You are viewing a plain text version of this content. The canonical link for it is here.
Posted to notifications@dubbo.apache.org by GitBox <gi...@apache.org> on 2020/08/15 15:10:46 UTC
[GitHub] [dubbo-go-hessian2] zhangshen023 opened a new pull request #219: java.sql.Time & java.sql.Date
zhangshen023 opened a new pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219
**What this PR does**:
java class java.sql.Time & java.sql.Date refer to hessian.Time & hessian.Date
**Which issue(s) this PR fixes**:
Fixes #
**Special notes for your reviewer**:
**Does this PR introduce a user-facing change?**:
can use hessian.Time & hessian.Date in go for communicating with java.sql.Time & java.sql.Date in java
```release-note
```
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674487720
ok,i will make a try
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r471177263
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
+ return t.Time.Second()
+}
+
+func (t *Time) setTime(time time.Time) {
+ t.Time = time
+}
+
+func (t *Time) ValueOf(timeStr string) error {
+ time, err := time.Parse("15:04:05", timeStr)
+ if err != nil {
+ return err
+ }
+ t.Time = time
+ return nil
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+func SetJavaSqlTimeSerialize(time JavaSqlTime) {
+ name := time.JavaClassName()
+ var typ = reflect.TypeOf(time)
+ SetSerializer(name, JavaSqlTimeSerializer{})
+ //RegisterPOJO(time)
+ javaSqlTimeTypeMap[name] = typ
+}
+
+func getJavaSqlTimeSerialize(name string) reflect.Type {
+ return javaSqlTimeTypeMap[name]
+}
+
+type JavaSqlTimeSerializer struct {
+}
+
+func (JavaSqlTimeSerializer) EncObject(e *Encoder, vv POJO) error {
+
+ var (
+ idx int
+ idx1 int
+ i int
+ err error
+ clsDef classInfo
+ )
+ v, ok := vv.(JavaSqlTime)
+ if !ok {
+ return perrors.New("can not be converted into java sql time object")
+ }
+ className := v.JavaClassName()
+ if className == "" {
+ return perrors.New("class name empty")
+ }
+
+ tValue := reflect.ValueOf(vv)
+ // check ref
+ if n, ok := e.checkRefMap(tValue); ok {
+ e.buffer = encRef(e.buffer, n)
+ return nil
+ }
+
+ // write object definition
+ idx = -1
+ for i = range e.classInfoList {
+ if v.JavaClassName() == e.classInfoList[i].javaName {
+ idx = i
+ break
+ }
+ }
+
+ if idx == -1 {
+ idx1, ok = checkPOJORegistry(typeof(v))
+ if !ok {
+ if reflect.TypeOf(v).Implements(javaEnumType) {
+ idx1 = RegisterJavaEnum(v.(POJOEnum))
+ } else {
+ idx1 = RegisterPOJO(v)
+ }
+ }
+ _, clsDef, err = getStructDefByIndex(idx1)
+ if err != nil {
+ return perrors.WithStack(err)
+ }
+
+ i = len(e.classInfoList)
+ e.classInfoList = append(e.classInfoList, clsDef)
+ e.buffer = append(e.buffer, clsDef.buffer...)
+ e.buffer = e.buffer[0 : len(e.buffer)-1]
+ }
+
+ if idx == -1 {
Review comment:
optimized
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r479706008
##########
File path: java_sql_time/java_sql_time.go
##########
@@ -0,0 +1,30 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package java_sql_time
+
+import "time"
+
+type JavaSqlTime interface {
+ // ValueOf parse time string which format likes '2006-01-02 15:04:05'
+ ValueOf(timeStr string) error
+ // SetTime for decode time
+ SetTime(time time.Time)
+ JavaClassName() string
Review comment:
IMO,may cause circular reference. The hierarchy of package java_sql_time which struct 'JavaSqlTime' is kept is lower than interface Pojo's.
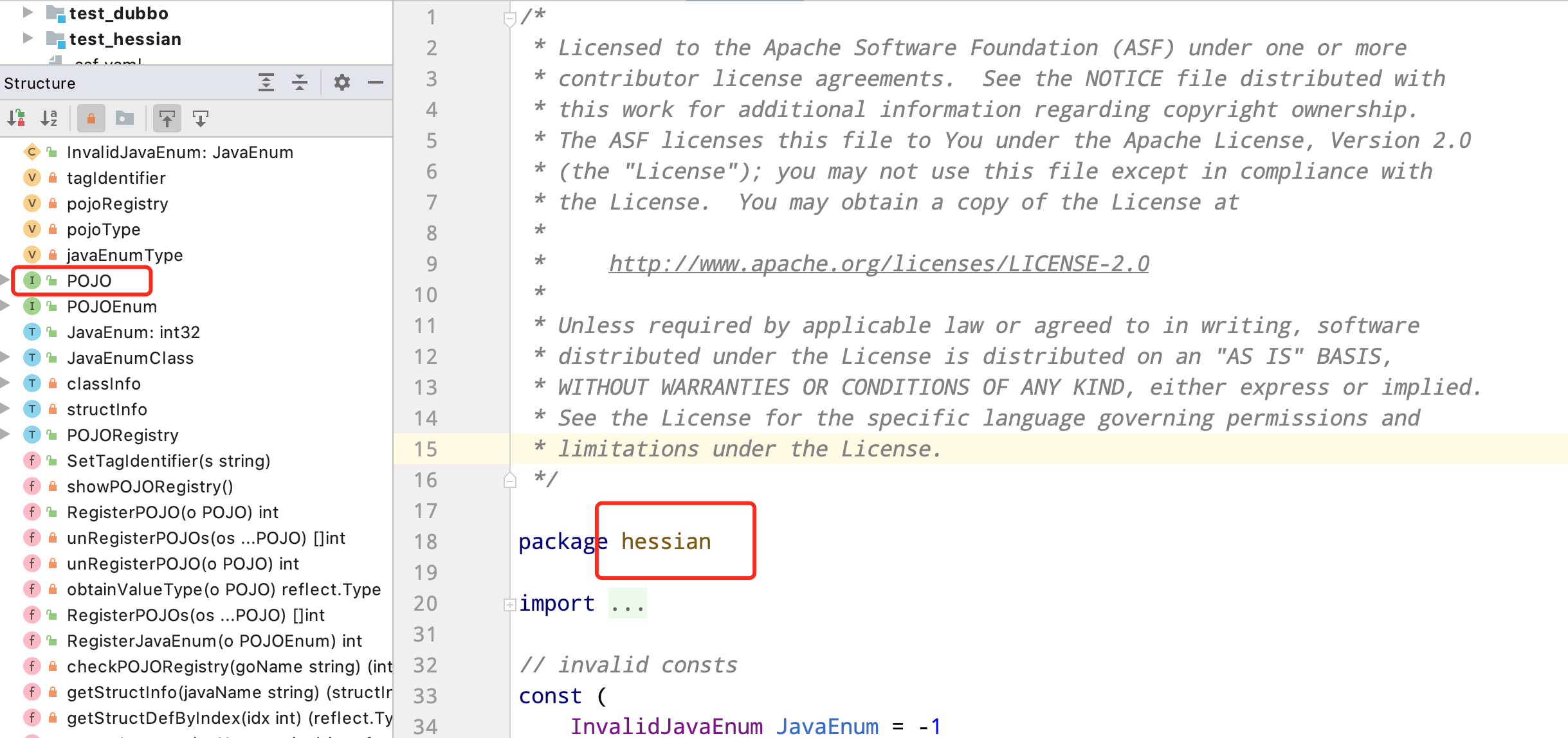
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] AlexStocks merged pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
AlexStocks merged pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] wongoo commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
wongoo commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r476494466
##########
File path: java_sql_time/java_sql_time.go
##########
@@ -0,0 +1,30 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package java_sql_time
+
+import "time"
+
+type JavaSqlTime interface {
+ // ValueOf parse time string which format likes '2006-01-02 15:04:05'
+ ValueOf(timeStr string) error
+ // SetTime for decode time
+ SetTime(time time.Time)
+ JavaClassName() string
Review comment:
should extend POJO interface
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] AlexStocks commented on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
AlexStocks commented on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674487394
@zhangshen023 pls fix the travis failure.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674487629
> @zhangshen023 pls fix the travis failure.
ok, i have a try
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r479706058
##########
File path: java_sql_time/java_sql_time.go
##########
@@ -0,0 +1,30 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package java_sql_time
+
+import "time"
+
+type JavaSqlTime interface {
+ // ValueOf parse time string which format likes '2006-01-02 15:04:05'
+ ValueOf(timeStr string) error
+ // SetTime for decode time
+ SetTime(time time.Time)
+ JavaClassName() string
Review comment:
pls see https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r479706008
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 removed a comment on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 removed a comment on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674487629
> @zhangshen023 pls fix the travis failure.
ok, i have a try
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] wongoo commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
wongoo commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r473649043
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,152 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+import (
+ "github.com/apache/dubbo-go-hessian2/java_sql_time"
+)
+
+func init() {
+ RegisterPOJO(&java_sql_time.Date{})
+ RegisterPOJO(&java_sql_time.Time{})
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+// SetJavaSqlTimeSerialize register serializer for java.sql.Time & java.sql.Date
+func SetJavaSqlTimeSerialize(time java_sql_time.JavaSqlTime) {
+ name := time.JavaClassName()
+ var typ = reflect.TypeOf(time)
+ SetSerializer(name, JavaSqlTimeSerializer{})
+ javaSqlTimeTypeMap[name] = typ
+}
+
+// nolint
+func getJavaSqlTimeSerialize(name string) reflect.Type {
+ return javaSqlTimeTypeMap[name]
+}
+
+// JavaSqlTimeSerializer used to encode & decode java.sql.Time & java.sql.Date
+type JavaSqlTimeSerializer struct {
Review comment:
move all code to package `java_sql_time`, only keep the init func in hessian package
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,152 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+import (
+ "github.com/apache/dubbo-go-hessian2/java_sql_time"
+)
+
+func init() {
+ RegisterPOJO(&java_sql_time.Date{})
+ RegisterPOJO(&java_sql_time.Time{})
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
Review comment:
javaSqlTimeTypeMap is unused, is it necessary?
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,152 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+import (
+ "github.com/apache/dubbo-go-hessian2/java_sql_time"
+)
+
+func init() {
+ RegisterPOJO(&java_sql_time.Date{})
+ RegisterPOJO(&java_sql_time.Time{})
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+// SetJavaSqlTimeSerialize register serializer for java.sql.Time & java.sql.Date
+func SetJavaSqlTimeSerialize(time java_sql_time.JavaSqlTime) {
+ name := time.JavaClassName()
+ var typ = reflect.TypeOf(time)
+ SetSerializer(name, JavaSqlTimeSerializer{})
+ javaSqlTimeTypeMap[name] = typ
+}
+
+// nolint
+func getJavaSqlTimeSerialize(name string) reflect.Type {
+ return javaSqlTimeTypeMap[name]
+}
+
+// JavaSqlTimeSerializer used to encode & decode java.sql.Time & java.sql.Date
+type JavaSqlTimeSerializer struct {
+}
+
+// nolint
+func (JavaSqlTimeSerializer) EncObject(e *Encoder, vv POJO) error {
+
+ var (
+ i int
+ idx int
+ err error
+ clsDef classInfo
+ className string
+ ptrV reflect.Value
+ )
+
+ // ensure ptrV is pointer to know vv is type JavaSqlTime or not
+ ptrV = reflect.ValueOf(vv)
+ if reflect.TypeOf(vv).Kind() != reflect.Ptr {
+ ptrV = PackPtr(ptrV)
+ }
+ v, ok := ptrV.Interface().(java_sql_time.JavaSqlTime)
+ if !ok {
+ return perrors.New("can not be converted into java sql time object")
+ }
+ className = v.JavaClassName()
+ if className == "" {
+ return perrors.New("class name empty")
+ }
+ tValue := reflect.ValueOf(vv)
+ // check ref
+ if n, ok := e.checkRefMap(tValue); ok {
+ e.buffer = encRef(e.buffer, n)
+ return nil
+ }
+
+ // write object definition
+ idx = -1
+ for i = range e.classInfoList {
+ if v.JavaClassName() == e.classInfoList[i].javaName {
+ idx = i
+ break
+ }
+ }
+ if idx == -1 {
+ idx, ok = checkPOJORegistry(typeof(vv))
+ if !ok {
+ idx = RegisterPOJO(v)
+ }
+ _, clsDef, err = getStructDefByIndex(idx)
+ if err != nil {
+ return perrors.WithStack(err)
+ }
+ idx = len(e.classInfoList)
+ e.classInfoList = append(e.classInfoList, clsDef)
+ e.buffer = append(e.buffer, clsDef.buffer...)
+ }
+ e.buffer = e.buffer[0 : len(e.buffer)-1]
+ e.buffer = encInt32(e.buffer, 1)
+ e.buffer = encString(e.buffer, "value")
+
+ // write object instance
+ if byte(idx) <= OBJECT_DIRECT_MAX {
+ e.buffer = encByte(e.buffer, byte(idx)+BC_OBJECT_DIRECT)
+ } else {
+ e.buffer = encByte(e.buffer, BC_OBJECT)
+ e.buffer = encInt32(e.buffer, int32(idx))
+ }
+ e.buffer = encDateInMs(e.buffer, v.GetTime())
+ return nil
+}
+
+// nolint
+func (JavaSqlTimeSerializer) DecObject(d *Decoder, typ reflect.Type, cls classInfo) (interface{}, error) {
+
+ if typ.Kind() != reflect.Struct {
+ return nil, perrors.Errorf("wrong type expect Struct but get:%s", typ.String())
+ }
+
+ vRef := reflect.New(typ)
+ // add pointer ref so that ref the same object
+ d.appendRefs(vRef.Interface())
+
+ tag, err := d.readByte()
+ if err == io.EOF {
+ return nil, err
+ }
+ date, err := d.decDate(int32(tag))
+ if err != nil {
+ return nil, perrors.WithStack(err)
+ }
+ sqlTime := vRef.Interface()
+
+ result, ok := sqlTime.(java_sql_time.JavaSqlTime)
+ result.SetTime(date)
Review comment:
set after checking ok
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,152 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+import (
+ "github.com/apache/dubbo-go-hessian2/java_sql_time"
+)
+
+func init() {
+ RegisterPOJO(&java_sql_time.Date{})
+ RegisterPOJO(&java_sql_time.Time{})
Review comment:
should JavaSqlTimeSerializer be registered here?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] AlexStocks commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
AlexStocks commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r471112035
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
Review comment:
add comment. especially u should introduce u date format is "2006-01-02".
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
+ return t.Time.Second()
+}
+
+func (t *Time) setTime(time time.Time) {
+ t.Time = time
+}
+
+func (t *Time) ValueOf(timeStr string) error {
+ time, err := time.Parse("15:04:05", timeStr)
+ if err != nil {
+ return err
+ }
+ t.Time = time
+ return nil
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+func SetJavaSqlTimeSerialize(time JavaSqlTime) {
+ name := time.JavaClassName()
+ var typ = reflect.TypeOf(time)
+ SetSerializer(name, JavaSqlTimeSerializer{})
+ //RegisterPOJO(time)
+ javaSqlTimeTypeMap[name] = typ
+}
+
+func getJavaSqlTimeSerialize(name string) reflect.Type {
+ return javaSqlTimeTypeMap[name]
+}
+
+type JavaSqlTimeSerializer struct {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
Review comment:
add comment
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
+ return t.Time.Second()
+}
+
+func (t *Time) setTime(time time.Time) {
+ t.Time = time
+}
+
+func (t *Time) ValueOf(timeStr string) error {
+ time, err := time.Parse("15:04:05", timeStr)
+ if err != nil {
+ return err
+ }
+ t.Time = time
+ return nil
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+func SetJavaSqlTimeSerialize(time JavaSqlTime) {
+ name := time.JavaClassName()
+ var typ = reflect.TypeOf(time)
+ SetSerializer(name, JavaSqlTimeSerializer{})
+ //RegisterPOJO(time)
+ javaSqlTimeTypeMap[name] = typ
+}
+
+func getJavaSqlTimeSerialize(name string) reflect.Type {
+ return javaSqlTimeTypeMap[name]
+}
+
+type JavaSqlTimeSerializer struct {
+}
+
+func (JavaSqlTimeSerializer) EncObject(e *Encoder, vv POJO) error {
+
+ var (
+ idx int
+ idx1 int
+ i int
+ err error
+ clsDef classInfo
+ )
+ v, ok := vv.(JavaSqlTime)
+ if !ok {
+ return perrors.New("can not be converted into java sql time object")
+ }
+ className := v.JavaClassName()
+ if className == "" {
+ return perrors.New("class name empty")
+ }
+
+ tValue := reflect.ValueOf(vv)
+ // check ref
+ if n, ok := e.checkRefMap(tValue); ok {
+ e.buffer = encRef(e.buffer, n)
+ return nil
+ }
+
+ // write object definition
+ idx = -1
+ for i = range e.classInfoList {
+ if v.JavaClassName() == e.classInfoList[i].javaName {
+ idx = i
+ break
+ }
+ }
+
+ if idx == -1 {
+ idx1, ok = checkPOJORegistry(typeof(v))
+ if !ok {
+ if reflect.TypeOf(v).Implements(javaEnumType) {
+ idx1 = RegisterJavaEnum(v.(POJOEnum))
+ } else {
+ idx1 = RegisterPOJO(v)
+ }
+ }
+ _, clsDef, err = getStructDefByIndex(idx1)
+ if err != nil {
+ return perrors.WithStack(err)
+ }
+
+ i = len(e.classInfoList)
+ e.classInfoList = append(e.classInfoList, clsDef)
+ e.buffer = append(e.buffer, clsDef.buffer...)
+ e.buffer = e.buffer[0 : len(e.buffer)-1]
+ }
+
+ if idx == -1 {
Review comment:
why using another if-clause as its condition is the same as the above if?
##########
File path: java_sql_time_test.go
##########
@@ -0,0 +1,97 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "github.com/stretchr/testify/assert"
Review comment:
pls split the if clause.
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
Review comment:
add comment
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
+ return t.Time.Second()
+}
+
+func (t *Time) setTime(time time.Time) {
+ t.Time = time
+}
+
+func (t *Time) ValueOf(timeStr string) error {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
+ return t.Time.Second()
+}
+
+func (t *Time) setTime(time time.Time) {
+ t.Time = time
+}
+
+func (t *Time) ValueOf(timeStr string) error {
+ time, err := time.Parse("15:04:05", timeStr)
+ if err != nil {
+ return err
+ }
+ t.Time = time
+ return nil
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+func SetJavaSqlTimeSerialize(time JavaSqlTime) {
Review comment:
add comment
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
Review comment:
add comment or "// nolint"
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,230 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+ "time"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+func init() {
+ RegisterPOJO(&Date{})
+ RegisterPOJO(&Time{})
+}
+
+type JavaSqlTime interface {
+ ValueOf(timeStr string) error
+ setTime(time time.Time)
+ JavaClassName() string
+ time() time.Time
+}
+
+type Date struct {
+ time.Time
+}
+
+func (d *Date) time() time.Time {
+ return d.Time
+}
+
+func (d *Date) setTime(time time.Time) {
+ d.Time = time
+}
+
+func (Date) JavaClassName() string {
+ return "java.sql.Date"
+}
+
+func (d *Date) ValueOf(dateStr string) error {
+ time, err := time.Parse("2006-01-02", dateStr)
+ if err != nil {
+ return err
+ }
+ d.Time = time
+ return nil
+}
+
+func (d *Date) Year() int {
+ return d.Time.Year()
+}
+
+func (d *Date) Month() time.Month {
+ return d.Time.Month()
+}
+
+func (d *Date) Day() int {
+ return d.Time.Day()
+}
+
+type Time struct {
+ time.Time
+}
+
+func (Time) JavaClassName() string {
+ return "java.sql.Time"
+}
+
+func (t Time) time() time.Time {
+ return t.Time
+}
+
+func (t *Time) Hours() int {
+ return t.Time.Hour()
+}
+
+func (t *Time) Minutes() int {
+ return t.Time.Minute()
+}
+
+func (t *Time) Seconds() int {
+ return t.Time.Second()
+}
+
+func (t *Time) setTime(time time.Time) {
+ t.Time = time
+}
+
+func (t *Time) ValueOf(timeStr string) error {
+ time, err := time.Parse("15:04:05", timeStr)
+ if err != nil {
+ return err
+ }
+ t.Time = time
+ return nil
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
+
+func SetJavaSqlTimeSerialize(time JavaSqlTime) {
+ name := time.JavaClassName()
+ var typ = reflect.TypeOf(time)
+ SetSerializer(name, JavaSqlTimeSerializer{})
+ //RegisterPOJO(time)
+ javaSqlTimeTypeMap[name] = typ
+}
+
+func getJavaSqlTimeSerialize(name string) reflect.Type {
+ return javaSqlTimeTypeMap[name]
+}
+
+type JavaSqlTimeSerializer struct {
+}
+
+func (JavaSqlTimeSerializer) EncObject(e *Encoder, vv POJO) error {
Review comment:
add comment
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] wongoo commented on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
wongoo commented on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674747804
NOT AGREE to add the Date&Time definition in hessian package, and there already java8_time package in which it contains date and time definition. If it's really necessary , suggestion to move to a new package `java_sql_time`.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674791927
> NOT AGREE to add the Date&Time definition in hessian package, and there already java8_time package in which it contains date and time definition. If it's really necessary , suggestion to move to a new package `java_sql_time`.
good suggestion.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r471177379
##########
File path: java_sql_time_test.go
##########
@@ -0,0 +1,97 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "github.com/stretchr/testify/assert"
Review comment:
Can you tell me in more detail
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674791505
> java_sql_time
good suggestion.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] AlexStocks commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
AlexStocks commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r471488169
##########
File path: java_sql_time_test.go
##########
@@ -0,0 +1,97 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "github.com/stretchr/testify/assert"
Review comment:
what I mean is u should split the import into two parts as other dubbogo files.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] AlexStocks closed pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
AlexStocks closed pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zouyx commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zouyx commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r479659363
##########
File path: java_sql_time/java_sql_time.go
##########
@@ -0,0 +1,30 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package java_sql_time
+
+import "time"
+
+type JavaSqlTime interface {
+ // ValueOf parse time string which format likes '2006-01-02 15:04:05'
+ ValueOf(timeStr string) error
+ // SetTime for decode time
+ SetTime(time time.Time)
+ JavaClassName() string
Review comment:
Should fix this problem?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r471523136
##########
File path: java_sql_time_test.go
##########
@@ -0,0 +1,97 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "github.com/stretchr/testify/assert"
Review comment:
> what I mean is u should split the import into two parts as other dubbogo files.
ok
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 commented on a change in pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 commented on a change in pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#discussion_r475300346
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,152 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+import (
+ "github.com/apache/dubbo-go-hessian2/java_sql_time"
+)
+
+func init() {
+ RegisterPOJO(&java_sql_time.Date{})
+ RegisterPOJO(&java_sql_time.Time{})
+}
+
+var javaSqlTimeTypeMap = make(map[string]reflect.Type, 16)
Review comment:
LGTM
##########
File path: java_sql_time.go
##########
@@ -0,0 +1,152 @@
+/*
+ * Licensed to the Apache Software Foundation (ASF) under one or more
+ * contributor license agreements. See the NOTICE file distributed with
+ * this work for additional information regarding copyright ownership.
+ * The ASF licenses this file to You under the Apache License, Version 2.0
+ * (the "License"); you may not use this file except in compliance with
+ * the License. You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ */
+
+package hessian
+
+import (
+ "io"
+ "reflect"
+)
+
+import (
+ perrors "github.com/pkg/errors"
+)
+
+import (
+ "github.com/apache/dubbo-go-hessian2/java_sql_time"
+)
+
+func init() {
+ RegisterPOJO(&java_sql_time.Date{})
+ RegisterPOJO(&java_sql_time.Time{})
Review comment:
LGTM
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org
[GitHub] [dubbo-go-hessian2] zhangshen023 removed a comment on pull request #219: java.sql.Time & java.sql.Date
Posted by GitBox <gi...@apache.org>.
zhangshen023 removed a comment on pull request #219:
URL: https://github.com/apache/dubbo-go-hessian2/pull/219#issuecomment-674791505
> java_sql_time
good suggestion.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
---------------------------------------------------------------------
To unsubscribe, e-mail: notifications-unsubscribe@dubbo.apache.org
For additional commands, e-mail: notifications-help@dubbo.apache.org