
+ {
+ fprintf(stderr, "ERROR: Skipping malformed line in file: %s\n",
+ line);
+ continue;
+ }
+
+ /* NUL-terminate the variable string */
+
+ *value++ = '\0';
+
+ /* Process the variable assignment */
+
+ if (strcmp(variable, "DEVICE") == 0)
+ {
+ /* Just assure that it matches the filename */
+
+ if (strcmp(value, netdev) != 0)
+ {
+ fprintf(stderr, "ERROR: Bad device in file: %s=%s\n",
+ variable, value);
+ }
+ }
+ else if (strcmp(variable, "IPv6PROTO") == 0)
+ {
+ if (strcmp(value, "none") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_NONE;
+ }
+ else if (strcmp(value, "static") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_STATIC;
+ }
+ else if (strcmp(value, "autoconf") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_AUTOCONF;
+ }
+ else if (strcmp(value, "fallback") == 0)
+ {
+ ipv6cfg->proto = IPv6PROTO_FALLBACK;
+ }
+ else
+ {
+ fprintf(stderr, "ERROR: Unrecognized IPv6PROTO: %s=%s\n",
+ variable, value);
+ }
+
+ /* Assume IPv4 settings are present if the IPv6BOOTPROTO
+ * setting is encountered.
+ */
+
+ found = true;
+ }
+ else if (strcmp(variable, "IPv6IPADDR") == 0)
+ {
+ ret = inet_pton(AF_INET6, value, &ipv6cfg->ipaddr);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: inet_pton() failed: %d\n", ret);
+ return ret;
+ }
+ }
+ else if (strcmp(variable, "IPv6NETMASK") == 0)
+ {
+ ret = inet_pton(AF_INET6, value, &ipv6cfg->netmask);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: inet_pton() failed: %d\n", ret);
+ return ret;
+ }
+ }
+ else if (strcmp(variable, "IPv6ROUTER") == 0)
+ {
+ ret = inet_pton(AF_INET6, value, &ipv6cfg->router);
+ if (ret < 0)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: inet_pton() failed: %d\n", ret);
+ return ret;
+ }
+ }
+
+ /* Anything other than some IPv4 settings would be an error.
+ * This is a sloppy check because it does not detect invalid
+ * names variables that begin with "IPv4".
+ */
+
+ else if (strncmp(variable, "IPv4", 4) != 0)
+ {
+ fprintf(stderr, "ERROR: Unrecognized variable: %s=%s\n",
+ variable, value);
+ }
+ }
+ }
+
+ /* Close the file and return */
+
+ fclose(stream);
+ return found ? OK : -ENOENT;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+int ipcfg_write_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR const struct ipv4cfg_s *ipv4cfg)
+{
+#ifdef CONFIG_NET_IPv6
+ struct ipv6cfg_s ipv6cfg;
+ bool ipv6 = false;
+#endif
+ FAR FILE *stream;
+ int ret;
+
+ DEBUGASSERT(stream != NULL && ipv4cfg != NULL);
+
+#ifdef CONFIG_NET_IPv6
+ /* Read any IPv6 data in the file */
+
+ ret = ipcfg_read_text_ipv6(path, netdev, &ipv6cfg);
+ if (ret < 0)
+ {
+ /* -ENOENT is not an error. It simply means that there is no IPv6
+ * configuration in the file.
+ */
+
+ if (ret != -ENOENT)
+ {
+ return ret;
+ }
+ }
+ else
+ {
+ ipv6 = true;
+ }
+#endif
+
+ /* Open the file for writing (truncates) */
+
+ stream = fopen(path, "w");
+ if (stream == NULL)
+ {
+ ret = -errno;
+ fprintf(stderr, "ERROR: Failed to open %s: %d\n", path, ret);
+ return ret;
+ }
+
+ /* Save the device name */
+
+ fprintf(stream, "DEVICE=%s\n", netdev);
+
+ /* Write the IPv4 configuration */
+
+ ret = ipcfg_write_ipv4(stream, ipv4cfg);
+ if (ret < 0)
+ {
+ return ret;
+ }
+
+#ifdef CONFIG_NET_IPv6
+ /* Followed by any IPv6 data in the file */
+
+ if (ipv6)
+ {
+ ret = ipcfg_write_ipv6(stream, &ipv6cfg);
+ }
+#endif
+
+ fclose(stream);
+ return ret;
+}
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a binary IP Configuration file.
Review comment:
binary->text
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
+};
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv4)
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv6)
+int ipcfg_read_binary_ipv6(FAR const char *path,
+ FAR struct ipv6cfg_s *ipv6cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_binary_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_IPCFG_BINARY) && \
+ defined(CONFIG_NET_IPv4)
+int ipcfg_write_binary_ipv4(FAR const char *path,
+ FAR const struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_binary_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv6cfg - The IPv6 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_IPCFG_BINARY) && \
+ defined(CONFIG_NET_IPv6)
+int ipcfg_write_binary_ipv6(FAR const char *path,
+ FAR const struct ipv6cfg_s *ipv6cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv4
+int ipcfg_read_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_text_ipv6
+ *
+ * Description:
+ * Read IPv6 configuration from a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv6cfg - Location to read IPv6 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#ifdef CONFIG_NET_IPv6
+int ipcfg_read_text_ipv6(FAR const char *path, FAR const char *netdev,
+ FAR struct ipv6cfg_s *ipv6cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv4
+ *
+ * Description:
+ * Write the IPv4 configuration to a human-readable, text IP Configuration
+ * file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * netdev - Network device name string
+ * ipv4cfg - The IPv4 configration to write
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_WRITABLE) && defined(CONFIG_NET_IPv4)
+int ipcfg_write_text_ipv4(FAR const char *path, FAR const char *netdev,
+ FAR const struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_write_text_ipv6
+ *
+ * Description:
+ * Write the IPv6 configuration to a binary IP Configuration file.
Review comment:
binary->text
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703282339
@xiaoxiang781216 Thanks for the merge.
I have some concerns about byte ordering.
- apps/fsutils/ipcfg does not care about network vs. host byte ordering. It saves and returns addresses in the same order that they were provided. Perhaps this should be specified as network order?
- But it does call inet_addr() which expects the string representation in host order, but returns the binary in network order.
- It also calls inet_pton() and inet_ntop(). inet_pton() expects the string representation in host order. The comments say that it returns the binary in network order, but I don't see that. It looks like it returns the data in host order (but I might be wrong).
- inet_ntop() comments say that the input in network byte ordering. That is true, but it seems to be hard coded to work only on a little endian machine of the IPv4 conversion. The IPv6 conversion calls ntohs() for each 16-bit value. I think that is okay.
- apps/examples/provides addresses in host order. But in testing, the final addresses are printed in the correct host byte order. Now I am thinking that is suspicious.
- David's netinit change assumes that the IPv4 values returned by ipcfg are in host order and uses HTONL() to convert the addresses to network order.
This is confusing. I think the byte ordering needs to be clearly stated (network ordering), inet_pton() et al should be verified, and netinit.c should not call HTONL. I will look at this more when I incorporate the ipcfg changes in netinit. Perhaps there is no problem that a few comments cannot fix.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on a change in pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on a change in pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#discussion_r499249779
##########
File path: fsutils/ipcfg/ipcfg.h
##########
@@ -0,0 +1,237 @@
+/****************************************************************************
+ * apps/fsutils/ipcfg/ipcfg.h
+ *
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership. The
+ * ASF licenses this file to you under the Apache License, Version 2.0 (the
+ * "License"); you may not use this file except in compliance with the
+ * License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
+ * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
+ * License for the specific language governing permissions and limitations
+ * under the License.
+ *
+ ****************************************************************************/
+
+#ifndef __APPS_FSUTILS_IPCFG_IPCFG_H
+#define __APPS_FSUTILS_IPCFG_IPCFG_H
+
+/****************************************************************************
+ * Included Files
+ ****************************************************************************/
+
+#include <nuttx/config.h>
+
+#include <stdint.h>
+
+#include <netinet/in.h>
+
+#include "fsutils/ipcfg.h"
+
+/****************************************************************************
+ * Pre-processor Definitions
+ ****************************************************************************/
+
+#define MAX_LINESIZE 80
+#define MAX_IPv4PROTO IPv4PROTO_FALLBACK
+#define MAX_IPv6PROTO IPv6PROTO_FALLBACK
+
+/****************************************************************************
+ * Public Types
+ ****************************************************************************/
+
+/* IP Configuration record header. */
+
+struct ipcfg_header_s
+{
+ uint8_t next; /* Offset to the next IP configuration record */
+ sa_family_t type; /* Must be AF_INET */
+};
+
+/****************************************************************************
+ * Public Function Prototypes
+ ****************************************************************************/
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv4
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
+ *
+ * Input Parameters:
+ * path - The full path to the IP configuration file
+ * ipv4cfg - Location to read IPv4 configration to
+ *
+ * Returned Value:
+ * Zero is returned on success; a negated errno value is returned on any
+ * failure.
+ *
+ ****************************************************************************/
+
+#if defined(CONFIG_IPCFG_BINARY) && defined(CONFIG_NET_IPv4)
+int ipcfg_read_binary_ipv4(FAR const char *path,
+ FAR struct ipv4cfg_s *ipv4cfg);
+#endif
+
+/****************************************************************************
+ * Name: ipcfg_read_binary_ipv6
+ *
+ * Description:
+ * Read IPv4 configuration from a binary IP Configuration file.
Review comment:
fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] patacongo commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
patacongo commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703261007
@xiaoxiang781216 I believe that all of you comments have been addressed.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [incubator-nuttx-apps] davids5 commented on pull request #417: apps/fsutils/ipcfg: Add support for IPv6
Posted by GitBox <gi...@apache.org>.
davids5 commented on pull request #417:
URL: https://github.com/apache/incubator-nuttx-apps/pull/417#issuecomment-703577385
@patacongo based on the last comment above, can you please use the extra 16 bit in the header as a version number?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org