You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@cordova.apache.org by st...@apache.org on 2016/03/04 00:54:41 UTC
[01/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Repository: cordova-osx
Updated Branches:
refs/heads/4.0.x c81645cf4 -> a8e7d75aa
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/src/chmod.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/chmod.js b/node_modules/shelljs/src/chmod.js
new file mode 100644
index 0000000..6c6de10
--- /dev/null
+++ b/node_modules/shelljs/src/chmod.js
@@ -0,0 +1,215 @@
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+var PERMS = (function (base) {
+ return {
+ OTHER_EXEC : base.EXEC,
+ OTHER_WRITE : base.WRITE,
+ OTHER_READ : base.READ,
+
+ GROUP_EXEC : base.EXEC << 3,
+ GROUP_WRITE : base.WRITE << 3,
+ GROUP_READ : base.READ << 3,
+
+ OWNER_EXEC : base.EXEC << 6,
+ OWNER_WRITE : base.WRITE << 6,
+ OWNER_READ : base.READ << 6,
+
+ // Literal octal numbers are apparently not allowed in "strict" javascript. Using parseInt is
+ // the preferred way, else a jshint warning is thrown.
+ STICKY : parseInt('01000', 8),
+ SETGID : parseInt('02000', 8),
+ SETUID : parseInt('04000', 8),
+
+ TYPE_MASK : parseInt('0770000', 8)
+ };
+})({
+ EXEC : 1,
+ WRITE : 2,
+ READ : 4
+});
+
+//@
+//@ ### chmod(octal_mode || octal_string, file)
+//@ ### chmod(symbolic_mode, file)
+//@
+//@ Available options:
+//@
+//@ + `-v`: output a diagnostic for every file processed//@
+//@ + `-c`: like verbose but report only when a change is made//@
+//@ + `-R`: change files and directories recursively//@
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ chmod(755, '/Users/brandon');
+//@ chmod('755', '/Users/brandon'); // same as above
+//@ chmod('u+x', '/Users/brandon');
+//@ ```
+//@
+//@ Alters the permissions of a file or directory by either specifying the
+//@ absolute permissions in octal form or expressing the changes in symbols.
+//@ This command tries to mimic the POSIX behavior as much as possible.
+//@ Notable exceptions:
+//@
+//@ + In symbolic modes, 'a-r' and '-r' are identical. No consideration is
+//@ given to the umask.
+//@ + There is no "quiet" option since default behavior is to run silent.
+function _chmod(options, mode, filePattern) {
+ if (!filePattern) {
+ if (options.length > 0 && options.charAt(0) === '-') {
+ // Special case where the specified file permissions started with - to subtract perms, which
+ // get picked up by the option parser as command flags.
+ // If we are down by one argument and options starts with -, shift everything over.
+ filePattern = mode;
+ mode = options;
+ options = '';
+ }
+ else {
+ common.error('You must specify a file.');
+ }
+ }
+
+ options = common.parseOptions(options, {
+ 'R': 'recursive',
+ 'c': 'changes',
+ 'v': 'verbose'
+ });
+
+ if (typeof filePattern === 'string') {
+ filePattern = [ filePattern ];
+ }
+
+ var files;
+
+ if (options.recursive) {
+ files = [];
+ common.expand(filePattern).forEach(function addFile(expandedFile) {
+ var stat = fs.lstatSync(expandedFile);
+
+ if (!stat.isSymbolicLink()) {
+ files.push(expandedFile);
+
+ if (stat.isDirectory()) { // intentionally does not follow symlinks.
+ fs.readdirSync(expandedFile).forEach(function (child) {
+ addFile(expandedFile + '/' + child);
+ });
+ }
+ }
+ });
+ }
+ else {
+ files = common.expand(filePattern);
+ }
+
+ files.forEach(function innerChmod(file) {
+ file = path.resolve(file);
+ if (!fs.existsSync(file)) {
+ common.error('File not found: ' + file);
+ }
+
+ // When recursing, don't follow symlinks.
+ if (options.recursive && fs.lstatSync(file).isSymbolicLink()) {
+ return;
+ }
+
+ var stat = fs.statSync(file);
+ var isDir = stat.isDirectory();
+ var perms = stat.mode;
+ var type = perms & PERMS.TYPE_MASK;
+
+ var newPerms = perms;
+
+ if (isNaN(parseInt(mode, 8))) {
+ // parse options
+ mode.split(',').forEach(function (symbolicMode) {
+ /*jshint regexdash:true */
+ var pattern = /([ugoa]*)([=\+-])([rwxXst]*)/i;
+ var matches = pattern.exec(symbolicMode);
+
+ if (matches) {
+ var applyTo = matches[1];
+ var operator = matches[2];
+ var change = matches[3];
+
+ var changeOwner = applyTo.indexOf('u') != -1 || applyTo === 'a' || applyTo === '';
+ var changeGroup = applyTo.indexOf('g') != -1 || applyTo === 'a' || applyTo === '';
+ var changeOther = applyTo.indexOf('o') != -1 || applyTo === 'a' || applyTo === '';
+
+ var changeRead = change.indexOf('r') != -1;
+ var changeWrite = change.indexOf('w') != -1;
+ var changeExec = change.indexOf('x') != -1;
+ var changeExecDir = change.indexOf('X') != -1;
+ var changeSticky = change.indexOf('t') != -1;
+ var changeSetuid = change.indexOf('s') != -1;
+
+ if (changeExecDir && isDir)
+ changeExec = true;
+
+ var mask = 0;
+ if (changeOwner) {
+ mask |= (changeRead ? PERMS.OWNER_READ : 0) + (changeWrite ? PERMS.OWNER_WRITE : 0) + (changeExec ? PERMS.OWNER_EXEC : 0) + (changeSetuid ? PERMS.SETUID : 0);
+ }
+ if (changeGroup) {
+ mask |= (changeRead ? PERMS.GROUP_READ : 0) + (changeWrite ? PERMS.GROUP_WRITE : 0) + (changeExec ? PERMS.GROUP_EXEC : 0) + (changeSetuid ? PERMS.SETGID : 0);
+ }
+ if (changeOther) {
+ mask |= (changeRead ? PERMS.OTHER_READ : 0) + (changeWrite ? PERMS.OTHER_WRITE : 0) + (changeExec ? PERMS.OTHER_EXEC : 0);
+ }
+
+ // Sticky bit is special - it's not tied to user, group or other.
+ if (changeSticky) {
+ mask |= PERMS.STICKY;
+ }
+
+ switch (operator) {
+ case '+':
+ newPerms |= mask;
+ break;
+
+ case '-':
+ newPerms &= ~mask;
+ break;
+
+ case '=':
+ newPerms = type + mask;
+
+ // According to POSIX, when using = to explicitly set the permissions, setuid and setgid can never be cleared.
+ if (fs.statSync(file).isDirectory()) {
+ newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
+ }
+ break;
+ }
+
+ if (options.verbose) {
+ console.log(file + ' -> ' + newPerms.toString(8));
+ }
+
+ if (perms != newPerms) {
+ if (!options.verbose && options.changes) {
+ console.log(file + ' -> ' + newPerms.toString(8));
+ }
+ fs.chmodSync(file, newPerms);
+ perms = newPerms; // for the next round of changes!
+ }
+ }
+ else {
+ common.error('Invalid symbolic mode change: ' + symbolicMode);
+ }
+ });
+ }
+ else {
+ // they gave us a full number
+ newPerms = type + parseInt(mode, 8);
+
+ // POSIX rules are that setuid and setgid can only be added using numeric form, but not cleared.
+ if (fs.statSync(file).isDirectory()) {
+ newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
+ }
+
+ fs.chmodSync(file, newPerms);
+ }
+ });
+}
+module.exports = _chmod;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[03/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/workspace.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/workspace.xml b/node_modules/shelljs/.idea/workspace.xml
new file mode 100644
index 0000000..9247ca4
--- /dev/null
+++ b/node_modules/shelljs/.idea/workspace.xml
@@ -0,0 +1,764 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<project version="4">
+ <component name="ChangeListManager">
+ <list default="true" id="45933638-88e3-4f2a-b91c-9922c650f3b5" name="Default" comment="" />
+ <ignored path="shelljs.iws" />
+ <ignored path=".idea/workspace.xml" />
+ <option name="EXCLUDED_CONVERTED_TO_IGNORED" value="true" />
+ <option name="TRACKING_ENABLED" value="true" />
+ <option name="SHOW_DIALOG" value="false" />
+ <option name="HIGHLIGHT_CONFLICTS" value="true" />
+ <option name="HIGHLIGHT_NON_ACTIVE_CHANGELIST" value="false" />
+ <option name="LAST_RESOLUTION" value="IGNORE" />
+ </component>
+ <component name="ChangesViewManager" flattened_view="true" show_ignored="false" />
+ <component name="CreatePatchCommitExecutor">
+ <option name="PATCH_PATH" value="" />
+ </component>
+ <component name="ExecutionTargetManager" SELECTED_TARGET="default_target" />
+ <component name="FavoritesManager">
+ <favorites_list name="shelljs" />
+ </component>
+ <component name="FileEditorManager">
+ <leaf SIDE_TABS_SIZE_LIMIT_KEY="300">
+ <file leaf-file-name="common.js" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/src/common.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="104" column="7" selection-start-line="104" selection-start-column="7" selection-end-line="104" selection-end-column="7" />
+ <folding>
+ <marker date="1454630861000" expanded="true" signature="1550:1605" placeholder="//..." />
+ <marker date="1454630861000" expanded="true" signature="6173:6214" placeholder="//..." />
+ <marker date="1454630861000" expanded="true" signature="6173:6520" placeholder="{...}" />
+ <marker date="1454630861000" expanded="true" signature="6173:6891" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name="RELEASE.md" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/RELEASE.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="-0.0">
+ <caret line="0" column="15" selection-start-line="0" selection-start-column="15" selection-end-line="0" selection-end-column="15" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name="package.json" pinned="false" current-in-tab="true">
+ <entry file="file://$PROJECT_DIR$/package.json">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.7058824">
+ <caret line="40" column="0" selection-start-line="40" selection-start-column="0" selection-end-line="40" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name=".npmignore" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/.npmignore">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="9" column="0" selection-start-line="9" selection-start-column="0" selection-end-line="9" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name="README.md" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/README.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="-10.928572">
+ <caret line="72" column="15" selection-start-line="72" selection-start-column="15" selection-end-line="72" selection-end-column="15" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name="shjs" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/bin/shjs">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name="output.js" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/build/output.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </file>
+ <file leaf-file-name="cat.js" pinned="false" current-in-tab="false">
+ <entry file="file://$PROJECT_DIR$/src/cat.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="38" column="0" selection-start-line="38" selection-start-column="0" selection-end-line="38" selection-end-column="0" />
+ <folding>
+ <marker date="1454628318000" expanded="true" signature="59:506" placeholder="//..." />
+ <marker date="1454628318000" expanded="true" signature="537:958" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ </file>
+ </leaf>
+ </component>
+ <component name="Git.Settings">
+ <option name="RECENT_GIT_ROOT_PATH" value="$PROJECT_DIR$" />
+ </component>
+ <component name="IdeDocumentHistory">
+ <option name="CHANGED_PATHS">
+ <list>
+ <option value="$PROJECT_DIR$/.eslintrc" />
+ <option value="$PROJECT_DIR$/CONTRIBUTING.md" />
+ <option value="$PROJECT_DIR$/scripts/run-tests.js" />
+ <option value="$PROJECT_DIR$/src/rm.js" />
+ <option value="$PROJECT_DIR$/test/resources/issue44/main.js" />
+ <option value="$PROJECT_DIR$/test/ln.js" />
+ <option value="$PROJECT_DIR$/test/common.js" />
+ <option value="$PROJECT_DIR$/src/ln.js" />
+ <option value="$PROJECT_DIR$/src/ls.js" />
+ <option value="$PROJECT_DIR$/test/which.js" />
+ <option value="$PROJECT_DIR$/.eslintrc.json" />
+ <option value="$PROJECT_DIR$/src/cat.js" />
+ <option value="$PROJECT_DIR$/src/common.js" />
+ <option value="$PROJECT_DIR$/test/TODO" />
+ <option value="$PROJECT_DIR$/CHANGELOG.md" />
+ <option value="$PROJECT_DIR$/maked.js" />
+ <option value="$PROJECT_DIR$/docs/my_docs.md" />
+ <option value="$PROJECT_DIR$/.npmignore" />
+ <option value="$PROJECT_DIR$/m.js" />
+ <option value="$PROJECT_DIR$/README.md" />
+ <option value="$PROJECT_DIR$/package.json" />
+ </list>
+ </option>
+ </component>
+ <component name="JsBuildToolGruntFileManager" detection-done="true" />
+ <component name="JsBuildToolPackageJson" detection-done="true">
+ <package-json value="$PROJECT_DIR$/package.json" />
+ </component>
+ <component name="JsGulpfileManager">
+ <detection-done>true</detection-done>
+ </component>
+ <component name="ProjectFrameBounds">
+ <option name="width" value="1920" />
+ <option name="height" value="1200" />
+ </component>
+ <component name="ProjectLevelVcsManager" settingsEditedManually="true">
+ <OptionsSetting value="true" id="Add" />
+ <OptionsSetting value="true" id="Remove" />
+ <OptionsSetting value="true" id="Checkout" />
+ <OptionsSetting value="true" id="Update" />
+ <OptionsSetting value="true" id="Status" />
+ <OptionsSetting value="true" id="Edit" />
+ <ConfirmationsSetting value="0" id="Add" />
+ <ConfirmationsSetting value="0" id="Remove" />
+ </component>
+ <component name="ProjectView">
+ <navigator currentView="ProjectPane" proportions="" version="1">
+ <flattenPackages />
+ <showMembers />
+ <showModules />
+ <showLibraryContents />
+ <hideEmptyPackages />
+ <abbreviatePackageNames />
+ <autoscrollToSource />
+ <autoscrollFromSource />
+ <sortByType />
+ <manualOrder />
+ <foldersAlwaysOnTop value="true" />
+ </navigator>
+ <panes>
+ <pane id="ProjectPane">
+ <subPane>
+ <PATH>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
+ </PATH_ELEMENT>
+ </PATH>
+ <PATH>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
+ </PATH_ELEMENT>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
+ </PATH_ELEMENT>
+ </PATH>
+ <PATH>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
+ </PATH_ELEMENT>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
+ </PATH_ELEMENT>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="build" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
+ </PATH_ELEMENT>
+ </PATH>
+ <PATH>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
+ </PATH_ELEMENT>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="shelljs" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
+ </PATH_ELEMENT>
+ <PATH_ELEMENT>
+ <option name="myItemId" value="bin" />
+ <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
+ </PATH_ELEMENT>
+ </PATH>
+ </subPane>
+ </pane>
+ <pane id="Scratches" />
+ <pane id="Scope" />
+ </panes>
+ </component>
+ <component name="PropertiesComponent">
+ <property name="settings.editor.selected.configurable" value="Markdown" />
+ <property name="settings.editor.splitter.proportion" value="0.2" />
+ <property name="last_opened_file_path" value="$PROJECT_DIR$" />
+ <property name="WebServerToolWindowFactoryState" value="false" />
+ <property name="js-jscs-nodeInterpreter" value="$USER_HOME$/n/bin/node" />
+ <property name="HbShouldOpenHtmlAsHb" value="" />
+ <property name="jsx.switch.disabled" value="true" />
+ <property name="FullScreen" value="true" />
+ </component>
+ <component name="RunManager">
+ <configuration default="true" type="BashConfigurationType" factoryName="Bash">
+ <option name="INTERPRETER_OPTIONS" value="" />
+ <option name="INTERPRETER_PATH" value="/bin/bash" />
+ <option name="WORKING_DIRECTORY" value="" />
+ <option name="PARENT_ENVS" value="true" />
+ <option name="SCRIPT_NAME" value="" />
+ <option name="PARAMETERS" value="" />
+ <module name="" />
+ <envs />
+ <method />
+ </configuration>
+ <configuration default="true" type="DartCommandLineRunConfigurationType" factoryName="Dart Command Line Application">
+ <method />
+ </configuration>
+ <configuration default="true" type="DartTestRunConfigurationType" factoryName="Dart Test">
+ <method />
+ </configuration>
+ <configuration default="true" type="JavaScriptTestRunnerKarma" factoryName="Karma" config-file="">
+ <envs />
+ <method />
+ </configuration>
+ <configuration default="true" type="JavascriptDebugType" factoryName="JavaScript Debug">
+ <method />
+ </configuration>
+ <configuration default="true" type="NodeJSConfigurationType" factoryName="Node.js" working-dir="">
+ <method />
+ </configuration>
+ <configuration default="true" type="cucumber.js" factoryName="Cucumber.js">
+ <option name="cucumberJsArguments" value="" />
+ <option name="executablePath" />
+ <option name="filePath" />
+ <method />
+ </configuration>
+ <configuration default="true" type="js.build_tools.gulp" factoryName="Gulp.js">
+ <node-options />
+ <gulpfile />
+ <tasks />
+ <arguments />
+ <envs />
+ <method />
+ </configuration>
+ <configuration default="true" type="js.build_tools.npm" factoryName="npm">
+ <command value="run-script" />
+ <scripts />
+ <envs />
+ <method />
+ </configuration>
+ <configuration default="true" type="mocha-javascript-test-runner" factoryName="Mocha">
+ <node-options />
+ <working-directory>$PROJECT_DIR$</working-directory>
+ <pass-parent-env>true</pass-parent-env>
+ <envs />
+ <ui>bdd</ui>
+ <extra-mocha-options />
+ <test-kind>DIRECTORY</test-kind>
+ <test-directory />
+ <recursive>false</recursive>
+ <method />
+ </configuration>
+ </component>
+ <component name="ShelveChangesManager" show_recycled="false" />
+ <component name="SvnConfiguration">
+ <configuration />
+ </component>
+ <component name="TaskManager">
+ <task active="true" id="Default" summary="Default task">
+ <changelist id="45933638-88e3-4f2a-b91c-9922c650f3b5" name="Default" comment="" />
+ <created>1453951651105</created>
+ <option name="number" value="Default" />
+ <updated>1453951651105</updated>
+ </task>
+ <servers />
+ </component>
+ <component name="ToolWindowManager">
+ <frame x="0" y="0" width="1920" height="1200" extended-state="6" />
+ <editor active="false" />
+ <layout>
+ <window_info id="Project" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="true" show_stripe_button="true" weight="0.15745129" sideWeight="0.5" order="0" side_tool="false" content_ui="tabs" />
+ <window_info id="TODO" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="6" side_tool="false" content_ui="tabs" />
+ <window_info id="Event Log" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="7" side_tool="true" content_ui="tabs" />
+ <window_info id="npm" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="2" side_tool="true" content_ui="tabs" />
+ <window_info id="Version Control" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="7" side_tool="false" content_ui="tabs" />
+ <window_info id="Run" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="2" side_tool="false" content_ui="tabs" />
+ <window_info id="Structure" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="1" side_tool="false" content_ui="tabs" />
+ <window_info id="Terminal" active="true" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="true" show_stripe_button="true" weight="0.20318021" sideWeight="0.5" order="7" side_tool="false" content_ui="tabs" />
+ <window_info id="Favorites" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="2" side_tool="true" content_ui="tabs" />
+ <window_info id="Debug" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.4" sideWeight="0.5" order="3" side_tool="false" content_ui="tabs" />
+ <window_info id="Cvs" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="4" side_tool="false" content_ui="tabs" />
+ <window_info id="Message" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="0" side_tool="false" content_ui="tabs" />
+ <window_info id="Commander" active="false" anchor="right" auto_hide="false" internal_type="SLIDING" type="SLIDING" visible="false" show_stripe_button="true" weight="0.4" sideWeight="0.5" order="0" side_tool="false" content_ui="tabs" />
+ <window_info id="Inspection" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.4" sideWeight="0.5" order="5" side_tool="false" content_ui="tabs" />
+ <window_info id="Hierarchy" active="false" anchor="right" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="2" side_tool="false" content_ui="combo" />
+ <window_info id="Find" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="1" side_tool="false" content_ui="tabs" />
+ <window_info id="Ant Build" active="false" anchor="right" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="1" side_tool="false" content_ui="tabs" />
+ </layout>
+ </component>
+ <component name="Vcs.Log.UiProperties">
+ <option name="RECENTLY_FILTERED_USER_GROUPS">
+ <collection />
+ </option>
+ <option name="RECENTLY_FILTERED_BRANCH_GROUPS">
+ <collection />
+ </option>
+ </component>
+ <component name="VcsContentAnnotationSettings">
+ <option name="myLimit" value="2678400000" />
+ </component>
+ <component name="XDebuggerManager">
+ <breakpoint-manager />
+ <watches-manager />
+ </component>
+ <component name="editorHistoryManager">
+ <entry file="file://$PROJECT_DIR$/src/common.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ <folding>
+ <marker date="1454630861000" expanded="true" signature="1550:1605" placeholder="//..." />
+ <marker date="1454630861000" expanded="true" signature="6173:6214" placeholder="//..." />
+ <marker date="1454630861000" expanded="true" signature="6173:6520" placeholder="{...}" />
+ <marker date="1454630861000" expanded="true" signature="6173:6891" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/shell.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/global.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/.gitignore">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="2" column="0" selection-start-line="2" selection-start-column="0" selection-end-line="2" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/which.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/RELEASE.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/package.json">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="29" column="23" selection-start-line="29" selection-start-column="23" selection-end-line="29" selection-end-column="23" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/README.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="43" column="0" selection-start-line="43" selection-start-column="0" selection-end-line="43" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/scripts/generate-docs.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/scripts/run-tests.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="26" column="50" selection-start-line="26" selection-start-column="50" selection-end-line="26" selection-end-column="50" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/shell.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/global.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/.gitignore">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="2" column="0" selection-start-line="2" selection-start-column="0" selection-end-line="2" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/which.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/RELEASE.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/package.json">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="29" column="23" selection-start-line="29" selection-start-column="23" selection-end-line="29" selection-end-column="23" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/README.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="43" column="0" selection-start-line="43" selection-start-column="0" selection-end-line="43" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/scripts/generate-docs.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/scripts/run-tests.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="26" column="50" selection-start-line="26" selection-start-column="50" selection-end-line="26" selection-end-column="50" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/shell.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/global.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/.gitignore">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/.gitignore">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="2" column="0" selection-start-line="2" selection-start-column="0" selection-end-line="2" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/scripts/generate-docs.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/sed.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="1" column="0" selection-start-line="1" selection-start-column="0" selection-end-line="1" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/grep.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/shell.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/global.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/sed.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="1" column="0" selection-start-line="1" selection-start-column="0" selection-end-line="1" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/pwd.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/scripts/run-tests.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="-0.17814727">
+ <caret line="24" column="16" selection-start-line="24" selection-start-column="16" selection-end-line="24" selection-end-column="16" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/rm.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="189" column="0" selection-start-line="189" selection-start-column="0" selection-end-line="189" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/node_modules/glob/README.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="30.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/rm.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="137" column="25" selection-start-line="137" selection-start-column="25" selection-end-line="137" selection-end-column="25" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/resources/issue44/main.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="1" column="0" selection-start-line="1" selection-start-column="0" selection-end-line="1" selection-end-column="0" />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/ln.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="20" column="1" selection-start-line="20" selection-start-column="1" selection-end-line="20" selection-end-column="1" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/common.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="46" column="10" selection-start-line="46" selection-start-column="10" selection-end-line="46" selection-end-column="10" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/ls.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="15" column="18" selection-start-line="15" selection-start-column="18" selection-end-line="15" selection-end-column="18" />
+ <folding>
+ <marker date="1454625654000" expanded="true" signature="11066:11146" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/ln.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="48" column="9" selection-start-line="48" selection-start-column="9" selection-end-line="48" selection-end-column="9" />
+ <folding>
+ <marker date="1454625654000" expanded="true" signature="1476:1565" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/ls.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="168" column="0" selection-start-line="168" selection-start-column="0" selection-end-line="168" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/test/which.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="19" column="0" selection-start-line="19" selection-start-column="0" selection-end-line="19" selection-end-column="0" />
+ <folding>
+ <marker date="1454625654000" expanded="true" signature="711:743" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/cat.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="38" column="0" selection-start-line="38" selection-start-column="0" selection-end-line="38" selection-end-column="0" />
+ <folding>
+ <marker date="1454628318000" expanded="true" signature="59:506" placeholder="//..." />
+ <marker date="1454628318000" expanded="true" signature="537:958" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/src/common.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="104" column="7" selection-start-line="104" selection-start-column="7" selection-end-line="104" selection-end-column="7" />
+ <folding>
+ <marker date="1454630861000" expanded="true" signature="1550:1605" placeholder="//..." />
+ <marker date="1454630861000" expanded="true" signature="6173:6214" placeholder="//..." />
+ <marker date="1454630861000" expanded="true" signature="6173:6520" placeholder="{...}" />
+ <marker date="1454630861000" expanded="true" signature="6173:6891" placeholder="{...}" />
+ </folding>
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/.npmignore">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="9" column="0" selection-start-line="9" selection-start-column="0" selection-end-line="9" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/RELEASE.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="-0.0">
+ <caret line="0" column="15" selection-start-line="0" selection-start-column="15" selection-end-line="0" selection-end-column="15" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/bin/shjs">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/build/output.js">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.0">
+ <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/README.md">
+ <provider editor-type-id="MarkdownPreviewEditor">
+ <state />
+ </provider>
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="-10.928572">
+ <caret line="72" column="15" selection-start-line="72" selection-start-column="15" selection-end-line="72" selection-end-column="15" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ <entry file="file://$PROJECT_DIR$/package.json">
+ <provider selected="true" editor-type-id="text-editor">
+ <state vertical-scroll-proportion="0.7058824">
+ <caret line="40" column="0" selection-start-line="40" selection-start-column="0" selection-end-line="40" selection-end-column="0" />
+ <folding />
+ </state>
+ </provider>
+ </entry>
+ </component>
+</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.npmignore b/node_modules/shelljs/.npmignore
new file mode 100644
index 0000000..8b693ff
--- /dev/null
+++ b/node_modules/shelljs/.npmignore
@@ -0,0 +1,9 @@
+test/
+tmp/
+.documentup.json
+.gitignore
+.jshintrc
+.lgtm
+.travis.yml
+appveyor.yml
+RELEASE.md
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/LICENSE b/node_modules/shelljs/LICENSE
new file mode 100644
index 0000000..0f0f119
--- /dev/null
+++ b/node_modules/shelljs/LICENSE
@@ -0,0 +1,26 @@
+Copyright (c) 2012, Artur Adib <ar...@gmail.com>
+All rights reserved.
+
+You may use this project under the terms of the New BSD license as follows:
+
+Redistribution and use in source and binary forms, with or without
+modification, are permitted provided that the following conditions are met:
+ * Redistributions of source code must retain the above copyright
+ notice, this list of conditions and the following disclaimer.
+ * Redistributions in binary form must reproduce the above copyright
+ notice, this list of conditions and the following disclaimer in the
+ documentation and/or other materials provided with the distribution.
+ * Neither the name of Artur Adib nor the
+ names of the contributors may be used to endorse or promote products
+ derived from this software without specific prior written permission.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+ARE DISCLAIMED. IN NO EVENT SHALL ARTUR ADIB BE LIABLE FOR ANY
+DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
+(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
+LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
+ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
+(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
+THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/MAINTAINERS
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/MAINTAINERS b/node_modules/shelljs/MAINTAINERS
new file mode 100644
index 0000000..3f94761
--- /dev/null
+++ b/node_modules/shelljs/MAINTAINERS
@@ -0,0 +1,3 @@
+Ari Porad <ar...@ariporad.com> (@ariporad)
+Nate Fischer <nt...@gmail.com> (@nfischer)
+Artur Adib <ar...@gmail.com> (@arturadib)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/README.md
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/README.md b/node_modules/shelljs/README.md
new file mode 100644
index 0000000..d6dcb63
--- /dev/null
+++ b/node_modules/shelljs/README.md
@@ -0,0 +1,658 @@
+# ShellJS - Unix shell commands for Node.js
+
+[](https://gitter.im/shelljs/shelljs?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
+[](http://travis-ci.org/shelljs/shelljs)
+[](https://ci.appveyor.com/project/shelljs/shelljs)
+
+ShellJS is a portable **(Windows/Linux/OS X)** implementation of Unix shell commands on top of the Node.js API. You can use it to eliminate your shell script's dependency on Unix while still keeping its familiar and powerful commands. You can also install it globally so you can run it from outside Node projects - say goodbye to those gnarly Bash scripts!
+
+The project is [unit-tested](http://travis-ci.org/shelljs/shelljs) and battled-tested in projects like:
+
++ [PDF.js](http://github.com/mozilla/pdf.js) - Firefox's next-gen PDF reader
++ [Firebug](http://getfirebug.com/) - Firefox's infamous debugger
++ [JSHint](http://jshint.com) - Most popular JavaScript linter
++ [Zepto](http://zeptojs.com) - jQuery-compatible JavaScript library for modern browsers
++ [Yeoman](http://yeoman.io/) - Web application stack and development tool
++ [Deployd.com](http://deployd.com) - Open source PaaS for quick API backend generation
+
+and [many more](https://npmjs.org/browse/depended/shelljs).
+
+If you have feedback, suggestions, or need help, feel free to post in our [issue tracker](https://github.com/shelljs/shelljs/issues).
+
+## Installing
+
+Via npm:
+
+```bash
+$ npm install [-g] shelljs
+```
+
+If the global option `-g` is specified, the binary `shjs` will be installed. This makes it possible to
+run ShellJS scripts much like any shell script from the command line, i.e. without requiring a `node_modules` folder:
+
+```bash
+$ shjs my_script
+```
+
+## Examples
+
+### JavaScript
+
+```javascript
+require('shelljs/global');
+
+if (!which('git')) {
+ echo('Sorry, this script requires git');
+ exit(1);
+}
+
+// Copy files to release dir
+mkdir('-p', 'out/Release');
+cp('-R', 'stuff/*', 'out/Release');
+
+// Replace macros in each .js file
+cd('lib');
+ls('*.js').forEach(function(file) {
+ sed('-i', 'BUILD_VERSION', 'v0.1.2', file);
+ sed('-i', /.*REMOVE_THIS_LINE.*\n/, '', file);
+ sed('-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file);
+});
+cd('..');
+
+// Run external tool synchronously
+if (exec('git commit -am "Auto-commit"').code !== 0) {
+ echo('Error: Git commit failed');
+ exit(1);
+}
+```
+
+### CoffeeScript
+
+CoffeeScript is also supported automatically:
+
+```coffeescript
+require 'shelljs/global'
+
+if not which 'git'
+ echo 'Sorry, this script requires git'
+ exit 1
+
+# Copy files to release dir
+mkdir '-p', 'out/Release'
+cp '-R', 'stuff/*', 'out/Release'
+
+# Replace macros in each .js file
+cd 'lib'
+for file in ls '*.js'
+ sed '-i', 'BUILD_VERSION', 'v0.1.2', file
+ sed '-i', /.*REMOVE_THIS_LINE.*\n/, '', file
+ sed '-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file
+cd '..'
+
+# Run external tool synchronously
+if (exec 'git commit -am "Auto-commit"').code != 0
+ echo 'Error: Git commit failed'
+ exit 1
+```
+
+## Global vs. Local
+
+The example above uses the convenience script `shelljs/global` to reduce verbosity. If polluting your global namespace is not desirable, simply require `shelljs`.
+
+Example:
+
+```javascript
+var shell = require('shelljs');
+shell.echo('hello world');
+```
+
+## Make tool
+
+A convenience script `shelljs/make` is also provided to mimic the behavior of a Unix Makefile.
+In this case all shell objects are global, and command line arguments will cause the script to
+execute only the corresponding function in the global `target` object. To avoid redundant calls,
+target functions are executed only once per script.
+
+Example:
+
+```javascript
+require('shelljs/make');
+
+target.all = function() {
+ target.bundle();
+ target.docs();
+};
+
+target.bundle = function() {
+ cd(__dirname);
+ mkdir('-p', 'build');
+ cd('src');
+ cat('*.js').to('../build/output.js');
+};
+
+target.docs = function() {
+ cd(__dirname);
+ mkdir('-p', 'docs');
+ var files = ls('src/*.js');
+ for(var i = 0; i < files.length; i++) {
+ var text = grep('//@', files[i]); // extract special comments
+ text = text.replace(/\/\/@/g, ''); // remove comment tags
+ text.toEnd('docs/my_docs.md');
+ }
+};
+```
+
+To run the target `all`, call the above script without arguments: `$ node make`. To run the target `docs`: `$ node make docs`.
+
+You can also pass arguments to your targets by using the `--` separator. For example, to pass `arg1` and `arg2` to a target `bundle`, do `$ node make bundle -- arg1 arg2`:
+
+```javascript
+require('shelljs/make');
+
+target.bundle = function(argsArray) {
+ // argsArray = ['arg1', 'arg2']
+ /* ... */
+}
+```
+
+
+<!-- DO NOT MODIFY BEYOND THIS POINT - IT'S AUTOMATICALLY GENERATED -->
+
+
+## Command reference
+
+
+All commands run synchronously, unless otherwise stated.
+
+
+### cd([dir])
+Changes to directory `dir` for the duration of the script. Changes to home
+directory if no argument is supplied.
+
+
+### pwd()
+Returns the current directory.
+
+
+### ls([options,] [path, ...])
+### ls([options,] path_array)
+Available options:
+
++ `-R`: recursive
++ `-A`: all files (include files beginning with `.`, except for `.` and `..`)
++ `-d`: list directories themselves, not their contents
++ `-l`: list objects representing each file, each with fields containing `ls
+ -l` output fields. See
+ [fs.Stats](https://nodejs.org/api/fs.html#fs_class_fs_stats)
+ for more info
+
+Examples:
+
+```javascript
+ls('projs/*.js');
+ls('-R', '/users/me', '/tmp');
+ls('-R', ['/users/me', '/tmp']); // same as above
+ls('-l', 'file.txt'); // { name: 'file.txt', mode: 33188, nlink: 1, ...}
+```
+
+Returns array of files in the given path, or in current directory if no path provided.
+
+
+### find(path [, path ...])
+### find(path_array)
+Examples:
+
+```javascript
+find('src', 'lib');
+find(['src', 'lib']); // same as above
+find('.').filter(function(file) { return file.match(/\.js$/); });
+```
+
+Returns array of all files (however deep) in the given paths.
+
+The main difference from `ls('-R', path)` is that the resulting file names
+include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
+
+
+### cp([options,] source [, source ...], dest)
+### cp([options,] source_array, dest)
+Available options:
+
++ `-f`: force (default behavior)
++ `-n`: no-clobber
++ `-r, -R`: recursive
+
+Examples:
+
+```javascript
+cp('file1', 'dir1');
+cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
+cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
+```
+
+Copies files. The wildcard `*` is accepted.
+
+
+### rm([options,] file [, file ...])
+### rm([options,] file_array)
+Available options:
+
++ `-f`: force
++ `-r, -R`: recursive
+
+Examples:
+
+```javascript
+rm('-rf', '/tmp/*');
+rm('some_file.txt', 'another_file.txt');
+rm(['some_file.txt', 'another_file.txt']); // same as above
+```
+
+Removes files. The wildcard `*` is accepted.
+
+
+### mv([options ,] source [, source ...], dest')
+### mv([options ,] source_array, dest')
+Available options:
+
++ `-f`: force (default behavior)
++ `-n`: no-clobber
+
+Examples:
+
+```javascript
+mv('-n', 'file', 'dir/');
+mv('file1', 'file2', 'dir/');
+mv(['file1', 'file2'], 'dir/'); // same as above
+```
+
+Moves files. The wildcard `*` is accepted.
+
+
+### mkdir([options,] dir [, dir ...])
+### mkdir([options,] dir_array)
+Available options:
+
++ `-p`: full path (will create intermediate dirs if necessary)
+
+Examples:
+
+```javascript
+mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
+mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
+```
+
+Creates directories.
+
+
+### test(expression)
+Available expression primaries:
+
++ `'-b', 'path'`: true if path is a block device
++ `'-c', 'path'`: true if path is a character device
++ `'-d', 'path'`: true if path is a directory
++ `'-e', 'path'`: true if path exists
++ `'-f', 'path'`: true if path is a regular file
++ `'-L', 'path'`: true if path is a symbolic link
++ `'-p', 'path'`: true if path is a pipe (FIFO)
++ `'-S', 'path'`: true if path is a socket
+
+Examples:
+
+```javascript
+if (test('-d', path)) { /* do something with dir */ };
+if (!test('-f', path)) continue; // skip if it's a regular file
+```
+
+Evaluates expression using the available primaries and returns corresponding value.
+
+
+### cat(file [, file ...])
+### cat(file_array)
+
+Examples:
+
+```javascript
+var str = cat('file*.txt');
+var str = cat('file1', 'file2');
+var str = cat(['file1', 'file2']); // same as above
+```
+
+Returns a string containing the given file, or a concatenated string
+containing the files if more than one file is given (a new line character is
+introduced between each file). Wildcard `*` accepted.
+
+
+### 'string'.to(file)
+
+Examples:
+
+```javascript
+cat('input.txt').to('output.txt');
+```
+
+Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
+those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
+
+
+### 'string'.toEnd(file)
+
+Examples:
+
+```javascript
+cat('input.txt').toEnd('output.txt');
+```
+
+Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
+those returned by `cat`, `grep`, etc).
+
+
+### sed([options,] search_regex, replacement, file [, file ...])
+### sed([options,] search_regex, replacement, file_array)
+Available options:
+
++ `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
+
+Examples:
+
+```javascript
+sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
+sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
+```
+
+Reads an input string from `files` and performs a JavaScript `replace()` on the input
+using the given search regex and replacement string or function. Returns the new string after replacement.
+
+
+### grep([options,] regex_filter, file [, file ...])
+### grep([options,] regex_filter, file_array)
+Available options:
+
++ `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
+
+Examples:
+
+```javascript
+grep('-v', 'GLOBAL_VARIABLE', '*.js');
+grep('GLOBAL_VARIABLE', '*.js');
+```
+
+Reads input string from given files and returns a string containing all lines of the
+file that match the given `regex_filter`. Wildcard `*` accepted.
+
+
+### which(command)
+
+Examples:
+
+```javascript
+var nodeExec = which('node');
+```
+
+Searches for `command` in the system's PATH. On Windows, this uses the
+`PATHEXT` variable to append the extension if it's not already executable.
+Returns string containing the absolute path to the command.
+
+
+### echo(string [, string ...])
+
+Examples:
+
+```javascript
+echo('hello world');
+var str = echo('hello world');
+```
+
+Prints string to stdout, and returns string with additional utility methods
+like `.to()`.
+
+
+### pushd([options,] [dir | '-N' | '+N'])
+
+Available options:
+
++ `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
+
+Arguments:
+
++ `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
++ `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
++ `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
+
+Examples:
+
+```javascript
+// process.cwd() === '/usr'
+pushd('/etc'); // Returns /etc /usr
+pushd('+1'); // Returns /usr /etc
+```
+
+Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
+
+### popd([options,] ['-N' | '+N'])
+
+Available options:
+
++ `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
+
+Arguments:
+
++ `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
++ `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
+
+Examples:
+
+```javascript
+echo(process.cwd()); // '/usr'
+pushd('/etc'); // '/etc /usr'
+echo(process.cwd()); // '/etc'
+popd(); // '/usr'
+echo(process.cwd()); // '/usr'
+```
+
+When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
+
+### dirs([options | '+N' | '-N'])
+
+Available options:
+
++ `-c`: Clears the directory stack by deleting all of the elements.
+
+Arguments:
+
++ `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
++ `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
+
+Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
+
+See also: pushd, popd
+
+
+### ln([options,] source, dest)
+Available options:
+
++ `-s`: symlink
++ `-f`: force
+
+Examples:
+
+```javascript
+ln('file', 'newlink');
+ln('-sf', 'file', 'existing');
+```
+
+Links source to dest. Use -f to force the link, should dest already exist.
+
+
+### exit(code)
+Exits the current process with the given exit code.
+
+### env['VAR_NAME']
+Object containing environment variables (both getter and setter). Shortcut to process.env.
+
+### exec(command [, options] [, callback])
+Available options (all `false` by default):
+
++ `async`: Asynchronous execution. If a callback is provided, it will be set to
+ `true`, regardless of the passed value.
++ `silent`: Do not echo program output to console.
++ and any option available to NodeJS's
+ [child_process.exec()](https://nodejs.org/api/child_process.html#child_process_child_process_exec_command_options_callback)
+
+Examples:
+
+```javascript
+var version = exec('node --version', {silent:true}).stdout;
+
+var child = exec('some_long_running_process', {async:true});
+child.stdout.on('data', function(data) {
+ /* ... do something with data ... */
+});
+
+exec('some_long_running_process', function(code, stdout, stderr) {
+ console.log('Exit code:', code);
+ console.log('Program output:', stdout);
+ console.log('Program stderr:', stderr);
+});
+```
+
+Executes the given `command` _synchronously_, unless otherwise specified. When in synchronous
+mode returns the object `{ code:..., stdout:... , stderr:... }`, containing the program's
+`stdout`, `stderr`, and its exit `code`. Otherwise returns the child process object,
+and the `callback` gets the arguments `(code, stdout, stderr)`.
+
+**Note:** For long-lived processes, it's best to run `exec()` asynchronously as
+the current synchronous implementation uses a lot of CPU. This should be getting
+fixed soon.
+
+
+### chmod(octal_mode || octal_string, file)
+### chmod(symbolic_mode, file)
+
+Available options:
+
++ `-v`: output a diagnostic for every file processed
++ `-c`: like verbose but report only when a change is made
++ `-R`: change files and directories recursively
+
+Examples:
+
+```javascript
+chmod(755, '/Users/brandon');
+chmod('755', '/Users/brandon'); // same as above
+chmod('u+x', '/Users/brandon');
+```
+
+Alters the permissions of a file or directory by either specifying the
+absolute permissions in octal form or expressing the changes in symbols.
+This command tries to mimic the POSIX behavior as much as possible.
+Notable exceptions:
+
++ In symbolic modes, 'a-r' and '-r' are identical. No consideration is
+ given to the umask.
++ There is no "quiet" option since default behavior is to run silent.
+
+
+### touch([options,] file)
+Available options:
+
++ `-a`: Change only the access time
++ `-c`: Do not create any files
++ `-m`: Change only the modification time
++ `-d DATE`: Parse DATE and use it instead of current time
++ `-r FILE`: Use FILE's times instead of current time
+
+Examples:
+
+```javascript
+touch('source.js');
+touch('-c', '/path/to/some/dir/source.js');
+touch({ '-r': FILE }, '/path/to/some/dir/source.js');
+```
+
+Update the access and modification times of each FILE to the current time.
+A FILE argument that does not exist is created empty, unless -c is supplied.
+This is a partial implementation of *[touch(1)](http://linux.die.net/man/1/touch)*.
+
+
+### set(options)
+Available options:
+
++ `+/-e`: exit upon error (`config.fatal`)
++ `+/-v`: verbose: show all commands (`config.verbose`)
+
+Examples:
+
+```javascript
+set('-e'); // exit upon first error
+set('+e'); // this undoes a "set('-e')"
+```
+
+Sets global configuration variables
+
+
+## Non-Unix commands
+
+
+### tempdir()
+
+Examples:
+
+```javascript
+var tmp = tempdir(); // "/tmp" for most *nix platforms
+```
+
+Searches and returns string containing a writeable, platform-dependent temporary directory.
+Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
+
+
+### error()
+Tests if error occurred in the last command. Returns `null` if no error occurred,
+otherwise returns string explaining the error
+
+
+## Configuration
+
+
+### config.silent
+Example:
+
+```javascript
+var sh = require('shelljs');
+var silentState = sh.config.silent; // save old silent state
+sh.config.silent = true;
+/* ... */
+sh.config.silent = silentState; // restore old silent state
+```
+
+Suppresses all command output if `true`, except for `echo()` calls.
+Default is `false`.
+
+### config.fatal
+Example:
+
+```javascript
+require('shelljs/global');
+config.fatal = true; // or set('-e');
+cp('this_file_does_not_exist', '/dev/null'); // dies here
+/* more commands... */
+```
+
+If `true` the script will die on errors. Default is `false`. This is
+analogous to Bash's `set -e`
+
+### config.verbose
+Example:
+
+```javascript
+config.verbose = true; // or set('-v');
+cd('dir/');
+ls('subdir/');
+```
+
+Will print each command as follows:
+
+```
+cd dir/
+ls subdir/
+```
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/bin/shjs
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/bin/shjs b/node_modules/shelljs/bin/shjs
new file mode 100755
index 0000000..aae3bc6
--- /dev/null
+++ b/node_modules/shelljs/bin/shjs
@@ -0,0 +1,55 @@
+#!/usr/bin/env node
+require('../global');
+
+if (process.argv.length < 3) {
+ console.log('ShellJS: missing argument (script name)');
+ console.log();
+ process.exit(1);
+}
+
+var args,
+ scriptName = process.argv[2];
+env['NODE_PATH'] = __dirname + '/../..';
+
+if (!scriptName.match(/\.js/) && !scriptName.match(/\.coffee/)) {
+ if (test('-f', scriptName + '.js'))
+ scriptName += '.js';
+ if (test('-f', scriptName + '.coffee'))
+ scriptName += '.coffee';
+}
+
+if (!test('-f', scriptName)) {
+ console.log('ShellJS: script not found ('+scriptName+')');
+ console.log();
+ process.exit(1);
+}
+
+args = process.argv.slice(3);
+
+for (var i = 0, l = args.length; i < l; i++) {
+ if (args[i][0] !== "-"){
+ args[i] = '"' + args[i] + '"'; // fixes arguments with multiple words
+ }
+}
+
+if (scriptName.match(/\.coffee$/)) {
+ //
+ // CoffeeScript
+ //
+ if (which('coffee')) {
+ exec('coffee "' + scriptName + '" ' + args.join(' '), function(code) {
+ process.exit(code);
+ });
+ } else {
+ console.log('ShellJS: CoffeeScript interpreter not found');
+ console.log();
+ process.exit(1);
+ }
+} else {
+ //
+ // JavaScript
+ //
+ exec('node "' + scriptName + '" ' + args.join(' '), function(code) {
+ process.exit(code);
+ });
+}
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[44/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/README.md
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/README.md b/node_modules/elementtree/README.md
new file mode 100644
index 0000000..738420c
--- /dev/null
+++ b/node_modules/elementtree/README.md
@@ -0,0 +1,141 @@
+node-elementtree
+====================
+
+node-elementtree is a [Node.js](http://nodejs.org) XML parser and serializer based upon the [Python ElementTree v1.3](http://effbot.org/zone/element-index.htm) module.
+
+Installation
+====================
+
+ $ npm install elementtree
+
+Using the library
+====================
+
+For the usage refer to the Python ElementTree library documentation - [http://effbot.org/zone/element-index.htm#usage](http://effbot.org/zone/element-index.htm#usage).
+
+Supported XPath expressions in `find`, `findall` and `findtext` methods are listed on [http://effbot.org/zone/element-xpath.htm](http://effbot.org/zone/element-xpath.htm).
+
+Example 1 – Creating An XML Document
+====================
+
+This example shows how to build a valid XML document that can be published to
+Atom Hopper. Atom Hopper is used internally as a bridge from products all the
+way to collecting revenue, called “Usage.” MaaS and other products send similar
+events to it every time user performs an action on a resource
+(e.g. creates,updates or deletes). Below is an example of leveraging the API
+to create a new XML document.
+
+```javascript
+var et = require('elementtree');
+var XML = et.XML;
+var ElementTree = et.ElementTree;
+var element = et.Element;
+var subElement = et.SubElement;
+
+var date, root, tenantId, serviceName, eventType, usageId, dataCenter, region,
+checks, resourceId, category, startTime, resourceName, etree, xml;
+
+date = new Date();
+
+root = element('entry');
+root.set('xmlns', 'http://www.w3.org/2005/Atom');
+
+tenantId = subElement(root, 'TenantId');
+tenantId.text = '12345';
+
+serviceName = subElement(root, 'ServiceName');
+serviceName.text = 'MaaS';
+
+resourceId = subElement(root, 'ResourceID');
+resourceId.text = 'enAAAA';
+
+usageId = subElement(root, 'UsageID');
+usageId.text = '550e8400-e29b-41d4-a716-446655440000';
+
+eventType = subElement(root, 'EventType');
+eventType.text = 'create';
+
+category = subElement(root, 'category');
+category.set('term', 'monitoring.entity.create');
+
+dataCenter = subElement(root, 'DataCenter');
+dataCenter.text = 'global';
+
+region = subElement(root, 'Region');
+region.text = 'global';
+
+startTime = subElement(root, 'StartTime');
+startTime.text = date;
+
+resourceName = subElement(root, 'ResourceName');
+resourceName.text = 'entity';
+
+etree = new ElementTree(root);
+xml = etree.write({'xml_declaration': false});
+console.log(xml);
+```
+
+As you can see, both et.Element and et.SubElement are factory methods which
+return a new instance of Element and SubElement class, respectively.
+When you create a new element (tag) you can use set method to set an attribute.
+To set the tag value, assign a value to the .text attribute.
+
+This example would output a document that looks like this:
+
+```xml
+<entry xmlns="http://www.w3.org/2005/Atom">
+ <TenantId>12345</TenantId>
+ <ServiceName>MaaS</ServiceName>
+ <ResourceID>enAAAA</ResourceID>
+ <UsageID>550e8400-e29b-41d4-a716-446655440000</UsageID>
+ <EventType>create</EventType>
+ <category term="monitoring.entity.create"/>
+ <DataCenter>global</DataCenter>
+ <Region>global</Region>
+ <StartTime>Sun Apr 29 2012 16:37:32 GMT-0700 (PDT)</StartTime>
+ <ResourceName>entity</ResourceName>
+</entry>
+```
+
+Example 2 – Parsing An XML Document
+====================
+
+This example shows how to parse an XML document and use simple XPath selectors.
+For demonstration purposes, we will use the XML document located at
+https://gist.github.com/2554343.
+
+Behind the scenes, node-elementtree uses Isaac’s sax library for parsing XML,
+but the library has a concept of “parsers,” which means it’s pretty simple to
+add support for a different parser.
+
+```javascript
+var fs = require('fs');
+
+var et = require('elementtree');
+
+var XML = et.XML;
+var ElementTree = et.ElementTree;
+var element = et.Element;
+var subElement = et.SubElement;
+
+var data, etree;
+
+data = fs.readFileSync('document.xml').toString();
+etree = et.parse(data);
+
+console.log(etree.findall('./entry/TenantId').length); // 2
+console.log(etree.findtext('./entry/ServiceName')); // MaaS
+console.log(etree.findall('./entry/category')[0].get('term')); // monitoring.entity.create
+console.log(etree.findall('*/category/[@term="monitoring.entity.update"]').length); // 1
+```
+
+Build status
+====================
+
+[](http://travis-ci.org/racker/node-elementtree)
+
+
+License
+====================
+
+node-elementtree is distributed under the [Apache license](http://www.apache.org/licenses/LICENSE-2.0.html).
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/constants.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/constants.js b/node_modules/elementtree/lib/constants.js
new file mode 100644
index 0000000..b057faf
--- /dev/null
+++ b/node_modules/elementtree/lib/constants.js
@@ -0,0 +1,20 @@
+/*
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+var DEFAULT_PARSER = 'sax';
+
+exports.DEFAULT_PARSER = DEFAULT_PARSER;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/elementpath.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/elementpath.js b/node_modules/elementtree/lib/elementpath.js
new file mode 100644
index 0000000..2e93f47
--- /dev/null
+++ b/node_modules/elementtree/lib/elementpath.js
@@ -0,0 +1,343 @@
+/**
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+var sprintf = require('./sprintf').sprintf;
+
+var utils = require('./utils');
+var SyntaxError = require('./errors').SyntaxError;
+
+var _cache = {};
+
+var RE = new RegExp(
+ "(" +
+ "'[^']*'|\"[^\"]*\"|" +
+ "::|" +
+ "//?|" +
+ "\\.\\.|" +
+ "\\(\\)|" +
+ "[/.*:\\[\\]\\(\\)@=])|" +
+ "((?:\\{[^}]+\\})?[^/\\[\\]\\(\\)@=\\s]+)|" +
+ "\\s+", 'g'
+);
+
+var xpath_tokenizer = utils.findall.bind(null, RE);
+
+function prepare_tag(next, token) {
+ var tag = token[0];
+
+ function select(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+ elem._children.forEach(function(e) {
+ if (e.tag === tag) {
+ rv.push(e);
+ }
+ });
+ }
+
+ return rv;
+ }
+
+ return select;
+}
+
+function prepare_star(next, token) {
+ function select(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+ elem._children.forEach(function(e) {
+ rv.push(e);
+ });
+ }
+
+ return rv;
+ }
+
+ return select;
+}
+
+function prepare_dot(next, token) {
+ function select(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+ rv.push(elem);
+ }
+
+ return rv;
+ }
+
+ return select;
+}
+
+function prepare_iter(next, token) {
+ var tag;
+ token = next();
+
+ if (token[1] === '*') {
+ tag = '*';
+ }
+ else if (!token[1]) {
+ tag = token[0] || '';
+ }
+ else {
+ throw new SyntaxError(token);
+ }
+
+ function select(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+ elem.iter(tag, function(e) {
+ if (e !== elem) {
+ rv.push(e);
+ }
+ });
+ }
+
+ return rv;
+ }
+
+ return select;
+}
+
+function prepare_dot_dot(next, token) {
+ function select(context, result) {
+ var i, len, elem, rv = [], parent_map = context.parent_map;
+
+ if (!parent_map) {
+ context.parent_map = parent_map = {};
+
+ context.root.iter(null, function(p) {
+ p._children.forEach(function(e) {
+ parent_map[e] = p;
+ });
+ });
+ }
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+
+ if (parent_map.hasOwnProperty(elem)) {
+ rv.push(parent_map[elem]);
+ }
+ }
+
+ return rv;
+ }
+
+ return select;
+}
+
+
+function prepare_predicate(next, token) {
+ var tag, key, value, select;
+ token = next();
+
+ if (token[1] === '@') {
+ // attribute
+ token = next();
+
+ if (token[1]) {
+ throw new SyntaxError(token, 'Invalid attribute predicate');
+ }
+
+ key = token[0];
+ token = next();
+
+ if (token[1] === ']') {
+ select = function(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+
+ if (elem.get(key)) {
+ rv.push(elem);
+ }
+ }
+
+ return rv;
+ };
+ }
+ else if (token[1] === '=') {
+ value = next()[1];
+
+ if (value[0] === '"' || value[value.length - 1] === '\'') {
+ value = value.slice(1, value.length - 1);
+ }
+ else {
+ throw new SyntaxError(token, 'Ivalid comparison target');
+ }
+
+ token = next();
+ select = function(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+
+ if (elem.get(key) === value) {
+ rv.push(elem);
+ }
+ }
+
+ return rv;
+ };
+ }
+
+ if (token[1] !== ']') {
+ throw new SyntaxError(token, 'Invalid attribute predicate');
+ }
+ }
+ else if (!token[1]) {
+ tag = token[0] || '';
+ token = next();
+
+ if (token[1] !== ']') {
+ throw new SyntaxError(token, 'Invalid node predicate');
+ }
+
+ select = function(context, result) {
+ var i, len, elem, rv = [];
+
+ for (i = 0, len = result.length; i < len; i++) {
+ elem = result[i];
+
+ if (elem.find(tag)) {
+ rv.push(elem);
+ }
+ }
+
+ return rv;
+ };
+ }
+ else {
+ throw new SyntaxError(null, 'Invalid predicate');
+ }
+
+ return select;
+}
+
+
+
+var ops = {
+ "": prepare_tag,
+ "*": prepare_star,
+ ".": prepare_dot,
+ "..": prepare_dot_dot,
+ "//": prepare_iter,
+ "[": prepare_predicate,
+};
+
+function _SelectorContext(root) {
+ this.parent_map = null;
+ this.root = root;
+}
+
+function findall(elem, path) {
+ var selector, result, i, len, token, value, select, context;
+
+ if (_cache.hasOwnProperty(path)) {
+ selector = _cache[path];
+ }
+ else {
+ // TODO: Use smarter cache purging approach
+ if (Object.keys(_cache).length > 100) {
+ _cache = {};
+ }
+
+ if (path.charAt(0) === '/') {
+ throw new SyntaxError(null, 'Cannot use absolute path on element');
+ }
+
+ result = xpath_tokenizer(path);
+ selector = [];
+
+ function getToken() {
+ return result.shift();
+ }
+
+ token = getToken();
+ while (true) {
+ var c = token[1] || '';
+ value = ops[c](getToken, token);
+
+ if (!value) {
+ throw new SyntaxError(null, sprintf('Invalid path: %s', path));
+ }
+
+ selector.push(value);
+ token = getToken();
+
+ if (!token) {
+ break;
+ }
+ else if (token[1] === '/') {
+ token = getToken();
+ }
+
+ if (!token) {
+ break;
+ }
+ }
+
+ _cache[path] = selector;
+ }
+
+ // Execute slector pattern
+ result = [elem];
+ context = new _SelectorContext(elem);
+
+ for (i = 0, len = selector.length; i < len; i++) {
+ select = selector[i];
+ result = select(context, result);
+ }
+
+ return result || [];
+}
+
+function find(element, path) {
+ var resultElements = findall(element, path);
+
+ if (resultElements && resultElements.length > 0) {
+ return resultElements[0];
+ }
+
+ return null;
+}
+
+function findtext(element, path, defvalue) {
+ var resultElements = findall(element, path);
+
+ if (resultElements && resultElements.length > 0) {
+ return resultElements[0].text;
+ }
+
+ return defvalue;
+}
+
+
+exports.find = find;
+exports.findall = findall;
+exports.findtext = findtext;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/elementtree.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/elementtree.js b/node_modules/elementtree/lib/elementtree.js
new file mode 100644
index 0000000..61d9276
--- /dev/null
+++ b/node_modules/elementtree/lib/elementtree.js
@@ -0,0 +1,611 @@
+/**
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+var sprintf = require('./sprintf').sprintf;
+
+var utils = require('./utils');
+var ElementPath = require('./elementpath');
+var TreeBuilder = require('./treebuilder').TreeBuilder;
+var get_parser = require('./parser').get_parser;
+var constants = require('./constants');
+
+var element_ids = 0;
+
+function Element(tag, attrib)
+{
+ this._id = element_ids++;
+ this.tag = tag;
+ this.attrib = {};
+ this.text = null;
+ this.tail = null;
+ this._children = [];
+
+ if (attrib) {
+ this.attrib = utils.merge(this.attrib, attrib);
+ }
+}
+
+Element.prototype.toString = function()
+{
+ return sprintf("<Element %s at %s>", this.tag, this._id);
+};
+
+Element.prototype.makeelement = function(tag, attrib)
+{
+ return new Element(tag, attrib);
+};
+
+Element.prototype.len = function()
+{
+ return this._children.length;
+};
+
+Element.prototype.getItem = function(index)
+{
+ return this._children[index];
+};
+
+Element.prototype.setItem = function(index, element)
+{
+ this._children[index] = element;
+};
+
+Element.prototype.delItem = function(index)
+{
+ this._children.splice(index, 1);
+};
+
+Element.prototype.getSlice = function(start, stop)
+{
+ return this._children.slice(start, stop);
+};
+
+Element.prototype.setSlice = function(start, stop, elements)
+{
+ var i;
+ var k = 0;
+ for (i = start; i < stop; i++, k++) {
+ this._children[i] = elements[k];
+ }
+};
+
+Element.prototype.delSlice = function(start, stop)
+{
+ this._children.splice(start, stop - start);
+};
+
+Element.prototype.append = function(element)
+{
+ this._children.push(element);
+};
+
+Element.prototype.extend = function(elements)
+{
+ this._children.concat(elements);
+};
+
+Element.prototype.insert = function(index, element)
+{
+ this._children[index] = element;
+};
+
+Element.prototype.remove = function(element)
+{
+ this._children = this._children.filter(function(e) {
+ /* TODO: is this the right way to do this? */
+ if (e._id === element._id) {
+ return false;
+ }
+ return true;
+ });
+};
+
+Element.prototype.getchildren = function() {
+ return this._children;
+};
+
+Element.prototype.find = function(path)
+{
+ return ElementPath.find(this, path);
+};
+
+Element.prototype.findtext = function(path, defvalue)
+{
+ return ElementPath.findtext(this, path, defvalue);
+};
+
+Element.prototype.findall = function(path, defvalue)
+{
+ return ElementPath.findall(this, path, defvalue);
+};
+
+Element.prototype.clear = function()
+{
+ this.attrib = {};
+ this._children = [];
+ this.text = null;
+ this.tail = null;
+};
+
+Element.prototype.get = function(key, defvalue)
+{
+ if (this.attrib[key] !== undefined) {
+ return this.attrib[key];
+ }
+ else {
+ return defvalue;
+ }
+};
+
+Element.prototype.set = function(key, value)
+{
+ this.attrib[key] = value;
+};
+
+Element.prototype.keys = function()
+{
+ return Object.keys(this.attrib);
+};
+
+Element.prototype.items = function()
+{
+ return utils.items(this.attrib);
+};
+
+/*
+ * In python this uses a generator, but in v8 we don't have em,
+ * so we use a callback instead.
+ **/
+Element.prototype.iter = function(tag, callback)
+{
+ var self = this;
+ var i, child;
+
+ if (tag === "*") {
+ tag = null;
+ }
+
+ if (tag === null || this.tag === tag) {
+ callback(self);
+ }
+
+ for (i = 0; i < this._children.length; i++) {
+ child = this._children[i];
+ child.iter(tag, function(e) {
+ callback(e);
+ });
+ }
+};
+
+Element.prototype.itertext = function(callback)
+{
+ this.iter(null, function(e) {
+ if (e.text) {
+ callback(e.text);
+ }
+
+ if (e.tail) {
+ callback(e.tail);
+ }
+ });
+};
+
+
+function SubElement(parent, tag, attrib) {
+ var element = parent.makeelement(tag, attrib);
+ parent.append(element);
+ return element;
+}
+
+function Comment(text) {
+ var element = new Element(Comment);
+ if (text) {
+ element.text = text;
+ }
+ return element;
+}
+
+function CData(text) {
+ var element = new Element(CData);
+ if (text) {
+ element.text = text;
+ }
+ return element;
+}
+
+function ProcessingInstruction(target, text)
+{
+ var element = new Element(ProcessingInstruction);
+ element.text = target;
+ if (text) {
+ element.text = element.text + " " + text;
+ }
+ return element;
+}
+
+function QName(text_or_uri, tag)
+{
+ if (tag) {
+ text_or_uri = sprintf("{%s}%s", text_or_uri, tag);
+ }
+ this.text = text_or_uri;
+}
+
+QName.prototype.toString = function() {
+ return this.text;
+};
+
+function ElementTree(element)
+{
+ this._root = element;
+}
+
+ElementTree.prototype.getroot = function() {
+ return this._root;
+};
+
+ElementTree.prototype._setroot = function(element) {
+ this._root = element;
+};
+
+ElementTree.prototype.parse = function(source, parser) {
+ if (!parser) {
+ parser = get_parser(constants.DEFAULT_PARSER);
+ parser = new parser.XMLParser(new TreeBuilder());
+ }
+
+ parser.feed(source);
+ this._root = parser.close();
+ return this._root;
+};
+
+ElementTree.prototype.iter = function(tag, callback) {
+ this._root.iter(tag, callback);
+};
+
+ElementTree.prototype.find = function(path) {
+ return this._root.find(path);
+};
+
+ElementTree.prototype.findtext = function(path, defvalue) {
+ return this._root.findtext(path, defvalue);
+};
+
+ElementTree.prototype.findall = function(path) {
+ return this._root.findall(path);
+};
+
+/**
+ * Unlike ElementTree, we don't write to a file, we return you a string.
+ */
+ElementTree.prototype.write = function(options) {
+ var sb = [];
+ options = utils.merge({
+ encoding: 'utf-8',
+ xml_declaration: null,
+ default_namespace: null,
+ method: 'xml'}, options);
+
+ if (options.xml_declaration !== false) {
+ sb.push("<?xml version='1.0' encoding='"+options.encoding +"'?>\n");
+ }
+
+ if (options.method === "text") {
+ _serialize_text(sb, self._root, encoding);
+ }
+ else {
+ var qnames, namespaces, indent, indent_string;
+ var x = _namespaces(this._root, options.encoding, options.default_namespace);
+ qnames = x[0];
+ namespaces = x[1];
+
+ if (options.hasOwnProperty('indent')) {
+ indent = 0;
+ indent_string = new Array(options.indent + 1).join(' ');
+ }
+ else {
+ indent = false;
+ }
+
+ if (options.method === "xml") {
+ _serialize_xml(function(data) {
+ sb.push(data);
+ }, this._root, options.encoding, qnames, namespaces, indent, indent_string);
+ }
+ else {
+ /* TODO: html */
+ throw new Error("unknown serialization method "+ options.method);
+ }
+ }
+
+ return sb.join("");
+};
+
+var _namespace_map = {
+ /* "well-known" namespace prefixes */
+ "http://www.w3.org/XML/1998/namespace": "xml",
+ "http://www.w3.org/1999/xhtml": "html",
+ "http://www.w3.org/1999/02/22-rdf-syntax-ns#": "rdf",
+ "http://schemas.xmlsoap.org/wsdl/": "wsdl",
+ /* xml schema */
+ "http://www.w3.org/2001/XMLSchema": "xs",
+ "http://www.w3.org/2001/XMLSchema-instance": "xsi",
+ /* dublic core */
+ "http://purl.org/dc/elements/1.1/": "dc",
+};
+
+function register_namespace(prefix, uri) {
+ if (/ns\d+$/.test(prefix)) {
+ throw new Error('Prefix format reserved for internal use');
+ }
+
+ if (_namespace_map.hasOwnProperty(uri) && _namespace_map[uri] === prefix) {
+ delete _namespace_map[uri];
+ }
+
+ _namespace_map[uri] = prefix;
+}
+
+
+function _escape(text, encoding, isAttribute, isText) {
+ if (text) {
+ text = text.toString();
+ text = text.replace(/&/g, '&');
+ text = text.replace(/</g, '<');
+ text = text.replace(/>/g, '>');
+ if (!isText) {
+ text = text.replace(/\n/g, '
');
+ text = text.replace(/\r/g, '
');
+ }
+ if (isAttribute) {
+ text = text.replace(/"/g, '"');
+ }
+ }
+ return text;
+}
+
+/* TODO: benchmark single regex */
+function _escape_attrib(text, encoding) {
+ return _escape(text, encoding, true);
+}
+
+function _escape_cdata(text, encoding) {
+ return _escape(text, encoding, false);
+}
+
+function _escape_text(text, encoding) {
+ return _escape(text, encoding, false, true);
+}
+
+function _namespaces(elem, encoding, default_namespace) {
+ var qnames = {};
+ var namespaces = {};
+
+ if (default_namespace) {
+ namespaces[default_namespace] = "";
+ }
+
+ function encode(text) {
+ return text;
+ }
+
+ function add_qname(qname) {
+ if (qname[0] === "{") {
+ var tmp = qname.substring(1).split("}", 2);
+ var uri = tmp[0];
+ var tag = tmp[1];
+ var prefix = namespaces[uri];
+
+ if (prefix === undefined) {
+ prefix = _namespace_map[uri];
+ if (prefix === undefined) {
+ prefix = "ns" + Object.keys(namespaces).length;
+ }
+ if (prefix !== "xml") {
+ namespaces[uri] = prefix;
+ }
+ }
+
+ if (prefix) {
+ qnames[qname] = sprintf("%s:%s", prefix, tag);
+ }
+ else {
+ qnames[qname] = tag;
+ }
+ }
+ else {
+ if (default_namespace) {
+ throw new Error('cannot use non-qualified names with default_namespace option');
+ }
+
+ qnames[qname] = qname;
+ }
+ }
+
+
+ elem.iter(null, function(e) {
+ var i;
+ var tag = e.tag;
+ var text = e.text;
+ var items = e.items();
+
+ if (tag instanceof QName && qnames[tag.text] === undefined) {
+ add_qname(tag.text);
+ }
+ else if (typeof(tag) === "string") {
+ add_qname(tag);
+ }
+ else if (tag !== null && tag !== Comment && tag !== CData && tag !== ProcessingInstruction) {
+ throw new Error('Invalid tag type for serialization: '+ tag);
+ }
+
+ if (text instanceof QName && qnames[text.text] === undefined) {
+ add_qname(text.text);
+ }
+
+ items.forEach(function(item) {
+ var key = item[0],
+ value = item[1];
+ if (key instanceof QName) {
+ key = key.text;
+ }
+
+ if (qnames[key] === undefined) {
+ add_qname(key);
+ }
+
+ if (value instanceof QName && qnames[value.text] === undefined) {
+ add_qname(value.text);
+ }
+ });
+ });
+ return [qnames, namespaces];
+}
+
+function _serialize_xml(write, elem, encoding, qnames, namespaces, indent, indent_string) {
+ var tag = elem.tag;
+ var text = elem.text;
+ var items;
+ var i;
+
+ var newlines = indent || (indent === 0);
+ write(Array(indent + 1).join(indent_string));
+
+ if (tag === Comment) {
+ write(sprintf("<!--%s-->", _escape_cdata(text, encoding)));
+ }
+ else if (tag === ProcessingInstruction) {
+ write(sprintf("<?%s?>", _escape_cdata(text, encoding)));
+ }
+ else if (tag === CData) {
+ text = text || '';
+ write(sprintf("<![CDATA[%s]]>", text));
+ }
+ else {
+ tag = qnames[tag];
+ if (tag === undefined) {
+ if (text) {
+ write(_escape_text(text, encoding));
+ }
+ elem.iter(function(e) {
+ _serialize_xml(write, e, encoding, qnames, null, newlines ? indent + 1 : false, indent_string);
+ });
+ }
+ else {
+ write("<" + tag);
+ items = elem.items();
+
+ if (items || namespaces) {
+ items.sort(); // lexical order
+
+ items.forEach(function(item) {
+ var k = item[0],
+ v = item[1];
+
+ if (k instanceof QName) {
+ k = k.text;
+ }
+
+ if (v instanceof QName) {
+ v = qnames[v.text];
+ }
+ else {
+ v = _escape_attrib(v, encoding);
+ }
+ write(sprintf(" %s=\"%s\"", qnames[k], v));
+ });
+
+ if (namespaces) {
+ items = utils.items(namespaces);
+ items.sort(function(a, b) { return a[1] < b[1]; });
+
+ items.forEach(function(item) {
+ var k = item[1],
+ v = item[0];
+
+ if (k) {
+ k = ':' + k;
+ }
+
+ write(sprintf(" xmlns%s=\"%s\"", k, _escape_attrib(v, encoding)));
+ });
+ }
+ }
+
+ if (text || elem.len()) {
+ if (text && text.toString().match(/^\s*$/)) {
+ text = null;
+ }
+
+ write(">");
+ if (!text && newlines) {
+ write("\n");
+ }
+
+ if (text) {
+ write(_escape_text(text, encoding));
+ }
+ elem._children.forEach(function(e) {
+ _serialize_xml(write, e, encoding, qnames, null, newlines ? indent + 1 : false, indent_string);
+ });
+
+ if (!text && indent) {
+ write(Array(indent + 1).join(indent_string));
+ }
+ write("</" + tag + ">");
+ }
+ else {
+ write(" />");
+ }
+ }
+ }
+
+ if (newlines) {
+ write("\n");
+ }
+}
+
+function parse(source, parser) {
+ var tree = new ElementTree();
+ tree.parse(source, parser);
+ return tree;
+}
+
+function tostring(element, options) {
+ return new ElementTree(element).write(options);
+}
+
+exports.PI = ProcessingInstruction;
+exports.Comment = Comment;
+exports.CData = CData;
+exports.ProcessingInstruction = ProcessingInstruction;
+exports.SubElement = SubElement;
+exports.QName = QName;
+exports.ElementTree = ElementTree;
+exports.ElementPath = ElementPath;
+exports.Element = function(tag, attrib) {
+ return new Element(tag, attrib);
+};
+
+exports.XML = function(data) {
+ var et = new ElementTree();
+ return et.parse(data);
+};
+
+exports.parse = parse;
+exports.register_namespace = register_namespace;
+exports.tostring = tostring;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/errors.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/errors.js b/node_modules/elementtree/lib/errors.js
new file mode 100644
index 0000000..e8742be
--- /dev/null
+++ b/node_modules/elementtree/lib/errors.js
@@ -0,0 +1,31 @@
+/**
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+var util = require('util');
+
+var sprintf = require('./sprintf').sprintf;
+
+function SyntaxError(token, msg) {
+ msg = msg || sprintf('Syntax Error at token %s', token.toString());
+ this.token = token;
+ this.message = msg;
+ Error.call(this, msg);
+}
+
+util.inherits(SyntaxError, Error);
+
+exports.SyntaxError = SyntaxError;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/parser.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/parser.js b/node_modules/elementtree/lib/parser.js
new file mode 100644
index 0000000..7307ee4
--- /dev/null
+++ b/node_modules/elementtree/lib/parser.js
@@ -0,0 +1,33 @@
+/*
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+/* TODO: support node-expat C++ module optionally */
+
+var util = require('util');
+var parsers = require('./parsers/index');
+
+function get_parser(name) {
+ if (name === 'sax') {
+ return parsers.sax;
+ }
+ else {
+ throw new Error('Invalid parser: ' + name);
+ }
+}
+
+
+exports.get_parser = get_parser;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/parsers/index.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/parsers/index.js b/node_modules/elementtree/lib/parsers/index.js
new file mode 100644
index 0000000..5eac5c8
--- /dev/null
+++ b/node_modules/elementtree/lib/parsers/index.js
@@ -0,0 +1 @@
+exports.sax = require('./sax');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/parsers/sax.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/parsers/sax.js b/node_modules/elementtree/lib/parsers/sax.js
new file mode 100644
index 0000000..69b0a59
--- /dev/null
+++ b/node_modules/elementtree/lib/parsers/sax.js
@@ -0,0 +1,56 @@
+var util = require('util');
+
+var sax = require('sax');
+
+var TreeBuilder = require('./../treebuilder').TreeBuilder;
+
+function XMLParser(target) {
+ this.parser = sax.parser(true);
+
+ this.target = (target) ? target : new TreeBuilder();
+
+ this.parser.onopentag = this._handleOpenTag.bind(this);
+ this.parser.ontext = this._handleText.bind(this);
+ this.parser.oncdata = this._handleCdata.bind(this);
+ this.parser.ondoctype = this._handleDoctype.bind(this);
+ this.parser.oncomment = this._handleComment.bind(this);
+ this.parser.onclosetag = this._handleCloseTag.bind(this);
+ this.parser.onerror = this._handleError.bind(this);
+}
+
+XMLParser.prototype._handleOpenTag = function(tag) {
+ this.target.start(tag.name, tag.attributes);
+};
+
+XMLParser.prototype._handleText = function(text) {
+ this.target.data(text);
+};
+
+XMLParser.prototype._handleCdata = function(text) {
+ this.target.data(text);
+};
+
+XMLParser.prototype._handleDoctype = function(text) {
+};
+
+XMLParser.prototype._handleComment = function(comment) {
+};
+
+XMLParser.prototype._handleCloseTag = function(tag) {
+ this.target.end(tag);
+};
+
+XMLParser.prototype._handleError = function(err) {
+ throw err;
+};
+
+XMLParser.prototype.feed = function(chunk) {
+ this.parser.write(chunk);
+};
+
+XMLParser.prototype.close = function() {
+ this.parser.close();
+ return this.target.close();
+};
+
+exports.XMLParser = XMLParser;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/sprintf.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/sprintf.js b/node_modules/elementtree/lib/sprintf.js
new file mode 100644
index 0000000..f802c1b
--- /dev/null
+++ b/node_modules/elementtree/lib/sprintf.js
@@ -0,0 +1,86 @@
+/*
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+var cache = {};
+
+
+// Do any others need escaping?
+var TO_ESCAPE = {
+ '\'': '\\\'',
+ '\n': '\\n'
+};
+
+
+function populate(formatter) {
+ var i, type,
+ key = formatter,
+ prev = 0,
+ arg = 1,
+ builder = 'return \'';
+
+ for (i = 0; i < formatter.length; i++) {
+ if (formatter[i] === '%') {
+ type = formatter[i + 1];
+
+ switch (type) {
+ case 's':
+ builder += formatter.slice(prev, i) + '\' + arguments[' + arg + '] + \'';
+ prev = i + 2;
+ arg++;
+ break;
+ case 'j':
+ builder += formatter.slice(prev, i) + '\' + JSON.stringify(arguments[' + arg + ']) + \'';
+ prev = i + 2;
+ arg++;
+ break;
+ case '%':
+ builder += formatter.slice(prev, i + 1);
+ prev = i + 2;
+ i++;
+ break;
+ }
+
+
+ } else if (TO_ESCAPE[formatter[i]]) {
+ builder += formatter.slice(prev, i) + TO_ESCAPE[formatter[i]];
+ prev = i + 1;
+ }
+ }
+
+ builder += formatter.slice(prev) + '\';';
+ cache[key] = new Function(builder);
+}
+
+
+/**
+ * A fast version of sprintf(), which currently only supports the %s and %j.
+ * This caches a formatting function for each format string that is used, so
+ * you should only use this sprintf() will be called many times with a single
+ * format string and a limited number of format strings will ever be used (in
+ * general this means that format strings should be string literals).
+ *
+ * @param {String} formatter A format string.
+ * @param {...String} var_args Values that will be formatted by %s and %j.
+ * @return {String} The formatted output.
+ */
+exports.sprintf = function(formatter, var_args) {
+ if (!cache[formatter]) {
+ populate(formatter);
+ }
+
+ return cache[formatter].apply(null, arguments);
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/treebuilder.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/treebuilder.js b/node_modules/elementtree/lib/treebuilder.js
new file mode 100644
index 0000000..393a98f
--- /dev/null
+++ b/node_modules/elementtree/lib/treebuilder.js
@@ -0,0 +1,60 @@
+function TreeBuilder(element_factory) {
+ this._data = [];
+ this._elem = [];
+ this._last = null;
+ this._tail = null;
+ if (!element_factory) {
+ /* evil circular dep */
+ element_factory = require('./elementtree').Element;
+ }
+ this._factory = element_factory;
+}
+
+TreeBuilder.prototype.close = function() {
+ return this._last;
+};
+
+TreeBuilder.prototype._flush = function() {
+ if (this._data) {
+ if (this._last !== null) {
+ var text = this._data.join("");
+ if (this._tail) {
+ this._last.tail = text;
+ }
+ else {
+ this._last.text = text;
+ }
+ }
+ this._data = [];
+ }
+};
+
+TreeBuilder.prototype.data = function(data) {
+ this._data.push(data);
+};
+
+TreeBuilder.prototype.start = function(tag, attrs) {
+ this._flush();
+ var elem = this._factory(tag, attrs);
+ this._last = elem;
+
+ if (this._elem.length) {
+ this._elem[this._elem.length - 1].append(elem);
+ }
+
+ this._elem.push(elem);
+
+ this._tail = null;
+};
+
+TreeBuilder.prototype.end = function(tag) {
+ this._flush();
+ this._last = this._elem.pop();
+ if (this._last.tag !== tag) {
+ throw new Error("end tag mismatch");
+ }
+ this._tail = 1;
+ return this._last;
+};
+
+exports.TreeBuilder = TreeBuilder;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/lib/utils.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/lib/utils.js b/node_modules/elementtree/lib/utils.js
new file mode 100644
index 0000000..b08a670
--- /dev/null
+++ b/node_modules/elementtree/lib/utils.js
@@ -0,0 +1,72 @@
+/**
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+/**
+ * @param {Object} hash.
+ * @param {Array} ignored.
+ */
+function items(hash, ignored) {
+ ignored = ignored || null;
+ var k, rv = [];
+
+ function is_ignored(key) {
+ if (!ignored || ignored.length === 0) {
+ return false;
+ }
+
+ return ignored.indexOf(key);
+ }
+
+ for (k in hash) {
+ if (hash.hasOwnProperty(k) && !(is_ignored(ignored))) {
+ rv.push([k, hash[k]]);
+ }
+ }
+
+ return rv;
+}
+
+
+function findall(re, str) {
+ var match, matches = [];
+
+ while ((match = re.exec(str))) {
+ matches.push(match);
+ }
+
+ return matches;
+}
+
+function merge(a, b) {
+ var c = {}, attrname;
+
+ for (attrname in a) {
+ if (a.hasOwnProperty(attrname)) {
+ c[attrname] = a[attrname];
+ }
+ }
+ for (attrname in b) {
+ if (b.hasOwnProperty(attrname)) {
+ c[attrname] = b[attrname];
+ }
+ }
+ return c;
+}
+
+exports.items = items;
+exports.findall = findall;
+exports.merge = merge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/package.json
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/package.json b/node_modules/elementtree/package.json
new file mode 100644
index 0000000..f6d1ed1
--- /dev/null
+++ b/node_modules/elementtree/package.json
@@ -0,0 +1,100 @@
+{
+ "_args": [
+ [
+ "elementtree@^0.1.6",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "elementtree@>=0.1.6 <0.2.0",
+ "_id": "elementtree@0.1.6",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/elementtree",
+ "_npmUser": {
+ "email": "ryan@trolocsis.com",
+ "name": "rphillips"
+ },
+ "_npmVersion": "1.3.24",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "elementtree",
+ "raw": "elementtree@^0.1.6",
+ "rawSpec": "^0.1.6",
+ "scope": null,
+ "spec": ">=0.1.6 <0.2.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/elementtree/-/elementtree-0.1.6.tgz",
+ "_shasum": "2ac4c46ea30516c8c4cbdb5e3ac7418e592de20c",
+ "_shrinkwrap": null,
+ "_spec": "elementtree@^0.1.6",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "name": "Rackspace US, Inc."
+ },
+ "bugs": {
+ "url": "https://github.com/racker/node-elementtree/issues"
+ },
+ "contributors": [
+ {
+ "name": "Paul Querna",
+ "email": "paul.querna@rackspace.com"
+ },
+ {
+ "name": "Tomaz Muraus",
+ "email": "tomaz.muraus@rackspace.com"
+ }
+ ],
+ "dependencies": {
+ "sax": "0.3.5"
+ },
+ "description": "XML Serialization and Parsing module based on Python's ElementTree.",
+ "devDependencies": {
+ "whiskey": "0.8.x"
+ },
+ "directories": {
+ "lib": "lib"
+ },
+ "dist": {
+ "shasum": "2ac4c46ea30516c8c4cbdb5e3ac7418e592de20c",
+ "tarball": "http://registry.npmjs.org/elementtree/-/elementtree-0.1.6.tgz"
+ },
+ "engines": {
+ "node": ">= 0.4.0"
+ },
+ "homepage": "https://github.com/racker/node-elementtree",
+ "keywords": [
+ "elementtree",
+ "parser",
+ "sax",
+ "seralization",
+ "xml"
+ ],
+ "licenses": [
+ {
+ "type": "Apache",
+ "url": "http://www.apache.org/licenses/LICENSE-2.0.html"
+ }
+ ],
+ "main": "lib/elementtree.js",
+ "maintainers": [
+ {
+ "name": "rphillips",
+ "email": "ryan@trolocsis.com"
+ }
+ ],
+ "name": "elementtree",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/racker/node-elementtree.git"
+ },
+ "scripts": {
+ "test": "make test"
+ },
+ "version": "0.1.6"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/tests/data/xml1.xml
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/tests/data/xml1.xml b/node_modules/elementtree/tests/data/xml1.xml
new file mode 100644
index 0000000..72c33ae
--- /dev/null
+++ b/node_modules/elementtree/tests/data/xml1.xml
@@ -0,0 +1,17 @@
+<?xml version="1.0"?>
+<container name="test_container_1" xmlns:android="http://schemas.android.com/apk/res/android">
+ <object>dd
+ <name>test_object_1</name>
+ <hash>4281c348eaf83e70ddce0e07221c3d28</hash>
+ <bytes android:type="cool">14</bytes>
+ <content_type>application/octetstream</content_type>
+ <last_modified>2009-02-03T05:26:32.612278</last_modified>
+ </object>
+ <object>
+ <name>test_object_2</name>
+ <hash>b039efe731ad111bc1b0ef221c3849d0</hash>
+ <bytes android:type="lame">64</bytes>
+ <content_type>application/octetstream</content_type>
+ <last_modified>2009-02-03T05:26:32.612278</last_modified>
+ </object>
+</container>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/tests/data/xml2.xml
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/tests/data/xml2.xml b/node_modules/elementtree/tests/data/xml2.xml
new file mode 100644
index 0000000..5f94bbd
--- /dev/null
+++ b/node_modules/elementtree/tests/data/xml2.xml
@@ -0,0 +1,14 @@
+<?xml version="1.0"?>
+<object>
+ <title>
+ Hello World
+ </title>
+ <children>
+ <object id="obj1" />
+ <object id="obj2" />
+ <object id="obj3" />
+ </children>
+ <text><![CDATA[
+ Test & Test & Test
+ ]]></text>
+</object>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/tests/test-simple.js
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/tests/test-simple.js b/node_modules/elementtree/tests/test-simple.js
new file mode 100644
index 0000000..1fc04b8
--- /dev/null
+++ b/node_modules/elementtree/tests/test-simple.js
@@ -0,0 +1,339 @@
+/**
+ * Copyright 2011 Rackspace
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+var fs = require('fs');
+var path = require('path');
+
+var sprintf = require('./../lib/sprintf').sprintf;
+var et = require('elementtree');
+var XML = et.XML;
+var ElementTree = et.ElementTree;
+var Element = et.Element;
+var SubElement = et.SubElement;
+var SyntaxError = require('./../lib/errors').SyntaxError;
+
+function readFile(name) {
+ return fs.readFileSync(path.join(__dirname, '/data/', name), 'utf8');
+}
+
+exports['test_simplest'] = function(test, assert) {
+ /* Ported from <https://github.com/lxml/lxml/blob/master/src/lxml/tests/test_elementtree.py> */
+ var Element = et.Element;
+ var root = Element('root');
+ root.append(Element('one'));
+ root.append(Element('two'));
+ root.append(Element('three'));
+ assert.equal(3, root.len());
+ assert.equal('one', root.getItem(0).tag);
+ assert.equal('two', root.getItem(1).tag);
+ assert.equal('three', root.getItem(2).tag);
+ test.finish();
+};
+
+
+exports['test_attribute_values'] = function(test, assert) {
+ var XML = et.XML;
+ var root = XML('<doc alpha="Alpha" beta="Beta" gamma="Gamma"/>');
+ assert.equal('Alpha', root.attrib['alpha']);
+ assert.equal('Beta', root.attrib['beta']);
+ assert.equal('Gamma', root.attrib['gamma']);
+ test.finish();
+};
+
+
+exports['test_findall'] = function(test, assert) {
+ var XML = et.XML;
+ var root = XML('<a><b><c/></b><b/><c><b/></c></a>');
+
+ assert.equal(root.findall("c").length, 1);
+ assert.equal(root.findall(".//c").length, 2);
+ assert.equal(root.findall(".//b").length, 3);
+ assert.equal(root.findall(".//b")[0]._children.length, 1);
+ assert.equal(root.findall(".//b")[1]._children.length, 0);
+ assert.equal(root.findall(".//b")[2]._children.length, 0);
+ assert.deepEqual(root.findall('.//b')[0], root.getchildren()[0]);
+
+ test.finish();
+};
+
+exports['test_find'] = function(test, assert) {
+ var a = Element('a');
+ var b = SubElement(a, 'b');
+ var c = SubElement(a, 'c');
+
+ assert.deepEqual(a.find('./b/..'), a);
+ test.finish();
+};
+
+exports['test_elementtree_find_qname'] = function(test, assert) {
+ var tree = new et.ElementTree(XML('<a><b><c/></b><b/><c><b/></c></a>'));
+ assert.deepEqual(tree.find(new et.QName('c')), tree.getroot()._children[2]);
+ test.finish();
+};
+
+exports['test_attrib_ns_clear'] = function(test, assert) {
+ var attribNS = '{http://foo/bar}x';
+
+ var par = Element('par');
+ par.set(attribNS, 'a');
+ var child = SubElement(par, 'child');
+ child.set(attribNS, 'b');
+
+ assert.equal('a', par.get(attribNS));
+ assert.equal('b', child.get(attribNS));
+
+ par.clear();
+ assert.equal(null, par.get(attribNS));
+ assert.equal('b', child.get(attribNS));
+ test.finish();
+};
+
+exports['test_create_tree_and_parse_simple'] = function(test, assert) {
+ var i = 0;
+ var e = new Element('bar', {});
+ var expected = "<?xml version='1.0' encoding='utf-8'?>\n" +
+ '<bar><blah a="11" /><blah a="12" /><gag a="13" b="abc">ponies</gag></bar>';
+
+ SubElement(e, "blah", {a: 11});
+ SubElement(e, "blah", {a: 12});
+ var se = et.SubElement(e, "gag", {a: '13', b: 'abc'});
+ se.text = 'ponies';
+
+ se.itertext(function(text) {
+ assert.equal(text, 'ponies');
+ i++;
+ });
+
+ assert.equal(i, 1);
+ var etree = new ElementTree(e);
+ var xml = etree.write();
+ assert.equal(xml, expected);
+ test.finish();
+};
+
+exports['test_write_with_options'] = function(test, assert) {
+ var i = 0;
+ var e = new Element('bar', {});
+ var expected1 = "<?xml version='1.0' encoding='utf-8'?>\n" +
+ '<bar>\n' +
+ ' <blah a="11">\n' +
+ ' <baz d="11">test</baz>\n' +
+ ' </blah>\n' +
+ ' <blah a="12" />\n' +
+ ' <gag a="13" b="abc">ponies</gag>\n' +
+ '</bar>\n';
+ var expected2 = "<?xml version='1.0' encoding='utf-8'?>\n" +
+ '<bar>\n' +
+ ' <blah a="11">\n' +
+ ' <baz d="11">test</baz>\n' +
+ ' </blah>\n' +
+ ' <blah a="12" />\n' +
+ ' <gag a="13" b="abc">ponies</gag>\n' +
+ '</bar>\n';
+
+ var expected3 = "<?xml version='1.0' encoding='utf-8'?>\n" +
+ '<object>\n' +
+ ' <title>\n' +
+ ' Hello World\n' +
+ ' </title>\n' +
+ ' <children>\n' +
+ ' <object id="obj1" />\n' +
+ ' <object id="obj2" />\n' +
+ ' <object id="obj3" />\n' +
+ ' </children>\n' +
+ ' <text>\n' +
+ ' Test & Test & Test\n' +
+ ' </text>\n' +
+ '</object>\n';
+
+ var se1 = SubElement(e, "blah", {a: 11});
+ var se2 = SubElement(se1, "baz", {d: 11});
+ se2.text = 'test';
+ SubElement(e, "blah", {a: 12});
+ var se = et.SubElement(e, "gag", {a: '13', b: 'abc'});
+ se.text = 'ponies';
+
+ se.itertext(function(text) {
+ assert.equal(text, 'ponies');
+ i++;
+ });
+
+ assert.equal(i, 1);
+ var etree = new ElementTree(e);
+ var xml1 = etree.write({'indent': 4});
+ var xml2 = etree.write({'indent': 2});
+ assert.equal(xml1, expected1);
+ assert.equal(xml2, expected2);
+
+ var file = readFile('xml2.xml');
+ var etree2 = et.parse(file);
+ var xml3 = etree2.write({'indent': 4});
+ assert.equal(xml3, expected3);
+ test.finish();
+};
+
+exports['test_parse_and_find_2'] = function(test, assert) {
+ var data = readFile('xml1.xml');
+ var etree = et.parse(data);
+
+ assert.equal(etree.findall('./object').length, 2);
+ assert.equal(etree.findall('[@name]').length, 1);
+ assert.equal(etree.findall('[@name="test_container_1"]').length, 1);
+ assert.equal(etree.findall('[@name=\'test_container_1\']').length, 1);
+ assert.equal(etree.findall('./object')[0].findtext('name'), 'test_object_1');
+ assert.equal(etree.findtext('./object/name'), 'test_object_1');
+ assert.equal(etree.findall('.//bytes').length, 2);
+ assert.equal(etree.findall('*/bytes').length, 2);
+ assert.equal(etree.findall('*/foobar').length, 0);
+
+ test.finish();
+};
+
+exports['test_namespaced_attribute'] = function(test, assert) {
+ var data = readFile('xml1.xml');
+ var etree = et.parse(data);
+
+ assert.equal(etree.findall('*/bytes[@android:type="cool"]').length, 1);
+
+ test.finish();
+}
+
+exports['test_syntax_errors'] = function(test, assert) {
+ var expressions = [ './/@bar', '[@bar', '[@foo=bar]', '[@', '/bar' ];
+ var errCount = 0;
+ var data = readFile('xml1.xml');
+ var etree = et.parse(data);
+
+ expressions.forEach(function(expression) {
+ try {
+ etree.findall(expression);
+ }
+ catch (err) {
+ errCount++;
+ }
+ });
+
+ assert.equal(errCount, expressions.length);
+ test.finish();
+};
+
+exports['test_register_namespace'] = function(test, assert){
+ var prefix = 'TESTPREFIX';
+ var namespace = 'http://seriously.unknown/namespace/URI';
+ var errCount = 0;
+
+ var etree = Element(sprintf('{%s}test', namespace));
+ assert.equal(et.tostring(etree, { 'xml_declaration': false}),
+ sprintf('<ns0:test xmlns:ns0="%s" />', namespace));
+
+ et.register_namespace(prefix, namespace);
+ var etree = Element(sprintf('{%s}test', namespace));
+ assert.equal(et.tostring(etree, { 'xml_declaration': false}),
+ sprintf('<%s:test xmlns:%s="%s" />', prefix, prefix, namespace));
+
+ try {
+ et.register_namespace('ns25', namespace);
+ }
+ catch (err) {
+ errCount++;
+ }
+
+ assert.equal(errCount, 1, 'Reserved prefix used, but exception was not thrown');
+ test.finish();
+};
+
+exports['test_tostring'] = function(test, assert) {
+ var a = Element('a');
+ var b = SubElement(a, 'b');
+ var c = SubElement(a, 'c');
+ c.text = 543;
+
+ assert.equal(et.tostring(a, { 'xml_declaration': false }), '<a><b /><c>543</c></a>');
+ assert.equal(et.tostring(c, { 'xml_declaration': false }), '<c>543</c>');
+ test.finish();
+};
+
+exports['test_escape'] = function(test, assert) {
+ var a = Element('a');
+ var b = SubElement(a, 'b');
+ b.text = '&&&&<>"\n\r';
+
+ assert.equal(et.tostring(a, { 'xml_declaration': false }), '<a><b>&&&&<>\"\n\r</b></a>');
+ test.finish();
+};
+
+exports['test_find_null'] = function(test, assert) {
+ var root = Element('root');
+ var node = SubElement(root, 'node');
+ var leaf = SubElement(node, 'leaf');
+ leaf.text = 'ipsum';
+
+ assert.equal(root.find('node/leaf'), leaf);
+ assert.equal(root.find('no-such-node/leaf'), null);
+ test.finish();
+};
+
+exports['test_findtext_null'] = function(test, assert) {
+ var root = Element('root');
+ var node = SubElement(root, 'node');
+ var leaf = SubElement(node, 'leaf');
+ leaf.text = 'ipsum';
+
+ assert.equal(root.findtext('node/leaf'), 'ipsum');
+ assert.equal(root.findtext('no-such-node/leaf'), null);
+ test.finish();
+};
+
+exports['test_remove'] = function(test, assert) {
+ var root = Element('root');
+ var node1 = SubElement(root, 'node1');
+ var node2 = SubElement(root, 'node2');
+ var node3 = SubElement(root, 'node3');
+
+ assert.equal(root.len(), 3);
+
+ root.remove(node2);
+
+ assert.equal(root.len(), 2);
+ assert.equal(root.getItem(0).tag, 'node1')
+ assert.equal(root.getItem(1).tag, 'node3')
+
+ test.finish();
+};
+
+exports['test_cdata_write'] = function(test, assert) {
+ var root, etree, xml, values, value, i;
+
+ values = [
+ 'if(0>1) then true;',
+ '<test1>ponies hello</test1>',
+ ''
+ ];
+
+ for (i = 0; i < values.length; i++) {
+ value = values[i];
+
+ root = Element('root');
+ root.append(et.CData(value));
+ etree = new ElementTree(root);
+ xml = etree.write({'xml_declaration': false});
+
+ assert.equal(xml, sprintf('<root><![CDATA[%s]]></root>', value));
+ }
+
+ test.finish();
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/glob/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/glob/LICENSE b/node_modules/glob/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/glob/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/glob/README.md
----------------------------------------------------------------------
diff --git a/node_modules/glob/README.md b/node_modules/glob/README.md
new file mode 100644
index 0000000..063cf95
--- /dev/null
+++ b/node_modules/glob/README.md
@@ -0,0 +1,377 @@
+[](https://travis-ci.org/isaacs/node-glob/) [](https://david-dm.org/isaacs/node-glob) [](https://david-dm.org/isaacs/node-glob#info=devDependencies) [](https://david-dm.org/isaacs/node-glob#info=optionalDependencies)
+
+# Glob
+
+Match files using the patterns the shell uses, like stars and stuff.
+
+This is a glob implementation in JavaScript. It uses the `minimatch`
+library to do its matching.
+
+
+
+## Usage
+
+```javascript
+var glob = require("glob")
+
+// options is optional
+glob("**/*.js", options, function (er, files) {
+ // files is an array of filenames.
+ // If the `nonull` option is set, and nothing
+ // was found, then files is ["**/*.js"]
+ // er is an error object or null.
+})
+```
+
+## Glob Primer
+
+"Globs" are the patterns you type when you do stuff like `ls *.js` on
+the command line, or put `build/*` in a `.gitignore` file.
+
+Before parsing the path part patterns, braced sections are expanded
+into a set. Braced sections start with `{` and end with `}`, with any
+number of comma-delimited sections within. Braced sections may contain
+slash characters, so `a{/b/c,bcd}` would expand into `a/b/c` and `abcd`.
+
+The following characters have special magic meaning when used in a
+path portion:
+
+* `*` Matches 0 or more characters in a single path portion
+* `?` Matches 1 character
+* `[...]` Matches a range of characters, similar to a RegExp range.
+ If the first character of the range is `!` or `^` then it matches
+ any character not in the range.
+* `!(pattern|pattern|pattern)` Matches anything that does not match
+ any of the patterns provided.
+* `?(pattern|pattern|pattern)` Matches zero or one occurrence of the
+ patterns provided.
+* `+(pattern|pattern|pattern)` Matches one or more occurrences of the
+ patterns provided.
+* `*(a|b|c)` Matches zero or more occurrences of the patterns provided
+* `@(pattern|pat*|pat?erN)` Matches exactly one of the patterns
+ provided
+* `**` If a "globstar" is alone in a path portion, then it matches
+ zero or more directories and subdirectories searching for matches.
+ It does not crawl symlinked directories.
+
+### Dots
+
+If a file or directory path portion has a `.` as the first character,
+then it will not match any glob pattern unless that pattern's
+corresponding path part also has a `.` as its first character.
+
+For example, the pattern `a/.*/c` would match the file at `a/.b/c`.
+However the pattern `a/*/c` would not, because `*` does not start with
+a dot character.
+
+You can make glob treat dots as normal characters by setting
+`dot:true` in the options.
+
+### Basename Matching
+
+If you set `matchBase:true` in the options, and the pattern has no
+slashes in it, then it will seek for any file anywhere in the tree
+with a matching basename. For example, `*.js` would match
+`test/simple/basic.js`.
+
+### Negation
+
+The intent for negation would be for a pattern starting with `!` to
+match everything that *doesn't* match the supplied pattern. However,
+the implementation is weird, and for the time being, this should be
+avoided. The behavior is deprecated in version 5, and will be removed
+entirely in version 6.
+
+### Empty Sets
+
+If no matching files are found, then an empty array is returned. This
+differs from the shell, where the pattern itself is returned. For
+example:
+
+ $ echo a*s*d*f
+ a*s*d*f
+
+To get the bash-style behavior, set the `nonull:true` in the options.
+
+### See Also:
+
+* `man sh`
+* `man bash` (Search for "Pattern Matching")
+* `man 3 fnmatch`
+* `man 5 gitignore`
+* [minimatch documentation](https://github.com/isaacs/minimatch)
+
+## glob.hasMagic(pattern, [options])
+
+Returns `true` if there are any special characters in the pattern, and
+`false` otherwise.
+
+Note that the options affect the results. If `noext:true` is set in
+the options object, then `+(a|b)` will not be considered a magic
+pattern. If the pattern has a brace expansion, like `a/{b/c,x/y}`
+then that is considered magical, unless `nobrace:true` is set in the
+options.
+
+## glob(pattern, [options], cb)
+
+* `pattern` {String} Pattern to be matched
+* `options` {Object}
+* `cb` {Function}
+ * `err` {Error | null}
+ * `matches` {Array<String>} filenames found matching the pattern
+
+Perform an asynchronous glob search.
+
+## glob.sync(pattern, [options])
+
+* `pattern` {String} Pattern to be matched
+* `options` {Object}
+* return: {Array<String>} filenames found matching the pattern
+
+Perform a synchronous glob search.
+
+## Class: glob.Glob
+
+Create a Glob object by instantiating the `glob.Glob` class.
+
+```javascript
+var Glob = require("glob").Glob
+var mg = new Glob(pattern, options, cb)
+```
+
+It's an EventEmitter, and starts walking the filesystem to find matches
+immediately.
+
+### new glob.Glob(pattern, [options], [cb])
+
+* `pattern` {String} pattern to search for
+* `options` {Object}
+* `cb` {Function} Called when an error occurs, or matches are found
+ * `err` {Error | null}
+ * `matches` {Array<String>} filenames found matching the pattern
+
+Note that if the `sync` flag is set in the options, then matches will
+be immediately available on the `g.found` member.
+
+### Properties
+
+* `minimatch` The minimatch object that the glob uses.
+* `options` The options object passed in.
+* `aborted` Boolean which is set to true when calling `abort()`. There
+ is no way at this time to continue a glob search after aborting, but
+ you can re-use the statCache to avoid having to duplicate syscalls.
+* `cache` Convenience object. Each field has the following possible
+ values:
+ * `false` - Path does not exist
+ * `true` - Path exists
+ * `'DIR'` - Path exists, and is not a directory
+ * `'FILE'` - Path exists, and is a directory
+ * `[file, entries, ...]` - Path exists, is a directory, and the
+ array value is the results of `fs.readdir`
+* `statCache` Cache of `fs.stat` results, to prevent statting the same
+ path multiple times.
+* `symlinks` A record of which paths are symbolic links, which is
+ relevant in resolving `**` patterns.
+* `realpathCache` An optional object which is passed to `fs.realpath`
+ to minimize unnecessary syscalls. It is stored on the instantiated
+ Glob object, and may be re-used.
+
+### Events
+
+* `end` When the matching is finished, this is emitted with all the
+ matches found. If the `nonull` option is set, and no match was found,
+ then the `matches` list contains the original pattern. The matches
+ are sorted, unless the `nosort` flag is set.
+* `match` Every time a match is found, this is emitted with the matched.
+* `error` Emitted when an unexpected error is encountered, or whenever
+ any fs error occurs if `options.strict` is set.
+* `abort` When `abort()` is called, this event is raised.
+
+### Methods
+
+* `pause` Temporarily stop the search
+* `resume` Resume the search
+* `abort` Stop the search forever
+
+### Options
+
+All the options that can be passed to Minimatch can also be passed to
+Glob to change pattern matching behavior. Also, some have been added,
+or have glob-specific ramifications.
+
+All options are false by default, unless otherwise noted.
+
+All options are added to the Glob object, as well.
+
+If you are running many `glob` operations, you can pass a Glob object
+as the `options` argument to a subsequent operation to shortcut some
+`stat` and `readdir` calls. At the very least, you may pass in shared
+`symlinks`, `statCache`, `realpathCache`, and `cache` options, so that
+parallel glob operations will be sped up by sharing information about
+the filesystem.
+
+* `cwd` The current working directory in which to search. Defaults
+ to `process.cwd()`.
+* `root` The place where patterns starting with `/` will be mounted
+ onto. Defaults to `path.resolve(options.cwd, "/")` (`/` on Unix
+ systems, and `C:\` or some such on Windows.)
+* `dot` Include `.dot` files in normal matches and `globstar` matches.
+ Note that an explicit dot in a portion of the pattern will always
+ match dot files.
+* `nomount` By default, a pattern starting with a forward-slash will be
+ "mounted" onto the root setting, so that a valid filesystem path is
+ returned. Set this flag to disable that behavior.
+* `mark` Add a `/` character to directory matches. Note that this
+ requires additional stat calls.
+* `nosort` Don't sort the results.
+* `stat` Set to true to stat *all* results. This reduces performance
+ somewhat, and is completely unnecessary, unless `readdir` is presumed
+ to be an untrustworthy indicator of file existence.
+* `silent` When an unusual error is encountered when attempting to
+ read a directory, a warning will be printed to stderr. Set the
+ `silent` option to true to suppress these warnings.
+* `strict` When an unusual error is encountered when attempting to
+ read a directory, the process will just continue on in search of
+ other matches. Set the `strict` option to raise an error in these
+ cases.
+* `cache` See `cache` property above. Pass in a previously generated
+ cache object to save some fs calls.
+* `statCache` A cache of results of filesystem information, to prevent
+ unnecessary stat calls. While it should not normally be necessary
+ to set this, you may pass the statCache from one glob() call to the
+ options object of another, if you know that the filesystem will not
+ change between calls. (See "Race Conditions" below.)
+* `symlinks` A cache of known symbolic links. You may pass in a
+ previously generated `symlinks` object to save `lstat` calls when
+ resolving `**` matches.
+* `sync` DEPRECATED: use `glob.sync(pattern, opts)` instead.
+* `nounique` In some cases, brace-expanded patterns can result in the
+ same file showing up multiple times in the result set. By default,
+ this implementation prevents duplicates in the result set. Set this
+ flag to disable that behavior.
+* `nonull` Set to never return an empty set, instead returning a set
+ containing the pattern itself. This is the default in glob(3).
+* `debug` Set to enable debug logging in minimatch and glob.
+* `nobrace` Do not expand `{a,b}` and `{1..3}` brace sets.
+* `noglobstar` Do not match `**` against multiple filenames. (Ie,
+ treat it as a normal `*` instead.)
+* `noext` Do not match `+(a|b)` "extglob" patterns.
+* `nocase` Perform a case-insensitive match. Note: on
+ case-insensitive filesystems, non-magic patterns will match by
+ default, since `stat` and `readdir` will not raise errors.
+* `matchBase` Perform a basename-only match if the pattern does not
+ contain any slash characters. That is, `*.js` would be treated as
+ equivalent to `**/*.js`, matching all js files in all directories.
+* `nodir` Do not match directories, only files. (Note: to match
+ *only* directories, simply put a `/` at the end of the pattern.)
+* `ignore` Add a pattern or an array of patterns to exclude matches.
+* `follow` Follow symlinked directories when expanding `**` patterns.
+ Note that this can result in a lot of duplicate references in the
+ presence of cyclic links.
+* `realpath` Set to true to call `fs.realpath` on all of the results.
+ In the case of a symlink that cannot be resolved, the full absolute
+ path to the matched entry is returned (though it will usually be a
+ broken symlink)
+* `nonegate` Suppress deprecated `negate` behavior. (See below.)
+ Default=true
+* `nocomment` Suppress deprecated `comment` behavior. (See below.)
+ Default=true
+
+## Comparisons to other fnmatch/glob implementations
+
+While strict compliance with the existing standards is a worthwhile
+goal, some discrepancies exist between node-glob and other
+implementations, and are intentional.
+
+The double-star character `**` is supported by default, unless the
+`noglobstar` flag is set. This is supported in the manner of bsdglob
+and bash 4.3, where `**` only has special significance if it is the only
+thing in a path part. That is, `a/**/b` will match `a/x/y/b`, but
+`a/**b` will not.
+
+Note that symlinked directories are not crawled as part of a `**`,
+though their contents may match against subsequent portions of the
+pattern. This prevents infinite loops and duplicates and the like.
+
+If an escaped pattern has no matches, and the `nonull` flag is set,
+then glob returns the pattern as-provided, rather than
+interpreting the character escapes. For example,
+`glob.match([], "\\*a\\?")` will return `"\\*a\\?"` rather than
+`"*a?"`. This is akin to setting the `nullglob` option in bash, except
+that it does not resolve escaped pattern characters.
+
+If brace expansion is not disabled, then it is performed before any
+other interpretation of the glob pattern. Thus, a pattern like
+`+(a|{b),c)}`, which would not be valid in bash or zsh, is expanded
+**first** into the set of `+(a|b)` and `+(a|c)`, and those patterns are
+checked for validity. Since those two are valid, matching proceeds.
+
+### Comments and Negation
+
+**Note**: In version 5 of this module, negation and comments are
+**disabled** by default. You can explicitly set `nonegate:false` or
+`nocomment:false` to re-enable them. They are going away entirely in
+version 6.
+
+The intent for negation would be for a pattern starting with `!` to
+match everything that *doesn't* match the supplied pattern. However,
+the implementation is weird. It is better to use the `ignore` option
+to set a pattern or set of patterns to exclude from matches. If you
+want the "everything except *x*" type of behavior, you can use `**` as
+the main pattern, and set an `ignore` for the things to exclude.
+
+The comments feature is added in minimatch, primarily to more easily
+support use cases like ignore files, where a `#` at the start of a
+line makes the pattern "empty". However, in the context of a
+straightforward filesystem globber, "comments" don't make much sense.
+
+## Windows
+
+**Please only use forward-slashes in glob expressions.**
+
+Though windows uses either `/` or `\` as its path separator, only `/`
+characters are used by this glob implementation. You must use
+forward-slashes **only** in glob expressions. Back-slashes will always
+be interpreted as escape characters, not path separators.
+
+Results from absolute patterns such as `/foo/*` are mounted onto the
+root setting using `path.join`. On windows, this will by default result
+in `/foo/*` matching `C:\foo\bar.txt`.
+
+## Race Conditions
+
+Glob searching, by its very nature, is susceptible to race conditions,
+since it relies on directory walking and such.
+
+As a result, it is possible that a file that exists when glob looks for
+it may have been deleted or modified by the time it returns the result.
+
+As part of its internal implementation, this program caches all stat
+and readdir calls that it makes, in order to cut down on system
+overhead. However, this also makes it even more susceptible to races,
+especially if the cache or statCache objects are reused between glob
+calls.
+
+Users are thus advised not to use a glob result as a guarantee of
+filesystem state in the face of rapid changes. For the vast majority
+of operations, this is never a problem.
+
+## Contributing
+
+Any change to behavior (including bugfixes) must come with a test.
+
+Patches that fail tests or reduce performance will be rejected.
+
+```
+# to run tests
+npm test
+
+# to re-generate test fixtures
+npm run test-regen
+
+# to benchmark against bash/zsh
+npm run bench
+
+# to profile javascript
+npm run prof
+```
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/glob/common.js
----------------------------------------------------------------------
diff --git a/node_modules/glob/common.js b/node_modules/glob/common.js
new file mode 100644
index 0000000..e36a631
--- /dev/null
+++ b/node_modules/glob/common.js
@@ -0,0 +1,245 @@
+exports.alphasort = alphasort
+exports.alphasorti = alphasorti
+exports.setopts = setopts
+exports.ownProp = ownProp
+exports.makeAbs = makeAbs
+exports.finish = finish
+exports.mark = mark
+exports.isIgnored = isIgnored
+exports.childrenIgnored = childrenIgnored
+
+function ownProp (obj, field) {
+ return Object.prototype.hasOwnProperty.call(obj, field)
+}
+
+var path = require("path")
+var minimatch = require("minimatch")
+var isAbsolute = require("path-is-absolute")
+var Minimatch = minimatch.Minimatch
+
+function alphasorti (a, b) {
+ return a.toLowerCase().localeCompare(b.toLowerCase())
+}
+
+function alphasort (a, b) {
+ return a.localeCompare(b)
+}
+
+function setupIgnores (self, options) {
+ self.ignore = options.ignore || []
+
+ if (!Array.isArray(self.ignore))
+ self.ignore = [self.ignore]
+
+ if (self.ignore.length) {
+ self.ignore = self.ignore.map(ignoreMap)
+ }
+}
+
+function ignoreMap (pattern) {
+ var gmatcher = null
+ if (pattern.slice(-3) === '/**') {
+ var gpattern = pattern.replace(/(\/\*\*)+$/, '')
+ gmatcher = new Minimatch(gpattern)
+ }
+
+ return {
+ matcher: new Minimatch(pattern),
+ gmatcher: gmatcher
+ }
+}
+
+function setopts (self, pattern, options) {
+ if (!options)
+ options = {}
+
+ // base-matching: just use globstar for that.
+ if (options.matchBase && -1 === pattern.indexOf("/")) {
+ if (options.noglobstar) {
+ throw new Error("base matching requires globstar")
+ }
+ pattern = "**/" + pattern
+ }
+
+ self.silent = !!options.silent
+ self.pattern = pattern
+ self.strict = options.strict !== false
+ self.realpath = !!options.realpath
+ self.realpathCache = options.realpathCache || Object.create(null)
+ self.follow = !!options.follow
+ self.dot = !!options.dot
+ self.mark = !!options.mark
+ self.nodir = !!options.nodir
+ if (self.nodir)
+ self.mark = true
+ self.sync = !!options.sync
+ self.nounique = !!options.nounique
+ self.nonull = !!options.nonull
+ self.nosort = !!options.nosort
+ self.nocase = !!options.nocase
+ self.stat = !!options.stat
+ self.noprocess = !!options.noprocess
+
+ self.maxLength = options.maxLength || Infinity
+ self.cache = options.cache || Object.create(null)
+ self.statCache = options.statCache || Object.create(null)
+ self.symlinks = options.symlinks || Object.create(null)
+
+ setupIgnores(self, options)
+
+ self.changedCwd = false
+ var cwd = process.cwd()
+ if (!ownProp(options, "cwd"))
+ self.cwd = cwd
+ else {
+ self.cwd = options.cwd
+ self.changedCwd = path.resolve(options.cwd) !== cwd
+ }
+
+ self.root = options.root || path.resolve(self.cwd, "/")
+ self.root = path.resolve(self.root)
+ if (process.platform === "win32")
+ self.root = self.root.replace(/\\/g, "/")
+
+ self.nomount = !!options.nomount
+
+ // disable comments and negation unless the user explicitly
+ // passes in false as the option.
+ options.nonegate = options.nonegate === false ? false : true
+ options.nocomment = options.nocomment === false ? false : true
+ deprecationWarning(options)
+
+ self.minimatch = new Minimatch(pattern, options)
+ self.options = self.minimatch.options
+}
+
+// TODO(isaacs): remove entirely in v6
+// exported to reset in tests
+exports.deprecationWarned
+function deprecationWarning(options) {
+ if (!options.nonegate || !options.nocomment) {
+ if (process.noDeprecation !== true && !exports.deprecationWarned) {
+ var msg = 'glob WARNING: comments and negation will be disabled in v6'
+ if (process.throwDeprecation)
+ throw new Error(msg)
+ else if (process.traceDeprecation)
+ console.trace(msg)
+ else
+ console.error(msg)
+
+ exports.deprecationWarned = true
+ }
+ }
+}
+
+function finish (self) {
+ var nou = self.nounique
+ var all = nou ? [] : Object.create(null)
+
+ for (var i = 0, l = self.matches.length; i < l; i ++) {
+ var matches = self.matches[i]
+ if (!matches || Object.keys(matches).length === 0) {
+ if (self.nonull) {
+ // do like the shell, and spit out the literal glob
+ var literal = self.minimatch.globSet[i]
+ if (nou)
+ all.push(literal)
+ else
+ all[literal] = true
+ }
+ } else {
+ // had matches
+ var m = Object.keys(matches)
+ if (nou)
+ all.push.apply(all, m)
+ else
+ m.forEach(function (m) {
+ all[m] = true
+ })
+ }
+ }
+
+ if (!nou)
+ all = Object.keys(all)
+
+ if (!self.nosort)
+ all = all.sort(self.nocase ? alphasorti : alphasort)
+
+ // at *some* point we statted all of these
+ if (self.mark) {
+ for (var i = 0; i < all.length; i++) {
+ all[i] = self._mark(all[i])
+ }
+ if (self.nodir) {
+ all = all.filter(function (e) {
+ return !(/\/$/.test(e))
+ })
+ }
+ }
+
+ if (self.ignore.length)
+ all = all.filter(function(m) {
+ return !isIgnored(self, m)
+ })
+
+ self.found = all
+}
+
+function mark (self, p) {
+ var abs = makeAbs(self, p)
+ var c = self.cache[abs]
+ var m = p
+ if (c) {
+ var isDir = c === 'DIR' || Array.isArray(c)
+ var slash = p.slice(-1) === '/'
+
+ if (isDir && !slash)
+ m += '/'
+ else if (!isDir && slash)
+ m = m.slice(0, -1)
+
+ if (m !== p) {
+ var mabs = makeAbs(self, m)
+ self.statCache[mabs] = self.statCache[abs]
+ self.cache[mabs] = self.cache[abs]
+ }
+ }
+
+ return m
+}
+
+// lotta situps...
+function makeAbs (self, f) {
+ var abs = f
+ if (f.charAt(0) === '/') {
+ abs = path.join(self.root, f)
+ } else if (isAbsolute(f) || f === '') {
+ abs = f
+ } else if (self.changedCwd) {
+ abs = path.resolve(self.cwd, f)
+ } else {
+ abs = path.resolve(f)
+ }
+ return abs
+}
+
+
+// Return true, if pattern ends with globstar '**', for the accompanying parent directory.
+// Ex:- If node_modules/** is the pattern, add 'node_modules' to ignore list along with it's contents
+function isIgnored (self, path) {
+ if (!self.ignore.length)
+ return false
+
+ return self.ignore.some(function(item) {
+ return item.matcher.match(path) || !!(item.gmatcher && item.gmatcher.match(path))
+ })
+}
+
+function childrenIgnored (self, path) {
+ if (!self.ignore.length)
+ return false
+
+ return self.ignore.some(function(item) {
+ return !!(item.gmatcher && item.gmatcher.match(path))
+ })
+}
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[24/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isIterateeCall.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isIterateeCall.js b/node_modules/lodash/internal/isIterateeCall.js
new file mode 100644
index 0000000..07490f2
--- /dev/null
+++ b/node_modules/lodash/internal/isIterateeCall.js
@@ -0,0 +1,28 @@
+var isArrayLike = require('./isArrayLike'),
+ isIndex = require('./isIndex'),
+ isObject = require('../lang/isObject');
+
+/**
+ * Checks if the provided arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call, else `false`.
+ */
+function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)) {
+ var other = object[index];
+ return value === value ? (value === other) : (other !== other);
+ }
+ return false;
+}
+
+module.exports = isIterateeCall;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isKey.js b/node_modules/lodash/internal/isKey.js
new file mode 100644
index 0000000..44ccfd4
--- /dev/null
+++ b/node_modules/lodash/internal/isKey.js
@@ -0,0 +1,28 @@
+var isArray = require('../lang/isArray'),
+ toObject = require('./toObject');
+
+/** Used to match property names within property paths. */
+var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\n\\]|\\.)*?\1)\]/,
+ reIsPlainProp = /^\w*$/;
+
+/**
+ * Checks if `value` is a property name and not a property path.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {Object} [object] The object to query keys on.
+ * @returns {boolean} Returns `true` if `value` is a property name, else `false`.
+ */
+function isKey(value, object) {
+ var type = typeof value;
+ if ((type == 'string' && reIsPlainProp.test(value)) || type == 'number') {
+ return true;
+ }
+ if (isArray(value)) {
+ return false;
+ }
+ var result = !reIsDeepProp.test(value);
+ return result || (object != null && value in toObject(object));
+}
+
+module.exports = isKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isLaziable.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isLaziable.js b/node_modules/lodash/internal/isLaziable.js
new file mode 100644
index 0000000..475fab1
--- /dev/null
+++ b/node_modules/lodash/internal/isLaziable.js
@@ -0,0 +1,27 @@
+var LazyWrapper = require('./LazyWrapper'),
+ getData = require('./getData'),
+ getFuncName = require('./getFuncName'),
+ lodash = require('../chain/lodash');
+
+/**
+ * Checks if `func` has a lazy counterpart.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` has a lazy counterpart, else `false`.
+ */
+function isLaziable(func) {
+ var funcName = getFuncName(func),
+ other = lodash[funcName];
+
+ if (typeof other != 'function' || !(funcName in LazyWrapper.prototype)) {
+ return false;
+ }
+ if (func === other) {
+ return true;
+ }
+ var data = getData(other);
+ return !!data && func === data[0];
+}
+
+module.exports = isLaziable;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isLength.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isLength.js b/node_modules/lodash/internal/isLength.js
new file mode 100644
index 0000000..2092987
--- /dev/null
+++ b/node_modules/lodash/internal/isLength.js
@@ -0,0 +1,20 @@
+/**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This function is based on [`ToLength`](http://ecma-international.org/ecma-262/6.0/#sec-tolength).
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ */
+function isLength(value) {
+ return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+module.exports = isLength;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isObjectLike.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isObjectLike.js b/node_modules/lodash/internal/isObjectLike.js
new file mode 100644
index 0000000..8ca0585
--- /dev/null
+++ b/node_modules/lodash/internal/isObjectLike.js
@@ -0,0 +1,12 @@
+/**
+ * Checks if `value` is object-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+module.exports = isObjectLike;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isSpace.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isSpace.js b/node_modules/lodash/internal/isSpace.js
new file mode 100644
index 0000000..16ea6f3
--- /dev/null
+++ b/node_modules/lodash/internal/isSpace.js
@@ -0,0 +1,14 @@
+/**
+ * Used by `trimmedLeftIndex` and `trimmedRightIndex` to determine if a
+ * character code is whitespace.
+ *
+ * @private
+ * @param {number} charCode The character code to inspect.
+ * @returns {boolean} Returns `true` if `charCode` is whitespace, else `false`.
+ */
+function isSpace(charCode) {
+ return ((charCode <= 160 && (charCode >= 9 && charCode <= 13) || charCode == 32 || charCode == 160) || charCode == 5760 || charCode == 6158 ||
+ (charCode >= 8192 && (charCode <= 8202 || charCode == 8232 || charCode == 8233 || charCode == 8239 || charCode == 8287 || charCode == 12288 || charCode == 65279)));
+}
+
+module.exports = isSpace;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isStrictComparable.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isStrictComparable.js b/node_modules/lodash/internal/isStrictComparable.js
new file mode 100644
index 0000000..0a53eba
--- /dev/null
+++ b/node_modules/lodash/internal/isStrictComparable.js
@@ -0,0 +1,15 @@
+var isObject = require('../lang/isObject');
+
+/**
+ * Checks if `value` is suitable for strict equality comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` if suitable for strict
+ * equality comparisons, else `false`.
+ */
+function isStrictComparable(value) {
+ return value === value && !isObject(value);
+}
+
+module.exports = isStrictComparable;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/lazyClone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/lazyClone.js b/node_modules/lodash/internal/lazyClone.js
new file mode 100644
index 0000000..04c222b
--- /dev/null
+++ b/node_modules/lodash/internal/lazyClone.js
@@ -0,0 +1,23 @@
+var LazyWrapper = require('./LazyWrapper'),
+ arrayCopy = require('./arrayCopy');
+
+/**
+ * Creates a clone of the lazy wrapper object.
+ *
+ * @private
+ * @name clone
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the cloned `LazyWrapper` object.
+ */
+function lazyClone() {
+ var result = new LazyWrapper(this.__wrapped__);
+ result.__actions__ = arrayCopy(this.__actions__);
+ result.__dir__ = this.__dir__;
+ result.__filtered__ = this.__filtered__;
+ result.__iteratees__ = arrayCopy(this.__iteratees__);
+ result.__takeCount__ = this.__takeCount__;
+ result.__views__ = arrayCopy(this.__views__);
+ return result;
+}
+
+module.exports = lazyClone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/lazyReverse.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/lazyReverse.js b/node_modules/lodash/internal/lazyReverse.js
new file mode 100644
index 0000000..c658402
--- /dev/null
+++ b/node_modules/lodash/internal/lazyReverse.js
@@ -0,0 +1,23 @@
+var LazyWrapper = require('./LazyWrapper');
+
+/**
+ * Reverses the direction of lazy iteration.
+ *
+ * @private
+ * @name reverse
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the new reversed `LazyWrapper` object.
+ */
+function lazyReverse() {
+ if (this.__filtered__) {
+ var result = new LazyWrapper(this);
+ result.__dir__ = -1;
+ result.__filtered__ = true;
+ } else {
+ result = this.clone();
+ result.__dir__ *= -1;
+ }
+ return result;
+}
+
+module.exports = lazyReverse;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/lazyValue.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/lazyValue.js b/node_modules/lodash/internal/lazyValue.js
new file mode 100644
index 0000000..8de68e6
--- /dev/null
+++ b/node_modules/lodash/internal/lazyValue.js
@@ -0,0 +1,72 @@
+var baseWrapperValue = require('./baseWrapperValue'),
+ getView = require('./getView'),
+ isArray = require('../lang/isArray');
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/** Used to indicate the type of lazy iteratees. */
+var LAZY_FILTER_FLAG = 1,
+ LAZY_MAP_FLAG = 2;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Extracts the unwrapped value from its lazy wrapper.
+ *
+ * @private
+ * @name value
+ * @memberOf LazyWrapper
+ * @returns {*} Returns the unwrapped value.
+ */
+function lazyValue() {
+ var array = this.__wrapped__.value(),
+ dir = this.__dir__,
+ isArr = isArray(array),
+ isRight = dir < 0,
+ arrLength = isArr ? array.length : 0,
+ view = getView(0, arrLength, this.__views__),
+ start = view.start,
+ end = view.end,
+ length = end - start,
+ index = isRight ? end : (start - 1),
+ iteratees = this.__iteratees__,
+ iterLength = iteratees.length,
+ resIndex = 0,
+ takeCount = nativeMin(length, this.__takeCount__);
+
+ if (!isArr || arrLength < LARGE_ARRAY_SIZE || (arrLength == length && takeCount == length)) {
+ return baseWrapperValue(array, this.__actions__);
+ }
+ var result = [];
+
+ outer:
+ while (length-- && resIndex < takeCount) {
+ index += dir;
+
+ var iterIndex = -1,
+ value = array[index];
+
+ while (++iterIndex < iterLength) {
+ var data = iteratees[iterIndex],
+ iteratee = data.iteratee,
+ type = data.type,
+ computed = iteratee(value);
+
+ if (type == LAZY_MAP_FLAG) {
+ value = computed;
+ } else if (!computed) {
+ if (type == LAZY_FILTER_FLAG) {
+ continue outer;
+ } else {
+ break outer;
+ }
+ }
+ }
+ result[resIndex++] = value;
+ }
+ return result;
+}
+
+module.exports = lazyValue;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/mapDelete.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/mapDelete.js b/node_modules/lodash/internal/mapDelete.js
new file mode 100644
index 0000000..8b7fd53
--- /dev/null
+++ b/node_modules/lodash/internal/mapDelete.js
@@ -0,0 +1,14 @@
+/**
+ * Removes `key` and its value from the cache.
+ *
+ * @private
+ * @name delete
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed successfully, else `false`.
+ */
+function mapDelete(key) {
+ return this.has(key) && delete this.__data__[key];
+}
+
+module.exports = mapDelete;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/mapGet.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/mapGet.js b/node_modules/lodash/internal/mapGet.js
new file mode 100644
index 0000000..1f22295
--- /dev/null
+++ b/node_modules/lodash/internal/mapGet.js
@@ -0,0 +1,14 @@
+/**
+ * Gets the cached value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the cached value.
+ */
+function mapGet(key) {
+ return key == '__proto__' ? undefined : this.__data__[key];
+}
+
+module.exports = mapGet;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/mapHas.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/mapHas.js b/node_modules/lodash/internal/mapHas.js
new file mode 100644
index 0000000..6d94ce4
--- /dev/null
+++ b/node_modules/lodash/internal/mapHas.js
@@ -0,0 +1,20 @@
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if a cached value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function mapHas(key) {
+ return key != '__proto__' && hasOwnProperty.call(this.__data__, key);
+}
+
+module.exports = mapHas;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/mapSet.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/mapSet.js b/node_modules/lodash/internal/mapSet.js
new file mode 100644
index 0000000..0434c3f
--- /dev/null
+++ b/node_modules/lodash/internal/mapSet.js
@@ -0,0 +1,18 @@
+/**
+ * Sets `value` to `key` of the cache.
+ *
+ * @private
+ * @name set
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the value to cache.
+ * @param {*} value The value to cache.
+ * @returns {Object} Returns the cache object.
+ */
+function mapSet(key, value) {
+ if (key != '__proto__') {
+ this.__data__[key] = value;
+ }
+ return this;
+}
+
+module.exports = mapSet;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/mergeData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/mergeData.js b/node_modules/lodash/internal/mergeData.js
new file mode 100644
index 0000000..29297c7
--- /dev/null
+++ b/node_modules/lodash/internal/mergeData.js
@@ -0,0 +1,89 @@
+var arrayCopy = require('./arrayCopy'),
+ composeArgs = require('./composeArgs'),
+ composeArgsRight = require('./composeArgsRight'),
+ replaceHolders = require('./replaceHolders');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1,
+ CURRY_BOUND_FLAG = 4,
+ CURRY_FLAG = 8,
+ ARY_FLAG = 128,
+ REARG_FLAG = 256;
+
+/** Used as the internal argument placeholder. */
+var PLACEHOLDER = '__lodash_placeholder__';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Merges the function metadata of `source` into `data`.
+ *
+ * Merging metadata reduces the number of wrappers required to invoke a function.
+ * This is possible because methods like `_.bind`, `_.curry`, and `_.partial`
+ * may be applied regardless of execution order. Methods like `_.ary` and `_.rearg`
+ * augment function arguments, making the order in which they are executed important,
+ * preventing the merging of metadata. However, we make an exception for a safe
+ * common case where curried functions have `_.ary` and or `_.rearg` applied.
+ *
+ * @private
+ * @param {Array} data The destination metadata.
+ * @param {Array} source The source metadata.
+ * @returns {Array} Returns `data`.
+ */
+function mergeData(data, source) {
+ var bitmask = data[1],
+ srcBitmask = source[1],
+ newBitmask = bitmask | srcBitmask,
+ isCommon = newBitmask < ARY_FLAG;
+
+ var isCombo =
+ (srcBitmask == ARY_FLAG && bitmask == CURRY_FLAG) ||
+ (srcBitmask == ARY_FLAG && bitmask == REARG_FLAG && data[7].length <= source[8]) ||
+ (srcBitmask == (ARY_FLAG | REARG_FLAG) && bitmask == CURRY_FLAG);
+
+ // Exit early if metadata can't be merged.
+ if (!(isCommon || isCombo)) {
+ return data;
+ }
+ // Use source `thisArg` if available.
+ if (srcBitmask & BIND_FLAG) {
+ data[2] = source[2];
+ // Set when currying a bound function.
+ newBitmask |= (bitmask & BIND_FLAG) ? 0 : CURRY_BOUND_FLAG;
+ }
+ // Compose partial arguments.
+ var value = source[3];
+ if (value) {
+ var partials = data[3];
+ data[3] = partials ? composeArgs(partials, value, source[4]) : arrayCopy(value);
+ data[4] = partials ? replaceHolders(data[3], PLACEHOLDER) : arrayCopy(source[4]);
+ }
+ // Compose partial right arguments.
+ value = source[5];
+ if (value) {
+ partials = data[5];
+ data[5] = partials ? composeArgsRight(partials, value, source[6]) : arrayCopy(value);
+ data[6] = partials ? replaceHolders(data[5], PLACEHOLDER) : arrayCopy(source[6]);
+ }
+ // Use source `argPos` if available.
+ value = source[7];
+ if (value) {
+ data[7] = arrayCopy(value);
+ }
+ // Use source `ary` if it's smaller.
+ if (srcBitmask & ARY_FLAG) {
+ data[8] = data[8] == null ? source[8] : nativeMin(data[8], source[8]);
+ }
+ // Use source `arity` if one is not provided.
+ if (data[9] == null) {
+ data[9] = source[9];
+ }
+ // Use source `func` and merge bitmasks.
+ data[0] = source[0];
+ data[1] = newBitmask;
+
+ return data;
+}
+
+module.exports = mergeData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/mergeDefaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/mergeDefaults.js b/node_modules/lodash/internal/mergeDefaults.js
new file mode 100644
index 0000000..dcd967e
--- /dev/null
+++ b/node_modules/lodash/internal/mergeDefaults.js
@@ -0,0 +1,15 @@
+var merge = require('../object/merge');
+
+/**
+ * Used by `_.defaultsDeep` to customize its `_.merge` use.
+ *
+ * @private
+ * @param {*} objectValue The destination object property value.
+ * @param {*} sourceValue The source object property value.
+ * @returns {*} Returns the value to assign to the destination object.
+ */
+function mergeDefaults(objectValue, sourceValue) {
+ return objectValue === undefined ? sourceValue : merge(objectValue, sourceValue, mergeDefaults);
+}
+
+module.exports = mergeDefaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/metaMap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/metaMap.js b/node_modules/lodash/internal/metaMap.js
new file mode 100644
index 0000000..59bfd5f
--- /dev/null
+++ b/node_modules/lodash/internal/metaMap.js
@@ -0,0 +1,9 @@
+var getNative = require('./getNative');
+
+/** Native method references. */
+var WeakMap = getNative(global, 'WeakMap');
+
+/** Used to store function metadata. */
+var metaMap = WeakMap && new WeakMap;
+
+module.exports = metaMap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/pickByArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/pickByArray.js b/node_modules/lodash/internal/pickByArray.js
new file mode 100644
index 0000000..0999d90
--- /dev/null
+++ b/node_modules/lodash/internal/pickByArray.js
@@ -0,0 +1,28 @@
+var toObject = require('./toObject');
+
+/**
+ * A specialized version of `_.pick` which picks `object` properties specified
+ * by `props`.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {string[]} props The property names to pick.
+ * @returns {Object} Returns the new object.
+ */
+function pickByArray(object, props) {
+ object = toObject(object);
+
+ var index = -1,
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ if (key in object) {
+ result[key] = object[key];
+ }
+ }
+ return result;
+}
+
+module.exports = pickByArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/pickByCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/pickByCallback.js b/node_modules/lodash/internal/pickByCallback.js
new file mode 100644
index 0000000..79d3cdc
--- /dev/null
+++ b/node_modules/lodash/internal/pickByCallback.js
@@ -0,0 +1,22 @@
+var baseForIn = require('./baseForIn');
+
+/**
+ * A specialized version of `_.pick` which picks `object` properties `predicate`
+ * returns truthy for.
+ *
+ * @private
+ * @param {Object} object The source object.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Object} Returns the new object.
+ */
+function pickByCallback(object, predicate) {
+ var result = {};
+ baseForIn(object, function(value, key, object) {
+ if (predicate(value, key, object)) {
+ result[key] = value;
+ }
+ });
+ return result;
+}
+
+module.exports = pickByCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/reEscape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/reEscape.js b/node_modules/lodash/internal/reEscape.js
new file mode 100644
index 0000000..7f47eda
--- /dev/null
+++ b/node_modules/lodash/internal/reEscape.js
@@ -0,0 +1,4 @@
+/** Used to match template delimiters. */
+var reEscape = /<%-([\s\S]+?)%>/g;
+
+module.exports = reEscape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/reEvaluate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/reEvaluate.js b/node_modules/lodash/internal/reEvaluate.js
new file mode 100644
index 0000000..6adfc31
--- /dev/null
+++ b/node_modules/lodash/internal/reEvaluate.js
@@ -0,0 +1,4 @@
+/** Used to match template delimiters. */
+var reEvaluate = /<%([\s\S]+?)%>/g;
+
+module.exports = reEvaluate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/reInterpolate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/reInterpolate.js b/node_modules/lodash/internal/reInterpolate.js
new file mode 100644
index 0000000..d02ff0b
--- /dev/null
+++ b/node_modules/lodash/internal/reInterpolate.js
@@ -0,0 +1,4 @@
+/** Used to match template delimiters. */
+var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+module.exports = reInterpolate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/realNames.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/realNames.js b/node_modules/lodash/internal/realNames.js
new file mode 100644
index 0000000..aa0d529
--- /dev/null
+++ b/node_modules/lodash/internal/realNames.js
@@ -0,0 +1,4 @@
+/** Used to lookup unminified function names. */
+var realNames = {};
+
+module.exports = realNames;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/reorder.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/reorder.js b/node_modules/lodash/internal/reorder.js
new file mode 100644
index 0000000..9424927
--- /dev/null
+++ b/node_modules/lodash/internal/reorder.js
@@ -0,0 +1,29 @@
+var arrayCopy = require('./arrayCopy'),
+ isIndex = require('./isIndex');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Reorder `array` according to the specified indexes where the element at
+ * the first index is assigned as the first element, the element at
+ * the second index is assigned as the second element, and so on.
+ *
+ * @private
+ * @param {Array} array The array to reorder.
+ * @param {Array} indexes The arranged array indexes.
+ * @returns {Array} Returns `array`.
+ */
+function reorder(array, indexes) {
+ var arrLength = array.length,
+ length = nativeMin(indexes.length, arrLength),
+ oldArray = arrayCopy(array);
+
+ while (length--) {
+ var index = indexes[length];
+ array[length] = isIndex(index, arrLength) ? oldArray[index] : undefined;
+ }
+ return array;
+}
+
+module.exports = reorder;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/replaceHolders.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/replaceHolders.js b/node_modules/lodash/internal/replaceHolders.js
new file mode 100644
index 0000000..3089e75
--- /dev/null
+++ b/node_modules/lodash/internal/replaceHolders.js
@@ -0,0 +1,28 @@
+/** Used as the internal argument placeholder. */
+var PLACEHOLDER = '__lodash_placeholder__';
+
+/**
+ * Replaces all `placeholder` elements in `array` with an internal placeholder
+ * and returns an array of their indexes.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {*} placeholder The placeholder to replace.
+ * @returns {Array} Returns the new array of placeholder indexes.
+ */
+function replaceHolders(array, placeholder) {
+ var index = -1,
+ length = array.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ if (array[index] === placeholder) {
+ array[index] = PLACEHOLDER;
+ result[++resIndex] = index;
+ }
+ }
+ return result;
+}
+
+module.exports = replaceHolders;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/setData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/setData.js b/node_modules/lodash/internal/setData.js
new file mode 100644
index 0000000..7eb3f40
--- /dev/null
+++ b/node_modules/lodash/internal/setData.js
@@ -0,0 +1,41 @@
+var baseSetData = require('./baseSetData'),
+ now = require('../date/now');
+
+/** Used to detect when a function becomes hot. */
+var HOT_COUNT = 150,
+ HOT_SPAN = 16;
+
+/**
+ * Sets metadata for `func`.
+ *
+ * **Note:** If this function becomes hot, i.e. is invoked a lot in a short
+ * period of time, it will trip its breaker and transition to an identity function
+ * to avoid garbage collection pauses in V8. See [V8 issue 2070](https://code.google.com/p/v8/issues/detail?id=2070)
+ * for more details.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+var setData = (function() {
+ var count = 0,
+ lastCalled = 0;
+
+ return function(key, value) {
+ var stamp = now(),
+ remaining = HOT_SPAN - (stamp - lastCalled);
+
+ lastCalled = stamp;
+ if (remaining > 0) {
+ if (++count >= HOT_COUNT) {
+ return key;
+ }
+ } else {
+ count = 0;
+ }
+ return baseSetData(key, value);
+ };
+}());
+
+module.exports = setData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/shimKeys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/shimKeys.js b/node_modules/lodash/internal/shimKeys.js
new file mode 100644
index 0000000..189e492
--- /dev/null
+++ b/node_modules/lodash/internal/shimKeys.js
@@ -0,0 +1,41 @@
+var isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isIndex = require('./isIndex'),
+ isLength = require('./isLength'),
+ keysIn = require('../object/keysIn');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A fallback implementation of `Object.keys` which creates an array of the
+ * own enumerable property names of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function shimKeys(object) {
+ var props = keysIn(object),
+ propsLength = props.length,
+ length = propsLength && object.length;
+
+ var allowIndexes = !!length && isLength(length) &&
+ (isArray(object) || isArguments(object));
+
+ var index = -1,
+ result = [];
+
+ while (++index < propsLength) {
+ var key = props[index];
+ if ((allowIndexes && isIndex(key, length)) || hasOwnProperty.call(object, key)) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = shimKeys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/sortedUniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/sortedUniq.js b/node_modules/lodash/internal/sortedUniq.js
new file mode 100644
index 0000000..3ede46a
--- /dev/null
+++ b/node_modules/lodash/internal/sortedUniq.js
@@ -0,0 +1,29 @@
+/**
+ * An implementation of `_.uniq` optimized for sorted arrays without support
+ * for callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The function invoked per iteration.
+ * @returns {Array} Returns the new duplicate free array.
+ */
+function sortedUniq(array, iteratee) {
+ var seen,
+ index = -1,
+ length = array.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value, index, array) : value;
+
+ if (!index || seen !== computed) {
+ seen = computed;
+ result[++resIndex] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = sortedUniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/toIterable.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/toIterable.js b/node_modules/lodash/internal/toIterable.js
new file mode 100644
index 0000000..c0a5b28
--- /dev/null
+++ b/node_modules/lodash/internal/toIterable.js
@@ -0,0 +1,22 @@
+var isArrayLike = require('./isArrayLike'),
+ isObject = require('../lang/isObject'),
+ values = require('../object/values');
+
+/**
+ * Converts `value` to an array-like object if it's not one.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {Array|Object} Returns the array-like object.
+ */
+function toIterable(value) {
+ if (value == null) {
+ return [];
+ }
+ if (!isArrayLike(value)) {
+ return values(value);
+ }
+ return isObject(value) ? value : Object(value);
+}
+
+module.exports = toIterable;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/toObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/toObject.js b/node_modules/lodash/internal/toObject.js
new file mode 100644
index 0000000..da4a008
--- /dev/null
+++ b/node_modules/lodash/internal/toObject.js
@@ -0,0 +1,14 @@
+var isObject = require('../lang/isObject');
+
+/**
+ * Converts `value` to an object if it's not one.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {Object} Returns the object.
+ */
+function toObject(value) {
+ return isObject(value) ? value : Object(value);
+}
+
+module.exports = toObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/toPath.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/toPath.js b/node_modules/lodash/internal/toPath.js
new file mode 100644
index 0000000..d29f1eb
--- /dev/null
+++ b/node_modules/lodash/internal/toPath.js
@@ -0,0 +1,28 @@
+var baseToString = require('./baseToString'),
+ isArray = require('../lang/isArray');
+
+/** Used to match property names within property paths. */
+var rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\n\\]|\\.)*?)\2)\]/g;
+
+/** Used to match backslashes in property paths. */
+var reEscapeChar = /\\(\\)?/g;
+
+/**
+ * Converts `value` to property path array if it's not one.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {Array} Returns the property path array.
+ */
+function toPath(value) {
+ if (isArray(value)) {
+ return value;
+ }
+ var result = [];
+ baseToString(value).replace(rePropName, function(match, number, quote, string) {
+ result.push(quote ? string.replace(reEscapeChar, '$1') : (number || match));
+ });
+ return result;
+}
+
+module.exports = toPath;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/trimmedLeftIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/trimmedLeftIndex.js b/node_modules/lodash/internal/trimmedLeftIndex.js
new file mode 100644
index 0000000..08aeb13
--- /dev/null
+++ b/node_modules/lodash/internal/trimmedLeftIndex.js
@@ -0,0 +1,19 @@
+var isSpace = require('./isSpace');
+
+/**
+ * Used by `_.trim` and `_.trimLeft` to get the index of the first non-whitespace
+ * character of `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the index of the first non-whitespace character.
+ */
+function trimmedLeftIndex(string) {
+ var index = -1,
+ length = string.length;
+
+ while (++index < length && isSpace(string.charCodeAt(index))) {}
+ return index;
+}
+
+module.exports = trimmedLeftIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/trimmedRightIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/trimmedRightIndex.js b/node_modules/lodash/internal/trimmedRightIndex.js
new file mode 100644
index 0000000..71b9e38
--- /dev/null
+++ b/node_modules/lodash/internal/trimmedRightIndex.js
@@ -0,0 +1,18 @@
+var isSpace = require('./isSpace');
+
+/**
+ * Used by `_.trim` and `_.trimRight` to get the index of the last non-whitespace
+ * character of `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the index of the last non-whitespace character.
+ */
+function trimmedRightIndex(string) {
+ var index = string.length;
+
+ while (index-- && isSpace(string.charCodeAt(index))) {}
+ return index;
+}
+
+module.exports = trimmedRightIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/unescapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/unescapeHtmlChar.js b/node_modules/lodash/internal/unescapeHtmlChar.js
new file mode 100644
index 0000000..28b3454
--- /dev/null
+++ b/node_modules/lodash/internal/unescapeHtmlChar.js
@@ -0,0 +1,22 @@
+/** Used to map HTML entities to characters. */
+var htmlUnescapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'",
+ '`': '`'
+};
+
+/**
+ * Used by `_.unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} chr The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+function unescapeHtmlChar(chr) {
+ return htmlUnescapes[chr];
+}
+
+module.exports = unescapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/wrapperClone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/wrapperClone.js b/node_modules/lodash/internal/wrapperClone.js
new file mode 100644
index 0000000..e5e10da
--- /dev/null
+++ b/node_modules/lodash/internal/wrapperClone.js
@@ -0,0 +1,18 @@
+var LazyWrapper = require('./LazyWrapper'),
+ LodashWrapper = require('./LodashWrapper'),
+ arrayCopy = require('./arrayCopy');
+
+/**
+ * Creates a clone of `wrapper`.
+ *
+ * @private
+ * @param {Object} wrapper The wrapper to clone.
+ * @returns {Object} Returns the cloned wrapper.
+ */
+function wrapperClone(wrapper) {
+ return wrapper instanceof LazyWrapper
+ ? wrapper.clone()
+ : new LodashWrapper(wrapper.__wrapped__, wrapper.__chain__, arrayCopy(wrapper.__actions__));
+}
+
+module.exports = wrapperClone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang.js b/node_modules/lodash/lang.js
new file mode 100644
index 0000000..8f0a364
--- /dev/null
+++ b/node_modules/lodash/lang.js
@@ -0,0 +1,32 @@
+module.exports = {
+ 'clone': require('./lang/clone'),
+ 'cloneDeep': require('./lang/cloneDeep'),
+ 'eq': require('./lang/eq'),
+ 'gt': require('./lang/gt'),
+ 'gte': require('./lang/gte'),
+ 'isArguments': require('./lang/isArguments'),
+ 'isArray': require('./lang/isArray'),
+ 'isBoolean': require('./lang/isBoolean'),
+ 'isDate': require('./lang/isDate'),
+ 'isElement': require('./lang/isElement'),
+ 'isEmpty': require('./lang/isEmpty'),
+ 'isEqual': require('./lang/isEqual'),
+ 'isError': require('./lang/isError'),
+ 'isFinite': require('./lang/isFinite'),
+ 'isFunction': require('./lang/isFunction'),
+ 'isMatch': require('./lang/isMatch'),
+ 'isNaN': require('./lang/isNaN'),
+ 'isNative': require('./lang/isNative'),
+ 'isNull': require('./lang/isNull'),
+ 'isNumber': require('./lang/isNumber'),
+ 'isObject': require('./lang/isObject'),
+ 'isPlainObject': require('./lang/isPlainObject'),
+ 'isRegExp': require('./lang/isRegExp'),
+ 'isString': require('./lang/isString'),
+ 'isTypedArray': require('./lang/isTypedArray'),
+ 'isUndefined': require('./lang/isUndefined'),
+ 'lt': require('./lang/lt'),
+ 'lte': require('./lang/lte'),
+ 'toArray': require('./lang/toArray'),
+ 'toPlainObject': require('./lang/toPlainObject')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/clone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/clone.js b/node_modules/lodash/lang/clone.js
new file mode 100644
index 0000000..85ee8fe
--- /dev/null
+++ b/node_modules/lodash/lang/clone.js
@@ -0,0 +1,70 @@
+var baseClone = require('../internal/baseClone'),
+ bindCallback = require('../internal/bindCallback'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates a clone of `value`. If `isDeep` is `true` nested objects are cloned,
+ * otherwise they are assigned by reference. If `customizer` is provided it's
+ * invoked to produce the cloned values. If `customizer` returns `undefined`
+ * cloning is handled by the method instead. The `customizer` is bound to
+ * `thisArg` and invoked with up to three argument; (value [, index|key, object]).
+ *
+ * **Note:** This method is loosely based on the
+ * [structured clone algorithm](http://www.w3.org/TR/html5/infrastructure.html#internal-structured-cloning-algorithm).
+ * The enumerable properties of `arguments` objects and objects created by
+ * constructors other than `Object` are cloned to plain `Object` objects. An
+ * empty object is returned for uncloneable values such as functions, DOM nodes,
+ * Maps, Sets, and WeakMaps.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @param {Function} [customizer] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {*} Returns the cloned value.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney' },
+ * { 'user': 'fred' }
+ * ];
+ *
+ * var shallow = _.clone(users);
+ * shallow[0] === users[0];
+ * // => true
+ *
+ * var deep = _.clone(users, true);
+ * deep[0] === users[0];
+ * // => false
+ *
+ * // using a customizer callback
+ * var el = _.clone(document.body, function(value) {
+ * if (_.isElement(value)) {
+ * return value.cloneNode(false);
+ * }
+ * });
+ *
+ * el === document.body
+ * // => false
+ * el.nodeName
+ * // => BODY
+ * el.childNodes.length;
+ * // => 0
+ */
+function clone(value, isDeep, customizer, thisArg) {
+ if (isDeep && typeof isDeep != 'boolean' && isIterateeCall(value, isDeep, customizer)) {
+ isDeep = false;
+ }
+ else if (typeof isDeep == 'function') {
+ thisArg = customizer;
+ customizer = isDeep;
+ isDeep = false;
+ }
+ return typeof customizer == 'function'
+ ? baseClone(value, isDeep, bindCallback(customizer, thisArg, 3))
+ : baseClone(value, isDeep);
+}
+
+module.exports = clone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/cloneDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/cloneDeep.js b/node_modules/lodash/lang/cloneDeep.js
new file mode 100644
index 0000000..c4d2517
--- /dev/null
+++ b/node_modules/lodash/lang/cloneDeep.js
@@ -0,0 +1,55 @@
+var baseClone = require('../internal/baseClone'),
+ bindCallback = require('../internal/bindCallback');
+
+/**
+ * Creates a deep clone of `value`. If `customizer` is provided it's invoked
+ * to produce the cloned values. If `customizer` returns `undefined` cloning
+ * is handled by the method instead. The `customizer` is bound to `thisArg`
+ * and invoked with up to three argument; (value [, index|key, object]).
+ *
+ * **Note:** This method is loosely based on the
+ * [structured clone algorithm](http://www.w3.org/TR/html5/infrastructure.html#internal-structured-cloning-algorithm).
+ * The enumerable properties of `arguments` objects and objects created by
+ * constructors other than `Object` are cloned to plain `Object` objects. An
+ * empty object is returned for uncloneable values such as functions, DOM nodes,
+ * Maps, Sets, and WeakMaps.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to deep clone.
+ * @param {Function} [customizer] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {*} Returns the deep cloned value.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney' },
+ * { 'user': 'fred' }
+ * ];
+ *
+ * var deep = _.cloneDeep(users);
+ * deep[0] === users[0];
+ * // => false
+ *
+ * // using a customizer callback
+ * var el = _.cloneDeep(document.body, function(value) {
+ * if (_.isElement(value)) {
+ * return value.cloneNode(true);
+ * }
+ * });
+ *
+ * el === document.body
+ * // => false
+ * el.nodeName
+ * // => BODY
+ * el.childNodes.length;
+ * // => 20
+ */
+function cloneDeep(value, customizer, thisArg) {
+ return typeof customizer == 'function'
+ ? baseClone(value, true, bindCallback(customizer, thisArg, 3))
+ : baseClone(value, true);
+}
+
+module.exports = cloneDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/eq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/eq.js b/node_modules/lodash/lang/eq.js
new file mode 100644
index 0000000..e6a5ce0
--- /dev/null
+++ b/node_modules/lodash/lang/eq.js
@@ -0,0 +1 @@
+module.exports = require('./isEqual');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/gt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/gt.js b/node_modules/lodash/lang/gt.js
new file mode 100644
index 0000000..ddaf5ea
--- /dev/null
+++ b/node_modules/lodash/lang/gt.js
@@ -0,0 +1,25 @@
+/**
+ * Checks if `value` is greater than `other`.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than `other`, else `false`.
+ * @example
+ *
+ * _.gt(3, 1);
+ * // => true
+ *
+ * _.gt(3, 3);
+ * // => false
+ *
+ * _.gt(1, 3);
+ * // => false
+ */
+function gt(value, other) {
+ return value > other;
+}
+
+module.exports = gt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/gte.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/gte.js b/node_modules/lodash/lang/gte.js
new file mode 100644
index 0000000..4a5ffb5
--- /dev/null
+++ b/node_modules/lodash/lang/gte.js
@@ -0,0 +1,25 @@
+/**
+ * Checks if `value` is greater than or equal to `other`.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is greater than or equal to `other`, else `false`.
+ * @example
+ *
+ * _.gte(3, 1);
+ * // => true
+ *
+ * _.gte(3, 3);
+ * // => true
+ *
+ * _.gte(1, 3);
+ * // => false
+ */
+function gte(value, other) {
+ return value >= other;
+}
+
+module.exports = gte;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isArguments.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isArguments.js b/node_modules/lodash/lang/isArguments.js
new file mode 100644
index 0000000..ce9763d
--- /dev/null
+++ b/node_modules/lodash/lang/isArguments.js
@@ -0,0 +1,34 @@
+var isArrayLike = require('../internal/isArrayLike'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Native method references. */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * Checks if `value` is classified as an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ return isObjectLike(value) && isArrayLike(value) &&
+ hasOwnProperty.call(value, 'callee') && !propertyIsEnumerable.call(value, 'callee');
+}
+
+module.exports = isArguments;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isArray.js b/node_modules/lodash/lang/isArray.js
new file mode 100644
index 0000000..9ab023a
--- /dev/null
+++ b/node_modules/lodash/lang/isArray.js
@@ -0,0 +1,40 @@
+var getNative = require('../internal/getNative'),
+ isLength = require('../internal/isLength'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var arrayTag = '[object Array]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeIsArray = getNative(Array, 'isArray');
+
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(function() { return arguments; }());
+ * // => false
+ */
+var isArray = nativeIsArray || function(value) {
+ return isObjectLike(value) && isLength(value.length) && objToString.call(value) == arrayTag;
+};
+
+module.exports = isArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isBoolean.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isBoolean.js b/node_modules/lodash/lang/isBoolean.js
new file mode 100644
index 0000000..460e6c5
--- /dev/null
+++ b/node_modules/lodash/lang/isBoolean.js
@@ -0,0 +1,35 @@
+var isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a boolean primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isBoolean(false);
+ * // => true
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+function isBoolean(value) {
+ return value === true || value === false || (isObjectLike(value) && objToString.call(value) == boolTag);
+}
+
+module.exports = isBoolean;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isDate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isDate.js b/node_modules/lodash/lang/isDate.js
new file mode 100644
index 0000000..29850d9
--- /dev/null
+++ b/node_modules/lodash/lang/isDate.js
@@ -0,0 +1,35 @@
+var isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var dateTag = '[object Date]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `Date` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ *
+ * _.isDate('Mon April 23 2012');
+ * // => false
+ */
+function isDate(value) {
+ return isObjectLike(value) && objToString.call(value) == dateTag;
+}
+
+module.exports = isDate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isElement.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isElement.js b/node_modules/lodash/lang/isElement.js
new file mode 100644
index 0000000..2e9c970
--- /dev/null
+++ b/node_modules/lodash/lang/isElement.js
@@ -0,0 +1,24 @@
+var isObjectLike = require('../internal/isObjectLike'),
+ isPlainObject = require('./isPlainObject');
+
+/**
+ * Checks if `value` is a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ *
+ * _.isElement('<body>');
+ * // => false
+ */
+function isElement(value) {
+ return !!value && value.nodeType === 1 && isObjectLike(value) && !isPlainObject(value);
+}
+
+module.exports = isElement;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isEmpty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isEmpty.js b/node_modules/lodash/lang/isEmpty.js
new file mode 100644
index 0000000..6b344a0
--- /dev/null
+++ b/node_modules/lodash/lang/isEmpty.js
@@ -0,0 +1,47 @@
+var isArguments = require('./isArguments'),
+ isArray = require('./isArray'),
+ isArrayLike = require('../internal/isArrayLike'),
+ isFunction = require('./isFunction'),
+ isObjectLike = require('../internal/isObjectLike'),
+ isString = require('./isString'),
+ keys = require('../object/keys');
+
+/**
+ * Checks if `value` is empty. A value is considered empty unless it's an
+ * `arguments` object, array, string, or jQuery-like collection with a length
+ * greater than `0` or an object with own enumerable properties.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {Array|Object|string} value The value to inspect.
+ * @returns {boolean} Returns `true` if `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty(null);
+ * // => true
+ *
+ * _.isEmpty(true);
+ * // => true
+ *
+ * _.isEmpty(1);
+ * // => true
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({ 'a': 1 });
+ * // => false
+ */
+function isEmpty(value) {
+ if (value == null) {
+ return true;
+ }
+ if (isArrayLike(value) && (isArray(value) || isString(value) || isArguments(value) ||
+ (isObjectLike(value) && isFunction(value.splice)))) {
+ return !value.length;
+ }
+ return !keys(value).length;
+}
+
+module.exports = isEmpty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isEqual.js b/node_modules/lodash/lang/isEqual.js
new file mode 100644
index 0000000..41bf568
--- /dev/null
+++ b/node_modules/lodash/lang/isEqual.js
@@ -0,0 +1,54 @@
+var baseIsEqual = require('../internal/baseIsEqual'),
+ bindCallback = require('../internal/bindCallback');
+
+/**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent. If `customizer` is provided it's invoked to compare values.
+ * If `customizer` returns `undefined` comparisons are handled by the method
+ * instead. The `customizer` is bound to `thisArg` and invoked with up to
+ * three arguments: (value, other [, index|key]).
+ *
+ * **Note:** This method supports comparing arrays, booleans, `Date` objects,
+ * numbers, `Object` objects, regexes, and strings. Objects are compared by
+ * their own, not inherited, enumerable properties. Functions and DOM nodes
+ * are **not** supported. Provide a customizer function to extend support
+ * for comparing other values.
+ *
+ * @static
+ * @memberOf _
+ * @alias eq
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize value comparisons.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'user': 'fred' };
+ * var other = { 'user': 'fred' };
+ *
+ * object == other;
+ * // => false
+ *
+ * _.isEqual(object, other);
+ * // => true
+ *
+ * // using a customizer callback
+ * var array = ['hello', 'goodbye'];
+ * var other = ['hi', 'goodbye'];
+ *
+ * _.isEqual(array, other, function(value, other) {
+ * if (_.every([value, other], RegExp.prototype.test, /^h(?:i|ello)$/)) {
+ * return true;
+ * }
+ * });
+ * // => true
+ */
+function isEqual(value, other, customizer, thisArg) {
+ customizer = typeof customizer == 'function' ? bindCallback(customizer, thisArg, 3) : undefined;
+ var result = customizer ? customizer(value, other) : undefined;
+ return result === undefined ? baseIsEqual(value, other, customizer) : !!result;
+}
+
+module.exports = isEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isError.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isError.js b/node_modules/lodash/lang/isError.js
new file mode 100644
index 0000000..a7bb0d0
--- /dev/null
+++ b/node_modules/lodash/lang/isError.js
@@ -0,0 +1,36 @@
+var isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var errorTag = '[object Error]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is an `Error`, `EvalError`, `RangeError`, `ReferenceError`,
+ * `SyntaxError`, `TypeError`, or `URIError` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an error object, else `false`.
+ * @example
+ *
+ * _.isError(new Error);
+ * // => true
+ *
+ * _.isError(Error);
+ * // => false
+ */
+function isError(value) {
+ return isObjectLike(value) && typeof value.message == 'string' && objToString.call(value) == errorTag;
+}
+
+module.exports = isError;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isFinite.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isFinite.js b/node_modules/lodash/lang/isFinite.js
new file mode 100644
index 0000000..e01a307
--- /dev/null
+++ b/node_modules/lodash/lang/isFinite.js
@@ -0,0 +1,35 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeIsFinite = global.isFinite;
+
+/**
+ * Checks if `value` is a finite primitive number.
+ *
+ * **Note:** This method is based on [`Number.isFinite`](http://ecma-international.org/ecma-262/6.0/#sec-number.isfinite).
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a finite number, else `false`.
+ * @example
+ *
+ * _.isFinite(10);
+ * // => true
+ *
+ * _.isFinite('10');
+ * // => false
+ *
+ * _.isFinite(true);
+ * // => false
+ *
+ * _.isFinite(Object(10));
+ * // => false
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ */
+function isFinite(value) {
+ return typeof value == 'number' && nativeIsFinite(value);
+}
+
+module.exports = isFinite;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isFunction.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isFunction.js b/node_modules/lodash/lang/isFunction.js
new file mode 100644
index 0000000..abe5668
--- /dev/null
+++ b/node_modules/lodash/lang/isFunction.js
@@ -0,0 +1,38 @@
+var isObject = require('./isObject');
+
+/** `Object#toString` result references. */
+var funcTag = '[object Function]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in older versions of Chrome and Safari which return 'function' for regexes
+ // and Safari 8 which returns 'object' for typed array constructors.
+ return isObject(value) && objToString.call(value) == funcTag;
+}
+
+module.exports = isFunction;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isMatch.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isMatch.js b/node_modules/lodash/lang/isMatch.js
new file mode 100644
index 0000000..0a51d49
--- /dev/null
+++ b/node_modules/lodash/lang/isMatch.js
@@ -0,0 +1,49 @@
+var baseIsMatch = require('../internal/baseIsMatch'),
+ bindCallback = require('../internal/bindCallback'),
+ getMatchData = require('../internal/getMatchData');
+
+/**
+ * Performs a deep comparison between `object` and `source` to determine if
+ * `object` contains equivalent property values. If `customizer` is provided
+ * it's invoked to compare values. If `customizer` returns `undefined`
+ * comparisons are handled by the method instead. The `customizer` is bound
+ * to `thisArg` and invoked with three arguments: (value, other, index|key).
+ *
+ * **Note:** This method supports comparing properties of arrays, booleans,
+ * `Date` objects, numbers, `Object` objects, regexes, and strings. Functions
+ * and DOM nodes are **not** supported. Provide a customizer function to extend
+ * support for comparing other values.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {Object} object The object to inspect.
+ * @param {Object} source The object of property values to match.
+ * @param {Function} [customizer] The function to customize value comparisons.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ * @example
+ *
+ * var object = { 'user': 'fred', 'age': 40 };
+ *
+ * _.isMatch(object, { 'age': 40 });
+ * // => true
+ *
+ * _.isMatch(object, { 'age': 36 });
+ * // => false
+ *
+ * // using a customizer callback
+ * var object = { 'greeting': 'hello' };
+ * var source = { 'greeting': 'hi' };
+ *
+ * _.isMatch(object, source, function(value, other) {
+ * return _.every([value, other], RegExp.prototype.test, /^h(?:i|ello)$/) || undefined;
+ * });
+ * // => true
+ */
+function isMatch(object, source, customizer, thisArg) {
+ customizer = typeof customizer == 'function' ? bindCallback(customizer, thisArg, 3) : undefined;
+ return baseIsMatch(object, getMatchData(source), customizer);
+}
+
+module.exports = isMatch;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isNaN.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isNaN.js b/node_modules/lodash/lang/isNaN.js
new file mode 100644
index 0000000..cf83d56
--- /dev/null
+++ b/node_modules/lodash/lang/isNaN.js
@@ -0,0 +1,34 @@
+var isNumber = require('./isNumber');
+
+/**
+ * Checks if `value` is `NaN`.
+ *
+ * **Note:** This method is not the same as [`isNaN`](https://es5.github.io/#x15.1.2.4)
+ * which returns `true` for `undefined` and other non-numeric values.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+function isNaN(value) {
+ // An `NaN` primitive is the only value that is not equal to itself.
+ // Perform the `toStringTag` check first to avoid errors with some host objects in IE.
+ return isNumber(value) && value != +value;
+}
+
+module.exports = isNaN;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isNative.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isNative.js b/node_modules/lodash/lang/isNative.js
new file mode 100644
index 0000000..3ad7144
--- /dev/null
+++ b/node_modules/lodash/lang/isNative.js
@@ -0,0 +1,48 @@
+var isFunction = require('./isFunction'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** Used to detect host constructors (Safari > 5). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var fnToString = Function.prototype.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ fnToString.call(hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function, else `false`.
+ * @example
+ *
+ * _.isNative(Array.prototype.push);
+ * // => true
+ *
+ * _.isNative(_);
+ * // => false
+ */
+function isNative(value) {
+ if (value == null) {
+ return false;
+ }
+ if (isFunction(value)) {
+ return reIsNative.test(fnToString.call(value));
+ }
+ return isObjectLike(value) && reIsHostCtor.test(value);
+}
+
+module.exports = isNative;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isNull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isNull.js b/node_modules/lodash/lang/isNull.js
new file mode 100644
index 0000000..ec66c4d
--- /dev/null
+++ b/node_modules/lodash/lang/isNull.js
@@ -0,0 +1,21 @@
+/**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(void 0);
+ * // => false
+ */
+function isNull(value) {
+ return value === null;
+}
+
+module.exports = isNull;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isNumber.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isNumber.js b/node_modules/lodash/lang/isNumber.js
new file mode 100644
index 0000000..6764d6f
--- /dev/null
+++ b/node_modules/lodash/lang/isNumber.js
@@ -0,0 +1,41 @@
+var isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var numberTag = '[object Number]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `Number` primitive or object.
+ *
+ * **Note:** To exclude `Infinity`, `-Infinity`, and `NaN`, which are classified
+ * as numbers, use the `_.isFinite` method.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isNumber(8.4);
+ * // => true
+ *
+ * _.isNumber(NaN);
+ * // => true
+ *
+ * _.isNumber('8.4');
+ * // => false
+ */
+function isNumber(value) {
+ return typeof value == 'number' || (isObjectLike(value) && objToString.call(value) == numberTag);
+}
+
+module.exports = isNumber;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isObject.js b/node_modules/lodash/lang/isObject.js
new file mode 100644
index 0000000..6db5998
--- /dev/null
+++ b/node_modules/lodash/lang/isObject.js
@@ -0,0 +1,28 @@
+/**
+ * Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // Avoid a V8 JIT bug in Chrome 19-20.
+ // See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+module.exports = isObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isPlainObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isPlainObject.js b/node_modules/lodash/lang/isPlainObject.js
new file mode 100644
index 0000000..5b34c83
--- /dev/null
+++ b/node_modules/lodash/lang/isPlainObject.js
@@ -0,0 +1,71 @@
+var baseForIn = require('../internal/baseForIn'),
+ isArguments = require('./isArguments'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var objectTag = '[object Object]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is a plain object, that is, an object created by the
+ * `Object` constructor or one with a `[[Prototype]]` of `null`.
+ *
+ * **Note:** This method assumes objects created by the `Object` constructor
+ * have no inherited enumerable properties.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * _.isPlainObject(new Foo);
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'x': 0, 'y': 0 });
+ * // => true
+ *
+ * _.isPlainObject(Object.create(null));
+ * // => true
+ */
+function isPlainObject(value) {
+ var Ctor;
+
+ // Exit early for non `Object` objects.
+ if (!(isObjectLike(value) && objToString.call(value) == objectTag && !isArguments(value)) ||
+ (!hasOwnProperty.call(value, 'constructor') && (Ctor = value.constructor, typeof Ctor == 'function' && !(Ctor instanceof Ctor)))) {
+ return false;
+ }
+ // IE < 9 iterates inherited properties before own properties. If the first
+ // iterated property is an object's own property then there are no inherited
+ // enumerable properties.
+ var result;
+ // In most environments an object's own properties are iterated before
+ // its inherited properties. If the last iterated property is an object's
+ // own property then there are no inherited enumerable properties.
+ baseForIn(value, function(subValue, key) {
+ result = key;
+ });
+ return result === undefined || hasOwnProperty.call(value, result);
+}
+
+module.exports = isPlainObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isRegExp.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isRegExp.js b/node_modules/lodash/lang/isRegExp.js
new file mode 100644
index 0000000..f029cbd
--- /dev/null
+++ b/node_modules/lodash/lang/isRegExp.js
@@ -0,0 +1,35 @@
+var isObject = require('./isObject');
+
+/** `Object#toString` result references. */
+var regexpTag = '[object RegExp]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `RegExp` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isRegExp(/abc/);
+ * // => true
+ *
+ * _.isRegExp('/abc/');
+ * // => false
+ */
+function isRegExp(value) {
+ return isObject(value) && objToString.call(value) == regexpTag;
+}
+
+module.exports = isRegExp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isString.js b/node_modules/lodash/lang/isString.js
new file mode 100644
index 0000000..8b28ee1
--- /dev/null
+++ b/node_modules/lodash/lang/isString.js
@@ -0,0 +1,35 @@
+var isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var stringTag = '[object String]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `String` primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isString('abc');
+ * // => true
+ *
+ * _.isString(1);
+ * // => false
+ */
+function isString(value) {
+ return typeof value == 'string' || (isObjectLike(value) && objToString.call(value) == stringTag);
+}
+
+module.exports = isString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isTypedArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isTypedArray.js b/node_modules/lodash/lang/isTypedArray.js
new file mode 100644
index 0000000..6e8a6e0
--- /dev/null
+++ b/node_modules/lodash/lang/isTypedArray.js
@@ -0,0 +1,74 @@
+var isLength = require('../internal/isLength'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/** Used to identify `toStringTag` values of typed arrays. */
+var typedArrayTags = {};
+typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
+typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
+typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
+typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
+typedArrayTags[uint32Tag] = true;
+typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
+typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
+typedArrayTags[dateTag] = typedArrayTags[errorTag] =
+typedArrayTags[funcTag] = typedArrayTags[mapTag] =
+typedArrayTags[numberTag] = typedArrayTags[objectTag] =
+typedArrayTags[regexpTag] = typedArrayTags[setTag] =
+typedArrayTags[stringTag] = typedArrayTags[weakMapTag] = false;
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a typed array.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isTypedArray(new Uint8Array);
+ * // => true
+ *
+ * _.isTypedArray([]);
+ * // => false
+ */
+function isTypedArray(value) {
+ return isObjectLike(value) && isLength(value.length) && !!typedArrayTags[objToString.call(value)];
+}
+
+module.exports = isTypedArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/isUndefined.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/isUndefined.js b/node_modules/lodash/lang/isUndefined.js
new file mode 100644
index 0000000..d64e560
--- /dev/null
+++ b/node_modules/lodash/lang/isUndefined.js
@@ -0,0 +1,21 @@
+/**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ *
+ * _.isUndefined(null);
+ * // => false
+ */
+function isUndefined(value) {
+ return value === undefined;
+}
+
+module.exports = isUndefined;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/lt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/lt.js b/node_modules/lodash/lang/lt.js
new file mode 100644
index 0000000..4439870
--- /dev/null
+++ b/node_modules/lodash/lang/lt.js
@@ -0,0 +1,25 @@
+/**
+ * Checks if `value` is less than `other`.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than `other`, else `false`.
+ * @example
+ *
+ * _.lt(1, 3);
+ * // => true
+ *
+ * _.lt(3, 3);
+ * // => false
+ *
+ * _.lt(3, 1);
+ * // => false
+ */
+function lt(value, other) {
+ return value < other;
+}
+
+module.exports = lt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/lte.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/lte.js b/node_modules/lodash/lang/lte.js
new file mode 100644
index 0000000..e2b8ab1
--- /dev/null
+++ b/node_modules/lodash/lang/lte.js
@@ -0,0 +1,25 @@
+/**
+ * Checks if `value` is less than or equal to `other`.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if `value` is less than or equal to `other`, else `false`.
+ * @example
+ *
+ * _.lte(1, 3);
+ * // => true
+ *
+ * _.lte(3, 3);
+ * // => true
+ *
+ * _.lte(3, 1);
+ * // => false
+ */
+function lte(value, other) {
+ return value <= other;
+}
+
+module.exports = lte;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/toArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/toArray.js b/node_modules/lodash/lang/toArray.js
new file mode 100644
index 0000000..72b0b46
--- /dev/null
+++ b/node_modules/lodash/lang/toArray.js
@@ -0,0 +1,32 @@
+var arrayCopy = require('../internal/arrayCopy'),
+ getLength = require('../internal/getLength'),
+ isLength = require('../internal/isLength'),
+ values = require('../object/values');
+
+/**
+ * Converts `value` to an array.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {Array} Returns the converted array.
+ * @example
+ *
+ * (function() {
+ * return _.toArray(arguments).slice(1);
+ * }(1, 2, 3));
+ * // => [2, 3]
+ */
+function toArray(value) {
+ var length = value ? getLength(value) : 0;
+ if (!isLength(length)) {
+ return values(value);
+ }
+ if (!length) {
+ return [];
+ }
+ return arrayCopy(value);
+}
+
+module.exports = toArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/lang/toPlainObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/lang/toPlainObject.js b/node_modules/lodash/lang/toPlainObject.js
new file mode 100644
index 0000000..6315176
--- /dev/null
+++ b/node_modules/lodash/lang/toPlainObject.js
@@ -0,0 +1,31 @@
+var baseCopy = require('../internal/baseCopy'),
+ keysIn = require('../object/keysIn');
+
+/**
+ * Converts `value` to a plain object flattening inherited enumerable
+ * properties of `value` to own properties of the plain object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {Object} Returns the converted plain object.
+ * @example
+ *
+ * function Foo() {
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.assign({ 'a': 1 }, new Foo);
+ * // => { 'a': 1, 'b': 2 }
+ *
+ * _.assign({ 'a': 1 }, _.toPlainObject(new Foo));
+ * // => { 'a': 1, 'b': 2, 'c': 3 }
+ */
+function toPlainObject(value) {
+ return baseCopy(value, keysIn(value));
+}
+
+module.exports = toPlainObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math.js b/node_modules/lodash/math.js
new file mode 100644
index 0000000..21409ce
--- /dev/null
+++ b/node_modules/lodash/math.js
@@ -0,0 +1,9 @@
+module.exports = {
+ 'add': require('./math/add'),
+ 'ceil': require('./math/ceil'),
+ 'floor': require('./math/floor'),
+ 'max': require('./math/max'),
+ 'min': require('./math/min'),
+ 'round': require('./math/round'),
+ 'sum': require('./math/sum')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/add.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/add.js b/node_modules/lodash/math/add.js
new file mode 100644
index 0000000..59ced2f
--- /dev/null
+++ b/node_modules/lodash/math/add.js
@@ -0,0 +1,19 @@
+/**
+ * Adds two numbers.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {number} augend The first number to add.
+ * @param {number} addend The second number to add.
+ * @returns {number} Returns the sum.
+ * @example
+ *
+ * _.add(6, 4);
+ * // => 10
+ */
+function add(augend, addend) {
+ return (+augend || 0) + (+addend || 0);
+}
+
+module.exports = add;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/ceil.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/ceil.js b/node_modules/lodash/math/ceil.js
new file mode 100644
index 0000000..9dbf0c2
--- /dev/null
+++ b/node_modules/lodash/math/ceil.js
@@ -0,0 +1,25 @@
+var createRound = require('../internal/createRound');
+
+/**
+ * Calculates `n` rounded up to `precision`.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {number} n The number to round up.
+ * @param {number} [precision=0] The precision to round up to.
+ * @returns {number} Returns the rounded up number.
+ * @example
+ *
+ * _.ceil(4.006);
+ * // => 5
+ *
+ * _.ceil(6.004, 2);
+ * // => 6.01
+ *
+ * _.ceil(6040, -2);
+ * // => 6100
+ */
+var ceil = createRound('ceil');
+
+module.exports = ceil;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/floor.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/floor.js b/node_modules/lodash/math/floor.js
new file mode 100644
index 0000000..e4dcae8
--- /dev/null
+++ b/node_modules/lodash/math/floor.js
@@ -0,0 +1,25 @@
+var createRound = require('../internal/createRound');
+
+/**
+ * Calculates `n` rounded down to `precision`.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {number} n The number to round down.
+ * @param {number} [precision=0] The precision to round down to.
+ * @returns {number} Returns the rounded down number.
+ * @example
+ *
+ * _.floor(4.006);
+ * // => 4
+ *
+ * _.floor(0.046, 2);
+ * // => 0.04
+ *
+ * _.floor(4060, -2);
+ * // => 4000
+ */
+var floor = createRound('floor');
+
+module.exports = floor;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/max.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/max.js b/node_modules/lodash/math/max.js
new file mode 100644
index 0000000..220c105
--- /dev/null
+++ b/node_modules/lodash/math/max.js
@@ -0,0 +1,56 @@
+var createExtremum = require('../internal/createExtremum'),
+ gt = require('../lang/gt');
+
+/** Used as references for `-Infinity` and `Infinity`. */
+var NEGATIVE_INFINITY = Number.NEGATIVE_INFINITY;
+
+/**
+ * Gets the maximum value of `collection`. If `collection` is empty or falsey
+ * `-Infinity` is returned. If an iteratee function is provided it's invoked
+ * for each value in `collection` to generate the criterion by which the value
+ * is ranked. The `iteratee` is bound to `thisArg` and invoked with three
+ * arguments: (value, index, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {*} Returns the maximum value.
+ * @example
+ *
+ * _.max([4, 2, 8, 6]);
+ * // => 8
+ *
+ * _.max([]);
+ * // => -Infinity
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.max(users, function(chr) {
+ * return chr.age;
+ * });
+ * // => { 'user': 'fred', 'age': 40 }
+ *
+ * // using the `_.property` callback shorthand
+ * _.max(users, 'age');
+ * // => { 'user': 'fred', 'age': 40 }
+ */
+var max = createExtremum(gt, NEGATIVE_INFINITY);
+
+module.exports = max;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[43/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/glob/glob.js
----------------------------------------------------------------------
diff --git a/node_modules/glob/glob.js b/node_modules/glob/glob.js
new file mode 100644
index 0000000..022d2ac
--- /dev/null
+++ b/node_modules/glob/glob.js
@@ -0,0 +1,752 @@
+// Approach:
+//
+// 1. Get the minimatch set
+// 2. For each pattern in the set, PROCESS(pattern, false)
+// 3. Store matches per-set, then uniq them
+//
+// PROCESS(pattern, inGlobStar)
+// Get the first [n] items from pattern that are all strings
+// Join these together. This is PREFIX.
+// If there is no more remaining, then stat(PREFIX) and
+// add to matches if it succeeds. END.
+//
+// If inGlobStar and PREFIX is symlink and points to dir
+// set ENTRIES = []
+// else readdir(PREFIX) as ENTRIES
+// If fail, END
+//
+// with ENTRIES
+// If pattern[n] is GLOBSTAR
+// // handle the case where the globstar match is empty
+// // by pruning it out, and testing the resulting pattern
+// PROCESS(pattern[0..n] + pattern[n+1 .. $], false)
+// // handle other cases.
+// for ENTRY in ENTRIES (not dotfiles)
+// // attach globstar + tail onto the entry
+// // Mark that this entry is a globstar match
+// PROCESS(pattern[0..n] + ENTRY + pattern[n .. $], true)
+//
+// else // not globstar
+// for ENTRY in ENTRIES (not dotfiles, unless pattern[n] is dot)
+// Test ENTRY against pattern[n]
+// If fails, continue
+// If passes, PROCESS(pattern[0..n] + item + pattern[n+1 .. $])
+//
+// Caveat:
+// Cache all stats and readdirs results to minimize syscall. Since all
+// we ever care about is existence and directory-ness, we can just keep
+// `true` for files, and [children,...] for directories, or `false` for
+// things that don't exist.
+
+module.exports = glob
+
+var fs = require('fs')
+var minimatch = require('minimatch')
+var Minimatch = minimatch.Minimatch
+var inherits = require('inherits')
+var EE = require('events').EventEmitter
+var path = require('path')
+var assert = require('assert')
+var isAbsolute = require('path-is-absolute')
+var globSync = require('./sync.js')
+var common = require('./common.js')
+var alphasort = common.alphasort
+var alphasorti = common.alphasorti
+var setopts = common.setopts
+var ownProp = common.ownProp
+var inflight = require('inflight')
+var util = require('util')
+var childrenIgnored = common.childrenIgnored
+var isIgnored = common.isIgnored
+
+var once = require('once')
+
+function glob (pattern, options, cb) {
+ if (typeof options === 'function') cb = options, options = {}
+ if (!options) options = {}
+
+ if (options.sync) {
+ if (cb)
+ throw new TypeError('callback provided to sync glob')
+ return globSync(pattern, options)
+ }
+
+ return new Glob(pattern, options, cb)
+}
+
+glob.sync = globSync
+var GlobSync = glob.GlobSync = globSync.GlobSync
+
+// old api surface
+glob.glob = glob
+
+glob.hasMagic = function (pattern, options_) {
+ var options = util._extend({}, options_)
+ options.noprocess = true
+
+ var g = new Glob(pattern, options)
+ var set = g.minimatch.set
+ if (set.length > 1)
+ return true
+
+ for (var j = 0; j < set[0].length; j++) {
+ if (typeof set[0][j] !== 'string')
+ return true
+ }
+
+ return false
+}
+
+glob.Glob = Glob
+inherits(Glob, EE)
+function Glob (pattern, options, cb) {
+ if (typeof options === 'function') {
+ cb = options
+ options = null
+ }
+
+ if (options && options.sync) {
+ if (cb)
+ throw new TypeError('callback provided to sync glob')
+ return new GlobSync(pattern, options)
+ }
+
+ if (!(this instanceof Glob))
+ return new Glob(pattern, options, cb)
+
+ setopts(this, pattern, options)
+ this._didRealPath = false
+
+ // process each pattern in the minimatch set
+ var n = this.minimatch.set.length
+
+ // The matches are stored as {<filename>: true,...} so that
+ // duplicates are automagically pruned.
+ // Later, we do an Object.keys() on these.
+ // Keep them as a list so we can fill in when nonull is set.
+ this.matches = new Array(n)
+
+ if (typeof cb === 'function') {
+ cb = once(cb)
+ this.on('error', cb)
+ this.on('end', function (matches) {
+ cb(null, matches)
+ })
+ }
+
+ var self = this
+ var n = this.minimatch.set.length
+ this._processing = 0
+ this.matches = new Array(n)
+
+ this._emitQueue = []
+ this._processQueue = []
+ this.paused = false
+
+ if (this.noprocess)
+ return this
+
+ if (n === 0)
+ return done()
+
+ for (var i = 0; i < n; i ++) {
+ this._process(this.minimatch.set[i], i, false, done)
+ }
+
+ function done () {
+ --self._processing
+ if (self._processing <= 0)
+ self._finish()
+ }
+}
+
+Glob.prototype._finish = function () {
+ assert(this instanceof Glob)
+ if (this.aborted)
+ return
+
+ if (this.realpath && !this._didRealpath)
+ return this._realpath()
+
+ common.finish(this)
+ this.emit('end', this.found)
+}
+
+Glob.prototype._realpath = function () {
+ if (this._didRealpath)
+ return
+
+ this._didRealpath = true
+
+ var n = this.matches.length
+ if (n === 0)
+ return this._finish()
+
+ var self = this
+ for (var i = 0; i < this.matches.length; i++)
+ this._realpathSet(i, next)
+
+ function next () {
+ if (--n === 0)
+ self._finish()
+ }
+}
+
+Glob.prototype._realpathSet = function (index, cb) {
+ var matchset = this.matches[index]
+ if (!matchset)
+ return cb()
+
+ var found = Object.keys(matchset)
+ var self = this
+ var n = found.length
+
+ if (n === 0)
+ return cb()
+
+ var set = this.matches[index] = Object.create(null)
+ found.forEach(function (p, i) {
+ // If there's a problem with the stat, then it means that
+ // one or more of the links in the realpath couldn't be
+ // resolved. just return the abs value in that case.
+ p = self._makeAbs(p)
+ fs.realpath(p, self.realpathCache, function (er, real) {
+ if (!er)
+ set[real] = true
+ else if (er.syscall === 'stat')
+ set[p] = true
+ else
+ self.emit('error', er) // srsly wtf right here
+
+ if (--n === 0) {
+ self.matches[index] = set
+ cb()
+ }
+ })
+ })
+}
+
+Glob.prototype._mark = function (p) {
+ return common.mark(this, p)
+}
+
+Glob.prototype._makeAbs = function (f) {
+ return common.makeAbs(this, f)
+}
+
+Glob.prototype.abort = function () {
+ this.aborted = true
+ this.emit('abort')
+}
+
+Glob.prototype.pause = function () {
+ if (!this.paused) {
+ this.paused = true
+ this.emit('pause')
+ }
+}
+
+Glob.prototype.resume = function () {
+ if (this.paused) {
+ this.emit('resume')
+ this.paused = false
+ if (this._emitQueue.length) {
+ var eq = this._emitQueue.slice(0)
+ this._emitQueue.length = 0
+ for (var i = 0; i < eq.length; i ++) {
+ var e = eq[i]
+ this._emitMatch(e[0], e[1])
+ }
+ }
+ if (this._processQueue.length) {
+ var pq = this._processQueue.slice(0)
+ this._processQueue.length = 0
+ for (var i = 0; i < pq.length; i ++) {
+ var p = pq[i]
+ this._processing--
+ this._process(p[0], p[1], p[2], p[3])
+ }
+ }
+ }
+}
+
+Glob.prototype._process = function (pattern, index, inGlobStar, cb) {
+ assert(this instanceof Glob)
+ assert(typeof cb === 'function')
+
+ if (this.aborted)
+ return
+
+ this._processing++
+ if (this.paused) {
+ this._processQueue.push([pattern, index, inGlobStar, cb])
+ return
+ }
+
+ //console.error('PROCESS %d', this._processing, pattern)
+
+ // Get the first [n] parts of pattern that are all strings.
+ var n = 0
+ while (typeof pattern[n] === 'string') {
+ n ++
+ }
+ // now n is the index of the first one that is *not* a string.
+
+ // see if there's anything else
+ var prefix
+ switch (n) {
+ // if not, then this is rather simple
+ case pattern.length:
+ this._processSimple(pattern.join('/'), index, cb)
+ return
+
+ case 0:
+ // pattern *starts* with some non-trivial item.
+ // going to readdir(cwd), but not include the prefix in matches.
+ prefix = null
+ break
+
+ default:
+ // pattern has some string bits in the front.
+ // whatever it starts with, whether that's 'absolute' like /foo/bar,
+ // or 'relative' like '../baz'
+ prefix = pattern.slice(0, n).join('/')
+ break
+ }
+
+ var remain = pattern.slice(n)
+
+ // get the list of entries.
+ var read
+ if (prefix === null)
+ read = '.'
+ else if (isAbsolute(prefix) || isAbsolute(pattern.join('/'))) {
+ if (!prefix || !isAbsolute(prefix))
+ prefix = '/' + prefix
+ read = prefix
+ } else
+ read = prefix
+
+ var abs = this._makeAbs(read)
+
+ //if ignored, skip _processing
+ if (childrenIgnored(this, read))
+ return cb()
+
+ var isGlobStar = remain[0] === minimatch.GLOBSTAR
+ if (isGlobStar)
+ this._processGlobStar(prefix, read, abs, remain, index, inGlobStar, cb)
+ else
+ this._processReaddir(prefix, read, abs, remain, index, inGlobStar, cb)
+}
+
+Glob.prototype._processReaddir = function (prefix, read, abs, remain, index, inGlobStar, cb) {
+ var self = this
+ this._readdir(abs, inGlobStar, function (er, entries) {
+ return self._processReaddir2(prefix, read, abs, remain, index, inGlobStar, entries, cb)
+ })
+}
+
+Glob.prototype._processReaddir2 = function (prefix, read, abs, remain, index, inGlobStar, entries, cb) {
+
+ // if the abs isn't a dir, then nothing can match!
+ if (!entries)
+ return cb()
+
+ // It will only match dot entries if it starts with a dot, or if
+ // dot is set. Stuff like @(.foo|.bar) isn't allowed.
+ var pn = remain[0]
+ var negate = !!this.minimatch.negate
+ var rawGlob = pn._glob
+ var dotOk = this.dot || rawGlob.charAt(0) === '.'
+
+ var matchedEntries = []
+ for (var i = 0; i < entries.length; i++) {
+ var e = entries[i]
+ if (e.charAt(0) !== '.' || dotOk) {
+ var m
+ if (negate && !prefix) {
+ m = !e.match(pn)
+ } else {
+ m = e.match(pn)
+ }
+ if (m)
+ matchedEntries.push(e)
+ }
+ }
+
+ //console.error('prd2', prefix, entries, remain[0]._glob, matchedEntries)
+
+ var len = matchedEntries.length
+ // If there are no matched entries, then nothing matches.
+ if (len === 0)
+ return cb()
+
+ // if this is the last remaining pattern bit, then no need for
+ // an additional stat *unless* the user has specified mark or
+ // stat explicitly. We know they exist, since readdir returned
+ // them.
+
+ if (remain.length === 1 && !this.mark && !this.stat) {
+ if (!this.matches[index])
+ this.matches[index] = Object.create(null)
+
+ for (var i = 0; i < len; i ++) {
+ var e = matchedEntries[i]
+ if (prefix) {
+ if (prefix !== '/')
+ e = prefix + '/' + e
+ else
+ e = prefix + e
+ }
+
+ if (e.charAt(0) === '/' && !this.nomount) {
+ e = path.join(this.root, e)
+ }
+ this._emitMatch(index, e)
+ }
+ // This was the last one, and no stats were needed
+ return cb()
+ }
+
+ // now test all matched entries as stand-ins for that part
+ // of the pattern.
+ remain.shift()
+ for (var i = 0; i < len; i ++) {
+ var e = matchedEntries[i]
+ var newPattern
+ if (prefix) {
+ if (prefix !== '/')
+ e = prefix + '/' + e
+ else
+ e = prefix + e
+ }
+ this._process([e].concat(remain), index, inGlobStar, cb)
+ }
+ cb()
+}
+
+Glob.prototype._emitMatch = function (index, e) {
+ if (this.aborted)
+ return
+
+ if (this.matches[index][e])
+ return
+
+ if (isIgnored(this, e))
+ return
+
+ if (this.paused) {
+ this._emitQueue.push([index, e])
+ return
+ }
+
+ var abs = this._makeAbs(e)
+
+ if (this.nodir) {
+ var c = this.cache[abs]
+ if (c === 'DIR' || Array.isArray(c))
+ return
+ }
+
+ if (this.mark)
+ e = this._mark(e)
+
+ this.matches[index][e] = true
+
+ var st = this.statCache[abs]
+ if (st)
+ this.emit('stat', e, st)
+
+ this.emit('match', e)
+}
+
+Glob.prototype._readdirInGlobStar = function (abs, cb) {
+ if (this.aborted)
+ return
+
+ // follow all symlinked directories forever
+ // just proceed as if this is a non-globstar situation
+ if (this.follow)
+ return this._readdir(abs, false, cb)
+
+ var lstatkey = 'lstat\0' + abs
+ var self = this
+ var lstatcb = inflight(lstatkey, lstatcb_)
+
+ if (lstatcb)
+ fs.lstat(abs, lstatcb)
+
+ function lstatcb_ (er, lstat) {
+ if (er)
+ return cb()
+
+ var isSym = lstat.isSymbolicLink()
+ self.symlinks[abs] = isSym
+
+ // If it's not a symlink or a dir, then it's definitely a regular file.
+ // don't bother doing a readdir in that case.
+ if (!isSym && !lstat.isDirectory()) {
+ self.cache[abs] = 'FILE'
+ cb()
+ } else
+ self._readdir(abs, false, cb)
+ }
+}
+
+Glob.prototype._readdir = function (abs, inGlobStar, cb) {
+ if (this.aborted)
+ return
+
+ cb = inflight('readdir\0'+abs+'\0'+inGlobStar, cb)
+ if (!cb)
+ return
+
+ //console.error('RD %j %j', +inGlobStar, abs)
+ if (inGlobStar && !ownProp(this.symlinks, abs))
+ return this._readdirInGlobStar(abs, cb)
+
+ if (ownProp(this.cache, abs)) {
+ var c = this.cache[abs]
+ if (!c || c === 'FILE')
+ return cb()
+
+ if (Array.isArray(c))
+ return cb(null, c)
+ }
+
+ var self = this
+ fs.readdir(abs, readdirCb(this, abs, cb))
+}
+
+function readdirCb (self, abs, cb) {
+ return function (er, entries) {
+ if (er)
+ self._readdirError(abs, er, cb)
+ else
+ self._readdirEntries(abs, entries, cb)
+ }
+}
+
+Glob.prototype._readdirEntries = function (abs, entries, cb) {
+ if (this.aborted)
+ return
+
+ // if we haven't asked to stat everything, then just
+ // assume that everything in there exists, so we can avoid
+ // having to stat it a second time.
+ if (!this.mark && !this.stat) {
+ for (var i = 0; i < entries.length; i ++) {
+ var e = entries[i]
+ if (abs === '/')
+ e = abs + e
+ else
+ e = abs + '/' + e
+ this.cache[e] = true
+ }
+ }
+
+ this.cache[abs] = entries
+ return cb(null, entries)
+}
+
+Glob.prototype._readdirError = function (f, er, cb) {
+ if (this.aborted)
+ return
+
+ // handle errors, and cache the information
+ switch (er.code) {
+ case 'ENOTSUP': // https://github.com/isaacs/node-glob/issues/205
+ case 'ENOTDIR': // totally normal. means it *does* exist.
+ this.cache[this._makeAbs(f)] = 'FILE'
+ break
+
+ case 'ENOENT': // not terribly unusual
+ case 'ELOOP':
+ case 'ENAMETOOLONG':
+ case 'UNKNOWN':
+ this.cache[this._makeAbs(f)] = false
+ break
+
+ default: // some unusual error. Treat as failure.
+ this.cache[this._makeAbs(f)] = false
+ if (this.strict) {
+ this.emit('error', er)
+ // If the error is handled, then we abort
+ // if not, we threw out of here
+ this.abort()
+ }
+ if (!this.silent)
+ console.error('glob error', er)
+ break
+ }
+
+ return cb()
+}
+
+Glob.prototype._processGlobStar = function (prefix, read, abs, remain, index, inGlobStar, cb) {
+ var self = this
+ this._readdir(abs, inGlobStar, function (er, entries) {
+ self._processGlobStar2(prefix, read, abs, remain, index, inGlobStar, entries, cb)
+ })
+}
+
+
+Glob.prototype._processGlobStar2 = function (prefix, read, abs, remain, index, inGlobStar, entries, cb) {
+ //console.error('pgs2', prefix, remain[0], entries)
+
+ // no entries means not a dir, so it can never have matches
+ // foo.txt/** doesn't match foo.txt
+ if (!entries)
+ return cb()
+
+ // test without the globstar, and with every child both below
+ // and replacing the globstar.
+ var remainWithoutGlobStar = remain.slice(1)
+ var gspref = prefix ? [ prefix ] : []
+ var noGlobStar = gspref.concat(remainWithoutGlobStar)
+
+ // the noGlobStar pattern exits the inGlobStar state
+ this._process(noGlobStar, index, false, cb)
+
+ var isSym = this.symlinks[abs]
+ var len = entries.length
+
+ // If it's a symlink, and we're in a globstar, then stop
+ if (isSym && inGlobStar)
+ return cb()
+
+ for (var i = 0; i < len; i++) {
+ var e = entries[i]
+ if (e.charAt(0) === '.' && !this.dot)
+ continue
+
+ // these two cases enter the inGlobStar state
+ var instead = gspref.concat(entries[i], remainWithoutGlobStar)
+ this._process(instead, index, true, cb)
+
+ var below = gspref.concat(entries[i], remain)
+ this._process(below, index, true, cb)
+ }
+
+ cb()
+}
+
+Glob.prototype._processSimple = function (prefix, index, cb) {
+ // XXX review this. Shouldn't it be doing the mounting etc
+ // before doing stat? kinda weird?
+ var self = this
+ this._stat(prefix, function (er, exists) {
+ self._processSimple2(prefix, index, er, exists, cb)
+ })
+}
+Glob.prototype._processSimple2 = function (prefix, index, er, exists, cb) {
+
+ //console.error('ps2', prefix, exists)
+
+ if (!this.matches[index])
+ this.matches[index] = Object.create(null)
+
+ // If it doesn't exist, then just mark the lack of results
+ if (!exists)
+ return cb()
+
+ if (prefix && isAbsolute(prefix) && !this.nomount) {
+ var trail = /[\/\\]$/.test(prefix)
+ if (prefix.charAt(0) === '/') {
+ prefix = path.join(this.root, prefix)
+ } else {
+ prefix = path.resolve(this.root, prefix)
+ if (trail)
+ prefix += '/'
+ }
+ }
+
+ if (process.platform === 'win32')
+ prefix = prefix.replace(/\\/g, '/')
+
+ // Mark this as a match
+ this._emitMatch(index, prefix)
+ cb()
+}
+
+// Returns either 'DIR', 'FILE', or false
+Glob.prototype._stat = function (f, cb) {
+ var abs = this._makeAbs(f)
+ var needDir = f.slice(-1) === '/'
+
+ if (f.length > this.maxLength)
+ return cb()
+
+ if (!this.stat && ownProp(this.cache, abs)) {
+ var c = this.cache[abs]
+
+ if (Array.isArray(c))
+ c = 'DIR'
+
+ // It exists, but maybe not how we need it
+ if (!needDir || c === 'DIR')
+ return cb(null, c)
+
+ if (needDir && c === 'FILE')
+ return cb()
+
+ // otherwise we have to stat, because maybe c=true
+ // if we know it exists, but not what it is.
+ }
+
+ var exists
+ var stat = this.statCache[abs]
+ if (stat !== undefined) {
+ if (stat === false)
+ return cb(null, stat)
+ else {
+ var type = stat.isDirectory() ? 'DIR' : 'FILE'
+ if (needDir && type === 'FILE')
+ return cb()
+ else
+ return cb(null, type, stat)
+ }
+ }
+
+ var self = this
+ var statcb = inflight('stat\0' + abs, lstatcb_)
+ if (statcb)
+ fs.lstat(abs, statcb)
+
+ function lstatcb_ (er, lstat) {
+ if (lstat && lstat.isSymbolicLink()) {
+ // If it's a symlink, then treat it as the target, unless
+ // the target does not exist, then treat it as a file.
+ return fs.stat(abs, function (er, stat) {
+ if (er)
+ self._stat2(f, abs, null, lstat, cb)
+ else
+ self._stat2(f, abs, er, stat, cb)
+ })
+ } else {
+ self._stat2(f, abs, er, lstat, cb)
+ }
+ }
+}
+
+Glob.prototype._stat2 = function (f, abs, er, stat, cb) {
+ if (er) {
+ this.statCache[abs] = false
+ return cb()
+ }
+
+ var needDir = f.slice(-1) === '/'
+ this.statCache[abs] = stat
+
+ if (abs.slice(-1) === '/' && !stat.isDirectory())
+ return cb(null, false, stat)
+
+ var c = stat.isDirectory() ? 'DIR' : 'FILE'
+ this.cache[abs] = this.cache[abs] || c
+
+ if (needDir && c !== 'DIR')
+ return cb()
+
+ return cb(null, c, stat)
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/glob/package.json
----------------------------------------------------------------------
diff --git a/node_modules/glob/package.json b/node_modules/glob/package.json
new file mode 100644
index 0000000..ed9e928
--- /dev/null
+++ b/node_modules/glob/package.json
@@ -0,0 +1,98 @@
+{
+ "_args": [
+ [
+ "glob@^5.0.13",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "glob@>=5.0.13 <6.0.0",
+ "_id": "glob@5.0.15",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/glob",
+ "_nodeVersion": "4.0.0",
+ "_npmUser": {
+ "email": "isaacs@npmjs.com",
+ "name": "isaacs"
+ },
+ "_npmVersion": "3.3.2",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "glob",
+ "raw": "glob@^5.0.13",
+ "rawSpec": "^5.0.13",
+ "scope": null,
+ "spec": ">=5.0.13 <6.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/glob/-/glob-5.0.15.tgz",
+ "_shasum": "1bc936b9e02f4a603fcc222ecf7633d30b8b93b1",
+ "_shrinkwrap": null,
+ "_spec": "glob@^5.0.13",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me/"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/node-glob/issues"
+ },
+ "dependencies": {
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "2 || 3",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "description": "a little globber",
+ "devDependencies": {
+ "mkdirp": "0",
+ "rimraf": "^2.2.8",
+ "tap": "^1.1.4",
+ "tick": "0.0.6"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "1bc936b9e02f4a603fcc222ecf7633d30b8b93b1",
+ "tarball": "http://registry.npmjs.org/glob/-/glob-5.0.15.tgz"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "files": [
+ "common.js",
+ "glob.js",
+ "sync.js"
+ ],
+ "gitHead": "3a7e71d453dd80e75b196fd262dd23ed54beeceb",
+ "homepage": "https://github.com/isaacs/node-glob#readme",
+ "license": "ISC",
+ "main": "glob.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "name": "glob",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/node-glob.git"
+ },
+ "scripts": {
+ "bench": "bash benchmark.sh",
+ "benchclean": "node benchclean.js",
+ "prepublish": "npm run benchclean",
+ "prof": "bash prof.sh && cat profile.txt",
+ "profclean": "rm -f v8.log profile.txt",
+ "test": "tap test/*.js --cov",
+ "test-regen": "npm run profclean && TEST_REGEN=1 node test/00-setup.js"
+ },
+ "version": "5.0.15"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/glob/sync.js
----------------------------------------------------------------------
diff --git a/node_modules/glob/sync.js b/node_modules/glob/sync.js
new file mode 100644
index 0000000..09883d2
--- /dev/null
+++ b/node_modules/glob/sync.js
@@ -0,0 +1,460 @@
+module.exports = globSync
+globSync.GlobSync = GlobSync
+
+var fs = require('fs')
+var minimatch = require('minimatch')
+var Minimatch = minimatch.Minimatch
+var Glob = require('./glob.js').Glob
+var util = require('util')
+var path = require('path')
+var assert = require('assert')
+var isAbsolute = require('path-is-absolute')
+var common = require('./common.js')
+var alphasort = common.alphasort
+var alphasorti = common.alphasorti
+var setopts = common.setopts
+var ownProp = common.ownProp
+var childrenIgnored = common.childrenIgnored
+
+function globSync (pattern, options) {
+ if (typeof options === 'function' || arguments.length === 3)
+ throw new TypeError('callback provided to sync glob\n'+
+ 'See: https://github.com/isaacs/node-glob/issues/167')
+
+ return new GlobSync(pattern, options).found
+}
+
+function GlobSync (pattern, options) {
+ if (!pattern)
+ throw new Error('must provide pattern')
+
+ if (typeof options === 'function' || arguments.length === 3)
+ throw new TypeError('callback provided to sync glob\n'+
+ 'See: https://github.com/isaacs/node-glob/issues/167')
+
+ if (!(this instanceof GlobSync))
+ return new GlobSync(pattern, options)
+
+ setopts(this, pattern, options)
+
+ if (this.noprocess)
+ return this
+
+ var n = this.minimatch.set.length
+ this.matches = new Array(n)
+ for (var i = 0; i < n; i ++) {
+ this._process(this.minimatch.set[i], i, false)
+ }
+ this._finish()
+}
+
+GlobSync.prototype._finish = function () {
+ assert(this instanceof GlobSync)
+ if (this.realpath) {
+ var self = this
+ this.matches.forEach(function (matchset, index) {
+ var set = self.matches[index] = Object.create(null)
+ for (var p in matchset) {
+ try {
+ p = self._makeAbs(p)
+ var real = fs.realpathSync(p, self.realpathCache)
+ set[real] = true
+ } catch (er) {
+ if (er.syscall === 'stat')
+ set[self._makeAbs(p)] = true
+ else
+ throw er
+ }
+ }
+ })
+ }
+ common.finish(this)
+}
+
+
+GlobSync.prototype._process = function (pattern, index, inGlobStar) {
+ assert(this instanceof GlobSync)
+
+ // Get the first [n] parts of pattern that are all strings.
+ var n = 0
+ while (typeof pattern[n] === 'string') {
+ n ++
+ }
+ // now n is the index of the first one that is *not* a string.
+
+ // See if there's anything else
+ var prefix
+ switch (n) {
+ // if not, then this is rather simple
+ case pattern.length:
+ this._processSimple(pattern.join('/'), index)
+ return
+
+ case 0:
+ // pattern *starts* with some non-trivial item.
+ // going to readdir(cwd), but not include the prefix in matches.
+ prefix = null
+ break
+
+ default:
+ // pattern has some string bits in the front.
+ // whatever it starts with, whether that's 'absolute' like /foo/bar,
+ // or 'relative' like '../baz'
+ prefix = pattern.slice(0, n).join('/')
+ break
+ }
+
+ var remain = pattern.slice(n)
+
+ // get the list of entries.
+ var read
+ if (prefix === null)
+ read = '.'
+ else if (isAbsolute(prefix) || isAbsolute(pattern.join('/'))) {
+ if (!prefix || !isAbsolute(prefix))
+ prefix = '/' + prefix
+ read = prefix
+ } else
+ read = prefix
+
+ var abs = this._makeAbs(read)
+
+ //if ignored, skip processing
+ if (childrenIgnored(this, read))
+ return
+
+ var isGlobStar = remain[0] === minimatch.GLOBSTAR
+ if (isGlobStar)
+ this._processGlobStar(prefix, read, abs, remain, index, inGlobStar)
+ else
+ this._processReaddir(prefix, read, abs, remain, index, inGlobStar)
+}
+
+
+GlobSync.prototype._processReaddir = function (prefix, read, abs, remain, index, inGlobStar) {
+ var entries = this._readdir(abs, inGlobStar)
+
+ // if the abs isn't a dir, then nothing can match!
+ if (!entries)
+ return
+
+ // It will only match dot entries if it starts with a dot, or if
+ // dot is set. Stuff like @(.foo|.bar) isn't allowed.
+ var pn = remain[0]
+ var negate = !!this.minimatch.negate
+ var rawGlob = pn._glob
+ var dotOk = this.dot || rawGlob.charAt(0) === '.'
+
+ var matchedEntries = []
+ for (var i = 0; i < entries.length; i++) {
+ var e = entries[i]
+ if (e.charAt(0) !== '.' || dotOk) {
+ var m
+ if (negate && !prefix) {
+ m = !e.match(pn)
+ } else {
+ m = e.match(pn)
+ }
+ if (m)
+ matchedEntries.push(e)
+ }
+ }
+
+ var len = matchedEntries.length
+ // If there are no matched entries, then nothing matches.
+ if (len === 0)
+ return
+
+ // if this is the last remaining pattern bit, then no need for
+ // an additional stat *unless* the user has specified mark or
+ // stat explicitly. We know they exist, since readdir returned
+ // them.
+
+ if (remain.length === 1 && !this.mark && !this.stat) {
+ if (!this.matches[index])
+ this.matches[index] = Object.create(null)
+
+ for (var i = 0; i < len; i ++) {
+ var e = matchedEntries[i]
+ if (prefix) {
+ if (prefix.slice(-1) !== '/')
+ e = prefix + '/' + e
+ else
+ e = prefix + e
+ }
+
+ if (e.charAt(0) === '/' && !this.nomount) {
+ e = path.join(this.root, e)
+ }
+ this.matches[index][e] = true
+ }
+ // This was the last one, and no stats were needed
+ return
+ }
+
+ // now test all matched entries as stand-ins for that part
+ // of the pattern.
+ remain.shift()
+ for (var i = 0; i < len; i ++) {
+ var e = matchedEntries[i]
+ var newPattern
+ if (prefix)
+ newPattern = [prefix, e]
+ else
+ newPattern = [e]
+ this._process(newPattern.concat(remain), index, inGlobStar)
+ }
+}
+
+
+GlobSync.prototype._emitMatch = function (index, e) {
+ var abs = this._makeAbs(e)
+ if (this.mark)
+ e = this._mark(e)
+
+ if (this.matches[index][e])
+ return
+
+ if (this.nodir) {
+ var c = this.cache[this._makeAbs(e)]
+ if (c === 'DIR' || Array.isArray(c))
+ return
+ }
+
+ this.matches[index][e] = true
+ if (this.stat)
+ this._stat(e)
+}
+
+
+GlobSync.prototype._readdirInGlobStar = function (abs) {
+ // follow all symlinked directories forever
+ // just proceed as if this is a non-globstar situation
+ if (this.follow)
+ return this._readdir(abs, false)
+
+ var entries
+ var lstat
+ var stat
+ try {
+ lstat = fs.lstatSync(abs)
+ } catch (er) {
+ // lstat failed, doesn't exist
+ return null
+ }
+
+ var isSym = lstat.isSymbolicLink()
+ this.symlinks[abs] = isSym
+
+ // If it's not a symlink or a dir, then it's definitely a regular file.
+ // don't bother doing a readdir in that case.
+ if (!isSym && !lstat.isDirectory())
+ this.cache[abs] = 'FILE'
+ else
+ entries = this._readdir(abs, false)
+
+ return entries
+}
+
+GlobSync.prototype._readdir = function (abs, inGlobStar) {
+ var entries
+
+ if (inGlobStar && !ownProp(this.symlinks, abs))
+ return this._readdirInGlobStar(abs)
+
+ if (ownProp(this.cache, abs)) {
+ var c = this.cache[abs]
+ if (!c || c === 'FILE')
+ return null
+
+ if (Array.isArray(c))
+ return c
+ }
+
+ try {
+ return this._readdirEntries(abs, fs.readdirSync(abs))
+ } catch (er) {
+ this._readdirError(abs, er)
+ return null
+ }
+}
+
+GlobSync.prototype._readdirEntries = function (abs, entries) {
+ // if we haven't asked to stat everything, then just
+ // assume that everything in there exists, so we can avoid
+ // having to stat it a second time.
+ if (!this.mark && !this.stat) {
+ for (var i = 0; i < entries.length; i ++) {
+ var e = entries[i]
+ if (abs === '/')
+ e = abs + e
+ else
+ e = abs + '/' + e
+ this.cache[e] = true
+ }
+ }
+
+ this.cache[abs] = entries
+
+ // mark and cache dir-ness
+ return entries
+}
+
+GlobSync.prototype._readdirError = function (f, er) {
+ // handle errors, and cache the information
+ switch (er.code) {
+ case 'ENOTSUP': // https://github.com/isaacs/node-glob/issues/205
+ case 'ENOTDIR': // totally normal. means it *does* exist.
+ this.cache[this._makeAbs(f)] = 'FILE'
+ break
+
+ case 'ENOENT': // not terribly unusual
+ case 'ELOOP':
+ case 'ENAMETOOLONG':
+ case 'UNKNOWN':
+ this.cache[this._makeAbs(f)] = false
+ break
+
+ default: // some unusual error. Treat as failure.
+ this.cache[this._makeAbs(f)] = false
+ if (this.strict)
+ throw er
+ if (!this.silent)
+ console.error('glob error', er)
+ break
+ }
+}
+
+GlobSync.prototype._processGlobStar = function (prefix, read, abs, remain, index, inGlobStar) {
+
+ var entries = this._readdir(abs, inGlobStar)
+
+ // no entries means not a dir, so it can never have matches
+ // foo.txt/** doesn't match foo.txt
+ if (!entries)
+ return
+
+ // test without the globstar, and with every child both below
+ // and replacing the globstar.
+ var remainWithoutGlobStar = remain.slice(1)
+ var gspref = prefix ? [ prefix ] : []
+ var noGlobStar = gspref.concat(remainWithoutGlobStar)
+
+ // the noGlobStar pattern exits the inGlobStar state
+ this._process(noGlobStar, index, false)
+
+ var len = entries.length
+ var isSym = this.symlinks[abs]
+
+ // If it's a symlink, and we're in a globstar, then stop
+ if (isSym && inGlobStar)
+ return
+
+ for (var i = 0; i < len; i++) {
+ var e = entries[i]
+ if (e.charAt(0) === '.' && !this.dot)
+ continue
+
+ // these two cases enter the inGlobStar state
+ var instead = gspref.concat(entries[i], remainWithoutGlobStar)
+ this._process(instead, index, true)
+
+ var below = gspref.concat(entries[i], remain)
+ this._process(below, index, true)
+ }
+}
+
+GlobSync.prototype._processSimple = function (prefix, index) {
+ // XXX review this. Shouldn't it be doing the mounting etc
+ // before doing stat? kinda weird?
+ var exists = this._stat(prefix)
+
+ if (!this.matches[index])
+ this.matches[index] = Object.create(null)
+
+ // If it doesn't exist, then just mark the lack of results
+ if (!exists)
+ return
+
+ if (prefix && isAbsolute(prefix) && !this.nomount) {
+ var trail = /[\/\\]$/.test(prefix)
+ if (prefix.charAt(0) === '/') {
+ prefix = path.join(this.root, prefix)
+ } else {
+ prefix = path.resolve(this.root, prefix)
+ if (trail)
+ prefix += '/'
+ }
+ }
+
+ if (process.platform === 'win32')
+ prefix = prefix.replace(/\\/g, '/')
+
+ // Mark this as a match
+ this.matches[index][prefix] = true
+}
+
+// Returns either 'DIR', 'FILE', or false
+GlobSync.prototype._stat = function (f) {
+ var abs = this._makeAbs(f)
+ var needDir = f.slice(-1) === '/'
+
+ if (f.length > this.maxLength)
+ return false
+
+ if (!this.stat && ownProp(this.cache, abs)) {
+ var c = this.cache[abs]
+
+ if (Array.isArray(c))
+ c = 'DIR'
+
+ // It exists, but maybe not how we need it
+ if (!needDir || c === 'DIR')
+ return c
+
+ if (needDir && c === 'FILE')
+ return false
+
+ // otherwise we have to stat, because maybe c=true
+ // if we know it exists, but not what it is.
+ }
+
+ var exists
+ var stat = this.statCache[abs]
+ if (!stat) {
+ var lstat
+ try {
+ lstat = fs.lstatSync(abs)
+ } catch (er) {
+ return false
+ }
+
+ if (lstat.isSymbolicLink()) {
+ try {
+ stat = fs.statSync(abs)
+ } catch (er) {
+ stat = lstat
+ }
+ } else {
+ stat = lstat
+ }
+ }
+
+ this.statCache[abs] = stat
+
+ var c = stat.isDirectory() ? 'DIR' : 'FILE'
+ this.cache[abs] = this.cache[abs] || c
+
+ if (needDir && c !== 'DIR')
+ return false
+
+ return c
+}
+
+GlobSync.prototype._mark = function (p) {
+ return common.mark(this, p)
+}
+
+GlobSync.prototype._makeAbs = function (f) {
+ return common.makeAbs(this, f)
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inflight/.eslintrc
----------------------------------------------------------------------
diff --git a/node_modules/inflight/.eslintrc b/node_modules/inflight/.eslintrc
new file mode 100644
index 0000000..b7a1550
--- /dev/null
+++ b/node_modules/inflight/.eslintrc
@@ -0,0 +1,17 @@
+{
+ "env" : {
+ "node" : true
+ },
+ "rules" : {
+ "semi": [2, "never"],
+ "strict": 0,
+ "quotes": [1, "single", "avoid-escape"],
+ "no-use-before-define": 0,
+ "curly": 0,
+ "no-underscore-dangle": 0,
+ "no-lonely-if": 1,
+ "no-unused-vars": [2, {"vars" : "all", "args" : "after-used"}],
+ "no-mixed-requires": 0,
+ "space-infix-ops": 0
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inflight/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/inflight/LICENSE b/node_modules/inflight/LICENSE
new file mode 100644
index 0000000..05eeeb8
--- /dev/null
+++ b/node_modules/inflight/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inflight/README.md
----------------------------------------------------------------------
diff --git a/node_modules/inflight/README.md b/node_modules/inflight/README.md
new file mode 100644
index 0000000..6dc8929
--- /dev/null
+++ b/node_modules/inflight/README.md
@@ -0,0 +1,37 @@
+# inflight
+
+Add callbacks to requests in flight to avoid async duplication
+
+## USAGE
+
+```javascript
+var inflight = require('inflight')
+
+// some request that does some stuff
+function req(key, callback) {
+ // key is any random string. like a url or filename or whatever.
+ //
+ // will return either a falsey value, indicating that the
+ // request for this key is already in flight, or a new callback
+ // which when called will call all callbacks passed to inflightk
+ // with the same key
+ callback = inflight(key, callback)
+
+ // If we got a falsey value back, then there's already a req going
+ if (!callback) return
+
+ // this is where you'd fetch the url or whatever
+ // callback is also once()-ified, so it can safely be assigned
+ // to multiple events etc. First call wins.
+ setTimeout(function() {
+ callback(null, key)
+ }, 100)
+}
+
+// only assigns a single setTimeout
+// when it dings, all cbs get called
+req('foo', cb1)
+req('foo', cb2)
+req('foo', cb3)
+req('foo', cb4)
+```
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inflight/inflight.js
----------------------------------------------------------------------
diff --git a/node_modules/inflight/inflight.js b/node_modules/inflight/inflight.js
new file mode 100644
index 0000000..8bc96cb
--- /dev/null
+++ b/node_modules/inflight/inflight.js
@@ -0,0 +1,44 @@
+var wrappy = require('wrappy')
+var reqs = Object.create(null)
+var once = require('once')
+
+module.exports = wrappy(inflight)
+
+function inflight (key, cb) {
+ if (reqs[key]) {
+ reqs[key].push(cb)
+ return null
+ } else {
+ reqs[key] = [cb]
+ return makeres(key)
+ }
+}
+
+function makeres (key) {
+ return once(function RES () {
+ var cbs = reqs[key]
+ var len = cbs.length
+ var args = slice(arguments)
+ for (var i = 0; i < len; i++) {
+ cbs[i].apply(null, args)
+ }
+ if (cbs.length > len) {
+ // added more in the interim.
+ // de-zalgo, just in case, but don't call again.
+ cbs.splice(0, len)
+ process.nextTick(function () {
+ RES.apply(null, args)
+ })
+ } else {
+ delete reqs[key]
+ }
+ })
+}
+
+function slice (args) {
+ var length = args.length
+ var array = []
+
+ for (var i = 0; i < length; i++) array[i] = args[i]
+ return array
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inflight/package.json
----------------------------------------------------------------------
diff --git a/node_modules/inflight/package.json b/node_modules/inflight/package.json
new file mode 100644
index 0000000..05813cb
--- /dev/null
+++ b/node_modules/inflight/package.json
@@ -0,0 +1,86 @@
+{
+ "_args": [
+ [
+ "inflight@^1.0.4",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob"
+ ]
+ ],
+ "_from": "inflight@>=1.0.4 <2.0.0",
+ "_id": "inflight@1.0.4",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/inflight",
+ "_nodeVersion": "0.10.32",
+ "_npmUser": {
+ "email": "ogd@aoaioxxysz.net",
+ "name": "othiym23"
+ },
+ "_npmVersion": "2.1.3",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "inflight",
+ "raw": "inflight@^1.0.4",
+ "rawSpec": "^1.0.4",
+ "scope": null,
+ "spec": ">=1.0.4 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/glob"
+ ],
+ "_resolved": "http://registry.npmjs.org/inflight/-/inflight-1.0.4.tgz",
+ "_shasum": "6cbb4521ebd51ce0ec0a936bfd7657ef7e9b172a",
+ "_shrinkwrap": null,
+ "_spec": "inflight@^1.0.4",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me/"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/inflight/issues"
+ },
+ "dependencies": {
+ "once": "^1.3.0",
+ "wrappy": "1"
+ },
+ "description": "Add callbacks to requests in flight to avoid async duplication",
+ "devDependencies": {
+ "tap": "^0.4.10"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "6cbb4521ebd51ce0ec0a936bfd7657ef7e9b172a",
+ "tarball": "http://registry.npmjs.org/inflight/-/inflight-1.0.4.tgz"
+ },
+ "gitHead": "c7b5531d572a867064d4a1da9e013e8910b7d1ba",
+ "homepage": "https://github.com/isaacs/inflight",
+ "license": "ISC",
+ "main": "inflight.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ {
+ "name": "othiym23",
+ "email": "ogd@aoaioxxysz.net"
+ },
+ {
+ "name": "iarna",
+ "email": "me@re-becca.org"
+ }
+ ],
+ "name": "inflight",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/inflight.git"
+ },
+ "scripts": {
+ "test": "tap test.js"
+ },
+ "version": "1.0.4"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inflight/test.js
----------------------------------------------------------------------
diff --git a/node_modules/inflight/test.js b/node_modules/inflight/test.js
new file mode 100644
index 0000000..2bb75b3
--- /dev/null
+++ b/node_modules/inflight/test.js
@@ -0,0 +1,97 @@
+var test = require('tap').test
+var inf = require('./inflight.js')
+
+
+function req (key, cb) {
+ cb = inf(key, cb)
+ if (cb) setTimeout(function () {
+ cb(key)
+ cb(key)
+ })
+ return cb
+}
+
+test('basic', function (t) {
+ var calleda = false
+ var a = req('key', function (k) {
+ t.notOk(calleda)
+ calleda = true
+ t.equal(k, 'key')
+ if (calledb) t.end()
+ })
+ t.ok(a, 'first returned cb function')
+
+ var calledb = false
+ var b = req('key', function (k) {
+ t.notOk(calledb)
+ calledb = true
+ t.equal(k, 'key')
+ if (calleda) t.end()
+ })
+
+ t.notOk(b, 'second should get falsey inflight response')
+})
+
+test('timing', function (t) {
+ var expect = [
+ 'method one',
+ 'start one',
+ 'end one',
+ 'two',
+ 'tick',
+ 'three'
+ ]
+ var i = 0
+
+ function log (m) {
+ t.equal(m, expect[i], m + ' === ' + expect[i])
+ ++i
+ if (i === expect.length)
+ t.end()
+ }
+
+ function method (name, cb) {
+ log('method ' + name)
+ process.nextTick(cb)
+ }
+
+ var one = inf('foo', function () {
+ log('start one')
+ var three = inf('foo', function () {
+ log('three')
+ })
+ if (three) method('three', three)
+ log('end one')
+ })
+
+ method('one', one)
+
+ var two = inf('foo', function () {
+ log('two')
+ })
+ if (two) method('one', two)
+
+ process.nextTick(log.bind(null, 'tick'))
+})
+
+test('parameters', function (t) {
+ t.plan(8)
+
+ var a = inf('key', function (first, second, third) {
+ t.equal(first, 1)
+ t.equal(second, 2)
+ t.equal(third, 3)
+ })
+ t.ok(a, 'first returned cb function')
+
+ var b = inf('key', function (first, second, third) {
+ t.equal(first, 1)
+ t.equal(second, 2)
+ t.equal(third, 3)
+ })
+ t.notOk(b, 'second should get falsey inflight response')
+
+ setTimeout(function () {
+ a(1, 2, 3)
+ })
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inherits/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/inherits/LICENSE b/node_modules/inherits/LICENSE
new file mode 100644
index 0000000..dea3013
--- /dev/null
+++ b/node_modules/inherits/LICENSE
@@ -0,0 +1,16 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
+REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY AND
+FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
+INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
+LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
+OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
+PERFORMANCE OF THIS SOFTWARE.
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inherits/README.md
----------------------------------------------------------------------
diff --git a/node_modules/inherits/README.md b/node_modules/inherits/README.md
new file mode 100644
index 0000000..b1c5665
--- /dev/null
+++ b/node_modules/inherits/README.md
@@ -0,0 +1,42 @@
+Browser-friendly inheritance fully compatible with standard node.js
+[inherits](http://nodejs.org/api/util.html#util_util_inherits_constructor_superconstructor).
+
+This package exports standard `inherits` from node.js `util` module in
+node environment, but also provides alternative browser-friendly
+implementation through [browser
+field](https://gist.github.com/shtylman/4339901). Alternative
+implementation is a literal copy of standard one located in standalone
+module to avoid requiring of `util`. It also has a shim for old
+browsers with no `Object.create` support.
+
+While keeping you sure you are using standard `inherits`
+implementation in node.js environment, it allows bundlers such as
+[browserify](https://github.com/substack/node-browserify) to not
+include full `util` package to your client code if all you need is
+just `inherits` function. It worth, because browser shim for `util`
+package is large and `inherits` is often the single function you need
+from it.
+
+It's recommended to use this package instead of
+`require('util').inherits` for any code that has chances to be used
+not only in node.js but in browser too.
+
+## usage
+
+```js
+var inherits = require('inherits');
+// then use exactly as the standard one
+```
+
+## note on version ~1.0
+
+Version ~1.0 had completely different motivation and is not compatible
+neither with 2.0 nor with standard node.js `inherits`.
+
+If you are using version ~1.0 and planning to switch to ~2.0, be
+careful:
+
+* new version uses `super_` instead of `super` for referencing
+ superclass
+* new version overwrites current prototype while old one preserves any
+ existing fields on it
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inherits/inherits.js
----------------------------------------------------------------------
diff --git a/node_modules/inherits/inherits.js b/node_modules/inherits/inherits.js
new file mode 100644
index 0000000..29f5e24
--- /dev/null
+++ b/node_modules/inherits/inherits.js
@@ -0,0 +1 @@
+module.exports = require('util').inherits
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inherits/inherits_browser.js
----------------------------------------------------------------------
diff --git a/node_modules/inherits/inherits_browser.js b/node_modules/inherits/inherits_browser.js
new file mode 100644
index 0000000..c1e78a7
--- /dev/null
+++ b/node_modules/inherits/inherits_browser.js
@@ -0,0 +1,23 @@
+if (typeof Object.create === 'function') {
+ // implementation from standard node.js 'util' module
+ module.exports = function inherits(ctor, superCtor) {
+ ctor.super_ = superCtor
+ ctor.prototype = Object.create(superCtor.prototype, {
+ constructor: {
+ value: ctor,
+ enumerable: false,
+ writable: true,
+ configurable: true
+ }
+ });
+ };
+} else {
+ // old school shim for old browsers
+ module.exports = function inherits(ctor, superCtor) {
+ ctor.super_ = superCtor
+ var TempCtor = function () {}
+ TempCtor.prototype = superCtor.prototype
+ ctor.prototype = new TempCtor()
+ ctor.prototype.constructor = ctor
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inherits/package.json
----------------------------------------------------------------------
diff --git a/node_modules/inherits/package.json b/node_modules/inherits/package.json
new file mode 100644
index 0000000..245ab13
--- /dev/null
+++ b/node_modules/inherits/package.json
@@ -0,0 +1,77 @@
+{
+ "_args": [
+ [
+ "inherits@2",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob"
+ ]
+ ],
+ "_from": "inherits@>=2.0.0 <3.0.0",
+ "_id": "inherits@2.0.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/inherits",
+ "_npmUser": {
+ "email": "i@izs.me",
+ "name": "isaacs"
+ },
+ "_npmVersion": "1.3.8",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "inherits",
+ "raw": "inherits@2",
+ "rawSpec": "2",
+ "scope": null,
+ "spec": ">=2.0.0 <3.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/glob"
+ ],
+ "_resolved": "http://registry.npmjs.org/inherits/-/inherits-2.0.1.tgz",
+ "_shasum": "b17d08d326b4423e568eff719f91b0b1cbdf69f1",
+ "_shrinkwrap": null,
+ "_spec": "inherits@2",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob",
+ "browser": "./inherits_browser.js",
+ "bugs": {
+ "url": "https://github.com/isaacs/inherits/issues"
+ },
+ "dependencies": {},
+ "description": "Browser-friendly inheritance fully compatible with standard node.js inherits()",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "b17d08d326b4423e568eff719f91b0b1cbdf69f1",
+ "tarball": "http://registry.npmjs.org/inherits/-/inherits-2.0.1.tgz"
+ },
+ "homepage": "https://github.com/isaacs/inherits#readme",
+ "keywords": [
+ "browser",
+ "browserify",
+ "class",
+ "inheritance",
+ "inherits",
+ "klass",
+ "object-oriented",
+ "oop"
+ ],
+ "license": "ISC",
+ "main": "./inherits.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "name": "inherits",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/inherits.git"
+ },
+ "scripts": {
+ "test": "node test"
+ },
+ "version": "2.0.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/inherits/test.js
----------------------------------------------------------------------
diff --git a/node_modules/inherits/test.js b/node_modules/inherits/test.js
new file mode 100644
index 0000000..fc53012
--- /dev/null
+++ b/node_modules/inherits/test.js
@@ -0,0 +1,25 @@
+var inherits = require('./inherits.js')
+var assert = require('assert')
+
+function test(c) {
+ assert(c.constructor === Child)
+ assert(c.constructor.super_ === Parent)
+ assert(Object.getPrototypeOf(c) === Child.prototype)
+ assert(Object.getPrototypeOf(Object.getPrototypeOf(c)) === Parent.prototype)
+ assert(c instanceof Child)
+ assert(c instanceof Parent)
+}
+
+function Child() {
+ Parent.call(this)
+ test(this)
+}
+
+function Parent() {}
+
+inherits(Child, Parent)
+
+var c = new Child
+test(c)
+
+console.log('ok')
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/LICENSE.txt
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/LICENSE.txt b/node_modules/lodash-node/LICENSE.txt
new file mode 100644
index 0000000..49869bb
--- /dev/null
+++ b/node_modules/lodash-node/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+Based on Underscore.js 1.5.2, copyright 2009-2013 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors <http://underscorejs.org/>
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/README.md
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/README.md b/node_modules/lodash-node/README.md
new file mode 100644
index 0000000..3bc410e
--- /dev/null
+++ b/node_modules/lodash-node/README.md
@@ -0,0 +1,44 @@
+# lodash-node v2.4.1
+
+A collection of [Lo-Dash](http://lodash.com/) methods as [Node.js](http://nodejs.org/) modules generated by [lodash-cli](https://npmjs.org/package/lodash-cli).
+
+## Installation & usage
+
+Using [`npm`](http://npmjs.org/):
+
+```bash
+npm i --save lodash-node
+
+{sudo} npm i -g lodash-node
+npm ln lodash-node
+```
+
+In Node.js:
+
+```js
+var _ = require('lodash-node');
+
+// or as Underscore
+var _ = require('lodash-node/underscore');
+
+// or by method category
+var collections = require('lodash-node/modern/collections');
+
+// or individual methods
+var isEqual = require('lodash-node/modern/objects/isEqual');
+var findWhere = require('lodash-node/underscore/collections/findWhere');
+```
+
+## Author
+
+| [](https://twitter.com/jdalton "Follow @jdalton on Twitter") |
+|---|
+| [John-David Dalton](http://allyoucanleet.com/) |
+
+## Contributors
+
+| [](https://twitter.com/blainebublitz "Follow @BlaineBublitz on Twitter") | [](https://twitter.com/kitcambridge "Follow @kitcambridge on Twitter") | [](https://twitter.com/mathias "Follow @mathias on Twitter") |
+|---|---|---|
+| [Blaine Bublitz](http://www.iceddev.com/) | [Kit Cambridge](http://kitcambridge.be/) | [Mathias Bynens](http://mathiasbynens.be/) |
+
+[](https://bitdeli.com/free "Bitdeli Badge")
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays.js b/node_modules/lodash-node/compat/arrays.js
new file mode 100644
index 0000000..86f6dcf
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'compact': require('./arrays/compact'),
+ 'difference': require('./arrays/difference'),
+ 'drop': require('./arrays/rest'),
+ 'findIndex': require('./arrays/findIndex'),
+ 'findLastIndex': require('./arrays/findLastIndex'),
+ 'first': require('./arrays/first'),
+ 'flatten': require('./arrays/flatten'),
+ 'head': require('./arrays/first'),
+ 'indexOf': require('./arrays/indexOf'),
+ 'initial': require('./arrays/initial'),
+ 'intersection': require('./arrays/intersection'),
+ 'last': require('./arrays/last'),
+ 'lastIndexOf': require('./arrays/lastIndexOf'),
+ 'object': require('./arrays/zipObject'),
+ 'pull': require('./arrays/pull'),
+ 'range': require('./arrays/range'),
+ 'remove': require('./arrays/remove'),
+ 'rest': require('./arrays/rest'),
+ 'sortedIndex': require('./arrays/sortedIndex'),
+ 'tail': require('./arrays/rest'),
+ 'take': require('./arrays/first'),
+ 'union': require('./arrays/union'),
+ 'uniq': require('./arrays/uniq'),
+ 'unique': require('./arrays/uniq'),
+ 'unzip': require('./arrays/zip'),
+ 'without': require('./arrays/without'),
+ 'xor': require('./arrays/xor'),
+ 'zip': require('./arrays/zip'),
+ 'zipObject': require('./arrays/zipObject')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/compact.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/compact.js b/node_modules/lodash-node/compat/arrays/compact.js
new file mode 100644
index 0000000..f52dc22
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/compact.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are all falsey.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+function compact(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = compact;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/difference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/difference.js b/node_modules/lodash-node/compat/arrays/difference.js
new file mode 100644
index 0000000..f646752
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/difference.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseFlatten = require('../internals/baseFlatten');
+
+/**
+ * Creates an array excluding all values of the provided arrays using strict
+ * equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {...Array} [values] The arrays of values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.difference([1, 2, 3, 4, 5], [5, 2, 10]);
+ * // => [1, 3, 4]
+ */
+function difference(array) {
+ return baseDifference(array, baseFlatten(arguments, true, true, 1));
+}
+
+module.exports = difference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/findIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/findIndex.js b/node_modules/lodash-node/compat/arrays/findIndex.js
new file mode 100644
index 0000000..cabf7c0
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/findIndex.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback');
+
+/**
+ * This method is like `_.find` except that it returns the index of the first
+ * element that passes the callback check, instead of the element itself.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ * ];
+ *
+ * _.findIndex(characters, function(chr) {
+ * return chr.age < 20;
+ * });
+ * // => 2
+ *
+ * // using "_.where" callback shorthand
+ * _.findIndex(characters, { 'age': 36 });
+ * // => 0
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findIndex(characters, 'blocked');
+ * // => 1
+ */
+function findIndex(array, callback, thisArg) {
+ var index = -1,
+ length = array ? array.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length) {
+ if (callback(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = findIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/findLastIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/findLastIndex.js b/node_modules/lodash-node/compat/arrays/findLastIndex.js
new file mode 100644
index 0000000..2a74e31
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/findLastIndex.js
@@ -0,0 +1,63 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback');
+
+/**
+ * This method is like `_.findIndex` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': true },
+ * { 'name': 'fred', 'age': 40, 'blocked': false },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': true }
+ * ];
+ *
+ * _.findLastIndex(characters, function(chr) {
+ * return chr.age > 30;
+ * });
+ * // => 1
+ *
+ * // using "_.where" callback shorthand
+ * _.findLastIndex(characters, { 'age': 36 });
+ * // => 0
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findLastIndex(characters, 'blocked');
+ * // => 2
+ */
+function findLastIndex(array, callback, thisArg) {
+ var length = array ? array.length : 0;
+ callback = createCallback(callback, thisArg, 3);
+ while (length--) {
+ if (callback(array[length], length, array)) {
+ return length;
+ }
+ }
+ return -1;
+}
+
+module.exports = findLastIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/first.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/first.js b/node_modules/lodash-node/compat/arrays/first.js
new file mode 100644
index 0000000..c4b1c38
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/first.js
@@ -0,0 +1,86 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the first element or first `n` elements of an array. If a callback
+ * is provided elements at the beginning of the array are returned as long
+ * as the callback returns truey. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias head, take
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the first element(s) of `array`.
+ * @example
+ *
+ * _.first([1, 2, 3]);
+ * // => 1
+ *
+ * _.first([1, 2, 3], 2);
+ * // => [1, 2]
+ *
+ * _.first([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [1, 2]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.first(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'blocked': true, 'employer': 'slate' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.pluck(_.first(characters, { 'employer': 'slate' }), 'name');
+ * // => ['barney', 'fred']
+ */
+function first(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = -1;
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array ? array[0] : undefined;
+ }
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, n), length));
+}
+
+module.exports = first;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/flatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/flatten.js b/node_modules/lodash-node/compat/arrays/flatten.js
new file mode 100644
index 0000000..54dc494
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/flatten.js
@@ -0,0 +1,66 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ map = require('../collections/map');
+
+/**
+ * Flattens a nested array (the nesting can be to any depth). If `isShallow`
+ * is truey, the array will only be flattened a single level. If a callback
+ * is provided each element of the array is passed through the callback before
+ * flattening. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isShallow=false] A flag to restrict flattening to a single level.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2], [3, [[4]]]]);
+ * // => [1, 2, 3, 4];
+ *
+ * _.flatten([1, [2], [3, [[4]]]], true);
+ * // => [1, 2, 3, [[4]]];
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 30, 'pets': ['hoppy'] },
+ * { 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.flatten(characters, 'pets');
+ * // => ['hoppy', 'baby puss', 'dino']
+ */
+function flatten(array, isShallow, callback, thisArg) {
+ // juggle arguments
+ if (typeof isShallow != 'boolean' && isShallow != null) {
+ thisArg = callback;
+ callback = (typeof isShallow != 'function' && thisArg && thisArg[isShallow] === array) ? null : isShallow;
+ isShallow = false;
+ }
+ if (callback != null) {
+ array = map(array, callback, thisArg);
+ }
+ return baseFlatten(array, isShallow);
+}
+
+module.exports = flatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/indexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/indexOf.js b/node_modules/lodash-node/compat/arrays/indexOf.js
new file mode 100644
index 0000000..19238b5
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/indexOf.js
@@ -0,0 +1,50 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ sortedIndex = require('./sortedIndex');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Gets the index at which the first occurrence of `value` is found using
+ * strict equality for comparisons, i.e. `===`. If the array is already sorted
+ * providing `true` for `fromIndex` will run a faster binary search.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {boolean|number} [fromIndex=0] The index to search from or `true`
+ * to perform a binary search on a sorted array.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 1
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 4
+ *
+ * _.indexOf([1, 1, 2, 2, 3, 3], 2, true);
+ * // => 2
+ */
+function indexOf(array, value, fromIndex) {
+ if (typeof fromIndex == 'number') {
+ var length = array ? array.length : 0;
+ fromIndex = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex || 0);
+ } else if (fromIndex) {
+ var index = sortedIndex(array, value);
+ return array[index] === value ? index : -1;
+ }
+ return baseIndexOf(array, value, fromIndex);
+}
+
+module.exports = indexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/initial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/initial.js b/node_modules/lodash-node/compat/arrays/initial.js
new file mode 100644
index 0000000..77ea68b
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/initial.js
@@ -0,0 +1,82 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets all but the last element or last `n` elements of an array. If a
+ * callback is provided elements at the end of the array are excluded from
+ * the result as long as the callback returns truey. The callback is bound
+ * to `thisArg` and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.initial([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.initial([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [1]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.initial(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'blocked': false, 'employer': 'slate' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.pluck(_.initial(characters, { 'employer': 'na' }), 'name');
+ * // => ['barney', 'fred']
+ */
+function initial(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg, 3);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : callback || n;
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, length - n), length));
+}
+
+module.exports = initial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/intersection.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/intersection.js b/node_modules/lodash-node/compat/arrays/intersection.js
new file mode 100644
index 0000000..fd7bf65
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/intersection.js
@@ -0,0 +1,83 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ cacheIndexOf = require('../internals/cacheIndexOf'),
+ createCache = require('../internals/createCache'),
+ getArray = require('../internals/getArray'),
+ isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray'),
+ largeArraySize = require('../internals/largeArraySize'),
+ releaseArray = require('../internals/releaseArray'),
+ releaseObject = require('../internals/releaseObject');
+
+/**
+ * Creates an array of unique values present in all provided arrays using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of shared values.
+ * @example
+ *
+ * _.intersection([1, 2, 3], [5, 2, 1, 4], [2, 1]);
+ * // => [1, 2]
+ */
+function intersection() {
+ var args = [],
+ argsIndex = -1,
+ argsLength = arguments.length,
+ caches = getArray(),
+ indexOf = baseIndexOf,
+ trustIndexOf = indexOf === baseIndexOf,
+ seen = getArray();
+
+ while (++argsIndex < argsLength) {
+ var value = arguments[argsIndex];
+ if (isArray(value) || isArguments(value)) {
+ args.push(value);
+ caches.push(trustIndexOf && value.length >= largeArraySize &&
+ createCache(argsIndex ? args[argsIndex] : seen));
+ }
+ }
+ var array = args[0],
+ index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ outer:
+ while (++index < length) {
+ var cache = caches[0];
+ value = array[index];
+
+ if ((cache ? cacheIndexOf(cache, value) : indexOf(seen, value)) < 0) {
+ argsIndex = argsLength;
+ (cache || seen).push(value);
+ while (--argsIndex) {
+ cache = caches[argsIndex];
+ if ((cache ? cacheIndexOf(cache, value) : indexOf(args[argsIndex], value)) < 0) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ }
+ while (argsLength--) {
+ cache = caches[argsLength];
+ if (cache) {
+ releaseObject(cache);
+ }
+ }
+ releaseArray(caches);
+ releaseArray(seen);
+ return result;
+}
+
+module.exports = intersection;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/last.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/last.js b/node_modules/lodash-node/compat/arrays/last.js
new file mode 100644
index 0000000..b54f370
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/last.js
@@ -0,0 +1,84 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Gets the last element or last `n` elements of an array. If a callback is
+ * provided elements at the end of the array are returned as long as the
+ * callback returns truey. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the last element(s) of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ *
+ * _.last([1, 2, 3], 2);
+ * // => [2, 3]
+ *
+ * _.last([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [2, 3]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.pluck(_.last(characters, 'blocked'), 'name');
+ * // => ['fred', 'pebbles']
+ *
+ * // using "_.where" callback shorthand
+ * _.last(characters, { 'employer': 'na' });
+ * // => [{ 'name': 'pebbles', 'blocked': true, 'employer': 'na' }]
+ */
+function last(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg, 3);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array ? array[length - 1] : undefined;
+ }
+ }
+ return slice(array, nativeMax(0, length - n));
+}
+
+module.exports = last;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/lastIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/lastIndexOf.js b/node_modules/lodash-node/compat/arrays/lastIndexOf.js
new file mode 100644
index 0000000..c7b8817
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/lastIndexOf.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the index at which the last occurrence of `value` is found using strict
+ * equality for comparisons, i.e. `===`. If `fromIndex` is negative, it is used
+ * as the offset from the end of the collection.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 4
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 1
+ */
+function lastIndexOf(array, value, fromIndex) {
+ var index = array ? array.length : 0;
+ if (typeof fromIndex == 'number') {
+ index = (fromIndex < 0 ? nativeMax(0, index + fromIndex) : nativeMin(fromIndex, index - 1)) + 1;
+ }
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = lastIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/pull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/pull.js b/node_modules/lodash-node/compat/arrays/pull.js
new file mode 100644
index 0000000..d7f60d0
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/pull.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var splice = arrayRef.splice;
+
+/**
+ * Removes all provided values from the given array using strict equality for
+ * comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to modify.
+ * @param {...*} [value] The values to remove.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3, 1, 2, 3];
+ * _.pull(array, 2, 3);
+ * console.log(array);
+ * // => [1, 1]
+ */
+function pull(array) {
+ var args = arguments,
+ argsIndex = 0,
+ argsLength = args.length,
+ length = array ? array.length : 0;
+
+ while (++argsIndex < argsLength) {
+ var index = -1,
+ value = args[argsIndex];
+ while (++index < length) {
+ if (array[index] === value) {
+ splice.call(array, index--, 1);
+ length--;
+ }
+ }
+ }
+ return array;
+}
+
+module.exports = pull;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[18/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/examples/json.pegjs
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/examples/json.pegjs b/node_modules/pegjs/examples/json.pegjs
new file mode 100644
index 0000000..f2a34b1
--- /dev/null
+++ b/node_modules/pegjs/examples/json.pegjs
@@ -0,0 +1,120 @@
+/* JSON parser based on the grammar described at http://json.org/. */
+
+/* ===== Syntactical Elements ===== */
+
+start
+ = _ object:object { return object; }
+
+object
+ = "{" _ "}" _ { return {}; }
+ / "{" _ members:members "}" _ { return members; }
+
+members
+ = head:pair tail:("," _ pair)* {
+ var result = {};
+ result[head[0]] = head[1];
+ for (var i = 0; i < tail.length; i++) {
+ result[tail[i][2][0]] = tail[i][2][1];
+ }
+ return result;
+ }
+
+pair
+ = name:string ":" _ value:value { return [name, value]; }
+
+array
+ = "[" _ "]" _ { return []; }
+ / "[" _ elements:elements "]" _ { return elements; }
+
+elements
+ = head:value tail:("," _ value)* {
+ var result = [head];
+ for (var i = 0; i < tail.length; i++) {
+ result.push(tail[i][2]);
+ }
+ return result;
+ }
+
+value
+ = string
+ / number
+ / object
+ / array
+ / "true" _ { return true; }
+ / "false" _ { return false; }
+ // FIXME: We can't return null here because that would mean parse failure.
+ / "null" _ { return "null"; }
+
+/* ===== Lexical Elements ===== */
+
+string "string"
+ = '"' '"' _ { return ""; }
+ / '"' chars:chars '"' _ { return chars; }
+
+chars
+ = chars:char+ { return chars.join(""); }
+
+char
+ // In the original JSON grammar: "any-Unicode-character-except-"-or-\-or-control-character"
+ = [^"\\\0-\x1F\x7f]
+ / '\\"' { return '"'; }
+ / "\\\\" { return "\\"; }
+ / "\\/" { return "/"; }
+ / "\\b" { return "\b"; }
+ / "\\f" { return "\f"; }
+ / "\\n" { return "\n"; }
+ / "\\r" { return "\r"; }
+ / "\\t" { return "\t"; }
+ / "\\u" h1:hexDigit h2:hexDigit h3:hexDigit h4:hexDigit {
+ return String.fromCharCode(parseInt("0x" + h1 + h2 + h3 + h4));
+ }
+
+number "number"
+ = int_:int frac:frac exp:exp _ { return parseFloat(int_ + frac + exp); }
+ / int_:int frac:frac _ { return parseFloat(int_ + frac); }
+ / int_:int exp:exp _ { return parseFloat(int_ + exp); }
+ / int_:int _ { return parseFloat(int_); }
+
+int
+ = digit19:digit19 digits:digits { return digit19 + digits; }
+ / digit:digit
+ / "-" digit19:digit19 digits:digits { return "-" + digit19 + digits; }
+ / "-" digit:digit { return "-" + digit; }
+
+frac
+ = "." digits:digits { return "." + digits; }
+
+exp
+ = e:e digits:digits { return e + digits; }
+
+digits
+ = digits:digit+ { return digits.join(""); }
+
+e
+ = e:[eE] sign:[+-]? { return e + sign; }
+
+/*
+ * The following rules are not present in the original JSON gramar, but they are
+ * assumed to exist implicitly.
+ *
+ * FIXME: Define them according to ECMA-262, 5th ed.
+ */
+
+digit
+ = [0-9]
+
+digit19
+ = [1-9]
+
+hexDigit
+ = [0-9a-fA-F]
+
+/* ===== Whitespace ===== */
+
+_ "whitespace"
+ = whitespace*
+
+// Whitespace is undefined in the original JSON grammar, so I assume a simple
+// conventional definition consistent with ECMA-262, 5th ed.
+whitespace
+ = [ \t\n\r]
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[33/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/intersection.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/intersection.js b/node_modules/lodash-node/underscore/arrays/intersection.js
new file mode 100644
index 0000000..71a01d1
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/intersection.js
@@ -0,0 +1,60 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates an array of unique values present in all provided arrays using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of shared values.
+ * @example
+ *
+ * _.intersection([1, 2, 3], [5, 2, 1, 4], [2, 1]);
+ * // => [1, 2]
+ */
+function intersection() {
+ var args = [],
+ argsIndex = -1,
+ argsLength = arguments.length;
+
+ while (++argsIndex < argsLength) {
+ var value = arguments[argsIndex];
+ if (isArray(value) || isArguments(value)) {
+ args.push(value);
+ }
+ }
+ var array = args[0],
+ index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ result = [];
+
+ outer:
+ while (++index < length) {
+ value = array[index];
+ if (indexOf(result, value) < 0) {
+ var argsIndex = argsLength;
+ while (--argsIndex) {
+ if (indexOf(args[argsIndex], value) < 0) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = intersection;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/last.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/last.js b/node_modules/lodash-node/underscore/arrays/last.js
new file mode 100644
index 0000000..ba418b7
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/last.js
@@ -0,0 +1,84 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Gets the last element or last `n` elements of an array. If a callback is
+ * provided elements at the end of the array are returned as long as the
+ * callback returns truey. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the last element(s) of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ *
+ * _.last([1, 2, 3], 2);
+ * // => [2, 3]
+ *
+ * _.last([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [2, 3]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.pluck(_.last(characters, 'blocked'), 'name');
+ * // => ['fred', 'pebbles']
+ *
+ * // using "_.where" callback shorthand
+ * _.last(characters, { 'employer': 'na' });
+ * // => [{ 'name': 'pebbles', 'blocked': true, 'employer': 'na' }]
+ */
+function last(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg, 3);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array ? array[length - 1] : undefined;
+ }
+ }
+ return slice(array, nativeMax(0, length - n));
+}
+
+module.exports = last;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/lastIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/lastIndexOf.js b/node_modules/lodash-node/underscore/arrays/lastIndexOf.js
new file mode 100644
index 0000000..e791147
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/lastIndexOf.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the index at which the last occurrence of `value` is found using strict
+ * equality for comparisons, i.e. `===`. If `fromIndex` is negative, it is used
+ * as the offset from the end of the collection.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 4
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 1
+ */
+function lastIndexOf(array, value, fromIndex) {
+ var index = array ? array.length : 0;
+ if (typeof fromIndex == 'number') {
+ index = (fromIndex < 0 ? nativeMax(0, index + fromIndex) : nativeMin(fromIndex, index - 1)) + 1;
+ }
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = lastIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/range.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/range.js b/node_modules/lodash-node/underscore/arrays/range.js
new file mode 100644
index 0000000..0075159
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/range.js
@@ -0,0 +1,69 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Native method shortcuts */
+var ceil = Math.ceil;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Creates an array of numbers (positive and/or negative) progressing from
+ * `start` up to but not including `end`. If `start` is less than `stop` a
+ * zero-length range is created unless a negative `step` is specified.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @param {number} [step=1] The value to increment or decrement by.
+ * @returns {Array} Returns a new range array.
+ * @example
+ *
+ * _.range(4);
+ * // => [0, 1, 2, 3]
+ *
+ * _.range(1, 5);
+ * // => [1, 2, 3, 4]
+ *
+ * _.range(0, 20, 5);
+ * // => [0, 5, 10, 15]
+ *
+ * _.range(0, -4, -1);
+ * // => [0, -1, -2, -3]
+ *
+ * _.range(1, 4, 0);
+ * // => [1, 1, 1]
+ *
+ * _.range(0);
+ * // => []
+ */
+function range(start, end, step) {
+ start = +start || 0;
+ step = (+step || 1);
+
+ if (end == null) {
+ end = start;
+ start = 0;
+ }
+ // use `Array(length)` so engines like Chakra and V8 avoid slower modes
+ // http://youtu.be/XAqIpGU8ZZk#t=17m25s
+ var index = -1,
+ length = nativeMax(0, ceil((end - start) / step)),
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = start;
+ start += step;
+ }
+ return result;
+}
+
+module.exports = range;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/rest.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/rest.js b/node_modules/lodash-node/underscore/arrays/rest.js
new file mode 100644
index 0000000..284608c
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/rest.js
@@ -0,0 +1,83 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * The opposite of `_.initial` this method gets all but the first element or
+ * first `n` elements of an array. If a callback function is provided elements
+ * at the beginning of the array are excluded from the result as long as the
+ * callback returns truey. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias drop, tail
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.rest([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.rest([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.rest([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [3]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.pluck(_.rest(characters, 'blocked'), 'name');
+ * // => ['fred', 'pebbles']
+ *
+ * // using "_.where" callback shorthand
+ * _.rest(characters, { 'employer': 'slate' });
+ * // => [{ 'name': 'pebbles', 'blocked': true, 'employer': 'na' }]
+ */
+function rest(array, callback, thisArg) {
+ if (typeof callback != 'number' && callback != null) {
+ var n = 0,
+ index = -1,
+ length = array ? array.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : nativeMax(0, callback);
+ }
+ return slice(array, n);
+}
+
+module.exports = rest;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/sortedIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/sortedIndex.js b/node_modules/lodash-node/underscore/arrays/sortedIndex.js
new file mode 100644
index 0000000..2358d08
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/sortedIndex.js
@@ -0,0 +1,77 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ identity = require('../utilities/identity');
+
+/**
+ * Uses a binary search to determine the smallest index at which a value
+ * should be inserted into a given sorted array in order to maintain the sort
+ * order of the array. If a callback is provided it will be executed for
+ * `value` and each element of `array` to compute their sort ranking. The
+ * callback is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedIndex([20, 30, 50], 40);
+ * // => 2
+ *
+ * // using "_.pluck" callback shorthand
+ * _.sortedIndex([{ 'x': 20 }, { 'x': 30 }, { 'x': 50 }], { 'x': 40 }, 'x');
+ * // => 2
+ *
+ * var dict = {
+ * 'wordToNumber': { 'twenty': 20, 'thirty': 30, 'fourty': 40, 'fifty': 50 }
+ * };
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return dict.wordToNumber[word];
+ * });
+ * // => 2
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return this.wordToNumber[word];
+ * }, dict);
+ * // => 2
+ */
+function sortedIndex(array, value, callback, thisArg) {
+ var low = 0,
+ high = array ? array.length : low;
+
+ // explicitly reference `identity` for better inlining in Firefox
+ callback = callback ? createCallback(callback, thisArg, 1) : identity;
+ value = callback(value);
+
+ while (low < high) {
+ var mid = (low + high) >>> 1;
+ (callback(array[mid]) < value)
+ ? low = mid + 1
+ : high = mid;
+ }
+ return low;
+}
+
+module.exports = sortedIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/union.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/union.js b/node_modules/lodash-node/underscore/arrays/union.js
new file mode 100644
index 0000000..5fe15c6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/union.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ baseUniq = require('../internals/baseUniq');
+
+/**
+ * Creates an array of unique values, in order, of the provided arrays using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of combined values.
+ * @example
+ *
+ * _.union([1, 2, 3], [5, 2, 1, 4], [2, 1]);
+ * // => [1, 2, 3, 5, 4]
+ */
+function union() {
+ return baseUniq(baseFlatten(arguments, true, true));
+}
+
+module.exports = union;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/uniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/uniq.js b/node_modules/lodash-node/underscore/arrays/uniq.js
new file mode 100644
index 0000000..e403ea1
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/uniq.js
@@ -0,0 +1,69 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseUniq = require('../internals/baseUniq'),
+ createCallback = require('../functions/createCallback');
+
+/**
+ * Creates a duplicate-value-free version of an array using strict equality
+ * for comparisons, i.e. `===`. If the array is sorted, providing
+ * `true` for `isSorted` will use a faster algorithm. If a callback is provided
+ * each element of `array` is passed through the callback before uniqueness
+ * is computed. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias unique
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {boolean} [isSorted=false] A flag to indicate that `array` is sorted.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a duplicate-value-free array.
+ * @example
+ *
+ * _.uniq([1, 2, 1, 3, 1]);
+ * // => [1, 2, 3]
+ *
+ * _.uniq([1, 1, 2, 2, 3], true);
+ * // => [1, 2, 3]
+ *
+ * _.uniq(['A', 'b', 'C', 'a', 'B', 'c'], function(letter) { return letter.toLowerCase(); });
+ * // => ['A', 'b', 'C']
+ *
+ * _.uniq([1, 2.5, 3, 1.5, 2, 3.5], function(num) { return this.floor(num); }, Math);
+ * // => [1, 2.5, 3]
+ *
+ * // using "_.pluck" callback shorthand
+ * _.uniq([{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+function uniq(array, isSorted, callback, thisArg) {
+ // juggle arguments
+ if (typeof isSorted != 'boolean' && isSorted != null) {
+ thisArg = callback;
+ callback = (typeof isSorted != 'function' && thisArg && thisArg[isSorted] === array) ? null : isSorted;
+ isSorted = false;
+ }
+ if (callback != null) {
+ callback = createCallback(callback, thisArg, 3);
+ }
+ return baseUniq(array, isSorted, callback);
+}
+
+module.exports = uniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/without.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/without.js b/node_modules/lodash-node/underscore/arrays/without.js
new file mode 100644
index 0000000..b42b19d
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/without.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates an array excluding all provided values using strict equality for
+ * comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to filter.
+ * @param {...*} [value] The values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.without([1, 2, 1, 0, 3, 1, 4], 0, 1);
+ * // => [2, 3, 4]
+ */
+function without(array) {
+ return baseDifference(array, slice(arguments, 1));
+}
+
+module.exports = without;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/zip.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/zip.js b/node_modules/lodash-node/underscore/arrays/zip.js
new file mode 100644
index 0000000..080e7b6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/zip.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var max = require('../collections/max'),
+ pluck = require('../collections/pluck');
+
+/**
+ * Creates an array of grouped elements, the first of which contains the first
+ * elements of the given arrays, the second of which contains the second
+ * elements of the given arrays, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @alias unzip
+ * @category Arrays
+ * @param {...Array} [array] Arrays to process.
+ * @returns {Array} Returns a new array of grouped elements.
+ * @example
+ *
+ * _.zip(['fred', 'barney'], [30, 40], [true, false]);
+ * // => [['fred', 30, true], ['barney', 40, false]]
+ */
+function zip() {
+ var index = -1,
+ length = max(pluck(arguments, 'length')),
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = pluck(arguments, index);
+ }
+ return result;
+}
+
+module.exports = zip;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/zipObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/zipObject.js b/node_modules/lodash-node/underscore/arrays/zipObject.js
new file mode 100644
index 0000000..6fbcdef
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/zipObject.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArray = require('../objects/isArray');
+
+/**
+ * Creates an object composed from arrays of `keys` and `values`. Provide
+ * either a single two dimensional array, i.e. `[[key1, value1], [key2, value2]]`
+ * or two arrays, one of `keys` and one of corresponding `values`.
+ *
+ * @static
+ * @memberOf _
+ * @alias object
+ * @category Arrays
+ * @param {Array} keys The array of keys.
+ * @param {Array} [values=[]] The array of values.
+ * @returns {Object} Returns an object composed of the given keys and
+ * corresponding values.
+ * @example
+ *
+ * _.zipObject(['fred', 'barney'], [30, 40]);
+ * // => { 'fred': 30, 'barney': 40 }
+ */
+function zipObject(keys, values) {
+ var index = -1,
+ length = keys ? keys.length : 0,
+ result = {};
+
+ if (!values && length && !isArray(keys[0])) {
+ values = [];
+ }
+ while (++index < length) {
+ var key = keys[index];
+ if (values) {
+ result[key] = values[index];
+ } else if (key) {
+ result[key[0]] = key[1];
+ }
+ }
+ return result;
+}
+
+module.exports = zipObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/chaining.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/chaining.js b/node_modules/lodash-node/underscore/chaining.js
new file mode 100644
index 0000000..00bb0d7
--- /dev/null
+++ b/node_modules/lodash-node/underscore/chaining.js
@@ -0,0 +1,16 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'chain': require('./chaining/chain'),
+ 'tap': require('./chaining/tap'),
+ 'value': require('./chaining/wrapperValueOf'),
+ 'wrapperChain': require('./chaining/wrapperChain'),
+ 'wrapperValueOf': require('./chaining/wrapperValueOf')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/chaining/chain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/chaining/chain.js b/node_modules/lodash-node/underscore/chaining/chain.js
new file mode 100644
index 0000000..bce59d9
--- /dev/null
+++ b/node_modules/lodash-node/underscore/chaining/chain.js
@@ -0,0 +1,41 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var lodashWrapper = require('../internals/lodashWrapper');
+
+/**
+ * Creates a `lodash` object that wraps the given value with explicit
+ * method chaining enabled.
+ *
+ * @static
+ * @memberOf _
+ * @category Chaining
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the wrapper object.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _.chain(characters)
+ * .sortBy('age')
+ * .map(function(chr) { return chr.name + ' is ' + chr.age; })
+ * .first()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+function chain(value) {
+ value = new lodashWrapper(value);
+ value.__chain__ = true;
+ return value;
+}
+
+module.exports = chain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/chaining/tap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/chaining/tap.js b/node_modules/lodash-node/underscore/chaining/tap.js
new file mode 100644
index 0000000..4f46c86
--- /dev/null
+++ b/node_modules/lodash-node/underscore/chaining/tap.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Invokes `interceptor` with the `value` as the first argument and then
+ * returns `value`. The purpose of this method is to "tap into" a method
+ * chain in order to perform operations on intermediate results within
+ * the chain.
+ *
+ * @static
+ * @memberOf _
+ * @category Chaining
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * _([1, 2, 3, 4])
+ * .tap(function(array) { array.pop(); })
+ * .reverse()
+ * .value();
+ * // => [3, 2, 1]
+ */
+function tap(value, interceptor) {
+ interceptor(value);
+ return value;
+}
+
+module.exports = tap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/chaining/wrapperChain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/chaining/wrapperChain.js b/node_modules/lodash-node/underscore/chaining/wrapperChain.js
new file mode 100644
index 0000000..7a88080
--- /dev/null
+++ b/node_modules/lodash-node/underscore/chaining/wrapperChain.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Enables explicit method chaining on the wrapper object.
+ *
+ * @name chain
+ * @memberOf _
+ * @category Chaining
+ * @returns {*} Returns the wrapper object.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // without explicit chaining
+ * _(characters).first();
+ * // => { 'name': 'barney', 'age': 36 }
+ *
+ * // with explicit chaining
+ * _(characters).chain()
+ * .first()
+ * .pick('age')
+ * .value();
+ * // => { 'age': 36 }
+ */
+function wrapperChain() {
+ this.__chain__ = true;
+ return this;
+}
+
+module.exports = wrapperChain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/chaining/wrapperValueOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/chaining/wrapperValueOf.js b/node_modules/lodash-node/underscore/chaining/wrapperValueOf.js
new file mode 100644
index 0000000..541a489
--- /dev/null
+++ b/node_modules/lodash-node/underscore/chaining/wrapperValueOf.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ support = require('../support');
+
+/**
+ * Extracts the wrapped value.
+ *
+ * @name valueOf
+ * @memberOf _
+ * @alias value
+ * @category Chaining
+ * @returns {*} Returns the wrapped value.
+ * @example
+ *
+ * _([1, 2, 3]).valueOf();
+ * // => [1, 2, 3]
+ */
+function wrapperValueOf() {
+ return this.__wrapped__;
+}
+
+module.exports = wrapperValueOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections.js b/node_modules/lodash-node/underscore/collections.js
new file mode 100644
index 0000000..83220bc
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'all': require('./collections/every'),
+ 'any': require('./collections/some'),
+ 'collect': require('./collections/map'),
+ 'contains': require('./collections/contains'),
+ 'countBy': require('./collections/countBy'),
+ 'detect': require('./collections/find'),
+ 'each': require('./collections/forEach'),
+ 'eachRight': require('./collections/forEachRight'),
+ 'every': require('./collections/every'),
+ 'filter': require('./collections/filter'),
+ 'find': require('./collections/find'),
+ 'findWhere': require('./collections/findWhere'),
+ 'foldl': require('./collections/reduce'),
+ 'foldr': require('./collections/reduceRight'),
+ 'forEach': require('./collections/forEach'),
+ 'forEachRight': require('./collections/forEachRight'),
+ 'groupBy': require('./collections/groupBy'),
+ 'include': require('./collections/contains'),
+ 'indexBy': require('./collections/indexBy'),
+ 'inject': require('./collections/reduce'),
+ 'invoke': require('./collections/invoke'),
+ 'map': require('./collections/map'),
+ 'max': require('./collections/max'),
+ 'min': require('./collections/min'),
+ 'pluck': require('./collections/pluck'),
+ 'reduce': require('./collections/reduce'),
+ 'reduceRight': require('./collections/reduceRight'),
+ 'reject': require('./collections/reject'),
+ 'sample': require('./collections/sample'),
+ 'select': require('./collections/filter'),
+ 'shuffle': require('./collections/shuffle'),
+ 'size': require('./collections/size'),
+ 'some': require('./collections/some'),
+ 'sortBy': require('./collections/sortBy'),
+ 'toArray': require('./collections/toArray'),
+ 'where': require('./collections/where')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/contains.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/contains.js b/node_modules/lodash-node/underscore/collections/contains.js
new file mode 100644
index 0000000..72a78b6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/contains.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ forOwn = require('../objects/forOwn'),
+ indicatorObject = require('../internals/indicatorObject');
+
+/**
+ * Checks if a given value is present in a collection using strict equality
+ * for comparisons, i.e. `===`. If `fromIndex` is negative, it is used as the
+ * offset from the end of the collection.
+ *
+ * @static
+ * @memberOf _
+ * @alias include
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {*} target The value to check for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {boolean} Returns `true` if the `target` element is found, else `false`.
+ * @example
+ *
+ * _.contains([1, 2, 3], 1);
+ * // => true
+ *
+ * _.contains([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.contains({ 'name': 'fred', 'age': 40 }, 'fred');
+ * // => true
+ *
+ * _.contains('pebbles', 'eb');
+ * // => true
+ */
+function contains(collection, target) {
+ var indexOf = baseIndexOf,
+ length = collection ? collection.length : 0,
+ result = false;
+ if (length && typeof length == 'number') {
+ result = indexOf(collection, target) > -1;
+ } else {
+ forOwn(collection, function(value) {
+ return (result = value === target) && indicatorObject;
+ });
+ }
+ return result;
+}
+
+module.exports = contains;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/countBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/countBy.js b/node_modules/lodash-node/underscore/collections/countBy.js
new file mode 100644
index 0000000..6791bd2
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/countBy.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` through the callback. The corresponding value
+ * of each key is the number of times the key was returned by the callback.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+var countBy = createAggregator(function(result, value, key) {
+ (hasOwnProperty.call(result, key) ? result[key]++ : result[key] = 1);
+});
+
+module.exports = countBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/every.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/every.js b/node_modules/lodash-node/underscore/collections/every.js
new file mode 100644
index 0000000..aa09512
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/every.js
@@ -0,0 +1,75 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn'),
+ indicatorObject = require('../internals/indicatorObject');
+
+/**
+ * Checks if the given callback returns truey value for **all** elements of
+ * a collection. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if all elements passed the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes']);
+ * // => false
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.every(characters, 'age');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.every(characters, { 'age': 36 });
+ * // => false
+ */
+function every(collection, callback, thisArg) {
+ var result = true;
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ if (!(result = !!callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ return !(result = !!callback(value, index, collection)) && indicatorObject;
+ });
+ }
+ return result;
+}
+
+module.exports = every;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/filter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/filter.js b/node_modules/lodash-node/underscore/collections/filter.js
new file mode 100644
index 0000000..5b96f19
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/filter.js
@@ -0,0 +1,76 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Iterates over elements of a collection, returning an array of all elements
+ * the callback returns truey for. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias select
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that passed the callback check.
+ * @example
+ *
+ * var evens = _.filter([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [2, 4, 6]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.filter(characters, 'blocked');
+ * // => [{ 'name': 'fred', 'age': 40, 'blocked': true }]
+ *
+ * // using "_.where" callback shorthand
+ * _.filter(characters, { 'age': 36 });
+ * // => [{ 'name': 'barney', 'age': 36, 'blocked': false }]
+ */
+function filter(collection, callback, thisArg) {
+ var result = [];
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = filter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/find.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/find.js b/node_modules/lodash-node/underscore/collections/find.js
new file mode 100644
index 0000000..beae5be
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/find.js
@@ -0,0 +1,81 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn'),
+ indicatorObject = require('../internals/indicatorObject');
+
+/**
+ * Iterates over elements of a collection, returning the first element that
+ * the callback returns truey for. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias detect, findWhere
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the found element, else `undefined`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ * ];
+ *
+ * _.find(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => { 'name': 'barney', 'age': 36, 'blocked': false }
+ *
+ * // using "_.where" callback shorthand
+ * _.find(characters, { 'age': 1 });
+ * // => { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.find(characters, 'blocked');
+ * // => { 'name': 'fred', 'age': 40, 'blocked': true }
+ */
+function find(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ return value;
+ }
+ }
+ } else {
+ var result;
+ forOwn(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result = value;
+ return indicatorObject;
+ }
+ });
+ return result;
+ }
+}
+
+module.exports = find;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/findWhere.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/findWhere.js b/node_modules/lodash-node/underscore/collections/findWhere.js
new file mode 100644
index 0000000..5ef75f6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/findWhere.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var where = require('./where');
+
+/**
+ * Examines each element in a `collection`, returning the first that
+ * has the given properties. When checking `properties`, this method
+ * performs a deep comparison between values to determine if they are
+ * equivalent to each other.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Object} properties The object of property values to filter by.
+ * @returns {*} Returns the found element, else `undefined`.
+ * @example
+ *
+ * var food = [
+ * { 'name': 'apple', 'organic': false, 'type': 'fruit' },
+ * { 'name': 'banana', 'organic': true, 'type': 'fruit' },
+ * { 'name': 'beet', 'organic': false, 'type': 'vegetable' }
+ * ];
+ *
+ * _.findWhere(food, { 'type': 'vegetable' });
+ * // => { 'name': 'beet', 'organic': false, 'type': 'vegetable' }
+ */
+function findWhere(object, properties) {
+ return where(object, properties, true);
+}
+
+module.exports = findWhere;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/forEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/forEach.js b/node_modules/lodash-node/underscore/collections/forEach.js
new file mode 100644
index 0000000..8e1e2be
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/forEach.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ forOwn = require('../objects/forOwn'),
+ indicatorObject = require('../internals/indicatorObject');
+
+/**
+ * Iterates over elements of a collection, executing the callback for each
+ * element. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection). Callbacks may exit iteration early by
+ * explicitly returning `false`.
+ *
+ * Note: As with other "Collections" methods, objects with a `length` property
+ * are iterated like arrays. To avoid this behavior `_.forIn` or `_.forOwn`
+ * may be used for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @alias each
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEach(function(num) { console.log(num); }).join(',');
+ * // => logs each number and returns '1,2,3'
+ *
+ * _.forEach({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { console.log(num); });
+ * // => logs each number and returns the object (property order is not guaranteed across environments)
+ */
+function forEach(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3);
+ if (typeof length == 'number') {
+ while (++index < length) {
+ if (callback(collection[index], index, collection) === indicatorObject) {
+ break;
+ }
+ }
+ } else {
+ forOwn(collection, callback);
+ }
+}
+
+module.exports = forEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/forEachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/forEachRight.js b/node_modules/lodash-node/underscore/collections/forEachRight.js
new file mode 100644
index 0000000..1cf6173
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/forEachRight.js
@@ -0,0 +1,51 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ forOwn = require('../objects/forOwn'),
+ indicatorObject = require('../internals/indicatorObject'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString'),
+ keys = require('../objects/keys');
+
+/**
+ * This method is like `_.forEach` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias eachRight
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEachRight(function(num) { console.log(num); }).join(',');
+ * // => logs each number from right to left and returns '3,2,1'
+ */
+function forEachRight(collection, callback) {
+ var length = collection ? collection.length : 0;
+ if (typeof length == 'number') {
+ while (length--) {
+ if (callback(collection[length], length, collection) === false) {
+ break;
+ }
+ }
+ } else {
+ var props = keys(collection);
+ length = props.length;
+ forOwn(collection, function(value, key, collection) {
+ key = props ? props[--length] : --length;
+ return callback(collection[key], key, collection) === false && indicatorObject;
+ });
+ }
+}
+
+module.exports = forEachRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/groupBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/groupBy.js b/node_modules/lodash-node/underscore/collections/groupBy.js
new file mode 100644
index 0000000..739312c
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/groupBy.js
@@ -0,0 +1,56 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of a collection through the callback. The corresponding value
+ * of each key is an array of the elements responsible for generating the key.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+var groupBy = createAggregator(function(result, value, key) {
+ (hasOwnProperty.call(result, key) ? result[key] : result[key] = []).push(value);
+});
+
+module.exports = groupBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/indexBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/indexBy.js b/node_modules/lodash-node/underscore/collections/indexBy.js
new file mode 100644
index 0000000..795256f
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/indexBy.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of the collection through the given callback. The corresponding
+ * value of each key is the last element responsible for generating the key.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * var keys = [
+ * { 'dir': 'left', 'code': 97 },
+ * { 'dir': 'right', 'code': 100 }
+ * ];
+ *
+ * _.indexBy(keys, 'dir');
+ * // => { 'left': { 'dir': 'left', 'code': 97 }, 'right': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(keys, function(key) { return String.fromCharCode(key.code); });
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(characters, function(key) { this.fromCharCode(key.code); }, String);
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ */
+var indexBy = createAggregator(function(result, value, key) {
+ result[key] = value;
+});
+
+module.exports = indexBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/invoke.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/invoke.js b/node_modules/lodash-node/underscore/collections/invoke.js
new file mode 100644
index 0000000..3f36076
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/invoke.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('./forEach'),
+ slice = require('../internals/slice');
+
+/**
+ * Invokes the method named by `methodName` on each element in the `collection`
+ * returning an array of the results of each invoked method. Additional arguments
+ * will be provided to each invoked method. If `methodName` is a function it
+ * will be invoked for, and `this` bound to, each element in the `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|string} methodName The name of the method to invoke or
+ * the function invoked per iteration.
+ * @param {...*} [arg] Arguments to invoke the method with.
+ * @returns {Array} Returns a new array of the results of each invoked method.
+ * @example
+ *
+ * _.invoke([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invoke([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+function invoke(collection, methodName) {
+ var args = slice(arguments, 2),
+ index = -1,
+ isFunc = typeof methodName == 'function',
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ result[++index] = (isFunc ? methodName : value[methodName]).apply(value, args);
+ });
+ return result;
+}
+
+module.exports = invoke;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/map.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/map.js b/node_modules/lodash-node/underscore/collections/map.js
new file mode 100644
index 0000000..85b4469
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/map.js
@@ -0,0 +1,70 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Creates an array of values by running each element in the collection
+ * through the callback. The callback is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias collect
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of the results of each `callback` execution.
+ * @example
+ *
+ * _.map([1, 2, 3], function(num) { return num * 3; });
+ * // => [3, 6, 9]
+ *
+ * _.map({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { return num * 3; });
+ * // => [3, 6, 9] (property order is not guaranteed across environments)
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(characters, 'name');
+ * // => ['barney', 'fred']
+ */
+function map(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ if (typeof length == 'number') {
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = callback(collection[index], index, collection);
+ }
+ } else {
+ result = [];
+ forOwn(collection, function(value, key, collection) {
+ result[++index] = callback(value, key, collection);
+ });
+ }
+ return result;
+}
+
+module.exports = map;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/max.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/max.js b/node_modules/lodash-node/underscore/collections/max.js
new file mode 100644
index 0000000..576e634
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/max.js
@@ -0,0 +1,86 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Retrieves the maximum value of a collection. If the collection is empty or
+ * falsey `-Infinity` is returned. If a callback is provided it will be executed
+ * for each value in the collection to generate the criterion by which the value
+ * is ranked. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the maximum value.
+ * @example
+ *
+ * _.max([4, 2, 8, 6]);
+ * // => 8
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.max(characters, function(chr) { return chr.age; });
+ * // => { 'name': 'fred', 'age': 40 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.max(characters, 'age');
+ * // => { 'name': 'fred', 'age': 40 };
+ */
+function max(collection, callback, thisArg) {
+ var computed = -Infinity,
+ result = computed;
+
+ // allows working with functions like `_.map` without using
+ // their `index` argument as a callback
+ if (typeof callback != 'function' && thisArg && thisArg[callback] === collection) {
+ callback = null;
+ }
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (callback == null && typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ if (value > result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = createCallback(callback, thisArg, 3);
+
+ forEach(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current > computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = max;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/min.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/min.js b/node_modules/lodash-node/underscore/collections/min.js
new file mode 100644
index 0000000..21ef973
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/min.js
@@ -0,0 +1,86 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Retrieves the minimum value of a collection. If the collection is empty or
+ * falsey `Infinity` is returned. If a callback is provided it will be executed
+ * for each value in the collection to generate the criterion by which the value
+ * is ranked. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the minimum value.
+ * @example
+ *
+ * _.min([4, 2, 8, 6]);
+ * // => 2
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.min(characters, function(chr) { return chr.age; });
+ * // => { 'name': 'barney', 'age': 36 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.min(characters, 'age');
+ * // => { 'name': 'barney', 'age': 36 };
+ */
+function min(collection, callback, thisArg) {
+ var computed = Infinity,
+ result = computed;
+
+ // allows working with functions like `_.map` without using
+ // their `index` argument as a callback
+ if (typeof callback != 'function' && thisArg && thisArg[callback] === collection) {
+ callback = null;
+ }
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (callback == null && typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ if (value < result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = createCallback(callback, thisArg, 3);
+
+ forEach(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current < computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = min;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/pluck.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/pluck.js b/node_modules/lodash-node/underscore/collections/pluck.js
new file mode 100644
index 0000000..66855c9
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/pluck.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var map = require('./map');
+
+/**
+ * Retrieves the value of a specified property from all elements in the collection.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {string} property The name of the property to pluck.
+ * @returns {Array} Returns a new array of property values.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.pluck(characters, 'name');
+ * // => ['barney', 'fred']
+ */
+var pluck = map;
+
+module.exports = pluck;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/reduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/reduce.js b/node_modules/lodash-node/underscore/collections/reduce.js
new file mode 100644
index 0000000..5f0a15f
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/reduce.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Reduces a collection to a value which is the accumulated result of running
+ * each element in the collection through the callback, where each successive
+ * callback execution consumes the return value of the previous execution. If
+ * `accumulator` is not provided the first element of the collection will be
+ * used as the initial `accumulator` value. The callback is bound to `thisArg`
+ * and invoked with four arguments; (accumulator, value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @alias foldl, inject
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] Initial value of the accumulator.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var sum = _.reduce([1, 2, 3], function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * var mapped = _.reduce({ 'a': 1, 'b': 2, 'c': 3 }, function(result, num, key) {
+ * result[key] = num * 3;
+ * return result;
+ * }, {});
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ */
+function reduce(collection, callback, accumulator, thisArg) {
+ if (!collection) return accumulator;
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+
+ var index = -1,
+ length = collection.length;
+
+ if (typeof length == 'number') {
+ if (noaccum) {
+ accumulator = collection[++index];
+ }
+ while (++index < length) {
+ accumulator = callback(accumulator, collection[index], index, collection);
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection)
+ });
+ }
+ return accumulator;
+}
+
+module.exports = reduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/reduceRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/reduceRight.js b/node_modules/lodash-node/underscore/collections/reduceRight.js
new file mode 100644
index 0000000..6ebae93
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/reduceRight.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEachRight = require('./forEachRight');
+
+/**
+ * This method is like `_.reduce` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias foldr
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] Initial value of the accumulator.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var list = [[0, 1], [2, 3], [4, 5]];
+ * var flat = _.reduceRight(list, function(a, b) { return a.concat(b); }, []);
+ * // => [4, 5, 2, 3, 0, 1]
+ */
+function reduceRight(collection, callback, accumulator, thisArg) {
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+ forEachRight(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection);
+ });
+ return accumulator;
+}
+
+module.exports = reduceRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/reject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/reject.js b/node_modules/lodash-node/underscore/collections/reject.js
new file mode 100644
index 0000000..31b32e7
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/reject.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ filter = require('./filter');
+
+/**
+ * The opposite of `_.filter` this method returns the elements of a
+ * collection that the callback does **not** return truey for.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that failed the callback check.
+ * @example
+ *
+ * var odds = _.reject([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [1, 3, 5]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.reject(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'age': 36, 'blocked': false }]
+ *
+ * // using "_.where" callback shorthand
+ * _.reject(characters, { 'age': 36 });
+ * // => [{ 'name': 'fred', 'age': 40, 'blocked': true }]
+ */
+function reject(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg, 3);
+ return filter(collection, function(value, index, collection) {
+ return !callback(value, index, collection);
+ });
+}
+
+module.exports = reject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/sample.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/sample.js b/node_modules/lodash-node/underscore/collections/sample.js
new file mode 100644
index 0000000..b851923
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/sample.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom'),
+ isString = require('../objects/isString'),
+ shuffle = require('./shuffle'),
+ values = require('../objects/values');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Retrieves a random element or `n` random elements from a collection.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to sample.
+ * @param {number} [n] The number of elements to sample.
+ * @param- {Object} [guard] Allows working with functions like `_.map`
+ * without using their `index` arguments as `n`.
+ * @returns {Array} Returns the random sample(s) of `collection`.
+ * @example
+ *
+ * _.sample([1, 2, 3, 4]);
+ * // => 2
+ *
+ * _.sample([1, 2, 3, 4], 2);
+ * // => [3, 1]
+ */
+function sample(collection, n, guard) {
+ if (collection && typeof collection.length != 'number') {
+ collection = values(collection);
+ }
+ if (n == null || guard) {
+ return collection ? collection[baseRandom(0, collection.length - 1)] : undefined;
+ }
+ var result = shuffle(collection);
+ result.length = nativeMin(nativeMax(0, n), result.length);
+ return result;
+}
+
+module.exports = sample;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[49/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/big-integer/BigInteger.js
----------------------------------------------------------------------
diff --git a/node_modules/big-integer/BigInteger.js b/node_modules/big-integer/BigInteger.js
new file mode 100644
index 0000000..39b6006
--- /dev/null
+++ b/node_modules/big-integer/BigInteger.js
@@ -0,0 +1,1189 @@
+var bigInt = (function (undefined) {
+ "use strict";
+
+ var BASE = 1e7,
+ LOG_BASE = 7,
+ MAX_INT = 9007199254740992,
+ MAX_INT_ARR = smallToArray(MAX_INT),
+ LOG_MAX_INT = Math.log(MAX_INT);
+
+ function BigInteger(value, sign) {
+ this.value = value;
+ this.sign = sign;
+ this.isSmall = false;
+ }
+
+ function SmallInteger(value) {
+ this.value = value;
+ this.sign = value < 0;
+ this.isSmall = true;
+ }
+
+ function isPrecise(n) {
+ return -MAX_INT < n && n < MAX_INT;
+ }
+
+ function smallToArray(n) { // For performance reasons doesn't reference BASE, need to change this function if BASE changes
+ if (n < 1e7)
+ return [n];
+ if (n < 1e14)
+ return [n % 1e7, Math.floor(n / 1e7)];
+ return [n % 1e7, Math.floor(n / 1e7) % 1e7, Math.floor(n / 1e14)];
+ }
+
+ function arrayToSmall(arr) { // If BASE changes this function may need to change
+ trim(arr);
+ var length = arr.length;
+ if (length < 4 && compareAbs(arr, MAX_INT_ARR) < 0) {
+ switch (length) {
+ case 0: return 0;
+ case 1: return arr[0];
+ case 2: return arr[0] + arr[1] * BASE;
+ default: return arr[0] + (arr[1] + arr[2] * BASE) * BASE;
+ }
+ }
+ return arr;
+ }
+
+ function trim(v) {
+ var i = v.length;
+ while (v[--i] === 0);
+ v.length = i + 1;
+ }
+
+ function createArray(length) { // function shamelessly stolen from Yaffle's library https://github.com/Yaffle/BigInteger
+ var x = new Array(length);
+ var i = -1;
+ while (++i < length) {
+ x[i] = 0;
+ }
+ return x;
+ }
+
+ function truncate(n) {
+ if (n > 0) return Math.floor(n);
+ return Math.ceil(n);
+ }
+
+ function add(a, b) { // assumes a and b are arrays with a.length >= b.length
+ var l_a = a.length,
+ l_b = b.length,
+ r = new Array(l_a),
+ carry = 0,
+ base = BASE,
+ sum, i;
+ for (i = 0; i < l_b; i++) {
+ sum = a[i] + b[i] + carry;
+ carry = sum >= base ? 1 : 0;
+ r[i] = sum - carry * base;
+ }
+ while (i < l_a) {
+ sum = a[i] + carry;
+ carry = sum === base ? 1 : 0;
+ r[i++] = sum - carry * base;
+ }
+ if (carry > 0) r.push(carry);
+ return r;
+ }
+
+ function addAny(a, b) {
+ if (a.length >= b.length) return add(a, b);
+ return add(b, a);
+ }
+
+ function addSmall(a, carry) { // assumes a is array, carry is number with 0 <= carry < MAX_INT
+ var l = a.length,
+ r = new Array(l),
+ base = BASE,
+ sum, i;
+ for (i = 0; i < l; i++) {
+ sum = a[i] - base + carry;
+ carry = Math.floor(sum / base);
+ r[i] = sum - carry * base;
+ carry += 1;
+ }
+ while (carry > 0) {
+ r[i++] = carry % base;
+ carry = Math.floor(carry / base);
+ }
+ return r;
+ }
+
+ BigInteger.prototype.add = function (v) {
+ var value, n = parseValue(v);
+ if (this.sign !== n.sign) {
+ return this.subtract(n.negate());
+ }
+ var a = this.value, b = n.value;
+ if (n.isSmall) {
+ return new BigInteger(addSmall(a, Math.abs(b)), this.sign);
+ }
+ return new BigInteger(addAny(a, b), this.sign);
+ };
+ BigInteger.prototype.plus = BigInteger.prototype.add;
+
+ SmallInteger.prototype.add = function (v) {
+ var n = parseValue(v);
+ var a = this.value;
+ if (a < 0 !== n.sign) {
+ return this.subtract(n.negate());
+ }
+ var b = n.value;
+ if (n.isSmall) {
+ if (isPrecise(a + b)) return new SmallInteger(a + b);
+ b = smallToArray(Math.abs(b));
+ }
+ return new BigInteger(addSmall(b, Math.abs(a)), a < 0);
+ };
+ SmallInteger.prototype.plus = SmallInteger.prototype.add;
+
+ function subtract(a, b) { // assumes a and b are arrays with a >= b
+ var a_l = a.length,
+ b_l = b.length,
+ r = new Array(a_l),
+ borrow = 0,
+ base = BASE,
+ i, difference;
+ for (i = 0; i < b_l; i++) {
+ difference = a[i] - borrow - b[i];
+ if (difference < 0) {
+ difference += base;
+ borrow = 1;
+ } else borrow = 0;
+ r[i] = difference;
+ }
+ for (i = b_l; i < a_l; i++) {
+ difference = a[i] - borrow;
+ if (difference < 0) difference += base;
+ else {
+ r[i++] = difference;
+ break;
+ }
+ r[i] = difference;
+ }
+ for (; i < a_l; i++) {
+ r[i] = a[i];
+ }
+ trim(r);
+ return r;
+ }
+
+ function subtractAny(a, b, sign) {
+ var value, isSmall;
+ if (compareAbs(a, b) >= 0) {
+ value = subtract(a,b);
+ } else {
+ value = subtract(b, a);
+ sign = !sign;
+ }
+ value = arrayToSmall(value);
+ if (typeof value === "number") {
+ if (sign) value = -value;
+ return new SmallInteger(value);
+ }
+ return new BigInteger(value, sign);
+ }
+
+ function subtractSmall(a, b, sign) { // assumes a is array, b is number with 0 <= b < MAX_INT
+ var l = a.length,
+ r = new Array(l),
+ carry = -b,
+ base = BASE,
+ i, difference;
+ for (i = 0; i < l; i++) {
+ difference = a[i] + carry;
+ carry = Math.floor(difference / base);
+ difference %= base;
+ r[i] = difference < 0 ? difference + base : difference;
+ }
+ r = arrayToSmall(r);
+ if (typeof r === "number") {
+ if (sign) r = -r;
+ return new SmallInteger(r);
+ } return new BigInteger(r, sign);
+ }
+
+ BigInteger.prototype.subtract = function (v) {
+ var n = parseValue(v);
+ if (this.sign !== n.sign) {
+ return this.add(n.negate());
+ }
+ var a = this.value, b = n.value;
+ if (n.isSmall)
+ return subtractSmall(a, Math.abs(b), this.sign);
+ return subtractAny(a, b, this.sign);
+ };
+ BigInteger.prototype.minus = BigInteger.prototype.subtract;
+
+ SmallInteger.prototype.subtract = function (v) {
+ var n = parseValue(v);
+ var a = this.value;
+ if (a < 0 !== n.sign) {
+ return this.add(n.negate());
+ }
+ var b = n.value;
+ if (n.isSmall) {
+ return new SmallInteger(a - b);
+ }
+ return subtractSmall(b, Math.abs(a), a >= 0);
+ };
+ SmallInteger.prototype.minus = SmallInteger.prototype.subtract;
+
+ BigInteger.prototype.negate = function () {
+ return new BigInteger(this.value, !this.sign);
+ };
+ SmallInteger.prototype.negate = function () {
+ var sign = this.sign;
+ var small = new SmallInteger(-this.value);
+ small.sign = !sign;
+ return small;
+ };
+
+ BigInteger.prototype.abs = function () {
+ return new BigInteger(this.value, false);
+ };
+ SmallInteger.prototype.abs = function () {
+ return new SmallInteger(Math.abs(this.value));
+ };
+
+ function multiplyLong(a, b) {
+ var a_l = a.length,
+ b_l = b.length,
+ l = a_l + b_l,
+ r = createArray(l),
+ base = BASE,
+ product, carry, i, a_i, b_j;
+ for (i = 0; i < a_l; ++i) {
+ a_i = a[i];
+ for (var j = 0; j < b_l; ++j) {
+ b_j = b[j];
+ product = a_i * b_j + r[i + j];
+ carry = Math.floor(product / base);
+ r[i + j] = product - carry * base;
+ r[i + j + 1] += carry;
+ }
+ }
+ trim(r);
+ return r;
+ }
+
+ function multiplySmall(a, b) { // assumes a is array, b is number with |b| < BASE
+ var l = a.length,
+ r = new Array(l),
+ base = BASE,
+ carry = 0,
+ product, i;
+ for (i = 0; i < l; i++) {
+ product = a[i] * b + carry;
+ carry = Math.floor(product / base);
+ r[i] = product - carry * base;
+ }
+ while (carry > 0) {
+ r[i++] = carry % base;
+ carry = Math.floor(carry / base);
+ }
+ return r;
+ }
+
+ function shiftLeft(x, n) {
+ var r = [];
+ while (n-- > 0) r.push(0);
+ return r.concat(x);
+ }
+
+ function multiplyKaratsuba(x, y) {
+ var n = Math.max(x.length, y.length);
+
+ if (n <= 30) return multiplyLong(x, y);
+ n = Math.ceil(n / 2);
+
+ var b = x.slice(n),
+ a = x.slice(0, n),
+ d = y.slice(n),
+ c = y.slice(0, n);
+
+ var ac = multiplyKaratsuba(a, c),
+ bd = multiplyKaratsuba(b, d),
+ abcd = multiplyKaratsuba(addAny(a, b), addAny(c, d));
+
+ var product = addAny(addAny(ac, shiftLeft(subtract(subtract(abcd, ac), bd), n)), shiftLeft(bd, 2 * n));
+ trim(product);
+ return product;
+ }
+
+ // The following function is derived from a surface fit of a graph plotting the performance difference
+ // between long multiplication and karatsuba multiplication versus the lengths of the two arrays.
+ function useKaratsuba(l1, l2) {
+ return -0.012 * l1 - 0.012 * l2 + 0.000015 * l1 * l2 > 0;
+ }
+
+ BigInteger.prototype.multiply = function (v) {
+ var value, n = parseValue(v),
+ a = this.value, b = n.value,
+ sign = this.sign !== n.sign,
+ abs;
+ if (n.isSmall) {
+ if (b === 0) return CACHE[0];
+ if (b === 1) return this;
+ if (b === -1) return this.negate();
+ abs = Math.abs(b);
+ if (abs < BASE) {
+ return new BigInteger(multiplySmall(a, abs), sign);
+ }
+ b = smallToArray(abs);
+ }
+ if (useKaratsuba(a.length, b.length)) // Karatsuba is only faster for certain array sizes
+ return new BigInteger(multiplyKaratsuba(a, b), sign);
+ return new BigInteger(multiplyLong(a, b), sign);
+ };
+
+ BigInteger.prototype.times = BigInteger.prototype.multiply;
+
+ function multiplySmallAndArray(a, b, sign) { // a >= 0
+ if (a < BASE) {
+ return new BigInteger(multiplySmall(b, a), sign);
+ }
+ return new BigInteger(multiplyLong(b, smallToArray(a)), sign);
+ }
+ SmallInteger.prototype["_multiplyBySmall"] = function (a) {
+ if (isPrecise(a.value * this.value)) {
+ return new SmallInteger(a.value * this.value);
+ }
+ return multiplySmallAndArray(Math.abs(a.value), smallToArray(Math.abs(this.value)), this.sign !== a.sign);
+ };
+ BigInteger.prototype["_multiplyBySmall"] = function (a) {
+ if (a.value === 0) return CACHE[0];
+ if (a.value === 1) return this;
+ if (a.value === -1) return this.negate();
+ return multiplySmallAndArray(Math.abs(a.value), this.value, this.sign !== a.sign);
+ };
+ SmallInteger.prototype.multiply = function (v) {
+ return parseValue(v)["_multiplyBySmall"](this);
+ };
+ SmallInteger.prototype.times = SmallInteger.prototype.multiply;
+
+ function square(a) {
+ var l = a.length,
+ r = createArray(l + l),
+ base = BASE,
+ product, carry, i, a_i, a_j;
+ for (i = 0; i < l; i++) {
+ a_i = a[i];
+ for (var j = 0; j < l; j++) {
+ a_j = a[j];
+ product = a_i * a_j + r[i + j];
+ carry = Math.floor(product / base);
+ r[i + j] = product - carry * base;
+ r[i + j + 1] += carry;
+ }
+ }
+ trim(r);
+ return r;
+ }
+
+ BigInteger.prototype.square = function () {
+ return new BigInteger(square(this.value), false);
+ };
+
+ SmallInteger.prototype.square = function () {
+ var value = this.value * this.value;
+ if (isPrecise(value)) return new SmallInteger(value);
+ return new BigInteger(square(smallToArray(Math.abs(this.value))), false);
+ };
+
+ function divMod1(a, b) { // Left over from previous version. Performs faster than divMod2 on smaller input sizes.
+ var a_l = a.length,
+ b_l = b.length,
+ base = BASE,
+ result = createArray(b.length),
+ divisorMostSignificantDigit = b[b_l - 1],
+ // normalization
+ lambda = Math.ceil(base / (2 * divisorMostSignificantDigit)),
+ remainder = multiplySmall(a, lambda),
+ divisor = multiplySmall(b, lambda),
+ quotientDigit, shift, carry, borrow, i, l, q;
+ if (remainder.length <= a_l) remainder.push(0);
+ divisor.push(0);
+ divisorMostSignificantDigit = divisor[b_l - 1];
+ for (shift = a_l - b_l; shift >= 0; shift--) {
+ quotientDigit = base - 1;
+ if (remainder[shift + b_l] !== divisorMostSignificantDigit) {
+ quotientDigit = Math.floor((remainder[shift + b_l] * base + remainder[shift + b_l - 1]) / divisorMostSignificantDigit);
+ }
+ // quotientDigit <= base - 1
+ carry = 0;
+ borrow = 0;
+ l = divisor.length;
+ for (i = 0; i < l; i++) {
+ carry += quotientDigit * divisor[i];
+ q = Math.floor(carry / base);
+ borrow += remainder[shift + i] - (carry - q * base);
+ carry = q;
+ if (borrow < 0) {
+ remainder[shift + i] = borrow + base;
+ borrow = -1;
+ } else {
+ remainder[shift + i] = borrow;
+ borrow = 0;
+ }
+ }
+ while (borrow !== 0) {
+ quotientDigit -= 1;
+ carry = 0;
+ for (i = 0; i < l; i++) {
+ carry += remainder[shift + i] - base + divisor[i];
+ if (carry < 0) {
+ remainder[shift + i] = carry + base;
+ carry = 0;
+ } else {
+ remainder[shift + i] = carry;
+ carry = 1;
+ }
+ }
+ borrow += carry;
+ }
+ result[shift] = quotientDigit;
+ }
+ // denormalization
+ remainder = divModSmall(remainder, lambda)[0];
+ return [arrayToSmall(result), arrayToSmall(remainder)];
+ }
+
+ function divMod2(a, b) { // Implementation idea shamelessly stolen from Silent Matt's library http://silentmatt.com/biginteger/
+ // Performs faster than divMod1 on larger input sizes.
+ var a_l = a.length,
+ b_l = b.length,
+ result = [],
+ part = [],
+ base = BASE,
+ guess, xlen, highx, highy, check;
+ while (a_l) {
+ part.unshift(a[--a_l]);
+ if (compareAbs(part, b) < 0) {
+ result.push(0);
+ continue;
+ }
+ xlen = part.length;
+ highx = part[xlen - 1] * base + part[xlen - 2];
+ highy = b[b_l - 1] * base + b[b_l - 2];
+ if (xlen > b_l) {
+ highx = (highx + 1) * base;
+ }
+ guess = Math.ceil(highx / highy);
+ do {
+ check = multiplySmall(b, guess);
+ if (compareAbs(check, part) <= 0) break;
+ guess--;
+ } while (guess);
+ result.push(guess);
+ part = subtract(part, check);
+ }
+ result.reverse();
+ return [arrayToSmall(result), arrayToSmall(part)];
+ }
+
+ function divModSmall(value, lambda) {
+ var length = value.length,
+ quotient = createArray(length),
+ base = BASE,
+ i, q, remainder, divisor;
+ remainder = 0;
+ for (i = length - 1; i >= 0; --i) {
+ divisor = remainder * base + value[i];
+ q = truncate(divisor / lambda);
+ remainder = divisor - q * lambda;
+ quotient[i] = q | 0;
+ }
+ return [quotient, remainder | 0];
+ }
+
+ function divModAny(self, v) {
+ var value, n = parseValue(v);
+ var a = self.value, b = n.value;
+ var quotient;
+ if (b === 0) throw new Error("Cannot divide by zero");
+ if (self.isSmall) {
+ if (n.isSmall) {
+ return [new SmallInteger(truncate(a / b)), new SmallInteger(a % b)];
+ }
+ return [CACHE[0], self];
+ }
+ if (n.isSmall) {
+ if (b === 1) return [self, CACHE[0]];
+ if (b == -1) return [self.negate(), CACHE[0]];
+ var abs = Math.abs(b);
+ if (abs < BASE) {
+ value = divModSmall(a, abs);
+ quotient = arrayToSmall(value[0]);
+ var remainder = value[1];
+ if (self.sign) remainder = -remainder;
+ if (typeof quotient === "number") {
+ if (self.sign !== n.sign) quotient = -quotient;
+ return [new SmallInteger(quotient), new SmallInteger(remainder)];
+ }
+ return [new BigInteger(quotient, self.sign !== n.sign), new SmallInteger(remainder)];
+ }
+ b = smallToArray(abs);
+ }
+ var comparison = compareAbs(a, b);
+ if (comparison === -1) return [CACHE[0], self];
+ if (comparison === 0) return [CACHE[self.sign === n.sign ? 1 : -1], CACHE[0]];
+
+ // divMod1 is faster on smaller input sizes
+ if (a.length + b.length <= 200)
+ value = divMod1(a, b);
+ else value = divMod2(a, b);
+
+ quotient = value[0];
+ var qSign = self.sign !== n.sign,
+ mod = value[1],
+ mSign = self.sign;
+ if (typeof quotient === "number") {
+ if (qSign) quotient = -quotient;
+ quotient = new SmallInteger(quotient);
+ } else quotient = new BigInteger(quotient, qSign);
+ if (typeof mod === "number") {
+ if (mSign) mod = -mod;
+ mod = new SmallInteger(mod);
+ } else mod = new BigInteger(mod, mSign);
+ return [quotient, mod];
+ }
+
+ BigInteger.prototype.divmod = function (v) {
+ var result = divModAny(this, v);
+ return {
+ quotient: result[0],
+ remainder: result[1]
+ };
+ };
+ SmallInteger.prototype.divmod = BigInteger.prototype.divmod;
+
+ BigInteger.prototype.divide = function (v) {
+ return divModAny(this, v)[0];
+ };
+ SmallInteger.prototype.over = SmallInteger.prototype.divide = BigInteger.prototype.over = BigInteger.prototype.divide;
+
+ BigInteger.prototype.mod = function (v) {
+ return divModAny(this, v)[1];
+ };
+ SmallInteger.prototype.remainder = SmallInteger.prototype.mod = BigInteger.prototype.remainder = BigInteger.prototype.mod;
+
+ BigInteger.prototype.pow = function (v) {
+ var n = parseValue(v),
+ a = this.value,
+ b = n.value,
+ value, x, y;
+ if (b === 0) return CACHE[1];
+ if (a === 0) return CACHE[0];
+ if (a === 1) return CACHE[1];
+ if (a === -1) return n.isEven() ? CACHE[1] : CACHE[-1];
+ if (n.sign) {
+ return CACHE[0];
+ }
+ if (!n.isSmall) throw new Error("The exponent " + n.toString() + " is too large.");
+ if (this.isSmall) {
+ if (isPrecise(value = Math.pow(a, b)))
+ return new SmallInteger(truncate(value));
+ }
+ x = this;
+ y = CACHE[1];
+ while (true) {
+ if (b & 1 === 1) {
+ y = y.times(x);
+ --b;
+ }
+ if (b === 0) break;
+ b /= 2;
+ x = x.square();
+ }
+ return y;
+ };
+ SmallInteger.prototype.pow = BigInteger.prototype.pow;
+
+ BigInteger.prototype.modPow = function (exp, mod) {
+ exp = parseValue(exp);
+ mod = parseValue(mod);
+ if (mod.isZero()) throw new Error("Cannot take modPow with modulus 0");
+ var r = CACHE[1],
+ base = this.mod(mod);
+ while (exp.isPositive()) {
+ if (base.isZero()) return CACHE[0];
+ if (exp.isOdd()) r = r.multiply(base).mod(mod);
+ exp = exp.divide(2);
+ base = base.square().mod(mod);
+ }
+ return r;
+ };
+ SmallInteger.prototype.modPow = BigInteger.prototype.modPow;
+
+ function compareAbs(a, b) {
+ if (a.length !== b.length) {
+ return a.length > b.length ? 1 : -1;
+ }
+ for (var i = a.length - 1; i >= 0; i--) {
+ if (a[i] !== b[i]) return a[i] > b[i] ? 1 : -1;
+ }
+ return 0;
+ }
+
+ BigInteger.prototype.compareAbs = function (v) {
+ var n = parseValue(v),
+ a = this.value,
+ b = n.value;
+ if (n.isSmall) return 1;
+ return compareAbs(a, b);
+ };
+ SmallInteger.prototype.compareAbs = function (v) {
+ var n = parseValue(v),
+ a = Math.abs(this.value),
+ b = n.value;
+ if (n.isSmall) {
+ b = Math.abs(b);
+ return a === b ? 0 : a > b ? 1 : -1;
+ }
+ return -1;
+ };
+
+ BigInteger.prototype.compare = function (v) {
+ // See discussion about comparison with Infinity:
+ // https://github.com/peterolson/BigInteger.js/issues/61
+ if (v === Infinity) {
+ return -1;
+ }
+ if (v === -Infinity) {
+ return 1;
+ }
+
+ var n = parseValue(v),
+ a = this.value,
+ b = n.value;
+ if (this.sign !== n.sign) {
+ return n.sign ? 1 : -1;
+ }
+ if (n.isSmall) {
+ return this.sign ? -1 : 1;
+ }
+ return compareAbs(a, b) * (this.sign ? -1 : 1);
+ };
+ BigInteger.prototype.compareTo = BigInteger.prototype.compare;
+
+ SmallInteger.prototype.compare = function (v) {
+ if (v === Infinity) {
+ return -1;
+ }
+ if (v === -Infinity) {
+ return 1;
+ }
+
+ var n = parseValue(v),
+ a = this.value,
+ b = n.value;
+ if (n.isSmall) {
+ return a == b ? 0 : a > b ? 1 : -1;
+ }
+ if (a < 0 !== n.sign) {
+ return a < 0 ? -1 : 1;
+ }
+ return a < 0 ? 1 : -1;
+ };
+ SmallInteger.prototype.compareTo = SmallInteger.prototype.compare;
+
+ BigInteger.prototype.equals = function (v) {
+ return this.compare(v) === 0;
+ };
+ SmallInteger.prototype.eq = SmallInteger.prototype.equals = BigInteger.prototype.eq = BigInteger.prototype.equals;
+
+ BigInteger.prototype.notEquals = function (v) {
+ return this.compare(v) !== 0;
+ };
+ SmallInteger.prototype.neq = SmallInteger.prototype.notEquals = BigInteger.prototype.neq = BigInteger.prototype.notEquals;
+
+ BigInteger.prototype.greater = function (v) {
+ return this.compare(v) > 0;
+ };
+ SmallInteger.prototype.gt = SmallInteger.prototype.greater = BigInteger.prototype.gt = BigInteger.prototype.greater;
+
+ BigInteger.prototype.lesser = function (v) {
+ return this.compare(v) < 0;
+ };
+ SmallInteger.prototype.lt = SmallInteger.prototype.lesser = BigInteger.prototype.lt = BigInteger.prototype.lesser;
+
+ BigInteger.prototype.greaterOrEquals = function (v) {
+ return this.compare(v) >= 0;
+ };
+ SmallInteger.prototype.geq = SmallInteger.prototype.greaterOrEquals = BigInteger.prototype.geq = BigInteger.prototype.greaterOrEquals;
+
+ BigInteger.prototype.lesserOrEquals = function (v) {
+ return this.compare(v) <= 0;
+ };
+ SmallInteger.prototype.leq = SmallInteger.prototype.lesserOrEquals = BigInteger.prototype.leq = BigInteger.prototype.lesserOrEquals;
+
+ BigInteger.prototype.isEven = function () {
+ return (this.value[0] & 1) === 0;
+ };
+ SmallInteger.prototype.isEven = function () {
+ return (this.value & 1) === 0;
+ };
+
+ BigInteger.prototype.isOdd = function () {
+ return (this.value[0] & 1) === 1;
+ };
+ SmallInteger.prototype.isOdd = function () {
+ return (this.value & 1) === 1;
+ };
+
+ BigInteger.prototype.isPositive = function () {
+ return !this.sign;
+ };
+ SmallInteger.prototype.isPositive = function () {
+ return this.value > 0;
+ };
+
+ BigInteger.prototype.isNegative = function () {
+ return this.sign;
+ };
+ SmallInteger.prototype.isNegative = function () {
+ return this.value < 0;
+ };
+
+ BigInteger.prototype.isUnit = function () {
+ return false;
+ };
+ SmallInteger.prototype.isUnit = function () {
+ return Math.abs(this.value) === 1;
+ };
+
+ BigInteger.prototype.isZero = function () {
+ return false;
+ };
+ SmallInteger.prototype.isZero = function () {
+ return this.value === 0;
+ };
+ BigInteger.prototype.isDivisibleBy = function (v) {
+ var n = parseValue(v);
+ var value = n.value;
+ if (value === 0) return false;
+ if (value === 1) return true;
+ if (value === 2) return this.isEven();
+ return this.mod(n).equals(CACHE[0]);
+ };
+ SmallInteger.prototype.isDivisibleBy = BigInteger.prototype.isDivisibleBy;
+
+ function isBasicPrime(v) {
+ var n = v.abs();
+ if (n.isUnit()) return false;
+ if (n.equals(2) || n.equals(3) || n.equals(5)) return true;
+ if (n.isEven() || n.isDivisibleBy(3) || n.isDivisibleBy(5)) return false;
+ if (n.lesser(25)) return true;
+ // we don't know if it's prime: let the other functions figure it out
+ };
+
+ BigInteger.prototype.isPrime = function () {
+ var isPrime = isBasicPrime(this);
+ if (isPrime !== undefined) return isPrime;
+ var n = this.abs(),
+ nPrev = n.prev();
+ var a = [2, 3, 5, 7, 11, 13, 17, 19],
+ b = nPrev,
+ d, t, i, x;
+ while (b.isEven()) b = b.divide(2);
+ for (i = 0; i < a.length; i++) {
+ x = bigInt(a[i]).modPow(b, n);
+ if (x.equals(CACHE[1]) || x.equals(nPrev)) continue;
+ for (t = true, d = b; t && d.lesser(nPrev) ; d = d.multiply(2)) {
+ x = x.square().mod(n);
+ if (x.equals(nPrev)) t = false;
+ }
+ if (t) return false;
+ }
+ return true;
+ };
+ SmallInteger.prototype.isPrime = BigInteger.prototype.isPrime;
+
+ BigInteger.prototype.isProbablePrime = function (iterations) {
+ var isPrime = isBasicPrime(this);
+ if (isPrime !== undefined) return isPrime;
+ var n = this.abs();
+ var t = iterations === undefined ? 5 : iterations;
+ // use the Fermat primality test
+ for (var i = 0; i < t; i++) {
+ var a = bigInt.randBetween(2, n.minus(2));
+ if (!a.modPow(n.prev(), n).isUnit()) return false; // definitely composite
+ }
+ return true; // large chance of being prime
+ };
+ SmallInteger.prototype.isProbablePrime = BigInteger.prototype.isProbablePrime;
+
+ BigInteger.prototype.next = function () {
+ var value = this.value;
+ if (this.sign) {
+ return subtractSmall(value, 1, this.sign);
+ }
+ return new BigInteger(addSmall(value, 1), this.sign);
+ };
+ SmallInteger.prototype.next = function () {
+ var value = this.value;
+ if (value + 1 < MAX_INT) return new SmallInteger(value + 1);
+ return new BigInteger(MAX_INT_ARR, false);
+ };
+
+ BigInteger.prototype.prev = function () {
+ var value = this.value;
+ if (this.sign) {
+ return new BigInteger(addSmall(value, 1), true);
+ }
+ return subtractSmall(value, 1, this.sign);
+ };
+ SmallInteger.prototype.prev = function () {
+ var value = this.value;
+ if (value - 1 > -MAX_INT) return new SmallInteger(value - 1);
+ return new BigInteger(MAX_INT_ARR, true);
+ };
+
+ var powersOfTwo = [1];
+ while (powersOfTwo[powersOfTwo.length - 1] <= BASE) powersOfTwo.push(2 * powersOfTwo[powersOfTwo.length - 1]);
+ var powers2Length = powersOfTwo.length, highestPower2 = powersOfTwo[powers2Length - 1];
+
+ function shift_isSmall(n) {
+ return ((typeof n === "number" || typeof n === "string") && +Math.abs(n) <= BASE) ||
+ (n instanceof BigInteger && n.value.length <= 1);
+ }
+
+ BigInteger.prototype.shiftLeft = function (n) {
+ if (!shift_isSmall(n)) {
+ throw new Error(String(n) + " is too large for shifting.");
+ }
+ n = +n;
+ if (n < 0) return this.shiftRight(-n);
+ var result = this;
+ while (n >= powers2Length) {
+ result = result.multiply(highestPower2);
+ n -= powers2Length - 1;
+ }
+ return result.multiply(powersOfTwo[n]);
+ };
+ SmallInteger.prototype.shiftLeft = BigInteger.prototype.shiftLeft;
+
+ BigInteger.prototype.shiftRight = function (n) {
+ var remQuo;
+ if (!shift_isSmall(n)) {
+ throw new Error(String(n) + " is too large for shifting.");
+ }
+ n = +n;
+ if (n < 0) return this.shiftLeft(-n);
+ var result = this;
+ while (n >= powers2Length) {
+ if (result.isZero()) return result;
+ remQuo = divModAny(result, highestPower2);
+ result = remQuo[1].isNegative() ? remQuo[0].prev() : remQuo[0];
+ n -= powers2Length - 1;
+ }
+ remQuo = divModAny(result, powersOfTwo[n]);
+ return remQuo[1].isNegative() ? remQuo[0].prev() : remQuo[0];
+ };
+ SmallInteger.prototype.shiftRight = BigInteger.prototype.shiftRight;
+
+ function bitwise(x, y, fn) {
+ y = parseValue(y);
+ var xSign = x.isNegative(), ySign = y.isNegative();
+ var xRem = xSign ? x.not() : x,
+ yRem = ySign ? y.not() : y;
+ var xBits = [], yBits = [];
+ var xStop = false, yStop = false;
+ while (!xStop || !yStop) {
+ if (xRem.isZero()) { // virtual sign extension for simulating two's complement
+ xStop = true;
+ xBits.push(xSign ? 1 : 0);
+ }
+ else if (xSign) xBits.push(xRem.isEven() ? 1 : 0); // two's complement for negative numbers
+ else xBits.push(xRem.isEven() ? 0 : 1);
+
+ if (yRem.isZero()) {
+ yStop = true;
+ yBits.push(ySign ? 1 : 0);
+ }
+ else if (ySign) yBits.push(yRem.isEven() ? 1 : 0);
+ else yBits.push(yRem.isEven() ? 0 : 1);
+
+ xRem = xRem.over(2);
+ yRem = yRem.over(2);
+ }
+ var result = [];
+ for (var i = 0; i < xBits.length; i++) result.push(fn(xBits[i], yBits[i]));
+ var sum = bigInt(result.pop()).negate().times(bigInt(2).pow(result.length));
+ while (result.length) {
+ sum = sum.add(bigInt(result.pop()).times(bigInt(2).pow(result.length)));
+ }
+ return sum;
+ }
+
+ BigInteger.prototype.not = function () {
+ return this.negate().prev();
+ };
+ SmallInteger.prototype.not = BigInteger.prototype.not;
+
+ BigInteger.prototype.and = function (n) {
+ return bitwise(this, n, function (a, b) { return a & b; });
+ };
+ SmallInteger.prototype.and = BigInteger.prototype.and;
+
+ BigInteger.prototype.or = function (n) {
+ return bitwise(this, n, function (a, b) { return a | b; });
+ };
+ SmallInteger.prototype.or = BigInteger.prototype.or;
+
+ BigInteger.prototype.xor = function (n) {
+ return bitwise(this, n, function (a, b) { return a ^ b; });
+ };
+ SmallInteger.prototype.xor = BigInteger.prototype.xor;
+
+ var LOBMASK_I = 1 << 30, LOBMASK_BI = (BASE & -BASE) * (BASE & -BASE) | LOBMASK_I;
+ function roughLOB(n) { // get lowestOneBit (rough)
+ // SmallInteger: return Min(lowestOneBit(n), 1 << 30)
+ // BigInteger: return Min(lowestOneBit(n), 1 << 14) [BASE=1e7]
+ var v = n.value, x = typeof v === "number" ? v | LOBMASK_I : v[0] + v[1] * BASE | LOBMASK_BI;
+ return x & -x;
+ }
+
+ function max(a, b) {
+ a = parseValue(a);
+ b = parseValue(b);
+ return a.greater(b) ? a : b;
+ }
+ function min(a,b) {
+ a = parseValue(a);
+ b = parseValue(b);
+ return a.lesser(b) ? a : b;
+ }
+ function gcd(a, b) {
+ a = parseValue(a).abs();
+ b = parseValue(b).abs();
+ if (a.equals(b)) return a;
+ if (a.isZero()) return b;
+ if (b.isZero()) return a;
+ var c = CACHE[1], d, t;
+ while (a.isEven() && b.isEven()) {
+ d = Math.min(roughLOB(a), roughLOB(b));
+ a = a.divide(d);
+ b = b.divide(d);
+ c = c.multiply(d);
+ }
+ while (a.isEven()) {
+ a = a.divide(roughLOB(a));
+ }
+ do {
+ while (b.isEven()) {
+ b = b.divide(roughLOB(b));
+ }
+ if (a.greater(b)) {
+ t = b; b = a; a = t;
+ }
+ b = b.subtract(a);
+ } while (!b.isZero());
+ return c.isUnit() ? a : a.multiply(c);
+ }
+ function lcm(a, b) {
+ a = parseValue(a).abs();
+ b = parseValue(b).abs();
+ return a.divide(gcd(a, b)).multiply(b);
+ }
+ function randBetween(a, b) {
+ a = parseValue(a);
+ b = parseValue(b);
+ var low = min(a, b), high = max(a, b);
+ var range = high.subtract(low);
+ if (range.isSmall) return low.add(Math.round(Math.random() * range));
+ var length = range.value.length - 1;
+ var result = [], restricted = true;
+ for (var i = length; i >= 0; i--) {
+ var top = restricted ? range.value[i] : BASE;
+ var digit = truncate(Math.random() * top);
+ result.unshift(digit);
+ if (digit < top) restricted = false;
+ }
+ result = arrayToSmall(result);
+ return low.add(typeof result === "number" ? new SmallInteger(result) : new BigInteger(result, false));
+ }
+ var parseBase = function (text, base) {
+ var val = CACHE[0], pow = CACHE[1],
+ length = text.length;
+ if (2 <= base && base <= 36) {
+ if (length <= LOG_MAX_INT / Math.log(base)) {
+ return new SmallInteger(parseInt(text, base));
+ }
+ }
+ base = parseValue(base);
+ var digits = [];
+ var i;
+ var isNegative = text[0] === "-";
+ for (i = isNegative ? 1 : 0; i < text.length; i++) {
+ var c = text[i].toLowerCase(),
+ charCode = c.charCodeAt(0);
+ if (48 <= charCode && charCode <= 57) digits.push(parseValue(c));
+ else if (97 <= charCode && charCode <= 122) digits.push(parseValue(c.charCodeAt(0) - 87));
+ else if (c === "<") {
+ var start = i;
+ do { i++; } while (text[i] !== ">");
+ digits.push(parseValue(text.slice(start + 1, i)));
+ }
+ else throw new Error(c + " is not a valid character");
+ }
+ digits.reverse();
+ for (i = 0; i < digits.length; i++) {
+ val = val.add(digits[i].times(pow));
+ pow = pow.times(base);
+ }
+ return isNegative ? val.negate() : val;
+ };
+
+ function stringify(digit) {
+ var v = digit.value;
+ if (typeof v === "number") v = [v];
+ if (v.length === 1 && v[0] <= 35) {
+ return "0123456789abcdefghijklmnopqrstuvwxyz".charAt(v[0]);
+ }
+ return "<" + v + ">";
+ }
+ function toBase(n, base) {
+ base = bigInt(base);
+ if (base.isZero()) {
+ if (n.isZero()) return "0";
+ throw new Error("Cannot convert nonzero numbers to base 0.");
+ }
+ if (base.equals(-1)) {
+ if (n.isZero()) return "0";
+ if (n.isNegative()) return new Array(1 - n).join("10");
+ return "1" + new Array(+n).join("01");
+ }
+ var minusSign = "";
+ if (n.isNegative() && base.isPositive()) {
+ minusSign = "-";
+ n = n.abs();
+ }
+ if (base.equals(1)) {
+ if (n.isZero()) return "0";
+ return minusSign + new Array(+n + 1).join(1);
+ }
+ var out = [];
+ var left = n, divmod;
+ while (left.isNegative() || left.compareAbs(base) >= 0) {
+ divmod = left.divmod(base);
+ left = divmod.quotient;
+ var digit = divmod.remainder;
+ if (digit.isNegative()) {
+ digit = base.minus(digit).abs();
+ left = left.next();
+ }
+ out.push(stringify(digit));
+ }
+ out.push(stringify(left));
+ return minusSign + out.reverse().join("");
+ }
+
+ BigInteger.prototype.toString = function (radix) {
+ if (radix === undefined) radix = 10;
+ if (radix !== 10) return toBase(this, radix);
+ var v = this.value, l = v.length, str = String(v[--l]), zeros = "0000000", digit;
+ while (--l >= 0) {
+ digit = String(v[l]);
+ str += zeros.slice(digit.length) + digit;
+ }
+ var sign = this.sign ? "-" : "";
+ return sign + str;
+ };
+ SmallInteger.prototype.toString = function (radix) {
+ if (radix === undefined) radix = 10;
+ if (radix != 10) return toBase(this, radix);
+ return String(this.value);
+ };
+
+ BigInteger.prototype.valueOf = function () {
+ return +this.toString();
+ };
+ BigInteger.prototype.toJSNumber = BigInteger.prototype.valueOf;
+
+ SmallInteger.prototype.valueOf = function () {
+ return this.value;
+ };
+ SmallInteger.prototype.toJSNumber = SmallInteger.prototype.valueOf;
+
+ function parseStringValue(v) {
+ if (isPrecise(+v)) {
+ var x = +v;
+ if (x === truncate(x))
+ return new SmallInteger(x);
+ throw "Invalid integer: " + v;
+ }
+ var sign = v[0] === "-";
+ if (sign) v = v.slice(1);
+ var split = v.split(/e/i);
+ if (split.length > 2) throw new Error("Invalid integer: " + text.join("e"));
+ if (split.length === 2) {
+ var exp = split[1];
+ if (exp[0] === "+") exp = exp.slice(1);
+ exp = +exp;
+ if (exp !== truncate(exp) || !isPrecise(exp)) throw new Error("Invalid integer: " + exp + " is not a valid exponent.");
+ var text = split[0];
+ var decimalPlace = text.indexOf(".");
+ if (decimalPlace >= 0) {
+ exp -= text.length - decimalPlace - 1;
+ text = text.slice(0, decimalPlace) + text.slice(decimalPlace + 1);
+ }
+ if (exp < 0) throw new Error("Cannot include negative exponent part for integers");
+ text += (new Array(exp + 1)).join("0");
+ v = text;
+ }
+ var isValid = /^([0-9][0-9]*)$/.test(v);
+ if (!isValid) throw new Error("Invalid integer: " + v);
+ var r = [], max = v.length, l = LOG_BASE, min = max - l;
+ while (max > 0) {
+ r.push(+v.slice(min, max));
+ min -= l;
+ if (min < 0) min = 0;
+ max -= l;
+ }
+ trim(r);
+ return new BigInteger(r, sign);
+ }
+
+ function parseNumberValue(v) {
+ if (isPrecise(v)) return new SmallInteger(v);
+ return parseStringValue(v.toString());
+ }
+
+ function parseValue(v) {
+ if (typeof v === "number") {
+ return parseNumberValue(v);
+ }
+ if (typeof v === "string") {
+ return parseStringValue(v);
+ }
+ return v;
+ }
+ // Pre-define numbers in range [-999,999]
+ var CACHE = function (v, radix) {
+ if (typeof v === "undefined") return CACHE[0];
+ if (typeof radix !== "undefined") return +radix === 10 ? parseValue(v) : parseBase(v, radix);
+ return parseValue(v);
+ };
+ for (var i = 0; i < 1000; i++) {
+ CACHE[i] = new SmallInteger(i);
+ if (i > 0) CACHE[-i] = new SmallInteger(-i);
+ }
+ // Backwards compatibility
+ CACHE.one = CACHE[1];
+ CACHE.zero = CACHE[0];
+ CACHE.minusOne = CACHE[-1];
+ CACHE.max = max;
+ CACHE.min = min;
+ CACHE.gcd = gcd;
+ CACHE.lcm = lcm;
+ CACHE.isInstance = function (x) { return x instanceof BigInteger || x instanceof SmallInteger; };
+ CACHE.randBetween = randBetween;
+ return CACHE;
+})();
+
+// Node.js check
+if (typeof module !== "undefined" && module.hasOwnProperty("exports")) {
+ module.exports = bigInt;
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/big-integer/BigInteger.min.js
----------------------------------------------------------------------
diff --git a/node_modules/big-integer/BigInteger.min.js b/node_modules/big-integer/BigInteger.min.js
new file mode 100644
index 0000000..53480ea
--- /dev/null
+++ b/node_modules/big-integer/BigInteger.min.js
@@ -0,0 +1 @@
+var bigInt=function(e){"use strict";function o(e,t){this.value=e,this.sign=t,this.isSmall=!1}function u(e){this.value=e,this.sign=e<0,this.isSmall=!0}function a(e){return-r<e&&e<r}function f(e){return e<1e7?[e]:e<1e14?[e%1e7,Math.floor(e/1e7)]:[e%1e7,Math.floor(e/1e7)%1e7,Math.floor(e/1e14)]}function l(e){c(e);var n=e.length;if(n<4&&M(e,i)<0)switch(n){case 0:return 0;case 1:return e[0];case 2:return e[0]+e[1]*t;default:return e[0]+(e[1]+e[2]*t)*t}return e}function c(e){var t=e.length;while(e[--t]===0);e.length=t+1}function h(e){var t=new Array(e),n=-1;while(++n<e)t[n]=0;return t}function p(e){return e>0?Math.floor(e):Math.ceil(e)}function d(e,n){var r=e.length,i=n.length,s=new Array(r),o=0,u=t,a,f;for(f=0;f<i;f++)a=e[f]+n[f]+o,o=a>=u?1:0,s[f]=a-o*u;while(f<r)a=e[f]+o,o=a===u?1:0,s[f++]=a-o*u;return o>0&&s.push(o),s}function v(e,t){return e.length>=t.length?d(e,t):d(t,e)}function m(e,n){var r=e.length,i=new Array(r),s=t,o,u;for(u=0;u<r;u++)o=e[u]-s+n,n=Math.floor(o/s),i[u]=o-n*s,n+=1
;while(n>0)i[u++]=n%s,n=Math.floor(n/s);return i}function g(e,n){var r=e.length,i=n.length,s=new Array(r),o=0,u=t,a,f;for(a=0;a<i;a++)f=e[a]-o-n[a],f<0?(f+=u,o=1):o=0,s[a]=f;for(a=i;a<r;a++){f=e[a]-o;if(!(f<0)){s[a++]=f;break}f+=u,s[a]=f}for(;a<r;a++)s[a]=e[a];return c(s),s}function y(e,t,n){var r,i;return M(e,t)>=0?r=g(e,t):(r=g(t,e),n=!n),r=l(r),typeof r=="number"?(n&&(r=-r),new u(r)):new o(r,n)}function b(e,n,r){var i=e.length,s=new Array(i),a=-n,f=t,c,h;for(c=0;c<i;c++)h=e[c]+a,a=Math.floor(h/f),h%=f,s[c]=h<0?h+f:h;return s=l(s),typeof s=="number"?(r&&(s=-s),new u(s)):new o(s,r)}function w(e,n){var r=e.length,i=n.length,s=r+i,o=h(s),u=t,a,f,l,p,d;for(l=0;l<r;++l){p=e[l];for(var v=0;v<i;++v)d=n[v],a=p*d+o[l+v],f=Math.floor(a/u),o[l+v]=a-f*u,o[l+v+1]+=f}return c(o),o}function E(e,n){var r=e.length,i=new Array(r),s=t,o=0,u,a;for(a=0;a<r;a++)u=e[a]*n+o,o=Math.floor(u/s),i[a]=u-o*s;while(o>0)i[a++]=o%s,o=Math.floor(o/s);return i}function S(e,t){var n=[];while(t-->0)n.push(0);return n
.concat(e)}function x(e,t){var n=Math.max(e.length,t.length);if(n<=30)return w(e,t);n=Math.ceil(n/2);var r=e.slice(n),i=e.slice(0,n),s=t.slice(n),o=t.slice(0,n),u=x(i,o),a=x(r,s),f=x(v(i,r),v(o,s)),l=v(v(u,S(g(g(f,u),a),n)),S(a,2*n));return c(l),l}function T(e,t){return-0.012*e-.012*t+15e-6*e*t>0}function N(e,n,r){return e<t?new o(E(n,e),r):new o(w(n,f(e)),r)}function C(e){var n=e.length,r=h(n+n),i=t,s,o,u,a,f;for(u=0;u<n;u++){a=e[u];for(var l=0;l<n;l++)f=e[l],s=a*f+r[u+l],o=Math.floor(s/i),r[u+l]=s-o*i,r[u+l+1]+=o}return c(r),r}function k(e,n){var r=e.length,i=n.length,s=t,o=h(n.length),u=n[i-1],a=Math.ceil(s/(2*u)),f=E(e,a),c=E(n,a),p,d,v,m,g,y,b;f.length<=r&&f.push(0),c.push(0),u=c[i-1];for(d=r-i;d>=0;d--){p=s-1,f[d+i]!==u&&(p=Math.floor((f[d+i]*s+f[d+i-1])/u)),v=0,m=0,y=c.length;for(g=0;g<y;g++)v+=p*c[g],b=Math.floor(v/s),m+=f[d+g]-(v-b*s),v=b,m<0?(f[d+g]=m+s,m=-1):(f[d+g]=m,m=0);while(m!==0){p-=1,v=0;for(g=0;g<y;g++)v+=f[d+g]-s+c[g],v<0?(f[d+g]=v+s,v=0):(f[d+g]=v,v=1);m+=v}o[d]
=p}return f=A(f,a)[0],[l(o),l(f)]}function L(e,n){var r=e.length,i=n.length,s=[],o=[],u=t,a,f,c,h,p;while(r){o.unshift(e[--r]);if(M(o,n)<0){s.push(0);continue}f=o.length,c=o[f-1]*u+o[f-2],h=n[i-1]*u+n[i-2],f>i&&(c=(c+1)*u),a=Math.ceil(c/h);do{p=E(n,a);if(M(p,o)<=0)break;a--}while(a);s.push(a),o=g(o,p)}return s.reverse(),[l(s),l(o)]}function A(e,n){var r=e.length,i=h(r),s=t,o,u,a,f;a=0;for(o=r-1;o>=0;--o)f=a*s+e[o],u=p(f/n),a=f-u*n,i[o]=u|0;return[i,a|0]}function O(e,n){var r,i=G(n),s=e.value,a=i.value,c;if(a===0)throw new Error("Cannot divide by zero");if(e.isSmall)return i.isSmall?[new u(p(s/a)),new u(s%a)]:[Y[0],e];if(i.isSmall){if(a===1)return[e,Y[0]];if(a==-1)return[e.negate(),Y[0]];var h=Math.abs(a);if(h<t){r=A(s,h),c=l(r[0]);var d=r[1];return e.sign&&(d=-d),typeof c=="number"?(e.sign!==i.sign&&(c=-c),[new u(c),new u(d)]):[new o(c,e.sign!==i.sign),new u(d)]}a=f(h)}var v=M(s,a);if(v===-1)return[Y[0],e];if(v===0)return[Y[e.sign===i.sign?1:-1],Y[0]];s.length+a.length<=200?r=k(s,a)
:r=L(s,a),c=r[0];var m=e.sign!==i.sign,g=r[1],y=e.sign;return typeof c=="number"?(m&&(c=-c),c=new u(c)):c=new o(c,m),typeof g=="number"?(y&&(g=-g),g=new u(g)):g=new o(g,y),[c,g]}function M(e,t){if(e.length!==t.length)return e.length>t.length?1:-1;for(var n=e.length-1;n>=0;n--)if(e[n]!==t[n])return e[n]>t[n]?1:-1;return 0}function _(e){var t=e.abs();if(t.isUnit())return!1;if(t.equals(2)||t.equals(3)||t.equals(5))return!0;if(t.isEven()||t.isDivisibleBy(3)||t.isDivisibleBy(5))return!1;if(t.lesser(25))return!0}function B(e){return(typeof e=="number"||typeof e=="string")&&+Math.abs(e)<=t||e instanceof o&&e.value.length<=1}function j(e,t,n){t=G(t);var r=e.isNegative(),i=t.isNegative(),s=r?e.not():e,o=i?t.not():t,u=[],a=[],f=!1,l=!1;while(!f||!l)s.isZero()?(f=!0,u.push(r?1:0)):r?u.push(s.isEven()?1:0):u.push(s.isEven()?0:1),o.isZero()?(l=!0,a.push(i?1:0)):i?a.push(o.isEven()?1:0):a.push(o.isEven()?0:1),s=s.over(2),o=o.over(2);var c=[];for(var h=0;h<u.length;h++)c.push(n(u[h],a[h]));var p=b
igInt(c.pop()).negate().times(bigInt(2).pow(c.length));while(c.length)p=p.add(bigInt(c.pop()).times(bigInt(2).pow(c.length)));return p}function q(e){var n=e.value,r=typeof n=="number"?n|F:n[0]+n[1]*t|I;return r&-r}function R(e,t){return e=G(e),t=G(t),e.greater(t)?e:t}function U(e,t){return e=G(e),t=G(t),e.lesser(t)?e:t}function z(e,t){e=G(e).abs(),t=G(t).abs();if(e.equals(t))return e;if(e.isZero())return t;if(t.isZero())return e;var n=Y[1],r,i;while(e.isEven()&&t.isEven())r=Math.min(q(e),q(t)),e=e.divide(r),t=t.divide(r),n=n.multiply(r);while(e.isEven())e=e.divide(q(e));do{while(t.isEven())t=t.divide(q(t));e.greater(t)&&(i=t,t=e,e=i),t=t.subtract(e)}while(!t.isZero());return n.isUnit()?e:e.multiply(n)}function W(e,t){return e=G(e).abs(),t=G(t).abs(),e.divide(z(e,t)).multiply(t)}function X(e,n){e=G(e),n=G(n);var r=U(e,n),i=R(e,n),s=i.subtract(r);if(s.isSmall)return r.add(Math.round(Math.random()*s));var a=s.value.length-1,f=[],c=!0;for(var h=a;h>=0;h--){var d=c?s.value[h]:t,v=p(Math.
random()*d);f.unshift(v),v<d&&(c=!1)}return f=l(f),r.add(typeof f=="number"?new u(f):new o(f,!1))}function $(e){var t=e.value;return typeof t=="number"&&(t=[t]),t.length===1&&t[0]<=35?"0123456789abcdefghijklmnopqrstuvwxyz".charAt(t[0]):"<"+t+">"}function J(e,t){t=bigInt(t);if(t.isZero()){if(e.isZero())return"0";throw new Error("Cannot convert nonzero numbers to base 0.")}if(t.equals(-1))return e.isZero()?"0":e.isNegative()?(new Array(1-e)).join("10"):"1"+(new Array(+e)).join("01");var n="";e.isNegative()&&t.isPositive()&&(n="-",e=e.abs());if(t.equals(1))return e.isZero()?"0":n+(new Array(+e+1)).join(1);var r=[],i=e,s;while(i.isNegative()||i.compareAbs(t)>=0){s=i.divmod(t),i=s.quotient;var o=s.remainder;o.isNegative()&&(o=t.minus(o).abs(),i=i.next()),r.push($(o))}return r.push($(i)),n+r.reverse().join("")}function K(e){if(a(+e)){var t=+e;if(t===p(t))return new u(t);throw"Invalid integer: "+e}var r=e[0]==="-";r&&(e=e.slice(1));var i=e.split(/e/i);if(i.length>2)throw new Error("Invalid
integer: "+f.join("e"));if(i.length===2){var s=i[1];s[0]==="+"&&(s=s.slice(1)),s=+s;if(s!==p(s)||!a(s))throw new Error("Invalid integer: "+s+" is not a valid exponent.");var f=i[0],l=f.indexOf(".");l>=0&&(s-=f.length-l-1,f=f.slice(0,l)+f.slice(l+1));if(s<0)throw new Error("Cannot include negative exponent part for integers");f+=(new Array(s+1)).join("0"),e=f}var h=/^([0-9][0-9]*)$/.test(e);if(!h)throw new Error("Invalid integer: "+e);var d=[],v=e.length,m=n,g=v-m;while(v>0)d.push(+e.slice(g,v)),g-=m,g<0&&(g=0),v-=m;return c(d),new o(d,r)}function Q(e){return a(e)?new u(e):K(e.toString())}function G(e){return typeof e=="number"?Q(e):typeof e=="string"?K(e):e}var t=1e7,n=7,r=9007199254740992,i=f(r),s=Math.log(r);o.prototype.add=function(e){var t,n=G(e);if(this.sign!==n.sign)return this.subtract(n.negate());var r=this.value,i=n.value;return n.isSmall?new o(m(r,Math.abs(i)),this.sign):new o(v(r,i),this.sign)},o.prototype.plus=o.prototype.add,u.prototype.add=function(e){var t=G(e),n=thi
s.value;if(n<0!==t.sign)return this.subtract(t.negate());var r=t.value;if(t.isSmall){if(a(n+r))return new u(n+r);r=f(Math.abs(r))}return new o(m(r,Math.abs(n)),n<0)},u.prototype.plus=u.prototype.add,o.prototype.subtract=function(e){var t=G(e);if(this.sign!==t.sign)return this.add(t.negate());var n=this.value,r=t.value;return t.isSmall?b(n,Math.abs(r),this.sign):y(n,r,this.sign)},o.prototype.minus=o.prototype.subtract,u.prototype.subtract=function(e){var t=G(e),n=this.value;if(n<0!==t.sign)return this.add(t.negate());var r=t.value;return t.isSmall?new u(n-r):b(r,Math.abs(n),n>=0)},u.prototype.minus=u.prototype.subtract,o.prototype.negate=function(){return new o(this.value,!this.sign)},u.prototype.negate=function(){var e=this.sign,t=new u(-this.value);return t.sign=!e,t},o.prototype.abs=function(){return new o(this.value,!1)},u.prototype.abs=function(){return new u(Math.abs(this.value))},o.prototype.multiply=function(e){var n,r=G(e),i=this.value,s=r.value,u=this.sign!==r.sign,a;if(r.i
sSmall){if(s===0)return Y[0];if(s===1)return this;if(s===-1)return this.negate();a=Math.abs(s);if(a<t)return new o(E(i,a),u);s=f(a)}return T(i.length,s.length)?new o(x(i,s),u):new o(w(i,s),u)},o.prototype.times=o.prototype.multiply,u.prototype._multiplyBySmall=function(e){return a(e.value*this.value)?new u(e.value*this.value):N(Math.abs(e.value),f(Math.abs(this.value)),this.sign!==e.sign)},o.prototype._multiplyBySmall=function(e){return e.value===0?Y[0]:e.value===1?this:e.value===-1?this.negate():N(Math.abs(e.value),this.value,this.sign!==e.sign)},u.prototype.multiply=function(e){return G(e)._multiplyBySmall(this)},u.prototype.times=u.prototype.multiply,o.prototype.square=function(){return new o(C(this.value),!1)},u.prototype.square=function(){var e=this.value*this.value;return a(e)?new u(e):new o(C(f(Math.abs(this.value))),!1)},o.prototype.divmod=function(e){var t=O(this,e);return{quotient:t[0],remainder:t[1]}},u.prototype.divmod=o.prototype.divmod,o.prototype.divide=function(e){re
turn O(this,e)[0]},u.prototype.over=u.prototype.divide=o.prototype.over=o.prototype.divide,o.prototype.mod=function(e){return O(this,e)[1]},u.prototype.remainder=u.prototype.mod=o.prototype.remainder=o.prototype.mod,o.prototype.pow=function(e){var t=G(e),n=this.value,r=t.value,i,s,o;if(r===0)return Y[1];if(n===0)return Y[0];if(n===1)return Y[1];if(n===-1)return t.isEven()?Y[1]:Y[-1];if(t.sign)return Y[0];if(!t.isSmall)throw new Error("The exponent "+t.toString()+" is too large.");if(this.isSmall&&a(i=Math.pow(n,r)))return new u(p(i));s=this,o=Y[1];for(;;){r&!0&&(o=o.times(s),--r);if(r===0)break;r/=2,s=s.square()}return o},u.prototype.pow=o.prototype.pow,o.prototype.modPow=function(e,t){e=G(e),t=G(t);if(t.isZero())throw new Error("Cannot take modPow with modulus 0");var n=Y[1],r=this.mod(t);while(e.isPositive()){if(r.isZero())return Y[0];e.isOdd()&&(n=n.multiply(r).mod(t)),e=e.divide(2),r=r.square().mod(t)}return n},u.prototype.modPow=o.prototype.modPow,o.prototype.compareAbs=functio
n(e){var t=G(e),n=this.value,r=t.value;return t.isSmall?1:M(n,r)},u.prototype.compareAbs=function(e){var t=G(e),n=Math.abs(this.value),r=t.value;return t.isSmall?(r=Math.abs(r),n===r?0:n>r?1:-1):-1},o.prototype.compare=function(e){if(e===Infinity)return-1;if(e===-Infinity)return 1;var t=G(e),n=this.value,r=t.value;return this.sign!==t.sign?t.sign?1:-1:t.isSmall?this.sign?-1:1:M(n,r)*(this.sign?-1:1)},o.prototype.compareTo=o.prototype.compare,u.prototype.compare=function(e){if(e===Infinity)return-1;if(e===-Infinity)return 1;var t=G(e),n=this.value,r=t.value;return t.isSmall?n==r?0:n>r?1:-1:n<0!==t.sign?n<0?-1:1:n<0?1:-1},u.prototype.compareTo=u.prototype.compare,o.prototype.equals=function(e){return this.compare(e)===0},u.prototype.eq=u.prototype.equals=o.prototype.eq=o.prototype.equals,o.prototype.notEquals=function(e){return this.compare(e)!==0},u.prototype.neq=u.prototype.notEquals=o.prototype.neq=o.prototype.notEquals,o.prototype.greater=function(e){return this.compare(e)>0},u.pr
ototype.gt=u.prototype.greater=o.prototype.gt=o.prototype.greater,o.prototype.lesser=function(e){return this.compare(e)<0},u.prototype.lt=u.prototype.lesser=o.prototype.lt=o.prototype.lesser,o.prototype.greaterOrEquals=function(e){return this.compare(e)>=0},u.prototype.geq=u.prototype.greaterOrEquals=o.prototype.geq=o.prototype.greaterOrEquals,o.prototype.lesserOrEquals=function(e){return this.compare(e)<=0},u.prototype.leq=u.prototype.lesserOrEquals=o.prototype.leq=o.prototype.lesserOrEquals,o.prototype.isEven=function(){return(this.value[0]&1)===0},u.prototype.isEven=function(){return(this.value&1)===0},o.prototype.isOdd=function(){return(this.value[0]&1)===1},u.prototype.isOdd=function(){return(this.value&1)===1},o.prototype.isPositive=function(){return!this.sign},u.prototype.isPositive=function(){return this.value>0},o.prototype.isNegative=function(){return this.sign},u.prototype.isNegative=function(){return this.value<0},o.prototype.isUnit=function(){return!1},u.prototype.isUni
t=function(){return Math.abs(this.value)===1},o.prototype.isZero=function(){return!1},u.prototype.isZero=function(){return this.value===0},o.prototype.isDivisibleBy=function(e){var t=G(e),n=t.value;return n===0?!1:n===1?!0:n===2?this.isEven():this.mod(t).equals(Y[0])},u.prototype.isDivisibleBy=o.prototype.isDivisibleBy,o.prototype.isPrime=function(){var t=_(this);if(t!==e)return t;var n=this.abs(),r=n.prev(),i=[2,3,5,7,11,13,17,19],s=r,o,u,a,f;while(s.isEven())s=s.divide(2);for(a=0;a<i.length;a++){f=bigInt(i[a]).modPow(s,n);if(f.equals(Y[1])||f.equals(r))continue;for(u=!0,o=s;u&&o.lesser(r);o=o.multiply(2))f=f.square().mod(n),f.equals(r)&&(u=!1);if(u)return!1}return!0},u.prototype.isPrime=o.prototype.isPrime,o.prototype.isProbablePrime=function(t){var n=_(this);if(n!==e)return n;var r=this.abs(),i=t===e?5:t;for(var s=0;s<i;s++){var o=bigInt.randBetween(2,r.minus(2));if(!o.modPow(r.prev(),r).isUnit())return!1}return!0},u.prototype.isProbablePrime=o.prototype.isProbablePrime,o.prototy
pe.next=function(){var e=this.value;return this.sign?b(e,1,this.sign):new o(m(e,1),this.sign)},u.prototype.next=function(){var e=this.value;return e+1<r?new u(e+1):new o(i,!1)},o.prototype.prev=function(){var e=this.value;return this.sign?new o(m(e,1),!0):b(e,1,this.sign)},u.prototype.prev=function(){var e=this.value;return e-1>-r?new u(e-1):new o(i,!0)};var D=[1];while(D[D.length-1]<=t)D.push(2*D[D.length-1]);var P=D.length,H=D[P-1];o.prototype.shiftLeft=function(e){if(!B(e))throw new Error(String(e)+" is too large for shifting.");e=+e;if(e<0)return this.shiftRight(-e);var t=this;while(e>=P)t=t.multiply(H),e-=P-1;return t.multiply(D[e])},u.prototype.shiftLeft=o.prototype.shiftLeft,o.prototype.shiftRight=function(e){var t;if(!B(e))throw new Error(String(e)+" is too large for shifting.");e=+e;if(e<0)return this.shiftLeft(-e);var n=this;while(e>=P){if(n.isZero())return n;t=O(n,H),n=t[1].isNegative()?t[0].prev():t[0],e-=P-1}return t=O(n,D[e]),t[1].isNegative()?t[0].prev():t[0]},u.proto
type.shiftRight=o.prototype.shiftRight,o.prototype.not=function(){return this.negate().prev()},u.prototype.not=o.prototype.not,o.prototype.and=function(e){return j(this,e,function(e,t){return e&t})},u.prototype.and=o.prototype.and,o.prototype.or=function(e){return j(this,e,function(e,t){return e|t})},u.prototype.or=o.prototype.or,o.prototype.xor=function(e){return j(this,e,function(e,t){return e^t})},u.prototype.xor=o.prototype.xor;var F=1<<30,I=(t&-t)*(t&-t)|F,V=function(e,t){var n=Y[0],r=Y[1],i=e.length;if(2<=t&&t<=36&&i<=s/Math.log(t))return new u(parseInt(e,t));t=G(t);var o=[],a,f=e[0]==="-";for(a=f?1:0;a<e.length;a++){var l=e[a].toLowerCase(),c=l.charCodeAt(0);if(48<=c&&c<=57)o.push(G(l));else if(97<=c&&c<=122)o.push(G(l.charCodeAt(0)-87));else{if(l!=="<")throw new Error(l+" is not a valid character");var h=a;do a++;while(e[a]!==">");o.push(G(e.slice(h+1,a)))}}o.reverse();for(a=0;a<o.length;a++)n=n.add(o[a].times(r)),r=r.times(t);return f?n.negate():n};o.prototype.toString=func
tion(t){t===e&&(t=10);if(t!==10)return J(this,t);var n=this.value,r=n.length,i=String(n[--r]),s="0000000",o;while(--r>=0)o=String(n[r]),i+=s.slice(o.length)+o;var u=this.sign?"-":"";return u+i},u.prototype.toString=function(t){return t===e&&(t=10),t!=10?J(this,t):String(this.value)},o.prototype.valueOf=function(){return+this.toString()},o.prototype.toJSNumber=o.prototype.valueOf,u.prototype.valueOf=function(){return this.value},u.prototype.toJSNumber=u.prototype.valueOf;var Y=function(e,t){return typeof e=="undefined"?Y[0]:typeof t!="undefined"?+t===10?G(e):V(e,t):G(e)};for(var Z=0;Z<1e3;Z++)Y[Z]=new u(Z),Z>0&&(Y[-Z]=new u(-Z));return Y.one=Y[1],Y.zero=Y[0],Y.minusOne=Y[-1],Y.max=R,Y.min=U,Y.gcd=z,Y.lcm=W,Y.isInstance=function(e){return e instanceof o||e instanceof u},Y.randBetween=X,Y}();typeof module!="undefined"&&module.hasOwnProperty("exports")&&(module.exports=bigInt);
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/big-integer/README.md
----------------------------------------------------------------------
diff --git a/node_modules/big-integer/README.md b/node_modules/big-integer/README.md
new file mode 100644
index 0000000..e608118
--- /dev/null
+++ b/node_modules/big-integer/README.md
@@ -0,0 +1,506 @@
+# BigInteger.js [![Build Status][travis-img]][travis-url] [![Coverage Status][coveralls-img]][coveralls-url] [![Monthly Downloads][downloads-img]][downloads-url]
+
+[travis-url]: https://travis-ci.org/peterolson/BigInteger.js
+[travis-img]: https://travis-ci.org/peterolson/BigInteger.js.svg?branch=master
+[coveralls-url]: https://coveralls.io/github/peterolson/BigInteger.js?branch=master
+[coveralls-img]: https://coveralls.io/repos/peterolson/BigInteger.js/badge.svg?branch=master&service=github
+[downloads-url]: https://www.npmjs.com/package/big-integer
+[downloads-img]: https://img.shields.io/npm/dm/big-integer.svg
+
+**BigInteger.js** is an arbitrary-length integer library for Javascript, allowing arithmetic operations on integers of unlimited size, notwithstanding memory and time limitations.
+
+## Installation
+
+If you are using a browser, you can download [BigInteger.js from GitHub](http://peterolson.github.com/BigInteger.js/BigInteger.min.js) or just hotlink to it:
+
+ <script src="http://peterolson.github.com/BigInteger.js/BigInteger.min.js"></script>
+
+If you are using node, you can install BigInteger with [npm](https://npmjs.org/).
+
+ npm install big-integer
+
+Then you can include it in your code:
+
+ var bigInt = require("big-integer");
+
+
+## Usage
+### `bigInt(number, [base])`
+
+You can create a bigInt by calling the `bigInt` function. You can pass in
+
+ - a string, which it will parse as an bigInt and throw an `"Invalid integer"` error if the parsing fails.
+ - a Javascript number, which it will parse as an bigInt and throw an `"Invalid integer"` error if the parsing fails.
+ - another bigInt.
+ - nothing, and it will return `bigInt.zero`.
+
+ If you provide a second parameter, then it will parse `number` as a number in base `base`. Note that `base` can be any bigInt (even negative or zero). The letters "a-z" and "A-Z" will be interpreted as the numbers 10 to 35. Higher digits can be specified in angle brackets (`<` and `>`).
+
+Examples:
+
+ var zero = bigInt();
+ var ninetyThree = bigInt(93);
+ var largeNumber = bigInt("75643564363473453456342378564387956906736546456235345");
+ var googol = bigInt("1e100");
+ var bigNumber = bigInt(largeNumber);
+
+ var maximumByte = bigInt("FF", 16);
+ var fiftyFiveGoogol = bigInt("<55>0", googol);
+
+Note that Javascript numbers larger than `9007199254740992` and smaller than `-9007199254740992` are not precisely represented numbers and will not produce exact results. If you are dealing with numbers outside that range, it is better to pass in strings.
+
+### Method Chaining
+
+Note that bigInt operations return bigInts, which allows you to chain methods, for example:
+
+ var salary = bigInt(dollarsPerHour).times(hoursWorked).plus(randomBonuses)
+
+### Constants
+
+There are three named constants already stored that you do not have to construct with the `bigInt` function yourself:
+
+ - `bigInt.one`, equivalent to `bigInt(1)`
+ - `bigInt.zero`, equivalent to `bigInt(0)`
+ - `bigInt.minusOne`, equivalent to `bigInt(-1)`
+
+The numbers from -999 to 999 are also already prestored and can be accessed using `bigInt[index]`, for example:
+
+ - `bigInt[-999]`, equivalent to `bigInt(-999)`
+ - `bigInt[256]`, equivalent to `bigInt(256)`
+
+### Methods
+
+#### `abs()`
+
+Returns the absolute value of a bigInt.
+
+ - `bigInt(-45).abs()` => `45`
+ - `bigInt(45).abs()` => `45`
+
+#### `add(number)`
+
+Performs addition.
+
+ - `bigInt(5).add(7)` => `12`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Addition)
+
+#### `and(number)`
+
+Performs the bitwise AND operation. The operands are treated as if they were represented using [two's complement representation](http://en.wikipedia.org/wiki/Two%27s_complement).
+
+ - `bigInt(6).and(3)` => `2`
+ - `bigInt(6).and(-3)` => `4`
+
+#### `compare(number)`
+
+Performs a comparison between two numbers. If the numbers are equal, it returns `0`. If the first number is greater, it returns `1`. If the first number is lesser, it returns `-1`.
+
+ - `bigInt(5).compare(5)` => `0`
+ - `bigInt(5).compare(4)` => `1`
+ - `bigInt(4).compare(5)` => `-1`
+
+#### `compareAbs(number)`
+
+Performs a comparison between the absolute value of two numbers.
+
+ - `bigInt(5).compareAbs(-5)` => `0`
+ - `bigInt(5).compareAbs(4)` => `1`
+ - `bigInt(4).compareAbs(-5)` => `-1`
+
+#### `compareTo(number)`
+
+Alias for the `compare` method.
+
+#### `divide(number)`
+
+Performs integer division, disregarding the remainder.
+
+ - `bigInt(59).divide(5)` => `11`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Division)
+
+#### `divmod(number)`
+
+Performs division and returns an object with two properties: `quotient` and `remainder`. The sign of the remainder will match the sign of the dividend.
+
+ - `bigInt(59).divmod(5)` => `{quotient: bigInt(11), remainder: bigInt(4) }`
+ - `bigInt(-5).divmod(2)` => `{quotient: bigInt(-2), remainder: bigInt(-1) }`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Division)
+
+#### `eq(number)`
+
+Alias for the `equals` method.
+
+#### `equals(number)`
+
+Checks if two numbers are equal.
+
+ - `bigInt(5).equals(5)` => `true`
+ - `bigInt(4).equals(7)` => `false`
+
+#### `geq(number)`
+
+Alias for the `greaterOrEquals` method.
+
+
+#### `greater(number)`
+
+Checks if the first number is greater than the second.
+
+ - `bigInt(5).greater(6)` => `false`
+ - `bigInt(5).greater(5)` => `false`
+ - `bigInt(5).greater(4)` => `true`
+
+#### `greaterOrEquals(number)`
+
+Checks if the first number is greater than or equal to the second.
+
+ - `bigInt(5).greaterOrEquals(6)` => `false`
+ - `bigInt(5).greaterOrEquals(5)` => `true`
+ - `bigInt(5).greaterOrEquals(4)` => `true`
+
+#### `gt(number)`
+
+Alias for the `greater` method.
+
+#### `isDivisibleBy(number)`
+
+Returns `true` if the first number is divisible by the second number, `false` otherwise.
+
+ - `bigInt(999).isDivisibleBy(333)` => `true`
+ - `bigInt(99).isDivisibleBy(5)` => `false`
+
+#### `isEven()`
+
+Returns `true` if the number is even, `false` otherwise.
+
+ - `bigInt(6).isEven()` => `true`
+ - `bigInt(3).isEven()` => `false`
+
+#### `isNegative()`
+
+Returns `true` if the number is negative, `false` otherwise.
+Returns `false` for `0` and `-0`.
+
+ - `bigInt(-23).isNegative()` => `true`
+ - `bigInt(50).isNegative()` => `false`
+
+#### `isOdd()`
+
+Returns `true` if the number is odd, `false` otherwise.
+
+ - `bigInt(13).isOdd()` => `true`
+ - `bigInt(40).isOdd()` => `false`
+
+#### `isPositive()`
+
+Return `true` if the number is positive, `false` otherwise.
+Returns `false` for `0` and `-0`.
+
+ - `bigInt(54).isPositive()` => `true`
+ - `bigInt(-1).isPositive()` => `false`
+
+#### `isPrime()`
+
+Returns `true` if the number is prime, `false` otherwise.
+
+ - `bigInt(5).isPrime()` => `true`
+ - `bigInt(6).isPrime()` => `false`
+
+#### `isProbablePrime([iterations])`
+
+Returns `true` if the number is very likely to be positive, `false` otherwise.
+Argument is optional and determines the amount of iterations of the test (default: `5`). The more iterations, the lower chance of getting a false positive.
+This uses the [Fermat primality test](https://en.wikipedia.org/wiki/Fermat_primality_test).
+
+ - `bigInt(5).isProbablePrime()` => `true`
+ - `bigInt(49).isProbablePrime()` => `false`
+ - `bigInt(1729).isProbablePrime(50)` => `false`
+
+Note that this function is not deterministic, since it relies on random sampling of factors, so the result for some numbers is not always the same. [Carmichael numbers](https://en.wikipedia.org/wiki/Carmichael_number) are particularly prone to give unreliable results.
+
+For example, `bigInt(1729).isProbablePrime()` returns `false` about 76% of the time and `true` about 24% of the time. The correct result is `false`.
+
+#### `isUnit()`
+
+Returns `true` if the number is `1` or `-1`, `false` otherwise.
+
+ - `bigInt.one.isUnit()` => `true`
+ - `bigInt.minusOne.isUnit()` => `true`
+ - `bigInt(5).isUnit()` => `false`
+
+#### `isZero()`
+
+Return `true` if the number is `0` or `-0`, `false` otherwise.
+
+ - `bigInt.zero.isZero()` => `true`
+ - `bigInt("-0").isZero()` => `true`
+ - `bigInt(50).isZero()` => `false`
+
+#### `leq(number)`
+
+Alias for the `lesserOrEquals` method.
+
+#### `lesser(number)`
+
+Checks if the first number is lesser than the second.
+
+ - `bigInt(5).lesser(6)` => `true`
+ - `bigInt(5).lesser(5)` => `false`
+ - `bigInt(5).lesser(4)` => `false`
+
+#### `lesserOrEquals(number)`
+
+Checks if the first number is less than or equal to the second.
+
+ - `bigInt(5).lesserOrEquals(6)` => `true`
+ - `bigInt(5).lesserOrEquals(5)` => `true`
+ - `bigInt(5).lesserOrEquals(4)` => `false`
+
+#### `lt(number)`
+
+Alias for the `lesser` method.
+
+#### `minus(number)`
+
+Alias for the `subtract` method.
+
+ - `bigInt(3).minus(5)` => `-2`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Subtraction)
+
+#### `mod(number)`
+
+Performs division and returns the remainder, disregarding the quotient. The sign of the remainder will match the sign of the dividend.
+
+ - `bigInt(59).mod(5)` => `4`
+ - `bigInt(-5).mod(2)` => `-1`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Division)
+
+#### `modPow(exp, mod)`
+
+Takes the number to the power `exp` modulo `mod`.
+
+ - `bigInt(10).modPow(3, 30)` => `10`
+
+#### `multiply(number)`
+
+Performs multiplication.
+
+ - `bigInt(111).multiply(111)` => `12321`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Multiplication)
+
+#### `neq(number)`
+
+Alias for the `notEquals` method.
+
+#### `next()`
+
+Adds one to the number.
+
+ - `bigInt(6).next()` => `7`
+
+#### `not()`
+
+Performs the bitwise NOT operation. The operands are treated as if they were represented using [two's complement representation](http://en.wikipedia.org/wiki/Two%27s_complement).
+
+ - `bigInt(10).not()` => `-11`
+ - `bigInt(0).not()` => `-1`
+
+#### `notEquals(number)`
+
+Checks if two numbers are not equal.
+
+ - `bigInt(5).notEquals(5)` => `false`
+ - `bigInt(4).notEquals(7)` => `true`
+
+#### `or(number)`
+
+Performs the bitwise OR operation. The operands are treated as if they were represented using [two's complement representation](http://en.wikipedia.org/wiki/Two%27s_complement).
+
+ - `bigInt(13).or(10)` => `15`
+ - `bigInt(13).or(-8)` => `-3`
+
+#### `over(number)`
+
+Alias for the `divide` method.
+
+ - `bigInt(59).over(5)` => `11`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Division)
+
+#### `plus(number)`
+
+Alias for the `add` method.
+
+ - `bigInt(5).plus(7)` => `12`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Addition)
+
+#### `pow(number)`
+
+Performs exponentiation. If the exponent is less than `0`, `pow` returns `0`. `bigInt.zero.pow(0)` returns `1`.
+
+ - `bigInt(16).pow(16)` => `18446744073709551616`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Exponentiation)
+
+#### `prev(number)`
+
+Subtracts one from the number.
+
+ - `bigInt(6).prev()` => `5`
+
+#### `remainder(number)`
+
+Alias for the `mod` method.
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Division)
+
+#### `shiftLeft(n)`
+
+Shifts the number left by `n` places in its binary representation. If a negative number is provided, it will shift right. Throws an error if `n` is outside of the range `[-9007199254740992, 9007199254740992]`.
+
+ - `bigInt(8).shiftLeft(2)` => `32`
+ - `bigInt(8).shiftLeft(-2)` => `2`
+
+#### `shiftRight(n)`
+
+Shifts the number right by `n` places in its binary representation. If a negative number is provided, it will shift left. Throws an error if `n` is outside of the range `[-9007199254740992, 9007199254740992]`.
+
+ - `bigInt(8).shiftRight(2)` => `2`
+ - `bigInt(8).shiftRight(-2)` => `32`
+
+#### `square()`
+
+Squares the number
+
+ - `bigInt(3).square()` => `9`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Squaring)
+
+#### `subtract(number)`
+
+Performs subtraction.
+
+ - `bigInt(3).subtract(5)` => `-2`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Subtraction)
+
+#### `times(number)`
+
+Alias for the `multiply` method.
+
+ - `bigInt(111).times(111)` => `12321`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#Multiplication)
+
+#### `toJSNumber()`
+
+Converts a bigInt into a native Javascript number. Loses precision for numbers outside the range `[-9007199254740992, 9007199254740992]`.
+
+ - `bigInt("18446744073709551616").toJSNumber()` => `18446744073709552000`
+
+#### `xor(number)`
+
+Performs the bitwise XOR operation. The operands are treated as if they were represented using [two's complement representation](http://en.wikipedia.org/wiki/Two%27s_complement).
+
+ - `bigInt(12).xor(5)` => `9`
+ - `bigInt(12).xor(-5)` => `-9`
+
+### Static Methods
+
+#### `gcd(a, b)`
+
+Finds the greatest common denominator of `a` and `b`.
+
+ - `bigInt.gcd(42,56)` => `14`
+
+#### `isInstance(x)`
+
+Returns `true` if `x` is a BigInteger, `false` otherwise.
+
+ - `bigInt.isInstance(bigInt(14))` => `true`
+ - `bigInt.isInstance(14)` => `false`
+
+#### `lcm(a,b)`
+
+Finds the least common multiple of `a` and `b`.
+
+ - `bigInt.lcm(21, 6)` => `42`
+
+#### `max(a,b)`
+
+Returns the largest of `a` and `b`.
+
+ - `bigInt.max(77, 432)` => `432`
+
+#### `min(a,b)`
+
+Returns the smallest of `a` and `b`.
+
+ - `bigInt.min(77, 432)` => `77`
+
+#### `randBetween(min, max)`
+
+Returns a random number between `min` and `max`.
+
+ - `bigInt.randBetween("-1e100", "1e100")` => (for example) `8494907165436643479673097939554427056789510374838494147955756275846226209006506706784609314471378745`
+
+
+### Override Methods
+
+#### `toString(radix = 10)`
+
+Converts a bigInt to a string. There is an optional radix parameter (which defaults to 10) that converts the number to the given radix. Digits in the range `10-35` will use the letters `a-z`.
+
+ - `bigInt("1e9").toString()` => `"1000000000"`
+ - `bigInt("1e9").toString(16)` => `"3b9aca00"`
+
+**Note that arithmetical operators will trigger the `valueOf` function rather than the `toString` function.** When converting a bigInteger to a string, you should use the `toString` method or the `String` function instead of adding the empty string.
+
+ - `bigInt("999999999999999999").toString()` => `"999999999999999999"`
+ - `String(bigInt("999999999999999999"))` => `"999999999999999999"`
+ - `bigInt("999999999999999999") + ""` => `1000000000000000000`
+
+Bases larger than 36 are supported. If a digit is greater than or equal to 36, it will be enclosed in angle brackets.
+
+ - `bigInt(567890).toString(100)` => `"<56><78><90>"`
+
+Negative bases are also supported.
+
+ - `bigInt(12345).toString(-10)` => `"28465"`
+
+Base 1 and base -1 are also supported.
+
+ - `bigInt(-15).toString(1)` => `"-111111111111111"`
+ - `bigInt(-15).toString(-1)` => `"101010101010101010101010101010"`
+
+Base 0 is only allowed for the number zero.
+
+ - `bigInt(0).toString(0)` => `0`
+ - `bigInt(1).toString(0)` => `Error: Cannot convert nonzero numbers to base 0.`
+
+[View benchmarks for this method](http://peterolson.github.io/BigInteger.js/benchmark/#toString)
+
+#### `valueOf()`
+
+Converts a bigInt to a native Javascript number. This override allows you to use native arithmetic operators without explicit conversion:
+
+ - `bigInt("100") + bigInt("200") === 300; //true`
+
+## Contributors
+
+To contribute, just fork the project, make some changes, and submit a pull request. Please verify that the unit tests pass before submitting.
+
+The unit tests are contained in the `spec/spec.js` file. You can run them locally by opening the `spec/SpecRunner.html` or file or running `npm test`. You can also [run the tests online from GitHub](http://peterolson.github.io/BigInteger.js/spec/SpecRunner.html).
+
+There are performance benchmarks that can be viewed from the `benchmarks/index.html` page. You can [run them online from GitHub](http://peterolson.github.io/BigInteger.js/benchmark/).
+
+## License
+
+This project is public domain. For more details, read about the [Unlicense](http://unlicense.org/).
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/big-integer/package.json
----------------------------------------------------------------------
diff --git a/node_modules/big-integer/package.json b/node_modules/big-integer/package.json
new file mode 100644
index 0000000..0ca677f
--- /dev/null
+++ b/node_modules/big-integer/package.json
@@ -0,0 +1,100 @@
+{
+ "_args": [
+ [
+ "big-integer@^1.6.7",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/bplist-parser"
+ ]
+ ],
+ "_from": "big-integer@>=1.6.7 <2.0.0",
+ "_id": "big-integer@1.6.12",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/big-integer",
+ "_nodeVersion": "0.12.3",
+ "_npmOperationalInternal": {
+ "host": "packages-6-west.internal.npmjs.com",
+ "tmp": "tmp/big-integer-1.6.12.tgz_1455702804335_0.11810904298909009"
+ },
+ "_npmUser": {
+ "email": "peter.e.c.olson+npm@gmail.com",
+ "name": "peterolson"
+ },
+ "_npmVersion": "2.9.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "big-integer",
+ "raw": "big-integer@^1.6.7",
+ "rawSpec": "^1.6.7",
+ "scope": null,
+ "spec": ">=1.6.7 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/bplist-parser"
+ ],
+ "_resolved": "http://registry.npmjs.org/big-integer/-/big-integer-1.6.12.tgz",
+ "_shasum": "39afcddafcd5c4480864efb757337d508938bb26",
+ "_shrinkwrap": null,
+ "_spec": "big-integer@^1.6.7",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/bplist-parser",
+ "author": {
+ "email": "peter.e.c.olson+npm@gmail.com",
+ "name": "Peter Olson"
+ },
+ "bin": {},
+ "bugs": {
+ "url": "https://github.com/peterolson/BigInteger.js/issues"
+ },
+ "contributors": [],
+ "dependencies": {},
+ "description": "An arbitrary length integer library for Javascript",
+ "devDependencies": {
+ "coveralls": "^2.11.4",
+ "jasmine": "2.1.x",
+ "jasmine-core": "^2.3.4",
+ "karma": "^0.13.3",
+ "karma-coverage": "^0.4.2",
+ "karma-jasmine": "^0.3.6",
+ "karma-phantomjs-launcher": "~0.1"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "39afcddafcd5c4480864efb757337d508938bb26",
+ "tarball": "http://registry.npmjs.org/big-integer/-/big-integer-1.6.12.tgz"
+ },
+ "engines": {
+ "node": ">=0.6"
+ },
+ "gitHead": "56f449108e31542f939e701f1fe562a46e6c1fab",
+ "homepage": "https://github.com/peterolson/BigInteger.js#readme",
+ "keywords": [
+ "arbitrary",
+ "arithmetic",
+ "big",
+ "bigint",
+ "biginteger",
+ "bignum",
+ "integer",
+ "math",
+ "precision"
+ ],
+ "license": "Unlicense",
+ "main": "./BigInteger",
+ "maintainers": [
+ {
+ "name": "peterolson",
+ "email": "peter.e.c.olson+npm@gmail.com"
+ }
+ ],
+ "name": "big-integer",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+ssh://git@github.com/peterolson/BigInteger.js.git"
+ },
+ "scripts": {
+ "test": "karma start my.conf.js"
+ },
+ "version": "1.6.12"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/.npmignore b/node_modules/bplist-creator/.npmignore
new file mode 100644
index 0000000..a9b46ea
--- /dev/null
+++ b/node_modules/bplist-creator/.npmignore
@@ -0,0 +1,8 @@
+/build/*
+node_modules
+*.node
+*.sh
+*.swp
+.lock*
+npm-debug.log
+.idea
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/README.md
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/README.md b/node_modules/bplist-creator/README.md
new file mode 100644
index 0000000..bf3080b
--- /dev/null
+++ b/node_modules/bplist-creator/README.md
@@ -0,0 +1,64 @@
+bplist-parser
+=============
+
+Binary Mac OS X Plist (property list) creator.
+
+## Installation
+
+```bash
+$ npm install bplist-creator
+```
+
+## Quick Examples
+
+```javascript
+var bplist = require('bplist-creator');
+
+var buffer = bplist({
+ key1: [1, 2, 3]
+});
+```
+
+## Real/Double/Float handling
+
+Javascript don't have different types for `1` and `1.0`. This package
+will automatically store numbers as the appropriate type, but can't
+detect floats that is also integers.
+
+If you need to force a value to be written with the `real` type pass
+an instance of `Real`.
+
+```javascript
+var buffer = bplist({
+ backgroundRed: new bplist.Real(1),
+ backgroundGreen: new bplist.Real(0),
+ backgroundBlue: new bplist.Real(0)
+});
+```
+
+In `xml` the corresponding tags is `<integer>` and `<real>`.
+
+## License
+
+(The MIT License)
+
+Copyright (c) 2012 Near Infinity Corporation
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[48/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/bplistCreator.js
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/bplistCreator.js b/node_modules/bplist-creator/bplistCreator.js
new file mode 100644
index 0000000..b480826
--- /dev/null
+++ b/node_modules/bplist-creator/bplistCreator.js
@@ -0,0 +1,404 @@
+'use strict';
+
+// adapted from http://code.google.com/p/plist/source/browse/trunk/src/main/java/com/dd/plist/BinaryPropertyListWriter.java
+
+var streamBuffers = require("stream-buffers");
+
+var debug = false;
+
+function Real(value) {
+ this.value = value;
+}
+
+module.exports = function(dicts) {
+ var buffer = new streamBuffers.WritableStreamBuffer();
+ buffer.write(new Buffer("bplist00"));
+
+ if (debug) {
+ console.log('create', require('util').inspect(dicts, false, 10));
+ }
+
+ if (dicts instanceof Array && dicts.length === 1) {
+ dicts = dicts[0];
+ }
+
+ var entries = toEntries(dicts);
+ if (debug) {
+ console.log('entries', entries);
+ }
+ var idSizeInBytes = computeIdSizeInBytes(entries.length);
+ var offsets = [];
+ var offsetSizeInBytes;
+ var offsetTableOffset;
+
+ updateEntryIds();
+
+ entries.forEach(function(entry, entryIdx) {
+ offsets[entryIdx] = buffer.size();
+ if (!entry) {
+ buffer.write(0x00);
+ } else {
+ write(entry);
+ }
+ });
+
+ writeOffsetTable();
+ writeTrailer();
+ return buffer.getContents();
+
+ function updateEntryIds() {
+ var strings = {};
+ var entryId = 0;
+ entries.forEach(function(entry) {
+ if (entry.id) {
+ return;
+ }
+ if (entry.type === 'string') {
+ if (!entry.bplistOverride && strings.hasOwnProperty(entry.value)) {
+ entry.type = 'stringref';
+ entry.id = strings[entry.value];
+ } else {
+ strings[entry.value] = entry.id = entryId++;
+ }
+ } else {
+ entry.id = entryId++;
+ }
+ });
+
+ entries = entries.filter(function(entry) {
+ return (entry.type !== 'stringref');
+ });
+ }
+
+ function writeTrailer() {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeTrailer');
+ }
+ // 6 null bytes
+ buffer.write(new Buffer([0, 0, 0, 0, 0, 0]));
+
+ // size of an offset
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeTrailer(offsetSizeInBytes):', offsetSizeInBytes);
+ }
+ writeByte(offsetSizeInBytes);
+
+ // size of a ref
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeTrailer(offsetSizeInBytes):', idSizeInBytes);
+ }
+ writeByte(idSizeInBytes);
+
+ // number of objects
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeTrailer(number of objects):', entries.length);
+ }
+ writeLong(entries.length);
+
+ // top object
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeTrailer(top object)');
+ }
+ writeLong(0);
+
+ // offset table offset
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeTrailer(offset table offset):', offsetTableOffset);
+ }
+ writeLong(offsetTableOffset);
+ }
+
+ function writeOffsetTable() {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeOffsetTable');
+ }
+ offsetTableOffset = buffer.size();
+ offsetSizeInBytes = computeOffsetSizeInBytes(offsetTableOffset);
+ offsets.forEach(function(offset) {
+ writeBytes(offset, offsetSizeInBytes);
+ });
+ }
+
+ function write(entry) {
+ switch (entry.type) {
+ case 'dict':
+ writeDict(entry);
+ break;
+ case 'number':
+ case 'double':
+ writeNumber(entry);
+ break;
+ case 'array':
+ writeArray(entry);
+ break;
+ case 'boolean':
+ writeBoolean(entry);
+ break;
+ case 'string':
+ case 'string-utf16':
+ writeString(entry);
+ break;
+ case 'data':
+ writeData(entry);
+ break;
+ default:
+ throw new Error("unhandled entry type: " + entry.type);
+ }
+ }
+
+ function writeDict(entry) {
+ if (debug) {
+ var keysStr = entry.entryKeys.map(function(k) {return k.id;});
+ var valsStr = entry.entryValues.map(function(k) {return k.id;});
+ console.log('0x' + buffer.size().toString(16), 'writeDict', '(id: ' + entry.id + ')', '(keys: ' + keysStr + ')', '(values: ' + valsStr + ')');
+ }
+ writeIntHeader(0xD, entry.entryKeys.length);
+ entry.entryKeys.forEach(function(entry) {
+ writeID(entry.id);
+ });
+ entry.entryValues.forEach(function(entry) {
+ writeID(entry.id);
+ });
+ }
+
+ function writeNumber(entry) {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeNumber', entry.value, ' (type: ' + entry.type + ')', '(id: ' + entry.id + ')');
+ }
+
+ if (entry.type !== 'double' && parseFloat(entry.value.toFixed()) == entry.value) {
+ if (entry.value < 0) {
+ writeByte(0x13);
+ writeBytes(entry.value, 8);
+ } else if (entry.value <= 0xff) {
+ writeByte(0x10);
+ writeBytes(entry.value, 1);
+ } else if (entry.value <= 0xffff) {
+ writeByte(0x11);
+ writeBytes(entry.value, 2);
+ } else if (entry.value <= 0xffffffff) {
+ writeByte(0x12);
+ writeBytes(entry.value, 4);
+ } else {
+ writeByte(0x13);
+ writeBytes(entry.value, 8);
+ }
+ } else {
+ writeByte(0x23);
+ writeDouble(entry.value);
+ }
+ }
+
+ function writeArray(entry) {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeArray (length: ' + entry.entries.length + ')', '(id: ' + entry.id + ')');
+ }
+ writeIntHeader(0xA, entry.entries.length);
+ entry.entries.forEach(function(e) {
+ writeID(e.id);
+ });
+ }
+
+ function writeBoolean(entry) {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeBoolean', entry.value, '(id: ' + entry.id + ')');
+ }
+ writeByte(entry.value ? 0x09 : 0x08);
+ }
+
+ function writeString(entry) {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeString', entry.value, '(id: ' + entry.id + ')');
+ }
+ if (entry.type === 'string-utf16') {
+ var utf16 = new Buffer(entry.value, 'ucs2');
+ writeIntHeader(0x6, utf16.length / 2);
+ // needs to be big endian so swap the bytes
+ for (var i = 0; i < utf16.length; i += 2) {
+ var t = utf16[i + 0];
+ utf16[i + 0] = utf16[i + 1];
+ utf16[i + 1] = t;
+ }
+ buffer.write(utf16);
+ } else {
+ var utf8 = new Buffer(entry.value, 'utf8');
+ writeIntHeader(0x5, utf8.length);
+ buffer.write(utf8);
+ }
+ }
+
+ function writeData(entry) {
+ if (debug) {
+ console.log('0x' + buffer.size().toString(16), 'writeData', entry.value, '(id: ' + entry.id + ')');
+ }
+ writeIntHeader(0x4, entry.value.length);
+ buffer.write(entry.value);
+ }
+
+ function writeLong(l) {
+ writeBytes(l, 8);
+ }
+
+ function writeByte(b) {
+ buffer.write(new Buffer([b]));
+ }
+
+ function writeDouble(v) {
+ var buf = new Buffer(8);
+ buf.writeDoubleBE(v, 0);
+ buffer.write(buf);
+ }
+
+ function writeIntHeader(kind, value) {
+ if (value < 15) {
+ writeByte((kind << 4) + value);
+ } else if (value < 256) {
+ writeByte((kind << 4) + 15);
+ writeByte(0x10);
+ writeBytes(value, 1);
+ } else if (value < 65536) {
+ writeByte((kind << 4) + 15);
+ writeByte(0x11);
+ writeBytes(value, 2);
+ } else {
+ writeByte((kind << 4) + 15);
+ writeByte(0x12);
+ writeBytes(value, 4);
+ }
+ }
+
+ function writeID(id) {
+ writeBytes(id, idSizeInBytes);
+ }
+
+ function writeBytes(value, bytes) {
+ // write low-order bytes big-endian style
+ var buf = new Buffer(bytes);
+ var z = 0;
+ // javascript doesn't handle large numbers
+ while (bytes > 4) {
+ buf[z++] = 0;
+ bytes--;
+ }
+ for (var i = bytes - 1; i >= 0; i--) {
+ buf[z++] = value >> (8 * i);
+ }
+ buffer.write(buf);
+ }
+};
+
+function toEntries(dicts) {
+ if (dicts.bplistOverride) {
+ return [dicts];
+ }
+
+ if (dicts instanceof Array) {
+ return toEntriesArray(dicts);
+ } else if (dicts instanceof Buffer) {
+ return [
+ {
+ type: 'data',
+ value: dicts
+ }
+ ];
+ } else if (dicts instanceof Real) {
+ return [
+ {
+ type: 'double',
+ value: dicts.value
+ }
+ ];
+ } else if (typeof(dicts) === 'object') {
+ return toEntriesObject(dicts);
+ } else if (typeof(dicts) === 'string') {
+ return [
+ {
+ type: 'string',
+ value: dicts
+ }
+ ];
+ } else if (typeof(dicts) === 'number') {
+ return [
+ {
+ type: 'number',
+ value: dicts
+ }
+ ];
+ } else if (typeof(dicts) === 'boolean') {
+ return [
+ {
+ type: 'boolean',
+ value: dicts
+ }
+ ];
+ } else {
+ throw new Error('unhandled entry: ' + dicts);
+ }
+}
+
+function toEntriesArray(arr) {
+ if (debug) {
+ console.log('toEntriesArray');
+ }
+ var results = [
+ {
+ type: 'array',
+ entries: []
+ }
+ ];
+ arr.forEach(function(v) {
+ var entry = toEntries(v);
+ results[0].entries.push(entry[0]);
+ results = results.concat(entry);
+ });
+ return results;
+}
+
+function toEntriesObject(dict) {
+ if (debug) {
+ console.log('toEntriesObject');
+ }
+ var results = [
+ {
+ type: 'dict',
+ entryKeys: [],
+ entryValues: []
+ }
+ ];
+ Object.keys(dict).forEach(function(key) {
+ var entryKey = toEntries(key);
+ results[0].entryKeys.push(entryKey[0]);
+ results = results.concat(entryKey[0]);
+ });
+ Object.keys(dict).forEach(function(key) {
+ var entryValue = toEntries(dict[key]);
+ results[0].entryValues.push(entryValue[0]);
+ results = results.concat(entryValue);
+ });
+ return results;
+}
+
+function computeOffsetSizeInBytes(maxOffset) {
+ if (maxOffset < 256) {
+ return 1;
+ }
+ if (maxOffset < 65536) {
+ return 2;
+ }
+ if (maxOffset < 4294967296) {
+ return 4;
+ }
+ return 8;
+}
+
+function computeIdSizeInBytes(numberOfIds) {
+ if (numberOfIds < 256) {
+ return 1;
+ }
+ if (numberOfIds < 65536) {
+ return 2;
+ }
+ return 4;
+}
+
+module.exports.Real = Real;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/package.json
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/package.json b/node_modules/bplist-creator/package.json
new file mode 100644
index 0000000..3d8d90b
--- /dev/null
+++ b/node_modules/bplist-creator/package.json
@@ -0,0 +1,79 @@
+{
+ "_args": [
+ [
+ "bplist-creator@0.0.4",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/simple-plist"
+ ]
+ ],
+ "_from": "bplist-creator@0.0.4",
+ "_id": "bplist-creator@0.0.4",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/bplist-creator",
+ "_npmUser": {
+ "email": "joe@fernsroth.com",
+ "name": "joeferner"
+ },
+ "_npmVersion": "1.4.10",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "bplist-creator",
+ "raw": "bplist-creator@0.0.4",
+ "rawSpec": "0.0.4",
+ "scope": null,
+ "spec": "0.0.4",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/simple-plist"
+ ],
+ "_resolved": "http://registry.npmjs.org/bplist-creator/-/bplist-creator-0.0.4.tgz",
+ "_shasum": "4ac0496782e127a85c1d2026a4f5eb22a7aff991",
+ "_shrinkwrap": null,
+ "_spec": "bplist-creator@0.0.4",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/simple-plist",
+ "author": {
+ "name": "https://github.com/nearinfinity/node-bplist-parser.git"
+ },
+ "bugs": {
+ "url": "https://github.com/nearinfinity/node-bplist-creator/issues"
+ },
+ "dependencies": {
+ "stream-buffers": "~0.2.3"
+ },
+ "description": "Binary Mac OS X Plist (property list) creator.",
+ "devDependencies": {
+ "bplist-parser": "0.0.4",
+ "nodeunit": "0.7.4"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "4ac0496782e127a85c1d2026a4f5eb22a7aff991",
+ "tarball": "http://registry.npmjs.org/bplist-creator/-/bplist-creator-0.0.4.tgz"
+ },
+ "homepage": "https://github.com/nearinfinity/node-bplist-creator",
+ "keywords": [
+ "bplist",
+ "creator",
+ "plist"
+ ],
+ "license": "MIT",
+ "main": "bplistCreator.js",
+ "maintainers": [
+ {
+ "name": "joeferner",
+ "email": "joe@fernsroth.com"
+ }
+ ],
+ "name": "bplist-creator",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/nearinfinity/node-bplist-creator.git"
+ },
+ "scripts": {
+ "test": "./node_modules/nodeunit/bin/nodeunit test"
+ },
+ "version": "0.0.4"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/airplay.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/airplay.bplist b/node_modules/bplist-creator/test/airplay.bplist
new file mode 100644
index 0000000..931adea
Binary files /dev/null and b/node_modules/bplist-creator/test/airplay.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/binaryData.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/binaryData.bplist b/node_modules/bplist-creator/test/binaryData.bplist
new file mode 100644
index 0000000..4c03581
Binary files /dev/null and b/node_modules/bplist-creator/test/binaryData.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/creatorTest.js
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/creatorTest.js b/node_modules/bplist-creator/test/creatorTest.js
new file mode 100644
index 0000000..af427a1
--- /dev/null
+++ b/node_modules/bplist-creator/test/creatorTest.js
@@ -0,0 +1,197 @@
+'use strict';
+
+var fs = require('fs');
+var path = require('path');
+var nodeunit = require('nodeunit');
+var bplistParser = require('bplist-parser');
+var bplistCreator = require('../');
+
+module.exports = {
+// 'iTunes Small': function(test) {
+// var file = path.join(__dirname, "iTunes-small.bplist");
+// testFile(test, file);
+// },
+
+ 'sample1': function(test) {
+ var file = path.join(__dirname, "sample1.bplist");
+ testFile(test, file);
+ },
+
+ 'sample2': function(test) {
+ var file = path.join(__dirname, "sample2.bplist");
+ testFile(test, file);
+ },
+
+ 'binary data': function(test) {
+ var file = path.join(__dirname, "binaryData.bplist");
+ testFile(test, file);
+ },
+
+ 'airplay': function(test) {
+ var file = path.join(__dirname, "airplay.bplist");
+ testFile(test, file);
+ },
+
+// 'utf16': function(test) {
+// var file = path.join(__dirname, "utf16.bplist");
+// testFile(test, file);
+// },
+
+// 'uid': function(test) {
+// var file = path.join(__dirname, "uid.bplist");
+// testFile(test, file);
+// }
+};
+
+function testFile(test, file) {
+ fs.readFile(file, function(err, fileData) {
+ if (err) {
+ return test.done(err);
+ }
+
+ bplistParser.parseFile(file, function(err, dicts) {
+ if (err) {
+ return test.done(err);
+ }
+
+ // airplay overrides
+ if (dicts && dicts[0] && dicts[0].loadedTimeRanges && dicts[0].loadedTimeRanges[0] && dicts[0].loadedTimeRanges[0].hasOwnProperty('start')) {
+ dicts[0].loadedTimeRanges[0].start = {
+ bplistOverride: true,
+ type: 'double',
+ value: dicts[0].loadedTimeRanges[0].start
+ };
+ }
+ if (dicts && dicts[0] && dicts[0].loadedTimeRanges && dicts[0].seekableTimeRanges[0] && dicts[0].seekableTimeRanges[0].hasOwnProperty('start')) {
+ dicts[0].seekableTimeRanges[0].start = {
+ bplistOverride: true,
+ type: 'double',
+ value: dicts[0].seekableTimeRanges[0].start
+ };
+ }
+ if (dicts && dicts[0] && dicts[0].hasOwnProperty('rate')) {
+ dicts[0].rate = {
+ bplistOverride: true,
+ type: 'double',
+ value: dicts[0].rate
+ };
+ }
+
+ // utf16
+ if (dicts && dicts[0] && dicts[0].hasOwnProperty('NSHumanReadableCopyright')) {
+ dicts[0].NSHumanReadableCopyright = {
+ bplistOverride: true,
+ type: 'string-utf16',
+ value: dicts[0].NSHumanReadableCopyright
+ };
+ }
+ if (dicts && dicts[0] && dicts[0].hasOwnProperty('CFBundleExecutable')) {
+ dicts[0].CFBundleExecutable = {
+ bplistOverride: true,
+ type: 'string',
+ value: dicts[0].CFBundleExecutable
+ };
+ }
+ if (dicts && dicts[0] && dicts[0].CFBundleURLTypes && dicts[0].CFBundleURLTypes[0] && dicts[0].CFBundleURLTypes[0].hasOwnProperty('CFBundleURLSchemes')) {
+ dicts[0].CFBundleURLTypes[0].CFBundleURLSchemes[0] = {
+ bplistOverride: true,
+ type: 'string',
+ value: dicts[0].CFBundleURLTypes[0].CFBundleURLSchemes[0]
+ };
+ }
+ if (dicts && dicts[0] && dicts[0].hasOwnProperty('CFBundleDisplayName')) {
+ dicts[0].CFBundleDisplayName = {
+ bplistOverride: true,
+ type: 'string',
+ value: dicts[0].CFBundleDisplayName
+ };
+ }
+ if (dicts && dicts[0] && dicts[0].hasOwnProperty('DTPlatformBuild')) {
+ dicts[0].DTPlatformBuild = {
+ bplistOverride: true,
+ type: 'string',
+ value: dicts[0].DTPlatformBuild
+ };
+ }
+
+ var buf = bplistCreator(dicts);
+ compareBuffers(test, buf, fileData);
+ return test.done();
+ });
+ });
+}
+
+function compareBuffers(test, buf1, buf2) {
+ if (buf1.length !== buf2.length) {
+ printBuffers(buf1, buf2);
+ return test.fail("buffer size mismatch. found: " + buf1.length + ", expected: " + buf2.length + ".");
+ }
+ for (var i = 0; i < buf1.length; i++) {
+ if (buf1[i] !== buf2[i]) {
+ printBuffers(buf1, buf2);
+ return test.fail("buffer mismatch at offset 0x" + i.toString(16) + ". found: 0x" + buf1[i].toString(16) + ", expected: 0x" + buf2[i].toString(16) + ".");
+ }
+ }
+}
+
+function printBuffers(buf1, buf2) {
+ var i, t;
+ for (var lineOffset = 0; lineOffset < buf1.length || lineOffset < buf2.length; lineOffset += 16) {
+ var line = '';
+
+ t = ('000000000' + lineOffset.toString(16));
+ line += t.substr(t.length - 8) + ': ';
+
+ for (i = 0; i < 16; i++) {
+ if (i == 8) {
+ line += ' ';
+ }
+ if (lineOffset + i < buf1.length) {
+ t = ('00' + buf1[lineOffset + i].toString(16));
+ line += t.substr(t.length - 2) + ' ';
+ } else {
+ line += ' ';
+ }
+ }
+ line += ' ';
+ for (i = 0; i < 16; i++) {
+ if (lineOffset + i < buf1.length) {
+ t = String.fromCharCode(buf1[lineOffset + i]);
+ if (t < ' ' || t > '~') {
+ t = '.';
+ }
+ line += t;
+ } else {
+ line += ' ';
+ }
+ }
+
+ line += ' - ';
+
+ for (i = 0; i < 16; i++) {
+ if (i == 8) {
+ line += ' ';
+ }
+ if (lineOffset + i < buf2.length) {
+ t = ('00' + buf2[lineOffset + i].toString(16));
+ line += t.substr(t.length - 2) + ' ';
+ } else {
+ line += ' ';
+ }
+ }
+ line += ' ';
+ for (i = 0; i < 16; i++) {
+ if (lineOffset + i < buf2.length) {
+ t = String.fromCharCode(buf2[lineOffset + i]);
+ if (t < ' ' || t > '~') {
+ t = '.';
+ }
+ line += t;
+ } else {
+ line += ' ';
+ }
+ }
+
+ console.log(line);
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/iTunes-small.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/iTunes-small.bplist b/node_modules/bplist-creator/test/iTunes-small.bplist
new file mode 100644
index 0000000..b7edb14
Binary files /dev/null and b/node_modules/bplist-creator/test/iTunes-small.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/sample1.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/sample1.bplist b/node_modules/bplist-creator/test/sample1.bplist
new file mode 100644
index 0000000..5b808ff
Binary files /dev/null and b/node_modules/bplist-creator/test/sample1.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/sample2.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/sample2.bplist b/node_modules/bplist-creator/test/sample2.bplist
new file mode 100644
index 0000000..fc42979
Binary files /dev/null and b/node_modules/bplist-creator/test/sample2.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/uid.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/uid.bplist b/node_modules/bplist-creator/test/uid.bplist
new file mode 100644
index 0000000..59f341e
Binary files /dev/null and b/node_modules/bplist-creator/test/uid.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-creator/test/utf16.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-creator/test/utf16.bplist b/node_modules/bplist-creator/test/utf16.bplist
new file mode 100644
index 0000000..ba4bcfa
Binary files /dev/null and b/node_modules/bplist-creator/test/utf16.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/.npmignore b/node_modules/bplist-parser/.npmignore
new file mode 100644
index 0000000..a9b46ea
--- /dev/null
+++ b/node_modules/bplist-parser/.npmignore
@@ -0,0 +1,8 @@
+/build/*
+node_modules
+*.node
+*.sh
+*.swp
+.lock*
+npm-debug.log
+.idea
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/README.md
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/README.md b/node_modules/bplist-parser/README.md
new file mode 100644
index 0000000..37e5e1c
--- /dev/null
+++ b/node_modules/bplist-parser/README.md
@@ -0,0 +1,47 @@
+bplist-parser
+=============
+
+Binary Mac OS X Plist (property list) parser.
+
+## Installation
+
+```bash
+$ npm install bplist-parser
+```
+
+## Quick Examples
+
+```javascript
+var bplist = require('bplist-parser');
+
+bplist.parseFile('myPlist.bplist', function(err, obj) {
+ if (err) throw err;
+
+ console.log(JSON.stringify(obj));
+});
+```
+
+## License
+
+(The MIT License)
+
+Copyright (c) 2012 Near Infinity Corporation
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/bplistParser.js
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/bplistParser.js b/node_modules/bplist-parser/bplistParser.js
new file mode 100644
index 0000000..f8335bc
--- /dev/null
+++ b/node_modules/bplist-parser/bplistParser.js
@@ -0,0 +1,357 @@
+'use strict';
+
+// adapted from http://code.google.com/p/plist/source/browse/trunk/src/com/dd/plist/BinaryPropertyListParser.java
+
+var fs = require('fs');
+var bigInt = require("big-integer");
+var debug = false;
+
+exports.maxObjectSize = 100 * 1000 * 1000; // 100Meg
+exports.maxObjectCount = 32768;
+
+// EPOCH = new SimpleDateFormat("yyyy MM dd zzz").parse("2001 01 01 GMT").getTime();
+// ...but that's annoying in a static initializer because it can throw exceptions, ick.
+// So we just hardcode the correct value.
+var EPOCH = 978307200000;
+
+// UID object definition
+var UID = exports.UID = function(id) {
+ this.UID = id;
+}
+
+var parseFile = exports.parseFile = function (fileNameOrBuffer, callback) {
+ function tryParseBuffer(buffer) {
+ var err = null;
+ var result;
+ try {
+ result = parseBuffer(buffer);
+ } catch (ex) {
+ err = ex;
+ }
+ callback(err, result);
+ }
+
+ if (Buffer.isBuffer(fileNameOrBuffer)) {
+ return tryParseBuffer(fileNameOrBuffer);
+ } else {
+ fs.readFile(fileNameOrBuffer, function (err, data) {
+ if (err) { return callback(err); }
+ tryParseBuffer(data);
+ });
+ }
+};
+
+var parseBuffer = exports.parseBuffer = function (buffer) {
+ var result = {};
+
+ // check header
+ var header = buffer.slice(0, 'bplist'.length).toString('utf8');
+ if (header !== 'bplist') {
+ throw new Error("Invalid binary plist. Expected 'bplist' at offset 0.");
+ }
+
+ // Handle trailer, last 32 bytes of the file
+ var trailer = buffer.slice(buffer.length - 32, buffer.length);
+ // 6 null bytes (index 0 to 5)
+ var offsetSize = trailer.readUInt8(6);
+ if (debug) {
+ console.log("offsetSize: " + offsetSize);
+ }
+ var objectRefSize = trailer.readUInt8(7);
+ if (debug) {
+ console.log("objectRefSize: " + objectRefSize);
+ }
+ var numObjects = readUInt64BE(trailer, 8);
+ if (debug) {
+ console.log("numObjects: " + numObjects);
+ }
+ var topObject = readUInt64BE(trailer, 16);
+ if (debug) {
+ console.log("topObject: " + topObject);
+ }
+ var offsetTableOffset = readUInt64BE(trailer, 24);
+ if (debug) {
+ console.log("offsetTableOffset: " + offsetTableOffset);
+ }
+
+ if (numObjects > exports.maxObjectCount) {
+ throw new Error("maxObjectCount exceeded");
+ }
+
+ // Handle offset table
+ var offsetTable = [];
+
+ for (var i = 0; i < numObjects; i++) {
+ var offsetBytes = buffer.slice(offsetTableOffset + i * offsetSize, offsetTableOffset + (i + 1) * offsetSize);
+ offsetTable[i] = readUInt(offsetBytes, 0);
+ if (debug) {
+ console.log("Offset for Object #" + i + " is " + offsetTable[i] + " [" + offsetTable[i].toString(16) + "]");
+ }
+ }
+
+ // Parses an object inside the currently parsed binary property list.
+ // For the format specification check
+ // <a href="http://www.opensource.apple.com/source/CF/CF-635/CFBinaryPList.c">
+ // Apple's binary property list parser implementation</a>.
+ function parseObject(tableOffset) {
+ var offset = offsetTable[tableOffset];
+ var type = buffer[offset];
+ var objType = (type & 0xF0) >> 4; //First 4 bits
+ var objInfo = (type & 0x0F); //Second 4 bits
+ switch (objType) {
+ case 0x0:
+ return parseSimple();
+ case 0x1:
+ return parseInteger();
+ case 0x8:
+ return parseUID();
+ case 0x2:
+ return parseReal();
+ case 0x3:
+ return parseDate();
+ case 0x4:
+ return parseData();
+ case 0x5: // ASCII
+ return parsePlistString();
+ case 0x6: // UTF-16
+ return parsePlistString(true);
+ case 0xA:
+ return parseArray();
+ case 0xD:
+ return parseDictionary();
+ default:
+ throw new Error("Unhandled type 0x" + objType.toString(16));
+ }
+
+ function parseSimple() {
+ //Simple
+ switch (objInfo) {
+ case 0x0: // null
+ return null;
+ case 0x8: // false
+ return false;
+ case 0x9: // true
+ return true;
+ case 0xF: // filler byte
+ return null;
+ default:
+ throw new Error("Unhandled simple type 0x" + objType.toString(16));
+ }
+ }
+
+ function bufferToHexString(buffer) {
+ var str = '';
+ var i;
+ for (i = 0; i < buffer.length; i++) {
+ if (buffer[i] != 0x00) {
+ break;
+ }
+ }
+ for (; i < buffer.length; i++) {
+ var part = '00' + buffer[i].toString(16);
+ str += part.substr(part.length - 2);
+ }
+ return str;
+ }
+
+ function parseInteger() {
+ var length = Math.pow(2, objInfo);
+ if (length > 4) {
+ var data = buffer.slice(offset + 1, offset + 1 + length);
+ var str = bufferToHexString(data);
+ return bigInt(str, 16);
+ } if (length < exports.maxObjectSize) {
+ return readUInt(buffer.slice(offset + 1, offset + 1 + length));
+ } else {
+ throw new Error("To little heap space available! Wanted to read " + length + " bytes, but only " + exports.maxObjectSize + " are available.");
+ }
+ }
+
+ function parseUID() {
+ var length = objInfo + 1;
+ if (length < exports.maxObjectSize) {
+ return new UID(readUInt(buffer.slice(offset + 1, offset + 1 + length)));
+ } else {
+ throw new Error("To little heap space available! Wanted to read " + length + " bytes, but only " + exports.maxObjectSize + " are available.");
+ }
+ }
+
+ function parseReal() {
+ var length = Math.pow(2, objInfo);
+ if (length < exports.maxObjectSize) {
+ var realBuffer = buffer.slice(offset + 1, offset + 1 + length);
+ if (length === 4) {
+ return realBuffer.readFloatBE(0);
+ }
+ else if (length === 8) {
+ return realBuffer.readDoubleBE(0);
+ }
+ } else {
+ throw new Error("To little heap space available! Wanted to read " + length + " bytes, but only " + exports.maxObjectSize + " are available.");
+ }
+ }
+
+ function parseDate() {
+ if (objInfo != 0x3) {
+ console.error("Unknown date type :" + objInfo + ". Parsing anyway...");
+ }
+ var dateBuffer = buffer.slice(offset + 1, offset + 9);
+ return new Date(EPOCH + (1000 * dateBuffer.readDoubleBE(0)));
+ }
+
+ function parseData() {
+ var dataoffset = 1;
+ var length = objInfo;
+ if (objInfo == 0xF) {
+ var int_type = buffer[offset + 1];
+ var intType = (int_type & 0xF0) / 0x10;
+ if (intType != 0x1) {
+ console.error("0x4: UNEXPECTED LENGTH-INT TYPE! " + intType);
+ }
+ var intInfo = int_type & 0x0F;
+ var intLength = Math.pow(2, intInfo);
+ dataoffset = 2 + intLength;
+ if (intLength < 3) {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ } else {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ }
+ }
+ if (length < exports.maxObjectSize) {
+ return buffer.slice(offset + dataoffset, offset + dataoffset + length);
+ } else {
+ throw new Error("To little heap space available! Wanted to read " + length + " bytes, but only " + exports.maxObjectSize + " are available.");
+ }
+ }
+
+ function parsePlistString (isUtf16) {
+ isUtf16 = isUtf16 || 0;
+ var enc = "utf8";
+ var length = objInfo;
+ var stroffset = 1;
+ if (objInfo == 0xF) {
+ var int_type = buffer[offset + 1];
+ var intType = (int_type & 0xF0) / 0x10;
+ if (intType != 0x1) {
+ console.err("UNEXPECTED LENGTH-INT TYPE! " + intType);
+ }
+ var intInfo = int_type & 0x0F;
+ var intLength = Math.pow(2, intInfo);
+ var stroffset = 2 + intLength;
+ if (intLength < 3) {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ } else {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ }
+ }
+ // length is String length -> to get byte length multiply by 2, as 1 character takes 2 bytes in UTF-16
+ length *= (isUtf16 + 1);
+ if (length < exports.maxObjectSize) {
+ var plistString = new Buffer(buffer.slice(offset + stroffset, offset + stroffset + length));
+ if (isUtf16) {
+ plistString = swapBytes(plistString);
+ enc = "ucs2";
+ }
+ return plistString.toString(enc);
+ } else {
+ throw new Error("To little heap space available! Wanted to read " + length + " bytes, but only " + exports.maxObjectSize + " are available.");
+ }
+ }
+
+ function parseArray() {
+ var length = objInfo;
+ var arrayoffset = 1;
+ if (objInfo == 0xF) {
+ var int_type = buffer[offset + 1];
+ var intType = (int_type & 0xF0) / 0x10;
+ if (intType != 0x1) {
+ console.error("0xa: UNEXPECTED LENGTH-INT TYPE! " + intType);
+ }
+ var intInfo = int_type & 0x0F;
+ var intLength = Math.pow(2, intInfo);
+ arrayoffset = 2 + intLength;
+ if (intLength < 3) {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ } else {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ }
+ }
+ if (length * objectRefSize > exports.maxObjectSize) {
+ throw new Error("To little heap space available!");
+ }
+ var array = [];
+ for (var i = 0; i < length; i++) {
+ var objRef = readUInt(buffer.slice(offset + arrayoffset + i * objectRefSize, offset + arrayoffset + (i + 1) * objectRefSize));
+ array[i] = parseObject(objRef);
+ }
+ return array;
+ }
+
+ function parseDictionary() {
+ var length = objInfo;
+ var dictoffset = 1;
+ if (objInfo == 0xF) {
+ var int_type = buffer[offset + 1];
+ var intType = (int_type & 0xF0) / 0x10;
+ if (intType != 0x1) {
+ console.error("0xD: UNEXPECTED LENGTH-INT TYPE! " + intType);
+ }
+ var intInfo = int_type & 0x0F;
+ var intLength = Math.pow(2, intInfo);
+ dictoffset = 2 + intLength;
+ if (intLength < 3) {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ } else {
+ length = readUInt(buffer.slice(offset + 2, offset + 2 + intLength));
+ }
+ }
+ if (length * 2 * objectRefSize > exports.maxObjectSize) {
+ throw new Error("To little heap space available!");
+ }
+ if (debug) {
+ console.log("Parsing dictionary #" + tableOffset);
+ }
+ var dict = {};
+ for (var i = 0; i < length; i++) {
+ var keyRef = readUInt(buffer.slice(offset + dictoffset + i * objectRefSize, offset + dictoffset + (i + 1) * objectRefSize));
+ var valRef = readUInt(buffer.slice(offset + dictoffset + (length * objectRefSize) + i * objectRefSize, offset + dictoffset + (length * objectRefSize) + (i + 1) * objectRefSize));
+ var key = parseObject(keyRef);
+ var val = parseObject(valRef);
+ if (debug) {
+ console.log(" DICT #" + tableOffset + ": Mapped " + key + " to " + val);
+ }
+ dict[key] = val;
+ }
+ return dict;
+ }
+ }
+
+ return [ parseObject(topObject) ];
+};
+
+function readUInt(buffer, start) {
+ start = start || 0;
+
+ var l = 0;
+ for (var i = start; i < buffer.length; i++) {
+ l <<= 8;
+ l |= buffer[i] & 0xFF;
+ }
+ return l;
+}
+
+// we're just going to toss the high order bits because javascript doesn't have 64-bit ints
+function readUInt64BE(buffer, start) {
+ var data = buffer.slice(start, start + 8);
+ return data.readUInt32BE(4, 8);
+}
+
+function swapBytes(buffer) {
+ var len = buffer.length;
+ for (var i = 0; i < len; i += 2) {
+ var a = buffer[i];
+ buffer[i] = buffer[i+1];
+ buffer[i+1] = a;
+ }
+ return buffer;
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/package.json
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/package.json b/node_modules/bplist-parser/package.json
new file mode 100644
index 0000000..f4d2d0f
--- /dev/null
+++ b/node_modules/bplist-parser/package.json
@@ -0,0 +1,81 @@
+{
+ "_args": [
+ [
+ "bplist-parser@^0.1.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "bplist-parser@>=0.1.0 <0.2.0",
+ "_id": "bplist-parser@0.1.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/bplist-parser",
+ "_nodeVersion": "5.1.0",
+ "_npmUser": {
+ "email": "joe@fernsroth.com",
+ "name": "joeferner"
+ },
+ "_npmVersion": "3.4.0",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "bplist-parser",
+ "raw": "bplist-parser@^0.1.0",
+ "rawSpec": "^0.1.0",
+ "scope": null,
+ "spec": ">=0.1.0 <0.2.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/bplist-parser/-/bplist-parser-0.1.1.tgz",
+ "_shasum": "d60d5dcc20cba6dc7e1f299b35d3e1f95dafbae6",
+ "_shrinkwrap": null,
+ "_spec": "bplist-parser@^0.1.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "email": "joe.ferner@nearinfinity.com",
+ "name": "Joe Ferner"
+ },
+ "bugs": {
+ "url": "https://github.com/nearinfinity/node-bplist-parser/issues"
+ },
+ "dependencies": {
+ "big-integer": "^1.6.7"
+ },
+ "description": "Binary plist parser.",
+ "devDependencies": {
+ "nodeunit": "~0.9.1"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "d60d5dcc20cba6dc7e1f299b35d3e1f95dafbae6",
+ "tarball": "http://registry.npmjs.org/bplist-parser/-/bplist-parser-0.1.1.tgz"
+ },
+ "gitHead": "c4f22650de2cc95edd21a6e609ff0654a2b951bd",
+ "homepage": "https://github.com/nearinfinity/node-bplist-parser#readme",
+ "keywords": [
+ "bplist",
+ "parser",
+ "plist"
+ ],
+ "license": "MIT",
+ "main": "bplistParser.js",
+ "maintainers": [
+ {
+ "name": "joeferner",
+ "email": "joe@fernsroth.com"
+ }
+ ],
+ "name": "bplist-parser",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/nearinfinity/node-bplist-parser.git"
+ },
+ "scripts": {
+ "test": "./node_modules/nodeunit/bin/nodeunit test"
+ },
+ "version": "0.1.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/airplay.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/airplay.bplist b/node_modules/bplist-parser/test/airplay.bplist
new file mode 100644
index 0000000..931adea
Binary files /dev/null and b/node_modules/bplist-parser/test/airplay.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/iTunes-small.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/iTunes-small.bplist b/node_modules/bplist-parser/test/iTunes-small.bplist
new file mode 100644
index 0000000..b7edb14
Binary files /dev/null and b/node_modules/bplist-parser/test/iTunes-small.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/int64.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/int64.bplist b/node_modules/bplist-parser/test/int64.bplist
new file mode 100644
index 0000000..6da9c04
Binary files /dev/null and b/node_modules/bplist-parser/test/int64.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/int64.xml
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/int64.xml b/node_modules/bplist-parser/test/int64.xml
new file mode 100644
index 0000000..cc6cb03
--- /dev/null
+++ b/node_modules/bplist-parser/test/int64.xml
@@ -0,0 +1,10 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<!DOCTYPE plist PUBLIC "-//Apple Computer//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
+<plist version="1.0">
+ <dict>
+ <key>zero</key>
+ <integer>0</integer>
+ <key>int64item</key>
+ <integer>12345678901234567890</integer>
+ </dict>
+</plist>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/parseTest.js
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/parseTest.js b/node_modules/bplist-parser/test/parseTest.js
new file mode 100644
index 0000000..67e7bfa
--- /dev/null
+++ b/node_modules/bplist-parser/test/parseTest.js
@@ -0,0 +1,159 @@
+'use strict';
+
+// tests are adapted from https://github.com/TooTallNate/node-plist
+
+var path = require('path');
+var nodeunit = require('nodeunit');
+var bplist = require('../');
+
+module.exports = {
+ 'iTunes Small': function (test) {
+ var file = path.join(__dirname, "iTunes-small.bplist");
+ var startTime1 = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime1) + 'ms');
+ var dict = dicts[0];
+ test.equal(dict['Application Version'], "9.0.3");
+ test.equal(dict['Library Persistent ID'], "6F81D37F95101437");
+ test.done();
+ });
+ },
+
+ 'sample1': function (test) {
+ var file = path.join(__dirname, "sample1.bplist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+ var dict = dicts[0];
+ test.equal(dict['CFBundleIdentifier'], 'com.apple.dictionary.MySample');
+ test.done();
+ });
+ },
+
+ 'sample2': function (test) {
+ var file = path.join(__dirname, "sample2.bplist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+ var dict = dicts[0];
+ test.equal(dict['PopupMenu'][2]['Key'], "\n #import <Cocoa/Cocoa.h>\n\n#import <MacRuby/MacRuby.h>\n\nint main(int argc, char *argv[])\n{\n return macruby_main(\"rb_main.rb\", argc, argv);\n}\n");
+ test.done();
+ });
+ },
+
+ 'airplay': function (test) {
+ var file = path.join(__dirname, "airplay.bplist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+
+ var dict = dicts[0];
+ test.equal(dict['duration'], 5555.0495000000001);
+ test.equal(dict['position'], 4.6269989039999997);
+ test.done();
+ });
+ },
+
+ 'utf16': function (test) {
+ var file = path.join(__dirname, "utf16.bplist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+
+ var dict = dicts[0];
+ test.equal(dict['CFBundleName'], 'sellStuff');
+ test.equal(dict['CFBundleShortVersionString'], '2.6.1');
+ test.equal(dict['NSHumanReadableCopyright'], '©2008-2012, sellStuff, Inc.');
+ test.done();
+ });
+ },
+
+ 'utf16chinese': function (test) {
+ var file = path.join(__dirname, "utf16_chinese.plist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+
+ var dict = dicts[0];
+ test.equal(dict['CFBundleName'], '天翼阅读');
+ test.equal(dict['CFBundleDisplayName'], '天翼阅读');
+ test.done();
+ });
+ },
+
+
+
+ 'uid': function (test) {
+ var file = path.join(__dirname, "uid.bplist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+
+ var dict = dicts[0];
+ test.deepEqual(dict['$objects'][1]['NS.keys'], [{UID:2}, {UID:3}, {UID:4}]);
+ test.deepEqual(dict['$objects'][1]['NS.objects'], [{UID: 5}, {UID:6}, {UID:7}]);
+ test.deepEqual(dict['$top']['root'], {UID:1});
+ test.done();
+ });
+ },
+
+ 'int64': function (test) {
+ var file = path.join(__dirname, "int64.bplist");
+ var startTime = new Date();
+
+ bplist.parseFile(file, function (err, dicts) {
+ if (err) {
+ throw err;
+ }
+
+ var endTime = new Date();
+ console.log('Parsed "' + file + '" in ' + (endTime - startTime) + 'ms');
+ var dict = dicts[0];
+ test.equal(dict['zero'], '0');
+ test.equal(dict['int64item'], '12345678901234567890');
+ test.done();
+ });
+ }
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/sample1.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/sample1.bplist b/node_modules/bplist-parser/test/sample1.bplist
new file mode 100644
index 0000000..5b808ff
Binary files /dev/null and b/node_modules/bplist-parser/test/sample1.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/sample2.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/sample2.bplist b/node_modules/bplist-parser/test/sample2.bplist
new file mode 100644
index 0000000..fc42979
Binary files /dev/null and b/node_modules/bplist-parser/test/sample2.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/uid.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/uid.bplist b/node_modules/bplist-parser/test/uid.bplist
new file mode 100644
index 0000000..59f341e
Binary files /dev/null and b/node_modules/bplist-parser/test/uid.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/utf16.bplist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/utf16.bplist b/node_modules/bplist-parser/test/utf16.bplist
new file mode 100644
index 0000000..ba4bcfa
Binary files /dev/null and b/node_modules/bplist-parser/test/utf16.bplist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/bplist-parser/test/utf16_chinese.plist
----------------------------------------------------------------------
diff --git a/node_modules/bplist-parser/test/utf16_chinese.plist b/node_modules/bplist-parser/test/utf16_chinese.plist
new file mode 100755
index 0000000..ba1e2d7
Binary files /dev/null and b/node_modules/bplist-parser/test/utf16_chinese.plist differ
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/brace-expansion/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/brace-expansion/.npmignore b/node_modules/brace-expansion/.npmignore
new file mode 100644
index 0000000..353546a
--- /dev/null
+++ b/node_modules/brace-expansion/.npmignore
@@ -0,0 +1,3 @@
+test
+.gitignore
+.travis.yml
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/brace-expansion/README.md
----------------------------------------------------------------------
diff --git a/node_modules/brace-expansion/README.md b/node_modules/brace-expansion/README.md
new file mode 100644
index 0000000..1793929
--- /dev/null
+++ b/node_modules/brace-expansion/README.md
@@ -0,0 +1,122 @@
+# brace-expansion
+
+[Brace expansion](https://www.gnu.org/software/bash/manual/html_node/Brace-Expansion.html),
+as known from sh/bash, in JavaScript.
+
+[](http://travis-ci.org/juliangruber/brace-expansion)
+[](https://www.npmjs.org/package/brace-expansion)
+
+[](https://ci.testling.com/juliangruber/brace-expansion)
+
+## Example
+
+```js
+var expand = require('brace-expansion');
+
+expand('file-{a,b,c}.jpg')
+// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg']
+
+expand('-v{,,}')
+// => ['-v', '-v', '-v']
+
+expand('file{0..2}.jpg')
+// => ['file0.jpg', 'file1.jpg', 'file2.jpg']
+
+expand('file-{a..c}.jpg')
+// => ['file-a.jpg', 'file-b.jpg', 'file-c.jpg']
+
+expand('file{2..0}.jpg')
+// => ['file2.jpg', 'file1.jpg', 'file0.jpg']
+
+expand('file{0..4..2}.jpg')
+// => ['file0.jpg', 'file2.jpg', 'file4.jpg']
+
+expand('file-{a..e..2}.jpg')
+// => ['file-a.jpg', 'file-c.jpg', 'file-e.jpg']
+
+expand('file{00..10..5}.jpg')
+// => ['file00.jpg', 'file05.jpg', 'file10.jpg']
+
+expand('{{A..C},{a..c}}')
+// => ['A', 'B', 'C', 'a', 'b', 'c']
+
+expand('ppp{,config,oe{,conf}}')
+// => ['ppp', 'pppconfig', 'pppoe', 'pppoeconf']
+```
+
+## API
+
+```js
+var expand = require('brace-expansion');
+```
+
+### var expanded = expand(str)
+
+Return an array of all possible and valid expansions of `str`. If none are
+found, `[str]` is returned.
+
+Valid expansions are:
+
+```js
+/^(.*,)+(.+)?$/
+// {a,b,...}
+```
+
+A comma seperated list of options, like `{a,b}` or `{a,{b,c}}` or `{,a,}`.
+
+```js
+/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/
+// {x..y[..incr]}
+```
+
+A numeric sequence from `x` to `y` inclusive, with optional increment.
+If `x` or `y` start with a leading `0`, all the numbers will be padded
+to have equal length. Negative numbers and backwards iteration work too.
+
+```js
+/^-?\d+\.\.-?\d+(\.\.-?\d+)?$/
+// {x..y[..incr]}
+```
+
+An alphabetic sequence from `x` to `y` inclusive, with optional increment.
+`x` and `y` must be exactly one character, and if given, `incr` must be a
+number.
+
+For compatibility reasons, the string `${` is not eligible for brace expansion.
+
+## Installation
+
+With [npm](https://npmjs.org) do:
+
+```bash
+npm install brace-expansion
+```
+
+## Contributors
+
+- [Julian Gruber](https://github.com/juliangruber)
+- [Isaac Z. Schlueter](https://github.com/isaacs)
+
+## License
+
+(MIT)
+
+Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
+of the Software, and to permit persons to whom the Software is furnished to do
+so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/brace-expansion/example.js
----------------------------------------------------------------------
diff --git a/node_modules/brace-expansion/example.js b/node_modules/brace-expansion/example.js
new file mode 100644
index 0000000..60ecfc7
--- /dev/null
+++ b/node_modules/brace-expansion/example.js
@@ -0,0 +1,8 @@
+var expand = require('./');
+
+console.log(expand('http://any.org/archive{1996..1999}/vol{1..4}/part{a,b,c}.html'));
+console.log(expand('http://www.numericals.com/file{1..100..10}.txt'));
+console.log(expand('http://www.letters.com/file{a..z..2}.txt'));
+console.log(expand('mkdir /usr/local/src/bash/{old,new,dist,bugs}'));
+console.log(expand('chown root /usr/{ucb/{ex,edit},lib/{ex?.?*,how_ex}}'));
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/brace-expansion/index.js
----------------------------------------------------------------------
diff --git a/node_modules/brace-expansion/index.js b/node_modules/brace-expansion/index.js
new file mode 100644
index 0000000..932718f
--- /dev/null
+++ b/node_modules/brace-expansion/index.js
@@ -0,0 +1,191 @@
+var concatMap = require('concat-map');
+var balanced = require('balanced-match');
+
+module.exports = expandTop;
+
+var escSlash = '\0SLASH'+Math.random()+'\0';
+var escOpen = '\0OPEN'+Math.random()+'\0';
+var escClose = '\0CLOSE'+Math.random()+'\0';
+var escComma = '\0COMMA'+Math.random()+'\0';
+var escPeriod = '\0PERIOD'+Math.random()+'\0';
+
+function numeric(str) {
+ return parseInt(str, 10) == str
+ ? parseInt(str, 10)
+ : str.charCodeAt(0);
+}
+
+function escapeBraces(str) {
+ return str.split('\\\\').join(escSlash)
+ .split('\\{').join(escOpen)
+ .split('\\}').join(escClose)
+ .split('\\,').join(escComma)
+ .split('\\.').join(escPeriod);
+}
+
+function unescapeBraces(str) {
+ return str.split(escSlash).join('\\')
+ .split(escOpen).join('{')
+ .split(escClose).join('}')
+ .split(escComma).join(',')
+ .split(escPeriod).join('.');
+}
+
+
+// Basically just str.split(","), but handling cases
+// where we have nested braced sections, which should be
+// treated as individual members, like {a,{b,c},d}
+function parseCommaParts(str) {
+ if (!str)
+ return [''];
+
+ var parts = [];
+ var m = balanced('{', '}', str);
+
+ if (!m)
+ return str.split(',');
+
+ var pre = m.pre;
+ var body = m.body;
+ var post = m.post;
+ var p = pre.split(',');
+
+ p[p.length-1] += '{' + body + '}';
+ var postParts = parseCommaParts(post);
+ if (post.length) {
+ p[p.length-1] += postParts.shift();
+ p.push.apply(p, postParts);
+ }
+
+ parts.push.apply(parts, p);
+
+ return parts;
+}
+
+function expandTop(str) {
+ if (!str)
+ return [];
+
+ return expand(escapeBraces(str), true).map(unescapeBraces);
+}
+
+function identity(e) {
+ return e;
+}
+
+function embrace(str) {
+ return '{' + str + '}';
+}
+function isPadded(el) {
+ return /^-?0\d/.test(el);
+}
+
+function lte(i, y) {
+ return i <= y;
+}
+function gte(i, y) {
+ return i >= y;
+}
+
+function expand(str, isTop) {
+ var expansions = [];
+
+ var m = balanced('{', '}', str);
+ if (!m || /\$$/.test(m.pre)) return [str];
+
+ var isNumericSequence = /^-?\d+\.\.-?\d+(?:\.\.-?\d+)?$/.test(m.body);
+ var isAlphaSequence = /^[a-zA-Z]\.\.[a-zA-Z](?:\.\.-?\d+)?$/.test(m.body);
+ var isSequence = isNumericSequence || isAlphaSequence;
+ var isOptions = /^(.*,)+(.+)?$/.test(m.body);
+ if (!isSequence && !isOptions) {
+ // {a},b}
+ if (m.post.match(/,.*\}/)) {
+ str = m.pre + '{' + m.body + escClose + m.post;
+ return expand(str);
+ }
+ return [str];
+ }
+
+ var n;
+ if (isSequence) {
+ n = m.body.split(/\.\./);
+ } else {
+ n = parseCommaParts(m.body);
+ if (n.length === 1) {
+ // x{{a,b}}y ==> x{a}y x{b}y
+ n = expand(n[0], false).map(embrace);
+ if (n.length === 1) {
+ var post = m.post.length
+ ? expand(m.post, false)
+ : [''];
+ return post.map(function(p) {
+ return m.pre + n[0] + p;
+ });
+ }
+ }
+ }
+
+ // at this point, n is the parts, and we know it's not a comma set
+ // with a single entry.
+
+ // no need to expand pre, since it is guaranteed to be free of brace-sets
+ var pre = m.pre;
+ var post = m.post.length
+ ? expand(m.post, false)
+ : [''];
+
+ var N;
+
+ if (isSequence) {
+ var x = numeric(n[0]);
+ var y = numeric(n[1]);
+ var width = Math.max(n[0].length, n[1].length)
+ var incr = n.length == 3
+ ? Math.abs(numeric(n[2]))
+ : 1;
+ var test = lte;
+ var reverse = y < x;
+ if (reverse) {
+ incr *= -1;
+ test = gte;
+ }
+ var pad = n.some(isPadded);
+
+ N = [];
+
+ for (var i = x; test(i, y); i += incr) {
+ var c;
+ if (isAlphaSequence) {
+ c = String.fromCharCode(i);
+ if (c === '\\')
+ c = '';
+ } else {
+ c = String(i);
+ if (pad) {
+ var need = width - c.length;
+ if (need > 0) {
+ var z = new Array(need + 1).join('0');
+ if (i < 0)
+ c = '-' + z + c.slice(1);
+ else
+ c = z + c;
+ }
+ }
+ }
+ N.push(c);
+ }
+ } else {
+ N = concatMap(n, function(el) { return expand(el, false) });
+ }
+
+ for (var j = 0; j < N.length; j++) {
+ for (var k = 0; k < post.length; k++) {
+ var expansion = pre + N[j] + post[k];
+ if (!isTop || isSequence || expansion)
+ expansions.push(expansion);
+ }
+ }
+
+ return expansions;
+}
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/brace-expansion/package.json
----------------------------------------------------------------------
diff --git a/node_modules/brace-expansion/package.json b/node_modules/brace-expansion/package.json
new file mode 100644
index 0000000..acbbaa3
--- /dev/null
+++ b/node_modules/brace-expansion/package.json
@@ -0,0 +1,104 @@
+{
+ "_args": [
+ [
+ "brace-expansion@^1.0.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/minimatch"
+ ]
+ ],
+ "_from": "brace-expansion@>=1.0.0 <2.0.0",
+ "_id": "brace-expansion@1.1.3",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/brace-expansion",
+ "_nodeVersion": "5.5.0",
+ "_npmOperationalInternal": {
+ "host": "packages-6-west.internal.npmjs.com",
+ "tmp": "tmp/brace-expansion-1.1.3.tgz_1455216688668_0.948847763473168"
+ },
+ "_npmUser": {
+ "email": "julian@juliangruber.com",
+ "name": "juliangruber"
+ },
+ "_npmVersion": "3.3.12",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "brace-expansion",
+ "raw": "brace-expansion@^1.0.0",
+ "rawSpec": "^1.0.0",
+ "scope": null,
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/minimatch"
+ ],
+ "_resolved": "http://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.3.tgz",
+ "_shasum": "46bff50115d47fc9ab89854abb87d98078a10991",
+ "_shrinkwrap": null,
+ "_spec": "brace-expansion@^1.0.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/minimatch",
+ "author": {
+ "email": "mail@juliangruber.com",
+ "name": "Julian Gruber",
+ "url": "http://juliangruber.com"
+ },
+ "bugs": {
+ "url": "https://github.com/juliangruber/brace-expansion/issues"
+ },
+ "dependencies": {
+ "balanced-match": "^0.3.0",
+ "concat-map": "0.0.1"
+ },
+ "description": "Brace expansion as known from sh/bash",
+ "devDependencies": {
+ "tape": "4.4.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "46bff50115d47fc9ab89854abb87d98078a10991",
+ "tarball": "http://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.3.tgz"
+ },
+ "gitHead": "f0da1bb668e655f67b6b2d660c6e1c19e2a6f231",
+ "homepage": "https://github.com/juliangruber/brace-expansion",
+ "keywords": [],
+ "license": "MIT",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "juliangruber",
+ "email": "julian@juliangruber.com"
+ },
+ {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ }
+ ],
+ "name": "brace-expansion",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/juliangruber/brace-expansion.git"
+ },
+ "scripts": {
+ "gentest": "bash test/generate.sh",
+ "test": "tape test/*.js"
+ },
+ "testling": {
+ "browsers": [
+ "android-browser/4.2..latest",
+ "chrome/25..latest",
+ "chrome/canary",
+ "firefox/20..latest",
+ "firefox/nightly",
+ "ie/8..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest"
+ ],
+ "files": "test/*.js"
+ },
+ "version": "1.1.3"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/.travis.yml b/node_modules/concat-map/.travis.yml
new file mode 100644
index 0000000..f1d0f13
--- /dev/null
+++ b/node_modules/concat-map/.travis.yml
@@ -0,0 +1,4 @@
+language: node_js
+node_js:
+ - 0.4
+ - 0.6
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/LICENSE b/node_modules/concat-map/LICENSE
new file mode 100644
index 0000000..ee27ba4
--- /dev/null
+++ b/node_modules/concat-map/LICENSE
@@ -0,0 +1,18 @@
+This software is released under the MIT license:
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
+the Software, and to permit persons to whom the Software is furnished to do so,
+subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
+FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
+COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
+IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
+CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/README.markdown
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/README.markdown b/node_modules/concat-map/README.markdown
new file mode 100644
index 0000000..408f70a
--- /dev/null
+++ b/node_modules/concat-map/README.markdown
@@ -0,0 +1,62 @@
+concat-map
+==========
+
+Concatenative mapdashery.
+
+[](http://ci.testling.com/substack/node-concat-map)
+
+[](http://travis-ci.org/substack/node-concat-map)
+
+example
+=======
+
+``` js
+var concatMap = require('concat-map');
+var xs = [ 1, 2, 3, 4, 5, 6 ];
+var ys = concatMap(xs, function (x) {
+ return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
+});
+console.dir(ys);
+```
+
+***
+
+```
+[ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]
+```
+
+methods
+=======
+
+``` js
+var concatMap = require('concat-map')
+```
+
+concatMap(xs, fn)
+-----------------
+
+Return an array of concatenated elements by calling `fn(x, i)` for each element
+`x` and each index `i` in the array `xs`.
+
+When `fn(x, i)` returns an array, its result will be concatenated with the
+result array. If `fn(x, i)` returns anything else, that value will be pushed
+onto the end of the result array.
+
+install
+=======
+
+With [npm](http://npmjs.org) do:
+
+```
+npm install concat-map
+```
+
+license
+=======
+
+MIT
+
+notes
+=====
+
+This module was written while sitting high above the ground in a tree.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/example/map.js
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/example/map.js b/node_modules/concat-map/example/map.js
new file mode 100644
index 0000000..3365621
--- /dev/null
+++ b/node_modules/concat-map/example/map.js
@@ -0,0 +1,6 @@
+var concatMap = require('../');
+var xs = [ 1, 2, 3, 4, 5, 6 ];
+var ys = concatMap(xs, function (x) {
+ return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
+});
+console.dir(ys);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/index.js
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/index.js b/node_modules/concat-map/index.js
new file mode 100644
index 0000000..b29a781
--- /dev/null
+++ b/node_modules/concat-map/index.js
@@ -0,0 +1,13 @@
+module.exports = function (xs, fn) {
+ var res = [];
+ for (var i = 0; i < xs.length; i++) {
+ var x = fn(xs[i], i);
+ if (isArray(x)) res.push.apply(res, x);
+ else res.push(x);
+ }
+ return res;
+};
+
+var isArray = Array.isArray || function (xs) {
+ return Object.prototype.toString.call(xs) === '[object Array]';
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/package.json
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/package.json b/node_modules/concat-map/package.json
new file mode 100644
index 0000000..586e812
--- /dev/null
+++ b/node_modules/concat-map/package.json
@@ -0,0 +1,109 @@
+{
+ "_args": [
+ [
+ "concat-map@0.0.1",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/brace-expansion"
+ ]
+ ],
+ "_from": "concat-map@0.0.1",
+ "_id": "concat-map@0.0.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/concat-map",
+ "_npmUser": {
+ "email": "mail@substack.net",
+ "name": "substack"
+ },
+ "_npmVersion": "1.3.21",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "concat-map",
+ "raw": "concat-map@0.0.1",
+ "rawSpec": "0.0.1",
+ "scope": null,
+ "spec": "0.0.1",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/brace-expansion"
+ ],
+ "_resolved": "http://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz",
+ "_shasum": "d8a96bd77fd68df7793a73036a3ba0d5405d477b",
+ "_shrinkwrap": null,
+ "_spec": "concat-map@0.0.1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/brace-expansion",
+ "author": {
+ "email": "mail@substack.net",
+ "name": "James Halliday",
+ "url": "http://substack.net"
+ },
+ "bugs": {
+ "url": "https://github.com/substack/node-concat-map/issues"
+ },
+ "dependencies": {},
+ "description": "concatenative mapdashery",
+ "devDependencies": {
+ "tape": "~2.4.0"
+ },
+ "directories": {
+ "example": "example",
+ "test": "test"
+ },
+ "dist": {
+ "shasum": "d8a96bd77fd68df7793a73036a3ba0d5405d477b",
+ "tarball": "http://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz"
+ },
+ "homepage": "https://github.com/substack/node-concat-map",
+ "keywords": [
+ "concat",
+ "concatMap",
+ "functional",
+ "higher-order",
+ "map"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "substack",
+ "email": "mail@substack.net"
+ }
+ ],
+ "name": "concat-map",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/substack/node-concat-map.git"
+ },
+ "scripts": {
+ "test": "tape test/*.js"
+ },
+ "testling": {
+ "browsers": {
+ "chrome": [
+ 10,
+ 22
+ ],
+ "ff": [
+ 10,
+ 15,
+ 3.5
+ ],
+ "ie": [
+ 6,
+ 7,
+ 8,
+ 9
+ ],
+ "opera": [
+ 12
+ ],
+ "safari": [
+ 5.1
+ ]
+ },
+ "files": "test/*.js"
+ },
+ "version": "0.0.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/concat-map/test/map.js
----------------------------------------------------------------------
diff --git a/node_modules/concat-map/test/map.js b/node_modules/concat-map/test/map.js
new file mode 100644
index 0000000..fdbd702
--- /dev/null
+++ b/node_modules/concat-map/test/map.js
@@ -0,0 +1,39 @@
+var concatMap = require('../');
+var test = require('tape');
+
+test('empty or not', function (t) {
+ var xs = [ 1, 2, 3, 4, 5, 6 ];
+ var ixes = [];
+ var ys = concatMap(xs, function (x, ix) {
+ ixes.push(ix);
+ return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
+ });
+ t.same(ys, [ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]);
+ t.same(ixes, [ 0, 1, 2, 3, 4, 5 ]);
+ t.end();
+});
+
+test('always something', function (t) {
+ var xs = [ 'a', 'b', 'c', 'd' ];
+ var ys = concatMap(xs, function (x) {
+ return x === 'b' ? [ 'B', 'B', 'B' ] : [ x ];
+ });
+ t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]);
+ t.end();
+});
+
+test('scalars', function (t) {
+ var xs = [ 'a', 'b', 'c', 'd' ];
+ var ys = concatMap(xs, function (x) {
+ return x === 'b' ? [ 'B', 'B', 'B' ] : x;
+ });
+ t.same(ys, [ 'a', 'B', 'B', 'B', 'c', 'd' ]);
+ t.end();
+});
+
+test('undefs', function (t) {
+ var xs = [ 'a', 'b', 'c', 'd' ];
+ var ys = concatMap(xs, function () {});
+ t.same(ys, [ undefined, undefined, undefined, undefined ]);
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/.jscs.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/.jscs.json b/node_modules/cordova-common/.jscs.json
new file mode 100644
index 0000000..5cc7e26
--- /dev/null
+++ b/node_modules/cordova-common/.jscs.json
@@ -0,0 +1,24 @@
+{
+ "disallowMixedSpacesAndTabs": true,
+ "disallowTrailingWhitespace": true,
+ "validateLineBreaks": "LF",
+ "validateIndentation": 4,
+ "requireLineFeedAtFileEnd": true,
+
+ "disallowSpaceAfterPrefixUnaryOperators": true,
+ "disallowSpaceBeforePostfixUnaryOperators": true,
+ "requireSpaceAfterLineComment": true,
+ "requireCapitalizedConstructors": true,
+
+ "disallowSpacesInNamedFunctionExpression": {
+ "beforeOpeningRoundBrace": true
+ },
+
+ "requireSpaceAfterKeywords": [
+ "if",
+ "else",
+ "for",
+ "while",
+ "do"
+ ]
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/.jshintignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/.jshintignore b/node_modules/cordova-common/.jshintignore
new file mode 100644
index 0000000..d606f61
--- /dev/null
+++ b/node_modules/cordova-common/.jshintignore
@@ -0,0 +1 @@
+spec/fixtures/*
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/.npmignore b/node_modules/cordova-common/.npmignore
new file mode 100644
index 0000000..5d14118
--- /dev/null
+++ b/node_modules/cordova-common/.npmignore
@@ -0,0 +1,2 @@
+spec
+coverage
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/.ratignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/.ratignore b/node_modules/cordova-common/.ratignore
new file mode 100644
index 0000000..26f7205
--- /dev/null
+++ b/node_modules/cordova-common/.ratignore
@@ -0,0 +1,2 @@
+fixtures
+coverage
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/README.md b/node_modules/cordova-common/README.md
new file mode 100644
index 0000000..6454481
--- /dev/null
+++ b/node_modules/cordova-common/README.md
@@ -0,0 +1,153 @@
+<!--
+#
+# Licensed to the Apache Software Foundation (ASF) under one
+# or more contributor license agreements. See the NOTICE file
+# distributed with this work for additional information
+# regarding copyright ownership. The ASF licenses this file
+# to you under the Apache License, Version 2.0 (the
+# "License"); you may not use this file except in compliance
+# with the License. You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing,
+# software distributed under the License is distributed on an
+# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+# KIND, either express or implied. See the License for the
+# specific language governing permissions and limitations
+# under the License.
+#
+-->
+
+# cordova-common
+Expoeses shared functionality used by [cordova-lib](https://github.com/apache/cordova-lib/) and Cordova platforms.
+## Exposed APIs
+
+### `events`
+
+Represents special instance of NodeJS EventEmitter which is intended to be used to post events to cordova-lib and cordova-cli
+
+Usage:
+```
+var events = require('cordova-common').events;
+events.emit('warn', 'Some warning message')
+```
+
+There are the following events supported by cordova-cli: `verbose`, `log`, `info`, `warn`, `error`.
+
+### `CordovaError`
+
+An error class used by Cordova to throw cordova-specific errors. The CordovaError class is inherited from Error, so CordovaError instances is also valid Error instances (`instanceof` check succeeds).
+
+Usage:
+
+```
+var CordovaError = require('cordova-common').CordovaError;
+throw new CordovaError('Some error message', SOME_ERR_CODE);
+```
+
+See [CordovaError](src/CordovaError/CordovaError.js) for supported error codes.
+
+### `ConfigParser`
+
+Exposes functionality to deal with cordova project `config.xml` files. For ConfigParser API reference check [ConfigParser Readme](src/ConfigParser/README.md).
+
+Usage:
+```
+var ConfigParser = require('cordova-common').ConfigParser;
+var appConfig = new ConfigParser('path/to/cordova-app/config.xml');
+console.log(appconfig.name() + ':' + appConfig.version());
+```
+
+### `PluginInfoProvider` and `PluginInfo`
+
+`PluginInfo` is a wrapper for cordova plugins' `plugin.xml` files. This class may be instantiated directly or via `PluginInfoProvider`. The difference is that `PluginInfoProvider` caches `PluginInfo` instances based on plugin source directory.
+
+Usage:
+```
+var PluginInfo: require('cordova-common').PluginInfo;
+var PluginInfoProvider: require('cordova-common').PluginInfoProvider;
+
+// The following instances are equal
+var plugin1 = new PluginInfo('path/to/plugin_directory');
+var plugin2 = new PluginInfoProvider().get('path/to/plugin_directory');
+
+console.log('The plugin ' + plugin1.id + ' has version ' + plugin1.version)
+```
+
+### `ActionStack`
+
+Utility module for dealing with sequential tasks. Provides a set of tasks that are needed to be done and reverts all tasks that are already completed if one of those tasks fail to complete. Used internally by cordova-lib and platform's plugin installation routines.
+
+Usage:
+```
+var ActionStack = require('cordova-common').ActionStack;
+var stack = new ActionStack()
+
+var action1 = stack.createAction(task1, [<task parameters>], task1_reverter, [<reverter_parameters>]);
+var action2 = stack.createAction(task2, [<task parameters>], task2_reverter, [<reverter_parameters>]);
+
+stack.push(action1);
+stack.push(action2);
+
+stack.process()
+.then(function() {
+ // all actions succeded
+})
+.catch(function(error){
+ // One of actions failed with error
+})
+```
+
+### `superspawn`
+
+Module for spawning child processes with some advanced logic.
+
+Usage:
+```
+var superspawn = require('cordova-common').superspawn;
+superspawn.spawn('adb', ['devices'])
+.progress(function(data){
+ if (data.stderr)
+ console.error('"adb devices" raised an error: ' + data.stderr);
+})
+.then(function(devices){
+ // Do something...
+})
+```
+
+### `xmlHelpers`
+
+A set of utility methods for dealing with xml files.
+
+Usage:
+```
+var xml = require('cordova-common').xmlHelpers;
+
+var xmlDoc1 = xml.parseElementtreeSync('some/xml/file');
+var xmlDoc2 = xml.parseElementtreeSync('another/xml/file');
+
+xml.mergeXml(doc1, doc2); // doc2 now contains all the nodes from doc1
+```
+
+### Other APIs
+
+The APIs listed below are also exposed but are intended to be only used internally by cordova plugin installation routines.
+
+```
+PlatformJson
+ConfigChanges
+ConfigKeeper
+ConfigFile
+mungeUtil
+```
+
+## Setup
+* Clone this repository onto your local machine
+ `git clone https://git-wip-us.apache.org/repos/asf/cordova-lib.git`
+* In terminal, navigate to the inner cordova-common directory
+ `cd cordova-lib/cordova-common`
+* Install dependencies and npm-link
+ `npm install && npm link`
+* Navigate to cordova-lib directory and link cordova-common
+ `cd ../cordova-lib && npm link cordova-common && npm install`
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/RELEASENOTES.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/RELEASENOTES.md b/node_modules/cordova-common/RELEASENOTES.md
new file mode 100644
index 0000000..e7db69c
--- /dev/null
+++ b/node_modules/cordova-common/RELEASENOTES.md
@@ -0,0 +1,42 @@
+<!--
+#
+# Licensed to the Apache Software Foundation (ASF) under one
+# or more contributor license agreements. See the NOTICE file
+# distributed with this work for additional information
+# regarding copyright ownership. The ASF licenses this file
+# to you under the Apache License, Version 2.0 (the
+# "License"); you may not use this file except in compliance
+# with the License. You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing,
+# software distributed under the License is distributed on an
+# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+# KIND, either express or implied. See the License for the
+# specific language governing permissions and limitations
+# under the License.
+#
+-->
+# Cordova-common Release Notes
+
+### 1.1.0 (Feb 16, 2016)
+* CB-10482 Remove references to windows8 from cordova-lib/cli
+* CB-10430 Adds forwardEvents method to easily connect two EventEmitters
+* CB-10176 Adds CordovaLogger class, based on logger module from cordova-cli
+* CB-10052 Expose child process' io streams via promise progress notification
+* CB-10497 Prefer .bat over .cmd on windows platform
+* CB-9984 Bumps plist version and fixes failing cordova-common test
+
+### 1.0.0 (Oct 29, 2015)
+
+* CB-9890 Documents cordova-common
+* CB-9598 Correct cordova-lib -> cordova-common in README
+* Pick ConfigParser changes from apache@0c3614e
+* CB-9743 Removes system frameworks handling from ConfigChanges
+* CB-9598 Cleans out code which has been moved to `cordova-common`
+* Pick ConfigParser changes from apache@ddb027b
+* Picking CordovaError changes from apache@a3b1fca
+* CB-9598 Adds tests and fixtures based on existing cordova-lib ones
+* CB-9598 Initial implementation for cordova-common
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/cordova-common.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/cordova-common.js b/node_modules/cordova-common/cordova-common.js
new file mode 100644
index 0000000..22e90a7
--- /dev/null
+++ b/node_modules/cordova-common/cordova-common.js
@@ -0,0 +1,43 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+/* jshint node:true */
+
+// For now expose plugman and cordova just as they were in the old repos
+exports = module.exports = {
+ events: require('./src/events'),
+ superspawn: require('./src/superspawn'),
+
+ ActionStack: require('./src/ActionStack'),
+ CordovaError: require('./src/CordovaError/CordovaError'),
+ CordovaLogger: require('./src/CordovaLogger'),
+ CordovaExternalToolErrorContext: require('./src/CordovaError/CordovaExternalToolErrorContext'),
+ PlatformJson: require('./src/PlatformJson'),
+ ConfigParser: require('./src/ConfigParser/ConfigParser.js'),
+
+ PluginInfo: require('./src/PluginInfo/PluginInfo.js'),
+ PluginInfoProvider: require('./src/PluginInfo/PluginInfoProvider.js'),
+
+ ConfigChanges: require('./src/ConfigChanges/ConfigChanges.js'),
+ ConfigKeeper: require('./src/ConfigChanges/ConfigKeeper.js'),
+ ConfigFile: require('./src/ConfigChanges/ConfigFile.js'),
+ mungeUtil: require('./src/ConfigChanges/munge-util.js'),
+
+ xmlHelpers: require('./src/util/xml-helpers')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/.bin/shjs
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/.bin/shjs b/node_modules/cordova-common/node_modules/.bin/shjs
new file mode 120000
index 0000000..a044997
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/.bin/shjs
@@ -0,0 +1 @@
+../shelljs/bin/shjs
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/.documentup.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.documentup.json b/node_modules/cordova-common/node_modules/shelljs/.documentup.json
new file mode 100644
index 0000000..57fe301
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/.documentup.json
@@ -0,0 +1,6 @@
+{
+ "name": "ShellJS",
+ "twitter": [
+ "r2r"
+ ]
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.jshintrc b/node_modules/cordova-common/node_modules/shelljs/.jshintrc
new file mode 100644
index 0000000..a80c559
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/.jshintrc
@@ -0,0 +1,7 @@
+{
+ "loopfunc": true,
+ "sub": true,
+ "undef": true,
+ "unused": true,
+ "node": true
+}
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.npmignore b/node_modules/cordova-common/node_modules/shelljs/.npmignore
new file mode 100644
index 0000000..6b20c38
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/.npmignore
@@ -0,0 +1,2 @@
+test/
+tmp/
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.travis.yml b/node_modules/cordova-common/node_modules/shelljs/.travis.yml
new file mode 100644
index 0000000..1b3280a
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/.travis.yml
@@ -0,0 +1,6 @@
+language: node_js
+node_js:
+ - "0.10"
+ - "0.11"
+ - "0.12"
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/LICENSE b/node_modules/cordova-common/node_modules/shelljs/LICENSE
new file mode 100644
index 0000000..1b35ee9
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/LICENSE
@@ -0,0 +1,26 @@
+Copyright (c) 2012, Artur Adib <aa...@mozilla.com>
+All rights reserved.
+
+You may use this project under the terms of the New BSD license as follows:
+
+Redistribution and use in source and binary forms, with or without
+modification, are permitted provided that the following conditions are met:
+ * Redistributions of source code must retain the above copyright
+ notice, this list of conditions and the following disclaimer.
+ * Redistributions in binary form must reproduce the above copyright
+ notice, this list of conditions and the following disclaimer in the
+ documentation and/or other materials provided with the distribution.
+ * Neither the name of Artur Adib nor the
+ names of the contributors may be used to endorse or promote products
+ derived from this software without specific prior written permission.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
+ARE DISCLAIMED. IN NO EVENT SHALL ARTUR ADIB BE LIABLE FOR ANY
+DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
+(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
+LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
+ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
+(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
+THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[51/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
CB-10668 added node_modules directory
Project: http://git-wip-us.apache.org/repos/asf/cordova-osx/repo
Commit: http://git-wip-us.apache.org/repos/asf/cordova-osx/commit/42cbe9fd
Tree: http://git-wip-us.apache.org/repos/asf/cordova-osx/tree/42cbe9fd
Diff: http://git-wip-us.apache.org/repos/asf/cordova-osx/diff/42cbe9fd
Branch: refs/heads/4.0.x
Commit: 42cbe9fd3a7215c66e9d4dd31ac179a2206b0eb8
Parents: be5fba3
Author: Steve Gill <st...@gmail.com>
Authored: Mon Feb 22 11:55:25 2016 -0800
Committer: Steve Gill <st...@gmail.com>
Committed: Thu Mar 3 15:22:57 2016 -0800
----------------------------------------------------------------------
node_modules/abbrev/.npmignore | 4 +
node_modules/abbrev/.travis.yml | 5 +
node_modules/abbrev/CONTRIBUTING.md | 3 +
node_modules/abbrev/LICENSE | 15 +
node_modules/abbrev/README.md | 23 +
node_modules/abbrev/abbrev.js | 62 +
node_modules/abbrev/package.json | 74 +
node_modules/abbrev/test.js | 47 +
node_modules/ansi/.jshintrc | 4 +
node_modules/ansi/.npmignore | 1 +
node_modules/ansi/History.md | 23 +
node_modules/ansi/LICENSE | 24 +
node_modules/ansi/README.md | 98 +
node_modules/ansi/examples/beep/index.js | 16 +
node_modules/ansi/examples/clear/index.js | 15 +
node_modules/ansi/examples/cursorPosition.js | 32 +
node_modules/ansi/examples/progress/index.js | 87 +
node_modules/ansi/lib/ansi.js | 405 +
node_modules/ansi/lib/newlines.js | 71 +
node_modules/ansi/package.json | 85 +
node_modules/balanced-match/.npmignore | 2 +
node_modules/balanced-match/.travis.yml | 3 +
node_modules/balanced-match/LICENSE.md | 21 +
node_modules/balanced-match/Makefile | 6 +
node_modules/balanced-match/README.md | 89 +
node_modules/balanced-match/example.js | 5 +
node_modules/balanced-match/index.js | 50 +
node_modules/balanced-match/package.json | 98 +
node_modules/balanced-match/test/balanced.js | 84 +
node_modules/base64-js/.travis.yml | 5 +
node_modules/base64-js/LICENSE.MIT | 21 +
node_modules/base64-js/README.md | 31 +
node_modules/base64-js/bench/bench.js | 19 +
node_modules/base64-js/lib/b64.js | 124 +
node_modules/base64-js/package.json | 93 +
node_modules/base64-js/test/convert.js | 51 +
node_modules/base64-js/test/url-safe.js | 18 +
node_modules/big-integer/BigInteger.js | 1189 ++
node_modules/big-integer/BigInteger.min.js | 1 +
node_modules/big-integer/README.md | 506 +
node_modules/big-integer/package.json | 100 +
node_modules/bplist-creator/.npmignore | 8 +
node_modules/bplist-creator/README.md | 64 +
node_modules/bplist-creator/bplistCreator.js | 404 +
node_modules/bplist-creator/package.json | 79 +
node_modules/bplist-creator/test/airplay.bplist | Bin 0 -> 341 bytes
.../bplist-creator/test/binaryData.bplist | Bin 0 -> 7153 bytes
node_modules/bplist-creator/test/creatorTest.js | 197 +
.../bplist-creator/test/iTunes-small.bplist | Bin 0 -> 24433 bytes
node_modules/bplist-creator/test/sample1.bplist | Bin 0 -> 605 bytes
node_modules/bplist-creator/test/sample2.bplist | Bin 0 -> 384 bytes
node_modules/bplist-creator/test/uid.bplist | Bin 0 -> 365 bytes
node_modules/bplist-creator/test/utf16.bplist | Bin 0 -> 1273 bytes
node_modules/bplist-parser/.npmignore | 8 +
node_modules/bplist-parser/README.md | 47 +
node_modules/bplist-parser/bplistParser.js | 357 +
node_modules/bplist-parser/package.json | 81 +
node_modules/bplist-parser/test/airplay.bplist | Bin 0 -> 341 bytes
.../bplist-parser/test/iTunes-small.bplist | Bin 0 -> 24433 bytes
node_modules/bplist-parser/test/int64.bplist | Bin 0 -> 84 bytes
node_modules/bplist-parser/test/int64.xml | 10 +
node_modules/bplist-parser/test/parseTest.js | 159 +
node_modules/bplist-parser/test/sample1.bplist | Bin 0 -> 605 bytes
node_modules/bplist-parser/test/sample2.bplist | Bin 0 -> 384 bytes
node_modules/bplist-parser/test/uid.bplist | Bin 0 -> 365 bytes
node_modules/bplist-parser/test/utf16.bplist | Bin 0 -> 1273 bytes
.../bplist-parser/test/utf16_chinese.plist | Bin 0 -> 2362 bytes
node_modules/brace-expansion/.npmignore | 3 +
node_modules/brace-expansion/README.md | 122 +
node_modules/brace-expansion/example.js | 8 +
node_modules/brace-expansion/index.js | 191 +
node_modules/brace-expansion/package.json | 104 +
node_modules/concat-map/.travis.yml | 4 +
node_modules/concat-map/LICENSE | 18 +
node_modules/concat-map/README.markdown | 62 +
node_modules/concat-map/example/map.js | 6 +
node_modules/concat-map/index.js | 13 +
node_modules/concat-map/package.json | 109 +
node_modules/concat-map/test/map.js | 39 +
node_modules/cordova-common/.jscs.json | 24 +
node_modules/cordova-common/.jshintignore | 1 +
node_modules/cordova-common/.npmignore | 2 +
node_modules/cordova-common/.ratignore | 2 +
node_modules/cordova-common/README.md | 153 +
node_modules/cordova-common/RELEASENOTES.md | 42 +
node_modules/cordova-common/cordova-common.js | 43 +
.../cordova-common/node_modules/.bin/shjs | 1 +
.../node_modules/shelljs/.documentup.json | 6 +
.../node_modules/shelljs/.jshintrc | 7 +
.../node_modules/shelljs/.npmignore | 2 +
.../node_modules/shelljs/.travis.yml | 6 +
.../cordova-common/node_modules/shelljs/LICENSE | 26 +
.../node_modules/shelljs/README.md | 579 +
.../node_modules/shelljs/RELEASE.md | 9 +
.../node_modules/shelljs/bin/shjs | 51 +
.../node_modules/shelljs/global.js | 3 +
.../cordova-common/node_modules/shelljs/make.js | 56 +
.../node_modules/shelljs/package.json | 88 +
.../shelljs/scripts/generate-docs.js | 21 +
.../node_modules/shelljs/scripts/run-tests.js | 50 +
.../node_modules/shelljs/shell.js | 159 +
.../node_modules/shelljs/src/cat.js | 43 +
.../node_modules/shelljs/src/cd.js | 19 +
.../node_modules/shelljs/src/chmod.js | 208 +
.../node_modules/shelljs/src/common.js | 203 +
.../node_modules/shelljs/src/cp.js | 204 +
.../node_modules/shelljs/src/dirs.js | 191 +
.../node_modules/shelljs/src/echo.js | 20 +
.../node_modules/shelljs/src/error.js | 10 +
.../node_modules/shelljs/src/exec.js | 216 +
.../node_modules/shelljs/src/find.js | 51 +
.../node_modules/shelljs/src/grep.js | 52 +
.../node_modules/shelljs/src/ln.js | 53 +
.../node_modules/shelljs/src/ls.js | 126 +
.../node_modules/shelljs/src/mkdir.js | 68 +
.../node_modules/shelljs/src/mv.js | 80 +
.../node_modules/shelljs/src/popd.js | 1 +
.../node_modules/shelljs/src/pushd.js | 1 +
.../node_modules/shelljs/src/pwd.js | 11 +
.../node_modules/shelljs/src/rm.js | 163 +
.../node_modules/shelljs/src/sed.js | 43 +
.../node_modules/shelljs/src/tempdir.js | 56 +
.../node_modules/shelljs/src/test.js | 85 +
.../node_modules/shelljs/src/to.js | 29 +
.../node_modules/shelljs/src/toEnd.js | 29 +
.../node_modules/shelljs/src/which.js | 83 +
node_modules/cordova-common/package.json | 119 +
node_modules/cordova-common/src/.jshintrc | 10 +
node_modules/cordova-common/src/ActionStack.js | 85 +
.../src/ConfigChanges/ConfigChanges.js | 323 +
.../src/ConfigChanges/ConfigFile.js | 208 +
.../src/ConfigChanges/ConfigKeeper.js | 65 +
.../src/ConfigChanges/munge-util.js | 160 +
.../src/ConfigParser/ConfigParser.js | 499 +
.../cordova-common/src/ConfigParser/README.md | 86 +
.../src/CordovaError/CordovaError.js | 91 +
.../CordovaExternalToolErrorContext.js | 48 +
.../cordova-common/src/CordovaLogger.js | 203 +
node_modules/cordova-common/src/PlatformJson.js | 155 +
.../cordova-common/src/PluginInfo/PluginInfo.js | 410 +
.../src/PluginInfo/PluginInfoProvider.js | 82 +
node_modules/cordova-common/src/events.js | 65 +
node_modules/cordova-common/src/superspawn.js | 184 +
.../cordova-common/src/util/plist-helpers.js | 101 +
.../cordova-common/src/util/xml-helpers.js | 266 +
node_modules/cordova-registry-mapper/.npmignore | 1 +
.../cordova-registry-mapper/.travis.yml | 7 +
node_modules/cordova-registry-mapper/README.md | 14 +
node_modules/cordova-registry-mapper/index.js | 204 +
.../cordova-registry-mapper/package.json | 77 +
.../cordova-registry-mapper/tests/test.js | 11 +
node_modules/elementtree/.npmignore | 1 +
node_modules/elementtree/.travis.yml | 10 +
node_modules/elementtree/CHANGES.md | 39 +
node_modules/elementtree/LICENSE.txt | 203 +
node_modules/elementtree/Makefile | 21 +
node_modules/elementtree/NOTICE | 5 +
node_modules/elementtree/README.md | 141 +
node_modules/elementtree/lib/constants.js | 20 +
node_modules/elementtree/lib/elementpath.js | 343 +
node_modules/elementtree/lib/elementtree.js | 611 +
node_modules/elementtree/lib/errors.js | 31 +
node_modules/elementtree/lib/parser.js | 33 +
node_modules/elementtree/lib/parsers/index.js | 1 +
node_modules/elementtree/lib/parsers/sax.js | 56 +
node_modules/elementtree/lib/sprintf.js | 86 +
node_modules/elementtree/lib/treebuilder.js | 60 +
node_modules/elementtree/lib/utils.js | 72 +
node_modules/elementtree/package.json | 100 +
node_modules/elementtree/tests/data/xml1.xml | 17 +
node_modules/elementtree/tests/data/xml2.xml | 14 +
node_modules/elementtree/tests/test-simple.js | 339 +
node_modules/glob/LICENSE | 15 +
node_modules/glob/README.md | 377 +
node_modules/glob/common.js | 245 +
node_modules/glob/glob.js | 752 +
node_modules/glob/package.json | 98 +
node_modules/glob/sync.js | 460 +
node_modules/inflight/.eslintrc | 17 +
node_modules/inflight/LICENSE | 15 +
node_modules/inflight/README.md | 37 +
node_modules/inflight/inflight.js | 44 +
node_modules/inflight/package.json | 86 +
node_modules/inflight/test.js | 97 +
node_modules/inherits/LICENSE | 16 +
node_modules/inherits/README.md | 42 +
node_modules/inherits/inherits.js | 1 +
node_modules/inherits/inherits_browser.js | 23 +
node_modules/inherits/package.json | 77 +
node_modules/inherits/test.js | 25 +
node_modules/lodash-node/LICENSE.txt | 22 +
node_modules/lodash-node/README.md | 44 +
node_modules/lodash-node/compat/arrays.js | 40 +
.../lodash-node/compat/arrays/compact.js | 38 +
.../lodash-node/compat/arrays/difference.js | 31 +
.../lodash-node/compat/arrays/findIndex.js | 65 +
.../lodash-node/compat/arrays/findLastIndex.js | 63 +
node_modules/lodash-node/compat/arrays/first.js | 86 +
.../lodash-node/compat/arrays/flatten.js | 66 +
.../lodash-node/compat/arrays/indexOf.js | 50 +
.../lodash-node/compat/arrays/initial.js | 82 +
.../lodash-node/compat/arrays/intersection.js | 83 +
node_modules/lodash-node/compat/arrays/last.js | 84 +
.../lodash-node/compat/arrays/lastIndexOf.js | 54 +
node_modules/lodash-node/compat/arrays/pull.js | 57 +
node_modules/lodash-node/compat/arrays/range.js | 69 +
.../lodash-node/compat/arrays/remove.js | 71 +
node_modules/lodash-node/compat/arrays/rest.js | 83 +
.../lodash-node/compat/arrays/sortedIndex.js | 77 +
node_modules/lodash-node/compat/arrays/union.js | 30 +
node_modules/lodash-node/compat/arrays/uniq.js | 69 +
.../lodash-node/compat/arrays/without.js | 31 +
node_modules/lodash-node/compat/arrays/xor.js | 46 +
node_modules/lodash-node/compat/arrays/zip.js | 40 +
.../lodash-node/compat/arrays/zipObject.js | 48 +
node_modules/lodash-node/compat/chaining.js | 17 +
.../lodash-node/compat/chaining/chain.js | 41 +
node_modules/lodash-node/compat/chaining/tap.js | 35 +
.../lodash-node/compat/chaining/wrapperChain.js | 40 +
.../compat/chaining/wrapperToString.js | 26 +
.../compat/chaining/wrapperValueOf.js | 28 +
node_modules/lodash-node/compat/collections.js | 49 +
.../lodash-node/compat/collections/at.js | 50 +
.../lodash-node/compat/collections/contains.js | 65 +
.../lodash-node/compat/collections/countBy.js | 55 +
.../lodash-node/compat/collections/every.js | 75 +
.../lodash-node/compat/collections/filter.js | 77 +
.../lodash-node/compat/collections/find.js | 81 +
.../lodash-node/compat/collections/findLast.js | 44 +
.../lodash-node/compat/collections/forEach.js | 55 +
.../compat/collections/forEachRight.js | 59 +
.../lodash-node/compat/collections/groupBy.js | 56 +
.../lodash-node/compat/collections/indexBy.js | 54 +
.../lodash-node/compat/collections/invoke.js | 47 +
.../lodash-node/compat/collections/map.js | 70 +
.../lodash-node/compat/collections/max.js | 90 +
.../lodash-node/compat/collections/min.js | 90 +
.../lodash-node/compat/collections/pluck.js | 33 +
.../lodash-node/compat/collections/reduce.js | 67 +
.../compat/collections/reduceRight.js | 42 +
.../lodash-node/compat/collections/reject.js | 57 +
.../lodash-node/compat/collections/sample.js | 52 +
.../lodash-node/compat/collections/shuffle.js | 39 +
.../lodash-node/compat/collections/size.js | 36 +
.../lodash-node/compat/collections/some.js | 76 +
.../lodash-node/compat/collections/sortBy.js | 101 +
.../lodash-node/compat/collections/toArray.js | 36 +
.../lodash-node/compat/collections/where.js | 38 +
node_modules/lodash-node/compat/functions.js | 27 +
.../lodash-node/compat/functions/after.js | 46 +
.../lodash-node/compat/functions/bind.js | 41 +
.../lodash-node/compat/functions/bindAll.js | 49 +
.../lodash-node/compat/functions/bindKey.js | 52 +
.../lodash-node/compat/functions/compose.js | 61 +
.../compat/functions/createCallback.js | 81 +
.../lodash-node/compat/functions/curry.js | 44 +
.../lodash-node/compat/functions/debounce.js | 156 +
.../lodash-node/compat/functions/defer.js | 35 +
.../lodash-node/compat/functions/delay.js | 36 +
.../lodash-node/compat/functions/memoize.js | 71 +
.../lodash-node/compat/functions/once.js | 48 +
.../lodash-node/compat/functions/partial.js | 34 +
.../compat/functions/partialRight.js | 43 +
.../lodash-node/compat/functions/throttle.js | 71 +
.../lodash-node/compat/functions/wrap.js | 36 +
node_modules/lodash-node/compat/index.js | 376 +
.../lodash-node/compat/internals/arrayPool.js | 13 +
.../lodash-node/compat/internals/baseBind.js | 62 +
.../lodash-node/compat/internals/baseClone.js | 154 +
.../lodash-node/compat/internals/baseCreate.js | 42 +
.../compat/internals/baseCreateCallback.js | 80 +
.../compat/internals/baseCreateWrapper.js | 78 +
.../compat/internals/baseDifference.js | 52 +
.../lodash-node/compat/internals/baseEach.js | 28 +
.../lodash-node/compat/internals/baseFlatten.js | 52 +
.../lodash-node/compat/internals/baseIndexOf.js | 32 +
.../lodash-node/compat/internals/baseIsEqual.js | 212 +
.../lodash-node/compat/internals/baseMerge.js | 79 +
.../lodash-node/compat/internals/baseRandom.js | 29 +
.../lodash-node/compat/internals/baseUniq.js | 64 +
.../compat/internals/cacheIndexOf.js | 39 +
.../lodash-node/compat/internals/cachePush.js | 38 +
.../compat/internals/charAtCallback.js | 22 +
.../compat/internals/compareAscending.js | 47 +
.../compat/internals/createAggregator.js | 45 +
.../lodash-node/compat/internals/createCache.js | 45 +
.../compat/internals/createIterator.js | 127 +
.../compat/internals/createWrapper.js | 106 +
.../compat/internals/defaultsIteratorOptions.js | 26 +
.../compat/internals/eachIteratorOptions.js | 20 +
.../compat/internals/escapeHtmlChar.js | 22 +
.../compat/internals/escapeStringChar.js | 33 +
.../compat/internals/forOwnIteratorOptions.js | 17 +
.../lodash-node/compat/internals/getArray.js | 21 +
.../lodash-node/compat/internals/getObject.js | 35 +
.../lodash-node/compat/internals/htmlEscapes.js | 26 +
.../compat/internals/htmlUnescapes.js | 15 +
.../compat/internals/indicatorObject.js | 13 +
.../lodash-node/compat/internals/isNative.js | 34 +
.../lodash-node/compat/internals/isNode.js | 23 +
.../compat/internals/iteratorTemplate.js | 109 +
.../lodash-node/compat/internals/keyPrefix.js | 13 +
.../compat/internals/largeArraySize.js | 13 +
.../compat/internals/lodashWrapper.js | 23 +
.../lodash-node/compat/internals/maxPoolSize.js | 13 +
.../lodash-node/compat/internals/objectPool.js | 13 +
.../lodash-node/compat/internals/objectTypes.js | 20 +
.../compat/internals/reEscapedHtml.js | 15 +
.../compat/internals/reInterpolate.js | 13 +
.../compat/internals/reUnescapedHtml.js | 15 +
.../compat/internals/releaseArray.js | 25 +
.../compat/internals/releaseObject.js | 29 +
.../lodash-node/compat/internals/setBindData.js | 43 +
.../compat/internals/shimIsPlainObject.js | 67 +
.../lodash-node/compat/internals/shimKeys.js | 27 +
.../lodash-node/compat/internals/slice.js | 38 +
.../compat/internals/unescapeHtmlChar.js | 22 +
node_modules/lodash-node/compat/objects.js | 52 +
.../lodash-node/compat/objects/assign.js | 55 +
.../lodash-node/compat/objects/clone.js | 63 +
.../lodash-node/compat/objects/cloneDeep.js | 57 +
.../lodash-node/compat/objects/create.js | 48 +
.../lodash-node/compat/objects/defaults.js | 34 +
.../lodash-node/compat/objects/findKey.js | 65 +
.../lodash-node/compat/objects/findLastKey.js | 65 +
.../lodash-node/compat/objects/forIn.js | 48 +
.../lodash-node/compat/objects/forInRight.js | 57 +
.../lodash-node/compat/objects/forOwn.js | 36 +
.../lodash-node/compat/objects/forOwnRight.js | 44 +
.../lodash-node/compat/objects/functions.js | 37 +
node_modules/lodash-node/compat/objects/has.js | 35 +
.../lodash-node/compat/objects/invert.js | 37 +
.../lodash-node/compat/objects/isArguments.js | 52 +
.../lodash-node/compat/objects/isArray.js | 45 +
.../lodash-node/compat/objects/isBoolean.js | 37 +
.../lodash-node/compat/objects/isDate.js | 36 +
.../lodash-node/compat/objects/isElement.js | 27 +
.../lodash-node/compat/objects/isEmpty.js | 66 +
.../lodash-node/compat/objects/isEqual.js | 54 +
.../lodash-node/compat/objects/isFinite.js | 46 +
.../lodash-node/compat/objects/isFunction.js | 42 +
.../lodash-node/compat/objects/isNaN.js | 42 +
.../lodash-node/compat/objects/isNull.js | 30 +
.../lodash-node/compat/objects/isNumber.js | 39 +
.../lodash-node/compat/objects/isObject.js | 39 +
.../lodash-node/compat/objects/isPlainObject.js | 62 +
.../lodash-node/compat/objects/isRegExp.js | 37 +
.../lodash-node/compat/objects/isString.js | 37 +
.../lodash-node/compat/objects/isUndefined.js | 27 +
node_modules/lodash-node/compat/objects/keys.js | 42 +
.../lodash-node/compat/objects/mapValues.js | 58 +
.../lodash-node/compat/objects/merge.js | 97 +
node_modules/lodash-node/compat/objects/omit.js | 67 +
.../lodash-node/compat/objects/pairs.js | 38 +
node_modules/lodash-node/compat/objects/pick.js | 65 +
.../lodash-node/compat/objects/transform.js | 67 +
.../lodash-node/compat/objects/values.js | 36 +
node_modules/lodash-node/compat/support.js | 177 +
node_modules/lodash-node/compat/utilities.js | 28 +
.../lodash-node/compat/utilities/constant.js | 31 +
.../lodash-node/compat/utilities/escape.js | 31 +
.../lodash-node/compat/utilities/identity.js | 28 +
.../lodash-node/compat/utilities/mixin.js | 88 +
.../lodash-node/compat/utilities/noConflict.js | 30 +
.../lodash-node/compat/utilities/noop.js | 26 +
.../lodash-node/compat/utilities/now.js | 28 +
.../lodash-node/compat/utilities/parseInt.js | 53 +
.../lodash-node/compat/utilities/property.js | 40 +
.../lodash-node/compat/utilities/random.js | 73 +
.../lodash-node/compat/utilities/result.js | 45 +
.../lodash-node/compat/utilities/template.js | 216 +
.../compat/utilities/templateSettings.js | 73 +
.../lodash-node/compat/utilities/times.js | 46 +
.../lodash-node/compat/utilities/unescape.js | 32 +
.../lodash-node/compat/utilities/uniqueId.js | 34 +
node_modules/lodash-node/modern/arrays.js | 40 +
.../lodash-node/modern/arrays/compact.js | 38 +
.../lodash-node/modern/arrays/difference.js | 31 +
.../lodash-node/modern/arrays/findIndex.js | 65 +
.../lodash-node/modern/arrays/findLastIndex.js | 63 +
node_modules/lodash-node/modern/arrays/first.js | 86 +
.../lodash-node/modern/arrays/flatten.js | 66 +
.../lodash-node/modern/arrays/indexOf.js | 50 +
.../lodash-node/modern/arrays/initial.js | 82 +
.../lodash-node/modern/arrays/intersection.js | 83 +
node_modules/lodash-node/modern/arrays/last.js | 84 +
.../lodash-node/modern/arrays/lastIndexOf.js | 54 +
node_modules/lodash-node/modern/arrays/pull.js | 57 +
node_modules/lodash-node/modern/arrays/range.js | 69 +
.../lodash-node/modern/arrays/remove.js | 71 +
node_modules/lodash-node/modern/arrays/rest.js | 83 +
.../lodash-node/modern/arrays/sortedIndex.js | 77 +
node_modules/lodash-node/modern/arrays/union.js | 30 +
node_modules/lodash-node/modern/arrays/uniq.js | 69 +
.../lodash-node/modern/arrays/without.js | 31 +
node_modules/lodash-node/modern/arrays/xor.js | 46 +
node_modules/lodash-node/modern/arrays/zip.js | 40 +
.../lodash-node/modern/arrays/zipObject.js | 48 +
node_modules/lodash-node/modern/chaining.js | 17 +
.../lodash-node/modern/chaining/chain.js | 41 +
node_modules/lodash-node/modern/chaining/tap.js | 35 +
.../lodash-node/modern/chaining/wrapperChain.js | 40 +
.../modern/chaining/wrapperToString.js | 26 +
.../modern/chaining/wrapperValueOf.js | 29 +
node_modules/lodash-node/modern/collections.js | 49 +
.../lodash-node/modern/collections/at.js | 46 +
.../lodash-node/modern/collections/contains.js | 65 +
.../lodash-node/modern/collections/countBy.js | 55 +
.../lodash-node/modern/collections/every.js | 74 +
.../lodash-node/modern/collections/filter.js | 76 +
.../lodash-node/modern/collections/find.js | 80 +
.../lodash-node/modern/collections/findLast.js | 44 +
.../lodash-node/modern/collections/forEach.js | 55 +
.../modern/collections/forEachRight.js | 52 +
.../lodash-node/modern/collections/groupBy.js | 56 +
.../lodash-node/modern/collections/indexBy.js | 54 +
.../lodash-node/modern/collections/invoke.js | 47 +
.../lodash-node/modern/collections/map.js | 70 +
.../lodash-node/modern/collections/max.js | 91 +
.../lodash-node/modern/collections/min.js | 91 +
.../lodash-node/modern/collections/pluck.js | 33 +
.../lodash-node/modern/collections/reduce.js | 67 +
.../modern/collections/reduceRight.js | 42 +
.../lodash-node/modern/collections/reject.js | 57 +
.../lodash-node/modern/collections/sample.js | 49 +
.../lodash-node/modern/collections/shuffle.js | 39 +
.../lodash-node/modern/collections/size.js | 36 +
.../lodash-node/modern/collections/some.js | 76 +
.../lodash-node/modern/collections/sortBy.js | 101 +
.../lodash-node/modern/collections/toArray.js | 33 +
.../lodash-node/modern/collections/where.js | 38 +
node_modules/lodash-node/modern/functions.js | 27 +
.../lodash-node/modern/functions/after.js | 46 +
.../lodash-node/modern/functions/bind.js | 40 +
.../lodash-node/modern/functions/bindAll.js | 49 +
.../lodash-node/modern/functions/bindKey.js | 52 +
.../lodash-node/modern/functions/compose.js | 61 +
.../modern/functions/createCallback.js | 81 +
.../lodash-node/modern/functions/curry.js | 44 +
.../lodash-node/modern/functions/debounce.js | 156 +
.../lodash-node/modern/functions/defer.js | 35 +
.../lodash-node/modern/functions/delay.js | 36 +
.../lodash-node/modern/functions/memoize.js | 71 +
.../lodash-node/modern/functions/once.js | 48 +
.../lodash-node/modern/functions/partial.js | 34 +
.../modern/functions/partialRight.js | 43 +
.../lodash-node/modern/functions/throttle.js | 71 +
.../lodash-node/modern/functions/wrap.js | 36 +
node_modules/lodash-node/modern/index.js | 354 +
.../lodash-node/modern/internals/arrayPool.js | 13 +
.../lodash-node/modern/internals/baseBind.js | 62 +
.../lodash-node/modern/internals/baseClone.js | 152 +
.../lodash-node/modern/internals/baseCreate.js | 42 +
.../modern/internals/baseCreateCallback.js | 80 +
.../modern/internals/baseCreateWrapper.js | 78 +
.../modern/internals/baseDifference.js | 52 +
.../lodash-node/modern/internals/baseFlatten.js | 52 +
.../lodash-node/modern/internals/baseIndexOf.js | 32 +
.../lodash-node/modern/internals/baseIsEqual.js | 209 +
.../lodash-node/modern/internals/baseMerge.js | 79 +
.../lodash-node/modern/internals/baseRandom.js | 29 +
.../lodash-node/modern/internals/baseUniq.js | 64 +
.../modern/internals/cacheIndexOf.js | 39 +
.../lodash-node/modern/internals/cachePush.js | 38 +
.../modern/internals/charAtCallback.js | 22 +
.../modern/internals/compareAscending.js | 47 +
.../modern/internals/createAggregator.js | 45 +
.../lodash-node/modern/internals/createCache.js | 45 +
.../modern/internals/createWrapper.js | 106 +
.../modern/internals/escapeHtmlChar.js | 22 +
.../modern/internals/escapeStringChar.js | 33 +
.../lodash-node/modern/internals/getArray.js | 21 +
.../lodash-node/modern/internals/getObject.js | 35 +
.../lodash-node/modern/internals/htmlEscapes.js | 26 +
.../modern/internals/htmlUnescapes.js | 15 +
.../lodash-node/modern/internals/isNative.js | 34 +
.../lodash-node/modern/internals/keyPrefix.js | 13 +
.../modern/internals/largeArraySize.js | 13 +
.../modern/internals/lodashWrapper.js | 23 +
.../lodash-node/modern/internals/maxPoolSize.js | 13 +
.../lodash-node/modern/internals/objectPool.js | 13 +
.../lodash-node/modern/internals/objectTypes.js | 20 +
.../modern/internals/reEscapedHtml.js | 15 +
.../modern/internals/reInterpolate.js | 13 +
.../modern/internals/reUnescapedHtml.js | 15 +
.../modern/internals/releaseArray.js | 25 +
.../modern/internals/releaseObject.js | 29 +
.../lodash-node/modern/internals/setBindData.js | 43 +
.../modern/internals/shimIsPlainObject.js | 52 +
.../lodash-node/modern/internals/shimKeys.js | 38 +
.../lodash-node/modern/internals/slice.js | 38 +
.../modern/internals/unescapeHtmlChar.js | 22 +
node_modules/lodash-node/modern/objects.js | 52 +
.../lodash-node/modern/objects/assign.js | 70 +
.../lodash-node/modern/objects/clone.js | 63 +
.../lodash-node/modern/objects/cloneDeep.js | 57 +
.../lodash-node/modern/objects/create.js | 48 +
.../lodash-node/modern/objects/defaults.js | 54 +
.../lodash-node/modern/objects/findKey.js | 65 +
.../lodash-node/modern/objects/findLastKey.js | 65 +
.../lodash-node/modern/objects/forIn.js | 54 +
.../lodash-node/modern/objects/forInRight.js | 57 +
.../lodash-node/modern/objects/forOwn.js | 50 +
.../lodash-node/modern/objects/forOwnRight.js | 44 +
.../lodash-node/modern/objects/functions.js | 37 +
node_modules/lodash-node/modern/objects/has.js | 35 +
.../lodash-node/modern/objects/invert.js | 37 +
.../lodash-node/modern/objects/isArguments.js | 40 +
.../lodash-node/modern/objects/isArray.js | 45 +
.../lodash-node/modern/objects/isBoolean.js | 37 +
.../lodash-node/modern/objects/isDate.js | 36 +
.../lodash-node/modern/objects/isElement.js | 27 +
.../lodash-node/modern/objects/isEmpty.js | 63 +
.../lodash-node/modern/objects/isEqual.js | 54 +
.../lodash-node/modern/objects/isFinite.js | 46 +
.../lodash-node/modern/objects/isFunction.js | 27 +
.../lodash-node/modern/objects/isNaN.js | 42 +
.../lodash-node/modern/objects/isNull.js | 30 +
.../lodash-node/modern/objects/isNumber.js | 39 +
.../lodash-node/modern/objects/isObject.js | 39 +
.../lodash-node/modern/objects/isPlainObject.js | 60 +
.../lodash-node/modern/objects/isRegExp.js | 36 +
.../lodash-node/modern/objects/isString.js | 37 +
.../lodash-node/modern/objects/isUndefined.js | 27 +
node_modules/lodash-node/modern/objects/keys.js | 36 +
.../lodash-node/modern/objects/mapValues.js | 58 +
.../lodash-node/modern/objects/merge.js | 97 +
node_modules/lodash-node/modern/objects/omit.js | 67 +
.../lodash-node/modern/objects/pairs.js | 38 +
node_modules/lodash-node/modern/objects/pick.js | 65 +
.../lodash-node/modern/objects/transform.js | 67 +
.../lodash-node/modern/objects/values.js | 36 +
node_modules/lodash-node/modern/support.js | 40 +
node_modules/lodash-node/modern/utilities.js | 28 +
.../lodash-node/modern/utilities/constant.js | 31 +
.../lodash-node/modern/utilities/escape.js | 31 +
.../lodash-node/modern/utilities/identity.js | 28 +
.../lodash-node/modern/utilities/mixin.js | 88 +
.../lodash-node/modern/utilities/noConflict.js | 30 +
.../lodash-node/modern/utilities/noop.js | 26 +
.../lodash-node/modern/utilities/now.js | 28 +
.../lodash-node/modern/utilities/parseInt.js | 53 +
.../lodash-node/modern/utilities/property.js | 40 +
.../lodash-node/modern/utilities/random.js | 73 +
.../lodash-node/modern/utilities/result.js | 45 +
.../lodash-node/modern/utilities/template.js | 216 +
.../modern/utilities/templateSettings.js | 73 +
.../lodash-node/modern/utilities/times.js | 46 +
.../lodash-node/modern/utilities/unescape.js | 32 +
.../lodash-node/modern/utilities/uniqueId.js | 34 +
node_modules/lodash-node/package.json | 111 +
node_modules/lodash-node/underscore/arrays.js | 35 +
.../lodash-node/underscore/arrays/compact.js | 38 +
.../lodash-node/underscore/arrays/difference.js | 31 +
.../lodash-node/underscore/arrays/first.js | 86 +
.../lodash-node/underscore/arrays/flatten.js | 56 +
.../lodash-node/underscore/arrays/indexOf.js | 50 +
.../lodash-node/underscore/arrays/initial.js | 82 +
.../underscore/arrays/intersection.js | 60 +
.../lodash-node/underscore/arrays/last.js | 84 +
.../underscore/arrays/lastIndexOf.js | 54 +
.../lodash-node/underscore/arrays/range.js | 69 +
.../lodash-node/underscore/arrays/rest.js | 83 +
.../underscore/arrays/sortedIndex.js | 77 +
.../lodash-node/underscore/arrays/union.js | 30 +
.../lodash-node/underscore/arrays/uniq.js | 69 +
.../lodash-node/underscore/arrays/without.js | 31 +
.../lodash-node/underscore/arrays/zip.js | 39 +
.../lodash-node/underscore/arrays/zipObject.js | 48 +
node_modules/lodash-node/underscore/chaining.js | 16 +
.../lodash-node/underscore/chaining/chain.js | 41 +
.../lodash-node/underscore/chaining/tap.js | 35 +
.../underscore/chaining/wrapperChain.js | 40 +
.../underscore/chaining/wrapperValueOf.js | 29 +
.../lodash-node/underscore/collections.js | 47 +
.../underscore/collections/contains.js | 54 +
.../underscore/collections/countBy.js | 55 +
.../lodash-node/underscore/collections/every.js | 75 +
.../underscore/collections/filter.js | 76 +
.../lodash-node/underscore/collections/find.js | 81 +
.../underscore/collections/findWhere.js | 38 +
.../underscore/collections/forEach.js | 55 +
.../underscore/collections/forEachRight.js | 51 +
.../underscore/collections/groupBy.js | 56 +
.../underscore/collections/indexBy.js | 54 +
.../underscore/collections/invoke.js | 47 +
.../lodash-node/underscore/collections/map.js | 70 +
.../lodash-node/underscore/collections/max.js | 86 +
.../lodash-node/underscore/collections/min.js | 86 +
.../lodash-node/underscore/collections/pluck.js | 33 +
.../underscore/collections/reduce.js | 67 +
.../underscore/collections/reduceRight.js | 42 +
.../underscore/collections/reject.js | 57 +
.../underscore/collections/sample.js | 49 +
.../underscore/collections/shuffle.js | 39 +
.../lodash-node/underscore/collections/size.js | 36 +
.../lodash-node/underscore/collections/some.js | 77 +
.../underscore/collections/sortBy.js | 86 +
.../underscore/collections/toArray.js | 37 +
.../lodash-node/underscore/collections/where.js | 44 +
.../lodash-node/underscore/functions.js | 24 +
.../lodash-node/underscore/functions/after.js | 46 +
.../lodash-node/underscore/functions/bind.js | 40 +
.../lodash-node/underscore/functions/bindAll.js | 49 +
.../lodash-node/underscore/functions/compose.js | 61 +
.../underscore/functions/createCallback.js | 67 +
.../underscore/functions/debounce.js | 156 +
.../lodash-node/underscore/functions/defer.js | 35 +
.../lodash-node/underscore/functions/delay.js | 36 +
.../lodash-node/underscore/functions/memoize.js | 65 +
.../lodash-node/underscore/functions/once.js | 48 +
.../lodash-node/underscore/functions/partial.js | 34 +
.../underscore/functions/throttle.js | 65 +
.../lodash-node/underscore/functions/wrap.js | 36 +
node_modules/lodash-node/underscore/index.js | 284 +
.../underscore/internals/baseBind.js | 60 +
.../underscore/internals/baseCreate.js | 42 +
.../underscore/internals/baseCreateCallback.js | 47 +
.../underscore/internals/baseCreateWrapper.js | 76 +
.../underscore/internals/baseDifference.js | 35 +
.../underscore/internals/baseFlatten.js | 52 +
.../underscore/internals/baseIndexOf.js | 32 +
.../underscore/internals/baseIsEqual.js | 149 +
.../underscore/internals/baseRandom.js | 29 +
.../underscore/internals/baseUniq.js | 45 +
.../underscore/internals/compareAscending.js | 47 +
.../underscore/internals/createAggregator.js | 45 +
.../underscore/internals/createWrapper.js | 60 +
.../underscore/internals/escapeHtmlChar.js | 22 +
.../underscore/internals/escapeStringChar.js | 33 +
.../underscore/internals/htmlEscapes.js | 26 +
.../underscore/internals/htmlUnescapes.js | 15 +
.../underscore/internals/indicatorObject.js | 13 +
.../underscore/internals/isNative.js | 34 +
.../underscore/internals/keyPrefix.js | 13 +
.../underscore/internals/lodashWrapper.js | 23 +
.../underscore/internals/objectTypes.js | 20 +
.../underscore/internals/reEscapedHtml.js | 15 +
.../underscore/internals/reInterpolate.js | 13 +
.../underscore/internals/reUnescapedHtml.js | 15 +
.../underscore/internals/shimKeys.js | 38 +
.../lodash-node/underscore/internals/slice.js | 38 +
.../underscore/internals/unescapeHtmlChar.js | 22 +
node_modules/lodash-node/underscore/objects.js | 42 +
.../lodash-node/underscore/objects/assign.js | 58 +
.../lodash-node/underscore/objects/clone.js | 61 +
.../lodash-node/underscore/objects/defaults.js | 49 +
.../lodash-node/underscore/objects/forIn.js | 54 +
.../lodash-node/underscore/objects/forOwn.js | 53 +
.../lodash-node/underscore/objects/functions.js | 37 +
.../lodash-node/underscore/objects/has.js | 35 +
.../lodash-node/underscore/objects/invert.js | 37 +
.../underscore/objects/isArguments.js | 51 +
.../lodash-node/underscore/objects/isArray.js | 45 +
.../lodash-node/underscore/objects/isBoolean.js | 37 +
.../lodash-node/underscore/objects/isDate.js | 36 +
.../lodash-node/underscore/objects/isElement.js | 27 +
.../lodash-node/underscore/objects/isEmpty.js | 54 +
.../lodash-node/underscore/objects/isEqual.js | 53 +
.../lodash-node/underscore/objects/isFinite.js | 46 +
.../underscore/objects/isFunction.js | 42 +
.../lodash-node/underscore/objects/isNaN.js | 42 +
.../lodash-node/underscore/objects/isNull.js | 30 +
.../lodash-node/underscore/objects/isNumber.js | 39 +
.../lodash-node/underscore/objects/isObject.js | 39 +
.../lodash-node/underscore/objects/isRegExp.js | 37 +
.../lodash-node/underscore/objects/isString.js | 37 +
.../underscore/objects/isUndefined.js | 27 +
.../lodash-node/underscore/objects/keys.js | 36 +
.../lodash-node/underscore/objects/omit.js | 57 +
.../lodash-node/underscore/objects/pairs.js | 38 +
.../lodash-node/underscore/objects/pick.js | 53 +
.../lodash-node/underscore/objects/values.js | 36 +
node_modules/lodash-node/underscore/support.js | 46 +
.../lodash-node/underscore/utilities.js | 26 +
.../lodash-node/underscore/utilities/escape.js | 31 +
.../underscore/utilities/identity.js | 28 +
.../lodash-node/underscore/utilities/mixin.js | 74 +
.../underscore/utilities/noConflict.js | 30 +
.../lodash-node/underscore/utilities/noop.js | 26 +
.../lodash-node/underscore/utilities/now.js | 28 +
.../underscore/utilities/property.js | 40 +
.../lodash-node/underscore/utilities/random.js | 58 +
.../lodash-node/underscore/utilities/result.js | 45 +
.../underscore/utilities/template.js | 163 +
.../underscore/utilities/templateSettings.js | 73 +
.../lodash-node/underscore/utilities/times.js | 46 +
.../underscore/utilities/unescape.js | 32 +
.../underscore/utilities/uniqueId.js | 34 +
node_modules/lodash/LICENSE | 22 +
node_modules/lodash/README.md | 121 +
node_modules/lodash/array.js | 44 +
node_modules/lodash/array/chunk.js | 46 +
node_modules/lodash/array/compact.js | 30 +
node_modules/lodash/array/difference.js | 29 +
node_modules/lodash/array/drop.js | 39 +
node_modules/lodash/array/dropRight.js | 40 +
node_modules/lodash/array/dropRightWhile.js | 59 +
node_modules/lodash/array/dropWhile.js | 59 +
node_modules/lodash/array/fill.js | 44 +
node_modules/lodash/array/findIndex.js | 53 +
node_modules/lodash/array/findLastIndex.js | 53 +
node_modules/lodash/array/first.js | 22 +
node_modules/lodash/array/flatten.js | 32 +
node_modules/lodash/array/flattenDeep.js | 21 +
node_modules/lodash/array/head.js | 1 +
node_modules/lodash/array/indexOf.js | 53 +
node_modules/lodash/array/initial.js | 20 +
node_modules/lodash/array/intersection.js | 58 +
node_modules/lodash/array/last.js | 19 +
node_modules/lodash/array/lastIndexOf.js | 60 +
node_modules/lodash/array/object.js | 1 +
node_modules/lodash/array/pull.js | 52 +
node_modules/lodash/array/pullAt.js | 40 +
node_modules/lodash/array/remove.js | 64 +
node_modules/lodash/array/rest.js | 21 +
node_modules/lodash/array/slice.js | 30 +
node_modules/lodash/array/sortedIndex.js | 53 +
node_modules/lodash/array/sortedLastIndex.js | 25 +
node_modules/lodash/array/tail.js | 1 +
node_modules/lodash/array/take.js | 39 +
node_modules/lodash/array/takeRight.js | 40 +
node_modules/lodash/array/takeRightWhile.js | 59 +
node_modules/lodash/array/takeWhile.js | 59 +
node_modules/lodash/array/union.js | 24 +
node_modules/lodash/array/uniq.js | 71 +
node_modules/lodash/array/unique.js | 1 +
node_modules/lodash/array/unzip.js | 47 +
node_modules/lodash/array/unzipWith.js | 41 +
node_modules/lodash/array/without.js | 27 +
node_modules/lodash/array/xor.js | 35 +
node_modules/lodash/array/zip.js | 21 +
node_modules/lodash/array/zipObject.js | 43 +
node_modules/lodash/array/zipWith.js | 36 +
node_modules/lodash/chain.js | 16 +
node_modules/lodash/chain/chain.js | 35 +
node_modules/lodash/chain/commit.js | 1 +
node_modules/lodash/chain/concat.js | 1 +
node_modules/lodash/chain/lodash.js | 125 +
node_modules/lodash/chain/plant.js | 1 +
node_modules/lodash/chain/reverse.js | 1 +
node_modules/lodash/chain/run.js | 1 +
node_modules/lodash/chain/tap.js | 29 +
node_modules/lodash/chain/thru.js | 26 +
node_modules/lodash/chain/toJSON.js | 1 +
node_modules/lodash/chain/toString.js | 1 +
node_modules/lodash/chain/value.js | 1 +
node_modules/lodash/chain/valueOf.js | 1 +
node_modules/lodash/chain/wrapperChain.js | 32 +
node_modules/lodash/chain/wrapperCommit.js | 32 +
node_modules/lodash/chain/wrapperConcat.js | 34 +
node_modules/lodash/chain/wrapperPlant.js | 45 +
node_modules/lodash/chain/wrapperReverse.js | 43 +
node_modules/lodash/chain/wrapperToString.js | 17 +
node_modules/lodash/chain/wrapperValue.js | 20 +
node_modules/lodash/collection.js | 44 +
node_modules/lodash/collection/all.js | 1 +
node_modules/lodash/collection/any.js | 1 +
node_modules/lodash/collection/at.js | 29 +
node_modules/lodash/collection/collect.js | 1 +
node_modules/lodash/collection/contains.js | 1 +
node_modules/lodash/collection/countBy.js | 54 +
node_modules/lodash/collection/detect.js | 1 +
node_modules/lodash/collection/each.js | 1 +
node_modules/lodash/collection/eachRight.js | 1 +
node_modules/lodash/collection/every.js | 66 +
node_modules/lodash/collection/filter.js | 61 +
node_modules/lodash/collection/find.js | 56 +
node_modules/lodash/collection/findLast.js | 25 +
node_modules/lodash/collection/findWhere.js | 37 +
node_modules/lodash/collection/foldl.js | 1 +
node_modules/lodash/collection/foldr.js | 1 +
node_modules/lodash/collection/forEach.js | 37 +
node_modules/lodash/collection/forEachRight.js | 26 +
node_modules/lodash/collection/groupBy.js | 59 +
node_modules/lodash/collection/include.js | 1 +
node_modules/lodash/collection/includes.js | 57 +
node_modules/lodash/collection/indexBy.js | 53 +
node_modules/lodash/collection/inject.js | 1 +
node_modules/lodash/collection/invoke.js | 42 +
node_modules/lodash/collection/map.js | 68 +
node_modules/lodash/collection/max.js | 1 +
node_modules/lodash/collection/min.js | 1 +
node_modules/lodash/collection/partition.js | 66 +
node_modules/lodash/collection/pluck.js | 31 +
node_modules/lodash/collection/reduce.js | 44 +
node_modules/lodash/collection/reduceRight.js | 29 +
node_modules/lodash/collection/reject.js | 50 +
node_modules/lodash/collection/sample.js | 50 +
node_modules/lodash/collection/select.js | 1 +
node_modules/lodash/collection/shuffle.js | 24 +
node_modules/lodash/collection/size.js | 30 +
node_modules/lodash/collection/some.js | 67 +
node_modules/lodash/collection/sortBy.js | 71 +
node_modules/lodash/collection/sortByAll.js | 52 +
node_modules/lodash/collection/sortByOrder.js | 55 +
node_modules/lodash/collection/sum.js | 1 +
node_modules/lodash/collection/where.js | 37 +
node_modules/lodash/date.js | 3 +
node_modules/lodash/date/now.js | 24 +
node_modules/lodash/function.js | 28 +
node_modules/lodash/function/after.js | 48 +
node_modules/lodash/function/ary.js | 34 +
node_modules/lodash/function/backflow.js | 1 +
node_modules/lodash/function/before.js | 42 +
node_modules/lodash/function/bind.js | 56 +
node_modules/lodash/function/bindAll.js | 50 +
node_modules/lodash/function/bindKey.js | 66 +
node_modules/lodash/function/compose.js | 1 +
node_modules/lodash/function/curry.js | 51 +
node_modules/lodash/function/curryRight.js | 48 +
node_modules/lodash/function/debounce.js | 181 +
node_modules/lodash/function/defer.js | 25 +
node_modules/lodash/function/delay.js | 26 +
node_modules/lodash/function/flow.js | 25 +
node_modules/lodash/function/flowRight.js | 25 +
node_modules/lodash/function/memoize.js | 80 +
node_modules/lodash/function/modArgs.js | 58 +
node_modules/lodash/function/negate.js | 32 +
node_modules/lodash/function/once.js | 24 +
node_modules/lodash/function/partial.js | 43 +
node_modules/lodash/function/partialRight.js | 42 +
node_modules/lodash/function/rearg.js | 40 +
node_modules/lodash/function/restParam.js | 58 +
node_modules/lodash/function/spread.js | 44 +
node_modules/lodash/function/throttle.js | 62 +
node_modules/lodash/function/wrap.js | 33 +
node_modules/lodash/index.js | 12351 ++++++++++++++
node_modules/lodash/internal/LazyWrapper.js | 26 +
node_modules/lodash/internal/LodashWrapper.js | 21 +
node_modules/lodash/internal/MapCache.js | 24 +
node_modules/lodash/internal/SetCache.js | 29 +
node_modules/lodash/internal/arrayConcat.js | 25 +
node_modules/lodash/internal/arrayCopy.js | 20 +
node_modules/lodash/internal/arrayEach.js | 22 +
node_modules/lodash/internal/arrayEachRight.js | 21 +
node_modules/lodash/internal/arrayEvery.js | 23 +
node_modules/lodash/internal/arrayExtremum.js | 30 +
node_modules/lodash/internal/arrayFilter.js | 25 +
node_modules/lodash/internal/arrayMap.js | 21 +
node_modules/lodash/internal/arrayPush.js | 20 +
node_modules/lodash/internal/arrayReduce.js | 26 +
.../lodash/internal/arrayReduceRight.js | 24 +
node_modules/lodash/internal/arraySome.js | 23 +
node_modules/lodash/internal/arraySum.js | 20 +
node_modules/lodash/internal/assignDefaults.js | 13 +
.../lodash/internal/assignOwnDefaults.js | 26 +
node_modules/lodash/internal/assignWith.js | 32 +
node_modules/lodash/internal/baseAssign.js | 19 +
node_modules/lodash/internal/baseAt.js | 32 +
node_modules/lodash/internal/baseCallback.js | 35 +
node_modules/lodash/internal/baseClone.js | 128 +
.../lodash/internal/baseCompareAscending.js | 34 +
node_modules/lodash/internal/baseCopy.js | 23 +
node_modules/lodash/internal/baseCreate.js | 23 +
node_modules/lodash/internal/baseDelay.js | 21 +
node_modules/lodash/internal/baseDifference.js | 55 +
node_modules/lodash/internal/baseEach.js | 15 +
node_modules/lodash/internal/baseEachRight.js | 15 +
node_modules/lodash/internal/baseEvery.js | 22 +
node_modules/lodash/internal/baseExtremum.js | 29 +
node_modules/lodash/internal/baseFill.js | 31 +
node_modules/lodash/internal/baseFilter.js | 22 +
node_modules/lodash/internal/baseFind.js | 25 +
node_modules/lodash/internal/baseFindIndex.js | 23 +
node_modules/lodash/internal/baseFlatten.js | 41 +
node_modules/lodash/internal/baseFor.js | 17 +
node_modules/lodash/internal/baseForIn.js | 17 +
node_modules/lodash/internal/baseForOwn.js | 17 +
node_modules/lodash/internal/baseForOwnRight.js | 17 +
node_modules/lodash/internal/baseForRight.js | 15 +
node_modules/lodash/internal/baseFunctions.js | 27 +
node_modules/lodash/internal/baseGet.js | 29 +
node_modules/lodash/internal/baseIndexOf.js | 27 +
node_modules/lodash/internal/baseIsEqual.js | 28 +
node_modules/lodash/internal/baseIsEqualDeep.js | 102 +
node_modules/lodash/internal/baseIsFunction.js | 15 +
node_modules/lodash/internal/baseIsMatch.js | 52 +
node_modules/lodash/internal/baseLodash.js | 10 +
node_modules/lodash/internal/baseMap.js | 23 +
node_modules/lodash/internal/baseMatches.js | 30 +
.../lodash/internal/baseMatchesProperty.js | 45 +
node_modules/lodash/internal/baseMerge.js | 56 +
node_modules/lodash/internal/baseMergeDeep.js | 67 +
node_modules/lodash/internal/baseProperty.js | 14 +
.../lodash/internal/basePropertyDeep.js | 19 +
node_modules/lodash/internal/basePullAt.js | 30 +
node_modules/lodash/internal/baseRandom.js | 18 +
node_modules/lodash/internal/baseReduce.js | 24 +
node_modules/lodash/internal/baseSetData.js | 17 +
node_modules/lodash/internal/baseSlice.js | 32 +
node_modules/lodash/internal/baseSome.js | 23 +
node_modules/lodash/internal/baseSortBy.js | 21 +
node_modules/lodash/internal/baseSortByOrder.js | 31 +
node_modules/lodash/internal/baseSum.js | 20 +
node_modules/lodash/internal/baseToString.js | 13 +
node_modules/lodash/internal/baseUniq.js | 60 +
node_modules/lodash/internal/baseValues.js | 22 +
node_modules/lodash/internal/baseWhile.js | 24 +
.../lodash/internal/baseWrapperValue.js | 29 +
node_modules/lodash/internal/binaryIndex.js | 39 +
node_modules/lodash/internal/binaryIndexBy.js | 57 +
node_modules/lodash/internal/bindCallback.js | 39 +
node_modules/lodash/internal/bufferClone.js | 20 +
node_modules/lodash/internal/cacheIndexOf.js | 19 +
node_modules/lodash/internal/cachePush.js | 20 +
node_modules/lodash/internal/charsLeftIndex.js | 18 +
node_modules/lodash/internal/charsRightIndex.js | 17 +
.../lodash/internal/compareAscending.js | 16 +
node_modules/lodash/internal/compareMultiple.js | 44 +
node_modules/lodash/internal/composeArgs.js | 34 +
.../lodash/internal/composeArgsRight.js | 36 +
.../lodash/internal/createAggregator.js | 35 +
node_modules/lodash/internal/createAssigner.js | 41 +
node_modules/lodash/internal/createBaseEach.js | 31 +
node_modules/lodash/internal/createBaseFor.js | 27 +
.../lodash/internal/createBindWrapper.js | 22 +
node_modules/lodash/internal/createCache.js | 21 +
.../lodash/internal/createCompounder.js | 26 +
.../lodash/internal/createCtorWrapper.js | 37 +
node_modules/lodash/internal/createCurry.js | 23 +
node_modules/lodash/internal/createDefaults.js | 22 +
node_modules/lodash/internal/createExtremum.js | 33 +
node_modules/lodash/internal/createFind.js | 25 +
node_modules/lodash/internal/createFindIndex.js | 21 +
node_modules/lodash/internal/createFindKey.js | 18 +
node_modules/lodash/internal/createFlow.js | 74 +
node_modules/lodash/internal/createForEach.js | 20 +
node_modules/lodash/internal/createForIn.js | 20 +
node_modules/lodash/internal/createForOwn.js | 19 +
.../lodash/internal/createHybridWrapper.js | 111 +
.../lodash/internal/createObjectMapper.js | 26 +
node_modules/lodash/internal/createPadDir.js | 18 +
node_modules/lodash/internal/createPadding.js | 29 +
node_modules/lodash/internal/createPartial.js | 20 +
.../lodash/internal/createPartialWrapper.js | 43 +
node_modules/lodash/internal/createReduce.js | 22 +
node_modules/lodash/internal/createRound.js | 23 +
.../lodash/internal/createSortedIndex.js | 20 +
node_modules/lodash/internal/createWrapper.js | 86 +
node_modules/lodash/internal/deburrLetter.js | 33 +
node_modules/lodash/internal/equalArrays.js | 51 +
node_modules/lodash/internal/equalByTag.js | 48 +
node_modules/lodash/internal/equalObjects.js | 67 +
node_modules/lodash/internal/escapeHtmlChar.js | 22 +
.../lodash/internal/escapeRegExpChar.js | 38 +
.../lodash/internal/escapeStringChar.js | 22 +
node_modules/lodash/internal/getData.js | 15 +
node_modules/lodash/internal/getFuncName.js | 25 +
node_modules/lodash/internal/getLength.js | 15 +
node_modules/lodash/internal/getMatchData.js | 21 +
node_modules/lodash/internal/getNative.js | 16 +
node_modules/lodash/internal/getView.js | 33 +
node_modules/lodash/internal/indexOfNaN.js | 23 +
node_modules/lodash/internal/initCloneArray.js | 26 +
node_modules/lodash/internal/initCloneByTag.js | 63 +
node_modules/lodash/internal/initCloneObject.js | 16 +
node_modules/lodash/internal/invokePath.js | 26 +
node_modules/lodash/internal/isArrayLike.js | 15 +
node_modules/lodash/internal/isIndex.js | 24 +
node_modules/lodash/internal/isIterateeCall.js | 28 +
node_modules/lodash/internal/isKey.js | 28 +
node_modules/lodash/internal/isLaziable.js | 27 +
node_modules/lodash/internal/isLength.js | 20 +
node_modules/lodash/internal/isObjectLike.js | 12 +
node_modules/lodash/internal/isSpace.js | 14 +
.../lodash/internal/isStrictComparable.js | 15 +
node_modules/lodash/internal/lazyClone.js | 23 +
node_modules/lodash/internal/lazyReverse.js | 23 +
node_modules/lodash/internal/lazyValue.js | 72 +
node_modules/lodash/internal/mapDelete.js | 14 +
node_modules/lodash/internal/mapGet.js | 14 +
node_modules/lodash/internal/mapHas.js | 20 +
node_modules/lodash/internal/mapSet.js | 18 +
node_modules/lodash/internal/mergeData.js | 89 +
node_modules/lodash/internal/mergeDefaults.js | 15 +
node_modules/lodash/internal/metaMap.js | 9 +
node_modules/lodash/internal/pickByArray.js | 28 +
node_modules/lodash/internal/pickByCallback.js | 22 +
node_modules/lodash/internal/reEscape.js | 4 +
node_modules/lodash/internal/reEvaluate.js | 4 +
node_modules/lodash/internal/reInterpolate.js | 4 +
node_modules/lodash/internal/realNames.js | 4 +
node_modules/lodash/internal/reorder.js | 29 +
node_modules/lodash/internal/replaceHolders.js | 28 +
node_modules/lodash/internal/setData.js | 41 +
node_modules/lodash/internal/shimKeys.js | 41 +
node_modules/lodash/internal/sortedUniq.js | 29 +
node_modules/lodash/internal/toIterable.js | 22 +
node_modules/lodash/internal/toObject.js | 14 +
node_modules/lodash/internal/toPath.js | 28 +
.../lodash/internal/trimmedLeftIndex.js | 19 +
.../lodash/internal/trimmedRightIndex.js | 18 +
.../lodash/internal/unescapeHtmlChar.js | 22 +
node_modules/lodash/internal/wrapperClone.js | 18 +
node_modules/lodash/lang.js | 32 +
node_modules/lodash/lang/clone.js | 70 +
node_modules/lodash/lang/cloneDeep.js | 55 +
node_modules/lodash/lang/eq.js | 1 +
node_modules/lodash/lang/gt.js | 25 +
node_modules/lodash/lang/gte.js | 25 +
node_modules/lodash/lang/isArguments.js | 34 +
node_modules/lodash/lang/isArray.js | 40 +
node_modules/lodash/lang/isBoolean.js | 35 +
node_modules/lodash/lang/isDate.js | 35 +
node_modules/lodash/lang/isElement.js | 24 +
node_modules/lodash/lang/isEmpty.js | 47 +
node_modules/lodash/lang/isEqual.js | 54 +
node_modules/lodash/lang/isError.js | 36 +
node_modules/lodash/lang/isFinite.js | 35 +
node_modules/lodash/lang/isFunction.js | 38 +
node_modules/lodash/lang/isMatch.js | 49 +
node_modules/lodash/lang/isNaN.js | 34 +
node_modules/lodash/lang/isNative.js | 48 +
node_modules/lodash/lang/isNull.js | 21 +
node_modules/lodash/lang/isNumber.js | 41 +
node_modules/lodash/lang/isObject.js | 28 +
node_modules/lodash/lang/isPlainObject.js | 71 +
node_modules/lodash/lang/isRegExp.js | 35 +
node_modules/lodash/lang/isString.js | 35 +
node_modules/lodash/lang/isTypedArray.js | 74 +
node_modules/lodash/lang/isUndefined.js | 21 +
node_modules/lodash/lang/lt.js | 25 +
node_modules/lodash/lang/lte.js | 25 +
node_modules/lodash/lang/toArray.js | 32 +
node_modules/lodash/lang/toPlainObject.js | 31 +
node_modules/lodash/math.js | 9 +
node_modules/lodash/math/add.js | 19 +
node_modules/lodash/math/ceil.js | 25 +
node_modules/lodash/math/floor.js | 25 +
node_modules/lodash/math/max.js | 56 +
node_modules/lodash/math/min.js | 56 +
node_modules/lodash/math/round.js | 25 +
node_modules/lodash/math/sum.js | 50 +
node_modules/lodash/number.js | 4 +
node_modules/lodash/number/inRange.js | 47 +
node_modules/lodash/number/random.js | 70 +
node_modules/lodash/object.js | 31 +
node_modules/lodash/object/assign.js | 43 +
node_modules/lodash/object/create.js | 47 +
node_modules/lodash/object/defaults.js | 25 +
node_modules/lodash/object/defaultsDeep.js | 25 +
node_modules/lodash/object/extend.js | 1 +
node_modules/lodash/object/findKey.js | 54 +
node_modules/lodash/object/findLastKey.js | 54 +
node_modules/lodash/object/forIn.js | 33 +
node_modules/lodash/object/forInRight.js | 31 +
node_modules/lodash/object/forOwn.js | 33 +
node_modules/lodash/object/forOwnRight.js | 31 +
node_modules/lodash/object/functions.js | 23 +
node_modules/lodash/object/get.js | 33 +
node_modules/lodash/object/has.js | 57 +
node_modules/lodash/object/invert.js | 60 +
node_modules/lodash/object/keys.js | 45 +
node_modules/lodash/object/keysIn.js | 64 +
node_modules/lodash/object/mapKeys.js | 25 +
node_modules/lodash/object/mapValues.js | 46 +
node_modules/lodash/object/merge.js | 54 +
node_modules/lodash/object/methods.js | 1 +
node_modules/lodash/object/omit.js | 47 +
node_modules/lodash/object/pairs.js | 33 +
node_modules/lodash/object/pick.js | 42 +
node_modules/lodash/object/result.js | 49 +
node_modules/lodash/object/set.js | 55 +
node_modules/lodash/object/transform.js | 61 +
node_modules/lodash/object/values.js | 33 +
node_modules/lodash/object/valuesIn.js | 31 +
node_modules/lodash/package.json | 121 +
node_modules/lodash/string.js | 25 +
node_modules/lodash/string/camelCase.js | 27 +
node_modules/lodash/string/capitalize.js | 21 +
node_modules/lodash/string/deburr.js | 29 +
node_modules/lodash/string/endsWith.js | 40 +
node_modules/lodash/string/escape.js | 48 +
node_modules/lodash/string/escapeRegExp.js | 32 +
node_modules/lodash/string/kebabCase.js | 26 +
node_modules/lodash/string/pad.js | 47 +
node_modules/lodash/string/padLeft.js | 27 +
node_modules/lodash/string/padRight.js | 27 +
node_modules/lodash/string/parseInt.js | 46 +
node_modules/lodash/string/repeat.js | 47 +
node_modules/lodash/string/snakeCase.js | 26 +
node_modules/lodash/string/startCase.js | 26 +
node_modules/lodash/string/startsWith.js | 36 +
node_modules/lodash/string/template.js | 226 +
node_modules/lodash/string/templateSettings.js | 67 +
node_modules/lodash/string/trim.js | 42 +
node_modules/lodash/string/trimLeft.js | 36 +
node_modules/lodash/string/trimRight.js | 36 +
node_modules/lodash/string/trunc.js | 105 +
node_modules/lodash/string/unescape.js | 33 +
node_modules/lodash/string/words.js | 38 +
node_modules/lodash/support.js | 10 +
node_modules/lodash/utility.js | 18 +
node_modules/lodash/utility/attempt.js | 32 +
node_modules/lodash/utility/callback.js | 53 +
node_modules/lodash/utility/constant.js | 23 +
node_modules/lodash/utility/identity.js | 20 +
node_modules/lodash/utility/iteratee.js | 1 +
node_modules/lodash/utility/matches.js | 33 +
node_modules/lodash/utility/matchesProperty.js | 32 +
node_modules/lodash/utility/method.js | 33 +
node_modules/lodash/utility/methodOf.js | 32 +
node_modules/lodash/utility/mixin.js | 82 +
node_modules/lodash/utility/noop.js | 19 +
node_modules/lodash/utility/property.js | 31 +
node_modules/lodash/utility/propertyOf.js | 30 +
node_modules/lodash/utility/range.js | 66 +
node_modules/lodash/utility/times.js | 60 +
node_modules/lodash/utility/uniqueId.js | 27 +
node_modules/minimatch/LICENSE | 15 +
node_modules/minimatch/README.md | 216 +
node_modules/minimatch/minimatch.js | 912 +
node_modules/minimatch/package.json | 85 +
node_modules/node-uuid/.npmignore | 2 +
node_modules/node-uuid/LICENSE.md | 3 +
node_modules/node-uuid/README.md | 199 +
node_modules/node-uuid/benchmark/README.md | 53 +
node_modules/node-uuid/benchmark/bench.gnu | 174 +
node_modules/node-uuid/benchmark/bench.sh | 34 +
.../node-uuid/benchmark/benchmark-native.c | 34 +
node_modules/node-uuid/benchmark/benchmark.js | 84 +
node_modules/node-uuid/package.json | 85 +
node_modules/node-uuid/test/compare_v1.js | 63 +
node_modules/node-uuid/test/test.html | 17 +
node_modules/node-uuid/test/test.js | 240 +
node_modules/node-uuid/uuid.js | 249 +
node_modules/nopt/.npmignore | 1 +
node_modules/nopt/.travis.yml | 9 +
node_modules/nopt/LICENSE | 15 +
node_modules/nopt/README.md | 211 +
node_modules/nopt/bin/nopt.js | 54 +
node_modules/nopt/examples/my-program.js | 30 +
node_modules/nopt/lib/nopt.js | 415 +
node_modules/nopt/package.json | 88 +
node_modules/nopt/test/basic.js | 273 +
node_modules/once/LICENSE | 15 +
node_modules/once/README.md | 51 +
node_modules/once/once.js | 21 +
node_modules/once/package.json | 89 +
node_modules/os-homedir/index.js | 24 +
node_modules/os-homedir/license | 21 +
node_modules/os-homedir/package.json | 96 +
node_modules/os-homedir/readme.md | 33 +
node_modules/os-tmpdir/index.js | 25 +
node_modules/os-tmpdir/license | 21 +
node_modules/os-tmpdir/package.json | 96 +
node_modules/os-tmpdir/readme.md | 36 +
node_modules/osenv/.npmignore | 13 +
node_modules/osenv/.travis.yml | 9 +
node_modules/osenv/LICENSE | 15 +
node_modules/osenv/README.md | 63 +
node_modules/osenv/osenv.js | 72 +
node_modules/osenv/package.json | 101 +
node_modules/osenv/test/unix.js | 71 +
node_modules/osenv/test/windows.js | 74 +
node_modules/osenv/x.tap | 39 +
node_modules/path-is-absolute/index.js | 20 +
node_modules/path-is-absolute/license | 21 +
node_modules/path-is-absolute/package.json | 97 +
node_modules/path-is-absolute/readme.md | 51 +
node_modules/pegjs/CHANGELOG | 146 +
node_modules/pegjs/LICENSE | 22 +
node_modules/pegjs/README.md | 226 +
node_modules/pegjs/VERSION | 1 +
node_modules/pegjs/bin/pegjs | 142 +
node_modules/pegjs/examples/arithmetics.pegjs | 22 +
node_modules/pegjs/examples/css.pegjs | 554 +
node_modules/pegjs/examples/javascript.pegjs | 1530 ++
node_modules/pegjs/examples/json.pegjs | 120 +
node_modules/pegjs/lib/peg.js | 5141 ++++++
node_modules/pegjs/package.json | 82 +
node_modules/plist/.jshintrc | 4 +
node_modules/plist/.travis.yml | 34 +
node_modules/plist/History.md | 122 +
node_modules/plist/LICENSE | 24 +
node_modules/plist/Makefile | 76 +
node_modules/plist/README.md | 113 +
node_modules/plist/dist/plist-build.js | 3982 +++++
node_modules/plist/dist/plist-parse.js | 4055 +++++
node_modules/plist/dist/plist.js | 7987 +++++++++
node_modules/plist/examples/browser/index.html | 14 +
node_modules/plist/lib/build.js | 138 +
node_modules/plist/lib/node.js | 49 +
node_modules/plist/lib/parse.js | 200 +
node_modules/plist/lib/plist.js | 23 +
node_modules/plist/package.json | 117 +
node_modules/q/CHANGES.md | 786 +
node_modules/q/LICENSE | 18 +
node_modules/q/README.md | 881 +
node_modules/q/package.json | 146 +
node_modules/q/q.js | 2048 +++
node_modules/q/queue.js | 35 +
node_modules/sax/AUTHORS | 9 +
node_modules/sax/LICENSE | 23 +
node_modules/sax/README.md | 213 +
node_modules/sax/examples/big-not-pretty.xml | 8002 +++++++++
node_modules/sax/examples/example.js | 41 +
node_modules/sax/examples/get-products.js | 58 +
node_modules/sax/examples/hello-world.js | 4 +
node_modules/sax/examples/not-pretty.xml | 8 +
node_modules/sax/examples/pretty-print.js | 74 +
node_modules/sax/examples/shopping.xml | 2 +
node_modules/sax/examples/strict.dtd | 870 +
node_modules/sax/examples/switch-bench.js | 45 +
node_modules/sax/examples/test.html | 15 +
node_modules/sax/examples/test.xml | 1254 ++
node_modules/sax/lib/sax.js | 1006 ++
node_modules/sax/package.json | 114 +
node_modules/sax/test/buffer-overrun.js | 25 +
node_modules/sax/test/cdata-chunked.js | 11 +
node_modules/sax/test/cdata-end-split.js | 15 +
node_modules/sax/test/cdata-fake-end.js | 28 +
node_modules/sax/test/cdata-multiple.js | 15 +
node_modules/sax/test/cdata.js | 10 +
node_modules/sax/test/index.js | 86 +
node_modules/sax/test/issue-23.js | 43 +
node_modules/sax/test/issue-30.js | 24 +
node_modules/sax/test/issue-35.js | 15 +
node_modules/sax/test/issue-47.js | 13 +
node_modules/sax/test/issue-49.js | 31 +
node_modules/sax/test/parser-position.js | 28 +
node_modules/sax/test/script.js | 12 +
.../sax/test/self-closing-child-strict.js | 40 +
node_modules/sax/test/self-closing-child.js | 40 +
node_modules/sax/test/self-closing-tag.js | 25 +
node_modules/sax/test/stray-ending.js | 17 +
.../sax/test/trailing-non-whitespace.js | 17 +
node_modules/sax/test/unquoted.js | 17 +
node_modules/sax/test/xmlns-issue-41.js | 67 +
node_modules/sax/test/xmlns-rebinding.js | 59 +
node_modules/sax/test/xmlns-strict.js | 71 +
node_modules/sax/test/xmlns-unbound.js | 15 +
.../test/xmlns-xml-default-prefix-attribute.js | 35 +
.../sax/test/xmlns-xml-default-prefix.js | 20 +
.../sax/test/xmlns-xml-default-redefine.js | 40 +
node_modules/semver/.npmignore | 4 +
node_modules/semver/.travis.yml | 5 +
node_modules/semver/LICENSE | 15 +
node_modules/semver/README.md | 327 +
node_modules/semver/bin/semver | 133 +
node_modules/semver/package.json | 77 +
node_modules/semver/range.bnf | 16 +
node_modules/semver/semver.js | 1188 ++
node_modules/semver/test/big-numbers.js | 31 +
node_modules/semver/test/clean.js | 29 +
node_modules/semver/test/gtr.js | 173 +
node_modules/semver/test/index.js | 698 +
node_modules/semver/test/ltr.js | 181 +
node_modules/semver/test/major-minor-patch.js | 72 +
node_modules/shelljs/.idea/.name | 1 +
node_modules/shelljs/.idea/encodings.xml | 6 +
.../inspectionProfiles/Project_Default.xml | 7 +
.../inspectionProfiles/profiles_settings.xml | 7 +
.../shelljs/.idea/jsLibraryMappings.xml | 6 +
.../.idea/libraries/shelljs_node_modules.xml | 14 +
node_modules/shelljs/.idea/misc.xml | 28 +
node_modules/shelljs/.idea/modules.xml | 8 +
node_modules/shelljs/.idea/shelljs.iml | 9 +
node_modules/shelljs/.idea/vcs.xml | 6 +
node_modules/shelljs/.idea/workspace.xml | 764 +
node_modules/shelljs/.npmignore | 9 +
node_modules/shelljs/LICENSE | 26 +
node_modules/shelljs/MAINTAINERS | 3 +
node_modules/shelljs/README.md | 658 +
node_modules/shelljs/bin/shjs | 55 +
node_modules/shelljs/build/output.js | 2411 +++
node_modules/shelljs/global.js | 3 +
node_modules/shelljs/make.js | 57 +
node_modules/shelljs/package.json | 112 +
node_modules/shelljs/scripts/generate-docs.js | 26 +
node_modules/shelljs/scripts/run-tests.js | 55 +
node_modules/shelljs/shell.js | 184 +
node_modules/shelljs/src/cat.js | 40 +
node_modules/shelljs/src/cd.js | 28 +
node_modules/shelljs/src/chmod.js | 215 +
node_modules/shelljs/src/common.js | 257 +
node_modules/shelljs/src/cp.js | 210 +
node_modules/shelljs/src/dirs.js | 191 +
node_modules/shelljs/src/echo.js | 20 +
node_modules/shelljs/src/error.js | 10 +
node_modules/shelljs/src/exec.js | 249 +
node_modules/shelljs/src/find.js | 51 +
node_modules/shelljs/src/grep.js | 52 +
node_modules/shelljs/src/ln.js | 69 +
node_modules/shelljs/src/ls.js | 168 +
node_modules/shelljs/src/mkdir.js | 68 +
node_modules/shelljs/src/mv.js | 82 +
node_modules/shelljs/src/popd.js | 1 +
node_modules/shelljs/src/pushd.js | 1 +
node_modules/shelljs/src/pwd.js | 11 +
node_modules/shelljs/src/rm.js | 163 +
node_modules/shelljs/src/sed.js | 64 +
node_modules/shelljs/src/set.js | 49 +
node_modules/shelljs/src/tempdir.js | 57 +
node_modules/shelljs/src/test.js | 85 +
node_modules/shelljs/src/to.js | 30 +
node_modules/shelljs/src/toEnd.js | 30 +
node_modules/shelljs/src/touch.js | 109 +
node_modules/shelljs/src/which.js | 98 +
node_modules/simple-plist/.npmignore | 14 +
node_modules/simple-plist/LICENSE | 20 +
node_modules/simple-plist/README.md | 47 +
.../node_modules/base64-js/.travis.yml | 5 +
.../node_modules/base64-js/LICENSE.MIT | 21 +
.../node_modules/base64-js/README.md | 31 +
.../node_modules/base64-js/bench/bench.js | 19 +
.../node_modules/base64-js/lib/b64.js | 121 +
.../node_modules/base64-js/package.json | 87 +
.../node_modules/base64-js/test/convert.js | 52 +
.../node_modules/bplist-parser/.npmignore | 8 +
.../node_modules/bplist-parser/README.md | 47 +
.../node_modules/bplist-parser/bplistParser.js | 332 +
.../node_modules/bplist-parser/package.json | 78 +
.../bplist-parser/test/airplay.bplist | Bin 0 -> 341 bytes
.../bplist-parser/test/iTunes-small.bplist | Bin 0 -> 24433 bytes
.../bplist-parser/test/parseTest.js | 120 +
.../bplist-parser/test/sample1.bplist | Bin 0 -> 605 bytes
.../bplist-parser/test/sample2.bplist | Bin 0 -> 384 bytes
.../node_modules/bplist-parser/test/uid.bplist | Bin 0 -> 365 bytes
.../bplist-parser/test/utf16.bplist | Bin 0 -> 1273 bytes
.../simple-plist/node_modules/plist/.jshintrc | 4 +
.../simple-plist/node_modules/plist/.travis.yml | 32 +
.../simple-plist/node_modules/plist/History.md | 112 +
.../simple-plist/node_modules/plist/LICENSE | 24 +
.../simple-plist/node_modules/plist/Makefile | 76 +
.../simple-plist/node_modules/plist/README.md | 113 +
.../node_modules/plist/dist/plist-build.js | 12596 ++++++++++++++
.../node_modules/plist/dist/plist-parse.js | 3628 ++++
.../node_modules/plist/dist/plist.js | 14920 +++++++++++++++++
.../plist/examples/browser/index.html | 14 +
.../node_modules/plist/lib/build.js | 136 +
.../simple-plist/node_modules/plist/lib/node.js | 49 +
.../node_modules/plist/lib/parse.js | 200 +
.../node_modules/plist/lib/plist.js | 23 +
.../node_modules/plist/package.json | 115 +
.../node_modules/plist/test/build.js | 133 +
.../node_modules/plist/test/parse.js | 448 +
.../node_modules/util-deprecate/History.md | 5 +
.../node_modules/util-deprecate/LICENSE | 24 +
.../node_modules/util-deprecate/README.md | 53 +
.../node_modules/util-deprecate/browser.js | 55 +
.../node_modules/util-deprecate/node.js | 6 +
.../node_modules/util-deprecate/package.json | 79 +
.../node_modules/xmlbuilder/.npmignore | 4 +
.../node_modules/xmlbuilder/LICENSE | 21 +
.../node_modules/xmlbuilder/README.md | 82 +
.../node_modules/xmlbuilder/lib/XMLAttribute.js | 32 +
.../node_modules/xmlbuilder/lib/XMLBuilder.js | 70 +
.../node_modules/xmlbuilder/lib/XMLCData.js | 48 +
.../node_modules/xmlbuilder/lib/XMLComment.js | 48 +
.../xmlbuilder/lib/XMLDTDAttList.js | 71 +
.../xmlbuilder/lib/XMLDTDElement.js | 49 +
.../node_modules/xmlbuilder/lib/XMLDTDEntity.js | 85 +
.../xmlbuilder/lib/XMLDTDNotation.js | 59 +
.../xmlbuilder/lib/XMLDeclaration.js | 70 +
.../node_modules/xmlbuilder/lib/XMLDocType.js | 183 +
.../node_modules/xmlbuilder/lib/XMLElement.js | 190 +
.../node_modules/xmlbuilder/lib/XMLNode.js | 304 +
.../xmlbuilder/lib/XMLProcessingInstruction.js | 50 +
.../node_modules/xmlbuilder/lib/XMLRaw.js | 48 +
.../xmlbuilder/lib/XMLStringifier.js | 163 +
.../node_modules/xmlbuilder/lib/XMLText.js | 49 +
.../node_modules/xmlbuilder/lib/index.js | 14 +
.../node_modules/xmlbuilder/package.json | 90 +
node_modules/simple-plist/package.json | 85 +
node_modules/simple-plist/simple-plist.js | 68 +
node_modules/stream-buffers/.mailmap | 0
node_modules/stream-buffers/.travis.yml | 15 +
node_modules/stream-buffers/README.md | 116 +
node_modules/stream-buffers/UNLICENSE | 24 +
node_modules/stream-buffers/lib/constants.js | 6 +
.../stream-buffers/lib/readable_streambuffer.js | 116 +
node_modules/stream-buffers/lib/streambuffer.js | 3 +
.../stream-buffers/lib/writable_streambuffer.js | 94 +
node_modules/stream-buffers/package.json | 83 +
node_modules/underscore/LICENSE | 23 +
node_modules/underscore/README.md | 22 +
node_modules/underscore/package.json | 97 +
node_modules/underscore/underscore-min.js | 6 +
node_modules/underscore/underscore-min.map | 1 +
node_modules/underscore/underscore.js | 1548 ++
node_modules/unorm/LICENSE.md | 42 +
node_modules/unorm/README.md | 118 +
node_modules/unorm/lib/unorm.js | 442 +
node_modules/unorm/package.json | 96 +
node_modules/util-deprecate/History.md | 16 +
node_modules/util-deprecate/LICENSE | 24 +
node_modules/util-deprecate/README.md | 53 +
node_modules/util-deprecate/browser.js | 67 +
node_modules/util-deprecate/node.js | 6 +
node_modules/util-deprecate/package.json | 81 +
node_modules/wrappy/LICENSE | 15 +
node_modules/wrappy/README.md | 36 +
node_modules/wrappy/package.json | 78 +
node_modules/wrappy/test/basic.js | 51 +
node_modules/wrappy/wrappy.js | 33 +
node_modules/xcode/.npmignore | 1 +
node_modules/xcode/AUTHORS | 6 +
node_modules/xcode/LICENSE | 14 +
node_modules/xcode/Makefile | 5 +
node_modules/xcode/README.md | 42 +
node_modules/xcode/index.js | 1 +
node_modules/xcode/lib/parseJob.js | 15 +
node_modules/xcode/lib/parser/pbxproj.js | 1843 ++
node_modules/xcode/lib/parser/pbxproj.pegjs | 273 +
node_modules/xcode/lib/pbxFile.js | 199 +
node_modules/xcode/lib/pbxProject.js | 1810 ++
node_modules/xcode/lib/pbxWriter.js | 282 +
node_modules/xcode/package.json | 117 +
node_modules/xmlbuilder/.npmignore | 5 +
node_modules/xmlbuilder/LICENSE | 21 +
node_modules/xmlbuilder/README.md | 86 +
node_modules/xmlbuilder/lib/XMLAttribute.js | 32 +
node_modules/xmlbuilder/lib/XMLBuilder.js | 69 +
node_modules/xmlbuilder/lib/XMLCData.js | 49 +
node_modules/xmlbuilder/lib/XMLComment.js | 49 +
node_modules/xmlbuilder/lib/XMLDTDAttList.js | 68 +
node_modules/xmlbuilder/lib/XMLDTDElement.js | 46 +
node_modules/xmlbuilder/lib/XMLDTDEntity.js | 84 +
node_modules/xmlbuilder/lib/XMLDTDNotation.js | 56 +
node_modules/xmlbuilder/lib/XMLDeclaration.js | 65 +
node_modules/xmlbuilder/lib/XMLDocType.js | 188 +
node_modules/xmlbuilder/lib/XMLElement.js | 212 +
node_modules/xmlbuilder/lib/XMLNode.js | 331 +
.../xmlbuilder/lib/XMLProcessingInstruction.js | 51 +
node_modules/xmlbuilder/lib/XMLRaw.js | 49 +
node_modules/xmlbuilder/lib/XMLStringifier.js | 165 +
node_modules/xmlbuilder/lib/XMLText.js | 49 +
node_modules/xmlbuilder/lib/index.js | 14 +
node_modules/xmlbuilder/package.json | 89 +
node_modules/xmldom/.npmignore | 5 +
node_modules/xmldom/.travis.yml | 22 +
node_modules/xmldom/LICENSE | 8 +
node_modules/xmldom/__package__.js | 4 +
node_modules/xmldom/changelog | 14 +
node_modules/xmldom/component.json | 10 +
node_modules/xmldom/dom-parser.js | 249 +
node_modules/xmldom/dom.js | 1147 ++
node_modules/xmldom/package.json | 128 +
node_modules/xmldom/readme.md | 219 +
node_modules/xmldom/sax.js | 586 +
1442 files changed, 173244 insertions(+)
----------------------------------------------------------------------
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/.npmignore b/node_modules/abbrev/.npmignore
new file mode 100644
index 0000000..9d6cd2f
--- /dev/null
+++ b/node_modules/abbrev/.npmignore
@@ -0,0 +1,4 @@
+.nyc_output
+nyc_output
+node_modules
+coverage
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/.travis.yml b/node_modules/abbrev/.travis.yml
new file mode 100644
index 0000000..991d04b
--- /dev/null
+++ b/node_modules/abbrev/.travis.yml
@@ -0,0 +1,5 @@
+language: node_js
+node_js:
+ - '0.10'
+ - '0.12'
+ - 'iojs'
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/CONTRIBUTING.md
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/CONTRIBUTING.md b/node_modules/abbrev/CONTRIBUTING.md
new file mode 100644
index 0000000..2f30261
--- /dev/null
+++ b/node_modules/abbrev/CONTRIBUTING.md
@@ -0,0 +1,3 @@
+ To get started, <a
+ href="http://www.clahub.com/agreements/isaacs/abbrev-js">sign the
+ Contributor License Agreement</a>.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/LICENSE b/node_modules/abbrev/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/abbrev/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/README.md
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/README.md b/node_modules/abbrev/README.md
new file mode 100644
index 0000000..99746fe
--- /dev/null
+++ b/node_modules/abbrev/README.md
@@ -0,0 +1,23 @@
+# abbrev-js
+
+Just like [ruby's Abbrev](http://apidock.com/ruby/Abbrev).
+
+Usage:
+
+ var abbrev = require("abbrev");
+ abbrev("foo", "fool", "folding", "flop");
+
+ // returns:
+ { fl: 'flop'
+ , flo: 'flop'
+ , flop: 'flop'
+ , fol: 'folding'
+ , fold: 'folding'
+ , foldi: 'folding'
+ , foldin: 'folding'
+ , folding: 'folding'
+ , foo: 'foo'
+ , fool: 'fool'
+ }
+
+This is handy for command-line scripts, or other cases where you want to be able to accept shorthands.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[12/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/examples/browser/index.html
----------------------------------------------------------------------
diff --git a/node_modules/plist/examples/browser/index.html b/node_modules/plist/examples/browser/index.html
new file mode 100644
index 0000000..8ce7d92
--- /dev/null
+++ b/node_modules/plist/examples/browser/index.html
@@ -0,0 +1,14 @@
+<!DOCTYPE html>
+<html>
+ <head>
+ <title>plist.js browser example</title>
+ <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
+ </head>
+ <body>
+ <script src="../../dist/plist.js"></script>
+ <script>
+ // TODO: add <input type=file> drag and drop example
+ console.log(plist);
+ </script>
+ </body>
+</html>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/lib/build.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/lib/build.js b/node_modules/plist/lib/build.js
new file mode 100644
index 0000000..e2b9454
--- /dev/null
+++ b/node_modules/plist/lib/build.js
@@ -0,0 +1,138 @@
+
+/**
+ * Module dependencies.
+ */
+
+var base64 = require('base64-js');
+var xmlbuilder = require('xmlbuilder');
+
+/**
+ * Module exports.
+ */
+
+exports.build = build;
+
+/**
+ * Accepts a `Date` instance and returns an ISO date string.
+ *
+ * @param {Date} d - Date instance to serialize
+ * @returns {String} ISO date string representation of `d`
+ * @api private
+ */
+
+function ISODateString(d){
+ function pad(n){
+ return n < 10 ? '0' + n : n;
+ }
+ return d.getUTCFullYear()+'-'
+ + pad(d.getUTCMonth()+1)+'-'
+ + pad(d.getUTCDate())+'T'
+ + pad(d.getUTCHours())+':'
+ + pad(d.getUTCMinutes())+':'
+ + pad(d.getUTCSeconds())+'Z';
+}
+
+/**
+ * Returns the internal "type" of `obj` via the
+ * `Object.prototype.toString()` trick.
+ *
+ * @param {Mixed} obj - any value
+ * @returns {String} the internal "type" name
+ * @api private
+ */
+
+var toString = Object.prototype.toString;
+function type (obj) {
+ var m = toString.call(obj).match(/\[object (.*)\]/);
+ return m ? m[1] : m;
+}
+
+/**
+ * Generate an XML plist string from the input object `obj`.
+ *
+ * @param {Object} obj - the object to convert
+ * @param {Object} [opts] - optional options object
+ * @returns {String} converted plist XML string
+ * @api public
+ */
+
+function build (obj, opts) {
+ var XMLHDR = {
+ version: '1.0',
+ encoding: 'UTF-8'
+ };
+
+ var XMLDTD = {
+ pubid: '-//Apple//DTD PLIST 1.0//EN',
+ sysid: 'http://www.apple.com/DTDs/PropertyList-1.0.dtd'
+ };
+
+ var doc = xmlbuilder.create('plist');
+
+ doc.dec(XMLHDR.version, XMLHDR.encoding, XMLHDR.standalone);
+ doc.dtd(XMLDTD.pubid, XMLDTD.sysid);
+ doc.att('version', '1.0');
+
+ walk_obj(obj, doc);
+
+ if (!opts) opts = {};
+ // default `pretty` to `true`
+ opts.pretty = opts.pretty !== false;
+ return doc.end(opts);
+}
+
+/**
+ * depth first, recursive traversal of a javascript object. when complete,
+ * next_child contains a reference to the build XML object.
+ *
+ * @api private
+ */
+
+function walk_obj(next, next_child) {
+ var tag_type, i, prop;
+ var name = type(next);
+
+ if ('Undefined' == name) {
+ return;
+ } else if (Array.isArray(next)) {
+ next_child = next_child.ele('array');
+ for (i = 0; i < next.length; i++) {
+ walk_obj(next[i], next_child);
+ }
+
+ } else if (Buffer.isBuffer(next)) {
+ next_child.ele('data').raw(next.toString('base64'));
+
+ } else if ('Object' == name) {
+ next_child = next_child.ele('dict');
+ for (prop in next) {
+ if (next.hasOwnProperty(prop)) {
+ next_child.ele('key').txt(prop);
+ walk_obj(next[prop], next_child);
+ }
+ }
+
+ } else if ('Number' == name) {
+ // detect if this is an integer or real
+ // TODO: add an ability to force one way or another via a "cast"
+ tag_type = (next % 1 === 0) ? 'integer' : 'real';
+ next_child.ele(tag_type).txt(next.toString());
+
+ } else if ('Date' == name) {
+ next_child.ele('date').txt(ISODateString(new Date(next)));
+
+ } else if ('Boolean' == name) {
+ next_child.ele(next ? 'true' : 'false');
+
+ } else if ('String' == name) {
+ next_child.ele('string').txt(next);
+
+ } else if ('ArrayBuffer' == name) {
+ next_child.ele('data').raw(base64.fromByteArray(next));
+
+ } else if (next && next.buffer && 'ArrayBuffer' == type(next.buffer)) {
+ // a typed array
+ next_child.ele('data').raw(base64.fromByteArray(new Uint8Array(next.buffer), next_child));
+
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/lib/node.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/lib/node.js b/node_modules/plist/lib/node.js
new file mode 100644
index 0000000..ac18e32
--- /dev/null
+++ b/node_modules/plist/lib/node.js
@@ -0,0 +1,49 @@
+/**
+ * Module dependencies.
+ */
+
+var fs = require('fs');
+var parse = require('./parse');
+var deprecate = require('util-deprecate');
+
+/**
+ * Module exports.
+ */
+
+exports.parseFile = deprecate(parseFile, '`parseFile()` is deprecated. ' +
+ 'Use `parseString()` instead.');
+exports.parseFileSync = deprecate(parseFileSync, '`parseFileSync()` is deprecated. ' +
+ 'Use `parseStringSync()` instead.');
+
+/**
+ * Parses file `filename` as a .plist file.
+ * Invokes `fn` callback function when done.
+ *
+ * @param {String} filename - name of the file to read
+ * @param {Function} fn - callback function
+ * @api public
+ * @deprecated use parseString() instead
+ */
+
+function parseFile (filename, fn) {
+ fs.readFile(filename, { encoding: 'utf8' }, onread);
+ function onread (err, inxml) {
+ if (err) return fn(err);
+ parse.parseString(inxml, fn);
+ }
+}
+
+/**
+ * Parses file `filename` as a .plist file.
+ * Returns a when done.
+ *
+ * @param {String} filename - name of the file to read
+ * @param {Function} fn - callback function
+ * @api public
+ * @deprecated use parseStringSync() instead
+ */
+
+function parseFileSync (filename) {
+ var inxml = fs.readFileSync(filename, 'utf8');
+ return parse.parseStringSync(inxml);
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/lib/parse.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/lib/parse.js b/node_modules/plist/lib/parse.js
new file mode 100644
index 0000000..c154384
--- /dev/null
+++ b/node_modules/plist/lib/parse.js
@@ -0,0 +1,200 @@
+
+/**
+ * Module dependencies.
+ */
+
+var deprecate = require('util-deprecate');
+var DOMParser = require('xmldom').DOMParser;
+
+/**
+ * Module exports.
+ */
+
+exports.parse = parse;
+exports.parseString = deprecate(parseString, '`parseString()` is deprecated. ' +
+ 'It\'s not actually async. Use `parse()` instead.');
+exports.parseStringSync = deprecate(parseStringSync, '`parseStringSync()` is ' +
+ 'deprecated. Use `parse()` instead.');
+
+/**
+ * We ignore raw text (usually whitespace), <!-- xml comments -->,
+ * and raw CDATA nodes.
+ *
+ * @param {Element} node
+ * @returns {Boolean}
+ * @api private
+ */
+
+function shouldIgnoreNode (node) {
+ return node.nodeType === 3 // text
+ || node.nodeType === 8 // comment
+ || node.nodeType === 4; // cdata
+}
+
+
+/**
+ * Parses a Plist XML string. Returns an Object.
+ *
+ * @param {String} xml - the XML String to decode
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ */
+
+function parse (xml) {
+ var doc = new DOMParser().parseFromString(xml);
+ if (doc.documentElement.nodeName !== 'plist') {
+ throw new Error('malformed document. First element should be <plist>');
+ }
+ var plist = parsePlistXML(doc.documentElement);
+
+ // the root <plist> node gets interpreted as an Array,
+ // so pull out the inner data first
+ if (plist.length == 1) plist = plist[0];
+
+ return plist;
+}
+
+/**
+ * Parses a Plist XML string. Returns an Object. Takes a `callback` function.
+ *
+ * @param {String} xml - the XML String to decode
+ * @param {Function} callback - callback function
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ * @deprecated not actually async. use parse() instead
+ */
+
+function parseString (xml, callback) {
+ var doc, error, plist;
+ try {
+ doc = new DOMParser().parseFromString(xml);
+ plist = parsePlistXML(doc.documentElement);
+ } catch(e) {
+ error = e;
+ }
+ callback(error, plist);
+}
+
+/**
+ * Parses a Plist XML string. Returns an Object.
+ *
+ * @param {String} xml - the XML String to decode
+ * @param {Function} callback - callback function
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ * @deprecated use parse() instead
+ */
+
+function parseStringSync (xml) {
+ var doc = new DOMParser().parseFromString(xml);
+ var plist;
+ if (doc.documentElement.nodeName !== 'plist') {
+ throw new Error('malformed document. First element should be <plist>');
+ }
+ plist = parsePlistXML(doc.documentElement);
+
+ // if the plist is an array with 1 element, pull it out of the array
+ if (plist.length == 1) {
+ plist = plist[0];
+ }
+ return plist;
+}
+
+/**
+ * Convert an XML based plist document into a JSON representation.
+ *
+ * @param {Object} xml_node - current XML node in the plist
+ * @returns {Mixed} built up JSON object
+ * @api private
+ */
+
+function parsePlistXML (node) {
+ var i, new_obj, key, val, new_arr, res, d;
+
+ if (!node)
+ return null;
+
+ if (node.nodeName === 'plist') {
+ new_arr = [];
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ new_arr.push( parsePlistXML(node.childNodes[i]));
+ }
+ }
+ return new_arr;
+
+ } else if (node.nodeName === 'dict') {
+ new_obj = {};
+ key = null;
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ if (key === null) {
+ key = parsePlistXML(node.childNodes[i]);
+ } else {
+ new_obj[key] = parsePlistXML(node.childNodes[i]);
+ key = null;
+ }
+ }
+ }
+ return new_obj;
+
+ } else if (node.nodeName === 'array') {
+ new_arr = [];
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ res = parsePlistXML(node.childNodes[i]);
+ if (null != res) new_arr.push(res);
+ }
+ }
+ return new_arr;
+
+ } else if (node.nodeName === '#text') {
+ // TODO: what should we do with text types? (CDATA sections)
+
+ } else if (node.nodeName === 'key') {
+ return node.childNodes[0].nodeValue;
+
+ } else if (node.nodeName === 'string') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ res += node.childNodes[d].nodeValue;
+ }
+ return res;
+
+ } else if (node.nodeName === 'integer') {
+ // parse as base 10 integer
+ return parseInt(node.childNodes[0].nodeValue, 10);
+
+ } else if (node.nodeName === 'real') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ if (node.childNodes[d].nodeType === 3) {
+ res += node.childNodes[d].nodeValue;
+ }
+ }
+ return parseFloat(res);
+
+ } else if (node.nodeName === 'data') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ if (node.childNodes[d].nodeType === 3) {
+ res += node.childNodes[d].nodeValue.replace(/\s+/g, '');
+ }
+ }
+
+ // decode base64 data to a Buffer instance
+ return new Buffer(res, 'base64');
+
+ } else if (node.nodeName === 'date') {
+ return new Date(node.childNodes[0].nodeValue);
+
+ } else if (node.nodeName === 'true') {
+ return true;
+
+ } else if (node.nodeName === 'false') {
+ return false;
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/lib/plist.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/lib/plist.js b/node_modules/plist/lib/plist.js
new file mode 100644
index 0000000..00a4167
--- /dev/null
+++ b/node_modules/plist/lib/plist.js
@@ -0,0 +1,23 @@
+
+var i;
+
+/**
+ * Parser functions.
+ */
+
+var parserFunctions = require('./parse');
+for (i in parserFunctions) exports[i] = parserFunctions[i];
+
+/**
+ * Builder functions.
+ */
+
+var builderFunctions = require('./build');
+for (i in builderFunctions) exports[i] = builderFunctions[i];
+
+/**
+ * Add Node.js-specific functions (they're deprecated…).
+ */
+
+var nodeFunctions = require('./node');
+for (i in nodeFunctions) exports[i] = nodeFunctions[i];
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/package.json
----------------------------------------------------------------------
diff --git a/node_modules/plist/package.json b/node_modules/plist/package.json
new file mode 100644
index 0000000..26e4a52
--- /dev/null
+++ b/node_modules/plist/package.json
@@ -0,0 +1,117 @@
+{
+ "_args": [
+ [
+ "plist@^1.2.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "plist@>=1.2.0 <2.0.0",
+ "_id": "plist@1.2.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/plist",
+ "_nodeVersion": "5.0.0",
+ "_npmUser": {
+ "email": "reinstein.mike@gmail.com",
+ "name": "mreinstein"
+ },
+ "_npmVersion": "3.3.11",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "plist",
+ "raw": "plist@^1.2.0",
+ "rawSpec": "^1.2.0",
+ "scope": null,
+ "spec": ">=1.2.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/plist/-/plist-1.2.0.tgz",
+ "_shasum": "084b5093ddc92506e259f874b8d9b1afb8c79593",
+ "_shrinkwrap": null,
+ "_spec": "plist@^1.2.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "email": "nathan@tootallnate.net",
+ "name": "Nathan Rajlich"
+ },
+ "bugs": {
+ "url": "https://github.com/TooTallNate/node-plist/issues"
+ },
+ "contributors": [
+ {
+ "name": "Hans Huebner",
+ "email": "hans.huebner@gmail.com"
+ },
+ {
+ "name": "Pierre Metrailler"
+ },
+ {
+ "name": "Mike Reinstein",
+ "email": "reinstein.mike@gmail.com"
+ },
+ {
+ "name": "Vladimir Tsvang"
+ },
+ {
+ "name": "Mathieu D'Amours"
+ }
+ ],
+ "dependencies": {
+ "base64-js": "0.0.8",
+ "util-deprecate": "1.0.2",
+ "xmlbuilder": "4.0.0",
+ "xmldom": "0.1.x"
+ },
+ "description": "Mac OS X Plist parser/builder for Node.js and browsers",
+ "devDependencies": {
+ "browserify": "12.0.1",
+ "mocha": "2.3.3",
+ "multiline": "1.0.2",
+ "zuul": "3.7.2"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "084b5093ddc92506e259f874b8d9b1afb8c79593",
+ "tarball": "http://registry.npmjs.org/plist/-/plist-1.2.0.tgz"
+ },
+ "gitHead": "69520574f27864145192338b72e608fbe1bda6f7",
+ "homepage": "https://github.com/TooTallNate/node-plist#readme",
+ "keywords": [
+ "apple",
+ "browser",
+ "mac",
+ "parser",
+ "plist",
+ "xml"
+ ],
+ "license": "MIT",
+ "main": "lib/plist.js",
+ "maintainers": [
+ {
+ "name": "TooTallNate",
+ "email": "nathan@tootallnate.net"
+ },
+ {
+ "name": "tootallnate",
+ "email": "nathan@tootallnate.net"
+ },
+ {
+ "name": "mreinstein",
+ "email": "reinstein.mike@gmail.com"
+ }
+ ],
+ "name": "plist",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/TooTallNate/node-plist.git"
+ },
+ "scripts": {
+ "test": "make test"
+ },
+ "version": "1.2.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/q/CHANGES.md
----------------------------------------------------------------------
diff --git a/node_modules/q/CHANGES.md b/node_modules/q/CHANGES.md
new file mode 100644
index 0000000..cd351fd
--- /dev/null
+++ b/node_modules/q/CHANGES.md
@@ -0,0 +1,786 @@
+
+## 1.4.1
+
+ - Address an issue that prevented Q from being used as a `<script>` for
+ Firefox add-ons. Q can now be used in any environment that provides `window`
+ or `self` globals, favoring `window` since add-ons have an an immutable
+ `self` that is distinct from `window`.
+
+## 1.4.0
+
+ - Add `noConflict` support for use in `<script>` (@jahnjw).
+
+## 1.3.0
+
+ - Add tracking for unhandled and handled rejections in Node.js (@benjamingr).
+
+## 1.2.1
+
+ - Fix Node.js environment detection for modern Browserify (@kahnjw).
+
+## 1.2.0
+
+ - Added Q.any(promisesArray) method (@vergara).
+ Returns a promise fulfilled with the value of the first resolved promise in
+ promisesArray. If all promises in promisesArray are rejected, it returns
+ a rejected promise.
+
+## 1.1.2
+
+ - Removed extraneous files from the npm package by using the "files"
+ whitelist in package.json instead of the .npmignore blacklist.
+ (@anton-rudeshko)
+
+## 1.1.1
+
+ - Fix a pair of regressions in bootstrapping, one which precluded
+ WebWorker support, and another that precluded support in
+ ``<script>`` usage outright. #607
+
+## 1.1.0
+
+ - Adds support for enabling long stack traces in node.js by setting
+ environment variable `Q_DEBUG=1`.
+ - Introduces the `tap` method to promises, which will see a value
+ pass through without alteration.
+ - Use instanceof to recognize own promise instances as opposed to
+ thenables.
+ - Construct timeout errors with `code === ETIMEDOUT` (Kornel Lesiński)
+ - More descriminant CommonJS module environment detection.
+ - Dropped continuous integration for Node.js 0.6 and 0.8 because of
+ changes to npm that preclude the use of new `^` version predicate
+ operator in any transitive dependency.
+ - Users can now override `Q.nextTick`.
+
+## 1.0.1
+
+ - Adds support for `Q.Promise`, which implements common usage of the
+ ES6 `Promise` constructor and its methods. `Promise` does not have
+ a valid promise constructor and a proper implementation awaits
+ version 2 of Q.
+ - Removes the console stopgap for a promise inspector. This no longer
+ works with any degree of reliability.
+ - Fixes support for content security policies that forbid eval. Now
+ using the `StopIteration` global to distinguish SpiderMonkey
+ generators from ES6 generators, assuming that they will never
+ coexist.
+
+## 1.0.0
+
+:cake: This is all but a re-release of version 0.9, which has settled
+into a gentle maintenance mode and rightly deserves an official 1.0.
+An ambitious 2.0 release is already around the corner, but 0.9/1.0
+have been distributed far and wide and demand long term support.
+
+ - Q will now attempt to post a debug message in browsers regardless
+ of whether window.Touch is defined. Chrome at least now has this
+ property regardless of whether touch is supported by the underlying
+ hardware.
+ - Remove deprecation warning from `promise.valueOf`. The function is
+ called by the browser in various ways so there is no way to
+ distinguish usage that should be migrated from usage that cannot be
+ altered.
+
+## 0.9.7
+
+ - :warning: `q.min.js` is no longer checked-in. It is however still
+ created by Grunt and NPM.
+ - Fixes a bug that inhibited `Q.async` with implementations of the new
+ ES6 generators.
+ - Fixes a bug with `nextTick` affecting Safari 6.0.5 the first time a
+ page loads when an `iframe` is involved.
+ - Introduces `passByCopy`, `join`, and `race`.
+ - Shows stack traces or error messages on the console, instead of
+ `Error` objects.
+ - Elimintates wrapper methods for improved performance.
+ - `Q.all` now propagates progress notifications of the form you might
+ expect of ES6 iterations, `{value, index}` where the `value` is the
+ progress notification from the promise at `index`.
+
+## 0.9.6
+
+ - Fixes a bug in recognizing the difference between compatible Q
+ promises, and Q promises from before the implementation of "inspect".
+ The latter are now coerced.
+ - Fixes an infinite asynchronous coercion cycle introduced by former
+ solution, in two independently sufficient ways. 1.) All promises
+ returned by makePromise now implement "inspect", albeit a default
+ that reports that the promise has an "unknown" state. 2.) The
+ implementation of "then/when" is now in "then" instead of "when", so
+ that the responsibility to "coerce" the given promise rests solely in
+ the "when" method and the "then" method may assume that "this" is a
+ promise of the right type.
+ - Refactors `nextTick` to use an unrolled microtask within Q regardless
+ of how new ticks a requested. #316 @rkatic
+
+## 0.9.5
+
+ - Introduces `inspect` for getting the state of a promise as
+ `{state: "fulfilled" | "rejected" | "pending", value | reason}`.
+ - Introduces `allSettled` which produces an array of promises states
+ for the input promises once they have all "settled". This is in
+ accordance with a discussion on Promises/A+ that "settled" refers to
+ a promise that is "fulfilled" or "rejected". "resolved" refers to a
+ deferred promise that has been "resolved" to another promise,
+ "sealing its fate" to the fate of the successor promise.
+ - Long stack traces are now off by default. Set `Q.longStackSupport`
+ to true to enable long stack traces.
+ - Long stack traces can now follow the entire asynchronous history of a
+ promise, not just a single jump.
+ - Introduces `spawn` for an immediately invoked asychronous generator.
+ @jlongster
+ - Support for *experimental* synonyms `mapply`, `mcall`, `nmapply`,
+ `nmcall` for method invocation.
+
+## 0.9.4
+
+ - `isPromise` and `isPromiseAlike` now always returns a boolean
+ (even for falsy values). #284 @lfac-pt
+ - Support for ES6 Generators in `async` #288 @andywingo
+ - Clear duplicate promise rejections from dispatch methods #238 @SLaks
+ - Unhandled rejection API #296 @domenic
+ `stopUnhandledRejectionTracking`, `getUnhandledReasons`,
+ `resetUnhandledRejections`.
+
+## 0.9.3
+
+ - Add the ability to give `Q.timeout`'s errors a custom error message. #270
+ @jgrenon
+ - Fix Q's call-stack busting behavior in Node.js 0.10, by switching from
+ `process.nextTick` to `setImmediate`. #254 #259
+ - Fix Q's behavior when used with the Mocha test runner in the browser, since
+ Mocha introduces a fake `process` global without a `nextTick` property. #267
+ - Fix some, but not all, cases wherein Q would give false positives in its
+ unhandled rejection detection (#252). A fix for other cases (#238) is
+ hopefully coming soon.
+ - Made `Q.promise` throw early if given a non-function.
+
+## 0.9.2
+
+ - Pass through progress notifications when using `timeout`. #229 @omares
+ - Pass through progress notifications when using `delay`.
+ - Fix `nbind` to actually bind the `thisArg`. #232 @davidpadbury
+
+## 0.9.1
+
+ - Made the AMD detection compatible with the RequireJS optimizer's `namespace`
+ option. #225 @terinjokes
+ - Fix side effects from `valueOf`, and thus from `isFulfilled`, `isRejected`,
+ and `isPending`. #226 @benjamn
+
+## 0.9.0
+
+This release removes many layers of deprecated methods and brings Q closer to
+alignment with Mark Miller’s TC39 [strawman][] for concurrency. At the same
+time, it fixes many bugs and adds a few features around error handling. Finally,
+it comes with an updated and comprehensive [API Reference][].
+
+[strawman]: http://wiki.ecmascript.org/doku.php?id=strawman:concurrency
+[API Reference]: https://github.com/kriskowal/q/wiki/API-Reference
+
+### API Cleanup
+
+The following deprecated or undocumented methods have been removed.
+Their replacements are listed here:
+
+<table>
+ <thead>
+ <tr>
+ <th>0.8.x method</th>
+ <th>0.9 replacement</th>
+ </tr>
+ </thead>
+ <tbody>
+ <tr>
+ <td><code>Q.ref</code></td>
+ <td><code>Q</code></td>
+ </tr>
+ <tr>
+ <td><code>call</code>, <code>apply</code>, <code>bind</code> (*)</td>
+ <td><code>fcall</code>/<code>invoke</code>, <code>fapply</code>/<code>post</code>, <code>fbind</code></td>
+ </tr>
+ <tr>
+ <td><code>ncall</code>, <code>napply</code> (*)</td>
+ <td><code>nfcall</code>/<code>ninvoke</code>, <code>nfapply</code>/<code>npost</code></td>
+ </tr>
+ <tr>
+ <td><code>end</code></td>
+ <td><code>done</code></td>
+ </tr>
+ <tr>
+ <td><code>put</code></td>
+ <td><code>set</code></td>
+ </tr>
+ <tr>
+ <td><code>node</code></td>
+ <td><code>nbind</code></td>
+ </tr>
+ <tr>
+ <td><code>nend</code></td>
+ <td><code>nodeify</code></td>
+ </tr>
+ <tr>
+ <td><code>isResolved</code></td>
+ <td><code>isPending</code></td>
+ </tr>
+ <tr>
+ <td><code>deferred.node</code></td>
+ <td><code>deferred.makeNodeResolver</code></td>
+ </tr>
+ <tr>
+ <td><code>Method</code>, <code>sender</code></td>
+ <td><code>dispatcher</code></td>
+ </tr>
+ <tr>
+ <td><code>send</code></td>
+ <td><code>dispatch</code></td>
+ </tr>
+ <tr>
+ <td><code>view</code>, <code>viewInfo</code></td>
+ <td>(none)</td>
+ </tr>
+ </tbody>
+</table>
+
+
+(*) Use of ``thisp`` is discouraged. For calling methods, use ``post`` or
+``invoke``.
+
+### Alignment with the Concurrency Strawman
+
+- Q now exports a `Q(value)` function, an alias for `resolve`.
+ `Q.call`, `Q.apply`, and `Q.bind` were removed to make room for the
+ same methods on the function prototype.
+- `invoke` has been aliased to `send` in all its forms.
+- `post` with no method name acts like `fapply`.
+
+### Error Handling
+
+- Long stack traces can be turned off by setting `Q.stackJumpLimit` to zero.
+ In the future, this property will be used to fine tune how many stack jumps
+ are retained in long stack traces; for now, anything nonzero is treated as
+ one (since Q only tracks one stack jump at the moment, see #144). #168
+- In Node.js, if there are unhandled rejections when the process exits, they
+ are output to the console. #115
+
+### Other
+
+- `delete` and `set` (née `put`) no longer have a fulfillment value.
+- Q promises are no longer frozen, which
+ [helps with performance](http://code.google.com/p/v8/issues/detail?id=1858).
+- `thenReject` is now included, as a counterpart to `thenResolve`.
+- The included browser `nextTick` shim is now faster. #195 @rkatic.
+
+### Bug Fixes
+
+- Q now works in Internet Explorer 10. #186 @ForbesLindesay
+- `fbind` no longer hard-binds the returned function's `this` to `undefined`.
+ #202
+- `Q.reject` no longer leaks memory. #148
+- `npost` with no arguments now works. #207
+- `allResolved` now works with non-Q promises ("thenables"). #179
+- `keys` behavior is now correct even in browsers without native
+ `Object.keys`. #192 @rkatic
+- `isRejected` and the `exception` property now work correctly if the
+ rejection reason is falsy. #198
+
+### Internals and Advanced
+
+- The internal interface for a promise now uses
+ `dispatchPromise(resolve, op, operands)` instead of `sendPromise(op,
+ resolve, ...operands)`, which reduces the cases where Q needs to do
+ argument slicing.
+- The internal protocol uses different operands. "put" is now "set".
+ "del" is now "delete". "view" and "viewInfo" have been removed.
+- `Q.fulfill` has been added. It is distinct from `Q.resolve` in that
+ it does not pass promises through, nor coerces promises from other
+ systems. The promise becomes the fulfillment value. This is only
+ recommended for use when trying to fulfill a promise with an object that has
+ a `then` function that is at the same time not a promise.
+
+## 0.8.12
+- Treat foreign promises as unresolved in `Q.isFulfilled`; this lets `Q.all`
+ work on arrays containing foreign promises. #154
+- Fix minor incompliances with the [Promises/A+ spec][] and [test suite][]. #157
+ #158
+
+[Promises/A+ spec]: http://promises-aplus.github.com/promises-spec/
+[test suite]: https://github.com/promises-aplus/promises-tests
+
+## 0.8.11
+
+ - Added ``nfcall``, ``nfapply``, and ``nfbind`` as ``thisp``-less versions of
+ ``ncall``, ``napply``, and ``nbind``. The latter are now deprecated. #142
+ - Long stack traces no longer cause linearly-growing memory usage when chaining
+ promises together. #111
+ - Inspecting ``error.stack`` in a rejection handler will now give a long stack
+ trace. #103
+ - Fixed ``Q.timeout`` to clear its timeout handle when the promise is rejected;
+ previously, it kept the event loop alive until the timeout period expired.
+ #145 @dfilatov
+ - Added `q/queue` module, which exports an infinite promise queue
+ constructor.
+
+## 0.8.10
+
+ - Added ``done`` as a replacement for ``end``, taking the usual fulfillment,
+ rejection, and progress handlers. It's essentially equivalent to
+ ``then(f, r, p).end()``.
+ - Added ``Q.onerror``, a settable error trap that you can use to get full stack
+ traces for uncaught errors. #94
+ - Added ``thenResolve`` as a shortcut for returning a constant value once a
+ promise is fulfilled. #108 @ForbesLindesay
+ - Various tweaks to progress notification, including propagation and
+ transformation of progress values and only forwarding a single progress
+ object.
+ - Renamed ``nend`` to ``nodeify``. It no longer returns an always-fulfilled
+ promise when a Node callback is passed.
+ - ``deferred.resolve`` and ``deferred.reject`` no longer (sometimes) return
+ ``deferred.promise``.
+ - Fixed stack traces getting mangled if they hit ``end`` twice. #116 #121 @ef4
+ - Fixed ``ninvoke`` and ``npost`` to work on promises for objects with Node
+ methods. #134
+ - Fixed accidental coercion of objects with nontrivial ``valueOf`` methods,
+ like ``Date``s, by the promise's ``valueOf`` method. #135
+ - Fixed ``spread`` not calling the passed rejection handler if given a rejected
+ promise.
+
+## 0.8.9
+
+ - Added ``nend``
+ - Added preliminary progress notification support, via
+ ``promise.then(onFulfilled, onRejected, onProgress)``,
+ ``promise.progress(onProgress)``, and ``deferred.notify(...progressData)``.
+ - Made ``put`` and ``del`` return the object acted upon for easier chaining.
+ #84
+ - Fixed coercion cycles with cooperating promises. #106
+
+## 0.8.7
+
+ - Support [Montage Require](http://github.com/kriskowal/mr)
+
+## 0.8.6
+
+ - Fixed ``npost`` and ``ninvoke`` to pass the correct ``thisp``. #74
+ - Fixed various cases involving unorthodox rejection reasons. #73 #90
+ @ef4
+ - Fixed double-resolving of misbehaved custom promises. #75
+ - Sped up ``Q.all`` for arrays contain already-resolved promises or scalar
+ values. @ForbesLindesay
+ - Made stack trace filtering work when concatenating assets. #93 @ef4
+ - Added warnings for deprecated methods. @ForbesLindesay
+ - Added ``.npmignore`` file so that dependent packages get a slimmer
+ ``node_modules`` directory.
+
+## 0.8.5
+
+ - Added preliminary support for long traces (@domenic)
+ - Added ``fapply``, ``fcall``, ``fbind`` for non-thisp
+ promised function calls.
+ - Added ``return`` for async generators, where generators
+ are implemented.
+ - Rejected promises now have an "exception" property. If an object
+ isRejected(object), then object.valueOf().exception will
+ be the wrapped error.
+ - Added Jasmine specifications
+ - Support Internet Explorers 7–9 (with multiple bug fixes @domenic)
+ - Support Firefox 12
+ - Support Safari 5.1.5
+ - Support Chrome 18
+
+## 0.8.4
+
+ - WARNING: ``promise.timeout`` is now rejected with an ``Error`` object
+ and the message now includes the duration of the timeout in
+ miliseconds. This doesn't constitute (in my opinion) a
+ backward-incompatibility since it is a change of an undocumented and
+ unspecified public behavior, but if you happened to depend on the
+ exception being a string, you will need to revise your code.
+ - Added ``deferred.makeNodeResolver()`` to replace the more cryptic
+ ``deferred.node()`` method.
+ - Added experimental ``Q.promise(maker(resolve, reject))`` to make a
+ promise inside a callback, such that thrown exceptions in the
+ callback are converted and the resolver and rejecter are arguments.
+ This is a shorthand for making a deferred directly and inspired by
+ @gozala’s stream constructor pattern and the Microsoft Windows Metro
+ Promise constructor interface.
+ - Added experimental ``Q.begin()`` that is intended to kick off chains
+ of ``.then`` so that each of these can be reordered without having to
+ edit the new and former first step.
+
+## 0.8.3
+
+ - Added ``isFulfilled``, ``isRejected``, and ``isResolved``
+ to the promise prototype.
+ - Added ``allResolved`` for waiting for every promise to either be
+ fulfilled or rejected, without propagating an error. @utvara #53
+ - Added ``Q.bind`` as a method to transform functions that
+ return and throw into promise-returning functions. See
+ [an example](https://gist.github.com/1782808). @domenic
+ - Renamed ``node`` export to ``nbind``, and added ``napply`` to
+ complete the set. ``node`` remains as deprecated. @domenic #58
+ - Renamed ``Method`` export to ``sender``. ``Method``
+ remains as deprecated and will be removed in the next
+ major version since I expect it has very little usage.
+ - Added browser console message indicating a live list of
+ unhandled errors.
+ - Added support for ``msSetImmediate`` (IE10) or ``setImmediate``
+ (available via [polyfill](https://github.com/NobleJS/setImmediate))
+ as a browser-side ``nextTick`` implementation. #44 #50 #59
+ - Stopped using the event-queue dependency, which was in place for
+ Narwhal support: now directly using ``process.nextTick``.
+ - WARNING: EXPERIMENTAL: added ``finally`` alias for ``fin``, ``catch``
+ alias for ``fail``, ``try`` alias for ``call``, and ``delete`` alias
+ for ``del``. These properties are enquoted in the library for
+ cross-browser compatibility, but may be used as property names in
+ modern engines.
+
+## 0.8.2
+
+ - Deprecated ``ref`` in favor of ``resolve`` as recommended by
+ @domenic.
+ - Update event-queue dependency.
+
+## 0.8.1
+
+ - Fixed Opera bug. #35 @cadorn
+ - Fixed ``Q.all([])`` #32 @domenic
+
+## 0.8.0
+
+ - WARNING: ``enqueue`` removed. Use ``nextTick`` instead.
+ This is more consistent with NodeJS and (subjectively)
+ more explicit and intuitive.
+ - WARNING: ``def`` removed. Use ``master`` instead. The
+ term ``def`` was too confusing to new users.
+ - WARNING: ``spy`` removed in favor of ``fin``.
+ - WARNING: ``wait`` removed. Do ``all(args).get(0)`` instead.
+ - WARNING: ``join`` removed. Do ``all(args).spread(callback)`` instead.
+ - WARNING: Removed the ``Q`` function module.exports alias
+ for ``Q.ref``. It conflicts with ``Q.apply`` in weird
+ ways, making it uncallable.
+ - Revised ``delay`` so that it accepts both ``(value,
+ timeout)`` and ``(timeout)`` variations based on
+ arguments length.
+ - Added ``ref().spread(cb(...args))``, a variant of
+ ``then`` that spreads an array across multiple arguments.
+ Useful with ``all()``.
+ - Added ``defer().node()`` Node callback generator. The
+ callback accepts ``(error, value)`` or ``(error,
+ ...values)``. For multiple value arguments, the
+ fulfillment value is an array, useful in conjunction with
+ ``spread``.
+ - Added ``node`` and ``ncall``, both with the signature
+ ``(fun, thisp_opt, ...args)``. The former is a decorator
+ and the latter calls immediately. ``node`` optional
+ binds and partially applies. ``ncall`` can bind and pass
+ arguments.
+
+## 0.7.2
+
+ - Fixed thenable promise assimilation.
+
+## 0.7.1
+
+ - Stopped shimming ``Array.prototype.reduce``. The
+ enumerable property has bad side-effects. Libraries that
+ depend on this (for example, QQ) will need to be revised.
+
+## 0.7.0 - BACKWARD INCOMPATIBILITY
+
+ - WARNING: Removed ``report`` and ``asap``
+ - WARNING: The ``callback`` argument of the ``fin``
+ function no longer receives any arguments. Thus, it can
+ be used to call functions that should not receive
+ arguments on resolution. Use ``when``, ``then``, or
+ ``fail`` if you need a value.
+ - IMPORTANT: Fixed a bug in the use of ``MessageChannel``
+ for ``nextTick``.
+ - Renamed ``enqueue`` to ``nextTick``.
+ - Added experimental ``view`` and ``viewInfo`` for creating
+ views of promises either when or before they're
+ fulfilled.
+ - Shims are now externally applied so subsequent scripts or
+ dependees can use them.
+ - Improved minification results.
+ - Improved readability.
+
+## 0.6.0 - BACKWARD INCOMPATIBILITY
+
+ - WARNING: In practice, the implementation of ``spy`` and
+ the name ``fin`` were useful. I've removed the old
+ ``fin`` implementation and renamed/aliased ``spy``.
+ - The "q" module now exports its ``ref`` function as a "Q"
+ constructor, with module systems that support exports
+ assignment including NodeJS, RequireJS, and when used as
+ a ``<script>`` tag. Notably, strictly compliant CommonJS
+ does not support this, but UncommonJS does.
+ - Added ``async`` decorator for generators that use yield
+ to "trampoline" promises. In engines that support
+ generators (SpiderMonkey), this will greatly reduce the
+ need for nested callbacks.
+ - Made ``when`` chainable.
+ - Made ``all`` chainable.
+
+## 0.5.3
+
+ - Added ``all`` and refactored ``join`` and ``wait`` to use
+ it. All of these will now reject at the earliest
+ rejection.
+
+## 0.5.2
+
+ - Minor improvement to ``spy``; now waits for resolution of
+ callback promise.
+
+## 0.5.1
+
+ - Made most Q API methods chainable on promise objects, and
+ turned the previous promise-methods of ``join``,
+ ``wait``, and ``report`` into Q API methods.
+ - Added ``apply`` and ``call`` to the Q API, and ``apply``
+ as a promise handler.
+ - Added ``fail``, ``fin``, and ``spy`` to Q and the promise
+ prototype for convenience when observing rejection,
+ fulfillment and rejection, or just observing without
+ affecting the resolution.
+ - Renamed ``def`` (although ``def`` remains shimmed until
+ the next major release) to ``master``.
+ - Switched to using ``MessageChannel`` for next tick task
+ enqueue in browsers that support it.
+
+## 0.5.0 - MINOR BACKWARD INCOMPATIBILITY
+
+ - Exceptions are no longer reported when consumed.
+ - Removed ``error`` from the API. Since exceptions are
+ getting consumed, throwing them in an errback causes the
+ exception to silently disappear. Use ``end``.
+ - Added ``end`` as both an API method and a promise-chain
+ ending method. It causes propagated rejections to be
+ thrown, which allows Node to write stack traces and
+ emit ``uncaughtException`` events, and browsers to
+ likewise emit ``onerror`` and log to the console.
+ - Added ``join`` and ``wait`` as promise chain functions,
+ so you can wait for variadic promises, returning your own
+ promise back, or join variadic promises, resolving with a
+ callback that receives variadic fulfillment values.
+
+## 0.4.4
+
+ - ``end`` no longer returns a promise. It is the end of the
+ promise chain.
+ - Stopped reporting thrown exceptions in ``when`` callbacks
+ and errbacks. These must be explicitly reported through
+ ``.end()``, ``.then(null, Q.error)``, or some other
+ mechanism.
+ - Added ``report`` as an API method, which can be used as
+ an errback to report and propagate an error.
+ - Added ``report`` as a promise-chain method, so an error
+ can be reported if it passes such a gate.
+
+## 0.4.3
+
+ - Fixed ``<script>`` support that regressed with 0.4.2
+ because of "use strict" in the module system
+ multi-plexer.
+
+## 0.4.2
+
+ - Added support for RequireJS (jburke)
+
+## 0.4.1
+
+ - Added an "end" method to the promise prototype,
+ as a shorthand for waiting for the promise to
+ be resolved gracefully, and failing to do so,
+ to dump an error message.
+
+## 0.4.0 - BACKWARD INCOMPATIBLE*
+
+ - *Removed the utility modules. NPM and Node no longer
+ expose any module except the main module. These have
+ been moved and merged into the "qq" package.
+ - *In a non-CommonJS browser, q.js can be used as a script.
+ It now creates a Q global variable.
+ - Fixed thenable assimilation.
+ - Fixed some issues with asap, when it resolves to
+ undefined, or throws an exception.
+
+## 0.3.0 - BACKWARD-INCOMPATIBLE
+
+ - The `post` method has been reverted to its original
+ signature, as provided in Tyler Close's `ref_send` API.
+ That is, `post` accepts two arguments, the second of
+ which is an arbitrary object, but usually invocation
+ arguments as an `Array`. To provide variadic arguments
+ to `post`, there is a new `invoke` function that posts
+ the variadic arguments to the value given in the first
+ argument.
+ - The `defined` method has been moved from `q` to `q/util`
+ since it gets no use in practice but is still
+ theoretically useful.
+ - The `Promise` constructor has been renamed to
+ `makePromise` to be consistent with the convention that
+ functions that do not require the `new` keyword to be
+ used as constructors have camelCase names.
+ - The `isResolved` function has been renamed to
+ `isFulfilled`. There is a new `isResolved` function that
+ indicates whether a value is not a promise or, if it is a
+ promise, whether it has been either fulfilled or
+ rejected. The code has been revised to reflect this
+ nuance in terminology.
+
+## 0.2.10
+
+ - Added `join` to `"q/util"` for variadically joining
+ multiple promises.
+
+## 0.2.9
+
+ - The future-compatible `invoke` method has been added,
+ to replace `post`, since `post` will become backward-
+ incompatible in the next major release.
+ - Exceptions thrown in the callbacks of a `when` call are
+ now emitted to Node's `"uncaughtException"` `process`
+ event in addition to being returned as a rejection reason.
+
+## 0.2.8
+
+ - Exceptions thrown in the callbacks of a `when` call
+ are now consumed, warned, and transformed into
+ rejections of the promise returned by `when`.
+
+## 0.2.7
+
+ - Fixed a minor bug in thenable assimilation, regressed
+ because of the change in the forwarding protocol.
+ - Fixed behavior of "q/util" `deep` method on dates and
+ other primitives. Github issue #11.
+
+## 0.2.6
+
+ - Thenables (objects with a "then" method) are accepted
+ and provided, bringing this implementation of Q
+ into conformance with Promises/A, B, and D.
+ - Added `makePromise`, to replace the `Promise` function
+ eventually.
+ - Rejections are now also duck-typed. A rejection is a
+ promise with a valueOf method that returns a rejection
+ descriptor. A rejection descriptor has a
+ "promiseRejected" property equal to "true" and a
+ "reason" property corresponding to the rejection reason.
+ - Altered the `makePromise` API such that the `fallback`
+ method no longer receives a superfluous `resolved` method
+ after the `operator`. The fallback method is responsible
+ only for returning a resolution. This breaks an
+ undocumented API, so third-party API's depending on the
+ previous undocumented behavior may break.
+
+## 0.2.5
+
+ - Changed promises into a duck-type such that multiple
+ instances of the Q module can exchange promise objects.
+ A promise is now defined as "an object that implements the
+ `promiseSend(op, resolved, ...)` method and `valueOf`".
+ - Exceptions in promises are now captured and returned
+ as rejections.
+
+## 0.2.4
+
+ - Fixed bug in `ref` that prevented `del` messages from
+ being received (gozala)
+ - Fixed a conflict with FireFox 4; constructor property
+ is now read-only.
+
+## 0.2.3
+
+ - Added `keys` message to promises and to the promise API.
+
+## 0.2.2
+
+ - Added boilerplate to `q/queue` and `q/util`.
+ - Fixed missing dependency to `q/queue`.
+
+## 0.2.1
+
+ - The `resolve` and `reject` methods of `defer` objects now
+ return the resolution promise for convenience.
+ - Added `q/util`, which provides `step`, `delay`, `shallow`,
+ `deep`, and three reduction orders.
+ - Added `q/queue` module for a promise `Queue`.
+ - Added `q-comm` to the list of compatible libraries.
+ - Deprecated `defined` from `q`, with intent to move it to
+ `q/util`.
+
+## 0.2.0 - BACKWARD INCOMPATIBLE
+
+ - Changed post(ref, name, args) to variadic
+ post(ref, name, ...args). BACKWARD INCOMPATIBLE
+ - Added a def(value) method to annotate an object as being
+ necessarily a local value that cannot be serialized, such
+ that inter-process/worker/vat promise communication
+ libraries will send messages to it, but never send it
+ back.
+ - Added a send(value, op, ...args) method to the public API, for
+ forwarding messages to a value or promise in a future turn.
+
+## 0.1.9
+
+ - Added isRejected() for testing whether a value is a rejected
+ promise. isResolved() retains the behavior of stating
+ that rejected promises are not resolved.
+
+## 0.1.8
+
+ - Fixed isResolved(null) and isResolved(undefined) [issue #9]
+ - Fixed a problem with the Object.create shim
+
+## 0.1.7
+
+ - shimmed ES5 Object.create in addition to Object.freeze
+ for compatibility on non-ES5 engines (gozala)
+
+## 0.1.6
+
+ - Q.isResolved added
+ - promise.valueOf() now returns the value of resolved
+ and near values
+ - asap retried
+ - promises are frozen when possible
+
+## 0.1.5
+
+ - fixed dependency list for Teleport (gozala)
+ - all unit tests now pass (gozala)
+
+## 0.1.4
+
+ - added support for Teleport as an engine (gozala)
+ - simplified and updated methods for getting internal
+ print and enqueue functions universally (gozala)
+
+## 0.1.3
+
+ - fixed erroneous link to the q module in package.json
+
+## 0.1.2
+
+ - restructured for overlay style package compatibility
+
+## 0.1.0
+
+ - removed asap because it was broken, probably down to the
+ philosophy.
+
+## 0.0.3
+
+ - removed q-util
+ - fixed asap so it returns a value if completed
+
+## 0.0.2
+
+ - added q-util
+
+## 0.0.1
+
+ - initial version
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/q/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/q/LICENSE b/node_modules/q/LICENSE
new file mode 100644
index 0000000..8a706b5
--- /dev/null
+++ b/node_modules/q/LICENSE
@@ -0,0 +1,18 @@
+Copyright 2009–2014 Kristopher Michael Kowal. All rights reserved.
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to
+deal in the Software without restriction, including without limitation the
+rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
+sell copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
+IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/q/README.md
----------------------------------------------------------------------
diff --git a/node_modules/q/README.md b/node_modules/q/README.md
new file mode 100644
index 0000000..9065bfa
--- /dev/null
+++ b/node_modules/q/README.md
@@ -0,0 +1,881 @@
+[](http://travis-ci.org/kriskowal/q)
+
+<a href="http://promises-aplus.github.com/promises-spec">
+ <img src="http://kriskowal.github.io/q/q.png"
+ align="right" alt="Q logo" />
+</a>
+
+*This is Q version 1, from the `v1` branch in Git. This documentation applies to
+the latest of both the version 1 and version 0.9 release trains. These releases
+are stable. There will be no further releases of 0.9 after 0.9.7 which is nearly
+equivalent to version 1.0.0. All further releases of `q@~1.0` will be backward
+compatible. The version 2 release train introduces significant and
+backward-incompatible changes and is experimental at this time.*
+
+If a function cannot return a value or throw an exception without
+blocking, it can return a promise instead. A promise is an object
+that represents the return value or the thrown exception that the
+function may eventually provide. A promise can also be used as a
+proxy for a [remote object][Q-Connection] to overcome latency.
+
+[Q-Connection]: https://github.com/kriskowal/q-connection
+
+On the first pass, promises can mitigate the “[Pyramid of
+Doom][POD]”: the situation where code marches to the right faster
+than it marches forward.
+
+[POD]: http://calculist.org/blog/2011/12/14/why-coroutines-wont-work-on-the-web/
+
+```javascript
+step1(function (value1) {
+ step2(value1, function(value2) {
+ step3(value2, function(value3) {
+ step4(value3, function(value4) {
+ // Do something with value4
+ });
+ });
+ });
+});
+```
+
+With a promise library, you can flatten the pyramid.
+
+```javascript
+Q.fcall(promisedStep1)
+.then(promisedStep2)
+.then(promisedStep3)
+.then(promisedStep4)
+.then(function (value4) {
+ // Do something with value4
+})
+.catch(function (error) {
+ // Handle any error from all above steps
+})
+.done();
+```
+
+With this approach, you also get implicit error propagation, just like `try`,
+`catch`, and `finally`. An error in `promisedStep1` will flow all the way to
+the `catch` function, where it’s caught and handled. (Here `promisedStepN` is
+a version of `stepN` that returns a promise.)
+
+The callback approach is called an “inversion of control”.
+A function that accepts a callback instead of a return value
+is saying, “Don’t call me, I’ll call you.”. Promises
+[un-invert][IOC] the inversion, cleanly separating the input
+arguments from control flow arguments. This simplifies the
+use and creation of API’s, particularly variadic,
+rest and spread arguments.
+
+[IOC]: http://www.slideshare.net/domenicdenicola/callbacks-promises-and-coroutines-oh-my-the-evolution-of-asynchronicity-in-javascript
+
+
+## Getting Started
+
+The Q module can be loaded as:
+
+- A ``<script>`` tag (creating a ``Q`` global variable): ~2.5 KB minified and
+ gzipped.
+- A Node.js and CommonJS module, available in [npm](https://npmjs.org/) as
+ the [q](https://npmjs.org/package/q) package
+- An AMD module
+- A [component](https://github.com/component/component) as ``microjs/q``
+- Using [bower](http://bower.io/) as `q#1.0.1`
+- Using [NuGet](http://nuget.org/) as [Q](https://nuget.org/packages/q)
+
+Q can exchange promises with jQuery, Dojo, When.js, WinJS, and more.
+
+## Resources
+
+Our [wiki][] contains a number of useful resources, including:
+
+- A method-by-method [Q API reference][reference].
+- A growing [examples gallery][examples], showing how Q can be used to make
+ everything better. From XHR to database access to accessing the Flickr API,
+ Q is there for you.
+- There are many libraries that produce and consume Q promises for everything
+ from file system/database access or RPC to templating. For a list of some of
+ the more popular ones, see [Libraries][].
+- If you want materials that introduce the promise concept generally, and the
+ below tutorial isn't doing it for you, check out our collection of
+ [presentations, blog posts, and podcasts][resources].
+- A guide for those [coming from jQuery's `$.Deferred`][jquery].
+
+We'd also love to have you join the Q-Continuum [mailing list][].
+
+[wiki]: https://github.com/kriskowal/q/wiki
+[reference]: https://github.com/kriskowal/q/wiki/API-Reference
+[examples]: https://github.com/kriskowal/q/wiki/Examples-Gallery
+[Libraries]: https://github.com/kriskowal/q/wiki/Libraries
+[resources]: https://github.com/kriskowal/q/wiki/General-Promise-Resources
+[jquery]: https://github.com/kriskowal/q/wiki/Coming-from-jQuery
+[mailing list]: https://groups.google.com/forum/#!forum/q-continuum
+
+
+## Tutorial
+
+Promises have a ``then`` method, which you can use to get the eventual
+return value (fulfillment) or thrown exception (rejection).
+
+```javascript
+promiseMeSomething()
+.then(function (value) {
+}, function (reason) {
+});
+```
+
+If ``promiseMeSomething`` returns a promise that gets fulfilled later
+with a return value, the first function (the fulfillment handler) will be
+called with the value. However, if the ``promiseMeSomething`` function
+gets rejected later by a thrown exception, the second function (the
+rejection handler) will be called with the exception.
+
+Note that resolution of a promise is always asynchronous: that is, the
+fulfillment or rejection handler will always be called in the next turn of the
+event loop (i.e. `process.nextTick` in Node). This gives you a nice
+guarantee when mentally tracing the flow of your code, namely that
+``then`` will always return before either handler is executed.
+
+In this tutorial, we begin with how to consume and work with promises. We'll
+talk about how to create them, and thus create functions like
+`promiseMeSomething` that return promises, [below](#the-beginning).
+
+
+### Propagation
+
+The ``then`` method returns a promise, which in this example, I’m
+assigning to ``outputPromise``.
+
+```javascript
+var outputPromise = getInputPromise()
+.then(function (input) {
+}, function (reason) {
+});
+```
+
+The ``outputPromise`` variable becomes a new promise for the return
+value of either handler. Since a function can only either return a
+value or throw an exception, only one handler will ever be called and it
+will be responsible for resolving ``outputPromise``.
+
+- If you return a value in a handler, ``outputPromise`` will get
+ fulfilled.
+
+- If you throw an exception in a handler, ``outputPromise`` will get
+ rejected.
+
+- If you return a **promise** in a handler, ``outputPromise`` will
+ “become” that promise. Being able to become a new promise is useful
+ for managing delays, combining results, or recovering from errors.
+
+If the ``getInputPromise()`` promise gets rejected and you omit the
+rejection handler, the **error** will go to ``outputPromise``:
+
+```javascript
+var outputPromise = getInputPromise()
+.then(function (value) {
+});
+```
+
+If the input promise gets fulfilled and you omit the fulfillment handler, the
+**value** will go to ``outputPromise``:
+
+```javascript
+var outputPromise = getInputPromise()
+.then(null, function (error) {
+});
+```
+
+Q promises provide a ``fail`` shorthand for ``then`` when you are only
+interested in handling the error:
+
+```javascript
+var outputPromise = getInputPromise()
+.fail(function (error) {
+});
+```
+
+If you are writing JavaScript for modern engines only or using
+CoffeeScript, you may use `catch` instead of `fail`.
+
+Promises also have a ``fin`` function that is like a ``finally`` clause.
+The final handler gets called, with no arguments, when the promise
+returned by ``getInputPromise()`` either returns a value or throws an
+error. The value returned or error thrown by ``getInputPromise()``
+passes directly to ``outputPromise`` unless the final handler fails, and
+may be delayed if the final handler returns a promise.
+
+```javascript
+var outputPromise = getInputPromise()
+.fin(function () {
+ // close files, database connections, stop servers, conclude tests
+});
+```
+
+- If the handler returns a value, the value is ignored
+- If the handler throws an error, the error passes to ``outputPromise``
+- If the handler returns a promise, ``outputPromise`` gets postponed. The
+ eventual value or error has the same effect as an immediate return
+ value or thrown error: a value would be ignored, an error would be
+ forwarded.
+
+If you are writing JavaScript for modern engines only or using
+CoffeeScript, you may use `finally` instead of `fin`.
+
+### Chaining
+
+There are two ways to chain promises. You can chain promises either
+inside or outside handlers. The next two examples are equivalent.
+
+```javascript
+return getUsername()
+.then(function (username) {
+ return getUser(username)
+ .then(function (user) {
+ // if we get here without an error,
+ // the value returned here
+ // or the exception thrown here
+ // resolves the promise returned
+ // by the first line
+ })
+});
+```
+
+```javascript
+return getUsername()
+.then(function (username) {
+ return getUser(username);
+})
+.then(function (user) {
+ // if we get here without an error,
+ // the value returned here
+ // or the exception thrown here
+ // resolves the promise returned
+ // by the first line
+});
+```
+
+The only difference is nesting. It’s useful to nest handlers if you
+need to capture multiple input values in your closure.
+
+```javascript
+function authenticate() {
+ return getUsername()
+ .then(function (username) {
+ return getUser(username);
+ })
+ // chained because we will not need the user name in the next event
+ .then(function (user) {
+ return getPassword()
+ // nested because we need both user and password next
+ .then(function (password) {
+ if (user.passwordHash !== hash(password)) {
+ throw new Error("Can't authenticate");
+ }
+ });
+ });
+}
+```
+
+
+### Combination
+
+You can turn an array of promises into a promise for the whole,
+fulfilled array using ``all``.
+
+```javascript
+return Q.all([
+ eventualAdd(2, 2),
+ eventualAdd(10, 20)
+]);
+```
+
+If you have a promise for an array, you can use ``spread`` as a
+replacement for ``then``. The ``spread`` function “spreads” the
+values over the arguments of the fulfillment handler. The rejection handler
+will get called at the first sign of failure. That is, whichever of
+the received promises fails first gets handled by the rejection handler.
+
+```javascript
+function eventualAdd(a, b) {
+ return Q.spread([a, b], function (a, b) {
+ return a + b;
+ })
+}
+```
+
+But ``spread`` calls ``all`` initially, so you can skip it in chains.
+
+```javascript
+return getUsername()
+.then(function (username) {
+ return [username, getUser(username)];
+})
+.spread(function (username, user) {
+});
+```
+
+The ``all`` function returns a promise for an array of values. When this
+promise is fulfilled, the array contains the fulfillment values of the original
+promises, in the same order as those promises. If one of the given promises
+is rejected, the returned promise is immediately rejected, not waiting for the
+rest of the batch. If you want to wait for all of the promises to either be
+fulfilled or rejected, you can use ``allSettled``.
+
+```javascript
+Q.allSettled(promises)
+.then(function (results) {
+ results.forEach(function (result) {
+ if (result.state === "fulfilled") {
+ var value = result.value;
+ } else {
+ var reason = result.reason;
+ }
+ });
+});
+```
+
+The ``any`` function accepts an array of promises and returns a promise that is
+fulfilled by the first given promise to be fulfilled, or rejected if all of the
+given promises are rejected.
+
+```javascript
+Q.any(promises)
+.then(function (first) {
+ // Any of the promises was fulfilled.
+}, function (error) {
+ // All of the promises were rejected.
+});
+```
+
+### Sequences
+
+If you have a number of promise-producing functions that need
+to be run sequentially, you can of course do so manually:
+
+```javascript
+return foo(initialVal).then(bar).then(baz).then(qux);
+```
+
+However, if you want to run a dynamically constructed sequence of
+functions, you'll want something like this:
+
+```javascript
+var funcs = [foo, bar, baz, qux];
+
+var result = Q(initialVal);
+funcs.forEach(function (f) {
+ result = result.then(f);
+});
+return result;
+```
+
+You can make this slightly more compact using `reduce`:
+
+```javascript
+return funcs.reduce(function (soFar, f) {
+ return soFar.then(f);
+}, Q(initialVal));
+```
+
+Or, you could use the ultra-compact version:
+
+```javascript
+return funcs.reduce(Q.when, Q(initialVal));
+```
+
+### Handling Errors
+
+One sometimes-unintuive aspect of promises is that if you throw an
+exception in the fulfillment handler, it will not be caught by the error
+handler.
+
+```javascript
+return foo()
+.then(function (value) {
+ throw new Error("Can't bar.");
+}, function (error) {
+ // We only get here if "foo" fails
+});
+```
+
+To see why this is, consider the parallel between promises and
+``try``/``catch``. We are ``try``-ing to execute ``foo()``: the error
+handler represents a ``catch`` for ``foo()``, while the fulfillment handler
+represents code that happens *after* the ``try``/``catch`` block.
+That code then needs its own ``try``/``catch`` block.
+
+In terms of promises, this means chaining your rejection handler:
+
+```javascript
+return foo()
+.then(function (value) {
+ throw new Error("Can't bar.");
+})
+.fail(function (error) {
+ // We get here with either foo's error or bar's error
+});
+```
+
+### Progress Notification
+
+It's possible for promises to report their progress, e.g. for tasks that take a
+long time like a file upload. Not all promises will implement progress
+notifications, but for those that do, you can consume the progress values using
+a third parameter to ``then``:
+
+```javascript
+return uploadFile()
+.then(function () {
+ // Success uploading the file
+}, function (err) {
+ // There was an error, and we get the reason for error
+}, function (progress) {
+ // We get notified of the upload's progress as it is executed
+});
+```
+
+Like `fail`, Q also provides a shorthand for progress callbacks
+called `progress`:
+
+```javascript
+return uploadFile().progress(function (progress) {
+ // We get notified of the upload's progress
+});
+```
+
+### The End
+
+When you get to the end of a chain of promises, you should either
+return the last promise or end the chain. Since handlers catch
+errors, it’s an unfortunate pattern that the exceptions can go
+unobserved.
+
+So, either return it,
+
+```javascript
+return foo()
+.then(function () {
+ return "bar";
+});
+```
+
+Or, end it.
+
+```javascript
+foo()
+.then(function () {
+ return "bar";
+})
+.done();
+```
+
+Ending a promise chain makes sure that, if an error doesn’t get
+handled before the end, it will get rethrown and reported.
+
+This is a stopgap. We are exploring ways to make unhandled errors
+visible without any explicit handling.
+
+
+### The Beginning
+
+Everything above assumes you get a promise from somewhere else. This
+is the common case. Every once in a while, you will need to create a
+promise from scratch.
+
+#### Using ``Q.fcall``
+
+You can create a promise from a value using ``Q.fcall``. This returns a
+promise for 10.
+
+```javascript
+return Q.fcall(function () {
+ return 10;
+});
+```
+
+You can also use ``fcall`` to get a promise for an exception.
+
+```javascript
+return Q.fcall(function () {
+ throw new Error("Can't do it");
+});
+```
+
+As the name implies, ``fcall`` can call functions, or even promised
+functions. This uses the ``eventualAdd`` function above to add two
+numbers.
+
+```javascript
+return Q.fcall(eventualAdd, 2, 2);
+```
+
+
+#### Using Deferreds
+
+If you have to interface with asynchronous functions that are callback-based
+instead of promise-based, Q provides a few shortcuts (like ``Q.nfcall`` and
+friends). But much of the time, the solution will be to use *deferreds*.
+
+```javascript
+var deferred = Q.defer();
+FS.readFile("foo.txt", "utf-8", function (error, text) {
+ if (error) {
+ deferred.reject(new Error(error));
+ } else {
+ deferred.resolve(text);
+ }
+});
+return deferred.promise;
+```
+
+Note that a deferred can be resolved with a value or a promise. The
+``reject`` function is a shorthand for resolving with a rejected
+promise.
+
+```javascript
+// this:
+deferred.reject(new Error("Can't do it"));
+
+// is shorthand for:
+var rejection = Q.fcall(function () {
+ throw new Error("Can't do it");
+});
+deferred.resolve(rejection);
+```
+
+This is a simplified implementation of ``Q.delay``.
+
+```javascript
+function delay(ms) {
+ var deferred = Q.defer();
+ setTimeout(deferred.resolve, ms);
+ return deferred.promise;
+}
+```
+
+This is a simplified implementation of ``Q.timeout``
+
+```javascript
+function timeout(promise, ms) {
+ var deferred = Q.defer();
+ Q.when(promise, deferred.resolve);
+ delay(ms).then(function () {
+ deferred.reject(new Error("Timed out"));
+ });
+ return deferred.promise;
+}
+```
+
+Finally, you can send a progress notification to the promise with
+``deferred.notify``.
+
+For illustration, this is a wrapper for XML HTTP requests in the browser. Note
+that a more [thorough][XHR] implementation would be in order in practice.
+
+[XHR]: https://github.com/montagejs/mr/blob/71e8df99bb4f0584985accd6f2801ef3015b9763/browser.js#L29-L73
+
+```javascript
+function requestOkText(url) {
+ var request = new XMLHttpRequest();
+ var deferred = Q.defer();
+
+ request.open("GET", url, true);
+ request.onload = onload;
+ request.onerror = onerror;
+ request.onprogress = onprogress;
+ request.send();
+
+ function onload() {
+ if (request.status === 200) {
+ deferred.resolve(request.responseText);
+ } else {
+ deferred.reject(new Error("Status code was " + request.status));
+ }
+ }
+
+ function onerror() {
+ deferred.reject(new Error("Can't XHR " + JSON.stringify(url)));
+ }
+
+ function onprogress(event) {
+ deferred.notify(event.loaded / event.total);
+ }
+
+ return deferred.promise;
+}
+```
+
+Below is an example of how to use this ``requestOkText`` function:
+
+```javascript
+requestOkText("http://localhost:3000")
+.then(function (responseText) {
+ // If the HTTP response returns 200 OK, log the response text.
+ console.log(responseText);
+}, function (error) {
+ // If there's an error or a non-200 status code, log the error.
+ console.error(error);
+}, function (progress) {
+ // Log the progress as it comes in.
+ console.log("Request progress: " + Math.round(progress * 100) + "%");
+});
+```
+
+#### Using `Q.Promise`
+
+This is an alternative promise-creation API that has the same power as
+the deferred concept, but without introducing another conceptual entity.
+
+Rewriting the `requestOkText` example above using `Q.Promise`:
+
+```javascript
+function requestOkText(url) {
+ return Q.Promise(function(resolve, reject, notify) {
+ var request = new XMLHttpRequest();
+
+ request.open("GET", url, true);
+ request.onload = onload;
+ request.onerror = onerror;
+ request.onprogress = onprogress;
+ request.send();
+
+ function onload() {
+ if (request.status === 200) {
+ resolve(request.responseText);
+ } else {
+ reject(new Error("Status code was " + request.status));
+ }
+ }
+
+ function onerror() {
+ reject(new Error("Can't XHR " + JSON.stringify(url)));
+ }
+
+ function onprogress(event) {
+ notify(event.loaded / event.total);
+ }
+ });
+}
+```
+
+If `requestOkText` were to throw an exception, the returned promise would be
+rejected with that thrown exception as the rejection reason.
+
+### The Middle
+
+If you are using a function that may return a promise, but just might
+return a value if it doesn’t need to defer, you can use the “static”
+methods of the Q library.
+
+The ``when`` function is the static equivalent for ``then``.
+
+```javascript
+return Q.when(valueOrPromise, function (value) {
+}, function (error) {
+});
+```
+
+All of the other methods on a promise have static analogs with the
+same name.
+
+The following are equivalent:
+
+```javascript
+return Q.all([a, b]);
+```
+
+```javascript
+return Q.fcall(function () {
+ return [a, b];
+})
+.all();
+```
+
+When working with promises provided by other libraries, you should
+convert it to a Q promise. Not all promise libraries make the same
+guarantees as Q and certainly don’t provide all of the same methods.
+Most libraries only provide a partially functional ``then`` method.
+This thankfully is all we need to turn them into vibrant Q promises.
+
+```javascript
+return Q($.ajax(...))
+.then(function () {
+});
+```
+
+If there is any chance that the promise you receive is not a Q promise
+as provided by your library, you should wrap it using a Q function.
+You can even use ``Q.invoke`` as a shorthand.
+
+```javascript
+return Q.invoke($, 'ajax', ...)
+.then(function () {
+});
+```
+
+
+### Over the Wire
+
+A promise can serve as a proxy for another object, even a remote
+object. There are methods that allow you to optimistically manipulate
+properties or call functions. All of these interactions return
+promises, so they can be chained.
+
+```
+direct manipulation using a promise as a proxy
+-------------------------- -------------------------------
+value.foo promise.get("foo")
+value.foo = value promise.put("foo", value)
+delete value.foo promise.del("foo")
+value.foo(...args) promise.post("foo", [args])
+value.foo(...args) promise.invoke("foo", ...args)
+value(...args) promise.fapply([args])
+value(...args) promise.fcall(...args)
+```
+
+If the promise is a proxy for a remote object, you can shave
+round-trips by using these functions instead of ``then``. To take
+advantage of promises for remote objects, check out [Q-Connection][].
+
+[Q-Connection]: https://github.com/kriskowal/q-connection
+
+Even in the case of non-remote objects, these methods can be used as
+shorthand for particularly-simple fulfillment handlers. For example, you
+can replace
+
+```javascript
+return Q.fcall(function () {
+ return [{ foo: "bar" }, { foo: "baz" }];
+})
+.then(function (value) {
+ return value[0].foo;
+});
+```
+
+with
+
+```javascript
+return Q.fcall(function () {
+ return [{ foo: "bar" }, { foo: "baz" }];
+})
+.get(0)
+.get("foo");
+```
+
+
+### Adapting Node
+
+If you're working with functions that make use of the Node.js callback pattern,
+where callbacks are in the form of `function(err, result)`, Q provides a few
+useful utility functions for converting between them. The most straightforward
+are probably `Q.nfcall` and `Q.nfapply` ("Node function call/apply") for calling
+Node.js-style functions and getting back a promise:
+
+```javascript
+return Q.nfcall(FS.readFile, "foo.txt", "utf-8");
+return Q.nfapply(FS.readFile, ["foo.txt", "utf-8"]);
+```
+
+If you are working with methods, instead of simple functions, you can easily
+run in to the usual problems where passing a method to another function—like
+`Q.nfcall`—"un-binds" the method from its owner. To avoid this, you can either
+use `Function.prototype.bind` or some nice shortcut methods we provide:
+
+```javascript
+return Q.ninvoke(redisClient, "get", "user:1:id");
+return Q.npost(redisClient, "get", ["user:1:id"]);
+```
+
+You can also create reusable wrappers with `Q.denodeify` or `Q.nbind`:
+
+```javascript
+var readFile = Q.denodeify(FS.readFile);
+return readFile("foo.txt", "utf-8");
+
+var redisClientGet = Q.nbind(redisClient.get, redisClient);
+return redisClientGet("user:1:id");
+```
+
+Finally, if you're working with raw deferred objects, there is a
+`makeNodeResolver` method on deferreds that can be handy:
+
+```javascript
+var deferred = Q.defer();
+FS.readFile("foo.txt", "utf-8", deferred.makeNodeResolver());
+return deferred.promise;
+```
+
+### Long Stack Traces
+
+Q comes with optional support for “long stack traces,” wherein the `stack`
+property of `Error` rejection reasons is rewritten to be traced along
+asynchronous jumps instead of stopping at the most recent one. As an example:
+
+```js
+function theDepthsOfMyProgram() {
+ Q.delay(100).done(function explode() {
+ throw new Error("boo!");
+ });
+}
+
+theDepthsOfMyProgram();
+```
+
+usually would give a rather unhelpful stack trace looking something like
+
+```
+Error: boo!
+ at explode (/path/to/test.js:3:11)
+ at _fulfilled (/path/to/test.js:q:54)
+ at resolvedValue.promiseDispatch.done (/path/to/q.js:823:30)
+ at makePromise.promise.promiseDispatch (/path/to/q.js:496:13)
+ at pending (/path/to/q.js:397:39)
+ at process.startup.processNextTick.process._tickCallback (node.js:244:9)
+```
+
+But, if you turn this feature on by setting
+
+```js
+Q.longStackSupport = true;
+```
+
+then the above code gives a nice stack trace to the tune of
+
+```
+Error: boo!
+ at explode (/path/to/test.js:3:11)
+From previous event:
+ at theDepthsOfMyProgram (/path/to/test.js:2:16)
+ at Object.<anonymous> (/path/to/test.js:7:1)
+```
+
+Note how you can see the function that triggered the async operation in the
+stack trace! This is very helpful for debugging, as otherwise you end up getting
+only the first line, plus a bunch of Q internals, with no sign of where the
+operation started.
+
+In node.js, this feature can also be enabled through the Q_DEBUG environment
+variable:
+
+```
+Q_DEBUG=1 node server.js
+```
+
+This will enable long stack support in every instance of Q.
+
+This feature does come with somewhat-serious performance and memory overhead,
+however. If you're working with lots of promises, or trying to scale a server
+to many users, you should probably keep it off. But in development, go for it!
+
+## Tests
+
+You can view the results of the Q test suite [in your browser][tests]!
+
+[tests]: https://rawgithub.com/kriskowal/q/v1/spec/q-spec.html
+
+## License
+
+Copyright 2009–2015 Kristopher Michael Kowal and contributors
+MIT License (enclosed)
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/q/package.json
----------------------------------------------------------------------
diff --git a/node_modules/q/package.json b/node_modules/q/package.json
new file mode 100644
index 0000000..63689f4
--- /dev/null
+++ b/node_modules/q/package.json
@@ -0,0 +1,146 @@
+{
+ "_args": [
+ [
+ "q@^1.4.1",
+ "/Users/steveng/repo/cordova/cordova-osx"
+ ]
+ ],
+ "_from": "q@>=1.4.1 <2.0.0",
+ "_id": "q@1.4.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/q",
+ "_nodeVersion": "1.8.1",
+ "_npmUser": {
+ "email": "kris.kowal@cixar.com",
+ "name": "kriskowal"
+ },
+ "_npmVersion": "2.8.3",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "q",
+ "raw": "q@^1.4.1",
+ "rawSpec": "^1.4.1",
+ "scope": null,
+ "spec": ">=1.4.1 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/",
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/q/-/q-1.4.1.tgz",
+ "_shasum": "55705bcd93c5f3673530c2c2cbc0c2b3addc286e",
+ "_shrinkwrap": null,
+ "_spec": "q@^1.4.1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx",
+ "author": {
+ "email": "kris@cixar.com",
+ "name": "Kris Kowal",
+ "url": "https://github.com/kriskowal"
+ },
+ "bugs": {
+ "url": "http://github.com/kriskowal/q/issues"
+ },
+ "contributors": [
+ {
+ "name": "Kris Kowal",
+ "email": "kris@cixar.com",
+ "url": "https://github.com/kriskowal"
+ },
+ {
+ "name": "Irakli Gozalishvili",
+ "email": "rfobic@gmail.com",
+ "url": "http://jeditoolkit.com"
+ },
+ {
+ "name": "Domenic Denicola",
+ "email": "domenic@domenicdenicola.com",
+ "url": "http://domenicdenicola.com"
+ }
+ ],
+ "dependencies": {},
+ "description": "A library for promises (CommonJS/Promises/A,B,D)",
+ "devDependencies": {
+ "cover": "*",
+ "grunt": "~0.4.1",
+ "grunt-cli": "~0.1.9",
+ "grunt-contrib-uglify": "~0.9.1",
+ "jasmine-node": "1.11.0",
+ "jshint": "~2.1.9",
+ "matcha": "~0.2.0",
+ "opener": "*",
+ "promises-aplus-tests": "1.x"
+ },
+ "directories": {
+ "test": "./spec"
+ },
+ "dist": {
+ "shasum": "55705bcd93c5f3673530c2c2cbc0c2b3addc286e",
+ "tarball": "http://registry.npmjs.org/q/-/q-1.4.1.tgz"
+ },
+ "engines": {
+ "node": ">=0.6.0",
+ "teleport": ">=0.2.0"
+ },
+ "files": [
+ "LICENSE",
+ "q.js",
+ "queue.js"
+ ],
+ "gitHead": "d373079d3620152e3d60e82f27265a09ee0e81bd",
+ "homepage": "https://github.com/kriskowal/q",
+ "keywords": [
+ "async",
+ "browser",
+ "deferred",
+ "flow control",
+ "fluent",
+ "future",
+ "node",
+ "promise",
+ "promises",
+ "promises-a",
+ "promises-aplus",
+ "q"
+ ],
+ "license": {
+ "type": "MIT",
+ "url": "http://github.com/kriskowal/q/raw/master/LICENSE"
+ },
+ "main": "q.js",
+ "maintainers": [
+ {
+ "name": "kriskowal",
+ "email": "kris.kowal@cixar.com"
+ },
+ {
+ "name": "domenic",
+ "email": "domenic@domenicdenicola.com"
+ }
+ ],
+ "name": "q",
+ "optionalDependencies": {},
+ "overlay": {
+ "teleport": {
+ "dependencies": {
+ "system": ">=0.0.4"
+ }
+ }
+ },
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/kriskowal/q.git"
+ },
+ "scripts": {
+ "benchmark": "matcha",
+ "cover": "cover run jasmine-node spec && cover report html && opener cover_html/index.html",
+ "lint": "jshint q.js",
+ "minify": "grunt",
+ "prepublish": "grunt",
+ "test": "jasmine-node spec && promises-aplus-tests spec/aplus-adapter",
+ "test-browser": "opener spec/q-spec.html"
+ },
+ "version": "1.4.1"
+}
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[15/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/dist/plist-build.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/dist/plist-build.js b/node_modules/plist/dist/plist-build.js
new file mode 100644
index 0000000..4fcd378
--- /dev/null
+++ b/node_modules/plist/dist/plist-build.js
@@ -0,0 +1,3982 @@
+(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.plist = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){
+(function (Buffer){
+
+/**
+ * Module dependencies.
+ */
+
+var base64 = require('base64-js');
+var xmlbuilder = require('xmlbuilder');
+
+/**
+ * Module exports.
+ */
+
+exports.build = build;
+
+/**
+ * Accepts a `Date` instance and returns an ISO date string.
+ *
+ * @param {Date} d - Date instance to serialize
+ * @returns {String} ISO date string representation of `d`
+ * @api private
+ */
+
+function ISODateString(d){
+ function pad(n){
+ return n < 10 ? '0' + n : n;
+ }
+ return d.getUTCFullYear()+'-'
+ + pad(d.getUTCMonth()+1)+'-'
+ + pad(d.getUTCDate())+'T'
+ + pad(d.getUTCHours())+':'
+ + pad(d.getUTCMinutes())+':'
+ + pad(d.getUTCSeconds())+'Z';
+}
+
+/**
+ * Returns the internal "type" of `obj` via the
+ * `Object.prototype.toString()` trick.
+ *
+ * @param {Mixed} obj - any value
+ * @returns {String} the internal "type" name
+ * @api private
+ */
+
+var toString = Object.prototype.toString;
+function type (obj) {
+ var m = toString.call(obj).match(/\[object (.*)\]/);
+ return m ? m[1] : m;
+}
+
+/**
+ * Generate an XML plist string from the input object `obj`.
+ *
+ * @param {Object} obj - the object to convert
+ * @param {Object} [opts] - optional options object
+ * @returns {String} converted plist XML string
+ * @api public
+ */
+
+function build (obj, opts) {
+ var XMLHDR = {
+ version: '1.0',
+ encoding: 'UTF-8'
+ };
+
+ var XMLDTD = {
+ pubid: '-//Apple//DTD PLIST 1.0//EN',
+ sysid: 'http://www.apple.com/DTDs/PropertyList-1.0.dtd'
+ };
+
+ var doc = xmlbuilder.create('plist');
+
+ doc.dec(XMLHDR.version, XMLHDR.encoding, XMLHDR.standalone);
+ doc.dtd(XMLDTD.pubid, XMLDTD.sysid);
+ doc.att('version', '1.0');
+
+ walk_obj(obj, doc);
+
+ if (!opts) opts = {};
+ // default `pretty` to `true`
+ opts.pretty = opts.pretty !== false;
+ return doc.end(opts);
+}
+
+/**
+ * depth first, recursive traversal of a javascript object. when complete,
+ * next_child contains a reference to the build XML object.
+ *
+ * @api private
+ */
+
+function walk_obj(next, next_child) {
+ var tag_type, i, prop;
+ var name = type(next);
+
+ if ('Undefined' == name) {
+ return;
+ } else if (Array.isArray(next)) {
+ next_child = next_child.ele('array');
+ for (i = 0; i < next.length; i++) {
+ walk_obj(next[i], next_child);
+ }
+
+ } else if (Buffer.isBuffer(next)) {
+ next_child.ele('data').raw(next.toString('base64'));
+
+ } else if ('Object' == name) {
+ next_child = next_child.ele('dict');
+ for (prop in next) {
+ if (next.hasOwnProperty(prop)) {
+ next_child.ele('key').txt(prop);
+ walk_obj(next[prop], next_child);
+ }
+ }
+
+ } else if ('Number' == name) {
+ // detect if this is an integer or real
+ // TODO: add an ability to force one way or another via a "cast"
+ tag_type = (next % 1 === 0) ? 'integer' : 'real';
+ next_child.ele(tag_type).txt(next.toString());
+
+ } else if ('Date' == name) {
+ next_child.ele('date').txt(ISODateString(new Date(next)));
+
+ } else if ('Boolean' == name) {
+ next_child.ele(next ? 'true' : 'false');
+
+ } else if ('String' == name) {
+ next_child.ele('string').txt(next);
+
+ } else if ('ArrayBuffer' == name) {
+ next_child.ele('data').raw(base64.fromByteArray(next));
+
+ } else if (next && next.buffer && 'ArrayBuffer' == type(next.buffer)) {
+ // a typed array
+ next_child.ele('data').raw(base64.fromByteArray(new Uint8Array(next.buffer), next_child));
+
+ }
+}
+
+}).call(this,{"isBuffer":require("../node_modules/is-buffer/index.js")})
+},{"../node_modules/is-buffer/index.js":3,"base64-js":2,"xmlbuilder":79}],2:[function(require,module,exports){
+var lookup = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+;(function (exports) {
+ 'use strict';
+
+ var Arr = (typeof Uint8Array !== 'undefined')
+ ? Uint8Array
+ : Array
+
+ var PLUS = '+'.charCodeAt(0)
+ var SLASH = '/'.charCodeAt(0)
+ var NUMBER = '0'.charCodeAt(0)
+ var LOWER = 'a'.charCodeAt(0)
+ var UPPER = 'A'.charCodeAt(0)
+ var PLUS_URL_SAFE = '-'.charCodeAt(0)
+ var SLASH_URL_SAFE = '_'.charCodeAt(0)
+
+ function decode (elt) {
+ var code = elt.charCodeAt(0)
+ if (code === PLUS ||
+ code === PLUS_URL_SAFE)
+ return 62 // '+'
+ if (code === SLASH ||
+ code === SLASH_URL_SAFE)
+ return 63 // '/'
+ if (code < NUMBER)
+ return -1 //no match
+ if (code < NUMBER + 10)
+ return code - NUMBER + 26 + 26
+ if (code < UPPER + 26)
+ return code - UPPER
+ if (code < LOWER + 26)
+ return code - LOWER + 26
+ }
+
+ function b64ToByteArray (b64) {
+ var i, j, l, tmp, placeHolders, arr
+
+ if (b64.length % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4')
+ }
+
+ // the number of equal signs (place holders)
+ // if there are two placeholders, than the two characters before it
+ // represent one byte
+ // if there is only one, then the three characters before it represent 2 bytes
+ // this is just a cheap hack to not do indexOf twice
+ var len = b64.length
+ placeHolders = '=' === b64.charAt(len - 2) ? 2 : '=' === b64.charAt(len - 1) ? 1 : 0
+
+ // base64 is 4/3 + up to two characters of the original data
+ arr = new Arr(b64.length * 3 / 4 - placeHolders)
+
+ // if there are placeholders, only get up to the last complete 4 chars
+ l = placeHolders > 0 ? b64.length - 4 : b64.length
+
+ var L = 0
+
+ function push (v) {
+ arr[L++] = v
+ }
+
+ for (i = 0, j = 0; i < l; i += 4, j += 3) {
+ tmp = (decode(b64.charAt(i)) << 18) | (decode(b64.charAt(i + 1)) << 12) | (decode(b64.charAt(i + 2)) << 6) | decode(b64.charAt(i + 3))
+ push((tmp & 0xFF0000) >> 16)
+ push((tmp & 0xFF00) >> 8)
+ push(tmp & 0xFF)
+ }
+
+ if (placeHolders === 2) {
+ tmp = (decode(b64.charAt(i)) << 2) | (decode(b64.charAt(i + 1)) >> 4)
+ push(tmp & 0xFF)
+ } else if (placeHolders === 1) {
+ tmp = (decode(b64.charAt(i)) << 10) | (decode(b64.charAt(i + 1)) << 4) | (decode(b64.charAt(i + 2)) >> 2)
+ push((tmp >> 8) & 0xFF)
+ push(tmp & 0xFF)
+ }
+
+ return arr
+ }
+
+ function uint8ToBase64 (uint8) {
+ var i,
+ extraBytes = uint8.length % 3, // if we have 1 byte left, pad 2 bytes
+ output = "",
+ temp, length
+
+ function encode (num) {
+ return lookup.charAt(num)
+ }
+
+ function tripletToBase64 (num) {
+ return encode(num >> 18 & 0x3F) + encode(num >> 12 & 0x3F) + encode(num >> 6 & 0x3F) + encode(num & 0x3F)
+ }
+
+ // go through the array every three bytes, we'll deal with trailing stuff later
+ for (i = 0, length = uint8.length - extraBytes; i < length; i += 3) {
+ temp = (uint8[i] << 16) + (uint8[i + 1] << 8) + (uint8[i + 2])
+ output += tripletToBase64(temp)
+ }
+
+ // pad the end with zeros, but make sure to not forget the extra bytes
+ switch (extraBytes) {
+ case 1:
+ temp = uint8[uint8.length - 1]
+ output += encode(temp >> 2)
+ output += encode((temp << 4) & 0x3F)
+ output += '=='
+ break
+ case 2:
+ temp = (uint8[uint8.length - 2] << 8) + (uint8[uint8.length - 1])
+ output += encode(temp >> 10)
+ output += encode((temp >> 4) & 0x3F)
+ output += encode((temp << 2) & 0x3F)
+ output += '='
+ break
+ }
+
+ return output
+ }
+
+ exports.toByteArray = b64ToByteArray
+ exports.fromByteArray = uint8ToBase64
+}(typeof exports === 'undefined' ? (this.base64js = {}) : exports))
+
+},{}],3:[function(require,module,exports){
+/**
+ * Determine if an object is Buffer
+ *
+ * Author: Feross Aboukhadijeh <fe...@feross.org> <http://feross.org>
+ * License: MIT
+ *
+ * `npm install is-buffer`
+ */
+
+module.exports = function (obj) {
+ return !!(obj != null &&
+ (obj._isBuffer || // For Safari 5-7 (missing Object.prototype.constructor)
+ (obj.constructor &&
+ typeof obj.constructor.isBuffer === 'function' &&
+ obj.constructor.isBuffer(obj))
+ ))
+}
+
+},{}],4:[function(require,module,exports){
+/**
+ * Gets the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the last element of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ */
+function last(array) {
+ var length = array ? array.length : 0;
+ return length ? array[length - 1] : undefined;
+}
+
+module.exports = last;
+
+},{}],5:[function(require,module,exports){
+var arrayEvery = require('../internal/arrayEvery'),
+ baseCallback = require('../internal/baseCallback'),
+ baseEvery = require('../internal/baseEvery'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Checks if `predicate` returns truthy for **all** elements of `collection`.
+ * The predicate is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.every(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.every(users, 'active', false);
+ * // => true
+ *
+ * // using the `_.property` callback shorthand
+ * _.every(users, 'active');
+ * // => false
+ */
+function every(collection, predicate, thisArg) {
+ var func = isArray(collection) ? arrayEvery : baseEvery;
+ if (thisArg && isIterateeCall(collection, predicate, thisArg)) {
+ predicate = undefined;
+ }
+ if (typeof predicate != 'function' || thisArg !== undefined) {
+ predicate = baseCallback(predicate, thisArg, 3);
+ }
+ return func(collection, predicate);
+}
+
+module.exports = every;
+
+},{"../internal/arrayEvery":7,"../internal/baseCallback":11,"../internal/baseEvery":15,"../internal/isIterateeCall":40,"../lang/isArray":49}],6:[function(require,module,exports){
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of the
+ * created function and arguments from `start` and beyond provided as an array.
+ *
+ * **Note:** This method is based on the [rest parameter](https://developer.mozilla.org/Web/JavaScript/Reference/Functions/rest_parameters).
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.restParam(function(what, names) {
+ * return what + ' ' + _.initial(names).join(', ') +
+ * (_.size(names) > 1 ? ', & ' : '') + _.last(names);
+ * });
+ *
+ * say('hello', 'fred', 'barney', 'pebbles');
+ * // => 'hello fred, barney, & pebbles'
+ */
+function restParam(func, start) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ start = nativeMax(start === undefined ? (func.length - 1) : (+start || 0), 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ rest = Array(length);
+
+ while (++index < length) {
+ rest[index] = args[start + index];
+ }
+ switch (start) {
+ case 0: return func.call(this, rest);
+ case 1: return func.call(this, args[0], rest);
+ case 2: return func.call(this, args[0], args[1], rest);
+ }
+ var otherArgs = Array(start + 1);
+ index = -1;
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = rest;
+ return func.apply(this, otherArgs);
+ };
+}
+
+module.exports = restParam;
+
+},{}],7:[function(require,module,exports){
+/**
+ * A specialized version of `_.every` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ */
+function arrayEvery(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (!predicate(array[index], index, array)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = arrayEvery;
+
+},{}],8:[function(require,module,exports){
+/**
+ * A specialized version of `_.some` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+function arraySome(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (predicate(array[index], index, array)) {
+ return true;
+ }
+ }
+ return false;
+}
+
+module.exports = arraySome;
+
+},{}],9:[function(require,module,exports){
+var keys = require('../object/keys');
+
+/**
+ * A specialized version of `_.assign` for customizing assigned values without
+ * support for argument juggling, multiple sources, and `this` binding `customizer`
+ * functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ */
+function assignWith(object, source, customizer) {
+ var index = -1,
+ props = keys(source),
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index],
+ value = object[key],
+ result = customizer(value, source[key], key, object, source);
+
+ if ((result === result ? (result !== value) : (value === value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = result;
+ }
+ }
+ return object;
+}
+
+module.exports = assignWith;
+
+},{"../object/keys":58}],10:[function(require,module,exports){
+var baseCopy = require('./baseCopy'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.assign` without support for argument juggling,
+ * multiple sources, and `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssign(object, source) {
+ return source == null
+ ? object
+ : baseCopy(source, keys(source), object);
+}
+
+module.exports = baseAssign;
+
+},{"../object/keys":58,"./baseCopy":12}],11:[function(require,module,exports){
+var baseMatches = require('./baseMatches'),
+ baseMatchesProperty = require('./baseMatchesProperty'),
+ bindCallback = require('./bindCallback'),
+ identity = require('../utility/identity'),
+ property = require('../utility/property');
+
+/**
+ * The base implementation of `_.callback` which supports specifying the
+ * number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Returns the callback.
+ */
+function baseCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (type == 'function') {
+ return thisArg === undefined
+ ? func
+ : bindCallback(func, thisArg, argCount);
+ }
+ if (func == null) {
+ return identity;
+ }
+ if (type == 'object') {
+ return baseMatches(func);
+ }
+ return thisArg === undefined
+ ? property(func)
+ : baseMatchesProperty(func, thisArg);
+}
+
+module.exports = baseCallback;
+
+},{"../utility/identity":61,"../utility/property":62,"./baseMatches":22,"./baseMatchesProperty":23,"./bindCallback":28}],12:[function(require,module,exports){
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property names to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @returns {Object} Returns `object`.
+ */
+function baseCopy(source, props, object) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ object[key] = source[key];
+ }
+ return object;
+}
+
+module.exports = baseCopy;
+
+},{}],13:[function(require,module,exports){
+var isObject = require('../lang/isObject');
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+var baseCreate = (function() {
+ function object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ object.prototype = prototype;
+ var result = new object;
+ object.prototype = undefined;
+ }
+ return result || {};
+ };
+}());
+
+module.exports = baseCreate;
+
+},{"../lang/isObject":53}],14:[function(require,module,exports){
+var baseForOwn = require('./baseForOwn'),
+ createBaseEach = require('./createBaseEach');
+
+/**
+ * The base implementation of `_.forEach` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+var baseEach = createBaseEach(baseForOwn);
+
+module.exports = baseEach;
+
+},{"./baseForOwn":17,"./createBaseEach":30}],15:[function(require,module,exports){
+var baseEach = require('./baseEach');
+
+/**
+ * The base implementation of `_.every` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+}
+
+module.exports = baseEvery;
+
+},{"./baseEach":14}],16:[function(require,module,exports){
+var createBaseFor = require('./createBaseFor');
+
+/**
+ * The base implementation of `baseForIn` and `baseForOwn` which iterates
+ * over `object` properties returned by `keysFunc` invoking `iteratee` for
+ * each property. Iteratee functions may exit iteration early by explicitly
+ * returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseFor = createBaseFor();
+
+module.exports = baseFor;
+
+},{"./createBaseFor":31}],17:[function(require,module,exports){
+var baseFor = require('./baseFor'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.forOwn` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForOwn(object, iteratee) {
+ return baseFor(object, iteratee, keys);
+}
+
+module.exports = baseForOwn;
+
+},{"../object/keys":58,"./baseFor":16}],18:[function(require,module,exports){
+var toObject = require('./toObject');
+
+/**
+ * The base implementation of `get` without support for string paths
+ * and default values.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} path The path of the property to get.
+ * @param {string} [pathKey] The key representation of path.
+ * @returns {*} Returns the resolved value.
+ */
+function baseGet(object, path, pathKey) {
+ if (object == null) {
+ return;
+ }
+ if (pathKey !== undefined && pathKey in toObject(object)) {
+ path = [pathKey];
+ }
+ var index = 0,
+ length = path.length;
+
+ while (object != null && index < length) {
+ object = object[path[index++]];
+ }
+ return (index && index == length) ? object : undefined;
+}
+
+module.exports = baseGet;
+
+},{"./toObject":46}],19:[function(require,module,exports){
+var baseIsEqualDeep = require('./baseIsEqualDeep'),
+ isObject = require('../lang/isObject'),
+ isObjectLike = require('./isObjectLike');
+
+/**
+ * The base implementation of `_.isEqual` without support for `this` binding
+ * `customizer` functions.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize comparing values.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(value, other, customizer, isLoose, stackA, stackB) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, baseIsEqual, customizer, isLoose, stackA, stackB);
+}
+
+module.exports = baseIsEqual;
+
+},{"../lang/isObject":53,"./baseIsEqualDeep":20,"./isObjectLike":43}],20:[function(require,module,exports){
+var equalArrays = require('./equalArrays'),
+ equalByTag = require('./equalByTag'),
+ equalObjects = require('./equalObjects'),
+ isArray = require('../lang/isArray'),
+ isTypedArray = require('../lang/isTypedArray');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ objectTag = '[object Object]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `value` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function baseIsEqualDeep(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = arrayTag,
+ othTag = arrayTag;
+
+ if (!objIsArr) {
+ objTag = objToString.call(object);
+ if (objTag == argsTag) {
+ objTag = objectTag;
+ } else if (objTag != objectTag) {
+ objIsArr = isTypedArray(object);
+ }
+ }
+ if (!othIsArr) {
+ othTag = objToString.call(other);
+ if (othTag == argsTag) {
+ othTag = objectTag;
+ } else if (othTag != objectTag) {
+ othIsArr = isTypedArray(other);
+ }
+ }
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ if (isSameTag && !(objIsArr || objIsObj)) {
+ return equalByTag(object, other, objTag);
+ }
+ if (!isLoose) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ return equalFunc(objIsWrapped ? object.value() : object, othIsWrapped ? other.value() : other, customizer, isLoose, stackA, stackB);
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ // Assume cyclic values are equal.
+ // For more information on detecting circular references see https://es5.github.io/#JO.
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == object) {
+ return stackB[length] == other;
+ }
+ }
+ // Add `object` and `other` to the stack of traversed objects.
+ stackA.push(object);
+ stackB.push(other);
+
+ var result = (objIsArr ? equalArrays : equalObjects)(object, other, equalFunc, customizer, isLoose, stackA, stackB);
+
+ stackA.pop();
+ stackB.pop();
+
+ return result;
+}
+
+module.exports = baseIsEqualDeep;
+
+},{"../lang/isArray":49,"../lang/isTypedArray":55,"./equalArrays":32,"./equalByTag":33,"./equalObjects":34}],21:[function(require,module,exports){
+var baseIsEqual = require('./baseIsEqual'),
+ toObject = require('./toObject');
+
+/**
+ * The base implementation of `_.isMatch` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} matchData The propery names, values, and compare flags to match.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ */
+function baseIsMatch(object, matchData, customizer) {
+ var index = matchData.length,
+ length = index,
+ noCustomizer = !customizer;
+
+ if (object == null) {
+ return !length;
+ }
+ object = toObject(object);
+ while (index--) {
+ var data = matchData[index];
+ if ((noCustomizer && data[2])
+ ? data[1] !== object[data[0]]
+ : !(data[0] in object)
+ ) {
+ return false;
+ }
+ }
+ while (++index < length) {
+ data = matchData[index];
+ var key = data[0],
+ objValue = object[key],
+ srcValue = data[1];
+
+ if (noCustomizer && data[2]) {
+ if (objValue === undefined && !(key in object)) {
+ return false;
+ }
+ } else {
+ var result = customizer ? customizer(objValue, srcValue, key) : undefined;
+ if (!(result === undefined ? baseIsEqual(srcValue, objValue, customizer, true) : result)) {
+ return false;
+ }
+ }
+ }
+ return true;
+}
+
+module.exports = baseIsMatch;
+
+},{"./baseIsEqual":19,"./toObject":46}],22:[function(require,module,exports){
+var baseIsMatch = require('./baseIsMatch'),
+ getMatchData = require('./getMatchData'),
+ toObject = require('./toObject');
+
+/**
+ * The base implementation of `_.matches` which does not clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new function.
+ */
+function baseMatches(source) {
+ var matchData = getMatchData(source);
+ if (matchData.length == 1 && matchData[0][2]) {
+ var key = matchData[0][0],
+ value = matchData[0][1];
+
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ return object[key] === value && (value !== undefined || (key in toObject(object)));
+ };
+ }
+ return function(object) {
+ return baseIsMatch(object, matchData);
+ };
+}
+
+module.exports = baseMatches;
+
+},{"./baseIsMatch":21,"./getMatchData":36,"./toObject":46}],23:[function(require,module,exports){
+var baseGet = require('./baseGet'),
+ baseIsEqual = require('./baseIsEqual'),
+ baseSlice = require('./baseSlice'),
+ isArray = require('../lang/isArray'),
+ isKey = require('./isKey'),
+ isStrictComparable = require('./isStrictComparable'),
+ last = require('../array/last'),
+ toObject = require('./toObject'),
+ toPath = require('./toPath');
+
+/**
+ * The base implementation of `_.matchesProperty` which does not clone `srcValue`.
+ *
+ * @private
+ * @param {string} path The path of the property to get.
+ * @param {*} srcValue The value to compare.
+ * @returns {Function} Returns the new function.
+ */
+function baseMatchesProperty(path, srcValue) {
+ var isArr = isArray(path),
+ isCommon = isKey(path) && isStrictComparable(srcValue),
+ pathKey = (path + '');
+
+ path = toPath(path);
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ var key = pathKey;
+ object = toObject(object);
+ if ((isArr || !isCommon) && !(key in object)) {
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ if (object == null) {
+ return false;
+ }
+ key = last(path);
+ object = toObject(object);
+ }
+ return object[key] === srcValue
+ ? (srcValue !== undefined || (key in object))
+ : baseIsEqual(srcValue, object[key], undefined, true);
+ };
+}
+
+module.exports = baseMatchesProperty;
+
+},{"../array/last":4,"../lang/isArray":49,"./baseGet":18,"./baseIsEqual":19,"./baseSlice":26,"./isKey":41,"./isStrictComparable":44,"./toObject":46,"./toPath":47}],24:[function(require,module,exports){
+/**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+module.exports = baseProperty;
+
+},{}],25:[function(require,module,exports){
+var baseGet = require('./baseGet'),
+ toPath = require('./toPath');
+
+/**
+ * A specialized version of `baseProperty` which supports deep paths.
+ *
+ * @private
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function basePropertyDeep(path) {
+ var pathKey = (path + '');
+ path = toPath(path);
+ return function(object) {
+ return baseGet(object, path, pathKey);
+ };
+}
+
+module.exports = basePropertyDeep;
+
+},{"./baseGet":18,"./toPath":47}],26:[function(require,module,exports){
+/**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ start = start == null ? 0 : (+start || 0);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : (+end || 0);
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+}
+
+module.exports = baseSlice;
+
+},{}],27:[function(require,module,exports){
+/**
+ * Converts `value` to a string if it's not one. An empty string is returned
+ * for `null` or `undefined` values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+function baseToString(value) {
+ return value == null ? '' : (value + '');
+}
+
+module.exports = baseToString;
+
+},{}],28:[function(require,module,exports){
+var identity = require('../utility/identity');
+
+/**
+ * A specialized version of `baseCallback` which only supports `this` binding
+ * and specifying the number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Returns the callback.
+ */
+function bindCallback(func, thisArg, argCount) {
+ if (typeof func != 'function') {
+ return identity;
+ }
+ if (thisArg === undefined) {
+ return func;
+ }
+ switch (argCount) {
+ case 1: return function(value) {
+ return func.call(thisArg, value);
+ };
+ case 3: return function(value, index, collection) {
+ return func.call(thisArg, value, index, collection);
+ };
+ case 4: return function(accumulator, value, index, collection) {
+ return func.call(thisArg, accumulator, value, index, collection);
+ };
+ case 5: return function(value, other, key, object, source) {
+ return func.call(thisArg, value, other, key, object, source);
+ };
+ }
+ return function() {
+ return func.apply(thisArg, arguments);
+ };
+}
+
+module.exports = bindCallback;
+
+},{"../utility/identity":61}],29:[function(require,module,exports){
+var bindCallback = require('./bindCallback'),
+ isIterateeCall = require('./isIterateeCall'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates a `_.assign`, `_.defaults`, or `_.merge` function.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+function createAssigner(assigner) {
+ return restParam(function(object, sources) {
+ var index = -1,
+ length = object == null ? 0 : sources.length,
+ customizer = length > 2 ? sources[length - 2] : undefined,
+ guard = length > 2 ? sources[2] : undefined,
+ thisArg = length > 1 ? sources[length - 1] : undefined;
+
+ if (typeof customizer == 'function') {
+ customizer = bindCallback(customizer, thisArg, 5);
+ length -= 2;
+ } else {
+ customizer = typeof thisArg == 'function' ? thisArg : undefined;
+ length -= (customizer ? 1 : 0);
+ }
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ customizer = length < 3 ? undefined : customizer;
+ length = 1;
+ }
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, customizer);
+ }
+ }
+ return object;
+ });
+}
+
+module.exports = createAssigner;
+
+},{"../function/restParam":6,"./bindCallback":28,"./isIterateeCall":40}],30:[function(require,module,exports){
+var getLength = require('./getLength'),
+ isLength = require('./isLength'),
+ toObject = require('./toObject');
+
+/**
+ * Creates a `baseEach` or `baseEachRight` function.
+ *
+ * @private
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseEach(eachFunc, fromRight) {
+ return function(collection, iteratee) {
+ var length = collection ? getLength(collection) : 0;
+ if (!isLength(length)) {
+ return eachFunc(collection, iteratee);
+ }
+ var index = fromRight ? length : -1,
+ iterable = toObject(collection);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (iteratee(iterable[index], index, iterable) === false) {
+ break;
+ }
+ }
+ return collection;
+ };
+}
+
+module.exports = createBaseEach;
+
+},{"./getLength":35,"./isLength":42,"./toObject":46}],31:[function(require,module,exports){
+var toObject = require('./toObject');
+
+/**
+ * Creates a base function for `_.forIn` or `_.forInRight`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseFor(fromRight) {
+ return function(object, iteratee, keysFunc) {
+ var iterable = toObject(object),
+ props = keysFunc(object),
+ length = props.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length)) {
+ var key = props[index];
+ if (iteratee(iterable[key], key, iterable) === false) {
+ break;
+ }
+ }
+ return object;
+ };
+}
+
+module.exports = createBaseFor;
+
+},{"./toObject":46}],32:[function(require,module,exports){
+var arraySome = require('./arraySome');
+
+/**
+ * A specialized version of `baseIsEqualDeep` for arrays with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Array} array The array to compare.
+ * @param {Array} other The other array to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing arrays.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
+ */
+function equalArrays(array, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var index = -1,
+ arrLength = array.length,
+ othLength = other.length;
+
+ if (arrLength != othLength && !(isLoose && othLength > arrLength)) {
+ return false;
+ }
+ // Ignore non-index properties.
+ while (++index < arrLength) {
+ var arrValue = array[index],
+ othValue = other[index],
+ result = customizer ? customizer(isLoose ? othValue : arrValue, isLoose ? arrValue : othValue, index) : undefined;
+
+ if (result !== undefined) {
+ if (result) {
+ continue;
+ }
+ return false;
+ }
+ // Recursively compare arrays (susceptible to call stack limits).
+ if (isLoose) {
+ if (!arraySome(other, function(othValue) {
+ return arrValue === othValue || equalFunc(arrValue, othValue, customizer, isLoose, stackA, stackB);
+ })) {
+ return false;
+ }
+ } else if (!(arrValue === othValue || equalFunc(arrValue, othValue, customizer, isLoose, stackA, stackB))) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = equalArrays;
+
+},{"./arraySome":8}],33:[function(require,module,exports){
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ numberTag = '[object Number]',
+ regexpTag = '[object RegExp]',
+ stringTag = '[object String]';
+
+/**
+ * A specialized version of `baseIsEqualDeep` for comparing objects of
+ * the same `toStringTag`.
+ *
+ * **Note:** This function only supports comparing values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {string} tag The `toStringTag` of the objects to compare.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function equalByTag(object, other, tag) {
+ switch (tag) {
+ case boolTag:
+ case dateTag:
+ // Coerce dates and booleans to numbers, dates to milliseconds and booleans
+ // to `1` or `0` treating invalid dates coerced to `NaN` as not equal.
+ return +object == +other;
+
+ case errorTag:
+ return object.name == other.name && object.message == other.message;
+
+ case numberTag:
+ // Treat `NaN` vs. `NaN` as equal.
+ return (object != +object)
+ ? other != +other
+ : object == +other;
+
+ case regexpTag:
+ case stringTag:
+ // Coerce regexes to strings and treat strings primitives and string
+ // objects as equal. See https://es5.github.io/#x15.10.6.4 for more details.
+ return object == (other + '');
+ }
+ return false;
+}
+
+module.exports = equalByTag;
+
+},{}],34:[function(require,module,exports){
+var keys = require('../object/keys');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A specialized version of `baseIsEqualDeep` for objects with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing values.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function equalObjects(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var objProps = keys(object),
+ objLength = objProps.length,
+ othProps = keys(other),
+ othLength = othProps.length;
+
+ if (objLength != othLength && !isLoose) {
+ return false;
+ }
+ var index = objLength;
+ while (index--) {
+ var key = objProps[index];
+ if (!(isLoose ? key in other : hasOwnProperty.call(other, key))) {
+ return false;
+ }
+ }
+ var skipCtor = isLoose;
+ while (++index < objLength) {
+ key = objProps[index];
+ var objValue = object[key],
+ othValue = other[key],
+ result = customizer ? customizer(isLoose ? othValue : objValue, isLoose? objValue : othValue, key) : undefined;
+
+ // Recursively compare objects (susceptible to call stack limits).
+ if (!(result === undefined ? equalFunc(objValue, othValue, customizer, isLoose, stackA, stackB) : result)) {
+ return false;
+ }
+ skipCtor || (skipCtor = key == 'constructor');
+ }
+ if (!skipCtor) {
+ var objCtor = object.constructor,
+ othCtor = other.constructor;
+
+ // Non `Object` object instances with different constructors are not equal.
+ if (objCtor != othCtor &&
+ ('constructor' in object && 'constructor' in other) &&
+ !(typeof objCtor == 'function' && objCtor instanceof objCtor &&
+ typeof othCtor == 'function' && othCtor instanceof othCtor)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = equalObjects;
+
+},{"../object/keys":58}],35:[function(require,module,exports){
+var baseProperty = require('./baseProperty');
+
+/**
+ * Gets the "length" property value of `object`.
+ *
+ * **Note:** This function is used to avoid a [JIT bug](https://bugs.webkit.org/show_bug.cgi?id=142792)
+ * that affects Safari on at least iOS 8.1-8.3 ARM64.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {*} Returns the "length" value.
+ */
+var getLength = baseProperty('length');
+
+module.exports = getLength;
+
+},{"./baseProperty":24}],36:[function(require,module,exports){
+var isStrictComparable = require('./isStrictComparable'),
+ pairs = require('../object/pairs');
+
+/**
+ * Gets the propery names, values, and compare flags of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the match data of `object`.
+ */
+function getMatchData(object) {
+ var result = pairs(object),
+ length = result.length;
+
+ while (length--) {
+ result[length][2] = isStrictComparable(result[length][1]);
+ }
+ return result;
+}
+
+module.exports = getMatchData;
+
+},{"../object/pairs":60,"./isStrictComparable":44}],37:[function(require,module,exports){
+var isNative = require('../lang/isNative');
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = object == null ? undefined : object[key];
+ return isNative(value) ? value : undefined;
+}
+
+module.exports = getNative;
+
+},{"../lang/isNative":52}],38:[function(require,module,exports){
+var getLength = require('./getLength'),
+ isLength = require('./isLength');
+
+/**
+ * Checks if `value` is array-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ */
+function isArrayLike(value) {
+ return value != null && isLength(getLength(value));
+}
+
+module.exports = isArrayLike;
+
+},{"./getLength":35,"./isLength":42}],39:[function(require,module,exports){
+/** Used to detect unsigned integer values. */
+var reIsUint = /^\d+$/;
+
+/**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ value = (typeof value == 'number' || reIsUint.test(value)) ? +value : -1;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+ return value > -1 && value % 1 == 0 && value < length;
+}
+
+module.exports = isIndex;
+
+},{}],40:[function(require,module,exports){
+var isArrayLike = require('./isArrayLike'),
+ isIndex = require('./isIndex'),
+ isObject = require('../lang/isObject');
+
+/**
+ * Checks if the provided arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call, else `false`.
+ */
+function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)) {
+ var other = object[index];
+ return value === value ? (value === other) : (other !== other);
+ }
+ return false;
+}
+
+module.exports = isIterateeCall;
+
+},{"../lang/isObject":53,"./isArrayLike":38,"./isIndex":39}],41:[function(require,module,exports){
+var isArray = require('../lang/isArray'),
+ toObject = require('./toObject');
+
+/** Used to match property names within property paths. */
+var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\n\\]|\\.)*?\1)\]/,
+ reIsPlainProp = /^\w*$/;
+
+/**
+ * Checks if `value` is a property name and not a property path.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {Object} [object] The object to query keys on.
+ * @returns {boolean} Returns `true` if `value` is a property name, else `false`.
+ */
+function isKey(value, object) {
+ var type = typeof value;
+ if ((type == 'string' && reIsPlainProp.test(value)) || type == 'number') {
+ return true;
+ }
+ if (isArray(value)) {
+ return false;
+ }
+ var result = !reIsDeepProp.test(value);
+ return result || (object != null && value in toObject(object));
+}
+
+module.exports = isKey;
+
+},{"../lang/isArray":49,"./toObject":46}],42:[function(require,module,exports){
+/**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This function is based on [`ToLength`](http://ecma-international.org/ecma-262/6.0/#sec-tolength).
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ */
+function isLength(value) {
+ return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+module.exports = isLength;
+
+},{}],43:[function(require,module,exports){
+/**
+ * Checks if `value` is object-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+module.exports = isObjectLike;
+
+},{}],44:[function(require,module,exports){
+var isObject = require('../lang/isObject');
+
+/**
+ * Checks if `value` is suitable for strict equality comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` if suitable for strict
+ * equality comparisons, else `false`.
+ */
+function isStrictComparable(value) {
+ return value === value && !isObject(value);
+}
+
+module.exports = isStrictComparable;
+
+},{"../lang/isObject":53}],45:[function(require,module,exports){
+var isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isIndex = require('./isIndex'),
+ isLength = require('./isLength'),
+ keysIn = require('../object/keysIn');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A fallback implementation of `Object.keys` which creates an array of the
+ * own enumerable property names of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function shimKeys(object) {
+ var props = keysIn(object),
+ propsLength = props.length,
+ length = propsLength && object.length;
+
+ var allowIndexes = !!length && isLength(length) &&
+ (isArray(object) || isArguments(object));
+
+ var index = -1,
+ result = [];
+
+ while (++index < propsLength) {
+ var key = props[index];
+ if ((allowIndexes && isIndex(key, length)) || hasOwnProperty.call(object, key)) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = shimKeys;
+
+},{"../lang/isArguments":48,"../lang/isArray":49,"../object/keysIn":59,"./isIndex":39,"./isLength":42}],46:[function(require,module,exports){
+var isObject = require('../lang/isObject');
+
+/**
+ * Converts `value` to an object if it's not one.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {Object} Returns the object.
+ */
+function toObject(value) {
+ return isObject(value) ? value : Object(value);
+}
+
+module.exports = toObject;
+
+},{"../lang/isObject":53}],47:[function(require,module,exports){
+var baseToString = require('./baseToString'),
+ isArray = require('../lang/isArray');
+
+/** Used to match property names within property paths. */
+var rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\n\\]|\\.)*?)\2)\]/g;
+
+/** Used to match backslashes in property paths. */
+var reEscapeChar = /\\(\\)?/g;
+
+/**
+ * Converts `value` to property path array if it's not one.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {Array} Returns the property path array.
+ */
+function toPath(value) {
+ if (isArray(value)) {
+ return value;
+ }
+ var result = [];
+ baseToString(value).replace(rePropName, function(match, number, quote, string) {
+ result.push(quote ? string.replace(reEscapeChar, '$1') : (number || match));
+ });
+ return result;
+}
+
+module.exports = toPath;
+
+},{"../lang/isArray":49,"./baseToString":27}],48:[function(require,module,exports){
+var isArrayLike = require('../internal/isArrayLike'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Native method references. */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * Checks if `value` is classified as an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ return isObjectLike(value) && isArrayLike(value) &&
+ hasOwnProperty.call(value, 'callee') && !propertyIsEnumerable.call(value, 'callee');
+}
+
+module.exports = isArguments;
+
+},{"../internal/isArrayLike":38,"../internal/isObjectLike":43}],49:[function(require,module,exports){
+var getNative = require('../internal/getNative'),
+ isLength = require('../internal/isLength'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var arrayTag = '[object Array]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeIsArray = getNative(Array, 'isArray');
+
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(function() { return arguments; }());
+ * // => false
+ */
+var isArray = nativeIsArray || function(value) {
+ return isObjectLike(value) && isLength(value.length) && objToString.call(value) == arrayTag;
+};
+
+module.exports = isArray;
+
+},{"../internal/getNative":37,"../internal/isLength":42,"../internal/isObjectLike":43}],50:[function(require,module,exports){
+var isArguments = require('./isArguments'),
+ isArray = require('./isArray'),
+ isArrayLike = require('../internal/isArrayLike'),
+ isFunction = require('./isFunction'),
+ isObjectLike = require('../internal/isObjectLike'),
+ isString = require('./isString'),
+ keys = require('../object/keys');
+
+/**
+ * Checks if `value` is empty. A value is considered empty unless it's an
+ * `arguments` object, array, string, or jQuery-like collection with a length
+ * greater than `0` or an object with own enumerable properties.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {Array|Object|string} value The value to inspect.
+ * @returns {boolean} Returns `true` if `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty(null);
+ * // => true
+ *
+ * _.isEmpty(true);
+ * // => true
+ *
+ * _.isEmpty(1);
+ * // => true
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({ 'a': 1 });
+ * // => false
+ */
+function isEmpty(value) {
+ if (value == null) {
+ return true;
+ }
+ if (isArrayLike(value) && (isArray(value) || isString(value) || isArguments(value) ||
+ (isObjectLike(value) && isFunction(value.splice)))) {
+ return !value.length;
+ }
+ return !keys(value).length;
+}
+
+module.exports = isEmpty;
+
+},{"../internal/isArrayLike":38,"../internal/isObjectLike":43,"../object/keys":58,"./isArguments":48,"./isArray":49,"./isFunction":51,"./isString":54}],51:[function(require,module,exports){
+var isObject = require('./isObject');
+
+/** `Object#toString` result references. */
+var funcTag = '[object Function]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in older versions of Chrome and Safari which return 'function' for regexes
+ // and Safari 8 which returns 'object' for typed array constructors.
+ return isObject(value) && objToString.call(value) == funcTag;
+}
+
+module.exports = isFunction;
+
+},{"./isObject":53}],52:[function(require,module,exports){
+var isFunction = require('./isFunction'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** Used to detect host constructors (Safari > 5). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var fnToString = Function.prototype.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ fnToString.call(hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function, else `false`.
+ * @example
+ *
+ * _.isNative(Array.prototype.push);
+ * // => true
+ *
+ * _.isNative(_);
+ * // => false
+ */
+function isNative(value) {
+ if (value == null) {
+ return false;
+ }
+ if (isFunction(value)) {
+ return reIsNative.test(fnToString.call(value));
+ }
+ return isObjectLike(value) && reIsHostCtor.test(value);
+}
+
+module.exports = isNative;
+
+},{"../internal/isObjectLike":43,"./isFunction":51}],53:[function(require,module,exports){
+/**
+ * Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // Avoid a V8 JIT bug in Chrome 19-20.
+ // See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+module.exports = isObject;
+
+},{}],54:[function(require,module,exports){
+var isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var stringTag = '[object String]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a `String` primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isString('abc');
+ * // => true
+ *
+ * _.isString(1);
+ * // => false
+ */
+function isString(value) {
+ return typeof value == 'string' || (isObjectLike(value) && objToString.call(value) == stringTag);
+}
+
+module.exports = isString;
+
+},{"../internal/isObjectLike":43}],55:[function(require,module,exports){
+var isLength = require('../internal/isLength'),
+ isObjectLike = require('../internal/isObjectLike');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/** Used to identify `toStringTag` values of typed arrays. */
+var typedArrayTags = {};
+typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
+typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
+typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
+typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
+typedArrayTags[uint32Tag] = true;
+typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
+typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
+typedArrayTags[dateTag] = typedArrayTags[errorTag] =
+typedArrayTags[funcTag] = typedArrayTags[mapTag] =
+typedArrayTags[numberTag] = typedArrayTags[objectTag] =
+typedArrayTags[regexpTag] = typedArrayTags[setTag] =
+typedArrayTags[stringTag] = typedArrayTags[weakMapTag] = false;
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Checks if `value` is classified as a typed array.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isTypedArray(new Uint8Array);
+ * // => true
+ *
+ * _.isTypedArray([]);
+ * // => false
+ */
+function isTypedArray(value) {
+ return isObjectLike(value) && isLength(value.length) && !!typedArrayTags[objToString.call(value)];
+}
+
+module.exports = isTypedArray;
+
+},{"../internal/isLength":42,"../internal/isObjectLike":43}],56:[function(require,module,exports){
+var assignWith = require('../internal/assignWith'),
+ baseAssign = require('../internal/baseAssign'),
+ createAssigner = require('../internal/createAssigner');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object. Subsequent sources overwrite property assignments of previous sources.
+ * If `customizer` is provided it's invoked to produce the assigned values.
+ * The `customizer` is bound to `thisArg` and invoked with five arguments:
+ * (objectValue, sourceValue, key, object, source).
+ *
+ * **Note:** This method mutates `object` and is based on
+ * [`Object.assign`](http://ecma-international.org/ecma-262/6.0/#sec-object.assign).
+ *
+ * @static
+ * @memberOf _
+ * @alias extend
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.assign({ 'user': 'barney' }, { 'age': 40 }, { 'user': 'fred' });
+ * // => { 'user': 'fred', 'age': 40 }
+ *
+ * // using a customizer callback
+ * var defaults = _.partialRight(_.assign, function(value, other) {
+ * return _.isUndefined(value) ? other : value;
+ * });
+ *
+ * defaults({ 'user': 'barney' }, { 'age': 36 }, { 'user': 'fred' });
+ * // => { 'user': 'barney', 'age': 36 }
+ */
+var assign = createAssigner(function(object, source, customizer) {
+ return customizer
+ ? assignWith(object, source, customizer)
+ : baseAssign(object, source);
+});
+
+module.exports = assign;
+
+},{"../internal/assignWith":9,"../internal/baseAssign":10,"../internal/createAssigner":29}],57:[function(require,module,exports){
+var baseAssign = require('../internal/baseAssign'),
+ baseCreate = require('../internal/baseCreate'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates an object that inherits from the given `prototype` object. If a
+ * `properties` object is provided its own enumerable properties are assigned
+ * to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, {
+ * 'constructor': Circle
+ * });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+function create(prototype, properties, guard) {
+ var result = baseCreate(prototype);
+ if (guard && isIterateeCall(prototype, properties, guard)) {
+ properties = undefined;
+ }
+ return properties ? baseAssign(result, properties) : result;
+}
+
+module.exports = create;
+
+},{"../internal/baseAssign":10,"../internal/baseCreate":13,"../internal/isIterateeCall":40}],58:[function(require,module,exports){
+var getNative = require('../internal/getNative'),
+ isArrayLike = require('../internal/isArrayLike'),
+ isObject = require('../lang/isObject'),
+ shimKeys = require('../internal/shimKeys');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeKeys = getNative(Object, 'keys');
+
+/**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/6.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+var keys = !nativeKeys ? shimKeys : function(object) {
+ var Ctor = object == null ? undefined : object.constructor;
+ if ((typeof Ctor == 'function' && Ctor.prototype === object) ||
+ (typeof object != 'function' && isArrayLike(object))) {
+ return shimKeys(object);
+ }
+ return isObject(object) ? nativeKeys(object) : [];
+};
+
+module.exports = keys;
+
+},{"../internal/getNative":37,"../internal/isArrayLike":38,"../internal/shimKeys":45,"../lang/isObject":53}],59:[function(require,module,exports){
+var isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isIndex = require('../internal/isIndex'),
+ isLength = require('../internal/isLength'),
+ isObject = require('../lang/isObject');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+function keysIn(object) {
+ if (object == null) {
+ return [];
+ }
+ if (!isObject(object)) {
+ object = Object(object);
+ }
+ var length = object.length;
+ length = (length && isLength(length) &&
+ (isArray(object) || isArguments(object)) && length) || 0;
+
+ var Ctor = object.constructor,
+ index = -1,
+ isProto = typeof Ctor == 'function' && Ctor.prototype === object,
+ result = Array(length),
+ skipIndexes = length > 0;
+
+ while (++index < length) {
+ result[index] = (index + '');
+ }
+ for (var key in object) {
+ if (!(skipIndexes && isIndex(key, length)) &&
+ !(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = keysIn;
+
+},{"../internal/isIndex":39,"../internal/isLength":42,"../lang/isArguments":48,"../lang/isArray":49,"../lang/isObject":53}],60:[function(require,module,exports){
+var keys = require('./keys'),
+ toObject = require('../internal/toObject');
+
+/**
+ * Creates a two dimensional array of the key-value pairs for `object`,
+ * e.g. `[[key1, value1], [key2, value2]]`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the new array of key-value pairs.
+ * @example
+ *
+ * _.pairs({ 'barney': 36, 'fred': 40 });
+ * // => [['barney', 36], ['fred', 40]] (iteration order is not guaranteed)
+ */
+function pairs(object) {
+ object = toObject(object);
+
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ var key = props[index];
+ result[index] = [key, object[key]];
+ }
+ return result;
+}
+
+module.exports = pairs;
+
+},{"../internal/toObject":46,"./keys":58}],61:[function(require,module,exports){
+/**
+ * This method returns the first argument provided to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * _.identity(object) === object;
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+module.exports = identity;
+
+},{}],62:[function(require,module,exports){
+var baseProperty = require('../internal/baseProperty'),
+ basePropertyDeep = require('../internal/basePropertyDeep'),
+ isKey = require('../internal/isKey');
+
+/**
+ * Creates a function that returns the property value at `path` on a
+ * given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var objects = [
+ * { 'a': { 'b': { 'c': 2 } } },
+ * { 'a': { 'b': { 'c': 1 } } }
+ * ];
+ *
+ * _.map(objects, _.property('a.b.c'));
+ * // => [2, 1]
+ *
+ * _.pluck(_.sortBy(objects, _.property(['a', 'b', 'c'])), 'a.b.c');
+ * // => [1, 2]
+ */
+function property(path) {
+ return isKey(path) ? baseProperty(path) : basePropertyDeep(path);
+}
+
+module.exports = property;
+
+},{"../internal/baseProperty":24,"../internal/basePropertyDeep":25,"../internal/isKey":41}],63:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLAttribute, create;
+
+ create = require('lodash/object/create');
+
+ module.exports = XMLAttribute = (function() {
+ function XMLAttribute(parent, name, value) {
+ this.stringify = parent.stringify;
+ if (name == null) {
+ throw new Error("Missing attribute name of element " + parent.name);
+ }
+ if (value == null) {
+ throw new Error("Missing attribute value for attribute " + name + " of element " + parent.name);
+ }
+ this.name = this.stringify.attName(name);
+ this.value = this.stringify.attValue(value);
+ }
+
+ XMLAttribute.prototype.clone = function() {
+ return create(XMLAttribute.prototype, this);
+ };
+
+ XMLAttribute.prototype.toString = function(options, level) {
+ return ' ' + this.name + '="' + this.value + '"';
+ };
+
+ return XMLAttribute;
+
+ })();
+
+}).call(this);
+
+},{"lodash/object/create":57}],64:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLBuilder, XMLDeclaration, XMLDocType, XMLElement, XMLStringifier;
+
+ XMLStringifier = require('./XMLStringifier');
+
+ XMLDeclaration = require('./XMLDeclaration');
+
+ XMLDocType = require('./XMLDocType');
+
+ XMLElement = require('./XMLElement');
+
+ module.exports = XMLBuilder = (function() {
+ function XMLBuilder(name, options) {
+ var root, temp;
+ if (name == null) {
+ throw new Error("Root element needs a name");
+ }
+ if (options == null) {
+ options = {};
+ }
+ this.options = options;
+ this.stringify = new XMLStringifier(options);
+ temp = new XMLElement(this, 'doc');
+ root = temp.element(name);
+ root.isRoot = true;
+ root.documentObject = this;
+ this.rootObject = root;
+ if (!options.headless) {
+ root.declaration(options);
+ if ((options.pubID != null) || (options.sysID != null)) {
+ root.doctype(options);
+ }
+ }
+ }
+
+ XMLBuilder.prototype.root = function() {
+ return this.rootObject;
+ };
+
+ XMLBuilder.prototype.end = function(options) {
+ return this.toString(options);
+ };
+
+ XMLBuilder.prototype.toString = function(options) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ r = '';
+ if (this.xmldec != null) {
+ r += this.xmldec.toString(options);
+ }
+ if (this.doctype != null) {
+ r += this.doctype.toString(options);
+ }
+ r += this.rootObject.toString(options);
+ if (pretty && r.slice(-newline.length) === newline) {
+ r = r.slice(0, -newline.length);
+ }
+ return r;
+ };
+
+ return XMLBuilder;
+
+ })();
+
+}).call(this);
+
+},{"./XMLDeclaration":71,"./XMLDocType":72,"./XMLElement":73,"./XMLStringifier":77}],65:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLCData, XMLNode, create,
+ extend = function(child, parent) { for (var key in parent) { if (hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; },
+ hasProp = {}.hasOwnProperty;
+
+ create = require('lodash/object/create');
+
+ XMLNode = require('./XMLNode');
+
+ module.exports = XMLCData = (function(superClass) {
+ extend(XMLCData, superClass);
+
+ function XMLCData(parent, text) {
+ XMLCData.__super__.constructor.call(this, parent);
+ if (text == null) {
+ throw new Error("Missing CDATA text");
+ }
+ this.text = this.stringify.cdata(text);
+ }
+
+ XMLCData.prototype.clone = function() {
+ return create(XMLCData.prototype, this);
+ };
+
+ XMLCData.prototype.toString = function(options, level) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2, space;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ level || (level = 0);
+ space = new Array(level + offset + 1).join(indent);
+ r = '';
+ if (pretty) {
+ r += space;
+ }
+ r += '<![CDATA[' + this.text + ']]>';
+ if (pretty) {
+ r += newline;
+ }
+ return r;
+ };
+
+ return XMLCData;
+
+ })(XMLNode);
+
+}).call(this);
+
+},{"./XMLNode":74,"lodash/object/create":57}],66:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLComment, XMLNode, create,
+ extend = function(child, parent) { for (var key in parent) { if (hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; },
+ hasProp = {}.hasOwnProperty;
+
+ create = require('lodash/object/create');
+
+ XMLNode = require('./XMLNode');
+
+ module.exports = XMLComment = (function(superClass) {
+ extend(XMLComment, superClass);
+
+ function XMLComment(parent, text) {
+ XMLComment.__super__.constructor.call(this, parent);
+ if (text == null) {
+ throw new Error("Missing comment text");
+ }
+ this.text = this.stringify.comment(text);
+ }
+
+ XMLComment.prototype.clone = function() {
+ return create(XMLComment.prototype, this);
+ };
+
+ XMLComment.prototype.toString = function(options, level) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2, space;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ level || (level = 0);
+ space = new Array(level + offset + 1).join(indent);
+ r = '';
+ if (pretty) {
+ r += space;
+ }
+ r += '<!-- ' + this.text + ' -->';
+ if (pretty) {
+ r += newline;
+ }
+ return r;
+ };
+
+ return XMLComment;
+
+ })(XMLNode);
+
+}).call(this);
+
+},{"./XMLNode":74,"lodash/object/create":57}],67:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLDTDAttList, create;
+
+ create = require('lodash/object/create');
+
+ module.exports = XMLDTDAttList = (function() {
+ function XMLDTDAttList(parent, elementName, attributeName, attributeType, defaultValueType, defaultValue) {
+ this.stringify = parent.stringify;
+ if (elementName == null) {
+ throw new Error("Missing DTD element name");
+ }
+ if (attributeName == null) {
+ throw new Error("Missing DTD attribute name");
+ }
+ if (!attributeType) {
+ throw new Error("Missing DTD attribute type");
+ }
+ if (!defaultValueType) {
+ throw new Error("Missing DTD attribute default");
+ }
+ if (defaultValueType.indexOf('#') !== 0) {
+ defaultValueType = '#' + defaultValueType;
+ }
+ if (!defaultValueType.match(/^(#REQUIRED|#IMPLIED|#FIXED|#DEFAULT)$/)) {
+ throw new Error("Invalid default value type; expected: #REQUIRED, #IMPLIED, #FIXED or #DEFAULT");
+ }
+ if (defaultValue && !defaultValueType.match(/^(#FIXED|#DEFAULT)$/)) {
+ throw new Error("Default value only applies to #FIXED or #DEFAULT");
+ }
+ this.elementName = this.stringify.eleName(elementName);
+ this.attributeName = this.stringify.attName(attributeName);
+ this.attributeType = this.stringify.dtdAttType(attributeType);
+ this.defaultValue = this.stringify.dtdAttDefault(defaultValue);
+ this.defaultValueType = defaultValueType;
+ }
+
+ XMLDTDAttList.prototype.toString = function(options, level) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2, space;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ level || (level = 0);
+ space = new Array(level + offset + 1).join(indent);
+ r = '';
+ if (pretty) {
+ r += space;
+ }
+ r += '<!ATTLIST ' + this.elementName + ' ' + this.attributeName + ' ' + this.attributeType;
+ if (this.defaultValueType !== '#DEFAULT') {
+ r += ' ' + this.defaultValueType;
+ }
+ if (this.defaultValue) {
+ r += ' "' + this.defaultValue + '"';
+ }
+ r += '>';
+ if (pretty) {
+ r += newline;
+ }
+ return r;
+ };
+
+ return XMLDTDAttList;
+
+ })();
+
+}).call(this);
+
+},{"lodash/object/create":57}],68:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLDTDElement, create;
+
+ create = require('lodash/object/create');
+
+ module.exports = XMLDTDElement = (function() {
+ function XMLDTDElement(parent, name, value) {
+ this.stringify = parent.stringify;
+ if (name == null) {
+ throw new Error("Missing DTD element name");
+ }
+ if (!value) {
+ value = '(#PCDATA)';
+ }
+ if (Array.isArray(value)) {
+ value = '(' + value.join(',') + ')';
+ }
+ this.name = this.stringify.eleName(name);
+ this.value = this.stringify.dtdElementValue(value);
+ }
+
+ XMLDTDElement.prototype.toString = function(options, level) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2, space;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ level || (level = 0);
+ space = new Array(level + offset + 1).join(indent);
+ r = '';
+ if (pretty) {
+ r += space;
+ }
+ r += '<!ELEMENT ' + this.name + ' ' + this.value + '>';
+ if (pretty) {
+ r += newline;
+ }
+ return r;
+ };
+
+ return XMLDTDElement;
+
+ })();
+
+}).call(this);
+
+},{"lodash/object/create":57}],69:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLDTDEntity, create, isObject;
+
+ create = require('lodash/object/create');
+
+ isObject = require('lodash/lang/isObject');
+
+ module.exports = XMLDTDEntity = (function() {
+ function XMLDTDEntity(parent, pe, name, value) {
+ this.stringify = parent.stringify;
+ if (name == null) {
+ throw new Error("Missing entity name");
+ }
+ if (value == null) {
+ throw new Error("Missing entity value");
+ }
+ this.pe = !!pe;
+ this.name = this.stringify.eleName(name);
+ if (!isObject(value)) {
+ this.value = this.stringify.dtdEntityValue(value);
+ } else {
+ if (!value.pubID && !value.sysID) {
+ throw new Error("Public and/or system identifiers are required for an external entity");
+ }
+ if (value.pubID && !value.sysID) {
+ throw new Error("System identifier is required for a public external entity");
+ }
+ if (value.pubID != null) {
+ this.pubID = this.stringify.dtdPubID(value.pubID);
+ }
+ if (value.sysID != null) {
+ this.sysID = this.stringify.dtdSysID(value.sysID);
+ }
+ if (value.nData != null) {
+ this.nData = this.stringify.dtdNData(value.nData);
+ }
+ if (this.pe && this.nData) {
+ throw new Error("Notation declaration is not allowed in a parameter entity");
+ }
+ }
+ }
+
+ XMLDTDEntity.prototype.toString = function(options, level) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2, space;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ level || (level = 0);
+ space = new Array(level + offset + 1).join(indent);
+ r = '';
+ if (pretty) {
+ r += space;
+ }
+ r += '<!ENTITY';
+ if (this.pe) {
+ r += ' %';
+ }
+ r += ' ' + this.name;
+ if (this.value) {
+ r += ' "' + this.value + '"';
+ } else {
+ if (this.pubID && this.sysID) {
+ r += ' PUBLIC "' + this.pubID + '" "' + this.sysID + '"';
+ } else if (this.sysID) {
+ r += ' SYSTEM "' + this.sysID + '"';
+ }
+ if (this.nData) {
+ r += ' NDATA ' + this.nData;
+ }
+ }
+ r += '>';
+ if (pretty) {
+ r += newline;
+ }
+ return r;
+ };
+
+ return XMLDTDEntity;
+
+ })();
+
+}).call(this);
+
+},{"lodash/lang/isObject":53,"lodash/object/create":57}],70:[function(require,module,exports){
+// Generated by CoffeeScript 1.9.1
+(function() {
+ var XMLDTDNotation, create;
+
+ create = require('lodash/object/create');
+
+ module.exports = XMLDTDNotation = (function() {
+ function XMLDTDNotation(parent, name, value) {
+ this.stringify = parent.stringify;
+ if (name == null) {
+ throw new Error("Missing notation name");
+ }
+ if (!value.pubID && !value.sysID) {
+ throw new Error("Public or system identifiers are required for an external entity");
+ }
+ this.name = this.stringify.eleName(name);
+ if (value.pubID != null) {
+ this.pubID = this.stringify.dtdPubID(value.pubID);
+ }
+ if (value.sysID != null) {
+ this.sysID = this.stringify.dtdSysID(value.sysID);
+ }
+ }
+
+ XMLDTDNotation.prototype.toString = function(options, level) {
+ var indent, newline, offset, pretty, r, ref, ref1, ref2, space;
+ pretty = (options != null ? options.pretty : void 0) || false;
+ indent = (ref = options != null ? options.indent : void 0) != null ? ref : ' ';
+ offset = (ref1 = options != null ? options.offset : void 0) != null ? ref1 : 0;
+ newline = (ref2 = options != null ? options.newline : void 0) != null ? ref2 : '\n';
+ level || (level = 0);
+ space = new Array(level + offset + 1).join(indent);
+ r = '';
+ if (pretty) {
+ r +=
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[20/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-homedir/readme.md
----------------------------------------------------------------------
diff --git a/node_modules/os-homedir/readme.md b/node_modules/os-homedir/readme.md
new file mode 100644
index 0000000..4851f10
--- /dev/null
+++ b/node_modules/os-homedir/readme.md
@@ -0,0 +1,33 @@
+# os-homedir [](https://travis-ci.org/sindresorhus/os-homedir)
+
+> io.js 2.3.0 [`os.homedir()`](https://iojs.org/api/os.html#os_os_homedir) ponyfill
+
+> Ponyfill: A polyfill that doesn't overwrite the native method
+
+
+## Install
+
+```
+$ npm install --save os-homedir
+```
+
+
+## Usage
+
+```js
+var osHomedir = require('os-homedir');
+
+console.log(osHomedir());
+//=> /Users/sindresorhus
+```
+
+
+## Related
+
+- [user-home](https://github.com/sindresorhus/user-home) - Same as this module but caches the result
+- [home-or-tmp](https://github.com/sindresorhus/home-or-tmp) - Get the user home directory with fallback to the system temp directory
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-tmpdir/index.js
----------------------------------------------------------------------
diff --git a/node_modules/os-tmpdir/index.js b/node_modules/os-tmpdir/index.js
new file mode 100644
index 0000000..52d90bf
--- /dev/null
+++ b/node_modules/os-tmpdir/index.js
@@ -0,0 +1,25 @@
+'use strict';
+var isWindows = process.platform === 'win32';
+var trailingSlashRe = isWindows ? /[^:]\\$/ : /.\/$/;
+
+// https://github.com/nodejs/io.js/blob/3e7a14381497a3b73dda68d05b5130563cdab420/lib/os.js#L25-L43
+module.exports = function () {
+ var path;
+
+ if (isWindows) {
+ path = process.env.TEMP ||
+ process.env.TMP ||
+ (process.env.SystemRoot || process.env.windir) + '\\temp';
+ } else {
+ path = process.env.TMPDIR ||
+ process.env.TMP ||
+ process.env.TEMP ||
+ '/tmp';
+ }
+
+ if (trailingSlashRe.test(path)) {
+ path = path.slice(0, -1);
+ }
+
+ return path;
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-tmpdir/license
----------------------------------------------------------------------
diff --git a/node_modules/os-tmpdir/license b/node_modules/os-tmpdir/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/os-tmpdir/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus <si...@gmail.com> (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-tmpdir/package.json
----------------------------------------------------------------------
diff --git a/node_modules/os-tmpdir/package.json b/node_modules/os-tmpdir/package.json
new file mode 100644
index 0000000..097f803
--- /dev/null
+++ b/node_modules/os-tmpdir/package.json
@@ -0,0 +1,96 @@
+{
+ "_args": [
+ [
+ "os-tmpdir@^1.0.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/osenv"
+ ]
+ ],
+ "_from": "os-tmpdir@>=1.0.0 <2.0.0",
+ "_id": "os-tmpdir@1.0.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/os-tmpdir",
+ "_nodeVersion": "0.12.3",
+ "_npmUser": {
+ "email": "sindresorhus@gmail.com",
+ "name": "sindresorhus"
+ },
+ "_npmVersion": "2.9.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "os-tmpdir",
+ "raw": "os-tmpdir@^1.0.0",
+ "rawSpec": "^1.0.0",
+ "scope": null,
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/osenv"
+ ],
+ "_resolved": "http://registry.npmjs.org/os-tmpdir/-/os-tmpdir-1.0.1.tgz",
+ "_shasum": "e9b423a1edaf479882562e92ed71d7743a071b6e",
+ "_shrinkwrap": null,
+ "_spec": "os-tmpdir@^1.0.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/osenv",
+ "author": {
+ "email": "sindresorhus@gmail.com",
+ "name": "Sindre Sorhus",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/os-tmpdir/issues"
+ },
+ "dependencies": {},
+ "description": "Node.js os.tmpdir() ponyfill",
+ "devDependencies": {
+ "ava": "0.0.4"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "e9b423a1edaf479882562e92ed71d7743a071b6e",
+ "tarball": "http://registry.npmjs.org/os-tmpdir/-/os-tmpdir-1.0.1.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "5c5d355f81378980db629d60128ad03e02b1c1e5",
+ "homepage": "https://github.com/sindresorhus/os-tmpdir",
+ "keywords": [
+ "built-in",
+ "core",
+ "dir",
+ "directory",
+ "env",
+ "environment",
+ "os",
+ "polyfill",
+ "ponyfill",
+ "shim",
+ "temp",
+ "tempdir",
+ "tmp",
+ "tmpdir"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "os-tmpdir",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/os-tmpdir.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "1.0.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-tmpdir/readme.md
----------------------------------------------------------------------
diff --git a/node_modules/os-tmpdir/readme.md b/node_modules/os-tmpdir/readme.md
new file mode 100644
index 0000000..54d4c6e
--- /dev/null
+++ b/node_modules/os-tmpdir/readme.md
@@ -0,0 +1,36 @@
+# os-tmpdir [](https://travis-ci.org/sindresorhus/os-tmpdir)
+
+> Node.js [`os.tmpdir()`](https://nodejs.org/api/os.html#os_os_tmpdir) ponyfill
+
+> Ponyfill: A polyfill that doesn't overwrite the native method
+
+Use this instead of `require('os').tmpdir()` to get a consistent behaviour on different Node.js versions (even 0.8).
+
+*This is actually taken from io.js 2.0.2 as it contains some fixes that haven't bubbled up to Node.js yet.*
+
+
+## Install
+
+```
+$ npm install --save os-tmpdir
+```
+
+
+## Usage
+
+```js
+var osTmpdir = require('os-tmpdir');
+
+osTmpdir();
+//=> /var/folders/m3/5574nnhn0yj488ccryqr7tc80000gn/T
+```
+
+
+## API
+
+See the [`os.tmpdir()` docs](https://nodejs.org/api/os.html#os_os_tmpdir).
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/osenv/.npmignore b/node_modules/osenv/.npmignore
new file mode 100644
index 0000000..8c23dee
--- /dev/null
+++ b/node_modules/osenv/.npmignore
@@ -0,0 +1,13 @@
+*.swp
+.*.swp
+
+.DS_Store
+*~
+.project
+.settings
+npm-debug.log
+coverage.html
+.idea
+lib-cov
+
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/osenv/.travis.yml b/node_modules/osenv/.travis.yml
new file mode 100644
index 0000000..99f2bbf
--- /dev/null
+++ b/node_modules/osenv/.travis.yml
@@ -0,0 +1,9 @@
+language: node_js
+language: node_js
+node_js:
+ - '0.8'
+ - '0.10'
+ - '0.12'
+ - 'iojs'
+before_install:
+ - npm install -g npm@latest
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/osenv/LICENSE b/node_modules/osenv/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/osenv/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/README.md
----------------------------------------------------------------------
diff --git a/node_modules/osenv/README.md b/node_modules/osenv/README.md
new file mode 100644
index 0000000..08fd900
--- /dev/null
+++ b/node_modules/osenv/README.md
@@ -0,0 +1,63 @@
+# osenv
+
+Look up environment settings specific to different operating systems.
+
+## Usage
+
+```javascript
+var osenv = require('osenv')
+var path = osenv.path()
+var user = osenv.user()
+// etc.
+
+// Some things are not reliably in the env, and have a fallback command:
+var h = osenv.hostname(function (er, hostname) {
+ h = hostname
+})
+// This will still cause it to be memoized, so calling osenv.hostname()
+// is now an immediate operation.
+
+// You can always send a cb, which will get called in the nextTick
+// if it's been memoized, or wait for the fallback data if it wasn't
+// found in the environment.
+osenv.hostname(function (er, hostname) {
+ if (er) console.error('error looking up hostname')
+ else console.log('this machine calls itself %s', hostname)
+})
+```
+
+## osenv.hostname()
+
+The machine name. Calls `hostname` if not found.
+
+## osenv.user()
+
+The currently logged-in user. Calls `whoami` if not found.
+
+## osenv.prompt()
+
+Either PS1 on unix, or PROMPT on Windows.
+
+## osenv.tmpdir()
+
+The place where temporary files should be created.
+
+## osenv.home()
+
+No place like it.
+
+## osenv.path()
+
+An array of the places that the operating system will search for
+executables.
+
+## osenv.editor()
+
+Return the executable name of the editor program. This uses the EDITOR
+and VISUAL environment variables, and falls back to `vi` on Unix, or
+`notepad.exe` on Windows.
+
+## osenv.shell()
+
+The SHELL on Unix, which Windows calls the ComSpec. Defaults to 'bash'
+or 'cmd'.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/osenv.js
----------------------------------------------------------------------
diff --git a/node_modules/osenv/osenv.js b/node_modules/osenv/osenv.js
new file mode 100644
index 0000000..702a95b
--- /dev/null
+++ b/node_modules/osenv/osenv.js
@@ -0,0 +1,72 @@
+var isWindows = process.platform === 'win32'
+var path = require('path')
+var exec = require('child_process').exec
+var osTmpdir = require('os-tmpdir')
+var osHomedir = require('os-homedir')
+
+// looking up envs is a bit costly.
+// Also, sometimes we want to have a fallback
+// Pass in a callback to wait for the fallback on failures
+// After the first lookup, always returns the same thing.
+function memo (key, lookup, fallback) {
+ var fell = false
+ var falling = false
+ exports[key] = function (cb) {
+ var val = lookup()
+ if (!val && !fell && !falling && fallback) {
+ fell = true
+ falling = true
+ exec(fallback, function (er, output, stderr) {
+ falling = false
+ if (er) return // oh well, we tried
+ val = output.trim()
+ })
+ }
+ exports[key] = function (cb) {
+ if (cb) process.nextTick(cb.bind(null, null, val))
+ return val
+ }
+ if (cb && !falling) process.nextTick(cb.bind(null, null, val))
+ return val
+ }
+}
+
+memo('user', function () {
+ return ( isWindows
+ ? process.env.USERDOMAIN + '\\' + process.env.USERNAME
+ : process.env.USER
+ )
+}, 'whoami')
+
+memo('prompt', function () {
+ return isWindows ? process.env.PROMPT : process.env.PS1
+})
+
+memo('hostname', function () {
+ return isWindows ? process.env.COMPUTERNAME : process.env.HOSTNAME
+}, 'hostname')
+
+memo('tmpdir', function () {
+ return osTmpdir()
+})
+
+memo('home', function () {
+ return osHomedir()
+})
+
+memo('path', function () {
+ return (process.env.PATH ||
+ process.env.Path ||
+ process.env.path).split(isWindows ? ';' : ':')
+})
+
+memo('editor', function () {
+ return process.env.EDITOR ||
+ process.env.VISUAL ||
+ (isWindows ? 'notepad.exe' : 'vi')
+})
+
+memo('shell', function () {
+ return isWindows ? process.env.ComSpec || 'cmd'
+ : process.env.SHELL || 'bash'
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/package.json
----------------------------------------------------------------------
diff --git a/node_modules/osenv/package.json b/node_modules/osenv/package.json
new file mode 100644
index 0000000..8360a97
--- /dev/null
+++ b/node_modules/osenv/package.json
@@ -0,0 +1,101 @@
+{
+ "_args": [
+ [
+ "osenv@^0.1.3",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "osenv@>=0.1.3 <0.2.0",
+ "_id": "osenv@0.1.3",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/osenv",
+ "_nodeVersion": "2.2.1",
+ "_npmUser": {
+ "email": "isaacs@npmjs.com",
+ "name": "isaacs"
+ },
+ "_npmVersion": "3.0.0",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "osenv",
+ "raw": "osenv@^0.1.3",
+ "rawSpec": "^0.1.3",
+ "scope": null,
+ "spec": ">=0.1.3 <0.2.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/osenv/-/osenv-0.1.3.tgz",
+ "_shasum": "83cf05c6d6458fc4d5ac6362ea325d92f2754217",
+ "_shrinkwrap": null,
+ "_spec": "osenv@^0.1.3",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me/"
+ },
+ "bugs": {
+ "url": "https://github.com/npm/osenv/issues"
+ },
+ "dependencies": {
+ "os-homedir": "^1.0.0",
+ "os-tmpdir": "^1.0.0"
+ },
+ "description": "Look up environment settings specific to different operating systems",
+ "devDependencies": {
+ "tap": "^1.2.0"
+ },
+ "directories": {
+ "test": "test"
+ },
+ "dist": {
+ "shasum": "83cf05c6d6458fc4d5ac6362ea325d92f2754217",
+ "tarball": "http://registry.npmjs.org/osenv/-/osenv-0.1.3.tgz"
+ },
+ "gitHead": "f746b3405d8f9e28054d11b97e1436f6a15016c4",
+ "homepage": "https://github.com/npm/osenv#readme",
+ "keywords": [
+ "environment",
+ "home",
+ "path",
+ "prompt",
+ "ps1",
+ "tmpdir",
+ "variable"
+ ],
+ "license": "ISC",
+ "main": "osenv.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ {
+ "name": "robertkowalski",
+ "email": "rok@kowalski.gd"
+ },
+ {
+ "name": "othiym23",
+ "email": "ogd@aoaioxxysz.net"
+ },
+ {
+ "name": "iarna",
+ "email": "me@re-becca.org"
+ }
+ ],
+ "name": "osenv",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/npm/osenv.git"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "version": "0.1.3"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/test/unix.js
----------------------------------------------------------------------
diff --git a/node_modules/osenv/test/unix.js b/node_modules/osenv/test/unix.js
new file mode 100644
index 0000000..f87cbfb
--- /dev/null
+++ b/node_modules/osenv/test/unix.js
@@ -0,0 +1,71 @@
+// only run this test on windows
+// pretending to be another platform is too hacky, since it breaks
+// how the underlying system looks up module paths and runs
+// child processes, and all that stuff is cached.
+if (process.platform === 'win32') {
+ console.log('TAP Version 13\n' +
+ '1..0\n' +
+ '# Skip unix tests, this is not unix\n')
+ return
+}
+var tap = require('tap')
+
+// like unix, but funny
+process.env.USER = 'sirUser'
+process.env.HOME = '/home/sirUser'
+process.env.HOSTNAME = 'my-machine'
+process.env.TMPDIR = '/tmpdir'
+process.env.TMP = '/tmp'
+process.env.TEMP = '/temp'
+process.env.PATH = '/opt/local/bin:/usr/local/bin:/usr/bin/:bin'
+process.env.PS1 = '(o_o) $ '
+process.env.EDITOR = 'edit'
+process.env.VISUAL = 'visualedit'
+process.env.SHELL = 'zsh'
+
+tap.test('basic unix sanity test', function (t) {
+ var osenv = require('../osenv.js')
+
+ t.equal(osenv.user(), process.env.USER)
+ t.equal(osenv.home(), process.env.HOME)
+ t.equal(osenv.hostname(), process.env.HOSTNAME)
+ t.same(osenv.path(), process.env.PATH.split(':'))
+ t.equal(osenv.prompt(), process.env.PS1)
+ t.equal(osenv.tmpdir(), process.env.TMPDIR)
+
+ // mildly evil, but it's for a test.
+ process.env.TMPDIR = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.tmpdir(), process.env.TMP)
+
+ process.env.TMP = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.tmpdir(), process.env.TEMP)
+
+ process.env.TEMP = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ osenv.home = function () { return null }
+ t.equal(osenv.tmpdir(), '/tmp')
+
+ t.equal(osenv.editor(), 'edit')
+ process.env.EDITOR = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.editor(), 'visualedit')
+
+ process.env.VISUAL = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.editor(), 'vi')
+
+ t.equal(osenv.shell(), 'zsh')
+ process.env.SHELL = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.shell(), 'bash')
+
+ t.end()
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/test/windows.js
----------------------------------------------------------------------
diff --git a/node_modules/osenv/test/windows.js b/node_modules/osenv/test/windows.js
new file mode 100644
index 0000000..c9d837a
--- /dev/null
+++ b/node_modules/osenv/test/windows.js
@@ -0,0 +1,74 @@
+// only run this test on windows
+// pretending to be another platform is too hacky, since it breaks
+// how the underlying system looks up module paths and runs
+// child processes, and all that stuff is cached.
+if (process.platform !== 'win32') {
+ console.log('TAP version 13\n' +
+ '1..0 # Skip windows tests, this is not windows\n')
+ return
+}
+
+// load this before clubbing the platform name.
+var tap = require('tap')
+
+process.env.windir = 'c:\\windows'
+process.env.USERDOMAIN = 'some-domain'
+process.env.USERNAME = 'sirUser'
+process.env.USERPROFILE = 'C:\\Users\\sirUser'
+process.env.COMPUTERNAME = 'my-machine'
+process.env.TMPDIR = 'C:\\tmpdir'
+process.env.TMP = 'C:\\tmp'
+process.env.TEMP = 'C:\\temp'
+process.env.Path = 'C:\\Program Files\\;C:\\Binary Stuff\\bin'
+process.env.PROMPT = '(o_o) $ '
+process.env.EDITOR = 'edit'
+process.env.VISUAL = 'visualedit'
+process.env.ComSpec = 'some-com'
+
+tap.test('basic windows sanity test', function (t) {
+ var osenv = require('../osenv.js')
+
+ t.equal(osenv.user(),
+ process.env.USERDOMAIN + '\\' + process.env.USERNAME)
+ t.equal(osenv.home(), process.env.USERPROFILE)
+ t.equal(osenv.hostname(), process.env.COMPUTERNAME)
+ t.same(osenv.path(), process.env.Path.split(';'))
+ t.equal(osenv.prompt(), process.env.PROMPT)
+ t.equal(osenv.tmpdir(), process.env.TMPDIR)
+
+ // mildly evil, but it's for a test.
+ process.env.TMPDIR = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.tmpdir(), process.env.TMP)
+
+ process.env.TMP = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.tmpdir(), process.env.TEMP)
+
+ process.env.TEMP = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ osenv.home = function () { return null }
+ t.equal(osenv.tmpdir(), 'c:\\windows\\temp')
+
+ t.equal(osenv.editor(), 'edit')
+ process.env.EDITOR = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.editor(), 'visualedit')
+
+ process.env.VISUAL = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.editor(), 'notepad.exe')
+
+ t.equal(osenv.shell(), 'some-com')
+ process.env.ComSpec = ''
+ delete require.cache[require.resolve('../osenv.js')]
+ var osenv = require('../osenv.js')
+ t.equal(osenv.shell(), 'cmd')
+
+ t.end()
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/osenv/x.tap
----------------------------------------------------------------------
diff --git a/node_modules/osenv/x.tap b/node_modules/osenv/x.tap
new file mode 100644
index 0000000..90d8472
--- /dev/null
+++ b/node_modules/osenv/x.tap
@@ -0,0 +1,39 @@
+TAP version 13
+ # Subtest: test/unix.js
+ TAP version 13
+ # Subtest: basic unix sanity test
+ ok 1 - should be equal
+ ok 2 - should be equal
+ ok 3 - should be equal
+ ok 4 - should be equivalent
+ ok 5 - should be equal
+ ok 6 - should be equal
+ ok 7 - should be equal
+ ok 8 - should be equal
+ ok 9 - should be equal
+ ok 10 - should be equal
+ ok 11 - should be equal
+ ok 12 - should be equal
+ ok 13 - should be equal
+ ok 14 - should be equal
+ 1..14
+ ok 1 - basic unix sanity test # time=10.712ms
+
+ 1..1
+ # time=18.422ms
+ok 1 - test/unix.js # time=169.827ms
+
+ # Subtest: test/windows.js
+ TAP version 13
+ 1..0 # Skip windows tests, this is not windows
+
+ok 2 - test/windows.js # SKIP Skip windows tests, this is not windows
+
+ # Subtest: test/nada.js
+ TAP version 13
+ 1..0
+
+ok 2 - test/nada.js
+
+1..3
+# time=274.247ms
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/path-is-absolute/index.js
----------------------------------------------------------------------
diff --git a/node_modules/path-is-absolute/index.js b/node_modules/path-is-absolute/index.js
new file mode 100644
index 0000000..19f103f
--- /dev/null
+++ b/node_modules/path-is-absolute/index.js
@@ -0,0 +1,20 @@
+'use strict';
+
+function posix(path) {
+ return path.charAt(0) === '/';
+};
+
+function win32(path) {
+ // https://github.com/joyent/node/blob/b3fcc245fb25539909ef1d5eaa01dbf92e168633/lib/path.js#L56
+ var splitDeviceRe = /^([a-zA-Z]:|[\\\/]{2}[^\\\/]+[\\\/]+[^\\\/]+)?([\\\/])?([\s\S]*?)$/;
+ var result = splitDeviceRe.exec(path);
+ var device = result[1] || '';
+ var isUnc = !!device && device.charAt(1) !== ':';
+
+ // UNC paths are always absolute
+ return !!result[2] || isUnc;
+};
+
+module.exports = process.platform === 'win32' ? win32 : posix;
+module.exports.posix = posix;
+module.exports.win32 = win32;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/path-is-absolute/license
----------------------------------------------------------------------
diff --git a/node_modules/path-is-absolute/license b/node_modules/path-is-absolute/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/path-is-absolute/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus <si...@gmail.com> (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/path-is-absolute/package.json
----------------------------------------------------------------------
diff --git a/node_modules/path-is-absolute/package.json b/node_modules/path-is-absolute/package.json
new file mode 100644
index 0000000..def9f9f
--- /dev/null
+++ b/node_modules/path-is-absolute/package.json
@@ -0,0 +1,97 @@
+{
+ "_args": [
+ [
+ "path-is-absolute@^1.0.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob"
+ ]
+ ],
+ "_from": "path-is-absolute@>=1.0.0 <2.0.0",
+ "_id": "path-is-absolute@1.0.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/path-is-absolute",
+ "_nodeVersion": "0.12.0",
+ "_npmUser": {
+ "email": "sindresorhus@gmail.com",
+ "name": "sindresorhus"
+ },
+ "_npmVersion": "2.5.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "path-is-absolute",
+ "raw": "path-is-absolute@^1.0.0",
+ "rawSpec": "^1.0.0",
+ "scope": null,
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/glob"
+ ],
+ "_resolved": "http://registry.npmjs.org/path-is-absolute/-/path-is-absolute-1.0.0.tgz",
+ "_shasum": "263dada66ab3f2fb10bf7f9d24dd8f3e570ef912",
+ "_shrinkwrap": null,
+ "_spec": "path-is-absolute@^1.0.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob",
+ "author": {
+ "email": "sindresorhus@gmail.com",
+ "name": "Sindre Sorhus",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/path-is-absolute/issues"
+ },
+ "dependencies": {},
+ "description": "Node.js 0.12 path.isAbsolute() ponyfill",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "263dada66ab3f2fb10bf7f9d24dd8f3e570ef912",
+ "tarball": "http://registry.npmjs.org/path-is-absolute/-/path-is-absolute-1.0.0.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "7a76a0c9f2263192beedbe0a820e4d0baee5b7a1",
+ "homepage": "https://github.com/sindresorhus/path-is-absolute",
+ "keywords": [
+ "absolute",
+ "built-in",
+ "check",
+ "core",
+ "detect",
+ "dir",
+ "file",
+ "is",
+ "is-absolute",
+ "isabsolute",
+ "path",
+ "paths",
+ "polyfill",
+ "ponyfill",
+ "shim",
+ "util",
+ "utils"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "path-is-absolute",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/path-is-absolute.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "1.0.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/path-is-absolute/readme.md
----------------------------------------------------------------------
diff --git a/node_modules/path-is-absolute/readme.md b/node_modules/path-is-absolute/readme.md
new file mode 100644
index 0000000..cdf94f4
--- /dev/null
+++ b/node_modules/path-is-absolute/readme.md
@@ -0,0 +1,51 @@
+# path-is-absolute [](https://travis-ci.org/sindresorhus/path-is-absolute)
+
+> Node.js 0.12 [`path.isAbsolute()`](http://nodejs.org/api/path.html#path_path_isabsolute_path) ponyfill
+
+> Ponyfill: A polyfill that doesn't overwrite the native method
+
+
+## Install
+
+```
+$ npm install --save path-is-absolute
+```
+
+
+## Usage
+
+```js
+var pathIsAbsolute = require('path-is-absolute');
+
+// Linux
+pathIsAbsolute('/home/foo');
+//=> true
+
+// Windows
+pathIsAbsolute('C:/Users/');
+//=> true
+
+// Any OS
+pathIsAbsolute.posix('/home/foo');
+//=> true
+```
+
+
+## API
+
+See the [`path.isAbsolute()` docs](http://nodejs.org/api/path.html#path_path_isabsolute_path).
+
+### pathIsAbsolute(path)
+
+### pathIsAbsolute.posix(path)
+
+The Posix specific version.
+
+### pathIsAbsolute.win32(path)
+
+The Windows specific version.
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/CHANGELOG
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/CHANGELOG b/node_modules/pegjs/CHANGELOG
new file mode 100644
index 0000000..e5967cf
--- /dev/null
+++ b/node_modules/pegjs/CHANGELOG
@@ -0,0 +1,146 @@
+0.6.2 (2011-08-20)
+------------------
+
+Small Changes:
+
+* Reset parser position when action returns |null|.
+* Fixed typo in JavaScript example grammar.
+
+0.6.1 (2011-04-14)
+------------------
+
+Small Changes:
+
+* Use --ascii option when generating a minified version.
+
+0.6.0 (2011-04-14)
+------------------
+
+Big Changes:
+
+* Rewrote the command-line mode to be based on Node.js instead of Rhino -- no
+ more Java dependency. This also means that PEG.js is available as a Node.js
+ package and can be required as a module.
+* Version for the browser is built separately from the command-ine one in two
+ flavors (normal and minified).
+* Parser variable name is no longer required argument of bin/pegjs -- it is
+ "module.exports" by default and can be set using the -e/--export-var option.
+ This makes parsers generated by /bin/pegjs Node.js modules by default.
+* Added ability to start parsing from any grammar rule.
+* Added several compiler optimizations -- 0.6 is ~12% faster than 0.5.1 in the
+ benchmark on V8.
+
+Small Changes:
+
+* Split the source code into multiple files combined together using a build
+ system.
+* Jake is now used instead of Rake for build scripts -- no more Ruby dependency.
+* Test suite can be run from the command-line.
+* Benchmark suite can be run from the command-line.
+* Benchmark browser runner improvements (users can specify number of runs,
+ benchmarks are run using |setTimeout|, table is centered and fixed-width).
+* Added PEG.js version to "Generated by..." line in generated parsers.
+* Added PEG.js version information and homepage header to peg.js.
+* Generated code improvements and fixes.
+* Internal code improvements and fixes.
+* Rewrote README.md.
+
+0.5.1 (2010-11-28)
+------------------
+
+Small Changes:
+
+* Fixed a problem where "SyntaxError: Invalid range in character class." error
+ appeared when using command-line version on Widnows (GH-13).
+* Fixed wrong version reported by "bin/pegjs --version".
+* Removed two unused variables in the code.
+* Fixed incorrect variable name on two places.
+
+0.5 (2010-06-10)
+----------------
+
+Big Changes:
+
+* Syntax change: Use labeled expressions and variables instead of $1, $2, etc.
+* Syntax change: Replaced ":" after a rule name with "=".
+* Syntax change: Allow trailing semicolon (";") for rules
+* Semantic change: Start rule of the grammar is now implicitly its first rule.
+* Implemented semantic predicates.
+* Implemented initializers.
+* Removed ability to change the start rule when generating the parser.
+* Added several compiler optimizations -- 0.5 is ~11% faster than 0.4 in the
+ benchmark on V8.
+
+Small Changes:
+
+* PEG.buildParser now accepts grammars only in string format.
+* Added "Generated by ..." message to the generated parsers.
+* Formatted all grammars more consistently and transparently.
+* Added notes about ECMA-262, 5th ed. compatibility to the JSON example grammar.
+* Guarded against redefinition of |undefined|.
+* Made bin/pegjs work when called via a symlink (issue #1).
+* Fixed bug causing incorrect error messages (issue #2).
+* Fixed error message for invalid character range.
+* Fixed string literal parsing in the JavaScript grammar.
+* Generated code improvements and fixes.
+* Internal code improvements and fixes.
+* Improved README.md.
+
+0.4 (2010-04-17)
+----------------
+
+Big Changes:
+
+* Improved IE compatibility -- IE6+ is now fully supported.
+* Generated parsers are now standalone (no runtime is required).
+* Added example grammars for JavaScript, CSS and JSON.
+* Added a benchmark suite.
+* Implemented negative character classes (e.g. [^a-z]).
+* Project moved from BitBucket to GitHub.
+
+Small Changes:
+
+* Code generated for the character classes is now regexp-based (= simpler and
+ more scalable).
+* Added \uFEFF (BOM) to the definition of whitespace in the metagrammar.
+* When building a parser, left-recursive rules (both direct and indirect) are
+ reported as errors.
+* When building a parser, missing rules are reported as errors.
+* Expected items in the error messages do not contain duplicates and they are
+ sorted.
+* Fixed several bugs in the example arithmetics grammar.
+* Converted README to GitHub Flavored Markdown and improved it.
+* Added CHANGELOG.
+* Internal code improvements.
+
+0.3 (2010-03-14)
+----------------
+
+* Wrote README.
+* Bootstrapped the grammar parser.
+* Metagrammar recognizes JavaScript-like comments.
+* Changed standard grammar extension from .peg to .pegjs (it is more specific).
+* Simplified the example arithmetics grammar + added comment.
+* Fixed a bug with reporting of invalid ranges such as [b-a] in the metagrammar.
+* Fixed --start vs. --start-rule inconsistency between help and actual option
+ processing code.
+* Avoided ugliness in QUnit output.
+* Fixed typo in help: "parserVar" -> "parser_var".
+* Internal code improvements.
+
+0.2.1 (2010-03-08)
+------------------
+
+* Added "pegjs-" prefix to the name of the minified runtime file.
+
+0.2 (2010-03-08)
+----------------
+
+* Added Rakefile that builds minified runtime using Google Closure Compiler API.
+* Removed trailing commas in object initializers (Google Closure does not like
+ them).
+
+0.1 (2010-03-08)
+----------------
+
+* Initial release.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/LICENSE b/node_modules/pegjs/LICENSE
new file mode 100644
index 0000000..48ab3e5
--- /dev/null
+++ b/node_modules/pegjs/LICENSE
@@ -0,0 +1,22 @@
+Copyright (c) 2010-2011 David Majda
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/README.md
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/README.md b/node_modules/pegjs/README.md
new file mode 100644
index 0000000..7a4c17e
--- /dev/null
+++ b/node_modules/pegjs/README.md
@@ -0,0 +1,226 @@
+PEG.js
+======
+
+PEG.js is a simple parser generator for JavaScript that produces fast parsers with excellent error reporting. You can use it to process complex data or computer languages and build transformers, interpreters, compilers and other tools easily.
+
+Features
+--------
+
+ * Simple and expressive grammar syntax
+ * Integrates both lexical and syntactical analysis
+ * Parsers have excellent error reporting out of the box
+ * Based on [parsing expression grammar](http://en.wikipedia.org/wiki/Parsing_expression_grammar) formalism — more powerful than traditional LL(*k*) and LR(*k*) parsers
+ * Usable [from your browser](http://pegjs.majda.cz/online), from the command line, or via JavaScript API
+
+Getting Started
+---------------
+
+[Online version](http://pegjs.majda.cz/online) is the easiest way to generate a parser. Just enter your grammar, try parsing few inputs, and download generated parser code.
+
+Installation
+------------
+
+### Command Line / Server-side
+
+To use command-line version, install [Node.js](http://nodejs.org/) and [npm](http://npmjs.org/) first. You can then install PEG.js:
+
+ $ npm install pegjs
+
+Once installed, you can use the `pegjs` command to generate your parser from a grammar and use the JavaScript API from Node.js.
+
+### Browser
+
+[Download](http://pegjs.majda.cz/#download) the PEG.js library (regular or minified version) and include it in your web page or application using the `<script>` tag.
+
+Generating a Parser
+-------------------
+
+PEG.js generates parser from a grammar that describes expected input and can specify what the parser returns (using semantic actions on matched parts of the input). Generated parser itself is a JavaScript object with a simple API.
+
+### Command Line
+
+To generate a parser from your grammar, use the `pegjs` command:
+
+ $ pegjs arithmetics.pegjs
+
+This writes parser source code into a file with the same name as the grammar file but with “.js” extension. You can also specify the output file explicitly:
+
+ $ pegjs arithmetics.pegjs arithmetics-parser.js
+
+If you omit both input and ouptut file, standard input and output are used.
+
+By default, the parser object is assigned to `module.exports`, which makes the output a Node.js module. You can assign it to another variable by passing a variable name using the `-e`/`--export-var` option. This may be helpful if you want to use the parser in browser environment.
+
+### JavaScript API
+
+In Node.js, require the PEG.js parser generator module:
+
+ var PEG = require("pegjs");
+
+In browser, include the PEG.js library in your web page or application using the `<script>` tag. The API will be available through the `PEG` global object.
+
+To generate a parser, call the `PEG.buildParser` method and pass your grammar as a parameter:
+
+ var parser = PEG.buildParser("start = ('a' / 'b')+");
+
+The method will return generated parser object or throw an exception if the grammar is invalid. The exception will contain `message` property with more details about the error.
+
+To get parser’s source code, call the `toSource` method on the parser.
+
+Using the Parser
+----------------
+
+Using the generated parser is simple — just call its `parse` method and pass an input string as a parameter. The method will return a parse result (the exact value depends on the grammar used to build the parser) or throw an exception if the input is invalid. The exception will contain `line`, `column` and `message` properties with more details about the error.
+
+ parser.parse("abba"); // returns ["a", "b", "b", "a"]
+
+ parser.parse("abcd"); // throws an exception
+
+You can also start parsing from a specific rule in the grammar. Just pass the rule name to the `parse` method as a second parameter.
+
+Grammar Syntax and Semantics
+----------------------------
+
+The grammar syntax is similar to JavaScript in that it is not line-oriented and ignores whitespace between tokens. You can also use JavaScript-style comments (`// ...` and `/* ... */`).
+
+Let's look at example grammar that recognizes simple arithmetic expressions like `2*(3+4)`. A parser generated from this grammar computes their values.
+
+ start
+ = additive
+
+ additive
+ = left:multiplicative "+" right:additive { return left + right; }
+ / multiplicative
+
+ multiplicative
+ = left:primary "*" right:multiplicative { return left * right; }
+ / primary
+
+ primary
+ = integer
+ / "(" additive:additive ")" { return additive; }
+
+ integer "integer"
+ = digits:[0-9]+ { return parseInt(digits.join(""), 10); }
+
+On the top level, the grammar consists of *rules* (in our example, there are five of them). Each rule has a *name* (e.g. `integer`) that identifies the rule, and a *parsing expression* (e.g. `digits:[0-9]+ { return parseInt(digits.join(""), 10); }`) that defines a pattern to match against the input text and possibly contains some JavaScript code that determines what happens when the pattern matches successfully. A rule can also contain *human-readable name* that is used in error messages (in our example, only the `integer` rule has a human-readable name). The parsing starts at the first rule, which is also called the *start rule*.
+
+A rule name must be a JavaScript identifier. It is followed by an equality sign (“=”) and a parsing expression. If the rule has a human-readable name, it is written as a JavaScript string between the name and separating equality sign. Rules need to be separated only by whitespace (their beginning is easily recognizable), but a semicolon (“;”) after the parsing expression is allowed.
+
+Rules can be preceded by an *initializer* — a piece of JavaScript code in curly braces (“{” and “}”). This code is executed before the generated parser starts parsing. All variables and functions defined in the initializer are accessible in rule actions and semantic predicates. Curly braces in the initializer code must be balanced.
+
+The parsing expressions of the rules are used to match the input text to the grammar. There are various types of expressions — matching characters or character classes, indicating optional parts and repetition, etc. Expressions can also contain references to other rules. See detailed description below.
+
+If an expression successfully matches a part of the text when running the generated parser, it produces a *match result*, which is a JavaScript value. For example:
+
+ * An expression matching a literal string produces a JavaScript string containing matched part of the input.
+ * An expression matching repeated occurrence of some subexpression produces a JavaScript array with all the matches.
+
+The match results propagate through the rules when the rule names are used in expressions, up to the start rule. The generated parser returns start rule's match result when parsing is successful.
+
+One special case of parser expression is a *parser action* — a piece of JavaScript code inside curly braces (“{” and “}”) that takes match results of some of the the preceding expressions and returns a JavaScript value. This value is considered match result of the preceding expression (in other words, the parser action is a match result transformer).
+
+In our arithmetics example, there are many parser actions. Consider the action in expression `digits:[0-9]+ { return parseInt(digits.join(""), 10); }`. It takes the match result of the expression [0-9]+, which is an array of strings containing digits, as its parameter. It joins the digits together to form a number and converts it to a JavaScript `number` object.
+
+### Parsing Expression Types
+
+There are several types of parsing expressions, some of them containing subexpressions and thus forming a recursive structure:
+
+#### "*literal*"<br>'*literal*'
+
+Match exact literal string and return it. The string syntax is the same as in JavaScript.
+
+#### .
+
+Match exactly one character and return it as a string.
+
+#### [*characters*]
+
+Match one character from a set and return it as a string. The characters in the list can be escaped in exactly the same way as in JavaScript string. The list of characters can also contain ranges (e.g. `[a-z]` means “all lowercase letters”). Preceding the characters with `^` inverts the matched set (e.g. `[^a-z]` means “all character but lowercase letters”).
+
+#### *rule*
+
+Match a parsing expression of a rule recursively and return its match result.
+
+#### ( *expression* )
+
+Match a subexpression and return its match result.
+
+#### *expression* \*
+
+Match zero or more repetitions of the expression and return their match results in an array. The matching is greedy, i.e. the parser tries to match the expression as many times as possible.
+
+#### *expression* +
+
+Match one or more repetitions of the expression and return their match results in an array. The matching is greedy, i.e. the parser tries to match the expression as many times as possible.
+
+#### *expression* ?
+
+Try to match the expression. If the match succeeds, return its match result, otherwise return an empty string.
+
+#### & *expression*
+
+Try to match the expression. If the match succeeds, just return an empty string and do not advance the parser position, otherwise consider the match failed.
+
+#### ! *expression*
+
+Try to match the expression and. If the match does not succeed, just return an empty string and do not advance the parser position, otherwise consider the match failed.
+
+#### & { *predicate* }
+
+The predicate is a piece of JavaScript code that is executed as if it was inside a function. It should return some JavaScript value using the `return` statement. If the returned value evaluates to `true` in boolean context, just return an empty string and do not advance the parser position; otherwise consider the match failed.
+
+The code inside the predicate has access to all variables and functions defined in the initializer at the beginning of the grammar. Curly braces in the predicate code must be balanced.
+
+#### ! { *predicate* }
+
+The predicate is a piece of JavaScript code that is executed as if it was inside a function. It should return some JavaScript value using the `return` statement. If the returned value evaluates to `false` in boolean context, just return an empty string and do not advance the parser position; otherwise consider the match failed.
+
+The code inside the predicate has access to all variables and functions defined in the initializer at the beginning of the grammar. Curly braces in the predicate code must be balanced.
+
+#### *label* : *expression*
+
+Match the expression and remember its match result under given lablel. The label must be a JavaScript identifier.
+
+Labeled expressions are useful together with actions, where saved match results can be accessed by action's JavaScript code.
+
+#### *expression<sub>1</sub>* *expression<sub>2</sub>* ... *expression<sub>n</sub>*
+
+Match a sequence of expressions and return their match results in an array.
+
+#### *expression* { *action* }
+
+Match the expression. If the match is successful, run the action, otherwise consider the match failed.
+
+The action is a piece of JavaScript code that is executed as if it was inside a function. It gets the match results of labeled expressions in preceding expression as its arguments. The action should return some JavaScript value using the `return` statement. This value is considered match result of the preceding expression. The action can return `null` to indicate a match failure.
+
+The code inside the action has access to all variables and functions defined in the initializer at the beginning of the grammar. Curly braces in the action code must be balanced.
+
+#### *expression<sub>1</sub>* / *expression<sub>2</sub>* / ... / *expression<sub>n</sub>*
+
+Try to match the first expression, if it does not succeed, try the second one, etc. Return the match result of the first successfully matched expression. If no expression matches, consider the match failed.
+
+Compatibility
+-------------
+
+Both the parser generator and generated parsers should run well in the following environments:
+
+ * Node.js 0.4.4+
+ * IE 6+
+ * Firefox
+ * Chrome
+ * Safari
+ * Opera
+
+Development
+-----------
+
+ * [Project website](https://pegjs.majda.cz/)
+ * [Source code](https://github.com/dmajda/pegjs)
+ * [Issue tracker](https://github.com/dmajda/pegjs/issues)
+ * [Google Group](http://groups.google.com/group/pegjs)
+ * [Twitter](http://twitter.com/peg_js)
+
+PEG.js is developed by [David Majda](http://majda.cz/) ([@dmajda](http://twitter.com/dmajda)). You are welcome to contribute code. Unless your contribution is really trivial you should get in touch with me first — this can prevent wasted effort on both sides. You can send code both as patch or GitHub pull request.
+
+Note that PEG.js is still very much work in progress. There are no compatibility guarantees until version 1.0.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/VERSION
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/VERSION b/node_modules/pegjs/VERSION
new file mode 100644
index 0000000..b616048
--- /dev/null
+++ b/node_modules/pegjs/VERSION
@@ -0,0 +1 @@
+0.6.2
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/bin/pegjs
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/bin/pegjs b/node_modules/pegjs/bin/pegjs
new file mode 100755
index 0000000..c24246e
--- /dev/null
+++ b/node_modules/pegjs/bin/pegjs
@@ -0,0 +1,142 @@
+#!/usr/bin/env node
+
+var sys = require("sys");
+var fs = require("fs");
+var PEG = require("../lib/peg");
+
+/* Helpers */
+
+function printVersion() {
+ sys.puts("PEG.js " + PEG.VERSION);
+}
+
+function printHelp() {
+ sys.puts("Usage: pegjs [options] [--] [<input_file>] [<output_file>]");
+ sys.puts("");
+ sys.puts("Generates a parser from the PEG grammar specified in the <input_file> and");
+ sys.puts("writes it to the <output_file>.");
+ sys.puts("");
+ sys.puts("If the <output_file> is omitted, its name is generated by changing the");
+ sys.puts("<input_file> extension to \".js\". If both <input_file> and <output_file> are");
+ sys.puts("omitted, standard input and output are used.");
+ sys.puts("");
+ sys.puts("Options:");
+ sys.puts(" -e, --export-var <variable> name of the variable where the parser object");
+ sys.puts(" will be stored (default: \"module.exports\")");
+ sys.puts(" -v, --version print version information and exit");
+ sys.puts(" -h, --help print help and exit");
+}
+
+function exitSuccess() {
+ process.exit(0);
+}
+
+function exitFailure() {
+ process.exit(1);
+}
+
+function abort(message) {
+ sys.error(message);
+ exitFailure();
+}
+
+/* Arguments */
+
+var args = process.argv.slice(2); // Trim "node" and the script path.
+
+function isOption(arg) {
+ return /-.+/.test(arg);
+}
+
+function nextArg() {
+ args.shift();
+}
+
+/* Files */
+
+function readStream(inputStream, callback) {
+ var input = "";
+ inputStream.on("data", function(data) { input += data; });
+ inputStream.on("end", function() { callback(input); });
+}
+
+/* Main */
+
+/* This makes the generated parser a CommonJS module by default. */
+var exportVar = "module.exports";
+
+while (args.length > 0 && isOption(args[0])) {
+ switch (args[0]) {
+ case "-e":
+ case "--export-var":
+ nextArg();
+ if (args.length === 0) {
+ abort("Missing parameter of the -e/--export-var option.");
+ }
+ exportVar = args[0];
+ break;
+
+ case "-v":
+ case "--version":
+ printVersion();
+ exitSuccess();
+ break;
+
+ case "-h":
+ case "--help":
+ printHelp();
+ exitSuccess();
+ break;
+
+ case "--":
+ nextArg();
+ break;
+
+ default:
+ abort("Unknown option: " + args[0] + ".");
+ }
+ nextArg();
+}
+
+switch (args.length) {
+ case 0:
+ var inputStream = process.openStdin();
+ var outputStream = process.stdout;
+ break;
+
+ case 1:
+ case 2:
+ var inputFile = args[0];
+ var inputStream = fs.createReadStream(inputFile);
+ inputStream.on("error", function() {
+ abort("Can't read from file \"" + inputFile + "\".");
+ });
+
+ var outputFile = args.length == 1
+ ? args[0].replace(/\.[^.]*$/, ".js")
+ : args[1];
+ var outputStream = fs.createWriteStream(outputFile);
+ outputStream.on("error", function() {
+ abort("Can't write to file \"" + outputFile + "\".");
+ });
+
+ break;
+
+ default:
+ abort("Too many arguments.");
+}
+
+readStream(inputStream, function(input) {
+ try {
+ var parser = PEG.buildParser(input);
+ } catch (e) {
+ if (e.line !== undefined && e.column !== undefined) {
+ abort(e.line + ":" + e.column + ": " + e.message);
+ } else {
+ abort(e.message);
+ }
+ }
+
+ outputStream.write(exportVar + " = " + parser.toSource() + ";\n");
+ outputStream.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/examples/arithmetics.pegjs
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/examples/arithmetics.pegjs b/node_modules/pegjs/examples/arithmetics.pegjs
new file mode 100644
index 0000000..52fae2a
--- /dev/null
+++ b/node_modules/pegjs/examples/arithmetics.pegjs
@@ -0,0 +1,22 @@
+/*
+ * Classic example grammar, which recognizes simple arithmetic expressions like
+ * "2*(3+4)". The parser generated from this grammar then computes their value.
+ */
+
+start
+ = additive
+
+additive
+ = left:multiplicative "+" right:additive { return left + right; }
+ / multiplicative
+
+multiplicative
+ = left:primary "*" right:multiplicative { return left * right; }
+ / primary
+
+primary
+ = integer
+ / "(" additive:additive ")" { return additive; }
+
+integer "integer"
+ = digits:[0-9]+ { return parseInt(digits.join(""), 10); }
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/examples/css.pegjs
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/examples/css.pegjs b/node_modules/pegjs/examples/css.pegjs
new file mode 100644
index 0000000..5593d3c
--- /dev/null
+++ b/node_modules/pegjs/examples/css.pegjs
@@ -0,0 +1,554 @@
+/*
+ * CSS parser based on the grammar described at http://www.w3.org/TR/CSS2/grammar.html.
+ *
+ * The parser builds a tree representing the parsed CSS, composed of basic
+ * JavaScript values, arrays and objects (basically JSON). It can be easily
+ * used by various CSS processors, transformers, etc.
+ *
+ * Note that the parser does not handle errors in CSS according to the
+ * specification -- many errors which it should recover from (e.g. malformed
+ * declarations or unexpected end of stylesheet) are simply fatal. This is a
+ * result of straightforward rewrite of the CSS grammar to PEG.js and it should
+ * be fixed sometimes.
+ */
+
+/* ===== Syntactical Elements ===== */
+
+start
+ = stylesheet:stylesheet comment* { return stylesheet; }
+
+stylesheet
+ = charset:(CHARSET_SYM STRING ";")? (S / CDO / CDC)*
+ imports:(import (CDO S* / CDC S*)*)*
+ rules:((ruleset / media / page) (CDO S* / CDC S*)*)* {
+ var importsConverted = [];
+ for (var i = 0; i < imports.length; i++) {
+ importsConverted.push(imports[i][0]);
+ }
+
+ var rulesConverted = [];
+ for (i = 0; i < rules.length; i++) {
+ rulesConverted.push(rules[i][0]);
+ }
+
+ return {
+ type: "stylesheet",
+ charset: charset !== "" ? charset[1] : null,
+ imports: importsConverted,
+ rules: rulesConverted
+ };
+ }
+
+import
+ = IMPORT_SYM S* href:(STRING / URI) S* media:media_list? ";" S* {
+ return {
+ type: "import_rule",
+ href: href,
+ media: media !== "" ? media : []
+ };
+ }
+
+media
+ = MEDIA_SYM S* media:media_list "{" S* rules:ruleset* "}" S* {
+ return {
+ type: "media_rule",
+ media: media,
+ rules: rules
+ };
+ }
+
+media_list
+ = head:medium tail:("," S* medium)* {
+ var result = [head];
+ for (var i = 0; i < tail.length; i++) {
+ result.push(tail[i][2]);
+ }
+ return result;
+ }
+
+medium
+ = ident:IDENT S* { return ident; }
+
+page
+ = PAGE_SYM S* qualifier:pseudo_page?
+ "{" S*
+ declarationsHead:declaration?
+ declarationsTail:(";" S* declaration?)*
+ "}" S* {
+ var declarations = declarationsHead !== "" ? [declarationsHead] : [];
+ for (var i = 0; i < declarationsTail.length; i++) {
+ if (declarationsTail[i][2] !== "") {
+ declarations.push(declarationsTail[i][2]);
+ }
+ }
+
+ return {
+ type: "page_rule",
+ qualifier: qualifier !== "" ? qualifier : null,
+ declarations: declarations
+ };
+ }
+
+pseudo_page
+ = ":" ident:IDENT S* { return ident; }
+
+operator
+ = "/" S* { return "/"; }
+ / "," S* { return ","; }
+
+combinator
+ = "+" S* { return "+"; }
+ / ">" S* { return ">"; }
+
+unary_operator
+ = "+"
+ / "-"
+
+property
+ = ident:IDENT S* { return ident; }
+
+ruleset
+ = selectorsHead:selector
+ selectorsTail:("," S* selector)*
+ "{" S*
+ declarationsHead:declaration?
+ declarationsTail:(";" S* declaration?)*
+ "}" S* {
+ var selectors = [selectorsHead];
+ for (var i = 0; i < selectorsTail.length; i++) {
+ selectors.push(selectorsTail[i][2]);
+ }
+
+ var declarations = declarationsHead !== "" ? [declarationsHead] : [];
+ for (i = 0; i < declarationsTail.length; i++) {
+ if (declarationsTail[i][2] !== "") {
+ declarations.push(declarationsTail[i][2]);
+ }
+ }
+
+ return {
+ type: "ruleset",
+ selectors: selectors,
+ declarations: declarations
+ };
+ }
+
+selector
+ = left:simple_selector S* combinator:combinator right:selector {
+ return {
+ type: "selector",
+ combinator: combinator,
+ left: left,
+ right: right
+ };
+ }
+ / left:simple_selector S* right:selector {
+ return {
+ type: "selector",
+ combinator: " ",
+ left: left,
+ right: right
+ };
+ }
+ / selector:simple_selector S* { return selector; }
+
+simple_selector
+ = element:element_name
+ qualifiers:(
+ id:HASH { return { type: "ID selector", id: id.substr(1) }; }
+ / class
+ / attrib
+ / pseudo
+ )* {
+ return {
+ type: "simple_selector",
+ element: element,
+ qualifiers: qualifiers
+ };
+ }
+ / qualifiers:(
+ id:HASH { return { type: "ID selector", id: id.substr(1) }; }
+ / class
+ / attrib
+ / pseudo
+ )+ {
+ return {
+ type: "simple_selector",
+ element: "*",
+ qualifiers: qualifiers
+ };
+ }
+
+class
+ = "." class_:IDENT { return { type: "class_selector", "class": class_ }; }
+
+element_name
+ = IDENT / '*'
+
+attrib
+ = "[" S*
+ attribute:IDENT S*
+ operatorAndValue:(
+ ('=' / INCLUDES / DASHMATCH) S*
+ (IDENT / STRING) S*
+ )?
+ "]" {
+ return {
+ type: "attribute_selector",
+ attribute: attribute,
+ operator: operatorAndValue !== "" ? operatorAndValue[0] : null,
+ value: operatorAndValue !== "" ? operatorAndValue[2] : null
+ };
+ }
+
+pseudo
+ = ":"
+ value:(
+ name:FUNCTION S* params:(IDENT S*)? ")" {
+ return {
+ type: "function",
+ name: name,
+ params: params !== "" ? [params[0]] : []
+ };
+ }
+ / IDENT
+ ) {
+ /*
+ * The returned object has somewhat vague property names and values because
+ * the rule matches both pseudo-classes and pseudo-elements (they look the
+ * same at the syntactic level).
+ */
+ return {
+ type: "pseudo_selector",
+ value: value
+ };
+ }
+
+declaration
+ = property:property ":" S* expression:expr important:prio? {
+ return {
+ type: "declaration",
+ property: property,
+ expression: expression,
+ important: important !== "" ? true : false
+ };
+ }
+
+prio
+ = IMPORTANT_SYM S*
+
+expr
+ = head:term tail:(operator? term)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "expression",
+ operator: tail[i][0],
+ left: result,
+ right: tail[i][1]
+ };
+ }
+ return result;
+ }
+
+term
+ = operator:unary_operator?
+ value:(
+ EMS S*
+ / EXS S*
+ / LENGTH S*
+ / ANGLE S*
+ / TIME S*
+ / FREQ S*
+ / PERCENTAGE S*
+ / NUMBER S*
+ ) { return { type: "value", value: operator + value[0] }; }
+ / value:URI S* { return { type: "uri", value: value }; }
+ / function
+ / hexcolor
+ / value:STRING S* { return { type: "string", value: value }; }
+ / value:IDENT S* { return { type: "ident", value: value }; }
+
+function
+ = name:FUNCTION S* params:expr ")" S* {
+ return {
+ type: "function",
+ name: name,
+ params: params
+ };
+ }
+
+hexcolor
+ = value:HASH S* { return { type: "hexcolor", value: value}; }
+
+/* ===== Lexical Elements ===== */
+
+/* Macros */
+
+h
+ = [0-9a-fA-F]
+
+nonascii
+ = [\x80-\xFF]
+
+unicode
+ = "\\" h1:h h2:h? h3:h? h4:h? h5:h? h6:h? ("\r\n" / [ \t\r\n\f])? {
+ return String.fromCharCode(parseInt("0x" + h1 + h2 + h3 + h4 + h5 + h6));
+ }
+
+escape
+ = unicode
+ / "\\" char_:[^\r\n\f0-9a-fA-F] { return char_; }
+
+nmstart
+ = [_a-zA-Z]
+ / nonascii
+ / escape
+
+nmchar
+ = [_a-zA-Z0-9-]
+ / nonascii
+ / escape
+
+integer
+ = digits:[0-9]+ { return parseInt(digits.join("")); }
+
+float
+ = before:[0-9]* "." after:[0-9]+ {
+ return parseFloat(before.join("") + "." + after.join(""));
+ }
+
+string1
+ = '"' chars:([^\n\r\f\\"] / "\\" nl:nl { return nl } / escape)* '"' {
+ return chars.join("");
+ }
+
+string2
+ = "'" chars:([^\n\r\f\\'] / "\\" nl:nl { return nl } / escape)* "'" {
+ return chars.join("");
+ }
+
+comment
+ = "/*" [^*]* "*"+ ([^/*] [^*]* "*"+)* "/"
+
+ident
+ = dash:"-"? nmstart:nmstart nmchars:nmchar* {
+ return dash + nmstart + nmchars.join("");
+ }
+
+name
+ = nmchars:nmchar+ { return nmchars.join(""); }
+
+num
+ = float
+ / integer
+
+string
+ = string1
+ / string2
+
+url
+ = chars:([!#$%&*-~] / nonascii / escape)* { return chars.join(""); }
+
+s
+ = [ \t\r\n\f]+
+
+w
+ = s?
+
+nl
+ = "\n"
+ / "\r\n"
+ / "\r"
+ / "\f"
+
+A
+ = [aA]
+ / "\\" "0"? "0"? "0"? "0"? "41" ("\r\n" / [ \t\r\n\f])? { return "A"; }
+ / "\\" "0"? "0"? "0"? "0"? "61" ("\r\n" / [ \t\r\n\f])? { return "a"; }
+
+C
+ = [cC]
+ / "\\" "0"? "0"? "0"? "0"? "43" ("\r\n" / [ \t\r\n\f])? { return "C"; }
+ / "\\" "0"? "0"? "0"? "0"? "63" ("\r\n" / [ \t\r\n\f])? { return "c"; }
+
+D
+ = [dD]
+ / "\\" "0"? "0"? "0"? "0"? "44" ("\r\n" / [ \t\r\n\f])? { return "D"; }
+ / "\\" "0"? "0"? "0"? "0"? "64" ("\r\n" / [ \t\r\n\f])? { return "d"; }
+
+E
+ = [eE]
+ / "\\" "0"? "0"? "0"? "0"? "45" ("\r\n" / [ \t\r\n\f])? { return "E"; }
+ / "\\" "0"? "0"? "0"? "0"? "65" ("\r\n" / [ \t\r\n\f])? { return "e"; }
+
+G
+ = [gG]
+ / "\\" "0"? "0"? "0"? "0"? "47" ("\r\n" / [ \t\r\n\f])? { return "G"; }
+ / "\\" "0"? "0"? "0"? "0"? "67" ("\r\n" / [ \t\r\n\f])? { return "g"; }
+ / "\\" char_:[gG] { return char_; }
+
+H
+ = h:[hH]
+ / "\\" "0"? "0"? "0"? "0"? "48" ("\r\n" / [ \t\r\n\f])? { return "H"; }
+ / "\\" "0"? "0"? "0"? "0"? "68" ("\r\n" / [ \t\r\n\f])? { return "h"; }
+ / "\\" char_:[hH] { return char_; }
+
+I
+ = i:[iI]
+ / "\\" "0"? "0"? "0"? "0"? "49" ("\r\n" / [ \t\r\n\f])? { return "I"; }
+ / "\\" "0"? "0"? "0"? "0"? "69" ("\r\n" / [ \t\r\n\f])? { return "i"; }
+ / "\\" char_:[iI] { return char_; }
+
+K
+ = [kK]
+ / "\\" "0"? "0"? "0"? "0"? "4" [bB] ("\r\n" / [ \t\r\n\f])? { return "K"; }
+ / "\\" "0"? "0"? "0"? "0"? "6" [bB] ("\r\n" / [ \t\r\n\f])? { return "k"; }
+ / "\\" char_:[kK] { return char_; }
+
+L
+ = [lL]
+ / "\\" "0"? "0"? "0"? "0"? "4" [cC] ("\r\n" / [ \t\r\n\f])? { return "L"; }
+ / "\\" "0"? "0"? "0"? "0"? "6" [cC] ("\r\n" / [ \t\r\n\f])? { return "l"; }
+ / "\\" char_:[lL] { return char_; }
+
+M
+ = [mM]
+ / "\\" "0"? "0"? "0"? "0"? "4" [dD] ("\r\n" / [ \t\r\n\f])? { return "M"; }
+ / "\\" "0"? "0"? "0"? "0"? "6" [dD] ("\r\n" / [ \t\r\n\f])? { return "m"; }
+ / "\\" char_:[mM] { return char_; }
+
+N
+ = [nN]
+ / "\\" "0"? "0"? "0"? "0"? "4" [eE] ("\r\n" / [ \t\r\n\f])? { return "N"; }
+ / "\\" "0"? "0"? "0"? "0"? "6" [eE] ("\r\n" / [ \t\r\n\f])? { return "n"; }
+ / "\\" char_:[nN] { return char_; }
+
+O
+ = [oO]
+ / "\\" "0"? "0"? "0"? "0"? "4" [fF] ("\r\n" / [ \t\r\n\f])? { return "O"; }
+ / "\\" "0"? "0"? "0"? "0"? "6" [fF] ("\r\n" / [ \t\r\n\f])? { return "o"; }
+ / "\\" char_:[oO] { return char_; }
+
+P
+ = [pP]
+ / "\\" "0"? "0"? "0"? "0"? "50" ("\r\n" / [ \t\r\n\f])? { return "P"; }
+ / "\\" "0"? "0"? "0"? "0"? "70" ("\r\n" / [ \t\r\n\f])? { return "p"; }
+ / "\\" char_:[pP] { return char_; }
+
+R
+ = [rR]
+ / "\\" "0"? "0"? "0"? "0"? "52" ("\r\n" / [ \t\r\n\f])? { return "R"; }
+ / "\\" "0"? "0"? "0"? "0"? "72" ("\r\n" / [ \t\r\n\f])? { return "r"; }
+ / "\\" char_:[rR] { return char_; }
+
+S_
+ = [sS]
+ / "\\" "0"? "0"? "0"? "0"? "53" ("\r\n" / [ \t\r\n\f])? { return "S"; }
+ / "\\" "0"? "0"? "0"? "0"? "73" ("\r\n" / [ \t\r\n\f])? { return "s"; }
+ / "\\" char_:[sS] { return char_; }
+
+T
+ = [tT]
+ / "\\" "0"? "0"? "0"? "0"? "54" ("\r\n" / [ \t\r\n\f])? { return "T"; }
+ / "\\" "0"? "0"? "0"? "0"? "74" ("\r\n" / [ \t\r\n\f])? { return "t"; }
+ / "\\" char_:[tT] { return char_; }
+
+U
+ = [uU]
+ / "\\" "0"? "0"? "0"? "0"? "55" ("\r\n" / [ \t\r\n\f])? { return "U"; }
+ / "\\" "0"? "0"? "0"? "0"? "75" ("\r\n" / [ \t\r\n\f])? { return "u"; }
+ / "\\" char_:[uU] { return char_; }
+
+X
+ = [xX]
+ / "\\" "0"? "0"? "0"? "0"? "58" ("\r\n" / [ \t\r\n\f])? { return "X"; }
+ / "\\" "0"? "0"? "0"? "0"? "78" ("\r\n" / [ \t\r\n\f])? { return "x"; }
+ / "\\" char_:[xX] { return char_; }
+
+Z
+ = [zZ]
+ / "\\" "0"? "0"? "0"? "0"? "5" [aA] ("\r\n" / [ \t\r\n\f])? { return "Z"; }
+ / "\\" "0"? "0"? "0"? "0"? "7" [aA] ("\r\n" / [ \t\r\n\f])? { return "z"; }
+ / "\\" char_:[zZ] { return char_; }
+
+/* Tokens */
+
+S "whitespace"
+ = comment* s
+
+CDO "<!--"
+ = comment* "<!--"
+
+CDC "-->"
+ = comment* "-->"
+
+INCLUDES "~="
+ = comment* "~="
+
+DASHMATCH "|="
+ = comment* "|="
+
+STRING "string"
+ = comment* string:string { return string; }
+
+IDENT "identifier"
+ = comment* ident:ident { return ident; }
+
+HASH "hash"
+ = comment* "#" name:name { return "#" + name; }
+
+IMPORT_SYM "@import"
+ = comment* "@" I M P O R T
+
+PAGE_SYM "@page"
+ = comment* "@" P A G E
+
+MEDIA_SYM "@media"
+ = comment* "@" M E D I A
+
+CHARSET_SYM "@charset"
+ = comment* "@charset "
+
+/* Note: We replace "w" with "s" here to avoid infinite recursion. */
+IMPORTANT_SYM "!important"
+ = comment* "!" (s / comment)* I M P O R T A N T { return "!important"; }
+
+EMS "length"
+ = comment* num:num e:E m:M { return num + e + m; }
+
+EXS "length"
+ = comment* num:num e:E x:X { return num + e + x; }
+
+LENGTH "length"
+ = comment* num:num unit:(P X / C M / M M / I N / P T / P C) {
+ return num + unit.join("");
+ }
+
+ANGLE "angle"
+ = comment* num:num unit:(D E G / R A D / G R A D) {
+ return num + unit.join("");
+ }
+
+TIME "time"
+ = comment* num:num unit:(m:M s:S_ { return m + s; } / S_) {
+ return num + unit;
+ }
+
+FREQ "frequency"
+ = comment* num:num unit:(H Z / K H Z) { return num + unit.join(""); }
+
+DIMENSION "dimension"
+ = comment* num:num unit:ident { return num + unit; }
+
+PERCENTAGE "percentage"
+ = comment* num:num "%" { return num + "%"; }
+
+NUMBER "number"
+ = comment* num:num { return num; }
+
+URI "uri"
+ = comment* U R L "(" w value:(string / url) w ")" { return value; }
+
+FUNCTION "function"
+ = comment* name:ident "(" { return name; }
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[40/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseFlatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseFlatten.js b/node_modules/lodash-node/compat/internals/baseFlatten.js
new file mode 100644
index 0000000..aaad714
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseFlatten.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray');
+
+/**
+ * The base implementation of `_.flatten` without support for callback
+ * shorthands or `thisArg` binding.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isShallow=false] A flag to restrict flattening to a single level.
+ * @param {boolean} [isStrict=false] A flag to restrict flattening to arrays and `arguments` objects.
+ * @param {number} [fromIndex=0] The index to start from.
+ * @returns {Array} Returns a new flattened array.
+ */
+function baseFlatten(array, isShallow, isStrict, fromIndex) {
+ var index = (fromIndex || 0) - 1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+
+ if (value && typeof value == 'object' && typeof value.length == 'number'
+ && (isArray(value) || isArguments(value))) {
+ // recursively flatten arrays (susceptible to call stack limits)
+ if (!isShallow) {
+ value = baseFlatten(value, isShallow, isStrict);
+ }
+ var valIndex = -1,
+ valLength = value.length,
+ resIndex = result.length;
+
+ result.length += valLength;
+ while (++valIndex < valLength) {
+ result[resIndex++] = value[valIndex];
+ }
+ } else if (!isStrict) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseFlatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseIndexOf.js b/node_modules/lodash-node/compat/internals/baseIndexOf.js
new file mode 100644
index 0000000..bb12119
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseIndexOf.js
@@ -0,0 +1,32 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * The base implementation of `_.indexOf` without support for binary searches
+ * or `fromIndex` constraints.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ */
+function baseIndexOf(array, value, fromIndex) {
+ var index = (fromIndex || 0) - 1,
+ length = array ? array.length : 0;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseIsEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseIsEqual.js b/node_modules/lodash-node/compat/internals/baseIsEqual.js
new file mode 100644
index 0000000..79e26a9
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseIsEqual.js
@@ -0,0 +1,212 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('../objects/forIn'),
+ getArray = require('./getArray'),
+ isArguments = require('../objects/isArguments'),
+ isFunction = require('../objects/isFunction'),
+ isNode = require('./isNode'),
+ objectTypes = require('./objectTypes'),
+ releaseArray = require('./releaseArray'),
+ support = require('../support');
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * The base implementation of `_.isEqual`, without support for `thisArg` binding,
+ * that allows partial "_.where" style comparisons.
+ *
+ * @private
+ * @param {*} a The value to compare.
+ * @param {*} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {Function} [isWhere=false] A flag to indicate performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `a` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `b` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(a, b, callback, isWhere, stackA, stackB) {
+ // used to indicate that when comparing objects, `a` has at least the properties of `b`
+ if (callback) {
+ var result = callback(a, b);
+ if (typeof result != 'undefined') {
+ return !!result;
+ }
+ }
+ // exit early for identical values
+ if (a === b) {
+ // treat `+0` vs. `-0` as not equal
+ return a !== 0 || (1 / a == 1 / b);
+ }
+ var type = typeof a,
+ otherType = typeof b;
+
+ // exit early for unlike primitive values
+ if (a === a &&
+ !(a && objectTypes[type]) &&
+ !(b && objectTypes[otherType])) {
+ return false;
+ }
+ // exit early for `null` and `undefined` avoiding ES3's Function#call behavior
+ // http://es5.github.io/#x15.3.4.4
+ if (a == null || b == null) {
+ return a === b;
+ }
+ // compare [[Class]] names
+ var className = toString.call(a),
+ otherClass = toString.call(b);
+
+ if (className == argsClass) {
+ className = objectClass;
+ }
+ if (otherClass == argsClass) {
+ otherClass = objectClass;
+ }
+ if (className != otherClass) {
+ return false;
+ }
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ // coerce dates and booleans to numbers, dates to milliseconds and booleans
+ // to `1` or `0` treating invalid dates coerced to `NaN` as not equal
+ return +a == +b;
+
+ case numberClass:
+ // treat `NaN` vs. `NaN` as equal
+ return (a != +a)
+ ? b != +b
+ // but treat `+0` vs. `-0` as not equal
+ : (a == 0 ? (1 / a == 1 / b) : a == +b);
+
+ case regexpClass:
+ case stringClass:
+ // coerce regexes to strings (http://es5.github.io/#x15.10.6.4)
+ // treat string primitives and their corresponding object instances as equal
+ return a == String(b);
+ }
+ var isArr = className == arrayClass;
+ if (!isArr) {
+ // unwrap any `lodash` wrapped values
+ var aWrapped = hasOwnProperty.call(a, '__wrapped__'),
+ bWrapped = hasOwnProperty.call(b, '__wrapped__');
+
+ if (aWrapped || bWrapped) {
+ return baseIsEqual(aWrapped ? a.__wrapped__ : a, bWrapped ? b.__wrapped__ : b, callback, isWhere, stackA, stackB);
+ }
+ // exit for functions and DOM nodes
+ if (className != objectClass || (!support.nodeClass && (isNode(a) || isNode(b)))) {
+ return false;
+ }
+ // in older versions of Opera, `arguments` objects have `Array` constructors
+ var ctorA = !support.argsObject && isArguments(a) ? Object : a.constructor,
+ ctorB = !support.argsObject && isArguments(b) ? Object : b.constructor;
+
+ // non `Object` object instances with different constructors are not equal
+ if (ctorA != ctorB &&
+ !(isFunction(ctorA) && ctorA instanceof ctorA && isFunction(ctorB) && ctorB instanceof ctorB) &&
+ ('constructor' in a && 'constructor' in b)
+ ) {
+ return false;
+ }
+ }
+ // assume cyclic structures are equal
+ // the algorithm for detecting cyclic structures is adapted from ES 5.1
+ // section 15.12.3, abstract operation `JO` (http://es5.github.io/#x15.12.3)
+ var initedStack = !stackA;
+ stackA || (stackA = getArray());
+ stackB || (stackB = getArray());
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == a) {
+ return stackB[length] == b;
+ }
+ }
+ var size = 0;
+ result = true;
+
+ // add `a` and `b` to the stack of traversed objects
+ stackA.push(a);
+ stackB.push(b);
+
+ // recursively compare objects and arrays (susceptible to call stack limits)
+ if (isArr) {
+ // compare lengths to determine if a deep comparison is necessary
+ length = a.length;
+ size = b.length;
+ result = size == length;
+
+ if (result || isWhere) {
+ // deep compare the contents, ignoring non-numeric properties
+ while (size--) {
+ var index = length,
+ value = b[size];
+
+ if (isWhere) {
+ while (index--) {
+ if ((result = baseIsEqual(a[index], value, callback, isWhere, stackA, stackB))) {
+ break;
+ }
+ }
+ } else if (!(result = baseIsEqual(a[size], value, callback, isWhere, stackA, stackB))) {
+ break;
+ }
+ }
+ }
+ }
+ else {
+ // deep compare objects using `forIn`, instead of `forOwn`, to avoid `Object.keys`
+ // which, in this case, is more costly
+ forIn(b, function(value, key, b) {
+ if (hasOwnProperty.call(b, key)) {
+ // count the number of properties.
+ size++;
+ // deep compare each property value.
+ return (result = hasOwnProperty.call(a, key) && baseIsEqual(a[key], value, callback, isWhere, stackA, stackB));
+ }
+ });
+
+ if (result && !isWhere) {
+ // ensure both objects have the same number of properties
+ forIn(a, function(value, key, a) {
+ if (hasOwnProperty.call(a, key)) {
+ // `size` will be `-1` if `a` has more properties than `b`
+ return (result = --size > -1);
+ }
+ });
+ }
+ }
+ stackA.pop();
+ stackB.pop();
+
+ if (initedStack) {
+ releaseArray(stackA);
+ releaseArray(stackB);
+ }
+ return result;
+}
+
+module.exports = baseIsEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseMerge.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseMerge.js b/node_modules/lodash-node/compat/internals/baseMerge.js
new file mode 100644
index 0000000..f0dd885
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseMerge.js
@@ -0,0 +1,79 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray'),
+ isPlainObject = require('../objects/isPlainObject');
+
+/**
+ * The base implementation of `_.merge` without argument juggling or support
+ * for `thisArg` binding.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} [callback] The function to customize merging properties.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates values with source counterparts.
+ */
+function baseMerge(object, source, callback, stackA, stackB) {
+ (isArray(source) ? forEach : forOwn)(source, function(source, key) {
+ var found,
+ isArr,
+ result = source,
+ value = object[key];
+
+ if (source && ((isArr = isArray(source)) || isPlainObject(source))) {
+ // avoid merging previously merged cyclic sources
+ var stackLength = stackA.length;
+ while (stackLength--) {
+ if ((found = stackA[stackLength] == source)) {
+ value = stackB[stackLength];
+ break;
+ }
+ }
+ if (!found) {
+ var isShallow;
+ if (callback) {
+ result = callback(value, source);
+ if ((isShallow = typeof result != 'undefined')) {
+ value = result;
+ }
+ }
+ if (!isShallow) {
+ value = isArr
+ ? (isArray(value) ? value : [])
+ : (isPlainObject(value) ? value : {});
+ }
+ // add `source` and associated `value` to the stack of traversed objects
+ stackA.push(source);
+ stackB.push(value);
+
+ // recursively merge objects and arrays (susceptible to call stack limits)
+ if (!isShallow) {
+ baseMerge(value, source, callback, stackA, stackB);
+ }
+ }
+ }
+ else {
+ if (callback) {
+ result = callback(value, source);
+ if (typeof result == 'undefined') {
+ result = source;
+ }
+ }
+ if (typeof result != 'undefined') {
+ value = result;
+ }
+ }
+ object[key] = value;
+ });
+}
+
+module.exports = baseMerge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseRandom.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseRandom.js b/node_modules/lodash-node/compat/internals/baseRandom.js
new file mode 100644
index 0000000..a9f12b9
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseRandom.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Native method shortcuts */
+var floor = Math.floor;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeRandom = Math.random;
+
+/**
+ * The base implementation of `_.random` without argument juggling or support
+ * for returning floating-point numbers.
+ *
+ * @private
+ * @param {number} min The minimum possible value.
+ * @param {number} max The maximum possible value.
+ * @returns {number} Returns a random number.
+ */
+function baseRandom(min, max) {
+ return min + floor(nativeRandom() * (max - min + 1));
+}
+
+module.exports = baseRandom;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseUniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseUniq.js b/node_modules/lodash-node/compat/internals/baseUniq.js
new file mode 100644
index 0000000..1d96d63
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseUniq.js
@@ -0,0 +1,64 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf'),
+ cacheIndexOf = require('./cacheIndexOf'),
+ createCache = require('./createCache'),
+ getArray = require('./getArray'),
+ largeArraySize = require('./largeArraySize'),
+ releaseArray = require('./releaseArray'),
+ releaseObject = require('./releaseObject');
+
+/**
+ * The base implementation of `_.uniq` without support for callback shorthands
+ * or `thisArg` binding.
+ *
+ * @private
+ * @param {Array} array The array to process.
+ * @param {boolean} [isSorted=false] A flag to indicate that `array` is sorted.
+ * @param {Function} [callback] The function called per iteration.
+ * @returns {Array} Returns a duplicate-value-free array.
+ */
+function baseUniq(array, isSorted, callback) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ result = [];
+
+ var isLarge = !isSorted && length >= largeArraySize,
+ seen = (callback || isLarge) ? getArray() : result;
+
+ if (isLarge) {
+ var cache = createCache(seen);
+ indexOf = cacheIndexOf;
+ seen = cache;
+ }
+ while (++index < length) {
+ var value = array[index],
+ computed = callback ? callback(value, index, array) : value;
+
+ if (isSorted
+ ? !index || seen[seen.length - 1] !== computed
+ : indexOf(seen, computed) < 0
+ ) {
+ if (callback || isLarge) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ if (isLarge) {
+ releaseArray(seen.array);
+ releaseObject(seen);
+ } else if (callback) {
+ releaseArray(seen);
+ }
+ return result;
+}
+
+module.exports = baseUniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/cacheIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/cacheIndexOf.js b/node_modules/lodash-node/compat/internals/cacheIndexOf.js
new file mode 100644
index 0000000..7d540fd
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/cacheIndexOf.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf'),
+ keyPrefix = require('./keyPrefix');
+
+/**
+ * An implementation of `_.contains` for cache objects that mimics the return
+ * signature of `_.indexOf` by returning `0` if the value is found, else `-1`.
+ *
+ * @private
+ * @param {Object} cache The cache object to inspect.
+ * @param {*} value The value to search for.
+ * @returns {number} Returns `0` if `value` is found, else `-1`.
+ */
+function cacheIndexOf(cache, value) {
+ var type = typeof value;
+ cache = cache.cache;
+
+ if (type == 'boolean' || value == null) {
+ return cache[value] ? 0 : -1;
+ }
+ if (type != 'number' && type != 'string') {
+ type = 'object';
+ }
+ var key = type == 'number' ? value : keyPrefix + value;
+ cache = (cache = cache[type]) && cache[key];
+
+ return type == 'object'
+ ? (cache && baseIndexOf(cache, value) > -1 ? 0 : -1)
+ : (cache ? 0 : -1);
+}
+
+module.exports = cacheIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/cachePush.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/cachePush.js b/node_modules/lodash-node/compat/internals/cachePush.js
new file mode 100644
index 0000000..b16163a
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/cachePush.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keyPrefix = require('./keyPrefix');
+
+/**
+ * Adds a given value to the corresponding cache object.
+ *
+ * @private
+ * @param {*} value The value to add to the cache.
+ */
+function cachePush(value) {
+ var cache = this.cache,
+ type = typeof value;
+
+ if (type == 'boolean' || value == null) {
+ cache[value] = true;
+ } else {
+ if (type != 'number' && type != 'string') {
+ type = 'object';
+ }
+ var key = type == 'number' ? value : keyPrefix + value,
+ typeCache = cache[type] || (cache[type] = {});
+
+ if (type == 'object') {
+ (typeCache[key] || (typeCache[key] = [])).push(value);
+ } else {
+ typeCache[key] = true;
+ }
+ }
+}
+
+module.exports = cachePush;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/charAtCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/charAtCallback.js b/node_modules/lodash-node/compat/internals/charAtCallback.js
new file mode 100644
index 0000000..f754fe9
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/charAtCallback.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used by `_.max` and `_.min` as the default callback when a given
+ * collection is a string value.
+ *
+ * @private
+ * @param {string} value The character to inspect.
+ * @returns {number} Returns the code unit of given character.
+ */
+function charAtCallback(value) {
+ return value.charCodeAt(0);
+}
+
+module.exports = charAtCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/compareAscending.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/compareAscending.js b/node_modules/lodash-node/compat/internals/compareAscending.js
new file mode 100644
index 0000000..7387123
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/compareAscending.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used by `sortBy` to compare transformed `collection` elements, stable sorting
+ * them in ascending order.
+ *
+ * @private
+ * @param {Object} a The object to compare to `b`.
+ * @param {Object} b The object to compare to `a`.
+ * @returns {number} Returns the sort order indicator of `1` or `-1`.
+ */
+function compareAscending(a, b) {
+ var ac = a.criteria,
+ bc = b.criteria,
+ index = -1,
+ length = ac.length;
+
+ while (++index < length) {
+ var value = ac[index],
+ other = bc[index];
+
+ if (value !== other) {
+ if (value > other || typeof value == 'undefined') {
+ return 1;
+ }
+ if (value < other || typeof other == 'undefined') {
+ return -1;
+ }
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to return the same value for
+ // `a` and `b`. See https://github.com/jashkenas/underscore/pull/1247
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See http://code.google.com/p/v8/issues/detail?id=90
+ return a.index - b.index;
+}
+
+module.exports = compareAscending;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/createAggregator.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/createAggregator.js b/node_modules/lodash-node/compat/internals/createAggregator.js
new file mode 100644
index 0000000..cef4290
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/createAggregator.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('./baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates a function that aggregates a collection, creating an object composed
+ * of keys generated from the results of running each element of the collection
+ * through a callback. The given `setter` function sets the keys and values
+ * of the composed object.
+ *
+ * @private
+ * @param {Function} setter The setter function.
+ * @returns {Function} Returns the new aggregator function.
+ */
+function createAggregator(setter) {
+ return function(collection, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg, 3);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ setter(result, value, callback(value, index, collection), collection);
+ }
+ } else {
+ baseEach(collection, function(value, key, collection) {
+ setter(result, value, callback(value, key, collection), collection);
+ });
+ }
+ return result;
+ };
+}
+
+module.exports = createAggregator;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/createCache.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/createCache.js b/node_modules/lodash-node/compat/internals/createCache.js
new file mode 100644
index 0000000..fb94745
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/createCache.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var cachePush = require('./cachePush'),
+ getObject = require('./getObject'),
+ releaseObject = require('./releaseObject');
+
+/**
+ * Creates a cache object to optimize linear searches of large arrays.
+ *
+ * @private
+ * @param {Array} [array=[]] The array to search.
+ * @returns {null|Object} Returns the cache object or `null` if caching should not be used.
+ */
+function createCache(array) {
+ var index = -1,
+ length = array.length,
+ first = array[0],
+ mid = array[(length / 2) | 0],
+ last = array[length - 1];
+
+ if (first && typeof first == 'object' &&
+ mid && typeof mid == 'object' && last && typeof last == 'object') {
+ return false;
+ }
+ var cache = getObject();
+ cache['false'] = cache['null'] = cache['true'] = cache['undefined'] = false;
+
+ var result = getObject();
+ result.array = array;
+ result.cache = cache;
+ result.push = cachePush;
+
+ while (++index < length) {
+ result.push(array[index]);
+ }
+ return result;
+}
+
+module.exports = createCache;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/createIterator.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/createIterator.js b/node_modules/lodash-node/compat/internals/createIterator.js
new file mode 100644
index 0000000..2dab121
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/createIterator.js
@@ -0,0 +1,127 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('./baseCreateCallback'),
+ indicatorObject = require('./indicatorObject'),
+ isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString'),
+ iteratorTemplate = require('./iteratorTemplate'),
+ objectTypes = require('./objectTypes');
+
+/** Used to fix the JScript [[DontEnum]] bug */
+var shadowedProps = [
+ 'constructor', 'hasOwnProperty', 'isPrototypeOf', 'propertyIsEnumerable',
+ 'toLocaleString', 'toString', 'valueOf'
+];
+
+/** `Object#toString` result shortcuts */
+var arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ errorClass = '[object Error]',
+ funcClass = '[object Function]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+/** Used as the data object for `iteratorTemplate` */
+var iteratorData = {
+ 'args': '',
+ 'array': null,
+ 'bottom': '',
+ 'firstArg': '',
+ 'init': '',
+ 'keys': null,
+ 'loop': '',
+ 'shadowedProps': null,
+ 'support': null,
+ 'top': '',
+ 'useHas': false
+};
+
+/** Used for native method references */
+var errorProto = Error.prototype,
+ objectProto = Object.prototype,
+ stringProto = String.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to avoid iterating non-enumerable properties in IE < 9 */
+var nonEnumProps = {};
+nonEnumProps[arrayClass] = nonEnumProps[dateClass] = nonEnumProps[numberClass] = { 'constructor': true, 'toLocaleString': true, 'toString': true, 'valueOf': true };
+nonEnumProps[boolClass] = nonEnumProps[stringClass] = { 'constructor': true, 'toString': true, 'valueOf': true };
+nonEnumProps[errorClass] = nonEnumProps[funcClass] = nonEnumProps[regexpClass] = { 'constructor': true, 'toString': true };
+nonEnumProps[objectClass] = { 'constructor': true };
+
+(function() {
+ var length = shadowedProps.length;
+ while (length--) {
+ var key = shadowedProps[length];
+ for (var className in nonEnumProps) {
+ if (hasOwnProperty.call(nonEnumProps, className) && !hasOwnProperty.call(nonEnumProps[className], key)) {
+ nonEnumProps[className][key] = false;
+ }
+ }
+ }
+}());
+
+/**
+ * Creates compiled iteration functions.
+ *
+ * @private
+ * @param {...Object} [options] The compile options object(s).
+ * @param {string} [options.array] Code to determine if the iterable is an array or array-like.
+ * @param {boolean} [options.useHas] Specify using `hasOwnProperty` checks in the object loop.
+ * @param {Function} [options.keys] A reference to `_.keys` for use in own property iteration.
+ * @param {string} [options.args] A comma separated string of iteration function arguments.
+ * @param {string} [options.top] Code to execute before the iteration branches.
+ * @param {string} [options.loop] Code to execute in the object loop.
+ * @param {string} [options.bottom] Code to execute after the iteration branches.
+ * @returns {Function} Returns the compiled function.
+ */
+function createIterator() {
+ // data properties
+ iteratorData.shadowedProps = shadowedProps;
+
+ // iterator options
+ iteratorData.array = iteratorData.bottom = iteratorData.loop = iteratorData.top = '';
+ iteratorData.init = 'iterable';
+ iteratorData.useHas = true;
+
+ // merge options into a template data object
+ for (var object, index = 0; object = arguments[index]; index++) {
+ for (var key in object) {
+ iteratorData[key] = object[key];
+ }
+ }
+ var args = iteratorData.args;
+ iteratorData.firstArg = /^[^,]+/.exec(args)[0];
+
+ // create the function factory
+ var factory = Function(
+ 'baseCreateCallback, errorClass, errorProto, hasOwnProperty, ' +
+ 'indicatorObject, isArguments, isArray, isString, keys, objectProto, ' +
+ 'objectTypes, nonEnumProps, stringClass, stringProto, toString',
+ 'return function(' + args + ') {\n' + iteratorTemplate(iteratorData) + '\n}'
+ );
+
+ // return the compiled function
+ return factory(
+ baseCreateCallback, errorClass, errorProto, hasOwnProperty,
+ indicatorObject, isArguments, isArray, isString, iteratorData.keys, objectProto,
+ objectTypes, nonEnumProps, stringClass, stringProto, toString
+ );
+}
+
+module.exports = createIterator;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/createWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/createWrapper.js b/node_modules/lodash-node/compat/internals/createWrapper.js
new file mode 100644
index 0000000..2525e10
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/createWrapper.js
@@ -0,0 +1,106 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseBind = require('./baseBind'),
+ baseCreateWrapper = require('./baseCreateWrapper'),
+ isFunction = require('../objects/isFunction'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push,
+ unshift = arrayRef.unshift;
+
+/**
+ * Creates a function that, when called, either curries or invokes `func`
+ * with an optional `this` binding and partially applied arguments.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to reference.
+ * @param {number} bitmask The bitmask of method flags to compose.
+ * The bitmask may be composed of the following flags:
+ * 1 - `_.bind`
+ * 2 - `_.bindKey`
+ * 4 - `_.curry`
+ * 8 - `_.curry` (bound)
+ * 16 - `_.partial`
+ * 32 - `_.partialRight`
+ * @param {Array} [partialArgs] An array of arguments to prepend to those
+ * provided to the new function.
+ * @param {Array} [partialRightArgs] An array of arguments to append to those
+ * provided to the new function.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new function.
+ */
+function createWrapper(func, bitmask, partialArgs, partialRightArgs, thisArg, arity) {
+ var isBind = bitmask & 1,
+ isBindKey = bitmask & 2,
+ isCurry = bitmask & 4,
+ isCurryBound = bitmask & 8,
+ isPartial = bitmask & 16,
+ isPartialRight = bitmask & 32;
+
+ if (!isBindKey && !isFunction(func)) {
+ throw new TypeError;
+ }
+ if (isPartial && !partialArgs.length) {
+ bitmask &= ~16;
+ isPartial = partialArgs = false;
+ }
+ if (isPartialRight && !partialRightArgs.length) {
+ bitmask &= ~32;
+ isPartialRight = partialRightArgs = false;
+ }
+ var bindData = func && func.__bindData__;
+ if (bindData && bindData !== true) {
+ // clone `bindData`
+ bindData = slice(bindData);
+ if (bindData[2]) {
+ bindData[2] = slice(bindData[2]);
+ }
+ if (bindData[3]) {
+ bindData[3] = slice(bindData[3]);
+ }
+ // set `thisBinding` is not previously bound
+ if (isBind && !(bindData[1] & 1)) {
+ bindData[4] = thisArg;
+ }
+ // set if previously bound but not currently (subsequent curried functions)
+ if (!isBind && bindData[1] & 1) {
+ bitmask |= 8;
+ }
+ // set curried arity if not yet set
+ if (isCurry && !(bindData[1] & 4)) {
+ bindData[5] = arity;
+ }
+ // append partial left arguments
+ if (isPartial) {
+ push.apply(bindData[2] || (bindData[2] = []), partialArgs);
+ }
+ // append partial right arguments
+ if (isPartialRight) {
+ unshift.apply(bindData[3] || (bindData[3] = []), partialRightArgs);
+ }
+ // merge flags
+ bindData[1] |= bitmask;
+ return createWrapper.apply(null, bindData);
+ }
+ // fast path for `_.bind`
+ var creater = (bitmask == 1 || bitmask === 17) ? baseBind : baseCreateWrapper;
+ return creater([func, bitmask, partialArgs, partialRightArgs, thisArg, arity]);
+}
+
+module.exports = createWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/defaultsIteratorOptions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/defaultsIteratorOptions.js b/node_modules/lodash-node/compat/internals/defaultsIteratorOptions.js
new file mode 100644
index 0000000..97c87dd
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/defaultsIteratorOptions.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys');
+
+/** Reusable iterator options for `assign` and `defaults` */
+var defaultsIteratorOptions = {
+ 'args': 'object, source, guard',
+ 'top':
+ 'var args = arguments,\n' +
+ ' argsIndex = 0,\n' +
+ " argsLength = typeof guard == 'number' ? 2 : args.length;\n" +
+ 'while (++argsIndex < argsLength) {\n' +
+ ' iterable = args[argsIndex];\n' +
+ ' if (iterable && objectTypes[typeof iterable]) {',
+ 'keys': keys,
+ 'loop': "if (typeof result[index] == 'undefined') result[index] = iterable[index]",
+ 'bottom': ' }\n}'
+};
+
+module.exports = defaultsIteratorOptions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/eachIteratorOptions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/eachIteratorOptions.js b/node_modules/lodash-node/compat/internals/eachIteratorOptions.js
new file mode 100644
index 0000000..0112c82
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/eachIteratorOptions.js
@@ -0,0 +1,20 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys');
+
+/** Reusable iterator options shared by `each`, `forIn`, and `forOwn` */
+var eachIteratorOptions = {
+ 'args': 'collection, callback, thisArg',
+ 'top': "callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3)",
+ 'array': "typeof length == 'number'",
+ 'keys': keys,
+ 'loop': 'if (callback(iterable[index], index, collection) === false) return result'
+};
+
+module.exports = eachIteratorOptions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/escapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/escapeHtmlChar.js b/node_modules/lodash-node/compat/internals/escapeHtmlChar.js
new file mode 100644
index 0000000..10fe163
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/escapeHtmlChar.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes');
+
+/**
+ * Used by `escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} match The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeHtmlChar(match) {
+ return htmlEscapes[match];
+}
+
+module.exports = escapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/escapeStringChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/escapeStringChar.js b/node_modules/lodash-node/compat/internals/escapeStringChar.js
new file mode 100644
index 0000000..00ad70a
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/escapeStringChar.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to escape characters for inclusion in compiled string literals */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\t': 't',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `template` to escape characters for inclusion in compiled
+ * string literals.
+ *
+ * @private
+ * @param {string} match The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeStringChar(match) {
+ return '\\' + stringEscapes[match];
+}
+
+module.exports = escapeStringChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/forOwnIteratorOptions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/forOwnIteratorOptions.js b/node_modules/lodash-node/compat/internals/forOwnIteratorOptions.js
new file mode 100644
index 0000000..831d622
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/forOwnIteratorOptions.js
@@ -0,0 +1,17 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var eachIteratorOptions = require('./eachIteratorOptions');
+
+/** Reusable iterator options for `forIn` and `forOwn` */
+var forOwnIteratorOptions = {
+ 'top': 'if (!objectTypes[typeof iterable]) return result;\n' + eachIteratorOptions.top,
+ 'array': false
+};
+
+module.exports = forOwnIteratorOptions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/getArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/getArray.js b/node_modules/lodash-node/compat/internals/getArray.js
new file mode 100644
index 0000000..9420559
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/getArray.js
@@ -0,0 +1,21 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrayPool = require('./arrayPool');
+
+/**
+ * Gets an array from the array pool or creates a new one if the pool is empty.
+ *
+ * @private
+ * @returns {Array} The array from the pool.
+ */
+function getArray() {
+ return arrayPool.pop() || [];
+}
+
+module.exports = getArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/getObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/getObject.js b/node_modules/lodash-node/compat/internals/getObject.js
new file mode 100644
index 0000000..67b0cb5
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/getObject.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectPool = require('./objectPool');
+
+/**
+ * Gets an object from the object pool or creates a new one if the pool is empty.
+ *
+ * @private
+ * @returns {Object} The object from the pool.
+ */
+function getObject() {
+ return objectPool.pop() || {
+ 'array': null,
+ 'cache': null,
+ 'criteria': null,
+ 'false': false,
+ 'index': 0,
+ 'null': false,
+ 'number': null,
+ 'object': null,
+ 'push': null,
+ 'string': null,
+ 'true': false,
+ 'undefined': false,
+ 'value': null
+ };
+}
+
+module.exports = getObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/htmlEscapes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/htmlEscapes.js b/node_modules/lodash-node/compat/internals/htmlEscapes.js
new file mode 100644
index 0000000..0fd6c1d
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/htmlEscapes.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used to convert characters to HTML entities:
+ *
+ * Though the `>` character is escaped for symmetry, characters like `>` and `/`
+ * don't require escaping in HTML and have no special meaning unless they're part
+ * of a tag or an unquoted attribute value.
+ * http://mathiasbynens.be/notes/ambiguous-ampersands (under "semi-related fun fact")
+ */
+var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+};
+
+module.exports = htmlEscapes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/htmlUnescapes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/htmlUnescapes.js b/node_modules/lodash-node/compat/internals/htmlUnescapes.js
new file mode 100644
index 0000000..9401df9
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/htmlUnescapes.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes'),
+ invert = require('../objects/invert');
+
+/** Used to convert HTML entities to characters */
+var htmlUnescapes = invert(htmlEscapes);
+
+module.exports = htmlUnescapes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/indicatorObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/indicatorObject.js b/node_modules/lodash-node/compat/internals/indicatorObject.js
new file mode 100644
index 0000000..a0f71ef
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/indicatorObject.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used internally to indicate various things */
+var indicatorObject = {};
+
+module.exports = indicatorObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/isNative.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/isNative.js b/node_modules/lodash-node/compat/internals/isNative.js
new file mode 100644
index 0000000..8d729ff
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/isNative.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Used to detect if a method is native */
+var reNative = RegExp('^' +
+ String(toString)
+ .replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
+ .replace(/toString| for [^\]]+/g, '.*?') + '$'
+);
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a native function, else `false`.
+ */
+function isNative(value) {
+ return typeof value == 'function' && reNative.test(value);
+}
+
+module.exports = isNative;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/isNode.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/isNode.js b/node_modules/lodash-node/compat/internals/isNode.js
new file mode 100644
index 0000000..50c5c06
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/isNode.js
@@ -0,0 +1,23 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is a DOM node in IE < 9.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a DOM node, else `false`.
+ */
+function isNode(value) {
+ // IE < 9 presents DOM nodes as `Object` objects except they have `toString`
+ // methods that are `typeof` "string" and still can coerce nodes to strings
+ return typeof value.toString != 'function' && typeof (value + '') == 'string';
+}
+
+module.exports = isNode;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/iteratorTemplate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/iteratorTemplate.js b/node_modules/lodash-node/compat/internals/iteratorTemplate.js
new file mode 100644
index 0000000..e5526dc
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/iteratorTemplate.js
@@ -0,0 +1,109 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var support = require('../support');
+
+/**
+ * The template used to create iterator functions.
+ *
+ * @private
+ * @param {Object} data The data object used to populate the text.
+ * @returns {string} Returns the interpolated text.
+ */
+var iteratorTemplate = function(obj) {
+
+ var __p = 'var index, iterable = ' +
+ (obj.firstArg) +
+ ', result = ' +
+ (obj.init) +
+ ';\nif (!iterable) return result;\n' +
+ (obj.top) +
+ ';';
+ if (obj.array) {
+ __p += '\nvar length = iterable.length; index = -1;\nif (' +
+ (obj.array) +
+ ') { ';
+ if (support.unindexedChars) {
+ __p += '\n if (isString(iterable)) {\n iterable = iterable.split(\'\')\n } ';
+ }
+ __p += '\n while (++index < length) {\n ' +
+ (obj.loop) +
+ ';\n }\n}\nelse { ';
+ } else if (support.nonEnumArgs) {
+ __p += '\n var length = iterable.length; index = -1;\n if (length && isArguments(iterable)) {\n while (++index < length) {\n index += \'\';\n ' +
+ (obj.loop) +
+ ';\n }\n } else { ';
+ }
+
+ if (support.enumPrototypes) {
+ __p += '\n var skipProto = typeof iterable == \'function\';\n ';
+ }
+
+ if (support.enumErrorProps) {
+ __p += '\n var skipErrorProps = iterable === errorProto || iterable instanceof Error;\n ';
+ }
+
+ var conditions = []; if (support.enumPrototypes) { conditions.push('!(skipProto && index == "prototype")'); } if (support.enumErrorProps) { conditions.push('!(skipErrorProps && (index == "message" || index == "name"))'); }
+
+ if (obj.useHas && obj.keys) {
+ __p += '\n var ownIndex = -1,\n ownProps = objectTypes[typeof iterable] && keys(iterable),\n length = ownProps ? ownProps.length : 0;\n\n while (++ownIndex < length) {\n index = ownProps[ownIndex];\n';
+ if (conditions.length) {
+ __p += ' if (' +
+ (conditions.join(' && ')) +
+ ') {\n ';
+ }
+ __p +=
+ (obj.loop) +
+ '; ';
+ if (conditions.length) {
+ __p += '\n }';
+ }
+ __p += '\n } ';
+ } else {
+ __p += '\n for (index in iterable) {\n';
+ if (obj.useHas) { conditions.push("hasOwnProperty.call(iterable, index)"); } if (conditions.length) {
+ __p += ' if (' +
+ (conditions.join(' && ')) +
+ ') {\n ';
+ }
+ __p +=
+ (obj.loop) +
+ '; ';
+ if (conditions.length) {
+ __p += '\n }';
+ }
+ __p += '\n } ';
+ if (support.nonEnumShadows) {
+ __p += '\n\n if (iterable !== objectProto) {\n var ctor = iterable.constructor,\n isProto = iterable === (ctor && ctor.prototype),\n className = iterable === stringProto ? stringClass : iterable === errorProto ? errorClass : toString.call(iterable),\n nonEnum = nonEnumProps[className];\n ';
+ for (k = 0; k < 7; k++) {
+ __p += '\n index = \'' +
+ (obj.shadowedProps[k]) +
+ '\';\n if ((!(isProto && nonEnum[index]) && hasOwnProperty.call(iterable, index))';
+ if (!obj.useHas) {
+ __p += ' || (!nonEnum[index] && iterable[index] !== objectProto[index])';
+ }
+ __p += ') {\n ' +
+ (obj.loop) +
+ ';\n } ';
+ }
+ __p += '\n } ';
+ }
+
+ }
+
+ if (obj.array || support.nonEnumArgs) {
+ __p += '\n}';
+ }
+ __p +=
+ (obj.bottom) +
+ ';\nreturn result';
+
+ return __p
+};
+
+module.exports = iteratorTemplate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/keyPrefix.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/keyPrefix.js b/node_modules/lodash-node/compat/internals/keyPrefix.js
new file mode 100644
index 0000000..be57f36
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/keyPrefix.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to prefix keys to avoid issues with `__proto__` and properties on `Object.prototype` */
+var keyPrefix = +new Date + '';
+
+module.exports = keyPrefix;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/largeArraySize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/largeArraySize.js b/node_modules/lodash-node/compat/internals/largeArraySize.js
new file mode 100644
index 0000000..b61e52d
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/largeArraySize.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used as the size when optimizations are enabled for large arrays */
+var largeArraySize = 75;
+
+module.exports = largeArraySize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/lodashWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/lodashWrapper.js b/node_modules/lodash-node/compat/internals/lodashWrapper.js
new file mode 100644
index 0000000..b8d2442
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/lodashWrapper.js
@@ -0,0 +1,23 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * A fast path for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @param {boolean} chainAll A flag to enable chaining for all methods
+ * @returns {Object} Returns a `lodash` instance.
+ */
+function lodashWrapper(value, chainAll) {
+ this.__chain__ = !!chainAll;
+ this.__wrapped__ = value;
+}
+
+module.exports = lodashWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/maxPoolSize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/maxPoolSize.js b/node_modules/lodash-node/compat/internals/maxPoolSize.js
new file mode 100644
index 0000000..600c92b
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/maxPoolSize.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used as the max size of the `arrayPool` and `objectPool` */
+var maxPoolSize = 40;
+
+module.exports = maxPoolSize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/objectPool.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/objectPool.js b/node_modules/lodash-node/compat/internals/objectPool.js
new file mode 100644
index 0000000..ab798f1
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/objectPool.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to pool arrays and objects used internally */
+var objectPool = [];
+
+module.exports = objectPool;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/objectTypes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/objectTypes.js b/node_modules/lodash-node/compat/internals/objectTypes.js
new file mode 100644
index 0000000..42a9573
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/objectTypes.js
@@ -0,0 +1,20 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to determine if values are of the language type Object */
+var objectTypes = {
+ 'boolean': false,
+ 'function': true,
+ 'object': true,
+ 'number': false,
+ 'string': false,
+ 'undefined': false
+};
+
+module.exports = objectTypes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/reEscapedHtml.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/reEscapedHtml.js b/node_modules/lodash-node/compat/internals/reEscapedHtml.js
new file mode 100644
index 0000000..311180d
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/reEscapedHtml.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlUnescapes = require('./htmlUnescapes'),
+ keys = require('../objects/keys');
+
+/** Used to match HTML entities and HTML characters */
+var reEscapedHtml = RegExp('(' + keys(htmlUnescapes).join('|') + ')', 'g');
+
+module.exports = reEscapedHtml;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/reInterpolate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/reInterpolate.js b/node_modules/lodash-node/compat/internals/reInterpolate.js
new file mode 100644
index 0000000..18287e4
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/reInterpolate.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to match "interpolate" template delimiters */
+var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+module.exports = reInterpolate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/reUnescapedHtml.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/reUnescapedHtml.js b/node_modules/lodash-node/compat/internals/reUnescapedHtml.js
new file mode 100644
index 0000000..10d1871
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/reUnescapedHtml.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes'),
+ keys = require('../objects/keys');
+
+/** Used to match HTML entities and HTML characters */
+var reUnescapedHtml = RegExp('[' + keys(htmlEscapes).join('') + ']', 'g');
+
+module.exports = reUnescapedHtml;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/releaseArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/releaseArray.js b/node_modules/lodash-node/compat/internals/releaseArray.js
new file mode 100644
index 0000000..2c7ccba
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/releaseArray.js
@@ -0,0 +1,25 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrayPool = require('./arrayPool'),
+ maxPoolSize = require('./maxPoolSize');
+
+/**
+ * Releases the given array back to the array pool.
+ *
+ * @private
+ * @param {Array} [array] The array to release.
+ */
+function releaseArray(array) {
+ array.length = 0;
+ if (arrayPool.length < maxPoolSize) {
+ arrayPool.push(array);
+ }
+}
+
+module.exports = releaseArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/releaseObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/releaseObject.js b/node_modules/lodash-node/compat/internals/releaseObject.js
new file mode 100644
index 0000000..6ab3d91
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/releaseObject.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var maxPoolSize = require('./maxPoolSize'),
+ objectPool = require('./objectPool');
+
+/**
+ * Releases the given object back to the object pool.
+ *
+ * @private
+ * @param {Object} [object] The object to release.
+ */
+function releaseObject(object) {
+ var cache = object.cache;
+ if (cache) {
+ releaseObject(cache);
+ }
+ object.array = object.cache = object.criteria = object.object = object.number = object.string = object.value = null;
+ if (objectPool.length < maxPoolSize) {
+ objectPool.push(object);
+ }
+}
+
+module.exports = releaseObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/setBindData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/setBindData.js b/node_modules/lodash-node/compat/internals/setBindData.js
new file mode 100644
index 0000000..f12bd86
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/setBindData.js
@@ -0,0 +1,43 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./isNative'),
+ noop = require('../utilities/noop');
+
+/** Used as the property descriptor for `__bindData__` */
+var descriptor = {
+ 'configurable': false,
+ 'enumerable': false,
+ 'value': null,
+ 'writable': false
+};
+
+/** Used to set meta data on functions */
+var defineProperty = (function() {
+ // IE 8 only accepts DOM elements
+ try {
+ var o = {},
+ func = isNative(func = Object.defineProperty) && func,
+ result = func(o, o, o) && func;
+ } catch(e) { }
+ return result;
+}());
+
+/**
+ * Sets `this` binding data on a given function.
+ *
+ * @private
+ * @param {Function} func The function to set data on.
+ * @param {Array} value The data array to set.
+ */
+var setBindData = !defineProperty ? noop : function(func, value) {
+ descriptor.value = value;
+ defineProperty(func, '__bindData__', descriptor);
+};
+
+module.exports = setBindData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/shimIsPlainObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/shimIsPlainObject.js b/node_modules/lodash-node/compat/internals/shimIsPlainObject.js
new file mode 100644
index 0000000..32bf0f0
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/shimIsPlainObject.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('../objects/forIn'),
+ isArguments = require('../objects/isArguments'),
+ isFunction = require('../objects/isFunction'),
+ isNode = require('./isNode'),
+ support = require('../support');
+
+/** `Object#toString` result shortcuts */
+var objectClass = '[object Object]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A fallback implementation of `isPlainObject` which checks if a given value
+ * is an object created by the `Object` constructor, assuming objects created
+ * by the `Object` constructor have no inherited enumerable properties and that
+ * there are no `Object.prototype` extensions.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ */
+function shimIsPlainObject(value) {
+ var ctor,
+ result;
+
+ // avoid non Object objects, `arguments` objects, and DOM elements
+ if (!(value && toString.call(value) == objectClass) ||
+ (ctor = value.constructor, isFunction(ctor) && !(ctor instanceof ctor)) ||
+ (!support.argsClass && isArguments(value)) ||
+ (!support.nodeClass && isNode(value))) {
+ return false;
+ }
+ // IE < 9 iterates inherited properties before own properties. If the first
+ // iterated property is an object's own property then there are no inherited
+ // enumerable properties.
+ if (support.ownLast) {
+ forIn(value, function(value, key, object) {
+ result = hasOwnProperty.call(object, key);
+ return false;
+ });
+ return result !== false;
+ }
+ // In most environments an object's own properties are iterated before
+ // its inherited properties. If the last iterated property is an object's
+ // own property then there are no inherited enumerable properties.
+ forIn(value, function(value, key) {
+ result = key;
+ });
+ return typeof result == 'undefined' || hasOwnProperty.call(value, result);
+}
+
+module.exports = shimIsPlainObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/shimKeys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/shimKeys.js b/node_modules/lodash-node/compat/internals/shimKeys.js
new file mode 100644
index 0000000..6d70860
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/shimKeys.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createIterator = require('./createIterator');
+
+/**
+ * A fallback implementation of `Object.keys` which produces an array of the
+ * given object's own enumerable property names.
+ *
+ * @private
+ * @type Function
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names.
+ */
+var shimKeys = createIterator({
+ 'args': 'object',
+ 'init': '[]',
+ 'top': 'if (!(objectTypes[typeof object])) return result',
+ 'loop': 'result.push(index)'
+});
+
+module.exports = shimKeys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/slice.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/slice.js b/node_modules/lodash-node/compat/internals/slice.js
new file mode 100644
index 0000000..2f8318b
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/slice.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Slices the `collection` from the `start` index up to, but not including,
+ * the `end` index.
+ *
+ * Note: This function is used instead of `Array#slice` to support node lists
+ * in IE < 9 and to ensure dense arrays are returned.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to slice.
+ * @param {number} start The start index.
+ * @param {number} end The end index.
+ * @returns {Array} Returns the new array.
+ */
+function slice(array, start, end) {
+ start || (start = 0);
+ if (typeof end == 'undefined') {
+ end = array ? array.length : 0;
+ }
+ var index = -1,
+ length = end - start || 0,
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = array[start + index];
+ }
+ return result;
+}
+
+module.exports = slice;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/unescapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/unescapeHtmlChar.js b/node_modules/lodash-node/compat/internals/unescapeHtmlChar.js
new file mode 100644
index 0000000..3b8fd57
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/unescapeHtmlChar.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlUnescapes = require('./htmlUnescapes');
+
+/**
+ * Used by `unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} match The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+function unescapeHtmlChar(match) {
+ return htmlUnescapes[match];
+}
+
+module.exports = unescapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects.js b/node_modules/lodash-node/compat/objects.js
new file mode 100644
index 0000000..dca0d30
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'assign': require('./objects/assign'),
+ 'clone': require('./objects/clone'),
+ 'cloneDeep': require('./objects/cloneDeep'),
+ 'create': require('./objects/create'),
+ 'defaults': require('./objects/defaults'),
+ 'extend': require('./objects/assign'),
+ 'findKey': require('./objects/findKey'),
+ 'findLastKey': require('./objects/findLastKey'),
+ 'forIn': require('./objects/forIn'),
+ 'forInRight': require('./objects/forInRight'),
+ 'forOwn': require('./objects/forOwn'),
+ 'forOwnRight': require('./objects/forOwnRight'),
+ 'functions': require('./objects/functions'),
+ 'has': require('./objects/has'),
+ 'invert': require('./objects/invert'),
+ 'isArguments': require('./objects/isArguments'),
+ 'isArray': require('./objects/isArray'),
+ 'isBoolean': require('./objects/isBoolean'),
+ 'isDate': require('./objects/isDate'),
+ 'isElement': require('./objects/isElement'),
+ 'isEmpty': require('./objects/isEmpty'),
+ 'isEqual': require('./objects/isEqual'),
+ 'isFinite': require('./objects/isFinite'),
+ 'isFunction': require('./objects/isFunction'),
+ 'isNaN': require('./objects/isNaN'),
+ 'isNull': require('./objects/isNull'),
+ 'isNumber': require('./objects/isNumber'),
+ 'isObject': require('./objects/isObject'),
+ 'isPlainObject': require('./objects/isPlainObject'),
+ 'isRegExp': require('./objects/isRegExp'),
+ 'isString': require('./objects/isString'),
+ 'isUndefined': require('./objects/isUndefined'),
+ 'keys': require('./objects/keys'),
+ 'mapValues': require('./objects/mapValues'),
+ 'merge': require('./objects/merge'),
+ 'methods': require('./objects/functions'),
+ 'omit': require('./objects/omit'),
+ 'pairs': require('./objects/pairs'),
+ 'pick': require('./objects/pick'),
+ 'transform': require('./objects/transform'),
+ 'values': require('./objects/values')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/assign.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/assign.js b/node_modules/lodash-node/compat/objects/assign.js
new file mode 100644
index 0000000..73f88ae
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/assign.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createIterator = require('../internals/createIterator'),
+ defaultsIteratorOptions = require('../internals/defaultsIteratorOptions');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object. Subsequent sources will overwrite property assignments of previous
+ * sources. If a callback is provided it will be executed to produce the
+ * assigned values. The callback is bound to `thisArg` and invoked with two
+ * arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @alias extend
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param {Function} [callback] The function to customize assigning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * _.assign({ 'name': 'fred' }, { 'employer': 'slate' });
+ * // => { 'name': 'fred', 'employer': 'slate' }
+ *
+ * var defaults = _.partialRight(_.assign, function(a, b) {
+ * return typeof a == 'undefined' ? b : a;
+ * });
+ *
+ * var object = { 'name': 'barney' };
+ * defaults(object, { 'name': 'fred', 'employer': 'slate' });
+ * // => { 'name': 'barney', 'employer': 'slate' }
+ */
+var assign = createIterator(defaultsIteratorOptions, {
+ 'top':
+ defaultsIteratorOptions.top.replace(';',
+ ';\n' +
+ "if (argsLength > 3 && typeof args[argsLength - 2] == 'function') {\n" +
+ ' var callback = baseCreateCallback(args[--argsLength - 1], args[argsLength--], 2);\n' +
+ "} else if (argsLength > 2 && typeof args[argsLength - 1] == 'function') {\n" +
+ ' callback = args[--argsLength];\n' +
+ '}'
+ ),
+ 'loop': 'result[index] = callback ? callback(result[index], iterable[index]) : iterable[index]'
+});
+
+module.exports = assign;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/clone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/clone.js b/node_modules/lodash-node/compat/objects/clone.js
new file mode 100644
index 0000000..c81b204
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/clone.js
@@ -0,0 +1,63 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseClone = require('../internals/baseClone'),
+ baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Creates a clone of `value`. If `isDeep` is `true` nested objects will also
+ * be cloned, otherwise they will be assigned by reference. If a callback
+ * is provided it will be executed to produce the cloned values. If the
+ * callback returns `undefined` cloning will be handled by the method instead.
+ * The callback is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep=false] Specify a deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the cloned value.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * var shallow = _.clone(characters);
+ * shallow[0] === characters[0];
+ * // => true
+ *
+ * var deep = _.clone(characters, true);
+ * deep[0] === characters[0];
+ * // => false
+ *
+ * _.mixin({
+ * 'clone': _.partialRight(_.clone, function(value) {
+ * return _.isElement(value) ? value.cloneNode(false) : undefined;
+ * })
+ * });
+ *
+ * var clone = _.clone(document.body);
+ * clone.childNodes.length;
+ * // => 0
+ */
+function clone(value, isDeep, callback, thisArg) {
+ // allows working with "Collections" methods without using their `index`
+ // and `collection` arguments for `isDeep` and `callback`
+ if (typeof isDeep != 'boolean' && isDeep != null) {
+ thisArg = callback;
+ callback = isDeep;
+ isDeep = false;
+ }
+ return baseClone(value, isDeep, typeof callback == 'function' && baseCreateCallback(callback, thisArg, 1));
+}
+
+module.exports = clone;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[54/59] [abbrv] mac commit: CB-10668 fixed failing tests,
reverted shelljs to 0.5.3
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/ls.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/ls.js b/node_modules/cordova-common/node_modules/shelljs/src/ls.js
deleted file mode 100644
index 3345db4..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/ls.js
+++ /dev/null
@@ -1,126 +0,0 @@
-var path = require('path');
-var fs = require('fs');
-var common = require('./common');
-var _cd = require('./cd');
-var _pwd = require('./pwd');
-
-//@
-//@ ### ls([options ,] path [,path ...])
-//@ ### ls([options ,] path_array)
-//@ Available options:
-//@
-//@ + `-R`: recursive
-//@ + `-A`: all files (include files beginning with `.`, except for `.` and `..`)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ ls('projs/*.js');
-//@ ls('-R', '/users/me', '/tmp');
-//@ ls('-R', ['/users/me', '/tmp']); // same as above
-//@ ```
-//@
-//@ Returns array of files in the given path, or in current directory if no path provided.
-function _ls(options, paths) {
- options = common.parseOptions(options, {
- 'R': 'recursive',
- 'A': 'all',
- 'a': 'all_deprecated'
- });
-
- if (options.all_deprecated) {
- // We won't support the -a option as it's hard to image why it's useful
- // (it includes '.' and '..' in addition to '.*' files)
- // For backwards compatibility we'll dump a deprecated message and proceed as before
- common.log('ls: Option -a is deprecated. Use -A instead');
- options.all = true;
- }
-
- if (!paths)
- paths = ['.'];
- else if (typeof paths === 'object')
- paths = paths; // assume array
- else if (typeof paths === 'string')
- paths = [].slice.call(arguments, 1);
-
- var list = [];
-
- // Conditionally pushes file to list - returns true if pushed, false otherwise
- // (e.g. prevents hidden files to be included unless explicitly told so)
- function pushFile(file, query) {
- // hidden file?
- if (path.basename(file)[0] === '.') {
- // not explicitly asking for hidden files?
- if (!options.all && !(path.basename(query)[0] === '.' && path.basename(query).length > 1))
- return false;
- }
-
- if (common.platform === 'win')
- file = file.replace(/\\/g, '/');
-
- list.push(file);
- return true;
- }
-
- paths.forEach(function(p) {
- if (fs.existsSync(p)) {
- var stats = fs.statSync(p);
- // Simple file?
- if (stats.isFile()) {
- pushFile(p, p);
- return; // continue
- }
-
- // Simple dir?
- if (stats.isDirectory()) {
- // Iterate over p contents
- fs.readdirSync(p).forEach(function(file) {
- if (!pushFile(file, p))
- return;
-
- // Recursive?
- if (options.recursive) {
- var oldDir = _pwd();
- _cd('', p);
- if (fs.statSync(file).isDirectory())
- list = list.concat(_ls('-R'+(options.all?'A':''), file+'/*'));
- _cd('', oldDir);
- }
- });
- return; // continue
- }
- }
-
- // p does not exist - possible wildcard present
-
- var basename = path.basename(p);
- var dirname = path.dirname(p);
- // Wildcard present on an existing dir? (e.g. '/tmp/*.js')
- if (basename.search(/\*/) > -1 && fs.existsSync(dirname) && fs.statSync(dirname).isDirectory) {
- // Escape special regular expression chars
- var regexp = basename.replace(/(\^|\$|\(|\)|<|>|\[|\]|\{|\}|\.|\+|\?)/g, '\\$1');
- // Translates wildcard into regex
- regexp = '^' + regexp.replace(/\*/g, '.*') + '$';
- // Iterate over directory contents
- fs.readdirSync(dirname).forEach(function(file) {
- if (file.match(new RegExp(regexp))) {
- if (!pushFile(path.normalize(dirname+'/'+file), basename))
- return;
-
- // Recursive?
- if (options.recursive) {
- var pp = dirname + '/' + file;
- if (fs.lstatSync(pp).isDirectory())
- list = list.concat(_ls('-R'+(options.all?'A':''), pp+'/*'));
- } // recursive
- } // if file matches
- }); // forEach
- return;
- }
-
- common.error('no such file or directory: ' + p, true);
- });
-
- return list;
-}
-module.exports = _ls;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js b/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js
deleted file mode 100644
index 5a7088f..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js
+++ /dev/null
@@ -1,68 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-// Recursively creates 'dir'
-function mkdirSyncRecursive(dir) {
- var baseDir = path.dirname(dir);
-
- // Base dir exists, no recursion necessary
- if (fs.existsSync(baseDir)) {
- fs.mkdirSync(dir, parseInt('0777', 8));
- return;
- }
-
- // Base dir does not exist, go recursive
- mkdirSyncRecursive(baseDir);
-
- // Base dir created, can create dir
- fs.mkdirSync(dir, parseInt('0777', 8));
-}
-
-//@
-//@ ### mkdir([options ,] dir [, dir ...])
-//@ ### mkdir([options ,] dir_array)
-//@ Available options:
-//@
-//@ + `p`: full path (will create intermediate dirs if necessary)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
-//@ mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
-//@ ```
-//@
-//@ Creates directories.
-function _mkdir(options, dirs) {
- options = common.parseOptions(options, {
- 'p': 'fullpath'
- });
- if (!dirs)
- common.error('no paths given');
-
- if (typeof dirs === 'string')
- dirs = [].slice.call(arguments, 1);
- // if it's array leave it as it is
-
- dirs.forEach(function(dir) {
- if (fs.existsSync(dir)) {
- if (!options.fullpath)
- common.error('path already exists: ' + dir, true);
- return; // skip dir
- }
-
- // Base dir does not exist, and no -p option given
- var baseDir = path.dirname(dir);
- if (!fs.existsSync(baseDir) && !options.fullpath) {
- common.error('no such file or directory: ' + baseDir, true);
- return; // skip dir
- }
-
- if (options.fullpath)
- mkdirSyncRecursive(dir);
- else
- fs.mkdirSync(dir, parseInt('0777', 8));
- });
-} // mkdir
-module.exports = _mkdir;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/mv.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/mv.js b/node_modules/cordova-common/node_modules/shelljs/src/mv.js
deleted file mode 100644
index 11f9607..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/mv.js
+++ /dev/null
@@ -1,80 +0,0 @@
-var fs = require('fs');
-var path = require('path');
-var common = require('./common');
-
-//@
-//@ ### mv(source [, source ...], dest')
-//@ ### mv(source_array, dest')
-//@ Available options:
-//@
-//@ + `f`: force
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ mv('-f', 'file', 'dir/');
-//@ mv('file1', 'file2', 'dir/');
-//@ mv(['file1', 'file2'], 'dir/'); // same as above
-//@ ```
-//@
-//@ Moves files. The wildcard `*` is accepted.
-function _mv(options, sources, dest) {
- options = common.parseOptions(options, {
- 'f': 'force'
- });
-
- // Get sources, dest
- if (arguments.length < 3) {
- common.error('missing <source> and/or <dest>');
- } else if (arguments.length > 3) {
- sources = [].slice.call(arguments, 1, arguments.length - 1);
- dest = arguments[arguments.length - 1];
- } else if (typeof sources === 'string') {
- sources = [sources];
- } else if ('length' in sources) {
- sources = sources; // no-op for array
- } else {
- common.error('invalid arguments');
- }
-
- sources = common.expand(sources);
-
- var exists = fs.existsSync(dest),
- stats = exists && fs.statSync(dest);
-
- // Dest is not existing dir, but multiple sources given
- if ((!exists || !stats.isDirectory()) && sources.length > 1)
- common.error('dest is not a directory (too many sources)');
-
- // Dest is an existing file, but no -f given
- if (exists && stats.isFile() && !options.force)
- common.error('dest file already exists: ' + dest);
-
- sources.forEach(function(src) {
- if (!fs.existsSync(src)) {
- common.error('no such file or directory: '+src, true);
- return; // skip file
- }
-
- // If here, src exists
-
- // When copying to '/path/dir':
- // thisDest = '/path/dir/file1'
- var thisDest = dest;
- if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
- thisDest = path.normalize(dest + '/' + path.basename(src));
-
- if (fs.existsSync(thisDest) && !options.force) {
- common.error('dest file already exists: ' + thisDest, true);
- return; // skip file
- }
-
- if (path.resolve(src) === path.dirname(path.resolve(thisDest))) {
- common.error('cannot move to self: '+src, true);
- return; // skip file
- }
-
- fs.renameSync(src, thisDest);
- }); // forEach(src)
-} // mv
-module.exports = _mv;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/popd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/popd.js b/node_modules/cordova-common/node_modules/shelljs/src/popd.js
deleted file mode 100644
index 11ea24f..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/popd.js
+++ /dev/null
@@ -1 +0,0 @@
-// see dirs.js
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/pushd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/pushd.js b/node_modules/cordova-common/node_modules/shelljs/src/pushd.js
deleted file mode 100644
index 11ea24f..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/pushd.js
+++ /dev/null
@@ -1 +0,0 @@
-// see dirs.js
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/pwd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/pwd.js b/node_modules/cordova-common/node_modules/shelljs/src/pwd.js
deleted file mode 100644
index 41727bb..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/pwd.js
+++ /dev/null
@@ -1,11 +0,0 @@
-var path = require('path');
-var common = require('./common');
-
-//@
-//@ ### pwd()
-//@ Returns the current directory.
-function _pwd(options) {
- var pwd = path.resolve(process.cwd());
- return common.ShellString(pwd);
-}
-module.exports = _pwd;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/rm.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/rm.js b/node_modules/cordova-common/node_modules/shelljs/src/rm.js
deleted file mode 100644
index bd608cb..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/rm.js
+++ /dev/null
@@ -1,163 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-// Recursively removes 'dir'
-// Adapted from https://github.com/ryanmcgrath/wrench-js
-//
-// Copyright (c) 2010 Ryan McGrath
-// Copyright (c) 2012 Artur Adib
-//
-// Licensed under the MIT License
-// http://www.opensource.org/licenses/mit-license.php
-function rmdirSyncRecursive(dir, force) {
- var files;
-
- files = fs.readdirSync(dir);
-
- // Loop through and delete everything in the sub-tree after checking it
- for(var i = 0; i < files.length; i++) {
- var file = dir + "/" + files[i],
- currFile = fs.lstatSync(file);
-
- if(currFile.isDirectory()) { // Recursive function back to the beginning
- rmdirSyncRecursive(file, force);
- }
-
- else if(currFile.isSymbolicLink()) { // Unlink symlinks
- if (force || isWriteable(file)) {
- try {
- common.unlinkSync(file);
- } catch (e) {
- common.error('could not remove file (code '+e.code+'): ' + file, true);
- }
- }
- }
-
- else // Assume it's a file - perhaps a try/catch belongs here?
- if (force || isWriteable(file)) {
- try {
- common.unlinkSync(file);
- } catch (e) {
- common.error('could not remove file (code '+e.code+'): ' + file, true);
- }
- }
- }
-
- // Now that we know everything in the sub-tree has been deleted, we can delete the main directory.
- // Huzzah for the shopkeep.
-
- var result;
- try {
- // Retry on windows, sometimes it takes a little time before all the files in the directory are gone
- var start = Date.now();
- while (true) {
- try {
- result = fs.rmdirSync(dir);
- if (fs.existsSync(dir)) throw { code: "EAGAIN" }
- break;
- } catch(er) {
- // In addition to error codes, also check if the directory still exists and loop again if true
- if (process.platform === "win32" && (er.code === "ENOTEMPTY" || er.code === "EBUSY" || er.code === "EPERM" || er.code === "EAGAIN")) {
- if (Date.now() - start > 1000) throw er;
- } else if (er.code === "ENOENT") {
- // Directory did not exist, deletion was successful
- break;
- } else {
- throw er;
- }
- }
- }
- } catch(e) {
- common.error('could not remove directory (code '+e.code+'): ' + dir, true);
- }
-
- return result;
-} // rmdirSyncRecursive
-
-// Hack to determine if file has write permissions for current user
-// Avoids having to check user, group, etc, but it's probably slow
-function isWriteable(file) {
- var writePermission = true;
- try {
- var __fd = fs.openSync(file, 'a');
- fs.closeSync(__fd);
- } catch(e) {
- writePermission = false;
- }
-
- return writePermission;
-}
-
-//@
-//@ ### rm([options ,] file [, file ...])
-//@ ### rm([options ,] file_array)
-//@ Available options:
-//@
-//@ + `-f`: force
-//@ + `-r, -R`: recursive
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ rm('-rf', '/tmp/*');
-//@ rm('some_file.txt', 'another_file.txt');
-//@ rm(['some_file.txt', 'another_file.txt']); // same as above
-//@ ```
-//@
-//@ Removes files. The wildcard `*` is accepted.
-function _rm(options, files) {
- options = common.parseOptions(options, {
- 'f': 'force',
- 'r': 'recursive',
- 'R': 'recursive'
- });
- if (!files)
- common.error('no paths given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 1);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- files.forEach(function(file) {
- if (!fs.existsSync(file)) {
- // Path does not exist, no force flag given
- if (!options.force)
- common.error('no such file or directory: '+file, true);
-
- return; // skip file
- }
-
- // If here, path exists
-
- var stats = fs.lstatSync(file);
- if (stats.isFile() || stats.isSymbolicLink()) {
-
- // Do not check for file writing permissions
- if (options.force) {
- common.unlinkSync(file);
- return;
- }
-
- if (isWriteable(file))
- common.unlinkSync(file);
- else
- common.error('permission denied: '+file, true);
-
- return;
- } // simple file
-
- // Path is an existing directory, but no -r flag given
- if (stats.isDirectory() && !options.recursive) {
- common.error('path is a directory', true);
- return; // skip path
- }
-
- // Recursively remove existing directory
- if (stats.isDirectory() && options.recursive) {
- rmdirSyncRecursive(file, options.force);
- }
- }); // forEach(file)
-} // rm
-module.exports = _rm;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/sed.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/sed.js b/node_modules/cordova-common/node_modules/shelljs/src/sed.js
deleted file mode 100644
index 65f7cb4..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/sed.js
+++ /dev/null
@@ -1,43 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### sed([options ,] search_regex, replacement, file)
-//@ Available options:
-//@
-//@ + `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
-//@ sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
-//@ ```
-//@
-//@ Reads an input string from `file` and performs a JavaScript `replace()` on the input
-//@ using the given search regex and replacement string or function. Returns the new string after replacement.
-function _sed(options, regex, replacement, file) {
- options = common.parseOptions(options, {
- 'i': 'inplace'
- });
-
- if (typeof replacement === 'string' || typeof replacement === 'function')
- replacement = replacement; // no-op
- else if (typeof replacement === 'number')
- replacement = replacement.toString(); // fallback
- else
- common.error('invalid replacement string');
-
- if (!file)
- common.error('no file given');
-
- if (!fs.existsSync(file))
- common.error('no such file or directory: ' + file);
-
- var result = fs.readFileSync(file, 'utf8').replace(regex, replacement);
- if (options.inplace)
- fs.writeFileSync(file, result, 'utf8');
-
- return common.ShellString(result);
-}
-module.exports = _sed;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js b/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js
deleted file mode 100644
index 45953c2..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js
+++ /dev/null
@@ -1,56 +0,0 @@
-var common = require('./common');
-var os = require('os');
-var fs = require('fs');
-
-// Returns false if 'dir' is not a writeable directory, 'dir' otherwise
-function writeableDir(dir) {
- if (!dir || !fs.existsSync(dir))
- return false;
-
- if (!fs.statSync(dir).isDirectory())
- return false;
-
- var testFile = dir+'/'+common.randomFileName();
- try {
- fs.writeFileSync(testFile, ' ');
- common.unlinkSync(testFile);
- return dir;
- } catch (e) {
- return false;
- }
-}
-
-
-//@
-//@ ### tempdir()
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var tmp = tempdir(); // "/tmp" for most *nix platforms
-//@ ```
-//@
-//@ Searches and returns string containing a writeable, platform-dependent temporary directory.
-//@ Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
-function _tempDir() {
- var state = common.state;
- if (state.tempDir)
- return state.tempDir; // from cache
-
- state.tempDir = writeableDir(os.tempDir && os.tempDir()) || // node 0.8+
- writeableDir(process.env['TMPDIR']) ||
- writeableDir(process.env['TEMP']) ||
- writeableDir(process.env['TMP']) ||
- writeableDir(process.env['Wimp$ScrapDir']) || // RiscOS
- writeableDir('C:\\TEMP') || // Windows
- writeableDir('C:\\TMP') || // Windows
- writeableDir('\\TEMP') || // Windows
- writeableDir('\\TMP') || // Windows
- writeableDir('/tmp') ||
- writeableDir('/var/tmp') ||
- writeableDir('/usr/tmp') ||
- writeableDir('.'); // last resort
-
- return state.tempDir;
-}
-module.exports = _tempDir;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/test.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/test.js b/node_modules/cordova-common/node_modules/shelljs/src/test.js
deleted file mode 100644
index 8a4ac7d..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/test.js
+++ /dev/null
@@ -1,85 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### test(expression)
-//@ Available expression primaries:
-//@
-//@ + `'-b', 'path'`: true if path is a block device
-//@ + `'-c', 'path'`: true if path is a character device
-//@ + `'-d', 'path'`: true if path is a directory
-//@ + `'-e', 'path'`: true if path exists
-//@ + `'-f', 'path'`: true if path is a regular file
-//@ + `'-L', 'path'`: true if path is a symboilc link
-//@ + `'-p', 'path'`: true if path is a pipe (FIFO)
-//@ + `'-S', 'path'`: true if path is a socket
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ if (test('-d', path)) { /* do something with dir */ };
-//@ if (!test('-f', path)) continue; // skip if it's a regular file
-//@ ```
-//@
-//@ Evaluates expression using the available primaries and returns corresponding value.
-function _test(options, path) {
- if (!path)
- common.error('no path given');
-
- // hack - only works with unary primaries
- options = common.parseOptions(options, {
- 'b': 'block',
- 'c': 'character',
- 'd': 'directory',
- 'e': 'exists',
- 'f': 'file',
- 'L': 'link',
- 'p': 'pipe',
- 'S': 'socket'
- });
-
- var canInterpret = false;
- for (var key in options)
- if (options[key] === true) {
- canInterpret = true;
- break;
- }
-
- if (!canInterpret)
- common.error('could not interpret expression');
-
- if (options.link) {
- try {
- return fs.lstatSync(path).isSymbolicLink();
- } catch(e) {
- return false;
- }
- }
-
- if (!fs.existsSync(path))
- return false;
-
- if (options.exists)
- return true;
-
- var stats = fs.statSync(path);
-
- if (options.block)
- return stats.isBlockDevice();
-
- if (options.character)
- return stats.isCharacterDevice();
-
- if (options.directory)
- return stats.isDirectory();
-
- if (options.file)
- return stats.isFile();
-
- if (options.pipe)
- return stats.isFIFO();
-
- if (options.socket)
- return stats.isSocket();
-} // test
-module.exports = _test;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/to.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/to.js b/node_modules/cordova-common/node_modules/shelljs/src/to.js
deleted file mode 100644
index f029999..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/to.js
+++ /dev/null
@@ -1,29 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-//@
-//@ ### 'string'.to(file)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ cat('input.txt').to('output.txt');
-//@ ```
-//@
-//@ Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
-//@ those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
-function _to(options, file) {
- if (!file)
- common.error('wrong arguments');
-
- if (!fs.existsSync( path.dirname(file) ))
- common.error('no such file or directory: ' + path.dirname(file));
-
- try {
- fs.writeFileSync(file, this.toString(), 'utf8');
- } catch(e) {
- common.error('could not write to file (code '+e.code+'): '+file, true);
- }
-}
-module.exports = _to;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js b/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js
deleted file mode 100644
index f6d099d..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js
+++ /dev/null
@@ -1,29 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-//@
-//@ ### 'string'.toEnd(file)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ cat('input.txt').toEnd('output.txt');
-//@ ```
-//@
-//@ Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
-//@ those returned by `cat`, `grep`, etc).
-function _toEnd(options, file) {
- if (!file)
- common.error('wrong arguments');
-
- if (!fs.existsSync( path.dirname(file) ))
- common.error('no such file or directory: ' + path.dirname(file));
-
- try {
- fs.appendFileSync(file, this.toString(), 'utf8');
- } catch(e) {
- common.error('could not append to file (code '+e.code+'): '+file, true);
- }
-}
-module.exports = _toEnd;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/which.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/which.js b/node_modules/cordova-common/node_modules/shelljs/src/which.js
deleted file mode 100644
index 2822ecf..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/which.js
+++ /dev/null
@@ -1,83 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-// Cross-platform method for splitting environment PATH variables
-function splitPath(p) {
- for (i=1;i<2;i++) {}
-
- if (!p)
- return [];
-
- if (common.platform === 'win')
- return p.split(';');
- else
- return p.split(':');
-}
-
-function checkPath(path) {
- return fs.existsSync(path) && fs.statSync(path).isDirectory() == false;
-}
-
-//@
-//@ ### which(command)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var nodeExec = which('node');
-//@ ```
-//@
-//@ Searches for `command` in the system's PATH. On Windows looks for `.exe`, `.cmd`, and `.bat` extensions.
-//@ Returns string containing the absolute path to the command.
-function _which(options, cmd) {
- if (!cmd)
- common.error('must specify command');
-
- var pathEnv = process.env.path || process.env.Path || process.env.PATH,
- pathArray = splitPath(pathEnv),
- where = null;
-
- // No relative/absolute paths provided?
- if (cmd.search(/\//) === -1) {
- // Search for command in PATH
- pathArray.forEach(function(dir) {
- if (where)
- return; // already found it
-
- var attempt = path.resolve(dir + '/' + cmd);
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
-
- if (common.platform === 'win') {
- var baseAttempt = attempt;
- attempt = baseAttempt + '.exe';
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
- attempt = baseAttempt + '.cmd';
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
- attempt = baseAttempt + '.bat';
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
- } // if 'win'
- });
- }
-
- // Command not found anywhere?
- if (!checkPath(cmd) && !where)
- return null;
-
- where = where || path.resolve(cmd);
-
- return common.ShellString(where);
-}
-module.exports = _which;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/glob/package.json
----------------------------------------------------------------------
diff --git a/node_modules/glob/package.json b/node_modules/glob/package.json
index ed9e928..2b5aa7b 100644
--- a/node_modules/glob/package.json
+++ b/node_modules/glob/package.json
@@ -26,7 +26,8 @@
"type": "range"
},
"_requiredBy": [
- "/cordova-common"
+ "/cordova-common",
+ "/istanbul/fileset"
],
"_resolved": "http://registry.npmjs.org/glob/-/glob-5.0.15.tgz",
"_shasum": "1bc936b9e02f4a603fcc222ecf7633d30b8b93b1",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/inflight/package.json
----------------------------------------------------------------------
diff --git a/node_modules/inflight/package.json b/node_modules/inflight/package.json
index 05813cb..dd48a47 100644
--- a/node_modules/inflight/package.json
+++ b/node_modules/inflight/package.json
@@ -26,7 +26,11 @@
"type": "range"
},
"_requiredBy": [
- "/glob"
+ "/glob",
+ "/nyc/glob",
+ "/rimraf/glob",
+ "/tap-mocha-reporter/glob",
+ "/tap/glob"
],
"_resolved": "http://registry.npmjs.org/inflight/-/inflight-1.0.4.tgz",
"_shasum": "6cbb4521ebd51ce0ec0a936bfd7657ef7e9b172a",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/inherits/package.json
----------------------------------------------------------------------
diff --git a/node_modules/inherits/package.json b/node_modules/inherits/package.json
index 245ab13..dbf3ad1 100644
--- a/node_modules/inherits/package.json
+++ b/node_modules/inherits/package.json
@@ -25,7 +25,20 @@
"type": "range"
},
"_requiredBy": [
- "/glob"
+ "/bl/readable-stream",
+ "/cli/glob",
+ "/coveralls/readable-stream",
+ "/fileset/glob",
+ "/glob",
+ "/nyc/glob",
+ "/readable-stream",
+ "/rimraf/glob",
+ "/tap-mocha-reporter/glob",
+ "/tap-parser",
+ "/tap-parser/readable-stream",
+ "/tap/glob",
+ "/tap/readable-stream",
+ "/tape"
],
"_resolved": "http://registry.npmjs.org/inherits/-/inherits-2.0.1.tgz",
"_shasum": "b17d08d326b4423e568eff719f91b0b1cbdf69f1",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/lodash/package.json
----------------------------------------------------------------------
diff --git a/node_modules/lodash/package.json b/node_modules/lodash/package.json
index 99a91e2..455070b 100644
--- a/node_modules/lodash/package.json
+++ b/node_modules/lodash/package.json
@@ -26,6 +26,7 @@
"type": "range"
},
"_requiredBy": [
+ "/unicode-length",
"/xmlbuilder"
],
"_resolved": "http://registry.npmjs.org/lodash/-/lodash-3.10.1.tgz",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/minimatch/package.json
----------------------------------------------------------------------
diff --git a/node_modules/minimatch/package.json b/node_modules/minimatch/package.json
index 85c32dc..e0a9c84 100644
--- a/node_modules/minimatch/package.json
+++ b/node_modules/minimatch/package.json
@@ -26,7 +26,11 @@
"type": "range"
},
"_requiredBy": [
- "/glob"
+ "/glob",
+ "/nyc/glob",
+ "/rimraf/glob",
+ "/tap-mocha-reporter/glob",
+ "/tap/glob"
],
"_resolved": "http://registry.npmjs.org/minimatch/-/minimatch-3.0.0.tgz",
"_shasum": "5236157a51e4f004c177fb3c527ff7dd78f0ef83",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/nopt/package.json
----------------------------------------------------------------------
diff --git a/node_modules/nopt/package.json b/node_modules/nopt/package.json
index 4628882..e3d52e9 100644
--- a/node_modules/nopt/package.json
+++ b/node_modules/nopt/package.json
@@ -26,7 +26,8 @@
"type": "range"
},
"_requiredBy": [
- "/"
+ "/",
+ "/istanbul"
],
"_resolved": "http://registry.npmjs.org/nopt/-/nopt-3.0.6.tgz",
"_shasum": "c6465dbf08abcd4db359317f79ac68a646b28ff9",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/once/package.json
----------------------------------------------------------------------
diff --git a/node_modules/once/package.json b/node_modules/once/package.json
index 15cb893..fb5a7f1 100644
--- a/node_modules/once/package.json
+++ b/node_modules/once/package.json
@@ -27,7 +27,12 @@
},
"_requiredBy": [
"/glob",
- "/inflight"
+ "/inflight",
+ "/istanbul",
+ "/nyc/glob",
+ "/rimraf/glob",
+ "/tap-mocha-reporter/glob",
+ "/tap/glob"
],
"_resolved": "http://registry.npmjs.org/once/-/once-1.3.3.tgz",
"_shasum": "b2e261557ce4c314ec8304f3fa82663e4297ca20",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/os-homedir/package.json
----------------------------------------------------------------------
diff --git a/node_modules/os-homedir/package.json b/node_modules/os-homedir/package.json
index 2c7e01f..062614d 100644
--- a/node_modules/os-homedir/package.json
+++ b/node_modules/os-homedir/package.json
@@ -26,7 +26,8 @@
"type": "range"
},
"_requiredBy": [
- "/osenv"
+ "/osenv",
+ "/spawn-wrap"
],
"_resolved": "http://registry.npmjs.org/os-homedir/-/os-homedir-1.0.1.tgz",
"_shasum": "0d62bdf44b916fd3bbdcf2cab191948fb094f007",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/os-tmpdir/package.json
----------------------------------------------------------------------
diff --git a/node_modules/os-tmpdir/package.json b/node_modules/os-tmpdir/package.json
index 097f803..7faa398 100644
--- a/node_modules/os-tmpdir/package.json
+++ b/node_modules/os-tmpdir/package.json
@@ -26,7 +26,8 @@
"type": "range"
},
"_requiredBy": [
- "/osenv"
+ "/osenv",
+ "/tmp"
],
"_resolved": "http://registry.npmjs.org/os-tmpdir/-/os-tmpdir-1.0.1.tgz",
"_shasum": "e9b423a1edaf479882562e92ed71d7743a071b6e",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/path-is-absolute/package.json
----------------------------------------------------------------------
diff --git a/node_modules/path-is-absolute/package.json b/node_modules/path-is-absolute/package.json
index def9f9f..ac19376 100644
--- a/node_modules/path-is-absolute/package.json
+++ b/node_modules/path-is-absolute/package.json
@@ -26,7 +26,11 @@
"type": "range"
},
"_requiredBy": [
- "/glob"
+ "/glob",
+ "/nyc/glob",
+ "/rimraf/glob",
+ "/tap-mocha-reporter/glob",
+ "/tap/glob"
],
"_resolved": "http://registry.npmjs.org/path-is-absolute/-/path-is-absolute-1.0.0.tgz",
"_shasum": "263dada66ab3f2fb10bf7f9d24dd8f3e570ef912",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/semver/package.json
----------------------------------------------------------------------
diff --git a/node_modules/semver/package.json b/node_modules/semver/package.json
index cbd09b4..6e31d06 100644
--- a/node_modules/semver/package.json
+++ b/node_modules/semver/package.json
@@ -26,7 +26,8 @@
"type": "range"
},
"_requiredBy": [
- "/cordova-common"
+ "/cordova-common",
+ "/normalize-package-data"
],
"_resolved": "http://registry.npmjs.org/semver/-/semver-5.1.0.tgz",
"_shasum": "85f2cf8550465c4df000cf7d86f6b054106ab9e5",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/.name
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/.name b/node_modules/shelljs/.idea/.name
deleted file mode 100644
index 68840c9..0000000
--- a/node_modules/shelljs/.idea/.name
+++ /dev/null
@@ -1 +0,0 @@
-shelljs
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/encodings.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/encodings.xml b/node_modules/shelljs/.idea/encodings.xml
deleted file mode 100644
index 97626ba..0000000
--- a/node_modules/shelljs/.idea/encodings.xml
+++ /dev/null
@@ -1,6 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<project version="4">
- <component name="Encoding">
- <file url="PROJECT" charset="UTF-8" />
- </component>
-</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml b/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml
deleted file mode 100644
index e6d8387..0000000
--- a/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml
+++ /dev/null
@@ -1,7 +0,0 @@
-<component name="InspectionProjectProfileManager">
- <profile version="1.0">
- <option name="myName" value="Project Default" />
- <inspection_tool class="JSLastCommaInArrayLiteral" enabled="false" level="WARNING" enabled_by_default="false" />
- <inspection_tool class="JSLastCommaInObjectLiteral" enabled="false" level="WARNING" enabled_by_default="false" />
- </profile>
-</component>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml b/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml
deleted file mode 100644
index 3b31283..0000000
--- a/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml
+++ /dev/null
@@ -1,7 +0,0 @@
-<component name="InspectionProjectProfileManager">
- <settings>
- <option name="PROJECT_PROFILE" value="Project Default" />
- <option name="USE_PROJECT_PROFILE" value="true" />
- <version value="1.0" />
- </settings>
-</component>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/jsLibraryMappings.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/jsLibraryMappings.xml b/node_modules/shelljs/.idea/jsLibraryMappings.xml
deleted file mode 100644
index 40bdc77..0000000
--- a/node_modules/shelljs/.idea/jsLibraryMappings.xml
+++ /dev/null
@@ -1,6 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<project version="4">
- <component name="JavaScriptLibraryMappings">
- <file url="file://$PROJECT_DIR$" libraries="{shelljs node_modules}" />
- </component>
-</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml b/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml
deleted file mode 100644
index b1e5dce..0000000
--- a/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml
+++ /dev/null
@@ -1,14 +0,0 @@
-<component name="libraryTable">
- <library name="shelljs node_modules" type="javaScript">
- <properties>
- <option name="frameworkName" value="node_modules" />
- <sourceFilesUrls>
- <item url="file://$PROJECT_DIR$/node_modules" />
- </sourceFilesUrls>
- </properties>
- <CLASSES>
- <root url="file://$PROJECT_DIR$/node_modules" />
- </CLASSES>
- <SOURCES />
- </library>
-</component>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/misc.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/misc.xml b/node_modules/shelljs/.idea/misc.xml
deleted file mode 100644
index f524616..0000000
--- a/node_modules/shelljs/.idea/misc.xml
+++ /dev/null
@@ -1,28 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<project version="4">
- <component name="ProjectLevelVcsManager" settingsEditedManually="false">
- <OptionsSetting value="true" id="Add" />
- <OptionsSetting value="true" id="Remove" />
- <OptionsSetting value="true" id="Checkout" />
- <OptionsSetting value="true" id="Update" />
- <OptionsSetting value="true" id="Status" />
- <OptionsSetting value="true" id="Edit" />
- <ConfirmationsSetting value="0" id="Add" />
- <ConfirmationsSetting value="0" id="Remove" />
- </component>
- <component name="masterDetails">
- <states>
- <state key="ScopeChooserConfigurable.UI">
- <settings>
- <splitter-proportions>
- <option name="proportions">
- <list>
- <option value="0.2" />
- </list>
- </option>
- </splitter-proportions>
- </settings>
- </state>
- </states>
- </component>
-</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/modules.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/modules.xml b/node_modules/shelljs/.idea/modules.xml
deleted file mode 100644
index 26f2275..0000000
--- a/node_modules/shelljs/.idea/modules.xml
+++ /dev/null
@@ -1,8 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<project version="4">
- <component name="ProjectModuleManager">
- <modules>
- <module fileurl="file://$PROJECT_DIR$/.idea/shelljs.iml" filepath="$PROJECT_DIR$/.idea/shelljs.iml" />
- </modules>
- </component>
-</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/shelljs.iml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/shelljs.iml b/node_modules/shelljs/.idea/shelljs.iml
deleted file mode 100644
index 89f6e57..0000000
--- a/node_modules/shelljs/.idea/shelljs.iml
+++ /dev/null
@@ -1,9 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<module type="WEB_MODULE" version="4">
- <component name="NewModuleRootManager">
- <content url="file://$MODULE_DIR$" />
- <orderEntry type="inheritedJdk" />
- <orderEntry type="sourceFolder" forTests="false" />
- <orderEntry type="library" name="shelljs node_modules" level="project" />
- </component>
-</module>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/vcs.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/vcs.xml b/node_modules/shelljs/.idea/vcs.xml
deleted file mode 100644
index 94a25f7..0000000
--- a/node_modules/shelljs/.idea/vcs.xml
+++ /dev/null
@@ -1,6 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<project version="4">
- <component name="VcsDirectoryMappings">
- <mapping directory="$PROJECT_DIR$" vcs="Git" />
- </component>
-</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.idea/workspace.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/workspace.xml b/node_modules/shelljs/.idea/workspace.xml
deleted file mode 100644
index 9247ca4..0000000
--- a/node_modules/shelljs/.idea/workspace.xml
+++ /dev/null
@@ -1,764 +0,0 @@
-<?xml version="1.0" encoding="UTF-8"?>
-<project version="4">
- <component name="ChangeListManager">
- <list default="true" id="45933638-88e3-4f2a-b91c-9922c650f3b5" name="Default" comment="" />
- <ignored path="shelljs.iws" />
- <ignored path=".idea/workspace.xml" />
- <option name="EXCLUDED_CONVERTED_TO_IGNORED" value="true" />
- <option name="TRACKING_ENABLED" value="true" />
- <option name="SHOW_DIALOG" value="false" />
- <option name="HIGHLIGHT_CONFLICTS" value="true" />
- <option name="HIGHLIGHT_NON_ACTIVE_CHANGELIST" value="false" />
- <option name="LAST_RESOLUTION" value="IGNORE" />
- </component>
- <component name="ChangesViewManager" flattened_view="true" show_ignored="false" />
- <component name="CreatePatchCommitExecutor">
- <option name="PATCH_PATH" value="" />
- </component>
- <component name="ExecutionTargetManager" SELECTED_TARGET="default_target" />
- <component name="FavoritesManager">
- <favorites_list name="shelljs" />
- </component>
- <component name="FileEditorManager">
- <leaf SIDE_TABS_SIZE_LIMIT_KEY="300">
- <file leaf-file-name="common.js" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/src/common.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="104" column="7" selection-start-line="104" selection-start-column="7" selection-end-line="104" selection-end-column="7" />
- <folding>
- <marker date="1454630861000" expanded="true" signature="1550:1605" placeholder="//..." />
- <marker date="1454630861000" expanded="true" signature="6173:6214" placeholder="//..." />
- <marker date="1454630861000" expanded="true" signature="6173:6520" placeholder="{...}" />
- <marker date="1454630861000" expanded="true" signature="6173:6891" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name="RELEASE.md" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/RELEASE.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="-0.0">
- <caret line="0" column="15" selection-start-line="0" selection-start-column="15" selection-end-line="0" selection-end-column="15" />
- <folding />
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name="package.json" pinned="false" current-in-tab="true">
- <entry file="file://$PROJECT_DIR$/package.json">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.7058824">
- <caret line="40" column="0" selection-start-line="40" selection-start-column="0" selection-end-line="40" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name=".npmignore" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/.npmignore">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="9" column="0" selection-start-line="9" selection-start-column="0" selection-end-line="9" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name="README.md" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/README.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="-10.928572">
- <caret line="72" column="15" selection-start-line="72" selection-start-column="15" selection-end-line="72" selection-end-column="15" />
- <folding />
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name="shjs" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/bin/shjs">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name="output.js" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/build/output.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- </file>
- <file leaf-file-name="cat.js" pinned="false" current-in-tab="false">
- <entry file="file://$PROJECT_DIR$/src/cat.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="38" column="0" selection-start-line="38" selection-start-column="0" selection-end-line="38" selection-end-column="0" />
- <folding>
- <marker date="1454628318000" expanded="true" signature="59:506" placeholder="//..." />
- <marker date="1454628318000" expanded="true" signature="537:958" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- </file>
- </leaf>
- </component>
- <component name="Git.Settings">
- <option name="RECENT_GIT_ROOT_PATH" value="$PROJECT_DIR$" />
- </component>
- <component name="IdeDocumentHistory">
- <option name="CHANGED_PATHS">
- <list>
- <option value="$PROJECT_DIR$/.eslintrc" />
- <option value="$PROJECT_DIR$/CONTRIBUTING.md" />
- <option value="$PROJECT_DIR$/scripts/run-tests.js" />
- <option value="$PROJECT_DIR$/src/rm.js" />
- <option value="$PROJECT_DIR$/test/resources/issue44/main.js" />
- <option value="$PROJECT_DIR$/test/ln.js" />
- <option value="$PROJECT_DIR$/test/common.js" />
- <option value="$PROJECT_DIR$/src/ln.js" />
- <option value="$PROJECT_DIR$/src/ls.js" />
- <option value="$PROJECT_DIR$/test/which.js" />
- <option value="$PROJECT_DIR$/.eslintrc.json" />
- <option value="$PROJECT_DIR$/src/cat.js" />
- <option value="$PROJECT_DIR$/src/common.js" />
- <option value="$PROJECT_DIR$/test/TODO" />
- <option value="$PROJECT_DIR$/CHANGELOG.md" />
- <option value="$PROJECT_DIR$/maked.js" />
- <option value="$PROJECT_DIR$/docs/my_docs.md" />
- <option value="$PROJECT_DIR$/.npmignore" />
- <option value="$PROJECT_DIR$/m.js" />
- <option value="$PROJECT_DIR$/README.md" />
- <option value="$PROJECT_DIR$/package.json" />
- </list>
- </option>
- </component>
- <component name="JsBuildToolGruntFileManager" detection-done="true" />
- <component name="JsBuildToolPackageJson" detection-done="true">
- <package-json value="$PROJECT_DIR$/package.json" />
- </component>
- <component name="JsGulpfileManager">
- <detection-done>true</detection-done>
- </component>
- <component name="ProjectFrameBounds">
- <option name="width" value="1920" />
- <option name="height" value="1200" />
- </component>
- <component name="ProjectLevelVcsManager" settingsEditedManually="true">
- <OptionsSetting value="true" id="Add" />
- <OptionsSetting value="true" id="Remove" />
- <OptionsSetting value="true" id="Checkout" />
- <OptionsSetting value="true" id="Update" />
- <OptionsSetting value="true" id="Status" />
- <OptionsSetting value="true" id="Edit" />
- <ConfirmationsSetting value="0" id="Add" />
- <ConfirmationsSetting value="0" id="Remove" />
- </component>
- <component name="ProjectView">
- <navigator currentView="ProjectPane" proportions="" version="1">
- <flattenPackages />
- <showMembers />
- <showModules />
- <showLibraryContents />
- <hideEmptyPackages />
- <abbreviatePackageNames />
- <autoscrollToSource />
- <autoscrollFromSource />
- <sortByType />
- <manualOrder />
- <foldersAlwaysOnTop value="true" />
- </navigator>
- <panes>
- <pane id="ProjectPane">
- <subPane>
- <PATH>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
- </PATH_ELEMENT>
- </PATH>
- <PATH>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
- </PATH_ELEMENT>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
- </PATH_ELEMENT>
- </PATH>
- <PATH>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
- </PATH_ELEMENT>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
- </PATH_ELEMENT>
- <PATH_ELEMENT>
- <option name="myItemId" value="build" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
- </PATH_ELEMENT>
- </PATH>
- <PATH>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.ProjectViewProjectNode" />
- </PATH_ELEMENT>
- <PATH_ELEMENT>
- <option name="myItemId" value="shelljs" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
- </PATH_ELEMENT>
- <PATH_ELEMENT>
- <option name="myItemId" value="bin" />
- <option name="myItemType" value="com.intellij.ide.projectView.impl.nodes.PsiDirectoryNode" />
- </PATH_ELEMENT>
- </PATH>
- </subPane>
- </pane>
- <pane id="Scratches" />
- <pane id="Scope" />
- </panes>
- </component>
- <component name="PropertiesComponent">
- <property name="settings.editor.selected.configurable" value="Markdown" />
- <property name="settings.editor.splitter.proportion" value="0.2" />
- <property name="last_opened_file_path" value="$PROJECT_DIR$" />
- <property name="WebServerToolWindowFactoryState" value="false" />
- <property name="js-jscs-nodeInterpreter" value="$USER_HOME$/n/bin/node" />
- <property name="HbShouldOpenHtmlAsHb" value="" />
- <property name="jsx.switch.disabled" value="true" />
- <property name="FullScreen" value="true" />
- </component>
- <component name="RunManager">
- <configuration default="true" type="BashConfigurationType" factoryName="Bash">
- <option name="INTERPRETER_OPTIONS" value="" />
- <option name="INTERPRETER_PATH" value="/bin/bash" />
- <option name="WORKING_DIRECTORY" value="" />
- <option name="PARENT_ENVS" value="true" />
- <option name="SCRIPT_NAME" value="" />
- <option name="PARAMETERS" value="" />
- <module name="" />
- <envs />
- <method />
- </configuration>
- <configuration default="true" type="DartCommandLineRunConfigurationType" factoryName="Dart Command Line Application">
- <method />
- </configuration>
- <configuration default="true" type="DartTestRunConfigurationType" factoryName="Dart Test">
- <method />
- </configuration>
- <configuration default="true" type="JavaScriptTestRunnerKarma" factoryName="Karma" config-file="">
- <envs />
- <method />
- </configuration>
- <configuration default="true" type="JavascriptDebugType" factoryName="JavaScript Debug">
- <method />
- </configuration>
- <configuration default="true" type="NodeJSConfigurationType" factoryName="Node.js" working-dir="">
- <method />
- </configuration>
- <configuration default="true" type="cucumber.js" factoryName="Cucumber.js">
- <option name="cucumberJsArguments" value="" />
- <option name="executablePath" />
- <option name="filePath" />
- <method />
- </configuration>
- <configuration default="true" type="js.build_tools.gulp" factoryName="Gulp.js">
- <node-options />
- <gulpfile />
- <tasks />
- <arguments />
- <envs />
- <method />
- </configuration>
- <configuration default="true" type="js.build_tools.npm" factoryName="npm">
- <command value="run-script" />
- <scripts />
- <envs />
- <method />
- </configuration>
- <configuration default="true" type="mocha-javascript-test-runner" factoryName="Mocha">
- <node-options />
- <working-directory>$PROJECT_DIR$</working-directory>
- <pass-parent-env>true</pass-parent-env>
- <envs />
- <ui>bdd</ui>
- <extra-mocha-options />
- <test-kind>DIRECTORY</test-kind>
- <test-directory />
- <recursive>false</recursive>
- <method />
- </configuration>
- </component>
- <component name="ShelveChangesManager" show_recycled="false" />
- <component name="SvnConfiguration">
- <configuration />
- </component>
- <component name="TaskManager">
- <task active="true" id="Default" summary="Default task">
- <changelist id="45933638-88e3-4f2a-b91c-9922c650f3b5" name="Default" comment="" />
- <created>1453951651105</created>
- <option name="number" value="Default" />
- <updated>1453951651105</updated>
- </task>
- <servers />
- </component>
- <component name="ToolWindowManager">
- <frame x="0" y="0" width="1920" height="1200" extended-state="6" />
- <editor active="false" />
- <layout>
- <window_info id="Project" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="true" show_stripe_button="true" weight="0.15745129" sideWeight="0.5" order="0" side_tool="false" content_ui="tabs" />
- <window_info id="TODO" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="6" side_tool="false" content_ui="tabs" />
- <window_info id="Event Log" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="7" side_tool="true" content_ui="tabs" />
- <window_info id="npm" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="2" side_tool="true" content_ui="tabs" />
- <window_info id="Version Control" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="7" side_tool="false" content_ui="tabs" />
- <window_info id="Run" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="2" side_tool="false" content_ui="tabs" />
- <window_info id="Structure" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="1" side_tool="false" content_ui="tabs" />
- <window_info id="Terminal" active="true" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="true" show_stripe_button="true" weight="0.20318021" sideWeight="0.5" order="7" side_tool="false" content_ui="tabs" />
- <window_info id="Favorites" active="false" anchor="left" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="2" side_tool="true" content_ui="tabs" />
- <window_info id="Debug" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.4" sideWeight="0.5" order="3" side_tool="false" content_ui="tabs" />
- <window_info id="Cvs" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="4" side_tool="false" content_ui="tabs" />
- <window_info id="Message" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="0" side_tool="false" content_ui="tabs" />
- <window_info id="Commander" active="false" anchor="right" auto_hide="false" internal_type="SLIDING" type="SLIDING" visible="false" show_stripe_button="true" weight="0.4" sideWeight="0.5" order="0" side_tool="false" content_ui="tabs" />
- <window_info id="Inspection" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.4" sideWeight="0.5" order="5" side_tool="false" content_ui="tabs" />
- <window_info id="Hierarchy" active="false" anchor="right" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="2" side_tool="false" content_ui="combo" />
- <window_info id="Find" active="false" anchor="bottom" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.33" sideWeight="0.5" order="1" side_tool="false" content_ui="tabs" />
- <window_info id="Ant Build" active="false" anchor="right" auto_hide="false" internal_type="DOCKED" type="DOCKED" visible="false" show_stripe_button="true" weight="0.25" sideWeight="0.5" order="1" side_tool="false" content_ui="tabs" />
- </layout>
- </component>
- <component name="Vcs.Log.UiProperties">
- <option name="RECENTLY_FILTERED_USER_GROUPS">
- <collection />
- </option>
- <option name="RECENTLY_FILTERED_BRANCH_GROUPS">
- <collection />
- </option>
- </component>
- <component name="VcsContentAnnotationSettings">
- <option name="myLimit" value="2678400000" />
- </component>
- <component name="XDebuggerManager">
- <breakpoint-manager />
- <watches-manager />
- </component>
- <component name="editorHistoryManager">
- <entry file="file://$PROJECT_DIR$/src/common.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- <folding>
- <marker date="1454630861000" expanded="true" signature="1550:1605" placeholder="//..." />
- <marker date="1454630861000" expanded="true" signature="6173:6214" placeholder="//..." />
- <marker date="1454630861000" expanded="true" signature="6173:6520" placeholder="{...}" />
- <marker date="1454630861000" expanded="true" signature="6173:6891" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/shell.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/global.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/.gitignore">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="2" column="0" selection-start-line="2" selection-start-column="0" selection-end-line="2" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/which.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/RELEASE.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/package.json">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="29" column="23" selection-start-line="29" selection-start-column="23" selection-end-line="29" selection-end-column="23" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/README.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="43" column="0" selection-start-line="43" selection-start-column="0" selection-end-line="43" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/scripts/generate-docs.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/scripts/run-tests.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="26" column="50" selection-start-line="26" selection-start-column="50" selection-end-line="26" selection-end-column="50" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/shell.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/global.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/.gitignore">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="2" column="0" selection-start-line="2" selection-start-column="0" selection-end-line="2" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/which.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/RELEASE.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/package.json">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="29" column="23" selection-start-line="29" selection-start-column="23" selection-end-line="29" selection-end-column="23" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/README.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="43" column="0" selection-start-line="43" selection-start-column="0" selection-end-line="43" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/scripts/generate-docs.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/scripts/run-tests.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="26" column="50" selection-start-line="26" selection-start-column="50" selection-end-line="26" selection-end-column="50" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/shell.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/global.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/.gitignore">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/.gitignore">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="2" column="0" selection-start-line="2" selection-start-column="0" selection-end-line="2" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/scripts/generate-docs.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/sed.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="1" column="0" selection-start-line="1" selection-start-column="0" selection-end-line="1" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/grep.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/shell.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/global.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/sed.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="1" column="0" selection-start-line="1" selection-start-column="0" selection-end-line="1" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/pwd.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/scripts/run-tests.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="-0.17814727">
- <caret line="24" column="16" selection-start-line="24" selection-start-column="16" selection-end-line="24" selection-end-column="16" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/rm.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="189" column="0" selection-start-line="189" selection-start-column="0" selection-end-line="189" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/node_modules/glob/README.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="30.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/rm.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="137" column="25" selection-start-line="137" selection-start-column="25" selection-end-line="137" selection-end-column="25" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/resources/issue44/main.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="1" column="0" selection-start-line="1" selection-start-column="0" selection-end-line="1" selection-end-column="0" />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/ln.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="20" column="1" selection-start-line="20" selection-start-column="1" selection-end-line="20" selection-end-column="1" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/common.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="46" column="10" selection-start-line="46" selection-start-column="10" selection-end-line="46" selection-end-column="10" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/ls.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="15" column="18" selection-start-line="15" selection-start-column="18" selection-end-line="15" selection-end-column="18" />
- <folding>
- <marker date="1454625654000" expanded="true" signature="11066:11146" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/ln.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="48" column="9" selection-start-line="48" selection-start-column="9" selection-end-line="48" selection-end-column="9" />
- <folding>
- <marker date="1454625654000" expanded="true" signature="1476:1565" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/ls.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="168" column="0" selection-start-line="168" selection-start-column="0" selection-end-line="168" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/test/which.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="19" column="0" selection-start-line="19" selection-start-column="0" selection-end-line="19" selection-end-column="0" />
- <folding>
- <marker date="1454625654000" expanded="true" signature="711:743" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/cat.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="38" column="0" selection-start-line="38" selection-start-column="0" selection-end-line="38" selection-end-column="0" />
- <folding>
- <marker date="1454628318000" expanded="true" signature="59:506" placeholder="//..." />
- <marker date="1454628318000" expanded="true" signature="537:958" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/src/common.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="104" column="7" selection-start-line="104" selection-start-column="7" selection-end-line="104" selection-end-column="7" />
- <folding>
- <marker date="1454630861000" expanded="true" signature="1550:1605" placeholder="//..." />
- <marker date="1454630861000" expanded="true" signature="6173:6214" placeholder="//..." />
- <marker date="1454630861000" expanded="true" signature="6173:6520" placeholder="{...}" />
- <marker date="1454630861000" expanded="true" signature="6173:6891" placeholder="{...}" />
- </folding>
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/.npmignore">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="9" column="0" selection-start-line="9" selection-start-column="0" selection-end-line="9" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/RELEASE.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="-0.0">
- <caret line="0" column="15" selection-start-line="0" selection-start-column="15" selection-end-line="0" selection-end-column="15" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/bin/shjs">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/build/output.js">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.0">
- <caret line="0" column="0" selection-start-line="0" selection-start-column="0" selection-end-line="0" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/README.md">
- <provider editor-type-id="MarkdownPreviewEditor">
- <state />
- </provider>
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="-10.928572">
- <caret line="72" column="15" selection-start-line="72" selection-start-column="15" selection-end-line="72" selection-end-column="15" />
- <folding />
- </state>
- </provider>
- </entry>
- <entry file="file://$PROJECT_DIR$/package.json">
- <provider selected="true" editor-type-id="text-editor">
- <state vertical-scroll-proportion="0.7058824">
- <caret line="40" column="0" selection-start-line="40" selection-start-column="0" selection-end-line="40" selection-end-column="0" />
- <folding />
- </state>
- </provider>
- </entry>
- </component>
-</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.npmignore b/node_modules/shelljs/.npmignore
index 8b693ff..6b20c38 100644
--- a/node_modules/shelljs/.npmignore
+++ b/node_modules/shelljs/.npmignore
@@ -1,9 +1,2 @@
test/
-tmp/
-.documentup.json
-.gitignore
-.jshintrc
-.lgtm
-.travis.yml
-appveyor.yml
-RELEASE.md
+tmp/
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/LICENSE b/node_modules/shelljs/LICENSE
index 0f0f119..1b35ee9 100644
--- a/node_modules/shelljs/LICENSE
+++ b/node_modules/shelljs/LICENSE
@@ -1,4 +1,4 @@
-Copyright (c) 2012, Artur Adib <ar...@gmail.com>
+Copyright (c) 2012, Artur Adib <aa...@mozilla.com>
All rights reserved.
You may use this project under the terms of the New BSD license as follows:
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/MAINTAINERS
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/MAINTAINERS b/node_modules/shelljs/MAINTAINERS
deleted file mode 100644
index 3f94761..0000000
--- a/node_modules/shelljs/MAINTAINERS
+++ /dev/null
@@ -1,3 +0,0 @@
-Ari Porad <ar...@ariporad.com> (@ariporad)
-Nate Fischer <nt...@gmail.com> (@nfischer)
-Artur Adib <ar...@gmail.com> (@arturadib)
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[10/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/big-not-pretty.xml
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/big-not-pretty.xml b/node_modules/sax/examples/big-not-pretty.xml
new file mode 100644
index 0000000..fb5265d
--- /dev/null
+++ b/node_modules/sax/examples/big-not-pretty.xml
@@ -0,0 +1,8002 @@
+<big>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
+<root>
+ something<else> blerm <slurm
+
+
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[28/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/sortByOrder.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/sortByOrder.js b/node_modules/lodash/collection/sortByOrder.js
new file mode 100644
index 0000000..8b4fc19
--- /dev/null
+++ b/node_modules/lodash/collection/sortByOrder.js
@@ -0,0 +1,55 @@
+var baseSortByOrder = require('../internal/baseSortByOrder'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * This method is like `_.sortByAll` except that it allows specifying the
+ * sort orders of the iteratees to sort by. If `orders` is unspecified, all
+ * values are sorted in ascending order. Otherwise, a value is sorted in
+ * ascending order if its corresponding order is "asc", and descending if "desc".
+ *
+ * If a property name is provided for an iteratee the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If an object is provided for an iteratee the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function[]|Object[]|string[]} iteratees The iteratees to sort by.
+ * @param {boolean[]} [orders] The sort orders of `iteratees`.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.reduce`.
+ * @returns {Array} Returns the new sorted array.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'fred', 'age': 48 },
+ * { 'user': 'barney', 'age': 34 },
+ * { 'user': 'fred', 'age': 42 },
+ * { 'user': 'barney', 'age': 36 }
+ * ];
+ *
+ * // sort by `user` in ascending order and by `age` in descending order
+ * _.map(_.sortByOrder(users, ['user', 'age'], ['asc', 'desc']), _.values);
+ * // => [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 42]]
+ */
+function sortByOrder(collection, iteratees, orders, guard) {
+ if (collection == null) {
+ return [];
+ }
+ if (guard && isIterateeCall(iteratees, orders, guard)) {
+ orders = undefined;
+ }
+ if (!isArray(iteratees)) {
+ iteratees = iteratees == null ? [] : [iteratees];
+ }
+ if (!isArray(orders)) {
+ orders = orders == null ? [] : [orders];
+ }
+ return baseSortByOrder(collection, iteratees, orders);
+}
+
+module.exports = sortByOrder;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/sum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/sum.js b/node_modules/lodash/collection/sum.js
new file mode 100644
index 0000000..a2e9380
--- /dev/null
+++ b/node_modules/lodash/collection/sum.js
@@ -0,0 +1 @@
+module.exports = require('../math/sum');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/where.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/where.js b/node_modules/lodash/collection/where.js
new file mode 100644
index 0000000..f603bf8
--- /dev/null
+++ b/node_modules/lodash/collection/where.js
@@ -0,0 +1,37 @@
+var baseMatches = require('../internal/baseMatches'),
+ filter = require('./filter');
+
+/**
+ * Performs a deep comparison between each element in `collection` and the
+ * source object, returning an array of all elements that have equivalent
+ * property values.
+ *
+ * **Note:** This method supports comparing arrays, booleans, `Date` objects,
+ * numbers, `Object` objects, regexes, and strings. Objects are compared by
+ * their own, not inherited, enumerable properties. For comparing a single
+ * own or inherited property value see `_.matchesProperty`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {Object} source The object of property values to match.
+ * @returns {Array} Returns the new filtered array.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false, 'pets': ['hoppy'] },
+ * { 'user': 'fred', 'age': 40, 'active': true, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * _.pluck(_.where(users, { 'age': 36, 'active': false }), 'user');
+ * // => ['barney']
+ *
+ * _.pluck(_.where(users, { 'pets': ['dino'] }), 'user');
+ * // => ['fred']
+ */
+function where(collection, source) {
+ return filter(collection, baseMatches(source));
+}
+
+module.exports = where;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/date.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/date.js b/node_modules/lodash/date.js
new file mode 100644
index 0000000..195366e
--- /dev/null
+++ b/node_modules/lodash/date.js
@@ -0,0 +1,3 @@
+module.exports = {
+ 'now': require('./date/now')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/date/now.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/date/now.js b/node_modules/lodash/date/now.js
new file mode 100644
index 0000000..ffe3060
--- /dev/null
+++ b/node_modules/lodash/date/now.js
@@ -0,0 +1,24 @@
+var getNative = require('../internal/getNative');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeNow = getNative(Date, 'now');
+
+/**
+ * Gets the number of milliseconds that have elapsed since the Unix epoch
+ * (1 January 1970 00:00:00 UTC).
+ *
+ * @static
+ * @memberOf _
+ * @category Date
+ * @example
+ *
+ * _.defer(function(stamp) {
+ * console.log(_.now() - stamp);
+ * }, _.now());
+ * // => logs the number of milliseconds it took for the deferred function to be invoked
+ */
+var now = nativeNow || function() {
+ return new Date().getTime();
+};
+
+module.exports = now;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function.js b/node_modules/lodash/function.js
new file mode 100644
index 0000000..71f8ebe
--- /dev/null
+++ b/node_modules/lodash/function.js
@@ -0,0 +1,28 @@
+module.exports = {
+ 'after': require('./function/after'),
+ 'ary': require('./function/ary'),
+ 'backflow': require('./function/backflow'),
+ 'before': require('./function/before'),
+ 'bind': require('./function/bind'),
+ 'bindAll': require('./function/bindAll'),
+ 'bindKey': require('./function/bindKey'),
+ 'compose': require('./function/compose'),
+ 'curry': require('./function/curry'),
+ 'curryRight': require('./function/curryRight'),
+ 'debounce': require('./function/debounce'),
+ 'defer': require('./function/defer'),
+ 'delay': require('./function/delay'),
+ 'flow': require('./function/flow'),
+ 'flowRight': require('./function/flowRight'),
+ 'memoize': require('./function/memoize'),
+ 'modArgs': require('./function/modArgs'),
+ 'negate': require('./function/negate'),
+ 'once': require('./function/once'),
+ 'partial': require('./function/partial'),
+ 'partialRight': require('./function/partialRight'),
+ 'rearg': require('./function/rearg'),
+ 'restParam': require('./function/restParam'),
+ 'spread': require('./function/spread'),
+ 'throttle': require('./function/throttle'),
+ 'wrap': require('./function/wrap')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/after.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/after.js b/node_modules/lodash/function/after.js
new file mode 100644
index 0000000..96a51fd
--- /dev/null
+++ b/node_modules/lodash/function/after.js
@@ -0,0 +1,48 @@
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeIsFinite = global.isFinite;
+
+/**
+ * The opposite of `_.before`; this method creates a function that invokes
+ * `func` once it's called `n` or more times.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {number} n The number of calls before `func` is invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var saves = ['profile', 'settings'];
+ *
+ * var done = _.after(saves.length, function() {
+ * console.log('done saving!');
+ * });
+ *
+ * _.forEach(saves, function(type) {
+ * asyncSave({ 'type': type, 'complete': done });
+ * });
+ * // => logs 'done saving!' after the two async saves have completed
+ */
+function after(n, func) {
+ if (typeof func != 'function') {
+ if (typeof n == 'function') {
+ var temp = n;
+ n = func;
+ func = temp;
+ } else {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ }
+ n = nativeIsFinite(n = +n) ? n : 0;
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+}
+
+module.exports = after;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/ary.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/ary.js b/node_modules/lodash/function/ary.js
new file mode 100644
index 0000000..53a6913
--- /dev/null
+++ b/node_modules/lodash/function/ary.js
@@ -0,0 +1,34 @@
+var createWrapper = require('../internal/createWrapper'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var ARY_FLAG = 128;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that accepts up to `n` arguments ignoring any
+ * additional arguments.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to cap arguments for.
+ * @param {number} [n=func.length] The arity cap.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * _.map(['6', '8', '10'], _.ary(parseInt, 1));
+ * // => [6, 8, 10]
+ */
+function ary(func, n, guard) {
+ if (guard && isIterateeCall(func, n, guard)) {
+ n = undefined;
+ }
+ n = (func && n == null) ? func.length : nativeMax(+n || 0, 0);
+ return createWrapper(func, ARY_FLAG, undefined, undefined, undefined, undefined, n);
+}
+
+module.exports = ary;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/backflow.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/backflow.js b/node_modules/lodash/function/backflow.js
new file mode 100644
index 0000000..1954e94
--- /dev/null
+++ b/node_modules/lodash/function/backflow.js
@@ -0,0 +1 @@
+module.exports = require('./flowRight');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/before.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/before.js b/node_modules/lodash/function/before.js
new file mode 100644
index 0000000..3d94216
--- /dev/null
+++ b/node_modules/lodash/function/before.js
@@ -0,0 +1,42 @@
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a function that invokes `func`, with the `this` binding and arguments
+ * of the created function, while it's called less than `n` times. Subsequent
+ * calls to the created function return the result of the last `func` invocation.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {number} n The number of calls at which `func` is no longer invoked.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * jQuery('#add').on('click', _.before(5, addContactToList));
+ * // => allows adding up to 4 contacts to the list
+ */
+function before(n, func) {
+ var result;
+ if (typeof func != 'function') {
+ if (typeof n == 'function') {
+ var temp = n;
+ n = func;
+ func = temp;
+ } else {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ }
+ return function() {
+ if (--n > 0) {
+ result = func.apply(this, arguments);
+ }
+ if (n <= 1) {
+ func = undefined;
+ }
+ return result;
+ };
+}
+
+module.exports = before;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/bind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/bind.js b/node_modules/lodash/function/bind.js
new file mode 100644
index 0000000..0de126a
--- /dev/null
+++ b/node_modules/lodash/function/bind.js
@@ -0,0 +1,56 @@
+var createWrapper = require('../internal/createWrapper'),
+ replaceHolders = require('../internal/replaceHolders'),
+ restParam = require('./restParam');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1,
+ PARTIAL_FLAG = 32;
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of `thisArg`
+ * and prepends any additional `_.bind` arguments to those provided to the
+ * bound function.
+ *
+ * The `_.bind.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** Unlike native `Function#bind` this method does not set the "length"
+ * property of bound functions.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var greet = function(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * };
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * var bound = _.bind(greet, object, 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * // using placeholders
+ * var bound = _.bind(greet, object, _, '!');
+ * bound('hi');
+ * // => 'hi fred!'
+ */
+var bind = restParam(function(func, thisArg, partials) {
+ var bitmask = BIND_FLAG;
+ if (partials.length) {
+ var holders = replaceHolders(partials, bind.placeholder);
+ bitmask |= PARTIAL_FLAG;
+ }
+ return createWrapper(func, bitmask, thisArg, partials, holders);
+});
+
+// Assign default placeholders.
+bind.placeholder = {};
+
+module.exports = bind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/bindAll.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/bindAll.js b/node_modules/lodash/function/bindAll.js
new file mode 100644
index 0000000..a09e948
--- /dev/null
+++ b/node_modules/lodash/function/bindAll.js
@@ -0,0 +1,50 @@
+var baseFlatten = require('../internal/baseFlatten'),
+ createWrapper = require('../internal/createWrapper'),
+ functions = require('../object/functions'),
+ restParam = require('./restParam');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1;
+
+/**
+ * Binds methods of an object to the object itself, overwriting the existing
+ * method. Method names may be specified as individual arguments or as arrays
+ * of method names. If no method names are provided all enumerable function
+ * properties, own and inherited, of `object` are bound.
+ *
+ * **Note:** This method does not set the "length" property of bound functions.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Object} object The object to bind and assign the bound methods to.
+ * @param {...(string|string[])} [methodNames] The object method names to bind,
+ * specified as individual method names or arrays of method names.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'onClick': function() {
+ * console.log('clicked ' + this.label);
+ * }
+ * };
+ *
+ * _.bindAll(view);
+ * jQuery('#docs').on('click', view.onClick);
+ * // => logs 'clicked docs' when the element is clicked
+ */
+var bindAll = restParam(function(object, methodNames) {
+ methodNames = methodNames.length ? baseFlatten(methodNames) : functions(object);
+
+ var index = -1,
+ length = methodNames.length;
+
+ while (++index < length) {
+ var key = methodNames[index];
+ object[key] = createWrapper(object[key], BIND_FLAG, object);
+ }
+ return object;
+});
+
+module.exports = bindAll;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/bindKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/bindKey.js b/node_modules/lodash/function/bindKey.js
new file mode 100644
index 0000000..b787fe7
--- /dev/null
+++ b/node_modules/lodash/function/bindKey.js
@@ -0,0 +1,66 @@
+var createWrapper = require('../internal/createWrapper'),
+ replaceHolders = require('../internal/replaceHolders'),
+ restParam = require('./restParam');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1,
+ BIND_KEY_FLAG = 2,
+ PARTIAL_FLAG = 32;
+
+/**
+ * Creates a function that invokes the method at `object[key]` and prepends
+ * any additional `_.bindKey` arguments to those provided to the bound function.
+ *
+ * This method differs from `_.bind` by allowing bound functions to reference
+ * methods that may be redefined or don't yet exist.
+ * See [Peter Michaux's article](http://peter.michaux.ca/articles/lazy-function-definition-pattern)
+ * for more details.
+ *
+ * The `_.bindKey.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Object} object The object the method belongs to.
+ * @param {string} key The key of the method.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var object = {
+ * 'user': 'fred',
+ * 'greet': function(greeting, punctuation) {
+ * return greeting + ' ' + this.user + punctuation;
+ * }
+ * };
+ *
+ * var bound = _.bindKey(object, 'greet', 'hi');
+ * bound('!');
+ * // => 'hi fred!'
+ *
+ * object.greet = function(greeting, punctuation) {
+ * return greeting + 'ya ' + this.user + punctuation;
+ * };
+ *
+ * bound('!');
+ * // => 'hiya fred!'
+ *
+ * // using placeholders
+ * var bound = _.bindKey(object, 'greet', _, '!');
+ * bound('hi');
+ * // => 'hiya fred!'
+ */
+var bindKey = restParam(function(object, key, partials) {
+ var bitmask = BIND_FLAG | BIND_KEY_FLAG;
+ if (partials.length) {
+ var holders = replaceHolders(partials, bindKey.placeholder);
+ bitmask |= PARTIAL_FLAG;
+ }
+ return createWrapper(key, bitmask, object, partials, holders);
+});
+
+// Assign default placeholders.
+bindKey.placeholder = {};
+
+module.exports = bindKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/compose.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/compose.js b/node_modules/lodash/function/compose.js
new file mode 100644
index 0000000..1954e94
--- /dev/null
+++ b/node_modules/lodash/function/compose.js
@@ -0,0 +1 @@
+module.exports = require('./flowRight');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/curry.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/curry.js b/node_modules/lodash/function/curry.js
new file mode 100644
index 0000000..b7db3fd
--- /dev/null
+++ b/node_modules/lodash/function/curry.js
@@ -0,0 +1,51 @@
+var createCurry = require('../internal/createCurry');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var CURRY_FLAG = 8;
+
+/**
+ * Creates a function that accepts one or more arguments of `func` that when
+ * called either invokes `func` returning its result, if all `func` arguments
+ * have been provided, or returns a function that accepts one or more of the
+ * remaining `func` arguments, and so on. The arity of `func` may be specified
+ * if `func.length` is not sufficient.
+ *
+ * The `_.curry.placeholder` value, which defaults to `_` in monolithic builds,
+ * may be used as a placeholder for provided arguments.
+ *
+ * **Note:** This method does not set the "length" property of curried functions.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var abc = function(a, b, c) {
+ * return [a, b, c];
+ * };
+ *
+ * var curried = _.curry(abc);
+ *
+ * curried(1)(2)(3);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2)(3);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2, 3);
+ * // => [1, 2, 3]
+ *
+ * // using placeholders
+ * curried(1)(_, 3)(2);
+ * // => [1, 2, 3]
+ */
+var curry = createCurry(CURRY_FLAG);
+
+// Assign default placeholders.
+curry.placeholder = {};
+
+module.exports = curry;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/curryRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/curryRight.js b/node_modules/lodash/function/curryRight.js
new file mode 100644
index 0000000..11c5403
--- /dev/null
+++ b/node_modules/lodash/function/curryRight.js
@@ -0,0 +1,48 @@
+var createCurry = require('../internal/createCurry');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var CURRY_RIGHT_FLAG = 16;
+
+/**
+ * This method is like `_.curry` except that arguments are applied to `func`
+ * in the manner of `_.partialRight` instead of `_.partial`.
+ *
+ * The `_.curryRight.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for provided arguments.
+ *
+ * **Note:** This method does not set the "length" property of curried functions.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var abc = function(a, b, c) {
+ * return [a, b, c];
+ * };
+ *
+ * var curried = _.curryRight(abc);
+ *
+ * curried(3)(2)(1);
+ * // => [1, 2, 3]
+ *
+ * curried(2, 3)(1);
+ * // => [1, 2, 3]
+ *
+ * curried(1, 2, 3);
+ * // => [1, 2, 3]
+ *
+ * // using placeholders
+ * curried(3)(1, _)(2);
+ * // => [1, 2, 3]
+ */
+var curryRight = createCurry(CURRY_RIGHT_FLAG);
+
+// Assign default placeholders.
+curryRight.placeholder = {};
+
+module.exports = curryRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/debounce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/debounce.js b/node_modules/lodash/function/debounce.js
new file mode 100644
index 0000000..163af90
--- /dev/null
+++ b/node_modules/lodash/function/debounce.js
@@ -0,0 +1,181 @@
+var isObject = require('../lang/isObject'),
+ now = require('../date/now');
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a debounced function that delays invoking `func` until after `wait`
+ * milliseconds have elapsed since the last time the debounced function was
+ * invoked. The debounced function comes with a `cancel` method to cancel
+ * delayed invocations. Provide an options object to indicate that `func`
+ * should be invoked on the leading and/or trailing edge of the `wait` timeout.
+ * Subsequent calls to the debounced function return the result of the last
+ * `func` invocation.
+ *
+ * **Note:** If `leading` and `trailing` options are `true`, `func` is invoked
+ * on the trailing edge of the timeout only if the the debounced function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * See [David Corbacho's article](http://drupalmotion.com/article/debounce-and-throttle-visual-explanation)
+ * for details over the differences between `_.debounce` and `_.throttle`.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to debounce.
+ * @param {number} [wait=0] The number of milliseconds to delay.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=false] Specify invoking on the leading
+ * edge of the timeout.
+ * @param {number} [options.maxWait] The maximum time `func` is allowed to be
+ * delayed before it's invoked.
+ * @param {boolean} [options.trailing=true] Specify invoking on the trailing
+ * edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * // avoid costly calculations while the window size is in flux
+ * jQuery(window).on('resize', _.debounce(calculateLayout, 150));
+ *
+ * // invoke `sendMail` when the click event is fired, debouncing subsequent calls
+ * jQuery('#postbox').on('click', _.debounce(sendMail, 300, {
+ * 'leading': true,
+ * 'trailing': false
+ * }));
+ *
+ * // ensure `batchLog` is invoked once after 1 second of debounced calls
+ * var source = new EventSource('/stream');
+ * jQuery(source).on('message', _.debounce(batchLog, 250, {
+ * 'maxWait': 1000
+ * }));
+ *
+ * // cancel a debounced call
+ * var todoChanges = _.debounce(batchLog, 1000);
+ * Object.observe(models.todo, todoChanges);
+ *
+ * Object.observe(models, function(changes) {
+ * if (_.find(changes, { 'user': 'todo', 'type': 'delete'})) {
+ * todoChanges.cancel();
+ * }
+ * }, ['delete']);
+ *
+ * // ...at some point `models.todo` is changed
+ * models.todo.completed = true;
+ *
+ * // ...before 1 second has passed `models.todo` is deleted
+ * // which cancels the debounced `todoChanges` call
+ * delete models.todo;
+ */
+function debounce(func, wait, options) {
+ var args,
+ maxTimeoutId,
+ result,
+ stamp,
+ thisArg,
+ timeoutId,
+ trailingCall,
+ lastCalled = 0,
+ maxWait = false,
+ trailing = true;
+
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ wait = wait < 0 ? 0 : (+wait || 0);
+ if (options === true) {
+ var leading = true;
+ trailing = false;
+ } else if (isObject(options)) {
+ leading = !!options.leading;
+ maxWait = 'maxWait' in options && nativeMax(+options.maxWait || 0, wait);
+ trailing = 'trailing' in options ? !!options.trailing : trailing;
+ }
+
+ function cancel() {
+ if (timeoutId) {
+ clearTimeout(timeoutId);
+ }
+ if (maxTimeoutId) {
+ clearTimeout(maxTimeoutId);
+ }
+ lastCalled = 0;
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ }
+
+ function complete(isCalled, id) {
+ if (id) {
+ clearTimeout(id);
+ }
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (isCalled) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = undefined;
+ }
+ }
+ }
+
+ function delayed() {
+ var remaining = wait - (now() - stamp);
+ if (remaining <= 0 || remaining > wait) {
+ complete(trailingCall, maxTimeoutId);
+ } else {
+ timeoutId = setTimeout(delayed, remaining);
+ }
+ }
+
+ function maxDelayed() {
+ complete(trailing, timeoutId);
+ }
+
+ function debounced() {
+ args = arguments;
+ stamp = now();
+ thisArg = this;
+ trailingCall = trailing && (timeoutId || !leading);
+
+ if (maxWait === false) {
+ var leadingCall = leading && !timeoutId;
+ } else {
+ if (!maxTimeoutId && !leading) {
+ lastCalled = stamp;
+ }
+ var remaining = maxWait - (stamp - lastCalled),
+ isCalled = remaining <= 0 || remaining > maxWait;
+
+ if (isCalled) {
+ if (maxTimeoutId) {
+ maxTimeoutId = clearTimeout(maxTimeoutId);
+ }
+ lastCalled = stamp;
+ result = func.apply(thisArg, args);
+ }
+ else if (!maxTimeoutId) {
+ maxTimeoutId = setTimeout(maxDelayed, remaining);
+ }
+ }
+ if (isCalled && timeoutId) {
+ timeoutId = clearTimeout(timeoutId);
+ }
+ else if (!timeoutId && wait !== maxWait) {
+ timeoutId = setTimeout(delayed, wait);
+ }
+ if (leadingCall) {
+ isCalled = true;
+ result = func.apply(thisArg, args);
+ }
+ if (isCalled && !timeoutId && !maxTimeoutId) {
+ args = thisArg = undefined;
+ }
+ return result;
+ }
+ debounced.cancel = cancel;
+ return debounced;
+}
+
+module.exports = debounce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/defer.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/defer.js b/node_modules/lodash/function/defer.js
new file mode 100644
index 0000000..3accbf9
--- /dev/null
+++ b/node_modules/lodash/function/defer.js
@@ -0,0 +1,25 @@
+var baseDelay = require('../internal/baseDelay'),
+ restParam = require('./restParam');
+
+/**
+ * Defers invoking the `func` until the current call stack has cleared. Any
+ * additional arguments are provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to defer.
+ * @param {...*} [args] The arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) {
+ * console.log(text);
+ * }, 'deferred');
+ * // logs 'deferred' after one or more milliseconds
+ */
+var defer = restParam(function(func, args) {
+ return baseDelay(func, 1, args);
+});
+
+module.exports = defer;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/delay.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/delay.js b/node_modules/lodash/function/delay.js
new file mode 100644
index 0000000..d5eef27
--- /dev/null
+++ b/node_modules/lodash/function/delay.js
@@ -0,0 +1,26 @@
+var baseDelay = require('../internal/baseDelay'),
+ restParam = require('./restParam');
+
+/**
+ * Invokes `func` after `wait` milliseconds. Any additional arguments are
+ * provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {...*} [args] The arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) {
+ * console.log(text);
+ * }, 1000, 'later');
+ * // => logs 'later' after one second
+ */
+var delay = restParam(function(func, wait, args) {
+ return baseDelay(func, wait, args);
+});
+
+module.exports = delay;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/flow.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/flow.js b/node_modules/lodash/function/flow.js
new file mode 100644
index 0000000..a435a3d
--- /dev/null
+++ b/node_modules/lodash/function/flow.js
@@ -0,0 +1,25 @@
+var createFlow = require('../internal/createFlow');
+
+/**
+ * Creates a function that returns the result of invoking the provided
+ * functions with the `this` binding of the created function, where each
+ * successive invocation is supplied the return value of the previous.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {...Function} [funcs] Functions to invoke.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var addSquare = _.flow(_.add, square);
+ * addSquare(1, 2);
+ * // => 9
+ */
+var flow = createFlow();
+
+module.exports = flow;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/flowRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/flowRight.js b/node_modules/lodash/function/flowRight.js
new file mode 100644
index 0000000..23b9d76
--- /dev/null
+++ b/node_modules/lodash/function/flowRight.js
@@ -0,0 +1,25 @@
+var createFlow = require('../internal/createFlow');
+
+/**
+ * This method is like `_.flow` except that it creates a function that
+ * invokes the provided functions from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias backflow, compose
+ * @category Function
+ * @param {...Function} [funcs] Functions to invoke.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var addSquare = _.flowRight(square, _.add);
+ * addSquare(1, 2);
+ * // => 9
+ */
+var flowRight = createFlow(true);
+
+module.exports = flowRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/memoize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/memoize.js b/node_modules/lodash/function/memoize.js
new file mode 100644
index 0000000..f3b8d69
--- /dev/null
+++ b/node_modules/lodash/function/memoize.js
@@ -0,0 +1,80 @@
+var MapCache = require('../internal/MapCache');
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a function that memoizes the result of `func`. If `resolver` is
+ * provided it determines the cache key for storing the result based on the
+ * arguments provided to the memoized function. By default, the first argument
+ * provided to the memoized function is coerced to a string and used as the
+ * cache key. The `func` is invoked with the `this` binding of the memoized
+ * function.
+ *
+ * **Note:** The cache is exposed as the `cache` property on the memoized
+ * function. Its creation may be customized by replacing the `_.memoize.Cache`
+ * constructor with one whose instances implement the [`Map`](http://ecma-international.org/ecma-262/6.0/#sec-properties-of-the-map-prototype-object)
+ * method interface of `get`, `has`, and `set`.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to have its output memoized.
+ * @param {Function} [resolver] The function to resolve the cache key.
+ * @returns {Function} Returns the new memoizing function.
+ * @example
+ *
+ * var upperCase = _.memoize(function(string) {
+ * return string.toUpperCase();
+ * });
+ *
+ * upperCase('fred');
+ * // => 'FRED'
+ *
+ * // modifying the result cache
+ * upperCase.cache.set('fred', 'BARNEY');
+ * upperCase('fred');
+ * // => 'BARNEY'
+ *
+ * // replacing `_.memoize.Cache`
+ * var object = { 'user': 'fred' };
+ * var other = { 'user': 'barney' };
+ * var identity = _.memoize(_.identity);
+ *
+ * identity(object);
+ * // => { 'user': 'fred' }
+ * identity(other);
+ * // => { 'user': 'fred' }
+ *
+ * _.memoize.Cache = WeakMap;
+ * var identity = _.memoize(_.identity);
+ *
+ * identity(object);
+ * // => { 'user': 'fred' }
+ * identity(other);
+ * // => { 'user': 'barney' }
+ */
+function memoize(func, resolver) {
+ if (typeof func != 'function' || (resolver && typeof resolver != 'function')) {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var memoized = function() {
+ var args = arguments,
+ key = resolver ? resolver.apply(this, args) : args[0],
+ cache = memoized.cache;
+
+ if (cache.has(key)) {
+ return cache.get(key);
+ }
+ var result = func.apply(this, args);
+ memoized.cache = cache.set(key, result);
+ return result;
+ };
+ memoized.cache = new memoize.Cache;
+ return memoized;
+}
+
+// Assign cache to `_.memoize`.
+memoize.Cache = MapCache;
+
+module.exports = memoize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/modArgs.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/modArgs.js b/node_modules/lodash/function/modArgs.js
new file mode 100644
index 0000000..49b9b5e
--- /dev/null
+++ b/node_modules/lodash/function/modArgs.js
@@ -0,0 +1,58 @@
+var arrayEvery = require('../internal/arrayEvery'),
+ baseFlatten = require('../internal/baseFlatten'),
+ baseIsFunction = require('../internal/baseIsFunction'),
+ restParam = require('./restParam');
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Creates a function that runs each argument through a corresponding
+ * transform function.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to wrap.
+ * @param {...(Function|Function[])} [transforms] The functions to transform
+ * arguments, specified as individual functions or arrays of functions.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * function doubled(n) {
+ * return n * 2;
+ * }
+ *
+ * function square(n) {
+ * return n * n;
+ * }
+ *
+ * var modded = _.modArgs(function(x, y) {
+ * return [x, y];
+ * }, square, doubled);
+ *
+ * modded(1, 2);
+ * // => [1, 4]
+ *
+ * modded(5, 10);
+ * // => [25, 20]
+ */
+var modArgs = restParam(function(func, transforms) {
+ transforms = baseFlatten(transforms);
+ if (typeof func != 'function' || !arrayEvery(transforms, baseIsFunction)) {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var length = transforms.length;
+ return restParam(function(args) {
+ var index = nativeMin(args.length, length);
+ while (index--) {
+ args[index] = transforms[index](args[index]);
+ }
+ return func.apply(this, args);
+ });
+});
+
+module.exports = modArgs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/negate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/negate.js b/node_modules/lodash/function/negate.js
new file mode 100644
index 0000000..8247939
--- /dev/null
+++ b/node_modules/lodash/function/negate.js
@@ -0,0 +1,32 @@
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a function that negates the result of the predicate `func`. The
+ * `func` predicate is invoked with the `this` binding and arguments of the
+ * created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} predicate The predicate to negate.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * function isEven(n) {
+ * return n % 2 == 0;
+ * }
+ *
+ * _.filter([1, 2, 3, 4, 5, 6], _.negate(isEven));
+ * // => [1, 3, 5]
+ */
+function negate(predicate) {
+ if (typeof predicate != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return function() {
+ return !predicate.apply(this, arguments);
+ };
+}
+
+module.exports = negate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/once.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/once.js b/node_modules/lodash/function/once.js
new file mode 100644
index 0000000..0b5bd85
--- /dev/null
+++ b/node_modules/lodash/function/once.js
@@ -0,0 +1,24 @@
+var before = require('./before');
+
+/**
+ * Creates a function that is restricted to invoking `func` once. Repeat calls
+ * to the function return the value of the first call. The `func` is invoked
+ * with the `this` binding and arguments of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // `initialize` invokes `createApplication` once
+ */
+function once(func) {
+ return before(2, func);
+}
+
+module.exports = once;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/partial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/partial.js b/node_modules/lodash/function/partial.js
new file mode 100644
index 0000000..fb1d04f
--- /dev/null
+++ b/node_modules/lodash/function/partial.js
@@ -0,0 +1,43 @@
+var createPartial = require('../internal/createPartial');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var PARTIAL_FLAG = 32;
+
+/**
+ * Creates a function that invokes `func` with `partial` arguments prepended
+ * to those provided to the new function. This method is like `_.bind` except
+ * it does **not** alter the `this` binding.
+ *
+ * The `_.partial.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** This method does not set the "length" property of partially
+ * applied functions.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var greet = function(greeting, name) {
+ * return greeting + ' ' + name;
+ * };
+ *
+ * var sayHelloTo = _.partial(greet, 'hello');
+ * sayHelloTo('fred');
+ * // => 'hello fred'
+ *
+ * // using placeholders
+ * var greetFred = _.partial(greet, _, 'fred');
+ * greetFred('hi');
+ * // => 'hi fred'
+ */
+var partial = createPartial(PARTIAL_FLAG);
+
+// Assign default placeholders.
+partial.placeholder = {};
+
+module.exports = partial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/partialRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/partialRight.js b/node_modules/lodash/function/partialRight.js
new file mode 100644
index 0000000..634e6a4
--- /dev/null
+++ b/node_modules/lodash/function/partialRight.js
@@ -0,0 +1,42 @@
+var createPartial = require('../internal/createPartial');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var PARTIAL_RIGHT_FLAG = 64;
+
+/**
+ * This method is like `_.partial` except that partially applied arguments
+ * are appended to those provided to the new function.
+ *
+ * The `_.partialRight.placeholder` value, which defaults to `_` in monolithic
+ * builds, may be used as a placeholder for partially applied arguments.
+ *
+ * **Note:** This method does not set the "length" property of partially
+ * applied functions.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [partials] The arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var greet = function(greeting, name) {
+ * return greeting + ' ' + name;
+ * };
+ *
+ * var greetFred = _.partialRight(greet, 'fred');
+ * greetFred('hi');
+ * // => 'hi fred'
+ *
+ * // using placeholders
+ * var sayHelloTo = _.partialRight(greet, 'hello', _);
+ * sayHelloTo('fred');
+ * // => 'hello fred'
+ */
+var partialRight = createPartial(PARTIAL_RIGHT_FLAG);
+
+// Assign default placeholders.
+partialRight.placeholder = {};
+
+module.exports = partialRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/rearg.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/rearg.js b/node_modules/lodash/function/rearg.js
new file mode 100644
index 0000000..f2bd9c4
--- /dev/null
+++ b/node_modules/lodash/function/rearg.js
@@ -0,0 +1,40 @@
+var baseFlatten = require('../internal/baseFlatten'),
+ createWrapper = require('../internal/createWrapper'),
+ restParam = require('./restParam');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var REARG_FLAG = 256;
+
+/**
+ * Creates a function that invokes `func` with arguments arranged according
+ * to the specified indexes where the argument value at the first index is
+ * provided as the first argument, the argument value at the second index is
+ * provided as the second argument, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to rearrange arguments for.
+ * @param {...(number|number[])} indexes The arranged argument indexes,
+ * specified as individual indexes or arrays of indexes.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var rearged = _.rearg(function(a, b, c) {
+ * return [a, b, c];
+ * }, 2, 0, 1);
+ *
+ * rearged('b', 'c', 'a')
+ * // => ['a', 'b', 'c']
+ *
+ * var map = _.rearg(_.map, [1, 0]);
+ * map(function(n) {
+ * return n * 3;
+ * }, [1, 2, 3]);
+ * // => [3, 6, 9]
+ */
+var rearg = restParam(function(func, indexes) {
+ return createWrapper(func, REARG_FLAG, undefined, undefined, undefined, baseFlatten(indexes));
+});
+
+module.exports = rearg;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/restParam.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/restParam.js b/node_modules/lodash/function/restParam.js
new file mode 100644
index 0000000..8852286
--- /dev/null
+++ b/node_modules/lodash/function/restParam.js
@@ -0,0 +1,58 @@
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of the
+ * created function and arguments from `start` and beyond provided as an array.
+ *
+ * **Note:** This method is based on the [rest parameter](https://developer.mozilla.org/Web/JavaScript/Reference/Functions/rest_parameters).
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.restParam(function(what, names) {
+ * return what + ' ' + _.initial(names).join(', ') +
+ * (_.size(names) > 1 ? ', & ' : '') + _.last(names);
+ * });
+ *
+ * say('hello', 'fred', 'barney', 'pebbles');
+ * // => 'hello fred, barney, & pebbles'
+ */
+function restParam(func, start) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ start = nativeMax(start === undefined ? (func.length - 1) : (+start || 0), 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ rest = Array(length);
+
+ while (++index < length) {
+ rest[index] = args[start + index];
+ }
+ switch (start) {
+ case 0: return func.call(this, rest);
+ case 1: return func.call(this, args[0], rest);
+ case 2: return func.call(this, args[0], args[1], rest);
+ }
+ var otherArgs = Array(start + 1);
+ index = -1;
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = rest;
+ return func.apply(this, otherArgs);
+ };
+}
+
+module.exports = restParam;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/spread.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/spread.js b/node_modules/lodash/function/spread.js
new file mode 100644
index 0000000..780f504
--- /dev/null
+++ b/node_modules/lodash/function/spread.js
@@ -0,0 +1,44 @@
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of the created
+ * function and an array of arguments much like [`Function#apply`](https://es5.github.io/#x15.3.4.3).
+ *
+ * **Note:** This method is based on the [spread operator](https://developer.mozilla.org/Web/JavaScript/Reference/Operators/Spread_operator).
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to spread arguments over.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.spread(function(who, what) {
+ * return who + ' says ' + what;
+ * });
+ *
+ * say(['fred', 'hello']);
+ * // => 'fred says hello'
+ *
+ * // with a Promise
+ * var numbers = Promise.all([
+ * Promise.resolve(40),
+ * Promise.resolve(36)
+ * ]);
+ *
+ * numbers.then(_.spread(function(x, y) {
+ * return x + y;
+ * }));
+ * // => a Promise of 76
+ */
+function spread(func) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return function(array) {
+ return func.apply(this, array);
+ };
+}
+
+module.exports = spread;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/throttle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/throttle.js b/node_modules/lodash/function/throttle.js
new file mode 100644
index 0000000..1dd00ea
--- /dev/null
+++ b/node_modules/lodash/function/throttle.js
@@ -0,0 +1,62 @@
+var debounce = require('./debounce'),
+ isObject = require('../lang/isObject');
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a throttled function that only invokes `func` at most once per
+ * every `wait` milliseconds. The throttled function comes with a `cancel`
+ * method to cancel delayed invocations. Provide an options object to indicate
+ * that `func` should be invoked on the leading and/or trailing edge of the
+ * `wait` timeout. Subsequent calls to the throttled function return the
+ * result of the last `func` call.
+ *
+ * **Note:** If `leading` and `trailing` options are `true`, `func` is invoked
+ * on the trailing edge of the timeout only if the the throttled function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * See [David Corbacho's article](http://drupalmotion.com/article/debounce-and-throttle-visual-explanation)
+ * for details over the differences between `_.throttle` and `_.debounce`.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to throttle.
+ * @param {number} [wait=0] The number of milliseconds to throttle invocations to.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=true] Specify invoking on the leading
+ * edge of the timeout.
+ * @param {boolean} [options.trailing=true] Specify invoking on the trailing
+ * edge of the timeout.
+ * @returns {Function} Returns the new throttled function.
+ * @example
+ *
+ * // avoid excessively updating the position while scrolling
+ * jQuery(window).on('scroll', _.throttle(updatePosition, 100));
+ *
+ * // invoke `renewToken` when the click event is fired, but not more than once every 5 minutes
+ * jQuery('.interactive').on('click', _.throttle(renewToken, 300000, {
+ * 'trailing': false
+ * }));
+ *
+ * // cancel a trailing throttled call
+ * jQuery(window).on('popstate', throttled.cancel);
+ */
+function throttle(func, wait, options) {
+ var leading = true,
+ trailing = true;
+
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ if (options === false) {
+ leading = false;
+ } else if (isObject(options)) {
+ leading = 'leading' in options ? !!options.leading : leading;
+ trailing = 'trailing' in options ? !!options.trailing : trailing;
+ }
+ return debounce(func, wait, { 'leading': leading, 'maxWait': +wait, 'trailing': trailing });
+}
+
+module.exports = throttle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/function/wrap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/function/wrap.js b/node_modules/lodash/function/wrap.js
new file mode 100644
index 0000000..6a33c5e
--- /dev/null
+++ b/node_modules/lodash/function/wrap.js
@@ -0,0 +1,33 @@
+var createWrapper = require('../internal/createWrapper'),
+ identity = require('../utility/identity');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var PARTIAL_FLAG = 32;
+
+/**
+ * Creates a function that provides `value` to the wrapper function as its
+ * first argument. Any additional arguments provided to the function are
+ * appended to those provided to the wrapper function. The wrapper is invoked
+ * with the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {*} value The value to wrap.
+ * @param {Function} wrapper The wrapper function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var p = _.wrap(_.escape, function(func, text) {
+ * return '<p>' + func(text) + '</p>';
+ * });
+ *
+ * p('fred, barney, & pebbles');
+ * // => '<p>fred, barney, & pebbles</p>'
+ */
+function wrap(value, wrapper) {
+ wrapper = wrapper == null ? identity : wrapper;
+ return createWrapper(wrapper, PARTIAL_FLAG, undefined, [value], []);
+}
+
+module.exports = wrap;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[23/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/min.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/min.js b/node_modules/lodash/math/min.js
new file mode 100644
index 0000000..6d92d4f
--- /dev/null
+++ b/node_modules/lodash/math/min.js
@@ -0,0 +1,56 @@
+var createExtremum = require('../internal/createExtremum'),
+ lt = require('../lang/lt');
+
+/** Used as references for `-Infinity` and `Infinity`. */
+var POSITIVE_INFINITY = Number.POSITIVE_INFINITY;
+
+/**
+ * Gets the minimum value of `collection`. If `collection` is empty or falsey
+ * `Infinity` is returned. If an iteratee function is provided it's invoked
+ * for each value in `collection` to generate the criterion by which the value
+ * is ranked. The `iteratee` is bound to `thisArg` and invoked with three
+ * arguments: (value, index, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {*} Returns the minimum value.
+ * @example
+ *
+ * _.min([4, 2, 8, 6]);
+ * // => 2
+ *
+ * _.min([]);
+ * // => Infinity
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.min(users, function(chr) {
+ * return chr.age;
+ * });
+ * // => { 'user': 'barney', 'age': 36 }
+ *
+ * // using the `_.property` callback shorthand
+ * _.min(users, 'age');
+ * // => { 'user': 'barney', 'age': 36 }
+ */
+var min = createExtremum(lt, POSITIVE_INFINITY);
+
+module.exports = min;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/round.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/round.js b/node_modules/lodash/math/round.js
new file mode 100644
index 0000000..5c69d0c
--- /dev/null
+++ b/node_modules/lodash/math/round.js
@@ -0,0 +1,25 @@
+var createRound = require('../internal/createRound');
+
+/**
+ * Calculates `n` rounded to `precision`.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {number} n The number to round.
+ * @param {number} [precision=0] The precision to round to.
+ * @returns {number} Returns the rounded number.
+ * @example
+ *
+ * _.round(4.006);
+ * // => 4
+ *
+ * _.round(4.006, 2);
+ * // => 4.01
+ *
+ * _.round(4060, -2);
+ * // => 4100
+ */
+var round = createRound('round');
+
+module.exports = round;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/math/sum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/math/sum.js b/node_modules/lodash/math/sum.js
new file mode 100644
index 0000000..114ff1b
--- /dev/null
+++ b/node_modules/lodash/math/sum.js
@@ -0,0 +1,50 @@
+var arraySum = require('../internal/arraySum'),
+ baseCallback = require('../internal/baseCallback'),
+ baseSum = require('../internal/baseSum'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ toIterable = require('../internal/toIterable');
+
+/**
+ * Gets the sum of the values in `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Math
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {number} Returns the sum.
+ * @example
+ *
+ * _.sum([4, 6]);
+ * // => 10
+ *
+ * _.sum({ 'a': 4, 'b': 6 });
+ * // => 10
+ *
+ * var objects = [
+ * { 'n': 4 },
+ * { 'n': 6 }
+ * ];
+ *
+ * _.sum(objects, function(object) {
+ * return object.n;
+ * });
+ * // => 10
+ *
+ * // using the `_.property` callback shorthand
+ * _.sum(objects, 'n');
+ * // => 10
+ */
+function sum(collection, iteratee, thisArg) {
+ if (thisArg && isIterateeCall(collection, iteratee, thisArg)) {
+ iteratee = undefined;
+ }
+ iteratee = baseCallback(iteratee, thisArg, 3);
+ return iteratee.length == 1
+ ? arraySum(isArray(collection) ? collection : toIterable(collection), iteratee)
+ : baseSum(collection, iteratee);
+}
+
+module.exports = sum;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/number.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/number.js b/node_modules/lodash/number.js
new file mode 100644
index 0000000..afab9d9
--- /dev/null
+++ b/node_modules/lodash/number.js
@@ -0,0 +1,4 @@
+module.exports = {
+ 'inRange': require('./number/inRange'),
+ 'random': require('./number/random')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/number/inRange.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/number/inRange.js b/node_modules/lodash/number/inRange.js
new file mode 100644
index 0000000..30bf798
--- /dev/null
+++ b/node_modules/lodash/number/inRange.js
@@ -0,0 +1,47 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Checks if `n` is between `start` and up to but not including, `end`. If
+ * `end` is not specified it's set to `start` with `start` then set to `0`.
+ *
+ * @static
+ * @memberOf _
+ * @category Number
+ * @param {number} n The number to check.
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @returns {boolean} Returns `true` if `n` is in the range, else `false`.
+ * @example
+ *
+ * _.inRange(3, 2, 4);
+ * // => true
+ *
+ * _.inRange(4, 8);
+ * // => true
+ *
+ * _.inRange(4, 2);
+ * // => false
+ *
+ * _.inRange(2, 2);
+ * // => false
+ *
+ * _.inRange(1.2, 2);
+ * // => true
+ *
+ * _.inRange(5.2, 4);
+ * // => false
+ */
+function inRange(value, start, end) {
+ start = +start || 0;
+ if (end === undefined) {
+ end = start;
+ start = 0;
+ } else {
+ end = +end || 0;
+ }
+ return value >= nativeMin(start, end) && value < nativeMax(start, end);
+}
+
+module.exports = inRange;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/number/random.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/number/random.js b/node_modules/lodash/number/random.js
new file mode 100644
index 0000000..589d74e
--- /dev/null
+++ b/node_modules/lodash/number/random.js
@@ -0,0 +1,70 @@
+var baseRandom = require('../internal/baseRandom'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min,
+ nativeRandom = Math.random;
+
+/**
+ * Produces a random number between `min` and `max` (inclusive). If only one
+ * argument is provided a number between `0` and the given number is returned.
+ * If `floating` is `true`, or either `min` or `max` are floats, a floating-point
+ * number is returned instead of an integer.
+ *
+ * @static
+ * @memberOf _
+ * @category Number
+ * @param {number} [min=0] The minimum possible value.
+ * @param {number} [max=1] The maximum possible value.
+ * @param {boolean} [floating] Specify returning a floating-point number.
+ * @returns {number} Returns the random number.
+ * @example
+ *
+ * _.random(0, 5);
+ * // => an integer between 0 and 5
+ *
+ * _.random(5);
+ * // => also an integer between 0 and 5
+ *
+ * _.random(5, true);
+ * // => a floating-point number between 0 and 5
+ *
+ * _.random(1.2, 5.2);
+ * // => a floating-point number between 1.2 and 5.2
+ */
+function random(min, max, floating) {
+ if (floating && isIterateeCall(min, max, floating)) {
+ max = floating = undefined;
+ }
+ var noMin = min == null,
+ noMax = max == null;
+
+ if (floating == null) {
+ if (noMax && typeof min == 'boolean') {
+ floating = min;
+ min = 1;
+ }
+ else if (typeof max == 'boolean') {
+ floating = max;
+ noMax = true;
+ }
+ }
+ if (noMin && noMax) {
+ max = 1;
+ noMax = false;
+ }
+ min = +min || 0;
+ if (noMax) {
+ max = min;
+ min = 0;
+ } else {
+ max = +max || 0;
+ }
+ if (floating || min % 1 || max % 1) {
+ var rand = nativeRandom();
+ return nativeMin(min + (rand * (max - min + parseFloat('1e-' + ((rand + '').length - 1)))), max);
+ }
+ return baseRandom(min, max);
+}
+
+module.exports = random;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object.js b/node_modules/lodash/object.js
new file mode 100644
index 0000000..4beb005
--- /dev/null
+++ b/node_modules/lodash/object.js
@@ -0,0 +1,31 @@
+module.exports = {
+ 'assign': require('./object/assign'),
+ 'create': require('./object/create'),
+ 'defaults': require('./object/defaults'),
+ 'defaultsDeep': require('./object/defaultsDeep'),
+ 'extend': require('./object/extend'),
+ 'findKey': require('./object/findKey'),
+ 'findLastKey': require('./object/findLastKey'),
+ 'forIn': require('./object/forIn'),
+ 'forInRight': require('./object/forInRight'),
+ 'forOwn': require('./object/forOwn'),
+ 'forOwnRight': require('./object/forOwnRight'),
+ 'functions': require('./object/functions'),
+ 'get': require('./object/get'),
+ 'has': require('./object/has'),
+ 'invert': require('./object/invert'),
+ 'keys': require('./object/keys'),
+ 'keysIn': require('./object/keysIn'),
+ 'mapKeys': require('./object/mapKeys'),
+ 'mapValues': require('./object/mapValues'),
+ 'merge': require('./object/merge'),
+ 'methods': require('./object/methods'),
+ 'omit': require('./object/omit'),
+ 'pairs': require('./object/pairs'),
+ 'pick': require('./object/pick'),
+ 'result': require('./object/result'),
+ 'set': require('./object/set'),
+ 'transform': require('./object/transform'),
+ 'values': require('./object/values'),
+ 'valuesIn': require('./object/valuesIn')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/assign.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/assign.js b/node_modules/lodash/object/assign.js
new file mode 100644
index 0000000..4a765ed
--- /dev/null
+++ b/node_modules/lodash/object/assign.js
@@ -0,0 +1,43 @@
+var assignWith = require('../internal/assignWith'),
+ baseAssign = require('../internal/baseAssign'),
+ createAssigner = require('../internal/createAssigner');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object. Subsequent sources overwrite property assignments of previous sources.
+ * If `customizer` is provided it's invoked to produce the assigned values.
+ * The `customizer` is bound to `thisArg` and invoked with five arguments:
+ * (objectValue, sourceValue, key, object, source).
+ *
+ * **Note:** This method mutates `object` and is based on
+ * [`Object.assign`](http://ecma-international.org/ecma-262/6.0/#sec-object.assign).
+ *
+ * @static
+ * @memberOf _
+ * @alias extend
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.assign({ 'user': 'barney' }, { 'age': 40 }, { 'user': 'fred' });
+ * // => { 'user': 'fred', 'age': 40 }
+ *
+ * // using a customizer callback
+ * var defaults = _.partialRight(_.assign, function(value, other) {
+ * return _.isUndefined(value) ? other : value;
+ * });
+ *
+ * defaults({ 'user': 'barney' }, { 'age': 36 }, { 'user': 'fred' });
+ * // => { 'user': 'barney', 'age': 36 }
+ */
+var assign = createAssigner(function(object, source, customizer) {
+ return customizer
+ ? assignWith(object, source, customizer)
+ : baseAssign(object, source);
+});
+
+module.exports = assign;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/create.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/create.js b/node_modules/lodash/object/create.js
new file mode 100644
index 0000000..176294f
--- /dev/null
+++ b/node_modules/lodash/object/create.js
@@ -0,0 +1,47 @@
+var baseAssign = require('../internal/baseAssign'),
+ baseCreate = require('../internal/baseCreate'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates an object that inherits from the given `prototype` object. If a
+ * `properties` object is provided its own enumerable properties are assigned
+ * to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, {
+ * 'constructor': Circle
+ * });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+function create(prototype, properties, guard) {
+ var result = baseCreate(prototype);
+ if (guard && isIterateeCall(prototype, properties, guard)) {
+ properties = undefined;
+ }
+ return properties ? baseAssign(result, properties) : result;
+}
+
+module.exports = create;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/defaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/defaults.js b/node_modules/lodash/object/defaults.js
new file mode 100644
index 0000000..c05011e
--- /dev/null
+++ b/node_modules/lodash/object/defaults.js
@@ -0,0 +1,25 @@
+var assign = require('./assign'),
+ assignDefaults = require('../internal/assignDefaults'),
+ createDefaults = require('../internal/createDefaults');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object for all destination properties that resolve to `undefined`. Once a
+ * property is set, additional values of the same property are ignored.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.defaults({ 'user': 'barney' }, { 'age': 36 }, { 'user': 'fred' });
+ * // => { 'user': 'barney', 'age': 36 }
+ */
+var defaults = createDefaults(assign, assignDefaults);
+
+module.exports = defaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/defaultsDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/defaultsDeep.js b/node_modules/lodash/object/defaultsDeep.js
new file mode 100644
index 0000000..ec6e687
--- /dev/null
+++ b/node_modules/lodash/object/defaultsDeep.js
@@ -0,0 +1,25 @@
+var createDefaults = require('../internal/createDefaults'),
+ merge = require('./merge'),
+ mergeDefaults = require('../internal/mergeDefaults');
+
+/**
+ * This method is like `_.defaults` except that it recursively assigns
+ * default properties.
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.defaultsDeep({ 'user': { 'name': 'barney' } }, { 'user': { 'name': 'fred', 'age': 36 } });
+ * // => { 'user': { 'name': 'barney', 'age': 36 } }
+ *
+ */
+var defaultsDeep = createDefaults(merge, mergeDefaults);
+
+module.exports = defaultsDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/extend.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/extend.js b/node_modules/lodash/object/extend.js
new file mode 100644
index 0000000..dd0ca94
--- /dev/null
+++ b/node_modules/lodash/object/extend.js
@@ -0,0 +1 @@
+module.exports = require('./assign');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/findKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/findKey.js b/node_modules/lodash/object/findKey.js
new file mode 100644
index 0000000..1359df3
--- /dev/null
+++ b/node_modules/lodash/object/findKey.js
@@ -0,0 +1,54 @@
+var baseForOwn = require('../internal/baseForOwn'),
+ createFindKey = require('../internal/createFindKey');
+
+/**
+ * This method is like `_.find` except that it returns the key of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to search.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {string|undefined} Returns the key of the matched element, else `undefined`.
+ * @example
+ *
+ * var users = {
+ * 'barney': { 'age': 36, 'active': true },
+ * 'fred': { 'age': 40, 'active': false },
+ * 'pebbles': { 'age': 1, 'active': true }
+ * };
+ *
+ * _.findKey(users, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => 'barney' (iteration order is not guaranteed)
+ *
+ * // using the `_.matches` callback shorthand
+ * _.findKey(users, { 'age': 1, 'active': true });
+ * // => 'pebbles'
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.findKey(users, 'active', false);
+ * // => 'fred'
+ *
+ * // using the `_.property` callback shorthand
+ * _.findKey(users, 'active');
+ * // => 'barney'
+ */
+var findKey = createFindKey(baseForOwn);
+
+module.exports = findKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/findLastKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/findLastKey.js b/node_modules/lodash/object/findLastKey.js
new file mode 100644
index 0000000..42893a4
--- /dev/null
+++ b/node_modules/lodash/object/findLastKey.js
@@ -0,0 +1,54 @@
+var baseForOwnRight = require('../internal/baseForOwnRight'),
+ createFindKey = require('../internal/createFindKey');
+
+/**
+ * This method is like `_.findKey` except that it iterates over elements of
+ * a collection in the opposite order.
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to search.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {string|undefined} Returns the key of the matched element, else `undefined`.
+ * @example
+ *
+ * var users = {
+ * 'barney': { 'age': 36, 'active': true },
+ * 'fred': { 'age': 40, 'active': false },
+ * 'pebbles': { 'age': 1, 'active': true }
+ * };
+ *
+ * _.findLastKey(users, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => returns `pebbles` assuming `_.findKey` returns `barney`
+ *
+ * // using the `_.matches` callback shorthand
+ * _.findLastKey(users, { 'age': 36, 'active': true });
+ * // => 'barney'
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.findLastKey(users, 'active', false);
+ * // => 'fred'
+ *
+ * // using the `_.property` callback shorthand
+ * _.findLastKey(users, 'active');
+ * // => 'pebbles'
+ */
+var findLastKey = createFindKey(baseForOwnRight);
+
+module.exports = findLastKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/forIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/forIn.js b/node_modules/lodash/object/forIn.js
new file mode 100644
index 0000000..52d34af
--- /dev/null
+++ b/node_modules/lodash/object/forIn.js
@@ -0,0 +1,33 @@
+var baseFor = require('../internal/baseFor'),
+ createForIn = require('../internal/createForIn');
+
+/**
+ * Iterates over own and inherited enumerable properties of an object invoking
+ * `iteratee` for each property. The `iteratee` is bound to `thisArg` and invoked
+ * with three arguments: (value, key, object). Iteratee functions may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forIn(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'a', 'b', and 'c' (iteration order is not guaranteed)
+ */
+var forIn = createForIn(baseFor);
+
+module.exports = forIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/forInRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/forInRight.js b/node_modules/lodash/object/forInRight.js
new file mode 100644
index 0000000..6780b92
--- /dev/null
+++ b/node_modules/lodash/object/forInRight.js
@@ -0,0 +1,31 @@
+var baseForRight = require('../internal/baseForRight'),
+ createForIn = require('../internal/createForIn');
+
+/**
+ * This method is like `_.forIn` except that it iterates over properties of
+ * `object` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forInRight(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'c', 'b', and 'a' assuming `_.forIn ` logs 'a', 'b', and 'c'
+ */
+var forInRight = createForIn(baseForRight);
+
+module.exports = forInRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/forOwn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/forOwn.js b/node_modules/lodash/object/forOwn.js
new file mode 100644
index 0000000..747bb76
--- /dev/null
+++ b/node_modules/lodash/object/forOwn.js
@@ -0,0 +1,33 @@
+var baseForOwn = require('../internal/baseForOwn'),
+ createForOwn = require('../internal/createForOwn');
+
+/**
+ * Iterates over own enumerable properties of an object invoking `iteratee`
+ * for each property. The `iteratee` is bound to `thisArg` and invoked with
+ * three arguments: (value, key, object). Iteratee functions may exit iteration
+ * early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forOwn(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'a' and 'b' (iteration order is not guaranteed)
+ */
+var forOwn = createForOwn(baseForOwn);
+
+module.exports = forOwn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/forOwnRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/forOwnRight.js b/node_modules/lodash/object/forOwnRight.js
new file mode 100644
index 0000000..8122338
--- /dev/null
+++ b/node_modules/lodash/object/forOwnRight.js
@@ -0,0 +1,31 @@
+var baseForOwnRight = require('../internal/baseForOwnRight'),
+ createForOwn = require('../internal/createForOwn');
+
+/**
+ * This method is like `_.forOwn` except that it iterates over properties of
+ * `object` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.forOwnRight(new Foo, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'b' and 'a' assuming `_.forOwn` logs 'a' and 'b'
+ */
+var forOwnRight = createForOwn(baseForOwnRight);
+
+module.exports = forOwnRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/functions.js b/node_modules/lodash/object/functions.js
new file mode 100644
index 0000000..10799be
--- /dev/null
+++ b/node_modules/lodash/object/functions.js
@@ -0,0 +1,23 @@
+var baseFunctions = require('../internal/baseFunctions'),
+ keysIn = require('./keysIn');
+
+/**
+ * Creates an array of function property names from all enumerable properties,
+ * own and inherited, of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @alias methods
+ * @category Object
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns the new array of property names.
+ * @example
+ *
+ * _.functions(_);
+ * // => ['after', 'ary', 'assign', ...]
+ */
+function functions(object) {
+ return baseFunctions(object, keysIn(object));
+}
+
+module.exports = functions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/get.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/get.js b/node_modules/lodash/object/get.js
new file mode 100644
index 0000000..7e88f1e
--- /dev/null
+++ b/node_modules/lodash/object/get.js
@@ -0,0 +1,33 @@
+var baseGet = require('../internal/baseGet'),
+ toPath = require('../internal/toPath');
+
+/**
+ * Gets the property value at `path` of `object`. If the resolved value is
+ * `undefined` the `defaultValue` is used in its place.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to get.
+ * @param {*} [defaultValue] The value returned if the resolved value is `undefined`.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }] };
+ *
+ * _.get(object, 'a[0].b.c');
+ * // => 3
+ *
+ * _.get(object, ['a', '0', 'b', 'c']);
+ * // => 3
+ *
+ * _.get(object, 'a.b.c', 'default');
+ * // => 'default'
+ */
+function get(object, path, defaultValue) {
+ var result = object == null ? undefined : baseGet(object, toPath(path), (path + ''));
+ return result === undefined ? defaultValue : result;
+}
+
+module.exports = get;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/has.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/has.js b/node_modules/lodash/object/has.js
new file mode 100644
index 0000000..f356243
--- /dev/null
+++ b/node_modules/lodash/object/has.js
@@ -0,0 +1,57 @@
+var baseGet = require('../internal/baseGet'),
+ baseSlice = require('../internal/baseSlice'),
+ isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isIndex = require('../internal/isIndex'),
+ isKey = require('../internal/isKey'),
+ isLength = require('../internal/isLength'),
+ last = require('../array/last'),
+ toPath = require('../internal/toPath');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if `path` is a direct property.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path to check.
+ * @returns {boolean} Returns `true` if `path` is a direct property, else `false`.
+ * @example
+ *
+ * var object = { 'a': { 'b': { 'c': 3 } } };
+ *
+ * _.has(object, 'a');
+ * // => true
+ *
+ * _.has(object, 'a.b.c');
+ * // => true
+ *
+ * _.has(object, ['a', 'b', 'c']);
+ * // => true
+ */
+function has(object, path) {
+ if (object == null) {
+ return false;
+ }
+ var result = hasOwnProperty.call(object, path);
+ if (!result && !isKey(path)) {
+ path = toPath(path);
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ if (object == null) {
+ return false;
+ }
+ path = last(path);
+ result = hasOwnProperty.call(object, path);
+ }
+ return result || (isLength(object.length) && isIndex(path, object.length) &&
+ (isArray(object) || isArguments(object)));
+}
+
+module.exports = has;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/invert.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/invert.js b/node_modules/lodash/object/invert.js
new file mode 100644
index 0000000..54fb1f1
--- /dev/null
+++ b/node_modules/lodash/object/invert.js
@@ -0,0 +1,60 @@
+var isIterateeCall = require('../internal/isIterateeCall'),
+ keys = require('./keys');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of the inverted keys and values of `object`.
+ * If `object` contains duplicate values, subsequent values overwrite property
+ * assignments of previous values unless `multiValue` is `true`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to invert.
+ * @param {boolean} [multiValue] Allow multiple values per key.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Object} Returns the new inverted object.
+ * @example
+ *
+ * var object = { 'a': 1, 'b': 2, 'c': 1 };
+ *
+ * _.invert(object);
+ * // => { '1': 'c', '2': 'b' }
+ *
+ * // with `multiValue`
+ * _.invert(object, true);
+ * // => { '1': ['a', 'c'], '2': ['b'] }
+ */
+function invert(object, multiValue, guard) {
+ if (guard && isIterateeCall(object, multiValue, guard)) {
+ multiValue = undefined;
+ }
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index],
+ value = object[key];
+
+ if (multiValue) {
+ if (hasOwnProperty.call(result, value)) {
+ result[value].push(key);
+ } else {
+ result[value] = [key];
+ }
+ }
+ else {
+ result[value] = key;
+ }
+ }
+ return result;
+}
+
+module.exports = invert;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/keys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/keys.js b/node_modules/lodash/object/keys.js
new file mode 100644
index 0000000..4706fd6
--- /dev/null
+++ b/node_modules/lodash/object/keys.js
@@ -0,0 +1,45 @@
+var getNative = require('../internal/getNative'),
+ isArrayLike = require('../internal/isArrayLike'),
+ isObject = require('../lang/isObject'),
+ shimKeys = require('../internal/shimKeys');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeKeys = getNative(Object, 'keys');
+
+/**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/6.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+var keys = !nativeKeys ? shimKeys : function(object) {
+ var Ctor = object == null ? undefined : object.constructor;
+ if ((typeof Ctor == 'function' && Ctor.prototype === object) ||
+ (typeof object != 'function' && isArrayLike(object))) {
+ return shimKeys(object);
+ }
+ return isObject(object) ? nativeKeys(object) : [];
+};
+
+module.exports = keys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/keysIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/keysIn.js b/node_modules/lodash/object/keysIn.js
new file mode 100644
index 0000000..45a85d7
--- /dev/null
+++ b/node_modules/lodash/object/keysIn.js
@@ -0,0 +1,64 @@
+var isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isIndex = require('../internal/isIndex'),
+ isLength = require('../internal/isLength'),
+ isObject = require('../lang/isObject');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+function keysIn(object) {
+ if (object == null) {
+ return [];
+ }
+ if (!isObject(object)) {
+ object = Object(object);
+ }
+ var length = object.length;
+ length = (length && isLength(length) &&
+ (isArray(object) || isArguments(object)) && length) || 0;
+
+ var Ctor = object.constructor,
+ index = -1,
+ isProto = typeof Ctor == 'function' && Ctor.prototype === object,
+ result = Array(length),
+ skipIndexes = length > 0;
+
+ while (++index < length) {
+ result[index] = (index + '');
+ }
+ for (var key in object) {
+ if (!(skipIndexes && isIndex(key, length)) &&
+ !(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = keysIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/mapKeys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/mapKeys.js b/node_modules/lodash/object/mapKeys.js
new file mode 100644
index 0000000..680b29b
--- /dev/null
+++ b/node_modules/lodash/object/mapKeys.js
@@ -0,0 +1,25 @@
+var createObjectMapper = require('../internal/createObjectMapper');
+
+/**
+ * The opposite of `_.mapValues`; this method creates an object with the
+ * same values as `object` and keys generated by running each own enumerable
+ * property of `object` through `iteratee`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns the new mapped object.
+ * @example
+ *
+ * _.mapKeys({ 'a': 1, 'b': 2 }, function(value, key) {
+ * return key + value;
+ * });
+ * // => { 'a1': 1, 'b2': 2 }
+ */
+var mapKeys = createObjectMapper(true);
+
+module.exports = mapKeys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/mapValues.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/mapValues.js b/node_modules/lodash/object/mapValues.js
new file mode 100644
index 0000000..2afe6ba
--- /dev/null
+++ b/node_modules/lodash/object/mapValues.js
@@ -0,0 +1,46 @@
+var createObjectMapper = require('../internal/createObjectMapper');
+
+/**
+ * Creates an object with the same keys as `object` and values generated by
+ * running each own enumerable property of `object` through `iteratee`. The
+ * iteratee function is bound to `thisArg` and invoked with three arguments:
+ * (value, key, object).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns the new mapped object.
+ * @example
+ *
+ * _.mapValues({ 'a': 1, 'b': 2 }, function(n) {
+ * return n * 3;
+ * });
+ * // => { 'a': 3, 'b': 6 }
+ *
+ * var users = {
+ * 'fred': { 'user': 'fred', 'age': 40 },
+ * 'pebbles': { 'user': 'pebbles', 'age': 1 }
+ * };
+ *
+ * // using the `_.property` callback shorthand
+ * _.mapValues(users, 'age');
+ * // => { 'fred': 40, 'pebbles': 1 } (iteration order is not guaranteed)
+ */
+var mapValues = createObjectMapper();
+
+module.exports = mapValues;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/merge.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/merge.js b/node_modules/lodash/object/merge.js
new file mode 100644
index 0000000..86dd8af
--- /dev/null
+++ b/node_modules/lodash/object/merge.js
@@ -0,0 +1,54 @@
+var baseMerge = require('../internal/baseMerge'),
+ createAssigner = require('../internal/createAssigner');
+
+/**
+ * Recursively merges own enumerable properties of the source object(s), that
+ * don't resolve to `undefined` into the destination object. Subsequent sources
+ * overwrite property assignments of previous sources. If `customizer` is
+ * provided it's invoked to produce the merged values of the destination and
+ * source properties. If `customizer` returns `undefined` merging is handled
+ * by the method instead. The `customizer` is bound to `thisArg` and invoked
+ * with five arguments: (objectValue, sourceValue, key, object, source).
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @param {*} [thisArg] The `this` binding of `customizer`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var users = {
+ * 'data': [{ 'user': 'barney' }, { 'user': 'fred' }]
+ * };
+ *
+ * var ages = {
+ * 'data': [{ 'age': 36 }, { 'age': 40 }]
+ * };
+ *
+ * _.merge(users, ages);
+ * // => { 'data': [{ 'user': 'barney', 'age': 36 }, { 'user': 'fred', 'age': 40 }] }
+ *
+ * // using a customizer callback
+ * var object = {
+ * 'fruits': ['apple'],
+ * 'vegetables': ['beet']
+ * };
+ *
+ * var other = {
+ * 'fruits': ['banana'],
+ * 'vegetables': ['carrot']
+ * };
+ *
+ * _.merge(object, other, function(a, b) {
+ * if (_.isArray(a)) {
+ * return a.concat(b);
+ * }
+ * });
+ * // => { 'fruits': ['apple', 'banana'], 'vegetables': ['beet', 'carrot'] }
+ */
+var merge = createAssigner(baseMerge);
+
+module.exports = merge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/methods.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/methods.js b/node_modules/lodash/object/methods.js
new file mode 100644
index 0000000..8a304fe
--- /dev/null
+++ b/node_modules/lodash/object/methods.js
@@ -0,0 +1 @@
+module.exports = require('./functions');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/omit.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/omit.js b/node_modules/lodash/object/omit.js
new file mode 100644
index 0000000..fe3f485
--- /dev/null
+++ b/node_modules/lodash/object/omit.js
@@ -0,0 +1,47 @@
+var arrayMap = require('../internal/arrayMap'),
+ baseDifference = require('../internal/baseDifference'),
+ baseFlatten = require('../internal/baseFlatten'),
+ bindCallback = require('../internal/bindCallback'),
+ keysIn = require('./keysIn'),
+ pickByArray = require('../internal/pickByArray'),
+ pickByCallback = require('../internal/pickByCallback'),
+ restParam = require('../function/restParam');
+
+/**
+ * The opposite of `_.pick`; this method creates an object composed of the
+ * own and inherited enumerable properties of `object` that are not omitted.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {Function|...(string|string[])} [predicate] The function invoked per
+ * iteration or property names to omit, specified as individual property
+ * names or arrays of property names.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'user': 'fred', 'age': 40 };
+ *
+ * _.omit(object, 'age');
+ * // => { 'user': 'fred' }
+ *
+ * _.omit(object, _.isNumber);
+ * // => { 'user': 'fred' }
+ */
+var omit = restParam(function(object, props) {
+ if (object == null) {
+ return {};
+ }
+ if (typeof props[0] != 'function') {
+ var props = arrayMap(baseFlatten(props), String);
+ return pickByArray(object, baseDifference(keysIn(object), props));
+ }
+ var predicate = bindCallback(props[0], props[1], 3);
+ return pickByCallback(object, function(value, key, object) {
+ return !predicate(value, key, object);
+ });
+});
+
+module.exports = omit;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/pairs.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/pairs.js b/node_modules/lodash/object/pairs.js
new file mode 100644
index 0000000..fd4644c
--- /dev/null
+++ b/node_modules/lodash/object/pairs.js
@@ -0,0 +1,33 @@
+var keys = require('./keys'),
+ toObject = require('../internal/toObject');
+
+/**
+ * Creates a two dimensional array of the key-value pairs for `object`,
+ * e.g. `[[key1, value1], [key2, value2]]`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the new array of key-value pairs.
+ * @example
+ *
+ * _.pairs({ 'barney': 36, 'fred': 40 });
+ * // => [['barney', 36], ['fred', 40]] (iteration order is not guaranteed)
+ */
+function pairs(object) {
+ object = toObject(object);
+
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ var key = props[index];
+ result[index] = [key, object[key]];
+ }
+ return result;
+}
+
+module.exports = pairs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/pick.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/pick.js b/node_modules/lodash/object/pick.js
new file mode 100644
index 0000000..e318766
--- /dev/null
+++ b/node_modules/lodash/object/pick.js
@@ -0,0 +1,42 @@
+var baseFlatten = require('../internal/baseFlatten'),
+ bindCallback = require('../internal/bindCallback'),
+ pickByArray = require('../internal/pickByArray'),
+ pickByCallback = require('../internal/pickByCallback'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates an object composed of the picked `object` properties. Property
+ * names may be specified as individual arguments or as arrays of property
+ * names. If `predicate` is provided it's invoked for each property of `object`
+ * picking the properties `predicate` returns truthy for. The predicate is
+ * bound to `thisArg` and invoked with three arguments: (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The source object.
+ * @param {Function|...(string|string[])} [predicate] The function invoked per
+ * iteration or property names to pick, specified as individual property
+ * names or arrays of property names.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * var object = { 'user': 'fred', 'age': 40 };
+ *
+ * _.pick(object, 'user');
+ * // => { 'user': 'fred' }
+ *
+ * _.pick(object, _.isString);
+ * // => { 'user': 'fred' }
+ */
+var pick = restParam(function(object, props) {
+ if (object == null) {
+ return {};
+ }
+ return typeof props[0] == 'function'
+ ? pickByCallback(object, bindCallback(props[0], props[1], 3))
+ : pickByArray(object, baseFlatten(props));
+});
+
+module.exports = pick;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/result.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/result.js b/node_modules/lodash/object/result.js
new file mode 100644
index 0000000..29b38e6
--- /dev/null
+++ b/node_modules/lodash/object/result.js
@@ -0,0 +1,49 @@
+var baseGet = require('../internal/baseGet'),
+ baseSlice = require('../internal/baseSlice'),
+ isFunction = require('../lang/isFunction'),
+ isKey = require('../internal/isKey'),
+ last = require('../array/last'),
+ toPath = require('../internal/toPath');
+
+/**
+ * This method is like `_.get` except that if the resolved value is a function
+ * it's invoked with the `this` binding of its parent object and its result
+ * is returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the property to resolve.
+ * @param {*} [defaultValue] The value returned if the resolved value is `undefined`.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c1': 3, 'c2': _.constant(4) } }] };
+ *
+ * _.result(object, 'a[0].b.c1');
+ * // => 3
+ *
+ * _.result(object, 'a[0].b.c2');
+ * // => 4
+ *
+ * _.result(object, 'a.b.c', 'default');
+ * // => 'default'
+ *
+ * _.result(object, 'a.b.c', _.constant('default'));
+ * // => 'default'
+ */
+function result(object, path, defaultValue) {
+ var result = object == null ? undefined : object[path];
+ if (result === undefined) {
+ if (object != null && !isKey(path, object)) {
+ path = toPath(path);
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ result = object == null ? undefined : object[last(path)];
+ }
+ result = result === undefined ? defaultValue : result;
+ }
+ return isFunction(result) ? result.call(object) : result;
+}
+
+module.exports = result;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/set.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/set.js b/node_modules/lodash/object/set.js
new file mode 100644
index 0000000..7a1e4e9
--- /dev/null
+++ b/node_modules/lodash/object/set.js
@@ -0,0 +1,55 @@
+var isIndex = require('../internal/isIndex'),
+ isKey = require('../internal/isKey'),
+ isObject = require('../lang/isObject'),
+ toPath = require('../internal/toPath');
+
+/**
+ * Sets the property value of `path` on `object`. If a portion of `path`
+ * does not exist it's created.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to augment.
+ * @param {Array|string} path The path of the property to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var object = { 'a': [{ 'b': { 'c': 3 } }] };
+ *
+ * _.set(object, 'a[0].b.c', 4);
+ * console.log(object.a[0].b.c);
+ * // => 4
+ *
+ * _.set(object, 'x[0].y.z', 5);
+ * console.log(object.x[0].y.z);
+ * // => 5
+ */
+function set(object, path, value) {
+ if (object == null) {
+ return object;
+ }
+ var pathKey = (path + '');
+ path = (object[pathKey] != null || isKey(path, object)) ? [pathKey] : toPath(path);
+
+ var index = -1,
+ length = path.length,
+ lastIndex = length - 1,
+ nested = object;
+
+ while (nested != null && ++index < length) {
+ var key = path[index];
+ if (isObject(nested)) {
+ if (index == lastIndex) {
+ nested[key] = value;
+ } else if (nested[key] == null) {
+ nested[key] = isIndex(path[index + 1]) ? [] : {};
+ }
+ }
+ nested = nested[key];
+ }
+ return object;
+}
+
+module.exports = set;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/transform.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/transform.js b/node_modules/lodash/object/transform.js
new file mode 100644
index 0000000..9a814b1
--- /dev/null
+++ b/node_modules/lodash/object/transform.js
@@ -0,0 +1,61 @@
+var arrayEach = require('../internal/arrayEach'),
+ baseCallback = require('../internal/baseCallback'),
+ baseCreate = require('../internal/baseCreate'),
+ baseForOwn = require('../internal/baseForOwn'),
+ isArray = require('../lang/isArray'),
+ isFunction = require('../lang/isFunction'),
+ isObject = require('../lang/isObject'),
+ isTypedArray = require('../lang/isTypedArray');
+
+/**
+ * An alternative to `_.reduce`; this method transforms `object` to a new
+ * `accumulator` object which is the result of running each of its own enumerable
+ * properties through `iteratee`, with each invocation potentially mutating
+ * the `accumulator` object. The `iteratee` is bound to `thisArg` and invoked
+ * with four arguments: (accumulator, value, key, object). Iteratee functions
+ * may exit iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Array|Object} object The object to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The custom accumulator value.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * _.transform([2, 3, 4], function(result, n) {
+ * result.push(n *= n);
+ * return n % 2 == 0;
+ * });
+ * // => [4, 9]
+ *
+ * _.transform({ 'a': 1, 'b': 2 }, function(result, n, key) {
+ * result[key] = n * 3;
+ * });
+ * // => { 'a': 3, 'b': 6 }
+ */
+function transform(object, iteratee, accumulator, thisArg) {
+ var isArr = isArray(object) || isTypedArray(object);
+ iteratee = baseCallback(iteratee, thisArg, 4);
+
+ if (accumulator == null) {
+ if (isArr || isObject(object)) {
+ var Ctor = object.constructor;
+ if (isArr) {
+ accumulator = isArray(object) ? new Ctor : [];
+ } else {
+ accumulator = baseCreate(isFunction(Ctor) ? Ctor.prototype : undefined);
+ }
+ } else {
+ accumulator = {};
+ }
+ }
+ (isArr ? arrayEach : baseForOwn)(object, function(value, index, object) {
+ return iteratee(accumulator, value, index, object);
+ });
+ return accumulator;
+}
+
+module.exports = transform;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/values.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/values.js b/node_modules/lodash/object/values.js
new file mode 100644
index 0000000..0171515
--- /dev/null
+++ b/node_modules/lodash/object/values.js
@@ -0,0 +1,33 @@
+var baseValues = require('../internal/baseValues'),
+ keys = require('./keys');
+
+/**
+ * Creates an array of the own enumerable property values of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property values.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.values(new Foo);
+ * // => [1, 2] (iteration order is not guaranteed)
+ *
+ * _.values('hi');
+ * // => ['h', 'i']
+ */
+function values(object) {
+ return baseValues(object, keys(object));
+}
+
+module.exports = values;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/object/valuesIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/object/valuesIn.js b/node_modules/lodash/object/valuesIn.js
new file mode 100644
index 0000000..5f067c0
--- /dev/null
+++ b/node_modules/lodash/object/valuesIn.js
@@ -0,0 +1,31 @@
+var baseValues = require('../internal/baseValues'),
+ keysIn = require('./keysIn');
+
+/**
+ * Creates an array of the own and inherited enumerable property values
+ * of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property values.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.valuesIn(new Foo);
+ * // => [1, 2, 3] (iteration order is not guaranteed)
+ */
+function valuesIn(object) {
+ return baseValues(object, keysIn(object));
+}
+
+module.exports = valuesIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/package.json
----------------------------------------------------------------------
diff --git a/node_modules/lodash/package.json b/node_modules/lodash/package.json
new file mode 100644
index 0000000..99a91e2
--- /dev/null
+++ b/node_modules/lodash/package.json
@@ -0,0 +1,121 @@
+{
+ "_args": [
+ [
+ "lodash@^3.5.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/xmlbuilder"
+ ]
+ ],
+ "_from": "lodash@>=3.5.0 <4.0.0",
+ "_id": "lodash@3.10.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/lodash",
+ "_nodeVersion": "0.12.5",
+ "_npmUser": {
+ "email": "john.david.dalton@gmail.com",
+ "name": "jdalton"
+ },
+ "_npmVersion": "2.13.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "lodash",
+ "raw": "lodash@^3.5.0",
+ "rawSpec": "^3.5.0",
+ "scope": null,
+ "spec": ">=3.5.0 <4.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/xmlbuilder"
+ ],
+ "_resolved": "http://registry.npmjs.org/lodash/-/lodash-3.10.1.tgz",
+ "_shasum": "5bf45e8e49ba4189e17d482789dfd15bd140b7b6",
+ "_shrinkwrap": null,
+ "_spec": "lodash@^3.5.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/xmlbuilder",
+ "author": {
+ "email": "john.david.dalton@gmail.com",
+ "name": "John-David Dalton",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "dependencies": {},
+ "description": "The modern build of lodash modular utilities.",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "5bf45e8e49ba4189e17d482789dfd15bd140b7b6",
+ "tarball": "http://registry.npmjs.org/lodash/-/lodash-3.10.1.tgz"
+ },
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "modules",
+ "stdlib",
+ "util"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "jdalton",
+ "email": "john.david.dalton@gmail.com"
+ },
+ {
+ "name": "mathias",
+ "email": "mathias@qiwi.be"
+ },
+ {
+ "name": "phated",
+ "email": "blaine@iceddev.com"
+ },
+ {
+ "name": "kitcambridge",
+ "email": "github@kitcambridge.be"
+ },
+ {
+ "name": "d10",
+ "email": "demoneaux@gmail.com"
+ }
+ ],
+ "name": "lodash",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.10.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string.js b/node_modules/lodash/string.js
new file mode 100644
index 0000000..f777945
--- /dev/null
+++ b/node_modules/lodash/string.js
@@ -0,0 +1,25 @@
+module.exports = {
+ 'camelCase': require('./string/camelCase'),
+ 'capitalize': require('./string/capitalize'),
+ 'deburr': require('./string/deburr'),
+ 'endsWith': require('./string/endsWith'),
+ 'escape': require('./string/escape'),
+ 'escapeRegExp': require('./string/escapeRegExp'),
+ 'kebabCase': require('./string/kebabCase'),
+ 'pad': require('./string/pad'),
+ 'padLeft': require('./string/padLeft'),
+ 'padRight': require('./string/padRight'),
+ 'parseInt': require('./string/parseInt'),
+ 'repeat': require('./string/repeat'),
+ 'snakeCase': require('./string/snakeCase'),
+ 'startCase': require('./string/startCase'),
+ 'startsWith': require('./string/startsWith'),
+ 'template': require('./string/template'),
+ 'templateSettings': require('./string/templateSettings'),
+ 'trim': require('./string/trim'),
+ 'trimLeft': require('./string/trimLeft'),
+ 'trimRight': require('./string/trimRight'),
+ 'trunc': require('./string/trunc'),
+ 'unescape': require('./string/unescape'),
+ 'words': require('./string/words')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/camelCase.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/camelCase.js b/node_modules/lodash/string/camelCase.js
new file mode 100644
index 0000000..2d438f4
--- /dev/null
+++ b/node_modules/lodash/string/camelCase.js
@@ -0,0 +1,27 @@
+var createCompounder = require('../internal/createCompounder');
+
+/**
+ * Converts `string` to [camel case](https://en.wikipedia.org/wiki/CamelCase).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the camel cased string.
+ * @example
+ *
+ * _.camelCase('Foo Bar');
+ * // => 'fooBar'
+ *
+ * _.camelCase('--foo-bar');
+ * // => 'fooBar'
+ *
+ * _.camelCase('__foo_bar__');
+ * // => 'fooBar'
+ */
+var camelCase = createCompounder(function(result, word, index) {
+ word = word.toLowerCase();
+ return result + (index ? (word.charAt(0).toUpperCase() + word.slice(1)) : word);
+});
+
+module.exports = camelCase;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/capitalize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/capitalize.js b/node_modules/lodash/string/capitalize.js
new file mode 100644
index 0000000..f9222dc
--- /dev/null
+++ b/node_modules/lodash/string/capitalize.js
@@ -0,0 +1,21 @@
+var baseToString = require('../internal/baseToString');
+
+/**
+ * Capitalizes the first character of `string`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to capitalize.
+ * @returns {string} Returns the capitalized string.
+ * @example
+ *
+ * _.capitalize('fred');
+ * // => 'Fred'
+ */
+function capitalize(string) {
+ string = baseToString(string);
+ return string && (string.charAt(0).toUpperCase() + string.slice(1));
+}
+
+module.exports = capitalize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/deburr.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/deburr.js b/node_modules/lodash/string/deburr.js
new file mode 100644
index 0000000..0bd03e6
--- /dev/null
+++ b/node_modules/lodash/string/deburr.js
@@ -0,0 +1,29 @@
+var baseToString = require('../internal/baseToString'),
+ deburrLetter = require('../internal/deburrLetter');
+
+/** Used to match [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks). */
+var reComboMark = /[\u0300-\u036f\ufe20-\ufe23]/g;
+
+/** Used to match latin-1 supplementary letters (excluding mathematical operators). */
+var reLatin1 = /[\xc0-\xd6\xd8-\xde\xdf-\xf6\xf8-\xff]/g;
+
+/**
+ * Deburrs `string` by converting [latin-1 supplementary letters](https://en.wikipedia.org/wiki/Latin-1_Supplement_(Unicode_block)#Character_table)
+ * to basic latin letters and removing [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to deburr.
+ * @returns {string} Returns the deburred string.
+ * @example
+ *
+ * _.deburr('déjà vu');
+ * // => 'deja vu'
+ */
+function deburr(string) {
+ string = baseToString(string);
+ return string && string.replace(reLatin1, deburrLetter).replace(reComboMark, '');
+}
+
+module.exports = deburr;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/endsWith.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/endsWith.js b/node_modules/lodash/string/endsWith.js
new file mode 100644
index 0000000..26821e2
--- /dev/null
+++ b/node_modules/lodash/string/endsWith.js
@@ -0,0 +1,40 @@
+var baseToString = require('../internal/baseToString');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Checks if `string` ends with the given target string.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to search.
+ * @param {string} [target] The string to search for.
+ * @param {number} [position=string.length] The position to search from.
+ * @returns {boolean} Returns `true` if `string` ends with `target`, else `false`.
+ * @example
+ *
+ * _.endsWith('abc', 'c');
+ * // => true
+ *
+ * _.endsWith('abc', 'b');
+ * // => false
+ *
+ * _.endsWith('abc', 'b', 2);
+ * // => true
+ */
+function endsWith(string, target, position) {
+ string = baseToString(string);
+ target = (target + '');
+
+ var length = string.length;
+ position = position === undefined
+ ? length
+ : nativeMin(position < 0 ? 0 : (+position || 0), length);
+
+ position -= target.length;
+ return position >= 0 && string.indexOf(target, position) == position;
+}
+
+module.exports = endsWith;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/escape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/escape.js b/node_modules/lodash/string/escape.js
new file mode 100644
index 0000000..cd08a5d
--- /dev/null
+++ b/node_modules/lodash/string/escape.js
@@ -0,0 +1,48 @@
+var baseToString = require('../internal/baseToString'),
+ escapeHtmlChar = require('../internal/escapeHtmlChar');
+
+/** Used to match HTML entities and HTML characters. */
+var reUnescapedHtml = /[&<>"'`]/g,
+ reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
+
+/**
+ * Converts the characters "&", "<", ">", '"', "'", and "\`", in `string` to
+ * their corresponding HTML entities.
+ *
+ * **Note:** No other characters are escaped. To escape additional characters
+ * use a third-party library like [_he_](https://mths.be/he).
+ *
+ * Though the ">" character is escaped for symmetry, characters like
+ * ">" and "/" don't need escaping in HTML and have no special meaning
+ * unless they're part of a tag or unquoted attribute value.
+ * See [Mathias Bynens's article](https://mathiasbynens.be/notes/ambiguous-ampersands)
+ * (under "semi-related fun fact") for more details.
+ *
+ * Backticks are escaped because in Internet Explorer < 9, they can break out
+ * of attribute values or HTML comments. See [#59](https://html5sec.org/#59),
+ * [#102](https://html5sec.org/#102), [#108](https://html5sec.org/#108), and
+ * [#133](https://html5sec.org/#133) of the [HTML5 Security Cheatsheet](https://html5sec.org/)
+ * for more details.
+ *
+ * When working with HTML you should always [quote attribute values](http://wonko.com/post/html-escaping)
+ * to reduce XSS vectors.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles'
+ */
+function escape(string) {
+ // Reset `lastIndex` because in IE < 9 `String#replace` does not.
+ string = baseToString(string);
+ return (string && reHasUnescapedHtml.test(string))
+ ? string.replace(reUnescapedHtml, escapeHtmlChar)
+ : string;
+}
+
+module.exports = escape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/escapeRegExp.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/escapeRegExp.js b/node_modules/lodash/string/escapeRegExp.js
new file mode 100644
index 0000000..176137a
--- /dev/null
+++ b/node_modules/lodash/string/escapeRegExp.js
@@ -0,0 +1,32 @@
+var baseToString = require('../internal/baseToString'),
+ escapeRegExpChar = require('../internal/escapeRegExpChar');
+
+/**
+ * Used to match `RegExp` [syntax characters](http://ecma-international.org/ecma-262/6.0/#sec-patterns)
+ * and those outlined by [`EscapeRegExpPattern`](http://ecma-international.org/ecma-262/6.0/#sec-escaperegexppattern).
+ */
+var reRegExpChars = /^[:!,]|[\\^$.*+?()[\]{}|\/]|(^[0-9a-fA-Fnrtuvx])|([\n\r\u2028\u2029])/g,
+ reHasRegExpChars = RegExp(reRegExpChars.source);
+
+/**
+ * Escapes the `RegExp` special characters "\", "/", "^", "$", ".", "|", "?",
+ * "*", "+", "(", ")", "[", "]", "{" and "}" in `string`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escapeRegExp('[lodash](https://lodash.com/)');
+ * // => '\[lodash\]\(https:\/\/lodash\.com\/\)'
+ */
+function escapeRegExp(string) {
+ string = baseToString(string);
+ return (string && reHasRegExpChars.test(string))
+ ? string.replace(reRegExpChars, escapeRegExpChar)
+ : (string || '(?:)');
+}
+
+module.exports = escapeRegExp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/kebabCase.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/kebabCase.js b/node_modules/lodash/string/kebabCase.js
new file mode 100644
index 0000000..d29c2f9
--- /dev/null
+++ b/node_modules/lodash/string/kebabCase.js
@@ -0,0 +1,26 @@
+var createCompounder = require('../internal/createCompounder');
+
+/**
+ * Converts `string` to [kebab case](https://en.wikipedia.org/wiki/Letter_case#Special_case_styles).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the kebab cased string.
+ * @example
+ *
+ * _.kebabCase('Foo Bar');
+ * // => 'foo-bar'
+ *
+ * _.kebabCase('fooBar');
+ * // => 'foo-bar'
+ *
+ * _.kebabCase('__foo_bar__');
+ * // => 'foo-bar'
+ */
+var kebabCase = createCompounder(function(result, word, index) {
+ return result + (index ? '-' : '') + word.toLowerCase();
+});
+
+module.exports = kebabCase;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/pad.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/pad.js b/node_modules/lodash/string/pad.js
new file mode 100644
index 0000000..60e523b
--- /dev/null
+++ b/node_modules/lodash/string/pad.js
@@ -0,0 +1,47 @@
+var baseToString = require('../internal/baseToString'),
+ createPadding = require('../internal/createPadding');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil,
+ nativeFloor = Math.floor,
+ nativeIsFinite = global.isFinite;
+
+/**
+ * Pads `string` on the left and right sides if it's shorter than `length`.
+ * Padding characters are truncated if they can't be evenly divided by `length`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to pad.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padded string.
+ * @example
+ *
+ * _.pad('abc', 8);
+ * // => ' abc '
+ *
+ * _.pad('abc', 8, '_-');
+ * // => '_-abc_-_'
+ *
+ * _.pad('abc', 3);
+ * // => 'abc'
+ */
+function pad(string, length, chars) {
+ string = baseToString(string);
+ length = +length;
+
+ var strLength = string.length;
+ if (strLength >= length || !nativeIsFinite(length)) {
+ return string;
+ }
+ var mid = (length - strLength) / 2,
+ leftLength = nativeFloor(mid),
+ rightLength = nativeCeil(mid);
+
+ chars = createPadding('', rightLength, chars);
+ return chars.slice(0, leftLength) + string + chars;
+}
+
+module.exports = pad;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/padLeft.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/padLeft.js b/node_modules/lodash/string/padLeft.js
new file mode 100644
index 0000000..bb0c94d
--- /dev/null
+++ b/node_modules/lodash/string/padLeft.js
@@ -0,0 +1,27 @@
+var createPadDir = require('../internal/createPadDir');
+
+/**
+ * Pads `string` on the left side if it's shorter than `length`. Padding
+ * characters are truncated if they exceed `length`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to pad.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padded string.
+ * @example
+ *
+ * _.padLeft('abc', 6);
+ * // => ' abc'
+ *
+ * _.padLeft('abc', 6, '_-');
+ * // => '_-_abc'
+ *
+ * _.padLeft('abc', 3);
+ * // => 'abc'
+ */
+var padLeft = createPadDir();
+
+module.exports = padLeft;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/padRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/padRight.js b/node_modules/lodash/string/padRight.js
new file mode 100644
index 0000000..dc12f55
--- /dev/null
+++ b/node_modules/lodash/string/padRight.js
@@ -0,0 +1,27 @@
+var createPadDir = require('../internal/createPadDir');
+
+/**
+ * Pads `string` on the right side if it's shorter than `length`. Padding
+ * characters are truncated if they exceed `length`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to pad.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the padded string.
+ * @example
+ *
+ * _.padRight('abc', 6);
+ * // => 'abc '
+ *
+ * _.padRight('abc', 6, '_-');
+ * // => 'abc_-_'
+ *
+ * _.padRight('abc', 3);
+ * // => 'abc'
+ */
+var padRight = createPadDir(true);
+
+module.exports = padRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/parseInt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/parseInt.js b/node_modules/lodash/string/parseInt.js
new file mode 100644
index 0000000..f457711
--- /dev/null
+++ b/node_modules/lodash/string/parseInt.js
@@ -0,0 +1,46 @@
+var isIterateeCall = require('../internal/isIterateeCall'),
+ trim = require('./trim');
+
+/** Used to detect hexadecimal string values. */
+var reHasHexPrefix = /^0[xX]/;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeParseInt = global.parseInt;
+
+/**
+ * Converts `string` to an integer of the specified radix. If `radix` is
+ * `undefined` or `0`, a `radix` of `10` is used unless `value` is a hexadecimal,
+ * in which case a `radix` of `16` is used.
+ *
+ * **Note:** This method aligns with the [ES5 implementation](https://es5.github.io/#E)
+ * of `parseInt`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} string The string to convert.
+ * @param {number} [radix] The radix to interpret `value` by.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {number} Returns the converted integer.
+ * @example
+ *
+ * _.parseInt('08');
+ * // => 8
+ *
+ * _.map(['6', '08', '10'], _.parseInt);
+ * // => [6, 8, 10]
+ */
+function parseInt(string, radix, guard) {
+ // Firefox < 21 and Opera < 15 follow ES3 for `parseInt`.
+ // Chrome fails to trim leading <BOM> whitespace characters.
+ // See https://code.google.com/p/v8/issues/detail?id=3109 for more details.
+ if (guard ? isIterateeCall(string, radix, guard) : radix == null) {
+ radix = 0;
+ } else if (radix) {
+ radix = +radix;
+ }
+ string = trim(string);
+ return nativeParseInt(string, radix || (reHasHexPrefix.test(string) ? 16 : 10));
+}
+
+module.exports = parseInt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/repeat.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/repeat.js b/node_modules/lodash/string/repeat.js
new file mode 100644
index 0000000..2902123
--- /dev/null
+++ b/node_modules/lodash/string/repeat.js
@@ -0,0 +1,47 @@
+var baseToString = require('../internal/baseToString');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor,
+ nativeIsFinite = global.isFinite;
+
+/**
+ * Repeats the given string `n` times.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to repeat.
+ * @param {number} [n=0] The number of times to repeat the string.
+ * @returns {string} Returns the repeated string.
+ * @example
+ *
+ * _.repeat('*', 3);
+ * // => '***'
+ *
+ * _.repeat('abc', 2);
+ * // => 'abcabc'
+ *
+ * _.repeat('abc', 0);
+ * // => ''
+ */
+function repeat(string, n) {
+ var result = '';
+ string = baseToString(string);
+ n = +n;
+ if (n < 1 || !string || !nativeIsFinite(n)) {
+ return result;
+ }
+ // Leverage the exponentiation by squaring algorithm for a faster repeat.
+ // See https://en.wikipedia.org/wiki/Exponentiation_by_squaring for more details.
+ do {
+ if (n % 2) {
+ result += string;
+ }
+ n = nativeFloor(n / 2);
+ string += string;
+ } while (n);
+
+ return result;
+}
+
+module.exports = repeat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/snakeCase.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/snakeCase.js b/node_modules/lodash/string/snakeCase.js
new file mode 100644
index 0000000..c9ebffd
--- /dev/null
+++ b/node_modules/lodash/string/snakeCase.js
@@ -0,0 +1,26 @@
+var createCompounder = require('../internal/createCompounder');
+
+/**
+ * Converts `string` to [snake case](https://en.wikipedia.org/wiki/Snake_case).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the snake cased string.
+ * @example
+ *
+ * _.snakeCase('Foo Bar');
+ * // => 'foo_bar'
+ *
+ * _.snakeCase('fooBar');
+ * // => 'foo_bar'
+ *
+ * _.snakeCase('--foo-bar');
+ * // => 'foo_bar'
+ */
+var snakeCase = createCompounder(function(result, word, index) {
+ return result + (index ? '_' : '') + word.toLowerCase();
+});
+
+module.exports = snakeCase;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/startCase.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/startCase.js b/node_modules/lodash/string/startCase.js
new file mode 100644
index 0000000..740d48a
--- /dev/null
+++ b/node_modules/lodash/string/startCase.js
@@ -0,0 +1,26 @@
+var createCompounder = require('../internal/createCompounder');
+
+/**
+ * Converts `string` to [start case](https://en.wikipedia.org/wiki/Letter_case#Stylistic_or_specialised_usage).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to convert.
+ * @returns {string} Returns the start cased string.
+ * @example
+ *
+ * _.startCase('--foo-bar');
+ * // => 'Foo Bar'
+ *
+ * _.startCase('fooBar');
+ * // => 'Foo Bar'
+ *
+ * _.startCase('__foo_bar__');
+ * // => 'Foo Bar'
+ */
+var startCase = createCompounder(function(result, word, index) {
+ return result + (index ? ' ' : '') + (word.charAt(0).toUpperCase() + word.slice(1));
+});
+
+module.exports = startCase;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/startsWith.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/startsWith.js b/node_modules/lodash/string/startsWith.js
new file mode 100644
index 0000000..65fae2a
--- /dev/null
+++ b/node_modules/lodash/string/startsWith.js
@@ -0,0 +1,36 @@
+var baseToString = require('../internal/baseToString');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Checks if `string` starts with the given target string.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to search.
+ * @param {string} [target] The string to search for.
+ * @param {number} [position=0] The position to search from.
+ * @returns {boolean} Returns `true` if `string` starts with `target`, else `false`.
+ * @example
+ *
+ * _.startsWith('abc', 'a');
+ * // => true
+ *
+ * _.startsWith('abc', 'b');
+ * // => false
+ *
+ * _.startsWith('abc', 'b', 1);
+ * // => true
+ */
+function startsWith(string, target, position) {
+ string = baseToString(string);
+ position = position == null
+ ? 0
+ : nativeMin(position < 0 ? 0 : (+position || 0), string.length);
+
+ return string.lastIndexOf(target, position) == position;
+}
+
+module.exports = startsWith;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[47/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/README.md b/node_modules/cordova-common/node_modules/shelljs/README.md
new file mode 100644
index 0000000..d08d13e
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/README.md
@@ -0,0 +1,579 @@
+# ShellJS - Unix shell commands for Node.js [](http://travis-ci.org/arturadib/shelljs)
+
+ShellJS is a portable **(Windows/Linux/OS X)** implementation of Unix shell commands on top of the Node.js API. You can use it to eliminate your shell script's dependency on Unix while still keeping its familiar and powerful commands. You can also install it globally so you can run it from outside Node projects - say goodbye to those gnarly Bash scripts!
+
+The project is [unit-tested](http://travis-ci.org/arturadib/shelljs) and battled-tested in projects like:
+
++ [PDF.js](http://github.com/mozilla/pdf.js) - Firefox's next-gen PDF reader
++ [Firebug](http://getfirebug.com/) - Firefox's infamous debugger
++ [JSHint](http://jshint.com) - Most popular JavaScript linter
++ [Zepto](http://zeptojs.com) - jQuery-compatible JavaScript library for modern browsers
++ [Yeoman](http://yeoman.io/) - Web application stack and development tool
++ [Deployd.com](http://deployd.com) - Open source PaaS for quick API backend generation
+
+and [many more](https://npmjs.org/browse/depended/shelljs).
+
+Connect with [@r2r](http://twitter.com/r2r) on Twitter for questions, suggestions, etc.
+
+## Installing
+
+Via npm:
+
+```bash
+$ npm install [-g] shelljs
+```
+
+If the global option `-g` is specified, the binary `shjs` will be installed. This makes it possible to
+run ShellJS scripts much like any shell script from the command line, i.e. without requiring a `node_modules` folder:
+
+```bash
+$ shjs my_script
+```
+
+You can also just copy `shell.js` into your project's directory, and `require()` accordingly.
+
+
+## Examples
+
+### JavaScript
+
+```javascript
+require('shelljs/global');
+
+if (!which('git')) {
+ echo('Sorry, this script requires git');
+ exit(1);
+}
+
+// Copy files to release dir
+mkdir('-p', 'out/Release');
+cp('-R', 'stuff/*', 'out/Release');
+
+// Replace macros in each .js file
+cd('lib');
+ls('*.js').forEach(function(file) {
+ sed('-i', 'BUILD_VERSION', 'v0.1.2', file);
+ sed('-i', /.*REMOVE_THIS_LINE.*\n/, '', file);
+ sed('-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file);
+});
+cd('..');
+
+// Run external tool synchronously
+if (exec('git commit -am "Auto-commit"').code !== 0) {
+ echo('Error: Git commit failed');
+ exit(1);
+}
+```
+
+### CoffeeScript
+
+```coffeescript
+require 'shelljs/global'
+
+if not which 'git'
+ echo 'Sorry, this script requires git'
+ exit 1
+
+# Copy files to release dir
+mkdir '-p', 'out/Release'
+cp '-R', 'stuff/*', 'out/Release'
+
+# Replace macros in each .js file
+cd 'lib'
+for file in ls '*.js'
+ sed '-i', 'BUILD_VERSION', 'v0.1.2', file
+ sed '-i', /.*REMOVE_THIS_LINE.*\n/, '', file
+ sed '-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat 'macro.js', file
+cd '..'
+
+# Run external tool synchronously
+if (exec 'git commit -am "Auto-commit"').code != 0
+ echo 'Error: Git commit failed'
+ exit 1
+```
+
+## Global vs. Local
+
+The example above uses the convenience script `shelljs/global` to reduce verbosity. If polluting your global namespace is not desirable, simply require `shelljs`.
+
+Example:
+
+```javascript
+var shell = require('shelljs');
+shell.echo('hello world');
+```
+
+## Make tool
+
+A convenience script `shelljs/make` is also provided to mimic the behavior of a Unix Makefile. In this case all shell objects are global, and command line arguments will cause the script to execute only the corresponding function in the global `target` object. To avoid redundant calls, target functions are executed only once per script.
+
+Example (CoffeeScript):
+
+```coffeescript
+require 'shelljs/make'
+
+target.all = ->
+ target.bundle()
+ target.docs()
+
+target.bundle = ->
+ cd __dirname
+ mkdir 'build'
+ cd 'lib'
+ (cat '*.js').to '../build/output.js'
+
+target.docs = ->
+ cd __dirname
+ mkdir 'docs'
+ cd 'lib'
+ for file in ls '*.js'
+ text = grep '//@', file # extract special comments
+ text.replace '//@', '' # remove comment tags
+ text.to 'docs/my_docs.md'
+```
+
+To run the target `all`, call the above script without arguments: `$ node make`. To run the target `docs`: `$ node make docs`.
+
+You can also pass arguments to your targets by using the `--` separator. For example, to pass `arg1` and `arg2` to a target `bundle`, do `$ node make bundle -- arg1 arg2`:
+
+```javascript
+require('shelljs/make');
+
+target.bundle = function(argsArray) {
+ // argsArray = ['arg1', 'arg2']
+ /* ... */
+}
+```
+
+
+<!-- DO NOT MODIFY BEYOND THIS POINT - IT'S AUTOMATICALLY GENERATED -->
+
+
+## Command reference
+
+
+All commands run synchronously, unless otherwise stated.
+
+
+### cd('dir')
+Changes to directory `dir` for the duration of the script
+
+
+### pwd()
+Returns the current directory.
+
+
+### ls([options ,] path [,path ...])
+### ls([options ,] path_array)
+Available options:
+
++ `-R`: recursive
++ `-A`: all files (include files beginning with `.`, except for `.` and `..`)
+
+Examples:
+
+```javascript
+ls('projs/*.js');
+ls('-R', '/users/me', '/tmp');
+ls('-R', ['/users/me', '/tmp']); // same as above
+```
+
+Returns array of files in the given path, or in current directory if no path provided.
+
+
+### find(path [,path ...])
+### find(path_array)
+Examples:
+
+```javascript
+find('src', 'lib');
+find(['src', 'lib']); // same as above
+find('.').filter(function(file) { return file.match(/\.js$/); });
+```
+
+Returns array of all files (however deep) in the given paths.
+
+The main difference from `ls('-R', path)` is that the resulting file names
+include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
+
+
+### cp([options ,] source [,source ...], dest)
+### cp([options ,] source_array, dest)
+Available options:
+
++ `-f`: force
++ `-r, -R`: recursive
+
+Examples:
+
+```javascript
+cp('file1', 'dir1');
+cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
+cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
+```
+
+Copies files. The wildcard `*` is accepted.
+
+
+### rm([options ,] file [, file ...])
+### rm([options ,] file_array)
+Available options:
+
++ `-f`: force
++ `-r, -R`: recursive
+
+Examples:
+
+```javascript
+rm('-rf', '/tmp/*');
+rm('some_file.txt', 'another_file.txt');
+rm(['some_file.txt', 'another_file.txt']); // same as above
+```
+
+Removes files. The wildcard `*` is accepted.
+
+
+### mv(source [, source ...], dest')
+### mv(source_array, dest')
+Available options:
+
++ `f`: force
+
+Examples:
+
+```javascript
+mv('-f', 'file', 'dir/');
+mv('file1', 'file2', 'dir/');
+mv(['file1', 'file2'], 'dir/'); // same as above
+```
+
+Moves files. The wildcard `*` is accepted.
+
+
+### mkdir([options ,] dir [, dir ...])
+### mkdir([options ,] dir_array)
+Available options:
+
++ `p`: full path (will create intermediate dirs if necessary)
+
+Examples:
+
+```javascript
+mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
+mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
+```
+
+Creates directories.
+
+
+### test(expression)
+Available expression primaries:
+
++ `'-b', 'path'`: true if path is a block device
++ `'-c', 'path'`: true if path is a character device
++ `'-d', 'path'`: true if path is a directory
++ `'-e', 'path'`: true if path exists
++ `'-f', 'path'`: true if path is a regular file
++ `'-L', 'path'`: true if path is a symbolic link
++ `'-p', 'path'`: true if path is a pipe (FIFO)
++ `'-S', 'path'`: true if path is a socket
+
+Examples:
+
+```javascript
+if (test('-d', path)) { /* do something with dir */ };
+if (!test('-f', path)) continue; // skip if it's a regular file
+```
+
+Evaluates expression using the available primaries and returns corresponding value.
+
+
+### cat(file [, file ...])
+### cat(file_array)
+
+Examples:
+
+```javascript
+var str = cat('file*.txt');
+var str = cat('file1', 'file2');
+var str = cat(['file1', 'file2']); // same as above
+```
+
+Returns a string containing the given file, or a concatenated string
+containing the files if more than one file is given (a new line character is
+introduced between each file). Wildcard `*` accepted.
+
+
+### 'string'.to(file)
+
+Examples:
+
+```javascript
+cat('input.txt').to('output.txt');
+```
+
+Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
+those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
+
+
+### 'string'.toEnd(file)
+
+Examples:
+
+```javascript
+cat('input.txt').toEnd('output.txt');
+```
+
+Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
+those returned by `cat`, `grep`, etc).
+
+
+### sed([options ,] search_regex, replacement, file)
+Available options:
+
++ `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
+
+Examples:
+
+```javascript
+sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
+sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
+```
+
+Reads an input string from `file` and performs a JavaScript `replace()` on the input
+using the given search regex and replacement string or function. Returns the new string after replacement.
+
+
+### grep([options ,] regex_filter, file [, file ...])
+### grep([options ,] regex_filter, file_array)
+Available options:
+
++ `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
+
+Examples:
+
+```javascript
+grep('-v', 'GLOBAL_VARIABLE', '*.js');
+grep('GLOBAL_VARIABLE', '*.js');
+```
+
+Reads input string from given files and returns a string containing all lines of the
+file that match the given `regex_filter`. Wildcard `*` accepted.
+
+
+### which(command)
+
+Examples:
+
+```javascript
+var nodeExec = which('node');
+```
+
+Searches for `command` in the system's PATH. On Windows looks for `.exe`, `.cmd`, and `.bat` extensions.
+Returns string containing the absolute path to the command.
+
+
+### echo(string [,string ...])
+
+Examples:
+
+```javascript
+echo('hello world');
+var str = echo('hello world');
+```
+
+Prints string to stdout, and returns string with additional utility methods
+like `.to()`.
+
+
+### pushd([options,] [dir | '-N' | '+N'])
+
+Available options:
+
++ `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
+
+Arguments:
+
++ `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
++ `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
++ `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
+
+Examples:
+
+```javascript
+// process.cwd() === '/usr'
+pushd('/etc'); // Returns /etc /usr
+pushd('+1'); // Returns /usr /etc
+```
+
+Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
+
+### popd([options,] ['-N' | '+N'])
+
+Available options:
+
++ `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
+
+Arguments:
+
++ `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
++ `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
+
+Examples:
+
+```javascript
+echo(process.cwd()); // '/usr'
+pushd('/etc'); // '/etc /usr'
+echo(process.cwd()); // '/etc'
+popd(); // '/usr'
+echo(process.cwd()); // '/usr'
+```
+
+When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
+
+### dirs([options | '+N' | '-N'])
+
+Available options:
+
++ `-c`: Clears the directory stack by deleting all of the elements.
+
+Arguments:
+
++ `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
++ `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
+
+Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
+
+See also: pushd, popd
+
+
+### ln(options, source, dest)
+### ln(source, dest)
+Available options:
+
++ `s`: symlink
++ `f`: force
+
+Examples:
+
+```javascript
+ln('file', 'newlink');
+ln('-sf', 'file', 'existing');
+```
+
+Links source to dest. Use -f to force the link, should dest already exist.
+
+
+### exit(code)
+Exits the current process with the given exit code.
+
+### env['VAR_NAME']
+Object containing environment variables (both getter and setter). Shortcut to process.env.
+
+### exec(command [, options] [, callback])
+Available options (all `false` by default):
+
++ `async`: Asynchronous execution. Defaults to true if a callback is provided.
++ `silent`: Do not echo program output to console.
+
+Examples:
+
+```javascript
+var version = exec('node --version', {silent:true}).output;
+
+var child = exec('some_long_running_process', {async:true});
+child.stdout.on('data', function(data) {
+ /* ... do something with data ... */
+});
+
+exec('some_long_running_process', function(code, output) {
+ console.log('Exit code:', code);
+ console.log('Program output:', output);
+});
+```
+
+Executes the given `command` _synchronously_, unless otherwise specified.
+When in synchronous mode returns the object `{ code:..., output:... }`, containing the program's
+`output` (stdout + stderr) and its exit `code`. Otherwise returns the child process object, and
+the `callback` gets the arguments `(code, output)`.
+
+**Note:** For long-lived processes, it's best to run `exec()` asynchronously as
+the current synchronous implementation uses a lot of CPU. This should be getting
+fixed soon.
+
+
+### chmod(octal_mode || octal_string, file)
+### chmod(symbolic_mode, file)
+
+Available options:
+
++ `-v`: output a diagnostic for every file processed
++ `-c`: like verbose but report only when a change is made
++ `-R`: change files and directories recursively
+
+Examples:
+
+```javascript
+chmod(755, '/Users/brandon');
+chmod('755', '/Users/brandon'); // same as above
+chmod('u+x', '/Users/brandon');
+```
+
+Alters the permissions of a file or directory by either specifying the
+absolute permissions in octal form or expressing the changes in symbols.
+This command tries to mimic the POSIX behavior as much as possible.
+Notable exceptions:
+
++ In symbolic modes, 'a-r' and '-r' are identical. No consideration is
+ given to the umask.
++ There is no "quiet" option since default behavior is to run silent.
+
+
+## Non-Unix commands
+
+
+### tempdir()
+
+Examples:
+
+```javascript
+var tmp = tempdir(); // "/tmp" for most *nix platforms
+```
+
+Searches and returns string containing a writeable, platform-dependent temporary directory.
+Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
+
+
+### error()
+Tests if error occurred in the last command. Returns `null` if no error occurred,
+otherwise returns string explaining the error
+
+
+## Configuration
+
+
+### config.silent
+Example:
+
+```javascript
+var sh = require('shelljs');
+var silentState = sh.config.silent; // save old silent state
+sh.config.silent = true;
+/* ... */
+sh.config.silent = silentState; // restore old silent state
+```
+
+Suppresses all command output if `true`, except for `echo()` calls.
+Default is `false`.
+
+### config.fatal
+Example:
+
+```javascript
+require('shelljs/global');
+config.fatal = true;
+cp('this_file_does_not_exist', '/dev/null'); // dies here
+/* more commands... */
+```
+
+If `true` the script will die on errors. Default is `false`.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/RELEASE.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/RELEASE.md b/node_modules/cordova-common/node_modules/shelljs/RELEASE.md
new file mode 100644
index 0000000..69ef3fb
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/RELEASE.md
@@ -0,0 +1,9 @@
+# Release steps
+
+* Ensure master passes CI tests
+* Bump version in package.json. Any breaking change or new feature should bump minor (or even major). Non-breaking changes or fixes can just bump patch.
+* Update README manually if the changes are not documented in-code. If so, run `scripts/generate-docs.js`
+* Commit
+* `$ git tag <version>` (see `git tag -l` for latest)
+* `$ git push origin master --tags`
+* `$ npm publish .`
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/bin/shjs
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/bin/shjs b/node_modules/cordova-common/node_modules/shelljs/bin/shjs
new file mode 100755
index 0000000..d239a7a
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/bin/shjs
@@ -0,0 +1,51 @@
+#!/usr/bin/env node
+require('../global');
+
+if (process.argv.length < 3) {
+ console.log('ShellJS: missing argument (script name)');
+ console.log();
+ process.exit(1);
+}
+
+var args,
+ scriptName = process.argv[2];
+env['NODE_PATH'] = __dirname + '/../..';
+
+if (!scriptName.match(/\.js/) && !scriptName.match(/\.coffee/)) {
+ if (test('-f', scriptName + '.js'))
+ scriptName += '.js';
+ if (test('-f', scriptName + '.coffee'))
+ scriptName += '.coffee';
+}
+
+if (!test('-f', scriptName)) {
+ console.log('ShellJS: script not found ('+scriptName+')');
+ console.log();
+ process.exit(1);
+}
+
+args = process.argv.slice(3);
+
+for (var i = 0, l = args.length; i < l; i++) {
+ if (args[i][0] !== "-"){
+ args[i] = '"' + args[i] + '"'; // fixes arguments with multiple words
+ }
+}
+
+if (scriptName.match(/\.coffee$/)) {
+ //
+ // CoffeeScript
+ //
+ if (which('coffee')) {
+ exec('coffee ' + scriptName + ' ' + args.join(' '), { async: true });
+ } else {
+ console.log('ShellJS: CoffeeScript interpreter not found');
+ console.log();
+ process.exit(1);
+ }
+} else {
+ //
+ // JavaScript
+ //
+ exec('node ' + scriptName + ' ' + args.join(' '), { async: true });
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/global.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/global.js b/node_modules/cordova-common/node_modules/shelljs/global.js
new file mode 100644
index 0000000..97f0033
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/global.js
@@ -0,0 +1,3 @@
+var shell = require('./shell.js');
+for (var cmd in shell)
+ global[cmd] = shell[cmd];
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/make.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/make.js b/node_modules/cordova-common/node_modules/shelljs/make.js
new file mode 100644
index 0000000..f78b4cf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/make.js
@@ -0,0 +1,56 @@
+require('./global');
+
+global.config.fatal = true;
+global.target = {};
+
+var args = process.argv.slice(2),
+ targetArgs,
+ dashesLoc = args.indexOf('--');
+
+// split args, everything after -- if only for targets
+if (dashesLoc > -1) {
+ targetArgs = args.slice(dashesLoc + 1, args.length);
+ args = args.slice(0, dashesLoc);
+}
+
+// This ensures we only execute the script targets after the entire script has
+// been evaluated
+setTimeout(function() {
+ var t;
+
+ if (args.length === 1 && args[0] === '--help') {
+ console.log('Available targets:');
+ for (t in global.target)
+ console.log(' ' + t);
+ return;
+ }
+
+ // Wrap targets to prevent duplicate execution
+ for (t in global.target) {
+ (function(t, oldTarget){
+
+ // Wrap it
+ global.target[t] = function() {
+ if (oldTarget.done)
+ return;
+ oldTarget.done = true;
+ return oldTarget.apply(oldTarget, arguments);
+ };
+
+ })(t, global.target[t]);
+ }
+
+ // Execute desired targets
+ if (args.length > 0) {
+ args.forEach(function(arg) {
+ if (arg in global.target)
+ global.target[arg](targetArgs);
+ else {
+ console.log('no such target: ' + arg);
+ }
+ });
+ } else if ('all' in global.target) {
+ global.target.all(targetArgs);
+ }
+
+}, 0);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/package.json b/node_modules/cordova-common/node_modules/shelljs/package.json
new file mode 100644
index 0000000..f6f61cc
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/package.json
@@ -0,0 +1,88 @@
+{
+ "_args": [
+ [
+ "shelljs@^0.5.1",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "shelljs@>=0.5.1 <0.6.0",
+ "_id": "shelljs@0.5.3",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/cordova-common/shelljs",
+ "_nodeVersion": "1.2.0",
+ "_npmUser": {
+ "email": "arturadib@gmail.com",
+ "name": "artur"
+ },
+ "_npmVersion": "2.5.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "shelljs",
+ "raw": "shelljs@^0.5.1",
+ "rawSpec": "^0.5.1",
+ "scope": null,
+ "spec": ">=0.5.1 <0.6.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/shelljs/-/shelljs-0.5.3.tgz",
+ "_shasum": "c54982b996c76ef0c1e6b59fbdc5825f5b713113",
+ "_shrinkwrap": null,
+ "_spec": "shelljs@^0.5.1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "email": "arturadib@gmail.com",
+ "name": "Artur Adib"
+ },
+ "bin": {
+ "shjs": "./bin/shjs"
+ },
+ "bugs": {
+ "url": "https://github.com/arturadib/shelljs/issues"
+ },
+ "dependencies": {},
+ "description": "Portable Unix shell commands for Node.js",
+ "devDependencies": {
+ "jshint": "~2.1.11"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "c54982b996c76ef0c1e6b59fbdc5825f5b713113",
+ "tarball": "http://registry.npmjs.org/shelljs/-/shelljs-0.5.3.tgz"
+ },
+ "engines": {
+ "node": ">=0.8.0"
+ },
+ "gitHead": "22d0975040b9b8234755dc6e692d6869436e8485",
+ "homepage": "http://github.com/arturadib/shelljs",
+ "keywords": [
+ "jake",
+ "make",
+ "makefile",
+ "shell",
+ "synchronous",
+ "unix"
+ ],
+ "license": "BSD*",
+ "main": "./shell.js",
+ "maintainers": [
+ {
+ "name": "artur",
+ "email": "arturadib@gmail.com"
+ }
+ ],
+ "name": "shelljs",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/arturadib/shelljs.git"
+ },
+ "scripts": {
+ "test": "node scripts/run-tests"
+ },
+ "version": "0.5.3"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js b/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js
new file mode 100755
index 0000000..532fed9
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js
@@ -0,0 +1,21 @@
+#!/usr/bin/env node
+require('../global');
+
+echo('Appending docs to README.md');
+
+cd(__dirname + '/..');
+
+// Extract docs from shell.js
+var docs = grep('//@', 'shell.js');
+
+docs = docs.replace(/\/\/\@include (.+)/g, function(match, path) {
+ var file = path.match('.js$') ? path : path+'.js';
+ return grep('//@', file);
+});
+
+// Remove '//@'
+docs = docs.replace(/\/\/\@ ?/g, '');
+// Append docs to README
+sed('-i', /## Command reference(.|\n)*/, '## Command reference\n\n' + docs, 'README.md');
+
+echo('All done.');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js b/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js
new file mode 100755
index 0000000..f9d31e0
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js
@@ -0,0 +1,50 @@
+#!/usr/bin/env node
+require('../global');
+
+var path = require('path');
+
+var failed = false;
+
+//
+// Lint
+//
+JSHINT_BIN = './node_modules/jshint/bin/jshint';
+cd(__dirname + '/..');
+
+if (!test('-f', JSHINT_BIN)) {
+ echo('JSHint not found. Run `npm install` in the root dir first.');
+ exit(1);
+}
+
+if (exec(JSHINT_BIN + ' *.js test/*.js').code !== 0) {
+ failed = true;
+ echo('*** JSHINT FAILED! (return code != 0)');
+ echo();
+} else {
+ echo('All JSHint tests passed');
+ echo();
+}
+
+//
+// Unit tests
+//
+cd(__dirname + '/../test');
+ls('*.js').forEach(function(file) {
+ echo('Running test:', file);
+ if (exec('node ' + file).code !== 123) { // 123 avoids false positives (e.g. premature exit)
+ failed = true;
+ echo('*** TEST FAILED! (missing exit code "123")');
+ echo();
+ }
+});
+
+if (failed) {
+ echo();
+ echo('*******************************************************');
+ echo('WARNING: Some tests did not pass!');
+ echo('*******************************************************');
+ exit(1);
+} else {
+ echo();
+ echo('All tests passed.');
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/shell.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/shell.js b/node_modules/cordova-common/node_modules/shelljs/shell.js
new file mode 100644
index 0000000..bdeb559
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/shell.js
@@ -0,0 +1,159 @@
+//
+// ShellJS
+// Unix shell commands on top of Node's API
+//
+// Copyright (c) 2012 Artur Adib
+// http://github.com/arturadib/shelljs
+//
+
+var common = require('./src/common');
+
+
+//@
+//@ All commands run synchronously, unless otherwise stated.
+//@
+
+//@include ./src/cd
+var _cd = require('./src/cd');
+exports.cd = common.wrap('cd', _cd);
+
+//@include ./src/pwd
+var _pwd = require('./src/pwd');
+exports.pwd = common.wrap('pwd', _pwd);
+
+//@include ./src/ls
+var _ls = require('./src/ls');
+exports.ls = common.wrap('ls', _ls);
+
+//@include ./src/find
+var _find = require('./src/find');
+exports.find = common.wrap('find', _find);
+
+//@include ./src/cp
+var _cp = require('./src/cp');
+exports.cp = common.wrap('cp', _cp);
+
+//@include ./src/rm
+var _rm = require('./src/rm');
+exports.rm = common.wrap('rm', _rm);
+
+//@include ./src/mv
+var _mv = require('./src/mv');
+exports.mv = common.wrap('mv', _mv);
+
+//@include ./src/mkdir
+var _mkdir = require('./src/mkdir');
+exports.mkdir = common.wrap('mkdir', _mkdir);
+
+//@include ./src/test
+var _test = require('./src/test');
+exports.test = common.wrap('test', _test);
+
+//@include ./src/cat
+var _cat = require('./src/cat');
+exports.cat = common.wrap('cat', _cat);
+
+//@include ./src/to
+var _to = require('./src/to');
+String.prototype.to = common.wrap('to', _to);
+
+//@include ./src/toEnd
+var _toEnd = require('./src/toEnd');
+String.prototype.toEnd = common.wrap('toEnd', _toEnd);
+
+//@include ./src/sed
+var _sed = require('./src/sed');
+exports.sed = common.wrap('sed', _sed);
+
+//@include ./src/grep
+var _grep = require('./src/grep');
+exports.grep = common.wrap('grep', _grep);
+
+//@include ./src/which
+var _which = require('./src/which');
+exports.which = common.wrap('which', _which);
+
+//@include ./src/echo
+var _echo = require('./src/echo');
+exports.echo = _echo; // don't common.wrap() as it could parse '-options'
+
+//@include ./src/dirs
+var _dirs = require('./src/dirs').dirs;
+exports.dirs = common.wrap("dirs", _dirs);
+var _pushd = require('./src/dirs').pushd;
+exports.pushd = common.wrap('pushd', _pushd);
+var _popd = require('./src/dirs').popd;
+exports.popd = common.wrap("popd", _popd);
+
+//@include ./src/ln
+var _ln = require('./src/ln');
+exports.ln = common.wrap('ln', _ln);
+
+//@
+//@ ### exit(code)
+//@ Exits the current process with the given exit code.
+exports.exit = process.exit;
+
+//@
+//@ ### env['VAR_NAME']
+//@ Object containing environment variables (both getter and setter). Shortcut to process.env.
+exports.env = process.env;
+
+//@include ./src/exec
+var _exec = require('./src/exec');
+exports.exec = common.wrap('exec', _exec, {notUnix:true});
+
+//@include ./src/chmod
+var _chmod = require('./src/chmod');
+exports.chmod = common.wrap('chmod', _chmod);
+
+
+
+//@
+//@ ## Non-Unix commands
+//@
+
+//@include ./src/tempdir
+var _tempDir = require('./src/tempdir');
+exports.tempdir = common.wrap('tempdir', _tempDir);
+
+
+//@include ./src/error
+var _error = require('./src/error');
+exports.error = _error;
+
+
+
+//@
+//@ ## Configuration
+//@
+
+exports.config = common.config;
+
+//@
+//@ ### config.silent
+//@ Example:
+//@
+//@ ```javascript
+//@ var sh = require('shelljs');
+//@ var silentState = sh.config.silent; // save old silent state
+//@ sh.config.silent = true;
+//@ /* ... */
+//@ sh.config.silent = silentState; // restore old silent state
+//@ ```
+//@
+//@ Suppresses all command output if `true`, except for `echo()` calls.
+//@ Default is `false`.
+
+//@
+//@ ### config.fatal
+//@ Example:
+//@
+//@ ```javascript
+//@ require('shelljs/global');
+//@ config.fatal = true;
+//@ cp('this_file_does_not_exist', '/dev/null'); // dies here
+//@ /* more commands... */
+//@ ```
+//@
+//@ If `true` the script will die on errors. Default is `false`.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/cat.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/cat.js b/node_modules/cordova-common/node_modules/shelljs/src/cat.js
new file mode 100644
index 0000000..f6f4d25
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/cat.js
@@ -0,0 +1,43 @@
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### cat(file [, file ...])
+//@ ### cat(file_array)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var str = cat('file*.txt');
+//@ var str = cat('file1', 'file2');
+//@ var str = cat(['file1', 'file2']); // same as above
+//@ ```
+//@
+//@ Returns a string containing the given file, or a concatenated string
+//@ containing the files if more than one file is given (a new line character is
+//@ introduced between each file). Wildcard `*` accepted.
+function _cat(options, files) {
+ var cat = '';
+
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ files.forEach(function(file) {
+ if (!fs.existsSync(file))
+ common.error('no such file or directory: ' + file);
+
+ cat += fs.readFileSync(file, 'utf8') + '\n';
+ });
+
+ if (cat[cat.length-1] === '\n')
+ cat = cat.substring(0, cat.length-1);
+
+ return common.ShellString(cat);
+}
+module.exports = _cat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/cd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/cd.js b/node_modules/cordova-common/node_modules/shelljs/src/cd.js
new file mode 100644
index 0000000..230f432
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/cd.js
@@ -0,0 +1,19 @@
+var fs = require('fs');
+var common = require('./common');
+
+//@
+//@ ### cd('dir')
+//@ Changes to directory `dir` for the duration of the script
+function _cd(options, dir) {
+ if (!dir)
+ common.error('directory not specified');
+
+ if (!fs.existsSync(dir))
+ common.error('no such file or directory: ' + dir);
+
+ if (!fs.statSync(dir).isDirectory())
+ common.error('not a directory: ' + dir);
+
+ process.chdir(dir);
+}
+module.exports = _cd;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/chmod.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/chmod.js b/node_modules/cordova-common/node_modules/shelljs/src/chmod.js
new file mode 100644
index 0000000..f288893
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/chmod.js
@@ -0,0 +1,208 @@
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+var PERMS = (function (base) {
+ return {
+ OTHER_EXEC : base.EXEC,
+ OTHER_WRITE : base.WRITE,
+ OTHER_READ : base.READ,
+
+ GROUP_EXEC : base.EXEC << 3,
+ GROUP_WRITE : base.WRITE << 3,
+ GROUP_READ : base.READ << 3,
+
+ OWNER_EXEC : base.EXEC << 6,
+ OWNER_WRITE : base.WRITE << 6,
+ OWNER_READ : base.READ << 6,
+
+ // Literal octal numbers are apparently not allowed in "strict" javascript. Using parseInt is
+ // the preferred way, else a jshint warning is thrown.
+ STICKY : parseInt('01000', 8),
+ SETGID : parseInt('02000', 8),
+ SETUID : parseInt('04000', 8),
+
+ TYPE_MASK : parseInt('0770000', 8)
+ };
+})({
+ EXEC : 1,
+ WRITE : 2,
+ READ : 4
+});
+
+//@
+//@ ### chmod(octal_mode || octal_string, file)
+//@ ### chmod(symbolic_mode, file)
+//@
+//@ Available options:
+//@
+//@ + `-v`: output a diagnostic for every file processed//@
+//@ + `-c`: like verbose but report only when a change is made//@
+//@ + `-R`: change files and directories recursively//@
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ chmod(755, '/Users/brandon');
+//@ chmod('755', '/Users/brandon'); // same as above
+//@ chmod('u+x', '/Users/brandon');
+//@ ```
+//@
+//@ Alters the permissions of a file or directory by either specifying the
+//@ absolute permissions in octal form or expressing the changes in symbols.
+//@ This command tries to mimic the POSIX behavior as much as possible.
+//@ Notable exceptions:
+//@
+//@ + In symbolic modes, 'a-r' and '-r' are identical. No consideration is
+//@ given to the umask.
+//@ + There is no "quiet" option since default behavior is to run silent.
+function _chmod(options, mode, filePattern) {
+ if (!filePattern) {
+ if (options.length > 0 && options.charAt(0) === '-') {
+ // Special case where the specified file permissions started with - to subtract perms, which
+ // get picked up by the option parser as command flags.
+ // If we are down by one argument and options starts with -, shift everything over.
+ filePattern = mode;
+ mode = options;
+ options = '';
+ }
+ else {
+ common.error('You must specify a file.');
+ }
+ }
+
+ options = common.parseOptions(options, {
+ 'R': 'recursive',
+ 'c': 'changes',
+ 'v': 'verbose'
+ });
+
+ if (typeof filePattern === 'string') {
+ filePattern = [ filePattern ];
+ }
+
+ var files;
+
+ if (options.recursive) {
+ files = [];
+ common.expand(filePattern).forEach(function addFile(expandedFile) {
+ var stat = fs.lstatSync(expandedFile);
+
+ if (!stat.isSymbolicLink()) {
+ files.push(expandedFile);
+
+ if (stat.isDirectory()) { // intentionally does not follow symlinks.
+ fs.readdirSync(expandedFile).forEach(function (child) {
+ addFile(expandedFile + '/' + child);
+ });
+ }
+ }
+ });
+ }
+ else {
+ files = common.expand(filePattern);
+ }
+
+ files.forEach(function innerChmod(file) {
+ file = path.resolve(file);
+ if (!fs.existsSync(file)) {
+ common.error('File not found: ' + file);
+ }
+
+ // When recursing, don't follow symlinks.
+ if (options.recursive && fs.lstatSync(file).isSymbolicLink()) {
+ return;
+ }
+
+ var perms = fs.statSync(file).mode;
+ var type = perms & PERMS.TYPE_MASK;
+
+ var newPerms = perms;
+
+ if (isNaN(parseInt(mode, 8))) {
+ // parse options
+ mode.split(',').forEach(function (symbolicMode) {
+ /*jshint regexdash:true */
+ var pattern = /([ugoa]*)([=\+-])([rwxXst]*)/i;
+ var matches = pattern.exec(symbolicMode);
+
+ if (matches) {
+ var applyTo = matches[1];
+ var operator = matches[2];
+ var change = matches[3];
+
+ var changeOwner = applyTo.indexOf('u') != -1 || applyTo === 'a' || applyTo === '';
+ var changeGroup = applyTo.indexOf('g') != -1 || applyTo === 'a' || applyTo === '';
+ var changeOther = applyTo.indexOf('o') != -1 || applyTo === 'a' || applyTo === '';
+
+ var changeRead = change.indexOf('r') != -1;
+ var changeWrite = change.indexOf('w') != -1;
+ var changeExec = change.indexOf('x') != -1;
+ var changeSticky = change.indexOf('t') != -1;
+ var changeSetuid = change.indexOf('s') != -1;
+
+ var mask = 0;
+ if (changeOwner) {
+ mask |= (changeRead ? PERMS.OWNER_READ : 0) + (changeWrite ? PERMS.OWNER_WRITE : 0) + (changeExec ? PERMS.OWNER_EXEC : 0) + (changeSetuid ? PERMS.SETUID : 0);
+ }
+ if (changeGroup) {
+ mask |= (changeRead ? PERMS.GROUP_READ : 0) + (changeWrite ? PERMS.GROUP_WRITE : 0) + (changeExec ? PERMS.GROUP_EXEC : 0) + (changeSetuid ? PERMS.SETGID : 0);
+ }
+ if (changeOther) {
+ mask |= (changeRead ? PERMS.OTHER_READ : 0) + (changeWrite ? PERMS.OTHER_WRITE : 0) + (changeExec ? PERMS.OTHER_EXEC : 0);
+ }
+
+ // Sticky bit is special - it's not tied to user, group or other.
+ if (changeSticky) {
+ mask |= PERMS.STICKY;
+ }
+
+ switch (operator) {
+ case '+':
+ newPerms |= mask;
+ break;
+
+ case '-':
+ newPerms &= ~mask;
+ break;
+
+ case '=':
+ newPerms = type + mask;
+
+ // According to POSIX, when using = to explicitly set the permissions, setuid and setgid can never be cleared.
+ if (fs.statSync(file).isDirectory()) {
+ newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
+ }
+ break;
+ }
+
+ if (options.verbose) {
+ log(file + ' -> ' + newPerms.toString(8));
+ }
+
+ if (perms != newPerms) {
+ if (!options.verbose && options.changes) {
+ log(file + ' -> ' + newPerms.toString(8));
+ }
+ fs.chmodSync(file, newPerms);
+ }
+ }
+ else {
+ common.error('Invalid symbolic mode change: ' + symbolicMode);
+ }
+ });
+ }
+ else {
+ // they gave us a full number
+ newPerms = type + parseInt(mode, 8);
+
+ // POSIX rules are that setuid and setgid can only be added using numeric form, but not cleared.
+ if (fs.statSync(file).isDirectory()) {
+ newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
+ }
+
+ fs.chmodSync(file, newPerms);
+ }
+ });
+}
+module.exports = _chmod;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/common.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/common.js b/node_modules/cordova-common/node_modules/shelljs/src/common.js
new file mode 100644
index 0000000..d8c2312
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/common.js
@@ -0,0 +1,203 @@
+var os = require('os');
+var fs = require('fs');
+var _ls = require('./ls');
+
+// Module globals
+var config = {
+ silent: false,
+ fatal: false
+};
+exports.config = config;
+
+var state = {
+ error: null,
+ currentCmd: 'shell.js',
+ tempDir: null
+};
+exports.state = state;
+
+var platform = os.type().match(/^Win/) ? 'win' : 'unix';
+exports.platform = platform;
+
+function log() {
+ if (!config.silent)
+ console.log.apply(this, arguments);
+}
+exports.log = log;
+
+// Shows error message. Throws unless _continue or config.fatal are true
+function error(msg, _continue) {
+ if (state.error === null)
+ state.error = '';
+ state.error += state.currentCmd + ': ' + msg + '\n';
+
+ if (msg.length > 0)
+ log(state.error);
+
+ if (config.fatal)
+ process.exit(1);
+
+ if (!_continue)
+ throw '';
+}
+exports.error = error;
+
+// In the future, when Proxies are default, we can add methods like `.to()` to primitive strings.
+// For now, this is a dummy function to bookmark places we need such strings
+function ShellString(str) {
+ return str;
+}
+exports.ShellString = ShellString;
+
+// Returns {'alice': true, 'bob': false} when passed a dictionary, e.g.:
+// parseOptions('-a', {'a':'alice', 'b':'bob'});
+function parseOptions(str, map) {
+ if (!map)
+ error('parseOptions() internal error: no map given');
+
+ // All options are false by default
+ var options = {};
+ for (var letter in map)
+ options[map[letter]] = false;
+
+ if (!str)
+ return options; // defaults
+
+ if (typeof str !== 'string')
+ error('parseOptions() internal error: wrong str');
+
+ // e.g. match[1] = 'Rf' for str = '-Rf'
+ var match = str.match(/^\-(.+)/);
+ if (!match)
+ return options;
+
+ // e.g. chars = ['R', 'f']
+ var chars = match[1].split('');
+
+ chars.forEach(function(c) {
+ if (c in map)
+ options[map[c]] = true;
+ else
+ error('option not recognized: '+c);
+ });
+
+ return options;
+}
+exports.parseOptions = parseOptions;
+
+// Expands wildcards with matching (ie. existing) file names.
+// For example:
+// expand(['file*.js']) = ['file1.js', 'file2.js', ...]
+// (if the files 'file1.js', 'file2.js', etc, exist in the current dir)
+function expand(list) {
+ var expanded = [];
+ list.forEach(function(listEl) {
+ // Wildcard present on directory names ?
+ if(listEl.search(/\*[^\/]*\//) > -1 || listEl.search(/\*\*[^\/]*\//) > -1) {
+ var match = listEl.match(/^([^*]+\/|)(.*)/);
+ var root = match[1];
+ var rest = match[2];
+ var restRegex = rest.replace(/\*\*/g, ".*").replace(/\*/g, "[^\\/]*");
+ restRegex = new RegExp(restRegex);
+
+ _ls('-R', root).filter(function (e) {
+ return restRegex.test(e);
+ }).forEach(function(file) {
+ expanded.push(file);
+ });
+ }
+ // Wildcard present on file names ?
+ else if (listEl.search(/\*/) > -1) {
+ _ls('', listEl).forEach(function(file) {
+ expanded.push(file);
+ });
+ } else {
+ expanded.push(listEl);
+ }
+ });
+ return expanded;
+}
+exports.expand = expand;
+
+// Normalizes _unlinkSync() across platforms to match Unix behavior, i.e.
+// file can be unlinked even if it's read-only, see https://github.com/joyent/node/issues/3006
+function unlinkSync(file) {
+ try {
+ fs.unlinkSync(file);
+ } catch(e) {
+ // Try to override file permission
+ if (e.code === 'EPERM') {
+ fs.chmodSync(file, '0666');
+ fs.unlinkSync(file);
+ } else {
+ throw e;
+ }
+ }
+}
+exports.unlinkSync = unlinkSync;
+
+// e.g. 'shelljs_a5f185d0443ca...'
+function randomFileName() {
+ function randomHash(count) {
+ if (count === 1)
+ return parseInt(16*Math.random(), 10).toString(16);
+ else {
+ var hash = '';
+ for (var i=0; i<count; i++)
+ hash += randomHash(1);
+ return hash;
+ }
+ }
+
+ return 'shelljs_'+randomHash(20);
+}
+exports.randomFileName = randomFileName;
+
+// extend(target_obj, source_obj1 [, source_obj2 ...])
+// Shallow extend, e.g.:
+// extend({A:1}, {b:2}, {c:3}) returns {A:1, b:2, c:3}
+function extend(target) {
+ var sources = [].slice.call(arguments, 1);
+ sources.forEach(function(source) {
+ for (var key in source)
+ target[key] = source[key];
+ });
+
+ return target;
+}
+exports.extend = extend;
+
+// Common wrapper for all Unix-like commands
+function wrap(cmd, fn, options) {
+ return function() {
+ var retValue = null;
+
+ state.currentCmd = cmd;
+ state.error = null;
+
+ try {
+ var args = [].slice.call(arguments, 0);
+
+ if (options && options.notUnix) {
+ retValue = fn.apply(this, args);
+ } else {
+ if (args.length === 0 || typeof args[0] !== 'string' || args[0][0] !== '-')
+ args.unshift(''); // only add dummy option if '-option' not already present
+ retValue = fn.apply(this, args);
+ }
+ } catch (e) {
+ if (!state.error) {
+ // If state.error hasn't been set it's an error thrown by Node, not us - probably a bug...
+ console.log('shell.js: internal error');
+ console.log(e.stack || e);
+ process.exit(1);
+ }
+ if (config.fatal)
+ throw e;
+ }
+
+ state.currentCmd = 'shell.js';
+ return retValue;
+ };
+} // wrap
+exports.wrap = wrap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/cp.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/cp.js b/node_modules/cordova-common/node_modules/shelljs/src/cp.js
new file mode 100644
index 0000000..ef19f96
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/cp.js
@@ -0,0 +1,204 @@
+var fs = require('fs');
+var path = require('path');
+var common = require('./common');
+var os = require('os');
+
+// Buffered file copy, synchronous
+// (Using readFileSync() + writeFileSync() could easily cause a memory overflow
+// with large files)
+function copyFileSync(srcFile, destFile) {
+ if (!fs.existsSync(srcFile))
+ common.error('copyFileSync: no such file or directory: ' + srcFile);
+
+ var BUF_LENGTH = 64*1024,
+ buf = new Buffer(BUF_LENGTH),
+ bytesRead = BUF_LENGTH,
+ pos = 0,
+ fdr = null,
+ fdw = null;
+
+ try {
+ fdr = fs.openSync(srcFile, 'r');
+ } catch(e) {
+ common.error('copyFileSync: could not read src file ('+srcFile+')');
+ }
+
+ try {
+ fdw = fs.openSync(destFile, 'w');
+ } catch(e) {
+ common.error('copyFileSync: could not write to dest file (code='+e.code+'):'+destFile);
+ }
+
+ while (bytesRead === BUF_LENGTH) {
+ bytesRead = fs.readSync(fdr, buf, 0, BUF_LENGTH, pos);
+ fs.writeSync(fdw, buf, 0, bytesRead);
+ pos += bytesRead;
+ }
+
+ fs.closeSync(fdr);
+ fs.closeSync(fdw);
+
+ fs.chmodSync(destFile, fs.statSync(srcFile).mode);
+}
+
+// Recursively copies 'sourceDir' into 'destDir'
+// Adapted from https://github.com/ryanmcgrath/wrench-js
+//
+// Copyright (c) 2010 Ryan McGrath
+// Copyright (c) 2012 Artur Adib
+//
+// Licensed under the MIT License
+// http://www.opensource.org/licenses/mit-license.php
+function cpdirSyncRecursive(sourceDir, destDir, opts) {
+ if (!opts) opts = {};
+
+ /* Create the directory where all our junk is moving to; read the mode of the source directory and mirror it */
+ var checkDir = fs.statSync(sourceDir);
+ try {
+ fs.mkdirSync(destDir, checkDir.mode);
+ } catch (e) {
+ //if the directory already exists, that's okay
+ if (e.code !== 'EEXIST') throw e;
+ }
+
+ var files = fs.readdirSync(sourceDir);
+
+ for (var i = 0; i < files.length; i++) {
+ var srcFile = sourceDir + "/" + files[i];
+ var destFile = destDir + "/" + files[i];
+ var srcFileStat = fs.lstatSync(srcFile);
+
+ if (srcFileStat.isDirectory()) {
+ /* recursion this thing right on back. */
+ cpdirSyncRecursive(srcFile, destFile, opts);
+ } else if (srcFileStat.isSymbolicLink()) {
+ var symlinkFull = fs.readlinkSync(srcFile);
+ fs.symlinkSync(symlinkFull, destFile, os.platform() === "win32" ? "junction" : null);
+ } else {
+ /* At this point, we've hit a file actually worth copying... so copy it on over. */
+ if (fs.existsSync(destFile) && !opts.force) {
+ common.log('skipping existing file: ' + files[i]);
+ } else {
+ copyFileSync(srcFile, destFile);
+ }
+ }
+
+ } // for files
+} // cpdirSyncRecursive
+
+
+//@
+//@ ### cp([options ,] source [,source ...], dest)
+//@ ### cp([options ,] source_array, dest)
+//@ Available options:
+//@
+//@ + `-f`: force
+//@ + `-r, -R`: recursive
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ cp('file1', 'dir1');
+//@ cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
+//@ cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
+//@ ```
+//@
+//@ Copies files. The wildcard `*` is accepted.
+function _cp(options, sources, dest) {
+ options = common.parseOptions(options, {
+ 'f': 'force',
+ 'R': 'recursive',
+ 'r': 'recursive'
+ });
+
+ // Get sources, dest
+ if (arguments.length < 3) {
+ common.error('missing <source> and/or <dest>');
+ } else if (arguments.length > 3) {
+ sources = [].slice.call(arguments, 1, arguments.length - 1);
+ dest = arguments[arguments.length - 1];
+ } else if (typeof sources === 'string') {
+ sources = [sources];
+ } else if ('length' in sources) {
+ sources = sources; // no-op for array
+ } else {
+ common.error('invalid arguments');
+ }
+
+ var exists = fs.existsSync(dest),
+ stats = exists && fs.statSync(dest);
+
+ // Dest is not existing dir, but multiple sources given
+ if ((!exists || !stats.isDirectory()) && sources.length > 1)
+ common.error('dest is not a directory (too many sources)');
+
+ // Dest is an existing file, but no -f given
+ if (exists && stats.isFile() && !options.force)
+ common.error('dest file already exists: ' + dest);
+
+ if (options.recursive) {
+ // Recursive allows the shortcut syntax "sourcedir/" for "sourcedir/*"
+ // (see Github issue #15)
+ sources.forEach(function(src, i) {
+ if (src[src.length - 1] === '/')
+ sources[i] += '*';
+ });
+
+ // Create dest
+ try {
+ fs.mkdirSync(dest, parseInt('0777', 8));
+ } catch (e) {
+ // like Unix's cp, keep going even if we can't create dest dir
+ }
+ }
+
+ sources = common.expand(sources);
+
+ sources.forEach(function(src) {
+ if (!fs.existsSync(src)) {
+ common.error('no such file or directory: '+src, true);
+ return; // skip file
+ }
+
+ // If here, src exists
+ if (fs.statSync(src).isDirectory()) {
+ if (!options.recursive) {
+ // Non-Recursive
+ common.log(src + ' is a directory (not copied)');
+ } else {
+ // Recursive
+ // 'cp /a/source dest' should create 'source' in 'dest'
+ var newDest = path.join(dest, path.basename(src)),
+ checkDir = fs.statSync(src);
+ try {
+ fs.mkdirSync(newDest, checkDir.mode);
+ } catch (e) {
+ //if the directory already exists, that's okay
+ if (e.code !== 'EEXIST') {
+ common.error('dest file no such file or directory: ' + newDest, true);
+ throw e;
+ }
+ }
+
+ cpdirSyncRecursive(src, newDest, {force: options.force});
+ }
+ return; // done with dir
+ }
+
+ // If here, src is a file
+
+ // When copying to '/path/dir':
+ // thisDest = '/path/dir/file1'
+ var thisDest = dest;
+ if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
+ thisDest = path.normalize(dest + '/' + path.basename(src));
+
+ if (fs.existsSync(thisDest) && !options.force) {
+ common.error('dest file already exists: ' + thisDest, true);
+ return; // skip file
+ }
+
+ copyFileSync(src, thisDest);
+ }); // forEach(src)
+}
+module.exports = _cp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/dirs.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/dirs.js b/node_modules/cordova-common/node_modules/shelljs/src/dirs.js
new file mode 100644
index 0000000..58fae8b
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/dirs.js
@@ -0,0 +1,191 @@
+var common = require('./common');
+var _cd = require('./cd');
+var path = require('path');
+
+// Pushd/popd/dirs internals
+var _dirStack = [];
+
+function _isStackIndex(index) {
+ return (/^[\-+]\d+$/).test(index);
+}
+
+function _parseStackIndex(index) {
+ if (_isStackIndex(index)) {
+ if (Math.abs(index) < _dirStack.length + 1) { // +1 for pwd
+ return (/^-/).test(index) ? Number(index) - 1 : Number(index);
+ } else {
+ common.error(index + ': directory stack index out of range');
+ }
+ } else {
+ common.error(index + ': invalid number');
+ }
+}
+
+function _actualDirStack() {
+ return [process.cwd()].concat(_dirStack);
+}
+
+//@
+//@ ### pushd([options,] [dir | '-N' | '+N'])
+//@
+//@ Available options:
+//@
+//@ + `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
+//@
+//@ Arguments:
+//@
+//@ + `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
+//@ + `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
+//@ + `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ // process.cwd() === '/usr'
+//@ pushd('/etc'); // Returns /etc /usr
+//@ pushd('+1'); // Returns /usr /etc
+//@ ```
+//@
+//@ Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
+function _pushd(options, dir) {
+ if (_isStackIndex(options)) {
+ dir = options;
+ options = '';
+ }
+
+ options = common.parseOptions(options, {
+ 'n' : 'no-cd'
+ });
+
+ var dirs = _actualDirStack();
+
+ if (dir === '+0') {
+ return dirs; // +0 is a noop
+ } else if (!dir) {
+ if (dirs.length > 1) {
+ dirs = dirs.splice(1, 1).concat(dirs);
+ } else {
+ return common.error('no other directory');
+ }
+ } else if (_isStackIndex(dir)) {
+ var n = _parseStackIndex(dir);
+ dirs = dirs.slice(n).concat(dirs.slice(0, n));
+ } else {
+ if (options['no-cd']) {
+ dirs.splice(1, 0, dir);
+ } else {
+ dirs.unshift(dir);
+ }
+ }
+
+ if (options['no-cd']) {
+ dirs = dirs.slice(1);
+ } else {
+ dir = path.resolve(dirs.shift());
+ _cd('', dir);
+ }
+
+ _dirStack = dirs;
+ return _dirs('');
+}
+exports.pushd = _pushd;
+
+//@
+//@ ### popd([options,] ['-N' | '+N'])
+//@
+//@ Available options:
+//@
+//@ + `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
+//@
+//@ Arguments:
+//@
+//@ + `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
+//@ + `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ echo(process.cwd()); // '/usr'
+//@ pushd('/etc'); // '/etc /usr'
+//@ echo(process.cwd()); // '/etc'
+//@ popd(); // '/usr'
+//@ echo(process.cwd()); // '/usr'
+//@ ```
+//@
+//@ When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
+function _popd(options, index) {
+ if (_isStackIndex(options)) {
+ index = options;
+ options = '';
+ }
+
+ options = common.parseOptions(options, {
+ 'n' : 'no-cd'
+ });
+
+ if (!_dirStack.length) {
+ return common.error('directory stack empty');
+ }
+
+ index = _parseStackIndex(index || '+0');
+
+ if (options['no-cd'] || index > 0 || _dirStack.length + index === 0) {
+ index = index > 0 ? index - 1 : index;
+ _dirStack.splice(index, 1);
+ } else {
+ var dir = path.resolve(_dirStack.shift());
+ _cd('', dir);
+ }
+
+ return _dirs('');
+}
+exports.popd = _popd;
+
+//@
+//@ ### dirs([options | '+N' | '-N'])
+//@
+//@ Available options:
+//@
+//@ + `-c`: Clears the directory stack by deleting all of the elements.
+//@
+//@ Arguments:
+//@
+//@ + `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
+//@ + `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
+//@
+//@ Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
+//@
+//@ See also: pushd, popd
+function _dirs(options, index) {
+ if (_isStackIndex(options)) {
+ index = options;
+ options = '';
+ }
+
+ options = common.parseOptions(options, {
+ 'c' : 'clear'
+ });
+
+ if (options['clear']) {
+ _dirStack = [];
+ return _dirStack;
+ }
+
+ var stack = _actualDirStack();
+
+ if (index) {
+ index = _parseStackIndex(index);
+
+ if (index < 0) {
+ index = stack.length + index;
+ }
+
+ common.log(stack[index]);
+ return stack[index];
+ }
+
+ common.log(stack.join(' '));
+
+ return stack;
+}
+exports.dirs = _dirs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/echo.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/echo.js b/node_modules/cordova-common/node_modules/shelljs/src/echo.js
new file mode 100644
index 0000000..760ea84
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/echo.js
@@ -0,0 +1,20 @@
+var common = require('./common');
+
+//@
+//@ ### echo(string [,string ...])
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ echo('hello world');
+//@ var str = echo('hello world');
+//@ ```
+//@
+//@ Prints string to stdout, and returns string with additional utility methods
+//@ like `.to()`.
+function _echo() {
+ var messages = [].slice.call(arguments, 0);
+ console.log.apply(this, messages);
+ return common.ShellString(messages.join(' '));
+}
+module.exports = _echo;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/error.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/error.js b/node_modules/cordova-common/node_modules/shelljs/src/error.js
new file mode 100644
index 0000000..cca3efb
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/error.js
@@ -0,0 +1,10 @@
+var common = require('./common');
+
+//@
+//@ ### error()
+//@ Tests if error occurred in the last command. Returns `null` if no error occurred,
+//@ otherwise returns string explaining the error
+function error() {
+ return common.state.error;
+};
+module.exports = error;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/exec.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/exec.js b/node_modules/cordova-common/node_modules/shelljs/src/exec.js
new file mode 100644
index 0000000..d259a9f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/exec.js
@@ -0,0 +1,216 @@
+var common = require('./common');
+var _tempDir = require('./tempdir');
+var _pwd = require('./pwd');
+var path = require('path');
+var fs = require('fs');
+var child = require('child_process');
+
+// Hack to run child_process.exec() synchronously (sync avoids callback hell)
+// Uses a custom wait loop that checks for a flag file, created when the child process is done.
+// (Can't do a wait loop that checks for internal Node variables/messages as
+// Node is single-threaded; callbacks and other internal state changes are done in the
+// event loop).
+function execSync(cmd, opts) {
+ var tempDir = _tempDir();
+ var stdoutFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ codeFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ scriptFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ sleepFile = path.resolve(tempDir+'/'+common.randomFileName());
+
+ var options = common.extend({
+ silent: common.config.silent
+ }, opts);
+
+ var previousStdoutContent = '';
+ // Echoes stdout changes from running process, if not silent
+ function updateStdout() {
+ if (options.silent || !fs.existsSync(stdoutFile))
+ return;
+
+ var stdoutContent = fs.readFileSync(stdoutFile, 'utf8');
+ // No changes since last time?
+ if (stdoutContent.length <= previousStdoutContent.length)
+ return;
+
+ process.stdout.write(stdoutContent.substr(previousStdoutContent.length));
+ previousStdoutContent = stdoutContent;
+ }
+
+ function escape(str) {
+ return (str+'').replace(/([\\"'])/g, "\\$1").replace(/\0/g, "\\0");
+ }
+
+ if (fs.existsSync(scriptFile)) common.unlinkSync(scriptFile);
+ if (fs.existsSync(stdoutFile)) common.unlinkSync(stdoutFile);
+ if (fs.existsSync(codeFile)) common.unlinkSync(codeFile);
+
+ var execCommand = '"'+process.execPath+'" '+scriptFile;
+ var execOptions = {
+ env: process.env,
+ cwd: _pwd(),
+ maxBuffer: 20*1024*1024
+ };
+
+ if (typeof child.execSync === 'function') {
+ var script = [
+ "var child = require('child_process')",
+ " , fs = require('fs');",
+ "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
+ " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
+ "});",
+ "var stdoutStream = fs.createWriteStream('"+escape(stdoutFile)+"');",
+ "childProcess.stdout.pipe(stdoutStream, {end: false});",
+ "childProcess.stderr.pipe(stdoutStream, {end: false});",
+ "childProcess.stdout.pipe(process.stdout);",
+ "childProcess.stderr.pipe(process.stderr);",
+ "var stdoutEnded = false, stderrEnded = false;",
+ "function tryClosing(){ if(stdoutEnded && stderrEnded){ stdoutStream.end(); } }",
+ "childProcess.stdout.on('end', function(){ stdoutEnded = true; tryClosing(); });",
+ "childProcess.stderr.on('end', function(){ stderrEnded = true; tryClosing(); });"
+ ].join('\n');
+
+ fs.writeFileSync(scriptFile, script);
+
+ if (options.silent) {
+ execOptions.stdio = 'ignore';
+ } else {
+ execOptions.stdio = [0, 1, 2];
+ }
+
+ // Welcome to the future
+ child.execSync(execCommand, execOptions);
+ } else {
+ cmd += ' > '+stdoutFile+' 2>&1'; // works on both win/unix
+
+ var script = [
+ "var child = require('child_process')",
+ " , fs = require('fs');",
+ "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
+ " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
+ "});"
+ ].join('\n');
+
+ fs.writeFileSync(scriptFile, script);
+
+ child.exec(execCommand, execOptions);
+
+ // The wait loop
+ // sleepFile is used as a dummy I/O op to mitigate unnecessary CPU usage
+ // (tried many I/O sync ops, writeFileSync() seems to be only one that is effective in reducing
+ // CPU usage, though apparently not so much on Windows)
+ while (!fs.existsSync(codeFile)) { updateStdout(); fs.writeFileSync(sleepFile, 'a'); }
+ while (!fs.existsSync(stdoutFile)) { updateStdout(); fs.writeFileSync(sleepFile, 'a'); }
+ }
+
+ // At this point codeFile exists, but it's not necessarily flushed yet.
+ // Keep reading it until it is.
+ var code = parseInt('', 10);
+ while (isNaN(code)) {
+ code = parseInt(fs.readFileSync(codeFile, 'utf8'), 10);
+ }
+
+ var stdout = fs.readFileSync(stdoutFile, 'utf8');
+
+ // No biggie if we can't erase the files now -- they're in a temp dir anyway
+ try { common.unlinkSync(scriptFile); } catch(e) {}
+ try { common.unlinkSync(stdoutFile); } catch(e) {}
+ try { common.unlinkSync(codeFile); } catch(e) {}
+ try { common.unlinkSync(sleepFile); } catch(e) {}
+
+ // some shell return codes are defined as errors, per http://tldp.org/LDP/abs/html/exitcodes.html
+ if (code === 1 || code === 2 || code >= 126) {
+ common.error('', true); // unix/shell doesn't really give an error message after non-zero exit codes
+ }
+ // True if successful, false if not
+ var obj = {
+ code: code,
+ output: stdout
+ };
+ return obj;
+} // execSync()
+
+// Wrapper around exec() to enable echoing output to console in real time
+function execAsync(cmd, opts, callback) {
+ var output = '';
+
+ var options = common.extend({
+ silent: common.config.silent
+ }, opts);
+
+ var c = child.exec(cmd, {env: process.env, maxBuffer: 20*1024*1024}, function(err) {
+ if (callback)
+ callback(err ? err.code : 0, output);
+ });
+
+ c.stdout.on('data', function(data) {
+ output += data;
+ if (!options.silent)
+ process.stdout.write(data);
+ });
+
+ c.stderr.on('data', function(data) {
+ output += data;
+ if (!options.silent)
+ process.stdout.write(data);
+ });
+
+ return c;
+}
+
+//@
+//@ ### exec(command [, options] [, callback])
+//@ Available options (all `false` by default):
+//@
+//@ + `async`: Asynchronous execution. Defaults to true if a callback is provided.
+//@ + `silent`: Do not echo program output to console.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var version = exec('node --version', {silent:true}).output;
+//@
+//@ var child = exec('some_long_running_process', {async:true});
+//@ child.stdout.on('data', function(data) {
+//@ /* ... do something with data ... */
+//@ });
+//@
+//@ exec('some_long_running_process', function(code, output) {
+//@ console.log('Exit code:', code);
+//@ console.log('Program output:', output);
+//@ });
+//@ ```
+//@
+//@ Executes the given `command` _synchronously_, unless otherwise specified.
+//@ When in synchronous mode returns the object `{ code:..., output:... }`, containing the program's
+//@ `output` (stdout + stderr) and its exit `code`. Otherwise returns the child process object, and
+//@ the `callback` gets the arguments `(code, output)`.
+//@
+//@ **Note:** For long-lived processes, it's best to run `exec()` asynchronously as
+//@ the current synchronous implementation uses a lot of CPU. This should be getting
+//@ fixed soon.
+function _exec(command, options, callback) {
+ if (!command)
+ common.error('must specify command');
+
+ // Callback is defined instead of options.
+ if (typeof options === 'function') {
+ callback = options;
+ options = { async: true };
+ }
+
+ // Callback is defined with options.
+ if (typeof options === 'object' && typeof callback === 'function') {
+ options.async = true;
+ }
+
+ options = common.extend({
+ silent: common.config.silent,
+ async: false
+ }, options);
+
+ if (options.async)
+ return execAsync(command, options, callback);
+ else
+ return execSync(command, options);
+}
+module.exports = _exec;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/find.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/find.js b/node_modules/cordova-common/node_modules/shelljs/src/find.js
new file mode 100644
index 0000000..d9eeec2
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/find.js
@@ -0,0 +1,51 @@
+var fs = require('fs');
+var common = require('./common');
+var _ls = require('./ls');
+
+//@
+//@ ### find(path [,path ...])
+//@ ### find(path_array)
+//@ Examples:
+//@
+//@ ```javascript
+//@ find('src', 'lib');
+//@ find(['src', 'lib']); // same as above
+//@ find('.').filter(function(file) { return file.match(/\.js$/); });
+//@ ```
+//@
+//@ Returns array of all files (however deep) in the given paths.
+//@
+//@ The main difference from `ls('-R', path)` is that the resulting file names
+//@ include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
+function _find(options, paths) {
+ if (!paths)
+ common.error('no path specified');
+ else if (typeof paths === 'object')
+ paths = paths; // assume array
+ else if (typeof paths === 'string')
+ paths = [].slice.call(arguments, 1);
+
+ var list = [];
+
+ function pushFile(file) {
+ if (common.platform === 'win')
+ file = file.replace(/\\/g, '/');
+ list.push(file);
+ }
+
+ // why not simply do ls('-R', paths)? because the output wouldn't give the base dirs
+ // to get the base dir in the output, we need instead ls('-R', 'dir/*') for every directory
+
+ paths.forEach(function(file) {
+ pushFile(file);
+
+ if (fs.statSync(file).isDirectory()) {
+ _ls('-RA', file+'/*').forEach(function(subfile) {
+ pushFile(subfile);
+ });
+ }
+ });
+
+ return list;
+}
+module.exports = _find;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/grep.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/grep.js b/node_modules/cordova-common/node_modules/shelljs/src/grep.js
new file mode 100644
index 0000000..00c7d6a
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/grep.js
@@ -0,0 +1,52 @@
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### grep([options ,] regex_filter, file [, file ...])
+//@ ### grep([options ,] regex_filter, file_array)
+//@ Available options:
+//@
+//@ + `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ grep('-v', 'GLOBAL_VARIABLE', '*.js');
+//@ grep('GLOBAL_VARIABLE', '*.js');
+//@ ```
+//@
+//@ Reads input string from given files and returns a string containing all lines of the
+//@ file that match the given `regex_filter`. Wildcard `*` accepted.
+function _grep(options, regex, files) {
+ options = common.parseOptions(options, {
+ 'v': 'inverse'
+ });
+
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 2);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ var grep = '';
+ files.forEach(function(file) {
+ if (!fs.existsSync(file)) {
+ common.error('no such file or directory: ' + file, true);
+ return;
+ }
+
+ var contents = fs.readFileSync(file, 'utf8'),
+ lines = contents.split(/\r*\n/);
+ lines.forEach(function(line) {
+ var matched = line.match(regex);
+ if ((options.inverse && !matched) || (!options.inverse && matched))
+ grep += line + '\n';
+ });
+ });
+
+ return common.ShellString(grep);
+}
+module.exports = _grep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/ln.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/ln.js b/node_modules/cordova-common/node_modules/shelljs/src/ln.js
new file mode 100644
index 0000000..a7b9701
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/ln.js
@@ -0,0 +1,53 @@
+var fs = require('fs');
+var path = require('path');
+var common = require('./common');
+var os = require('os');
+
+//@
+//@ ### ln(options, source, dest)
+//@ ### ln(source, dest)
+//@ Available options:
+//@
+//@ + `s`: symlink
+//@ + `f`: force
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ ln('file', 'newlink');
+//@ ln('-sf', 'file', 'existing');
+//@ ```
+//@
+//@ Links source to dest. Use -f to force the link, should dest already exist.
+function _ln(options, source, dest) {
+ options = common.parseOptions(options, {
+ 's': 'symlink',
+ 'f': 'force'
+ });
+
+ if (!source || !dest) {
+ common.error('Missing <source> and/or <dest>');
+ }
+
+ source = path.resolve(process.cwd(), String(source));
+ dest = path.resolve(process.cwd(), String(dest));
+
+ if (!fs.existsSync(source)) {
+ common.error('Source file does not exist', true);
+ }
+
+ if (fs.existsSync(dest)) {
+ if (!options.force) {
+ common.error('Destination file exists', true);
+ }
+
+ fs.unlinkSync(dest);
+ }
+
+ if (options.symlink) {
+ fs.symlinkSync(source, dest, os.platform() === "win32" ? "junction" : null);
+ } else {
+ fs.linkSync(source, dest, os.platform() === "win32" ? "junction" : null);
+ }
+}
+module.exports = _ln;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/ls.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/ls.js b/node_modules/cordova-common/node_modules/shelljs/src/ls.js
new file mode 100644
index 0000000..3345db4
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/ls.js
@@ -0,0 +1,126 @@
+var path = require('path');
+var fs = require('fs');
+var common = require('./common');
+var _cd = require('./cd');
+var _pwd = require('./pwd');
+
+//@
+//@ ### ls([options ,] path [,path ...])
+//@ ### ls([options ,] path_array)
+//@ Available options:
+//@
+//@ + `-R`: recursive
+//@ + `-A`: all files (include files beginning with `.`, except for `.` and `..`)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ ls('projs/*.js');
+//@ ls('-R', '/users/me', '/tmp');
+//@ ls('-R', ['/users/me', '/tmp']); // same as above
+//@ ```
+//@
+//@ Returns array of files in the given path, or in current directory if no path provided.
+function _ls(options, paths) {
+ options = common.parseOptions(options, {
+ 'R': 'recursive',
+ 'A': 'all',
+ 'a': 'all_deprecated'
+ });
+
+ if (options.all_deprecated) {
+ // We won't support the -a option as it's hard to image why it's useful
+ // (it includes '.' and '..' in addition to '.*' files)
+ // For backwards compatibility we'll dump a deprecated message and proceed as before
+ common.log('ls: Option -a is deprecated. Use -A instead');
+ options.all = true;
+ }
+
+ if (!paths)
+ paths = ['.'];
+ else if (typeof paths === 'object')
+ paths = paths; // assume array
+ else if (typeof paths === 'string')
+ paths = [].slice.call(arguments, 1);
+
+ var list = [];
+
+ // Conditionally pushes file to list - returns true if pushed, false otherwise
+ // (e.g. prevents hidden files to be included unless explicitly told so)
+ function pushFile(file, query) {
+ // hidden file?
+ if (path.basename(file)[0] === '.') {
+ // not explicitly asking for hidden files?
+ if (!options.all && !(path.basename(query)[0] === '.' && path.basename(query).length > 1))
+ return false;
+ }
+
+ if (common.platform === 'win')
+ file = file.replace(/\\/g, '/');
+
+ list.push(file);
+ return true;
+ }
+
+ paths.forEach(function(p) {
+ if (fs.existsSync(p)) {
+ var stats = fs.statSync(p);
+ // Simple file?
+ if (stats.isFile()) {
+ pushFile(p, p);
+ return; // continue
+ }
+
+ // Simple dir?
+ if (stats.isDirectory()) {
+ // Iterate over p contents
+ fs.readdirSync(p).forEach(function(file) {
+ if (!pushFile(file, p))
+ return;
+
+ // Recursive?
+ if (options.recursive) {
+ var oldDir = _pwd();
+ _cd('', p);
+ if (fs.statSync(file).isDirectory())
+ list = list.concat(_ls('-R'+(options.all?'A':''), file+'/*'));
+ _cd('', oldDir);
+ }
+ });
+ return; // continue
+ }
+ }
+
+ // p does not exist - possible wildcard present
+
+ var basename = path.basename(p);
+ var dirname = path.dirname(p);
+ // Wildcard present on an existing dir? (e.g. '/tmp/*.js')
+ if (basename.search(/\*/) > -1 && fs.existsSync(dirname) && fs.statSync(dirname).isDirectory) {
+ // Escape special regular expression chars
+ var regexp = basename.replace(/(\^|\$|\(|\)|<|>|\[|\]|\{|\}|\.|\+|\?)/g, '\\$1');
+ // Translates wildcard into regex
+ regexp = '^' + regexp.replace(/\*/g, '.*') + '$';
+ // Iterate over directory contents
+ fs.readdirSync(dirname).forEach(function(file) {
+ if (file.match(new RegExp(regexp))) {
+ if (!pushFile(path.normalize(dirname+'/'+file), basename))
+ return;
+
+ // Recursive?
+ if (options.recursive) {
+ var pp = dirname + '/' + file;
+ if (fs.lstatSync(pp).isDirectory())
+ list = list.concat(_ls('-R'+(options.all?'A':''), pp+'/*'));
+ } // recursive
+ } // if file matches
+ }); // forEach
+ return;
+ }
+
+ common.error('no such file or directory: ' + p, true);
+ });
+
+ return list;
+}
+module.exports = _ls;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js b/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js
new file mode 100644
index 0000000..5a7088f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/mkdir.js
@@ -0,0 +1,68 @@
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+// Recursively creates 'dir'
+function mkdirSyncRecursive(dir) {
+ var baseDir = path.dirname(dir);
+
+ // Base dir exists, no recursion necessary
+ if (fs.existsSync(baseDir)) {
+ fs.mkdirSync(dir, parseInt('0777', 8));
+ return;
+ }
+
+ // Base dir does not exist, go recursive
+ mkdirSyncRecursive(baseDir);
+
+ // Base dir created, can create dir
+ fs.mkdirSync(dir, parseInt('0777', 8));
+}
+
+//@
+//@ ### mkdir([options ,] dir [, dir ...])
+//@ ### mkdir([options ,] dir_array)
+//@ Available options:
+//@
+//@ + `p`: full path (will create intermediate dirs if necessary)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
+//@ mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
+//@ ```
+//@
+//@ Creates directories.
+function _mkdir(options, dirs) {
+ options = common.parseOptions(options, {
+ 'p': 'fullpath'
+ });
+ if (!dirs)
+ common.error('no paths given');
+
+ if (typeof dirs === 'string')
+ dirs = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ dirs.forEach(function(dir) {
+ if (fs.existsSync(dir)) {
+ if (!options.fullpath)
+ common.error('path already exists: ' + dir, true);
+ return; // skip dir
+ }
+
+ // Base dir does not exist, and no -p option given
+ var baseDir = path.dirname(dir);
+ if (!fs.existsSync(baseDir) && !options.fullpath) {
+ common.error('no such file or directory: ' + baseDir, true);
+ return; // skip dir
+ }
+
+ if (options.fullpath)
+ mkdirSyncRecursive(dir);
+ else
+ fs.mkdirSync(dir, parseInt('0777', 8));
+ });
+} // mkdir
+module.exports = _mkdir;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/mv.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/mv.js b/node_modules/cordova-common/node_modules/shelljs/src/mv.js
new file mode 100644
index 0000000..11f9607
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/mv.js
@@ -0,0 +1,80 @@
+var fs = require('fs');
+var path = require('path');
+var common = require('./common');
+
+//@
+//@ ### mv(source [, source ...], dest')
+//@ ### mv(source_array, dest')
+//@ Available options:
+//@
+//@ + `f`: force
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ mv('-f', 'file', 'dir/');
+//@ mv('file1', 'file2', 'dir/');
+//@ mv(['file1', 'file2'], 'dir/'); // same as above
+//@ ```
+//@
+//@ Moves files. The wildcard `*` is accepted.
+function _mv(options, sources, dest) {
+ options = common.parseOptions(options, {
+ 'f': 'force'
+ });
+
+ // Get sources, dest
+ if (arguments.length < 3) {
+ common.error('missing <source> and/or <dest>');
+ } else if (arguments.length > 3) {
+ sources = [].slice.call(arguments, 1, arguments.length - 1);
+ dest = arguments[arguments.length - 1];
+ } else if (typeof sources === 'string') {
+ sources = [sources];
+ } else if ('length' in sources) {
+ sources = sources; // no-op for array
+ } else {
+ common.error('invalid arguments');
+ }
+
+ sources = common.expand(sources);
+
+ var exists = fs.existsSync(dest),
+ stats = exists && fs.statSync(dest);
+
+ // Dest is not existing dir, but multiple sources given
+ if ((!exists || !stats.isDirectory()) && sources.length > 1)
+ common.error('dest is not a directory (too many sources)');
+
+ // Dest is an existing file, but no -f given
+ if (exists && stats.isFile() && !options.force)
+ common.error('dest file already exists: ' + dest);
+
+ sources.forEach(function(src) {
+ if (!fs.existsSync(src)) {
+ common.error('no such file or directory: '+src, true);
+ return; // skip file
+ }
+
+ // If here, src exists
+
+ // When copying to '/path/dir':
+ // thisDest = '/path/dir/file1'
+ var thisDest = dest;
+ if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
+ thisDest = path.normalize(dest + '/' + path.basename(src));
+
+ if (fs.existsSync(thisDest) && !options.force) {
+ common.error('dest file already exists: ' + thisDest, true);
+ return; // skip file
+ }
+
+ if (path.resolve(src) === path.dirname(path.resolve(thisDest))) {
+ common.error('cannot move to self: '+src, true);
+ return; // skip file
+ }
+
+ fs.renameSync(src, thisDest);
+ }); // forEach(src)
+} // mv
+module.exports = _mv;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/popd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/popd.js b/node_modules/cordova-common/node_modules/shelljs/src/popd.js
new file mode 100644
index 0000000..11ea24f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/popd.js
@@ -0,0 +1 @@
+// see dirs.js
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/pushd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/pushd.js b/node_modules/cordova-common/node_modules/shelljs/src/pushd.js
new file mode 100644
index 0000000..11ea24f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/pushd.js
@@ -0,0 +1 @@
+// see dirs.js
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/pwd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/pwd.js b/node_modules/cordova-common/node_modules/shelljs/src/pwd.js
new file mode 100644
index 0000000..41727bb
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/pwd.js
@@ -0,0 +1,11 @@
+var path = require('path');
+var common = require('./common');
+
+//@
+//@ ### pwd()
+//@ Returns the current directory.
+function _pwd(options) {
+ var pwd = path.resolve(process.cwd());
+ return common.ShellString(pwd);
+}
+module.exports = _pwd;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[07/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/strict.dtd
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/strict.dtd b/node_modules/sax/examples/strict.dtd
new file mode 100644
index 0000000..b274559
--- /dev/null
+++ b/node_modules/sax/examples/strict.dtd
@@ -0,0 +1,870 @@
+<!--
+ This is HTML 4.01 Strict DTD, which excludes the presentation
+ attributes and elements that W3C expects to phase out as
+ support for style sheets matures. Authors should use the Strict
+ DTD when possible, but may use the Transitional DTD when support
+ for presentation attribute and elements is required.
+
+ HTML 4 includes mechanisms for style sheets, scripting,
+ embedding objects, improved support for right to left and mixed
+ direction text, and enhancements to forms for improved
+ accessibility for people with disabilities.
+
+ Draft: $Date: 1999/12/24 23:37:48 $
+
+ Authors:
+ Dave Raggett <ds...@w3.org>
+ Arnaud Le Hors <le...@w3.org>
+ Ian Jacobs <ij...@w3.org>
+
+ Further information about HTML 4.01 is available at:
+
+ http://www.w3.org/TR/1999/REC-html401-19991224
+
+
+ The HTML 4.01 specification includes additional
+ syntactic constraints that cannot be expressed within
+ the DTDs.
+
+-->
+<!--
+ Typical usage:
+
+ <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"
+ "http://www.w3.org/TR/html4/strict.dtd">
+ <html>
+ <head>
+ ...
+ </head>
+ <body>
+ ...
+ </body>
+ </html>
+
+ The URI used as a system identifier with the public identifier allows
+ the user agent to download the DTD and entity sets as needed.
+
+ The FPI for the Transitional HTML 4.01 DTD is:
+
+ "-//W3C//DTD HTML 4.01 Transitional//EN"
+
+ This version of the transitional DTD is:
+
+ http://www.w3.org/TR/1999/REC-html401-19991224/loose.dtd
+
+ If you are writing a document that includes frames, use
+ the following FPI:
+
+ "-//W3C//DTD HTML 4.01 Frameset//EN"
+
+ This version of the frameset DTD is:
+
+ http://www.w3.org/TR/1999/REC-html401-19991224/frameset.dtd
+
+ Use the following (relative) URIs to refer to
+ the DTDs and entity definitions of this specification:
+
+ "strict.dtd"
+ "loose.dtd"
+ "frameset.dtd"
+ "HTMLlat1.ent"
+ "HTMLsymbol.ent"
+ "HTMLspecial.ent"
+
+-->
+
+<!--================== Imported Names ====================================-->
+<!-- Feature Switch for frameset documents -->
+<!ENTITY % HTML.Frameset "IGNORE">
+
+<!ENTITY % ContentType "CDATA"
+ -- media type, as per [RFC2045]
+ -->
+
+<!ENTITY % ContentTypes "CDATA"
+ -- comma-separated list of media types, as per [RFC2045]
+ -->
+
+<!ENTITY % Charset "CDATA"
+ -- a character encoding, as per [RFC2045]
+ -->
+
+<!ENTITY % Charsets "CDATA"
+ -- a space-separated list of character encodings, as per [RFC2045]
+ -->
+
+<!ENTITY % LanguageCode "NAME"
+ -- a language code, as per [RFC1766]
+ -->
+
+<!ENTITY % Character "CDATA"
+ -- a single character from [ISO10646]
+ -->
+
+<!ENTITY % LinkTypes "CDATA"
+ -- space-separated list of link types
+ -->
+
+<!ENTITY % MediaDesc "CDATA"
+ -- single or comma-separated list of media descriptors
+ -->
+
+<!ENTITY % URI "CDATA"
+ -- a Uniform Resource Identifier,
+ see [URI]
+ -->
+
+<!ENTITY % Datetime "CDATA" -- date and time information. ISO date format -->
+
+
+<!ENTITY % Script "CDATA" -- script expression -->
+
+<!ENTITY % StyleSheet "CDATA" -- style sheet data -->
+
+
+
+<!ENTITY % Text "CDATA">
+
+
+<!-- Parameter Entities -->
+
+<!ENTITY % head.misc "SCRIPT|STYLE|META|LINK|OBJECT" -- repeatable head elements -->
+
+<!ENTITY % heading "H1|H2|H3|H4|H5|H6">
+
+<!ENTITY % list "UL | OL">
+
+<!ENTITY % preformatted "PRE">
+
+
+<!--================ Character mnemonic entities =========================-->
+
+<!ENTITY % HTMLlat1 PUBLIC
+ "-//W3C//ENTITIES Latin1//EN//HTML"
+ "HTMLlat1.ent">
+%HTMLlat1;
+
+<!ENTITY % HTMLsymbol PUBLIC
+ "-//W3C//ENTITIES Symbols//EN//HTML"
+ "HTMLsymbol.ent">
+%HTMLsymbol;
+
+<!ENTITY % HTMLspecial PUBLIC
+ "-//W3C//ENTITIES Special//EN//HTML"
+ "HTMLspecial.ent">
+%HTMLspecial;
+<!--=================== Generic Attributes ===============================-->
+
+<!ENTITY % coreattrs
+ "id ID #IMPLIED -- document-wide unique id --
+ class CDATA #IMPLIED -- space-separated list of classes --
+ style %StyleSheet; #IMPLIED -- associated style info --
+ title %Text; #IMPLIED -- advisory title --"
+ >
+
+<!ENTITY % i18n
+ "lang %LanguageCode; #IMPLIED -- language code --
+ dir (ltr|rtl) #IMPLIED -- direction for weak/neutral text --"
+ >
+
+<!ENTITY % events
+ "onclick %Script; #IMPLIED -- a pointer button was clicked --
+ ondblclick %Script; #IMPLIED -- a pointer button was double clicked--
+ onmousedown %Script; #IMPLIED -- a pointer button was pressed down --
+ onmouseup %Script; #IMPLIED -- a pointer button was released --
+ onmouseover %Script; #IMPLIED -- a pointer was moved onto --
+ onmousemove %Script; #IMPLIED -- a pointer was moved within --
+ onmouseout %Script; #IMPLIED -- a pointer was moved away --
+ onkeypress %Script; #IMPLIED -- a key was pressed and released --
+ onkeydown %Script; #IMPLIED -- a key was pressed down --
+ onkeyup %Script; #IMPLIED -- a key was released --"
+ >
+
+<!-- Reserved Feature Switch -->
+<!ENTITY % HTML.Reserved "IGNORE">
+
+<!-- The following attributes are reserved for possible future use -->
+<![ %HTML.Reserved; [
+<!ENTITY % reserved
+ "datasrc %URI; #IMPLIED -- a single or tabular Data Source --
+ datafld CDATA #IMPLIED -- the property or column name --
+ dataformatas (plaintext|html) plaintext -- text or html --"
+ >
+]]>
+
+<!ENTITY % reserved "">
+
+<!ENTITY % attrs "%coreattrs; %i18n; %events;">
+
+
+<!--=================== Text Markup ======================================-->
+
+<!ENTITY % fontstyle
+ "TT | I | B | BIG | SMALL">
+
+<!ENTITY % phrase "EM | STRONG | DFN | CODE |
+ SAMP | KBD | VAR | CITE | ABBR | ACRONYM" >
+
+<!ENTITY % special
+ "A | IMG | OBJECT | BR | SCRIPT | MAP | Q | SUB | SUP | SPAN | BDO">
+
+<!ENTITY % formctrl "INPUT | SELECT | TEXTAREA | LABEL | BUTTON">
+
+<!-- %inline; covers inline or "text-level" elements -->
+<!ENTITY % inline "#PCDATA | %fontstyle; | %phrase; | %special; | %formctrl;">
+
+<!ELEMENT (%fontstyle;|%phrase;) - - (%inline;)*>
+<!ATTLIST (%fontstyle;|%phrase;)
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!ELEMENT (SUB|SUP) - - (%inline;)* -- subscript, superscript -->
+<!ATTLIST (SUB|SUP)
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!ELEMENT SPAN - - (%inline;)* -- generic language/style container -->
+<!ATTLIST SPAN
+ %attrs; -- %coreattrs, %i18n, %events --
+ %reserved; -- reserved for possible future use --
+ >
+
+<!ELEMENT BDO - - (%inline;)* -- I18N BiDi over-ride -->
+<!ATTLIST BDO
+ %coreattrs; -- id, class, style, title --
+ lang %LanguageCode; #IMPLIED -- language code --
+ dir (ltr|rtl) #REQUIRED -- directionality --
+ >
+
+
+<!ELEMENT BR - O EMPTY -- forced line break -->
+<!ATTLIST BR
+ %coreattrs; -- id, class, style, title --
+ >
+
+<!--================== HTML content models ===============================-->
+
+<!--
+ HTML has two basic content models:
+
+ %inline; character level elements and text strings
+ %block; block-like elements e.g. paragraphs and lists
+-->
+
+<!ENTITY % block
+ "P | %heading; | %list; | %preformatted; | DL | DIV | NOSCRIPT |
+ BLOCKQUOTE | FORM | HR | TABLE | FIELDSET | ADDRESS">
+
+<!ENTITY % flow "%block; | %inline;">
+
+<!--=================== Document Body ====================================-->
+
+<!ELEMENT BODY O O (%block;|SCRIPT)+ +(INS|DEL) -- document body -->
+<!ATTLIST BODY
+ %attrs; -- %coreattrs, %i18n, %events --
+ onload %Script; #IMPLIED -- the document has been loaded --
+ onunload %Script; #IMPLIED -- the document has been removed --
+ >
+
+<!ELEMENT ADDRESS - - (%inline;)* -- information on author -->
+<!ATTLIST ADDRESS
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!ELEMENT DIV - - (%flow;)* -- generic language/style container -->
+<!ATTLIST DIV
+ %attrs; -- %coreattrs, %i18n, %events --
+ %reserved; -- reserved for possible future use --
+ >
+
+
+<!--================== The Anchor Element ================================-->
+
+<!ENTITY % Shape "(rect|circle|poly|default)">
+<!ENTITY % Coords "CDATA" -- comma-separated list of lengths -->
+
+<!ELEMENT A - - (%inline;)* -(A) -- anchor -->
+<!ATTLIST A
+ %attrs; -- %coreattrs, %i18n, %events --
+ charset %Charset; #IMPLIED -- char encoding of linked resource --
+ type %ContentType; #IMPLIED -- advisory content type --
+ name CDATA #IMPLIED -- named link end --
+ href %URI; #IMPLIED -- URI for linked resource --
+ hreflang %LanguageCode; #IMPLIED -- language code --
+ rel %LinkTypes; #IMPLIED -- forward link types --
+ rev %LinkTypes; #IMPLIED -- reverse link types --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ shape %Shape; rect -- for use with client-side image maps --
+ coords %Coords; #IMPLIED -- for use with client-side image maps --
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ >
+
+<!--================== Client-side image maps ============================-->
+
+<!-- These can be placed in the same document or grouped in a
+ separate document although this isn't yet widely supported -->
+
+<!ELEMENT MAP - - ((%block;) | AREA)+ -- client-side image map -->
+<!ATTLIST MAP
+ %attrs; -- %coreattrs, %i18n, %events --
+ name CDATA #REQUIRED -- for reference by usemap --
+ >
+
+<!ELEMENT AREA - O EMPTY -- client-side image map area -->
+<!ATTLIST AREA
+ %attrs; -- %coreattrs, %i18n, %events --
+ shape %Shape; rect -- controls interpretation of coords --
+ coords %Coords; #IMPLIED -- comma-separated list of lengths --
+ href %URI; #IMPLIED -- URI for linked resource --
+ nohref (nohref) #IMPLIED -- this region has no action --
+ alt %Text; #REQUIRED -- short description --
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ >
+
+<!--================== The LINK Element ==================================-->
+
+<!--
+ Relationship values can be used in principle:
+
+ a) for document specific toolbars/menus when used
+ with the LINK element in document head e.g.
+ start, contents, previous, next, index, end, help
+ b) to link to a separate style sheet (rel=stylesheet)
+ c) to make a link to a script (rel=script)
+ d) by stylesheets to control how collections of
+ html nodes are rendered into printed documents
+ e) to make a link to a printable version of this document
+ e.g. a postscript or pdf version (rel=alternate media=print)
+-->
+
+<!ELEMENT LINK - O EMPTY -- a media-independent link -->
+<!ATTLIST LINK
+ %attrs; -- %coreattrs, %i18n, %events --
+ charset %Charset; #IMPLIED -- char encoding of linked resource --
+ href %URI; #IMPLIED -- URI for linked resource --
+ hreflang %LanguageCode; #IMPLIED -- language code --
+ type %ContentType; #IMPLIED -- advisory content type --
+ rel %LinkTypes; #IMPLIED -- forward link types --
+ rev %LinkTypes; #IMPLIED -- reverse link types --
+ media %MediaDesc; #IMPLIED -- for rendering on these media --
+ >
+
+<!--=================== Images ===========================================-->
+
+<!-- Length defined in strict DTD for cellpadding/cellspacing -->
+<!ENTITY % Length "CDATA" -- nn for pixels or nn% for percentage length -->
+<!ENTITY % MultiLength "CDATA" -- pixel, percentage, or relative -->
+
+<![ %HTML.Frameset; [
+<!ENTITY % MultiLengths "CDATA" -- comma-separated list of MultiLength -->
+]]>
+
+<!ENTITY % Pixels "CDATA" -- integer representing length in pixels -->
+
+
+<!-- To avoid problems with text-only UAs as well as
+ to make image content understandable and navigable
+ to users of non-visual UAs, you need to provide
+ a description with ALT, and avoid server-side image maps -->
+<!ELEMENT IMG - O EMPTY -- Embedded image -->
+<!ATTLIST IMG
+ %attrs; -- %coreattrs, %i18n, %events --
+ src %URI; #REQUIRED -- URI of image to embed --
+ alt %Text; #REQUIRED -- short description --
+ longdesc %URI; #IMPLIED -- link to long description
+ (complements alt) --
+ name CDATA #IMPLIED -- name of image for scripting --
+ height %Length; #IMPLIED -- override height --
+ width %Length; #IMPLIED -- override width --
+ usemap %URI; #IMPLIED -- use client-side image map --
+ ismap (ismap) #IMPLIED -- use server-side image map --
+ >
+
+<!-- USEMAP points to a MAP element which may be in this document
+ or an external document, although the latter is not widely supported -->
+
+<!--==================== OBJECT ======================================-->
+<!--
+ OBJECT is used to embed objects as part of HTML pages
+ PARAM elements should precede other content. SGML mixed content
+ model technicality precludes specifying this formally ...
+-->
+
+<!ELEMENT OBJECT - - (PARAM | %flow;)*
+ -- generic embedded object -->
+<!ATTLIST OBJECT
+ %attrs; -- %coreattrs, %i18n, %events --
+ declare (declare) #IMPLIED -- declare but don't instantiate flag --
+ classid %URI; #IMPLIED -- identifies an implementation --
+ codebase %URI; #IMPLIED -- base URI for classid, data, archive--
+ data %URI; #IMPLIED -- reference to object's data --
+ type %ContentType; #IMPLIED -- content type for data --
+ codetype %ContentType; #IMPLIED -- content type for code --
+ archive CDATA #IMPLIED -- space-separated list of URIs --
+ standby %Text; #IMPLIED -- message to show while loading --
+ height %Length; #IMPLIED -- override height --
+ width %Length; #IMPLIED -- override width --
+ usemap %URI; #IMPLIED -- use client-side image map --
+ name CDATA #IMPLIED -- submit as part of form --
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ %reserved; -- reserved for possible future use --
+ >
+
+<!ELEMENT PARAM - O EMPTY -- named property value -->
+<!ATTLIST PARAM
+ id ID #IMPLIED -- document-wide unique id --
+ name CDATA #REQUIRED -- property name --
+ value CDATA #IMPLIED -- property value --
+ valuetype (DATA|REF|OBJECT) DATA -- How to interpret value --
+ type %ContentType; #IMPLIED -- content type for value
+ when valuetype=ref --
+ >
+
+
+<!--=================== Horizontal Rule ==================================-->
+
+<!ELEMENT HR - O EMPTY -- horizontal rule -->
+<!ATTLIST HR
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--=================== Paragraphs =======================================-->
+
+<!ELEMENT P - O (%inline;)* -- paragraph -->
+<!ATTLIST P
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--=================== Headings =========================================-->
+
+<!--
+ There are six levels of headings from H1 (the most important)
+ to H6 (the least important).
+-->
+
+<!ELEMENT (%heading;) - - (%inline;)* -- heading -->
+<!ATTLIST (%heading;)
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--=================== Preformatted Text ================================-->
+
+<!-- excludes markup for images and changes in font size -->
+<!ENTITY % pre.exclusion "IMG|OBJECT|BIG|SMALL|SUB|SUP">
+
+<!ELEMENT PRE - - (%inline;)* -(%pre.exclusion;) -- preformatted text -->
+<!ATTLIST PRE
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--===================== Inline Quotes ==================================-->
+
+<!ELEMENT Q - - (%inline;)* -- short inline quotation -->
+<!ATTLIST Q
+ %attrs; -- %coreattrs, %i18n, %events --
+ cite %URI; #IMPLIED -- URI for source document or msg --
+ >
+
+<!--=================== Block-like Quotes ================================-->
+
+<!ELEMENT BLOCKQUOTE - - (%block;|SCRIPT)+ -- long quotation -->
+<!ATTLIST BLOCKQUOTE
+ %attrs; -- %coreattrs, %i18n, %events --
+ cite %URI; #IMPLIED -- URI for source document or msg --
+ >
+
+<!--=================== Inserted/Deleted Text ============================-->
+
+
+<!-- INS/DEL are handled by inclusion on BODY -->
+<!ELEMENT (INS|DEL) - - (%flow;)* -- inserted text, deleted text -->
+<!ATTLIST (INS|DEL)
+ %attrs; -- %coreattrs, %i18n, %events --
+ cite %URI; #IMPLIED -- info on reason for change --
+ datetime %Datetime; #IMPLIED -- date and time of change --
+ >
+
+<!--=================== Lists ============================================-->
+
+<!-- definition lists - DT for term, DD for its definition -->
+
+<!ELEMENT DL - - (DT|DD)+ -- definition list -->
+<!ATTLIST DL
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!ELEMENT DT - O (%inline;)* -- definition term -->
+<!ELEMENT DD - O (%flow;)* -- definition description -->
+<!ATTLIST (DT|DD)
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+
+<!ELEMENT OL - - (LI)+ -- ordered list -->
+<!ATTLIST OL
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!-- Unordered Lists (UL) bullet styles -->
+<!ELEMENT UL - - (LI)+ -- unordered list -->
+<!ATTLIST UL
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+
+
+<!ELEMENT LI - O (%flow;)* -- list item -->
+<!ATTLIST LI
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--================ Forms ===============================================-->
+<!ELEMENT FORM - - (%block;|SCRIPT)+ -(FORM) -- interactive form -->
+<!ATTLIST FORM
+ %attrs; -- %coreattrs, %i18n, %events --
+ action %URI; #REQUIRED -- server-side form handler --
+ method (GET|POST) GET -- HTTP method used to submit the form--
+ enctype %ContentType; "application/x-www-form-urlencoded"
+ accept %ContentTypes; #IMPLIED -- list of MIME types for file upload --
+ name CDATA #IMPLIED -- name of form for scripting --
+ onsubmit %Script; #IMPLIED -- the form was submitted --
+ onreset %Script; #IMPLIED -- the form was reset --
+ accept-charset %Charsets; #IMPLIED -- list of supported charsets --
+ >
+
+<!-- Each label must not contain more than ONE field -->
+<!ELEMENT LABEL - - (%inline;)* -(LABEL) -- form field label text -->
+<!ATTLIST LABEL
+ %attrs; -- %coreattrs, %i18n, %events --
+ for IDREF #IMPLIED -- matches field ID value --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ >
+
+<!ENTITY % InputType
+ "(TEXT | PASSWORD | CHECKBOX |
+ RADIO | SUBMIT | RESET |
+ FILE | HIDDEN | IMAGE | BUTTON)"
+ >
+
+<!-- attribute name required for all but submit and reset -->
+<!ELEMENT INPUT - O EMPTY -- form control -->
+<!ATTLIST INPUT
+ %attrs; -- %coreattrs, %i18n, %events --
+ type %InputType; TEXT -- what kind of widget is needed --
+ name CDATA #IMPLIED -- submit as part of form --
+ value CDATA #IMPLIED -- Specify for radio buttons and checkboxes --
+ checked (checked) #IMPLIED -- for radio buttons and check boxes --
+ disabled (disabled) #IMPLIED -- unavailable in this context --
+ readonly (readonly) #IMPLIED -- for text and passwd --
+ size CDATA #IMPLIED -- specific to each type of field --
+ maxlength NUMBER #IMPLIED -- max chars for text fields --
+ src %URI; #IMPLIED -- for fields with images --
+ alt CDATA #IMPLIED -- short description --
+ usemap %URI; #IMPLIED -- use client-side image map --
+ ismap (ismap) #IMPLIED -- use server-side image map --
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ onselect %Script; #IMPLIED -- some text was selected --
+ onchange %Script; #IMPLIED -- the element value was changed --
+ accept %ContentTypes; #IMPLIED -- list of MIME types for file upload --
+ %reserved; -- reserved for possible future use --
+ >
+
+<!ELEMENT SELECT - - (OPTGROUP|OPTION)+ -- option selector -->
+<!ATTLIST SELECT
+ %attrs; -- %coreattrs, %i18n, %events --
+ name CDATA #IMPLIED -- field name --
+ size NUMBER #IMPLIED -- rows visible --
+ multiple (multiple) #IMPLIED -- default is single selection --
+ disabled (disabled) #IMPLIED -- unavailable in this context --
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ onchange %Script; #IMPLIED -- the element value was changed --
+ %reserved; -- reserved for possible future use --
+ >
+
+<!ELEMENT OPTGROUP - - (OPTION)+ -- option group -->
+<!ATTLIST OPTGROUP
+ %attrs; -- %coreattrs, %i18n, %events --
+ disabled (disabled) #IMPLIED -- unavailable in this context --
+ label %Text; #REQUIRED -- for use in hierarchical menus --
+ >
+
+<!ELEMENT OPTION - O (#PCDATA) -- selectable choice -->
+<!ATTLIST OPTION
+ %attrs; -- %coreattrs, %i18n, %events --
+ selected (selected) #IMPLIED
+ disabled (disabled) #IMPLIED -- unavailable in this context --
+ label %Text; #IMPLIED -- for use in hierarchical menus --
+ value CDATA #IMPLIED -- defaults to element content --
+ >
+
+<!ELEMENT TEXTAREA - - (#PCDATA) -- multi-line text field -->
+<!ATTLIST TEXTAREA
+ %attrs; -- %coreattrs, %i18n, %events --
+ name CDATA #IMPLIED
+ rows NUMBER #REQUIRED
+ cols NUMBER #REQUIRED
+ disabled (disabled) #IMPLIED -- unavailable in this context --
+ readonly (readonly) #IMPLIED
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ onselect %Script; #IMPLIED -- some text was selected --
+ onchange %Script; #IMPLIED -- the element value was changed --
+ %reserved; -- reserved for possible future use --
+ >
+
+<!--
+ #PCDATA is to solve the mixed content problem,
+ per specification only whitespace is allowed there!
+ -->
+<!ELEMENT FIELDSET - - (#PCDATA,LEGEND,(%flow;)*) -- form control group -->
+<!ATTLIST FIELDSET
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!ELEMENT LEGEND - - (%inline;)* -- fieldset legend -->
+
+<!ATTLIST LEGEND
+ %attrs; -- %coreattrs, %i18n, %events --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ >
+
+<!ELEMENT BUTTON - -
+ (%flow;)* -(A|%formctrl;|FORM|FIELDSET)
+ -- push button -->
+<!ATTLIST BUTTON
+ %attrs; -- %coreattrs, %i18n, %events --
+ name CDATA #IMPLIED
+ value CDATA #IMPLIED -- sent to server when submitted --
+ type (button|submit|reset) submit -- for use as form button --
+ disabled (disabled) #IMPLIED -- unavailable in this context --
+ tabindex NUMBER #IMPLIED -- position in tabbing order --
+ accesskey %Character; #IMPLIED -- accessibility key character --
+ onfocus %Script; #IMPLIED -- the element got the focus --
+ onblur %Script; #IMPLIED -- the element lost the focus --
+ %reserved; -- reserved for possible future use --
+ >
+
+<!--======================= Tables =======================================-->
+
+<!-- IETF HTML table standard, see [RFC1942] -->
+
+<!--
+ The BORDER attribute sets the thickness of the frame around the
+ table. The default units are screen pixels.
+
+ The FRAME attribute specifies which parts of the frame around
+ the table should be rendered. The values are not the same as
+ CALS to avoid a name clash with the VALIGN attribute.
+
+ The value "border" is included for backwards compatibility with
+ <TABLE BORDER> which yields frame=border and border=implied
+ For <TABLE BORDER=1> you get border=1 and frame=implied. In this
+ case, it is appropriate to treat this as frame=border for backwards
+ compatibility with deployed browsers.
+-->
+<!ENTITY % TFrame "(void|above|below|hsides|lhs|rhs|vsides|box|border)">
+
+<!--
+ The RULES attribute defines which rules to draw between cells:
+
+ If RULES is absent then assume:
+ "none" if BORDER is absent or BORDER=0 otherwise "all"
+-->
+
+<!ENTITY % TRules "(none | groups | rows | cols | all)">
+
+<!-- horizontal placement of table relative to document -->
+<!ENTITY % TAlign "(left|center|right)">
+
+<!-- horizontal alignment attributes for cell contents -->
+<!ENTITY % cellhalign
+ "align (left|center|right|justify|char) #IMPLIED
+ char %Character; #IMPLIED -- alignment char, e.g. char=':' --
+ charoff %Length; #IMPLIED -- offset for alignment char --"
+ >
+
+<!-- vertical alignment attributes for cell contents -->
+<!ENTITY % cellvalign
+ "valign (top|middle|bottom|baseline) #IMPLIED"
+ >
+
+<!ELEMENT TABLE - -
+ (CAPTION?, (COL*|COLGROUP*), THEAD?, TFOOT?, TBODY+)>
+<!ELEMENT CAPTION - - (%inline;)* -- table caption -->
+<!ELEMENT THEAD - O (TR)+ -- table header -->
+<!ELEMENT TFOOT - O (TR)+ -- table footer -->
+<!ELEMENT TBODY O O (TR)+ -- table body -->
+<!ELEMENT COLGROUP - O (COL)* -- table column group -->
+<!ELEMENT COL - O EMPTY -- table column -->
+<!ELEMENT TR - O (TH|TD)+ -- table row -->
+<!ELEMENT (TH|TD) - O (%flow;)* -- table header cell, table data cell-->
+
+<!ATTLIST TABLE -- table element --
+ %attrs; -- %coreattrs, %i18n, %events --
+ summary %Text; #IMPLIED -- purpose/structure for speech output--
+ width %Length; #IMPLIED -- table width --
+ border %Pixels; #IMPLIED -- controls frame width around table --
+ frame %TFrame; #IMPLIED -- which parts of frame to render --
+ rules %TRules; #IMPLIED -- rulings between rows and cols --
+ cellspacing %Length; #IMPLIED -- spacing between cells --
+ cellpadding %Length; #IMPLIED -- spacing within cells --
+ %reserved; -- reserved for possible future use --
+ datapagesize CDATA #IMPLIED -- reserved for possible future use --
+ >
+
+
+<!ATTLIST CAPTION
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--
+COLGROUP groups a set of COL elements. It allows you to group
+several semantically related columns together.
+-->
+<!ATTLIST COLGROUP
+ %attrs; -- %coreattrs, %i18n, %events --
+ span NUMBER 1 -- default number of columns in group --
+ width %MultiLength; #IMPLIED -- default width for enclosed COLs --
+ %cellhalign; -- horizontal alignment in cells --
+ %cellvalign; -- vertical alignment in cells --
+ >
+
+<!--
+ COL elements define the alignment properties for cells in
+ one or more columns.
+
+ The WIDTH attribute specifies the width of the columns, e.g.
+
+ width=64 width in screen pixels
+ width=0.5* relative width of 0.5
+
+ The SPAN attribute causes the attributes of one
+ COL element to apply to more than one column.
+-->
+<!ATTLIST COL -- column groups and properties --
+ %attrs; -- %coreattrs, %i18n, %events --
+ span NUMBER 1 -- COL attributes affect N columns --
+ width %MultiLength; #IMPLIED -- column width specification --
+ %cellhalign; -- horizontal alignment in cells --
+ %cellvalign; -- vertical alignment in cells --
+ >
+
+<!--
+ Use THEAD to duplicate headers when breaking table
+ across page boundaries, or for static headers when
+ TBODY sections are rendered in scrolling panel.
+
+ Use TFOOT to duplicate footers when breaking table
+ across page boundaries, or for static footers when
+ TBODY sections are rendered in scrolling panel.
+
+ Use multiple TBODY sections when rules are needed
+ between groups of table rows.
+-->
+<!ATTLIST (THEAD|TBODY|TFOOT) -- table section --
+ %attrs; -- %coreattrs, %i18n, %events --
+ %cellhalign; -- horizontal alignment in cells --
+ %cellvalign; -- vertical alignment in cells --
+ >
+
+<!ATTLIST TR -- table row --
+ %attrs; -- %coreattrs, %i18n, %events --
+ %cellhalign; -- horizontal alignment in cells --
+ %cellvalign; -- vertical alignment in cells --
+ >
+
+
+<!-- Scope is simpler than headers attribute for common tables -->
+<!ENTITY % Scope "(row|col|rowgroup|colgroup)">
+
+<!-- TH is for headers, TD for data, but for cells acting as both use TD -->
+<!ATTLIST (TH|TD) -- header or data cell --
+ %attrs; -- %coreattrs, %i18n, %events --
+ abbr %Text; #IMPLIED -- abbreviation for header cell --
+ axis CDATA #IMPLIED -- comma-separated list of related headers--
+ headers IDREFS #IMPLIED -- list of id's for header cells --
+ scope %Scope; #IMPLIED -- scope covered by header cells --
+ rowspan NUMBER 1 -- number of rows spanned by cell --
+ colspan NUMBER 1 -- number of cols spanned by cell --
+ %cellhalign; -- horizontal alignment in cells --
+ %cellvalign; -- vertical alignment in cells --
+ >
+
+
+<!--================ Document Head =======================================-->
+<!-- %head.misc; defined earlier on as "SCRIPT|STYLE|META|LINK|OBJECT" -->
+<!ENTITY % head.content "TITLE & BASE?">
+
+<!ELEMENT HEAD O O (%head.content;) +(%head.misc;) -- document head -->
+<!ATTLIST HEAD
+ %i18n; -- lang, dir --
+ profile %URI; #IMPLIED -- named dictionary of meta info --
+ >
+
+<!-- The TITLE element is not considered part of the flow of text.
+ It should be displayed, for example as the page header or
+ window title. Exactly one title is required per document.
+ -->
+<!ELEMENT TITLE - - (#PCDATA) -(%head.misc;) -- document title -->
+<!ATTLIST TITLE %i18n>
+
+
+<!ELEMENT BASE - O EMPTY -- document base URI -->
+<!ATTLIST BASE
+ href %URI; #REQUIRED -- URI that acts as base URI --
+ >
+
+<!ELEMENT META - O EMPTY -- generic metainformation -->
+<!ATTLIST META
+ %i18n; -- lang, dir, for use with content --
+ http-equiv NAME #IMPLIED -- HTTP response header name --
+ name NAME #IMPLIED -- metainformation name --
+ content CDATA #REQUIRED -- associated information --
+ scheme CDATA #IMPLIED -- select form of content --
+ >
+
+<!ELEMENT STYLE - - %StyleSheet -- style info -->
+<!ATTLIST STYLE
+ %i18n; -- lang, dir, for use with title --
+ type %ContentType; #REQUIRED -- content type of style language --
+ media %MediaDesc; #IMPLIED -- designed for use with these media --
+ title %Text; #IMPLIED -- advisory title --
+ >
+
+<!ELEMENT SCRIPT - - %Script; -- script statements -->
+<!ATTLIST SCRIPT
+ charset %Charset; #IMPLIED -- char encoding of linked resource --
+ type %ContentType; #REQUIRED -- content type of script language --
+ src %URI; #IMPLIED -- URI for an external script --
+ defer (defer) #IMPLIED -- UA may defer execution of script --
+ event CDATA #IMPLIED -- reserved for possible future use --
+ for %URI; #IMPLIED -- reserved for possible future use --
+ >
+
+<!ELEMENT NOSCRIPT - - (%block;)+
+ -- alternate content container for non script-based rendering -->
+<!ATTLIST NOSCRIPT
+ %attrs; -- %coreattrs, %i18n, %events --
+ >
+
+<!--================ Document Structure ==================================-->
+<!ENTITY % html.content "HEAD, BODY">
+
+<!ELEMENT HTML O O (%html.content;) -- document root element -->
+<!ATTLIST HTML
+ %i18n; -- lang, dir --
+ >
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/switch-bench.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/switch-bench.js b/node_modules/sax/examples/switch-bench.js
new file mode 100755
index 0000000..4d3cf14
--- /dev/null
+++ b/node_modules/sax/examples/switch-bench.js
@@ -0,0 +1,45 @@
+#!/usr/local/bin/node-bench
+
+var Promise = require("events").Promise;
+
+var xml = require("posix").cat("test.xml").wait(),
+ path = require("path"),
+ sax = require("../lib/sax"),
+ saxT = require("../lib/sax-trampoline"),
+
+ parser = sax.parser(false, {trim:true}),
+ parserT = saxT.parser(false, {trim:true}),
+
+ sys = require("sys");
+
+
+var count = exports.stepsPerLap = 500,
+ l = xml.length,
+ runs = 0;
+exports.countPerLap = 1000;
+exports.compare = {
+ "switch" : function () {
+ // sys.debug("switch runs: "+runs++);
+ // for (var x = 0; x < l; x += 1000) {
+ // parser.write(xml.substr(x, 1000))
+ // }
+ // for (var i = 0; i < count; i ++) {
+ parser.write(xml);
+ parser.close();
+ // }
+ // done();
+ },
+ trampoline : function () {
+ // sys.debug("trampoline runs: "+runs++);
+ // for (var x = 0; x < l; x += 1000) {
+ // parserT.write(xml.substr(x, 1000))
+ // }
+ // for (var i = 0; i < count; i ++) {
+ parserT.write(xml);
+ parserT.close();
+ // }
+ // done();
+ },
+};
+
+sys.debug("rock and roll...");
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/test.html
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/test.html b/node_modules/sax/examples/test.html
new file mode 100644
index 0000000..61f8f1a
--- /dev/null
+++ b/node_modules/sax/examples/test.html
@@ -0,0 +1,15 @@
+<!doctype html>
+
+<html lang="en">
+<head>
+ <title>testing the parser</title>
+</head>
+<body>
+
+<p>hello
+
+<script>
+
+</script>
+</body>
+</html>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[56/59] [abbrv] mac commit: updated .ratignore
Posted by st...@apache.org.
updated .ratignore
Project: http://git-wip-us.apache.org/repos/asf/cordova-osx/repo
Commit: http://git-wip-us.apache.org/repos/asf/cordova-osx/commit/e678ba9f
Tree: http://git-wip-us.apache.org/repos/asf/cordova-osx/tree/e678ba9f
Diff: http://git-wip-us.apache.org/repos/asf/cordova-osx/diff/e678ba9f
Branch: refs/heads/4.0.x
Commit: e678ba9f856d8ae89d37668de30ce52fa17730b4
Parents: 562f466
Author: Steve Gill <st...@gmail.com>
Authored: Thu Mar 3 15:20:34 2016 -0800
Committer: Steve Gill <st...@gmail.com>
Committed: Thu Mar 3 15:42:46 2016 -0800
----------------------------------------------------------------------
.ratignore | 2 ++
1 file changed, 2 insertions(+)
----------------------------------------------------------------------
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/e678ba9f/.ratignore
----------------------------------------------------------------------
diff --git a/.ratignore b/.ratignore
index 66b6d08..a4494e8 100644
--- a/.ratignore
+++ b/.ratignore
@@ -7,3 +7,5 @@ NSData+Base64.m
# AppIcon.appiconset
Contents.json
+
+cdv-test-project
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[04/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/semver.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/semver.js b/node_modules/semver/semver.js
new file mode 100644
index 0000000..71795f6
--- /dev/null
+++ b/node_modules/semver/semver.js
@@ -0,0 +1,1188 @@
+exports = module.exports = SemVer;
+
+// The debug function is excluded entirely from the minified version.
+/* nomin */ var debug;
+/* nomin */ if (typeof process === 'object' &&
+ /* nomin */ process.env &&
+ /* nomin */ process.env.NODE_DEBUG &&
+ /* nomin */ /\bsemver\b/i.test(process.env.NODE_DEBUG))
+ /* nomin */ debug = function() {
+ /* nomin */ var args = Array.prototype.slice.call(arguments, 0);
+ /* nomin */ args.unshift('SEMVER');
+ /* nomin */ console.log.apply(console, args);
+ /* nomin */ };
+/* nomin */ else
+ /* nomin */ debug = function() {};
+
+// Note: this is the semver.org version of the spec that it implements
+// Not necessarily the package version of this code.
+exports.SEMVER_SPEC_VERSION = '2.0.0';
+
+var MAX_LENGTH = 256;
+var MAX_SAFE_INTEGER = Number.MAX_SAFE_INTEGER || 9007199254740991;
+
+// The actual regexps go on exports.re
+var re = exports.re = [];
+var src = exports.src = [];
+var R = 0;
+
+// The following Regular Expressions can be used for tokenizing,
+// validating, and parsing SemVer version strings.
+
+// ## Numeric Identifier
+// A single `0`, or a non-zero digit followed by zero or more digits.
+
+var NUMERICIDENTIFIER = R++;
+src[NUMERICIDENTIFIER] = '0|[1-9]\\d*';
+var NUMERICIDENTIFIERLOOSE = R++;
+src[NUMERICIDENTIFIERLOOSE] = '[0-9]+';
+
+
+// ## Non-numeric Identifier
+// Zero or more digits, followed by a letter or hyphen, and then zero or
+// more letters, digits, or hyphens.
+
+var NONNUMERICIDENTIFIER = R++;
+src[NONNUMERICIDENTIFIER] = '\\d*[a-zA-Z-][a-zA-Z0-9-]*';
+
+
+// ## Main Version
+// Three dot-separated numeric identifiers.
+
+var MAINVERSION = R++;
+src[MAINVERSION] = '(' + src[NUMERICIDENTIFIER] + ')\\.' +
+ '(' + src[NUMERICIDENTIFIER] + ')\\.' +
+ '(' + src[NUMERICIDENTIFIER] + ')';
+
+var MAINVERSIONLOOSE = R++;
+src[MAINVERSIONLOOSE] = '(' + src[NUMERICIDENTIFIERLOOSE] + ')\\.' +
+ '(' + src[NUMERICIDENTIFIERLOOSE] + ')\\.' +
+ '(' + src[NUMERICIDENTIFIERLOOSE] + ')';
+
+// ## Pre-release Version Identifier
+// A numeric identifier, or a non-numeric identifier.
+
+var PRERELEASEIDENTIFIER = R++;
+src[PRERELEASEIDENTIFIER] = '(?:' + src[NUMERICIDENTIFIER] +
+ '|' + src[NONNUMERICIDENTIFIER] + ')';
+
+var PRERELEASEIDENTIFIERLOOSE = R++;
+src[PRERELEASEIDENTIFIERLOOSE] = '(?:' + src[NUMERICIDENTIFIERLOOSE] +
+ '|' + src[NONNUMERICIDENTIFIER] + ')';
+
+
+// ## Pre-release Version
+// Hyphen, followed by one or more dot-separated pre-release version
+// identifiers.
+
+var PRERELEASE = R++;
+src[PRERELEASE] = '(?:-(' + src[PRERELEASEIDENTIFIER] +
+ '(?:\\.' + src[PRERELEASEIDENTIFIER] + ')*))';
+
+var PRERELEASELOOSE = R++;
+src[PRERELEASELOOSE] = '(?:-?(' + src[PRERELEASEIDENTIFIERLOOSE] +
+ '(?:\\.' + src[PRERELEASEIDENTIFIERLOOSE] + ')*))';
+
+// ## Build Metadata Identifier
+// Any combination of digits, letters, or hyphens.
+
+var BUILDIDENTIFIER = R++;
+src[BUILDIDENTIFIER] = '[0-9A-Za-z-]+';
+
+// ## Build Metadata
+// Plus sign, followed by one or more period-separated build metadata
+// identifiers.
+
+var BUILD = R++;
+src[BUILD] = '(?:\\+(' + src[BUILDIDENTIFIER] +
+ '(?:\\.' + src[BUILDIDENTIFIER] + ')*))';
+
+
+// ## Full Version String
+// A main version, followed optionally by a pre-release version and
+// build metadata.
+
+// Note that the only major, minor, patch, and pre-release sections of
+// the version string are capturing groups. The build metadata is not a
+// capturing group, because it should not ever be used in version
+// comparison.
+
+var FULL = R++;
+var FULLPLAIN = 'v?' + src[MAINVERSION] +
+ src[PRERELEASE] + '?' +
+ src[BUILD] + '?';
+
+src[FULL] = '^' + FULLPLAIN + '$';
+
+// like full, but allows v1.2.3 and =1.2.3, which people do sometimes.
+// also, 1.0.0alpha1 (prerelease without the hyphen) which is pretty
+// common in the npm registry.
+var LOOSEPLAIN = '[v=\\s]*' + src[MAINVERSIONLOOSE] +
+ src[PRERELEASELOOSE] + '?' +
+ src[BUILD] + '?';
+
+var LOOSE = R++;
+src[LOOSE] = '^' + LOOSEPLAIN + '$';
+
+var GTLT = R++;
+src[GTLT] = '((?:<|>)?=?)';
+
+// Something like "2.*" or "1.2.x".
+// Note that "x.x" is a valid xRange identifer, meaning "any version"
+// Only the first item is strictly required.
+var XRANGEIDENTIFIERLOOSE = R++;
+src[XRANGEIDENTIFIERLOOSE] = src[NUMERICIDENTIFIERLOOSE] + '|x|X|\\*';
+var XRANGEIDENTIFIER = R++;
+src[XRANGEIDENTIFIER] = src[NUMERICIDENTIFIER] + '|x|X|\\*';
+
+var XRANGEPLAIN = R++;
+src[XRANGEPLAIN] = '[v=\\s]*(' + src[XRANGEIDENTIFIER] + ')' +
+ '(?:\\.(' + src[XRANGEIDENTIFIER] + ')' +
+ '(?:\\.(' + src[XRANGEIDENTIFIER] + ')' +
+ '(?:' + src[PRERELEASE] + ')?' +
+ src[BUILD] + '?' +
+ ')?)?';
+
+var XRANGEPLAINLOOSE = R++;
+src[XRANGEPLAINLOOSE] = '[v=\\s]*(' + src[XRANGEIDENTIFIERLOOSE] + ')' +
+ '(?:\\.(' + src[XRANGEIDENTIFIERLOOSE] + ')' +
+ '(?:\\.(' + src[XRANGEIDENTIFIERLOOSE] + ')' +
+ '(?:' + src[PRERELEASELOOSE] + ')?' +
+ src[BUILD] + '?' +
+ ')?)?';
+
+var XRANGE = R++;
+src[XRANGE] = '^' + src[GTLT] + '\\s*' + src[XRANGEPLAIN] + '$';
+var XRANGELOOSE = R++;
+src[XRANGELOOSE] = '^' + src[GTLT] + '\\s*' + src[XRANGEPLAINLOOSE] + '$';
+
+// Tilde ranges.
+// Meaning is "reasonably at or greater than"
+var LONETILDE = R++;
+src[LONETILDE] = '(?:~>?)';
+
+var TILDETRIM = R++;
+src[TILDETRIM] = '(\\s*)' + src[LONETILDE] + '\\s+';
+re[TILDETRIM] = new RegExp(src[TILDETRIM], 'g');
+var tildeTrimReplace = '$1~';
+
+var TILDE = R++;
+src[TILDE] = '^' + src[LONETILDE] + src[XRANGEPLAIN] + '$';
+var TILDELOOSE = R++;
+src[TILDELOOSE] = '^' + src[LONETILDE] + src[XRANGEPLAINLOOSE] + '$';
+
+// Caret ranges.
+// Meaning is "at least and backwards compatible with"
+var LONECARET = R++;
+src[LONECARET] = '(?:\\^)';
+
+var CARETTRIM = R++;
+src[CARETTRIM] = '(\\s*)' + src[LONECARET] + '\\s+';
+re[CARETTRIM] = new RegExp(src[CARETTRIM], 'g');
+var caretTrimReplace = '$1^';
+
+var CARET = R++;
+src[CARET] = '^' + src[LONECARET] + src[XRANGEPLAIN] + '$';
+var CARETLOOSE = R++;
+src[CARETLOOSE] = '^' + src[LONECARET] + src[XRANGEPLAINLOOSE] + '$';
+
+// A simple gt/lt/eq thing, or just "" to indicate "any version"
+var COMPARATORLOOSE = R++;
+src[COMPARATORLOOSE] = '^' + src[GTLT] + '\\s*(' + LOOSEPLAIN + ')$|^$';
+var COMPARATOR = R++;
+src[COMPARATOR] = '^' + src[GTLT] + '\\s*(' + FULLPLAIN + ')$|^$';
+
+
+// An expression to strip any whitespace between the gtlt and the thing
+// it modifies, so that `> 1.2.3` ==> `>1.2.3`
+var COMPARATORTRIM = R++;
+src[COMPARATORTRIM] = '(\\s*)' + src[GTLT] +
+ '\\s*(' + LOOSEPLAIN + '|' + src[XRANGEPLAIN] + ')';
+
+// this one has to use the /g flag
+re[COMPARATORTRIM] = new RegExp(src[COMPARATORTRIM], 'g');
+var comparatorTrimReplace = '$1$2$3';
+
+
+// Something like `1.2.3 - 1.2.4`
+// Note that these all use the loose form, because they'll be
+// checked against either the strict or loose comparator form
+// later.
+var HYPHENRANGE = R++;
+src[HYPHENRANGE] = '^\\s*(' + src[XRANGEPLAIN] + ')' +
+ '\\s+-\\s+' +
+ '(' + src[XRANGEPLAIN] + ')' +
+ '\\s*$';
+
+var HYPHENRANGELOOSE = R++;
+src[HYPHENRANGELOOSE] = '^\\s*(' + src[XRANGEPLAINLOOSE] + ')' +
+ '\\s+-\\s+' +
+ '(' + src[XRANGEPLAINLOOSE] + ')' +
+ '\\s*$';
+
+// Star ranges basically just allow anything at all.
+var STAR = R++;
+src[STAR] = '(<|>)?=?\\s*\\*';
+
+// Compile to actual regexp objects.
+// All are flag-free, unless they were created above with a flag.
+for (var i = 0; i < R; i++) {
+ debug(i, src[i]);
+ if (!re[i])
+ re[i] = new RegExp(src[i]);
+}
+
+exports.parse = parse;
+function parse(version, loose) {
+ if (version instanceof SemVer)
+ return version;
+
+ if (typeof version !== 'string')
+ return null;
+
+ if (version.length > MAX_LENGTH)
+ return null;
+
+ var r = loose ? re[LOOSE] : re[FULL];
+ if (!r.test(version))
+ return null;
+
+ try {
+ return new SemVer(version, loose);
+ } catch (er) {
+ return null;
+ }
+}
+
+exports.valid = valid;
+function valid(version, loose) {
+ var v = parse(version, loose);
+ return v ? v.version : null;
+}
+
+
+exports.clean = clean;
+function clean(version, loose) {
+ var s = parse(version.trim().replace(/^[=v]+/, ''), loose);
+ return s ? s.version : null;
+}
+
+exports.SemVer = SemVer;
+
+function SemVer(version, loose) {
+ if (version instanceof SemVer) {
+ if (version.loose === loose)
+ return version;
+ else
+ version = version.version;
+ } else if (typeof version !== 'string') {
+ throw new TypeError('Invalid Version: ' + version);
+ }
+
+ if (version.length > MAX_LENGTH)
+ throw new TypeError('version is longer than ' + MAX_LENGTH + ' characters')
+
+ if (!(this instanceof SemVer))
+ return new SemVer(version, loose);
+
+ debug('SemVer', version, loose);
+ this.loose = loose;
+ var m = version.trim().match(loose ? re[LOOSE] : re[FULL]);
+
+ if (!m)
+ throw new TypeError('Invalid Version: ' + version);
+
+ this.raw = version;
+
+ // these are actually numbers
+ this.major = +m[1];
+ this.minor = +m[2];
+ this.patch = +m[3];
+
+ if (this.major > MAX_SAFE_INTEGER || this.major < 0)
+ throw new TypeError('Invalid major version')
+
+ if (this.minor > MAX_SAFE_INTEGER || this.minor < 0)
+ throw new TypeError('Invalid minor version')
+
+ if (this.patch > MAX_SAFE_INTEGER || this.patch < 0)
+ throw new TypeError('Invalid patch version')
+
+ // numberify any prerelease numeric ids
+ if (!m[4])
+ this.prerelease = [];
+ else
+ this.prerelease = m[4].split('.').map(function(id) {
+ if (/^[0-9]+$/.test(id)) {
+ var num = +id
+ if (num >= 0 && num < MAX_SAFE_INTEGER)
+ return num
+ }
+ return id;
+ });
+
+ this.build = m[5] ? m[5].split('.') : [];
+ this.format();
+}
+
+SemVer.prototype.format = function() {
+ this.version = this.major + '.' + this.minor + '.' + this.patch;
+ if (this.prerelease.length)
+ this.version += '-' + this.prerelease.join('.');
+ return this.version;
+};
+
+SemVer.prototype.toString = function() {
+ return this.version;
+};
+
+SemVer.prototype.compare = function(other) {
+ debug('SemVer.compare', this.version, this.loose, other);
+ if (!(other instanceof SemVer))
+ other = new SemVer(other, this.loose);
+
+ return this.compareMain(other) || this.comparePre(other);
+};
+
+SemVer.prototype.compareMain = function(other) {
+ if (!(other instanceof SemVer))
+ other = new SemVer(other, this.loose);
+
+ return compareIdentifiers(this.major, other.major) ||
+ compareIdentifiers(this.minor, other.minor) ||
+ compareIdentifiers(this.patch, other.patch);
+};
+
+SemVer.prototype.comparePre = function(other) {
+ if (!(other instanceof SemVer))
+ other = new SemVer(other, this.loose);
+
+ // NOT having a prerelease is > having one
+ if (this.prerelease.length && !other.prerelease.length)
+ return -1;
+ else if (!this.prerelease.length && other.prerelease.length)
+ return 1;
+ else if (!this.prerelease.length && !other.prerelease.length)
+ return 0;
+
+ var i = 0;
+ do {
+ var a = this.prerelease[i];
+ var b = other.prerelease[i];
+ debug('prerelease compare', i, a, b);
+ if (a === undefined && b === undefined)
+ return 0;
+ else if (b === undefined)
+ return 1;
+ else if (a === undefined)
+ return -1;
+ else if (a === b)
+ continue;
+ else
+ return compareIdentifiers(a, b);
+ } while (++i);
+};
+
+// preminor will bump the version up to the next minor release, and immediately
+// down to pre-release. premajor and prepatch work the same way.
+SemVer.prototype.inc = function(release, identifier) {
+ switch (release) {
+ case 'premajor':
+ this.prerelease.length = 0;
+ this.patch = 0;
+ this.minor = 0;
+ this.major++;
+ this.inc('pre', identifier);
+ break;
+ case 'preminor':
+ this.prerelease.length = 0;
+ this.patch = 0;
+ this.minor++;
+ this.inc('pre', identifier);
+ break;
+ case 'prepatch':
+ // If this is already a prerelease, it will bump to the next version
+ // drop any prereleases that might already exist, since they are not
+ // relevant at this point.
+ this.prerelease.length = 0;
+ this.inc('patch', identifier);
+ this.inc('pre', identifier);
+ break;
+ // If the input is a non-prerelease version, this acts the same as
+ // prepatch.
+ case 'prerelease':
+ if (this.prerelease.length === 0)
+ this.inc('patch', identifier);
+ this.inc('pre', identifier);
+ break;
+
+ case 'major':
+ // If this is a pre-major version, bump up to the same major version.
+ // Otherwise increment major.
+ // 1.0.0-5 bumps to 1.0.0
+ // 1.1.0 bumps to 2.0.0
+ if (this.minor !== 0 || this.patch !== 0 || this.prerelease.length === 0)
+ this.major++;
+ this.minor = 0;
+ this.patch = 0;
+ this.prerelease = [];
+ break;
+ case 'minor':
+ // If this is a pre-minor version, bump up to the same minor version.
+ // Otherwise increment minor.
+ // 1.2.0-5 bumps to 1.2.0
+ // 1.2.1 bumps to 1.3.0
+ if (this.patch !== 0 || this.prerelease.length === 0)
+ this.minor++;
+ this.patch = 0;
+ this.prerelease = [];
+ break;
+ case 'patch':
+ // If this is not a pre-release version, it will increment the patch.
+ // If it is a pre-release it will bump up to the same patch version.
+ // 1.2.0-5 patches to 1.2.0
+ // 1.2.0 patches to 1.2.1
+ if (this.prerelease.length === 0)
+ this.patch++;
+ this.prerelease = [];
+ break;
+ // This probably shouldn't be used publicly.
+ // 1.0.0 "pre" would become 1.0.0-0 which is the wrong direction.
+ case 'pre':
+ if (this.prerelease.length === 0)
+ this.prerelease = [0];
+ else {
+ var i = this.prerelease.length;
+ while (--i >= 0) {
+ if (typeof this.prerelease[i] === 'number') {
+ this.prerelease[i]++;
+ i = -2;
+ }
+ }
+ if (i === -1) // didn't increment anything
+ this.prerelease.push(0);
+ }
+ if (identifier) {
+ // 1.2.0-beta.1 bumps to 1.2.0-beta.2,
+ // 1.2.0-beta.fooblz or 1.2.0-beta bumps to 1.2.0-beta.0
+ if (this.prerelease[0] === identifier) {
+ if (isNaN(this.prerelease[1]))
+ this.prerelease = [identifier, 0];
+ } else
+ this.prerelease = [identifier, 0];
+ }
+ break;
+
+ default:
+ throw new Error('invalid increment argument: ' + release);
+ }
+ this.format();
+ this.raw = this.version;
+ return this;
+};
+
+exports.inc = inc;
+function inc(version, release, loose, identifier) {
+ if (typeof(loose) === 'string') {
+ identifier = loose;
+ loose = undefined;
+ }
+
+ try {
+ return new SemVer(version, loose).inc(release, identifier).version;
+ } catch (er) {
+ return null;
+ }
+}
+
+exports.diff = diff;
+function diff(version1, version2) {
+ if (eq(version1, version2)) {
+ return null;
+ } else {
+ var v1 = parse(version1);
+ var v2 = parse(version2);
+ if (v1.prerelease.length || v2.prerelease.length) {
+ for (var key in v1) {
+ if (key === 'major' || key === 'minor' || key === 'patch') {
+ if (v1[key] !== v2[key]) {
+ return 'pre'+key;
+ }
+ }
+ }
+ return 'prerelease';
+ }
+ for (var key in v1) {
+ if (key === 'major' || key === 'minor' || key === 'patch') {
+ if (v1[key] !== v2[key]) {
+ return key;
+ }
+ }
+ }
+ }
+}
+
+exports.compareIdentifiers = compareIdentifiers;
+
+var numeric = /^[0-9]+$/;
+function compareIdentifiers(a, b) {
+ var anum = numeric.test(a);
+ var bnum = numeric.test(b);
+
+ if (anum && bnum) {
+ a = +a;
+ b = +b;
+ }
+
+ return (anum && !bnum) ? -1 :
+ (bnum && !anum) ? 1 :
+ a < b ? -1 :
+ a > b ? 1 :
+ 0;
+}
+
+exports.rcompareIdentifiers = rcompareIdentifiers;
+function rcompareIdentifiers(a, b) {
+ return compareIdentifiers(b, a);
+}
+
+exports.major = major;
+function major(a, loose) {
+ return new SemVer(a, loose).major;
+}
+
+exports.minor = minor;
+function minor(a, loose) {
+ return new SemVer(a, loose).minor;
+}
+
+exports.patch = patch;
+function patch(a, loose) {
+ return new SemVer(a, loose).patch;
+}
+
+exports.compare = compare;
+function compare(a, b, loose) {
+ return new SemVer(a, loose).compare(b);
+}
+
+exports.compareLoose = compareLoose;
+function compareLoose(a, b) {
+ return compare(a, b, true);
+}
+
+exports.rcompare = rcompare;
+function rcompare(a, b, loose) {
+ return compare(b, a, loose);
+}
+
+exports.sort = sort;
+function sort(list, loose) {
+ return list.sort(function(a, b) {
+ return exports.compare(a, b, loose);
+ });
+}
+
+exports.rsort = rsort;
+function rsort(list, loose) {
+ return list.sort(function(a, b) {
+ return exports.rcompare(a, b, loose);
+ });
+}
+
+exports.gt = gt;
+function gt(a, b, loose) {
+ return compare(a, b, loose) > 0;
+}
+
+exports.lt = lt;
+function lt(a, b, loose) {
+ return compare(a, b, loose) < 0;
+}
+
+exports.eq = eq;
+function eq(a, b, loose) {
+ return compare(a, b, loose) === 0;
+}
+
+exports.neq = neq;
+function neq(a, b, loose) {
+ return compare(a, b, loose) !== 0;
+}
+
+exports.gte = gte;
+function gte(a, b, loose) {
+ return compare(a, b, loose) >= 0;
+}
+
+exports.lte = lte;
+function lte(a, b, loose) {
+ return compare(a, b, loose) <= 0;
+}
+
+exports.cmp = cmp;
+function cmp(a, op, b, loose) {
+ var ret;
+ switch (op) {
+ case '===':
+ if (typeof a === 'object') a = a.version;
+ if (typeof b === 'object') b = b.version;
+ ret = a === b;
+ break;
+ case '!==':
+ if (typeof a === 'object') a = a.version;
+ if (typeof b === 'object') b = b.version;
+ ret = a !== b;
+ break;
+ case '': case '=': case '==': ret = eq(a, b, loose); break;
+ case '!=': ret = neq(a, b, loose); break;
+ case '>': ret = gt(a, b, loose); break;
+ case '>=': ret = gte(a, b, loose); break;
+ case '<': ret = lt(a, b, loose); break;
+ case '<=': ret = lte(a, b, loose); break;
+ default: throw new TypeError('Invalid operator: ' + op);
+ }
+ return ret;
+}
+
+exports.Comparator = Comparator;
+function Comparator(comp, loose) {
+ if (comp instanceof Comparator) {
+ if (comp.loose === loose)
+ return comp;
+ else
+ comp = comp.value;
+ }
+
+ if (!(this instanceof Comparator))
+ return new Comparator(comp, loose);
+
+ debug('comparator', comp, loose);
+ this.loose = loose;
+ this.parse(comp);
+
+ if (this.semver === ANY)
+ this.value = '';
+ else
+ this.value = this.operator + this.semver.version;
+
+ debug('comp', this);
+}
+
+var ANY = {};
+Comparator.prototype.parse = function(comp) {
+ var r = this.loose ? re[COMPARATORLOOSE] : re[COMPARATOR];
+ var m = comp.match(r);
+
+ if (!m)
+ throw new TypeError('Invalid comparator: ' + comp);
+
+ this.operator = m[1];
+ if (this.operator === '=')
+ this.operator = '';
+
+ // if it literally is just '>' or '' then allow anything.
+ if (!m[2])
+ this.semver = ANY;
+ else
+ this.semver = new SemVer(m[2], this.loose);
+};
+
+Comparator.prototype.toString = function() {
+ return this.value;
+};
+
+Comparator.prototype.test = function(version) {
+ debug('Comparator.test', version, this.loose);
+
+ if (this.semver === ANY)
+ return true;
+
+ if (typeof version === 'string')
+ version = new SemVer(version, this.loose);
+
+ return cmp(version, this.operator, this.semver, this.loose);
+};
+
+
+exports.Range = Range;
+function Range(range, loose) {
+ if ((range instanceof Range) && range.loose === loose)
+ return range;
+
+ if (!(this instanceof Range))
+ return new Range(range, loose);
+
+ this.loose = loose;
+
+ // First, split based on boolean or ||
+ this.raw = range;
+ this.set = range.split(/\s*\|\|\s*/).map(function(range) {
+ return this.parseRange(range.trim());
+ }, this).filter(function(c) {
+ // throw out any that are not relevant for whatever reason
+ return c.length;
+ });
+
+ if (!this.set.length) {
+ throw new TypeError('Invalid SemVer Range: ' + range);
+ }
+
+ this.format();
+}
+
+Range.prototype.format = function() {
+ this.range = this.set.map(function(comps) {
+ return comps.join(' ').trim();
+ }).join('||').trim();
+ return this.range;
+};
+
+Range.prototype.toString = function() {
+ return this.range;
+};
+
+Range.prototype.parseRange = function(range) {
+ var loose = this.loose;
+ range = range.trim();
+ debug('range', range, loose);
+ // `1.2.3 - 1.2.4` => `>=1.2.3 <=1.2.4`
+ var hr = loose ? re[HYPHENRANGELOOSE] : re[HYPHENRANGE];
+ range = range.replace(hr, hyphenReplace);
+ debug('hyphen replace', range);
+ // `> 1.2.3 < 1.2.5` => `>1.2.3 <1.2.5`
+ range = range.replace(re[COMPARATORTRIM], comparatorTrimReplace);
+ debug('comparator trim', range, re[COMPARATORTRIM]);
+
+ // `~ 1.2.3` => `~1.2.3`
+ range = range.replace(re[TILDETRIM], tildeTrimReplace);
+
+ // `^ 1.2.3` => `^1.2.3`
+ range = range.replace(re[CARETTRIM], caretTrimReplace);
+
+ // normalize spaces
+ range = range.split(/\s+/).join(' ');
+
+ // At this point, the range is completely trimmed and
+ // ready to be split into comparators.
+
+ var compRe = loose ? re[COMPARATORLOOSE] : re[COMPARATOR];
+ var set = range.split(' ').map(function(comp) {
+ return parseComparator(comp, loose);
+ }).join(' ').split(/\s+/);
+ if (this.loose) {
+ // in loose mode, throw out any that are not valid comparators
+ set = set.filter(function(comp) {
+ return !!comp.match(compRe);
+ });
+ }
+ set = set.map(function(comp) {
+ return new Comparator(comp, loose);
+ });
+
+ return set;
+};
+
+// Mostly just for testing and legacy API reasons
+exports.toComparators = toComparators;
+function toComparators(range, loose) {
+ return new Range(range, loose).set.map(function(comp) {
+ return comp.map(function(c) {
+ return c.value;
+ }).join(' ').trim().split(' ');
+ });
+}
+
+// comprised of xranges, tildes, stars, and gtlt's at this point.
+// already replaced the hyphen ranges
+// turn into a set of JUST comparators.
+function parseComparator(comp, loose) {
+ debug('comp', comp);
+ comp = replaceCarets(comp, loose);
+ debug('caret', comp);
+ comp = replaceTildes(comp, loose);
+ debug('tildes', comp);
+ comp = replaceXRanges(comp, loose);
+ debug('xrange', comp);
+ comp = replaceStars(comp, loose);
+ debug('stars', comp);
+ return comp;
+}
+
+function isX(id) {
+ return !id || id.toLowerCase() === 'x' || id === '*';
+}
+
+// ~, ~> --> * (any, kinda silly)
+// ~2, ~2.x, ~2.x.x, ~>2, ~>2.x ~>2.x.x --> >=2.0.0 <3.0.0
+// ~2.0, ~2.0.x, ~>2.0, ~>2.0.x --> >=2.0.0 <2.1.0
+// ~1.2, ~1.2.x, ~>1.2, ~>1.2.x --> >=1.2.0 <1.3.0
+// ~1.2.3, ~>1.2.3 --> >=1.2.3 <1.3.0
+// ~1.2.0, ~>1.2.0 --> >=1.2.0 <1.3.0
+function replaceTildes(comp, loose) {
+ return comp.trim().split(/\s+/).map(function(comp) {
+ return replaceTilde(comp, loose);
+ }).join(' ');
+}
+
+function replaceTilde(comp, loose) {
+ var r = loose ? re[TILDELOOSE] : re[TILDE];
+ return comp.replace(r, function(_, M, m, p, pr) {
+ debug('tilde', comp, _, M, m, p, pr);
+ var ret;
+
+ if (isX(M))
+ ret = '';
+ else if (isX(m))
+ ret = '>=' + M + '.0.0 <' + (+M + 1) + '.0.0';
+ else if (isX(p))
+ // ~1.2 == >=1.2.0- <1.3.0-
+ ret = '>=' + M + '.' + m + '.0 <' + M + '.' + (+m + 1) + '.0';
+ else if (pr) {
+ debug('replaceTilde pr', pr);
+ if (pr.charAt(0) !== '-')
+ pr = '-' + pr;
+ ret = '>=' + M + '.' + m + '.' + p + pr +
+ ' <' + M + '.' + (+m + 1) + '.0';
+ } else
+ // ~1.2.3 == >=1.2.3 <1.3.0
+ ret = '>=' + M + '.' + m + '.' + p +
+ ' <' + M + '.' + (+m + 1) + '.0';
+
+ debug('tilde return', ret);
+ return ret;
+ });
+}
+
+// ^ --> * (any, kinda silly)
+// ^2, ^2.x, ^2.x.x --> >=2.0.0 <3.0.0
+// ^2.0, ^2.0.x --> >=2.0.0 <3.0.0
+// ^1.2, ^1.2.x --> >=1.2.0 <2.0.0
+// ^1.2.3 --> >=1.2.3 <2.0.0
+// ^1.2.0 --> >=1.2.0 <2.0.0
+function replaceCarets(comp, loose) {
+ return comp.trim().split(/\s+/).map(function(comp) {
+ return replaceCaret(comp, loose);
+ }).join(' ');
+}
+
+function replaceCaret(comp, loose) {
+ debug('caret', comp, loose);
+ var r = loose ? re[CARETLOOSE] : re[CARET];
+ return comp.replace(r, function(_, M, m, p, pr) {
+ debug('caret', comp, _, M, m, p, pr);
+ var ret;
+
+ if (isX(M))
+ ret = '';
+ else if (isX(m))
+ ret = '>=' + M + '.0.0 <' + (+M + 1) + '.0.0';
+ else if (isX(p)) {
+ if (M === '0')
+ ret = '>=' + M + '.' + m + '.0 <' + M + '.' + (+m + 1) + '.0';
+ else
+ ret = '>=' + M + '.' + m + '.0 <' + (+M + 1) + '.0.0';
+ } else if (pr) {
+ debug('replaceCaret pr', pr);
+ if (pr.charAt(0) !== '-')
+ pr = '-' + pr;
+ if (M === '0') {
+ if (m === '0')
+ ret = '>=' + M + '.' + m + '.' + p + pr +
+ ' <' + M + '.' + m + '.' + (+p + 1);
+ else
+ ret = '>=' + M + '.' + m + '.' + p + pr +
+ ' <' + M + '.' + (+m + 1) + '.0';
+ } else
+ ret = '>=' + M + '.' + m + '.' + p + pr +
+ ' <' + (+M + 1) + '.0.0';
+ } else {
+ debug('no pr');
+ if (M === '0') {
+ if (m === '0')
+ ret = '>=' + M + '.' + m + '.' + p +
+ ' <' + M + '.' + m + '.' + (+p + 1);
+ else
+ ret = '>=' + M + '.' + m + '.' + p +
+ ' <' + M + '.' + (+m + 1) + '.0';
+ } else
+ ret = '>=' + M + '.' + m + '.' + p +
+ ' <' + (+M + 1) + '.0.0';
+ }
+
+ debug('caret return', ret);
+ return ret;
+ });
+}
+
+function replaceXRanges(comp, loose) {
+ debug('replaceXRanges', comp, loose);
+ return comp.split(/\s+/).map(function(comp) {
+ return replaceXRange(comp, loose);
+ }).join(' ');
+}
+
+function replaceXRange(comp, loose) {
+ comp = comp.trim();
+ var r = loose ? re[XRANGELOOSE] : re[XRANGE];
+ return comp.replace(r, function(ret, gtlt, M, m, p, pr) {
+ debug('xRange', comp, ret, gtlt, M, m, p, pr);
+ var xM = isX(M);
+ var xm = xM || isX(m);
+ var xp = xm || isX(p);
+ var anyX = xp;
+
+ if (gtlt === '=' && anyX)
+ gtlt = '';
+
+ if (xM) {
+ if (gtlt === '>' || gtlt === '<') {
+ // nothing is allowed
+ ret = '<0.0.0';
+ } else {
+ // nothing is forbidden
+ ret = '*';
+ }
+ } else if (gtlt && anyX) {
+ // replace X with 0
+ if (xm)
+ m = 0;
+ if (xp)
+ p = 0;
+
+ if (gtlt === '>') {
+ // >1 => >=2.0.0
+ // >1.2 => >=1.3.0
+ // >1.2.3 => >= 1.2.4
+ gtlt = '>=';
+ if (xm) {
+ M = +M + 1;
+ m = 0;
+ p = 0;
+ } else if (xp) {
+ m = +m + 1;
+ p = 0;
+ }
+ } else if (gtlt === '<=') {
+ // <=0.7.x is actually <0.8.0, since any 0.7.x should
+ // pass. Similarly, <=7.x is actually <8.0.0, etc.
+ gtlt = '<'
+ if (xm)
+ M = +M + 1
+ else
+ m = +m + 1
+ }
+
+ ret = gtlt + M + '.' + m + '.' + p;
+ } else if (xm) {
+ ret = '>=' + M + '.0.0 <' + (+M + 1) + '.0.0';
+ } else if (xp) {
+ ret = '>=' + M + '.' + m + '.0 <' + M + '.' + (+m + 1) + '.0';
+ }
+
+ debug('xRange return', ret);
+
+ return ret;
+ });
+}
+
+// Because * is AND-ed with everything else in the comparator,
+// and '' means "any version", just remove the *s entirely.
+function replaceStars(comp, loose) {
+ debug('replaceStars', comp, loose);
+ // Looseness is ignored here. star is always as loose as it gets!
+ return comp.trim().replace(re[STAR], '');
+}
+
+// This function is passed to string.replace(re[HYPHENRANGE])
+// M, m, patch, prerelease, build
+// 1.2 - 3.4.5 => >=1.2.0 <=3.4.5
+// 1.2.3 - 3.4 => >=1.2.0 <3.5.0 Any 3.4.x will do
+// 1.2 - 3.4 => >=1.2.0 <3.5.0
+function hyphenReplace($0,
+ from, fM, fm, fp, fpr, fb,
+ to, tM, tm, tp, tpr, tb) {
+
+ if (isX(fM))
+ from = '';
+ else if (isX(fm))
+ from = '>=' + fM + '.0.0';
+ else if (isX(fp))
+ from = '>=' + fM + '.' + fm + '.0';
+ else
+ from = '>=' + from;
+
+ if (isX(tM))
+ to = '';
+ else if (isX(tm))
+ to = '<' + (+tM + 1) + '.0.0';
+ else if (isX(tp))
+ to = '<' + tM + '.' + (+tm + 1) + '.0';
+ else if (tpr)
+ to = '<=' + tM + '.' + tm + '.' + tp + '-' + tpr;
+ else
+ to = '<=' + to;
+
+ return (from + ' ' + to).trim();
+}
+
+
+// if ANY of the sets match ALL of its comparators, then pass
+Range.prototype.test = function(version) {
+ if (!version)
+ return false;
+
+ if (typeof version === 'string')
+ version = new SemVer(version, this.loose);
+
+ for (var i = 0; i < this.set.length; i++) {
+ if (testSet(this.set[i], version))
+ return true;
+ }
+ return false;
+};
+
+function testSet(set, version) {
+ for (var i = 0; i < set.length; i++) {
+ if (!set[i].test(version))
+ return false;
+ }
+
+ if (version.prerelease.length) {
+ // Find the set of versions that are allowed to have prereleases
+ // For example, ^1.2.3-pr.1 desugars to >=1.2.3-pr.1 <2.0.0
+ // That should allow `1.2.3-pr.2` to pass.
+ // However, `1.2.4-alpha.notready` should NOT be allowed,
+ // even though it's within the range set by the comparators.
+ for (var i = 0; i < set.length; i++) {
+ debug(set[i].semver);
+ if (set[i].semver === ANY)
+ continue;
+
+ if (set[i].semver.prerelease.length > 0) {
+ var allowed = set[i].semver;
+ if (allowed.major === version.major &&
+ allowed.minor === version.minor &&
+ allowed.patch === version.patch)
+ return true;
+ }
+ }
+
+ // Version has a -pre, but it's not one of the ones we like.
+ return false;
+ }
+
+ return true;
+}
+
+exports.satisfies = satisfies;
+function satisfies(version, range, loose) {
+ try {
+ range = new Range(range, loose);
+ } catch (er) {
+ return false;
+ }
+ return range.test(version);
+}
+
+exports.maxSatisfying = maxSatisfying;
+function maxSatisfying(versions, range, loose) {
+ return versions.filter(function(version) {
+ return satisfies(version, range, loose);
+ }).sort(function(a, b) {
+ return rcompare(a, b, loose);
+ })[0] || null;
+}
+
+exports.validRange = validRange;
+function validRange(range, loose) {
+ try {
+ // Return '*' instead of '' so that truthiness works.
+ // This will throw if it's invalid anyway
+ return new Range(range, loose).range || '*';
+ } catch (er) {
+ return null;
+ }
+}
+
+// Determine if version is less than all the versions possible in the range
+exports.ltr = ltr;
+function ltr(version, range, loose) {
+ return outside(version, range, '<', loose);
+}
+
+// Determine if version is greater than all the versions possible in the range.
+exports.gtr = gtr;
+function gtr(version, range, loose) {
+ return outside(version, range, '>', loose);
+}
+
+exports.outside = outside;
+function outside(version, range, hilo, loose) {
+ version = new SemVer(version, loose);
+ range = new Range(range, loose);
+
+ var gtfn, ltefn, ltfn, comp, ecomp;
+ switch (hilo) {
+ case '>':
+ gtfn = gt;
+ ltefn = lte;
+ ltfn = lt;
+ comp = '>';
+ ecomp = '>=';
+ break;
+ case '<':
+ gtfn = lt;
+ ltefn = gte;
+ ltfn = gt;
+ comp = '<';
+ ecomp = '<=';
+ break;
+ default:
+ throw new TypeError('Must provide a hilo val of "<" or ">"');
+ }
+
+ // If it satisifes the range it is not outside
+ if (satisfies(version, range, loose)) {
+ return false;
+ }
+
+ // From now on, variable terms are as if we're in "gtr" mode.
+ // but note that everything is flipped for the "ltr" function.
+
+ for (var i = 0; i < range.set.length; ++i) {
+ var comparators = range.set[i];
+
+ var high = null;
+ var low = null;
+
+ comparators.forEach(function(comparator) {
+ if (comparator.semver === ANY) {
+ comparator = new Comparator('>=0.0.0')
+ }
+ high = high || comparator;
+ low = low || comparator;
+ if (gtfn(comparator.semver, high.semver, loose)) {
+ high = comparator;
+ } else if (ltfn(comparator.semver, low.semver, loose)) {
+ low = comparator;
+ }
+ });
+
+ // If the edge version comparator has a operator then our version
+ // isn't outside it
+ if (high.operator === comp || high.operator === ecomp) {
+ return false;
+ }
+
+ // If the lowest version comparator has an operator and our version
+ // is less than it then it isn't higher than the range
+ if ((!low.operator || low.operator === comp) &&
+ ltefn(version, low.semver)) {
+ return false;
+ } else if (low.operator === ecomp && ltfn(version, low.semver)) {
+ return false;
+ }
+ }
+ return true;
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/test/big-numbers.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/test/big-numbers.js b/node_modules/semver/test/big-numbers.js
new file mode 100644
index 0000000..c051864
--- /dev/null
+++ b/node_modules/semver/test/big-numbers.js
@@ -0,0 +1,31 @@
+var test = require('tap').test
+var semver = require('../')
+
+test('long version is too long', function (t) {
+ var v = '1.2.' + new Array(256).join('1')
+ t.throws(function () {
+ new semver.SemVer(v)
+ })
+ t.equal(semver.valid(v, false), null)
+ t.equal(semver.valid(v, true), null)
+ t.equal(semver.inc(v, 'patch'), null)
+ t.end()
+})
+
+test('big number is like too long version', function (t) {
+ var v = '1.2.' + new Array(100).join('1')
+ t.throws(function () {
+ new semver.SemVer(v)
+ })
+ t.equal(semver.valid(v, false), null)
+ t.equal(semver.valid(v, true), null)
+ t.equal(semver.inc(v, 'patch'), null)
+ t.end()
+})
+
+test('parsing null does not throw', function (t) {
+ t.equal(semver.parse(null), null)
+ t.equal(semver.parse({}), null)
+ t.equal(semver.parse(new semver.SemVer('1.2.3')).version, '1.2.3')
+ t.end()
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/test/clean.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/test/clean.js b/node_modules/semver/test/clean.js
new file mode 100644
index 0000000..9e268de
--- /dev/null
+++ b/node_modules/semver/test/clean.js
@@ -0,0 +1,29 @@
+var tap = require('tap');
+var test = tap.test;
+var semver = require('../semver.js');
+var clean = semver.clean;
+
+test('\nclean tests', function(t) {
+ // [range, version]
+ // Version should be detectable despite extra characters
+ [
+ ['1.2.3', '1.2.3'],
+ [' 1.2.3 ', '1.2.3'],
+ [' 1.2.3-4 ', '1.2.3-4'],
+ [' 1.2.3-pre ', '1.2.3-pre'],
+ [' =v1.2.3 ', '1.2.3'],
+ ['v1.2.3', '1.2.3'],
+ [' v1.2.3 ', '1.2.3'],
+ ['\t1.2.3', '1.2.3'],
+ ['>1.2.3', null],
+ ['~1.2.3', null],
+ ['<=1.2.3', null],
+ ['1.2.x', null]
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var msg = 'clean(' + range + ') = ' + version;
+ t.equal(clean(range), version, msg);
+ });
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/test/gtr.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/test/gtr.js b/node_modules/semver/test/gtr.js
new file mode 100644
index 0000000..bbb8789
--- /dev/null
+++ b/node_modules/semver/test/gtr.js
@@ -0,0 +1,173 @@
+var tap = require('tap');
+var test = tap.test;
+var semver = require('../semver.js');
+var gtr = semver.gtr;
+
+test('\ngtr tests', function(t) {
+ // [range, version, loose]
+ // Version should be greater than range
+ [
+ ['~1.2.2', '1.3.0'],
+ ['~0.6.1-1', '0.7.1-1'],
+ ['1.0.0 - 2.0.0', '2.0.1'],
+ ['1.0.0', '1.0.1-beta1'],
+ ['1.0.0', '2.0.0'],
+ ['<=2.0.0', '2.1.1'],
+ ['<=2.0.0', '3.2.9'],
+ ['<2.0.0', '2.0.0'],
+ ['0.1.20 || 1.2.4', '1.2.5'],
+ ['2.x.x', '3.0.0'],
+ ['1.2.x', '1.3.0'],
+ ['1.2.x || 2.x', '3.0.0'],
+ ['2.*.*', '5.0.1'],
+ ['1.2.*', '1.3.3'],
+ ['1.2.* || 2.*', '4.0.0'],
+ ['2', '3.0.0'],
+ ['2.3', '2.4.2'],
+ ['~2.4', '2.5.0'], // >=2.4.0 <2.5.0
+ ['~2.4', '2.5.5'],
+ ['~>3.2.1', '3.3.0'], // >=3.2.1 <3.3.0
+ ['~1', '2.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '2.2.4'],
+ ['~> 1', '3.2.3'],
+ ['~1.0', '1.1.2'], // >=1.0.0 <1.1.0
+ ['~ 1.0', '1.1.0'],
+ ['<1.2', '1.2.0'],
+ ['< 1.2', '1.2.1'],
+ ['1', '2.0.0beta', true],
+ ['~v0.5.4-pre', '0.6.0'],
+ ['~v0.5.4-pre', '0.6.1-pre'],
+ ['=0.7.x', '0.8.0'],
+ ['=0.7.x', '0.8.0-asdf'],
+ ['<0.7.x', '0.7.0'],
+ ['~1.2.2', '1.3.0'],
+ ['1.0.0 - 2.0.0', '2.2.3'],
+ ['1.0.0', '1.0.1'],
+ ['<=2.0.0', '3.0.0'],
+ ['<=2.0.0', '2.9999.9999'],
+ ['<=2.0.0', '2.2.9'],
+ ['<2.0.0', '2.9999.9999'],
+ ['<2.0.0', '2.2.9'],
+ ['2.x.x', '3.1.3'],
+ ['1.2.x', '1.3.3'],
+ ['1.2.x || 2.x', '3.1.3'],
+ ['2.*.*', '3.1.3'],
+ ['1.2.*', '1.3.3'],
+ ['1.2.* || 2.*', '3.1.3'],
+ ['2', '3.1.2'],
+ ['2.3', '2.4.1'],
+ ['~2.4', '2.5.0'], // >=2.4.0 <2.5.0
+ ['~>3.2.1', '3.3.2'], // >=3.2.1 <3.3.0
+ ['~1', '2.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '2.2.3'],
+ ['~1.0', '1.1.0'], // >=1.0.0 <1.1.0
+ ['<1', '1.0.0'],
+ ['1', '2.0.0beta', true],
+ ['<1', '1.0.0beta', true],
+ ['< 1', '1.0.0beta', true],
+ ['=0.7.x', '0.8.2'],
+ ['<0.7.x', '0.7.2']
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = 'gtr(' + version + ', ' + range + ', ' + loose + ')';
+ t.ok(gtr(version, range, loose), msg);
+ });
+ t.end();
+});
+
+test('\nnegative gtr tests', function(t) {
+ // [range, version, loose]
+ // Version should NOT be greater than range
+ [
+ ['~0.6.1-1', '0.6.1-1'],
+ ['1.0.0 - 2.0.0', '1.2.3'],
+ ['1.0.0 - 2.0.0', '0.9.9'],
+ ['1.0.0', '1.0.0'],
+ ['>=*', '0.2.4'],
+ ['', '1.0.0', true],
+ ['*', '1.2.3'],
+ ['*', 'v1.2.3-foo'],
+ ['>=1.0.0', '1.0.0'],
+ ['>=1.0.0', '1.0.1'],
+ ['>=1.0.0', '1.1.0'],
+ ['>1.0.0', '1.0.1'],
+ ['>1.0.0', '1.1.0'],
+ ['<=2.0.0', '2.0.0'],
+ ['<=2.0.0', '1.9999.9999'],
+ ['<=2.0.0', '0.2.9'],
+ ['<2.0.0', '1.9999.9999'],
+ ['<2.0.0', '0.2.9'],
+ ['>= 1.0.0', '1.0.0'],
+ ['>= 1.0.0', '1.0.1'],
+ ['>= 1.0.0', '1.1.0'],
+ ['> 1.0.0', '1.0.1'],
+ ['> 1.0.0', '1.1.0'],
+ ['<= 2.0.0', '2.0.0'],
+ ['<= 2.0.0', '1.9999.9999'],
+ ['<= 2.0.0', '0.2.9'],
+ ['< 2.0.0', '1.9999.9999'],
+ ['<\t2.0.0', '0.2.9'],
+ ['>=0.1.97', 'v0.1.97'],
+ ['>=0.1.97', '0.1.97'],
+ ['0.1.20 || 1.2.4', '1.2.4'],
+ ['0.1.20 || >1.2.4', '1.2.4'],
+ ['0.1.20 || 1.2.4', '1.2.3'],
+ ['0.1.20 || 1.2.4', '0.1.20'],
+ ['>=0.2.3 || <0.0.1', '0.0.0'],
+ ['>=0.2.3 || <0.0.1', '0.2.3'],
+ ['>=0.2.3 || <0.0.1', '0.2.4'],
+ ['||', '1.3.4'],
+ ['2.x.x', '2.1.3'],
+ ['1.2.x', '1.2.3'],
+ ['1.2.x || 2.x', '2.1.3'],
+ ['1.2.x || 2.x', '1.2.3'],
+ ['x', '1.2.3'],
+ ['2.*.*', '2.1.3'],
+ ['1.2.*', '1.2.3'],
+ ['1.2.* || 2.*', '2.1.3'],
+ ['1.2.* || 2.*', '1.2.3'],
+ ['1.2.* || 2.*', '1.2.3'],
+ ['*', '1.2.3'],
+ ['2', '2.1.2'],
+ ['2.3', '2.3.1'],
+ ['~2.4', '2.4.0'], // >=2.4.0 <2.5.0
+ ['~2.4', '2.4.5'],
+ ['~>3.2.1', '3.2.2'], // >=3.2.1 <3.3.0
+ ['~1', '1.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '1.2.3'],
+ ['~> 1', '1.2.3'],
+ ['~1.0', '1.0.2'], // >=1.0.0 <1.1.0
+ ['~ 1.0', '1.0.2'],
+ ['>=1', '1.0.0'],
+ ['>= 1', '1.0.0'],
+ ['<1.2', '1.1.1'],
+ ['< 1.2', '1.1.1'],
+ ['1', '1.0.0beta', true],
+ ['~v0.5.4-pre', '0.5.5'],
+ ['~v0.5.4-pre', '0.5.4'],
+ ['=0.7.x', '0.7.2'],
+ ['>=0.7.x', '0.7.2'],
+ ['=0.7.x', '0.7.0-asdf'],
+ ['>=0.7.x', '0.7.0-asdf'],
+ ['<=0.7.x', '0.6.2'],
+ ['>0.2.3 >0.2.4 <=0.2.5', '0.2.5'],
+ ['>=0.2.3 <=0.2.4', '0.2.4'],
+ ['1.0.0 - 2.0.0', '2.0.0'],
+ ['^1', '0.0.0-0'],
+ ['^3.0.0', '2.0.0'],
+ ['^1.0.0 || ~2.0.1', '2.0.0'],
+ ['^0.1.0 || ~3.0.1 || 5.0.0', '3.2.0'],
+ ['^0.1.0 || ~3.0.1 || 5.0.0', '1.0.0beta', true],
+ ['^0.1.0 || ~3.0.1 || 5.0.0', '5.0.0-0', true],
+ ['^0.1.0 || ~3.0.1 || >4 <=5.0.0', '3.5.0']
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = '!gtr(' + version + ', ' + range + ', ' + loose + ')';
+ t.notOk(gtr(version, range, loose), msg);
+ });
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/test/index.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/test/index.js b/node_modules/semver/test/index.js
new file mode 100644
index 0000000..47c3f5f
--- /dev/null
+++ b/node_modules/semver/test/index.js
@@ -0,0 +1,698 @@
+'use strict';
+
+var tap = require('tap');
+var test = tap.test;
+var semver = require('../semver.js');
+var eq = semver.eq;
+var gt = semver.gt;
+var lt = semver.lt;
+var neq = semver.neq;
+var cmp = semver.cmp;
+var gte = semver.gte;
+var lte = semver.lte;
+var satisfies = semver.satisfies;
+var validRange = semver.validRange;
+var inc = semver.inc;
+var diff = semver.diff;
+var replaceStars = semver.replaceStars;
+var toComparators = semver.toComparators;
+var SemVer = semver.SemVer;
+var Range = semver.Range;
+
+test('\ncomparison tests', function(t) {
+ // [version1, version2]
+ // version1 should be greater than version2
+ [['0.0.0', '0.0.0-foo'],
+ ['0.0.1', '0.0.0'],
+ ['1.0.0', '0.9.9'],
+ ['0.10.0', '0.9.0'],
+ ['0.99.0', '0.10.0'],
+ ['2.0.0', '1.2.3'],
+ ['v0.0.0', '0.0.0-foo', true],
+ ['v0.0.1', '0.0.0', true],
+ ['v1.0.0', '0.9.9', true],
+ ['v0.10.0', '0.9.0', true],
+ ['v0.99.0', '0.10.0', true],
+ ['v2.0.0', '1.2.3', true],
+ ['0.0.0', 'v0.0.0-foo', true],
+ ['0.0.1', 'v0.0.0', true],
+ ['1.0.0', 'v0.9.9', true],
+ ['0.10.0', 'v0.9.0', true],
+ ['0.99.0', 'v0.10.0', true],
+ ['2.0.0', 'v1.2.3', true],
+ ['1.2.3', '1.2.3-asdf'],
+ ['1.2.3', '1.2.3-4'],
+ ['1.2.3', '1.2.3-4-foo'],
+ ['1.2.3-5-foo', '1.2.3-5'],
+ ['1.2.3-5', '1.2.3-4'],
+ ['1.2.3-5-foo', '1.2.3-5-Foo'],
+ ['3.0.0', '2.7.2+asdf'],
+ ['1.2.3-a.10', '1.2.3-a.5'],
+ ['1.2.3-a.b', '1.2.3-a.5'],
+ ['1.2.3-a.b', '1.2.3-a'],
+ ['1.2.3-a.b.c.10.d.5', '1.2.3-a.b.c.5.d.100'],
+ ['1.2.3-r2', '1.2.3-r100'],
+ ['1.2.3-r100', '1.2.3-R2']
+ ].forEach(function(v) {
+ var v0 = v[0];
+ var v1 = v[1];
+ var loose = v[2];
+ t.ok(gt(v0, v1, loose), "gt('" + v0 + "', '" + v1 + "')");
+ t.ok(lt(v1, v0, loose), "lt('" + v1 + "', '" + v0 + "')");
+ t.ok(!gt(v1, v0, loose), "!gt('" + v1 + "', '" + v0 + "')");
+ t.ok(!lt(v0, v1, loose), "!lt('" + v0 + "', '" + v1 + "')");
+ t.ok(eq(v0, v0, loose), "eq('" + v0 + "', '" + v0 + "')");
+ t.ok(eq(v1, v1, loose), "eq('" + v1 + "', '" + v1 + "')");
+ t.ok(neq(v0, v1, loose), "neq('" + v0 + "', '" + v1 + "')");
+ t.ok(cmp(v1, '==', v1, loose), "cmp('" + v1 + "' == '" + v1 + "')");
+ t.ok(cmp(v0, '>=', v1, loose), "cmp('" + v0 + "' >= '" + v1 + "')");
+ t.ok(cmp(v1, '<=', v0, loose), "cmp('" + v1 + "' <= '" + v0 + "')");
+ t.ok(cmp(v0, '!=', v1, loose), "cmp('" + v0 + "' != '" + v1 + "')");
+ });
+ t.end();
+});
+
+test('\nequality tests', function(t) {
+ // [version1, version2]
+ // version1 should be equivalent to version2
+ [['1.2.3', 'v1.2.3', true],
+ ['1.2.3', '=1.2.3', true],
+ ['1.2.3', 'v 1.2.3', true],
+ ['1.2.3', '= 1.2.3', true],
+ ['1.2.3', ' v1.2.3', true],
+ ['1.2.3', ' =1.2.3', true],
+ ['1.2.3', ' v 1.2.3', true],
+ ['1.2.3', ' = 1.2.3', true],
+ ['1.2.3-0', 'v1.2.3-0', true],
+ ['1.2.3-0', '=1.2.3-0', true],
+ ['1.2.3-0', 'v 1.2.3-0', true],
+ ['1.2.3-0', '= 1.2.3-0', true],
+ ['1.2.3-0', ' v1.2.3-0', true],
+ ['1.2.3-0', ' =1.2.3-0', true],
+ ['1.2.3-0', ' v 1.2.3-0', true],
+ ['1.2.3-0', ' = 1.2.3-0', true],
+ ['1.2.3-1', 'v1.2.3-1', true],
+ ['1.2.3-1', '=1.2.3-1', true],
+ ['1.2.3-1', 'v 1.2.3-1', true],
+ ['1.2.3-1', '= 1.2.3-1', true],
+ ['1.2.3-1', ' v1.2.3-1', true],
+ ['1.2.3-1', ' =1.2.3-1', true],
+ ['1.2.3-1', ' v 1.2.3-1', true],
+ ['1.2.3-1', ' = 1.2.3-1', true],
+ ['1.2.3-beta', 'v1.2.3-beta', true],
+ ['1.2.3-beta', '=1.2.3-beta', true],
+ ['1.2.3-beta', 'v 1.2.3-beta', true],
+ ['1.2.3-beta', '= 1.2.3-beta', true],
+ ['1.2.3-beta', ' v1.2.3-beta', true],
+ ['1.2.3-beta', ' =1.2.3-beta', true],
+ ['1.2.3-beta', ' v 1.2.3-beta', true],
+ ['1.2.3-beta', ' = 1.2.3-beta', true],
+ ['1.2.3-beta+build', ' = 1.2.3-beta+otherbuild', true],
+ ['1.2.3+build', ' = 1.2.3+otherbuild', true],
+ ['1.2.3-beta+build', '1.2.3-beta+otherbuild'],
+ ['1.2.3+build', '1.2.3+otherbuild'],
+ [' v1.2.3+build', '1.2.3+otherbuild']
+ ].forEach(function(v) {
+ var v0 = v[0];
+ var v1 = v[1];
+ var loose = v[2];
+ t.ok(eq(v0, v1, loose), "eq('" + v0 + "', '" + v1 + "')");
+ t.ok(!neq(v0, v1, loose), "!neq('" + v0 + "', '" + v1 + "')");
+ t.ok(cmp(v0, '==', v1, loose), 'cmp(' + v0 + '==' + v1 + ')');
+ t.ok(!cmp(v0, '!=', v1, loose), '!cmp(' + v0 + '!=' + v1 + ')');
+ t.ok(!cmp(v0, '===', v1, loose), '!cmp(' + v0 + '===' + v1 + ')');
+ t.ok(cmp(v0, '!==', v1, loose), 'cmp(' + v0 + '!==' + v1 + ')');
+ t.ok(!gt(v0, v1, loose), "!gt('" + v0 + "', '" + v1 + "')");
+ t.ok(gte(v0, v1, loose), "gte('" + v0 + "', '" + v1 + "')");
+ t.ok(!lt(v0, v1, loose), "!lt('" + v0 + "', '" + v1 + "')");
+ t.ok(lte(v0, v1, loose), "lte('" + v0 + "', '" + v1 + "')");
+ });
+ t.end();
+});
+
+
+test('\nrange tests', function(t) {
+ // [range, version]
+ // version should be included by range
+ [['1.0.0 - 2.0.0', '1.2.3'],
+ ['^1.2.3+build', '1.2.3'],
+ ['^1.2.3+build', '1.3.0'],
+ ['1.2.3-pre+asdf - 2.4.3-pre+asdf', '1.2.3'],
+ ['1.2.3pre+asdf - 2.4.3-pre+asdf', '1.2.3', true],
+ ['1.2.3-pre+asdf - 2.4.3pre+asdf', '1.2.3', true],
+ ['1.2.3pre+asdf - 2.4.3pre+asdf', '1.2.3', true],
+ ['1.2.3-pre+asdf - 2.4.3-pre+asdf', '1.2.3-pre.2'],
+ ['1.2.3-pre+asdf - 2.4.3-pre+asdf', '2.4.3-alpha'],
+ ['1.2.3+asdf - 2.4.3+asdf', '1.2.3'],
+ ['1.0.0', '1.0.0'],
+ ['>=*', '0.2.4'],
+ ['', '1.0.0'],
+ ['*', '1.2.3'],
+ ['*', 'v1.2.3', true],
+ ['>=1.0.0', '1.0.0'],
+ ['>=1.0.0', '1.0.1'],
+ ['>=1.0.0', '1.1.0'],
+ ['>1.0.0', '1.0.1'],
+ ['>1.0.0', '1.1.0'],
+ ['<=2.0.0', '2.0.0'],
+ ['<=2.0.0', '1.9999.9999'],
+ ['<=2.0.0', '0.2.9'],
+ ['<2.0.0', '1.9999.9999'],
+ ['<2.0.0', '0.2.9'],
+ ['>= 1.0.0', '1.0.0'],
+ ['>= 1.0.0', '1.0.1'],
+ ['>= 1.0.0', '1.1.0'],
+ ['> 1.0.0', '1.0.1'],
+ ['> 1.0.0', '1.1.0'],
+ ['<= 2.0.0', '2.0.0'],
+ ['<= 2.0.0', '1.9999.9999'],
+ ['<= 2.0.0', '0.2.9'],
+ ['< 2.0.0', '1.9999.9999'],
+ ['<\t2.0.0', '0.2.9'],
+ ['>=0.1.97', 'v0.1.97', true],
+ ['>=0.1.97', '0.1.97'],
+ ['0.1.20 || 1.2.4', '1.2.4'],
+ ['>=0.2.3 || <0.0.1', '0.0.0'],
+ ['>=0.2.3 || <0.0.1', '0.2.3'],
+ ['>=0.2.3 || <0.0.1', '0.2.4'],
+ ['||', '1.3.4'],
+ ['2.x.x', '2.1.3'],
+ ['1.2.x', '1.2.3'],
+ ['1.2.x || 2.x', '2.1.3'],
+ ['1.2.x || 2.x', '1.2.3'],
+ ['x', '1.2.3'],
+ ['2.*.*', '2.1.3'],
+ ['1.2.*', '1.2.3'],
+ ['1.2.* || 2.*', '2.1.3'],
+ ['1.2.* || 2.*', '1.2.3'],
+ ['*', '1.2.3'],
+ ['2', '2.1.2'],
+ ['2.3', '2.3.1'],
+ ['~2.4', '2.4.0'], // >=2.4.0 <2.5.0
+ ['~2.4', '2.4.5'],
+ ['~>3.2.1', '3.2.2'], // >=3.2.1 <3.3.0,
+ ['~1', '1.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '1.2.3'],
+ ['~> 1', '1.2.3'],
+ ['~1.0', '1.0.2'], // >=1.0.0 <1.1.0,
+ ['~ 1.0', '1.0.2'],
+ ['~ 1.0.3', '1.0.12'],
+ ['>=1', '1.0.0'],
+ ['>= 1', '1.0.0'],
+ ['<1.2', '1.1.1'],
+ ['< 1.2', '1.1.1'],
+ ['~v0.5.4-pre', '0.5.5'],
+ ['~v0.5.4-pre', '0.5.4'],
+ ['=0.7.x', '0.7.2'],
+ ['<=0.7.x', '0.7.2'],
+ ['>=0.7.x', '0.7.2'],
+ ['<=0.7.x', '0.6.2'],
+ ['~1.2.1 >=1.2.3', '1.2.3'],
+ ['~1.2.1 =1.2.3', '1.2.3'],
+ ['~1.2.1 1.2.3', '1.2.3'],
+ ['~1.2.1 >=1.2.3 1.2.3', '1.2.3'],
+ ['~1.2.1 1.2.3 >=1.2.3', '1.2.3'],
+ ['~1.2.1 1.2.3', '1.2.3'],
+ ['>=1.2.1 1.2.3', '1.2.3'],
+ ['1.2.3 >=1.2.1', '1.2.3'],
+ ['>=1.2.3 >=1.2.1', '1.2.3'],
+ ['>=1.2.1 >=1.2.3', '1.2.3'],
+ ['>=1.2', '1.2.8'],
+ ['^1.2.3', '1.8.1'],
+ ['^0.1.2', '0.1.2'],
+ ['^0.1', '0.1.2'],
+ ['^1.2', '1.4.2'],
+ ['^1.2 ^1', '1.4.2'],
+ ['^1.2.3-alpha', '1.2.3-pre'],
+ ['^1.2.0-alpha', '1.2.0-pre'],
+ ['^0.0.1-alpha', '0.0.1-beta']
+ ].forEach(function(v) {
+ var range = v[0];
+ var ver = v[1];
+ var loose = v[2];
+ t.ok(satisfies(ver, range, loose), range + ' satisfied by ' + ver);
+ });
+ t.end();
+});
+
+test('\nnegative range tests', function(t) {
+ // [range, version]
+ // version should not be included by range
+ [['1.0.0 - 2.0.0', '2.2.3'],
+ ['1.2.3+asdf - 2.4.3+asdf', '1.2.3-pre.2'],
+ ['1.2.3+asdf - 2.4.3+asdf', '2.4.3-alpha'],
+ ['^1.2.3+build', '2.0.0'],
+ ['^1.2.3+build', '1.2.0'],
+ ['^1.2.3', '1.2.3-pre'],
+ ['^1.2', '1.2.0-pre'],
+ ['>1.2', '1.3.0-beta'],
+ ['<=1.2.3', '1.2.3-beta'],
+ ['^1.2.3', '1.2.3-beta'],
+ ['=0.7.x', '0.7.0-asdf'],
+ ['>=0.7.x', '0.7.0-asdf'],
+ ['1', '1.0.0beta', true],
+ ['<1', '1.0.0beta', true],
+ ['< 1', '1.0.0beta', true],
+ ['1.0.0', '1.0.1'],
+ ['>=1.0.0', '0.0.0'],
+ ['>=1.0.0', '0.0.1'],
+ ['>=1.0.0', '0.1.0'],
+ ['>1.0.0', '0.0.1'],
+ ['>1.0.0', '0.1.0'],
+ ['<=2.0.0', '3.0.0'],
+ ['<=2.0.0', '2.9999.9999'],
+ ['<=2.0.0', '2.2.9'],
+ ['<2.0.0', '2.9999.9999'],
+ ['<2.0.0', '2.2.9'],
+ ['>=0.1.97', 'v0.1.93', true],
+ ['>=0.1.97', '0.1.93'],
+ ['0.1.20 || 1.2.4', '1.2.3'],
+ ['>=0.2.3 || <0.0.1', '0.0.3'],
+ ['>=0.2.3 || <0.0.1', '0.2.2'],
+ ['2.x.x', '1.1.3'],
+ ['2.x.x', '3.1.3'],
+ ['1.2.x', '1.3.3'],
+ ['1.2.x || 2.x', '3.1.3'],
+ ['1.2.x || 2.x', '1.1.3'],
+ ['2.*.*', '1.1.3'],
+ ['2.*.*', '3.1.3'],
+ ['1.2.*', '1.3.3'],
+ ['1.2.* || 2.*', '3.1.3'],
+ ['1.2.* || 2.*', '1.1.3'],
+ ['2', '1.1.2'],
+ ['2.3', '2.4.1'],
+ ['~2.4', '2.5.0'], // >=2.4.0 <2.5.0
+ ['~2.4', '2.3.9'],
+ ['~>3.2.1', '3.3.2'], // >=3.2.1 <3.3.0
+ ['~>3.2.1', '3.2.0'], // >=3.2.1 <3.3.0
+ ['~1', '0.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '2.2.3'],
+ ['~1.0', '1.1.0'], // >=1.0.0 <1.1.0
+ ['<1', '1.0.0'],
+ ['>=1.2', '1.1.1'],
+ ['1', '2.0.0beta', true],
+ ['~v0.5.4-beta', '0.5.4-alpha'],
+ ['=0.7.x', '0.8.2'],
+ ['>=0.7.x', '0.6.2'],
+ ['<0.7.x', '0.7.2'],
+ ['<1.2.3', '1.2.3-beta'],
+ ['=1.2.3', '1.2.3-beta'],
+ ['>1.2', '1.2.8'],
+ ['^1.2.3', '2.0.0-alpha'],
+ ['^1.2.3', '1.2.2'],
+ ['^1.2', '1.1.9'],
+ ['*', 'v1.2.3-foo', true],
+ // invalid ranges never satisfied!
+ ['blerg', '1.2.3'],
+ ['git+https://user:password0123@github.com/foo', '123.0.0', true],
+ ['^1.2.3', '2.0.0-pre']
+ ].forEach(function(v) {
+ var range = v[0];
+ var ver = v[1];
+ var loose = v[2];
+ var found = satisfies(ver, range, loose);
+ t.ok(!found, ver + ' not satisfied by ' + range);
+ });
+ t.end();
+});
+
+test('\nincrement versions test', function(t) {
+// [version, inc, result, identifier]
+// inc(version, inc) -> result
+ [['1.2.3', 'major', '2.0.0'],
+ ['1.2.3', 'minor', '1.3.0'],
+ ['1.2.3', 'patch', '1.2.4'],
+ ['1.2.3tag', 'major', '2.0.0', true],
+ ['1.2.3-tag', 'major', '2.0.0'],
+ ['1.2.3', 'fake', null],
+ ['1.2.0-0', 'patch', '1.2.0'],
+ ['fake', 'major', null],
+ ['1.2.3-4', 'major', '2.0.0'],
+ ['1.2.3-4', 'minor', '1.3.0'],
+ ['1.2.3-4', 'patch', '1.2.3'],
+ ['1.2.3-alpha.0.beta', 'major', '2.0.0'],
+ ['1.2.3-alpha.0.beta', 'minor', '1.3.0'],
+ ['1.2.3-alpha.0.beta', 'patch', '1.2.3'],
+ ['1.2.4', 'prerelease', '1.2.5-0'],
+ ['1.2.3-0', 'prerelease', '1.2.3-1'],
+ ['1.2.3-alpha.0', 'prerelease', '1.2.3-alpha.1'],
+ ['1.2.3-alpha.1', 'prerelease', '1.2.3-alpha.2'],
+ ['1.2.3-alpha.2', 'prerelease', '1.2.3-alpha.3'],
+ ['1.2.3-alpha.0.beta', 'prerelease', '1.2.3-alpha.1.beta'],
+ ['1.2.3-alpha.1.beta', 'prerelease', '1.2.3-alpha.2.beta'],
+ ['1.2.3-alpha.2.beta', 'prerelease', '1.2.3-alpha.3.beta'],
+ ['1.2.3-alpha.10.0.beta', 'prerelease', '1.2.3-alpha.10.1.beta'],
+ ['1.2.3-alpha.10.1.beta', 'prerelease', '1.2.3-alpha.10.2.beta'],
+ ['1.2.3-alpha.10.2.beta', 'prerelease', '1.2.3-alpha.10.3.beta'],
+ ['1.2.3-alpha.10.beta.0', 'prerelease', '1.2.3-alpha.10.beta.1'],
+ ['1.2.3-alpha.10.beta.1', 'prerelease', '1.2.3-alpha.10.beta.2'],
+ ['1.2.3-alpha.10.beta.2', 'prerelease', '1.2.3-alpha.10.beta.3'],
+ ['1.2.3-alpha.9.beta', 'prerelease', '1.2.3-alpha.10.beta'],
+ ['1.2.3-alpha.10.beta', 'prerelease', '1.2.3-alpha.11.beta'],
+ ['1.2.3-alpha.11.beta', 'prerelease', '1.2.3-alpha.12.beta'],
+ ['1.2.0', 'prepatch', '1.2.1-0'],
+ ['1.2.0-1', 'prepatch', '1.2.1-0'],
+ ['1.2.0', 'preminor', '1.3.0-0'],
+ ['1.2.3-1', 'preminor', '1.3.0-0'],
+ ['1.2.0', 'premajor', '2.0.0-0'],
+ ['1.2.3-1', 'premajor', '2.0.0-0'],
+ ['1.2.0-1', 'minor', '1.2.0'],
+ ['1.0.0-1', 'major', '1.0.0'],
+
+ ['1.2.3', 'major', '2.0.0', false, 'dev'],
+ ['1.2.3', 'minor', '1.3.0', false, 'dev'],
+ ['1.2.3', 'patch', '1.2.4', false, 'dev'],
+ ['1.2.3tag', 'major', '2.0.0', true, 'dev'],
+ ['1.2.3-tag', 'major', '2.0.0', false, 'dev'],
+ ['1.2.3', 'fake', null, false, 'dev'],
+ ['1.2.0-0', 'patch', '1.2.0', false, 'dev'],
+ ['fake', 'major', null, false, 'dev'],
+ ['1.2.3-4', 'major', '2.0.0', false, 'dev'],
+ ['1.2.3-4', 'minor', '1.3.0', false, 'dev'],
+ ['1.2.3-4', 'patch', '1.2.3', false, 'dev'],
+ ['1.2.3-alpha.0.beta', 'major', '2.0.0', false, 'dev'],
+ ['1.2.3-alpha.0.beta', 'minor', '1.3.0', false, 'dev'],
+ ['1.2.3-alpha.0.beta', 'patch', '1.2.3', false, 'dev'],
+ ['1.2.4', 'prerelease', '1.2.5-dev.0', false, 'dev'],
+ ['1.2.3-0', 'prerelease', '1.2.3-dev.0', false, 'dev'],
+ ['1.2.3-alpha.0', 'prerelease', '1.2.3-dev.0', false, 'dev'],
+ ['1.2.3-alpha.0', 'prerelease', '1.2.3-alpha.1', false, 'alpha'],
+ ['1.2.3-alpha.0.beta', 'prerelease', '1.2.3-dev.0', false, 'dev'],
+ ['1.2.3-alpha.0.beta', 'prerelease', '1.2.3-alpha.1.beta', false, 'alpha'],
+ ['1.2.3-alpha.10.0.beta', 'prerelease', '1.2.3-dev.0', false, 'dev'],
+ ['1.2.3-alpha.10.0.beta', 'prerelease', '1.2.3-alpha.10.1.beta', false, 'alpha'],
+ ['1.2.3-alpha.10.1.beta', 'prerelease', '1.2.3-alpha.10.2.beta', false, 'alpha'],
+ ['1.2.3-alpha.10.2.beta', 'prerelease', '1.2.3-alpha.10.3.beta', false, 'alpha'],
+ ['1.2.3-alpha.10.beta.0', 'prerelease', '1.2.3-dev.0', false, 'dev'],
+ ['1.2.3-alpha.10.beta.0', 'prerelease', '1.2.3-alpha.10.beta.1', false, 'alpha'],
+ ['1.2.3-alpha.10.beta.1', 'prerelease', '1.2.3-alpha.10.beta.2', false, 'alpha'],
+ ['1.2.3-alpha.10.beta.2', 'prerelease', '1.2.3-alpha.10.beta.3', false, 'alpha'],
+ ['1.2.3-alpha.9.beta', 'prerelease', '1.2.3-dev.0', false, 'dev'],
+ ['1.2.3-alpha.9.beta', 'prerelease', '1.2.3-alpha.10.beta', false, 'alpha'],
+ ['1.2.3-alpha.10.beta', 'prerelease', '1.2.3-alpha.11.beta', false, 'alpha'],
+ ['1.2.3-alpha.11.beta', 'prerelease', '1.2.3-alpha.12.beta', false, 'alpha'],
+ ['1.2.0', 'prepatch', '1.2.1-dev.0', false, 'dev'],
+ ['1.2.0-1', 'prepatch', '1.2.1-dev.0', false, 'dev'],
+ ['1.2.0', 'preminor', '1.3.0-dev.0', false, 'dev'],
+ ['1.2.3-1', 'preminor', '1.3.0-dev.0', false, 'dev'],
+ ['1.2.0', 'premajor', '2.0.0-dev.0', false, 'dev'],
+ ['1.2.3-1', 'premajor', '2.0.0-dev.0', false, 'dev'],
+ ['1.2.0-1', 'minor', '1.2.0', false, 'dev'],
+ ['1.0.0-1', 'major', '1.0.0', false, 'dev'],
+ ['1.2.3-dev.bar', 'prerelease', '1.2.3-dev.0', false, 'dev']
+
+ ].forEach(function(v) {
+ var pre = v[0];
+ var what = v[1];
+ var wanted = v[2];
+ var loose = v[3];
+ var id = v[4];
+ var found = inc(pre, what, loose, id);
+ var cmd = 'inc(' + pre + ', ' + what + ', ' + id + ')';
+ t.equal(found, wanted, cmd + ' === ' + wanted);
+
+ var parsed = semver.parse(pre, loose);
+ if (wanted) {
+ parsed.inc(what, id);
+ t.equal(parsed.version, wanted, cmd + ' object version updated');
+ t.equal(parsed.raw, wanted, cmd + ' object raw field updated');
+ } else if (parsed) {
+ t.throws(function () {
+ parsed.inc(what, id)
+ })
+ } else {
+ t.equal(parsed, null)
+ }
+ });
+
+ t.end();
+});
+
+test('\ndiff versions test', function(t) {
+// [version1, version2, result]
+// diff(version1, version2) -> result
+ [['1.2.3', '0.2.3', 'major'],
+ ['1.4.5', '0.2.3', 'major'],
+ ['1.2.3', '2.0.0-pre', 'premajor'],
+ ['1.2.3', '1.3.3', 'minor'],
+ ['1.0.1', '1.1.0-pre', 'preminor'],
+ ['1.2.3', '1.2.4', 'patch'],
+ ['1.2.3', '1.2.4-pre', 'prepatch'],
+ ['0.0.1', '0.0.1-pre', 'prerelease'],
+ ['0.0.1', '0.0.1-pre-2', 'prerelease'],
+ ['1.1.0', '1.1.0-pre', 'prerelease'],
+ ['1.1.0-pre-1', '1.1.0-pre-2', 'prerelease'],
+ ['1.0.0', '1.0.0', null]
+
+ ].forEach(function(v) {
+ var version1 = v[0];
+ var version2 = v[1];
+ var wanted = v[2];
+ var found = diff(version1, version2);
+ var cmd = 'diff(' + version1 + ', ' + version2 + ')';
+ t.equal(found, wanted, cmd + ' === ' + wanted);
+ });
+
+ t.end();
+});
+
+test('\nvalid range test', function(t) {
+ // [range, result]
+ // validRange(range) -> result
+ // translate ranges into their canonical form
+ [['1.0.0 - 2.0.0', '>=1.0.0 <=2.0.0'],
+ ['1.0.0', '1.0.0'],
+ ['>=*', '*'],
+ ['', '*'],
+ ['*', '*'],
+ ['*', '*'],
+ ['>=1.0.0', '>=1.0.0'],
+ ['>1.0.0', '>1.0.0'],
+ ['<=2.0.0', '<=2.0.0'],
+ ['1', '>=1.0.0 <2.0.0'],
+ ['<=2.0.0', '<=2.0.0'],
+ ['<=2.0.0', '<=2.0.0'],
+ ['<2.0.0', '<2.0.0'],
+ ['<2.0.0', '<2.0.0'],
+ ['>= 1.0.0', '>=1.0.0'],
+ ['>= 1.0.0', '>=1.0.0'],
+ ['>= 1.0.0', '>=1.0.0'],
+ ['> 1.0.0', '>1.0.0'],
+ ['> 1.0.0', '>1.0.0'],
+ ['<= 2.0.0', '<=2.0.0'],
+ ['<= 2.0.0', '<=2.0.0'],
+ ['<= 2.0.0', '<=2.0.0'],
+ ['< 2.0.0', '<2.0.0'],
+ ['< 2.0.0', '<2.0.0'],
+ ['>=0.1.97', '>=0.1.97'],
+ ['>=0.1.97', '>=0.1.97'],
+ ['0.1.20 || 1.2.4', '0.1.20||1.2.4'],
+ ['>=0.2.3 || <0.0.1', '>=0.2.3||<0.0.1'],
+ ['>=0.2.3 || <0.0.1', '>=0.2.3||<0.0.1'],
+ ['>=0.2.3 || <0.0.1', '>=0.2.3||<0.0.1'],
+ ['||', '||'],
+ ['2.x.x', '>=2.0.0 <3.0.0'],
+ ['1.2.x', '>=1.2.0 <1.3.0'],
+ ['1.2.x || 2.x', '>=1.2.0 <1.3.0||>=2.0.0 <3.0.0'],
+ ['1.2.x || 2.x', '>=1.2.0 <1.3.0||>=2.0.0 <3.0.0'],
+ ['x', '*'],
+ ['2.*.*', '>=2.0.0 <3.0.0'],
+ ['1.2.*', '>=1.2.0 <1.3.0'],
+ ['1.2.* || 2.*', '>=1.2.0 <1.3.0||>=2.0.0 <3.0.0'],
+ ['*', '*'],
+ ['2', '>=2.0.0 <3.0.0'],
+ ['2.3', '>=2.3.0 <2.4.0'],
+ ['~2.4', '>=2.4.0 <2.5.0'],
+ ['~2.4', '>=2.4.0 <2.5.0'],
+ ['~>3.2.1', '>=3.2.1 <3.3.0'],
+ ['~1', '>=1.0.0 <2.0.0'],
+ ['~>1', '>=1.0.0 <2.0.0'],
+ ['~> 1', '>=1.0.0 <2.0.0'],
+ ['~1.0', '>=1.0.0 <1.1.0'],
+ ['~ 1.0', '>=1.0.0 <1.1.0'],
+ ['^0', '>=0.0.0 <1.0.0'],
+ ['^ 1', '>=1.0.0 <2.0.0'],
+ ['^0.1', '>=0.1.0 <0.2.0'],
+ ['^1.0', '>=1.0.0 <2.0.0'],
+ ['^1.2', '>=1.2.0 <2.0.0'],
+ ['^0.0.1', '>=0.0.1 <0.0.2'],
+ ['^0.0.1-beta', '>=0.0.1-beta <0.0.2'],
+ ['^0.1.2', '>=0.1.2 <0.2.0'],
+ ['^1.2.3', '>=1.2.3 <2.0.0'],
+ ['^1.2.3-beta.4', '>=1.2.3-beta.4 <2.0.0'],
+ ['<1', '<1.0.0'],
+ ['< 1', '<1.0.0'],
+ ['>=1', '>=1.0.0'],
+ ['>= 1', '>=1.0.0'],
+ ['<1.2', '<1.2.0'],
+ ['< 1.2', '<1.2.0'],
+ ['1', '>=1.0.0 <2.0.0'],
+ ['>01.02.03', '>1.2.3', true],
+ ['>01.02.03', null],
+ ['~1.2.3beta', '>=1.2.3-beta <1.3.0', true],
+ ['~1.2.3beta', null],
+ ['^ 1.2 ^ 1', '>=1.2.0 <2.0.0 >=1.0.0 <2.0.0']
+ ].forEach(function(v) {
+ var pre = v[0];
+ var wanted = v[1];
+ var loose = v[2];
+ var found = validRange(pre, loose);
+
+ t.equal(found, wanted, 'validRange(' + pre + ') === ' + wanted);
+ });
+
+ t.end();
+});
+
+test('\ncomparators test', function(t) {
+ // [range, comparators]
+ // turn range into a set of individual comparators
+ [['1.0.0 - 2.0.0', [['>=1.0.0', '<=2.0.0']]],
+ ['1.0.0', [['1.0.0']]],
+ ['>=*', [['']]],
+ ['', [['']]],
+ ['*', [['']]],
+ ['*', [['']]],
+ ['>=1.0.0', [['>=1.0.0']]],
+ ['>=1.0.0', [['>=1.0.0']]],
+ ['>=1.0.0', [['>=1.0.0']]],
+ ['>1.0.0', [['>1.0.0']]],
+ ['>1.0.0', [['>1.0.0']]],
+ ['<=2.0.0', [['<=2.0.0']]],
+ ['1', [['>=1.0.0', '<2.0.0']]],
+ ['<=2.0.0', [['<=2.0.0']]],
+ ['<=2.0.0', [['<=2.0.0']]],
+ ['<2.0.0', [['<2.0.0']]],
+ ['<2.0.0', [['<2.0.0']]],
+ ['>= 1.0.0', [['>=1.0.0']]],
+ ['>= 1.0.0', [['>=1.0.0']]],
+ ['>= 1.0.0', [['>=1.0.0']]],
+ ['> 1.0.0', [['>1.0.0']]],
+ ['> 1.0.0', [['>1.0.0']]],
+ ['<= 2.0.0', [['<=2.0.0']]],
+ ['<= 2.0.0', [['<=2.0.0']]],
+ ['<= 2.0.0', [['<=2.0.0']]],
+ ['< 2.0.0', [['<2.0.0']]],
+ ['<\t2.0.0', [['<2.0.0']]],
+ ['>=0.1.97', [['>=0.1.97']]],
+ ['>=0.1.97', [['>=0.1.97']]],
+ ['0.1.20 || 1.2.4', [['0.1.20'], ['1.2.4']]],
+ ['>=0.2.3 || <0.0.1', [['>=0.2.3'], ['<0.0.1']]],
+ ['>=0.2.3 || <0.0.1', [['>=0.2.3'], ['<0.0.1']]],
+ ['>=0.2.3 || <0.0.1', [['>=0.2.3'], ['<0.0.1']]],
+ ['||', [[''], ['']]],
+ ['2.x.x', [['>=2.0.0', '<3.0.0']]],
+ ['1.2.x', [['>=1.2.0', '<1.3.0']]],
+ ['1.2.x || 2.x', [['>=1.2.0', '<1.3.0'], ['>=2.0.0', '<3.0.0']]],
+ ['1.2.x || 2.x', [['>=1.2.0', '<1.3.0'], ['>=2.0.0', '<3.0.0']]],
+ ['x', [['']]],
+ ['2.*.*', [['>=2.0.0', '<3.0.0']]],
+ ['1.2.*', [['>=1.2.0', '<1.3.0']]],
+ ['1.2.* || 2.*', [['>=1.2.0', '<1.3.0'], ['>=2.0.0', '<3.0.0']]],
+ ['1.2.* || 2.*', [['>=1.2.0', '<1.3.0'], ['>=2.0.0', '<3.0.0']]],
+ ['*', [['']]],
+ ['2', [['>=2.0.0', '<3.0.0']]],
+ ['2.3', [['>=2.3.0', '<2.4.0']]],
+ ['~2.4', [['>=2.4.0', '<2.5.0']]],
+ ['~2.4', [['>=2.4.0', '<2.5.0']]],
+ ['~>3.2.1', [['>=3.2.1', '<3.3.0']]],
+ ['~1', [['>=1.0.0', '<2.0.0']]],
+ ['~>1', [['>=1.0.0', '<2.0.0']]],
+ ['~> 1', [['>=1.0.0', '<2.0.0']]],
+ ['~1.0', [['>=1.0.0', '<1.1.0']]],
+ ['~ 1.0', [['>=1.0.0', '<1.1.0']]],
+ ['~ 1.0.3', [['>=1.0.3', '<1.1.0']]],
+ ['~> 1.0.3', [['>=1.0.3', '<1.1.0']]],
+ ['<1', [['<1.0.0']]],
+ ['< 1', [['<1.0.0']]],
+ ['>=1', [['>=1.0.0']]],
+ ['>= 1', [['>=1.0.0']]],
+ ['<1.2', [['<1.2.0']]],
+ ['< 1.2', [['<1.2.0']]],
+ ['1', [['>=1.0.0', '<2.0.0']]],
+ ['1 2', [['>=1.0.0', '<2.0.0', '>=2.0.0', '<3.0.0']]],
+ ['1.2 - 3.4.5', [['>=1.2.0', '<=3.4.5']]],
+ ['1.2.3 - 3.4', [['>=1.2.3', '<3.5.0']]],
+ ['1.2.3 - 3', [['>=1.2.3', '<4.0.0']]],
+ ['>*', [['<0.0.0']]],
+ ['<*', [['<0.0.0']]]
+ ].forEach(function(v) {
+ var pre = v[0];
+ var wanted = v[1];
+ var found = toComparators(v[0]);
+ var jw = JSON.stringify(wanted);
+ t.equivalent(found, wanted, 'toComparators(' + pre + ') === ' + jw);
+ });
+
+ t.end();
+});
+
+test('\ninvalid version numbers', function(t) {
+ ['1.2.3.4',
+ 'NOT VALID',
+ 1.2,
+ null,
+ 'Infinity.NaN.Infinity'
+ ].forEach(function(v) {
+ t.throws(function() {
+ new SemVer(v);
+ }, {name:'TypeError', message:'Invalid Version: ' + v});
+ });
+
+ t.end();
+});
+
+test('\nstrict vs loose version numbers', function(t) {
+ [['=1.2.3', '1.2.3'],
+ ['01.02.03', '1.2.3'],
+ ['1.2.3-beta.01', '1.2.3-beta.1'],
+ [' =1.2.3', '1.2.3'],
+ ['1.2.3foo', '1.2.3-foo']
+ ].forEach(function(v) {
+ var loose = v[0];
+ var strict = v[1];
+ t.throws(function() {
+ new SemVer(loose);
+ });
+ var lv = new SemVer(loose, true);
+ t.equal(lv.version, strict);
+ t.ok(eq(loose, strict, true));
+ t.throws(function() {
+ eq(loose, strict);
+ });
+ t.throws(function() {
+ new SemVer(strict).compare(loose);
+ });
+ });
+ t.end();
+});
+
+test('\nstrict vs loose ranges', function(t) {
+ [['>=01.02.03', '>=1.2.3'],
+ ['~1.02.03beta', '>=1.2.3-beta <1.3.0']
+ ].forEach(function(v) {
+ var loose = v[0];
+ var comps = v[1];
+ t.throws(function() {
+ new Range(loose);
+ });
+ t.equal(new Range(loose, true).range, comps);
+ });
+ t.end();
+});
+
+test('\nmax satisfying', function(t) {
+ [[['1.2.3', '1.2.4'], '1.2', '1.2.4'],
+ [['1.2.4', '1.2.3'], '1.2', '1.2.4'],
+ [['1.2.3', '1.2.4', '1.2.5', '1.2.6'], '~1.2.3', '1.2.6'],
+ [['1.1.0', '1.2.0', '1.2.1', '1.3.0', '2.0.0b1', '2.0.0b2', '2.0.0b3', '2.0.0', '2.1.0'], '~2.0.0', '2.0.0', true]
+ ].forEach(function(v) {
+ var versions = v[0];
+ var range = v[1];
+ var expect = v[2];
+ var loose = v[3];
+ var actual = semver.maxSatisfying(versions, range, loose);
+ t.equal(actual, expect);
+ });
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/test/ltr.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/test/ltr.js b/node_modules/semver/test/ltr.js
new file mode 100644
index 0000000..0f7167d
--- /dev/null
+++ b/node_modules/semver/test/ltr.js
@@ -0,0 +1,181 @@
+var tap = require('tap');
+var test = tap.test;
+var semver = require('../semver.js');
+var ltr = semver.ltr;
+
+test('\nltr tests', function(t) {
+ // [range, version, loose]
+ // Version should be less than range
+ [
+ ['~1.2.2', '1.2.1'],
+ ['~0.6.1-1', '0.6.1-0'],
+ ['1.0.0 - 2.0.0', '0.0.1'],
+ ['1.0.0-beta.2', '1.0.0-beta.1'],
+ ['1.0.0', '0.0.0'],
+ ['>=2.0.0', '1.1.1'],
+ ['>=2.0.0', '1.2.9'],
+ ['>2.0.0', '2.0.0'],
+ ['0.1.20 || 1.2.4', '0.1.5'],
+ ['2.x.x', '1.0.0'],
+ ['1.2.x', '1.1.0'],
+ ['1.2.x || 2.x', '1.0.0'],
+ ['2.*.*', '1.0.1'],
+ ['1.2.*', '1.1.3'],
+ ['1.2.* || 2.*', '1.1.9999'],
+ ['2', '1.0.0'],
+ ['2.3', '2.2.2'],
+ ['~2.4', '2.3.0'], // >=2.4.0 <2.5.0
+ ['~2.4', '2.3.5'],
+ ['~>3.2.1', '3.2.0'], // >=3.2.1 <3.3.0
+ ['~1', '0.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '0.2.4'],
+ ['~> 1', '0.2.3'],
+ ['~1.0', '0.1.2'], // >=1.0.0 <1.1.0
+ ['~ 1.0', '0.1.0'],
+ ['>1.2', '1.2.0'],
+ ['> 1.2', '1.2.1'],
+ ['1', '0.0.0beta', true],
+ ['~v0.5.4-pre', '0.5.4-alpha'],
+ ['~v0.5.4-pre', '0.5.4-alpha'],
+ ['=0.7.x', '0.6.0'],
+ ['=0.7.x', '0.6.0-asdf'],
+ ['>=0.7.x', '0.6.0'],
+ ['~1.2.2', '1.2.1'],
+ ['1.0.0 - 2.0.0', '0.2.3'],
+ ['1.0.0', '0.0.1'],
+ ['>=2.0.0', '1.0.0'],
+ ['>=2.0.0', '1.9999.9999'],
+ ['>=2.0.0', '1.2.9'],
+ ['>2.0.0', '2.0.0'],
+ ['>2.0.0', '1.2.9'],
+ ['2.x.x', '1.1.3'],
+ ['1.2.x', '1.1.3'],
+ ['1.2.x || 2.x', '1.1.3'],
+ ['2.*.*', '1.1.3'],
+ ['1.2.*', '1.1.3'],
+ ['1.2.* || 2.*', '1.1.3'],
+ ['2', '1.9999.9999'],
+ ['2.3', '2.2.1'],
+ ['~2.4', '2.3.0'], // >=2.4.0 <2.5.0
+ ['~>3.2.1', '2.3.2'], // >=3.2.1 <3.3.0
+ ['~1', '0.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '0.2.3'],
+ ['~1.0', '0.0.0'], // >=1.0.0 <1.1.0
+ ['>1', '1.0.0'],
+ ['2', '1.0.0beta', true],
+ ['>1', '1.0.0beta', true],
+ ['> 1', '1.0.0beta', true],
+ ['=0.7.x', '0.6.2'],
+ ['=0.7.x', '0.7.0-asdf'],
+ ['^1', '1.0.0-0'],
+ ['>=0.7.x', '0.7.0-asdf'],
+ ['1', '1.0.0beta', true],
+ ['>=0.7.x', '0.6.2'],
+ ['>1.2.3', '1.3.0-alpha']
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = 'ltr(' + version + ', ' + range + ', ' + loose + ')';
+ t.ok(ltr(version, range, loose), msg);
+ });
+ t.end();
+});
+
+test('\nnegative ltr tests', function(t) {
+ // [range, version, loose]
+ // Version should NOT be less than range
+ [
+ ['~ 1.0', '1.1.0'],
+ ['~0.6.1-1', '0.6.1-1'],
+ ['1.0.0 - 2.0.0', '1.2.3'],
+ ['1.0.0 - 2.0.0', '2.9.9'],
+ ['1.0.0', '1.0.0'],
+ ['>=*', '0.2.4'],
+ ['', '1.0.0', true],
+ ['*', '1.2.3'],
+ ['>=1.0.0', '1.0.0'],
+ ['>=1.0.0', '1.0.1'],
+ ['>=1.0.0', '1.1.0'],
+ ['>1.0.0', '1.0.1'],
+ ['>1.0.0', '1.1.0'],
+ ['<=2.0.0', '2.0.0'],
+ ['<=2.0.0', '1.9999.9999'],
+ ['<=2.0.0', '0.2.9'],
+ ['<2.0.0', '1.9999.9999'],
+ ['<2.0.0', '0.2.9'],
+ ['>= 1.0.0', '1.0.0'],
+ ['>= 1.0.0', '1.0.1'],
+ ['>= 1.0.0', '1.1.0'],
+ ['> 1.0.0', '1.0.1'],
+ ['> 1.0.0', '1.1.0'],
+ ['<= 2.0.0', '2.0.0'],
+ ['<= 2.0.0', '1.9999.9999'],
+ ['<= 2.0.0', '0.2.9'],
+ ['< 2.0.0', '1.9999.9999'],
+ ['<\t2.0.0', '0.2.9'],
+ ['>=0.1.97', 'v0.1.97'],
+ ['>=0.1.97', '0.1.97'],
+ ['0.1.20 || 1.2.4', '1.2.4'],
+ ['0.1.20 || >1.2.4', '1.2.4'],
+ ['0.1.20 || 1.2.4', '1.2.3'],
+ ['0.1.20 || 1.2.4', '0.1.20'],
+ ['>=0.2.3 || <0.0.1', '0.0.0'],
+ ['>=0.2.3 || <0.0.1', '0.2.3'],
+ ['>=0.2.3 || <0.0.1', '0.2.4'],
+ ['||', '1.3.4'],
+ ['2.x.x', '2.1.3'],
+ ['1.2.x', '1.2.3'],
+ ['1.2.x || 2.x', '2.1.3'],
+ ['1.2.x || 2.x', '1.2.3'],
+ ['x', '1.2.3'],
+ ['2.*.*', '2.1.3'],
+ ['1.2.*', '1.2.3'],
+ ['1.2.* || 2.*', '2.1.3'],
+ ['1.2.* || 2.*', '1.2.3'],
+ ['1.2.* || 2.*', '1.2.3'],
+ ['*', '1.2.3'],
+ ['2', '2.1.2'],
+ ['2.3', '2.3.1'],
+ ['~2.4', '2.4.0'], // >=2.4.0 <2.5.0
+ ['~2.4', '2.4.5'],
+ ['~>3.2.1', '3.2.2'], // >=3.2.1 <3.3.0
+ ['~1', '1.2.3'], // >=1.0.0 <2.0.0
+ ['~>1', '1.2.3'],
+ ['~> 1', '1.2.3'],
+ ['~1.0', '1.0.2'], // >=1.0.0 <1.1.0
+ ['~ 1.0', '1.0.2'],
+ ['>=1', '1.0.0'],
+ ['>= 1', '1.0.0'],
+ ['<1.2', '1.1.1'],
+ ['< 1.2', '1.1.1'],
+ ['~v0.5.4-pre', '0.5.5'],
+ ['~v0.5.4-pre', '0.5.4'],
+ ['=0.7.x', '0.7.2'],
+ ['>=0.7.x', '0.7.2'],
+ ['<=0.7.x', '0.6.2'],
+ ['>0.2.3 >0.2.4 <=0.2.5', '0.2.5'],
+ ['>=0.2.3 <=0.2.4', '0.2.4'],
+ ['1.0.0 - 2.0.0', '2.0.0'],
+ ['^3.0.0', '4.0.0'],
+ ['^1.0.0 || ~2.0.1', '2.0.0'],
+ ['^0.1.0 || ~3.0.1 || 5.0.0', '3.2.0'],
+ ['^0.1.0 || ~3.0.1 || 5.0.0', '1.0.0beta', true],
+ ['^0.1.0 || ~3.0.1 || 5.0.0', '5.0.0-0', true],
+ ['^0.1.0 || ~3.0.1 || >4 <=5.0.0', '3.5.0'],
+ ['^1.0.0alpha', '1.0.0beta', true],
+ ['~1.0.0alpha', '1.0.0beta', true],
+ ['^1.0.0-alpha', '1.0.0beta', true],
+ ['~1.0.0-alpha', '1.0.0beta', true],
+ ['^1.0.0-alpha', '1.0.0-beta'],
+ ['~1.0.0-alpha', '1.0.0-beta'],
+ ['=0.1.0', '1.0.0']
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = '!ltr(' + version + ', ' + range + ', ' + loose + ')';
+ t.notOk(ltr(version, range, loose), msg);
+ });
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/test/major-minor-patch.js
----------------------------------------------------------------------
diff --git a/node_modules/semver/test/major-minor-patch.js b/node_modules/semver/test/major-minor-patch.js
new file mode 100644
index 0000000..e9d4039
--- /dev/null
+++ b/node_modules/semver/test/major-minor-patch.js
@@ -0,0 +1,72 @@
+var tap = require('tap');
+var test = tap.test;
+var semver = require('../semver.js');
+
+test('\nmajor tests', function(t) {
+ // [range, version]
+ // Version should be detectable despite extra characters
+ [
+ ['1.2.3', 1],
+ [' 1.2.3 ', 1],
+ [' 2.2.3-4 ', 2],
+ [' 3.2.3-pre ', 3],
+ ['v5.2.3', 5],
+ [' v8.2.3 ', 8],
+ ['\t13.2.3', 13],
+ ['=21.2.3', 21, true],
+ ['v=34.2.3', 34, true]
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = 'major(' + range + ') = ' + version;
+ t.equal(semver.major(range, loose), version, msg);
+ });
+ t.end();
+});
+
+test('\nminor tests', function(t) {
+ // [range, version]
+ // Version should be detectable despite extra characters
+ [
+ ['1.1.3', 1],
+ [' 1.1.3 ', 1],
+ [' 1.2.3-4 ', 2],
+ [' 1.3.3-pre ', 3],
+ ['v1.5.3', 5],
+ [' v1.8.3 ', 8],
+ ['\t1.13.3', 13],
+ ['=1.21.3', 21, true],
+ ['v=1.34.3', 34, true]
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = 'minor(' + range + ') = ' + version;
+ t.equal(semver.minor(range, loose), version, msg);
+ });
+ t.end();
+});
+
+test('\npatch tests', function(t) {
+ // [range, version]
+ // Version should be detectable despite extra characters
+ [
+ ['1.2.1', 1],
+ [' 1.2.1 ', 1],
+ [' 1.2.2-4 ', 2],
+ [' 1.2.3-pre ', 3],
+ ['v1.2.5', 5],
+ [' v1.2.8 ', 8],
+ ['\t1.2.13', 13],
+ ['=1.2.21', 21, true],
+ ['v=1.2.34', 34, true]
+ ].forEach(function(tuple) {
+ var range = tuple[0];
+ var version = tuple[1];
+ var loose = tuple[2] || false;
+ var msg = 'patch(' + range + ') = ' + version;
+ t.equal(semver.patch(range, loose), version, msg);
+ });
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/.name
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/.name b/node_modules/shelljs/.idea/.name
new file mode 100644
index 0000000..68840c9
--- /dev/null
+++ b/node_modules/shelljs/.idea/.name
@@ -0,0 +1 @@
+shelljs
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/encodings.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/encodings.xml b/node_modules/shelljs/.idea/encodings.xml
new file mode 100644
index 0000000..97626ba
--- /dev/null
+++ b/node_modules/shelljs/.idea/encodings.xml
@@ -0,0 +1,6 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<project version="4">
+ <component name="Encoding">
+ <file url="PROJECT" charset="UTF-8" />
+ </component>
+</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml b/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml
new file mode 100644
index 0000000..e6d8387
--- /dev/null
+++ b/node_modules/shelljs/.idea/inspectionProfiles/Project_Default.xml
@@ -0,0 +1,7 @@
+<component name="InspectionProjectProfileManager">
+ <profile version="1.0">
+ <option name="myName" value="Project Default" />
+ <inspection_tool class="JSLastCommaInArrayLiteral" enabled="false" level="WARNING" enabled_by_default="false" />
+ <inspection_tool class="JSLastCommaInObjectLiteral" enabled="false" level="WARNING" enabled_by_default="false" />
+ </profile>
+</component>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml b/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml
new file mode 100644
index 0000000..3b31283
--- /dev/null
+++ b/node_modules/shelljs/.idea/inspectionProfiles/profiles_settings.xml
@@ -0,0 +1,7 @@
+<component name="InspectionProjectProfileManager">
+ <settings>
+ <option name="PROJECT_PROFILE" value="Project Default" />
+ <option name="USE_PROJECT_PROFILE" value="true" />
+ <version value="1.0" />
+ </settings>
+</component>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/jsLibraryMappings.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/jsLibraryMappings.xml b/node_modules/shelljs/.idea/jsLibraryMappings.xml
new file mode 100644
index 0000000..40bdc77
--- /dev/null
+++ b/node_modules/shelljs/.idea/jsLibraryMappings.xml
@@ -0,0 +1,6 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<project version="4">
+ <component name="JavaScriptLibraryMappings">
+ <file url="file://$PROJECT_DIR$" libraries="{shelljs node_modules}" />
+ </component>
+</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml b/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml
new file mode 100644
index 0000000..b1e5dce
--- /dev/null
+++ b/node_modules/shelljs/.idea/libraries/shelljs_node_modules.xml
@@ -0,0 +1,14 @@
+<component name="libraryTable">
+ <library name="shelljs node_modules" type="javaScript">
+ <properties>
+ <option name="frameworkName" value="node_modules" />
+ <sourceFilesUrls>
+ <item url="file://$PROJECT_DIR$/node_modules" />
+ </sourceFilesUrls>
+ </properties>
+ <CLASSES>
+ <root url="file://$PROJECT_DIR$/node_modules" />
+ </CLASSES>
+ <SOURCES />
+ </library>
+</component>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/misc.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/misc.xml b/node_modules/shelljs/.idea/misc.xml
new file mode 100644
index 0000000..f524616
--- /dev/null
+++ b/node_modules/shelljs/.idea/misc.xml
@@ -0,0 +1,28 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<project version="4">
+ <component name="ProjectLevelVcsManager" settingsEditedManually="false">
+ <OptionsSetting value="true" id="Add" />
+ <OptionsSetting value="true" id="Remove" />
+ <OptionsSetting value="true" id="Checkout" />
+ <OptionsSetting value="true" id="Update" />
+ <OptionsSetting value="true" id="Status" />
+ <OptionsSetting value="true" id="Edit" />
+ <ConfirmationsSetting value="0" id="Add" />
+ <ConfirmationsSetting value="0" id="Remove" />
+ </component>
+ <component name="masterDetails">
+ <states>
+ <state key="ScopeChooserConfigurable.UI">
+ <settings>
+ <splitter-proportions>
+ <option name="proportions">
+ <list>
+ <option value="0.2" />
+ </list>
+ </option>
+ </splitter-proportions>
+ </settings>
+ </state>
+ </states>
+ </component>
+</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/modules.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/modules.xml b/node_modules/shelljs/.idea/modules.xml
new file mode 100644
index 0000000..26f2275
--- /dev/null
+++ b/node_modules/shelljs/.idea/modules.xml
@@ -0,0 +1,8 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<project version="4">
+ <component name="ProjectModuleManager">
+ <modules>
+ <module fileurl="file://$PROJECT_DIR$/.idea/shelljs.iml" filepath="$PROJECT_DIR$/.idea/shelljs.iml" />
+ </modules>
+ </component>
+</project>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/shelljs.iml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/shelljs.iml b/node_modules/shelljs/.idea/shelljs.iml
new file mode 100644
index 0000000..89f6e57
--- /dev/null
+++ b/node_modules/shelljs/.idea/shelljs.iml
@@ -0,0 +1,9 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<module type="WEB_MODULE" version="4">
+ <component name="NewModuleRootManager">
+ <content url="file://$MODULE_DIR$" />
+ <orderEntry type="inheritedJdk" />
+ <orderEntry type="sourceFolder" forTests="false" />
+ <orderEntry type="library" name="shelljs node_modules" level="project" />
+ </component>
+</module>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/.idea/vcs.xml
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/.idea/vcs.xml b/node_modules/shelljs/.idea/vcs.xml
new file mode 100644
index 0000000..94a25f7
--- /dev/null
+++ b/node_modules/shelljs/.idea/vcs.xml
@@ -0,0 +1,6 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<project version="4">
+ <component name="VcsDirectoryMappings">
+ <mapping directory="$PROJECT_DIR$" vcs="Git" />
+ </component>
+</project>
\ No newline at end of file
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[38/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/noConflict.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/noConflict.js b/node_modules/lodash-node/compat/utilities/noConflict.js
new file mode 100644
index 0000000..eb78149
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/noConflict.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to restore the original `_` reference in `noConflict` */
+var oldDash = global._;
+
+/**
+ * Reverts the '_' variable to its previous value and returns a reference to
+ * the `lodash` function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @returns {Function} Returns the `lodash` function.
+ * @example
+ *
+ * var lodash = _.noConflict();
+ */
+function noConflict() {
+ global._ = oldDash;
+ return this;
+}
+
+module.exports = noConflict;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/noop.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/noop.js b/node_modules/lodash-node/compat/utilities/noop.js
new file mode 100644
index 0000000..3cd2d53
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/noop.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * A no-operation function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * _.noop(object) === undefined;
+ * // => true
+ */
+function noop() {
+ // no operation performed
+}
+
+module.exports = noop;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/now.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/now.js b/node_modules/lodash-node/compat/utilities/now.js
new file mode 100644
index 0000000..ee091dc
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/now.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative');
+
+/**
+ * Gets the number of milliseconds that have elapsed since the Unix epoch
+ * (1 January 1970 00:00:00 UTC).
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @example
+ *
+ * var stamp = _.now();
+ * _.defer(function() { console.log(_.now() - stamp); });
+ * // => logs the number of milliseconds it took for the deferred function to be called
+ */
+var now = isNative(now = Date.now) && now || function() {
+ return new Date().getTime();
+};
+
+module.exports = now;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/parseInt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/parseInt.js b/node_modules/lodash-node/compat/utilities/parseInt.js
new file mode 100644
index 0000000..6227ccd
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/parseInt.js
@@ -0,0 +1,53 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isString = require('../objects/isString');
+
+/** Used to detect and test whitespace */
+var whitespace = (
+ // whitespace
+ ' \t\x0B\f\xA0\ufeff' +
+
+ // line terminators
+ '\n\r\u2028\u2029' +
+
+ // unicode category "Zs" space separators
+ '\u1680\u180e\u2000\u2001\u2002\u2003\u2004\u2005\u2006\u2007\u2008\u2009\u200a\u202f\u205f\u3000'
+);
+
+/** Used to match leading whitespace and zeros to be removed */
+var reLeadingSpacesAndZeros = RegExp('^[' + whitespace + ']*0+(?=.$)');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeParseInt = global.parseInt;
+
+/**
+ * Converts the given value into an integer of the specified radix.
+ * If `radix` is `undefined` or `0` a `radix` of `10` is used unless the
+ * `value` is a hexadecimal, in which case a `radix` of `16` is used.
+ *
+ * Note: This method avoids differences in native ES3 and ES5 `parseInt`
+ * implementations. See http://es5.github.io/#E.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} value The value to parse.
+ * @param {number} [radix] The radix used to interpret the value to parse.
+ * @returns {number} Returns the new integer value.
+ * @example
+ *
+ * _.parseInt('08');
+ * // => 8
+ */
+var parseInt = nativeParseInt(whitespace + '08') == 8 ? nativeParseInt : function(value, radix) {
+ // Firefox < 21 and Opera < 15 follow the ES3 specified implementation of `parseInt`
+ return nativeParseInt(isString(value) ? value.replace(reLeadingSpacesAndZeros, '') : value, radix || 0);
+};
+
+module.exports = parseInt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/property.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/property.js b/node_modules/lodash-node/compat/utilities/property.js
new file mode 100644
index 0000000..f3446dd
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/property.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates a "_.pluck" style function, which returns the `key` value of a
+ * given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} key The name of the property to retrieve.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'barney', 'age': 36 }
+ * ];
+ *
+ * var getName = _.property('name');
+ *
+ * _.map(characters, getName);
+ * // => ['barney', 'fred']
+ *
+ * _.sortBy(characters, getName);
+ * // => [{ 'name': 'barney', 'age': 36 }, { 'name': 'fred', 'age': 40 }]
+ */
+function property(key) {
+ return function(object) {
+ return object[key];
+ };
+}
+
+module.exports = property;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/random.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/random.js b/node_modules/lodash-node/compat/utilities/random.js
new file mode 100644
index 0000000..286bd1e
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/random.js
@@ -0,0 +1,73 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMin = Math.min,
+ nativeRandom = Math.random;
+
+/**
+ * Produces a random number between `min` and `max` (inclusive). If only one
+ * argument is provided a number between `0` and the given number will be
+ * returned. If `floating` is truey or either `min` or `max` are floats a
+ * floating-point number will be returned instead of an integer.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {number} [min=0] The minimum possible value.
+ * @param {number} [max=1] The maximum possible value.
+ * @param {boolean} [floating=false] Specify returning a floating-point number.
+ * @returns {number} Returns a random number.
+ * @example
+ *
+ * _.random(0, 5);
+ * // => an integer between 0 and 5
+ *
+ * _.random(5);
+ * // => also an integer between 0 and 5
+ *
+ * _.random(5, true);
+ * // => a floating-point number between 0 and 5
+ *
+ * _.random(1.2, 5.2);
+ * // => a floating-point number between 1.2 and 5.2
+ */
+function random(min, max, floating) {
+ var noMin = min == null,
+ noMax = max == null;
+
+ if (floating == null) {
+ if (typeof min == 'boolean' && noMax) {
+ floating = min;
+ min = 1;
+ }
+ else if (!noMax && typeof max == 'boolean') {
+ floating = max;
+ noMax = true;
+ }
+ }
+ if (noMin && noMax) {
+ max = 1;
+ }
+ min = +min || 0;
+ if (noMax) {
+ max = min;
+ min = 0;
+ } else {
+ max = +max || 0;
+ }
+ if (floating || min % 1 || max % 1) {
+ var rand = nativeRandom();
+ return nativeMin(min + (rand * (max - min + parseFloat('1e-' + ((rand +'').length - 1)))), max);
+ }
+ return baseRandom(min, max);
+}
+
+module.exports = random;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/result.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/result.js b/node_modules/lodash-node/compat/utilities/result.js
new file mode 100644
index 0000000..306b563
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/result.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Resolves the value of property `key` on `object`. If `key` is a function
+ * it will be invoked with the `this` binding of `object` and its result returned,
+ * else the property value is returned. If `object` is falsey then `undefined`
+ * is returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Object} object The object to inspect.
+ * @param {string} key The name of the property to resolve.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = {
+ * 'cheese': 'crumpets',
+ * 'stuff': function() {
+ * return 'nonsense';
+ * }
+ * };
+ *
+ * _.result(object, 'cheese');
+ * // => 'crumpets'
+ *
+ * _.result(object, 'stuff');
+ * // => 'nonsense'
+ */
+function result(object, key) {
+ if (object) {
+ var value = object[key];
+ return isFunction(value) ? object[key]() : value;
+ }
+}
+
+module.exports = result;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/template.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/template.js b/node_modules/lodash-node/compat/utilities/template.js
new file mode 100644
index 0000000..b4906b3
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/template.js
@@ -0,0 +1,216 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var defaults = require('../objects/defaults'),
+ escape = require('./escape'),
+ escapeStringChar = require('../internals/escapeStringChar'),
+ keys = require('../objects/keys'),
+ reInterpolate = require('../internals/reInterpolate'),
+ templateSettings = require('./templateSettings'),
+ values = require('../objects/values');
+
+/** Used to match empty string literals in compiled template source */
+var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+/**
+ * Used to match ES6 template delimiters
+ * http://people.mozilla.org/~jorendorff/es6-draft.html#sec-literals-string-literals
+ */
+var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+/** Used to ensure capturing order of template delimiters */
+var reNoMatch = /($^)/;
+
+/** Used to match unescaped characters in compiled string literals */
+var reUnescapedString = /['\n\r\t\u2028\u2029\\]/g;
+
+/**
+ * A micro-templating method that handles arbitrary delimiters, preserves
+ * whitespace, and correctly escapes quotes within interpolated code.
+ *
+ * Note: In the development build, `_.template` utilizes sourceURLs for easier
+ * debugging. See http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl
+ *
+ * For more information on precompiling templates see:
+ * http://lodash.com/custom-builds
+ *
+ * For more information on Chrome extension sandboxes see:
+ * http://developer.chrome.com/stable/extensions/sandboxingEval.html
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} text The template text.
+ * @param {Object} data The data object used to populate the text.
+ * @param {Object} [options] The options object.
+ * @param {RegExp} [options.escape] The "escape" delimiter.
+ * @param {RegExp} [options.evaluate] The "evaluate" delimiter.
+ * @param {Object} [options.imports] An object to import into the template as local variables.
+ * @param {RegExp} [options.interpolate] The "interpolate" delimiter.
+ * @param {string} [sourceURL] The sourceURL of the template's compiled source.
+ * @param {string} [variable] The data object variable name.
+ * @returns {Function|string} Returns a compiled function when no `data` object
+ * is given, else it returns the interpolated text.
+ * @example
+ *
+ * // using the "interpolate" delimiter to create a compiled template
+ * var compiled = _.template('hello <%= name %>');
+ * compiled({ 'name': 'fred' });
+ * // => 'hello fred'
+ *
+ * // using the "escape" delimiter to escape HTML in data property values
+ * _.template('<b><%- value %></b>', { 'value': '<script>' });
+ * // => '<b><script></b>'
+ *
+ * // using the "evaluate" delimiter to generate HTML
+ * var list = '<% _.forEach(people, function(name) { %><li><%- name %></li><% }); %>';
+ * _.template(list, { 'people': ['fred', 'barney'] });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the ES6 delimiter as an alternative to the default "interpolate" delimiter
+ * _.template('hello ${ name }', { 'name': 'pebbles' });
+ * // => 'hello pebbles'
+ *
+ * // using the internal `print` function in "evaluate" delimiters
+ * _.template('<% print("hello " + name); %>!', { 'name': 'barney' });
+ * // => 'hello barney!'
+ *
+ * // using a custom template delimiters
+ * _.templateSettings = {
+ * 'interpolate': /{{([\s\S]+?)}}/g
+ * };
+ *
+ * _.template('hello {{ name }}!', { 'name': 'mustache' });
+ * // => 'hello mustache!'
+ *
+ * // using the `imports` option to import jQuery
+ * var list = '<% jq.each(people, function(name) { %><li><%- name %></li><% }); %>';
+ * _.template(list, { 'people': ['fred', 'barney'] }, { 'imports': { 'jq': jQuery } });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the `sourceURL` option to specify a custom sourceURL for the template
+ * var compiled = _.template('hello <%= name %>', null, { 'sourceURL': '/basic/greeting.jst' });
+ * compiled(data);
+ * // => find the source of "greeting.jst" under the Sources tab or Resources panel of the web inspector
+ *
+ * // using the `variable` option to ensure a with-statement isn't used in the compiled template
+ * var compiled = _.template('hi <%= data.name %>!', null, { 'variable': 'data' });
+ * compiled.source;
+ * // => function(data) {
+ * var __t, __p = '', __e = _.escape;
+ * __p += 'hi ' + ((__t = ( data.name )) == null ? '' : __t) + '!';
+ * return __p;
+ * }
+ *
+ * // using the `source` property to inline compiled templates for meaningful
+ * // line numbers in error messages and a stack trace
+ * fs.writeFileSync(path.join(cwd, 'jst.js'), '\
+ * var JST = {\
+ * "main": ' + _.template(mainText).source + '\
+ * };\
+ * ');
+ */
+function template(text, data, options) {
+ // based on John Resig's `tmpl` implementation
+ // http://ejohn.org/blog/javascript-micro-templating/
+ // and Laura Doktorova's doT.js
+ // https://github.com/olado/doT
+ var settings = templateSettings.imports._.templateSettings || templateSettings;
+ text = String(text || '');
+
+ // avoid missing dependencies when `iteratorTemplate` is not defined
+ options = defaults({}, options, settings);
+
+ var imports = defaults({}, options.imports, settings.imports),
+ importsKeys = keys(imports),
+ importsValues = values(imports);
+
+ var isEvaluating,
+ index = 0,
+ interpolate = options.interpolate || reNoMatch,
+ source = "__p += '";
+
+ // compile the regexp to match each delimiter
+ var reDelimiters = RegExp(
+ (options.escape || reNoMatch).source + '|' +
+ interpolate.source + '|' +
+ (interpolate === reInterpolate ? reEsTemplate : reNoMatch).source + '|' +
+ (options.evaluate || reNoMatch).source + '|$'
+ , 'g');
+
+ text.replace(reDelimiters, function(match, escapeValue, interpolateValue, esTemplateValue, evaluateValue, offset) {
+ interpolateValue || (interpolateValue = esTemplateValue);
+
+ // escape characters that cannot be included in string literals
+ source += text.slice(index, offset).replace(reUnescapedString, escapeStringChar);
+
+ // replace delimiters with snippets
+ if (escapeValue) {
+ source += "' +\n__e(" + escapeValue + ") +\n'";
+ }
+ if (evaluateValue) {
+ isEvaluating = true;
+ source += "';\n" + evaluateValue + ";\n__p += '";
+ }
+ if (interpolateValue) {
+ source += "' +\n((__t = (" + interpolateValue + ")) == null ? '' : __t) +\n'";
+ }
+ index = offset + match.length;
+
+ // the JS engine embedded in Adobe products requires returning the `match`
+ // string in order to produce the correct `offset` value
+ return match;
+ });
+
+ source += "';\n";
+
+ // if `variable` is not specified, wrap a with-statement around the generated
+ // code to add the data object to the top of the scope chain
+ var variable = options.variable,
+ hasVariable = variable;
+
+ if (!hasVariable) {
+ variable = 'obj';
+ source = 'with (' + variable + ') {\n' + source + '\n}\n';
+ }
+ // cleanup code by stripping empty strings
+ source = (isEvaluating ? source.replace(reEmptyStringLeading, '') : source)
+ .replace(reEmptyStringMiddle, '$1')
+ .replace(reEmptyStringTrailing, '$1;');
+
+ // frame code as the function body
+ source = 'function(' + variable + ') {\n' +
+ (hasVariable ? '' : variable + ' || (' + variable + ' = {});\n') +
+ "var __t, __p = '', __e = _.escape" +
+ (isEvaluating
+ ? ', __j = Array.prototype.join;\n' +
+ "function print() { __p += __j.call(arguments, '') }\n"
+ : ';\n'
+ ) +
+ source +
+ 'return __p\n}';
+
+ try {
+ var result = Function(importsKeys, 'return ' + source ).apply(undefined, importsValues);
+ } catch(e) {
+ e.source = source;
+ throw e;
+ }
+ if (data) {
+ return result(data);
+ }
+ // provide the compiled function's source by its `toString` method, in
+ // supported environments, or the `source` property as a convenience for
+ // inlining compiled templates during the build process
+ result.source = source;
+ return result;
+}
+
+module.exports = template;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/templateSettings.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/templateSettings.js b/node_modules/lodash-node/compat/utilities/templateSettings.js
new file mode 100644
index 0000000..d8acdbf
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/templateSettings.js
@@ -0,0 +1,73 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var escape = require('./escape'),
+ reInterpolate = require('../internals/reInterpolate');
+
+/**
+ * By default, the template delimiters used by Lo-Dash are similar to those in
+ * embedded Ruby (ERB). Change the following template settings to use alternative
+ * delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'escape': /<%-([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'evaluate': /<%([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type string
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type Object
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type Function
+ */
+ '_': { 'escape': escape }
+ }
+};
+
+module.exports = templateSettings;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/times.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/times.js b/node_modules/lodash-node/compat/utilities/times.js
new file mode 100644
index 0000000..85e5c32
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/times.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Executes the callback `n` times, returning an array of the results
+ * of each callback execution. The callback is bound to `thisArg` and invoked
+ * with one argument; (index).
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {number} n The number of times to execute the callback.
+ * @param {Function} callback The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns an array of the results of each `callback` execution.
+ * @example
+ *
+ * var diceRolls = _.times(3, _.partial(_.random, 1, 6));
+ * // => [3, 6, 4]
+ *
+ * _.times(3, function(n) { mage.castSpell(n); });
+ * // => calls `mage.castSpell(n)` three times, passing `n` of `0`, `1`, and `2` respectively
+ *
+ * _.times(3, function(n) { this.cast(n); }, mage);
+ * // => also calls `mage.castSpell(n)` three times
+ */
+function times(n, callback, thisArg) {
+ n = (n = +n) > -1 ? n : 0;
+ var index = -1,
+ result = Array(n);
+
+ callback = baseCreateCallback(callback, thisArg, 1);
+ while (++index < n) {
+ result[index] = callback(index);
+ }
+ return result;
+}
+
+module.exports = times;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/unescape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/unescape.js b/node_modules/lodash-node/compat/utilities/unescape.js
new file mode 100644
index 0000000..dec526f
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/unescape.js
@@ -0,0 +1,32 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys'),
+ reEscapedHtml = require('../internals/reEscapedHtml'),
+ unescapeHtmlChar = require('../internals/unescapeHtmlChar');
+
+/**
+ * The inverse of `_.escape` this method converts the HTML entities
+ * `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding characters.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} string The string to unescape.
+ * @returns {string} Returns the unescaped string.
+ * @example
+ *
+ * _.unescape('Fred, Barney & Pebbles');
+ * // => 'Fred, Barney & Pebbles'
+ */
+function unescape(string) {
+ return string == null ? '' : String(string).replace(reEscapedHtml, unescapeHtmlChar);
+}
+
+module.exports = unescape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/uniqueId.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/uniqueId.js b/node_modules/lodash-node/compat/utilities/uniqueId.js
new file mode 100644
index 0000000..6c9346e
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/uniqueId.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to generate unique IDs */
+var idCounter = 0;
+
+/**
+ * Generates a unique ID. If `prefix` is provided the ID will be appended to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} [prefix] The value to prefix the ID with.
+ * @returns {string} Returns the unique ID.
+ * @example
+ *
+ * _.uniqueId('contact_');
+ * // => 'contact_104'
+ *
+ * _.uniqueId();
+ * // => '105'
+ */
+function uniqueId(prefix) {
+ var id = ++idCounter;
+ return String(prefix == null ? '' : prefix) + id;
+}
+
+module.exports = uniqueId;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays.js b/node_modules/lodash-node/modern/arrays.js
new file mode 100644
index 0000000..f6c37ff
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'compact': require('./arrays/compact'),
+ 'difference': require('./arrays/difference'),
+ 'drop': require('./arrays/rest'),
+ 'findIndex': require('./arrays/findIndex'),
+ 'findLastIndex': require('./arrays/findLastIndex'),
+ 'first': require('./arrays/first'),
+ 'flatten': require('./arrays/flatten'),
+ 'head': require('./arrays/first'),
+ 'indexOf': require('./arrays/indexOf'),
+ 'initial': require('./arrays/initial'),
+ 'intersection': require('./arrays/intersection'),
+ 'last': require('./arrays/last'),
+ 'lastIndexOf': require('./arrays/lastIndexOf'),
+ 'object': require('./arrays/zipObject'),
+ 'pull': require('./arrays/pull'),
+ 'range': require('./arrays/range'),
+ 'remove': require('./arrays/remove'),
+ 'rest': require('./arrays/rest'),
+ 'sortedIndex': require('./arrays/sortedIndex'),
+ 'tail': require('./arrays/rest'),
+ 'take': require('./arrays/first'),
+ 'union': require('./arrays/union'),
+ 'uniq': require('./arrays/uniq'),
+ 'unique': require('./arrays/uniq'),
+ 'unzip': require('./arrays/zip'),
+ 'without': require('./arrays/without'),
+ 'xor': require('./arrays/xor'),
+ 'zip': require('./arrays/zip'),
+ 'zipObject': require('./arrays/zipObject')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/compact.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/compact.js b/node_modules/lodash-node/modern/arrays/compact.js
new file mode 100644
index 0000000..f0bffba
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/compact.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are all falsey.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+function compact(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = compact;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/difference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/difference.js b/node_modules/lodash-node/modern/arrays/difference.js
new file mode 100644
index 0000000..22d16f0
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/difference.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseFlatten = require('../internals/baseFlatten');
+
+/**
+ * Creates an array excluding all values of the provided arrays using strict
+ * equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {...Array} [values] The arrays of values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.difference([1, 2, 3, 4, 5], [5, 2, 10]);
+ * // => [1, 3, 4]
+ */
+function difference(array) {
+ return baseDifference(array, baseFlatten(arguments, true, true, 1));
+}
+
+module.exports = difference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/findIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/findIndex.js b/node_modules/lodash-node/modern/arrays/findIndex.js
new file mode 100644
index 0000000..549d010
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/findIndex.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback');
+
+/**
+ * This method is like `_.find` except that it returns the index of the first
+ * element that passes the callback check, instead of the element itself.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ * ];
+ *
+ * _.findIndex(characters, function(chr) {
+ * return chr.age < 20;
+ * });
+ * // => 2
+ *
+ * // using "_.where" callback shorthand
+ * _.findIndex(characters, { 'age': 36 });
+ * // => 0
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findIndex(characters, 'blocked');
+ * // => 1
+ */
+function findIndex(array, callback, thisArg) {
+ var index = -1,
+ length = array ? array.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length) {
+ if (callback(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = findIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/findLastIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/findLastIndex.js b/node_modules/lodash-node/modern/arrays/findLastIndex.js
new file mode 100644
index 0000000..f487f71
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/findLastIndex.js
@@ -0,0 +1,63 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback');
+
+/**
+ * This method is like `_.findIndex` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': true },
+ * { 'name': 'fred', 'age': 40, 'blocked': false },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': true }
+ * ];
+ *
+ * _.findLastIndex(characters, function(chr) {
+ * return chr.age > 30;
+ * });
+ * // => 1
+ *
+ * // using "_.where" callback shorthand
+ * _.findLastIndex(characters, { 'age': 36 });
+ * // => 0
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findLastIndex(characters, 'blocked');
+ * // => 2
+ */
+function findLastIndex(array, callback, thisArg) {
+ var length = array ? array.length : 0;
+ callback = createCallback(callback, thisArg, 3);
+ while (length--) {
+ if (callback(array[length], length, array)) {
+ return length;
+ }
+ }
+ return -1;
+}
+
+module.exports = findLastIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/first.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/first.js b/node_modules/lodash-node/modern/arrays/first.js
new file mode 100644
index 0000000..f7d802e
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/first.js
@@ -0,0 +1,86 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the first element or first `n` elements of an array. If a callback
+ * is provided elements at the beginning of the array are returned as long
+ * as the callback returns truey. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias head, take
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the first element(s) of `array`.
+ * @example
+ *
+ * _.first([1, 2, 3]);
+ * // => 1
+ *
+ * _.first([1, 2, 3], 2);
+ * // => [1, 2]
+ *
+ * _.first([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [1, 2]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.first(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'blocked': true, 'employer': 'slate' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.pluck(_.first(characters, { 'employer': 'slate' }), 'name');
+ * // => ['barney', 'fred']
+ */
+function first(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = -1;
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array ? array[0] : undefined;
+ }
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, n), length));
+}
+
+module.exports = first;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/flatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/flatten.js b/node_modules/lodash-node/modern/arrays/flatten.js
new file mode 100644
index 0000000..49b9da9
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/flatten.js
@@ -0,0 +1,66 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ map = require('../collections/map');
+
+/**
+ * Flattens a nested array (the nesting can be to any depth). If `isShallow`
+ * is truey, the array will only be flattened a single level. If a callback
+ * is provided each element of the array is passed through the callback before
+ * flattening. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isShallow=false] A flag to restrict flattening to a single level.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2], [3, [[4]]]]);
+ * // => [1, 2, 3, 4];
+ *
+ * _.flatten([1, [2], [3, [[4]]]], true);
+ * // => [1, 2, 3, [[4]]];
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 30, 'pets': ['hoppy'] },
+ * { 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.flatten(characters, 'pets');
+ * // => ['hoppy', 'baby puss', 'dino']
+ */
+function flatten(array, isShallow, callback, thisArg) {
+ // juggle arguments
+ if (typeof isShallow != 'boolean' && isShallow != null) {
+ thisArg = callback;
+ callback = (typeof isShallow != 'function' && thisArg && thisArg[isShallow] === array) ? null : isShallow;
+ isShallow = false;
+ }
+ if (callback != null) {
+ array = map(array, callback, thisArg);
+ }
+ return baseFlatten(array, isShallow);
+}
+
+module.exports = flatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/indexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/indexOf.js b/node_modules/lodash-node/modern/arrays/indexOf.js
new file mode 100644
index 0000000..c7533e8
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/indexOf.js
@@ -0,0 +1,50 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ sortedIndex = require('./sortedIndex');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Gets the index at which the first occurrence of `value` is found using
+ * strict equality for comparisons, i.e. `===`. If the array is already sorted
+ * providing `true` for `fromIndex` will run a faster binary search.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {boolean|number} [fromIndex=0] The index to search from or `true`
+ * to perform a binary search on a sorted array.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 1
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 4
+ *
+ * _.indexOf([1, 1, 2, 2, 3, 3], 2, true);
+ * // => 2
+ */
+function indexOf(array, value, fromIndex) {
+ if (typeof fromIndex == 'number') {
+ var length = array ? array.length : 0;
+ fromIndex = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex || 0);
+ } else if (fromIndex) {
+ var index = sortedIndex(array, value);
+ return array[index] === value ? index : -1;
+ }
+ return baseIndexOf(array, value, fromIndex);
+}
+
+module.exports = indexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/initial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/initial.js b/node_modules/lodash-node/modern/arrays/initial.js
new file mode 100644
index 0000000..c1441a5
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/initial.js
@@ -0,0 +1,82 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets all but the last element or last `n` elements of an array. If a
+ * callback is provided elements at the end of the array are excluded from
+ * the result as long as the callback returns truey. The callback is bound
+ * to `thisArg` and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.initial([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.initial([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [1]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.initial(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'blocked': false, 'employer': 'slate' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.pluck(_.initial(characters, { 'employer': 'na' }), 'name');
+ * // => ['barney', 'fred']
+ */
+function initial(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg, 3);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : callback || n;
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, length - n), length));
+}
+
+module.exports = initial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/intersection.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/intersection.js b/node_modules/lodash-node/modern/arrays/intersection.js
new file mode 100644
index 0000000..a49371d
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/intersection.js
@@ -0,0 +1,83 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ cacheIndexOf = require('../internals/cacheIndexOf'),
+ createCache = require('../internals/createCache'),
+ getArray = require('../internals/getArray'),
+ isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray'),
+ largeArraySize = require('../internals/largeArraySize'),
+ releaseArray = require('../internals/releaseArray'),
+ releaseObject = require('../internals/releaseObject');
+
+/**
+ * Creates an array of unique values present in all provided arrays using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of shared values.
+ * @example
+ *
+ * _.intersection([1, 2, 3], [5, 2, 1, 4], [2, 1]);
+ * // => [1, 2]
+ */
+function intersection() {
+ var args = [],
+ argsIndex = -1,
+ argsLength = arguments.length,
+ caches = getArray(),
+ indexOf = baseIndexOf,
+ trustIndexOf = indexOf === baseIndexOf,
+ seen = getArray();
+
+ while (++argsIndex < argsLength) {
+ var value = arguments[argsIndex];
+ if (isArray(value) || isArguments(value)) {
+ args.push(value);
+ caches.push(trustIndexOf && value.length >= largeArraySize &&
+ createCache(argsIndex ? args[argsIndex] : seen));
+ }
+ }
+ var array = args[0],
+ index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ outer:
+ while (++index < length) {
+ var cache = caches[0];
+ value = array[index];
+
+ if ((cache ? cacheIndexOf(cache, value) : indexOf(seen, value)) < 0) {
+ argsIndex = argsLength;
+ (cache || seen).push(value);
+ while (--argsIndex) {
+ cache = caches[argsIndex];
+ if ((cache ? cacheIndexOf(cache, value) : indexOf(args[argsIndex], value)) < 0) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ }
+ while (argsLength--) {
+ cache = caches[argsLength];
+ if (cache) {
+ releaseObject(cache);
+ }
+ }
+ releaseArray(caches);
+ releaseArray(seen);
+ return result;
+}
+
+module.exports = intersection;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/last.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/last.js b/node_modules/lodash-node/modern/arrays/last.js
new file mode 100644
index 0000000..4cd82d8
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/last.js
@@ -0,0 +1,84 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Gets the last element or last `n` elements of an array. If a callback is
+ * provided elements at the end of the array are returned as long as the
+ * callback returns truey. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the last element(s) of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ *
+ * _.last([1, 2, 3], 2);
+ * // => [2, 3]
+ *
+ * _.last([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [2, 3]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.pluck(_.last(characters, 'blocked'), 'name');
+ * // => ['fred', 'pebbles']
+ *
+ * // using "_.where" callback shorthand
+ * _.last(characters, { 'employer': 'na' });
+ * // => [{ 'name': 'pebbles', 'blocked': true, 'employer': 'na' }]
+ */
+function last(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg, 3);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array ? array[length - 1] : undefined;
+ }
+ }
+ return slice(array, nativeMax(0, length - n));
+}
+
+module.exports = last;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/lastIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/lastIndexOf.js b/node_modules/lodash-node/modern/arrays/lastIndexOf.js
new file mode 100644
index 0000000..51c8210
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/lastIndexOf.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the index at which the last occurrence of `value` is found using strict
+ * equality for comparisons, i.e. `===`. If `fromIndex` is negative, it is used
+ * as the offset from the end of the collection.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=array.length-1] The index to search from.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 4
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 1
+ */
+function lastIndexOf(array, value, fromIndex) {
+ var index = array ? array.length : 0;
+ if (typeof fromIndex == 'number') {
+ index = (fromIndex < 0 ? nativeMax(0, index + fromIndex) : nativeMin(fromIndex, index - 1)) + 1;
+ }
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = lastIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/pull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/pull.js b/node_modules/lodash-node/modern/arrays/pull.js
new file mode 100644
index 0000000..d7526ea
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/pull.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var splice = arrayRef.splice;
+
+/**
+ * Removes all provided values from the given array using strict equality for
+ * comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to modify.
+ * @param {...*} [value] The values to remove.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3, 1, 2, 3];
+ * _.pull(array, 2, 3);
+ * console.log(array);
+ * // => [1, 1]
+ */
+function pull(array) {
+ var args = arguments,
+ argsIndex = 0,
+ argsLength = args.length,
+ length = array ? array.length : 0;
+
+ while (++argsIndex < argsLength) {
+ var index = -1,
+ value = args[argsIndex];
+ while (++index < length) {
+ if (array[index] === value) {
+ splice.call(array, index--, 1);
+ length--;
+ }
+ }
+ }
+ return array;
+}
+
+module.exports = pull;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/range.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/range.js b/node_modules/lodash-node/modern/arrays/range.js
new file mode 100644
index 0000000..bbc4ab5
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/range.js
@@ -0,0 +1,69 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Native method shortcuts */
+var ceil = Math.ceil;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Creates an array of numbers (positive and/or negative) progressing from
+ * `start` up to but not including `end`. If `start` is less than `stop` a
+ * zero-length range is created unless a negative `step` is specified.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @param {number} [step=1] The value to increment or decrement by.
+ * @returns {Array} Returns a new range array.
+ * @example
+ *
+ * _.range(4);
+ * // => [0, 1, 2, 3]
+ *
+ * _.range(1, 5);
+ * // => [1, 2, 3, 4]
+ *
+ * _.range(0, 20, 5);
+ * // => [0, 5, 10, 15]
+ *
+ * _.range(0, -4, -1);
+ * // => [0, -1, -2, -3]
+ *
+ * _.range(1, 4, 0);
+ * // => [1, 1, 1]
+ *
+ * _.range(0);
+ * // => []
+ */
+function range(start, end, step) {
+ start = +start || 0;
+ step = typeof step == 'number' ? step : (+step || 1);
+
+ if (end == null) {
+ end = start;
+ start = 0;
+ }
+ // use `Array(length)` so engines like Chakra and V8 avoid slower modes
+ // http://youtu.be/XAqIpGU8ZZk#t=17m25s
+ var index = -1,
+ length = nativeMax(0, ceil((end - start) / (step || 1))),
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = start;
+ start += step;
+ }
+ return result;
+}
+
+module.exports = range;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/remove.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/remove.js b/node_modules/lodash-node/modern/arrays/remove.js
new file mode 100644
index 0000000..dc818d5
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/remove.js
@@ -0,0 +1,71 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var splice = arrayRef.splice;
+
+/**
+ * Removes all elements from an array that the callback returns truey for
+ * and returns an array of removed elements. The callback is bound to `thisArg`
+ * and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to modify.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of removed elements.
+ * @example
+ *
+ * var array = [1, 2, 3, 4, 5, 6];
+ * var evens = _.remove(array, function(num) { return num % 2 == 0; });
+ *
+ * console.log(array);
+ * // => [1, 3, 5]
+ *
+ * console.log(evens);
+ * // => [2, 4, 6]
+ */
+function remove(array, callback, thisArg) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length) {
+ var value = array[index];
+ if (callback(value, index, array)) {
+ result.push(value);
+ splice.call(array, index--, 1);
+ length--;
+ }
+ }
+ return result;
+}
+
+module.exports = remove;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/rest.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/rest.js b/node_modules/lodash-node/modern/arrays/rest.js
new file mode 100644
index 0000000..216672e
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/rest.js
@@ -0,0 +1,83 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * The opposite of `_.initial` this method gets all but the first element or
+ * first `n` elements of an array. If a callback function is provided elements
+ * at the beginning of the array are excluded from the result as long as the
+ * callback returns truey. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias drop, tail
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.rest([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.rest([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.rest([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [3]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.pluck(_.rest(characters, 'blocked'), 'name');
+ * // => ['fred', 'pebbles']
+ *
+ * // using "_.where" callback shorthand
+ * _.rest(characters, { 'employer': 'slate' });
+ * // => [{ 'name': 'pebbles', 'blocked': true, 'employer': 'na' }]
+ */
+function rest(array, callback, thisArg) {
+ if (typeof callback != 'number' && callback != null) {
+ var n = 0,
+ index = -1,
+ length = array ? array.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : nativeMax(0, callback);
+ }
+ return slice(array, n);
+}
+
+module.exports = rest;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/sortedIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/sortedIndex.js b/node_modules/lodash-node/modern/arrays/sortedIndex.js
new file mode 100644
index 0000000..b4318b5
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/sortedIndex.js
@@ -0,0 +1,77 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ identity = require('../utilities/identity');
+
+/**
+ * Uses a binary search to determine the smallest index at which a value
+ * should be inserted into a given sorted array in order to maintain the sort
+ * order of the array. If a callback is provided it will be executed for
+ * `value` and each element of `array` to compute their sort ranking. The
+ * callback is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedIndex([20, 30, 50], 40);
+ * // => 2
+ *
+ * // using "_.pluck" callback shorthand
+ * _.sortedIndex([{ 'x': 20 }, { 'x': 30 }, { 'x': 50 }], { 'x': 40 }, 'x');
+ * // => 2
+ *
+ * var dict = {
+ * 'wordToNumber': { 'twenty': 20, 'thirty': 30, 'fourty': 40, 'fifty': 50 }
+ * };
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return dict.wordToNumber[word];
+ * });
+ * // => 2
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return this.wordToNumber[word];
+ * }, dict);
+ * // => 2
+ */
+function sortedIndex(array, value, callback, thisArg) {
+ var low = 0,
+ high = array ? array.length : low;
+
+ // explicitly reference `identity` for better inlining in Firefox
+ callback = callback ? createCallback(callback, thisArg, 1) : identity;
+ value = callback(value);
+
+ while (low < high) {
+ var mid = (low + high) >>> 1;
+ (callback(array[mid]) < value)
+ ? low = mid + 1
+ : high = mid;
+ }
+ return low;
+}
+
+module.exports = sortedIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/union.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/union.js b/node_modules/lodash-node/modern/arrays/union.js
new file mode 100644
index 0000000..bb1b2af
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/union.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ baseUniq = require('../internals/baseUniq');
+
+/**
+ * Creates an array of unique values, in order, of the provided arrays using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of combined values.
+ * @example
+ *
+ * _.union([1, 2, 3], [5, 2, 1, 4], [2, 1]);
+ * // => [1, 2, 3, 5, 4]
+ */
+function union() {
+ return baseUniq(baseFlatten(arguments, true, true));
+}
+
+module.exports = union;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/uniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/uniq.js b/node_modules/lodash-node/modern/arrays/uniq.js
new file mode 100644
index 0000000..8388c2a
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/uniq.js
@@ -0,0 +1,69 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseUniq = require('../internals/baseUniq'),
+ createCallback = require('../functions/createCallback');
+
+/**
+ * Creates a duplicate-value-free version of an array using strict equality
+ * for comparisons, i.e. `===`. If the array is sorted, providing
+ * `true` for `isSorted` will use a faster algorithm. If a callback is provided
+ * each element of `array` is passed through the callback before uniqueness
+ * is computed. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias unique
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {boolean} [isSorted=false] A flag to indicate that `array` is sorted.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a duplicate-value-free array.
+ * @example
+ *
+ * _.uniq([1, 2, 1, 3, 1]);
+ * // => [1, 2, 3]
+ *
+ * _.uniq([1, 1, 2, 2, 3], true);
+ * // => [1, 2, 3]
+ *
+ * _.uniq(['A', 'b', 'C', 'a', 'B', 'c'], function(letter) { return letter.toLowerCase(); });
+ * // => ['A', 'b', 'C']
+ *
+ * _.uniq([1, 2.5, 3, 1.5, 2, 3.5], function(num) { return this.floor(num); }, Math);
+ * // => [1, 2.5, 3]
+ *
+ * // using "_.pluck" callback shorthand
+ * _.uniq([{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+function uniq(array, isSorted, callback, thisArg) {
+ // juggle arguments
+ if (typeof isSorted != 'boolean' && isSorted != null) {
+ thisArg = callback;
+ callback = (typeof isSorted != 'function' && thisArg && thisArg[isSorted] === array) ? null : isSorted;
+ isSorted = false;
+ }
+ if (callback != null) {
+ callback = createCallback(callback, thisArg, 3);
+ }
+ return baseUniq(array, isSorted, callback);
+}
+
+module.exports = uniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/without.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/without.js b/node_modules/lodash-node/modern/arrays/without.js
new file mode 100644
index 0000000..f988395
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/without.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates an array excluding all provided values using strict equality for
+ * comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to filter.
+ * @param {...*} [value] The values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.without([1, 2, 1, 0, 3, 1, 4], 0, 1);
+ * // => [2, 3, 4]
+ */
+function without(array) {
+ return baseDifference(array, slice(arguments, 1));
+}
+
+module.exports = without;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/xor.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/xor.js b/node_modules/lodash-node/modern/arrays/xor.js
new file mode 100644
index 0000000..d76393f
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/xor.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseUniq = require('../internals/baseUniq'),
+ isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates an array that is the symmetric difference of the provided arrays.
+ * See http://en.wikipedia.org/wiki/Symmetric_difference.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of values.
+ * @example
+ *
+ * _.xor([1, 2, 3], [5, 2, 1, 4]);
+ * // => [3, 5, 4]
+ *
+ * _.xor([1, 2, 5], [2, 3, 5], [3, 4, 5]);
+ * // => [1, 4, 5]
+ */
+function xor() {
+ var index = -1,
+ length = arguments.length;
+
+ while (++index < length) {
+ var array = arguments[index];
+ if (isArray(array) || isArguments(array)) {
+ var result = result
+ ? baseUniq(baseDifference(result, array).concat(baseDifference(array, result)))
+ : array;
+ }
+ }
+ return result || [];
+}
+
+module.exports = xor;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/zip.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/zip.js b/node_modules/lodash-node/modern/arrays/zip.js
new file mode 100644
index 0000000..740d91c
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/zip.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var max = require('../collections/max'),
+ pluck = require('../collections/pluck');
+
+/**
+ * Creates an array of grouped elements, the first of which contains the first
+ * elements of the given arrays, the second of which contains the second
+ * elements of the given arrays, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @alias unzip
+ * @category Arrays
+ * @param {...Array} [array] Arrays to process.
+ * @returns {Array} Returns a new array of grouped elements.
+ * @example
+ *
+ * _.zip(['fred', 'barney'], [30, 40], [true, false]);
+ * // => [['fred', 30, true], ['barney', 40, false]]
+ */
+function zip() {
+ var array = arguments.length > 1 ? arguments : arguments[0],
+ index = -1,
+ length = array ? max(pluck(array, 'length')) : 0,
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = pluck(array, index);
+ }
+ return result;
+}
+
+module.exports = zip;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/arrays/zipObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/arrays/zipObject.js b/node_modules/lodash-node/modern/arrays/zipObject.js
new file mode 100644
index 0000000..889bea3
--- /dev/null
+++ b/node_modules/lodash-node/modern/arrays/zipObject.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArray = require('../objects/isArray');
+
+/**
+ * Creates an object composed from arrays of `keys` and `values`. Provide
+ * either a single two dimensional array, i.e. `[[key1, value1], [key2, value2]]`
+ * or two arrays, one of `keys` and one of corresponding `values`.
+ *
+ * @static
+ * @memberOf _
+ * @alias object
+ * @category Arrays
+ * @param {Array} keys The array of keys.
+ * @param {Array} [values=[]] The array of values.
+ * @returns {Object} Returns an object composed of the given keys and
+ * corresponding values.
+ * @example
+ *
+ * _.zipObject(['fred', 'barney'], [30, 40]);
+ * // => { 'fred': 30, 'barney': 40 }
+ */
+function zipObject(keys, values) {
+ var index = -1,
+ length = keys ? keys.length : 0,
+ result = {};
+
+ if (!values && length && !isArray(keys[0])) {
+ values = [];
+ }
+ while (++index < length) {
+ var key = keys[index];
+ if (values) {
+ result[key] = values[index];
+ } else if (key) {
+ result[key[0]] = key[1];
+ }
+ }
+ return result;
+}
+
+module.exports = zipObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/chaining.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/chaining.js b/node_modules/lodash-node/modern/chaining.js
new file mode 100644
index 0000000..f4b5258
--- /dev/null
+++ b/node_modules/lodash-node/modern/chaining.js
@@ -0,0 +1,17 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'chain': require('./chaining/chain'),
+ 'tap': require('./chaining/tap'),
+ 'value': require('./chaining/wrapperValueOf'),
+ 'wrapperChain': require('./chaining/wrapperChain'),
+ 'wrapperToString': require('./chaining/wrapperToString'),
+ 'wrapperValueOf': require('./chaining/wrapperValueOf')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/chaining/chain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/chaining/chain.js b/node_modules/lodash-node/modern/chaining/chain.js
new file mode 100644
index 0000000..05c0038
--- /dev/null
+++ b/node_modules/lodash-node/modern/chaining/chain.js
@@ -0,0 +1,41 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var lodashWrapper = require('../internals/lodashWrapper');
+
+/**
+ * Creates a `lodash` object that wraps the given value with explicit
+ * method chaining enabled.
+ *
+ * @static
+ * @memberOf _
+ * @category Chaining
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the wrapper object.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _.chain(characters)
+ * .sortBy('age')
+ * .map(function(chr) { return chr.name + ' is ' + chr.age; })
+ * .first()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+function chain(value) {
+ value = new lodashWrapper(value);
+ value.__chain__ = true;
+ return value;
+}
+
+module.exports = chain;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[27/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/index.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/index.js b/node_modules/lodash/index.js
new file mode 100644
index 0000000..5f17319
--- /dev/null
+++ b/node_modules/lodash/index.js
@@ -0,0 +1,12351 @@
+/**
+ * @license
+ * lodash 3.10.1 (Custom Build) <https://lodash.com/>
+ * Build: `lodash modern -d -o ./index.js`
+ * Copyright 2012-2015 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.8.3 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <https://lodash.com/license>
+ */
+;(function() {
+
+ /** Used as a safe reference for `undefined` in pre-ES5 environments. */
+ var undefined;
+
+ /** Used as the semantic version number. */
+ var VERSION = '3.10.1';
+
+ /** Used to compose bitmasks for wrapper metadata. */
+ var BIND_FLAG = 1,
+ BIND_KEY_FLAG = 2,
+ CURRY_BOUND_FLAG = 4,
+ CURRY_FLAG = 8,
+ CURRY_RIGHT_FLAG = 16,
+ PARTIAL_FLAG = 32,
+ PARTIAL_RIGHT_FLAG = 64,
+ ARY_FLAG = 128,
+ REARG_FLAG = 256;
+
+ /** Used as default options for `_.trunc`. */
+ var DEFAULT_TRUNC_LENGTH = 30,
+ DEFAULT_TRUNC_OMISSION = '...';
+
+ /** Used to detect when a function becomes hot. */
+ var HOT_COUNT = 150,
+ HOT_SPAN = 16;
+
+ /** Used as the size to enable large array optimizations. */
+ var LARGE_ARRAY_SIZE = 200;
+
+ /** Used to indicate the type of lazy iteratees. */
+ var LAZY_FILTER_FLAG = 1,
+ LAZY_MAP_FLAG = 2;
+
+ /** Used as the `TypeError` message for "Functions" methods. */
+ var FUNC_ERROR_TEXT = 'Expected a function';
+
+ /** Used as the internal argument placeholder. */
+ var PLACEHOLDER = '__lodash_placeholder__';
+
+ /** `Object#toString` result references. */
+ var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ weakMapTag = '[object WeakMap]';
+
+ var arrayBufferTag = '[object ArrayBuffer]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+ /** Used to match empty string literals in compiled template source. */
+ var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+ /** Used to match HTML entities and HTML characters. */
+ var reEscapedHtml = /&(?:amp|lt|gt|quot|#39|#96);/g,
+ reUnescapedHtml = /[&<>"'`]/g,
+ reHasEscapedHtml = RegExp(reEscapedHtml.source),
+ reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
+
+ /** Used to match template delimiters. */
+ var reEscape = /<%-([\s\S]+?)%>/g,
+ reEvaluate = /<%([\s\S]+?)%>/g,
+ reInterpolate = /<%=([\s\S]+?)%>/g;
+
+ /** Used to match property names within property paths. */
+ var reIsDeepProp = /\.|\[(?:[^[\]]*|(["'])(?:(?!\1)[^\n\\]|\\.)*?\1)\]/,
+ reIsPlainProp = /^\w*$/,
+ rePropName = /[^.[\]]+|\[(?:(-?\d+(?:\.\d+)?)|(["'])((?:(?!\2)[^\n\\]|\\.)*?)\2)\]/g;
+
+ /**
+ * Used to match `RegExp` [syntax characters](http://ecma-international.org/ecma-262/6.0/#sec-patterns)
+ * and those outlined by [`EscapeRegExpPattern`](http://ecma-international.org/ecma-262/6.0/#sec-escaperegexppattern).
+ */
+ var reRegExpChars = /^[:!,]|[\\^$.*+?()[\]{}|\/]|(^[0-9a-fA-Fnrtuvx])|([\n\r\u2028\u2029])/g,
+ reHasRegExpChars = RegExp(reRegExpChars.source);
+
+ /** Used to match [combining diacritical marks](https://en.wikipedia.org/wiki/Combining_Diacritical_Marks). */
+ var reComboMark = /[\u0300-\u036f\ufe20-\ufe23]/g;
+
+ /** Used to match backslashes in property paths. */
+ var reEscapeChar = /\\(\\)?/g;
+
+ /** Used to match [ES template delimiters](http://ecma-international.org/ecma-262/6.0/#sec-template-literal-lexical-components). */
+ var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+ /** Used to match `RegExp` flags from their coerced string values. */
+ var reFlags = /\w*$/;
+
+ /** Used to detect hexadecimal string values. */
+ var reHasHexPrefix = /^0[xX]/;
+
+ /** Used to detect host constructors (Safari > 5). */
+ var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+ /** Used to detect unsigned integer values. */
+ var reIsUint = /^\d+$/;
+
+ /** Used to match latin-1 supplementary letters (excluding mathematical operators). */
+ var reLatin1 = /[\xc0-\xd6\xd8-\xde\xdf-\xf6\xf8-\xff]/g;
+
+ /** Used to ensure capturing order of template delimiters. */
+ var reNoMatch = /($^)/;
+
+ /** Used to match unescaped characters in compiled string literals. */
+ var reUnescapedString = /['\n\r\u2028\u2029\\]/g;
+
+ /** Used to match words to create compound words. */
+ var reWords = (function() {
+ var upper = '[A-Z\\xc0-\\xd6\\xd8-\\xde]',
+ lower = '[a-z\\xdf-\\xf6\\xf8-\\xff]+';
+
+ return RegExp(upper + '+(?=' + upper + lower + ')|' + upper + '?' + lower + '|' + upper + '+|[0-9]+', 'g');
+ }());
+
+ /** Used to assign default `context` object properties. */
+ var contextProps = [
+ 'Array', 'ArrayBuffer', 'Date', 'Error', 'Float32Array', 'Float64Array',
+ 'Function', 'Int8Array', 'Int16Array', 'Int32Array', 'Math', 'Number',
+ 'Object', 'RegExp', 'Set', 'String', '_', 'clearTimeout', 'isFinite',
+ 'parseFloat', 'parseInt', 'setTimeout', 'TypeError', 'Uint8Array',
+ 'Uint8ClampedArray', 'Uint16Array', 'Uint32Array', 'WeakMap'
+ ];
+
+ /** Used to make template sourceURLs easier to identify. */
+ var templateCounter = -1;
+
+ /** Used to identify `toStringTag` values of typed arrays. */
+ var typedArrayTags = {};
+ typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
+ typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
+ typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
+ typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
+ typedArrayTags[uint32Tag] = true;
+ typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
+ typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
+ typedArrayTags[dateTag] = typedArrayTags[errorTag] =
+ typedArrayTags[funcTag] = typedArrayTags[mapTag] =
+ typedArrayTags[numberTag] = typedArrayTags[objectTag] =
+ typedArrayTags[regexpTag] = typedArrayTags[setTag] =
+ typedArrayTags[stringTag] = typedArrayTags[weakMapTag] = false;
+
+ /** Used to identify `toStringTag` values supported by `_.clone`. */
+ var cloneableTags = {};
+ cloneableTags[argsTag] = cloneableTags[arrayTag] =
+ cloneableTags[arrayBufferTag] = cloneableTags[boolTag] =
+ cloneableTags[dateTag] = cloneableTags[float32Tag] =
+ cloneableTags[float64Tag] = cloneableTags[int8Tag] =
+ cloneableTags[int16Tag] = cloneableTags[int32Tag] =
+ cloneableTags[numberTag] = cloneableTags[objectTag] =
+ cloneableTags[regexpTag] = cloneableTags[stringTag] =
+ cloneableTags[uint8Tag] = cloneableTags[uint8ClampedTag] =
+ cloneableTags[uint16Tag] = cloneableTags[uint32Tag] = true;
+ cloneableTags[errorTag] = cloneableTags[funcTag] =
+ cloneableTags[mapTag] = cloneableTags[setTag] =
+ cloneableTags[weakMapTag] = false;
+
+ /** Used to map latin-1 supplementary letters to basic latin letters. */
+ var deburredLetters = {
+ '\xc0': 'A', '\xc1': 'A', '\xc2': 'A', '\xc3': 'A', '\xc4': 'A', '\xc5': 'A',
+ '\xe0': 'a', '\xe1': 'a', '\xe2': 'a', '\xe3': 'a', '\xe4': 'a', '\xe5': 'a',
+ '\xc7': 'C', '\xe7': 'c',
+ '\xd0': 'D', '\xf0': 'd',
+ '\xc8': 'E', '\xc9': 'E', '\xca': 'E', '\xcb': 'E',
+ '\xe8': 'e', '\xe9': 'e', '\xea': 'e', '\xeb': 'e',
+ '\xcC': 'I', '\xcd': 'I', '\xce': 'I', '\xcf': 'I',
+ '\xeC': 'i', '\xed': 'i', '\xee': 'i', '\xef': 'i',
+ '\xd1': 'N', '\xf1': 'n',
+ '\xd2': 'O', '\xd3': 'O', '\xd4': 'O', '\xd5': 'O', '\xd6': 'O', '\xd8': 'O',
+ '\xf2': 'o', '\xf3': 'o', '\xf4': 'o', '\xf5': 'o', '\xf6': 'o', '\xf8': 'o',
+ '\xd9': 'U', '\xda': 'U', '\xdb': 'U', '\xdc': 'U',
+ '\xf9': 'u', '\xfa': 'u', '\xfb': 'u', '\xfc': 'u',
+ '\xdd': 'Y', '\xfd': 'y', '\xff': 'y',
+ '\xc6': 'Ae', '\xe6': 'ae',
+ '\xde': 'Th', '\xfe': 'th',
+ '\xdf': 'ss'
+ };
+
+ /** Used to map characters to HTML entities. */
+ var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": ''',
+ '`': '`'
+ };
+
+ /** Used to map HTML entities to characters. */
+ var htmlUnescapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'",
+ '`': '`'
+ };
+
+ /** Used to determine if values are of the language type `Object`. */
+ var objectTypes = {
+ 'function': true,
+ 'object': true
+ };
+
+ /** Used to escape characters for inclusion in compiled regexes. */
+ var regexpEscapes = {
+ '0': 'x30', '1': 'x31', '2': 'x32', '3': 'x33', '4': 'x34',
+ '5': 'x35', '6': 'x36', '7': 'x37', '8': 'x38', '9': 'x39',
+ 'A': 'x41', 'B': 'x42', 'C': 'x43', 'D': 'x44', 'E': 'x45', 'F': 'x46',
+ 'a': 'x61', 'b': 'x62', 'c': 'x63', 'd': 'x64', 'e': 'x65', 'f': 'x66',
+ 'n': 'x6e', 'r': 'x72', 't': 'x74', 'u': 'x75', 'v': 'x76', 'x': 'x78'
+ };
+
+ /** Used to escape characters for inclusion in compiled string literals. */
+ var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+ };
+
+ /** Detect free variable `exports`. */
+ var freeExports = objectTypes[typeof exports] && exports && !exports.nodeType && exports;
+
+ /** Detect free variable `module`. */
+ var freeModule = objectTypes[typeof module] && module && !module.nodeType && module;
+
+ /** Detect free variable `global` from Node.js. */
+ var freeGlobal = freeExports && freeModule && typeof global == 'object' && global && global.Object && global;
+
+ /** Detect free variable `self`. */
+ var freeSelf = objectTypes[typeof self] && self && self.Object && self;
+
+ /** Detect free variable `window`. */
+ var freeWindow = objectTypes[typeof window] && window && window.Object && window;
+
+ /** Detect the popular CommonJS extension `module.exports`. */
+ var moduleExports = freeModule && freeModule.exports === freeExports && freeExports;
+
+ /**
+ * Used as a reference to the global object.
+ *
+ * The `this` value is used if it's the global object to avoid Greasemonkey's
+ * restricted `window` object, otherwise the `window` object is used.
+ */
+ var root = freeGlobal || ((freeWindow !== (this && this.window)) && freeWindow) || freeSelf || this;
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * The base implementation of `compareAscending` which compares values and
+ * sorts them in ascending order without guaranteeing a stable sort.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {number} Returns the sort order indicator for `value`.
+ */
+ function baseCompareAscending(value, other) {
+ if (value !== other) {
+ var valIsNull = value === null,
+ valIsUndef = value === undefined,
+ valIsReflexive = value === value;
+
+ var othIsNull = other === null,
+ othIsUndef = other === undefined,
+ othIsReflexive = other === other;
+
+ if ((value > other && !othIsNull) || !valIsReflexive ||
+ (valIsNull && !othIsUndef && othIsReflexive) ||
+ (valIsUndef && othIsReflexive)) {
+ return 1;
+ }
+ if ((value < other && !valIsNull) || !othIsReflexive ||
+ (othIsNull && !valIsUndef && valIsReflexive) ||
+ (othIsUndef && valIsReflexive)) {
+ return -1;
+ }
+ }
+ return 0;
+ }
+
+ /**
+ * The base implementation of `_.findIndex` and `_.findLastIndex` without
+ * support for callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function baseFindIndex(array, predicate, fromRight) {
+ var length = array.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (predicate(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * The base implementation of `_.indexOf` without support for binary searches.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+ function baseIndexOf(array, value, fromIndex) {
+ if (value !== value) {
+ return indexOfNaN(array, fromIndex);
+ }
+ var index = fromIndex - 1,
+ length = array.length;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * The base implementation of `_.isFunction` without support for environments
+ * with incorrect `typeof` results.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ */
+ function baseIsFunction(value) {
+ // Avoid a Chakra JIT bug in compatibility modes of IE 11.
+ // See https://github.com/jashkenas/underscore/issues/1621 for more details.
+ return typeof value == 'function' || false;
+ }
+
+ /**
+ * Converts `value` to a string if it's not one. An empty string is returned
+ * for `null` or `undefined` values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+ function baseToString(value) {
+ return value == null ? '' : (value + '');
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimLeft` to get the index of the first character
+ * of `string` that is not found in `chars`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @param {string} chars The characters to find.
+ * @returns {number} Returns the index of the first character not found in `chars`.
+ */
+ function charsLeftIndex(string, chars) {
+ var index = -1,
+ length = string.length;
+
+ while (++index < length && chars.indexOf(string.charAt(index)) > -1) {}
+ return index;
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimRight` to get the index of the last character
+ * of `string` that is not found in `chars`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @param {string} chars The characters to find.
+ * @returns {number} Returns the index of the last character not found in `chars`.
+ */
+ function charsRightIndex(string, chars) {
+ var index = string.length;
+
+ while (index-- && chars.indexOf(string.charAt(index)) > -1) {}
+ return index;
+ }
+
+ /**
+ * Used by `_.sortBy` to compare transformed elements of a collection and stable
+ * sort them in ascending order.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @returns {number} Returns the sort order indicator for `object`.
+ */
+ function compareAscending(object, other) {
+ return baseCompareAscending(object.criteria, other.criteria) || (object.index - other.index);
+ }
+
+ /**
+ * Used by `_.sortByOrder` to compare multiple properties of a value to another
+ * and stable sort them.
+ *
+ * If `orders` is unspecified, all valuess are sorted in ascending order. Otherwise,
+ * a value is sorted in ascending order if its corresponding order is "asc", and
+ * descending if "desc".
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {boolean[]} orders The order to sort by for each property.
+ * @returns {number} Returns the sort order indicator for `object`.
+ */
+ function compareMultiple(object, other, orders) {
+ var index = -1,
+ objCriteria = object.criteria,
+ othCriteria = other.criteria,
+ length = objCriteria.length,
+ ordersLength = orders.length;
+
+ while (++index < length) {
+ var result = baseCompareAscending(objCriteria[index], othCriteria[index]);
+ if (result) {
+ if (index >= ordersLength) {
+ return result;
+ }
+ var order = orders[index];
+ return result * ((order === 'asc' || order === true) ? 1 : -1);
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to provide the same value for
+ // `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247
+ // for more details.
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See https://code.google.com/p/v8/issues/detail?id=90 for more details.
+ return object.index - other.index;
+ }
+
+ /**
+ * Used by `_.deburr` to convert latin-1 supplementary letters to basic latin letters.
+ *
+ * @private
+ * @param {string} letter The matched letter to deburr.
+ * @returns {string} Returns the deburred letter.
+ */
+ function deburrLetter(letter) {
+ return deburredLetters[letter];
+ }
+
+ /**
+ * Used by `_.escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+ function escapeHtmlChar(chr) {
+ return htmlEscapes[chr];
+ }
+
+ /**
+ * Used by `_.escapeRegExp` to escape characters for inclusion in compiled regexes.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @param {string} leadingChar The capture group for a leading character.
+ * @param {string} whitespaceChar The capture group for a whitespace character.
+ * @returns {string} Returns the escaped character.
+ */
+ function escapeRegExpChar(chr, leadingChar, whitespaceChar) {
+ if (leadingChar) {
+ chr = regexpEscapes[chr];
+ } else if (whitespaceChar) {
+ chr = stringEscapes[chr];
+ }
+ return '\\' + chr;
+ }
+
+ /**
+ * Used by `_.template` to escape characters for inclusion in compiled string literals.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+ function escapeStringChar(chr) {
+ return '\\' + stringEscapes[chr];
+ }
+
+ /**
+ * Gets the index at which the first occurrence of `NaN` is found in `array`.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {number} fromIndex The index to search from.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched `NaN`, else `-1`.
+ */
+ function indexOfNaN(array, fromIndex, fromRight) {
+ var length = array.length,
+ index = fromIndex + (fromRight ? 0 : -1);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ var other = array[index];
+ if (other !== other) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * Checks if `value` is object-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ */
+ function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+ }
+
+ /**
+ * Used by `trimmedLeftIndex` and `trimmedRightIndex` to determine if a
+ * character code is whitespace.
+ *
+ * @private
+ * @param {number} charCode The character code to inspect.
+ * @returns {boolean} Returns `true` if `charCode` is whitespace, else `false`.
+ */
+ function isSpace(charCode) {
+ return ((charCode <= 160 && (charCode >= 9 && charCode <= 13) || charCode == 32 || charCode == 160) || charCode == 5760 || charCode == 6158 ||
+ (charCode >= 8192 && (charCode <= 8202 || charCode == 8232 || charCode == 8233 || charCode == 8239 || charCode == 8287 || charCode == 12288 || charCode == 65279)));
+ }
+
+ /**
+ * Replaces all `placeholder` elements in `array` with an internal placeholder
+ * and returns an array of their indexes.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {*} placeholder The placeholder to replace.
+ * @returns {Array} Returns the new array of placeholder indexes.
+ */
+ function replaceHolders(array, placeholder) {
+ var index = -1,
+ length = array.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ if (array[index] === placeholder) {
+ array[index] = PLACEHOLDER;
+ result[++resIndex] = index;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * An implementation of `_.uniq` optimized for sorted arrays without support
+ * for callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The function invoked per iteration.
+ * @returns {Array} Returns the new duplicate-value-free array.
+ */
+ function sortedUniq(array, iteratee) {
+ var seen,
+ index = -1,
+ length = array.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value, index, array) : value;
+
+ if (!index || seen !== computed) {
+ seen = computed;
+ result[++resIndex] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimLeft` to get the index of the first non-whitespace
+ * character of `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the index of the first non-whitespace character.
+ */
+ function trimmedLeftIndex(string) {
+ var index = -1,
+ length = string.length;
+
+ while (++index < length && isSpace(string.charCodeAt(index))) {}
+ return index;
+ }
+
+ /**
+ * Used by `_.trim` and `_.trimRight` to get the index of the last non-whitespace
+ * character of `string`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @returns {number} Returns the index of the last non-whitespace character.
+ */
+ function trimmedRightIndex(string) {
+ var index = string.length;
+
+ while (index-- && isSpace(string.charCodeAt(index))) {}
+ return index;
+ }
+
+ /**
+ * Used by `_.unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} chr The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+ function unescapeHtmlChar(chr) {
+ return htmlUnescapes[chr];
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Create a new pristine `lodash` function using the given `context` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Object} [context=root] The context object.
+ * @returns {Function} Returns a new `lodash` function.
+ * @example
+ *
+ * _.mixin({ 'foo': _.constant('foo') });
+ *
+ * var lodash = _.runInContext();
+ * lodash.mixin({ 'bar': lodash.constant('bar') });
+ *
+ * _.isFunction(_.foo);
+ * // => true
+ * _.isFunction(_.bar);
+ * // => false
+ *
+ * lodash.isFunction(lodash.foo);
+ * // => false
+ * lodash.isFunction(lodash.bar);
+ * // => true
+ *
+ * // using `context` to mock `Date#getTime` use in `_.now`
+ * var mock = _.runInContext({
+ * 'Date': function() {
+ * return { 'getTime': getTimeMock };
+ * }
+ * });
+ *
+ * // or creating a suped-up `defer` in Node.js
+ * var defer = _.runInContext({ 'setTimeout': setImmediate }).defer;
+ */
+ function runInContext(context) {
+ // Avoid issues with some ES3 environments that attempt to use values, named
+ // after built-in constructors like `Object`, for the creation of literals.
+ // ES5 clears this up by stating that literals must use built-in constructors.
+ // See https://es5.github.io/#x11.1.5 for more details.
+ context = context ? _.defaults(root.Object(), context, _.pick(root, contextProps)) : root;
+
+ /** Native constructor references. */
+ var Array = context.Array,
+ Date = context.Date,
+ Error = context.Error,
+ Function = context.Function,
+ Math = context.Math,
+ Number = context.Number,
+ Object = context.Object,
+ RegExp = context.RegExp,
+ String = context.String,
+ TypeError = context.TypeError;
+
+ /** Used for native method references. */
+ var arrayProto = Array.prototype,
+ objectProto = Object.prototype,
+ stringProto = String.prototype;
+
+ /** Used to resolve the decompiled source of functions. */
+ var fnToString = Function.prototype.toString;
+
+ /** Used to check objects for own properties. */
+ var hasOwnProperty = objectProto.hasOwnProperty;
+
+ /** Used to generate unique IDs. */
+ var idCounter = 0;
+
+ /**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+ var objToString = objectProto.toString;
+
+ /** Used to restore the original `_` reference in `_.noConflict`. */
+ var oldDash = root._;
+
+ /** Used to detect if a method is native. */
+ var reIsNative = RegExp('^' +
+ fnToString.call(hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+ );
+
+ /** Native method references. */
+ var ArrayBuffer = context.ArrayBuffer,
+ clearTimeout = context.clearTimeout,
+ parseFloat = context.parseFloat,
+ pow = Math.pow,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable,
+ Set = getNative(context, 'Set'),
+ setTimeout = context.setTimeout,
+ splice = arrayProto.splice,
+ Uint8Array = context.Uint8Array,
+ WeakMap = getNative(context, 'WeakMap');
+
+ /* Native method references for those with the same name as other `lodash` methods. */
+ var nativeCeil = Math.ceil,
+ nativeCreate = getNative(Object, 'create'),
+ nativeFloor = Math.floor,
+ nativeIsArray = getNative(Array, 'isArray'),
+ nativeIsFinite = context.isFinite,
+ nativeKeys = getNative(Object, 'keys'),
+ nativeMax = Math.max,
+ nativeMin = Math.min,
+ nativeNow = getNative(Date, 'now'),
+ nativeParseInt = context.parseInt,
+ nativeRandom = Math.random;
+
+ /** Used as references for `-Infinity` and `Infinity`. */
+ var NEGATIVE_INFINITY = Number.NEGATIVE_INFINITY,
+ POSITIVE_INFINITY = Number.POSITIVE_INFINITY;
+
+ /** Used as references for the maximum length and index of an array. */
+ var MAX_ARRAY_LENGTH = 4294967295,
+ MAX_ARRAY_INDEX = MAX_ARRAY_LENGTH - 1,
+ HALF_MAX_ARRAY_LENGTH = MAX_ARRAY_LENGTH >>> 1;
+
+ /**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+ var MAX_SAFE_INTEGER = 9007199254740991;
+
+ /** Used to store function metadata. */
+ var metaMap = WeakMap && new WeakMap;
+
+ /** Used to lookup unminified function names. */
+ var realNames = {};
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a `lodash` object which wraps `value` to enable implicit chaining.
+ * Methods that operate on and return arrays, collections, and functions can
+ * be chained together. Methods that retrieve a single value or may return a
+ * primitive value will automatically end the chain returning the unwrapped
+ * value. Explicit chaining may be enabled using `_.chain`. The execution of
+ * chained methods is lazy, that is, execution is deferred until `_#value`
+ * is implicitly or explicitly called.
+ *
+ * Lazy evaluation allows several methods to support shortcut fusion. Shortcut
+ * fusion is an optimization strategy which merge iteratee calls; this can help
+ * to avoid the creation of intermediate data structures and greatly reduce the
+ * number of iteratee executions.
+ *
+ * Chaining is supported in custom builds as long as the `_#value` method is
+ * directly or indirectly included in the build.
+ *
+ * In addition to lodash methods, wrappers have `Array` and `String` methods.
+ *
+ * The wrapper `Array` methods are:
+ * `concat`, `join`, `pop`, `push`, `reverse`, `shift`, `slice`, `sort`,
+ * `splice`, and `unshift`
+ *
+ * The wrapper `String` methods are:
+ * `replace` and `split`
+ *
+ * The wrapper methods that support shortcut fusion are:
+ * `compact`, `drop`, `dropRight`, `dropRightWhile`, `dropWhile`, `filter`,
+ * `first`, `initial`, `last`, `map`, `pluck`, `reject`, `rest`, `reverse`,
+ * `slice`, `take`, `takeRight`, `takeRightWhile`, `takeWhile`, `toArray`,
+ * and `where`
+ *
+ * The chainable wrapper methods are:
+ * `after`, `ary`, `assign`, `at`, `before`, `bind`, `bindAll`, `bindKey`,
+ * `callback`, `chain`, `chunk`, `commit`, `compact`, `concat`, `constant`,
+ * `countBy`, `create`, `curry`, `debounce`, `defaults`, `defaultsDeep`,
+ * `defer`, `delay`, `difference`, `drop`, `dropRight`, `dropRightWhile`,
+ * `dropWhile`, `fill`, `filter`, `flatten`, `flattenDeep`, `flow`, `flowRight`,
+ * `forEach`, `forEachRight`, `forIn`, `forInRight`, `forOwn`, `forOwnRight`,
+ * `functions`, `groupBy`, `indexBy`, `initial`, `intersection`, `invert`,
+ * `invoke`, `keys`, `keysIn`, `map`, `mapKeys`, `mapValues`, `matches`,
+ * `matchesProperty`, `memoize`, `merge`, `method`, `methodOf`, `mixin`,
+ * `modArgs`, `negate`, `omit`, `once`, `pairs`, `partial`, `partialRight`,
+ * `partition`, `pick`, `plant`, `pluck`, `property`, `propertyOf`, `pull`,
+ * `pullAt`, `push`, `range`, `rearg`, `reject`, `remove`, `rest`, `restParam`,
+ * `reverse`, `set`, `shuffle`, `slice`, `sort`, `sortBy`, `sortByAll`,
+ * `sortByOrder`, `splice`, `spread`, `take`, `takeRight`, `takeRightWhile`,
+ * `takeWhile`, `tap`, `throttle`, `thru`, `times`, `toArray`, `toPlainObject`,
+ * `transform`, `union`, `uniq`, `unshift`, `unzip`, `unzipWith`, `values`,
+ * `valuesIn`, `where`, `without`, `wrap`, `xor`, `zip`, `zipObject`, `zipWith`
+ *
+ * The wrapper methods that are **not** chainable by default are:
+ * `add`, `attempt`, `camelCase`, `capitalize`, `ceil`, `clone`, `cloneDeep`,
+ * `deburr`, `endsWith`, `escape`, `escapeRegExp`, `every`, `find`, `findIndex`,
+ * `findKey`, `findLast`, `findLastIndex`, `findLastKey`, `findWhere`, `first`,
+ * `floor`, `get`, `gt`, `gte`, `has`, `identity`, `includes`, `indexOf`,
+ * `inRange`, `isArguments`, `isArray`, `isBoolean`, `isDate`, `isElement`,
+ * `isEmpty`, `isEqual`, `isError`, `isFinite` `isFunction`, `isMatch`,
+ * `isNative`, `isNaN`, `isNull`, `isNumber`, `isObject`, `isPlainObject`,
+ * `isRegExp`, `isString`, `isUndefined`, `isTypedArray`, `join`, `kebabCase`,
+ * `last`, `lastIndexOf`, `lt`, `lte`, `max`, `min`, `noConflict`, `noop`,
+ * `now`, `pad`, `padLeft`, `padRight`, `parseInt`, `pop`, `random`, `reduce`,
+ * `reduceRight`, `repeat`, `result`, `round`, `runInContext`, `shift`, `size`,
+ * `snakeCase`, `some`, `sortedIndex`, `sortedLastIndex`, `startCase`,
+ * `startsWith`, `sum`, `template`, `trim`, `trimLeft`, `trimRight`, `trunc`,
+ * `unescape`, `uniqueId`, `value`, and `words`
+ *
+ * The wrapper method `sample` will return a wrapped value when `n` is provided,
+ * otherwise an unwrapped value is returned.
+ *
+ * @name _
+ * @constructor
+ * @category Chain
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // returns an unwrapped value
+ * wrapped.reduce(function(total, n) {
+ * return total + n;
+ * });
+ * // => 6
+ *
+ * // returns a wrapped value
+ * var squares = wrapped.map(function(n) {
+ * return n * n;
+ * });
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+ function lodash(value) {
+ if (isObjectLike(value) && !isArray(value) && !(value instanceof LazyWrapper)) {
+ if (value instanceof LodashWrapper) {
+ return value;
+ }
+ if (hasOwnProperty.call(value, '__chain__') && hasOwnProperty.call(value, '__wrapped__')) {
+ return wrapperClone(value);
+ }
+ }
+ return new LodashWrapper(value);
+ }
+
+ /**
+ * The function whose prototype all chaining wrappers inherit from.
+ *
+ * @private
+ */
+ function baseLodash() {
+ // No operation performed.
+ }
+
+ /**
+ * The base constructor for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ * @param {boolean} [chainAll] Enable chaining for all wrapper methods.
+ * @param {Array} [actions=[]] Actions to peform to resolve the unwrapped value.
+ */
+ function LodashWrapper(value, chainAll, actions) {
+ this.__wrapped__ = value;
+ this.__actions__ = actions || [];
+ this.__chain__ = !!chainAll;
+ }
+
+ /**
+ * An object environment feature flags.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+ var support = lodash.support = {};
+
+ /**
+ * By default, the template delimiters used by lodash are like those in
+ * embedded Ruby (ERB). Change the following template settings to use
+ * alternative delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+ lodash.templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'escape': reEscape,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'evaluate': reEvaluate,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type string
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type Object
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type Function
+ */
+ '_': lodash
+ }
+ };
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a lazy wrapper object which wraps `value` to enable lazy evaluation.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ */
+ function LazyWrapper(value) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__dir__ = 1;
+ this.__filtered__ = false;
+ this.__iteratees__ = [];
+ this.__takeCount__ = POSITIVE_INFINITY;
+ this.__views__ = [];
+ }
+
+ /**
+ * Creates a clone of the lazy wrapper object.
+ *
+ * @private
+ * @name clone
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the cloned `LazyWrapper` object.
+ */
+ function lazyClone() {
+ var result = new LazyWrapper(this.__wrapped__);
+ result.__actions__ = arrayCopy(this.__actions__);
+ result.__dir__ = this.__dir__;
+ result.__filtered__ = this.__filtered__;
+ result.__iteratees__ = arrayCopy(this.__iteratees__);
+ result.__takeCount__ = this.__takeCount__;
+ result.__views__ = arrayCopy(this.__views__);
+ return result;
+ }
+
+ /**
+ * Reverses the direction of lazy iteration.
+ *
+ * @private
+ * @name reverse
+ * @memberOf LazyWrapper
+ * @returns {Object} Returns the new reversed `LazyWrapper` object.
+ */
+ function lazyReverse() {
+ if (this.__filtered__) {
+ var result = new LazyWrapper(this);
+ result.__dir__ = -1;
+ result.__filtered__ = true;
+ } else {
+ result = this.clone();
+ result.__dir__ *= -1;
+ }
+ return result;
+ }
+
+ /**
+ * Extracts the unwrapped value from its lazy wrapper.
+ *
+ * @private
+ * @name value
+ * @memberOf LazyWrapper
+ * @returns {*} Returns the unwrapped value.
+ */
+ function lazyValue() {
+ var array = this.__wrapped__.value(),
+ dir = this.__dir__,
+ isArr = isArray(array),
+ isRight = dir < 0,
+ arrLength = isArr ? array.length : 0,
+ view = getView(0, arrLength, this.__views__),
+ start = view.start,
+ end = view.end,
+ length = end - start,
+ index = isRight ? end : (start - 1),
+ iteratees = this.__iteratees__,
+ iterLength = iteratees.length,
+ resIndex = 0,
+ takeCount = nativeMin(length, this.__takeCount__);
+
+ if (!isArr || arrLength < LARGE_ARRAY_SIZE || (arrLength == length && takeCount == length)) {
+ return baseWrapperValue((isRight && isArr) ? array.reverse() : array, this.__actions__);
+ }
+ var result = [];
+
+ outer:
+ while (length-- && resIndex < takeCount) {
+ index += dir;
+
+ var iterIndex = -1,
+ value = array[index];
+
+ while (++iterIndex < iterLength) {
+ var data = iteratees[iterIndex],
+ iteratee = data.iteratee,
+ type = data.type,
+ computed = iteratee(value);
+
+ if (type == LAZY_MAP_FLAG) {
+ value = computed;
+ } else if (!computed) {
+ if (type == LAZY_FILTER_FLAG) {
+ continue outer;
+ } else {
+ break outer;
+ }
+ }
+ }
+ result[resIndex++] = value;
+ }
+ return result;
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a cache object to store key/value pairs.
+ *
+ * @private
+ * @static
+ * @name Cache
+ * @memberOf _.memoize
+ */
+ function MapCache() {
+ this.__data__ = {};
+ }
+
+ /**
+ * Removes `key` and its value from the cache.
+ *
+ * @private
+ * @name delete
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed successfully, else `false`.
+ */
+ function mapDelete(key) {
+ return this.has(key) && delete this.__data__[key];
+ }
+
+ /**
+ * Gets the cached value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the cached value.
+ */
+ function mapGet(key) {
+ return key == '__proto__' ? undefined : this.__data__[key];
+ }
+
+ /**
+ * Checks if a cached value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+ function mapHas(key) {
+ return key != '__proto__' && hasOwnProperty.call(this.__data__, key);
+ }
+
+ /**
+ * Sets `value` to `key` of the cache.
+ *
+ * @private
+ * @name set
+ * @memberOf _.memoize.Cache
+ * @param {string} key The key of the value to cache.
+ * @param {*} value The value to cache.
+ * @returns {Object} Returns the cache object.
+ */
+ function mapSet(key, value) {
+ if (key != '__proto__') {
+ this.__data__[key] = value;
+ }
+ return this;
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ *
+ * Creates a cache object to store unique values.
+ *
+ * @private
+ * @param {Array} [values] The values to cache.
+ */
+ function SetCache(values) {
+ var length = values ? values.length : 0;
+
+ this.data = { 'hash': nativeCreate(null), 'set': new Set };
+ while (length--) {
+ this.push(values[length]);
+ }
+ }
+
+ /**
+ * Checks if `value` is in `cache` mimicking the return signature of
+ * `_.indexOf` by returning `0` if the value is found, else `-1`.
+ *
+ * @private
+ * @param {Object} cache The cache to search.
+ * @param {*} value The value to search for.
+ * @returns {number} Returns `0` if `value` is found, else `-1`.
+ */
+ function cacheIndexOf(cache, value) {
+ var data = cache.data,
+ result = (typeof value == 'string' || isObject(value)) ? data.set.has(value) : data.hash[value];
+
+ return result ? 0 : -1;
+ }
+
+ /**
+ * Adds `value` to the cache.
+ *
+ * @private
+ * @name push
+ * @memberOf SetCache
+ * @param {*} value The value to cache.
+ */
+ function cachePush(value) {
+ var data = this.data;
+ if (typeof value == 'string' || isObject(value)) {
+ data.set.add(value);
+ } else {
+ data.hash[value] = true;
+ }
+ }
+
+ /*------------------------------------------------------------------------*/
+
+ /**
+ * Creates a new array joining `array` with `other`.
+ *
+ * @private
+ * @param {Array} array The array to join.
+ * @param {Array} other The other array to join.
+ * @returns {Array} Returns the new concatenated array.
+ */
+ function arrayConcat(array, other) {
+ var index = -1,
+ length = array.length,
+ othIndex = -1,
+ othLength = other.length,
+ result = Array(length + othLength);
+
+ while (++index < length) {
+ result[index] = array[index];
+ }
+ while (++othIndex < othLength) {
+ result[index++] = other[othIndex];
+ }
+ return result;
+ }
+
+ /**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayCopy(source, array) {
+ var index = -1,
+ length = source.length;
+
+ array || (array = Array(length));
+ while (++index < length) {
+ array[index] = source[index];
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.forEach` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayEach(array, iteratee) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (iteratee(array[index], index, array) === false) {
+ break;
+ }
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.forEachRight` for arrays without support for
+ * callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayEachRight(array, iteratee) {
+ var length = array.length;
+
+ while (length--) {
+ if (iteratee(array[length], length, array) === false) {
+ break;
+ }
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.every` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ */
+ function arrayEvery(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (!predicate(array[index], index, array)) {
+ return false;
+ }
+ }
+ return true;
+ }
+
+ /**
+ * A specialized version of `baseExtremum` for arrays which invokes `iteratee`
+ * with one argument: (value).
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} comparator The function used to compare values.
+ * @param {*} exValue The initial extremum value.
+ * @returns {*} Returns the extremum value.
+ */
+ function arrayExtremum(array, iteratee, comparator, exValue) {
+ var index = -1,
+ length = array.length,
+ computed = exValue,
+ result = computed;
+
+ while (++index < length) {
+ var value = array[index],
+ current = +iteratee(value);
+
+ if (comparator(current, computed)) {
+ computed = current;
+ result = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `_.filter` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+ function arrayFilter(array, predicate) {
+ var index = -1,
+ length = array.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (predicate(value, index, array)) {
+ result[++resIndex] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * A specialized version of `_.map` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+ function arrayMap(array, iteratee) {
+ var index = -1,
+ length = array.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = iteratee(array[index], index, array);
+ }
+ return result;
+ }
+
+ /**
+ * Appends the elements of `values` to `array`.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to append.
+ * @returns {Array} Returns `array`.
+ */
+ function arrayPush(array, values) {
+ var index = -1,
+ length = values.length,
+ offset = array.length;
+
+ while (++index < length) {
+ array[offset + index] = values[index];
+ }
+ return array;
+ }
+
+ /**
+ * A specialized version of `_.reduce` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initFromArray] Specify using the first element of `array`
+ * as the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+ function arrayReduce(array, iteratee, accumulator, initFromArray) {
+ var index = -1,
+ length = array.length;
+
+ if (initFromArray && length) {
+ accumulator = array[++index];
+ }
+ while (++index < length) {
+ accumulator = iteratee(accumulator, array[index], index, array);
+ }
+ return accumulator;
+ }
+
+ /**
+ * A specialized version of `_.reduceRight` for arrays without support for
+ * callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initFromArray] Specify using the last element of `array`
+ * as the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+ function arrayReduceRight(array, iteratee, accumulator, initFromArray) {
+ var length = array.length;
+ if (initFromArray && length) {
+ accumulator = array[--length];
+ }
+ while (length--) {
+ accumulator = iteratee(accumulator, array[length], length, array);
+ }
+ return accumulator;
+ }
+
+ /**
+ * A specialized version of `_.some` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+ function arraySome(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (predicate(array[index], index, array)) {
+ return true;
+ }
+ }
+ return false;
+ }
+
+ /**
+ * A specialized version of `_.sum` for arrays without support for callback
+ * shorthands and `this` binding..
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the sum.
+ */
+ function arraySum(array, iteratee) {
+ var length = array.length,
+ result = 0;
+
+ while (length--) {
+ result += +iteratee(array[length]) || 0;
+ }
+ return result;
+ }
+
+ /**
+ * Used by `_.defaults` to customize its `_.assign` use.
+ *
+ * @private
+ * @param {*} objectValue The destination object property value.
+ * @param {*} sourceValue The source object property value.
+ * @returns {*} Returns the value to assign to the destination object.
+ */
+ function assignDefaults(objectValue, sourceValue) {
+ return objectValue === undefined ? sourceValue : objectValue;
+ }
+
+ /**
+ * Used by `_.template` to customize its `_.assign` use.
+ *
+ * **Note:** This function is like `assignDefaults` except that it ignores
+ * inherited property values when checking if a property is `undefined`.
+ *
+ * @private
+ * @param {*} objectValue The destination object property value.
+ * @param {*} sourceValue The source object property value.
+ * @param {string} key The key associated with the object and source values.
+ * @param {Object} object The destination object.
+ * @returns {*} Returns the value to assign to the destination object.
+ */
+ function assignOwnDefaults(objectValue, sourceValue, key, object) {
+ return (objectValue === undefined || !hasOwnProperty.call(object, key))
+ ? sourceValue
+ : objectValue;
+ }
+
+ /**
+ * A specialized version of `_.assign` for customizing assigned values without
+ * support for argument juggling, multiple sources, and `this` binding `customizer`
+ * functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ */
+ function assignWith(object, source, customizer) {
+ var index = -1,
+ props = keys(source),
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index],
+ value = object[key],
+ result = customizer(value, source[key], key, object, source);
+
+ if ((result === result ? (result !== value) : (value === value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = result;
+ }
+ }
+ return object;
+ }
+
+ /**
+ * The base implementation of `_.assign` without support for argument juggling,
+ * multiple sources, and `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+ function baseAssign(object, source) {
+ return source == null
+ ? object
+ : baseCopy(source, keys(source), object);
+ }
+
+ /**
+ * The base implementation of `_.at` without support for string collections
+ * and individual key arguments.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {number[]|string[]} props The property names or indexes of elements to pick.
+ * @returns {Array} Returns the new array of picked elements.
+ */
+ function baseAt(collection, props) {
+ var index = -1,
+ isNil = collection == null,
+ isArr = !isNil && isArrayLike(collection),
+ length = isArr ? collection.length : 0,
+ propsLength = props.length,
+ result = Array(propsLength);
+
+ while(++index < propsLength) {
+ var key = props[index];
+ if (isArr) {
+ result[index] = isIndex(key, length) ? collection[key] : undefined;
+ } else {
+ result[index] = isNil ? undefined : collection[key];
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property names to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @returns {Object} Returns `object`.
+ */
+ function baseCopy(source, props, object) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ object[key] = source[key];
+ }
+ return object;
+ }
+
+ /**
+ * The base implementation of `_.callback` which supports specifying the
+ * number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Returns the callback.
+ */
+ function baseCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (type == 'function') {
+ return thisArg === undefined
+ ? func
+ : bindCallback(func, thisArg, argCount);
+ }
+ if (func == null) {
+ return identity;
+ }
+ if (type == 'object') {
+ return baseMatches(func);
+ }
+ return thisArg === undefined
+ ? property(func)
+ : baseMatchesProperty(func, thisArg);
+ }
+
+ /**
+ * The base implementation of `_.clone` without support for argument juggling
+ * and `this` binding `customizer` functions.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @param {Function} [customizer] The function to customize cloning values.
+ * @param {string} [key] The key of `value`.
+ * @param {Object} [object] The object `value` belongs to.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates clones with source counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+ function baseClone(value, isDeep, customizer, key, object, stackA, stackB) {
+ var result;
+ if (customizer) {
+ result = object ? customizer(value, key, object) : customizer(value);
+ }
+ if (result !== undefined) {
+ return result;
+ }
+ if (!isObject(value)) {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isArr) {
+ result = initCloneArray(value);
+ if (!isDeep) {
+ return arrayCopy(value, result);
+ }
+ } else {
+ var tag = objToString.call(value),
+ isFunc = tag == funcTag;
+
+ if (tag == objectTag || tag == argsTag || (isFunc && !object)) {
+ result = initCloneObject(isFunc ? {} : value);
+ if (!isDeep) {
+ return baseAssign(result, value);
+ }
+ } else {
+ return cloneableTags[tag]
+ ? initCloneByTag(value, tag, isDeep)
+ : (object ? value : {});
+ }
+ }
+ // Check for circular references and return its corresponding clone.
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == value) {
+ return stackB[length];
+ }
+ }
+ // Add the source value to the stack of traversed objects and associate it with its clone.
+ stackA.push(value);
+ stackB.push(result);
+
+ // Recursively populate clone (susceptible to call stack limits).
+ (isArr ? arrayEach : baseForOwn)(value, function(subValue, key) {
+ result[key] = baseClone(subValue, isDeep, customizer, key, value, stackA, stackB);
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+ var baseCreate = (function() {
+ function object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ object.prototype = prototype;
+ var result = new object;
+ object.prototype = undefined;
+ }
+ return result || {};
+ };
+ }());
+
+ /**
+ * The base implementation of `_.delay` and `_.defer` which accepts an index
+ * of where to slice the arguments to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {Object} args The arguments provide to `func`.
+ * @returns {number} Returns the timer id.
+ */
+ function baseDelay(func, wait, args) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+ }
+
+ /**
+ * The base implementation of `_.difference` which accepts a single array
+ * of values to exclude.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Array} values The values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ */
+ function baseDifference(array, values) {
+ var length = array ? array.length : 0,
+ result = [];
+
+ if (!length) {
+ return result;
+ }
+ var index = -1,
+ indexOf = getIndexOf(),
+ isCommon = indexOf == baseIndexOf,
+ cache = (isCommon && values.length >= LARGE_ARRAY_SIZE) ? createCache(values) : null,
+ valuesLength = values.length;
+
+ if (cache) {
+ indexOf = cacheIndexOf;
+ isCommon = false;
+ values = cache;
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index];
+
+ if (isCommon && value === value) {
+ var valuesIndex = valuesLength;
+ while (valuesIndex--) {
+ if (values[valuesIndex] === value) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ else if (indexOf(values, value, 0) < 0) {
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.forEach` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+ var baseEach = createBaseEach(baseForOwn);
+
+ /**
+ * The base implementation of `_.forEachRight` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+ var baseEachRight = createBaseEach(baseForOwnRight, true);
+
+ /**
+ * The base implementation of `_.every` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+ function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+ }
+
+ /**
+ * Gets the extremum value of `collection` invoking `iteratee` for each value
+ * in `collection` to generate the criterion by which the value is ranked.
+ * The `iteratee` is invoked with three arguments: (value, index|key, collection).
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} comparator The function used to compare values.
+ * @param {*} exValue The initial extremum value.
+ * @returns {*} Returns the extremum value.
+ */
+ function baseExtremum(collection, iteratee, comparator, exValue) {
+ var computed = exValue,
+ result = computed;
+
+ baseEach(collection, function(value, index, collection) {
+ var current = +iteratee(value, index, collection);
+ if (comparator(current, computed) || (current === exValue && current === result)) {
+ computed = current;
+ result = value;
+ }
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.fill` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ */
+ function baseFill(array, value, start, end) {
+ var length = array.length;
+
+ start = start == null ? 0 : (+start || 0);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : (+end || 0);
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : (end >>> 0);
+ start >>>= 0;
+
+ while (start < length) {
+ array[start++] = value;
+ }
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.filter` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+ function baseFilter(collection, predicate) {
+ var result = [];
+ baseEach(collection, function(value, index, collection) {
+ if (predicate(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.find`, `_.findLast`, `_.findKey`, and `_.findLastKey`,
+ * without support for callback shorthands and `this` binding, which iterates
+ * over `collection` using the provided `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @param {boolean} [retKey] Specify returning the key of the found element
+ * instead of the element itself.
+ * @returns {*} Returns the found element or its key, else `undefined`.
+ */
+ function baseFind(collection, predicate, eachFunc, retKey) {
+ var result;
+ eachFunc(collection, function(value, key, collection) {
+ if (predicate(value, key, collection)) {
+ result = retKey ? key : value;
+ return false;
+ }
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.flatten` with added support for restricting
+ * flattening and specifying the start index.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isDeep] Specify a deep flatten.
+ * @param {boolean} [isStrict] Restrict flattening to arrays-like objects.
+ * @param {Array} [result=[]] The initial result value.
+ * @returns {Array} Returns the new flattened array.
+ */
+ function baseFlatten(array, isDeep, isStrict, result) {
+ result || (result = []);
+
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var value = array[index];
+ if (isObjectLike(value) && isArrayLike(value) &&
+ (isStrict || isArray(value) || isArguments(value))) {
+ if (isDeep) {
+ // Recursively flatten arrays (susceptible to call stack limits).
+ baseFlatten(value, isDeep, isStrict, result);
+ } else {
+ arrayPush(result, value);
+ }
+ } else if (!isStrict) {
+ result[result.length] = value;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `baseForIn` and `baseForOwn` which iterates
+ * over `object` properties returned by `keysFunc` invoking `iteratee` for
+ * each property. Iteratee functions may exit iteration early by explicitly
+ * returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+ var baseFor = createBaseFor();
+
+ /**
+ * This function is like `baseFor` except that it iterates over properties
+ * in the opposite order.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+ var baseForRight = createBaseFor(true);
+
+ /**
+ * The base implementation of `_.forIn` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+ function baseForIn(object, iteratee) {
+ return baseFor(object, iteratee, keysIn);
+ }
+
+ /**
+ * The base implementation of `_.forOwn` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+ function baseForOwn(object, iteratee) {
+ return baseFor(object, iteratee, keys);
+ }
+
+ /**
+ * The base implementation of `_.forOwnRight` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+ function baseForOwnRight(object, iteratee) {
+ return baseForRight(object, iteratee, keys);
+ }
+
+ /**
+ * The base implementation of `_.functions` which creates an array of
+ * `object` function property names filtered from those provided.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} props The property names to filter.
+ * @returns {Array} Returns the new array of filtered property names.
+ */
+ function baseFunctions(object, props) {
+ var index = -1,
+ length = props.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var key = props[index];
+ if (isFunction(object[key])) {
+ result[++resIndex] = key;
+ }
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `get` without support for string paths
+ * and default values.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} path The path of the property to get.
+ * @param {string} [pathKey] The key representation of path.
+ * @returns {*} Returns the resolved value.
+ */
+ function baseGet(object, path, pathKey) {
+ if (object == null) {
+ return;
+ }
+ if (pathKey !== undefined && pathKey in toObject(object)) {
+ path = [pathKey];
+ }
+ var index = 0,
+ length = path.length;
+
+ while (object != null && index < length) {
+ object = object[path[index++]];
+ }
+ return (index && index == length) ? object : undefined;
+ }
+
+ /**
+ * The base implementation of `_.isEqual` without support for `this` binding
+ * `customizer` functions.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize comparing values.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+ function baseIsEqual(value, other, customizer, isLoose, stackA, stackB) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, baseIsEqual, customizer, isLoose, stackA, stackB);
+ }
+
+ /**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `value` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function baseIsEqualDeep(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = arrayTag,
+ othTag = arrayTag;
+
+ if (!objIsArr) {
+ objTag = objToString.call(object);
+ if (objTag == argsTag) {
+ objTag = objectTag;
+ } else if (objTag != objectTag) {
+ objIsArr = isTypedArray(object);
+ }
+ }
+ if (!othIsArr) {
+ othTag = objToString.call(other);
+ if (othTag == argsTag) {
+ othTag = objectTag;
+ } else if (othTag != objectTag) {
+ othIsArr = isTypedArray(other);
+ }
+ }
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ if (isSameTag && !(objIsArr || objIsObj)) {
+ return equalByTag(object, other, objTag);
+ }
+ if (!isLoose) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ return equalFunc(objIsWrapped ? object.value() : object, othIsWrapped ? other.value() : other, customizer, isLoose, stackA, stackB);
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ // Assume cyclic values are equal.
+ // For more information on detecting circular references see https://es5.github.io/#JO.
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == object) {
+ return stackB[length] == other;
+ }
+ }
+ // Add `object` and `other` to the stack of traversed objects.
+ stackA.push(object);
+ stackB.push(other);
+
+ var result = (objIsArr ? equalArrays : equalObjects)(object, other, equalFunc, customizer, isLoose, stackA, stackB);
+
+ stackA.pop();
+ stackB.pop();
+
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.isMatch` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} matchData The propery names, values, and compare flags to match.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ */
+ function baseIsMatch(object, matchData, customizer) {
+ var index = matchData.length,
+ length = index,
+ noCustomizer = !customizer;
+
+ if (object == null) {
+ return !length;
+ }
+ object = toObject(object);
+ while (index--) {
+ var data = matchData[index];
+ if ((noCustomizer && data[2])
+ ? data[1] !== object[data[0]]
+ : !(data[0] in object)
+ ) {
+ return false;
+ }
+ }
+ while (++index < length) {
+ data = matchData[index];
+ var key = data[0],
+ objValue = object[key],
+ srcValue = data[1];
+
+ if (noCustomizer && data[2]) {
+ if (objValue === undefined && !(key in object)) {
+ return false;
+ }
+ } else {
+ var result = customizer ? customizer(objValue, srcValue, key) : undefined;
+ if (!(result === undefined ? baseIsEqual(srcValue, objValue, customizer, true) : result)) {
+ return false;
+ }
+ }
+ }
+ return true;
+ }
+
+ /**
+ * The base implementation of `_.map` without support for callback shorthands
+ * and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+ function baseMap(collection, iteratee) {
+ var index = -1,
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value, key, collection) {
+ result[++index] = iteratee(value, key, collection);
+ });
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.matches` which does not clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new function.
+ */
+ function baseMatches(source) {
+ var matchData = getMatchData(source);
+ if (matchData.length == 1 && matchData[0][2]) {
+ var key = matchData[0][0],
+ value = matchData[0][1];
+
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ return object[key] === value && (value !== undefined || (key in toObject(object)));
+ };
+ }
+ return function(object) {
+ return baseIsMatch(object, matchData);
+ };
+ }
+
+ /**
+ * The base implementation of `_.matchesProperty` which does not clone `srcValue`.
+ *
+ * @private
+ * @param {string} path The path of the property to get.
+ * @param {*} srcValue The value to compare.
+ * @returns {Function} Returns the new function.
+ */
+ function baseMatchesProperty(path, srcValue) {
+ var isArr = isArray(path),
+ isCommon = isKey(path) && isStrictComparable(srcValue),
+ pathKey = (path + '');
+
+ path = toPath(path);
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ var key = pathKey;
+ object = toObject(object);
+ if ((isArr || !isCommon) && !(key in object)) {
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ if (object == null) {
+ return false;
+ }
+ key = last(path);
+ object = toObject(object);
+ }
+ return object[key] === srcValue
+ ? (srcValue !== undefined || (key in object))
+ : baseIsEqual(srcValue, object[key], undefined, true);
+ };
+ }
+
+ /**
+ * The base implementation of `_.merge` without support for argument juggling,
+ * multiple sources, and `this` binding `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates values with source counterparts.
+ * @returns {Object} Returns `object`.
+ */
+ function baseMerge(object, source, customizer, stackA, stackB) {
+ if (!isObject(object)) {
+ return object;
+ }
+ var isSrcArr = isArrayLike(source) && (isArray(source) || isTypedArray(source)),
+ props = isSrcArr ? undefined : keys(source);
+
+ arrayEach(props || source, function(srcValue, key) {
+ if (props) {
+ key = srcValue;
+ srcValue = source[key];
+ }
+ if (isObjectLike(srcValue)) {
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+ baseMergeDeep(object, source, key, baseMerge, customizer, stackA, stackB);
+ }
+ else {
+ var value = object[key],
+ result = customizer ? customizer(value, srcValue, key, object, source) : undefined,
+ isCommon = result === undefined;
+
+ if (isCommon) {
+ result = srcValue;
+ }
+ if ((result !== undefined || (isSrcArr && !(key in object))) &&
+ (isCommon || (result === result ? (result !== value) : (value === value)))) {
+ object[key] = result;
+ }
+ }
+ });
+ return object;
+ }
+
+ /**
+ * A specialized version of `baseMerge` for arrays and objects which performs
+ * deep merges and tracks traversed objects enabling objects with circular
+ * references to be merged.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {string} key The key of the value to merge.
+ * @param {Function} mergeFunc The function to merge values.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates values with source counterparts.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+ function baseMergeDeep(object, source, key, mergeFunc, customizer, stackA, stackB) {
+ var length = stackA.length,
+ srcValue = source[key];
+
+ while (length--) {
+ if (stackA[length] == srcValue) {
+ object[key] = stackB[length];
+ return;
+ }
+ }
+ var value = object[key],
+ result = customizer ? customizer(value, srcValue, key, object, source) : undefined,
+ isCommon = result === undefined;
+
+ if (isCommon) {
+ result = srcValue;
+ if (isArrayLike(srcValue) && (isArray(srcValue) || isTypedArray(srcValue))) {
+ result = isArray(value)
+ ? value
+ : (isArrayLike(value) ? arrayCopy(value) : []);
+ }
+ else if (isPlainObject(srcValue) || isArguments(srcValue)) {
+ result = isArguments(value)
+ ? toPlainObject(value)
+ : (isPlainObject(value) ? value : {});
+ }
+ else {
+ isCommon = false;
+ }
+ }
+ // Add the source value to the stack of traversed objects and associate
+ // it with its merged value.
+ stackA.push(srcValue);
+ stackB.push(result);
+
+ if (isCommon) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ object[key] = mergeFunc(result, srcValue, customizer, stackA, stackB);
+ } else if (result === result ? (result !== value) : (value === value)) {
+ object[key] = result;
+ }
+ }
+
+ /**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+ function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+ }
+
+ /**
+ * A specialized version of `baseProperty` which supports deep paths.
+ *
+ * @private
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+ function basePropertyDeep(path) {
+ var pathKey = (path + '');
+ path = toPath(path);
+ return function(object) {
+ return baseGet(object, path, pathKey);
+ };
+ }
+
+ /**
+ * The base implementation of `_.pullAt` without support for individual
+ * index arguments and capturing the removed elements.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {number[]} indexes The indexes of elements to remove.
+ * @returns {Array} Returns `array`.
+ */
+ function basePullAt(array, indexes) {
+ var length = array ? indexes.length : 0;
+ while (length--) {
+ var index = indexes[length];
+ if (index != previous && isIndex(index)) {
+ var previous = index;
+ splice.call(array, index, 1);
+ }
+ }
+ return array;
+ }
+
+ /**
+ * The base implementation of `_.random` without support for argument juggling
+ * and returning floating-point numbers.
+ *
+ * @private
+ * @param {number} min The minimum possible value.
+ * @param {number} max The maximum possible value.
+ * @returns {number} Returns the random number.
+ */
+ function baseRandom(min, max) {
+ return min + nativeFloor(nativeRandom() * (max - min + 1));
+ }
+
+ /**
+ * The base implementation of `_.reduce` and `_.reduceRight` without support
+ * for callback shorthands and `this` binding, which iterates over `collection`
+ * using the provided `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} accumulator The initial value.
+ * @param {boolean} initFromCollection Specify using the first or last element
+ * of `collection` as the initial value.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the accumulated value.
+ */
+ function baseReduce(collection, iteratee, accumulator, initFromCollection, eachFunc) {
+ eachFunc(collection, function(value, index, collection) {
+ accumulator = initFromCollection
+ ? (initFromCollection = false, value)
+ : iteratee(accumulator, value, index, collection);
+ });
+ return accumulator;
+ }
+
+ /**
+ * The base implementation of `setData` without support for hot loop detection.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+ var baseSetData = !metaMap ? identity : function(func, data) {
+ metaMap.set(func, data);
+ return func;
+ };
+
+ /**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+ function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ start = start == null ? 0 : (+start || 0);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : (+end || 0);
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+ }
+
+ /**
+ * The base implementation of `_.some` without support for callback shorthands
+ * and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[57/59] [abbrv] mac commit: updated releasenotes for 4.0.1 release
Posted by st...@apache.org.
updated releasenotes for 4.0.1 release
Project: http://git-wip-us.apache.org/repos/asf/cordova-osx/repo
Commit: http://git-wip-us.apache.org/repos/asf/cordova-osx/commit/1ed8a4ce
Tree: http://git-wip-us.apache.org/repos/asf/cordova-osx/tree/1ed8a4ce
Diff: http://git-wip-us.apache.org/repos/asf/cordova-osx/diff/1ed8a4ce
Branch: refs/heads/4.0.x
Commit: 1ed8a4ce6586241fe18007166316add87a6d591d
Parents: e678ba9
Author: Steve Gill <st...@gmail.com>
Authored: Thu Mar 3 15:42:34 2016 -0800
Committer: Steve Gill <st...@gmail.com>
Committed: Thu Mar 3 15:42:53 2016 -0800
----------------------------------------------------------------------
RELEASENOTES.md | 7 +++++++
1 file changed, 7 insertions(+)
----------------------------------------------------------------------
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/1ed8a4ce/RELEASENOTES.md
----------------------------------------------------------------------
diff --git a/RELEASENOTES.md b/RELEASENOTES.md
index a76cedd..d354f50 100644
--- a/RELEASENOTES.md
+++ b/RELEASENOTES.md
@@ -20,6 +20,13 @@
-->
## Release Notes for Cordova (OS X) ##
+### 4.0.1 (Mar 03, 2016)
+* updated .ratignore
+* CB-10668 added node_modules directory
+* CB-10668 removed bin/node_modules, updated create.js to use root node_modules
+* CB-10668 updated package.json, prepare.js and Api.js
+* CB-10646 Platform specific icons not copied to xcode project
+
### 4.0.0 (Feb 11, 2016)
* CB-10570 Compilation error on case sensitive filesystem
* CB-6789 **OSX** - Fix header licenses (Apache RAT report).
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[32/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/shuffle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/shuffle.js b/node_modules/lodash-node/underscore/collections/shuffle.js
new file mode 100644
index 0000000..526f1ce
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/shuffle.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom'),
+ forEach = require('./forEach');
+
+/**
+ * Creates an array of shuffled values, using a version of the Fisher-Yates
+ * shuffle. See http://en.wikipedia.org/wiki/Fisher-Yates_shuffle.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to shuffle.
+ * @returns {Array} Returns a new shuffled collection.
+ * @example
+ *
+ * _.shuffle([1, 2, 3, 4, 5, 6]);
+ * // => [4, 1, 6, 3, 5, 2]
+ */
+function shuffle(collection) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ var rand = baseRandom(0, ++index);
+ result[index] = result[rand];
+ result[rand] = value;
+ });
+ return result;
+}
+
+module.exports = shuffle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/size.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/size.js b/node_modules/lodash-node/underscore/collections/size.js
new file mode 100644
index 0000000..6ab8211
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/size.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys');
+
+/**
+ * Gets the size of the `collection` by returning `collection.length` for arrays
+ * and array-like objects or the number of own enumerable properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @returns {number} Returns `collection.length` or number of own enumerable properties.
+ * @example
+ *
+ * _.size([1, 2]);
+ * // => 2
+ *
+ * _.size({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => 3
+ *
+ * _.size('pebbles');
+ * // => 7
+ */
+function size(collection) {
+ var length = collection ? collection.length : 0;
+ return typeof length == 'number' ? length : keys(collection).length;
+}
+
+module.exports = size;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/some.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/some.js b/node_modules/lodash-node/underscore/collections/some.js
new file mode 100644
index 0000000..a10d1ba
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/some.js
@@ -0,0 +1,77 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn'),
+ indicatorObject = require('../internals/indicatorObject'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Checks if the callback returns a truey value for **any** element of a
+ * collection. The function returns as soon as it finds a passing value and
+ * does not iterate over the entire collection. The callback is bound to
+ * `thisArg` and invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias any
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if any element passed the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.some(characters, 'blocked');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.some(characters, { 'age': 1 });
+ * // => false
+ */
+function some(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ if ((result = callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ return (result = callback(value, index, collection)) && indicatorObject;
+ });
+ }
+ return !!result;
+}
+
+module.exports = some;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/sortBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/sortBy.js b/node_modules/lodash-node/underscore/collections/sortBy.js
new file mode 100644
index 0000000..2576da8
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/sortBy.js
@@ -0,0 +1,86 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var compareAscending = require('../internals/compareAscending'),
+ createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ isArray = require('../objects/isArray'),
+ map = require('./map');
+
+/**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in a collection through the callback. This method
+ * performs a stable sort, that is, it will preserve the original sort order
+ * of equal elements. The callback is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an array of property names is provided for `callback` the collection
+ * will be sorted by each property value.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Array|Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of sorted elements.
+ * @example
+ *
+ * _.sortBy([1, 2, 3], function(num) { return Math.sin(num); });
+ * // => [3, 1, 2]
+ *
+ * _.sortBy([1, 2, 3], function(num) { return this.sin(num); }, Math);
+ * // => [3, 1, 2]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'barney', 'age': 26 },
+ * { 'name': 'fred', 'age': 30 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(_.sortBy(characters, 'age'), _.values);
+ * // => [['barney', 26], ['fred', 30], ['barney', 36], ['fred', 40]]
+ *
+ * // sorting by multiple properties
+ * _.map(_.sortBy(characters, ['name', 'age']), _.values);
+ * // = > [['barney', 26], ['barney', 36], ['fred', 30], ['fred', 40]]
+ */
+function sortBy(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ callback = createCallback(callback, thisArg, 3);
+ forEach(collection, function(value, key, collection) {
+ result[++index] = {
+ 'criteria': [callback(value, key, collection)],
+ 'index': index,
+ 'value': value
+ };
+ });
+
+ length = result.length;
+ result.sort(compareAscending);
+ while (length--) {
+ result[length] = result[length].value;
+ }
+ return result;
+}
+
+module.exports = sortBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/toArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/toArray.js b/node_modules/lodash-node/underscore/collections/toArray.js
new file mode 100644
index 0000000..825585d
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/toArray.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArray = require('../objects/isArray'),
+ map = require('./map'),
+ slice = require('../internals/slice'),
+ values = require('../objects/values');
+
+/**
+ * Converts the `collection` to an array.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to convert.
+ * @returns {Array} Returns the new converted array.
+ * @example
+ *
+ * (function() { return _.toArray(arguments).slice(1); })(1, 2, 3, 4);
+ * // => [2, 3, 4]
+ */
+function toArray(collection) {
+ if (isArray(collection)) {
+ return slice(collection);
+ }
+ if (collection && typeof collection.length == 'number') {
+ return map(collection);
+ }
+ return values(collection);
+}
+
+module.exports = toArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/collections/where.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/collections/where.js b/node_modules/lodash-node/underscore/collections/where.js
new file mode 100644
index 0000000..20f7931
--- /dev/null
+++ b/node_modules/lodash-node/underscore/collections/where.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var filter = require('./filter'),
+ find = require('./find'),
+ isEmpty = require('../objects/isEmpty');
+
+/**
+ * Performs a deep comparison of each element in a `collection` to the given
+ * `properties` object, returning an array of all elements that have equivalent
+ * property values.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Object} props The object of property values to filter by.
+ * @returns {Array} Returns a new array of elements that have the given properties.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'pets': ['hoppy'] },
+ * { 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * _.where(characters, { 'age': 36 });
+ * // => [{ 'name': 'barney', 'age': 36, 'pets': ['hoppy'] }]
+ *
+ * _.where(characters, { 'pets': ['dino'] });
+ * // => [{ 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }]
+ */
+function where(collection, properties, first) {
+ return (first && isEmpty(properties))
+ ? undefined
+ : (first ? find : filter)(collection, properties);
+}
+
+module.exports = where;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions.js b/node_modules/lodash-node/underscore/functions.js
new file mode 100644
index 0000000..08a3320
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions.js
@@ -0,0 +1,24 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'after': require('./functions/after'),
+ 'bind': require('./functions/bind'),
+ 'bindAll': require('./functions/bindAll'),
+ 'compose': require('./functions/compose'),
+ 'createCallback': require('./functions/createCallback'),
+ 'debounce': require('./functions/debounce'),
+ 'defer': require('./functions/defer'),
+ 'delay': require('./functions/delay'),
+ 'memoize': require('./functions/memoize'),
+ 'once': require('./functions/once'),
+ 'partial': require('./functions/partial'),
+ 'throttle': require('./functions/throttle'),
+ 'wrap': require('./functions/wrap')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/after.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/after.js b/node_modules/lodash-node/underscore/functions/after.js
new file mode 100644
index 0000000..c9ba6e5
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/after.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that executes `func`, with the `this` binding and
+ * arguments of the created function, only after being called `n` times.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {number} n The number of times the function must be called before
+ * `func` is executed.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var saves = ['profile', 'settings'];
+ *
+ * var done = _.after(saves.length, function() {
+ * console.log('Done saving!');
+ * });
+ *
+ * _.forEach(saves, function(type) {
+ * asyncSave({ 'type': type, 'complete': done });
+ * });
+ * // => logs 'Done saving!', after all saves have completed
+ */
+function after(n, func) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+}
+
+module.exports = after;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/bind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/bind.js b/node_modules/lodash-node/underscore/functions/bind.js
new file mode 100644
index 0000000..96e6005
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/bind.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes `func` with the `this`
+ * binding of `thisArg` and prepends any additional `bind` arguments to those
+ * provided to the bound function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to bind.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var func = function(greeting) {
+ * return greeting + ' ' + this.name;
+ * };
+ *
+ * func = _.bind(func, { 'name': 'fred' }, 'hi');
+ * func();
+ * // => 'hi fred'
+ */
+function bind(func, thisArg) {
+ return arguments.length > 2
+ ? createWrapper(func, 17, slice(arguments, 2), null, thisArg)
+ : createWrapper(func, 1, null, null, thisArg);
+}
+
+module.exports = bind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/bindAll.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/bindAll.js b/node_modules/lodash-node/underscore/functions/bindAll.js
new file mode 100644
index 0000000..c06e606
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/bindAll.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ createWrapper = require('../internals/createWrapper'),
+ functions = require('../objects/functions');
+
+/**
+ * Binds methods of an object to the object itself, overwriting the existing
+ * method. Method names may be specified as individual arguments or as arrays
+ * of method names. If no method names are provided all the function properties
+ * of `object` will be bound.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object to bind and assign the bound methods to.
+ * @param {...string} [methodName] The object method names to
+ * bind, specified as individual method names or arrays of method names.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'onClick': function() { console.log('clicked ' + this.label); }
+ * };
+ *
+ * _.bindAll(view);
+ * jQuery('#docs').on('click', view.onClick);
+ * // => logs 'clicked docs', when the button is clicked
+ */
+function bindAll(object) {
+ var funcs = arguments.length > 1 ? baseFlatten(arguments, true, false, 1) : functions(object),
+ index = -1,
+ length = funcs.length;
+
+ while (++index < length) {
+ var key = funcs[index];
+ object[key] = createWrapper(object[key], 1, null, null, object);
+ }
+ return object;
+}
+
+module.exports = bindAll;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/compose.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/compose.js b/node_modules/lodash-node/underscore/functions/compose.js
new file mode 100644
index 0000000..65efb6a
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/compose.js
@@ -0,0 +1,61 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that is the composition of the provided functions,
+ * where each function consumes the return value of the function that follows.
+ * For example, composing the functions `f()`, `g()`, and `h()` produces `f(g(h()))`.
+ * Each function is executed with the `this` binding of the composed function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {...Function} [func] Functions to compose.
+ * @returns {Function} Returns the new composed function.
+ * @example
+ *
+ * var realNameMap = {
+ * 'pebbles': 'penelope'
+ * };
+ *
+ * var format = function(name) {
+ * name = realNameMap[name.toLowerCase()] || name;
+ * return name.charAt(0).toUpperCase() + name.slice(1).toLowerCase();
+ * };
+ *
+ * var greet = function(formatted) {
+ * return 'Hiya ' + formatted + '!';
+ * };
+ *
+ * var welcome = _.compose(greet, format);
+ * welcome('pebbles');
+ * // => 'Hiya Penelope!'
+ */
+function compose() {
+ var funcs = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ if (!isFunction(funcs[length])) {
+ throw new TypeError;
+ }
+ }
+ return function() {
+ var args = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ args = [funcs[length].apply(this, args)];
+ }
+ return args[0];
+ };
+}
+
+module.exports = compose;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/createCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/createCallback.js b/node_modules/lodash-node/underscore/functions/createCallback.js
new file mode 100644
index 0000000..7fb386c
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/createCallback.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ keys = require('../objects/keys'),
+ property = require('../utilities/property');
+
+/**
+ * Produces a callback bound to an optional `thisArg`. If `func` is a property
+ * name the created callback will return the property value for a given element.
+ * If `func` is an object the created callback will return `true` for elements
+ * that contain the equivalent object properties, otherwise it will return `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} [func=identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of the created callback.
+ * @param {number} [argCount] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // wrap to create custom callback shorthands
+ * _.createCallback = _.wrap(_.createCallback, function(func, callback, thisArg) {
+ * var match = /^(.+?)__([gl]t)(.+)$/.exec(callback);
+ * return !match ? func(callback, thisArg) : function(object) {
+ * return match[2] == 'gt' ? object[match[1]] > match[3] : object[match[1]] < match[3];
+ * };
+ * });
+ *
+ * _.filter(characters, 'age__gt38');
+ * // => [{ 'name': 'fred', 'age': 40 }]
+ */
+function createCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (func == null || type == 'function') {
+ return baseCreateCallback(func, thisArg, argCount);
+ }
+ // handle "_.pluck" style callback shorthands
+ if (type != 'object') {
+ return property(func);
+ }
+ var props = keys(func);
+ return function(object) {
+ var length = props.length,
+ result = false;
+
+ while (length--) {
+ if (!(result = object[props[length]] === func[props[length]])) {
+ break;
+ }
+ }
+ return result;
+ };
+}
+
+module.exports = createCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/debounce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/debounce.js b/node_modules/lodash-node/underscore/functions/debounce.js
new file mode 100644
index 0000000..fb0d645
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/debounce.js
@@ -0,0 +1,156 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject'),
+ now = require('../utilities/now');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that will delay the execution of `func` until after
+ * `wait` milliseconds have elapsed since the last time it was invoked.
+ * Provide an options object to indicate that `func` should be invoked on
+ * the leading and/or trailing edge of the `wait` timeout. Subsequent calls
+ * to the debounced function will return the result of the last `func` call.
+ *
+ * Note: If `leading` and `trailing` options are `true` `func` will be called
+ * on the trailing edge of the timeout only if the the debounced function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to debounce.
+ * @param {number} wait The number of milliseconds to delay.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=false] Specify execution on the leading edge of the timeout.
+ * @param {number} [options.maxWait] The maximum time `func` is allowed to be delayed before it's called.
+ * @param {boolean} [options.trailing=true] Specify execution on the trailing edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * // avoid costly calculations while the window size is in flux
+ * var lazyLayout = _.debounce(calculateLayout, 150);
+ * jQuery(window).on('resize', lazyLayout);
+ *
+ * // execute `sendMail` when the click event is fired, debouncing subsequent calls
+ * jQuery('#postbox').on('click', _.debounce(sendMail, 300, {
+ * 'leading': true,
+ * 'trailing': false
+ * });
+ *
+ * // ensure `batchLog` is executed once after 1 second of debounced calls
+ * var source = new EventSource('/stream');
+ * source.addEventListener('message', _.debounce(batchLog, 250, {
+ * 'maxWait': 1000
+ * }, false);
+ */
+function debounce(func, wait, options) {
+ var args,
+ maxTimeoutId,
+ result,
+ stamp,
+ thisArg,
+ timeoutId,
+ trailingCall,
+ lastCalled = 0,
+ maxWait = false,
+ trailing = true;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ wait = nativeMax(0, wait) || 0;
+ if (options === true) {
+ var leading = true;
+ trailing = false;
+ } else if (isObject(options)) {
+ leading = options.leading;
+ maxWait = 'maxWait' in options && (nativeMax(wait, options.maxWait) || 0);
+ trailing = 'trailing' in options ? options.trailing : trailing;
+ }
+ var delayed = function() {
+ var remaining = wait - (now() - stamp);
+ if (remaining <= 0) {
+ if (maxTimeoutId) {
+ clearTimeout(maxTimeoutId);
+ }
+ var isCalled = trailingCall;
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (isCalled) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ }
+ } else {
+ timeoutId = setTimeout(delayed, remaining);
+ }
+ };
+
+ var maxDelayed = function() {
+ if (timeoutId) {
+ clearTimeout(timeoutId);
+ }
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (trailing || (maxWait !== wait)) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ }
+ };
+
+ return function() {
+ args = arguments;
+ stamp = now();
+ thisArg = this;
+ trailingCall = trailing && (timeoutId || !leading);
+
+ if (maxWait === false) {
+ var leadingCall = leading && !timeoutId;
+ } else {
+ if (!maxTimeoutId && !leading) {
+ lastCalled = stamp;
+ }
+ var remaining = maxWait - (stamp - lastCalled),
+ isCalled = remaining <= 0;
+
+ if (isCalled) {
+ if (maxTimeoutId) {
+ maxTimeoutId = clearTimeout(maxTimeoutId);
+ }
+ lastCalled = stamp;
+ result = func.apply(thisArg, args);
+ }
+ else if (!maxTimeoutId) {
+ maxTimeoutId = setTimeout(maxDelayed, remaining);
+ }
+ }
+ if (isCalled && timeoutId) {
+ timeoutId = clearTimeout(timeoutId);
+ }
+ else if (!timeoutId && wait !== maxWait) {
+ timeoutId = setTimeout(delayed, wait);
+ }
+ if (leadingCall) {
+ isCalled = true;
+ result = func.apply(thisArg, args);
+ }
+ if (isCalled && !timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ return result;
+ };
+}
+
+module.exports = debounce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/defer.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/defer.js b/node_modules/lodash-node/underscore/functions/defer.js
new file mode 100644
index 0000000..3dc5873
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/defer.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ slice = require('../internals/slice');
+
+/**
+ * Defers executing the `func` function until the current call stack has cleared.
+ * Additional arguments will be provided to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to defer.
+ * @param {...*} [arg] Arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) { console.log(text); }, 'deferred');
+ * // logs 'deferred' after one or more milliseconds
+ */
+function defer(func) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var args = slice(arguments, 1);
+ return setTimeout(function() { func.apply(undefined, args); }, 1);
+}
+
+module.exports = defer;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/delay.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/delay.js b/node_modules/lodash-node/underscore/functions/delay.js
new file mode 100644
index 0000000..23e1560
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/delay.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ slice = require('../internals/slice');
+
+/**
+ * Executes the `func` function after `wait` milliseconds. Additional arguments
+ * will be provided to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay execution.
+ * @param {...*} [arg] Arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) { console.log(text); }, 1000, 'later');
+ * // => logs 'later' after one second
+ */
+function delay(func, wait) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var args = slice(arguments, 2);
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+}
+
+module.exports = delay;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/memoize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/memoize.js b/node_modules/lodash-node/underscore/functions/memoize.js
new file mode 100644
index 0000000..3f3a7e4
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/memoize.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ keyPrefix = require('../internals/keyPrefix');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates a function that memoizes the result of `func`. If `resolver` is
+ * provided it will be used to determine the cache key for storing the result
+ * based on the arguments provided to the memoized function. By default, the
+ * first argument provided to the memoized function is used as the cache key.
+ * The `func` is executed with the `this` binding of the memoized function.
+ * The result cache is exposed as the `cache` property on the memoized function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to have its output memoized.
+ * @param {Function} [resolver] A function used to resolve the cache key.
+ * @returns {Function} Returns the new memoizing function.
+ * @example
+ *
+ * var fibonacci = _.memoize(function(n) {
+ * return n < 2 ? n : fibonacci(n - 1) + fibonacci(n - 2);
+ * });
+ *
+ * fibonacci(9)
+ * // => 34
+ *
+ * var data = {
+ * 'fred': { 'name': 'fred', 'age': 40 },
+ * 'pebbles': { 'name': 'pebbles', 'age': 1 }
+ * };
+ *
+ * // modifying the result cache
+ * var get = _.memoize(function(name) { return data[name]; }, _.identity);
+ * get('pebbles');
+ * // => { 'name': 'pebbles', 'age': 1 }
+ *
+ * get.cache.pebbles.name = 'penelope';
+ * get('pebbles');
+ * // => { 'name': 'penelope', 'age': 1 }
+ */
+function memoize(func, resolver) {
+ var cache = {};
+ return function() {
+ var key = resolver ? resolver.apply(this, arguments) : keyPrefix + arguments[0];
+ return hasOwnProperty.call(cache, key)
+ ? cache[key]
+ : (cache[key] = func.apply(this, arguments));
+ };
+}
+
+module.exports = memoize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/once.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/once.js b/node_modules/lodash-node/underscore/functions/once.js
new file mode 100644
index 0000000..e5a1a28
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/once.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that is restricted to execute `func` once. Repeat calls to
+ * the function will return the value of the first call. The `func` is executed
+ * with the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // `initialize` executes `createApplication` once
+ */
+function once(func) {
+ var ran,
+ result;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ return function() {
+ if (ran) {
+ return result;
+ }
+ ran = true;
+ result = func.apply(this, arguments);
+
+ // clear the `func` variable so the function may be garbage collected
+ func = null;
+ return result;
+ };
+}
+
+module.exports = once;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/partial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/partial.js b/node_modules/lodash-node/underscore/functions/partial.js
new file mode 100644
index 0000000..a138531
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/partial.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes `func` with any additional
+ * `partial` arguments prepended to those provided to the new function. This
+ * method is similar to `_.bind` except it does **not** alter the `this` binding.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var greet = function(greeting, name) { return greeting + ' ' + name; };
+ * var hi = _.partial(greet, 'hi');
+ * hi('fred');
+ * // => 'hi fred'
+ */
+function partial(func) {
+ return createWrapper(func, 16, slice(arguments, 1));
+}
+
+module.exports = partial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/throttle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/throttle.js b/node_modules/lodash-node/underscore/functions/throttle.js
new file mode 100644
index 0000000..19bc529
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/throttle.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var debounce = require('./debounce'),
+ isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject');
+
+/**
+ * Creates a function that, when executed, will only call the `func` function
+ * at most once per every `wait` milliseconds. Provide an options object to
+ * indicate that `func` should be invoked on the leading and/or trailing edge
+ * of the `wait` timeout. Subsequent calls to the throttled function will
+ * return the result of the last `func` call.
+ *
+ * Note: If `leading` and `trailing` options are `true` `func` will be called
+ * on the trailing edge of the timeout only if the the throttled function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to throttle.
+ * @param {number} wait The number of milliseconds to throttle executions to.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=true] Specify execution on the leading edge of the timeout.
+ * @param {boolean} [options.trailing=true] Specify execution on the trailing edge of the timeout.
+ * @returns {Function} Returns the new throttled function.
+ * @example
+ *
+ * // avoid excessively updating the position while scrolling
+ * var throttled = _.throttle(updatePosition, 100);
+ * jQuery(window).on('scroll', throttled);
+ *
+ * // execute `renewToken` when the click event is fired, but not more than once every 5 minutes
+ * jQuery('.interactive').on('click', _.throttle(renewToken, 300000, {
+ * 'trailing': false
+ * }));
+ */
+function throttle(func, wait, options) {
+ var leading = true,
+ trailing = true;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ if (options === false) {
+ leading = false;
+ } else if (isObject(options)) {
+ leading = 'leading' in options ? options.leading : leading;
+ trailing = 'trailing' in options ? options.trailing : trailing;
+ }
+ options = {};
+ options.leading = leading;
+ options.maxWait = wait;
+ options.trailing = trailing;
+
+ return debounce(func, wait, options);
+}
+
+module.exports = throttle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/functions/wrap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/functions/wrap.js b/node_modules/lodash-node/underscore/functions/wrap.js
new file mode 100644
index 0000000..690849f
--- /dev/null
+++ b/node_modules/lodash-node/underscore/functions/wrap.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper');
+
+/**
+ * Creates a function that provides `value` to the wrapper function as its
+ * first argument. Additional arguments provided to the function are appended
+ * to those provided to the wrapper function. The wrapper is executed with
+ * the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {*} value The value to wrap.
+ * @param {Function} wrapper The wrapper function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var p = _.wrap(_.escape, function(func, text) {
+ * return '<p>' + func(text) + '</p>';
+ * });
+ *
+ * p('Fred, Wilma, & Pebbles');
+ * // => '<p>Fred, Wilma, & Pebbles</p>'
+ */
+function wrap(value, wrapper) {
+ return createWrapper(wrapper, 16, [value]);
+}
+
+module.exports = wrap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/index.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/index.js b/node_modules/lodash-node/underscore/index.js
new file mode 100644
index 0000000..ccbc67e
--- /dev/null
+++ b/node_modules/lodash-node/underscore/index.js
@@ -0,0 +1,284 @@
+/**
+ * @license
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrays = require('./arrays'),
+ chaining = require('./chaining'),
+ collections = require('./collections'),
+ functions = require('./functions'),
+ objects = require('./objects'),
+ utilities = require('./utilities'),
+ forEach = require('./collections/forEach'),
+ forOwn = require('./objects/forOwn'),
+ lodashWrapper = require('./internals/lodashWrapper'),
+ mixin = require('./utilities/mixin'),
+ support = require('./support'),
+ templateSettings = require('./utilities/templateSettings');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/**
+ * Creates a `lodash` object which wraps the given value to enable intuitive
+ * method chaining.
+ *
+ * In addition to Lo-Dash methods, wrappers also have the following `Array` methods:
+ * `concat`, `join`, `pop`, `push`, `reverse`, `shift`, `slice`, `sort`, `splice`,
+ * and `unshift`
+ *
+ * Chaining is supported in custom builds as long as the `value` method is
+ * implicitly or explicitly included in the build.
+ *
+ * The chainable wrapper functions are:
+ * `after`, `assign`, `bind`, `bindAll`, `bindKey`, `chain`, `compact`,
+ * `compose`, `concat`, `countBy`, `create`, `createCallback`, `curry`,
+ * `debounce`, `defaults`, `defer`, `delay`, `difference`, `filter`, `flatten`,
+ * `forEach`, `forEachRight`, `forIn`, `forInRight`, `forOwn`, `forOwnRight`,
+ * `functions`, `groupBy`, `indexBy`, `initial`, `intersection`, `invert`,
+ * `invoke`, `keys`, `map`, `max`, `memoize`, `merge`, `min`, `object`, `omit`,
+ * `once`, `pairs`, `partial`, `partialRight`, `pick`, `pluck`, `pull`, `push`,
+ * `range`, `reject`, `remove`, `rest`, `reverse`, `shuffle`, `slice`, `sort`,
+ * `sortBy`, `splice`, `tap`, `throttle`, `times`, `toArray`, `transform`,
+ * `union`, `uniq`, `unshift`, `unzip`, `values`, `where`, `without`, `wrap`,
+ * and `zip`
+ *
+ * The non-chainable wrapper functions are:
+ * `clone`, `cloneDeep`, `contains`, `escape`, `every`, `find`, `findIndex`,
+ * `findKey`, `findLast`, `findLastIndex`, `findLastKey`, `has`, `identity`,
+ * `indexOf`, `isArguments`, `isArray`, `isBoolean`, `isDate`, `isElement`,
+ * `isEmpty`, `isEqual`, `isFinite`, `isFunction`, `isNaN`, `isNull`, `isNumber`,
+ * `isObject`, `isPlainObject`, `isRegExp`, `isString`, `isUndefined`, `join`,
+ * `lastIndexOf`, `mixin`, `noConflict`, `parseInt`, `pop`, `random`, `reduce`,
+ * `reduceRight`, `result`, `shift`, `size`, `some`, `sortedIndex`, `runInContext`,
+ * `template`, `unescape`, `uniqueId`, and `value`
+ *
+ * The wrapper functions `first` and `last` return wrapped values when `n` is
+ * provided, otherwise they return unwrapped values.
+ *
+ * Explicit chaining can be enabled by using the `_.chain` method.
+ *
+ * @name _
+ * @constructor
+ * @category Chaining
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns a `lodash` instance.
+ * @example
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // returns an unwrapped value
+ * wrapped.reduce(function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * // returns a wrapped value
+ * var squares = wrapped.map(function(num) {
+ * return num * num;
+ * });
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+function lodash(value) {
+ return (value instanceof lodash)
+ ? value
+ : new lodashWrapper(value);
+}
+// ensure `new lodashWrapper` is an instance of `lodash`
+lodashWrapper.prototype = lodash.prototype;
+
+// wrap `_.mixin` so it works when provided only one argument
+mixin = (function(fn) {
+ return function(object, source) {
+ if (!source) {
+ source = object;
+ object = lodash;
+ }
+ return fn(object, source);
+ };
+}(mixin));
+
+// add functions that return wrapped values when chaining
+lodash.after = functions.after;
+lodash.bind = functions.bind;
+lodash.bindAll = functions.bindAll;
+lodash.chain = chaining.chain;
+lodash.compact = arrays.compact;
+lodash.compose = functions.compose;
+lodash.countBy = collections.countBy;
+lodash.debounce = functions.debounce;
+lodash.defaults = objects.defaults;
+lodash.defer = functions.defer;
+lodash.delay = functions.delay;
+lodash.difference = arrays.difference;
+lodash.filter = collections.filter;
+lodash.flatten = arrays.flatten;
+lodash.forEach = forEach;
+lodash.functions = objects.functions;
+lodash.groupBy = collections.groupBy;
+lodash.indexBy = collections.indexBy;
+lodash.initial = arrays.initial;
+lodash.intersection = arrays.intersection;
+lodash.invert = objects.invert;
+lodash.invoke = collections.invoke;
+lodash.keys = objects.keys;
+lodash.map = collections.map;
+lodash.max = collections.max;
+lodash.memoize = functions.memoize;
+lodash.min = collections.min;
+lodash.omit = objects.omit;
+lodash.once = functions.once;
+lodash.pairs = objects.pairs;
+lodash.partial = functions.partial;
+lodash.pick = objects.pick;
+lodash.pluck = collections.pluck;
+lodash.range = arrays.range;
+lodash.reject = collections.reject;
+lodash.rest = arrays.rest;
+lodash.shuffle = collections.shuffle;
+lodash.sortBy = collections.sortBy;
+lodash.tap = chaining.tap;
+lodash.throttle = functions.throttle;
+lodash.times = utilities.times;
+lodash.toArray = collections.toArray;
+lodash.union = arrays.union;
+lodash.uniq = arrays.uniq;
+lodash.values = objects.values;
+lodash.where = collections.where;
+lodash.without = arrays.without;
+lodash.wrap = functions.wrap;
+lodash.zip = arrays.zip;
+
+// add aliases
+lodash.collect = collections.map;
+lodash.drop = arrays.rest;
+lodash.each = forEach;
+lodash.extend = objects.assign;
+lodash.methods = objects.functions;
+lodash.object = arrays.zipObject;
+lodash.select = collections.filter;
+lodash.tail = arrays.rest;
+lodash.unique = arrays.uniq;
+
+// add functions that return unwrapped values when chaining
+lodash.clone = objects.clone;
+lodash.contains = collections.contains;
+lodash.escape = utilities.escape;
+lodash.every = collections.every;
+lodash.find = collections.find;
+lodash.has = objects.has;
+lodash.identity = utilities.identity;
+lodash.indexOf = arrays.indexOf;
+lodash.isArguments = objects.isArguments;
+lodash.isArray = objects.isArray;
+lodash.isBoolean = objects.isBoolean;
+lodash.isDate = objects.isDate;
+lodash.isElement = objects.isElement;
+lodash.isEmpty = objects.isEmpty;
+lodash.isEqual = objects.isEqual;
+lodash.isFinite = objects.isFinite;
+lodash.isFunction = objects.isFunction;
+lodash.isNaN = objects.isNaN;
+lodash.isNull = objects.isNull;
+lodash.isNumber = objects.isNumber;
+lodash.isObject = objects.isObject;
+lodash.isRegExp = objects.isRegExp;
+lodash.isString = objects.isString;
+lodash.isUndefined = objects.isUndefined;
+lodash.lastIndexOf = arrays.lastIndexOf;
+lodash.mixin = mixin;
+lodash.noConflict = utilities.noConflict;
+lodash.random = utilities.random;
+lodash.reduce = collections.reduce;
+lodash.reduceRight = collections.reduceRight;
+lodash.result = utilities.result;
+lodash.size = collections.size;
+lodash.some = collections.some;
+lodash.sortedIndex = arrays.sortedIndex;
+lodash.template = utilities.template;
+lodash.unescape = utilities.unescape;
+lodash.uniqueId = utilities.uniqueId;
+
+// add aliases
+lodash.all = collections.every;
+lodash.any = collections.some;
+lodash.detect = collections.find;
+lodash.findWhere = collections.findWhere;
+lodash.foldl = collections.reduce;
+lodash.foldr = collections.reduceRight;
+lodash.include = collections.contains;
+lodash.inject = collections.reduce;
+
+// add functions capable of returning wrapped and unwrapped values when chaining
+lodash.first = arrays.first;
+lodash.last = arrays.last;
+lodash.sample = collections.sample;
+
+// add aliases
+lodash.take = arrays.first;
+lodash.head = arrays.first;
+
+// add functions to `lodash.prototype`
+mixin(lodash);
+
+/**
+ * The semantic version number.
+ *
+ * @static
+ * @memberOf _
+ * @type string
+ */
+lodash.VERSION = '2.4.1';
+
+// add "Chaining" functions to the wrapper
+lodash.prototype.chain = chaining.wrapperChain;
+lodash.prototype.value = chaining.wrapperValueOf;
+
+ // add `Array` mutator functions to the wrapper
+ forEach(['pop', 'push', 'reverse', 'shift', 'sort', 'splice', 'unshift'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ var value = this.__wrapped__;
+ func.apply(value, arguments);
+
+ // avoid array-like object bugs with `Array#shift` and `Array#splice`
+ // in Firefox < 10 and IE < 9
+ if (!support.spliceObjects && value.length === 0) {
+ delete value[0];
+ }
+ return this;
+ };
+ });
+
+ // add `Array` accessor functions to the wrapper
+ forEach(['concat', 'join', 'slice'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ var value = this.__wrapped__,
+ result = func.apply(value, arguments);
+
+ if (this.__chain__) {
+ result = new lodashWrapper(result);
+ result.__chain__ = true;
+ }
+ return result;
+ };
+ });
+
+lodash.support = support;
+(lodash.templateSettings = utilities.templateSettings).imports._ = lodash;
+module.exports = lodash;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseBind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseBind.js b/node_modules/lodash-node/underscore/internals/baseBind.js
new file mode 100644
index 0000000..4ac6245
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseBind.js
@@ -0,0 +1,60 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('./baseCreate'),
+ isObject = require('../objects/isObject'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * The base implementation of `_.bind` that creates the bound function and
+ * sets its meta data.
+ *
+ * @private
+ * @param {Array} bindData The bind data array.
+ * @returns {Function} Returns the new bound function.
+ */
+function baseBind(bindData) {
+ var func = bindData[0],
+ partialArgs = bindData[2],
+ thisArg = bindData[4];
+
+ function bound() {
+ // `Function#bind` spec
+ // http://es5.github.io/#x15.3.4.5
+ if (partialArgs) {
+ // avoid `arguments` object deoptimizations by using `slice` instead
+ // of `Array.prototype.slice.call` and not assigning `arguments` to a
+ // variable as a ternary expression
+ var args = slice(partialArgs);
+ push.apply(args, arguments);
+ }
+ // mimic the constructor's `return` behavior
+ // http://es5.github.io/#x13.2.2
+ if (this instanceof bound) {
+ // ensure `new bound` is an instance of `func`
+ var thisBinding = baseCreate(func.prototype),
+ result = func.apply(thisBinding, args || arguments);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisArg, args || arguments);
+ }
+ return bound;
+}
+
+module.exports = baseBind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseCreate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseCreate.js b/node_modules/lodash-node/underscore/internals/baseCreate.js
new file mode 100644
index 0000000..2724d0b
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseCreate.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./isNative'),
+ isObject = require('../objects/isObject'),
+ noop = require('../utilities/noop');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeCreate = isNative(nativeCreate = Object.create) && nativeCreate;
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+function baseCreate(prototype, properties) {
+ return isObject(prototype) ? nativeCreate(prototype) : {};
+}
+// fallback for browsers without `Object.create`
+if (!nativeCreate) {
+ baseCreate = (function() {
+ function Object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ Object.prototype = prototype;
+ var result = new Object;
+ Object.prototype = null;
+ }
+ return result || global.Object();
+ };
+ }());
+}
+
+module.exports = baseCreate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseCreateCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseCreateCallback.js b/node_modules/lodash-node/underscore/internals/baseCreateCallback.js
new file mode 100644
index 0000000..be9a4a0
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseCreateCallback.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var bind = require('../functions/bind'),
+ identity = require('../utilities/identity');
+
+/**
+ * The base implementation of `_.createCallback` without support for creating
+ * "_.pluck" or "_.where" style callbacks.
+ *
+ * @private
+ * @param {*} [func=identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of the created callback.
+ * @param {number} [argCount] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ */
+function baseCreateCallback(func, thisArg, argCount) {
+ if (typeof func != 'function') {
+ return identity;
+ }
+ // exit early for no `thisArg` or already bound by `Function#bind`
+ if (typeof thisArg == 'undefined' || !('prototype' in func)) {
+ return func;
+ }
+ switch (argCount) {
+ case 1: return function(value) {
+ return func.call(thisArg, value);
+ };
+ case 2: return function(a, b) {
+ return func.call(thisArg, a, b);
+ };
+ case 3: return function(value, index, collection) {
+ return func.call(thisArg, value, index, collection);
+ };
+ case 4: return function(accumulator, value, index, collection) {
+ return func.call(thisArg, accumulator, value, index, collection);
+ };
+ }
+ return bind(func, thisArg);
+}
+
+module.exports = baseCreateCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseCreateWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseCreateWrapper.js b/node_modules/lodash-node/underscore/internals/baseCreateWrapper.js
new file mode 100644
index 0000000..61dd235
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseCreateWrapper.js
@@ -0,0 +1,76 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('./baseCreate'),
+ isObject = require('../objects/isObject'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * The base implementation of `createWrapper` that creates the wrapper and
+ * sets its meta data.
+ *
+ * @private
+ * @param {Array} bindData The bind data array.
+ * @returns {Function} Returns the new function.
+ */
+function baseCreateWrapper(bindData) {
+ var func = bindData[0],
+ bitmask = bindData[1],
+ partialArgs = bindData[2],
+ partialRightArgs = bindData[3],
+ thisArg = bindData[4],
+ arity = bindData[5];
+
+ var isBind = bitmask & 1,
+ isBindKey = bitmask & 2,
+ isCurry = bitmask & 4,
+ isCurryBound = bitmask & 8,
+ key = func;
+
+ function bound() {
+ var thisBinding = isBind ? thisArg : this;
+ if (partialArgs) {
+ var args = slice(partialArgs);
+ push.apply(args, arguments);
+ }
+ if (partialRightArgs || isCurry) {
+ args || (args = slice(arguments));
+ if (partialRightArgs) {
+ push.apply(args, partialRightArgs);
+ }
+ if (isCurry && args.length < arity) {
+ bitmask |= 16 & ~32;
+ return baseCreateWrapper([func, (isCurryBound ? bitmask : bitmask & ~3), args, null, thisArg, arity]);
+ }
+ }
+ args || (args = arguments);
+ if (isBindKey) {
+ func = thisBinding[key];
+ }
+ if (this instanceof bound) {
+ thisBinding = baseCreate(func.prototype);
+ var result = func.apply(thisBinding, args);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisBinding, args);
+ }
+ return bound;
+}
+
+module.exports = baseCreateWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseDifference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseDifference.js b/node_modules/lodash-node/underscore/internals/baseDifference.js
new file mode 100644
index 0000000..d31a28b
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseDifference.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf');
+
+/**
+ * The base implementation of `_.difference` that accepts a single array
+ * of values to exclude.
+ *
+ * @private
+ * @param {Array} array The array to process.
+ * @param {Array} [values] The array of values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ */
+function baseDifference(array, values) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (indexOf(values, value) < 0) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseDifference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseFlatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseFlatten.js b/node_modules/lodash-node/underscore/internals/baseFlatten.js
new file mode 100644
index 0000000..1b73a0b
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseFlatten.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray');
+
+/**
+ * The base implementation of `_.flatten` without support for callback
+ * shorthands or `thisArg` binding.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isShallow=false] A flag to restrict flattening to a single level.
+ * @param {boolean} [isStrict=false] A flag to restrict flattening to arrays and `arguments` objects.
+ * @param {number} [fromIndex=0] The index to start from.
+ * @returns {Array} Returns a new flattened array.
+ */
+function baseFlatten(array, isShallow, isStrict, fromIndex) {
+ var index = (fromIndex || 0) - 1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+
+ if (value && typeof value == 'object' && typeof value.length == 'number'
+ && (isArray(value) || isArguments(value))) {
+ // recursively flatten arrays (susceptible to call stack limits)
+ if (!isShallow) {
+ value = baseFlatten(value, isShallow, isStrict);
+ }
+ var valIndex = -1,
+ valLength = value.length,
+ resIndex = result.length;
+
+ result.length += valLength;
+ while (++valIndex < valLength) {
+ result[resIndex++] = value[valIndex];
+ }
+ } else if (!isStrict) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseFlatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseIndexOf.js b/node_modules/lodash-node/underscore/internals/baseIndexOf.js
new file mode 100644
index 0000000..43841ad
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseIndexOf.js
@@ -0,0 +1,32 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * The base implementation of `_.indexOf` without support for binary searches
+ * or `fromIndex` constraints.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ */
+function baseIndexOf(array, value, fromIndex) {
+ var index = (fromIndex || 0) - 1,
+ length = array ? array.length : 0;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseIsEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseIsEqual.js b/node_modules/lodash-node/underscore/internals/baseIsEqual.js
new file mode 100644
index 0000000..0944c77
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseIsEqual.js
@@ -0,0 +1,149 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('../objects/forIn'),
+ indicatorObject = require('./indicatorObject'),
+ isFunction = require('../objects/isFunction'),
+ objectTypes = require('./objectTypes');
+
+/** `Object#toString` result shortcuts */
+var arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * The base implementation of `_.isEqual`, without support for `thisArg` binding,
+ * that allows partial "_.where" style comparisons.
+ *
+ * @private
+ * @param {*} a The value to compare.
+ * @param {*} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {Function} [isWhere=false] A flag to indicate performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `a` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `b` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(a, b, stackA, stackB) {
+ if (a === b) {
+ return a !== 0 || (1 / a == 1 / b);
+ }
+ var type = typeof a,
+ otherType = typeof b;
+
+ if (a === a &&
+ !(a && objectTypes[type]) &&
+ !(b && objectTypes[otherType])) {
+ return false;
+ }
+ if (a == null || b == null) {
+ return a === b;
+ }
+ var className = toString.call(a),
+ otherClass = toString.call(b);
+
+ if (className != otherClass) {
+ return false;
+ }
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ return +a == +b;
+
+ case numberClass:
+ return a != +a
+ ? b != +b
+ : (a == 0 ? (1 / a == 1 / b) : a == +b);
+
+ case regexpClass:
+ case stringClass:
+ return a == String(b);
+ }
+ var isArr = className == arrayClass;
+ if (!isArr) {
+ var aWrapped = hasOwnProperty.call(a, '__wrapped__'),
+ bWrapped = hasOwnProperty.call(b, '__wrapped__');
+
+ if (aWrapped || bWrapped) {
+ return baseIsEqual(aWrapped ? a.__wrapped__ : a, bWrapped ? b.__wrapped__ : b, stackA, stackB);
+ }
+ if (className != objectClass) {
+ return false;
+ }
+ var ctorA = a.constructor,
+ ctorB = b.constructor;
+
+ if (ctorA != ctorB &&
+ !(isFunction(ctorA) && ctorA instanceof ctorA && isFunction(ctorB) && ctorB instanceof ctorB) &&
+ ('constructor' in a && 'constructor' in b)
+ ) {
+ return false;
+ }
+ }
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == a) {
+ return stackB[length] == b;
+ }
+ }
+ var result = true,
+ size = 0;
+
+ stackA.push(a);
+ stackB.push(b);
+
+ if (isArr) {
+ size = b.length;
+ result = size == a.length;
+
+ if (result) {
+ while (size--) {
+ if (!(result = baseIsEqual(a[size], b[size], stackA, stackB))) {
+ break;
+ }
+ }
+ }
+ }
+ else {
+ forIn(b, function(value, key, b) {
+ if (hasOwnProperty.call(b, key)) {
+ size++;
+ return !(result = hasOwnProperty.call(a, key) && baseIsEqual(a[key], value, stackA, stackB)) && indicatorObject;
+ }
+ });
+
+ if (result) {
+ forIn(a, function(value, key, a) {
+ if (hasOwnProperty.call(a, key)) {
+ return !(result = --size > -1) && indicatorObject;
+ }
+ });
+ }
+ }
+ stackA.pop();
+ stackB.pop();
+ return result;
+}
+
+module.exports = baseIsEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseRandom.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseRandom.js b/node_modules/lodash-node/underscore/internals/baseRandom.js
new file mode 100644
index 0000000..93ed76c
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseRandom.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Native method shortcuts */
+var floor = Math.floor;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeRandom = Math.random;
+
+/**
+ * The base implementation of `_.random` without argument juggling or support
+ * for returning floating-point numbers.
+ *
+ * @private
+ * @param {number} min The minimum possible value.
+ * @param {number} max The maximum possible value.
+ * @returns {number} Returns a random number.
+ */
+function baseRandom(min, max) {
+ return min + floor(nativeRandom() * (max - min + 1));
+}
+
+module.exports = baseRandom;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/baseUniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/baseUniq.js b/node_modules/lodash-node/underscore/internals/baseUniq.js
new file mode 100644
index 0000000..ed0c678
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/baseUniq.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf');
+
+/**
+ * The base implementation of `_.uniq` without support for callback shorthands
+ * or `thisArg` binding.
+ *
+ * @private
+ * @param {Array} array The array to process.
+ * @param {boolean} [isSorted=false] A flag to indicate that `array` is sorted.
+ * @param {Function} [callback] The function called per iteration.
+ * @returns {Array} Returns a duplicate-value-free array.
+ */
+function baseUniq(array, isSorted, callback) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ result = [],
+ seen = callback ? [] : result;
+
+ while (++index < length) {
+ var value = array[index],
+ computed = callback ? callback(value, index, array) : value;
+
+ if (isSorted
+ ? !index || seen[seen.length - 1] !== computed
+ : indexOf(seen, computed) < 0
+ ) {
+ if (callback) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseUniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/compareAscending.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/compareAscending.js b/node_modules/lodash-node/underscore/internals/compareAscending.js
new file mode 100644
index 0000000..b2d7af2
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/compareAscending.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used by `sortBy` to compare transformed `collection` elements, stable sorting
+ * them in ascending order.
+ *
+ * @private
+ * @param {Object} a The object to compare to `b`.
+ * @param {Object} b The object to compare to `a`.
+ * @returns {number} Returns the sort order indicator of `1` or `-1`.
+ */
+function compareAscending(a, b) {
+ var ac = a.criteria,
+ bc = b.criteria,
+ index = -1,
+ length = ac.length;
+
+ while (++index < length) {
+ var value = ac[index],
+ other = bc[index];
+
+ if (value !== other) {
+ if (value > other || typeof value == 'undefined') {
+ return 1;
+ }
+ if (value < other || typeof other == 'undefined') {
+ return -1;
+ }
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to return the same value for
+ // `a` and `b`. See https://github.com/jashkenas/underscore/pull/1247
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See http://code.google.com/p/v8/issues/detail?id=90
+ return a.index - b.index;
+}
+
+module.exports = compareAscending;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/createAggregator.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/createAggregator.js b/node_modules/lodash-node/underscore/internals/createAggregator.js
new file mode 100644
index 0000000..72206c5
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/createAggregator.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates a function that aggregates a collection, creating an object composed
+ * of keys generated from the results of running each element of the collection
+ * through a callback. The given `setter` function sets the keys and values
+ * of the composed object.
+ *
+ * @private
+ * @param {Function} setter The setter function.
+ * @returns {Function} Returns the new aggregator function.
+ */
+function createAggregator(setter) {
+ return function(collection, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ setter(result, value, callback(value, index, collection), collection);
+ }
+ } else {
+ forOwn(collection, function(value, key, collection) {
+ setter(result, value, callback(value, key, collection), collection);
+ });
+ }
+ return result;
+ };
+}
+
+module.exports = createAggregator;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/createWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/createWrapper.js b/node_modules/lodash-node/underscore/internals/createWrapper.js
new file mode 100644
index 0000000..82d8b00
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/createWrapper.js
@@ -0,0 +1,60 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseBind = require('./baseBind'),
+ baseCreateWrapper = require('./baseCreateWrapper'),
+ isFunction = require('../objects/isFunction'),
+ slice = require('./slice');
+
+/**
+ * Creates a function that, when called, either curries or invokes `func`
+ * with an optional `this` binding and partially applied arguments.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to reference.
+ * @param {number} bitmask The bitmask of method flags to compose.
+ * The bitmask may be composed of the following flags:
+ * 1 - `_.bind`
+ * 2 - `_.bindKey`
+ * 4 - `_.curry`
+ * 8 - `_.curry` (bound)
+ * 16 - `_.partial`
+ * 32 - `_.partialRight`
+ * @param {Array} [partialArgs] An array of arguments to prepend to those
+ * provided to the new function.
+ * @param {Array} [partialRightArgs] An array of arguments to append to those
+ * provided to the new function.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new function.
+ */
+function createWrapper(func, bitmask, partialArgs, partialRightArgs, thisArg, arity) {
+ var isBind = bitmask & 1,
+ isBindKey = bitmask & 2,
+ isCurry = bitmask & 4,
+ isCurryBound = bitmask & 8,
+ isPartial = bitmask & 16,
+ isPartialRight = bitmask & 32;
+
+ if (!isBindKey && !isFunction(func)) {
+ throw new TypeError;
+ }
+ if (isPartial && !partialArgs.length) {
+ bitmask &= ~16;
+ isPartial = partialArgs = false;
+ }
+ if (isPartialRight && !partialRightArgs.length) {
+ bitmask &= ~32;
+ isPartialRight = partialRightArgs = false;
+ }
+ // fast path for `_.bind`
+ var creater = (bitmask == 1 || bitmask === 17) ? baseBind : baseCreateWrapper;
+ return creater([func, bitmask, partialArgs, partialRightArgs, thisArg, arity]);
+}
+
+module.exports = createWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/escapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/escapeHtmlChar.js b/node_modules/lodash-node/underscore/internals/escapeHtmlChar.js
new file mode 100644
index 0000000..f1495bf
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/escapeHtmlChar.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes');
+
+/**
+ * Used by `escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} match The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeHtmlChar(match) {
+ return htmlEscapes[match];
+}
+
+module.exports = escapeHtmlChar;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[35/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/createWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/createWrapper.js b/node_modules/lodash-node/modern/internals/createWrapper.js
new file mode 100644
index 0000000..c39d340
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/createWrapper.js
@@ -0,0 +1,106 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseBind = require('./baseBind'),
+ baseCreateWrapper = require('./baseCreateWrapper'),
+ isFunction = require('../objects/isFunction'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push,
+ unshift = arrayRef.unshift;
+
+/**
+ * Creates a function that, when called, either curries or invokes `func`
+ * with an optional `this` binding and partially applied arguments.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to reference.
+ * @param {number} bitmask The bitmask of method flags to compose.
+ * The bitmask may be composed of the following flags:
+ * 1 - `_.bind`
+ * 2 - `_.bindKey`
+ * 4 - `_.curry`
+ * 8 - `_.curry` (bound)
+ * 16 - `_.partial`
+ * 32 - `_.partialRight`
+ * @param {Array} [partialArgs] An array of arguments to prepend to those
+ * provided to the new function.
+ * @param {Array} [partialRightArgs] An array of arguments to append to those
+ * provided to the new function.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new function.
+ */
+function createWrapper(func, bitmask, partialArgs, partialRightArgs, thisArg, arity) {
+ var isBind = bitmask & 1,
+ isBindKey = bitmask & 2,
+ isCurry = bitmask & 4,
+ isCurryBound = bitmask & 8,
+ isPartial = bitmask & 16,
+ isPartialRight = bitmask & 32;
+
+ if (!isBindKey && !isFunction(func)) {
+ throw new TypeError;
+ }
+ if (isPartial && !partialArgs.length) {
+ bitmask &= ~16;
+ isPartial = partialArgs = false;
+ }
+ if (isPartialRight && !partialRightArgs.length) {
+ bitmask &= ~32;
+ isPartialRight = partialRightArgs = false;
+ }
+ var bindData = func && func.__bindData__;
+ if (bindData && bindData !== true) {
+ // clone `bindData`
+ bindData = slice(bindData);
+ if (bindData[2]) {
+ bindData[2] = slice(bindData[2]);
+ }
+ if (bindData[3]) {
+ bindData[3] = slice(bindData[3]);
+ }
+ // set `thisBinding` is not previously bound
+ if (isBind && !(bindData[1] & 1)) {
+ bindData[4] = thisArg;
+ }
+ // set if previously bound but not currently (subsequent curried functions)
+ if (!isBind && bindData[1] & 1) {
+ bitmask |= 8;
+ }
+ // set curried arity if not yet set
+ if (isCurry && !(bindData[1] & 4)) {
+ bindData[5] = arity;
+ }
+ // append partial left arguments
+ if (isPartial) {
+ push.apply(bindData[2] || (bindData[2] = []), partialArgs);
+ }
+ // append partial right arguments
+ if (isPartialRight) {
+ unshift.apply(bindData[3] || (bindData[3] = []), partialRightArgs);
+ }
+ // merge flags
+ bindData[1] |= bitmask;
+ return createWrapper.apply(null, bindData);
+ }
+ // fast path for `_.bind`
+ var creater = (bitmask == 1 || bitmask === 17) ? baseBind : baseCreateWrapper;
+ return creater([func, bitmask, partialArgs, partialRightArgs, thisArg, arity]);
+}
+
+module.exports = createWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/escapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/escapeHtmlChar.js b/node_modules/lodash-node/modern/internals/escapeHtmlChar.js
new file mode 100644
index 0000000..1d7b6f1
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/escapeHtmlChar.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes');
+
+/**
+ * Used by `escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} match The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeHtmlChar(match) {
+ return htmlEscapes[match];
+}
+
+module.exports = escapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/escapeStringChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/escapeStringChar.js b/node_modules/lodash-node/modern/internals/escapeStringChar.js
new file mode 100644
index 0000000..9cf267f
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/escapeStringChar.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to escape characters for inclusion in compiled string literals */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\t': 't',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `template` to escape characters for inclusion in compiled
+ * string literals.
+ *
+ * @private
+ * @param {string} match The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeStringChar(match) {
+ return '\\' + stringEscapes[match];
+}
+
+module.exports = escapeStringChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/getArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/getArray.js b/node_modules/lodash-node/modern/internals/getArray.js
new file mode 100644
index 0000000..50fbc2f
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/getArray.js
@@ -0,0 +1,21 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrayPool = require('./arrayPool');
+
+/**
+ * Gets an array from the array pool or creates a new one if the pool is empty.
+ *
+ * @private
+ * @returns {Array} The array from the pool.
+ */
+function getArray() {
+ return arrayPool.pop() || [];
+}
+
+module.exports = getArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/getObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/getObject.js b/node_modules/lodash-node/modern/internals/getObject.js
new file mode 100644
index 0000000..ce1ee73
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/getObject.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectPool = require('./objectPool');
+
+/**
+ * Gets an object from the object pool or creates a new one if the pool is empty.
+ *
+ * @private
+ * @returns {Object} The object from the pool.
+ */
+function getObject() {
+ return objectPool.pop() || {
+ 'array': null,
+ 'cache': null,
+ 'criteria': null,
+ 'false': false,
+ 'index': 0,
+ 'null': false,
+ 'number': null,
+ 'object': null,
+ 'push': null,
+ 'string': null,
+ 'true': false,
+ 'undefined': false,
+ 'value': null
+ };
+}
+
+module.exports = getObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/htmlEscapes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/htmlEscapes.js b/node_modules/lodash-node/modern/internals/htmlEscapes.js
new file mode 100644
index 0000000..9933d60
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/htmlEscapes.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used to convert characters to HTML entities:
+ *
+ * Though the `>` character is escaped for symmetry, characters like `>` and `/`
+ * don't require escaping in HTML and have no special meaning unless they're part
+ * of a tag or an unquoted attribute value.
+ * http://mathiasbynens.be/notes/ambiguous-ampersands (under "semi-related fun fact")
+ */
+var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+};
+
+module.exports = htmlEscapes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/htmlUnescapes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/htmlUnescapes.js b/node_modules/lodash-node/modern/internals/htmlUnescapes.js
new file mode 100644
index 0000000..eeaaa1f
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/htmlUnescapes.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes'),
+ invert = require('../objects/invert');
+
+/** Used to convert HTML entities to characters */
+var htmlUnescapes = invert(htmlEscapes);
+
+module.exports = htmlUnescapes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/isNative.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/isNative.js b/node_modules/lodash-node/modern/internals/isNative.js
new file mode 100644
index 0000000..4053eed
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/isNative.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Used to detect if a method is native */
+var reNative = RegExp('^' +
+ String(toString)
+ .replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
+ .replace(/toString| for [^\]]+/g, '.*?') + '$'
+);
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a native function, else `false`.
+ */
+function isNative(value) {
+ return typeof value == 'function' && reNative.test(value);
+}
+
+module.exports = isNative;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/keyPrefix.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/keyPrefix.js b/node_modules/lodash-node/modern/internals/keyPrefix.js
new file mode 100644
index 0000000..a23cf32
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/keyPrefix.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to prefix keys to avoid issues with `__proto__` and properties on `Object.prototype` */
+var keyPrefix = +new Date + '';
+
+module.exports = keyPrefix;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/largeArraySize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/largeArraySize.js b/node_modules/lodash-node/modern/internals/largeArraySize.js
new file mode 100644
index 0000000..c876188
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/largeArraySize.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used as the size when optimizations are enabled for large arrays */
+var largeArraySize = 75;
+
+module.exports = largeArraySize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/lodashWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/lodashWrapper.js b/node_modules/lodash-node/modern/internals/lodashWrapper.js
new file mode 100644
index 0000000..a820145
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/lodashWrapper.js
@@ -0,0 +1,23 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * A fast path for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @param {boolean} chainAll A flag to enable chaining for all methods
+ * @returns {Object} Returns a `lodash` instance.
+ */
+function lodashWrapper(value, chainAll) {
+ this.__chain__ = !!chainAll;
+ this.__wrapped__ = value;
+}
+
+module.exports = lodashWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/maxPoolSize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/maxPoolSize.js b/node_modules/lodash-node/modern/internals/maxPoolSize.js
new file mode 100644
index 0000000..86dafab
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/maxPoolSize.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used as the max size of the `arrayPool` and `objectPool` */
+var maxPoolSize = 40;
+
+module.exports = maxPoolSize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/objectPool.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/objectPool.js b/node_modules/lodash-node/modern/internals/objectPool.js
new file mode 100644
index 0000000..fea0bf0
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/objectPool.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to pool arrays and objects used internally */
+var objectPool = [];
+
+module.exports = objectPool;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/objectTypes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/objectTypes.js b/node_modules/lodash-node/modern/internals/objectTypes.js
new file mode 100644
index 0000000..a100109
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/objectTypes.js
@@ -0,0 +1,20 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to determine if values are of the language type Object */
+var objectTypes = {
+ 'boolean': false,
+ 'function': true,
+ 'object': true,
+ 'number': false,
+ 'string': false,
+ 'undefined': false
+};
+
+module.exports = objectTypes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/reEscapedHtml.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/reEscapedHtml.js b/node_modules/lodash-node/modern/internals/reEscapedHtml.js
new file mode 100644
index 0000000..a52d67d
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/reEscapedHtml.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlUnescapes = require('./htmlUnescapes'),
+ keys = require('../objects/keys');
+
+/** Used to match HTML entities and HTML characters */
+var reEscapedHtml = RegExp('(' + keys(htmlUnescapes).join('|') + ')', 'g');
+
+module.exports = reEscapedHtml;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/reInterpolate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/reInterpolate.js b/node_modules/lodash-node/modern/internals/reInterpolate.js
new file mode 100644
index 0000000..bb4b865
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/reInterpolate.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to match "interpolate" template delimiters */
+var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+module.exports = reInterpolate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/reUnescapedHtml.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/reUnescapedHtml.js b/node_modules/lodash-node/modern/internals/reUnescapedHtml.js
new file mode 100644
index 0000000..75f6895
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/reUnescapedHtml.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes'),
+ keys = require('../objects/keys');
+
+/** Used to match HTML entities and HTML characters */
+var reUnescapedHtml = RegExp('[' + keys(htmlEscapes).join('') + ']', 'g');
+
+module.exports = reUnescapedHtml;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/releaseArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/releaseArray.js b/node_modules/lodash-node/modern/internals/releaseArray.js
new file mode 100644
index 0000000..5a75e51
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/releaseArray.js
@@ -0,0 +1,25 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrayPool = require('./arrayPool'),
+ maxPoolSize = require('./maxPoolSize');
+
+/**
+ * Releases the given array back to the array pool.
+ *
+ * @private
+ * @param {Array} [array] The array to release.
+ */
+function releaseArray(array) {
+ array.length = 0;
+ if (arrayPool.length < maxPoolSize) {
+ arrayPool.push(array);
+ }
+}
+
+module.exports = releaseArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/releaseObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/releaseObject.js b/node_modules/lodash-node/modern/internals/releaseObject.js
new file mode 100644
index 0000000..d2b9594
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/releaseObject.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var maxPoolSize = require('./maxPoolSize'),
+ objectPool = require('./objectPool');
+
+/**
+ * Releases the given object back to the object pool.
+ *
+ * @private
+ * @param {Object} [object] The object to release.
+ */
+function releaseObject(object) {
+ var cache = object.cache;
+ if (cache) {
+ releaseObject(cache);
+ }
+ object.array = object.cache = object.criteria = object.object = object.number = object.string = object.value = null;
+ if (objectPool.length < maxPoolSize) {
+ objectPool.push(object);
+ }
+}
+
+module.exports = releaseObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/setBindData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/setBindData.js b/node_modules/lodash-node/modern/internals/setBindData.js
new file mode 100644
index 0000000..0f800ee
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/setBindData.js
@@ -0,0 +1,43 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./isNative'),
+ noop = require('../utilities/noop');
+
+/** Used as the property descriptor for `__bindData__` */
+var descriptor = {
+ 'configurable': false,
+ 'enumerable': false,
+ 'value': null,
+ 'writable': false
+};
+
+/** Used to set meta data on functions */
+var defineProperty = (function() {
+ // IE 8 only accepts DOM elements
+ try {
+ var o = {},
+ func = isNative(func = Object.defineProperty) && func,
+ result = func(o, o, o) && func;
+ } catch(e) { }
+ return result;
+}());
+
+/**
+ * Sets `this` binding data on a given function.
+ *
+ * @private
+ * @param {Function} func The function to set data on.
+ * @param {Array} value The data array to set.
+ */
+var setBindData = !defineProperty ? noop : function(func, value) {
+ descriptor.value = value;
+ defineProperty(func, '__bindData__', descriptor);
+};
+
+module.exports = setBindData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/shimIsPlainObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/shimIsPlainObject.js b/node_modules/lodash-node/modern/internals/shimIsPlainObject.js
new file mode 100644
index 0000000..55c9875
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/shimIsPlainObject.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('../objects/forIn'),
+ isFunction = require('../objects/isFunction');
+
+/** `Object#toString` result shortcuts */
+var objectClass = '[object Object]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A fallback implementation of `isPlainObject` which checks if a given value
+ * is an object created by the `Object` constructor, assuming objects created
+ * by the `Object` constructor have no inherited enumerable properties and that
+ * there are no `Object.prototype` extensions.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ */
+function shimIsPlainObject(value) {
+ var ctor,
+ result;
+
+ // avoid non Object objects, `arguments` objects, and DOM elements
+ if (!(value && toString.call(value) == objectClass) ||
+ (ctor = value.constructor, isFunction(ctor) && !(ctor instanceof ctor))) {
+ return false;
+ }
+ // In most environments an object's own properties are iterated before
+ // its inherited properties. If the last iterated property is an object's
+ // own property then there are no inherited enumerable properties.
+ forIn(value, function(value, key) {
+ result = key;
+ });
+ return typeof result == 'undefined' || hasOwnProperty.call(value, result);
+}
+
+module.exports = shimIsPlainObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/shimKeys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/shimKeys.js b/node_modules/lodash-node/modern/internals/shimKeys.js
new file mode 100644
index 0000000..31adba1
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/shimKeys.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('./objectTypes');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A fallback implementation of `Object.keys` which produces an array of the
+ * given object's own enumerable property names.
+ *
+ * @private
+ * @type Function
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names.
+ */
+var shimKeys = function(object) {
+ var index, iterable = object, result = [];
+ if (!iterable) return result;
+ if (!(objectTypes[typeof object])) return result;
+ for (index in iterable) {
+ if (hasOwnProperty.call(iterable, index)) {
+ result.push(index);
+ }
+ }
+ return result
+};
+
+module.exports = shimKeys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/slice.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/slice.js b/node_modules/lodash-node/modern/internals/slice.js
new file mode 100644
index 0000000..ae00390
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/slice.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Slices the `collection` from the `start` index up to, but not including,
+ * the `end` index.
+ *
+ * Note: This function is used instead of `Array#slice` to support node lists
+ * in IE < 9 and to ensure dense arrays are returned.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to slice.
+ * @param {number} start The start index.
+ * @param {number} end The end index.
+ * @returns {Array} Returns the new array.
+ */
+function slice(array, start, end) {
+ start || (start = 0);
+ if (typeof end == 'undefined') {
+ end = array ? array.length : 0;
+ }
+ var index = -1,
+ length = end - start || 0,
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = array[start + index];
+ }
+ return result;
+}
+
+module.exports = slice;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/unescapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/unescapeHtmlChar.js b/node_modules/lodash-node/modern/internals/unescapeHtmlChar.js
new file mode 100644
index 0000000..b209f7a
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/unescapeHtmlChar.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlUnescapes = require('./htmlUnescapes');
+
+/**
+ * Used by `unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} match The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+function unescapeHtmlChar(match) {
+ return htmlUnescapes[match];
+}
+
+module.exports = unescapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects.js b/node_modules/lodash-node/modern/objects.js
new file mode 100644
index 0000000..d600832
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'assign': require('./objects/assign'),
+ 'clone': require('./objects/clone'),
+ 'cloneDeep': require('./objects/cloneDeep'),
+ 'create': require('./objects/create'),
+ 'defaults': require('./objects/defaults'),
+ 'extend': require('./objects/assign'),
+ 'findKey': require('./objects/findKey'),
+ 'findLastKey': require('./objects/findLastKey'),
+ 'forIn': require('./objects/forIn'),
+ 'forInRight': require('./objects/forInRight'),
+ 'forOwn': require('./objects/forOwn'),
+ 'forOwnRight': require('./objects/forOwnRight'),
+ 'functions': require('./objects/functions'),
+ 'has': require('./objects/has'),
+ 'invert': require('./objects/invert'),
+ 'isArguments': require('./objects/isArguments'),
+ 'isArray': require('./objects/isArray'),
+ 'isBoolean': require('./objects/isBoolean'),
+ 'isDate': require('./objects/isDate'),
+ 'isElement': require('./objects/isElement'),
+ 'isEmpty': require('./objects/isEmpty'),
+ 'isEqual': require('./objects/isEqual'),
+ 'isFinite': require('./objects/isFinite'),
+ 'isFunction': require('./objects/isFunction'),
+ 'isNaN': require('./objects/isNaN'),
+ 'isNull': require('./objects/isNull'),
+ 'isNumber': require('./objects/isNumber'),
+ 'isObject': require('./objects/isObject'),
+ 'isPlainObject': require('./objects/isPlainObject'),
+ 'isRegExp': require('./objects/isRegExp'),
+ 'isString': require('./objects/isString'),
+ 'isUndefined': require('./objects/isUndefined'),
+ 'keys': require('./objects/keys'),
+ 'mapValues': require('./objects/mapValues'),
+ 'merge': require('./objects/merge'),
+ 'methods': require('./objects/functions'),
+ 'omit': require('./objects/omit'),
+ 'pairs': require('./objects/pairs'),
+ 'pick': require('./objects/pick'),
+ 'transform': require('./objects/transform'),
+ 'values': require('./objects/values')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/assign.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/assign.js b/node_modules/lodash-node/modern/objects/assign.js
new file mode 100644
index 0000000..36e0690
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/assign.js
@@ -0,0 +1,70 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ keys = require('./keys'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object. Subsequent sources will overwrite property assignments of previous
+ * sources. If a callback is provided it will be executed to produce the
+ * assigned values. The callback is bound to `thisArg` and invoked with two
+ * arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @alias extend
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param {Function} [callback] The function to customize assigning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * _.assign({ 'name': 'fred' }, { 'employer': 'slate' });
+ * // => { 'name': 'fred', 'employer': 'slate' }
+ *
+ * var defaults = _.partialRight(_.assign, function(a, b) {
+ * return typeof a == 'undefined' ? b : a;
+ * });
+ *
+ * var object = { 'name': 'barney' };
+ * defaults(object, { 'name': 'fred', 'employer': 'slate' });
+ * // => { 'name': 'barney', 'employer': 'slate' }
+ */
+var assign = function(object, source, guard) {
+ var index, iterable = object, result = iterable;
+ if (!iterable) return result;
+ var args = arguments,
+ argsIndex = 0,
+ argsLength = typeof guard == 'number' ? 2 : args.length;
+ if (argsLength > 3 && typeof args[argsLength - 2] == 'function') {
+ var callback = baseCreateCallback(args[--argsLength - 1], args[argsLength--], 2);
+ } else if (argsLength > 2 && typeof args[argsLength - 1] == 'function') {
+ callback = args[--argsLength];
+ }
+ while (++argsIndex < argsLength) {
+ iterable = args[argsIndex];
+ if (iterable && objectTypes[typeof iterable]) {
+ var ownIndex = -1,
+ ownProps = objectTypes[typeof iterable] && keys(iterable),
+ length = ownProps ? ownProps.length : 0;
+
+ while (++ownIndex < length) {
+ index = ownProps[ownIndex];
+ result[index] = callback ? callback(result[index], iterable[index]) : iterable[index];
+ }
+ }
+ }
+ return result
+};
+
+module.exports = assign;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/clone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/clone.js b/node_modules/lodash-node/modern/objects/clone.js
new file mode 100644
index 0000000..6932a81
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/clone.js
@@ -0,0 +1,63 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseClone = require('../internals/baseClone'),
+ baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Creates a clone of `value`. If `isDeep` is `true` nested objects will also
+ * be cloned, otherwise they will be assigned by reference. If a callback
+ * is provided it will be executed to produce the cloned values. If the
+ * callback returns `undefined` cloning will be handled by the method instead.
+ * The callback is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep=false] Specify a deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the cloned value.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * var shallow = _.clone(characters);
+ * shallow[0] === characters[0];
+ * // => true
+ *
+ * var deep = _.clone(characters, true);
+ * deep[0] === characters[0];
+ * // => false
+ *
+ * _.mixin({
+ * 'clone': _.partialRight(_.clone, function(value) {
+ * return _.isElement(value) ? value.cloneNode(false) : undefined;
+ * })
+ * });
+ *
+ * var clone = _.clone(document.body);
+ * clone.childNodes.length;
+ * // => 0
+ */
+function clone(value, isDeep, callback, thisArg) {
+ // allows working with "Collections" methods without using their `index`
+ // and `collection` arguments for `isDeep` and `callback`
+ if (typeof isDeep != 'boolean' && isDeep != null) {
+ thisArg = callback;
+ callback = isDeep;
+ isDeep = false;
+ }
+ return baseClone(value, isDeep, typeof callback == 'function' && baseCreateCallback(callback, thisArg, 1));
+}
+
+module.exports = clone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/cloneDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/cloneDeep.js b/node_modules/lodash-node/modern/objects/cloneDeep.js
new file mode 100644
index 0000000..7bd6866
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/cloneDeep.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseClone = require('../internals/baseClone'),
+ baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Creates a deep clone of `value`. If a callback is provided it will be
+ * executed to produce the cloned values. If the callback returns `undefined`
+ * cloning will be handled by the method instead. The callback is bound to
+ * `thisArg` and invoked with one argument; (value).
+ *
+ * Note: This method is loosely based on the structured clone algorithm. Functions
+ * and DOM nodes are **not** cloned. The enumerable properties of `arguments` objects and
+ * objects created by constructors other than `Object` are cloned to plain `Object` objects.
+ * See http://www.w3.org/TR/html5/infrastructure.html#internal-structured-cloning-algorithm.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the deep cloned value.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * var deep = _.cloneDeep(characters);
+ * deep[0] === characters[0];
+ * // => false
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'node': element
+ * };
+ *
+ * var clone = _.cloneDeep(view, function(value) {
+ * return _.isElement(value) ? value.cloneNode(true) : undefined;
+ * });
+ *
+ * clone.node == view.node;
+ * // => false
+ */
+function cloneDeep(value, callback, thisArg) {
+ return baseClone(value, true, typeof callback == 'function' && baseCreateCallback(callback, thisArg, 1));
+}
+
+module.exports = cloneDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/create.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/create.js b/node_modules/lodash-node/modern/objects/create.js
new file mode 100644
index 0000000..fda6a94
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/create.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var assign = require('./assign'),
+ baseCreate = require('../internals/baseCreate');
+
+/**
+ * Creates an object that inherits from the given `prototype` object. If a
+ * `properties` object is provided its own enumerable properties are assigned
+ * to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, { 'constructor': Circle });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+function create(prototype, properties) {
+ var result = baseCreate(prototype);
+ return properties ? assign(result, properties) : result;
+}
+
+module.exports = create;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/defaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/defaults.js b/node_modules/lodash-node/modern/objects/defaults.js
new file mode 100644
index 0000000..6427fdc
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/defaults.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object for all destination properties that resolve to `undefined`. Once a
+ * property is set, additional defaults of the same property will be ignored.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param- {Object} [guard] Allows working with `_.reduce` without using its
+ * `key` and `object` arguments as sources.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var object = { 'name': 'barney' };
+ * _.defaults(object, { 'name': 'fred', 'employer': 'slate' });
+ * // => { 'name': 'barney', 'employer': 'slate' }
+ */
+var defaults = function(object, source, guard) {
+ var index, iterable = object, result = iterable;
+ if (!iterable) return result;
+ var args = arguments,
+ argsIndex = 0,
+ argsLength = typeof guard == 'number' ? 2 : args.length;
+ while (++argsIndex < argsLength) {
+ iterable = args[argsIndex];
+ if (iterable && objectTypes[typeof iterable]) {
+ var ownIndex = -1,
+ ownProps = objectTypes[typeof iterable] && keys(iterable),
+ length = ownProps ? ownProps.length : 0;
+
+ while (++ownIndex < length) {
+ index = ownProps[ownIndex];
+ if (typeof result[index] == 'undefined') result[index] = iterable[index];
+ }
+ }
+ }
+ return result
+};
+
+module.exports = defaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/findKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/findKey.js b/node_modules/lodash-node/modern/objects/findKey.js
new file mode 100644
index 0000000..6713253
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/findKey.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('./forOwn');
+
+/**
+ * This method is like `_.findIndex` except that it returns the key of the
+ * first element that passes the callback check, instead of the element itself.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to search.
+ * @param {Function|Object|string} [callback=identity] The function called per
+ * iteration. If a property name or object is provided it will be used to
+ * create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {string|undefined} Returns the key of the found element, else `undefined`.
+ * @example
+ *
+ * var characters = {
+ * 'barney': { 'age': 36, 'blocked': false },
+ * 'fred': { 'age': 40, 'blocked': true },
+ * 'pebbles': { 'age': 1, 'blocked': false }
+ * };
+ *
+ * _.findKey(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => 'barney' (property order is not guaranteed across environments)
+ *
+ * // using "_.where" callback shorthand
+ * _.findKey(characters, { 'age': 1 });
+ * // => 'pebbles'
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findKey(characters, 'blocked');
+ * // => 'fred'
+ */
+function findKey(object, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+ forOwn(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result = key;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = findKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/findLastKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/findLastKey.js b/node_modules/lodash-node/modern/objects/findLastKey.js
new file mode 100644
index 0000000..236c726
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/findLastKey.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwnRight = require('./forOwnRight');
+
+/**
+ * This method is like `_.findKey` except that it iterates over elements
+ * of a `collection` in the opposite order.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to search.
+ * @param {Function|Object|string} [callback=identity] The function called per
+ * iteration. If a property name or object is provided it will be used to
+ * create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {string|undefined} Returns the key of the found element, else `undefined`.
+ * @example
+ *
+ * var characters = {
+ * 'barney': { 'age': 36, 'blocked': true },
+ * 'fred': { 'age': 40, 'blocked': false },
+ * 'pebbles': { 'age': 1, 'blocked': true }
+ * };
+ *
+ * _.findLastKey(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => returns `pebbles`, assuming `_.findKey` returns `barney`
+ *
+ * // using "_.where" callback shorthand
+ * _.findLastKey(characters, { 'age': 40 });
+ * // => 'fred'
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findLastKey(characters, 'blocked');
+ * // => 'pebbles'
+ */
+function findLastKey(object, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+ forOwnRight(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result = key;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = findLastKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/forIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/forIn.js b/node_modules/lodash-node/modern/objects/forIn.js
new file mode 100644
index 0000000..2d827c3
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/forIn.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Iterates over own and inherited enumerable properties of an object,
+ * executing the callback for each property. The callback is bound to `thisArg`
+ * and invoked with three arguments; (value, key, object). Callbacks may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * Shape.prototype.move = function(x, y) {
+ * this.x += x;
+ * this.y += y;
+ * };
+ *
+ * _.forIn(new Shape, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'x', 'y', and 'move' (property order is not guaranteed across environments)
+ */
+var forIn = function(collection, callback, thisArg) {
+ var index, iterable = collection, result = iterable;
+ if (!iterable) return result;
+ if (!objectTypes[typeof iterable]) return result;
+ callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3);
+ for (index in iterable) {
+ if (callback(iterable[index], index, collection) === false) return result;
+ }
+ return result
+};
+
+module.exports = forIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/forInRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/forInRight.js b/node_modules/lodash-node/modern/objects/forInRight.js
new file mode 100644
index 0000000..e9f077a
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/forInRight.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ forIn = require('./forIn');
+
+/**
+ * This method is like `_.forIn` except that it iterates over elements
+ * of a `collection` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * Shape.prototype.move = function(x, y) {
+ * this.x += x;
+ * this.y += y;
+ * };
+ *
+ * _.forInRight(new Shape, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'move', 'y', and 'x' assuming `_.forIn ` logs 'x', 'y', and 'move'
+ */
+function forInRight(object, callback, thisArg) {
+ var pairs = [];
+
+ forIn(object, function(value, key) {
+ pairs.push(key, value);
+ });
+
+ var length = pairs.length;
+ callback = baseCreateCallback(callback, thisArg, 3);
+ while (length--) {
+ if (callback(pairs[length--], pairs[length], object) === false) {
+ break;
+ }
+ }
+ return object;
+}
+
+module.exports = forInRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/forOwn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/forOwn.js b/node_modules/lodash-node/modern/objects/forOwn.js
new file mode 100644
index 0000000..33d2297
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/forOwn.js
@@ -0,0 +1,50 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ keys = require('./keys'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Iterates over own enumerable properties of an object, executing the callback
+ * for each property. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, key, object). Callbacks may exit iteration early by
+ * explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.forOwn({ '0': 'zero', '1': 'one', 'length': 2 }, function(num, key) {
+ * console.log(key);
+ * });
+ * // => logs '0', '1', and 'length' (property order is not guaranteed across environments)
+ */
+var forOwn = function(collection, callback, thisArg) {
+ var index, iterable = collection, result = iterable;
+ if (!iterable) return result;
+ if (!objectTypes[typeof iterable]) return result;
+ callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3);
+ var ownIndex = -1,
+ ownProps = objectTypes[typeof iterable] && keys(iterable),
+ length = ownProps ? ownProps.length : 0;
+
+ while (++ownIndex < length) {
+ index = ownProps[ownIndex];
+ if (callback(iterable[index], index, collection) === false) return result;
+ }
+ return result
+};
+
+module.exports = forOwn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/forOwnRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/forOwnRight.js b/node_modules/lodash-node/modern/objects/forOwnRight.js
new file mode 100644
index 0000000..86e53e8
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/forOwnRight.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ keys = require('./keys');
+
+/**
+ * This method is like `_.forOwn` except that it iterates over elements
+ * of a `collection` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.forOwnRight({ '0': 'zero', '1': 'one', 'length': 2 }, function(num, key) {
+ * console.log(key);
+ * });
+ * // => logs 'length', '1', and '0' assuming `_.forOwn` logs '0', '1', and 'length'
+ */
+function forOwnRight(object, callback, thisArg) {
+ var props = keys(object),
+ length = props.length;
+
+ callback = baseCreateCallback(callback, thisArg, 3);
+ while (length--) {
+ var key = props[length];
+ if (callback(object[key], key, object) === false) {
+ break;
+ }
+ }
+ return object;
+}
+
+module.exports = forOwnRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/functions.js b/node_modules/lodash-node/modern/objects/functions.js
new file mode 100644
index 0000000..4cfd478
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/functions.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('./forIn'),
+ isFunction = require('./isFunction');
+
+/**
+ * Creates a sorted array of property names of all enumerable properties,
+ * own and inherited, of `object` that have function values.
+ *
+ * @static
+ * @memberOf _
+ * @alias methods
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names that have function values.
+ * @example
+ *
+ * _.functions(_);
+ * // => ['all', 'any', 'bind', 'bindAll', 'clone', 'compact', 'compose', ...]
+ */
+function functions(object) {
+ var result = [];
+ forIn(object, function(value, key) {
+ if (isFunction(value)) {
+ result.push(key);
+ }
+ });
+ return result.sort();
+}
+
+module.exports = functions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/has.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/has.js b/node_modules/lodash-node/modern/objects/has.js
new file mode 100644
index 0000000..e7f610c
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/has.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if the specified property name exists as a direct property of `object`,
+ * instead of an inherited property.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @param {string} key The name of the property to check.
+ * @returns {boolean} Returns `true` if key is a direct property, else `false`.
+ * @example
+ *
+ * _.has({ 'a': 1, 'b': 2, 'c': 3 }, 'b');
+ * // => true
+ */
+function has(object, key) {
+ return object ? hasOwnProperty.call(object, key) : false;
+}
+
+module.exports = has;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/invert.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/invert.js b/node_modules/lodash-node/modern/objects/invert.js
new file mode 100644
index 0000000..66d5949
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/invert.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates an object composed of the inverted keys and values of the given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to invert.
+ * @returns {Object} Returns the created inverted object.
+ * @example
+ *
+ * _.invert({ 'first': 'fred', 'second': 'barney' });
+ * // => { 'fred': 'first', 'barney': 'second' }
+ */
+function invert(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ result[object[key]] = key;
+ }
+ return result;
+}
+
+module.exports = invert;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isArguments.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isArguments.js b/node_modules/lodash-node/modern/objects/isArguments.js
new file mode 100644
index 0000000..eb87b80
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isArguments.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an `arguments` object, else `false`.
+ * @example
+ *
+ * (function() { return _.isArguments(arguments); })(1, 2, 3);
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ toString.call(value) == argsClass || false;
+}
+
+module.exports = isArguments;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isArray.js b/node_modules/lodash-node/modern/objects/isArray.js
new file mode 100644
index 0000000..c50e72c
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isArray.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative');
+
+/** `Object#toString` result shortcuts */
+var arrayClass = '[object Array]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeIsArray = isNative(nativeIsArray = Array.isArray) && nativeIsArray;
+
+/**
+ * Checks if `value` is an array.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an array, else `false`.
+ * @example
+ *
+ * (function() { return _.isArray(arguments); })();
+ * // => false
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ */
+var isArray = nativeIsArray || function(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ toString.call(value) == arrayClass || false;
+};
+
+module.exports = isArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isBoolean.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isBoolean.js b/node_modules/lodash-node/modern/objects/isBoolean.js
new file mode 100644
index 0000000..614b298
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isBoolean.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var boolClass = '[object Boolean]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a boolean value.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a boolean value, else `false`.
+ * @example
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+function isBoolean(value) {
+ return value === true || value === false ||
+ value && typeof value == 'object' && toString.call(value) == boolClass || false;
+}
+
+module.exports = isBoolean;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isDate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isDate.js b/node_modules/lodash-node/modern/objects/isDate.js
new file mode 100644
index 0000000..36ed1cf
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isDate.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var dateClass = '[object Date]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a date.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a date, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ */
+function isDate(value) {
+ return value && typeof value == 'object' && toString.call(value) == dateClass || false;
+}
+
+module.exports = isDate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isElement.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isElement.js b/node_modules/lodash-node/modern/objects/isElement.js
new file mode 100644
index 0000000..243b618
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isElement.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ */
+function isElement(value) {
+ return value && value.nodeType === 1 || false;
+}
+
+module.exports = isElement;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isEmpty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isEmpty.js b/node_modules/lodash-node/modern/objects/isEmpty.js
new file mode 100644
index 0000000..35fe0f7
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isEmpty.js
@@ -0,0 +1,63 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forOwn = require('./forOwn'),
+ isFunction = require('./isFunction');
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ objectClass = '[object Object]',
+ stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is empty. Arrays, strings, or `arguments` objects with a
+ * length of `0` and objects with no own enumerable properties are considered
+ * "empty".
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Array|Object|string} value The value to inspect.
+ * @returns {boolean} Returns `true` if the `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({});
+ * // => true
+ *
+ * _.isEmpty('');
+ * // => true
+ */
+function isEmpty(value) {
+ var result = true;
+ if (!value) {
+ return result;
+ }
+ var className = toString.call(value),
+ length = value.length;
+
+ if ((className == arrayClass || className == stringClass || className == argsClass ) ||
+ (className == objectClass && typeof length == 'number' && isFunction(value.splice))) {
+ return !length;
+ }
+ forOwn(value, function() {
+ return (result = false);
+ });
+ return result;
+}
+
+module.exports = isEmpty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isEqual.js b/node_modules/lodash-node/modern/objects/isEqual.js
new file mode 100644
index 0000000..54c83fe
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isEqual.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseIsEqual = require('../internals/baseIsEqual');
+
+/**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent to each other. If a callback is provided it will be executed
+ * to compare values. If the callback returns `undefined` comparisons will
+ * be handled by the method instead. The callback is bound to `thisArg` and
+ * invoked with two arguments; (a, b).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} a The value to compare.
+ * @param {*} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * var copy = { 'name': 'fred' };
+ *
+ * object == copy;
+ * // => false
+ *
+ * _.isEqual(object, copy);
+ * // => true
+ *
+ * var words = ['hello', 'goodbye'];
+ * var otherWords = ['hi', 'goodbye'];
+ *
+ * _.isEqual(words, otherWords, function(a, b) {
+ * var reGreet = /^(?:hello|hi)$/i,
+ * aGreet = _.isString(a) && reGreet.test(a),
+ * bGreet = _.isString(b) && reGreet.test(b);
+ *
+ * return (aGreet || bGreet) ? (aGreet == bGreet) : undefined;
+ * });
+ * // => true
+ */
+function isEqual(a, b, callback, thisArg) {
+ return baseIsEqual(a, b, typeof callback == 'function' && baseCreateCallback(callback, thisArg, 2));
+}
+
+module.exports = isEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isFinite.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isFinite.js b/node_modules/lodash-node/modern/objects/isFinite.js
new file mode 100644
index 0000000..8142a8b
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isFinite.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeIsFinite = global.isFinite,
+ nativeIsNaN = global.isNaN;
+
+/**
+ * Checks if `value` is, or can be coerced to, a finite number.
+ *
+ * Note: This is not the same as native `isFinite` which will return true for
+ * booleans and empty strings. See http://es5.github.io/#x15.1.2.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is finite, else `false`.
+ * @example
+ *
+ * _.isFinite(-101);
+ * // => true
+ *
+ * _.isFinite('10');
+ * // => true
+ *
+ * _.isFinite(true);
+ * // => false
+ *
+ * _.isFinite('');
+ * // => false
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ */
+function isFinite(value) {
+ return nativeIsFinite(value) && !nativeIsNaN(parseFloat(value));
+}
+
+module.exports = isFinite;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isFunction.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isFunction.js b/node_modules/lodash-node/modern/objects/isFunction.js
new file mode 100644
index 0000000..30a49f1
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isFunction.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is a function.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ */
+function isFunction(value) {
+ return typeof value == 'function';
+}
+
+module.exports = isFunction;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isNaN.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isNaN.js b/node_modules/lodash-node/modern/objects/isNaN.js
new file mode 100644
index 0000000..1fff994
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isNaN.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNumber = require('./isNumber');
+
+/**
+ * Checks if `value` is `NaN`.
+ *
+ * Note: This is not the same as native `isNaN` which will return `true` for
+ * `undefined` and other non-numeric values. See http://es5.github.io/#x15.1.2.4.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+function isNaN(value) {
+ // `NaN` as a primitive is the only value that is not equal to itself
+ // (perform the [[Class]] check first to avoid errors with some host objects in IE)
+ return isNumber(value) && value != +value;
+}
+
+module.exports = isNaN;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isNull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isNull.js b/node_modules/lodash-node/modern/objects/isNull.js
new file mode 100644
index 0000000..423296d
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isNull.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(undefined);
+ * // => false
+ */
+function isNull(value) {
+ return value === null;
+}
+
+module.exports = isNull;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[02/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/build/output.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/build/output.js b/node_modules/shelljs/build/output.js
new file mode 100644
index 0000000..1b778b9
--- /dev/null
+++ b/node_modules/shelljs/build/output.js
@@ -0,0 +1,2411 @@
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### cat(file [, file ...])
+//@ ### cat(file_array)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var str = cat('file*.txt');
+//@ var str = cat('file1', 'file2');
+//@ var str = cat(['file1', 'file2']); // same as above
+//@ ```
+//@
+//@ Returns a string containing the given file, or a concatenated string
+//@ containing the files if more than one file is given (a new line character is
+//@ introduced between each file). Wildcard `*` accepted.
+function _cat(options, files) {
+ var cat = '';
+
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ files.forEach(function(file) {
+ if (!fs.existsSync(file))
+ common.error('no such file or directory: ' + file);
+
+ cat += fs.readFileSync(file, 'utf8');
+ });
+
+ return common.ShellString(cat);
+}
+module.exports = _cat;
+
+var fs = require('fs');
+var common = require('./common');
+
+//@
+//@ ### cd([dir])
+//@ Changes to directory `dir` for the duration of the script. Changes to home
+//@ directory if no argument is supplied.
+function _cd(options, dir) {
+ if (!dir)
+ dir = common.getUserHome();
+
+ if (dir === '-') {
+ if (!common.state.previousDir)
+ common.error('could not find previous directory');
+ else
+ dir = common.state.previousDir;
+ }
+
+ if (!fs.existsSync(dir))
+ common.error('no such file or directory: ' + dir);
+
+ if (!fs.statSync(dir).isDirectory())
+ common.error('not a directory: ' + dir);
+
+ common.state.previousDir = process.cwd();
+ process.chdir(dir);
+}
+module.exports = _cd;
+
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+var PERMS = (function (base) {
+ return {
+ OTHER_EXEC : base.EXEC,
+ OTHER_WRITE : base.WRITE,
+ OTHER_READ : base.READ,
+
+ GROUP_EXEC : base.EXEC << 3,
+ GROUP_WRITE : base.WRITE << 3,
+ GROUP_READ : base.READ << 3,
+
+ OWNER_EXEC : base.EXEC << 6,
+ OWNER_WRITE : base.WRITE << 6,
+ OWNER_READ : base.READ << 6,
+
+ // Literal octal numbers are apparently not allowed in "strict" javascript. Using parseInt is
+ // the preferred way, else a jshint warning is thrown.
+ STICKY : parseInt('01000', 8),
+ SETGID : parseInt('02000', 8),
+ SETUID : parseInt('04000', 8),
+
+ TYPE_MASK : parseInt('0770000', 8)
+ };
+})({
+ EXEC : 1,
+ WRITE : 2,
+ READ : 4
+});
+
+//@
+//@ ### chmod(octal_mode || octal_string, file)
+//@ ### chmod(symbolic_mode, file)
+//@
+//@ Available options:
+//@
+//@ + `-v`: output a diagnostic for every file processed//@
+//@ + `-c`: like verbose but report only when a change is made//@
+//@ + `-R`: change files and directories recursively//@
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ chmod(755, '/Users/brandon');
+//@ chmod('755', '/Users/brandon'); // same as above
+//@ chmod('u+x', '/Users/brandon');
+//@ ```
+//@
+//@ Alters the permissions of a file or directory by either specifying the
+//@ absolute permissions in octal form or expressing the changes in symbols.
+//@ This command tries to mimic the POSIX behavior as much as possible.
+//@ Notable exceptions:
+//@
+//@ + In symbolic modes, 'a-r' and '-r' are identical. No consideration is
+//@ given to the umask.
+//@ + There is no "quiet" option since default behavior is to run silent.
+function _chmod(options, mode, filePattern) {
+ if (!filePattern) {
+ if (options.length > 0 && options.charAt(0) === '-') {
+ // Special case where the specified file permissions started with - to subtract perms, which
+ // get picked up by the option parser as command flags.
+ // If we are down by one argument and options starts with -, shift everything over.
+ filePattern = mode;
+ mode = options;
+ options = '';
+ }
+ else {
+ common.error('You must specify a file.');
+ }
+ }
+
+ options = common.parseOptions(options, {
+ 'R': 'recursive',
+ 'c': 'changes',
+ 'v': 'verbose'
+ });
+
+ if (typeof filePattern === 'string') {
+ filePattern = [ filePattern ];
+ }
+
+ var files;
+
+ if (options.recursive) {
+ files = [];
+ common.expand(filePattern).forEach(function addFile(expandedFile) {
+ var stat = fs.lstatSync(expandedFile);
+
+ if (!stat.isSymbolicLink()) {
+ files.push(expandedFile);
+
+ if (stat.isDirectory()) { // intentionally does not follow symlinks.
+ fs.readdirSync(expandedFile).forEach(function (child) {
+ addFile(expandedFile + '/' + child);
+ });
+ }
+ }
+ });
+ }
+ else {
+ files = common.expand(filePattern);
+ }
+
+ files.forEach(function innerChmod(file) {
+ file = path.resolve(file);
+ if (!fs.existsSync(file)) {
+ common.error('File not found: ' + file);
+ }
+
+ // When recursing, don't follow symlinks.
+ if (options.recursive && fs.lstatSync(file).isSymbolicLink()) {
+ return;
+ }
+
+ var stat = fs.statSync(file);
+ var isDir = stat.isDirectory();
+ var perms = stat.mode;
+ var type = perms & PERMS.TYPE_MASK;
+
+ var newPerms = perms;
+
+ if (isNaN(parseInt(mode, 8))) {
+ // parse options
+ mode.split(',').forEach(function (symbolicMode) {
+ /*jshint regexdash:true */
+ var pattern = /([ugoa]*)([=\+-])([rwxXst]*)/i;
+ var matches = pattern.exec(symbolicMode);
+
+ if (matches) {
+ var applyTo = matches[1];
+ var operator = matches[2];
+ var change = matches[3];
+
+ var changeOwner = applyTo.indexOf('u') != -1 || applyTo === 'a' || applyTo === '';
+ var changeGroup = applyTo.indexOf('g') != -1 || applyTo === 'a' || applyTo === '';
+ var changeOther = applyTo.indexOf('o') != -1 || applyTo === 'a' || applyTo === '';
+
+ var changeRead = change.indexOf('r') != -1;
+ var changeWrite = change.indexOf('w') != -1;
+ var changeExec = change.indexOf('x') != -1;
+ var changeExecDir = change.indexOf('X') != -1;
+ var changeSticky = change.indexOf('t') != -1;
+ var changeSetuid = change.indexOf('s') != -1;
+
+ if (changeExecDir && isDir)
+ changeExec = true;
+
+ var mask = 0;
+ if (changeOwner) {
+ mask |= (changeRead ? PERMS.OWNER_READ : 0) + (changeWrite ? PERMS.OWNER_WRITE : 0) + (changeExec ? PERMS.OWNER_EXEC : 0) + (changeSetuid ? PERMS.SETUID : 0);
+ }
+ if (changeGroup) {
+ mask |= (changeRead ? PERMS.GROUP_READ : 0) + (changeWrite ? PERMS.GROUP_WRITE : 0) + (changeExec ? PERMS.GROUP_EXEC : 0) + (changeSetuid ? PERMS.SETGID : 0);
+ }
+ if (changeOther) {
+ mask |= (changeRead ? PERMS.OTHER_READ : 0) + (changeWrite ? PERMS.OTHER_WRITE : 0) + (changeExec ? PERMS.OTHER_EXEC : 0);
+ }
+
+ // Sticky bit is special - it's not tied to user, group or other.
+ if (changeSticky) {
+ mask |= PERMS.STICKY;
+ }
+
+ switch (operator) {
+ case '+':
+ newPerms |= mask;
+ break;
+
+ case '-':
+ newPerms &= ~mask;
+ break;
+
+ case '=':
+ newPerms = type + mask;
+
+ // According to POSIX, when using = to explicitly set the permissions, setuid and setgid can never be cleared.
+ if (fs.statSync(file).isDirectory()) {
+ newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
+ }
+ break;
+ }
+
+ if (options.verbose) {
+ console.log(file + ' -> ' + newPerms.toString(8));
+ }
+
+ if (perms != newPerms) {
+ if (!options.verbose && options.changes) {
+ console.log(file + ' -> ' + newPerms.toString(8));
+ }
+ fs.chmodSync(file, newPerms);
+ perms = newPerms; // for the next round of changes!
+ }
+ }
+ else {
+ common.error('Invalid symbolic mode change: ' + symbolicMode);
+ }
+ });
+ }
+ else {
+ // they gave us a full number
+ newPerms = type + parseInt(mode, 8);
+
+ // POSIX rules are that setuid and setgid can only be added using numeric form, but not cleared.
+ if (fs.statSync(file).isDirectory()) {
+ newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
+ }
+
+ fs.chmodSync(file, newPerms);
+ }
+ });
+}
+module.exports = _chmod;
+
+var os = require('os');
+var fs = require('fs');
+var _ls = require('./ls');
+
+// Module globals
+var config = {
+ silent: false,
+ fatal: false,
+ verbose: false,
+};
+exports.config = config;
+
+var state = {
+ error: null,
+ currentCmd: 'shell.js',
+ previousDir: null,
+ tempDir: null
+};
+exports.state = state;
+
+var platform = os.type().match(/^Win/) ? 'win' : 'unix';
+exports.platform = platform;
+
+function log() {
+ if (!config.silent)
+ console.error.apply(console, arguments);
+}
+exports.log = log;
+
+// Shows error message. Throws unless _continue or config.fatal are true
+function error(msg, _continue) {
+ if (state.error === null)
+ state.error = '';
+ var log_entry = state.currentCmd + ': ' + msg;
+ if (state.error === '')
+ state.error = log_entry;
+ else
+ state.error += '\n' + log_entry;
+
+ if (msg.length > 0)
+ log(log_entry);
+
+ if (config.fatal)
+ process.exit(1);
+
+ if (!_continue)
+ throw '';
+}
+exports.error = error;
+
+// In the future, when Proxies are default, we can add methods like `.to()` to primitive strings.
+// For now, this is a dummy function to bookmark places we need such strings
+function ShellString(str) {
+ return str;
+}
+exports.ShellString = ShellString;
+
+// Return the home directory in a platform-agnostic way, with consideration for
+// older versions of node
+function getUserHome() {
+ var result;
+ if (os.homedir)
+ result = os.homedir(); // node 3+
+ else
+ result = process.env[(process.platform === 'win32') ? 'USERPROFILE' : 'HOME'];
+ return result;
+}
+exports.getUserHome = getUserHome;
+
+// Returns {'alice': true, 'bob': false} when passed a dictionary, e.g.:
+// parseOptions('-a', {'a':'alice', 'b':'bob'});
+function parseOptions(str, map) {
+ if (!map)
+ error('parseOptions() internal error: no map given');
+
+ // All options are false by default
+ var options = {};
+ for (var letter in map) {
+ if (!map[letter].match('^!'))
+ options[map[letter]] = false;
+ }
+
+ if (!str)
+ return options; // defaults
+
+ if (typeof str !== 'string')
+ error('parseOptions() internal error: wrong str');
+
+ // e.g. match[1] = 'Rf' for str = '-Rf'
+ var match = str.match(/^\-(.+)/);
+ if (!match)
+ return options;
+
+ // e.g. chars = ['R', 'f']
+ var chars = match[1].split('');
+
+ var opt;
+ chars.forEach(function(c) {
+ if (c in map) {
+ opt = map[c];
+ if (opt.match('^!'))
+ options[opt.slice(1, opt.length-1)] = false;
+ else
+ options[opt] = true;
+ } else {
+ error('option not recognized: '+c);
+ }
+ });
+
+ return options;
+}
+exports.parseOptions = parseOptions;
+
+// Expands wildcards with matching (ie. existing) file names.
+// For example:
+// expand(['file*.js']) = ['file1.js', 'file2.js', ...]
+// (if the files 'file1.js', 'file2.js', etc, exist in the current dir)
+function expand(list) {
+ var expanded = [];
+ list.forEach(function(listEl) {
+ // Wildcard present on directory names ?
+ if(listEl.search(/\*[^\/]*\//) > -1 || listEl.search(/\*\*[^\/]*\//) > -1) {
+ var match = listEl.match(/^([^*]+\/|)(.*)/);
+ var root = match[1];
+ var rest = match[2];
+ var restRegex = rest.replace(/\*\*/g, ".*").replace(/\*/g, "[^\\/]*");
+ restRegex = new RegExp(restRegex);
+
+ _ls('-R', root).filter(function (e) {
+ return restRegex.test(e);
+ }).forEach(function(file) {
+ expanded.push(file);
+ });
+ }
+ // Wildcard present on file names ?
+ else if (listEl.search(/\*/) > -1) {
+ _ls('', listEl).forEach(function(file) {
+ expanded.push(file);
+ });
+ } else {
+ expanded.push(listEl);
+ }
+ });
+ return expanded;
+}
+exports.expand = expand;
+
+// Normalizes _unlinkSync() across platforms to match Unix behavior, i.e.
+// file can be unlinked even if it's read-only, see https://github.com/joyent/node/issues/3006
+function unlinkSync(file) {
+ try {
+ fs.unlinkSync(file);
+ } catch(e) {
+ // Try to override file permission
+ if (e.code === 'EPERM') {
+ fs.chmodSync(file, '0666');
+ fs.unlinkSync(file);
+ } else {
+ throw e;
+ }
+ }
+}
+exports.unlinkSync = unlinkSync;
+
+// e.g. 'shelljs_a5f185d0443ca...'
+function randomFileName() {
+ function randomHash(count) {
+ if (count === 1)
+ return parseInt(16*Math.random(), 10).toString(16);
+ else {
+ var hash = '';
+ for (var i=0; i<count; i++)
+ hash += randomHash(1);
+ return hash;
+ }
+ }
+
+ return 'shelljs_'+randomHash(20);
+}
+exports.randomFileName = randomFileName;
+
+// extend(target_obj, source_obj1 [, source_obj2 ...])
+// Shallow extend, e.g.:
+// extend({A:1}, {b:2}, {c:3}) returns {A:1, b:2, c:3}
+function extend(target) {
+ var sources = [].slice.call(arguments, 1);
+ sources.forEach(function(source) {
+ for (var key in source)
+ target[key] = source[key];
+ });
+
+ return target;
+}
+exports.extend = extend;
+
+// Common wrapper for all Unix-like commands
+function wrap(cmd, fn, options) {
+ return function() {
+ var retValue = null;
+
+ state.currentCmd = cmd;
+ state.error = null;
+
+ try {
+ var args = [].slice.call(arguments, 0);
+
+ if (config.verbose) {
+ args.unshift(cmd);
+ console.log.apply(console, args);
+ args.shift();
+ }
+
+ if (options && options.notUnix) {
+ retValue = fn.apply(this, args);
+ } else {
+ if (args.length === 0 || typeof args[0] !== 'string' || args[0].length <= 1 || args[0][0] !== '-')
+ args.unshift(''); // only add dummy option if '-option' not already present
+ // Expand the '~' if appropriate
+ var homeDir = getUserHome();
+ args = args.map(function(arg) {
+ if (typeof arg === 'string' && arg.slice(0, 2) === '~/' || arg === '~')
+ return arg.replace(/^~/, homeDir);
+ else
+ return arg;
+ });
+ retValue = fn.apply(this, args);
+ }
+ } catch (e) {
+ if (!state.error) {
+ // If state.error hasn't been set it's an error thrown by Node, not us - probably a bug...
+ console.log('shell.js: internal error');
+ console.log(e.stack || e);
+ process.exit(1);
+ }
+ if (config.fatal)
+ throw e;
+ }
+
+ state.currentCmd = 'shell.js';
+ return retValue;
+ };
+} // wrap
+exports.wrap = wrap;
+
+var fs = require('fs');
+var path = require('path');
+var common = require('./common');
+var os = require('os');
+
+// Buffered file copy, synchronous
+// (Using readFileSync() + writeFileSync() could easily cause a memory overflow
+// with large files)
+function copyFileSync(srcFile, destFile) {
+ if (!fs.existsSync(srcFile))
+ common.error('copyFileSync: no such file or directory: ' + srcFile);
+
+ var BUF_LENGTH = 64*1024,
+ buf = new Buffer(BUF_LENGTH),
+ bytesRead = BUF_LENGTH,
+ pos = 0,
+ fdr = null,
+ fdw = null;
+
+ try {
+ fdr = fs.openSync(srcFile, 'r');
+ } catch(e) {
+ common.error('copyFileSync: could not read src file ('+srcFile+')');
+ }
+
+ try {
+ fdw = fs.openSync(destFile, 'w');
+ } catch(e) {
+ common.error('copyFileSync: could not write to dest file (code='+e.code+'):'+destFile);
+ }
+
+ while (bytesRead === BUF_LENGTH) {
+ bytesRead = fs.readSync(fdr, buf, 0, BUF_LENGTH, pos);
+ fs.writeSync(fdw, buf, 0, bytesRead);
+ pos += bytesRead;
+ }
+
+ fs.closeSync(fdr);
+ fs.closeSync(fdw);
+
+ fs.chmodSync(destFile, fs.statSync(srcFile).mode);
+}
+
+// Recursively copies 'sourceDir' into 'destDir'
+// Adapted from https://github.com/ryanmcgrath/wrench-js
+//
+// Copyright (c) 2010 Ryan McGrath
+// Copyright (c) 2012 Artur Adib
+//
+// Licensed under the MIT License
+// http://www.opensource.org/licenses/mit-license.php
+function cpdirSyncRecursive(sourceDir, destDir, opts) {
+ if (!opts) opts = {};
+
+ /* Create the directory where all our junk is moving to; read the mode of the source directory and mirror it */
+ var checkDir = fs.statSync(sourceDir);
+ try {
+ fs.mkdirSync(destDir, checkDir.mode);
+ } catch (e) {
+ //if the directory already exists, that's okay
+ if (e.code !== 'EEXIST') throw e;
+ }
+
+ var files = fs.readdirSync(sourceDir);
+
+ for (var i = 0; i < files.length; i++) {
+ var srcFile = sourceDir + "/" + files[i];
+ var destFile = destDir + "/" + files[i];
+ var srcFileStat = fs.lstatSync(srcFile);
+
+ if (srcFileStat.isDirectory()) {
+ /* recursion this thing right on back. */
+ cpdirSyncRecursive(srcFile, destFile, opts);
+ } else if (srcFileStat.isSymbolicLink()) {
+ var symlinkFull = fs.readlinkSync(srcFile);
+ fs.symlinkSync(symlinkFull, destFile, os.platform() === "win32" ? "junction" : null);
+ } else {
+ /* At this point, we've hit a file actually worth copying... so copy it on over. */
+ if (fs.existsSync(destFile) && opts.no_force) {
+ common.log('skipping existing file: ' + files[i]);
+ } else {
+ copyFileSync(srcFile, destFile);
+ }
+ }
+
+ } // for files
+} // cpdirSyncRecursive
+
+
+//@
+//@ ### cp([options,] source [, source ...], dest)
+//@ ### cp([options,] source_array, dest)
+//@ Available options:
+//@
+//@ + `-f`: force (default behavior)
+//@ + `-n`: no-clobber
+//@ + `-r, -R`: recursive
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ cp('file1', 'dir1');
+//@ cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
+//@ cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
+//@ ```
+//@
+//@ Copies files. The wildcard `*` is accepted.
+function _cp(options, sources, dest) {
+ options = common.parseOptions(options, {
+ 'f': '!no_force',
+ 'n': 'no_force',
+ 'R': 'recursive',
+ 'r': 'recursive'
+ });
+
+ // Get sources, dest
+ if (arguments.length < 3) {
+ common.error('missing <source> and/or <dest>');
+ } else if (arguments.length > 3) {
+ sources = [].slice.call(arguments, 1, arguments.length - 1);
+ dest = arguments[arguments.length - 1];
+ } else if (typeof sources === 'string') {
+ sources = [sources];
+ } else if ('length' in sources) {
+ sources = sources; // no-op for array
+ } else {
+ common.error('invalid arguments');
+ }
+
+ var exists = fs.existsSync(dest),
+ stats = exists && fs.statSync(dest);
+
+ // Dest is not existing dir, but multiple sources given
+ if ((!exists || !stats.isDirectory()) && sources.length > 1)
+ common.error('dest is not a directory (too many sources)');
+
+ // Dest is an existing file, but no -f given
+ if (exists && stats.isFile() && options.no_force)
+ common.error('dest file already exists: ' + dest);
+
+ if (options.recursive) {
+ // Recursive allows the shortcut syntax "sourcedir/" for "sourcedir/*"
+ // (see Github issue #15)
+ sources.forEach(function(src, i) {
+ if (src[src.length - 1] === '/') {
+ sources[i] += '*';
+ // If src is a directory and dest doesn't exist, 'cp -r src dest' should copy src/* into dest
+ } else if (fs.statSync(src).isDirectory() && !exists) {
+ sources[i] += '/*';
+ }
+ });
+
+ // Create dest
+ try {
+ fs.mkdirSync(dest, parseInt('0777', 8));
+ } catch (e) {
+ // like Unix's cp, keep going even if we can't create dest dir
+ }
+ }
+
+ sources = common.expand(sources);
+
+ sources.forEach(function(src) {
+ if (!fs.existsSync(src)) {
+ common.error('no such file or directory: '+src, true);
+ return; // skip file
+ }
+
+ // If here, src exists
+ if (fs.statSync(src).isDirectory()) {
+ if (!options.recursive) {
+ // Non-Recursive
+ common.log(src + ' is a directory (not copied)');
+ } else {
+ // Recursive
+ // 'cp /a/source dest' should create 'source' in 'dest'
+ var newDest = path.join(dest, path.basename(src)),
+ checkDir = fs.statSync(src);
+ try {
+ fs.mkdirSync(newDest, checkDir.mode);
+ } catch (e) {
+ //if the directory already exists, that's okay
+ if (e.code !== 'EEXIST') {
+ common.error('dest file no such file or directory: ' + newDest, true);
+ throw e;
+ }
+ }
+
+ cpdirSyncRecursive(src, newDest, {no_force: options.no_force});
+ }
+ return; // done with dir
+ }
+
+ // If here, src is a file
+
+ // When copying to '/path/dir':
+ // thisDest = '/path/dir/file1'
+ var thisDest = dest;
+ if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
+ thisDest = path.normalize(dest + '/' + path.basename(src));
+
+ if (fs.existsSync(thisDest) && options.no_force) {
+ common.error('dest file already exists: ' + thisDest, true);
+ return; // skip file
+ }
+
+ copyFileSync(src, thisDest);
+ }); // forEach(src)
+}
+module.exports = _cp;
+
+var common = require('./common');
+var _cd = require('./cd');
+var path = require('path');
+
+// Pushd/popd/dirs internals
+var _dirStack = [];
+
+function _isStackIndex(index) {
+ return (/^[\-+]\d+$/).test(index);
+}
+
+function _parseStackIndex(index) {
+ if (_isStackIndex(index)) {
+ if (Math.abs(index) < _dirStack.length + 1) { // +1 for pwd
+ return (/^-/).test(index) ? Number(index) - 1 : Number(index);
+ } else {
+ common.error(index + ': directory stack index out of range');
+ }
+ } else {
+ common.error(index + ': invalid number');
+ }
+}
+
+function _actualDirStack() {
+ return [process.cwd()].concat(_dirStack);
+}
+
+//@
+//@ ### pushd([options,] [dir | '-N' | '+N'])
+//@
+//@ Available options:
+//@
+//@ + `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
+//@
+//@ Arguments:
+//@
+//@ + `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
+//@ + `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
+//@ + `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ // process.cwd() === '/usr'
+//@ pushd('/etc'); // Returns /etc /usr
+//@ pushd('+1'); // Returns /usr /etc
+//@ ```
+//@
+//@ Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
+function _pushd(options, dir) {
+ if (_isStackIndex(options)) {
+ dir = options;
+ options = '';
+ }
+
+ options = common.parseOptions(options, {
+ 'n' : 'no-cd'
+ });
+
+ var dirs = _actualDirStack();
+
+ if (dir === '+0') {
+ return dirs; // +0 is a noop
+ } else if (!dir) {
+ if (dirs.length > 1) {
+ dirs = dirs.splice(1, 1).concat(dirs);
+ } else {
+ return common.error('no other directory');
+ }
+ } else if (_isStackIndex(dir)) {
+ var n = _parseStackIndex(dir);
+ dirs = dirs.slice(n).concat(dirs.slice(0, n));
+ } else {
+ if (options['no-cd']) {
+ dirs.splice(1, 0, dir);
+ } else {
+ dirs.unshift(dir);
+ }
+ }
+
+ if (options['no-cd']) {
+ dirs = dirs.slice(1);
+ } else {
+ dir = path.resolve(dirs.shift());
+ _cd('', dir);
+ }
+
+ _dirStack = dirs;
+ return _dirs('');
+}
+exports.pushd = _pushd;
+
+//@
+//@ ### popd([options,] ['-N' | '+N'])
+//@
+//@ Available options:
+//@
+//@ + `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
+//@
+//@ Arguments:
+//@
+//@ + `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
+//@ + `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ echo(process.cwd()); // '/usr'
+//@ pushd('/etc'); // '/etc /usr'
+//@ echo(process.cwd()); // '/etc'
+//@ popd(); // '/usr'
+//@ echo(process.cwd()); // '/usr'
+//@ ```
+//@
+//@ When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
+function _popd(options, index) {
+ if (_isStackIndex(options)) {
+ index = options;
+ options = '';
+ }
+
+ options = common.parseOptions(options, {
+ 'n' : 'no-cd'
+ });
+
+ if (!_dirStack.length) {
+ return common.error('directory stack empty');
+ }
+
+ index = _parseStackIndex(index || '+0');
+
+ if (options['no-cd'] || index > 0 || _dirStack.length + index === 0) {
+ index = index > 0 ? index - 1 : index;
+ _dirStack.splice(index, 1);
+ } else {
+ var dir = path.resolve(_dirStack.shift());
+ _cd('', dir);
+ }
+
+ return _dirs('');
+}
+exports.popd = _popd;
+
+//@
+//@ ### dirs([options | '+N' | '-N'])
+//@
+//@ Available options:
+//@
+//@ + `-c`: Clears the directory stack by deleting all of the elements.
+//@
+//@ Arguments:
+//@
+//@ + `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
+//@ + `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
+//@
+//@ Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
+//@
+//@ See also: pushd, popd
+function _dirs(options, index) {
+ if (_isStackIndex(options)) {
+ index = options;
+ options = '';
+ }
+
+ options = common.parseOptions(options, {
+ 'c' : 'clear'
+ });
+
+ if (options['clear']) {
+ _dirStack = [];
+ return _dirStack;
+ }
+
+ var stack = _actualDirStack();
+
+ if (index) {
+ index = _parseStackIndex(index);
+
+ if (index < 0) {
+ index = stack.length + index;
+ }
+
+ common.log(stack[index]);
+ return stack[index];
+ }
+
+ common.log(stack.join(' '));
+
+ return stack;
+}
+exports.dirs = _dirs;
+
+var common = require('./common');
+
+//@
+//@ ### echo(string [, string ...])
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ echo('hello world');
+//@ var str = echo('hello world');
+//@ ```
+//@
+//@ Prints string to stdout, and returns string with additional utility methods
+//@ like `.to()`.
+function _echo() {
+ var messages = [].slice.call(arguments, 0);
+ console.log.apply(console, messages);
+ return common.ShellString(messages.join(' '));
+}
+module.exports = _echo;
+
+var common = require('./common');
+
+//@
+//@ ### error()
+//@ Tests if error occurred in the last command. Returns `null` if no error occurred,
+//@ otherwise returns string explaining the error
+function error() {
+ return common.state.error;
+}
+module.exports = error;
+
+var common = require('./common');
+var _tempDir = require('./tempdir');
+var _pwd = require('./pwd');
+var path = require('path');
+var fs = require('fs');
+var child = require('child_process');
+
+// Hack to run child_process.exec() synchronously (sync avoids callback hell)
+// Uses a custom wait loop that checks for a flag file, created when the child process is done.
+// (Can't do a wait loop that checks for internal Node variables/messages as
+// Node is single-threaded; callbacks and other internal state changes are done in the
+// event loop).
+function execSync(cmd, opts) {
+ var tempDir = _tempDir();
+ var stdoutFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ stderrFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ codeFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ scriptFile = path.resolve(tempDir+'/'+common.randomFileName()),
+ sleepFile = path.resolve(tempDir+'/'+common.randomFileName());
+
+ var options = common.extend({
+ silent: common.config.silent
+ }, opts);
+
+ var previousStdoutContent = '',
+ previousStderrContent = '';
+ // Echoes stdout and stderr changes from running process, if not silent
+ function updateStream(streamFile) {
+ if (options.silent || !fs.existsSync(streamFile))
+ return;
+
+ var previousStreamContent,
+ proc_stream;
+ if (streamFile === stdoutFile) {
+ previousStreamContent = previousStdoutContent;
+ proc_stream = process.stdout;
+ } else { // assume stderr
+ previousStreamContent = previousStderrContent;
+ proc_stream = process.stderr;
+ }
+
+ var streamContent = fs.readFileSync(streamFile, 'utf8');
+ // No changes since last time?
+ if (streamContent.length <= previousStreamContent.length)
+ return;
+
+ proc_stream.write(streamContent.substr(previousStreamContent.length));
+ previousStreamContent = streamContent;
+ }
+
+ function escape(str) {
+ return (str+'').replace(/([\\"'])/g, "\\$1").replace(/\0/g, "\\0");
+ }
+
+ if (fs.existsSync(scriptFile)) common.unlinkSync(scriptFile);
+ if (fs.existsSync(stdoutFile)) common.unlinkSync(stdoutFile);
+ if (fs.existsSync(stderrFile)) common.unlinkSync(stderrFile);
+ if (fs.existsSync(codeFile)) common.unlinkSync(codeFile);
+
+ var execCommand = '"'+process.execPath+'" '+scriptFile;
+ var execOptions = {
+ env: process.env,
+ cwd: _pwd(),
+ maxBuffer: 20*1024*1024
+ };
+
+ var script;
+
+ if (typeof child.execSync === 'function') {
+ script = [
+ "var child = require('child_process')",
+ " , fs = require('fs');",
+ "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
+ " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
+ "});",
+ "var stdoutStream = fs.createWriteStream('"+escape(stdoutFile)+"');",
+ "var stderrStream = fs.createWriteStream('"+escape(stderrFile)+"');",
+ "childProcess.stdout.pipe(stdoutStream, {end: false});",
+ "childProcess.stderr.pipe(stderrStream, {end: false});",
+ "childProcess.stdout.pipe(process.stdout);",
+ "childProcess.stderr.pipe(process.stderr);",
+ "var stdoutEnded = false, stderrEnded = false;",
+ "function tryClosingStdout(){ if(stdoutEnded){ stdoutStream.end(); } }",
+ "function tryClosingStderr(){ if(stderrEnded){ stderrStream.end(); } }",
+ "childProcess.stdout.on('end', function(){ stdoutEnded = true; tryClosingStdout(); });",
+ "childProcess.stderr.on('end', function(){ stderrEnded = true; tryClosingStderr(); });"
+ ].join('\n');
+
+ fs.writeFileSync(scriptFile, script);
+
+ if (options.silent) {
+ execOptions.stdio = 'ignore';
+ } else {
+ execOptions.stdio = [0, 1, 2];
+ }
+
+ // Welcome to the future
+ child.execSync(execCommand, execOptions);
+ } else {
+ cmd += ' > '+stdoutFile+' 2> '+stderrFile; // works on both win/unix
+
+ script = [
+ "var child = require('child_process')",
+ " , fs = require('fs');",
+ "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
+ " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
+ "});"
+ ].join('\n');
+
+ fs.writeFileSync(scriptFile, script);
+
+ child.exec(execCommand, execOptions);
+
+ // The wait loop
+ // sleepFile is used as a dummy I/O op to mitigate unnecessary CPU usage
+ // (tried many I/O sync ops, writeFileSync() seems to be only one that is effective in reducing
+ // CPU usage, though apparently not so much on Windows)
+ while (!fs.existsSync(codeFile)) { updateStream(stdoutFile); fs.writeFileSync(sleepFile, 'a'); }
+ while (!fs.existsSync(stdoutFile)) { updateStream(stdoutFile); fs.writeFileSync(sleepFile, 'a'); }
+ while (!fs.existsSync(stderrFile)) { updateStream(stderrFile); fs.writeFileSync(sleepFile, 'a'); }
+ }
+
+ // At this point codeFile exists, but it's not necessarily flushed yet.
+ // Keep reading it until it is.
+ var code = parseInt('', 10);
+ while (isNaN(code)) {
+ code = parseInt(fs.readFileSync(codeFile, 'utf8'), 10);
+ }
+
+ var stdout = fs.readFileSync(stdoutFile, 'utf8');
+ var stderr = fs.readFileSync(stderrFile, 'utf8');
+
+ // No biggie if we can't erase the files now -- they're in a temp dir anyway
+ try { common.unlinkSync(scriptFile); } catch(e) {}
+ try { common.unlinkSync(stdoutFile); } catch(e) {}
+ try { common.unlinkSync(stderrFile); } catch(e) {}
+ try { common.unlinkSync(codeFile); } catch(e) {}
+ try { common.unlinkSync(sleepFile); } catch(e) {}
+
+ // some shell return codes are defined as errors, per http://tldp.org/LDP/abs/html/exitcodes.html
+ if (code === 1 || code === 2 || code >= 126) {
+ common.error('', true); // unix/shell doesn't really give an error message after non-zero exit codes
+ }
+ // True if successful, false if not
+ var obj = {
+ code: code,
+ output: stdout, // deprecated
+ stdout: stdout,
+ stderr: stderr
+ };
+ return obj;
+} // execSync()
+
+// Wrapper around exec() to enable echoing output to console in real time
+function execAsync(cmd, opts, callback) {
+ var stdout = '';
+ var stderr = '';
+
+ var options = common.extend({
+ silent: common.config.silent
+ }, opts);
+
+ var c = child.exec(cmd, {env: process.env, maxBuffer: 20*1024*1024}, function(err) {
+ if (callback)
+ callback(err ? err.code : 0, stdout, stderr);
+ });
+
+ c.stdout.on('data', function(data) {
+ stdout += data;
+ if (!options.silent)
+ process.stdout.write(data);
+ });
+
+ c.stderr.on('data', function(data) {
+ stderr += data;
+ if (!options.silent)
+ process.stderr.write(data);
+ });
+
+ return c;
+}
+
+//@
+//@ ### exec(command [, options] [, callback])
+//@ Available options (all `false` by default):
+//@
+//@ + `async`: Asynchronous execution. If a callback is provided, it will be set to
+//@ `true`, regardless of the passed value.
+//@ + `silent`: Do not echo program output to console.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var version = exec('node --version', {silent:true}).stdout;
+//@
+//@ var child = exec('some_long_running_process', {async:true});
+//@ child.stdout.on('data', function(data) {
+//@ /* ... do something with data ... */
+//@ });
+//@
+//@ exec('some_long_running_process', function(code, stdout, stderr) {
+//@ console.log('Exit code:', code);
+//@ console.log('Program output:', stdout);
+//@ console.log('Program stderr:', stderr);
+//@ });
+//@ ```
+//@
+//@ Executes the given `command` _synchronously_, unless otherwise specified. When in synchronous
+//@ mode returns the object `{ code:..., stdout:... , stderr:... }`, containing the program's
+//@ `stdout`, `stderr`, and its exit `code`. Otherwise returns the child process object,
+//@ and the `callback` gets the arguments `(code, stdout, stderr)`.
+//@
+//@ **Note:** For long-lived processes, it's best to run `exec()` asynchronously as
+//@ the current synchronous implementation uses a lot of CPU. This should be getting
+//@ fixed soon.
+function _exec(command, options, callback) {
+ if (!command)
+ common.error('must specify command');
+
+ // Callback is defined instead of options.
+ if (typeof options === 'function') {
+ callback = options;
+ options = { async: true };
+ }
+
+ // Callback is defined with options.
+ if (typeof options === 'object' && typeof callback === 'function') {
+ options.async = true;
+ }
+
+ options = common.extend({
+ silent: common.config.silent,
+ async: false
+ }, options);
+
+ if (options.async)
+ return execAsync(command, options, callback);
+ else
+ return execSync(command, options);
+}
+module.exports = _exec;
+
+var fs = require('fs');
+var common = require('./common');
+var _ls = require('./ls');
+
+//@
+//@ ### find(path [, path ...])
+//@ ### find(path_array)
+//@ Examples:
+//@
+//@ ```javascript
+//@ find('src', 'lib');
+//@ find(['src', 'lib']); // same as above
+//@ find('.').filter(function(file) { return file.match(/\.js$/); });
+//@ ```
+//@
+//@ Returns array of all files (however deep) in the given paths.
+//@
+//@ The main difference from `ls('-R', path)` is that the resulting file names
+//@ include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
+function _find(options, paths) {
+ if (!paths)
+ common.error('no path specified');
+ else if (typeof paths === 'object')
+ paths = paths; // assume array
+ else if (typeof paths === 'string')
+ paths = [].slice.call(arguments, 1);
+
+ var list = [];
+
+ function pushFile(file) {
+ if (common.platform === 'win')
+ file = file.replace(/\\/g, '/');
+ list.push(file);
+ }
+
+ // why not simply do ls('-R', paths)? because the output wouldn't give the base dirs
+ // to get the base dir in the output, we need instead ls('-R', 'dir/*') for every directory
+
+ paths.forEach(function(file) {
+ pushFile(file);
+
+ if (fs.statSync(file).isDirectory()) {
+ _ls('-RA', file+'/*').forEach(function(subfile) {
+ pushFile(subfile);
+ });
+ }
+ });
+
+ return list;
+}
+module.exports = _find;
+
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### grep([options,] regex_filter, file [, file ...])
+//@ ### grep([options,] regex_filter, file_array)
+//@ Available options:
+//@
+//@ + `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ grep('-v', 'GLOBAL_VARIABLE', '*.js');
+//@ grep('GLOBAL_VARIABLE', '*.js');
+//@ ```
+//@
+//@ Reads input string from given files and returns a string containing all lines of the
+//@ file that match the given `regex_filter`. Wildcard `*` accepted.
+function _grep(options, regex, files) {
+ options = common.parseOptions(options, {
+ 'v': 'inverse'
+ });
+
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 2);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ var grep = '';
+ files.forEach(function(file) {
+ if (!fs.existsSync(file)) {
+ common.error('no such file or directory: ' + file, true);
+ return;
+ }
+
+ var contents = fs.readFileSync(file, 'utf8'),
+ lines = contents.split(/\r*\n/);
+ lines.forEach(function(line) {
+ var matched = line.match(regex);
+ if ((options.inverse && !matched) || (!options.inverse && matched))
+ grep += line + '\n';
+ });
+ });
+
+ return common.ShellString(grep);
+}
+module.exports = _grep;
+
+var fs = require('fs');
+var path = require('path');
+var common = require('./common');
+
+//@
+//@ ### ln([options,] source, dest)
+//@ Available options:
+//@
+//@ + `-s`: symlink
+//@ + `-f`: force
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ ln('file', 'newlink');
+//@ ln('-sf', 'file', 'existing');
+//@ ```
+//@
+//@ Links source to dest. Use -f to force the link, should dest already exist.
+function _ln(options, source, dest) {
+ options = common.parseOptions(options, {
+ 's': 'symlink',
+ 'f': 'force'
+ });
+
+ if (!source || !dest) {
+ common.error('Missing <source> and/or <dest>');
+ }
+
+ source = String(source);
+ var sourcePath = path.normalize(source).replace(RegExp(path.sep + '$'), '');
+ var isAbsolute = (path.resolve(source) === sourcePath);
+ dest = path.resolve(process.cwd(), String(dest));
+
+ if (fs.existsSync(dest)) {
+ if (!options.force) {
+ common.error('Destination file exists', true);
+ }
+
+ fs.unlinkSync(dest);
+ }
+
+ if (options.symlink) {
+ var isWindows = common.platform === 'win';
+ var linkType = isWindows ? 'file' : null;
+ var resolvedSourcePath = isAbsolute ? sourcePath : path.resolve(process.cwd(), path.dirname(dest), source);
+ if (!fs.existsSync(resolvedSourcePath)) {
+ common.error('Source file does not exist', true);
+ } else if (isWindows && fs.statSync(resolvedSourcePath).isDirectory()) {
+ linkType = 'junction';
+ }
+
+ try {
+ fs.symlinkSync(linkType === 'junction' ? resolvedSourcePath: source, dest, linkType);
+ } catch (err) {
+ common.error(err.message);
+ }
+ } else {
+ if (!fs.existsSync(source)) {
+ common.error('Source file does not exist', true);
+ }
+ try {
+ fs.linkSync(source, dest);
+ } catch (err) {
+ common.error(err.message);
+ }
+ }
+}
+module.exports = _ln;
+
+var path = require('path');
+var fs = require('fs');
+var common = require('./common');
+var _cd = require('./cd');
+var _pwd = require('./pwd');
+
+//@
+//@ ### ls([options,] [path, ...])
+//@ ### ls([options,] path_array)
+//@ Available options:
+//@
+//@ + `-R`: recursive
+//@ + `-A`: all files (include files beginning with `.`, except for `.` and `..`)
+//@ + `-d`: list directories themselves, not their contents
+//@ + `-l`: list objects representing each file, each with fields containing `ls
+//@ -l` output fields. See
+//@ [fs.Stats](https://nodejs.org/api/fs.html#fs_class_fs_stats)
+//@ for more info
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ ls('projs/*.js');
+//@ ls('-R', '/users/me', '/tmp');
+//@ ls('-R', ['/users/me', '/tmp']); // same as above
+//@ ls('-l', 'file.txt'); // { name: 'file.txt', mode: 33188, nlink: 1, ...}
+//@ ```
+//@
+//@ Returns array of files in the given path, or in current directory if no path provided.
+function _ls(options, paths) {
+ options = common.parseOptions(options, {
+ 'R': 'recursive',
+ 'A': 'all',
+ 'a': 'all_deprecated',
+ 'd': 'directory',
+ 'l': 'long'
+ });
+
+ if (options.all_deprecated) {
+ // We won't support the -a option as it's hard to image why it's useful
+ // (it includes '.' and '..' in addition to '.*' files)
+ // For backwards compatibility we'll dump a deprecated message and proceed as before
+ common.log('ls: Option -a is deprecated. Use -A instead');
+ options.all = true;
+ }
+
+ if (!paths)
+ paths = ['.'];
+ else if (typeof paths === 'object')
+ paths = paths; // assume array
+ else if (typeof paths === 'string')
+ paths = [].slice.call(arguments, 1);
+
+ var list = [];
+
+ // Conditionally pushes file to list - returns true if pushed, false otherwise
+ // (e.g. prevents hidden files to be included unless explicitly told so)
+ function pushFile(file, query) {
+ var name = file.name || file;
+ // hidden file?
+ if (path.basename(name)[0] === '.') {
+ // not explicitly asking for hidden files?
+ if (!options.all && !(path.basename(query)[0] === '.' && path.basename(query).length > 1))
+ return false;
+ }
+
+ if (common.platform === 'win')
+ name = name.replace(/\\/g, '/');
+
+ if (file.name) {
+ file.name = name;
+ } else {
+ file = name;
+ }
+ list.push(file);
+ return true;
+ }
+
+ paths.forEach(function(p) {
+ if (fs.existsSync(p)) {
+ var stats = ls_stat(p);
+ // Simple file?
+ if (stats.isFile()) {
+ if (options.long) {
+ pushFile(stats, p);
+ } else {
+ pushFile(p, p);
+ }
+ return; // continue
+ }
+
+ // Simple dir?
+ if (options.directory) {
+ pushFile(p, p);
+ return;
+ } else if (stats.isDirectory()) {
+ // Iterate over p contents
+ fs.readdirSync(p).forEach(function(file) {
+ var orig_file = file;
+ if (options.long)
+ file = ls_stat(path.join(p, file));
+ if (!pushFile(file, p))
+ return;
+
+ // Recursive?
+ if (options.recursive) {
+ var oldDir = _pwd();
+ _cd('', p);
+ if (fs.statSync(orig_file).isDirectory())
+ list = list.concat(_ls('-R'+(options.all?'A':''), orig_file+'/*'));
+ _cd('', oldDir);
+ }
+ });
+ return; // continue
+ }
+ }
+
+ // p does not exist - possible wildcard present
+
+ var basename = path.basename(p);
+ var dirname = path.dirname(p);
+ // Wildcard present on an existing dir? (e.g. '/tmp/*.js')
+ if (basename.search(/\*/) > -1 && fs.existsSync(dirname) && fs.statSync(dirname).isDirectory) {
+ // Escape special regular expression chars
+ var regexp = basename.replace(/(\^|\$|\(|\)|<|>|\[|\]|\{|\}|\.|\+|\?)/g, '\\$1');
+ // Translates wildcard into regex
+ regexp = '^' + regexp.replace(/\*/g, '.*') + '$';
+ // Iterate over directory contents
+ fs.readdirSync(dirname).forEach(function(file) {
+ if (file.match(new RegExp(regexp))) {
+ var file_path = path.join(dirname, file);
+ file_path = options.long ? ls_stat(file_path) : file_path;
+ if (file_path.name)
+ file_path.name = path.normalize(file_path.name);
+ else
+ file_path = path.normalize(file_path);
+ if (!pushFile(file_path, basename))
+ return;
+
+ // Recursive?
+ if (options.recursive) {
+ var pp = dirname + '/' + file;
+ if (fs.lstatSync(pp).isDirectory())
+ list = list.concat(_ls('-R'+(options.all?'A':''), pp+'/*'));
+ } // recursive
+ } // if file matches
+ }); // forEach
+ return;
+ }
+
+ common.error('no such file or directory: ' + p, true);
+ });
+
+ return list;
+}
+module.exports = _ls;
+
+
+function ls_stat(path) {
+ var stats = fs.statSync(path);
+ // Note: this object will contain more information than .toString() returns
+ stats.name = path;
+ stats.toString = function() {
+ // Return a string resembling unix's `ls -l` format
+ return [this.mode, this.nlink, this.uid, this.gid, this.size, this.mtime, this.name].join(' ');
+ };
+ return stats;
+}
+
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+// Recursively creates 'dir'
+function mkdirSyncRecursive(dir) {
+ var baseDir = path.dirname(dir);
+
+ // Base dir exists, no recursion necessary
+ if (fs.existsSync(baseDir)) {
+ fs.mkdirSync(dir, parseInt('0777', 8));
+ return;
+ }
+
+ // Base dir does not exist, go recursive
+ mkdirSyncRecursive(baseDir);
+
+ // Base dir created, can create dir
+ fs.mkdirSync(dir, parseInt('0777', 8));
+}
+
+//@
+//@ ### mkdir([options,] dir [, dir ...])
+//@ ### mkdir([options,] dir_array)
+//@ Available options:
+//@
+//@ + `-p`: full path (will create intermediate dirs if necessary)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
+//@ mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
+//@ ```
+//@
+//@ Creates directories.
+function _mkdir(options, dirs) {
+ options = common.parseOptions(options, {
+ 'p': 'fullpath'
+ });
+ if (!dirs)
+ common.error('no paths given');
+
+ if (typeof dirs === 'string')
+ dirs = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ dirs.forEach(function(dir) {
+ if (fs.existsSync(dir)) {
+ if (!options.fullpath)
+ common.error('path already exists: ' + dir, true);
+ return; // skip dir
+ }
+
+ // Base dir does not exist, and no -p option given
+ var baseDir = path.dirname(dir);
+ if (!fs.existsSync(baseDir) && !options.fullpath) {
+ common.error('no such file or directory: ' + baseDir, true);
+ return; // skip dir
+ }
+
+ if (options.fullpath)
+ mkdirSyncRecursive(dir);
+ else
+ fs.mkdirSync(dir, parseInt('0777', 8));
+ });
+} // mkdir
+module.exports = _mkdir;
+
+var fs = require('fs');
+var path = require('path');
+var common = require('./common');
+
+//@
+//@ ### mv([options ,] source [, source ...], dest')
+//@ ### mv([options ,] source_array, dest')
+//@ Available options:
+//@
+//@ + `-f`: force (default behavior)
+//@ + `-n`: no-clobber
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ mv('-n', 'file', 'dir/');
+//@ mv('file1', 'file2', 'dir/');
+//@ mv(['file1', 'file2'], 'dir/'); // same as above
+//@ ```
+//@
+//@ Moves files. The wildcard `*` is accepted.
+function _mv(options, sources, dest) {
+ options = common.parseOptions(options, {
+ 'f': '!no_force',
+ 'n': 'no_force'
+ });
+
+ // Get sources, dest
+ if (arguments.length < 3) {
+ common.error('missing <source> and/or <dest>');
+ } else if (arguments.length > 3) {
+ sources = [].slice.call(arguments, 1, arguments.length - 1);
+ dest = arguments[arguments.length - 1];
+ } else if (typeof sources === 'string') {
+ sources = [sources];
+ } else if ('length' in sources) {
+ sources = sources; // no-op for array
+ } else {
+ common.error('invalid arguments');
+ }
+
+ sources = common.expand(sources);
+
+ var exists = fs.existsSync(dest),
+ stats = exists && fs.statSync(dest);
+
+ // Dest is not existing dir, but multiple sources given
+ if ((!exists || !stats.isDirectory()) && sources.length > 1)
+ common.error('dest is not a directory (too many sources)');
+
+ // Dest is an existing file, but no -f given
+ if (exists && stats.isFile() && options.no_force)
+ common.error('dest file already exists: ' + dest);
+
+ sources.forEach(function(src) {
+ if (!fs.existsSync(src)) {
+ common.error('no such file or directory: '+src, true);
+ return; // skip file
+ }
+
+ // If here, src exists
+
+ // When copying to '/path/dir':
+ // thisDest = '/path/dir/file1'
+ var thisDest = dest;
+ if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
+ thisDest = path.normalize(dest + '/' + path.basename(src));
+
+ if (fs.existsSync(thisDest) && options.no_force) {
+ common.error('dest file already exists: ' + thisDest, true);
+ return; // skip file
+ }
+
+ if (path.resolve(src) === path.dirname(path.resolve(thisDest))) {
+ common.error('cannot move to self: '+src, true);
+ return; // skip file
+ }
+
+ fs.renameSync(src, thisDest);
+ }); // forEach(src)
+} // mv
+module.exports = _mv;
+
+// see dirs.js
+// see dirs.js
+var path = require('path');
+var common = require('./common');
+
+//@
+//@ ### pwd()
+//@ Returns the current directory.
+function _pwd() {
+ var pwd = path.resolve(process.cwd());
+ return common.ShellString(pwd);
+}
+module.exports = _pwd;
+
+var common = require('./common');
+var fs = require('fs');
+
+// Recursively removes 'dir'
+// Adapted from https://github.com/ryanmcgrath/wrench-js
+//
+// Copyright (c) 2010 Ryan McGrath
+// Copyright (c) 2012 Artur Adib
+//
+// Licensed under the MIT License
+// http://www.opensource.org/licenses/mit-license.php
+function rmdirSyncRecursive(dir, force) {
+ var files;
+
+ files = fs.readdirSync(dir);
+
+ // Loop through and delete everything in the sub-tree after checking it
+ for(var i = 0; i < files.length; i++) {
+ var file = dir + "/" + files[i],
+ currFile = fs.lstatSync(file);
+
+ if(currFile.isDirectory()) { // Recursive function back to the beginning
+ rmdirSyncRecursive(file, force);
+ }
+
+ else if(currFile.isSymbolicLink()) { // Unlink symlinks
+ if (force || isWriteable(file)) {
+ try {
+ common.unlinkSync(file);
+ } catch (e) {
+ common.error('could not remove file (code '+e.code+'): ' + file, true);
+ }
+ }
+ }
+
+ else // Assume it's a file - perhaps a try/catch belongs here?
+ if (force || isWriteable(file)) {
+ try {
+ common.unlinkSync(file);
+ } catch (e) {
+ common.error('could not remove file (code '+e.code+'): ' + file, true);
+ }
+ }
+ }
+
+ // Now that we know everything in the sub-tree has been deleted, we can delete the main directory.
+ // Huzzah for the shopkeep.
+
+ var result;
+ try {
+ // Retry on windows, sometimes it takes a little time before all the files in the directory are gone
+ var start = Date.now();
+ while (true) {
+ try {
+ result = fs.rmdirSync(dir);
+ if (fs.existsSync(dir)) throw { code: "EAGAIN" };
+ break;
+ } catch(er) {
+ // In addition to error codes, also check if the directory still exists and loop again if true
+ if (process.platform === "win32" && (er.code === "ENOTEMPTY" || er.code === "EBUSY" || er.code === "EPERM" || er.code === "EAGAIN")) {
+ if (Date.now() - start > 1000) throw er;
+ } else if (er.code === "ENOENT") {
+ // Directory did not exist, deletion was successful
+ break;
+ } else {
+ throw er;
+ }
+ }
+ }
+ } catch(e) {
+ common.error('could not remove directory (code '+e.code+'): ' + dir, true);
+ }
+
+ return result;
+} // rmdirSyncRecursive
+
+// Hack to determine if file has write permissions for current user
+// Avoids having to check user, group, etc, but it's probably slow
+function isWriteable(file) {
+ var writePermission = true;
+ try {
+ var __fd = fs.openSync(file, 'a');
+ fs.closeSync(__fd);
+ } catch(e) {
+ writePermission = false;
+ }
+
+ return writePermission;
+}
+
+//@
+//@ ### rm([options,] file [, file ...])
+//@ ### rm([options,] file_array)
+//@ Available options:
+//@
+//@ + `-f`: force
+//@ + `-r, -R`: recursive
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ rm('-rf', '/tmp/*');
+//@ rm('some_file.txt', 'another_file.txt');
+//@ rm(['some_file.txt', 'another_file.txt']); // same as above
+//@ ```
+//@
+//@ Removes files. The wildcard `*` is accepted.
+function _rm(options, files) {
+ options = common.parseOptions(options, {
+ 'f': 'force',
+ 'r': 'recursive',
+ 'R': 'recursive'
+ });
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ files.forEach(function(file) {
+ if (!fs.existsSync(file)) {
+ // Path does not exist, no force flag given
+ if (!options.force)
+ common.error('no such file or directory: '+file, true);
+
+ return; // skip file
+ }
+
+ // If here, path exists
+
+ var stats = fs.lstatSync(file);
+ if (stats.isFile() || stats.isSymbolicLink()) {
+
+ // Do not check for file writing permissions
+ if (options.force) {
+ common.unlinkSync(file);
+ return;
+ }
+
+ if (isWriteable(file))
+ common.unlinkSync(file);
+ else
+ common.error('permission denied: '+file, true);
+
+ return;
+ } // simple file
+
+ // Path is an existing directory, but no -r flag given
+ if (stats.isDirectory() && !options.recursive) {
+ common.error('path is a directory', true);
+ return; // skip path
+ }
+
+ // Recursively remove existing directory
+ if (stats.isDirectory() && options.recursive) {
+ rmdirSyncRecursive(file, options.force);
+ }
+ }); // forEach(file)
+} // rm
+module.exports = _rm;
+
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### sed([options,] search_regex, replacement, file [, file ...])
+//@ ### sed([options,] search_regex, replacement, file_array)
+//@ Available options:
+//@
+//@ + `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
+//@ sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
+//@ ```
+//@
+//@ Reads an input string from `files` and performs a JavaScript `replace()` on the input
+//@ using the given search regex and replacement string or function. Returns the new string after replacement.
+function _sed(options, regex, replacement, files) {
+ options = common.parseOptions(options, {
+ 'i': 'inplace'
+ });
+
+ if (typeof replacement === 'string' || typeof replacement === 'function')
+ replacement = replacement; // no-op
+ else if (typeof replacement === 'number')
+ replacement = replacement.toString(); // fallback
+ else
+ common.error('invalid replacement string');
+
+ // Convert all search strings to RegExp
+ if (typeof regex === 'string')
+ regex = RegExp(regex);
+
+ if (!files)
+ common.error('no files given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 3);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ var sed = [];
+ files.forEach(function(file) {
+ if (!fs.existsSync(file)) {
+ common.error('no such file or directory: ' + file, true);
+ return;
+ }
+
+ var result = fs.readFileSync(file, 'utf8').split('\n').map(function (line) {
+ return line.replace(regex, replacement);
+ }).join('\n');
+
+ sed.push(result);
+
+ if (options.inplace)
+ fs.writeFileSync(file, result, 'utf8');
+ });
+
+ return common.ShellString(sed.join('\n'));
+}
+module.exports = _sed;
+
+var common = require('./common');
+
+//@
+//@ ### set(options)
+//@ Available options:
+//@
+//@ + `+/-e`: exit upon error (`config.fatal`)
+//@ + `+/-v`: verbose: show all commands (`config.verbose`)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ set('-e'); // exit upon first error
+//@ set('+e'); // this undoes a "set('-e')"
+//@ ```
+//@
+//@ Sets global configuration variables
+function _set(options) {
+ if (!options) {
+ var args = [].slice.call(arguments, 0);
+ if (args.length < 2)
+ common.error('must provide an argument');
+ options = args[1];
+ }
+ var negate = (options[0] === '+');
+ if (negate) {
+ options = '-' + options.slice(1); // parseOptions needs a '-' prefix
+ }
+ options = common.parseOptions(options, {
+ 'e': 'fatal',
+ 'v': 'verbose'
+ });
+
+ var key;
+ if (negate) {
+ for (key in options)
+ options[key] = !options[key];
+ }
+
+ for (key in options) {
+ // Only change the global config if `negate` is false and the option is true
+ // or if `negate` is true and the option is false (aka negate !== option)
+ if (negate !== options[key]) {
+ common.config[key] = options[key];
+ }
+ }
+ return;
+}
+module.exports = _set;
+
+var common = require('./common');
+var os = require('os');
+var fs = require('fs');
+
+// Returns false if 'dir' is not a writeable directory, 'dir' otherwise
+function writeableDir(dir) {
+ if (!dir || !fs.existsSync(dir))
+ return false;
+
+ if (!fs.statSync(dir).isDirectory())
+ return false;
+
+ var testFile = dir+'/'+common.randomFileName();
+ try {
+ fs.writeFileSync(testFile, ' ');
+ common.unlinkSync(testFile);
+ return dir;
+ } catch (e) {
+ return false;
+ }
+}
+
+
+//@
+//@ ### tempdir()
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var tmp = tempdir(); // "/tmp" for most *nix platforms
+//@ ```
+//@
+//@ Searches and returns string containing a writeable, platform-dependent temporary directory.
+//@ Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
+function _tempDir() {
+ var state = common.state;
+ if (state.tempDir)
+ return state.tempDir; // from cache
+
+ state.tempDir = writeableDir(os.tmpdir && os.tmpdir()) || // node 0.10+
+ writeableDir(os.tmpDir && os.tmpDir()) || // node 0.8+
+ writeableDir(process.env['TMPDIR']) ||
+ writeableDir(process.env['TEMP']) ||
+ writeableDir(process.env['TMP']) ||
+ writeableDir(process.env['Wimp$ScrapDir']) || // RiscOS
+ writeableDir('C:\\TEMP') || // Windows
+ writeableDir('C:\\TMP') || // Windows
+ writeableDir('\\TEMP') || // Windows
+ writeableDir('\\TMP') || // Windows
+ writeableDir('/tmp') ||
+ writeableDir('/var/tmp') ||
+ writeableDir('/usr/tmp') ||
+ writeableDir('.'); // last resort
+
+ return state.tempDir;
+}
+module.exports = _tempDir;
+
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### test(expression)
+//@ Available expression primaries:
+//@
+//@ + `'-b', 'path'`: true if path is a block device
+//@ + `'-c', 'path'`: true if path is a character device
+//@ + `'-d', 'path'`: true if path is a directory
+//@ + `'-e', 'path'`: true if path exists
+//@ + `'-f', 'path'`: true if path is a regular file
+//@ + `'-L', 'path'`: true if path is a symbolic link
+//@ + `'-p', 'path'`: true if path is a pipe (FIFO)
+//@ + `'-S', 'path'`: true if path is a socket
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ if (test('-d', path)) { /* do something with dir */ };
+//@ if (!test('-f', path)) continue; // skip if it's a regular file
+//@ ```
+//@
+//@ Evaluates expression using the available primaries and returns corresponding value.
+function _test(options, path) {
+ if (!path)
+ common.error('no path given');
+
+ // hack - only works with unary primaries
+ options = common.parseOptions(options, {
+ 'b': 'block',
+ 'c': 'character',
+ 'd': 'directory',
+ 'e': 'exists',
+ 'f': 'file',
+ 'L': 'link',
+ 'p': 'pipe',
+ 'S': 'socket'
+ });
+
+ var canInterpret = false;
+ for (var key in options)
+ if (options[key] === true) {
+ canInterpret = true;
+ break;
+ }
+
+ if (!canInterpret)
+ common.error('could not interpret expression');
+
+ if (options.link) {
+ try {
+ return fs.lstatSync(path).isSymbolicLink();
+ } catch(e) {
+ return false;
+ }
+ }
+
+ if (!fs.existsSync(path))
+ return false;
+
+ if (options.exists)
+ return true;
+
+ var stats = fs.statSync(path);
+
+ if (options.block)
+ return stats.isBlockDevice();
+
+ if (options.character)
+ return stats.isCharacterDevice();
+
+ if (options.directory)
+ return stats.isDirectory();
+
+ if (options.file)
+ return stats.isFile();
+
+ if (options.pipe)
+ return stats.isFIFO();
+
+ if (options.socket)
+ return stats.isSocket();
+} // test
+module.exports = _test;
+
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+//@
+//@ ### 'string'.to(file)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ cat('input.txt').to('output.txt');
+//@ ```
+//@
+//@ Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
+//@ those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
+function _to(options, file) {
+ if (!file)
+ common.error('wrong arguments');
+
+ if (!fs.existsSync( path.dirname(file) ))
+ common.error('no such file or directory: ' + path.dirname(file));
+
+ try {
+ fs.writeFileSync(file, this.toString(), 'utf8');
+ return this;
+ } catch(e) {
+ common.error('could not write to file (code '+e.code+'): '+file, true);
+ }
+}
+module.exports = _to;
+
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+//@
+//@ ### 'string'.toEnd(file)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ cat('input.txt').toEnd('output.txt');
+//@ ```
+//@
+//@ Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
+//@ those returned by `cat`, `grep`, etc).
+function _toEnd(options, file) {
+ if (!file)
+ common.error('wrong arguments');
+
+ if (!fs.existsSync( path.dirname(file) ))
+ common.error('no such file or directory: ' + path.dirname(file));
+
+ try {
+ fs.appendFileSync(file, this.toString(), 'utf8');
+ return this;
+ } catch(e) {
+ common.error('could not append to file (code '+e.code+'): '+file, true);
+ }
+}
+module.exports = _toEnd;
+
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### touch([options,] file)
+//@ Available options:
+//@
+//@ + `-a`: Change only the access time
+//@ + `-c`: Do not create any files
+//@ + `-m`: Change only the modification time
+//@ + `-d DATE`: Parse DATE and use it instead of current time
+//@ + `-r FILE`: Use FILE's times instead of current time
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ touch('source.js');
+//@ touch('-c', '/path/to/some/dir/source.js');
+//@ touch({ '-r': FILE }, '/path/to/some/dir/source.js');
+//@ ```
+//@
+//@ Update the access and modification times of each FILE to the current time.
+//@ A FILE argument that does not exist is created empty, unless -c is supplied.
+//@ This is a partial implementation of *[touch(1)](http://linux.die.net/man/1/touch)*.
+function _touch(opts, files) {
+ opts = common.parseOptions(opts, {
+ 'a': 'atime_only',
+ 'c': 'no_create',
+ 'd': 'date',
+ 'm': 'mtime_only',
+ 'r': 'reference',
+ });
+
+ if (!files) {
+ common.error('no paths given');
+ }
+
+
+ if (Array.isArray(files)) {
+ files.forEach(function(f) {
+ touchFile(opts, f);
+ });
+ } else if (typeof files === 'string') {
+ touchFile(opts, files);
+ } else {
+ common.error('file arg should be a string file path or an Array of string file paths');
+ }
+
+}
+
+function touchFile(opts, file) {
+ var stat = tryStatFile(file);
+
+ if (stat && stat.isDirectory()) {
+ // don't error just exit
+ return;
+ }
+
+ // if the file doesn't already exist and the user has specified --no-create then
+ // this script is finished
+ if (!stat && opts.no_create) {
+ return;
+ }
+
+ // open the file and then close it. this will create it if it doesn't exist but will
+ // not truncate the file
+ fs.closeSync(fs.openSync(file, 'a'));
+
+ //
+ // Set timestamps
+ //
+
+ // setup some defaults
+ var now = new Date();
+ var mtime = opts.date || now;
+ var atime = opts.date || now;
+
+ // use reference file
+ if (opts.reference) {
+ var refStat = tryStatFile(opts.reference);
+ if (!refStat) {
+ common.error('failed to get attributess of ' + opts.reference);
+ }
+ mtime = refStat.mtime;
+ atime = refStat.atime;
+ } else if (opts.date) {
+ mtime = opts.date;
+ atime = opts.date;
+ }
+
+ if (opts.atime_only && opts.mtime_only) {
+ // keep the new values of mtime and atime like GNU
+ } else if (opts.atime_only) {
+ mtime = stat.mtime;
+ } else if (opts.mtime_only) {
+ atime = stat.atime;
+ }
+
+ fs.utimesSync(file, atime, mtime);
+}
+
+module.exports = _touch;
+
+function tryStatFile(filePath) {
+ try {
+ return fs.statSync(filePath);
+ } catch (e) {
+ return null;
+ }
+}
+
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+// XP's system default value for PATHEXT system variable, just in case it's not
+// set on Windows.
+var XP_DEFAULT_PATHEXT = '.com;.exe;.bat;.cmd;.vbs;.vbe;.js;.jse;.wsf;.wsh';
+
+// Cross-platform method for splitting environment PATH variables
+function splitPath(p) {
+ if (!p)
+ return [];
+
+ if (common.platform === 'win')
+ return p.split(';');
+ else
+ return p.split(':');
+}
+
+function checkPath(path) {
+ return fs.existsSync(path) && !fs.statSync(path).isDirectory();
+}
+
+//@
+//@ ### which(command)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var nodeExec = which('node');
+//@ ```
+//@
+//@ Searches for `command` in the system's PATH. On Windows, this uses the
+//@ `PATHEXT` variable to append the extension if it's not already executable.
+//@ Returns string containing the absolute path to the command.
+function _which(options, cmd) {
+ if (!cmd)
+ common.error('must specify command');
+
+ var pathEnv = process.env.path || process.env.Path || process.env.PATH,
+ pathArray = splitPath(pathEnv),
+ where = null;
+
+ // No relative/absolute paths provided?
+ if (cmd.search(/\//) === -1) {
+ // Search for command in PATH
+ pathArray.forEach(function(dir) {
+ if (where)
+ return; // already found it
+
+ var attempt = path.resolve(dir, cmd);
+
+ if (common.platform === 'win') {
+ attempt = attempt.toUpperCase();
+
+ // In case the PATHEXT variable is somehow not set (e.g.
+ // child_process.spawn with an empty environment), use the XP default.
+ var pathExtEnv = process.env.PATHEXT || XP_DEFAULT_PATHEXT;
+ var pathExtArray = splitPath(pathExtEnv.toUpperCase());
+ var i;
+
+ // If the extension is already in PATHEXT, just return that.
+ for (i = 0; i < pathExtArray.length; i++) {
+ var ext = pathExtArray[i];
+ if (attempt.slice(-ext.length) === ext && checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ }
+
+ // Cycle through the PATHEXT variable
+ var baseAttempt = attempt;
+ for (i = 0; i < pathExtArray.length; i++) {
+ attempt = baseAttempt + pathExtArray[i];
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ }
+ } else {
+ // Assume it's Unix-like
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ }
+ });
+ }
+
+ // Command not found anywhere?
+ if (!checkPath(cmd) && !where)
+ return null;
+
+ where = where || path.resolve(cmd);
+
+ return common.ShellString(where);
+}
+module.exports = _which;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/global.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/global.js b/node_modules/shelljs/global.js
new file mode 100644
index 0000000..97f0033
--- /dev/null
+++ b/node_modules/shelljs/global.js
@@ -0,0 +1,3 @@
+var shell = require('./shell.js');
+for (var cmd in shell)
+ global[cmd] = shell[cmd];
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/make.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/make.js b/node_modules/shelljs/make.js
new file mode 100644
index 0000000..a8438c8
--- /dev/null
+++ b/node_modules/shelljs/make.js
@@ -0,0 +1,57 @@
+require('./global');
+
+global.config.fatal = true;
+global.target = {};
+
+var args = process.argv.slice(2),
+ targetArgs,
+ dashesLoc = args.indexOf('--');
+
+// split args, everything after -- if only for targets
+if (dashesLoc > -1) {
+ targetArgs = args.slice(dashesLoc + 1, args.length);
+ args = args.slice(0, dashesLoc);
+}
+
+// This ensures we only execute the script targets after the entire script has
+// been evaluated
+setTimeout(function() {
+ var t;
+
+ if (args.length === 1 && args[0] === '--help') {
+ console.log('Available targets:');
+ for (t in global.target)
+ console.log(' ' + t);
+ return;
+ }
+
+ // Wrap targets to prevent duplicate execution
+ for (t in global.target) {
+ (function(t, oldTarget){
+
+ // Wrap it
+ global.target[t] = function() {
+ if (!oldTarget.done){
+ oldTarget.done = true;
+ oldTarget.result = oldTarget.apply(oldTarget, arguments);
+ }
+ return oldTarget.result;
+ };
+
+ })(t, global.target[t]);
+ }
+
+ // Execute desired targets
+ if (args.length > 0) {
+ args.forEach(function(arg) {
+ if (arg in global.target)
+ global.target[arg](targetArgs);
+ else {
+ console.log('no such target: ' + arg);
+ }
+ });
+ } else if ('all' in global.target) {
+ global.target.all(targetArgs);
+ }
+
+}, 0);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/package.json
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/package.json b/node_modules/shelljs/package.json
new file mode 100644
index 0000000..2d0a809
--- /dev/null
+++ b/node_modules/shelljs/package.json
@@ -0,0 +1,112 @@
+{
+ "_args": [
+ [
+ "shelljs@^0.6.0",
+ "/Users/steveng/repo/cordova/cordova-osx"
+ ]
+ ],
+ "_from": "shelljs@>=0.6.0 <0.7.0",
+ "_id": "shelljs@0.6.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/shelljs",
+ "_nodeVersion": "5.4.0",
+ "_npmOperationalInternal": {
+ "host": "packages-6-west.internal.npmjs.com",
+ "tmp": "tmp/shelljs-0.6.0.tgz_1454632811074_0.5800695188809186"
+ },
+ "_npmUser": {
+ "email": "ari@ariporad.com",
+ "name": "ariporad"
+ },
+ "_npmVersion": "3.6.0",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "shelljs",
+ "raw": "shelljs@^0.6.0",
+ "rawSpec": "^0.6.0",
+ "scope": null,
+ "spec": ">=0.6.0 <0.7.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/"
+ ],
+ "_resolved": "http://registry.npmjs.org/shelljs/-/shelljs-0.6.0.tgz",
+ "_shasum": "ce1ed837b4b0e55b5ec3dab84251ab9dbdc0c7ec",
+ "_shrinkwrap": null,
+ "_spec": "shelljs@^0.6.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx",
+ "author": {
+ "email": "arturadib@gmail.com",
+ "name": "Artur Adib"
+ },
+ "bin": {
+ "shjs": "./bin/shjs"
+ },
+ "bugs": {
+ "url": "https://github.com/shelljs/shelljs/issues"
+ },
+ "contributors": [
+ {
+ "name": "Ari Porad",
+ "email": "ari@ariporad.com",
+ "url": "http://ariporad.com/"
+ },
+ {
+ "name": "Nate Fischer",
+ "email": "ntfschr@gmail.com"
+ }
+ ],
+ "dependencies": {},
+ "description": "Portable Unix shell commands for Node.js",
+ "devDependencies": {
+ "coffee-script": "^1.10.0",
+ "jshint": "~2.1.11"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "ce1ed837b4b0e55b5ec3dab84251ab9dbdc0c7ec",
+ "tarball": "http://registry.npmjs.org/shelljs/-/shelljs-0.6.0.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "gitHead": "fe06baf1173ec6f0a70cd58ddb7d373f4c6446f5",
+ "homepage": "http://github.com/shelljs/shelljs",
+ "keywords": [
+ "jake",
+ "make",
+ "makefile",
+ "shell",
+ "synchronous",
+ "unix"
+ ],
+ "license": "BSD-3-Clause",
+ "main": "./shell.js",
+ "maintainers": [
+ {
+ "name": "ariporad",
+ "email": "ari@ariporad.com"
+ },
+ {
+ "name": "artur",
+ "email": "arturadib@gmail.com"
+ },
+ {
+ "name": "nfischer",
+ "email": "ntfschr@gmail.com"
+ }
+ ],
+ "name": "shelljs",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/shelljs/shelljs.git"
+ },
+ "scripts": {
+ "test": "node scripts/run-tests"
+ },
+ "version": "0.6.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/scripts/generate-docs.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/scripts/generate-docs.js b/node_modules/shelljs/scripts/generate-docs.js
new file mode 100755
index 0000000..3a31a91
--- /dev/null
+++ b/node_modules/shelljs/scripts/generate-docs.js
@@ -0,0 +1,26 @@
+#!/usr/bin/env node
+/* globals cat, cd, echo, grep, sed */
+require('../global');
+
+echo('Appending docs to README.md');
+
+cd(__dirname + '/..');
+
+// Extract docs from shell.js
+var docs = grep('//@', 'shell.js');
+
+docs = docs.replace(/\/\/\@include (.+)/g, function(match, path) {
+ var file = path.match('.js$') ? path : path+'.js';
+ return grep('//@', file);
+});
+
+// Remove '//@'
+docs = docs.replace(/\/\/\@ ?/g, '');
+
+// Wipe out the old docs
+cat('README.md').replace(/## Command reference(.|\n)*/, '## Command reference').to('README.md');
+
+// Append new docs to README
+sed('-i', /## Command reference/, '## Command reference\n\n' + docs, 'README.md');
+
+echo('All done.');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/scripts/run-tests.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/scripts/run-tests.js b/node_modules/shelljs/scripts/run-tests.js
new file mode 100755
index 0000000..e8e7ff2
--- /dev/null
+++ b/node_modules/shelljs/scripts/run-tests.js
@@ -0,0 +1,55 @@
+#!/usr/bin/env node
+/* globals cd, echo, exec, exit, ls, pwd, test */
+require('../global');
+var common = require('../src/common');
+
+var failed = false;
+
+//
+// Lint
+//
+var JSHINT_BIN = 'node_modules/jshint/bin/jshint';
+cd(__dirname + '/..');
+
+if (!test('-f', JSHINT_BIN)) {
+ echo('JSHint not found. Run `npm install` in the root dir first.');
+ exit(1);
+}
+
+var jsfiles = common.expand([pwd() + '/*.js',
+ pwd() + '/scripts/*.js',
+ pwd() + '/src/*.js',
+ pwd() + '/test/*.js'
+ ]).join(' ');
+if (exec('node ' + pwd() + '/' + JSHINT_BIN + ' ' + jsfiles).code !== 0) {
+ failed = true;
+ echo('*** JSHINT FAILED! (return code != 0)');
+ echo();
+} else {
+ echo('All JSHint tests passed');
+ echo();
+}
+
+//
+// Unit tests
+//
+cd(__dirname + '/../test');
+ls('*.js').forEach(function(file) {
+ echo('Running test:', file);
+ if (exec('node ' + file).code !== 123) { // 123 avoids false positives (e.g. premature exit)
+ failed = true;
+ echo('*** TEST FAILED! (missing exit code "123")');
+ echo();
+ }
+});
+
+if (failed) {
+ echo();
+ echo('*******************************************************');
+ echo('WARNING: Some tests did not pass!');
+ echo('*******************************************************');
+ exit(1);
+} else {
+ echo();
+ echo('All tests passed.');
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/shell.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/shell.js b/node_modules/shelljs/shell.js
new file mode 100644
index 0000000..93aff70
--- /dev/null
+++ b/node_modules/shelljs/shell.js
@@ -0,0 +1,184 @@
+//
+// ShellJS
+// Unix shell commands on top of Node's API
+//
+// Copyright (c) 2012 Artur Adib
+// http://github.com/arturadib/shelljs
+//
+
+var common = require('./src/common');
+
+
+//@
+//@ All commands run synchronously, unless otherwise stated.
+//@
+
+//@include ./src/cd
+var _cd = require('./src/cd');
+exports.cd = common.wrap('cd', _cd);
+
+//@include ./src/pwd
+var _pwd = require('./src/pwd');
+exports.pwd = common.wrap('pwd', _pwd);
+
+//@include ./src/ls
+var _ls = require('./src/ls');
+exports.ls = common.wrap('ls', _ls);
+
+//@include ./src/find
+var _find = require('./src/find');
+exports.find = common.wrap('find', _find);
+
+//@include ./src/cp
+var _cp = require('./src/cp');
+exports.cp = common.wrap('cp', _cp);
+
+//@include ./src/rm
+var _rm = require('./src/rm');
+exports.rm = common.wrap('rm', _rm);
+
+//@include ./src/mv
+var _mv = require('./src/mv');
+exports.mv = common.wrap('mv', _mv);
+
+//@include ./src/mkdir
+var _mkdir = require('./src/mkdir');
+exports.mkdir = common.wrap('mkdir', _mkdir);
+
+//@include ./src/test
+var _test = require('./src/test');
+exports.test = common.wrap('test', _test);
+
+//@include ./src/cat
+var _cat = require('./src/cat');
+exports.cat = common.wrap('cat', _cat);
+
+//@include ./src/to
+var _to = require('./src/to');
+String.prototype.to = common.wrap('to', _to);
+
+//@include ./src/toEnd
+var _toEnd = require('./src/toEnd');
+String.prototype.toEnd = common.wrap('toEnd', _toEnd);
+
+//@include ./src/sed
+var _sed = require('./src/sed');
+exports.sed = common.wrap('sed', _sed);
+
+//@include ./src/grep
+var _grep = require('./src/grep');
+exports.grep = common.wrap('grep', _grep);
+
+//@include ./src/which
+var _which = require('./src/which');
+exports.which = common.wrap('which', _which);
+
+//@include ./src/echo
+var _echo = require('./src/echo');
+exports.echo = _echo; // don't common.wrap() as it could parse '-options'
+
+//@include ./src/dirs
+var _dirs = require('./src/dirs').dirs;
+exports.dirs = common.wrap("dirs", _dirs);
+var _pushd = require('./src/dirs').pushd;
+exports.pushd = common.wrap('pushd', _pushd);
+var _popd = require('./src/dirs').popd;
+exports.popd = common.wrap("popd", _popd);
+
+//@include ./src/ln
+var _ln = require('./src/ln');
+exports.ln = common.wrap('ln', _ln);
+
+//@
+//@ ### exit(code)
+//@ Exits the current process with the given exit code.
+exports.exit = process.exit;
+
+//@
+//@ ### env['VAR_NAME']
+//@ Object containing environment variables (both getter and setter). Shortcut to process.env.
+exports.env = process.env;
+
+//@include ./src/exec
+var _exec = require('./src/exec');
+exports.exec = common.wrap('exec', _exec, {notUnix:true});
+
+//@include ./src/chmod
+var _chmod = require('./src/chmod');
+exports.chmod = common.wrap('chmod', _chmod);
+
+//@include ./src/touch
+var _touch = require('./src/touch');
+exports.touch = common.wrap('touch', _touch);
+
+//@include ./src/set
+var _set = require('./src/set');
+exports.set = common.wrap('set', _set);
+
+
+//@
+//@ ## Non-Unix commands
+//@
+
+//@include ./src/tempdir
+var _tempDir = require('./src/tempdir');
+exports.tempdir = common.wrap('tempdir', _tempDir);
+
+
+//@include ./src/error
+var _error = require('./src/error');
+exports.error = _error;
+
+
+
+//@
+//@ ## Configuration
+//@
+
+exports.config = common.config;
+
+//@
+//@ ### config.silent
+//@ Example:
+//@
+//@ ```javascript
+//@ var sh = require('shelljs');
+//@ var silentState = sh.config.silent; // save old silent state
+//@ sh.config.silent = true;
+//@ /* ... */
+//@ sh.config.silent = silentState; // restore old silent state
+//@ ```
+//@
+//@ Suppresses all command output if `true`, except for `echo()` calls.
+//@ Default is `false`.
+
+//@
+//@ ### config.fatal
+//@ Example:
+//@
+//@ ```javascript
+//@ require('shelljs/global');
+//@ config.fatal = true; // or set('-e');
+//@ cp('this_file_does_not_exist', '/dev/null'); // dies here
+//@ /* more commands... */
+//@ ```
+//@
+//@ If `true` the script will die on errors. Default is `false`. This is
+//@ analogous to Bash's `set -e`
+
+//@
+//@ ### config.verbose
+//@ Example:
+//@
+//@ ```javascript
+//@ config.verbose = true; // or set('-v');
+//@ cd('dir/');
+//@ ls('subdir/');
+//@ ```
+//@
+//@ Will print each command as follows:
+//@
+//@ ```
+//@ cd dir/
+//@ ls subdir/
+//@ ```
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/src/cat.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/cat.js b/node_modules/shelljs/src/cat.js
new file mode 100644
index 0000000..5840b4e
--- /dev/null
+++ b/node_modules/shelljs/src/cat.js
@@ -0,0 +1,40 @@
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### cat(file [, file ...])
+//@ ### cat(file_array)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var str = cat('file*.txt');
+//@ var str = cat('file1', 'file2');
+//@ var str = cat(['file1', 'file2']); // same as above
+//@ ```
+//@
+//@ Returns a string containing the given file, or a concatenated string
+//@ containing the files if more than one file is given (a new line character is
+//@ introduced between each file). Wildcard `*` accepted.
+function _cat(options, files) {
+ var cat = '';
+
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ files.forEach(function(file) {
+ if (!fs.existsSync(file))
+ common.error('no such file or directory: ' + file);
+
+ cat += fs.readFileSync(file, 'utf8');
+ });
+
+ return common.ShellString(cat);
+}
+module.exports = _cat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/shelljs/src/cd.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/cd.js b/node_modules/shelljs/src/cd.js
new file mode 100644
index 0000000..b7b9931
--- /dev/null
+++ b/node_modules/shelljs/src/cd.js
@@ -0,0 +1,28 @@
+var fs = require('fs');
+var common = require('./common');
+
+//@
+//@ ### cd([dir])
+//@ Changes to directory `dir` for the duration of the script. Changes to home
+//@ directory if no argument is supplied.
+function _cd(options, dir) {
+ if (!dir)
+ dir = common.getUserHome();
+
+ if (dir === '-') {
+ if (!common.state.previousDir)
+ common.error('could not find previous directory');
+ else
+ dir = common.state.previousDir;
+ }
+
+ if (!fs.existsSync(dir))
+ common.error('no such file or directory: ' + dir);
+
+ if (!fs.statSync(dir).isDirectory())
+ common.error('not a directory: ' + dir);
+
+ common.state.previousDir = process.cwd();
+ process.chdir(dir);
+}
+module.exports = _cd;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[39/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/cloneDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/cloneDeep.js b/node_modules/lodash-node/compat/objects/cloneDeep.js
new file mode 100644
index 0000000..922214e
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/cloneDeep.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseClone = require('../internals/baseClone'),
+ baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Creates a deep clone of `value`. If a callback is provided it will be
+ * executed to produce the cloned values. If the callback returns `undefined`
+ * cloning will be handled by the method instead. The callback is bound to
+ * `thisArg` and invoked with one argument; (value).
+ *
+ * Note: This method is loosely based on the structured clone algorithm. Functions
+ * and DOM nodes are **not** cloned. The enumerable properties of `arguments` objects and
+ * objects created by constructors other than `Object` are cloned to plain `Object` objects.
+ * See http://www.w3.org/TR/html5/infrastructure.html#internal-structured-cloning-algorithm.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the deep cloned value.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * var deep = _.cloneDeep(characters);
+ * deep[0] === characters[0];
+ * // => false
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'node': element
+ * };
+ *
+ * var clone = _.cloneDeep(view, function(value) {
+ * return _.isElement(value) ? value.cloneNode(true) : undefined;
+ * });
+ *
+ * clone.node == view.node;
+ * // => false
+ */
+function cloneDeep(value, callback, thisArg) {
+ return baseClone(value, true, typeof callback == 'function' && baseCreateCallback(callback, thisArg, 1));
+}
+
+module.exports = cloneDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/create.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/create.js b/node_modules/lodash-node/compat/objects/create.js
new file mode 100644
index 0000000..ec60f54
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/create.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var assign = require('./assign'),
+ baseCreate = require('../internals/baseCreate');
+
+/**
+ * Creates an object that inherits from the given `prototype` object. If a
+ * `properties` object is provided its own enumerable properties are assigned
+ * to the created object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} prototype The object to inherit from.
+ * @param {Object} [properties] The properties to assign to the object.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * function Circle() {
+ * Shape.call(this);
+ * }
+ *
+ * Circle.prototype = _.create(Shape.prototype, { 'constructor': Circle });
+ *
+ * var circle = new Circle;
+ * circle instanceof Circle;
+ * // => true
+ *
+ * circle instanceof Shape;
+ * // => true
+ */
+function create(prototype, properties) {
+ var result = baseCreate(prototype);
+ return properties ? assign(result, properties) : result;
+}
+
+module.exports = create;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/defaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/defaults.js b/node_modules/lodash-node/compat/objects/defaults.js
new file mode 100644
index 0000000..64b5f4d
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/defaults.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createIterator = require('../internals/createIterator'),
+ defaultsIteratorOptions = require('../internals/defaultsIteratorOptions');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object for all destination properties that resolve to `undefined`. Once a
+ * property is set, additional defaults of the same property will be ignored.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param- {Object} [guard] Allows working with `_.reduce` without using its
+ * `key` and `object` arguments as sources.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var object = { 'name': 'barney' };
+ * _.defaults(object, { 'name': 'fred', 'employer': 'slate' });
+ * // => { 'name': 'barney', 'employer': 'slate' }
+ */
+var defaults = createIterator(defaultsIteratorOptions);
+
+module.exports = defaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/findKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/findKey.js b/node_modules/lodash-node/compat/objects/findKey.js
new file mode 100644
index 0000000..65a06d3
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/findKey.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('./forOwn');
+
+/**
+ * This method is like `_.findIndex` except that it returns the key of the
+ * first element that passes the callback check, instead of the element itself.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to search.
+ * @param {Function|Object|string} [callback=identity] The function called per
+ * iteration. If a property name or object is provided it will be used to
+ * create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {string|undefined} Returns the key of the found element, else `undefined`.
+ * @example
+ *
+ * var characters = {
+ * 'barney': { 'age': 36, 'blocked': false },
+ * 'fred': { 'age': 40, 'blocked': true },
+ * 'pebbles': { 'age': 1, 'blocked': false }
+ * };
+ *
+ * _.findKey(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => 'barney' (property order is not guaranteed across environments)
+ *
+ * // using "_.where" callback shorthand
+ * _.findKey(characters, { 'age': 1 });
+ * // => 'pebbles'
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findKey(characters, 'blocked');
+ * // => 'fred'
+ */
+function findKey(object, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+ forOwn(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result = key;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = findKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/findLastKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/findLastKey.js b/node_modules/lodash-node/compat/objects/findLastKey.js
new file mode 100644
index 0000000..86ca4dd
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/findLastKey.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwnRight = require('./forOwnRight');
+
+/**
+ * This method is like `_.findKey` except that it iterates over elements
+ * of a `collection` in the opposite order.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to search.
+ * @param {Function|Object|string} [callback=identity] The function called per
+ * iteration. If a property name or object is provided it will be used to
+ * create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {string|undefined} Returns the key of the found element, else `undefined`.
+ * @example
+ *
+ * var characters = {
+ * 'barney': { 'age': 36, 'blocked': true },
+ * 'fred': { 'age': 40, 'blocked': false },
+ * 'pebbles': { 'age': 1, 'blocked': true }
+ * };
+ *
+ * _.findLastKey(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => returns `pebbles`, assuming `_.findKey` returns `barney`
+ *
+ * // using "_.where" callback shorthand
+ * _.findLastKey(characters, { 'age': 40 });
+ * // => 'fred'
+ *
+ * // using "_.pluck" callback shorthand
+ * _.findLastKey(characters, 'blocked');
+ * // => 'pebbles'
+ */
+function findLastKey(object, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+ forOwnRight(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result = key;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = findLastKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/forIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/forIn.js b/node_modules/lodash-node/compat/objects/forIn.js
new file mode 100644
index 0000000..8f0c0f9
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/forIn.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createIterator = require('../internals/createIterator'),
+ eachIteratorOptions = require('../internals/eachIteratorOptions'),
+ forOwnIteratorOptions = require('../internals/forOwnIteratorOptions');
+
+/**
+ * Iterates over own and inherited enumerable properties of an object,
+ * executing the callback for each property. The callback is bound to `thisArg`
+ * and invoked with three arguments; (value, key, object). Callbacks may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * Shape.prototype.move = function(x, y) {
+ * this.x += x;
+ * this.y += y;
+ * };
+ *
+ * _.forIn(new Shape, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'x', 'y', and 'move' (property order is not guaranteed across environments)
+ */
+var forIn = createIterator(eachIteratorOptions, forOwnIteratorOptions, {
+ 'useHas': false
+});
+
+module.exports = forIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/forInRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/forInRight.js b/node_modules/lodash-node/compat/objects/forInRight.js
new file mode 100644
index 0000000..2cdabf5
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/forInRight.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ forIn = require('./forIn');
+
+/**
+ * This method is like `_.forIn` except that it iterates over elements
+ * of a `collection` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * Shape.prototype.move = function(x, y) {
+ * this.x += x;
+ * this.y += y;
+ * };
+ *
+ * _.forInRight(new Shape, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'move', 'y', and 'x' assuming `_.forIn ` logs 'x', 'y', and 'move'
+ */
+function forInRight(object, callback, thisArg) {
+ var pairs = [];
+
+ forIn(object, function(value, key) {
+ pairs.push(key, value);
+ });
+
+ var length = pairs.length;
+ callback = baseCreateCallback(callback, thisArg, 3);
+ while (length--) {
+ if (callback(pairs[length--], pairs[length], object) === false) {
+ break;
+ }
+ }
+ return object;
+}
+
+module.exports = forInRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/forOwn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/forOwn.js b/node_modules/lodash-node/compat/objects/forOwn.js
new file mode 100644
index 0000000..a4ab74c
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/forOwn.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createIterator = require('../internals/createIterator'),
+ eachIteratorOptions = require('../internals/eachIteratorOptions'),
+ forOwnIteratorOptions = require('../internals/forOwnIteratorOptions');
+
+/**
+ * Iterates over own enumerable properties of an object, executing the callback
+ * for each property. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, key, object). Callbacks may exit iteration early by
+ * explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.forOwn({ '0': 'zero', '1': 'one', 'length': 2 }, function(num, key) {
+ * console.log(key);
+ * });
+ * // => logs '0', '1', and 'length' (property order is not guaranteed across environments)
+ */
+var forOwn = createIterator(eachIteratorOptions, forOwnIteratorOptions);
+
+module.exports = forOwn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/forOwnRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/forOwnRight.js b/node_modules/lodash-node/compat/objects/forOwnRight.js
new file mode 100644
index 0000000..5d1e3b9
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/forOwnRight.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ keys = require('./keys');
+
+/**
+ * This method is like `_.forOwn` except that it iterates over elements
+ * of a `collection` in the opposite order.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.forOwnRight({ '0': 'zero', '1': 'one', 'length': 2 }, function(num, key) {
+ * console.log(key);
+ * });
+ * // => logs 'length', '1', and '0' assuming `_.forOwn` logs '0', '1', and 'length'
+ */
+function forOwnRight(object, callback, thisArg) {
+ var props = keys(object),
+ length = props.length;
+
+ callback = baseCreateCallback(callback, thisArg, 3);
+ while (length--) {
+ var key = props[length];
+ if (callback(object[key], key, object) === false) {
+ break;
+ }
+ }
+ return object;
+}
+
+module.exports = forOwnRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/functions.js b/node_modules/lodash-node/compat/objects/functions.js
new file mode 100644
index 0000000..3b78842
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/functions.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('./forIn'),
+ isFunction = require('./isFunction');
+
+/**
+ * Creates a sorted array of property names of all enumerable properties,
+ * own and inherited, of `object` that have function values.
+ *
+ * @static
+ * @memberOf _
+ * @alias methods
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names that have function values.
+ * @example
+ *
+ * _.functions(_);
+ * // => ['all', 'any', 'bind', 'bindAll', 'clone', 'compact', 'compose', ...]
+ */
+function functions(object) {
+ var result = [];
+ forIn(object, function(value, key) {
+ if (isFunction(value)) {
+ result.push(key);
+ }
+ });
+ return result.sort();
+}
+
+module.exports = functions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/has.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/has.js b/node_modules/lodash-node/compat/objects/has.js
new file mode 100644
index 0000000..055689c
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/has.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if the specified property name exists as a direct property of `object`,
+ * instead of an inherited property.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @param {string} key The name of the property to check.
+ * @returns {boolean} Returns `true` if key is a direct property, else `false`.
+ * @example
+ *
+ * _.has({ 'a': 1, 'b': 2, 'c': 3 }, 'b');
+ * // => true
+ */
+function has(object, key) {
+ return object ? hasOwnProperty.call(object, key) : false;
+}
+
+module.exports = has;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/invert.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/invert.js b/node_modules/lodash-node/compat/objects/invert.js
new file mode 100644
index 0000000..a623f7c
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/invert.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates an object composed of the inverted keys and values of the given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to invert.
+ * @returns {Object} Returns the created inverted object.
+ * @example
+ *
+ * _.invert({ 'first': 'fred', 'second': 'barney' });
+ * // => { 'fred': 'first', 'barney': 'second' }
+ */
+function invert(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ result[object[key]] = key;
+ }
+ return result;
+}
+
+module.exports = invert;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isArguments.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isArguments.js b/node_modules/lodash-node/compat/objects/isArguments.js
new file mode 100644
index 0000000..7efc264
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isArguments.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var support = require('../support');
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * Checks if `value` is an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an `arguments` object, else `false`.
+ * @example
+ *
+ * (function() { return _.isArguments(arguments); })(1, 2, 3);
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ toString.call(value) == argsClass || false;
+}
+// fallback for browsers that can't detect `arguments` objects by [[Class]]
+if (!support.argsClass) {
+ isArguments = function(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ hasOwnProperty.call(value, 'callee') && !propertyIsEnumerable.call(value, 'callee') || false;
+ };
+}
+
+module.exports = isArguments;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isArray.js b/node_modules/lodash-node/compat/objects/isArray.js
new file mode 100644
index 0000000..ceb9f39
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isArray.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative');
+
+/** `Object#toString` result shortcuts */
+var arrayClass = '[object Array]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeIsArray = isNative(nativeIsArray = Array.isArray) && nativeIsArray;
+
+/**
+ * Checks if `value` is an array.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an array, else `false`.
+ * @example
+ *
+ * (function() { return _.isArray(arguments); })();
+ * // => false
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ */
+var isArray = nativeIsArray || function(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ toString.call(value) == arrayClass || false;
+};
+
+module.exports = isArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isBoolean.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isBoolean.js b/node_modules/lodash-node/compat/objects/isBoolean.js
new file mode 100644
index 0000000..aa97cc4
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isBoolean.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var boolClass = '[object Boolean]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a boolean value.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a boolean value, else `false`.
+ * @example
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+function isBoolean(value) {
+ return value === true || value === false ||
+ value && typeof value == 'object' && toString.call(value) == boolClass || false;
+}
+
+module.exports = isBoolean;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isDate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isDate.js b/node_modules/lodash-node/compat/objects/isDate.js
new file mode 100644
index 0000000..9084a4c
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isDate.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var dateClass = '[object Date]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a date.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a date, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ */
+function isDate(value) {
+ return value && typeof value == 'object' && toString.call(value) == dateClass || false;
+}
+
+module.exports = isDate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isElement.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isElement.js b/node_modules/lodash-node/compat/objects/isElement.js
new file mode 100644
index 0000000..9538278
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isElement.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ */
+function isElement(value) {
+ return value && value.nodeType === 1 || false;
+}
+
+module.exports = isElement;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isEmpty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isEmpty.js b/node_modules/lodash-node/compat/objects/isEmpty.js
new file mode 100644
index 0000000..c9899e3
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isEmpty.js
@@ -0,0 +1,66 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forOwn = require('./forOwn'),
+ isArguments = require('./isArguments'),
+ isFunction = require('./isFunction'),
+ support = require('../support');
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ objectClass = '[object Object]',
+ stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is empty. Arrays, strings, or `arguments` objects with a
+ * length of `0` and objects with no own enumerable properties are considered
+ * "empty".
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Array|Object|string} value The value to inspect.
+ * @returns {boolean} Returns `true` if the `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({});
+ * // => true
+ *
+ * _.isEmpty('');
+ * // => true
+ */
+function isEmpty(value) {
+ var result = true;
+ if (!value) {
+ return result;
+ }
+ var className = toString.call(value),
+ length = value.length;
+
+ if ((className == arrayClass || className == stringClass ||
+ (support.argsClass ? className == argsClass : isArguments(value))) ||
+ (className == objectClass && typeof length == 'number' && isFunction(value.splice))) {
+ return !length;
+ }
+ forOwn(value, function() {
+ return (result = false);
+ });
+ return result;
+}
+
+module.exports = isEmpty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isEqual.js b/node_modules/lodash-node/compat/objects/isEqual.js
new file mode 100644
index 0000000..fbb4960
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isEqual.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseIsEqual = require('../internals/baseIsEqual');
+
+/**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent to each other. If a callback is provided it will be executed
+ * to compare values. If the callback returns `undefined` comparisons will
+ * be handled by the method instead. The callback is bound to `thisArg` and
+ * invoked with two arguments; (a, b).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} a The value to compare.
+ * @param {*} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * var copy = { 'name': 'fred' };
+ *
+ * object == copy;
+ * // => false
+ *
+ * _.isEqual(object, copy);
+ * // => true
+ *
+ * var words = ['hello', 'goodbye'];
+ * var otherWords = ['hi', 'goodbye'];
+ *
+ * _.isEqual(words, otherWords, function(a, b) {
+ * var reGreet = /^(?:hello|hi)$/i,
+ * aGreet = _.isString(a) && reGreet.test(a),
+ * bGreet = _.isString(b) && reGreet.test(b);
+ *
+ * return (aGreet || bGreet) ? (aGreet == bGreet) : undefined;
+ * });
+ * // => true
+ */
+function isEqual(a, b, callback, thisArg) {
+ return baseIsEqual(a, b, typeof callback == 'function' && baseCreateCallback(callback, thisArg, 2));
+}
+
+module.exports = isEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isFinite.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isFinite.js b/node_modules/lodash-node/compat/objects/isFinite.js
new file mode 100644
index 0000000..8546112
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isFinite.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeIsFinite = global.isFinite,
+ nativeIsNaN = global.isNaN;
+
+/**
+ * Checks if `value` is, or can be coerced to, a finite number.
+ *
+ * Note: This is not the same as native `isFinite` which will return true for
+ * booleans and empty strings. See http://es5.github.io/#x15.1.2.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is finite, else `false`.
+ * @example
+ *
+ * _.isFinite(-101);
+ * // => true
+ *
+ * _.isFinite('10');
+ * // => true
+ *
+ * _.isFinite(true);
+ * // => false
+ *
+ * _.isFinite('');
+ * // => false
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ */
+function isFinite(value) {
+ return nativeIsFinite(value) && !nativeIsNaN(parseFloat(value));
+}
+
+module.exports = isFinite;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isFunction.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isFunction.js b/node_modules/lodash-node/compat/objects/isFunction.js
new file mode 100644
index 0000000..22608cd
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isFunction.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var funcClass = '[object Function]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a function.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ */
+function isFunction(value) {
+ return typeof value == 'function';
+}
+// fallback for older versions of Chrome and Safari
+if (isFunction(/x/)) {
+ isFunction = function(value) {
+ return typeof value == 'function' && toString.call(value) == funcClass;
+ };
+}
+
+module.exports = isFunction;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isNaN.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isNaN.js b/node_modules/lodash-node/compat/objects/isNaN.js
new file mode 100644
index 0000000..426652d
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isNaN.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNumber = require('./isNumber');
+
+/**
+ * Checks if `value` is `NaN`.
+ *
+ * Note: This is not the same as native `isNaN` which will return `true` for
+ * `undefined` and other non-numeric values. See http://es5.github.io/#x15.1.2.4.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+function isNaN(value) {
+ // `NaN` as a primitive is the only value that is not equal to itself
+ // (perform the [[Class]] check first to avoid errors with some host objects in IE)
+ return isNumber(value) && value != +value;
+}
+
+module.exports = isNaN;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isNull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isNull.js b/node_modules/lodash-node/compat/objects/isNull.js
new file mode 100644
index 0000000..4789d5d
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isNull.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(undefined);
+ * // => false
+ */
+function isNull(value) {
+ return value === null;
+}
+
+module.exports = isNull;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isNumber.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isNumber.js b/node_modules/lodash-node/compat/objects/isNumber.js
new file mode 100644
index 0000000..f242787
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isNumber.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var numberClass = '[object Number]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a number.
+ *
+ * Note: `NaN` is considered a number. See http://es5.github.io/#x8.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(8.4 * 5);
+ * // => true
+ */
+function isNumber(value) {
+ return typeof value == 'number' ||
+ value && typeof value == 'object' && toString.call(value) == numberClass || false;
+}
+
+module.exports = isNumber;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isObject.js b/node_modules/lodash-node/compat/objects/isObject.js
new file mode 100644
index 0000000..a156308
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isObject.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('../internals/objectTypes');
+
+/**
+ * Checks if `value` is the language type of Object.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // check if the value is the ECMAScript language type of Object
+ // http://es5.github.io/#x8
+ // and avoid a V8 bug
+ // http://code.google.com/p/v8/issues/detail?id=2291
+ return !!(value && objectTypes[typeof value]);
+}
+
+module.exports = isObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isPlainObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isPlainObject.js b/node_modules/lodash-node/compat/objects/isPlainObject.js
new file mode 100644
index 0000000..9f2fe81
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isPlainObject.js
@@ -0,0 +1,62 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArguments = require('./isArguments'),
+ isNative = require('../internals/isNative'),
+ shimIsPlainObject = require('../internals/shimIsPlainObject'),
+ support = require('../support');
+
+/** `Object#toString` result shortcuts */
+var objectClass = '[object Object]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var getPrototypeOf = isNative(getPrototypeOf = Object.getPrototypeOf) && getPrototypeOf;
+
+/**
+ * Checks if `value` is an object created by the `Object` constructor.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * _.isPlainObject(new Shape);
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'x': 0, 'y': 0 });
+ * // => true
+ */
+var isPlainObject = !getPrototypeOf ? shimIsPlainObject : function(value) {
+ if (!(value && toString.call(value) == objectClass) || (!support.argsClass && isArguments(value))) {
+ return false;
+ }
+ var valueOf = value.valueOf,
+ objProto = isNative(valueOf) && (objProto = getPrototypeOf(valueOf)) && getPrototypeOf(objProto);
+
+ return objProto
+ ? (value == objProto || getPrototypeOf(value) == objProto)
+ : shimIsPlainObject(value);
+};
+
+module.exports = isPlainObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isRegExp.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isRegExp.js b/node_modules/lodash-node/compat/objects/isRegExp.js
new file mode 100644
index 0000000..d7800d0
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isRegExp.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('../internals/objectTypes');
+
+/** `Object#toString` result shortcuts */
+var regexpClass = '[object RegExp]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a regular expression.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a regular expression, else `false`.
+ * @example
+ *
+ * _.isRegExp(/fred/);
+ * // => true
+ */
+function isRegExp(value) {
+ return value && objectTypes[typeof value] && toString.call(value) == regexpClass || false;
+}
+
+module.exports = isRegExp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isString.js b/node_modules/lodash-node/compat/objects/isString.js
new file mode 100644
index 0000000..e1fabd5
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isString.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a string.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('fred');
+ * // => true
+ */
+function isString(value) {
+ return typeof value == 'string' ||
+ value && typeof value == 'object' && toString.call(value) == stringClass || false;
+}
+
+module.exports = isString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/isUndefined.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/isUndefined.js b/node_modules/lodash-node/compat/objects/isUndefined.js
new file mode 100644
index 0000000..1e28a1f
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/isUndefined.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ */
+function isUndefined(value) {
+ return typeof value == 'undefined';
+}
+
+module.exports = isUndefined;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/keys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/keys.js b/node_modules/lodash-node/compat/objects/keys.js
new file mode 100644
index 0000000..9959b2b
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/keys.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArguments = require('./isArguments'),
+ isNative = require('../internals/isNative'),
+ isObject = require('./isObject'),
+ shimKeys = require('../internals/shimKeys'),
+ support = require('../support');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeKeys = isNative(nativeKeys = Object.keys) && nativeKeys;
+
+/**
+ * Creates an array composed of the own enumerable property names of an object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names.
+ * @example
+ *
+ * _.keys({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => ['one', 'two', 'three'] (property order is not guaranteed across environments)
+ */
+var keys = !nativeKeys ? shimKeys : function(object) {
+ if (!isObject(object)) {
+ return [];
+ }
+ if ((support.enumPrototypes && typeof object == 'function') ||
+ (support.nonEnumArgs && object.length && isArguments(object))) {
+ return shimKeys(object);
+ }
+ return nativeKeys(object);
+};
+
+module.exports = keys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/mapValues.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/mapValues.js b/node_modules/lodash-node/compat/objects/mapValues.js
new file mode 100644
index 0000000..431f55b
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/mapValues.js
@@ -0,0 +1,58 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('./forOwn');
+
+/**
+ * Creates an object with the same keys as `object` and values generated by
+ * running each own enumerable property of `object` through the callback.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new object with values of the results of each `callback` execution.
+ * @example
+ *
+ * _.mapValues({ 'a': 1, 'b': 2, 'c': 3} , function(num) { return num * 3; });
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ *
+ * var characters = {
+ * 'fred': { 'name': 'fred', 'age': 40 },
+ * 'pebbles': { 'name': 'pebbles', 'age': 1 }
+ * };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.mapValues(characters, 'age');
+ * // => { 'fred': 40, 'pebbles': 1 }
+ */
+function mapValues(object, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg, 3);
+
+ forOwn(object, function(value, key, object) {
+ result[key] = callback(value, key, object);
+ });
+ return result;
+}
+
+module.exports = mapValues;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/merge.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/merge.js b/node_modules/lodash-node/compat/objects/merge.js
new file mode 100644
index 0000000..cb602a2
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/merge.js
@@ -0,0 +1,97 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseMerge = require('../internals/baseMerge'),
+ getArray = require('../internals/getArray'),
+ isObject = require('./isObject'),
+ releaseArray = require('../internals/releaseArray'),
+ slice = require('../internals/slice');
+
+/**
+ * Recursively merges own enumerable properties of the source object(s), that
+ * don't resolve to `undefined` into the destination object. Subsequent sources
+ * will overwrite property assignments of previous sources. If a callback is
+ * provided it will be executed to produce the merged values of the destination
+ * and source properties. If the callback returns `undefined` merging will
+ * be handled by the method instead. The callback is bound to `thisArg` and
+ * invoked with two arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param {Function} [callback] The function to customize merging properties.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var names = {
+ * 'characters': [
+ * { 'name': 'barney' },
+ * { 'name': 'fred' }
+ * ]
+ * };
+ *
+ * var ages = {
+ * 'characters': [
+ * { 'age': 36 },
+ * { 'age': 40 }
+ * ]
+ * };
+ *
+ * _.merge(names, ages);
+ * // => { 'characters': [{ 'name': 'barney', 'age': 36 }, { 'name': 'fred', 'age': 40 }] }
+ *
+ * var food = {
+ * 'fruits': ['apple'],
+ * 'vegetables': ['beet']
+ * };
+ *
+ * var otherFood = {
+ * 'fruits': ['banana'],
+ * 'vegetables': ['carrot']
+ * };
+ *
+ * _.merge(food, otherFood, function(a, b) {
+ * return _.isArray(a) ? a.concat(b) : undefined;
+ * });
+ * // => { 'fruits': ['apple', 'banana'], 'vegetables': ['beet', 'carrot] }
+ */
+function merge(object) {
+ var args = arguments,
+ length = 2;
+
+ if (!isObject(object)) {
+ return object;
+ }
+ // allows working with `_.reduce` and `_.reduceRight` without using
+ // their `index` and `collection` arguments
+ if (typeof args[2] != 'number') {
+ length = args.length;
+ }
+ if (length > 3 && typeof args[length - 2] == 'function') {
+ var callback = baseCreateCallback(args[--length - 1], args[length--], 2);
+ } else if (length > 2 && typeof args[length - 1] == 'function') {
+ callback = args[--length];
+ }
+ var sources = slice(arguments, 1, length),
+ index = -1,
+ stackA = getArray(),
+ stackB = getArray();
+
+ while (++index < length) {
+ baseMerge(object, sources[index], callback, stackA, stackB);
+ }
+ releaseArray(stackA);
+ releaseArray(stackB);
+ return object;
+}
+
+module.exports = merge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/omit.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/omit.js b/node_modules/lodash-node/compat/objects/omit.js
new file mode 100644
index 0000000..6517419
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/omit.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseFlatten = require('../internals/baseFlatten'),
+ createCallback = require('../functions/createCallback'),
+ forIn = require('./forIn');
+
+/**
+ * Creates a shallow clone of `object` excluding the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a callback is provided it will be executed for each
+ * property of `object` omitting the properties the callback returns truey
+ * for. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|...string|string[]} [callback] The properties to omit or the
+ * function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object without the omitted properties.
+ * @example
+ *
+ * _.omit({ 'name': 'fred', 'age': 40 }, 'age');
+ * // => { 'name': 'fred' }
+ *
+ * _.omit({ 'name': 'fred', 'age': 40 }, function(value) {
+ * return typeof value == 'number';
+ * });
+ * // => { 'name': 'fred' }
+ */
+function omit(object, callback, thisArg) {
+ var result = {};
+ if (typeof callback != 'function') {
+ var props = [];
+ forIn(object, function(value, key) {
+ props.push(key);
+ });
+ props = baseDifference(props, baseFlatten(arguments, true, false, 1));
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ result[key] = object[key];
+ }
+ } else {
+ callback = createCallback(callback, thisArg, 3);
+ forIn(object, function(value, key, object) {
+ if (!callback(value, key, object)) {
+ result[key] = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = omit;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/pairs.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/pairs.js b/node_modules/lodash-node/compat/objects/pairs.js
new file mode 100644
index 0000000..b334c4a
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/pairs.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates a two dimensional array of an object's key-value pairs,
+ * i.e. `[[key1, value1], [key2, value2]]`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns new array of key-value pairs.
+ * @example
+ *
+ * _.pairs({ 'barney': 36, 'fred': 40 });
+ * // => [['barney', 36], ['fred', 40]] (property order is not guaranteed across environments)
+ */
+function pairs(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ var key = props[index];
+ result[index] = [key, object[key]];
+ }
+ return result;
+}
+
+module.exports = pairs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/pick.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/pick.js b/node_modules/lodash-node/compat/objects/pick.js
new file mode 100644
index 0000000..fb581ad
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/pick.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ createCallback = require('../functions/createCallback'),
+ forIn = require('./forIn'),
+ isObject = require('./isObject');
+
+/**
+ * Creates a shallow clone of `object` composed of the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a callback is provided it will be executed for each
+ * property of `object` picking the properties the callback returns truey
+ * for. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|...string|string[]} [callback] The function called per
+ * iteration or property names to pick, specified as individual property
+ * names or arrays of property names.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object composed of the picked properties.
+ * @example
+ *
+ * _.pick({ 'name': 'fred', '_userid': 'fred1' }, 'name');
+ * // => { 'name': 'fred' }
+ *
+ * _.pick({ 'name': 'fred', '_userid': 'fred1' }, function(value, key) {
+ * return key.charAt(0) != '_';
+ * });
+ * // => { 'name': 'fred' }
+ */
+function pick(object, callback, thisArg) {
+ var result = {};
+ if (typeof callback != 'function') {
+ var index = -1,
+ props = baseFlatten(arguments, true, false, 1),
+ length = isObject(object) ? props.length : 0;
+
+ while (++index < length) {
+ var key = props[index];
+ if (key in object) {
+ result[key] = object[key];
+ }
+ }
+ } else {
+ callback = createCallback(callback, thisArg, 3);
+ forIn(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result[key] = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = pick;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/transform.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/transform.js b/node_modules/lodash-node/compat/objects/transform.js
new file mode 100644
index 0000000..f04b078
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/transform.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('../internals/baseCreate'),
+ baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ forOwn = require('./forOwn'),
+ isArray = require('./isArray');
+
+/**
+ * An alternative to `_.reduce` this method transforms `object` to a new
+ * `accumulator` object which is the result of running each of its own
+ * enumerable properties through a callback, with each callback execution
+ * potentially mutating the `accumulator` object. The callback is bound to
+ * `thisArg` and invoked with four arguments; (accumulator, value, key, object).
+ * Callbacks may exit iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Array|Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] The custom accumulator value.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var squares = _.transform([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], function(result, num) {
+ * num *= num;
+ * if (num % 2) {
+ * return result.push(num) < 3;
+ * }
+ * });
+ * // => [1, 9, 25]
+ *
+ * var mapped = _.transform({ 'a': 1, 'b': 2, 'c': 3 }, function(result, num, key) {
+ * result[key] = num * 3;
+ * });
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ */
+function transform(object, callback, accumulator, thisArg) {
+ var isArr = isArray(object);
+ if (accumulator == null) {
+ if (isArr) {
+ accumulator = [];
+ } else {
+ var ctor = object && object.constructor,
+ proto = ctor && ctor.prototype;
+
+ accumulator = baseCreate(proto);
+ }
+ }
+ if (callback) {
+ callback = createCallback(callback, thisArg, 4);
+ (isArr ? baseEach : forOwn)(object, function(value, index, object) {
+ return callback(accumulator, value, index, object);
+ });
+ }
+ return accumulator;
+}
+
+module.exports = transform;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/objects/values.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/objects/values.js b/node_modules/lodash-node/compat/objects/values.js
new file mode 100644
index 0000000..e3135ae
--- /dev/null
+++ b/node_modules/lodash-node/compat/objects/values.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates an array composed of the own enumerable property values of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property values.
+ * @example
+ *
+ * _.values({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => [1, 2, 3] (property order is not guaranteed across environments)
+ */
+function values(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = object[props[index]];
+ }
+ return result;
+}
+
+module.exports = values;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/support.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/support.js b/node_modules/lodash-node/compat/support.js
new file mode 100644
index 0000000..fb6ec48
--- /dev/null
+++ b/node_modules/lodash-node/compat/support.js
@@ -0,0 +1,177 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./internals/isNative');
+
+/** Used to detect functions containing a `this` reference */
+var reThis = /\bthis\b/;
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ objectClass = '[object Object]';
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Used for native method references */
+var errorProto = Error.prototype,
+ objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * An object used to flag environments features.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var support = {};
+
+(function() {
+ var ctor = function() { this.x = 1; },
+ object = { '0': 1, 'length': 1 },
+ props = [];
+
+ ctor.prototype = { 'valueOf': 1, 'y': 1 };
+ for (var key in new ctor) { props.push(key); }
+ for (key in arguments) { }
+
+ /**
+ * Detect if an `arguments` object's [[Class]] is resolvable (all but Firefox < 4, IE < 9).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.argsClass = toString.call(arguments) == argsClass;
+
+ /**
+ * Detect if `arguments` objects are `Object` objects (all but Narwhal and Opera < 10.5).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.argsObject = arguments.constructor == Object && !(arguments instanceof Array);
+
+ /**
+ * Detect if `name` or `message` properties of `Error.prototype` are
+ * enumerable by default. (IE < 9, Safari < 5.1)
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.enumErrorProps = propertyIsEnumerable.call(errorProto, 'message') || propertyIsEnumerable.call(errorProto, 'name');
+
+ /**
+ * Detect if `prototype` properties are enumerable by default.
+ *
+ * Firefox < 3.6, Opera > 9.50 - Opera < 11.60, and Safari < 5.1
+ * (if the prototype or a property on the prototype has been set)
+ * incorrectly sets a function's `prototype` property [[Enumerable]]
+ * value to `true`.
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.enumPrototypes = propertyIsEnumerable.call(ctor, 'prototype');
+
+ /**
+ * Detect if functions can be decompiled by `Function#toString`
+ * (all but PS3 and older Opera mobile browsers & avoided in Windows 8 apps).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.funcDecomp = !isNative(global.WinRTError) && reThis.test(function() { return this; });
+
+ /**
+ * Detect if `Function#name` is supported (all but IE).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.funcNames = typeof Function.name == 'string';
+
+ /**
+ * Detect if `arguments` object indexes are non-enumerable
+ * (Firefox < 4, IE < 9, PhantomJS, Safari < 5.1).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.nonEnumArgs = key != 0;
+
+ /**
+ * Detect if properties shadowing those on `Object.prototype` are non-enumerable.
+ *
+ * In IE < 9 an objects own properties, shadowing non-enumerable ones, are
+ * made non-enumerable as well (a.k.a the JScript [[DontEnum]] bug).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.nonEnumShadows = !/valueOf/.test(props);
+
+ /**
+ * Detect if own properties are iterated after inherited properties (all but IE < 9).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.ownLast = props[0] != 'x';
+
+ /**
+ * Detect if `Array#shift` and `Array#splice` augment array-like objects correctly.
+ *
+ * Firefox < 10, IE compatibility mode, and IE < 9 have buggy Array `shift()`
+ * and `splice()` functions that fail to remove the last element, `value[0]`,
+ * of array-like objects even though the `length` property is set to `0`.
+ * The `shift()` method is buggy in IE 8 compatibility mode, while `splice()`
+ * is buggy regardless of mode in IE < 9 and buggy in compatibility mode in IE 9.
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.spliceObjects = (arrayRef.splice.call(object, 0, 1), !object[0]);
+
+ /**
+ * Detect lack of support for accessing string characters by index.
+ *
+ * IE < 8 can't access characters by index and IE 8 can only access
+ * characters by index on string literals.
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.unindexedChars = ('x'[0] + Object('x')[0]) != 'xx';
+
+ /**
+ * Detect if a DOM node's [[Class]] is resolvable (all but IE < 9)
+ * and that the JS engine errors when attempting to coerce an object to
+ * a string without a `toString` function.
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ try {
+ support.nodeClass = !(toString.call(document) == objectClass && !({ 'toString': 0 } + ''));
+ } catch(e) {
+ support.nodeClass = true;
+ }
+}(1));
+
+module.exports = support;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities.js b/node_modules/lodash-node/compat/utilities.js
new file mode 100644
index 0000000..0fc2d98
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'constant': require('./utilities/constant'),
+ 'createCallback': require('./functions/createCallback'),
+ 'escape': require('./utilities/escape'),
+ 'identity': require('./utilities/identity'),
+ 'mixin': require('./utilities/mixin'),
+ 'noConflict': require('./utilities/noConflict'),
+ 'noop': require('./utilities/noop'),
+ 'now': require('./utilities/now'),
+ 'parseInt': require('./utilities/parseInt'),
+ 'property': require('./utilities/property'),
+ 'random': require('./utilities/random'),
+ 'result': require('./utilities/result'),
+ 'template': require('./utilities/template'),
+ 'templateSettings': require('./utilities/templateSettings'),
+ 'times': require('./utilities/times'),
+ 'unescape': require('./utilities/unescape'),
+ 'uniqueId': require('./utilities/uniqueId')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/constant.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/constant.js b/node_modules/lodash-node/compat/utilities/constant.js
new file mode 100644
index 0000000..ae112ed
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/constant.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates a function that returns `value`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} value The value to return from the new function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * var getter = _.constant(object);
+ * getter() === object;
+ * // => true
+ */
+function constant(value) {
+ return function() {
+ return value;
+ };
+}
+
+module.exports = constant;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/escape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/escape.js b/node_modules/lodash-node/compat/utilities/escape.js
new file mode 100644
index 0000000..00582b9
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/escape.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var escapeHtmlChar = require('../internals/escapeHtmlChar'),
+ keys = require('../objects/keys'),
+ reUnescapedHtml = require('../internals/reUnescapedHtml');
+
+/**
+ * Converts the characters `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding HTML entities.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} string The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('Fred, Wilma, & Pebbles');
+ * // => 'Fred, Wilma, & Pebbles'
+ */
+function escape(string) {
+ return string == null ? '' : String(string).replace(reUnescapedHtml, escapeHtmlChar);
+}
+
+module.exports = escape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/identity.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/identity.js b/node_modules/lodash-node/compat/utilities/identity.js
new file mode 100644
index 0000000..838700c
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/identity.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * This method returns the first argument provided to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * _.identity(object) === object;
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+module.exports = identity;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/utilities/mixin.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/utilities/mixin.js b/node_modules/lodash-node/compat/utilities/mixin.js
new file mode 100644
index 0000000..26db74f
--- /dev/null
+++ b/node_modules/lodash-node/compat/utilities/mixin.js
@@ -0,0 +1,88 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ functions = require('../objects/functions'),
+ isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * Adds function properties of a source object to the destination object.
+ * If `object` is a function methods will be added to its prototype as well.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Function|Object} [object=lodash] object The destination object.
+ * @param {Object} source The object of functions to add.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.chain=true] Specify whether the functions added are chainable.
+ * @example
+ *
+ * function capitalize(string) {
+ * return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase();
+ * }
+ *
+ * _.mixin({ 'capitalize': capitalize });
+ * _.capitalize('fred');
+ * // => 'Fred'
+ *
+ * _('fred').capitalize().value();
+ * // => 'Fred'
+ *
+ * _.mixin({ 'capitalize': capitalize }, { 'chain': false });
+ * _('fred').capitalize();
+ * // => 'Fred'
+ */
+function mixin(object, source, options) {
+ var chain = true,
+ methodNames = source && functions(source);
+
+ if (options === false) {
+ chain = false;
+ } else if (isObject(options) && 'chain' in options) {
+ chain = options.chain;
+ }
+ var ctor = object,
+ isFunc = isFunction(ctor);
+
+ forEach(methodNames, function(methodName) {
+ var func = object[methodName] = source[methodName];
+ if (isFunc) {
+ ctor.prototype[methodName] = function() {
+ var chainAll = this.__chain__,
+ value = this.__wrapped__,
+ args = [value];
+
+ push.apply(args, arguments);
+ var result = func.apply(object, args);
+ if (chain || chainAll) {
+ if (value === result && isObject(result)) {
+ return this;
+ }
+ result = new ctor(result);
+ result.__chain__ = chainAll;
+ }
+ return result;
+ };
+ }
+ });
+}
+
+module.exports = mixin;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[30/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/random.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/random.js b/node_modules/lodash-node/underscore/utilities/random.js
new file mode 100644
index 0000000..fb6640e
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/random.js
@@ -0,0 +1,58 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom');
+
+/** Native method shortcuts */
+var floor = Math.floor;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeRandom = Math.random;
+
+/**
+ * Produces a random number between `min` and `max` (inclusive). If only one
+ * argument is provided a number between `0` and the given number will be
+ * returned. If `floating` is truey or either `min` or `max` are floats a
+ * floating-point number will be returned instead of an integer.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {number} [min=0] The minimum possible value.
+ * @param {number} [max=1] The maximum possible value.
+ * @param {boolean} [floating=false] Specify returning a floating-point number.
+ * @returns {number} Returns a random number.
+ * @example
+ *
+ * _.random(0, 5);
+ * // => an integer between 0 and 5
+ *
+ * _.random(5);
+ * // => also an integer between 0 and 5
+ *
+ * _.random(5, true);
+ * // => a floating-point number between 0 and 5
+ *
+ * _.random(1.2, 5.2);
+ * // => a floating-point number between 1.2 and 5.2
+ */
+function random(min, max) {
+ if (min == null && max == null) {
+ max = 1;
+ }
+ min = +min || 0;
+ if (max == null) {
+ max = min;
+ min = 0;
+ } else {
+ max = +max || 0;
+ }
+ return min + floor(nativeRandom() * (max - min + 1));
+}
+
+module.exports = random;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/result.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/result.js b/node_modules/lodash-node/underscore/utilities/result.js
new file mode 100644
index 0000000..95d44c6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/result.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Resolves the value of property `key` on `object`. If `key` is a function
+ * it will be invoked with the `this` binding of `object` and its result returned,
+ * else the property value is returned. If `object` is falsey then `undefined`
+ * is returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Object} object The object to inspect.
+ * @param {string} key The name of the property to resolve.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = {
+ * 'cheese': 'crumpets',
+ * 'stuff': function() {
+ * return 'nonsense';
+ * }
+ * };
+ *
+ * _.result(object, 'cheese');
+ * // => 'crumpets'
+ *
+ * _.result(object, 'stuff');
+ * // => 'nonsense'
+ */
+function result(object, key) {
+ if (object) {
+ var value = object[key];
+ return isFunction(value) ? object[key]() : value;
+ }
+}
+
+module.exports = result;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/template.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/template.js b/node_modules/lodash-node/underscore/utilities/template.js
new file mode 100644
index 0000000..b6e8807
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/template.js
@@ -0,0 +1,163 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var defaults = require('../objects/defaults'),
+ escape = require('./escape'),
+ escapeStringChar = require('../internals/escapeStringChar'),
+ reInterpolate = require('../internals/reInterpolate'),
+ templateSettings = require('./templateSettings');
+
+/** Used to ensure capturing order of template delimiters */
+var reNoMatch = /($^)/;
+
+/** Used to match unescaped characters in compiled string literals */
+var reUnescapedString = /['\n\r\t\u2028\u2029\\]/g;
+
+/**
+ * A micro-templating method that handles arbitrary delimiters, preserves
+ * whitespace, and correctly escapes quotes within interpolated code.
+ *
+ * Note: In the development build, `_.template` utilizes sourceURLs for easier
+ * debugging. See http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl
+ *
+ * For more information on precompiling templates see:
+ * http://lodash.com/custom-builds
+ *
+ * For more information on Chrome extension sandboxes see:
+ * http://developer.chrome.com/stable/extensions/sandboxingEval.html
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} text The template text.
+ * @param {Object} data The data object used to populate the text.
+ * @param {Object} [options] The options object.
+ * @param {RegExp} [options.escape] The "escape" delimiter.
+ * @param {RegExp} [options.evaluate] The "evaluate" delimiter.
+ * @param {Object} [options.imports] An object to import into the template as local variables.
+ * @param {RegExp} [options.interpolate] The "interpolate" delimiter.
+ * @param {string} [sourceURL] The sourceURL of the template's compiled source.
+ * @param {string} [variable] The data object variable name.
+ * @returns {Function|string} Returns a compiled function when no `data` object
+ * is given, else it returns the interpolated text.
+ * @example
+ *
+ * // using the "interpolate" delimiter to create a compiled template
+ * var compiled = _.template('hello <%= name %>');
+ * compiled({ 'name': 'fred' });
+ * // => 'hello fred'
+ *
+ * // using the "escape" delimiter to escape HTML in data property values
+ * _.template('<b><%- value %></b>', { 'value': '<script>' });
+ * // => '<b><script></b>'
+ *
+ * // using the "evaluate" delimiter to generate HTML
+ * var list = '<% _.forEach(people, function(name) { %><li><%- name %></li><% }); %>';
+ * _.template(list, { 'people': ['fred', 'barney'] });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the ES6 delimiter as an alternative to the default "interpolate" delimiter
+ * _.template('hello ${ name }', { 'name': 'pebbles' });
+ * // => 'hello pebbles'
+ *
+ * // using the internal `print` function in "evaluate" delimiters
+ * _.template('<% print("hello " + name); %>!', { 'name': 'barney' });
+ * // => 'hello barney!'
+ *
+ * // using a custom template delimiters
+ * _.templateSettings = {
+ * 'interpolate': /{{([\s\S]+?)}}/g
+ * };
+ *
+ * _.template('hello {{ name }}!', { 'name': 'mustache' });
+ * // => 'hello mustache!'
+ *
+ * // using the `imports` option to import jQuery
+ * var list = '<% jq.each(people, function(name) { %><li><%- name %></li><% }); %>';
+ * _.template(list, { 'people': ['fred', 'barney'] }, { 'imports': { 'jq': jQuery } });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the `sourceURL` option to specify a custom sourceURL for the template
+ * var compiled = _.template('hello <%= name %>', null, { 'sourceURL': '/basic/greeting.jst' });
+ * compiled(data);
+ * // => find the source of "greeting.jst" under the Sources tab or Resources panel of the web inspector
+ *
+ * // using the `variable` option to ensure a with-statement isn't used in the compiled template
+ * var compiled = _.template('hi <%= data.name %>!', null, { 'variable': 'data' });
+ * compiled.source;
+ * // => function(data) {
+ * var __t, __p = '', __e = _.escape;
+ * __p += 'hi ' + ((__t = ( data.name )) == null ? '' : __t) + '!';
+ * return __p;
+ * }
+ *
+ * // using the `source` property to inline compiled templates for meaningful
+ * // line numbers in error messages and a stack trace
+ * fs.writeFileSync(path.join(cwd, 'jst.js'), '\
+ * var JST = {\
+ * "main": ' + _.template(mainText).source + '\
+ * };\
+ * ');
+ */
+function template(text, data, options) {
+ var _ = templateSettings.imports._,
+ settings = _.templateSettings || templateSettings;
+
+ text = String(text || '');
+ options = defaults({}, options, settings);
+
+ var index = 0,
+ source = "__p += '",
+ variable = options.variable;
+
+ var reDelimiters = RegExp(
+ (options.escape || reNoMatch).source + '|' +
+ (options.interpolate || reNoMatch).source + '|' +
+ (options.evaluate || reNoMatch).source + '|$'
+ , 'g');
+
+ text.replace(reDelimiters, function(match, escapeValue, interpolateValue, evaluateValue, offset) {
+ source += text.slice(index, offset).replace(reUnescapedString, escapeStringChar);
+ if (escapeValue) {
+ source += "' +\n_.escape(" + escapeValue + ") +\n'";
+ }
+ if (evaluateValue) {
+ source += "';\n" + evaluateValue + ";\n__p += '";
+ }
+ if (interpolateValue) {
+ source += "' +\n((__t = (" + interpolateValue + ")) == null ? '' : __t) +\n'";
+ }
+ index = offset + match.length;
+ return match;
+ });
+
+ source += "';\n";
+ if (!variable) {
+ variable = 'obj';
+ source = 'with (' + variable + ' || {}) {\n' + source + '\n}\n';
+ }
+ source = 'function(' + variable + ') {\n' +
+ "var __t, __p = '', __j = Array.prototype.join;\n" +
+ "function print() { __p += __j.call(arguments, '') }\n" +
+ source +
+ 'return __p\n}';
+
+ try {
+ var result = Function('_', 'return ' + source)(_);
+ } catch(e) {
+ e.source = source;
+ throw e;
+ }
+ if (data) {
+ return result(data);
+ }
+ result.source = source;
+ return result;
+}
+
+module.exports = template;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/templateSettings.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/templateSettings.js b/node_modules/lodash-node/underscore/utilities/templateSettings.js
new file mode 100644
index 0000000..8459664
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/templateSettings.js
@@ -0,0 +1,73 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var escape = require('./escape'),
+ reInterpolate = require('../internals/reInterpolate');
+
+/**
+ * By default, the template delimiters used by Lo-Dash are similar to those in
+ * embedded Ruby (ERB). Change the following template settings to use alternative
+ * delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'escape': /<%-([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'evaluate': /<%([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type string
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type Object
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type Function
+ */
+ '_': { 'escape': escape }
+ }
+};
+
+module.exports = templateSettings;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/times.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/times.js b/node_modules/lodash-node/underscore/utilities/times.js
new file mode 100644
index 0000000..b87e512
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/times.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Executes the callback `n` times, returning an array of the results
+ * of each callback execution. The callback is bound to `thisArg` and invoked
+ * with one argument; (index).
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {number} n The number of times to execute the callback.
+ * @param {Function} callback The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns an array of the results of each `callback` execution.
+ * @example
+ *
+ * var diceRolls = _.times(3, _.partial(_.random, 1, 6));
+ * // => [3, 6, 4]
+ *
+ * _.times(3, function(n) { mage.castSpell(n); });
+ * // => calls `mage.castSpell(n)` three times, passing `n` of `0`, `1`, and `2` respectively
+ *
+ * _.times(3, function(n) { this.cast(n); }, mage);
+ * // => also calls `mage.castSpell(n)` three times
+ */
+function times(n, callback, thisArg) {
+ n = (n = +n) > -1 ? n : 0;
+ var index = -1,
+ result = Array(n);
+
+ callback = baseCreateCallback(callback, thisArg, 1);
+ while (++index < n) {
+ result[index] = callback(index);
+ }
+ return result;
+}
+
+module.exports = times;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/unescape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/unescape.js b/node_modules/lodash-node/underscore/utilities/unescape.js
new file mode 100644
index 0000000..d6050ea
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/unescape.js
@@ -0,0 +1,32 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys'),
+ reEscapedHtml = require('../internals/reEscapedHtml'),
+ unescapeHtmlChar = require('../internals/unescapeHtmlChar');
+
+/**
+ * The inverse of `_.escape` this method converts the HTML entities
+ * `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding characters.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} string The string to unescape.
+ * @returns {string} Returns the unescaped string.
+ * @example
+ *
+ * _.unescape('Fred, Barney & Pebbles');
+ * // => 'Fred, Barney & Pebbles'
+ */
+function unescape(string) {
+ return string == null ? '' : String(string).replace(reEscapedHtml, unescapeHtmlChar);
+}
+
+module.exports = unescape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/uniqueId.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/uniqueId.js b/node_modules/lodash-node/underscore/utilities/uniqueId.js
new file mode 100644
index 0000000..e9178b6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/uniqueId.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to generate unique IDs */
+var idCounter = 0;
+
+/**
+ * Generates a unique ID. If `prefix` is provided the ID will be appended to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} [prefix] The value to prefix the ID with.
+ * @returns {string} Returns the unique ID.
+ * @example
+ *
+ * _.uniqueId('contact_');
+ * // => 'contact_104'
+ *
+ * _.uniqueId();
+ * // => '105'
+ */
+function uniqueId(prefix) {
+ var id = ++idCounter + '';
+ return prefix ? prefix + id : id;
+}
+
+module.exports = uniqueId;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/lodash/LICENSE b/node_modules/lodash/LICENSE
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation <http://dojofoundation.org/>
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors <http://underscorejs.org/>
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/README.md
----------------------------------------------------------------------
diff --git a/node_modules/lodash/README.md b/node_modules/lodash/README.md
new file mode 100644
index 0000000..fd98e5c
--- /dev/null
+++ b/node_modules/lodash/README.md
@@ -0,0 +1,121 @@
+# lodash v3.10.1
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash](https://lodash.com/) exported as [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) modules.
+
+Generated using [lodash-cli](https://www.npmjs.com/package/lodash-cli):
+```bash
+$ lodash modularize modern exports=node -o ./
+$ lodash modern -d -o ./index.js
+```
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash
+```
+
+In Node.js/io.js:
+
+```js
+// load the modern build
+var _ = require('lodash');
+// or a method category
+var array = require('lodash/array');
+// or a method (great for smaller builds with browserify/webpack)
+var chunk = require('lodash/array/chunk');
+```
+
+See the [package source](https://github.com/lodash/lodash/tree/3.10.1-npm) for more details.
+
+**Note:**<br>
+Don’t assign values to the [special variable](http://nodejs.org/api/repl.html#repl_repl_features) `_` when in the REPL.<br>
+Install [n_](https://www.npmjs.com/package/n_) for a REPL that includes lodash by default.
+
+## Module formats
+
+lodash is also available in a variety of other builds & module formats.
+
+ * npm packages for [modern](https://www.npmjs.com/package/lodash), [compatibility](https://www.npmjs.com/package/lodash-compat), & [per method](https://www.npmjs.com/browse/keyword/lodash-modularized) builds
+ * AMD modules for [modern](https://github.com/lodash/lodash/tree/3.10.1-amd) & [compatibility](https://github.com/lodash/lodash-compat/tree/3.10.1-amd) builds
+ * ES modules for the [modern](https://github.com/lodash/lodash/tree/3.10.1-es) build
+
+## Further Reading
+
+ * [API Documentation](https://lodash.com/docs)
+ * [Build Differences](https://github.com/lodash/lodash/wiki/Build-Differences)
+ * [Changelog](https://github.com/lodash/lodash/wiki/Changelog)
+ * [Roadmap](https://github.com/lodash/lodash/wiki/Roadmap)
+ * [More Resources](https://github.com/lodash/lodash/wiki/Resources)
+
+## Features
+
+ * ~100% [code coverage](https://coveralls.io/r/lodash)
+ * Follows [semantic versioning](http://semver.org/) for releases
+ * [Lazily evaluated](http://filimanjaro.com/blog/2014/introducing-lazy-evaluation/) chaining
+ * [_(…)](https://lodash.com/docs#_) supports implicit chaining
+ * [_.ary](https://lodash.com/docs#ary) & [_.rearg](https://lodash.com/docs#rearg) to change function argument limits & order
+ * [_.at](https://lodash.com/docs#at) for cherry-picking collection values
+ * [_.attempt](https://lodash.com/docs#attempt) to execute functions which may error without a try-catch
+ * [_.before](https://lodash.com/docs#before) to complement [_.after](https://lodash.com/docs#after)
+ * [_.bindKey](https://lodash.com/docs#bindKey) for binding [*“lazy”*](http://michaux.ca/articles/lazy-function-definition-pattern) defined methods
+ * [_.chunk](https://lodash.com/docs#chunk) for splitting an array into chunks of a given size
+ * [_.clone](https://lodash.com/docs#clone) supports shallow cloning of `Date` & `RegExp` objects
+ * [_.cloneDeep](https://lodash.com/docs#cloneDeep) for deep cloning arrays & objects
+ * [_.curry](https://lodash.com/docs#curry) & [_.curryRight](https://lodash.com/docs#curryRight) for creating [curried](http://hughfdjackson.com/javascript/why-curry-helps/) functions
+ * [_.debounce](https://lodash.com/docs#debounce) & [_.throttle](https://lodash.com/docs#throttle) are cancelable & accept options for more control
+ * [_.defaultsDeep](https://lodash.com/docs#defaultsDeep) for recursively assigning default properties
+ * [_.fill](https://lodash.com/docs#fill) to fill arrays with values
+ * [_.findKey](https://lodash.com/docs#findKey) for finding keys
+ * [_.flow](https://lodash.com/docs#flow) to complement [_.flowRight](https://lodash.com/docs#flowRight) (a.k.a `_.compose`)
+ * [_.forEach](https://lodash.com/docs#forEach) supports exiting early
+ * [_.forIn](https://lodash.com/docs#forIn) for iterating all enumerable properties
+ * [_.forOwn](https://lodash.com/docs#forOwn) for iterating own properties
+ * [_.get](https://lodash.com/docs#get) & [_.set](https://lodash.com/docs#set) for deep property getting & setting
+ * [_.gt](https://lodash.com/docs#gt), [_.gte](https://lodash.com/docs#gte), [_.lt](https://lodash.com/docs#lt), & [_.lte](https://lodash.com/docs#lte) relational methods
+ * [_.inRange](https://lodash.com/docs#inRange) for checking whether a number is within a given range
+ * [_.isNative](https://lodash.com/docs#isNative) to check for native functions
+ * [_.isPlainObject](https://lodash.com/docs#isPlainObject) & [_.toPlainObject](https://lodash.com/docs#toPlainObject) to check for & convert to `Object` objects
+ * [_.isTypedArray](https://lodash.com/docs#isTypedArray) to check for typed arrays
+ * [_.mapKeys](https://lodash.com/docs#mapKeys) for mapping keys to an object
+ * [_.matches](https://lodash.com/docs#matches) supports deep object comparisons
+ * [_.matchesProperty](https://lodash.com/docs#matchesProperty) to complement [_.matches](https://lodash.com/docs#matches) & [_.property](https://lodash.com/docs#property)
+ * [_.merge](https://lodash.com/docs#merge) for a deep [_.extend](https://lodash.com/docs#extend)
+ * [_.method](https://lodash.com/docs#method) & [_.methodOf](https://lodash.com/docs#methodOf) to create functions that invoke methods
+ * [_.modArgs](https://lodash.com/docs#modArgs) for more advanced functional composition
+ * [_.parseInt](https://lodash.com/docs#parseInt) for consistent cross-environment behavior
+ * [_.pull](https://lodash.com/docs#pull), [_.pullAt](https://lodash.com/docs#pullAt), & [_.remove](https://lodash.com/docs#remove) for mutating arrays
+ * [_.random](https://lodash.com/docs#random) supports returning floating-point numbers
+ * [_.restParam](https://lodash.com/docs#restParam) & [_.spread](https://lodash.com/docs#spread) for applying rest parameters & spreading arguments to functions
+ * [_.runInContext](https://lodash.com/docs#runInContext) for collisionless mixins & easier mocking
+ * [_.slice](https://lodash.com/docs#slice) for creating subsets of array-like values
+ * [_.sortByAll](https://lodash.com/docs#sortByAll) & [_.sortByOrder](https://lodash.com/docs#sortByOrder) for sorting by multiple properties & orders
+ * [_.support](https://lodash.com/docs#support) for flagging environment features
+ * [_.template](https://lodash.com/docs#template) supports [*“imports”*](https://lodash.com/docs#templateSettings-imports) options & [ES template delimiters](http://people.mozilla.org/~jorendorff/es6-draft.html#sec-template-literal-lexical-components)
+ * [_.transform](https://lodash.com/docs#transform) as a powerful alternative to [_.reduce](https://lodash.com/docs#reduce) for transforming objects
+ * [_.unzipWith](https://lodash.com/docs#unzipWith) & [_.zipWith](https://lodash.com/docs#zipWith) to specify how grouped values should be combined
+ * [_.valuesIn](https://lodash.com/docs#valuesIn) for getting values of all enumerable properties
+ * [_.xor](https://lodash.com/docs#xor) to complement [_.difference](https://lodash.com/docs#difference), [_.intersection](https://lodash.com/docs#intersection), & [_.union](https://lodash.com/docs#union)
+ * [_.add](https://lodash.com/docs#add), [_.round](https://lodash.com/docs#round), [_.sum](https://lodash.com/docs#sum), &
+ [more](https://lodash.com/docs "_.ceil & _.floor") math methods
+ * [_.bind](https://lodash.com/docs#bind), [_.curry](https://lodash.com/docs#curry), [_.partial](https://lodash.com/docs#partial), &
+ [more](https://lodash.com/docs "_.bindKey, _.curryRight, _.partialRight") support customizable argument placeholders
+ * [_.capitalize](https://lodash.com/docs#capitalize), [_.trim](https://lodash.com/docs#trim), &
+ [more](https://lodash.com/docs "_.camelCase, _.deburr, _.endsWith, _.escapeRegExp, _.kebabCase, _.pad, _.padLeft, _.padRight, _.repeat, _.snakeCase, _.startCase, _.startsWith, _.trimLeft, _.trimRight, _.trunc, _.words") string methods
+ * [_.clone](https://lodash.com/docs#clone), [_.isEqual](https://lodash.com/docs#isEqual), &
+ [more](https://lodash.com/docs "_.assign, _.cloneDeep, _.merge") accept customizer callbacks
+ * [_.dropWhile](https://lodash.com/docs#dropWhile), [_.takeWhile](https://lodash.com/docs#takeWhile), &
+ [more](https://lodash.com/docs "_.drop, _.dropRight, _.dropRightWhile, _.take, _.takeRight, _.takeRightWhile") to complement [_.first](https://lodash.com/docs#first), [_.initial](https://lodash.com/docs#initial), [_.last](https://lodash.com/docs#last), & [_.rest](https://lodash.com/docs#rest)
+ * [_.findLast](https://lodash.com/docs#findLast), [_.findLastKey](https://lodash.com/docs#findLastKey), &
+ [more](https://lodash.com/docs "_.curryRight, _.dropRight, _.dropRightWhile, _.flowRight, _.forEachRight, _.forInRight, _.forOwnRight, _.padRight, partialRight, _.takeRight, _.trimRight, _.takeRightWhile") right-associative methods
+ * [_.includes](https://lodash.com/docs#includes), [_.toArray](https://lodash.com/docs#toArray), &
+ [more](https://lodash.com/docs "_.at, _.countBy, _.every, _.filter, _.find, _.findLast, _.findWhere, _.forEach, _.forEachRight, _.groupBy, _.indexBy, _.invoke, _.map, _.max, _.min, _.partition, _.pluck, _.reduce, _.reduceRight, _.reject, _.shuffle, _.size, _.some, _.sortBy, _.sortByAll, _.sortByOrder, _.sum, _.where") accept strings
+ * [_#commit](https://lodash.com/docs#prototype-commit) & [_#plant](https://lodash.com/docs#prototype-plant) for working with chain sequences
+ * [_#thru](https://lodash.com/docs#thru) to pass values thru a chain sequence
+
+## Support
+
+Tested in Chrome 43-44, Firefox 38-39, IE 6-11, MS Edge, Safari 5-8, ChakraNode 0.12.2, io.js 2.5.0, Node.js 0.8.28, 0.10.40, & 0.12.7, PhantomJS 1.9.8, RingoJS 0.11, & Rhino 1.7.6.
+Automated [browser](https://saucelabs.com/u/lodash) & [CI](https://travis-ci.org/lodash/lodash/) test runs are available. Special thanks to [Sauce Labs](https://saucelabs.com/) for providing automated browser testing.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array.js b/node_modules/lodash/array.js
new file mode 100644
index 0000000..e5121fa
--- /dev/null
+++ b/node_modules/lodash/array.js
@@ -0,0 +1,44 @@
+module.exports = {
+ 'chunk': require('./array/chunk'),
+ 'compact': require('./array/compact'),
+ 'difference': require('./array/difference'),
+ 'drop': require('./array/drop'),
+ 'dropRight': require('./array/dropRight'),
+ 'dropRightWhile': require('./array/dropRightWhile'),
+ 'dropWhile': require('./array/dropWhile'),
+ 'fill': require('./array/fill'),
+ 'findIndex': require('./array/findIndex'),
+ 'findLastIndex': require('./array/findLastIndex'),
+ 'first': require('./array/first'),
+ 'flatten': require('./array/flatten'),
+ 'flattenDeep': require('./array/flattenDeep'),
+ 'head': require('./array/head'),
+ 'indexOf': require('./array/indexOf'),
+ 'initial': require('./array/initial'),
+ 'intersection': require('./array/intersection'),
+ 'last': require('./array/last'),
+ 'lastIndexOf': require('./array/lastIndexOf'),
+ 'object': require('./array/object'),
+ 'pull': require('./array/pull'),
+ 'pullAt': require('./array/pullAt'),
+ 'remove': require('./array/remove'),
+ 'rest': require('./array/rest'),
+ 'slice': require('./array/slice'),
+ 'sortedIndex': require('./array/sortedIndex'),
+ 'sortedLastIndex': require('./array/sortedLastIndex'),
+ 'tail': require('./array/tail'),
+ 'take': require('./array/take'),
+ 'takeRight': require('./array/takeRight'),
+ 'takeRightWhile': require('./array/takeRightWhile'),
+ 'takeWhile': require('./array/takeWhile'),
+ 'union': require('./array/union'),
+ 'uniq': require('./array/uniq'),
+ 'unique': require('./array/unique'),
+ 'unzip': require('./array/unzip'),
+ 'unzipWith': require('./array/unzipWith'),
+ 'without': require('./array/without'),
+ 'xor': require('./array/xor'),
+ 'zip': require('./array/zip'),
+ 'zipObject': require('./array/zipObject'),
+ 'zipWith': require('./array/zipWith')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/chunk.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/chunk.js b/node_modules/lodash/array/chunk.js
new file mode 100644
index 0000000..c8be1fb
--- /dev/null
+++ b/node_modules/lodash/array/chunk.js
@@ -0,0 +1,46 @@
+var baseSlice = require('../internal/baseSlice'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil,
+ nativeFloor = Math.floor,
+ nativeMax = Math.max;
+
+/**
+ * Creates an array of elements split into groups the length of `size`.
+ * If `collection` can't be split evenly, the final chunk will be the remaining
+ * elements.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to process.
+ * @param {number} [size=1] The length of each chunk.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the new array containing chunks.
+ * @example
+ *
+ * _.chunk(['a', 'b', 'c', 'd'], 2);
+ * // => [['a', 'b'], ['c', 'd']]
+ *
+ * _.chunk(['a', 'b', 'c', 'd'], 3);
+ * // => [['a', 'b', 'c'], ['d']]
+ */
+function chunk(array, size, guard) {
+ if (guard ? isIterateeCall(array, size, guard) : size == null) {
+ size = 1;
+ } else {
+ size = nativeMax(nativeFloor(size) || 1, 1);
+ }
+ var index = 0,
+ length = array ? array.length : 0,
+ resIndex = -1,
+ result = Array(nativeCeil(length / size));
+
+ while (index < length) {
+ result[++resIndex] = baseSlice(array, index, (index += size));
+ }
+ return result;
+}
+
+module.exports = chunk;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/compact.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/compact.js b/node_modules/lodash/array/compact.js
new file mode 100644
index 0000000..1dc1c55
--- /dev/null
+++ b/node_modules/lodash/array/compact.js
@@ -0,0 +1,30 @@
+/**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are falsey.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+function compact(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result[++resIndex] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = compact;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/difference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/difference.js b/node_modules/lodash/array/difference.js
new file mode 100644
index 0000000..128932a
--- /dev/null
+++ b/node_modules/lodash/array/difference.js
@@ -0,0 +1,29 @@
+var baseDifference = require('../internal/baseDifference'),
+ baseFlatten = require('../internal/baseFlatten'),
+ isArrayLike = require('../internal/isArrayLike'),
+ isObjectLike = require('../internal/isObjectLike'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates an array of unique `array` values not included in the other
+ * provided arrays using [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {...Array} [values] The arrays of values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.difference([1, 2, 3], [4, 2]);
+ * // => [1, 3]
+ */
+var difference = restParam(function(array, values) {
+ return (isObjectLike(array) && isArrayLike(array))
+ ? baseDifference(array, baseFlatten(values, false, true))
+ : [];
+});
+
+module.exports = difference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/drop.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/drop.js b/node_modules/lodash/array/drop.js
new file mode 100644
index 0000000..039a0b5
--- /dev/null
+++ b/node_modules/lodash/array/drop.js
@@ -0,0 +1,39 @@
+var baseSlice = require('../internal/baseSlice'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates a slice of `array` with `n` elements dropped from the beginning.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to drop.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.drop([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.drop([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.drop([1, 2, 3], 5);
+ * // => []
+ *
+ * _.drop([1, 2, 3], 0);
+ * // => [1, 2, 3]
+ */
+function drop(array, n, guard) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (guard ? isIterateeCall(array, n, guard) : n == null) {
+ n = 1;
+ }
+ return baseSlice(array, n < 0 ? 0 : n);
+}
+
+module.exports = drop;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/dropRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/dropRight.js b/node_modules/lodash/array/dropRight.js
new file mode 100644
index 0000000..14b5eb6
--- /dev/null
+++ b/node_modules/lodash/array/dropRight.js
@@ -0,0 +1,40 @@
+var baseSlice = require('../internal/baseSlice'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates a slice of `array` with `n` elements dropped from the end.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to drop.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.dropRight([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.dropRight([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.dropRight([1, 2, 3], 5);
+ * // => []
+ *
+ * _.dropRight([1, 2, 3], 0);
+ * // => [1, 2, 3]
+ */
+function dropRight(array, n, guard) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (guard ? isIterateeCall(array, n, guard) : n == null) {
+ n = 1;
+ }
+ n = length - (+n || 0);
+ return baseSlice(array, 0, n < 0 ? 0 : n);
+}
+
+module.exports = dropRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/dropRightWhile.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/dropRightWhile.js b/node_modules/lodash/array/dropRightWhile.js
new file mode 100644
index 0000000..be158bd
--- /dev/null
+++ b/node_modules/lodash/array/dropRightWhile.js
@@ -0,0 +1,59 @@
+var baseCallback = require('../internal/baseCallback'),
+ baseWhile = require('../internal/baseWhile');
+
+/**
+ * Creates a slice of `array` excluding elements dropped from the end.
+ * Elements are dropped until `predicate` returns falsey. The predicate is
+ * bound to `thisArg` and invoked with three arguments: (value, index, array).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that match the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.dropRightWhile([1, 2, 3], function(n) {
+ * return n > 1;
+ * });
+ * // => [1]
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.pluck(_.dropRightWhile(users, { 'user': 'pebbles', 'active': false }), 'user');
+ * // => ['barney', 'fred']
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.pluck(_.dropRightWhile(users, 'active', false), 'user');
+ * // => ['barney']
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.dropRightWhile(users, 'active'), 'user');
+ * // => ['barney', 'fred', 'pebbles']
+ */
+function dropRightWhile(array, predicate, thisArg) {
+ return (array && array.length)
+ ? baseWhile(array, baseCallback(predicate, thisArg, 3), true, true)
+ : [];
+}
+
+module.exports = dropRightWhile;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/dropWhile.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/dropWhile.js b/node_modules/lodash/array/dropWhile.js
new file mode 100644
index 0000000..d9eabae
--- /dev/null
+++ b/node_modules/lodash/array/dropWhile.js
@@ -0,0 +1,59 @@
+var baseCallback = require('../internal/baseCallback'),
+ baseWhile = require('../internal/baseWhile');
+
+/**
+ * Creates a slice of `array` excluding elements dropped from the beginning.
+ * Elements are dropped until `predicate` returns falsey. The predicate is
+ * bound to `thisArg` and invoked with three arguments: (value, index, array).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.dropWhile([1, 2, 3], function(n) {
+ * return n < 3;
+ * });
+ * // => [3]
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.pluck(_.dropWhile(users, { 'user': 'barney', 'active': false }), 'user');
+ * // => ['fred', 'pebbles']
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.pluck(_.dropWhile(users, 'active', false), 'user');
+ * // => ['pebbles']
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.dropWhile(users, 'active'), 'user');
+ * // => ['barney', 'fred', 'pebbles']
+ */
+function dropWhile(array, predicate, thisArg) {
+ return (array && array.length)
+ ? baseWhile(array, baseCallback(predicate, thisArg, 3), true)
+ : [];
+}
+
+module.exports = dropWhile;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/fill.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/fill.js b/node_modules/lodash/array/fill.js
new file mode 100644
index 0000000..2c8f6da
--- /dev/null
+++ b/node_modules/lodash/array/fill.js
@@ -0,0 +1,44 @@
+var baseFill = require('../internal/baseFill'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Fills elements of `array` with `value` from `start` up to, but not
+ * including, `end`.
+ *
+ * **Note:** This method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3];
+ *
+ * _.fill(array, 'a');
+ * console.log(array);
+ * // => ['a', 'a', 'a']
+ *
+ * _.fill(Array(3), 2);
+ * // => [2, 2, 2]
+ *
+ * _.fill([4, 6, 8], '*', 1, 2);
+ * // => [4, '*', 8]
+ */
+function fill(array, value, start, end) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (start && typeof start != 'number' && isIterateeCall(array, value, start)) {
+ start = 0;
+ end = length;
+ }
+ return baseFill(array, value, start, end);
+}
+
+module.exports = fill;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/findIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/findIndex.js b/node_modules/lodash/array/findIndex.js
new file mode 100644
index 0000000..2a6b8e1
--- /dev/null
+++ b/node_modules/lodash/array/findIndex.js
@@ -0,0 +1,53 @@
+var createFindIndex = require('../internal/createFindIndex');
+
+/**
+ * This method is like `_.find` except that it returns the index of the first
+ * element `predicate` returns truthy for instead of the element itself.
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to search.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * _.findIndex(users, function(chr) {
+ * return chr.user == 'barney';
+ * });
+ * // => 0
+ *
+ * // using the `_.matches` callback shorthand
+ * _.findIndex(users, { 'user': 'fred', 'active': false });
+ * // => 1
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.findIndex(users, 'active', false);
+ * // => 0
+ *
+ * // using the `_.property` callback shorthand
+ * _.findIndex(users, 'active');
+ * // => 2
+ */
+var findIndex = createFindIndex();
+
+module.exports = findIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/findLastIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/findLastIndex.js b/node_modules/lodash/array/findLastIndex.js
new file mode 100644
index 0000000..d6d8eca
--- /dev/null
+++ b/node_modules/lodash/array/findLastIndex.js
@@ -0,0 +1,53 @@
+var createFindIndex = require('../internal/createFindIndex');
+
+/**
+ * This method is like `_.findIndex` except that it iterates over elements
+ * of `collection` from right to left.
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to search.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {number} Returns the index of the found element, else `-1`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * _.findLastIndex(users, function(chr) {
+ * return chr.user == 'pebbles';
+ * });
+ * // => 2
+ *
+ * // using the `_.matches` callback shorthand
+ * _.findLastIndex(users, { 'user': 'barney', 'active': true });
+ * // => 0
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.findLastIndex(users, 'active', false);
+ * // => 2
+ *
+ * // using the `_.property` callback shorthand
+ * _.findLastIndex(users, 'active');
+ * // => 0
+ */
+var findLastIndex = createFindIndex(true);
+
+module.exports = findLastIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/first.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/first.js b/node_modules/lodash/array/first.js
new file mode 100644
index 0000000..b3b9c79
--- /dev/null
+++ b/node_modules/lodash/array/first.js
@@ -0,0 +1,22 @@
+/**
+ * Gets the first element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @alias head
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the first element of `array`.
+ * @example
+ *
+ * _.first([1, 2, 3]);
+ * // => 1
+ *
+ * _.first([]);
+ * // => undefined
+ */
+function first(array) {
+ return array ? array[0] : undefined;
+}
+
+module.exports = first;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/flatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/flatten.js b/node_modules/lodash/array/flatten.js
new file mode 100644
index 0000000..dc2eff8
--- /dev/null
+++ b/node_modules/lodash/array/flatten.js
@@ -0,0 +1,32 @@
+var baseFlatten = require('../internal/baseFlatten'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Flattens a nested array. If `isDeep` is `true` the array is recursively
+ * flattened, otherwise it's only flattened a single level.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isDeep] Specify a deep flatten.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2, 3, [4]]]);
+ * // => [1, 2, 3, [4]]
+ *
+ * // using `isDeep`
+ * _.flatten([1, [2, 3, [4]]], true);
+ * // => [1, 2, 3, 4]
+ */
+function flatten(array, isDeep, guard) {
+ var length = array ? array.length : 0;
+ if (guard && isIterateeCall(array, isDeep, guard)) {
+ isDeep = false;
+ }
+ return length ? baseFlatten(array, isDeep) : [];
+}
+
+module.exports = flatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/flattenDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/flattenDeep.js b/node_modules/lodash/array/flattenDeep.js
new file mode 100644
index 0000000..9f775fe
--- /dev/null
+++ b/node_modules/lodash/array/flattenDeep.js
@@ -0,0 +1,21 @@
+var baseFlatten = require('../internal/baseFlatten');
+
+/**
+ * Recursively flattens a nested array.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to recursively flatten.
+ * @returns {Array} Returns the new flattened array.
+ * @example
+ *
+ * _.flattenDeep([1, [2, 3, [4]]]);
+ * // => [1, 2, 3, 4]
+ */
+function flattenDeep(array) {
+ var length = array ? array.length : 0;
+ return length ? baseFlatten(array, true) : [];
+}
+
+module.exports = flattenDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/head.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/head.js b/node_modules/lodash/array/head.js
new file mode 100644
index 0000000..1961b08
--- /dev/null
+++ b/node_modules/lodash/array/head.js
@@ -0,0 +1 @@
+module.exports = require('./first');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/indexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/indexOf.js b/node_modules/lodash/array/indexOf.js
new file mode 100644
index 0000000..4cfc682
--- /dev/null
+++ b/node_modules/lodash/array/indexOf.js
@@ -0,0 +1,53 @@
+var baseIndexOf = require('../internal/baseIndexOf'),
+ binaryIndex = require('../internal/binaryIndex');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Gets the index at which the first occurrence of `value` is found in `array`
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons. If `fromIndex` is negative, it's used as the offset
+ * from the end of `array`. If `array` is sorted providing `true` for `fromIndex`
+ * performs a faster binary search.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {boolean|number} [fromIndex=0] The index to search from or `true`
+ * to perform a binary search on a sorted array.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 1, 2], 2);
+ * // => 1
+ *
+ * // using `fromIndex`
+ * _.indexOf([1, 2, 1, 2], 2, 2);
+ * // => 3
+ *
+ * // performing a binary search
+ * _.indexOf([1, 1, 2, 2], 2, true);
+ * // => 2
+ */
+function indexOf(array, value, fromIndex) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return -1;
+ }
+ if (typeof fromIndex == 'number') {
+ fromIndex = fromIndex < 0 ? nativeMax(length + fromIndex, 0) : fromIndex;
+ } else if (fromIndex) {
+ var index = binaryIndex(array, value);
+ if (index < length &&
+ (value === value ? (value === array[index]) : (array[index] !== array[index]))) {
+ return index;
+ }
+ return -1;
+ }
+ return baseIndexOf(array, value, fromIndex || 0);
+}
+
+module.exports = indexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/initial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/initial.js b/node_modules/lodash/array/initial.js
new file mode 100644
index 0000000..59b7a7d
--- /dev/null
+++ b/node_modules/lodash/array/initial.js
@@ -0,0 +1,20 @@
+var dropRight = require('./dropRight');
+
+/**
+ * Gets all but the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ */
+function initial(array) {
+ return dropRight(array, 1);
+}
+
+module.exports = initial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/intersection.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/intersection.js b/node_modules/lodash/array/intersection.js
new file mode 100644
index 0000000..f218432
--- /dev/null
+++ b/node_modules/lodash/array/intersection.js
@@ -0,0 +1,58 @@
+var baseIndexOf = require('../internal/baseIndexOf'),
+ cacheIndexOf = require('../internal/cacheIndexOf'),
+ createCache = require('../internal/createCache'),
+ isArrayLike = require('../internal/isArrayLike'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates an array of unique values that are included in all of the provided
+ * arrays using [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of shared values.
+ * @example
+ * _.intersection([1, 2], [4, 2], [2, 1]);
+ * // => [2]
+ */
+var intersection = restParam(function(arrays) {
+ var othLength = arrays.length,
+ othIndex = othLength,
+ caches = Array(length),
+ indexOf = baseIndexOf,
+ isCommon = true,
+ result = [];
+
+ while (othIndex--) {
+ var value = arrays[othIndex] = isArrayLike(value = arrays[othIndex]) ? value : [];
+ caches[othIndex] = (isCommon && value.length >= 120) ? createCache(othIndex && value) : null;
+ }
+ var array = arrays[0],
+ index = -1,
+ length = array ? array.length : 0,
+ seen = caches[0];
+
+ outer:
+ while (++index < length) {
+ value = array[index];
+ if ((seen ? cacheIndexOf(seen, value) : indexOf(result, value, 0)) < 0) {
+ var othIndex = othLength;
+ while (--othIndex) {
+ var cache = caches[othIndex];
+ if ((cache ? cacheIndexOf(cache, value) : indexOf(arrays[othIndex], value, 0)) < 0) {
+ continue outer;
+ }
+ }
+ if (seen) {
+ seen.push(value);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+});
+
+module.exports = intersection;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/last.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/last.js b/node_modules/lodash/array/last.js
new file mode 100644
index 0000000..299af31
--- /dev/null
+++ b/node_modules/lodash/array/last.js
@@ -0,0 +1,19 @@
+/**
+ * Gets the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the last element of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ */
+function last(array) {
+ var length = array ? array.length : 0;
+ return length ? array[length - 1] : undefined;
+}
+
+module.exports = last;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/lastIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/lastIndexOf.js b/node_modules/lodash/array/lastIndexOf.js
new file mode 100644
index 0000000..02b8062
--- /dev/null
+++ b/node_modules/lodash/array/lastIndexOf.js
@@ -0,0 +1,60 @@
+var binaryIndex = require('../internal/binaryIndex'),
+ indexOfNaN = require('../internal/indexOfNaN');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * This method is like `_.indexOf` except that it iterates over elements of
+ * `array` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {boolean|number} [fromIndex=array.length-1] The index to search from
+ * or `true` to perform a binary search on a sorted array.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 1, 2], 2);
+ * // => 3
+ *
+ * // using `fromIndex`
+ * _.lastIndexOf([1, 2, 1, 2], 2, 2);
+ * // => 1
+ *
+ * // performing a binary search
+ * _.lastIndexOf([1, 1, 2, 2], 2, true);
+ * // => 3
+ */
+function lastIndexOf(array, value, fromIndex) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return -1;
+ }
+ var index = length;
+ if (typeof fromIndex == 'number') {
+ index = (fromIndex < 0 ? nativeMax(length + fromIndex, 0) : nativeMin(fromIndex || 0, length - 1)) + 1;
+ } else if (fromIndex) {
+ index = binaryIndex(array, value, true) - 1;
+ var other = array[index];
+ if (value === value ? (value === other) : (other !== other)) {
+ return index;
+ }
+ return -1;
+ }
+ if (value !== value) {
+ return indexOfNaN(array, index, true);
+ }
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = lastIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/object.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/object.js b/node_modules/lodash/array/object.js
new file mode 100644
index 0000000..f4a3453
--- /dev/null
+++ b/node_modules/lodash/array/object.js
@@ -0,0 +1 @@
+module.exports = require('./zipObject');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/pull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/pull.js b/node_modules/lodash/array/pull.js
new file mode 100644
index 0000000..7bcbb4a
--- /dev/null
+++ b/node_modules/lodash/array/pull.js
@@ -0,0 +1,52 @@
+var baseIndexOf = require('../internal/baseIndexOf');
+
+/** Used for native method references. */
+var arrayProto = Array.prototype;
+
+/** Native method references. */
+var splice = arrayProto.splice;
+
+/**
+ * Removes all provided values from `array` using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * **Note:** Unlike `_.without`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {...*} [values] The values to remove.
+ * @returns {Array} Returns `array`.
+ * @example
+ *
+ * var array = [1, 2, 3, 1, 2, 3];
+ *
+ * _.pull(array, 2, 3);
+ * console.log(array);
+ * // => [1, 1]
+ */
+function pull() {
+ var args = arguments,
+ array = args[0];
+
+ if (!(array && array.length)) {
+ return array;
+ }
+ var index = 0,
+ indexOf = baseIndexOf,
+ length = args.length;
+
+ while (++index < length) {
+ var fromIndex = 0,
+ value = args[index];
+
+ while ((fromIndex = indexOf(array, value, fromIndex)) > -1) {
+ splice.call(array, fromIndex, 1);
+ }
+ }
+ return array;
+}
+
+module.exports = pull;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/pullAt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/pullAt.js b/node_modules/lodash/array/pullAt.js
new file mode 100644
index 0000000..4ca2476
--- /dev/null
+++ b/node_modules/lodash/array/pullAt.js
@@ -0,0 +1,40 @@
+var baseAt = require('../internal/baseAt'),
+ baseCompareAscending = require('../internal/baseCompareAscending'),
+ baseFlatten = require('../internal/baseFlatten'),
+ basePullAt = require('../internal/basePullAt'),
+ restParam = require('../function/restParam');
+
+/**
+ * Removes elements from `array` corresponding to the given indexes and returns
+ * an array of the removed elements. Indexes may be specified as an array of
+ * indexes or as individual arguments.
+ *
+ * **Note:** Unlike `_.at`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {...(number|number[])} [indexes] The indexes of elements to remove,
+ * specified as individual indexes or arrays of indexes.
+ * @returns {Array} Returns the new array of removed elements.
+ * @example
+ *
+ * var array = [5, 10, 15, 20];
+ * var evens = _.pullAt(array, 1, 3);
+ *
+ * console.log(array);
+ * // => [5, 15]
+ *
+ * console.log(evens);
+ * // => [10, 20]
+ */
+var pullAt = restParam(function(array, indexes) {
+ indexes = baseFlatten(indexes);
+
+ var result = baseAt(array, indexes);
+ basePullAt(array, indexes.sort(baseCompareAscending));
+ return result;
+});
+
+module.exports = pullAt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/remove.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/remove.js b/node_modules/lodash/array/remove.js
new file mode 100644
index 0000000..0cf979b
--- /dev/null
+++ b/node_modules/lodash/array/remove.js
@@ -0,0 +1,64 @@
+var baseCallback = require('../internal/baseCallback'),
+ basePullAt = require('../internal/basePullAt');
+
+/**
+ * Removes all elements from `array` that `predicate` returns truthy for
+ * and returns an array of the removed elements. The predicate is bound to
+ * `thisArg` and invoked with three arguments: (value, index, array).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * **Note:** Unlike `_.filter`, this method mutates `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to modify.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the new array of removed elements.
+ * @example
+ *
+ * var array = [1, 2, 3, 4];
+ * var evens = _.remove(array, function(n) {
+ * return n % 2 == 0;
+ * });
+ *
+ * console.log(array);
+ * // => [1, 3]
+ *
+ * console.log(evens);
+ * // => [2, 4]
+ */
+function remove(array, predicate, thisArg) {
+ var result = [];
+ if (!(array && array.length)) {
+ return result;
+ }
+ var index = -1,
+ indexes = [],
+ length = array.length;
+
+ predicate = baseCallback(predicate, thisArg, 3);
+ while (++index < length) {
+ var value = array[index];
+ if (predicate(value, index, array)) {
+ result.push(value);
+ indexes.push(index);
+ }
+ }
+ basePullAt(array, indexes);
+ return result;
+}
+
+module.exports = remove;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/rest.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/rest.js b/node_modules/lodash/array/rest.js
new file mode 100644
index 0000000..9bfb734
--- /dev/null
+++ b/node_modules/lodash/array/rest.js
@@ -0,0 +1,21 @@
+var drop = require('./drop');
+
+/**
+ * Gets all but the first element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @alias tail
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.rest([1, 2, 3]);
+ * // => [2, 3]
+ */
+function rest(array) {
+ return drop(array, 1);
+}
+
+module.exports = rest;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/slice.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/slice.js b/node_modules/lodash/array/slice.js
new file mode 100644
index 0000000..48ef1a1
--- /dev/null
+++ b/node_modules/lodash/array/slice.js
@@ -0,0 +1,30 @@
+var baseSlice = require('../internal/baseSlice'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates a slice of `array` from `start` up to, but not including, `end`.
+ *
+ * **Note:** This method is used instead of `Array#slice` to support node
+ * lists in IE < 9 and to ensure dense arrays are returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function slice(array, start, end) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (end && typeof end != 'number' && isIterateeCall(array, start, end)) {
+ start = 0;
+ end = length;
+ }
+ return baseSlice(array, start, end);
+}
+
+module.exports = slice;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/sortedIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/sortedIndex.js b/node_modules/lodash/array/sortedIndex.js
new file mode 100644
index 0000000..6903bca
--- /dev/null
+++ b/node_modules/lodash/array/sortedIndex.js
@@ -0,0 +1,53 @@
+var createSortedIndex = require('../internal/createSortedIndex');
+
+/**
+ * Uses a binary search to determine the lowest index at which `value` should
+ * be inserted into `array` in order to maintain its sort order. If an iteratee
+ * function is provided it's invoked for `value` and each element of `array`
+ * to compute their sort ranking. The iteratee is bound to `thisArg` and
+ * invoked with one argument; (value).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedIndex([30, 50], 40);
+ * // => 1
+ *
+ * _.sortedIndex([4, 4, 5, 5], 5);
+ * // => 2
+ *
+ * var dict = { 'data': { 'thirty': 30, 'forty': 40, 'fifty': 50 } };
+ *
+ * // using an iteratee function
+ * _.sortedIndex(['thirty', 'fifty'], 'forty', function(word) {
+ * return this.data[word];
+ * }, dict);
+ * // => 1
+ *
+ * // using the `_.property` callback shorthand
+ * _.sortedIndex([{ 'x': 30 }, { 'x': 50 }], { 'x': 40 }, 'x');
+ * // => 1
+ */
+var sortedIndex = createSortedIndex();
+
+module.exports = sortedIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/sortedLastIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/sortedLastIndex.js b/node_modules/lodash/array/sortedLastIndex.js
new file mode 100644
index 0000000..81a4a86
--- /dev/null
+++ b/node_modules/lodash/array/sortedLastIndex.js
@@ -0,0 +1,25 @@
+var createSortedIndex = require('../internal/createSortedIndex');
+
+/**
+ * This method is like `_.sortedIndex` except that it returns the highest
+ * index at which `value` should be inserted into `array` in order to
+ * maintain its sort order.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedLastIndex([4, 4, 5, 5], 5);
+ * // => 4
+ */
+var sortedLastIndex = createSortedIndex(true);
+
+module.exports = sortedLastIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/tail.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/tail.js b/node_modules/lodash/array/tail.js
new file mode 100644
index 0000000..c5dfe77
--- /dev/null
+++ b/node_modules/lodash/array/tail.js
@@ -0,0 +1 @@
+module.exports = require('./rest');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/take.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/take.js b/node_modules/lodash/array/take.js
new file mode 100644
index 0000000..875917a
--- /dev/null
+++ b/node_modules/lodash/array/take.js
@@ -0,0 +1,39 @@
+var baseSlice = require('../internal/baseSlice'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates a slice of `array` with `n` elements taken from the beginning.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to take.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.take([1, 2, 3]);
+ * // => [1]
+ *
+ * _.take([1, 2, 3], 2);
+ * // => [1, 2]
+ *
+ * _.take([1, 2, 3], 5);
+ * // => [1, 2, 3]
+ *
+ * _.take([1, 2, 3], 0);
+ * // => []
+ */
+function take(array, n, guard) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (guard ? isIterateeCall(array, n, guard) : n == null) {
+ n = 1;
+ }
+ return baseSlice(array, 0, n < 0 ? 0 : n);
+}
+
+module.exports = take;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/takeRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/takeRight.js b/node_modules/lodash/array/takeRight.js
new file mode 100644
index 0000000..6e89c87
--- /dev/null
+++ b/node_modules/lodash/array/takeRight.js
@@ -0,0 +1,40 @@
+var baseSlice = require('../internal/baseSlice'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates a slice of `array` with `n` elements taken from the end.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {number} [n=1] The number of elements to take.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.takeRight([1, 2, 3]);
+ * // => [3]
+ *
+ * _.takeRight([1, 2, 3], 2);
+ * // => [2, 3]
+ *
+ * _.takeRight([1, 2, 3], 5);
+ * // => [1, 2, 3]
+ *
+ * _.takeRight([1, 2, 3], 0);
+ * // => []
+ */
+function takeRight(array, n, guard) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (guard ? isIterateeCall(array, n, guard) : n == null) {
+ n = 1;
+ }
+ n = length - (+n || 0);
+ return baseSlice(array, n < 0 ? 0 : n);
+}
+
+module.exports = takeRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/takeRightWhile.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/takeRightWhile.js b/node_modules/lodash/array/takeRightWhile.js
new file mode 100644
index 0000000..5464d13
--- /dev/null
+++ b/node_modules/lodash/array/takeRightWhile.js
@@ -0,0 +1,59 @@
+var baseCallback = require('../internal/baseCallback'),
+ baseWhile = require('../internal/baseWhile');
+
+/**
+ * Creates a slice of `array` with elements taken from the end. Elements are
+ * taken until `predicate` returns falsey. The predicate is bound to `thisArg`
+ * and invoked with three arguments: (value, index, array).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.takeRightWhile([1, 2, 3], function(n) {
+ * return n > 1;
+ * });
+ * // => [2, 3]
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false },
+ * { 'user': 'pebbles', 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.pluck(_.takeRightWhile(users, { 'user': 'pebbles', 'active': false }), 'user');
+ * // => ['pebbles']
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.pluck(_.takeRightWhile(users, 'active', false), 'user');
+ * // => ['fred', 'pebbles']
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.takeRightWhile(users, 'active'), 'user');
+ * // => []
+ */
+function takeRightWhile(array, predicate, thisArg) {
+ return (array && array.length)
+ ? baseWhile(array, baseCallback(predicate, thisArg, 3), false, true)
+ : [];
+}
+
+module.exports = takeRightWhile;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/takeWhile.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/takeWhile.js b/node_modules/lodash/array/takeWhile.js
new file mode 100644
index 0000000..f7e28a1
--- /dev/null
+++ b/node_modules/lodash/array/takeWhile.js
@@ -0,0 +1,59 @@
+var baseCallback = require('../internal/baseCallback'),
+ baseWhile = require('../internal/baseWhile');
+
+/**
+ * Creates a slice of `array` with elements taken from the beginning. Elements
+ * are taken until `predicate` returns falsey. The predicate is bound to
+ * `thisArg` and invoked with three arguments: (value, index, array).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the slice of `array`.
+ * @example
+ *
+ * _.takeWhile([1, 2, 3], function(n) {
+ * return n < 3;
+ * });
+ * // => [1, 2]
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false},
+ * { 'user': 'pebbles', 'active': true }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.pluck(_.takeWhile(users, { 'user': 'barney', 'active': false }), 'user');
+ * // => ['barney']
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.pluck(_.takeWhile(users, 'active', false), 'user');
+ * // => ['barney', 'fred']
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.takeWhile(users, 'active'), 'user');
+ * // => []
+ */
+function takeWhile(array, predicate, thisArg) {
+ return (array && array.length)
+ ? baseWhile(array, baseCallback(predicate, thisArg, 3))
+ : [];
+}
+
+module.exports = takeWhile;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/union.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/union.js b/node_modules/lodash/array/union.js
new file mode 100644
index 0000000..53cefe4
--- /dev/null
+++ b/node_modules/lodash/array/union.js
@@ -0,0 +1,24 @@
+var baseFlatten = require('../internal/baseFlatten'),
+ baseUniq = require('../internal/baseUniq'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates an array of unique values, in order, from all of the provided arrays
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of combined values.
+ * @example
+ *
+ * _.union([1, 2], [4, 2], [2, 1]);
+ * // => [1, 2, 4]
+ */
+var union = restParam(function(arrays) {
+ return baseUniq(baseFlatten(arrays, false, true));
+});
+
+module.exports = union;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/uniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/uniq.js b/node_modules/lodash/array/uniq.js
new file mode 100644
index 0000000..ae937ef
--- /dev/null
+++ b/node_modules/lodash/array/uniq.js
@@ -0,0 +1,71 @@
+var baseCallback = require('../internal/baseCallback'),
+ baseUniq = require('../internal/baseUniq'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ sortedUniq = require('../internal/sortedUniq');
+
+/**
+ * Creates a duplicate-free version of an array, using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons, in which only the first occurence of each element
+ * is kept. Providing `true` for `isSorted` performs a faster search algorithm
+ * for sorted arrays. If an iteratee function is provided it's invoked for
+ * each element in the array to generate the criterion by which uniqueness
+ * is computed. The `iteratee` is bound to `thisArg` and invoked with three
+ * arguments: (value, index, array).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias unique
+ * @category Array
+ * @param {Array} array The array to inspect.
+ * @param {boolean} [isSorted] Specify the array is sorted.
+ * @param {Function|Object|string} [iteratee] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array} Returns the new duplicate-value-free array.
+ * @example
+ *
+ * _.uniq([2, 1, 2]);
+ * // => [2, 1]
+ *
+ * // using `isSorted`
+ * _.uniq([1, 1, 2], true);
+ * // => [1, 2]
+ *
+ * // using an iteratee function
+ * _.uniq([1, 2.5, 1.5, 2], function(n) {
+ * return this.floor(n);
+ * }, Math);
+ * // => [1, 2.5]
+ *
+ * // using the `_.property` callback shorthand
+ * _.uniq([{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+function uniq(array, isSorted, iteratee, thisArg) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ if (isSorted != null && typeof isSorted != 'boolean') {
+ thisArg = iteratee;
+ iteratee = isIterateeCall(array, isSorted, thisArg) ? undefined : isSorted;
+ isSorted = false;
+ }
+ iteratee = iteratee == null ? iteratee : baseCallback(iteratee, thisArg, 3);
+ return (isSorted)
+ ? sortedUniq(array, iteratee)
+ : baseUniq(array, iteratee);
+}
+
+module.exports = uniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/unique.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/unique.js b/node_modules/lodash/array/unique.js
new file mode 100644
index 0000000..396de1b
--- /dev/null
+++ b/node_modules/lodash/array/unique.js
@@ -0,0 +1 @@
+module.exports = require('./uniq');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/unzip.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/unzip.js b/node_modules/lodash/array/unzip.js
new file mode 100644
index 0000000..0a539fa
--- /dev/null
+++ b/node_modules/lodash/array/unzip.js
@@ -0,0 +1,47 @@
+var arrayFilter = require('../internal/arrayFilter'),
+ arrayMap = require('../internal/arrayMap'),
+ baseProperty = require('../internal/baseProperty'),
+ isArrayLike = require('../internal/isArrayLike');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * This method is like `_.zip` except that it accepts an array of grouped
+ * elements and creates an array regrouping the elements to their pre-zip
+ * configuration.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array of grouped elements to process.
+ * @returns {Array} Returns the new array of regrouped elements.
+ * @example
+ *
+ * var zipped = _.zip(['fred', 'barney'], [30, 40], [true, false]);
+ * // => [['fred', 30, true], ['barney', 40, false]]
+ *
+ * _.unzip(zipped);
+ * // => [['fred', 'barney'], [30, 40], [true, false]]
+ */
+function unzip(array) {
+ if (!(array && array.length)) {
+ return [];
+ }
+ var index = -1,
+ length = 0;
+
+ array = arrayFilter(array, function(group) {
+ if (isArrayLike(group)) {
+ length = nativeMax(group.length, length);
+ return true;
+ }
+ });
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = arrayMap(array, baseProperty(index));
+ }
+ return result;
+}
+
+module.exports = unzip;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/unzipWith.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/unzipWith.js b/node_modules/lodash/array/unzipWith.js
new file mode 100644
index 0000000..324a2b1
--- /dev/null
+++ b/node_modules/lodash/array/unzipWith.js
@@ -0,0 +1,41 @@
+var arrayMap = require('../internal/arrayMap'),
+ arrayReduce = require('../internal/arrayReduce'),
+ bindCallback = require('../internal/bindCallback'),
+ unzip = require('./unzip');
+
+/**
+ * This method is like `_.unzip` except that it accepts an iteratee to specify
+ * how regrouped values should be combined. The `iteratee` is bound to `thisArg`
+ * and invoked with four arguments: (accumulator, value, index, group).
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array of grouped elements to process.
+ * @param {Function} [iteratee] The function to combine regrouped values.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array} Returns the new array of regrouped elements.
+ * @example
+ *
+ * var zipped = _.zip([1, 2], [10, 20], [100, 200]);
+ * // => [[1, 10, 100], [2, 20, 200]]
+ *
+ * _.unzipWith(zipped, _.add);
+ * // => [3, 30, 300]
+ */
+function unzipWith(array, iteratee, thisArg) {
+ var length = array ? array.length : 0;
+ if (!length) {
+ return [];
+ }
+ var result = unzip(array);
+ if (iteratee == null) {
+ return result;
+ }
+ iteratee = bindCallback(iteratee, thisArg, 4);
+ return arrayMap(result, function(group) {
+ return arrayReduce(group, iteratee, undefined, true);
+ });
+}
+
+module.exports = unzipWith;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[50/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/abbrev.js
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/abbrev.js b/node_modules/abbrev/abbrev.js
new file mode 100644
index 0000000..69cfeac
--- /dev/null
+++ b/node_modules/abbrev/abbrev.js
@@ -0,0 +1,62 @@
+
+module.exports = exports = abbrev.abbrev = abbrev
+
+abbrev.monkeyPatch = monkeyPatch
+
+function monkeyPatch () {
+ Object.defineProperty(Array.prototype, 'abbrev', {
+ value: function () { return abbrev(this) },
+ enumerable: false, configurable: true, writable: true
+ })
+
+ Object.defineProperty(Object.prototype, 'abbrev', {
+ value: function () { return abbrev(Object.keys(this)) },
+ enumerable: false, configurable: true, writable: true
+ })
+}
+
+function abbrev (list) {
+ if (arguments.length !== 1 || !Array.isArray(list)) {
+ list = Array.prototype.slice.call(arguments, 0)
+ }
+ for (var i = 0, l = list.length, args = [] ; i < l ; i ++) {
+ args[i] = typeof list[i] === "string" ? list[i] : String(list[i])
+ }
+
+ // sort them lexicographically, so that they're next to their nearest kin
+ args = args.sort(lexSort)
+
+ // walk through each, seeing how much it has in common with the next and previous
+ var abbrevs = {}
+ , prev = ""
+ for (var i = 0, l = args.length ; i < l ; i ++) {
+ var current = args[i]
+ , next = args[i + 1] || ""
+ , nextMatches = true
+ , prevMatches = true
+ if (current === next) continue
+ for (var j = 0, cl = current.length ; j < cl ; j ++) {
+ var curChar = current.charAt(j)
+ nextMatches = nextMatches && curChar === next.charAt(j)
+ prevMatches = prevMatches && curChar === prev.charAt(j)
+ if (!nextMatches && !prevMatches) {
+ j ++
+ break
+ }
+ }
+ prev = current
+ if (j === cl) {
+ abbrevs[current] = current
+ continue
+ }
+ for (var a = current.substr(0, j) ; j <= cl ; j ++) {
+ abbrevs[a] = current
+ a += current.charAt(j)
+ }
+ }
+ return abbrevs
+}
+
+function lexSort (a, b) {
+ return a === b ? 0 : a > b ? 1 : -1
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/package.json
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/package.json b/node_modules/abbrev/package.json
new file mode 100644
index 0000000..3822a2d
--- /dev/null
+++ b/node_modules/abbrev/package.json
@@ -0,0 +1,74 @@
+{
+ "_args": [
+ [
+ "abbrev@1",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/nopt"
+ ]
+ ],
+ "_from": "abbrev@>=1.0.0 <2.0.0",
+ "_id": "abbrev@1.0.7",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/abbrev",
+ "_nodeVersion": "2.0.1",
+ "_npmUser": {
+ "email": "isaacs@npmjs.com",
+ "name": "isaacs"
+ },
+ "_npmVersion": "2.10.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "abbrev",
+ "raw": "abbrev@1",
+ "rawSpec": "1",
+ "scope": null,
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/nopt"
+ ],
+ "_resolved": "http://registry.npmjs.org/abbrev/-/abbrev-1.0.7.tgz",
+ "_shasum": "5b6035b2ee9d4fb5cf859f08a9be81b208491843",
+ "_shrinkwrap": null,
+ "_spec": "abbrev@1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/nopt",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/abbrev-js/issues"
+ },
+ "dependencies": {},
+ "description": "Like ruby's abbrev module, but in js",
+ "devDependencies": {
+ "tap": "^1.2.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "5b6035b2ee9d4fb5cf859f08a9be81b208491843",
+ "tarball": "http://registry.npmjs.org/abbrev/-/abbrev-1.0.7.tgz"
+ },
+ "gitHead": "821d09ce7da33627f91bbd8ed631497ed6f760c2",
+ "homepage": "https://github.com/isaacs/abbrev-js#readme",
+ "license": "ISC",
+ "main": "abbrev.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "name": "abbrev",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+ssh://git@github.com/isaacs/abbrev-js.git"
+ },
+ "scripts": {
+ "test": "tap test.js --cov"
+ },
+ "version": "1.0.7"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/abbrev/test.js
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/test.js b/node_modules/abbrev/test.js
new file mode 100644
index 0000000..eb30e42
--- /dev/null
+++ b/node_modules/abbrev/test.js
@@ -0,0 +1,47 @@
+var abbrev = require('./abbrev.js')
+var assert = require("assert")
+var util = require("util")
+
+console.log("TAP version 13")
+var count = 0
+
+function test (list, expect) {
+ count++
+ var actual = abbrev(list)
+ assert.deepEqual(actual, expect,
+ "abbrev("+util.inspect(list)+") === " + util.inspect(expect) + "\n"+
+ "actual: "+util.inspect(actual))
+ actual = abbrev.apply(exports, list)
+ assert.deepEqual(abbrev.apply(exports, list), expect,
+ "abbrev("+list.map(JSON.stringify).join(",")+") === " + util.inspect(expect) + "\n"+
+ "actual: "+util.inspect(actual))
+ console.log('ok - ' + list.join(' '))
+}
+
+test([ "ruby", "ruby", "rules", "rules", "rules" ],
+{ rub: 'ruby'
+, ruby: 'ruby'
+, rul: 'rules'
+, rule: 'rules'
+, rules: 'rules'
+})
+test(["fool", "foom", "pool", "pope"],
+{ fool: 'fool'
+, foom: 'foom'
+, poo: 'pool'
+, pool: 'pool'
+, pop: 'pope'
+, pope: 'pope'
+})
+test(["a", "ab", "abc", "abcd", "abcde", "acde"],
+{ a: 'a'
+, ab: 'ab'
+, abc: 'abc'
+, abcd: 'abcd'
+, abcde: 'abcde'
+, ac: 'acde'
+, acd: 'acde'
+, acde: 'acde'
+})
+
+console.log("1..%d", count)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/ansi/.jshintrc b/node_modules/ansi/.jshintrc
new file mode 100644
index 0000000..248c542
--- /dev/null
+++ b/node_modules/ansi/.jshintrc
@@ -0,0 +1,4 @@
+{
+ "laxcomma": true,
+ "asi": true
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/ansi/.npmignore b/node_modules/ansi/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/ansi/.npmignore
@@ -0,0 +1 @@
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/History.md
----------------------------------------------------------------------
diff --git a/node_modules/ansi/History.md b/node_modules/ansi/History.md
new file mode 100644
index 0000000..aea8aaf
--- /dev/null
+++ b/node_modules/ansi/History.md
@@ -0,0 +1,23 @@
+
+0.3.1 / 2016-01-14
+==================
+
+ * add MIT LICENSE file (#23, @kasicka)
+ * preserve chaining after redundant style-method calls (#19, @drewblaisdell)
+ * package: add "license" field (#16, @BenjaminTsai)
+
+0.3.0 / 2014-05-09
+==================
+
+ * package: remove "test" script and "devDependencies"
+ * package: remove "engines" section
+ * pacakge: remove "bin" section
+ * package: beautify
+ * examples: remove `starwars` example (#15)
+ * Documented goto, horizontalAbsolute, and eraseLine methods in README.md (#12, @Jammerwoch)
+ * add `.jshintrc` file
+
+< 0.3.0
+=======
+
+ * Prehistoric
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/ansi/LICENSE b/node_modules/ansi/LICENSE
new file mode 100644
index 0000000..2ea4dc5
--- /dev/null
+++ b/node_modules/ansi/LICENSE
@@ -0,0 +1,24 @@
+(The MIT License)
+
+Copyright (c) 2012 Nathan Rajlich <na...@tootallnate.net>
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/README.md
----------------------------------------------------------------------
diff --git a/node_modules/ansi/README.md b/node_modules/ansi/README.md
new file mode 100644
index 0000000..6ce1940
--- /dev/null
+++ b/node_modules/ansi/README.md
@@ -0,0 +1,98 @@
+ansi.js
+=========
+### Advanced ANSI formatting tool for Node.js
+
+`ansi.js` is a module for Node.js that provides an easy-to-use API for
+writing ANSI escape codes to `Stream` instances. ANSI escape codes are used to do
+fancy things in a terminal window, like render text in colors, delete characters,
+lines, the entire window, or hide and show the cursor, and lots more!
+
+#### Features:
+
+ * 256 color support for the terminal!
+ * Make a beep sound from your terminal!
+ * Works with *any* writable `Stream` instance.
+ * Allows you to move the cursor anywhere on the terminal window.
+ * Allows you to delete existing contents from the terminal window.
+ * Allows you to hide and show the cursor.
+ * Converts CSS color codes and RGB values into ANSI escape codes.
+ * Low-level; you are in control of when escape codes are used, it's not abstracted.
+
+
+Installation
+------------
+
+Install with `npm`:
+
+``` bash
+$ npm install ansi
+```
+
+
+Example
+-------
+
+``` js
+var ansi = require('ansi')
+ , cursor = ansi(process.stdout)
+
+// You can chain your calls forever:
+cursor
+ .red() // Set font color to red
+ .bg.grey() // Set background color to grey
+ .write('Hello World!') // Write 'Hello World!' to stdout
+ .bg.reset() // Reset the bgcolor before writing the trailing \n,
+ // to avoid Terminal glitches
+ .write('\n') // And a final \n to wrap things up
+
+// Rendering modes are persistent:
+cursor.hex('#660000').bold().underline()
+
+// You can use the regular logging functions, text will be green:
+console.log('This is blood red, bold text')
+
+// To reset just the foreground color:
+cursor.fg.reset()
+
+console.log('This will still be bold')
+
+// to go to a location (x,y) on the console
+// note: 1-indexed, not 0-indexed:
+cursor.goto(10, 5).write('Five down, ten over')
+
+// to clear the current line:
+cursor.horizontalAbsolute(0).eraseLine().write('Starting again')
+
+// to go to a different column on the current line:
+cursor.horizontalAbsolute(5).write('column five')
+
+// Clean up after yourself!
+cursor.reset()
+```
+
+
+License
+-------
+
+(The MIT License)
+
+Copyright (c) 2012 Nathan Rajlich <nathan@tootallnate.net>
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+'Software'), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
+IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
+CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
+TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
+SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/examples/beep/index.js
----------------------------------------------------------------------
diff --git a/node_modules/ansi/examples/beep/index.js b/node_modules/ansi/examples/beep/index.js
new file mode 100755
index 0000000..c1ec929
--- /dev/null
+++ b/node_modules/ansi/examples/beep/index.js
@@ -0,0 +1,16 @@
+#!/usr/bin/env node
+
+/**
+ * Invokes the terminal "beep" sound once per second on every exact second.
+ */
+
+process.title = 'beep'
+
+var cursor = require('../../')(process.stdout)
+
+function beep () {
+ cursor.beep()
+ setTimeout(beep, 1000 - (new Date()).getMilliseconds())
+}
+
+setTimeout(beep, 1000 - (new Date()).getMilliseconds())
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/examples/clear/index.js
----------------------------------------------------------------------
diff --git a/node_modules/ansi/examples/clear/index.js b/node_modules/ansi/examples/clear/index.js
new file mode 100755
index 0000000..6ac21ff
--- /dev/null
+++ b/node_modules/ansi/examples/clear/index.js
@@ -0,0 +1,15 @@
+#!/usr/bin/env node
+
+/**
+ * Like GNU ncurses "clear" command.
+ * https://github.com/mscdex/node-ncurses/blob/master/deps/ncurses/progs/clear.c
+ */
+
+process.title = 'clear'
+
+function lf () { return '\n' }
+
+require('../../')(process.stdout)
+ .write(Array.apply(null, Array(process.stdout.getWindowSize()[1])).map(lf).join(''))
+ .eraseData(2)
+ .goto(1, 1)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/examples/cursorPosition.js
----------------------------------------------------------------------
diff --git a/node_modules/ansi/examples/cursorPosition.js b/node_modules/ansi/examples/cursorPosition.js
new file mode 100755
index 0000000..50f9644
--- /dev/null
+++ b/node_modules/ansi/examples/cursorPosition.js
@@ -0,0 +1,32 @@
+#!/usr/bin/env node
+
+var tty = require('tty')
+var cursor = require('../')(process.stdout)
+
+// listen for the queryPosition report on stdin
+process.stdin.resume()
+raw(true)
+
+process.stdin.once('data', function (b) {
+ var match = /\[(\d+)\;(\d+)R$/.exec(b.toString())
+ if (match) {
+ var xy = match.slice(1, 3).reverse().map(Number)
+ console.error(xy)
+ }
+
+ // cleanup and close stdin
+ raw(false)
+ process.stdin.pause()
+})
+
+
+// send the query position request code to stdout
+cursor.queryPosition()
+
+function raw (mode) {
+ if (process.stdin.setRawMode) {
+ process.stdin.setRawMode(mode)
+ } else {
+ tty.setRawMode(mode)
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/examples/progress/index.js
----------------------------------------------------------------------
diff --git a/node_modules/ansi/examples/progress/index.js b/node_modules/ansi/examples/progress/index.js
new file mode 100644
index 0000000..d28dbda
--- /dev/null
+++ b/node_modules/ansi/examples/progress/index.js
@@ -0,0 +1,87 @@
+#!/usr/bin/env node
+
+var assert = require('assert')
+ , ansi = require('../../')
+
+function Progress (stream, width) {
+ this.cursor = ansi(stream)
+ this.delta = this.cursor.newlines
+ this.width = width | 0 || 10
+ this.open = '['
+ this.close = ']'
+ this.complete = '█'
+ this.incomplete = '_'
+
+ // initial render
+ this.progress = 0
+}
+
+Object.defineProperty(Progress.prototype, 'progress', {
+ get: get
+ , set: set
+ , configurable: true
+ , enumerable: true
+})
+
+function get () {
+ return this._progress
+}
+
+function set (v) {
+ this._progress = Math.max(0, Math.min(v, 100))
+
+ var w = this.width - this.complete.length - this.incomplete.length
+ , n = w * (this._progress / 100) | 0
+ , i = w - n
+ , com = c(this.complete, n)
+ , inc = c(this.incomplete, i)
+ , delta = this.cursor.newlines - this.delta
+
+ assert.equal(com.length + inc.length, w)
+
+ if (delta > 0) {
+ this.cursor.up(delta)
+ this.delta = this.cursor.newlines
+ }
+
+ this.cursor
+ .horizontalAbsolute(0)
+ .eraseLine(2)
+ .fg.white()
+ .write(this.open)
+ .fg.grey()
+ .bold()
+ .write(com)
+ .resetBold()
+ .write(inc)
+ .fg.white()
+ .write(this.close)
+ .fg.reset()
+ .write('\n')
+}
+
+function c (char, length) {
+ return Array.apply(null, Array(length)).map(function () {
+ return char
+ }).join('')
+}
+
+
+
+
+// Usage
+var width = parseInt(process.argv[2], 10) || process.stdout.getWindowSize()[0] / 2
+ , p = new Progress(process.stdout, width)
+
+;(function tick () {
+ p.progress += Math.random() * 5
+ p.cursor
+ .eraseLine(2)
+ .write('Progress: ')
+ .bold().write(p.progress.toFixed(2))
+ .write('%')
+ .resetBold()
+ .write('\n')
+ if (p.progress < 100)
+ setTimeout(tick, 100)
+})()
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/lib/ansi.js
----------------------------------------------------------------------
diff --git a/node_modules/ansi/lib/ansi.js b/node_modules/ansi/lib/ansi.js
new file mode 100644
index 0000000..b1714e3
--- /dev/null
+++ b/node_modules/ansi/lib/ansi.js
@@ -0,0 +1,405 @@
+
+/**
+ * References:
+ *
+ * - http://en.wikipedia.org/wiki/ANSI_escape_code
+ * - http://www.termsys.demon.co.uk/vtansi.htm
+ *
+ */
+
+/**
+ * Module dependencies.
+ */
+
+var emitNewlineEvents = require('./newlines')
+ , prefix = '\x1b[' // For all escape codes
+ , suffix = 'm' // Only for color codes
+
+/**
+ * The ANSI escape sequences.
+ */
+
+var codes = {
+ up: 'A'
+ , down: 'B'
+ , forward: 'C'
+ , back: 'D'
+ , nextLine: 'E'
+ , previousLine: 'F'
+ , horizontalAbsolute: 'G'
+ , eraseData: 'J'
+ , eraseLine: 'K'
+ , scrollUp: 'S'
+ , scrollDown: 'T'
+ , savePosition: 's'
+ , restorePosition: 'u'
+ , queryPosition: '6n'
+ , hide: '?25l'
+ , show: '?25h'
+}
+
+/**
+ * Rendering ANSI codes.
+ */
+
+var styles = {
+ bold: 1
+ , italic: 3
+ , underline: 4
+ , inverse: 7
+}
+
+/**
+ * The negating ANSI code for the rendering modes.
+ */
+
+var reset = {
+ bold: 22
+ , italic: 23
+ , underline: 24
+ , inverse: 27
+}
+
+/**
+ * The standard, styleable ANSI colors.
+ */
+
+var colors = {
+ white: 37
+ , black: 30
+ , blue: 34
+ , cyan: 36
+ , green: 32
+ , magenta: 35
+ , red: 31
+ , yellow: 33
+ , grey: 90
+ , brightBlack: 90
+ , brightRed: 91
+ , brightGreen: 92
+ , brightYellow: 93
+ , brightBlue: 94
+ , brightMagenta: 95
+ , brightCyan: 96
+ , brightWhite: 97
+}
+
+
+/**
+ * Creates a Cursor instance based off the given `writable stream` instance.
+ */
+
+function ansi (stream, options) {
+ if (stream._ansicursor) {
+ return stream._ansicursor
+ } else {
+ return stream._ansicursor = new Cursor(stream, options)
+ }
+}
+module.exports = exports = ansi
+
+/**
+ * The `Cursor` class.
+ */
+
+function Cursor (stream, options) {
+ if (!(this instanceof Cursor)) {
+ return new Cursor(stream, options)
+ }
+ if (typeof stream != 'object' || typeof stream.write != 'function') {
+ throw new Error('a valid Stream instance must be passed in')
+ }
+
+ // the stream to use
+ this.stream = stream
+
+ // when 'enabled' is false then all the functions are no-ops except for write()
+ this.enabled = options && options.enabled
+ if (typeof this.enabled === 'undefined') {
+ this.enabled = stream.isTTY
+ }
+ this.enabled = !!this.enabled
+
+ // then `buffering` is true, then `write()` calls are buffered in
+ // memory until `flush()` is invoked
+ this.buffering = !!(options && options.buffering)
+ this._buffer = []
+
+ // controls the foreground and background colors
+ this.fg = this.foreground = new Colorer(this, 0)
+ this.bg = this.background = new Colorer(this, 10)
+
+ // defaults
+ this.Bold = false
+ this.Italic = false
+ this.Underline = false
+ this.Inverse = false
+
+ // keep track of the number of "newlines" that get encountered
+ this.newlines = 0
+ emitNewlineEvents(stream)
+ stream.on('newline', function () {
+ this.newlines++
+ }.bind(this))
+}
+exports.Cursor = Cursor
+
+/**
+ * Helper function that calls `write()` on the underlying Stream.
+ * Returns `this` instead of the write() return value to keep
+ * the chaining going.
+ */
+
+Cursor.prototype.write = function (data) {
+ if (this.buffering) {
+ this._buffer.push(arguments)
+ } else {
+ this.stream.write.apply(this.stream, arguments)
+ }
+ return this
+}
+
+/**
+ * Buffer `write()` calls into memory.
+ *
+ * @api public
+ */
+
+Cursor.prototype.buffer = function () {
+ this.buffering = true
+ return this
+}
+
+/**
+ * Write out the in-memory buffer.
+ *
+ * @api public
+ */
+
+Cursor.prototype.flush = function () {
+ this.buffering = false
+ var str = this._buffer.map(function (args) {
+ if (args.length != 1) throw new Error('unexpected args length! ' + args.length);
+ return args[0];
+ }).join('');
+ this._buffer.splice(0); // empty
+ this.write(str);
+ return this
+}
+
+
+/**
+ * The `Colorer` class manages both the background and foreground colors.
+ */
+
+function Colorer (cursor, base) {
+ this.current = null
+ this.cursor = cursor
+ this.base = base
+}
+exports.Colorer = Colorer
+
+/**
+ * Write an ANSI color code, ensuring that the same code doesn't get rewritten.
+ */
+
+Colorer.prototype._setColorCode = function setColorCode (code) {
+ var c = String(code)
+ if (this.current === c) return
+ this.cursor.enabled && this.cursor.write(prefix + c + suffix)
+ this.current = c
+ return this
+}
+
+
+/**
+ * Set up the positional ANSI codes.
+ */
+
+Object.keys(codes).forEach(function (name) {
+ var code = String(codes[name])
+ Cursor.prototype[name] = function () {
+ var c = code
+ if (arguments.length > 0) {
+ c = toArray(arguments).map(Math.round).join(';') + code
+ }
+ this.enabled && this.write(prefix + c)
+ return this
+ }
+})
+
+/**
+ * Set up the functions for the rendering ANSI codes.
+ */
+
+Object.keys(styles).forEach(function (style) {
+ var name = style[0].toUpperCase() + style.substring(1)
+ , c = styles[style]
+ , r = reset[style]
+
+ Cursor.prototype[style] = function () {
+ if (this[name]) return this
+ this.enabled && this.write(prefix + c + suffix)
+ this[name] = true
+ return this
+ }
+
+ Cursor.prototype['reset' + name] = function () {
+ if (!this[name]) return this
+ this.enabled && this.write(prefix + r + suffix)
+ this[name] = false
+ return this
+ }
+})
+
+/**
+ * Setup the functions for the standard colors.
+ */
+
+Object.keys(colors).forEach(function (color) {
+ var code = colors[color]
+
+ Colorer.prototype[color] = function () {
+ this._setColorCode(this.base + code)
+ return this.cursor
+ }
+
+ Cursor.prototype[color] = function () {
+ return this.foreground[color]()
+ }
+})
+
+/**
+ * Makes a beep sound!
+ */
+
+Cursor.prototype.beep = function () {
+ this.enabled && this.write('\x07')
+ return this
+}
+
+/**
+ * Moves cursor to specific position
+ */
+
+Cursor.prototype.goto = function (x, y) {
+ x = x | 0
+ y = y | 0
+ this.enabled && this.write(prefix + y + ';' + x + 'H')
+ return this
+}
+
+/**
+ * Resets the color.
+ */
+
+Colorer.prototype.reset = function () {
+ this._setColorCode(this.base + 39)
+ return this.cursor
+}
+
+/**
+ * Resets all ANSI formatting on the stream.
+ */
+
+Cursor.prototype.reset = function () {
+ this.enabled && this.write(prefix + '0' + suffix)
+ this.Bold = false
+ this.Italic = false
+ this.Underline = false
+ this.Inverse = false
+ this.foreground.current = null
+ this.background.current = null
+ return this
+}
+
+/**
+ * Sets the foreground color with the given RGB values.
+ * The closest match out of the 216 colors is picked.
+ */
+
+Colorer.prototype.rgb = function (r, g, b) {
+ var base = this.base + 38
+ , code = rgb(r, g, b)
+ this._setColorCode(base + ';5;' + code)
+ return this.cursor
+}
+
+/**
+ * Same as `cursor.fg.rgb(r, g, b)`.
+ */
+
+Cursor.prototype.rgb = function (r, g, b) {
+ return this.foreground.rgb(r, g, b)
+}
+
+/**
+ * Accepts CSS color codes for use with ANSI escape codes.
+ * For example: `#FF000` would be bright red.
+ */
+
+Colorer.prototype.hex = function (color) {
+ return this.rgb.apply(this, hex(color))
+}
+
+/**
+ * Same as `cursor.fg.hex(color)`.
+ */
+
+Cursor.prototype.hex = function (color) {
+ return this.foreground.hex(color)
+}
+
+
+// UTIL FUNCTIONS //
+
+/**
+ * Translates a 255 RGB value to a 0-5 ANSI RGV value,
+ * then returns the single ANSI color code to use.
+ */
+
+function rgb (r, g, b) {
+ var red = r / 255 * 5
+ , green = g / 255 * 5
+ , blue = b / 255 * 5
+ return rgb5(red, green, blue)
+}
+
+/**
+ * Turns rgb 0-5 values into a single ANSI color code to use.
+ */
+
+function rgb5 (r, g, b) {
+ var red = Math.round(r)
+ , green = Math.round(g)
+ , blue = Math.round(b)
+ return 16 + (red*36) + (green*6) + blue
+}
+
+/**
+ * Accepts a hex CSS color code string (# is optional) and
+ * translates it into an Array of 3 RGB 0-255 values, which
+ * can then be used with rgb().
+ */
+
+function hex (color) {
+ var c = color[0] === '#' ? color.substring(1) : color
+ , r = c.substring(0, 2)
+ , g = c.substring(2, 4)
+ , b = c.substring(4, 6)
+ return [parseInt(r, 16), parseInt(g, 16), parseInt(b, 16)]
+}
+
+/**
+ * Turns an array-like object into a real array.
+ */
+
+function toArray (a) {
+ var i = 0
+ , l = a.length
+ , rtn = []
+ for (; i<l; i++) {
+ rtn.push(a[i])
+ }
+ return rtn
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/lib/newlines.js
----------------------------------------------------------------------
diff --git a/node_modules/ansi/lib/newlines.js b/node_modules/ansi/lib/newlines.js
new file mode 100644
index 0000000..4e37a0a
--- /dev/null
+++ b/node_modules/ansi/lib/newlines.js
@@ -0,0 +1,71 @@
+
+/**
+ * Accepts any node Stream instance and hijacks its "write()" function,
+ * so that it can count any newlines that get written to the output.
+ *
+ * When a '\n' byte is encountered, then a "newline" event will be emitted
+ * on the stream, with no arguments. It is up to the listeners to determine
+ * any necessary deltas required for their use-case.
+ *
+ * Ex:
+ *
+ * var cursor = ansi(process.stdout)
+ * , ln = 0
+ * process.stdout.on('newline', function () {
+ * ln++
+ * })
+ */
+
+/**
+ * Module dependencies.
+ */
+
+var assert = require('assert')
+var NEWLINE = '\n'.charCodeAt(0)
+
+function emitNewlineEvents (stream) {
+ if (stream._emittingNewlines) {
+ // already emitting newline events
+ return
+ }
+
+ var write = stream.write
+
+ stream.write = function (data) {
+ // first write the data
+ var rtn = write.apply(stream, arguments)
+
+ if (stream.listeners('newline').length > 0) {
+ var len = data.length
+ , i = 0
+ // now try to calculate any deltas
+ if (typeof data == 'string') {
+ for (; i<len; i++) {
+ processByte(stream, data.charCodeAt(i))
+ }
+ } else {
+ // buffer
+ for (; i<len; i++) {
+ processByte(stream, data[i])
+ }
+ }
+ }
+
+ return rtn
+ }
+
+ stream._emittingNewlines = true
+}
+module.exports = emitNewlineEvents
+
+
+/**
+ * Processes an individual byte being written to a stream
+ */
+
+function processByte (stream, b) {
+ assert.equal(typeof b, 'number')
+ if (b === NEWLINE) {
+ stream.emit('newline')
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/ansi/package.json
----------------------------------------------------------------------
diff --git a/node_modules/ansi/package.json b/node_modules/ansi/package.json
new file mode 100644
index 0000000..44bf5f4
--- /dev/null
+++ b/node_modules/ansi/package.json
@@ -0,0 +1,85 @@
+{
+ "_args": [
+ [
+ "ansi@^0.3.1",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "ansi@>=0.3.1 <0.4.0",
+ "_id": "ansi@0.3.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/ansi",
+ "_nodeVersion": "5.3.0",
+ "_npmUser": {
+ "email": "nathan@tootallnate.net",
+ "name": "tootallnate"
+ },
+ "_npmVersion": "3.3.12",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "ansi",
+ "raw": "ansi@^0.3.1",
+ "rawSpec": "^0.3.1",
+ "scope": null,
+ "spec": ">=0.3.1 <0.4.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/ansi/-/ansi-0.3.1.tgz",
+ "_shasum": "0c42d4fb17160d5a9af1e484bace1c66922c1b21",
+ "_shrinkwrap": null,
+ "_spec": "ansi@^0.3.1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "email": "nathan@tootallnate.net",
+ "name": "Nathan Rajlich",
+ "url": "http://tootallnate.net"
+ },
+ "bugs": {
+ "url": "https://github.com/TooTallNate/ansi.js/issues"
+ },
+ "dependencies": {},
+ "description": "Advanced ANSI formatting tool for Node.js",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "0c42d4fb17160d5a9af1e484bace1c66922c1b21",
+ "tarball": "http://registry.npmjs.org/ansi/-/ansi-0.3.1.tgz"
+ },
+ "gitHead": "4d0d4af94e0bdaa648bd7262acd3bde4b98d5246",
+ "homepage": "https://github.com/TooTallNate/ansi.js#readme",
+ "keywords": [
+ "256",
+ "ansi",
+ "color",
+ "cursor",
+ "formatting",
+ "rgb",
+ "stream",
+ "terminal"
+ ],
+ "license": "MIT",
+ "main": "./lib/ansi.js",
+ "maintainers": [
+ {
+ "name": "TooTallNate",
+ "email": "nathan@tootallnate.net"
+ },
+ {
+ "name": "tootallnate",
+ "email": "nathan@tootallnate.net"
+ }
+ ],
+ "name": "ansi",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/TooTallNate/ansi.js.git"
+ },
+ "scripts": {},
+ "version": "0.3.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/.npmignore b/node_modules/balanced-match/.npmignore
new file mode 100644
index 0000000..fd4f2b0
--- /dev/null
+++ b/node_modules/balanced-match/.npmignore
@@ -0,0 +1,2 @@
+node_modules
+.DS_Store
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/.travis.yml b/node_modules/balanced-match/.travis.yml
new file mode 100644
index 0000000..6e5919d
--- /dev/null
+++ b/node_modules/balanced-match/.travis.yml
@@ -0,0 +1,3 @@
+language: node_js
+node_js:
+ - "0.10"
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/LICENSE.md
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/LICENSE.md b/node_modules/balanced-match/LICENSE.md
new file mode 100644
index 0000000..2cdc8e4
--- /dev/null
+++ b/node_modules/balanced-match/LICENSE.md
@@ -0,0 +1,21 @@
+(MIT)
+
+Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
+of the Software, and to permit persons to whom the Software is furnished to do
+so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/Makefile
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/Makefile b/node_modules/balanced-match/Makefile
new file mode 100644
index 0000000..fa5da71
--- /dev/null
+++ b/node_modules/balanced-match/Makefile
@@ -0,0 +1,6 @@
+
+test:
+ @node_modules/.bin/tape test/*.js
+
+.PHONY: test
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/README.md
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/README.md b/node_modules/balanced-match/README.md
new file mode 100644
index 0000000..421f3aa
--- /dev/null
+++ b/node_modules/balanced-match/README.md
@@ -0,0 +1,89 @@
+# balanced-match
+
+Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`.
+
+[](http://travis-ci.org/juliangruber/balanced-match)
+[](https://www.npmjs.org/package/balanced-match)
+
+[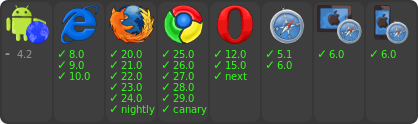](https://ci.testling.com/juliangruber/balanced-match)
+
+## Example
+
+Get the first matching pair of braces:
+
+```js
+var balanced = require('balanced-match');
+
+console.log(balanced('{', '}', 'pre{in{nested}}post'));
+console.log(balanced('{', '}', 'pre{first}between{second}post'));
+```
+
+The matches are:
+
+```bash
+$ node example.js
+{ start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' }
+{ start: 3,
+ end: 9,
+ pre: 'pre',
+ body: 'first',
+ post: 'between{second}post' }
+```
+
+## API
+
+### var m = balanced(a, b, str)
+
+For the first non-nested matching pair of `a` and `b` in `str`, return an
+object with those keys:
+
+* **start** the index of the first match of `a`
+* **end** the index of the matching `b`
+* **pre** the preamble, `a` and `b` not included
+* **body** the match, `a` and `b` not included
+* **post** the postscript, `a` and `b` not included
+
+If there's no match, `undefined` will be returned.
+
+If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']`.
+
+### var r = balanced.range(a, b, str)
+
+For the first non-nested matching pair of `a` and `b` in `str`, return an
+array with indexes: `[ <a index>, <b index> ]`.
+
+If there's no match, `undefined` will be returned.
+
+If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `[ 1, 3 ]`.
+
+## Installation
+
+With [npm](https://npmjs.org) do:
+
+```bash
+npm install balanced-match
+```
+
+## License
+
+(MIT)
+
+Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
+of the Software, and to permit persons to whom the Software is furnished to do
+so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/example.js
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/example.js b/node_modules/balanced-match/example.js
new file mode 100644
index 0000000..c02ad34
--- /dev/null
+++ b/node_modules/balanced-match/example.js
@@ -0,0 +1,5 @@
+var balanced = require('./');
+
+console.log(balanced('{', '}', 'pre{in{nested}}post'));
+console.log(balanced('{', '}', 'pre{first}between{second}post'));
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/index.js
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/index.js b/node_modules/balanced-match/index.js
new file mode 100644
index 0000000..75f3d71
--- /dev/null
+++ b/node_modules/balanced-match/index.js
@@ -0,0 +1,50 @@
+module.exports = balanced;
+function balanced(a, b, str) {
+ var r = range(a, b, str);
+
+ return r && {
+ start: r[0],
+ end: r[1],
+ pre: str.slice(0, r[0]),
+ body: str.slice(r[0] + a.length, r[1]),
+ post: str.slice(r[1] + b.length)
+ };
+}
+
+balanced.range = range;
+function range(a, b, str) {
+ var begs, beg, left, right, result;
+ var ai = str.indexOf(a);
+ var bi = str.indexOf(b, ai + 1);
+ var i = ai;
+
+ if (ai >= 0 && bi > 0) {
+ begs = [];
+ left = str.length;
+
+ while (i < str.length && i >= 0 && ! result) {
+ if (i == ai) {
+ begs.push(i);
+ ai = str.indexOf(a, i + 1);
+ } else if (begs.length == 1) {
+ result = [ begs.pop(), bi ];
+ } else {
+ beg = begs.pop();
+ if (beg < left) {
+ left = beg;
+ right = bi;
+ }
+
+ bi = str.indexOf(b, i + 1);
+ }
+
+ i = ai < bi && ai >= 0 ? ai : bi;
+ }
+
+ if (begs.length) {
+ result = [ left, right ];
+ }
+ }
+
+ return result;
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/package.json
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/package.json b/node_modules/balanced-match/package.json
new file mode 100644
index 0000000..18a029a
--- /dev/null
+++ b/node_modules/balanced-match/package.json
@@ -0,0 +1,98 @@
+{
+ "_args": [
+ [
+ "balanced-match@^0.3.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/brace-expansion"
+ ]
+ ],
+ "_from": "balanced-match@>=0.3.0 <0.4.0",
+ "_id": "balanced-match@0.3.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/balanced-match",
+ "_nodeVersion": "4.2.1",
+ "_npmUser": {
+ "email": "julian@juliangruber.com",
+ "name": "juliangruber"
+ },
+ "_npmVersion": "2.14.7",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "balanced-match",
+ "raw": "balanced-match@^0.3.0",
+ "rawSpec": "^0.3.0",
+ "scope": null,
+ "spec": ">=0.3.0 <0.4.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/brace-expansion"
+ ],
+ "_resolved": "http://registry.npmjs.org/balanced-match/-/balanced-match-0.3.0.tgz",
+ "_shasum": "a91cdd1ebef1a86659e70ff4def01625fc2d6756",
+ "_shrinkwrap": null,
+ "_spec": "balanced-match@^0.3.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/brace-expansion",
+ "author": {
+ "email": "mail@juliangruber.com",
+ "name": "Julian Gruber",
+ "url": "http://juliangruber.com"
+ },
+ "bugs": {
+ "url": "https://github.com/juliangruber/balanced-match/issues"
+ },
+ "dependencies": {},
+ "description": "Match balanced character pairs, like \"{\" and \"}\"",
+ "devDependencies": {
+ "tape": "~4.2.2"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "a91cdd1ebef1a86659e70ff4def01625fc2d6756",
+ "tarball": "http://registry.npmjs.org/balanced-match/-/balanced-match-0.3.0.tgz"
+ },
+ "gitHead": "a7114b0986554787e90b7ac595a043ca75ea77e5",
+ "homepage": "https://github.com/juliangruber/balanced-match",
+ "keywords": [
+ "balanced",
+ "match",
+ "parse",
+ "regexp",
+ "test"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "juliangruber",
+ "email": "julian@juliangruber.com"
+ }
+ ],
+ "name": "balanced-match",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/juliangruber/balanced-match.git"
+ },
+ "scripts": {
+ "test": "make test"
+ },
+ "testling": {
+ "browsers": [
+ "android-browser/4.2..latest",
+ "chrome/25..latest",
+ "chrome/canary",
+ "firefox/20..latest",
+ "firefox/nightly",
+ "ie/8..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest"
+ ],
+ "files": "test/*.js"
+ },
+ "version": "0.3.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/balanced-match/test/balanced.js
----------------------------------------------------------------------
diff --git a/node_modules/balanced-match/test/balanced.js b/node_modules/balanced-match/test/balanced.js
new file mode 100644
index 0000000..f5e98e3
--- /dev/null
+++ b/node_modules/balanced-match/test/balanced.js
@@ -0,0 +1,84 @@
+var test = require('tape');
+var balanced = require('..');
+
+test('balanced', function(t) {
+ t.deepEqual(balanced('{', '}', 'pre{in{nest}}post'), {
+ start: 3,
+ end: 12,
+ pre: 'pre',
+ body: 'in{nest}',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', '{{{{{{{{{in}post'), {
+ start: 8,
+ end: 11,
+ pre: '{{{{{{{{',
+ body: 'in',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre{body{in}post'), {
+ start: 8,
+ end: 11,
+ pre: 'pre{body',
+ body: 'in',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre}{in{nest}}post'), {
+ start: 4,
+ end: 13,
+ pre: 'pre}',
+ body: 'in{nest}',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre{body}between{body2}post'), {
+ start: 3,
+ end: 8,
+ pre: 'pre',
+ body: 'body',
+ post: 'between{body2}post'
+ });
+ t.notOk(balanced('{', '}', 'nope'), 'should be notOk');
+ t.deepEqual(balanced('<b>', '</b>', 'pre<b>in<b>nest</b></b>post'), {
+ start: 3,
+ end: 19,
+ pre: 'pre',
+ body: 'in<b>nest</b>',
+ post: 'post'
+ });
+ t.deepEqual(balanced('<b>', '</b>', 'pre</b><b>in<b>nest</b></b>post'), {
+ start: 7,
+ end: 23,
+ pre: 'pre</b>',
+ body: 'in<b>nest</b>',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{{', '}}', 'pre{{{in}}}post'), {
+ start: 3,
+ end: 9,
+ pre: 'pre',
+ body: '{in}',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{{{', '}}', 'pre{{{in}}}post'), {
+ start: 3,
+ end: 8,
+ pre: 'pre',
+ body: 'in',
+ post: '}post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre{{first}in{second}post'), {
+ start: 4,
+ end: 10,
+ pre: 'pre{',
+ body: 'first',
+ post: 'in{second}post'
+ });
+ t.deepEqual(balanced('<?', '?>', 'pre<?>post'), {
+ start: 3,
+ end: 4,
+ pre: 'pre',
+ body: '',
+ post: 'post'
+ });
+ t.end();
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/.travis.yml b/node_modules/base64-js/.travis.yml
new file mode 100644
index 0000000..939cb51
--- /dev/null
+++ b/node_modules/base64-js/.travis.yml
@@ -0,0 +1,5 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
+ - "0.11"
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/LICENSE.MIT
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/LICENSE.MIT b/node_modules/base64-js/LICENSE.MIT
new file mode 100644
index 0000000..96d3f68
--- /dev/null
+++ b/node_modules/base64-js/LICENSE.MIT
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2014
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/README.md
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/README.md b/node_modules/base64-js/README.md
new file mode 100644
index 0000000..ed31d1a
--- /dev/null
+++ b/node_modules/base64-js/README.md
@@ -0,0 +1,31 @@
+base64-js
+=========
+
+`base64-js` does basic base64 encoding/decoding in pure JS.
+
+[](http://travis-ci.org/beatgammit/base64-js)
+
+[](https://ci.testling.com/beatgammit/base64-js)
+
+Many browsers already have base64 encoding/decoding functionality, but it is for text data, not all-purpose binary data.
+
+Sometimes encoding/decoding binary data in the browser is useful, and that is what this module does.
+
+## install
+
+With [npm](https://npmjs.org) do:
+
+`npm install base64-js`
+
+## methods
+
+`var base64 = require('base64-js')`
+
+`base64` has two exposed functions, `toByteArray` and `fromByteArray`, which both take a single argument.
+
+* `toByteArray` - Takes a base64 string and returns a byte array
+* `fromByteArray` - Takes a byte array and returns a base64 string
+
+## license
+
+MIT
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/bench/bench.js
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/bench/bench.js b/node_modules/base64-js/bench/bench.js
new file mode 100644
index 0000000..0689e08
--- /dev/null
+++ b/node_modules/base64-js/bench/bench.js
@@ -0,0 +1,19 @@
+var random = require('crypto').pseudoRandomBytes
+
+var b64 = require('../')
+var fs = require('fs')
+var path = require('path')
+var data = random(1e6).toString('base64')
+//fs.readFileSync(path.join(__dirname, 'example.b64'), 'ascii').split('\n').join('')
+var start = Date.now()
+var raw = b64.toByteArray(data)
+var middle = Date.now()
+var data = b64.fromByteArray(raw)
+var end = Date.now()
+
+console.log('decode ms, decode ops/ms, encode ms, encode ops/ms')
+console.log(
+ middle - start, data.length / (middle - start),
+ end - middle, data.length / (end - middle))
+//console.log(data)
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/lib/b64.js
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/lib/b64.js b/node_modules/base64-js/lib/b64.js
new file mode 100644
index 0000000..46001d2
--- /dev/null
+++ b/node_modules/base64-js/lib/b64.js
@@ -0,0 +1,124 @@
+var lookup = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+;(function (exports) {
+ 'use strict';
+
+ var Arr = (typeof Uint8Array !== 'undefined')
+ ? Uint8Array
+ : Array
+
+ var PLUS = '+'.charCodeAt(0)
+ var SLASH = '/'.charCodeAt(0)
+ var NUMBER = '0'.charCodeAt(0)
+ var LOWER = 'a'.charCodeAt(0)
+ var UPPER = 'A'.charCodeAt(0)
+ var PLUS_URL_SAFE = '-'.charCodeAt(0)
+ var SLASH_URL_SAFE = '_'.charCodeAt(0)
+
+ function decode (elt) {
+ var code = elt.charCodeAt(0)
+ if (code === PLUS ||
+ code === PLUS_URL_SAFE)
+ return 62 // '+'
+ if (code === SLASH ||
+ code === SLASH_URL_SAFE)
+ return 63 // '/'
+ if (code < NUMBER)
+ return -1 //no match
+ if (code < NUMBER + 10)
+ return code - NUMBER + 26 + 26
+ if (code < UPPER + 26)
+ return code - UPPER
+ if (code < LOWER + 26)
+ return code - LOWER + 26
+ }
+
+ function b64ToByteArray (b64) {
+ var i, j, l, tmp, placeHolders, arr
+
+ if (b64.length % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4')
+ }
+
+ // the number of equal signs (place holders)
+ // if there are two placeholders, than the two characters before it
+ // represent one byte
+ // if there is only one, then the three characters before it represent 2 bytes
+ // this is just a cheap hack to not do indexOf twice
+ var len = b64.length
+ placeHolders = '=' === b64.charAt(len - 2) ? 2 : '=' === b64.charAt(len - 1) ? 1 : 0
+
+ // base64 is 4/3 + up to two characters of the original data
+ arr = new Arr(b64.length * 3 / 4 - placeHolders)
+
+ // if there are placeholders, only get up to the last complete 4 chars
+ l = placeHolders > 0 ? b64.length - 4 : b64.length
+
+ var L = 0
+
+ function push (v) {
+ arr[L++] = v
+ }
+
+ for (i = 0, j = 0; i < l; i += 4, j += 3) {
+ tmp = (decode(b64.charAt(i)) << 18) | (decode(b64.charAt(i + 1)) << 12) | (decode(b64.charAt(i + 2)) << 6) | decode(b64.charAt(i + 3))
+ push((tmp & 0xFF0000) >> 16)
+ push((tmp & 0xFF00) >> 8)
+ push(tmp & 0xFF)
+ }
+
+ if (placeHolders === 2) {
+ tmp = (decode(b64.charAt(i)) << 2) | (decode(b64.charAt(i + 1)) >> 4)
+ push(tmp & 0xFF)
+ } else if (placeHolders === 1) {
+ tmp = (decode(b64.charAt(i)) << 10) | (decode(b64.charAt(i + 1)) << 4) | (decode(b64.charAt(i + 2)) >> 2)
+ push((tmp >> 8) & 0xFF)
+ push(tmp & 0xFF)
+ }
+
+ return arr
+ }
+
+ function uint8ToBase64 (uint8) {
+ var i,
+ extraBytes = uint8.length % 3, // if we have 1 byte left, pad 2 bytes
+ output = "",
+ temp, length
+
+ function encode (num) {
+ return lookup.charAt(num)
+ }
+
+ function tripletToBase64 (num) {
+ return encode(num >> 18 & 0x3F) + encode(num >> 12 & 0x3F) + encode(num >> 6 & 0x3F) + encode(num & 0x3F)
+ }
+
+ // go through the array every three bytes, we'll deal with trailing stuff later
+ for (i = 0, length = uint8.length - extraBytes; i < length; i += 3) {
+ temp = (uint8[i] << 16) + (uint8[i + 1] << 8) + (uint8[i + 2])
+ output += tripletToBase64(temp)
+ }
+
+ // pad the end with zeros, but make sure to not forget the extra bytes
+ switch (extraBytes) {
+ case 1:
+ temp = uint8[uint8.length - 1]
+ output += encode(temp >> 2)
+ output += encode((temp << 4) & 0x3F)
+ output += '=='
+ break
+ case 2:
+ temp = (uint8[uint8.length - 2] << 8) + (uint8[uint8.length - 1])
+ output += encode(temp >> 10)
+ output += encode((temp >> 4) & 0x3F)
+ output += encode((temp << 2) & 0x3F)
+ output += '='
+ break
+ }
+
+ return output
+ }
+
+ exports.toByteArray = b64ToByteArray
+ exports.fromByteArray = uint8ToBase64
+}(typeof exports === 'undefined' ? (this.base64js = {}) : exports))
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/package.json
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/package.json b/node_modules/base64-js/package.json
new file mode 100644
index 0000000..c9eaf4d
--- /dev/null
+++ b/node_modules/base64-js/package.json
@@ -0,0 +1,93 @@
+{
+ "_args": [
+ [
+ "base64-js@0.0.8",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/plist"
+ ]
+ ],
+ "_from": "base64-js@0.0.8",
+ "_id": "base64-js@0.0.8",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/base64-js",
+ "_nodeVersion": "0.10.35",
+ "_npmUser": {
+ "email": "feross@feross.org",
+ "name": "feross"
+ },
+ "_npmVersion": "2.1.16",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "base64-js",
+ "raw": "base64-js@0.0.8",
+ "rawSpec": "0.0.8",
+ "scope": null,
+ "spec": "0.0.8",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/plist"
+ ],
+ "_resolved": "http://registry.npmjs.org/base64-js/-/base64-js-0.0.8.tgz",
+ "_shasum": "1101e9544f4a76b1bc3b26d452ca96d7a35e7978",
+ "_shrinkwrap": null,
+ "_spec": "base64-js@0.0.8",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/plist",
+ "author": {
+ "email": "t.jameson.little@gmail.com",
+ "name": "T. Jameson Little"
+ },
+ "bugs": {
+ "url": "https://github.com/beatgammit/base64-js/issues"
+ },
+ "dependencies": {},
+ "description": "Base64 encoding/decoding in pure JS",
+ "devDependencies": {
+ "tape": "~2.3.2"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "1101e9544f4a76b1bc3b26d452ca96d7a35e7978",
+ "tarball": "http://registry.npmjs.org/base64-js/-/base64-js-0.0.8.tgz"
+ },
+ "engines": {
+ "node": ">= 0.4"
+ },
+ "gitHead": "b4a8a5fa9b0caeddb5ad94dd1108253d8f2a315f",
+ "homepage": "https://github.com/beatgammit/base64-js",
+ "license": "MIT",
+ "main": "lib/b64.js",
+ "maintainers": [
+ {
+ "name": "beatgammit",
+ "email": "t.jameson.little@gmail.com"
+ },
+ {
+ "name": "feross",
+ "email": "feross@feross.org"
+ }
+ ],
+ "name": "base64-js",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/beatgammit/base64-js.git"
+ },
+ "scripts": {
+ "test": "tape test/*.js"
+ },
+ "testling": {
+ "browsers": [
+ "chrome/4..latest",
+ "firefox/3..latest",
+ "ie/6..latest",
+ "ipad/6",
+ "iphone/6",
+ "opera/11.0..latest",
+ "safari/5.1..latest"
+ ],
+ "files": "test/*.js"
+ },
+ "version": "0.0.8"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/test/convert.js
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/test/convert.js b/node_modules/base64-js/test/convert.js
new file mode 100644
index 0000000..60b09c0
--- /dev/null
+++ b/node_modules/base64-js/test/convert.js
@@ -0,0 +1,51 @@
+var test = require('tape'),
+ b64 = require('../lib/b64'),
+ checks = [
+ 'a',
+ 'aa',
+ 'aaa',
+ 'hi',
+ 'hi!',
+ 'hi!!',
+ 'sup',
+ 'sup?',
+ 'sup?!'
+ ];
+
+test('convert to base64 and back', function (t) {
+ t.plan(checks.length);
+
+ for (var i = 0; i < checks.length; i++) {
+ var check = checks[i],
+ b64Str,
+ arr,
+ str;
+
+ b64Str = b64.fromByteArray(map(check, function (char) { return char.charCodeAt(0); }));
+
+ arr = b64.toByteArray(b64Str);
+ str = map(arr, function (byte) { return String.fromCharCode(byte); }).join('');
+
+ t.equal(check, str, 'Checked ' + check);
+ }
+
+});
+
+function map (arr, callback) {
+ var res = [],
+ kValue,
+ mappedValue;
+
+ for (var k = 0, len = arr.length; k < len; k++) {
+ if ((typeof arr === 'string' && !!arr.charAt(k))) {
+ kValue = arr.charAt(k);
+ mappedValue = callback(kValue, k, arr);
+ res[k] = mappedValue;
+ } else if (typeof arr !== 'string' && k in arr) {
+ kValue = arr[k];
+ mappedValue = callback(kValue, k, arr);
+ res[k] = mappedValue;
+ }
+ }
+ return res;
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/base64-js/test/url-safe.js
----------------------------------------------------------------------
diff --git a/node_modules/base64-js/test/url-safe.js b/node_modules/base64-js/test/url-safe.js
new file mode 100644
index 0000000..dc437e9
--- /dev/null
+++ b/node_modules/base64-js/test/url-safe.js
@@ -0,0 +1,18 @@
+var test = require('tape'),
+ b64 = require('../lib/b64');
+
+test('decode url-safe style base64 strings', function (t) {
+ var expected = [0xff, 0xff, 0xbe, 0xff, 0xef, 0xbf, 0xfb, 0xef, 0xff];
+
+ var actual = b64.toByteArray('//++/++/++//');
+ for (var i = 0; i < actual.length; i++) {
+ t.equal(actual[i], expected[i])
+ }
+
+ actual = b64.toByteArray('__--_--_--__');
+ for (var i = 0; i < actual.length; i++) {
+ t.equal(actual[i], expected[i])
+ }
+
+ t.end();
+});
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[09/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/example.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/example.js b/node_modules/sax/examples/example.js
new file mode 100644
index 0000000..e7f81e6
--- /dev/null
+++ b/node_modules/sax/examples/example.js
@@ -0,0 +1,41 @@
+
+var fs = require("fs"),
+ sys = require("sys"),
+ path = require("path"),
+ xml = fs.cat(path.join(__dirname, "test.xml")),
+ sax = require("../lib/sax"),
+ strict = sax.parser(true),
+ loose = sax.parser(false, {trim:true}),
+ inspector = function (ev) { return function (data) {
+ // sys.error("");
+ // sys.error(ev+": "+sys.inspect(data));
+ // for (var i in data) sys.error(i+ " "+sys.inspect(data[i]));
+ // sys.error(this.line+":"+this.column);
+ }};
+
+xml.addCallback(function (xml) {
+ // strict.write(xml);
+
+ sax.EVENTS.forEach(function (ev) {
+ loose["on"+ev] = inspector(ev);
+ });
+ loose.onend = function () {
+ // sys.error("end");
+ // sys.error(sys.inspect(loose));
+ };
+
+ // do this one char at a time to verify that it works.
+ // (function () {
+ // if (xml) {
+ // loose.write(xml.substr(0,1000));
+ // xml = xml.substr(1000);
+ // process.nextTick(arguments.callee);
+ // } else loose.close();
+ // })();
+
+ for (var i = 0; i < 1000; i ++) {
+ loose.write(xml);
+ loose.close();
+ }
+
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/get-products.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/get-products.js b/node_modules/sax/examples/get-products.js
new file mode 100644
index 0000000..9e8d74a
--- /dev/null
+++ b/node_modules/sax/examples/get-products.js
@@ -0,0 +1,58 @@
+// pull out /GeneralSearchResponse/categories/category/items/product tags
+// the rest we don't care about.
+
+var sax = require("../lib/sax.js")
+var fs = require("fs")
+var path = require("path")
+var xmlFile = path.resolve(__dirname, "shopping.xml")
+var util = require("util")
+var http = require("http")
+
+fs.readFile(xmlFile, function (er, d) {
+ http.createServer(function (req, res) {
+ if (er) throw er
+ var xmlstr = d.toString("utf8")
+
+ var parser = sax.parser(true)
+ var products = []
+ var product = null
+ var currentTag = null
+
+ parser.onclosetag = function (tagName) {
+ if (tagName === "product") {
+ products.push(product)
+ currentTag = product = null
+ return
+ }
+ if (currentTag && currentTag.parent) {
+ var p = currentTag.parent
+ delete currentTag.parent
+ currentTag = p
+ }
+ }
+
+ parser.onopentag = function (tag) {
+ if (tag.name !== "product" && !product) return
+ if (tag.name === "product") {
+ product = tag
+ }
+ tag.parent = currentTag
+ tag.children = []
+ tag.parent && tag.parent.children.push(tag)
+ currentTag = tag
+ }
+
+ parser.ontext = function (text) {
+ if (currentTag) currentTag.children.push(text)
+ }
+
+ parser.onend = function () {
+ var out = util.inspect(products, false, 3, true)
+ res.writeHead(200, {"content-type":"application/json"})
+ res.end("{\"ok\":true}")
+ // res.end(JSON.stringify(products))
+ }
+
+ parser.write(xmlstr).end()
+ }).listen(1337)
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/hello-world.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/hello-world.js b/node_modules/sax/examples/hello-world.js
new file mode 100644
index 0000000..cbfa518
--- /dev/null
+++ b/node_modules/sax/examples/hello-world.js
@@ -0,0 +1,4 @@
+require("http").createServer(function (req, res) {
+ res.writeHead(200, {"content-type":"application/json"})
+ res.end(JSON.stringify({ok: true}))
+}).listen(1337)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/not-pretty.xml
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/not-pretty.xml b/node_modules/sax/examples/not-pretty.xml
new file mode 100644
index 0000000..9592852
--- /dev/null
+++ b/node_modules/sax/examples/not-pretty.xml
@@ -0,0 +1,8 @@
+<root>
+ something<else> blerm <slurm
+
+
+ attrib =
+ "blorg" ></else><!-- COMMENT!
+
+--><![CDATA[processing...]]> <selfclosing tag="blr>""/> a bit down here</root>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/pretty-print.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/pretty-print.js b/node_modules/sax/examples/pretty-print.js
new file mode 100644
index 0000000..cd6aca9
--- /dev/null
+++ b/node_modules/sax/examples/pretty-print.js
@@ -0,0 +1,74 @@
+var sax = require("../lib/sax")
+ , printer = sax.createStream(false, {lowercasetags:true, trim:true})
+ , fs = require("fs")
+
+function entity (str) {
+ return str.replace('"', '"')
+}
+
+printer.tabstop = 2
+printer.level = 0
+printer.indent = function () {
+ print("\n")
+ for (var i = this.level; i > 0; i --) {
+ for (var j = this.tabstop; j > 0; j --) {
+ print(" ")
+ }
+ }
+}
+printer.on("opentag", function (tag) {
+ this.indent()
+ this.level ++
+ print("<"+tag.name)
+ for (var i in tag.attributes) {
+ print(" "+i+"=\""+entity(tag.attributes[i])+"\"")
+ }
+ print(">")
+})
+
+printer.on("text", ontext)
+printer.on("doctype", ontext)
+function ontext (text) {
+ this.indent()
+ print(text)
+}
+
+printer.on("closetag", function (tag) {
+ this.level --
+ this.indent()
+ print("</"+tag+">")
+})
+
+printer.on("cdata", function (data) {
+ this.indent()
+ print("<![CDATA["+data+"]]>")
+})
+
+printer.on("comment", function (comment) {
+ this.indent()
+ print("<!--"+comment+"-->")
+})
+
+printer.on("error", function (error) {
+ console.error(error)
+ throw error
+})
+
+if (!process.argv[2]) {
+ throw new Error("Please provide an xml file to prettify\n"+
+ "TODO: read from stdin or take a file")
+}
+var xmlfile = require("path").join(process.cwd(), process.argv[2])
+var fstr = fs.createReadStream(xmlfile, { encoding: "utf8" })
+
+function print (c) {
+ if (!process.stdout.write(c)) {
+ fstr.pause()
+ }
+}
+
+process.stdout.on("drain", function () {
+ fstr.resume()
+})
+
+fstr.pipe(printer)
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[14/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/dist/plist-parse.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/dist/plist-parse.js b/node_modules/plist/dist/plist-parse.js
new file mode 100644
index 0000000..d84cf6e
--- /dev/null
+++ b/node_modules/plist/dist/plist-parse.js
@@ -0,0 +1,4055 @@
+(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.plist = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){
+(function (Buffer){
+
+/**
+ * Module dependencies.
+ */
+
+var deprecate = require('util-deprecate');
+var DOMParser = require('xmldom').DOMParser;
+
+/**
+ * Module exports.
+ */
+
+exports.parse = parse;
+exports.parseString = deprecate(parseString, '`parseString()` is deprecated. ' +
+ 'It\'s not actually async. Use `parse()` instead.');
+exports.parseStringSync = deprecate(parseStringSync, '`parseStringSync()` is ' +
+ 'deprecated. Use `parse()` instead.');
+
+/**
+ * We ignore raw text (usually whitespace), <!-- xml comments -->,
+ * and raw CDATA nodes.
+ *
+ * @param {Element} node
+ * @returns {Boolean}
+ * @api private
+ */
+
+function shouldIgnoreNode (node) {
+ return node.nodeType === 3 // text
+ || node.nodeType === 8 // comment
+ || node.nodeType === 4; // cdata
+}
+
+
+/**
+ * Parses a Plist XML string. Returns an Object.
+ *
+ * @param {String} xml - the XML String to decode
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ */
+
+function parse (xml) {
+ var doc = new DOMParser().parseFromString(xml);
+ if (doc.documentElement.nodeName !== 'plist') {
+ throw new Error('malformed document. First element should be <plist>');
+ }
+ var plist = parsePlistXML(doc.documentElement);
+
+ // the root <plist> node gets interpreted as an Array,
+ // so pull out the inner data first
+ if (plist.length == 1) plist = plist[0];
+
+ return plist;
+}
+
+/**
+ * Parses a Plist XML string. Returns an Object. Takes a `callback` function.
+ *
+ * @param {String} xml - the XML String to decode
+ * @param {Function} callback - callback function
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ * @deprecated not actually async. use parse() instead
+ */
+
+function parseString (xml, callback) {
+ var doc, error, plist;
+ try {
+ doc = new DOMParser().parseFromString(xml);
+ plist = parsePlistXML(doc.documentElement);
+ } catch(e) {
+ error = e;
+ }
+ callback(error, plist);
+}
+
+/**
+ * Parses a Plist XML string. Returns an Object.
+ *
+ * @param {String} xml - the XML String to decode
+ * @param {Function} callback - callback function
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ * @deprecated use parse() instead
+ */
+
+function parseStringSync (xml) {
+ var doc = new DOMParser().parseFromString(xml);
+ var plist;
+ if (doc.documentElement.nodeName !== 'plist') {
+ throw new Error('malformed document. First element should be <plist>');
+ }
+ plist = parsePlistXML(doc.documentElement);
+
+ // if the plist is an array with 1 element, pull it out of the array
+ if (plist.length == 1) {
+ plist = plist[0];
+ }
+ return plist;
+}
+
+/**
+ * Convert an XML based plist document into a JSON representation.
+ *
+ * @param {Object} xml_node - current XML node in the plist
+ * @returns {Mixed} built up JSON object
+ * @api private
+ */
+
+function parsePlistXML (node) {
+ var i, new_obj, key, val, new_arr, res, d;
+
+ if (!node)
+ return null;
+
+ if (node.nodeName === 'plist') {
+ new_arr = [];
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ new_arr.push( parsePlistXML(node.childNodes[i]));
+ }
+ }
+ return new_arr;
+
+ } else if (node.nodeName === 'dict') {
+ new_obj = {};
+ key = null;
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ if (key === null) {
+ key = parsePlistXML(node.childNodes[i]);
+ } else {
+ new_obj[key] = parsePlistXML(node.childNodes[i]);
+ key = null;
+ }
+ }
+ }
+ return new_obj;
+
+ } else if (node.nodeName === 'array') {
+ new_arr = [];
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ res = parsePlistXML(node.childNodes[i]);
+ if (null != res) new_arr.push(res);
+ }
+ }
+ return new_arr;
+
+ } else if (node.nodeName === '#text') {
+ // TODO: what should we do with text types? (CDATA sections)
+
+ } else if (node.nodeName === 'key') {
+ return node.childNodes[0].nodeValue;
+
+ } else if (node.nodeName === 'string') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ res += node.childNodes[d].nodeValue;
+ }
+ return res;
+
+ } else if (node.nodeName === 'integer') {
+ // parse as base 10 integer
+ return parseInt(node.childNodes[0].nodeValue, 10);
+
+ } else if (node.nodeName === 'real') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ if (node.childNodes[d].nodeType === 3) {
+ res += node.childNodes[d].nodeValue;
+ }
+ }
+ return parseFloat(res);
+
+ } else if (node.nodeName === 'data') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ if (node.childNodes[d].nodeType === 3) {
+ res += node.childNodes[d].nodeValue.replace(/\s+/g, '');
+ }
+ }
+
+ // decode base64 data to a Buffer instance
+ return new Buffer(res, 'base64');
+
+ } else if (node.nodeName === 'date') {
+ return new Date(node.childNodes[0].nodeValue);
+
+ } else if (node.nodeName === 'true') {
+ return true;
+
+ } else if (node.nodeName === 'false') {
+ return false;
+ }
+}
+
+}).call(this,require("buffer").Buffer)
+},{"buffer":3,"util-deprecate":6,"xmldom":7}],2:[function(require,module,exports){
+var lookup = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+;(function (exports) {
+ 'use strict';
+
+ var Arr = (typeof Uint8Array !== 'undefined')
+ ? Uint8Array
+ : Array
+
+ var PLUS = '+'.charCodeAt(0)
+ var SLASH = '/'.charCodeAt(0)
+ var NUMBER = '0'.charCodeAt(0)
+ var LOWER = 'a'.charCodeAt(0)
+ var UPPER = 'A'.charCodeAt(0)
+ var PLUS_URL_SAFE = '-'.charCodeAt(0)
+ var SLASH_URL_SAFE = '_'.charCodeAt(0)
+
+ function decode (elt) {
+ var code = elt.charCodeAt(0)
+ if (code === PLUS ||
+ code === PLUS_URL_SAFE)
+ return 62 // '+'
+ if (code === SLASH ||
+ code === SLASH_URL_SAFE)
+ return 63 // '/'
+ if (code < NUMBER)
+ return -1 //no match
+ if (code < NUMBER + 10)
+ return code - NUMBER + 26 + 26
+ if (code < UPPER + 26)
+ return code - UPPER
+ if (code < LOWER + 26)
+ return code - LOWER + 26
+ }
+
+ function b64ToByteArray (b64) {
+ var i, j, l, tmp, placeHolders, arr
+
+ if (b64.length % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4')
+ }
+
+ // the number of equal signs (place holders)
+ // if there are two placeholders, than the two characters before it
+ // represent one byte
+ // if there is only one, then the three characters before it represent 2 bytes
+ // this is just a cheap hack to not do indexOf twice
+ var len = b64.length
+ placeHolders = '=' === b64.charAt(len - 2) ? 2 : '=' === b64.charAt(len - 1) ? 1 : 0
+
+ // base64 is 4/3 + up to two characters of the original data
+ arr = new Arr(b64.length * 3 / 4 - placeHolders)
+
+ // if there are placeholders, only get up to the last complete 4 chars
+ l = placeHolders > 0 ? b64.length - 4 : b64.length
+
+ var L = 0
+
+ function push (v) {
+ arr[L++] = v
+ }
+
+ for (i = 0, j = 0; i < l; i += 4, j += 3) {
+ tmp = (decode(b64.charAt(i)) << 18) | (decode(b64.charAt(i + 1)) << 12) | (decode(b64.charAt(i + 2)) << 6) | decode(b64.charAt(i + 3))
+ push((tmp & 0xFF0000) >> 16)
+ push((tmp & 0xFF00) >> 8)
+ push(tmp & 0xFF)
+ }
+
+ if (placeHolders === 2) {
+ tmp = (decode(b64.charAt(i)) << 2) | (decode(b64.charAt(i + 1)) >> 4)
+ push(tmp & 0xFF)
+ } else if (placeHolders === 1) {
+ tmp = (decode(b64.charAt(i)) << 10) | (decode(b64.charAt(i + 1)) << 4) | (decode(b64.charAt(i + 2)) >> 2)
+ push((tmp >> 8) & 0xFF)
+ push(tmp & 0xFF)
+ }
+
+ return arr
+ }
+
+ function uint8ToBase64 (uint8) {
+ var i,
+ extraBytes = uint8.length % 3, // if we have 1 byte left, pad 2 bytes
+ output = "",
+ temp, length
+
+ function encode (num) {
+ return lookup.charAt(num)
+ }
+
+ function tripletToBase64 (num) {
+ return encode(num >> 18 & 0x3F) + encode(num >> 12 & 0x3F) + encode(num >> 6 & 0x3F) + encode(num & 0x3F)
+ }
+
+ // go through the array every three bytes, we'll deal with trailing stuff later
+ for (i = 0, length = uint8.length - extraBytes; i < length; i += 3) {
+ temp = (uint8[i] << 16) + (uint8[i + 1] << 8) + (uint8[i + 2])
+ output += tripletToBase64(temp)
+ }
+
+ // pad the end with zeros, but make sure to not forget the extra bytes
+ switch (extraBytes) {
+ case 1:
+ temp = uint8[uint8.length - 1]
+ output += encode(temp >> 2)
+ output += encode((temp << 4) & 0x3F)
+ output += '=='
+ break
+ case 2:
+ temp = (uint8[uint8.length - 2] << 8) + (uint8[uint8.length - 1])
+ output += encode(temp >> 10)
+ output += encode((temp >> 4) & 0x3F)
+ output += encode((temp << 2) & 0x3F)
+ output += '='
+ break
+ }
+
+ return output
+ }
+
+ exports.toByteArray = b64ToByteArray
+ exports.fromByteArray = uint8ToBase64
+}(typeof exports === 'undefined' ? (this.base64js = {}) : exports))
+
+},{}],3:[function(require,module,exports){
+(function (global){
+/*!
+ * The buffer module from node.js, for the browser.
+ *
+ * @author Feross Aboukhadijeh <fe...@feross.org> <http://feross.org>
+ * @license MIT
+ */
+/* eslint-disable no-proto */
+
+var base64 = require('base64-js')
+var ieee754 = require('ieee754')
+var isArray = require('is-array')
+
+exports.Buffer = Buffer
+exports.SlowBuffer = SlowBuffer
+exports.INSPECT_MAX_BYTES = 50
+Buffer.poolSize = 8192 // not used by this implementation
+
+var rootParent = {}
+
+/**
+ * If `Buffer.TYPED_ARRAY_SUPPORT`:
+ * === true Use Uint8Array implementation (fastest)
+ * === false Use Object implementation (most compatible, even IE6)
+ *
+ * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
+ * Opera 11.6+, iOS 4.2+.
+ *
+ * Due to various browser bugs, sometimes the Object implementation will be used even
+ * when the browser supports typed arrays.
+ *
+ * Note:
+ *
+ * - Firefox 4-29 lacks support for adding new properties to `Uint8Array` instances,
+ * See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438.
+ *
+ * - Safari 5-7 lacks support for changing the `Object.prototype.constructor` property
+ * on objects.
+ *
+ * - Chrome 9-10 is missing the `TypedArray.prototype.subarray` function.
+ *
+ * - IE10 has a broken `TypedArray.prototype.subarray` function which returns arrays of
+ * incorrect length in some situations.
+
+ * We detect these buggy browsers and set `Buffer.TYPED_ARRAY_SUPPORT` to `false` so they
+ * get the Object implementation, which is slower but behaves correctly.
+ */
+Buffer.TYPED_ARRAY_SUPPORT = global.TYPED_ARRAY_SUPPORT !== undefined
+ ? global.TYPED_ARRAY_SUPPORT
+ : typedArraySupport()
+
+function typedArraySupport () {
+ function Bar () {}
+ try {
+ var arr = new Uint8Array(1)
+ arr.foo = function () { return 42 }
+ arr.constructor = Bar
+ return arr.foo() === 42 && // typed array instances can be augmented
+ arr.constructor === Bar && // constructor can be set
+ typeof arr.subarray === 'function' && // chrome 9-10 lack `subarray`
+ arr.subarray(1, 1).byteLength === 0 // ie10 has broken `subarray`
+ } catch (e) {
+ return false
+ }
+}
+
+function kMaxLength () {
+ return Buffer.TYPED_ARRAY_SUPPORT
+ ? 0x7fffffff
+ : 0x3fffffff
+}
+
+/**
+ * Class: Buffer
+ * =============
+ *
+ * The Buffer constructor returns instances of `Uint8Array` that are augmented
+ * with function properties for all the node `Buffer` API functions. We use
+ * `Uint8Array` so that square bracket notation works as expected -- it returns
+ * a single octet.
+ *
+ * By augmenting the instances, we can avoid modifying the `Uint8Array`
+ * prototype.
+ */
+function Buffer (arg) {
+ if (!(this instanceof Buffer)) {
+ // Avoid going through an ArgumentsAdaptorTrampoline in the common case.
+ if (arguments.length > 1) return new Buffer(arg, arguments[1])
+ return new Buffer(arg)
+ }
+
+ this.length = 0
+ this.parent = undefined
+
+ // Common case.
+ if (typeof arg === 'number') {
+ return fromNumber(this, arg)
+ }
+
+ // Slightly less common case.
+ if (typeof arg === 'string') {
+ return fromString(this, arg, arguments.length > 1 ? arguments[1] : 'utf8')
+ }
+
+ // Unusual.
+ return fromObject(this, arg)
+}
+
+function fromNumber (that, length) {
+ that = allocate(that, length < 0 ? 0 : checked(length) | 0)
+ if (!Buffer.TYPED_ARRAY_SUPPORT) {
+ for (var i = 0; i < length; i++) {
+ that[i] = 0
+ }
+ }
+ return that
+}
+
+function fromString (that, string, encoding) {
+ if (typeof encoding !== 'string' || encoding === '') encoding = 'utf8'
+
+ // Assumption: byteLength() return value is always < kMaxLength.
+ var length = byteLength(string, encoding) | 0
+ that = allocate(that, length)
+
+ that.write(string, encoding)
+ return that
+}
+
+function fromObject (that, object) {
+ if (Buffer.isBuffer(object)) return fromBuffer(that, object)
+
+ if (isArray(object)) return fromArray(that, object)
+
+ if (object == null) {
+ throw new TypeError('must start with number, buffer, array or string')
+ }
+
+ if (typeof ArrayBuffer !== 'undefined') {
+ if (object.buffer instanceof ArrayBuffer) {
+ return fromTypedArray(that, object)
+ }
+ if (object instanceof ArrayBuffer) {
+ return fromArrayBuffer(that, object)
+ }
+ }
+
+ if (object.length) return fromArrayLike(that, object)
+
+ return fromJsonObject(that, object)
+}
+
+function fromBuffer (that, buffer) {
+ var length = checked(buffer.length) | 0
+ that = allocate(that, length)
+ buffer.copy(that, 0, 0, length)
+ return that
+}
+
+function fromArray (that, array) {
+ var length = checked(array.length) | 0
+ that = allocate(that, length)
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+// Duplicate of fromArray() to keep fromArray() monomorphic.
+function fromTypedArray (that, array) {
+ var length = checked(array.length) | 0
+ that = allocate(that, length)
+ // Truncating the elements is probably not what people expect from typed
+ // arrays with BYTES_PER_ELEMENT > 1 but it's compatible with the behavior
+ // of the old Buffer constructor.
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+function fromArrayBuffer (that, array) {
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ // Return an augmented `Uint8Array` instance, for best performance
+ array.byteLength
+ that = Buffer._augment(new Uint8Array(array))
+ } else {
+ // Fallback: Return an object instance of the Buffer class
+ that = fromTypedArray(that, new Uint8Array(array))
+ }
+ return that
+}
+
+function fromArrayLike (that, array) {
+ var length = checked(array.length) | 0
+ that = allocate(that, length)
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+// Deserialize { type: 'Buffer', data: [1,2,3,...] } into a Buffer object.
+// Returns a zero-length buffer for inputs that don't conform to the spec.
+function fromJsonObject (that, object) {
+ var array
+ var length = 0
+
+ if (object.type === 'Buffer' && isArray(object.data)) {
+ array = object.data
+ length = checked(array.length) | 0
+ }
+ that = allocate(that, length)
+
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+if (Buffer.TYPED_ARRAY_SUPPORT) {
+ Buffer.prototype.__proto__ = Uint8Array.prototype
+ Buffer.__proto__ = Uint8Array
+}
+
+function allocate (that, length) {
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ // Return an augmented `Uint8Array` instance, for best performance
+ that = Buffer._augment(new Uint8Array(length))
+ that.__proto__ = Buffer.prototype
+ } else {
+ // Fallback: Return an object instance of the Buffer class
+ that.length = length
+ that._isBuffer = true
+ }
+
+ var fromPool = length !== 0 && length <= Buffer.poolSize >>> 1
+ if (fromPool) that.parent = rootParent
+
+ return that
+}
+
+function checked (length) {
+ // Note: cannot use `length < kMaxLength` here because that fails when
+ // length is NaN (which is otherwise coerced to zero.)
+ if (length >= kMaxLength()) {
+ throw new RangeError('Attempt to allocate Buffer larger than maximum ' +
+ 'size: 0x' + kMaxLength().toString(16) + ' bytes')
+ }
+ return length | 0
+}
+
+function SlowBuffer (subject, encoding) {
+ if (!(this instanceof SlowBuffer)) return new SlowBuffer(subject, encoding)
+
+ var buf = new Buffer(subject, encoding)
+ delete buf.parent
+ return buf
+}
+
+Buffer.isBuffer = function isBuffer (b) {
+ return !!(b != null && b._isBuffer)
+}
+
+Buffer.compare = function compare (a, b) {
+ if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
+ throw new TypeError('Arguments must be Buffers')
+ }
+
+ if (a === b) return 0
+
+ var x = a.length
+ var y = b.length
+
+ var i = 0
+ var len = Math.min(x, y)
+ while (i < len) {
+ if (a[i] !== b[i]) break
+
+ ++i
+ }
+
+ if (i !== len) {
+ x = a[i]
+ y = b[i]
+ }
+
+ if (x < y) return -1
+ if (y < x) return 1
+ return 0
+}
+
+Buffer.isEncoding = function isEncoding (encoding) {
+ switch (String(encoding).toLowerCase()) {
+ case 'hex':
+ case 'utf8':
+ case 'utf-8':
+ case 'ascii':
+ case 'binary':
+ case 'base64':
+ case 'raw':
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return true
+ default:
+ return false
+ }
+}
+
+Buffer.concat = function concat (list, length) {
+ if (!isArray(list)) throw new TypeError('list argument must be an Array of Buffers.')
+
+ if (list.length === 0) {
+ return new Buffer(0)
+ }
+
+ var i
+ if (length === undefined) {
+ length = 0
+ for (i = 0; i < list.length; i++) {
+ length += list[i].length
+ }
+ }
+
+ var buf = new Buffer(length)
+ var pos = 0
+ for (i = 0; i < list.length; i++) {
+ var item = list[i]
+ item.copy(buf, pos)
+ pos += item.length
+ }
+ return buf
+}
+
+function byteLength (string, encoding) {
+ if (typeof string !== 'string') string = '' + string
+
+ var len = string.length
+ if (len === 0) return 0
+
+ // Use a for loop to avoid recursion
+ var loweredCase = false
+ for (;;) {
+ switch (encoding) {
+ case 'ascii':
+ case 'binary':
+ // Deprecated
+ case 'raw':
+ case 'raws':
+ return len
+ case 'utf8':
+ case 'utf-8':
+ return utf8ToBytes(string).length
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return len * 2
+ case 'hex':
+ return len >>> 1
+ case 'base64':
+ return base64ToBytes(string).length
+ default:
+ if (loweredCase) return utf8ToBytes(string).length // assume utf8
+ encoding = ('' + encoding).toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+Buffer.byteLength = byteLength
+
+// pre-set for values that may exist in the future
+Buffer.prototype.length = undefined
+Buffer.prototype.parent = undefined
+
+function slowToString (encoding, start, end) {
+ var loweredCase = false
+
+ start = start | 0
+ end = end === undefined || end === Infinity ? this.length : end | 0
+
+ if (!encoding) encoding = 'utf8'
+ if (start < 0) start = 0
+ if (end > this.length) end = this.length
+ if (end <= start) return ''
+
+ while (true) {
+ switch (encoding) {
+ case 'hex':
+ return hexSlice(this, start, end)
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Slice(this, start, end)
+
+ case 'ascii':
+ return asciiSlice(this, start, end)
+
+ case 'binary':
+ return binarySlice(this, start, end)
+
+ case 'base64':
+ return base64Slice(this, start, end)
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return utf16leSlice(this, start, end)
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
+ encoding = (encoding + '').toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+
+Buffer.prototype.toString = function toString () {
+ var length = this.length | 0
+ if (length === 0) return ''
+ if (arguments.length === 0) return utf8Slice(this, 0, length)
+ return slowToString.apply(this, arguments)
+}
+
+Buffer.prototype.equals = function equals (b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
+ if (this === b) return true
+ return Buffer.compare(this, b) === 0
+}
+
+Buffer.prototype.inspect = function inspect () {
+ var str = ''
+ var max = exports.INSPECT_MAX_BYTES
+ if (this.length > 0) {
+ str = this.toString('hex', 0, max).match(/.{2}/g).join(' ')
+ if (this.length > max) str += ' ... '
+ }
+ return '<Buffer ' + str + '>'
+}
+
+Buffer.prototype.compare = function compare (b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
+ if (this === b) return 0
+ return Buffer.compare(this, b)
+}
+
+Buffer.prototype.indexOf = function indexOf (val, byteOffset) {
+ if (byteOffset > 0x7fffffff) byteOffset = 0x7fffffff
+ else if (byteOffset < -0x80000000) byteOffset = -0x80000000
+ byteOffset >>= 0
+
+ if (this.length === 0) return -1
+ if (byteOffset >= this.length) return -1
+
+ // Negative offsets start from the end of the buffer
+ if (byteOffset < 0) byteOffset = Math.max(this.length + byteOffset, 0)
+
+ if (typeof val === 'string') {
+ if (val.length === 0) return -1 // special case: looking for empty string always fails
+ return String.prototype.indexOf.call(this, val, byteOffset)
+ }
+ if (Buffer.isBuffer(val)) {
+ return arrayIndexOf(this, val, byteOffset)
+ }
+ if (typeof val === 'number') {
+ if (Buffer.TYPED_ARRAY_SUPPORT && Uint8Array.prototype.indexOf === 'function') {
+ return Uint8Array.prototype.indexOf.call(this, val, byteOffset)
+ }
+ return arrayIndexOf(this, [ val ], byteOffset)
+ }
+
+ function arrayIndexOf (arr, val, byteOffset) {
+ var foundIndex = -1
+ for (var i = 0; byteOffset + i < arr.length; i++) {
+ if (arr[byteOffset + i] === val[foundIndex === -1 ? 0 : i - foundIndex]) {
+ if (foundIndex === -1) foundIndex = i
+ if (i - foundIndex + 1 === val.length) return byteOffset + foundIndex
+ } else {
+ foundIndex = -1
+ }
+ }
+ return -1
+ }
+
+ throw new TypeError('val must be string, number or Buffer')
+}
+
+// `get` is deprecated
+Buffer.prototype.get = function get (offset) {
+ console.log('.get() is deprecated. Access using array indexes instead.')
+ return this.readUInt8(offset)
+}
+
+// `set` is deprecated
+Buffer.prototype.set = function set (v, offset) {
+ console.log('.set() is deprecated. Access using array indexes instead.')
+ return this.writeUInt8(v, offset)
+}
+
+function hexWrite (buf, string, offset, length) {
+ offset = Number(offset) || 0
+ var remaining = buf.length - offset
+ if (!length) {
+ length = remaining
+ } else {
+ length = Number(length)
+ if (length > remaining) {
+ length = remaining
+ }
+ }
+
+ // must be an even number of digits
+ var strLen = string.length
+ if (strLen % 2 !== 0) throw new Error('Invalid hex string')
+
+ if (length > strLen / 2) {
+ length = strLen / 2
+ }
+ for (var i = 0; i < length; i++) {
+ var parsed = parseInt(string.substr(i * 2, 2), 16)
+ if (isNaN(parsed)) throw new Error('Invalid hex string')
+ buf[offset + i] = parsed
+ }
+ return i
+}
+
+function utf8Write (buf, string, offset, length) {
+ return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)
+}
+
+function asciiWrite (buf, string, offset, length) {
+ return blitBuffer(asciiToBytes(string), buf, offset, length)
+}
+
+function binaryWrite (buf, string, offset, length) {
+ return asciiWrite(buf, string, offset, length)
+}
+
+function base64Write (buf, string, offset, length) {
+ return blitBuffer(base64ToBytes(string), buf, offset, length)
+}
+
+function ucs2Write (buf, string, offset, length) {
+ return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)
+}
+
+Buffer.prototype.write = function write (string, offset, length, encoding) {
+ // Buffer#write(string)
+ if (offset === undefined) {
+ encoding = 'utf8'
+ length = this.length
+ offset = 0
+ // Buffer#write(string, encoding)
+ } else if (length === undefined && typeof offset === 'string') {
+ encoding = offset
+ length = this.length
+ offset = 0
+ // Buffer#write(string, offset[, length][, encoding])
+ } else if (isFinite(offset)) {
+ offset = offset | 0
+ if (isFinite(length)) {
+ length = length | 0
+ if (encoding === undefined) encoding = 'utf8'
+ } else {
+ encoding = length
+ length = undefined
+ }
+ // legacy write(string, encoding, offset, length) - remove in v0.13
+ } else {
+ var swap = encoding
+ encoding = offset
+ offset = length | 0
+ length = swap
+ }
+
+ var remaining = this.length - offset
+ if (length === undefined || length > remaining) length = remaining
+
+ if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {
+ throw new RangeError('attempt to write outside buffer bounds')
+ }
+
+ if (!encoding) encoding = 'utf8'
+
+ var loweredCase = false
+ for (;;) {
+ switch (encoding) {
+ case 'hex':
+ return hexWrite(this, string, offset, length)
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Write(this, string, offset, length)
+
+ case 'ascii':
+ return asciiWrite(this, string, offset, length)
+
+ case 'binary':
+ return binaryWrite(this, string, offset, length)
+
+ case 'base64':
+ // Warning: maxLength not taken into account in base64Write
+ return base64Write(this, string, offset, length)
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return ucs2Write(this, string, offset, length)
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
+ encoding = ('' + encoding).toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+
+Buffer.prototype.toJSON = function toJSON () {
+ return {
+ type: 'Buffer',
+ data: Array.prototype.slice.call(this._arr || this, 0)
+ }
+}
+
+function base64Slice (buf, start, end) {
+ if (start === 0 && end === buf.length) {
+ return base64.fromByteArray(buf)
+ } else {
+ return base64.fromByteArray(buf.slice(start, end))
+ }
+}
+
+function utf8Slice (buf, start, end) {
+ end = Math.min(buf.length, end)
+ var res = []
+
+ var i = start
+ while (i < end) {
+ var firstByte = buf[i]
+ var codePoint = null
+ var bytesPerSequence = (firstByte > 0xEF) ? 4
+ : (firstByte > 0xDF) ? 3
+ : (firstByte > 0xBF) ? 2
+ : 1
+
+ if (i + bytesPerSequence <= end) {
+ var secondByte, thirdByte, fourthByte, tempCodePoint
+
+ switch (bytesPerSequence) {
+ case 1:
+ if (firstByte < 0x80) {
+ codePoint = firstByte
+ }
+ break
+ case 2:
+ secondByte = buf[i + 1]
+ if ((secondByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)
+ if (tempCodePoint > 0x7F) {
+ codePoint = tempCodePoint
+ }
+ }
+ break
+ case 3:
+ secondByte = buf[i + 1]
+ thirdByte = buf[i + 2]
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)
+ if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
+ codePoint = tempCodePoint
+ }
+ }
+ break
+ case 4:
+ secondByte = buf[i + 1]
+ thirdByte = buf[i + 2]
+ fourthByte = buf[i + 3]
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)
+ if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
+ codePoint = tempCodePoint
+ }
+ }
+ }
+ }
+
+ if (codePoint === null) {
+ // we did not generate a valid codePoint so insert a
+ // replacement char (U+FFFD) and advance only 1 byte
+ codePoint = 0xFFFD
+ bytesPerSequence = 1
+ } else if (codePoint > 0xFFFF) {
+ // encode to utf16 (surrogate pair dance)
+ codePoint -= 0x10000
+ res.push(codePoint >>> 10 & 0x3FF | 0xD800)
+ codePoint = 0xDC00 | codePoint & 0x3FF
+ }
+
+ res.push(codePoint)
+ i += bytesPerSequence
+ }
+
+ return decodeCodePointsArray(res)
+}
+
+// Based on http://stackoverflow.com/a/22747272/680742, the browser with
+// the lowest limit is Chrome, with 0x10000 args.
+// We go 1 magnitude less, for safety
+var MAX_ARGUMENTS_LENGTH = 0x1000
+
+function decodeCodePointsArray (codePoints) {
+ var len = codePoints.length
+ if (len <= MAX_ARGUMENTS_LENGTH) {
+ return String.fromCharCode.apply(String, codePoints) // avoid extra slice()
+ }
+
+ // Decode in chunks to avoid "call stack size exceeded".
+ var res = ''
+ var i = 0
+ while (i < len) {
+ res += String.fromCharCode.apply(
+ String,
+ codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)
+ )
+ }
+ return res
+}
+
+function asciiSlice (buf, start, end) {
+ var ret = ''
+ end = Math.min(buf.length, end)
+
+ for (var i = start; i < end; i++) {
+ ret += String.fromCharCode(buf[i] & 0x7F)
+ }
+ return ret
+}
+
+function binarySlice (buf, start, end) {
+ var ret = ''
+ end = Math.min(buf.length, end)
+
+ for (var i = start; i < end; i++) {
+ ret += String.fromCharCode(buf[i])
+ }
+ return ret
+}
+
+function hexSlice (buf, start, end) {
+ var len = buf.length
+
+ if (!start || start < 0) start = 0
+ if (!end || end < 0 || end > len) end = len
+
+ var out = ''
+ for (var i = start; i < end; i++) {
+ out += toHex(buf[i])
+ }
+ return out
+}
+
+function utf16leSlice (buf, start, end) {
+ var bytes = buf.slice(start, end)
+ var res = ''
+ for (var i = 0; i < bytes.length; i += 2) {
+ res += String.fromCharCode(bytes[i] + bytes[i + 1] * 256)
+ }
+ return res
+}
+
+Buffer.prototype.slice = function slice (start, end) {
+ var len = this.length
+ start = ~~start
+ end = end === undefined ? len : ~~end
+
+ if (start < 0) {
+ start += len
+ if (start < 0) start = 0
+ } else if (start > len) {
+ start = len
+ }
+
+ if (end < 0) {
+ end += len
+ if (end < 0) end = 0
+ } else if (end > len) {
+ end = len
+ }
+
+ if (end < start) end = start
+
+ var newBuf
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ newBuf = Buffer._augment(this.subarray(start, end))
+ } else {
+ var sliceLen = end - start
+ newBuf = new Buffer(sliceLen, undefined)
+ for (var i = 0; i < sliceLen; i++) {
+ newBuf[i] = this[i + start]
+ }
+ }
+
+ if (newBuf.length) newBuf.parent = this.parent || this
+
+ return newBuf
+}
+
+/*
+ * Need to make sure that buffer isn't trying to write out of bounds.
+ */
+function checkOffset (offset, ext, length) {
+ if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')
+ if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')
+}
+
+Buffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var val = this[offset]
+ var mul = 1
+ var i = 0
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul
+ }
+
+ return val
+}
+
+Buffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) {
+ checkOffset(offset, byteLength, this.length)
+ }
+
+ var val = this[offset + --byteLength]
+ var mul = 1
+ while (byteLength > 0 && (mul *= 0x100)) {
+ val += this[offset + --byteLength] * mul
+ }
+
+ return val
+}
+
+Buffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 1, this.length)
+ return this[offset]
+}
+
+Buffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ return this[offset] | (this[offset + 1] << 8)
+}
+
+Buffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ return (this[offset] << 8) | this[offset + 1]
+}
+
+Buffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return ((this[offset]) |
+ (this[offset + 1] << 8) |
+ (this[offset + 2] << 16)) +
+ (this[offset + 3] * 0x1000000)
+}
+
+Buffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset] * 0x1000000) +
+ ((this[offset + 1] << 16) |
+ (this[offset + 2] << 8) |
+ this[offset + 3])
+}
+
+Buffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var val = this[offset]
+ var mul = 1
+ var i = 0
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul
+ }
+ mul *= 0x80
+
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength)
+
+ return val
+}
+
+Buffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var i = byteLength
+ var mul = 1
+ var val = this[offset + --i]
+ while (i > 0 && (mul *= 0x100)) {
+ val += this[offset + --i] * mul
+ }
+ mul *= 0x80
+
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength)
+
+ return val
+}
+
+Buffer.prototype.readInt8 = function readInt8 (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 1, this.length)
+ if (!(this[offset] & 0x80)) return (this[offset])
+ return ((0xff - this[offset] + 1) * -1)
+}
+
+Buffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ var val = this[offset] | (this[offset + 1] << 8)
+ return (val & 0x8000) ? val | 0xFFFF0000 : val
+}
+
+Buffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ var val = this[offset + 1] | (this[offset] << 8)
+ return (val & 0x8000) ? val | 0xFFFF0000 : val
+}
+
+Buffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset]) |
+ (this[offset + 1] << 8) |
+ (this[offset + 2] << 16) |
+ (this[offset + 3] << 24)
+}
+
+Buffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset] << 24) |
+ (this[offset + 1] << 16) |
+ (this[offset + 2] << 8) |
+ (this[offset + 3])
+}
+
+Buffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+ return ieee754.read(this, offset, true, 23, 4)
+}
+
+Buffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+ return ieee754.read(this, offset, false, 23, 4)
+}
+
+Buffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 8, this.length)
+ return ieee754.read(this, offset, true, 52, 8)
+}
+
+Buffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 8, this.length)
+ return ieee754.read(this, offset, false, 52, 8)
+}
+
+function checkInt (buf, value, offset, ext, max, min) {
+ if (!Buffer.isBuffer(buf)) throw new TypeError('buffer must be a Buffer instance')
+ if (value > max || value < min) throw new RangeError('value is out of bounds')
+ if (offset + ext > buf.length) throw new RangeError('index out of range')
+}
+
+Buffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0)
+
+ var mul = 1
+ var i = 0
+ this[offset] = value & 0xFF
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = (value / mul) & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0)
+
+ var i = byteLength - 1
+ var mul = 1
+ this[offset + i] = value & 0xFF
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = (value / mul) & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)
+ if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value)
+ this[offset] = (value & 0xff)
+ return offset + 1
+}
+
+function objectWriteUInt16 (buf, value, offset, littleEndian) {
+ if (value < 0) value = 0xffff + value + 1
+ for (var i = 0, j = Math.min(buf.length - offset, 2); i < j; i++) {
+ buf[offset + i] = (value & (0xff << (8 * (littleEndian ? i : 1 - i)))) >>>
+ (littleEndian ? i : 1 - i) * 8
+ }
+}
+
+Buffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ } else {
+ objectWriteUInt16(this, value, offset, true)
+ }
+ return offset + 2
+}
+
+Buffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 8)
+ this[offset + 1] = (value & 0xff)
+ } else {
+ objectWriteUInt16(this, value, offset, false)
+ }
+ return offset + 2
+}
+
+function objectWriteUInt32 (buf, value, offset, littleEndian) {
+ if (value < 0) value = 0xffffffff + value + 1
+ for (var i = 0, j = Math.min(buf.length - offset, 4); i < j; i++) {
+ buf[offset + i] = (value >>> (littleEndian ? i : 3 - i) * 8) & 0xff
+ }
+}
+
+Buffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset + 3] = (value >>> 24)
+ this[offset + 2] = (value >>> 16)
+ this[offset + 1] = (value >>> 8)
+ this[offset] = (value & 0xff)
+ } else {
+ objectWriteUInt32(this, value, offset, true)
+ }
+ return offset + 4
+}
+
+Buffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 24)
+ this[offset + 1] = (value >>> 16)
+ this[offset + 2] = (value >>> 8)
+ this[offset + 3] = (value & 0xff)
+ } else {
+ objectWriteUInt32(this, value, offset, false)
+ }
+ return offset + 4
+}
+
+Buffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1)
+
+ checkInt(this, value, offset, byteLength, limit - 1, -limit)
+ }
+
+ var i = 0
+ var mul = 1
+ var sub = value < 0 ? 1 : 0
+ this[offset] = value & 0xFF
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1)
+
+ checkInt(this, value, offset, byteLength, limit - 1, -limit)
+ }
+
+ var i = byteLength - 1
+ var mul = 1
+ var sub = value < 0 ? 1 : 0
+ this[offset + i] = value & 0xFF
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)
+ if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value)
+ if (value < 0) value = 0xff + value + 1
+ this[offset] = (value & 0xff)
+ return offset + 1
+}
+
+Buffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ } else {
+ objectWriteUInt16(this, value, offset, true)
+ }
+ return offset + 2
+}
+
+Buffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 8)
+ this[offset + 1] = (value & 0xff)
+ } else {
+ objectWriteUInt16(this, value, offset, false)
+ }
+ return offset + 2
+}
+
+Buffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ this[offset + 2] = (value >>> 16)
+ this[offset + 3] = (value >>> 24)
+ } else {
+ objectWriteUInt32(this, value, offset, true)
+ }
+ return offset + 4
+}
+
+Buffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
+ if (value < 0) value = 0xffffffff + value + 1
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 24)
+ this[offset + 1] = (value >>> 16)
+ this[offset + 2] = (value >>> 8)
+ this[offset + 3] = (value & 0xff)
+ } else {
+ objectWriteUInt32(this, value, offset, false)
+ }
+ return offset + 4
+}
+
+function checkIEEE754 (buf, value, offset, ext, max, min) {
+ if (value > max || value < min) throw new RangeError('value is out of bounds')
+ if (offset + ext > buf.length) throw new RangeError('index out of range')
+ if (offset < 0) throw new RangeError('index out of range')
+}
+
+function writeFloat (buf, value, offset, littleEndian, noAssert) {
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)
+ }
+ ieee754.write(buf, value, offset, littleEndian, 23, 4)
+ return offset + 4
+}
+
+Buffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {
+ return writeFloat(this, value, offset, true, noAssert)
+}
+
+Buffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {
+ return writeFloat(this, value, offset, false, noAssert)
+}
+
+function writeDouble (buf, value, offset, littleEndian, noAssert) {
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)
+ }
+ ieee754.write(buf, value, offset, littleEndian, 52, 8)
+ return offset + 8
+}
+
+Buffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {
+ return writeDouble(this, value, offset, true, noAssert)
+}
+
+Buffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {
+ return writeDouble(this, value, offset, false, noAssert)
+}
+
+// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
+Buffer.prototype.copy = function copy (target, targetStart, start, end) {
+ if (!start) start = 0
+ if (!end && end !== 0) end = this.length
+ if (targetStart >= target.length) targetStart = target.length
+ if (!targetStart) targetStart = 0
+ if (end > 0 && end < start) end = start
+
+ // Copy 0 bytes; we're done
+ if (end === start) return 0
+ if (target.length === 0 || this.length === 0) return 0
+
+ // Fatal error conditions
+ if (targetStart < 0) {
+ throw new RangeError('targetStart out of bounds')
+ }
+ if (start < 0 || start >= this.length) throw new RangeError('sourceStart out of bounds')
+ if (end < 0) throw new RangeError('sourceEnd out of bounds')
+
+ // Are we oob?
+ if (end > this.length) end = this.length
+ if (target.length - targetStart < end - start) {
+ end = target.length - targetStart + start
+ }
+
+ var len = end - start
+ var i
+
+ if (this === target && start < targetStart && targetStart < end) {
+ // descending copy from end
+ for (i = len - 1; i >= 0; i--) {
+ target[i + targetStart] = this[i + start]
+ }
+ } else if (len < 1000 || !Buffer.TYPED_ARRAY_SUPPORT) {
+ // ascending copy from start
+ for (i = 0; i < len; i++) {
+ target[i + targetStart] = this[i + start]
+ }
+ } else {
+ target._set(this.subarray(start, start + len), targetStart)
+ }
+
+ return len
+}
+
+// fill(value, start=0, end=buffer.length)
+Buffer.prototype.fill = function fill (value, start, end) {
+ if (!value) value = 0
+ if (!start) start = 0
+ if (!end) end = this.length
+
+ if (end < start) throw new RangeError('end < start')
+
+ // Fill 0 bytes; we're done
+ if (end === start) return
+ if (this.length === 0) return
+
+ if (start < 0 || start >= this.length) throw new RangeError('start out of bounds')
+ if (end < 0 || end > this.length) throw new RangeError('end out of bounds')
+
+ var i
+ if (typeof value === 'number') {
+ for (i = start; i < end; i++) {
+ this[i] = value
+ }
+ } else {
+ var bytes = utf8ToBytes(value.toString())
+ var len = bytes.length
+ for (i = start; i < end; i++) {
+ this[i] = bytes[i % len]
+ }
+ }
+
+ return this
+}
+
+/**
+ * Creates a new `ArrayBuffer` with the *copied* memory of the buffer instance.
+ * Added in Node 0.12. Only available in browsers that support ArrayBuffer.
+ */
+Buffer.prototype.toArrayBuffer = function toArrayBuffer () {
+ if (typeof Uint8Array !== 'undefined') {
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ return (new Buffer(this)).buffer
+ } else {
+ var buf = new Uint8Array(this.length)
+ for (var i = 0, len = buf.length; i < len; i += 1) {
+ buf[i] = this[i]
+ }
+ return buf.buffer
+ }
+ } else {
+ throw new TypeError('Buffer.toArrayBuffer not supported in this browser')
+ }
+}
+
+// HELPER FUNCTIONS
+// ================
+
+var BP = Buffer.prototype
+
+/**
+ * Augment a Uint8Array *instance* (not the Uint8Array class!) with Buffer methods
+ */
+Buffer._augment = function _augment (arr) {
+ arr.constructor = Buffer
+ arr._isBuffer = true
+
+ // save reference to original Uint8Array set method before overwriting
+ arr._set = arr.set
+
+ // deprecated
+ arr.get = BP.get
+ arr.set = BP.set
+
+ arr.write = BP.write
+ arr.toString = BP.toString
+ arr.toLocaleString = BP.toString
+ arr.toJSON = BP.toJSON
+ arr.equals = BP.equals
+ arr.compare = BP.compare
+ arr.indexOf = BP.indexOf
+ arr.copy = BP.copy
+ arr.slice = BP.slice
+ arr.readUIntLE = BP.readUIntLE
+ arr.readUIntBE = BP.readUIntBE
+ arr.readUInt8 = BP.readUInt8
+ arr.readUInt16LE = BP.readUInt16LE
+ arr.readUInt16BE = BP.readUInt16BE
+ arr.readUInt32LE = BP.readUInt32LE
+ arr.readUInt32BE = BP.readUInt32BE
+ arr.readIntLE = BP.readIntLE
+ arr.readIntBE = BP.readIntBE
+ arr.readInt8 = BP.readInt8
+ arr.readInt16LE = BP.readInt16LE
+ arr.readInt16BE = BP.readInt16BE
+ arr.readInt32LE = BP.readInt32LE
+ arr.readInt32BE = BP.readInt32BE
+ arr.readFloatLE = BP.readFloatLE
+ arr.readFloatBE = BP.readFloatBE
+ arr.readDoubleLE = BP.readDoubleLE
+ arr.readDoubleBE = BP.readDoubleBE
+ arr.writeUInt8 = BP.writeUInt8
+ arr.writeUIntLE = BP.writeUIntLE
+ arr.writeUIntBE = BP.writeUIntBE
+ arr.writeUInt16LE = BP.writeUInt16LE
+ arr.writeUInt16BE = BP.writeUInt16BE
+ arr.writeUInt32LE = BP.writeUInt32LE
+ arr.writeUInt32BE = BP.writeUInt32BE
+ arr.writeIntLE = BP.writeIntLE
+ arr.writeIntBE = BP.writeIntBE
+ arr.writeInt8 = BP.writeInt8
+ arr.writeInt16LE = BP.writeInt16LE
+ arr.writeInt16BE = BP.writeInt16BE
+ arr.writeInt32LE = BP.writeInt32LE
+ arr.writeInt32BE = BP.writeInt32BE
+ arr.writeFloatLE = BP.writeFloatLE
+ arr.writeFloatBE = BP.writeFloatBE
+ arr.writeDoubleLE = BP.writeDoubleLE
+ arr.writeDoubleBE = BP.writeDoubleBE
+ arr.fill = BP.fill
+ arr.inspect = BP.inspect
+ arr.toArrayBuffer = BP.toArrayBuffer
+
+ return arr
+}
+
+var INVALID_BASE64_RE = /[^+\/0-9A-Za-z-_]/g
+
+function base64clean (str) {
+ // Node strips out invalid characters like \n and \t from the string, base64-js does not
+ str = stringtrim(str).replace(INVALID_BASE64_RE, '')
+ // Node converts strings with length < 2 to ''
+ if (str.length < 2) return ''
+ // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
+ while (str.length % 4 !== 0) {
+ str = str + '='
+ }
+ return str
+}
+
+function stringtrim (str) {
+ if (str.trim) return str.trim()
+ return str.replace(/^\s+|\s+$/g, '')
+}
+
+function toHex (n) {
+ if (n < 16) return '0' + n.toString(16)
+ return n.toString(16)
+}
+
+function utf8ToBytes (string, units) {
+ units = units || Infinity
+ var codePoint
+ var length = string.length
+ var leadSurrogate = null
+ var bytes = []
+
+ for (var i = 0; i < length; i++) {
+ codePoint = string.charCodeAt(i)
+
+ // is surrogate component
+ if (codePoint > 0xD7FF && codePoint < 0xE000) {
+ // last char was a lead
+ if (!leadSurrogate) {
+ // no lead yet
+ if (codePoint > 0xDBFF) {
+ // unexpected trail
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ continue
+ } else if (i + 1 === length) {
+ // unpaired lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ continue
+ }
+
+ // valid lead
+ leadSurrogate = codePoint
+
+ continue
+ }
+
+ // 2 leads in a row
+ if (codePoint < 0xDC00) {
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ leadSurrogate = codePoint
+ continue
+ }
+
+ // valid surrogate pair
+ codePoint = leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00 | 0x10000
+ } else if (leadSurrogate) {
+ // valid bmp char, but last char was a lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ }
+
+ leadSurrogate = null
+
+ // encode utf8
+ if (codePoint < 0x80) {
+ if ((units -= 1) < 0) break
+ bytes.push(codePoint)
+ } else if (codePoint < 0x800) {
+ if ((units -= 2) < 0) break
+ bytes.push(
+ codePoint >> 0x6 | 0xC0,
+ codePoint & 0x3F | 0x80
+ )
+ } else if (codePoint < 0x10000) {
+ if ((units -= 3) < 0) break
+ bytes.push(
+ codePoint >> 0xC | 0xE0,
+ codePoint >> 0x6 & 0x3F | 0x80,
+ codePoint & 0x3F | 0x80
+ )
+ } else if (codePoint < 0x110000) {
+ if ((units -= 4) < 0) break
+ bytes.push(
+ codePoint >> 0x12 | 0xF0,
+ codePoint >> 0xC & 0x3F | 0x80,
+ codePoint >> 0x6 & 0x3F | 0x80,
+ codePoint & 0x3F | 0x80
+ )
+ } else {
+ throw new Error('Invalid code point')
+ }
+ }
+
+ return bytes
+}
+
+function asciiToBytes (str) {
+ var byteArray = []
+ for (var i = 0; i < str.length; i++) {
+ // Node's code seems to be doing this and not & 0x7F..
+ byteArray.push(str.charCodeAt(i) & 0xFF)
+ }
+ return byteArray
+}
+
+function utf16leToBytes (str, units) {
+ var c, hi, lo
+ var byteArray = []
+ for (var i = 0; i < str.length; i++) {
+ if ((units -= 2) < 0) break
+
+ c = str.charCodeAt(i)
+ hi = c >> 8
+ lo = c % 256
+ byteArray.push(lo)
+ byteArray.push(hi)
+ }
+
+ return byteArray
+}
+
+function base64ToBytes (str) {
+ return base64.toByteArray(base64clean(str))
+}
+
+function blitBuffer (src, dst, offset, length) {
+ for (var i = 0; i < length; i++) {
+ if ((i + offset >= dst.length) || (i >= src.length)) break
+ dst[i + offset] = src[i]
+ }
+ return i
+}
+
+}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
+},{"base64-js":2,"ieee754":4,"is-array":5}],4:[function(require,module,exports){
+exports.read = function (buffer, offset, isLE, mLen, nBytes) {
+ var e, m
+ var eLen = nBytes * 8 - mLen - 1
+ var eMax = (1 << eLen) - 1
+ var eBias = eMax >> 1
+ var nBits = -7
+ var i = isLE ? (nBytes - 1) : 0
+ var d = isLE ? -1 : 1
+ var s = buffer[offset + i]
+
+ i += d
+
+ e = s & ((1 << (-nBits)) - 1)
+ s >>= (-nBits)
+ nBits += eLen
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ m = e & ((1 << (-nBits)) - 1)
+ e >>= (-nBits)
+ nBits += mLen
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ if (e === 0) {
+ e = 1 - eBias
+ } else if (e === eMax) {
+ return m ? NaN : ((s ? -1 : 1) * Infinity)
+ } else {
+ m = m + Math.pow(2, mLen)
+ e = e - eBias
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen)
+}
+
+exports.write = function (buffer, value, offset, isLE, mLen, nBytes) {
+ var e, m, c
+ var eLen = nBytes * 8 - mLen - 1
+ var eMax = (1 << eLen) - 1
+ var eBias = eMax >> 1
+ var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)
+ var i = isLE ? 0 : (nBytes - 1)
+ var d = isLE ? 1 : -1
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0
+
+ value = Math.abs(value)
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0
+ e = eMax
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2)
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--
+ c *= 2
+ }
+ if (e + eBias >= 1) {
+ value += rt / c
+ } else {
+ value += rt * Math.pow(2, 1 - eBias)
+ }
+ if (value * c >= 2) {
+ e++
+ c /= 2
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0
+ e = eMax
+ } else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen)
+ e = e + eBias
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)
+ e = 0
+ }
+ }
+
+ for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
+
+ e = (e << mLen) | m
+ eLen += mLen
+ for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
+
+ buffer[offset + i - d] |= s * 128
+}
+
+},{}],5:[function(require,module,exports){
+
+/**
+ * isArray
+ */
+
+var isArray = Array.isArray;
+
+/**
+ * toString
+ */
+
+var str = Object.prototype.toString;
+
+/**
+ * Whether or not the given `val`
+ * is an array.
+ *
+ * example:
+ *
+ * isArray([]);
+ * // > true
+ * isArray(arguments);
+ * // > false
+ * isArray('');
+ * // > false
+ *
+ * @param {mixed} val
+ * @return {bool}
+ */
+
+module.exports = isArray || function (val) {
+ return !! val && '[object Array]' == str.call(val);
+};
+
+},{}],6:[function(require,module,exports){
+(function (global){
+
+/**
+ * Module exports.
+ */
+
+module.exports = deprecate;
+
+/**
+ * Mark that a method should not be used.
+ * Returns a modified function which warns once by default.
+ *
+ * If `localStorage.noDeprecation = true` is set, then it is a no-op.
+ *
+ * If `localStorage.throwDeprecation = true` is set, then deprecated functions
+ * will throw an Error when invoked.
+ *
+ * If `localStorage.traceDeprecation = true` is set, then deprecated functions
+ * will invoke `console.trace()` instead of `console.error()`.
+ *
+ * @param {Function} fn - the function to deprecate
+ * @param {String} msg - the string to print to the console when `fn` is invoked
+ * @returns {Function} a new "deprecated" version of `fn`
+ * @api public
+ */
+
+function deprecate (fn, msg) {
+ if (config('noDeprecation')) {
+ return fn;
+ }
+
+ var warned = false;
+ function deprecated() {
+ if (!warned) {
+ if (config('throwDeprecation')) {
+ throw new Error(msg);
+ } else if (config('traceDeprecation')) {
+ console.trace(msg);
+ } else {
+ console.warn(msg);
+ }
+ warned = true;
+ }
+ return fn.apply(this, arguments);
+ }
+
+ return deprecated;
+}
+
+/**
+ * Checks `localStorage` for boolean values for the given `name`.
+ *
+ * @param {String} name
+ * @returns {Boolean}
+ * @api private
+ */
+
+function config (name) {
+ // accessing global.localStorage can trigger a DOMException in sandboxed iframes
+ try {
+ if (!global.localStorage) return false;
+ } catch (_) {
+ return false;
+ }
+ var val = global.localStorage[name];
+ if (null == val) return false;
+ return String(val).toLowerCase() === 'true';
+}
+
+}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
+},{}],7:[function(require,module,exports){
+function DOMParser(options){
+ this.options = options ||{locator:{}};
+
+}
+DOMParser.prototype.parseFromString = function(source,mimeType){
+ var options = this.options;
+ var sax = new XMLReader();
+ var domBuilder = options.domBuilder || new DOMHandler();//contentHandler and LexicalHandler
+ var errorHandler = options.errorHandler;
+ var locator = options.locator;
+ var defaultNSMap = options.xmlns||{};
+ var entityMap = {'lt':'<','gt':'>','amp':'&','quot':'"','apos':"'"}
+ if(locator){
+ domBuilder.setDocumentLocator(locator)
+ }
+
+ sax.errorHandler = buildErrorHandler(errorHandler,domBuilder,locator);
+ sax.domBuilder = options.domBuilder || domBuilder;
+ if(/\/x?html?$/.test(mimeType)){
+ entityMap.nbsp = '\xa0';
+ entityMap.copy = '\xa9';
+ defaultNSMap['']= 'http://www.w3.org/1999/xhtml';
+ }
+ if(source){
+ sax.parse(source,defaultNSMap,entityMap);
+ }else{
+ sax.errorHandler.error("invalid document source");
+ }
+ return domBuilder.document;
+}
+function buildErrorHandler(errorImpl,domBuilder,locator){
+ if(!errorImpl){
+ if(domBuilder instanceof DOMHandler){
+ return domBuilder;
+ }
+ errorImpl = domBuilder ;
+ }
+ var errorHandler = {}
+ var isCallback = errorImpl instanceof Function;
+ locator = locator||{}
+ function build(key){
+ var fn = errorImpl[key];
+ if(!fn){
+ if(isCallback){
+ fn = errorImpl.length == 2?function(msg){errorImpl(key,msg)}:errorImpl;
+ }else{
+ var i=arguments.length;
+ while(--i){
+ if(fn = errorImpl[arguments[i]]){
+ break;
+ }
+ }
+ }
+ }
+ errorHandler[key] = fn && function(msg){
+ fn(msg+_locator(locator));
+ }||function(){};
+ }
+ build('warning','warn');
+ build('error','warn','warning');
+ build('fatalError','warn','warning','error');
+ return errorHandler;
+}
+/**
+ * +ContentHandler+ErrorHandler
+ * +LexicalHandler+EntityResolver2
+ * -DeclHandler-DTDHandler
+ *
+ * DefaultHandler:EntityResolver, DTDHandler, ContentHandler, ErrorHandler
+ * DefaultHandler2:DefaultHandler,LexicalHandler, DeclHandler, EntityResolver2
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/helpers/DefaultHandler.html
+ */
+function DOMHandler() {
+ this.cdata = false;
+}
+function position(locator,node){
+ node.lineNumber = locator.lineNumber;
+ node.columnNumber = locator.columnNumber;
+}
+/**
+ * @see org.xml.sax.ContentHandler#startDocument
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/ContentHandler.html
+ */
+DOMHandler.prototype = {
+ startDocument : function() {
+ this.document = new DOMImplementation().createDocument(null, null, null);
+ if (this.locator) {
+ this.document.documentURI = this.locator.systemId;
+ }
+ },
+ startElement:function(namespaceURI, localName, qName, attrs) {
+ var doc = this.document;
+ var el = doc.createElementNS(namespaceURI, qName||localName);
+ var len = attrs.length;
+ appendElement(this, el);
+ this.currentElement = el;
+
+ this.locator && position(this.locator,el)
+ for (var i = 0 ; i < len; i++) {
+ var namespaceURI = attrs.getURI(i);
+ var value = attrs.getValue(i);
+ var qName = attrs.getQName(i);
+ var attr = doc.createAttributeNS(namespaceURI, qName);
+ if( attr.getOffset){
+ position(attr.getOffset(1),attr)
+ }
+ attr.value = attr.nodeValue = value;
+ el.setAttributeNode(attr)
+ }
+ },
+ endElement:function(namespaceURI, localName, qName) {
+ var current = this.currentElement
+ var tagName = current.tagName;
+ this.currentElement = current.parentNode;
+ },
+ startPrefixMapping:function(prefix, uri) {
+ },
+ endPrefixMapping:function(prefix) {
+ },
+ processingInstruction:function(target, data) {
+ var ins = this.document.createProcessingInstruction(target, data);
+ this.locator && position(this.locator,ins)
+ appendElement(this, ins);
+ },
+ ignorableWhitespace:function(ch, start, length) {
+ },
+ characters:function(chars, start, length) {
+ chars = _toString.apply(this,arguments)
+ //console.log(chars)
+ if(this.currentElement && chars){
+ if (this.cdata) {
+ var charNode = this.document.createCDATASection(chars);
+ this.currentElement.appendChild(charNode);
+ } else {
+ var charNode = this.document.createTextNode(chars);
+ this.currentElement.appendChild(charNode);
+ }
+ this.locator && position(this.locator,charNode)
+ }
+ },
+ skippedEntity:function(name) {
+ },
+ endDocument:function() {
+ this.document.normalize();
+ },
+ setDocumentLocator:function (locator) {
+ if(this.locator = locator){// && !('lineNumber' in locator)){
+ locator.lineNumber = 0;
+ }
+ },
+ //LexicalHandler
+ comment:function(chars, start, length) {
+ chars = _toString.apply(this,arguments)
+ var comm = this.document.createComment(chars);
+ this.locator && position(this.locator,comm)
+ appendElement(this, comm);
+ },
+
+ startCDATA:function() {
+ //used in characters() methods
+ this.cdata = true;
+ },
+ endCDATA:function() {
+ this.cdata = false;
+ },
+
+ startDTD:function(name, publicId, systemId) {
+ var impl = this.document.implementation;
+ if (impl && impl.createDocumentType) {
+ var dt = impl.createDocumentType(name, publicId, systemId);
+ this.locator && position(this.locator,dt)
+ appendElement(this, dt);
+ }
+ },
+ /**
+ * @see org.xml.sax.ErrorHandler
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/ErrorHandler.html
+ */
+ warning:function(error) {
+ console.warn(error,_locator(this.locator));
+ },
+ error:function(error) {
+ console.error(error,_locator(this.locator));
+ },
+ fatalError:function(error) {
+ console.error(error,_locator(this.locator));
+ throw error;
+ }
+}
+function _locator(l){
+ if(l){
+ return '\n@'+(l.systemId ||'')+'#[line:'+l.lineNumber+',col:'+l.columnNumber+']'
+ }
+}
+function _toString(chars,start,length){
+ if(typeof chars == 'string'){
+ return chars.substr(start,length)
+ }else{//java sax connect width xmldom on rhino(what about: "? && !(chars instanceof String)")
+ if(chars.length >= start+length || start){
+ return new java.lang.String(chars,start,length)+'';
+ }
+ return chars;
+ }
+}
+
+/*
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/ext/LexicalHandler.html
+ * used method of org.xml.sax.ext.LexicalHandler:
+ * #comment(chars, start, length)
+ * #startCDATA()
+ * #endCDATA()
+ * #startDTD(name, publicId, systemId)
+ *
+ *
+ * IGNORED method of org.xml.sax.ext.LexicalHandler:
+ * #endDTD()
+ * #startEntity(name)
+ * #endEntity(name)
+ *
+ *
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/ext/DeclHandler.html
+ * IGNORED method of org.xml.sax.ext.DeclHandler
+ * #attributeDecl(eName, aName, type, mode, value)
+ * #elementDecl(name, model)
+ * #externalEntityDecl(name, publicId, systemId)
+ * #internalEntityDecl(name, value)
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/ext/EntityResolver2.html
+ * IGNORED method of org.xml.sax.EntityResolver2
+ * #resolveEntity(String name,String publicId,String baseURI,String systemId)
+ * #resolveEntity(publicId, systemId)
+ * #getExternalSubset(name, baseURI)
+ * @link http://www.saxproject.org/apidoc/org/xml/sax/DTDHandler.html
+ * IGNORED method of org.xml.sax.DTDHandler
+ * #notationDecl(name, publicId, systemId) {};
+ * #unparsedEntityDecl(name, publicId, systemId, notationName) {};
+ */
+"endDTD,startEntity,endEntity,attributeDecl,elementDecl,externalEntityDecl,internalEntityDecl,resolveEntity,getExternalSubset,notationDecl,unparsedEntityDecl".replace(/\w+/g,function(key){
+ DOMHandler.prototype[key] = function(){return null}
+})
+
+/* Private static helpers treated below as private instance methods, so don't need to add these to the public API; we might use a Relator to also get rid of non-standard public properties */
+function appendElement (hander,node) {
+ if (!hander.currentElement) {
+ hander.document.appendChild(node);
+ } else {
+ hander.currentElement.appendChild(node);
+ }
+}//appendChild and setAttributeNS are preformance key
+
+if(typeof require == 'function'){
+ var XMLReader = require('./sax').XMLReader;
+ var DOMImplementation = exports.DOMImplementation = require('./dom').DOMImplementation;
+ exports.XMLSerializer = require('./dom').XMLSerializer ;
+ exports.DOMParser = DOMParser;
+}
+
+},{"./dom":8,"./sax":9}],8:[function(require,module,exports){
+/*
+ * DOM Level 2
+ * Object DOMException
+ * @see http://www.w3.org/TR/REC-DOM-Level-1/ecma-script-language-binding.html
+ * @see http://www.w3.org/TR/2000/REC-DOM-Level-2-Core-20001113/ecma-script-binding.html
+ */
+
+function copy(src,dest){
+ for(var p in src){
+ dest[p] = src[p];
+ }
+}
+/**
+^\w+\.prototype\.([_\w]+)\s*=\s*((?:.*\{\s*?[\r\n][\s\S]*?^})|\S.*?(?=[;\r\n]));?
+^\w+\.prototype\.([_\w]+)\s*=\s*(\S.*?(?=[;\r\n]));?
+ */
+function _extends(Class,Super){
+ var pt = Class.prototype;
+ if(Object.create){
+ var ppt = Object.create(Super.prototype)
+ pt.__proto__ = ppt;
+ }
+ if(!(pt instanceof Super)){
+ function t(){};
+ t.prototype = Super.prototype;
+ t = new t();
+ copy(pt,t);
+ Class.prototype = pt = t;
+ }
+ if(pt.constructor != Class){
+ if(typeof Class != 'function'){
+ console.error("unknow Class:"+Class)
+ }
+ pt.constructor = Class
+ }
+}
+var htmlns = 'http://www.w3.org/1999/xhtml' ;
+// Node Types
+var NodeType = {}
+var ELEMENT_NODE = NodeType.ELEMENT_NODE = 1;
+var ATTRIBUTE_NODE = NodeType.ATTRIBUTE_NODE = 2;
+var TEXT_NODE = NodeType.TEXT_NODE = 3;
+var CDATA_SECTION_NODE = NodeType.CDATA_SECTION_NODE = 4;
+var ENTITY_REFERENCE_NODE = NodeType.ENTITY_REFERENCE_NODE = 5;
+var ENTITY_NODE = NodeType.ENTITY_NODE = 6;
+var PROCESSING_INSTRUCTION_NODE = NodeType.PROCESSING_INSTRUCTION_NODE = 7;
+var COMMENT_NODE = NodeType.COMMENT_NODE = 8;
+var DOCUMENT_NODE = NodeType.DOCUMENT_NODE = 9;
+var DOCUMENT_TYPE_NODE = NodeType.DOCUMENT_TYPE_NODE = 10;
+var DOCUMENT_FRAGMENT_NODE = NodeType.DOCUMENT_FRAGMENT_NODE = 11;
+var NOTATION_NODE = NodeType.NOTATION_NODE = 12;
+
+// ExceptionCode
+var ExceptionCode = {}
+var ExceptionMessage = {};
+var INDEX_SIZE_ERR = ExceptionCode.INDEX_SIZE_ERR = ((ExceptionMessage[1]="Index size error"),1);
+var DOMSTRING_SIZE_ERR = ExceptionCode.DOMSTRING_SIZE_ERR = ((ExceptionMessage[2]="DOMString size error"),2);
+var HIERARCHY_REQUEST_ERR = ExceptionCode.HIERARCHY_REQUEST_ERR = ((ExceptionMessage[3]="Hierarchy request error"),3);
+var WRONG_DOCUMENT_ERR = ExceptionCode.WRONG_DOCUMENT_ERR = ((ExceptionMessage[4]="Wrong document"),4);
+var INVALID_CHARACTER_ERR = ExceptionCode.INVALID_CHARACTER_ERR = ((ExceptionMessage[5]="Invalid character"),5);
+var NO_DATA_ALLOWED_ERR = ExceptionCode.NO_DATA_ALLOWED_ERR = ((ExceptionMessage[6]="No data allowed"),6);
+var NO_MODIFICATION_ALLOWED_ERR = ExceptionCode.NO_MODIFICATION_ALLOWED_ERR = ((ExceptionMessage[7]="No modification allowed"),7);
+var NOT_FOUND_ERR = ExceptionCode.NOT_FOUND_ERR = ((ExceptionMessage[8]="Not found"),8);
+var NOT_SUPPORTED_ERR = ExceptionCode.NOT_SUPPORTED_ERR = ((ExceptionMessage[9]="Not supported"),9);
+var INUSE_ATTRIBUTE_ERR = ExceptionCode.INUSE_ATTRIBUTE_ERR = ((ExceptionMessage[10]="Attribute in use"),10);
+//level2
+var INVALID_STATE_ERR = ExceptionCode.INVALID_STATE_ERR = ((ExceptionMessage[11]="Invalid state"),11);
+var SYNTAX_ERR = ExceptionCode.SYNTAX_ERR = ((ExceptionMessage[12]="Syntax error"),12);
+var INVALID_MODIFICATION_ERR = ExceptionCode.INVALID_MODIFICATION_ERR = ((ExceptionMessage[13]="Invalid modification"),13);
+var NAMESPACE_ERR = ExceptionCode.NAMESPACE_ERR = ((ExceptionMessage[14]="Invalid namespace"),14);
+var INVALID_ACCESS_ERR = ExceptionCode.INVALID_ACCESS_ERR = ((ExceptionMessage[15]="Invalid access"),15);
+
+
+function DOMException(code, message) {
+ if(message instanceof Error){
+ var error = message;
+ }else{
+ error = this;
+ Error.call(this, ExceptionMessage[code]);
+ this.message = ExceptionMessage[code];
+ if(Error.captureStackTrace) Error.captureStackTrace(this, DOMException);
+ }
+ error.code = code;
+ if(message) this.message = this.message + ": " + message;
+ return error;
+};
+DOMException.prototype = Error.prototype;
+copy(ExceptionCode,DOMException)
+/**
+ * @see http://www.w3.org/TR/2000/REC-DOM-Level-2-Core-20001113/core.html#ID-536297177
+ * The NodeList interface provides the abstraction of an ordered collection of nodes, without defining or constraining how this collection is implemented. NodeList objects in the DOM are live.
+ * The items in the NodeList are accessible via an integral index, starting from 0.
+ */
+function NodeList() {
+};
+NodeList.prototype = {
+ /**
+ * The number of nodes in the list. The range of valid child node indices is 0 to length-1 inclusive.
+ * @standard level1
+ */
+ length:0,
+ /**
+ * Returns the indexth item in the collection. If index is greater than or equal to the number of nodes in the list, this returns null.
+ * @standard level1
+ * @param index unsigned long
+ * Index into the collection.
+ * @return Node
+ * The node at the indexth position in the NodeList, or null if that is not a valid index.
+ */
+ item: function(index) {
+ return this[index] || null;
+ }
+};
+function LiveNodeList(node,refresh){
+ this._node = node;
+ this._refresh = refresh
+ _updateLiveList(this);
+}
+function _updateLiveList(list){
+ var inc = list._node._inc || list._node.ownerDocument._inc;
+ if(list._inc != inc){
+ var ls = list._refresh(list._node);
+ //console.log(ls.length)
+ __set__(list,'length',ls.length);
+ copy(ls,list);
+ list._inc = inc;
+ }
+}
+LiveNodeList.prototype.item = function(i){
+ _updateLiveList(this);
+ return this[i];
+}
+
+_extends(LiveNodeList,NodeList);
+/**
+ *
+ * Objects implementing the NamedNodeMap interface are used to represent collections of nodes that can be accessed by name. Note that NamedNodeMap does not inherit from NodeList; NamedNodeMaps are not maintained in any particular order. Objects contained in an object implementing NamedNodeMap may also be accessed by an ordinal index, but this is simply to allow convenient enumeration of the contents of a NamedNodeMap, and does not imply that the DOM specifies an order to these Nodes.
+ * NamedNodeMap objects in the DOM are live.
+ * used for attributes or DocumentType entities
+ */
+function NamedNodeMap() {
+};
+
+function _findNodeIndex(list,node){
+ var i = list.length;
+ while(i--){
+ if(list[i] === node){return i}
+ }
+}
+
+function _addNamedNode(el,list,newAttr,oldAttr){
+ if(oldAttr){
+ list[_findNodeIndex(list,oldAttr)] = newAttr;
+ }else{
+ list[list.length++] = newAttr;
+ }
+ if(el){
+ newAttr.ownerElement = el;
+ var doc = el.ownerDocument;
+ if(doc){
+ oldAttr && _onRemoveAttribute(doc,el,oldAttr);
+ _onAddAttribute(doc,el,newAttr);
+ }
+ }
+}
+function _removeNamedNode(el,list,attr){
+ var i = _findNodeIndex(list,attr);
+ if(i>=0){
+ var lastIndex = list.length-1
+ while(i<lastIndex){
+ list[i] = list[++i]
+ }
+ list.length = lastIndex;
+ if(el){
+ var doc = el.ownerDocument;
+ if(doc){
+ _onRemoveAttribute(doc,el,attr);
+ attr.ownerElement = null;
+ }
+ }
+ }else{
+ throw DOMException(NOT_FOUND_ERR,new Error())
+ }
+}
+NamedNodeMap.prototype = {
+ length:0,
+ item:NodeList.prototype.item,
+ getNamedItem: function(key) {
+// if(key.indexOf(':')>0 || key == 'xmlns'){
+// return null;
+// }
+ var i = this.length;
+ while(i--){
+ var attr = this[i];
+ if(attr.nodeName == key){
+ return attr;
+ }
+ }
+ },
+ setNamedItem: function(attr) {
+ var el = attr.ownerElement;
+ if(el && el!=this._ownerElement){
+ throw new DOMException(INUSE_ATTRIBUTE_ERR);
+ }
+ var oldAttr = this.getNamedItem(attr.nodeName);
+ _addNamedNode(this._ownerElement,this,attr,oldAttr);
+ return oldAttr;
+ },
+ /* returns Node */
+ setNamedItemNS: function(attr) {// raises: WRONG_DOCUMENT_ERR,NO_MODIFICATION_ALLOWED_ERR,INUSE_ATTRIBUTE_ERR
+ var el = attr.ownerElement, oldAttr;
+ if(el && el!=this._ownerElement){
+ throw new DOMException(INUSE_ATTRIBUTE_ERR);
+ }
+ oldAttr = this.getNamedItemNS(attr.namespaceURI,attr.localName);
+ _addNamedNode(this._ownerElement,this,attr,oldAttr);
+ return oldAttr;
+ },
+
+ /* returns Node */
+ removeNamedItem: function(key) {
+ var attr = this.getNamedItem(key);
+ _removeNamedNode(this._ownerElement,this,attr);
+ return attr;
+
+
+ },// raises: NOT_FOUND_ERR,NO_MODIFICATION_ALLOWED_ERR
+
+ //for level2
+ removeNamedItemNS:function(namespaceURI,localName){
+ var attr = this.getNamedItemNS(namespaceURI,localName);
+ _removeNamedNode(this._ownerElement,this,attr);
+ return attr;
+ },
+ getNamedItemNS: function(namespaceURI, localName) {
+ var i = this.length;
+ while(i--){
+ var node = this[i];
+ if(node.localName == localName && node.namespaceURI == namespaceURI){
+ return node;
+ }
+ }
+ return null;
+ }
+};
+/**
+ * @see http://www.w3.org/TR/REC-DOM-Level-1/level-one-core.html#ID-102161490
+ */
+function DOMImplementation(/* Object */ features) {
+ this._features = {};
+ if (features) {
+ for (var feature in features) {
+ this._features = features[feature];
+ }
+ }
+};
+
+DOMImplementation.prototype = {
+ hasFeature: function(/* string */ feature, /* string */ version) {
+ var versions = this._features[feature.toLowerCase()];
+ if (versions && (!version || version in versions)) {
+ return true;
+ } else {
+ return false;
+ }
+ },
+ // Introduced in DOM Level 2:
+ createDocument:function(namespaceURI, qualifiedName, doctype){// raises:INVALID_CHARACTER_ERR,NAMESPACE_ERR,WRONG_DOCUMENT_ERR
+ var doc = new Document();
+ doc.doctype = doctype;
+ if(doctype){
+ doc.appendChild(doctype);
+ }
+ doc.implementation = this;
+ doc.childNodes = new NodeList();
+ if(qualifiedName){
+ var root = doc.createElementNS(namespaceURI,qualifiedName);
+ doc.appendChild(root);
+ }
+ return doc;
+ },
+ // Introduced in DOM Level 2:
+ createDocumentType:function(qualifiedName, publicId, systemId){// raises:INVALID_CHARACTER_ERR,NAMESPACE_ERR
+ var node = new DocumentType();
+ node.name = qualifiedName;
+ node.nodeName = qualifiedName;
+ node.publicId = publicId;
+ node.systemId = systemId;
+ // Introduced in DOM Level 2:
+ //readonly attribute DOMString internalSubset;
+
+ //TODO:..
+ // readonly attribute NamedNodeMap entities;
+ // readonly attribute NamedNodeMap notations;
+ return node;
+ }
+};
+
+
+/**
+ * @see http://www.w3.org/TR/2000/REC-DOM-Level-2-Core-20001113/core.html#ID-1950641247
+ */
+
+function Node() {
+};
+
+Node.prototype = {
+ firstChild : null,
+ lastChild : null,
+ previousSibling : null,
+ nextSibling : null,
+ attributes : null,
+ parentNode : null,
+ childNodes : null,
+ ownerDocument : null,
+ nodeValue : null,
+ namespaceURI : null,
+ prefix : null,
+ localName : null,
+ // Modified in DOM Level 2:
+ insertBefore:function(newChild, refChild){//raises
+ return _insertBefore(this,newChild,refChild);
+ },
+ replaceChild:function(newChild, oldChild){//raises
+ this.insertBefore(newChild,oldChild);
+ if(oldChild){
+ this.removeChild(oldChild);
+ }
+ },
+ removeChild:function(oldChild){
+ return _removeChild(this,oldChild);
+ },
+ appendChild:function(newChild){
+ return this.insertBefore(newChild,null);
+ },
+ hasChildNodes:function(){
+ return this.firstChild != null;
+ },
+ cloneNode:function(deep){
+ return cloneNode(this.ownerDocument||this,this,deep);
+ },
+ // Modified in DOM Level 2:
+ normalize:function(){
+ var child = this.firstChild;
+ while(child){
+ var next = child.nextSibling;
+ if(next && next.nodeType == TEXT_NODE && child.nodeType == TEXT_NODE){
+ this.removeChild(next);
+ child.appendData(next.data);
+ }else{
+ child.normalize();
+ child = next;
+ }
+ }
+ },
+ // Introduced in DOM Level 2:
+ isSupported:function(feature, version){
+ return this.ownerDocument.implementation.hasFeature(feature,version);
+ },
+ // Introduced in DOM Level 2:
+ hasAttributes:function(){
+ return this.attributes.length>0;
+ },
+ lookupPrefix:function(namespaceURI){
+ var el = this;
+ while(el){
+ var map = el._nsMap;
+ //console.dir(map)
+ if(map){
+ for(var n in map){
+ if(map[n] == namespaceURI){
+ return n;
+ }
+ }
+ }
+ el = el.nodeType == 2?el.ownerDocument : el.parentNode;
+ }
+ return null;
+ },
+ // Introduced in DOM Level 3:
+ lookupNamespaceURI:function(prefix){
+ var el = this;
+ while(el){
+ var map = el._nsMap;
+ //console.dir(map)
+ if(map){
+ if(prefix in map){
+ return map[prefix] ;
+ }
+ }
+ el = el.nodeType == 2?el.ownerDocument : el.parentNode;
+ }
+ return null;
+ },
+ // Introduced in DOM Level 3:
+ isDefaultNamespace:function(namespaceURI){
+ var prefix = this.lookupPrefix(namespaceURI);
+ return prefix == null;
+ }
+};
+
+
+function _xmlEncoder(c){
+ return c == '<' && '<' ||
+ c == '>' && '>' ||
+ c == '&' && '&' ||
+ c == '"' && '"' ||
+ '&#'+c.charCodeAt()+';'
+}
+
+
+copy(NodeType,Node);
+copy(NodeType,Node.prototype);
+
+/**
+ * @param callback return true for continue,false for break
+ * @return boolean true: break visit;
+ */
+function _visitNode(node,callback){
+ if(callback(node)){
+ return true;
+ }
+ if(node = node.firstChild){
+ do{
+ if(_visitNode(node,callback)){return true}
+ }while(node=node.nextSibling)
+ }
+}
+
+
+
+function Document(){
+}
+function _onAddAttribute(doc,el,newAttr){
+ doc && doc._inc++;
+ var ns = newAttr.namespaceURI ;
+ if(ns == 'http://www.w3.org/2000/xmlns/'){
+ //update namespace
+ el._nsMap[newAttr.prefix?newAttr.localName:''] = newAttr.value
+ }
+}
+function _onRemoveAttribute(doc,el,newAttr,remove){
+ doc && doc._inc++;
+ var ns = newAttr.namespaceURI ;
+ if(ns == 'http://www.w3.org/2000/xmlns/'){
+ //update namespace
+ delete el._nsMap[newAttr.prefix?newAttr.localName:'']
+ }
+}
+function _onUpdateChild(doc,el,newChild){
+ if(doc && doc._inc){
+ doc._inc++;
+ //update childNodes
+ var cs = el.childNodes;
+ if(newChild){
+ cs[cs.length++] = newChild;
+ }else{
+ //console.log(1)
+ var child = el.firstChild;
+ var i = 0;
+ while(child){
+ cs[i++] = child;
+ child =child.nextSibling;
+ }
+ cs.length = i;
+ }
+ }
+}
+
+/**
+ * attributes;
+ * children;
+ *
+ * writeable properties:
+ * nodeValue,Attr:value,CharacterData:data
+ * prefix
+ */
+function _removeChild(parentNode,child){
+ var previous = child.previousSibling;
+ var next = child.nextSibling;
+ if(previous){
+ previous.nextSibling = next;
+ }else{
+ parentNode.firstChild = next
+ }
+ if(next){
+ next.previousSibling = previous;
+ }else{
+ parentNode.lastChild = previous;
+ }
+ _onUpdateChild(parentNode.ownerDocument,parentNode);
+ return child;
+}
+/**
+ * preformance key(refChild == null)
+ */
+function _insertBefore(parentNode,newChild,nextChild){
+ var cp = newChild.parentNode;
+ if(cp){
+ cp.removeChild(newChild);//remove and update
+ }
+ if(newChild.nodeType === DOCUMENT_FRAGMENT_NODE){
+ var newFirst = newChild.firstChild;
+ if (newFirst == null) {
+ return newChild;
+ }
+ var newLast = newChild.lastChild;
+ }else{
+ newFirst = newLast = newChild;
+ }
+ var pre = nextChild ? nextChild.previousSibling : parentNode.lastChild;
+
+ newFirst.previousSibling = pre;
+ newLast.nextSibling = nextChild;
+
+
+ if(pre){
+ pre.nextSibling = newFirst;
+ }else{
+ parentNode.firstChild = newFirst;
+ }
+ if(nextChild == null){
+ parentNode.lastChild = newLast;
+ }else{
+ nextChild.previousSibling = newLast;
+ }
+ do{
+ newFirst.parentNode = parentNode;
+ }while(newFirst !== newLast && (newFirst= newFirst.nextSibling))
+ _onUpdateChild(parentNode.ownerDocument||parentNode,parentNode);
+ //console.log(parentNode.lastChild.nextSibling == null)
+ if (newChild.nodeType == DOCUMENT_FRAGMENT_NODE) {
+ newChild.firstChild = newChild.lastChild = null;
+ }
+ return newChild;
+}
+function _appendSingleChild(parentNode,newChild){
+ var cp = newChild.parentNode;
+ if(cp){
+ var pre = parentNode.lastChild;
+ cp.removeChild(newChild);//remove and update
+ var pre = parentNode.lastChild;
+ }
+ var pre = parentNode.lastChild;
+ newChild.parentNode = parentNode;
+ newChild.previousSibling = pre;
+ newChild.nextSibling = null;
+ if(pre){
+ pre.nextSibling = newChild;
+ }else{
+ parentNode.firstChild = newChild;
+ }
+ parentNode.lastChild = newChild;
+ _onUpdateChild(parentNode.ownerDocument,parentNode,newChild);
+ return newChild;
+ //console.log("__aa",parentNode.lastChild.nextSibling == null)
+}
+Document.prototype = {
+ //implementation : null,
+ nodeName : '#document',
+ nodeType : DOCUMENT_NODE,
+ doctype : null,
+ documentElement : null,
+ _inc : 1,
+
+ insertBefore : function(newChild, refChild){//raises
+ if(newChild.nodeType == DOCUMENT_FRAGMENT_NODE){
+ var child = newChild.firstChild;
+ while(child){
+ var next = child.nextSibling;
+ this.insertBefore(child,refChild);
+ child = next;
+ }
+ return newChild;
+ }
+ if(this.documentElement == null && newChild.nodeType == 1){
+ this.documentElement = newChild;
+ }
+
+ return _insertBefore(this,newChild,refChild),(newChild.ownerDocument = this),newChild;
+ },
+ removeChild : function(oldChild){
+ if(this.documentElement == oldChild){
+ this.documentElement = null;
+ }
+ return _removeChild(this,oldChild);
+ },
+ // Introduced in DOM Level 2:
+ importNode : function(importedNode,deep){
+ return importNode(this,importedNode,deep);
+ },
+ // Introduced in DOM Level 2:
+ getElementById : function(id){
+ var rtv = null;
+ _visitNode(this.documentElement,function(node){
+ if(node.nodeType == 1){
+ if(node.getAttribute('id') == id){
+ rtv = node;
+ return true;
+ }
+ }
+ })
+ return rtv;
+ },
+
+ //document factory method:
+ createElement : function(tagName){
+ var node = new Element();
+ node.ownerDocument = this;
+ node.nodeName = tagName;
+ node.tagName = tagName;
+ node.childNodes = new NodeList();
+ var attrs = node.attributes = new NamedNodeMap();
+ attrs._ownerElement = node;
+ return node;
+ },
+ createDocumentFragment : function(){
+ var node = new DocumentFragment();
+ node.ownerDocument = this;
+ node.childNodes = new NodeList();
+ return node;
+ },
+ createTextNode : function(data){
+ var node = new Text();
+ node.ownerDocument = this;
+ node.appendData(data)
+ return node;
+ },
+ createComment : function(data){
+ var node = new Comment();
+ node.ownerDocument = this;
+ node.appendData(data)
+ return node;
+ },
+ createCDATASection : function(data){
+ var node = new CDATASection();
+ node.ownerDocument = this;
+ node.appendData(data)
+ return node;
+ },
+ createProcessingInstruction : function(target,data){
+ var node = new ProcessingInstruction();
+ node.ownerDocument = this;
+ node.tagName = node.target = target;
+ node.nodeValue= node.data = data;
+ return node;
+ },
+ createAttribute : function(name){
+ var node = new Attr();
+ node.ownerDocument = this;
+ node.name = name;
+ node.nodeName = name;
+ node.localName = name;
+ node.specified = true;
+ return node;
+ },
+ createEntityReference : function(name){
+ var node = new EntityReference();
+ node.ownerDocument = this;
+ node.nodeName = name;
+ return node;
+ },
+ // Introduced in DOM Level 2:
+ createElementNS : function(namespaceURI,qualifiedName){
+ var node = new Element();
+ var pl = qualifiedName.split(':');
+ var attrs = node.attributes = new NamedNodeMap();
+ node.childNodes = new NodeList();
+ node.ownerDocument = this;
+ node.nodeName = qualifiedName;
+ node.tagName = qualifiedName;
+ node.namespaceURI = namespaceURI;
+ if(pl.length == 2){
+ node.prefix = pl[0];
+ node.localName = pl[1];
+ }else{
+ //el.prefix = null;
+ node.localName = qualifiedName;
+ }
+ attrs._ownerElement = node;
+ return node;
+ },
+ // Introduced in DOM Level 2:
+ createAttributeNS : function(namespaceURI,qualifiedName){
+ var node = new Attr();
+ var pl = qualifiedName.split(':');
+ node.ownerDocument = this;
+ node.nodeName = qualifiedName;
+ node.name = qualifiedName;
+ node.namespaceURI = namespaceURI;
+ node.specified = true;
+ if(pl.length == 2){
+ node.prefix = pl[0];
+ node.localName = pl[1];
+ }else{
+ //el.prefix = null;
+ node.localName = qualifiedName;
+ }
+ return node;
+ }
+};
+_extends(Document,Node);
+
+
+function Element() {
+ this._nsMap = {};
+};
+Element.prototype = {
+ nodeType : ELEMENT_NODE,
+ hasAttribute : function(name){
+ return this.getAttributeNode(name)!=null;
+ },
+ getAttribute : function(name){
+ var attr = this.getAttributeNode(name);
+ return attr && attr.value || '';
+ },
+ getAttributeNode : function(name){
+ return this.attributes.getNamedItem(name);
+ },
+ setAttribute : function(name, value){
+ var attr = this.ownerDocument.createAttribute(name);
+ attr.value = attr.nodeValue = "" + value;
+ this.se
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[29/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/without.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/without.js b/node_modules/lodash/array/without.js
new file mode 100644
index 0000000..2ac3d11
--- /dev/null
+++ b/node_modules/lodash/array/without.js
@@ -0,0 +1,27 @@
+var baseDifference = require('../internal/baseDifference'),
+ isArrayLike = require('../internal/isArrayLike'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates an array excluding all provided values using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to filter.
+ * @param {...*} [values] The values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ * @example
+ *
+ * _.without([1, 2, 1, 3], 1, 2);
+ * // => [3]
+ */
+var without = restParam(function(array, values) {
+ return isArrayLike(array)
+ ? baseDifference(array, values)
+ : [];
+});
+
+module.exports = without;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/xor.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/xor.js b/node_modules/lodash/array/xor.js
new file mode 100644
index 0000000..04ef32a
--- /dev/null
+++ b/node_modules/lodash/array/xor.js
@@ -0,0 +1,35 @@
+var arrayPush = require('../internal/arrayPush'),
+ baseDifference = require('../internal/baseDifference'),
+ baseUniq = require('../internal/baseUniq'),
+ isArrayLike = require('../internal/isArrayLike');
+
+/**
+ * Creates an array of unique values that is the [symmetric difference](https://en.wikipedia.org/wiki/Symmetric_difference)
+ * of the provided arrays.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {...Array} [arrays] The arrays to inspect.
+ * @returns {Array} Returns the new array of values.
+ * @example
+ *
+ * _.xor([1, 2], [4, 2]);
+ * // => [1, 4]
+ */
+function xor() {
+ var index = -1,
+ length = arguments.length;
+
+ while (++index < length) {
+ var array = arguments[index];
+ if (isArrayLike(array)) {
+ var result = result
+ ? arrayPush(baseDifference(result, array), baseDifference(array, result))
+ : array;
+ }
+ }
+ return result ? baseUniq(result) : [];
+}
+
+module.exports = xor;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/zip.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/zip.js b/node_modules/lodash/array/zip.js
new file mode 100644
index 0000000..53a6f69
--- /dev/null
+++ b/node_modules/lodash/array/zip.js
@@ -0,0 +1,21 @@
+var restParam = require('../function/restParam'),
+ unzip = require('./unzip');
+
+/**
+ * Creates an array of grouped elements, the first of which contains the first
+ * elements of the given arrays, the second of which contains the second elements
+ * of the given arrays, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {...Array} [arrays] The arrays to process.
+ * @returns {Array} Returns the new array of grouped elements.
+ * @example
+ *
+ * _.zip(['fred', 'barney'], [30, 40], [true, false]);
+ * // => [['fred', 30, true], ['barney', 40, false]]
+ */
+var zip = restParam(unzip);
+
+module.exports = zip;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/zipObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/zipObject.js b/node_modules/lodash/array/zipObject.js
new file mode 100644
index 0000000..dec7a21
--- /dev/null
+++ b/node_modules/lodash/array/zipObject.js
@@ -0,0 +1,43 @@
+var isArray = require('../lang/isArray');
+
+/**
+ * The inverse of `_.pairs`; this method returns an object composed from arrays
+ * of property names and values. Provide either a single two dimensional array,
+ * e.g. `[[key1, value1], [key2, value2]]` or two arrays, one of property names
+ * and one of corresponding values.
+ *
+ * @static
+ * @memberOf _
+ * @alias object
+ * @category Array
+ * @param {Array} props The property names.
+ * @param {Array} [values=[]] The property values.
+ * @returns {Object} Returns the new object.
+ * @example
+ *
+ * _.zipObject([['fred', 30], ['barney', 40]]);
+ * // => { 'fred': 30, 'barney': 40 }
+ *
+ * _.zipObject(['fred', 'barney'], [30, 40]);
+ * // => { 'fred': 30, 'barney': 40 }
+ */
+function zipObject(props, values) {
+ var index = -1,
+ length = props ? props.length : 0,
+ result = {};
+
+ if (length && !values && !isArray(props[0])) {
+ values = [];
+ }
+ while (++index < length) {
+ var key = props[index];
+ if (values) {
+ result[key] = values[index];
+ } else if (key) {
+ result[key[0]] = key[1];
+ }
+ }
+ return result;
+}
+
+module.exports = zipObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/array/zipWith.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/array/zipWith.js b/node_modules/lodash/array/zipWith.js
new file mode 100644
index 0000000..ad10374
--- /dev/null
+++ b/node_modules/lodash/array/zipWith.js
@@ -0,0 +1,36 @@
+var restParam = require('../function/restParam'),
+ unzipWith = require('./unzipWith');
+
+/**
+ * This method is like `_.zip` except that it accepts an iteratee to specify
+ * how grouped values should be combined. The `iteratee` is bound to `thisArg`
+ * and invoked with four arguments: (accumulator, value, index, group).
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {...Array} [arrays] The arrays to process.
+ * @param {Function} [iteratee] The function to combine grouped values.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array} Returns the new array of grouped elements.
+ * @example
+ *
+ * _.zipWith([1, 2], [10, 20], [100, 200], _.add);
+ * // => [111, 222]
+ */
+var zipWith = restParam(function(arrays) {
+ var length = arrays.length,
+ iteratee = length > 2 ? arrays[length - 2] : undefined,
+ thisArg = length > 1 ? arrays[length - 1] : undefined;
+
+ if (length > 2 && typeof iteratee == 'function') {
+ length -= 2;
+ } else {
+ iteratee = (length > 1 && typeof thisArg == 'function') ? (--length, thisArg) : undefined;
+ thisArg = undefined;
+ }
+ arrays.length = length;
+ return unzipWith(arrays, iteratee, thisArg);
+});
+
+module.exports = zipWith;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain.js b/node_modules/lodash/chain.js
new file mode 100644
index 0000000..6439627
--- /dev/null
+++ b/node_modules/lodash/chain.js
@@ -0,0 +1,16 @@
+module.exports = {
+ 'chain': require('./chain/chain'),
+ 'commit': require('./chain/commit'),
+ 'concat': require('./chain/concat'),
+ 'lodash': require('./chain/lodash'),
+ 'plant': require('./chain/plant'),
+ 'reverse': require('./chain/reverse'),
+ 'run': require('./chain/run'),
+ 'tap': require('./chain/tap'),
+ 'thru': require('./chain/thru'),
+ 'toJSON': require('./chain/toJSON'),
+ 'toString': require('./chain/toString'),
+ 'value': require('./chain/value'),
+ 'valueOf': require('./chain/valueOf'),
+ 'wrapperChain': require('./chain/wrapperChain')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/chain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/chain.js b/node_modules/lodash/chain/chain.js
new file mode 100644
index 0000000..453ba1e
--- /dev/null
+++ b/node_modules/lodash/chain/chain.js
@@ -0,0 +1,35 @@
+var lodash = require('./lodash');
+
+/**
+ * Creates a `lodash` object that wraps `value` with explicit method
+ * chaining enabled.
+ *
+ * @static
+ * @memberOf _
+ * @category Chain
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 },
+ * { 'user': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _.chain(users)
+ * .sortBy('age')
+ * .map(function(chr) {
+ * return chr.user + ' is ' + chr.age;
+ * })
+ * .first()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+function chain(value) {
+ var result = lodash(value);
+ result.__chain__ = true;
+ return result;
+}
+
+module.exports = chain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/commit.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/commit.js b/node_modules/lodash/chain/commit.js
new file mode 100644
index 0000000..c732d1b
--- /dev/null
+++ b/node_modules/lodash/chain/commit.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperCommit');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/concat.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/concat.js b/node_modules/lodash/chain/concat.js
new file mode 100644
index 0000000..90607d1
--- /dev/null
+++ b/node_modules/lodash/chain/concat.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperConcat');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/lodash.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/lodash.js b/node_modules/lodash/chain/lodash.js
new file mode 100644
index 0000000..1c3467e
--- /dev/null
+++ b/node_modules/lodash/chain/lodash.js
@@ -0,0 +1,125 @@
+var LazyWrapper = require('../internal/LazyWrapper'),
+ LodashWrapper = require('../internal/LodashWrapper'),
+ baseLodash = require('../internal/baseLodash'),
+ isArray = require('../lang/isArray'),
+ isObjectLike = require('../internal/isObjectLike'),
+ wrapperClone = require('../internal/wrapperClone');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates a `lodash` object which wraps `value` to enable implicit chaining.
+ * Methods that operate on and return arrays, collections, and functions can
+ * be chained together. Methods that retrieve a single value or may return a
+ * primitive value will automatically end the chain returning the unwrapped
+ * value. Explicit chaining may be enabled using `_.chain`. The execution of
+ * chained methods is lazy, that is, execution is deferred until `_#value`
+ * is implicitly or explicitly called.
+ *
+ * Lazy evaluation allows several methods to support shortcut fusion. Shortcut
+ * fusion is an optimization strategy which merge iteratee calls; this can help
+ * to avoid the creation of intermediate data structures and greatly reduce the
+ * number of iteratee executions.
+ *
+ * Chaining is supported in custom builds as long as the `_#value` method is
+ * directly or indirectly included in the build.
+ *
+ * In addition to lodash methods, wrappers have `Array` and `String` methods.
+ *
+ * The wrapper `Array` methods are:
+ * `concat`, `join`, `pop`, `push`, `reverse`, `shift`, `slice`, `sort`,
+ * `splice`, and `unshift`
+ *
+ * The wrapper `String` methods are:
+ * `replace` and `split`
+ *
+ * The wrapper methods that support shortcut fusion are:
+ * `compact`, `drop`, `dropRight`, `dropRightWhile`, `dropWhile`, `filter`,
+ * `first`, `initial`, `last`, `map`, `pluck`, `reject`, `rest`, `reverse`,
+ * `slice`, `take`, `takeRight`, `takeRightWhile`, `takeWhile`, `toArray`,
+ * and `where`
+ *
+ * The chainable wrapper methods are:
+ * `after`, `ary`, `assign`, `at`, `before`, `bind`, `bindAll`, `bindKey`,
+ * `callback`, `chain`, `chunk`, `commit`, `compact`, `concat`, `constant`,
+ * `countBy`, `create`, `curry`, `debounce`, `defaults`, `defaultsDeep`,
+ * `defer`, `delay`, `difference`, `drop`, `dropRight`, `dropRightWhile`,
+ * `dropWhile`, `fill`, `filter`, `flatten`, `flattenDeep`, `flow`, `flowRight`,
+ * `forEach`, `forEachRight`, `forIn`, `forInRight`, `forOwn`, `forOwnRight`,
+ * `functions`, `groupBy`, `indexBy`, `initial`, `intersection`, `invert`,
+ * `invoke`, `keys`, `keysIn`, `map`, `mapKeys`, `mapValues`, `matches`,
+ * `matchesProperty`, `memoize`, `merge`, `method`, `methodOf`, `mixin`,
+ * `modArgs`, `negate`, `omit`, `once`, `pairs`, `partial`, `partialRight`,
+ * `partition`, `pick`, `plant`, `pluck`, `property`, `propertyOf`, `pull`,
+ * `pullAt`, `push`, `range`, `rearg`, `reject`, `remove`, `rest`, `restParam`,
+ * `reverse`, `set`, `shuffle`, `slice`, `sort`, `sortBy`, `sortByAll`,
+ * `sortByOrder`, `splice`, `spread`, `take`, `takeRight`, `takeRightWhile`,
+ * `takeWhile`, `tap`, `throttle`, `thru`, `times`, `toArray`, `toPlainObject`,
+ * `transform`, `union`, `uniq`, `unshift`, `unzip`, `unzipWith`, `values`,
+ * `valuesIn`, `where`, `without`, `wrap`, `xor`, `zip`, `zipObject`, `zipWith`
+ *
+ * The wrapper methods that are **not** chainable by default are:
+ * `add`, `attempt`, `camelCase`, `capitalize`, `ceil`, `clone`, `cloneDeep`,
+ * `deburr`, `endsWith`, `escape`, `escapeRegExp`, `every`, `find`, `findIndex`,
+ * `findKey`, `findLast`, `findLastIndex`, `findLastKey`, `findWhere`, `first`,
+ * `floor`, `get`, `gt`, `gte`, `has`, `identity`, `includes`, `indexOf`,
+ * `inRange`, `isArguments`, `isArray`, `isBoolean`, `isDate`, `isElement`,
+ * `isEmpty`, `isEqual`, `isError`, `isFinite` `isFunction`, `isMatch`,
+ * `isNative`, `isNaN`, `isNull`, `isNumber`, `isObject`, `isPlainObject`,
+ * `isRegExp`, `isString`, `isUndefined`, `isTypedArray`, `join`, `kebabCase`,
+ * `last`, `lastIndexOf`, `lt`, `lte`, `max`, `min`, `noConflict`, `noop`,
+ * `now`, `pad`, `padLeft`, `padRight`, `parseInt`, `pop`, `random`, `reduce`,
+ * `reduceRight`, `repeat`, `result`, `round`, `runInContext`, `shift`, `size`,
+ * `snakeCase`, `some`, `sortedIndex`, `sortedLastIndex`, `startCase`,
+ * `startsWith`, `sum`, `template`, `trim`, `trimLeft`, `trimRight`, `trunc`,
+ * `unescape`, `uniqueId`, `value`, and `words`
+ *
+ * The wrapper method `sample` will return a wrapped value when `n` is provided,
+ * otherwise an unwrapped value is returned.
+ *
+ * @name _
+ * @constructor
+ * @category Chain
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // returns an unwrapped value
+ * wrapped.reduce(function(total, n) {
+ * return total + n;
+ * });
+ * // => 6
+ *
+ * // returns a wrapped value
+ * var squares = wrapped.map(function(n) {
+ * return n * n;
+ * });
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+function lodash(value) {
+ if (isObjectLike(value) && !isArray(value) && !(value instanceof LazyWrapper)) {
+ if (value instanceof LodashWrapper) {
+ return value;
+ }
+ if (hasOwnProperty.call(value, '__chain__') && hasOwnProperty.call(value, '__wrapped__')) {
+ return wrapperClone(value);
+ }
+ }
+ return new LodashWrapper(value);
+}
+
+// Ensure wrappers are instances of `baseLodash`.
+lodash.prototype = baseLodash.prototype;
+
+module.exports = lodash;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/plant.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/plant.js b/node_modules/lodash/chain/plant.js
new file mode 100644
index 0000000..04099f2
--- /dev/null
+++ b/node_modules/lodash/chain/plant.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperPlant');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/reverse.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/reverse.js b/node_modules/lodash/chain/reverse.js
new file mode 100644
index 0000000..f72a64a
--- /dev/null
+++ b/node_modules/lodash/chain/reverse.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperReverse');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/run.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/run.js b/node_modules/lodash/chain/run.js
new file mode 100644
index 0000000..5e751a2
--- /dev/null
+++ b/node_modules/lodash/chain/run.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperValue');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/tap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/tap.js b/node_modules/lodash/chain/tap.js
new file mode 100644
index 0000000..3d0257e
--- /dev/null
+++ b/node_modules/lodash/chain/tap.js
@@ -0,0 +1,29 @@
+/**
+ * This method invokes `interceptor` and returns `value`. The interceptor is
+ * bound to `thisArg` and invoked with one argument; (value). The purpose of
+ * this method is to "tap into" a method chain in order to perform operations
+ * on intermediate results within the chain.
+ *
+ * @static
+ * @memberOf _
+ * @category Chain
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @param {*} [thisArg] The `this` binding of `interceptor`.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * _([1, 2, 3])
+ * .tap(function(array) {
+ * array.pop();
+ * })
+ * .reverse()
+ * .value();
+ * // => [2, 1]
+ */
+function tap(value, interceptor, thisArg) {
+ interceptor.call(thisArg, value);
+ return value;
+}
+
+module.exports = tap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/thru.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/thru.js b/node_modules/lodash/chain/thru.js
new file mode 100644
index 0000000..a715780
--- /dev/null
+++ b/node_modules/lodash/chain/thru.js
@@ -0,0 +1,26 @@
+/**
+ * This method is like `_.tap` except that it returns the result of `interceptor`.
+ *
+ * @static
+ * @memberOf _
+ * @category Chain
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @param {*} [thisArg] The `this` binding of `interceptor`.
+ * @returns {*} Returns the result of `interceptor`.
+ * @example
+ *
+ * _(' abc ')
+ * .chain()
+ * .trim()
+ * .thru(function(value) {
+ * return [value];
+ * })
+ * .value();
+ * // => ['abc']
+ */
+function thru(value, interceptor, thisArg) {
+ return interceptor.call(thisArg, value);
+}
+
+module.exports = thru;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/toJSON.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/toJSON.js b/node_modules/lodash/chain/toJSON.js
new file mode 100644
index 0000000..5e751a2
--- /dev/null
+++ b/node_modules/lodash/chain/toJSON.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperValue');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/toString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/toString.js b/node_modules/lodash/chain/toString.js
new file mode 100644
index 0000000..c7bcbf9
--- /dev/null
+++ b/node_modules/lodash/chain/toString.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperToString');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/value.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/value.js b/node_modules/lodash/chain/value.js
new file mode 100644
index 0000000..5e751a2
--- /dev/null
+++ b/node_modules/lodash/chain/value.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperValue');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/valueOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/valueOf.js b/node_modules/lodash/chain/valueOf.js
new file mode 100644
index 0000000..5e751a2
--- /dev/null
+++ b/node_modules/lodash/chain/valueOf.js
@@ -0,0 +1 @@
+module.exports = require('./wrapperValue');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperChain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperChain.js b/node_modules/lodash/chain/wrapperChain.js
new file mode 100644
index 0000000..3823481
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperChain.js
@@ -0,0 +1,32 @@
+var chain = require('./chain');
+
+/**
+ * Enables explicit method chaining on the wrapper object.
+ *
+ * @name chain
+ * @memberOf _
+ * @category Chain
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * // without explicit chaining
+ * _(users).first();
+ * // => { 'user': 'barney', 'age': 36 }
+ *
+ * // with explicit chaining
+ * _(users).chain()
+ * .first()
+ * .pick('user')
+ * .value();
+ * // => { 'user': 'barney' }
+ */
+function wrapperChain() {
+ return chain(this);
+}
+
+module.exports = wrapperChain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperCommit.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperCommit.js b/node_modules/lodash/chain/wrapperCommit.js
new file mode 100644
index 0000000..c3d2898
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperCommit.js
@@ -0,0 +1,32 @@
+var LodashWrapper = require('../internal/LodashWrapper');
+
+/**
+ * Executes the chained sequence and returns the wrapped result.
+ *
+ * @name commit
+ * @memberOf _
+ * @category Chain
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var array = [1, 2];
+ * var wrapped = _(array).push(3);
+ *
+ * console.log(array);
+ * // => [1, 2]
+ *
+ * wrapped = wrapped.commit();
+ * console.log(array);
+ * // => [1, 2, 3]
+ *
+ * wrapped.last();
+ * // => 3
+ *
+ * console.log(array);
+ * // => [1, 2, 3]
+ */
+function wrapperCommit() {
+ return new LodashWrapper(this.value(), this.__chain__);
+}
+
+module.exports = wrapperCommit;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperConcat.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperConcat.js b/node_modules/lodash/chain/wrapperConcat.js
new file mode 100644
index 0000000..799156c
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperConcat.js
@@ -0,0 +1,34 @@
+var arrayConcat = require('../internal/arrayConcat'),
+ baseFlatten = require('../internal/baseFlatten'),
+ isArray = require('../lang/isArray'),
+ restParam = require('../function/restParam'),
+ toObject = require('../internal/toObject');
+
+/**
+ * Creates a new array joining a wrapped array with any additional arrays
+ * and/or values.
+ *
+ * @name concat
+ * @memberOf _
+ * @category Chain
+ * @param {...*} [values] The values to concatenate.
+ * @returns {Array} Returns the new concatenated array.
+ * @example
+ *
+ * var array = [1];
+ * var wrapped = _(array).concat(2, [3], [[4]]);
+ *
+ * console.log(wrapped.value());
+ * // => [1, 2, 3, [4]]
+ *
+ * console.log(array);
+ * // => [1]
+ */
+var wrapperConcat = restParam(function(values) {
+ values = baseFlatten(values);
+ return this.thru(function(array) {
+ return arrayConcat(isArray(array) ? array : [toObject(array)], values);
+ });
+});
+
+module.exports = wrapperConcat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperPlant.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperPlant.js b/node_modules/lodash/chain/wrapperPlant.js
new file mode 100644
index 0000000..234fe41
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperPlant.js
@@ -0,0 +1,45 @@
+var baseLodash = require('../internal/baseLodash'),
+ wrapperClone = require('../internal/wrapperClone');
+
+/**
+ * Creates a clone of the chained sequence planting `value` as the wrapped value.
+ *
+ * @name plant
+ * @memberOf _
+ * @category Chain
+ * @returns {Object} Returns the new `lodash` wrapper instance.
+ * @example
+ *
+ * var array = [1, 2];
+ * var wrapped = _(array).map(function(value) {
+ * return Math.pow(value, 2);
+ * });
+ *
+ * var other = [3, 4];
+ * var otherWrapped = wrapped.plant(other);
+ *
+ * otherWrapped.value();
+ * // => [9, 16]
+ *
+ * wrapped.value();
+ * // => [1, 4]
+ */
+function wrapperPlant(value) {
+ var result,
+ parent = this;
+
+ while (parent instanceof baseLodash) {
+ var clone = wrapperClone(parent);
+ if (result) {
+ previous.__wrapped__ = clone;
+ } else {
+ result = clone;
+ }
+ var previous = clone;
+ parent = parent.__wrapped__;
+ }
+ previous.__wrapped__ = value;
+ return result;
+}
+
+module.exports = wrapperPlant;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperReverse.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperReverse.js b/node_modules/lodash/chain/wrapperReverse.js
new file mode 100644
index 0000000..6ba546d
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperReverse.js
@@ -0,0 +1,43 @@
+var LazyWrapper = require('../internal/LazyWrapper'),
+ LodashWrapper = require('../internal/LodashWrapper'),
+ thru = require('./thru');
+
+/**
+ * Reverses the wrapped array so the first element becomes the last, the
+ * second element becomes the second to last, and so on.
+ *
+ * **Note:** This method mutates the wrapped array.
+ *
+ * @name reverse
+ * @memberOf _
+ * @category Chain
+ * @returns {Object} Returns the new reversed `lodash` wrapper instance.
+ * @example
+ *
+ * var array = [1, 2, 3];
+ *
+ * _(array).reverse().value()
+ * // => [3, 2, 1]
+ *
+ * console.log(array);
+ * // => [3, 2, 1]
+ */
+function wrapperReverse() {
+ var value = this.__wrapped__;
+
+ var interceptor = function(value) {
+ return value.reverse();
+ };
+ if (value instanceof LazyWrapper) {
+ var wrapped = value;
+ if (this.__actions__.length) {
+ wrapped = new LazyWrapper(this);
+ }
+ wrapped = wrapped.reverse();
+ wrapped.__actions__.push({ 'func': thru, 'args': [interceptor], 'thisArg': undefined });
+ return new LodashWrapper(wrapped, this.__chain__);
+ }
+ return this.thru(interceptor);
+}
+
+module.exports = wrapperReverse;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperToString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperToString.js b/node_modules/lodash/chain/wrapperToString.js
new file mode 100644
index 0000000..db975a5
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperToString.js
@@ -0,0 +1,17 @@
+/**
+ * Produces the result of coercing the unwrapped value to a string.
+ *
+ * @name toString
+ * @memberOf _
+ * @category Chain
+ * @returns {string} Returns the coerced string value.
+ * @example
+ *
+ * _([1, 2, 3]).toString();
+ * // => '1,2,3'
+ */
+function wrapperToString() {
+ return (this.value() + '');
+}
+
+module.exports = wrapperToString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/chain/wrapperValue.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/chain/wrapperValue.js b/node_modules/lodash/chain/wrapperValue.js
new file mode 100644
index 0000000..2734e41
--- /dev/null
+++ b/node_modules/lodash/chain/wrapperValue.js
@@ -0,0 +1,20 @@
+var baseWrapperValue = require('../internal/baseWrapperValue');
+
+/**
+ * Executes the chained sequence to extract the unwrapped value.
+ *
+ * @name value
+ * @memberOf _
+ * @alias run, toJSON, valueOf
+ * @category Chain
+ * @returns {*} Returns the resolved unwrapped value.
+ * @example
+ *
+ * _([1, 2, 3]).value();
+ * // => [1, 2, 3]
+ */
+function wrapperValue() {
+ return baseWrapperValue(this.__wrapped__, this.__actions__);
+}
+
+module.exports = wrapperValue;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection.js b/node_modules/lodash/collection.js
new file mode 100644
index 0000000..0338857
--- /dev/null
+++ b/node_modules/lodash/collection.js
@@ -0,0 +1,44 @@
+module.exports = {
+ 'all': require('./collection/all'),
+ 'any': require('./collection/any'),
+ 'at': require('./collection/at'),
+ 'collect': require('./collection/collect'),
+ 'contains': require('./collection/contains'),
+ 'countBy': require('./collection/countBy'),
+ 'detect': require('./collection/detect'),
+ 'each': require('./collection/each'),
+ 'eachRight': require('./collection/eachRight'),
+ 'every': require('./collection/every'),
+ 'filter': require('./collection/filter'),
+ 'find': require('./collection/find'),
+ 'findLast': require('./collection/findLast'),
+ 'findWhere': require('./collection/findWhere'),
+ 'foldl': require('./collection/foldl'),
+ 'foldr': require('./collection/foldr'),
+ 'forEach': require('./collection/forEach'),
+ 'forEachRight': require('./collection/forEachRight'),
+ 'groupBy': require('./collection/groupBy'),
+ 'include': require('./collection/include'),
+ 'includes': require('./collection/includes'),
+ 'indexBy': require('./collection/indexBy'),
+ 'inject': require('./collection/inject'),
+ 'invoke': require('./collection/invoke'),
+ 'map': require('./collection/map'),
+ 'max': require('./math/max'),
+ 'min': require('./math/min'),
+ 'partition': require('./collection/partition'),
+ 'pluck': require('./collection/pluck'),
+ 'reduce': require('./collection/reduce'),
+ 'reduceRight': require('./collection/reduceRight'),
+ 'reject': require('./collection/reject'),
+ 'sample': require('./collection/sample'),
+ 'select': require('./collection/select'),
+ 'shuffle': require('./collection/shuffle'),
+ 'size': require('./collection/size'),
+ 'some': require('./collection/some'),
+ 'sortBy': require('./collection/sortBy'),
+ 'sortByAll': require('./collection/sortByAll'),
+ 'sortByOrder': require('./collection/sortByOrder'),
+ 'sum': require('./math/sum'),
+ 'where': require('./collection/where')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/all.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/all.js b/node_modules/lodash/collection/all.js
new file mode 100644
index 0000000..d0839f7
--- /dev/null
+++ b/node_modules/lodash/collection/all.js
@@ -0,0 +1 @@
+module.exports = require('./every');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/any.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/any.js b/node_modules/lodash/collection/any.js
new file mode 100644
index 0000000..900ac25
--- /dev/null
+++ b/node_modules/lodash/collection/any.js
@@ -0,0 +1 @@
+module.exports = require('./some');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/at.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/at.js b/node_modules/lodash/collection/at.js
new file mode 100644
index 0000000..29236e5
--- /dev/null
+++ b/node_modules/lodash/collection/at.js
@@ -0,0 +1,29 @@
+var baseAt = require('../internal/baseAt'),
+ baseFlatten = require('../internal/baseFlatten'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates an array of elements corresponding to the given keys, or indexes,
+ * of `collection`. Keys may be specified as individual arguments or as arrays
+ * of keys.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {...(number|number[]|string|string[])} [props] The property names
+ * or indexes of elements to pick, specified individually or in arrays.
+ * @returns {Array} Returns the new array of picked elements.
+ * @example
+ *
+ * _.at(['a', 'b', 'c'], [0, 2]);
+ * // => ['a', 'c']
+ *
+ * _.at(['barney', 'fred', 'pebbles'], 0, 2);
+ * // => ['barney', 'pebbles']
+ */
+var at = restParam(function(collection, props) {
+ return baseAt(collection, baseFlatten(props));
+});
+
+module.exports = at;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/collect.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/collect.js b/node_modules/lodash/collection/collect.js
new file mode 100644
index 0000000..0d1e1ab
--- /dev/null
+++ b/node_modules/lodash/collection/collect.js
@@ -0,0 +1 @@
+module.exports = require('./map');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/contains.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/contains.js b/node_modules/lodash/collection/contains.js
new file mode 100644
index 0000000..594722a
--- /dev/null
+++ b/node_modules/lodash/collection/contains.js
@@ -0,0 +1 @@
+module.exports = require('./includes');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/countBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/countBy.js b/node_modules/lodash/collection/countBy.js
new file mode 100644
index 0000000..e97dbb7
--- /dev/null
+++ b/node_modules/lodash/collection/countBy.js
@@ -0,0 +1,54 @@
+var createAggregator = require('../internal/createAggregator');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` through `iteratee`. The corresponding value
+ * of each key is the number of times the key was returned by `iteratee`.
+ * The `iteratee` is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(n) {
+ * return Math.floor(n);
+ * });
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(n) {
+ * return this.floor(n);
+ * }, Math);
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+var countBy = createAggregator(function(result, value, key) {
+ hasOwnProperty.call(result, key) ? ++result[key] : (result[key] = 1);
+});
+
+module.exports = countBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/detect.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/detect.js b/node_modules/lodash/collection/detect.js
new file mode 100644
index 0000000..2fb6303
--- /dev/null
+++ b/node_modules/lodash/collection/detect.js
@@ -0,0 +1 @@
+module.exports = require('./find');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/each.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/each.js b/node_modules/lodash/collection/each.js
new file mode 100644
index 0000000..8800f42
--- /dev/null
+++ b/node_modules/lodash/collection/each.js
@@ -0,0 +1 @@
+module.exports = require('./forEach');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/eachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/eachRight.js b/node_modules/lodash/collection/eachRight.js
new file mode 100644
index 0000000..3252b2a
--- /dev/null
+++ b/node_modules/lodash/collection/eachRight.js
@@ -0,0 +1 @@
+module.exports = require('./forEachRight');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/every.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/every.js b/node_modules/lodash/collection/every.js
new file mode 100644
index 0000000..5a2d0f5
--- /dev/null
+++ b/node_modules/lodash/collection/every.js
@@ -0,0 +1,66 @@
+var arrayEvery = require('../internal/arrayEvery'),
+ baseCallback = require('../internal/baseCallback'),
+ baseEvery = require('../internal/baseEvery'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Checks if `predicate` returns truthy for **all** elements of `collection`.
+ * The predicate is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.every(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.every(users, 'active', false);
+ * // => true
+ *
+ * // using the `_.property` callback shorthand
+ * _.every(users, 'active');
+ * // => false
+ */
+function every(collection, predicate, thisArg) {
+ var func = isArray(collection) ? arrayEvery : baseEvery;
+ if (thisArg && isIterateeCall(collection, predicate, thisArg)) {
+ predicate = undefined;
+ }
+ if (typeof predicate != 'function' || thisArg !== undefined) {
+ predicate = baseCallback(predicate, thisArg, 3);
+ }
+ return func(collection, predicate);
+}
+
+module.exports = every;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/filter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/filter.js b/node_modules/lodash/collection/filter.js
new file mode 100644
index 0000000..7620aa7
--- /dev/null
+++ b/node_modules/lodash/collection/filter.js
@@ -0,0 +1,61 @@
+var arrayFilter = require('../internal/arrayFilter'),
+ baseCallback = require('../internal/baseCallback'),
+ baseFilter = require('../internal/baseFilter'),
+ isArray = require('../lang/isArray');
+
+/**
+ * Iterates over elements of `collection`, returning an array of all elements
+ * `predicate` returns truthy for. The predicate is bound to `thisArg` and
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias select
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the new filtered array.
+ * @example
+ *
+ * _.filter([4, 5, 6], function(n) {
+ * return n % 2 == 0;
+ * });
+ * // => [4, 6]
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.pluck(_.filter(users, { 'age': 36, 'active': true }), 'user');
+ * // => ['barney']
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.pluck(_.filter(users, 'active', false), 'user');
+ * // => ['fred']
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.filter(users, 'active'), 'user');
+ * // => ['barney']
+ */
+function filter(collection, predicate, thisArg) {
+ var func = isArray(collection) ? arrayFilter : baseFilter;
+ predicate = baseCallback(predicate, thisArg, 3);
+ return func(collection, predicate);
+}
+
+module.exports = filter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/find.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/find.js b/node_modules/lodash/collection/find.js
new file mode 100644
index 0000000..7358cfe
--- /dev/null
+++ b/node_modules/lodash/collection/find.js
@@ -0,0 +1,56 @@
+var baseEach = require('../internal/baseEach'),
+ createFind = require('../internal/createFind');
+
+/**
+ * Iterates over elements of `collection`, returning the first element
+ * `predicate` returns truthy for. The predicate is bound to `thisArg` and
+ * invoked with three arguments: (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias detect
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false },
+ * { 'user': 'pebbles', 'age': 1, 'active': true }
+ * ];
+ *
+ * _.result(_.find(users, function(chr) {
+ * return chr.age < 40;
+ * }), 'user');
+ * // => 'barney'
+ *
+ * // using the `_.matches` callback shorthand
+ * _.result(_.find(users, { 'age': 1, 'active': true }), 'user');
+ * // => 'pebbles'
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.result(_.find(users, 'active', false), 'user');
+ * // => 'fred'
+ *
+ * // using the `_.property` callback shorthand
+ * _.result(_.find(users, 'active'), 'user');
+ * // => 'barney'
+ */
+var find = createFind(baseEach);
+
+module.exports = find;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/findLast.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/findLast.js b/node_modules/lodash/collection/findLast.js
new file mode 100644
index 0000000..75dbadc
--- /dev/null
+++ b/node_modules/lodash/collection/findLast.js
@@ -0,0 +1,25 @@
+var baseEachRight = require('../internal/baseEachRight'),
+ createFind = require('../internal/createFind');
+
+/**
+ * This method is like `_.find` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * _.findLast([1, 2, 3, 4], function(n) {
+ * return n % 2 == 1;
+ * });
+ * // => 3
+ */
+var findLast = createFind(baseEachRight, true);
+
+module.exports = findLast;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/findWhere.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/findWhere.js b/node_modules/lodash/collection/findWhere.js
new file mode 100644
index 0000000..2d62065
--- /dev/null
+++ b/node_modules/lodash/collection/findWhere.js
@@ -0,0 +1,37 @@
+var baseMatches = require('../internal/baseMatches'),
+ find = require('./find');
+
+/**
+ * Performs a deep comparison between each element in `collection` and the
+ * source object, returning the first element that has equivalent property
+ * values.
+ *
+ * **Note:** This method supports comparing arrays, booleans, `Date` objects,
+ * numbers, `Object` objects, regexes, and strings. Objects are compared by
+ * their own, not inherited, enumerable properties. For comparing a single
+ * own or inherited property value see `_.matchesProperty`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {Object} source The object of property values to match.
+ * @returns {*} Returns the matched element, else `undefined`.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * _.result(_.findWhere(users, { 'age': 36, 'active': true }), 'user');
+ * // => 'barney'
+ *
+ * _.result(_.findWhere(users, { 'age': 40, 'active': false }), 'user');
+ * // => 'fred'
+ */
+function findWhere(collection, source) {
+ return find(collection, baseMatches(source));
+}
+
+module.exports = findWhere;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/foldl.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/foldl.js b/node_modules/lodash/collection/foldl.js
new file mode 100644
index 0000000..26f53cf
--- /dev/null
+++ b/node_modules/lodash/collection/foldl.js
@@ -0,0 +1 @@
+module.exports = require('./reduce');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/foldr.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/foldr.js b/node_modules/lodash/collection/foldr.js
new file mode 100644
index 0000000..8fb199e
--- /dev/null
+++ b/node_modules/lodash/collection/foldr.js
@@ -0,0 +1 @@
+module.exports = require('./reduceRight');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/forEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/forEach.js b/node_modules/lodash/collection/forEach.js
new file mode 100644
index 0000000..05a8e21
--- /dev/null
+++ b/node_modules/lodash/collection/forEach.js
@@ -0,0 +1,37 @@
+var arrayEach = require('../internal/arrayEach'),
+ baseEach = require('../internal/baseEach'),
+ createForEach = require('../internal/createForEach');
+
+/**
+ * Iterates over elements of `collection` invoking `iteratee` for each element.
+ * The `iteratee` is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection). Iteratee functions may exit iteration early
+ * by explicitly returning `false`.
+ *
+ * **Note:** As with other "Collections" methods, objects with a "length" property
+ * are iterated like arrays. To avoid this behavior `_.forIn` or `_.forOwn`
+ * may be used for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @alias each
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2]).forEach(function(n) {
+ * console.log(n);
+ * }).value();
+ * // => logs each value from left to right and returns the array
+ *
+ * _.forEach({ 'a': 1, 'b': 2 }, function(n, key) {
+ * console.log(n, key);
+ * });
+ * // => logs each value-key pair and returns the object (iteration order is not guaranteed)
+ */
+var forEach = createForEach(arrayEach, baseEach);
+
+module.exports = forEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/forEachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/forEachRight.js b/node_modules/lodash/collection/forEachRight.js
new file mode 100644
index 0000000..3499711
--- /dev/null
+++ b/node_modules/lodash/collection/forEachRight.js
@@ -0,0 +1,26 @@
+var arrayEachRight = require('../internal/arrayEachRight'),
+ baseEachRight = require('../internal/baseEachRight'),
+ createForEach = require('../internal/createForEach');
+
+/**
+ * This method is like `_.forEach` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias eachRight
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2]).forEachRight(function(n) {
+ * console.log(n);
+ * }).value();
+ * // => logs each value from right to left and returns the array
+ */
+var forEachRight = createForEach(arrayEachRight, baseEachRight);
+
+module.exports = forEachRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/groupBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/groupBy.js b/node_modules/lodash/collection/groupBy.js
new file mode 100644
index 0000000..a925c89
--- /dev/null
+++ b/node_modules/lodash/collection/groupBy.js
@@ -0,0 +1,59 @@
+var createAggregator = require('../internal/createAggregator');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` through `iteratee`. The corresponding value
+ * of each key is an array of the elements responsible for generating the key.
+ * The `iteratee` is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(n) {
+ * return Math.floor(n);
+ * });
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(n) {
+ * return this.floor(n);
+ * }, Math);
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * // using the `_.property` callback shorthand
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+var groupBy = createAggregator(function(result, value, key) {
+ if (hasOwnProperty.call(result, key)) {
+ result[key].push(value);
+ } else {
+ result[key] = [value];
+ }
+});
+
+module.exports = groupBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/include.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/include.js b/node_modules/lodash/collection/include.js
new file mode 100644
index 0000000..594722a
--- /dev/null
+++ b/node_modules/lodash/collection/include.js
@@ -0,0 +1 @@
+module.exports = require('./includes');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/includes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/includes.js b/node_modules/lodash/collection/includes.js
new file mode 100644
index 0000000..329486a
--- /dev/null
+++ b/node_modules/lodash/collection/includes.js
@@ -0,0 +1,57 @@
+var baseIndexOf = require('../internal/baseIndexOf'),
+ getLength = require('../internal/getLength'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ isLength = require('../internal/isLength'),
+ isString = require('../lang/isString'),
+ values = require('../object/values');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Checks if `target` is in `collection` using
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/6.0/#sec-samevaluezero)
+ * for equality comparisons. If `fromIndex` is negative, it's used as the offset
+ * from the end of `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @alias contains, include
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {*} target The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.reduce`.
+ * @returns {boolean} Returns `true` if a matching element is found, else `false`.
+ * @example
+ *
+ * _.includes([1, 2, 3], 1);
+ * // => true
+ *
+ * _.includes([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.includes({ 'user': 'fred', 'age': 40 }, 'fred');
+ * // => true
+ *
+ * _.includes('pebbles', 'eb');
+ * // => true
+ */
+function includes(collection, target, fromIndex, guard) {
+ var length = collection ? getLength(collection) : 0;
+ if (!isLength(length)) {
+ collection = values(collection);
+ length = collection.length;
+ }
+ if (typeof fromIndex != 'number' || (guard && isIterateeCall(target, fromIndex, guard))) {
+ fromIndex = 0;
+ } else {
+ fromIndex = fromIndex < 0 ? nativeMax(length + fromIndex, 0) : (fromIndex || 0);
+ }
+ return (typeof collection == 'string' || !isArray(collection) && isString(collection))
+ ? (fromIndex <= length && collection.indexOf(target, fromIndex) > -1)
+ : (!!length && baseIndexOf(collection, target, fromIndex) > -1);
+}
+
+module.exports = includes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/indexBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/indexBy.js b/node_modules/lodash/collection/indexBy.js
new file mode 100644
index 0000000..34a941e
--- /dev/null
+++ b/node_modules/lodash/collection/indexBy.js
@@ -0,0 +1,53 @@
+var createAggregator = require('../internal/createAggregator');
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` through `iteratee`. The corresponding value
+ * of each key is the last element responsible for generating the key. The
+ * iteratee function is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * var keyData = [
+ * { 'dir': 'left', 'code': 97 },
+ * { 'dir': 'right', 'code': 100 }
+ * ];
+ *
+ * _.indexBy(keyData, 'dir');
+ * // => { 'left': { 'dir': 'left', 'code': 97 }, 'right': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(keyData, function(object) {
+ * return String.fromCharCode(object.code);
+ * });
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(keyData, function(object) {
+ * return this.fromCharCode(object.code);
+ * }, String);
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ */
+var indexBy = createAggregator(function(result, value, key) {
+ result[key] = value;
+});
+
+module.exports = indexBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/inject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/inject.js b/node_modules/lodash/collection/inject.js
new file mode 100644
index 0000000..26f53cf
--- /dev/null
+++ b/node_modules/lodash/collection/inject.js
@@ -0,0 +1 @@
+module.exports = require('./reduce');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/invoke.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/invoke.js b/node_modules/lodash/collection/invoke.js
new file mode 100644
index 0000000..6e71721
--- /dev/null
+++ b/node_modules/lodash/collection/invoke.js
@@ -0,0 +1,42 @@
+var baseEach = require('../internal/baseEach'),
+ invokePath = require('../internal/invokePath'),
+ isArrayLike = require('../internal/isArrayLike'),
+ isKey = require('../internal/isKey'),
+ restParam = require('../function/restParam');
+
+/**
+ * Invokes the method at `path` of each element in `collection`, returning
+ * an array of the results of each invoked method. Any additional arguments
+ * are provided to each invoked method. If `methodName` is a function it's
+ * invoked for, and `this` bound to, each element in `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Array|Function|string} path The path of the method to invoke or
+ * the function invoked per iteration.
+ * @param {...*} [args] The arguments to invoke the method with.
+ * @returns {Array} Returns the array of results.
+ * @example
+ *
+ * _.invoke([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invoke([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+var invoke = restParam(function(collection, path, args) {
+ var index = -1,
+ isFunc = typeof path == 'function',
+ isProp = isKey(path),
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value) {
+ var func = isFunc ? path : ((isProp && value != null) ? value[path] : undefined);
+ result[++index] = func ? func.apply(value, args) : invokePath(value, path, args);
+ });
+ return result;
+});
+
+module.exports = invoke;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/map.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/map.js b/node_modules/lodash/collection/map.js
new file mode 100644
index 0000000..5381110
--- /dev/null
+++ b/node_modules/lodash/collection/map.js
@@ -0,0 +1,68 @@
+var arrayMap = require('../internal/arrayMap'),
+ baseCallback = require('../internal/baseCallback'),
+ baseMap = require('../internal/baseMap'),
+ isArray = require('../lang/isArray');
+
+/**
+ * Creates an array of values by running each element in `collection` through
+ * `iteratee`. The `iteratee` is bound to `thisArg` and invoked with three
+ * arguments: (value, index|key, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * Many lodash methods are guarded to work as iteratees for methods like
+ * `_.every`, `_.filter`, `_.map`, `_.mapValues`, `_.reject`, and `_.some`.
+ *
+ * The guarded methods are:
+ * `ary`, `callback`, `chunk`, `clone`, `create`, `curry`, `curryRight`,
+ * `drop`, `dropRight`, `every`, `fill`, `flatten`, `invert`, `max`, `min`,
+ * `parseInt`, `slice`, `sortBy`, `take`, `takeRight`, `template`, `trim`,
+ * `trimLeft`, `trimRight`, `trunc`, `random`, `range`, `sample`, `some`,
+ * `sum`, `uniq`, and `words`
+ *
+ * @static
+ * @memberOf _
+ * @alias collect
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array} Returns the new mapped array.
+ * @example
+ *
+ * function timesThree(n) {
+ * return n * 3;
+ * }
+ *
+ * _.map([1, 2], timesThree);
+ * // => [3, 6]
+ *
+ * _.map({ 'a': 1, 'b': 2 }, timesThree);
+ * // => [3, 6] (iteration order is not guaranteed)
+ *
+ * var users = [
+ * { 'user': 'barney' },
+ * { 'user': 'fred' }
+ * ];
+ *
+ * // using the `_.property` callback shorthand
+ * _.map(users, 'user');
+ * // => ['barney', 'fred']
+ */
+function map(collection, iteratee, thisArg) {
+ var func = isArray(collection) ? arrayMap : baseMap;
+ iteratee = baseCallback(iteratee, thisArg, 3);
+ return func(collection, iteratee);
+}
+
+module.exports = map;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/max.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/max.js b/node_modules/lodash/collection/max.js
new file mode 100644
index 0000000..bb1d213
--- /dev/null
+++ b/node_modules/lodash/collection/max.js
@@ -0,0 +1 @@
+module.exports = require('../math/max');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/min.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/min.js b/node_modules/lodash/collection/min.js
new file mode 100644
index 0000000..eef13d0
--- /dev/null
+++ b/node_modules/lodash/collection/min.js
@@ -0,0 +1 @@
+module.exports = require('../math/min');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/partition.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/partition.js b/node_modules/lodash/collection/partition.js
new file mode 100644
index 0000000..ee35f27
--- /dev/null
+++ b/node_modules/lodash/collection/partition.js
@@ -0,0 +1,66 @@
+var createAggregator = require('../internal/createAggregator');
+
+/**
+ * Creates an array of elements split into two groups, the first of which
+ * contains elements `predicate` returns truthy for, while the second of which
+ * contains elements `predicate` returns falsey for. The predicate is bound
+ * to `thisArg` and invoked with three arguments: (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the array of grouped elements.
+ * @example
+ *
+ * _.partition([1, 2, 3], function(n) {
+ * return n % 2;
+ * });
+ * // => [[1, 3], [2]]
+ *
+ * _.partition([1.2, 2.3, 3.4], function(n) {
+ * return this.floor(n) % 2;
+ * }, Math);
+ * // => [[1.2, 3.4], [2.3]]
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': true },
+ * { 'user': 'pebbles', 'age': 1, 'active': false }
+ * ];
+ *
+ * var mapper = function(array) {
+ * return _.pluck(array, 'user');
+ * };
+ *
+ * // using the `_.matches` callback shorthand
+ * _.map(_.partition(users, { 'age': 1, 'active': false }), mapper);
+ * // => [['pebbles'], ['barney', 'fred']]
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.map(_.partition(users, 'active', false), mapper);
+ * // => [['barney', 'pebbles'], ['fred']]
+ *
+ * // using the `_.property` callback shorthand
+ * _.map(_.partition(users, 'active'), mapper);
+ * // => [['fred'], ['barney', 'pebbles']]
+ */
+var partition = createAggregator(function(result, value, key) {
+ result[key ? 0 : 1].push(value);
+}, function() { return [[], []]; });
+
+module.exports = partition;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/pluck.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/pluck.js b/node_modules/lodash/collection/pluck.js
new file mode 100644
index 0000000..5ee1ec8
--- /dev/null
+++ b/node_modules/lodash/collection/pluck.js
@@ -0,0 +1,31 @@
+var map = require('./map'),
+ property = require('../utility/property');
+
+/**
+ * Gets the property value of `path` from all elements in `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Array|string} path The path of the property to pluck.
+ * @returns {Array} Returns the property values.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.pluck(users, 'user');
+ * // => ['barney', 'fred']
+ *
+ * var userIndex = _.indexBy(users, 'user');
+ * _.pluck(userIndex, 'age');
+ * // => [36, 40] (iteration order is not guaranteed)
+ */
+function pluck(collection, path) {
+ return map(collection, property(path));
+}
+
+module.exports = pluck;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/reduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/reduce.js b/node_modules/lodash/collection/reduce.js
new file mode 100644
index 0000000..5d5e8c9
--- /dev/null
+++ b/node_modules/lodash/collection/reduce.js
@@ -0,0 +1,44 @@
+var arrayReduce = require('../internal/arrayReduce'),
+ baseEach = require('../internal/baseEach'),
+ createReduce = require('../internal/createReduce');
+
+/**
+ * Reduces `collection` to a value which is the accumulated result of running
+ * each element in `collection` through `iteratee`, where each successive
+ * invocation is supplied the return value of the previous. If `accumulator`
+ * is not provided the first element of `collection` is used as the initial
+ * value. The `iteratee` is bound to `thisArg` and invoked with four arguments:
+ * (accumulator, value, index|key, collection).
+ *
+ * Many lodash methods are guarded to work as iteratees for methods like
+ * `_.reduce`, `_.reduceRight`, and `_.transform`.
+ *
+ * The guarded methods are:
+ * `assign`, `defaults`, `defaultsDeep`, `includes`, `merge`, `sortByAll`,
+ * and `sortByOrder`
+ *
+ * @static
+ * @memberOf _
+ * @alias foldl, inject
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * _.reduce([1, 2], function(total, n) {
+ * return total + n;
+ * });
+ * // => 3
+ *
+ * _.reduce({ 'a': 1, 'b': 2 }, function(result, n, key) {
+ * result[key] = n * 3;
+ * return result;
+ * }, {});
+ * // => { 'a': 3, 'b': 6 } (iteration order is not guaranteed)
+ */
+var reduce = createReduce(arrayReduce, baseEach);
+
+module.exports = reduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/reduceRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/reduceRight.js b/node_modules/lodash/collection/reduceRight.js
new file mode 100644
index 0000000..5a5753b
--- /dev/null
+++ b/node_modules/lodash/collection/reduceRight.js
@@ -0,0 +1,29 @@
+var arrayReduceRight = require('../internal/arrayReduceRight'),
+ baseEachRight = require('../internal/baseEachRight'),
+ createReduce = require('../internal/createReduce');
+
+/**
+ * This method is like `_.reduce` except that it iterates over elements of
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias foldr
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var array = [[0, 1], [2, 3], [4, 5]];
+ *
+ * _.reduceRight(array, function(flattened, other) {
+ * return flattened.concat(other);
+ * }, []);
+ * // => [4, 5, 2, 3, 0, 1]
+ */
+var reduceRight = createReduce(arrayReduceRight, baseEachRight);
+
+module.exports = reduceRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/reject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/reject.js b/node_modules/lodash/collection/reject.js
new file mode 100644
index 0000000..5592453
--- /dev/null
+++ b/node_modules/lodash/collection/reject.js
@@ -0,0 +1,50 @@
+var arrayFilter = require('../internal/arrayFilter'),
+ baseCallback = require('../internal/baseCallback'),
+ baseFilter = require('../internal/baseFilter'),
+ isArray = require('../lang/isArray');
+
+/**
+ * The opposite of `_.filter`; this method returns the elements of `collection`
+ * that `predicate` does **not** return truthy for.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {Array} Returns the new filtered array.
+ * @example
+ *
+ * _.reject([1, 2, 3, 4], function(n) {
+ * return n % 2 == 0;
+ * });
+ * // => [1, 3]
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': false },
+ * { 'user': 'fred', 'age': 40, 'active': true }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.pluck(_.reject(users, { 'age': 40, 'active': true }), 'user');
+ * // => ['barney']
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.pluck(_.reject(users, 'active', false), 'user');
+ * // => ['fred']
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.reject(users, 'active'), 'user');
+ * // => ['barney']
+ */
+function reject(collection, predicate, thisArg) {
+ var func = isArray(collection) ? arrayFilter : baseFilter;
+ predicate = baseCallback(predicate, thisArg, 3);
+ return func(collection, function(value, index, collection) {
+ return !predicate(value, index, collection);
+ });
+}
+
+module.exports = reject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/sample.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/sample.js b/node_modules/lodash/collection/sample.js
new file mode 100644
index 0000000..8e01533
--- /dev/null
+++ b/node_modules/lodash/collection/sample.js
@@ -0,0 +1,50 @@
+var baseRandom = require('../internal/baseRandom'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ toArray = require('../lang/toArray'),
+ toIterable = require('../internal/toIterable');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMin = Math.min;
+
+/**
+ * Gets a random element or `n` random elements from a collection.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to sample.
+ * @param {number} [n] The number of elements to sample.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {*} Returns the random sample(s).
+ * @example
+ *
+ * _.sample([1, 2, 3, 4]);
+ * // => 2
+ *
+ * _.sample([1, 2, 3, 4], 2);
+ * // => [3, 1]
+ */
+function sample(collection, n, guard) {
+ if (guard ? isIterateeCall(collection, n, guard) : n == null) {
+ collection = toIterable(collection);
+ var length = collection.length;
+ return length > 0 ? collection[baseRandom(0, length - 1)] : undefined;
+ }
+ var index = -1,
+ result = toArray(collection),
+ length = result.length,
+ lastIndex = length - 1;
+
+ n = nativeMin(n < 0 ? 0 : (+n || 0), length);
+ while (++index < n) {
+ var rand = baseRandom(index, lastIndex),
+ value = result[rand];
+
+ result[rand] = result[index];
+ result[index] = value;
+ }
+ result.length = n;
+ return result;
+}
+
+module.exports = sample;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/select.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/select.js b/node_modules/lodash/collection/select.js
new file mode 100644
index 0000000..ade80f6
--- /dev/null
+++ b/node_modules/lodash/collection/select.js
@@ -0,0 +1 @@
+module.exports = require('./filter');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/shuffle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/shuffle.js b/node_modules/lodash/collection/shuffle.js
new file mode 100644
index 0000000..949689c
--- /dev/null
+++ b/node_modules/lodash/collection/shuffle.js
@@ -0,0 +1,24 @@
+var sample = require('./sample');
+
+/** Used as references for `-Infinity` and `Infinity`. */
+var POSITIVE_INFINITY = Number.POSITIVE_INFINITY;
+
+/**
+ * Creates an array of shuffled values, using a version of the
+ * [Fisher-Yates shuffle](https://en.wikipedia.org/wiki/Fisher-Yates_shuffle).
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to shuffle.
+ * @returns {Array} Returns the new shuffled array.
+ * @example
+ *
+ * _.shuffle([1, 2, 3, 4]);
+ * // => [4, 1, 3, 2]
+ */
+function shuffle(collection) {
+ return sample(collection, POSITIVE_INFINITY);
+}
+
+module.exports = shuffle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/size.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/size.js b/node_modules/lodash/collection/size.js
new file mode 100644
index 0000000..78dcf4c
--- /dev/null
+++ b/node_modules/lodash/collection/size.js
@@ -0,0 +1,30 @@
+var getLength = require('../internal/getLength'),
+ isLength = require('../internal/isLength'),
+ keys = require('../object/keys');
+
+/**
+ * Gets the size of `collection` by returning its length for array-like
+ * values or the number of own enumerable properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @returns {number} Returns the size of `collection`.
+ * @example
+ *
+ * _.size([1, 2, 3]);
+ * // => 3
+ *
+ * _.size({ 'a': 1, 'b': 2 });
+ * // => 2
+ *
+ * _.size('pebbles');
+ * // => 7
+ */
+function size(collection) {
+ var length = collection ? getLength(collection) : 0;
+ return isLength(length) ? length : keys(collection).length;
+}
+
+module.exports = size;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/some.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/some.js b/node_modules/lodash/collection/some.js
new file mode 100644
index 0000000..d0b09a4
--- /dev/null
+++ b/node_modules/lodash/collection/some.js
@@ -0,0 +1,67 @@
+var arraySome = require('../internal/arraySome'),
+ baseCallback = require('../internal/baseCallback'),
+ baseSome = require('../internal/baseSome'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Checks if `predicate` returns truthy for **any** element of `collection`.
+ * The function returns as soon as it finds a passing value and does not iterate
+ * over the entire collection. The predicate is bound to `thisArg` and invoked
+ * with three arguments: (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias any
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': true },
+ * { 'user': 'fred', 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.some(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.some(users, 'active', false);
+ * // => true
+ *
+ * // using the `_.property` callback shorthand
+ * _.some(users, 'active');
+ * // => true
+ */
+function some(collection, predicate, thisArg) {
+ var func = isArray(collection) ? arraySome : baseSome;
+ if (thisArg && isIterateeCall(collection, predicate, thisArg)) {
+ predicate = undefined;
+ }
+ if (typeof predicate != 'function' || thisArg !== undefined) {
+ predicate = baseCallback(predicate, thisArg, 3);
+ }
+ return func(collection, predicate);
+}
+
+module.exports = some;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/sortBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/sortBy.js b/node_modules/lodash/collection/sortBy.js
new file mode 100644
index 0000000..4401c77
--- /dev/null
+++ b/node_modules/lodash/collection/sortBy.js
@@ -0,0 +1,71 @@
+var baseCallback = require('../internal/baseCallback'),
+ baseMap = require('../internal/baseMap'),
+ baseSortBy = require('../internal/baseSortBy'),
+ compareAscending = require('../internal/compareAscending'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in a collection through `iteratee`. This method performs
+ * a stable sort, that is, it preserves the original sort order of equal elements.
+ * The `iteratee` is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `iteratee` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `iteratee` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [iteratee=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array} Returns the new sorted array.
+ * @example
+ *
+ * _.sortBy([1, 2, 3], function(n) {
+ * return Math.sin(n);
+ * });
+ * // => [3, 1, 2]
+ *
+ * _.sortBy([1, 2, 3], function(n) {
+ * return this.sin(n);
+ * }, Math);
+ * // => [3, 1, 2]
+ *
+ * var users = [
+ * { 'user': 'fred' },
+ * { 'user': 'pebbles' },
+ * { 'user': 'barney' }
+ * ];
+ *
+ * // using the `_.property` callback shorthand
+ * _.pluck(_.sortBy(users, 'user'), 'user');
+ * // => ['barney', 'fred', 'pebbles']
+ */
+function sortBy(collection, iteratee, thisArg) {
+ if (collection == null) {
+ return [];
+ }
+ if (thisArg && isIterateeCall(collection, iteratee, thisArg)) {
+ iteratee = undefined;
+ }
+ var index = -1;
+ iteratee = baseCallback(iteratee, thisArg, 3);
+
+ var result = baseMap(collection, function(value, key, collection) {
+ return { 'criteria': iteratee(value, key, collection), 'index': ++index, 'value': value };
+ });
+ return baseSortBy(result, compareAscending);
+}
+
+module.exports = sortBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/collection/sortByAll.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/collection/sortByAll.js b/node_modules/lodash/collection/sortByAll.js
new file mode 100644
index 0000000..4766c20
--- /dev/null
+++ b/node_modules/lodash/collection/sortByAll.js
@@ -0,0 +1,52 @@
+var baseFlatten = require('../internal/baseFlatten'),
+ baseSortByOrder = require('../internal/baseSortByOrder'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ restParam = require('../function/restParam');
+
+/**
+ * This method is like `_.sortBy` except that it can sort by multiple iteratees
+ * or property names.
+ *
+ * If a property name is provided for an iteratee the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If an object is provided for an iteratee the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {...(Function|Function[]|Object|Object[]|string|string[])} iteratees
+ * The iteratees to sort by, specified as individual values or arrays of values.
+ * @returns {Array} Returns the new sorted array.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'fred', 'age': 48 },
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 42 },
+ * { 'user': 'barney', 'age': 34 }
+ * ];
+ *
+ * _.map(_.sortByAll(users, ['user', 'age']), _.values);
+ * // => [['barney', 34], ['barney', 36], ['fred', 42], ['fred', 48]]
+ *
+ * _.map(_.sortByAll(users, 'user', function(chr) {
+ * return Math.floor(chr.age / 10);
+ * }), _.values);
+ * // => [['barney', 36], ['barney', 34], ['fred', 48], ['fred', 42]]
+ */
+var sortByAll = restParam(function(collection, iteratees) {
+ if (collection == null) {
+ return [];
+ }
+ var guard = iteratees[2];
+ if (guard && isIterateeCall(iteratees[0], iteratees[1], guard)) {
+ iteratees.length = 1;
+ }
+ return baseSortByOrder(collection, baseFlatten(iteratees), []);
+});
+
+module.exports = sortByAll;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[11/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/q/q.js
----------------------------------------------------------------------
diff --git a/node_modules/q/q.js b/node_modules/q/q.js
new file mode 100644
index 0000000..cf5339e
--- /dev/null
+++ b/node_modules/q/q.js
@@ -0,0 +1,2048 @@
+// vim:ts=4:sts=4:sw=4:
+/*!
+ *
+ * Copyright 2009-2012 Kris Kowal under the terms of the MIT
+ * license found at http://github.com/kriskowal/q/raw/master/LICENSE
+ *
+ * With parts by Tyler Close
+ * Copyright 2007-2009 Tyler Close under the terms of the MIT X license found
+ * at http://www.opensource.org/licenses/mit-license.html
+ * Forked at ref_send.js version: 2009-05-11
+ *
+ * With parts by Mark Miller
+ * Copyright (C) 2011 Google Inc.
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing, software
+ * distributed under the License is distributed on an "AS IS" BASIS,
+ * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ * See the License for the specific language governing permissions and
+ * limitations under the License.
+ *
+ */
+
+(function (definition) {
+ "use strict";
+
+ // This file will function properly as a <script> tag, or a module
+ // using CommonJS and NodeJS or RequireJS module formats. In
+ // Common/Node/RequireJS, the module exports the Q API and when
+ // executed as a simple <script>, it creates a Q global instead.
+
+ // Montage Require
+ if (typeof bootstrap === "function") {
+ bootstrap("promise", definition);
+
+ // CommonJS
+ } else if (typeof exports === "object" && typeof module === "object") {
+ module.exports = definition();
+
+ // RequireJS
+ } else if (typeof define === "function" && define.amd) {
+ define(definition);
+
+ // SES (Secure EcmaScript)
+ } else if (typeof ses !== "undefined") {
+ if (!ses.ok()) {
+ return;
+ } else {
+ ses.makeQ = definition;
+ }
+
+ // <script>
+ } else if (typeof window !== "undefined" || typeof self !== "undefined") {
+ // Prefer window over self for add-on scripts. Use self for
+ // non-windowed contexts.
+ var global = typeof window !== "undefined" ? window : self;
+
+ // Get the `window` object, save the previous Q global
+ // and initialize Q as a global.
+ var previousQ = global.Q;
+ global.Q = definition();
+
+ // Add a noConflict function so Q can be removed from the
+ // global namespace.
+ global.Q.noConflict = function () {
+ global.Q = previousQ;
+ return this;
+ };
+
+ } else {
+ throw new Error("This environment was not anticipated by Q. Please file a bug.");
+ }
+
+})(function () {
+"use strict";
+
+var hasStacks = false;
+try {
+ throw new Error();
+} catch (e) {
+ hasStacks = !!e.stack;
+}
+
+// All code after this point will be filtered from stack traces reported
+// by Q.
+var qStartingLine = captureLine();
+var qFileName;
+
+// shims
+
+// used for fallback in "allResolved"
+var noop = function () {};
+
+// Use the fastest possible means to execute a task in a future turn
+// of the event loop.
+var nextTick =(function () {
+ // linked list of tasks (single, with head node)
+ var head = {task: void 0, next: null};
+ var tail = head;
+ var flushing = false;
+ var requestTick = void 0;
+ var isNodeJS = false;
+ // queue for late tasks, used by unhandled rejection tracking
+ var laterQueue = [];
+
+ function flush() {
+ /* jshint loopfunc: true */
+ var task, domain;
+
+ while (head.next) {
+ head = head.next;
+ task = head.task;
+ head.task = void 0;
+ domain = head.domain;
+
+ if (domain) {
+ head.domain = void 0;
+ domain.enter();
+ }
+ runSingle(task, domain);
+
+ }
+ while (laterQueue.length) {
+ task = laterQueue.pop();
+ runSingle(task);
+ }
+ flushing = false;
+ }
+ // runs a single function in the async queue
+ function runSingle(task, domain) {
+ try {
+ task();
+
+ } catch (e) {
+ if (isNodeJS) {
+ // In node, uncaught exceptions are considered fatal errors.
+ // Re-throw them synchronously to interrupt flushing!
+
+ // Ensure continuation if the uncaught exception is suppressed
+ // listening "uncaughtException" events (as domains does).
+ // Continue in next event to avoid tick recursion.
+ if (domain) {
+ domain.exit();
+ }
+ setTimeout(flush, 0);
+ if (domain) {
+ domain.enter();
+ }
+
+ throw e;
+
+ } else {
+ // In browsers, uncaught exceptions are not fatal.
+ // Re-throw them asynchronously to avoid slow-downs.
+ setTimeout(function () {
+ throw e;
+ }, 0);
+ }
+ }
+
+ if (domain) {
+ domain.exit();
+ }
+ }
+
+ nextTick = function (task) {
+ tail = tail.next = {
+ task: task,
+ domain: isNodeJS && process.domain,
+ next: null
+ };
+
+ if (!flushing) {
+ flushing = true;
+ requestTick();
+ }
+ };
+
+ if (typeof process === "object" &&
+ process.toString() === "[object process]" && process.nextTick) {
+ // Ensure Q is in a real Node environment, with a `process.nextTick`.
+ // To see through fake Node environments:
+ // * Mocha test runner - exposes a `process` global without a `nextTick`
+ // * Browserify - exposes a `process.nexTick` function that uses
+ // `setTimeout`. In this case `setImmediate` is preferred because
+ // it is faster. Browserify's `process.toString()` yields
+ // "[object Object]", while in a real Node environment
+ // `process.nextTick()` yields "[object process]".
+ isNodeJS = true;
+
+ requestTick = function () {
+ process.nextTick(flush);
+ };
+
+ } else if (typeof setImmediate === "function") {
+ // In IE10, Node.js 0.9+, or https://github.com/NobleJS/setImmediate
+ if (typeof window !== "undefined") {
+ requestTick = setImmediate.bind(window, flush);
+ } else {
+ requestTick = function () {
+ setImmediate(flush);
+ };
+ }
+
+ } else if (typeof MessageChannel !== "undefined") {
+ // modern browsers
+ // http://www.nonblocking.io/2011/06/windownexttick.html
+ var channel = new MessageChannel();
+ // At least Safari Version 6.0.5 (8536.30.1) intermittently cannot create
+ // working message ports the first time a page loads.
+ channel.port1.onmessage = function () {
+ requestTick = requestPortTick;
+ channel.port1.onmessage = flush;
+ flush();
+ };
+ var requestPortTick = function () {
+ // Opera requires us to provide a message payload, regardless of
+ // whether we use it.
+ channel.port2.postMessage(0);
+ };
+ requestTick = function () {
+ setTimeout(flush, 0);
+ requestPortTick();
+ };
+
+ } else {
+ // old browsers
+ requestTick = function () {
+ setTimeout(flush, 0);
+ };
+ }
+ // runs a task after all other tasks have been run
+ // this is useful for unhandled rejection tracking that needs to happen
+ // after all `then`d tasks have been run.
+ nextTick.runAfter = function (task) {
+ laterQueue.push(task);
+ if (!flushing) {
+ flushing = true;
+ requestTick();
+ }
+ };
+ return nextTick;
+})();
+
+// Attempt to make generics safe in the face of downstream
+// modifications.
+// There is no situation where this is necessary.
+// If you need a security guarantee, these primordials need to be
+// deeply frozen anyway, and if you don’t need a security guarantee,
+// this is just plain paranoid.
+// However, this **might** have the nice side-effect of reducing the size of
+// the minified code by reducing x.call() to merely x()
+// See Mark Miller’s explanation of what this does.
+// http://wiki.ecmascript.org/doku.php?id=conventions:safe_meta_programming
+var call = Function.call;
+function uncurryThis(f) {
+ return function () {
+ return call.apply(f, arguments);
+ };
+}
+// This is equivalent, but slower:
+// uncurryThis = Function_bind.bind(Function_bind.call);
+// http://jsperf.com/uncurrythis
+
+var array_slice = uncurryThis(Array.prototype.slice);
+
+var array_reduce = uncurryThis(
+ Array.prototype.reduce || function (callback, basis) {
+ var index = 0,
+ length = this.length;
+ // concerning the initial value, if one is not provided
+ if (arguments.length === 1) {
+ // seek to the first value in the array, accounting
+ // for the possibility that is is a sparse array
+ do {
+ if (index in this) {
+ basis = this[index++];
+ break;
+ }
+ if (++index >= length) {
+ throw new TypeError();
+ }
+ } while (1);
+ }
+ // reduce
+ for (; index < length; index++) {
+ // account for the possibility that the array is sparse
+ if (index in this) {
+ basis = callback(basis, this[index], index);
+ }
+ }
+ return basis;
+ }
+);
+
+var array_indexOf = uncurryThis(
+ Array.prototype.indexOf || function (value) {
+ // not a very good shim, but good enough for our one use of it
+ for (var i = 0; i < this.length; i++) {
+ if (this[i] === value) {
+ return i;
+ }
+ }
+ return -1;
+ }
+);
+
+var array_map = uncurryThis(
+ Array.prototype.map || function (callback, thisp) {
+ var self = this;
+ var collect = [];
+ array_reduce(self, function (undefined, value, index) {
+ collect.push(callback.call(thisp, value, index, self));
+ }, void 0);
+ return collect;
+ }
+);
+
+var object_create = Object.create || function (prototype) {
+ function Type() { }
+ Type.prototype = prototype;
+ return new Type();
+};
+
+var object_hasOwnProperty = uncurryThis(Object.prototype.hasOwnProperty);
+
+var object_keys = Object.keys || function (object) {
+ var keys = [];
+ for (var key in object) {
+ if (object_hasOwnProperty(object, key)) {
+ keys.push(key);
+ }
+ }
+ return keys;
+};
+
+var object_toString = uncurryThis(Object.prototype.toString);
+
+function isObject(value) {
+ return value === Object(value);
+}
+
+// generator related shims
+
+// FIXME: Remove this function once ES6 generators are in SpiderMonkey.
+function isStopIteration(exception) {
+ return (
+ object_toString(exception) === "[object StopIteration]" ||
+ exception instanceof QReturnValue
+ );
+}
+
+// FIXME: Remove this helper and Q.return once ES6 generators are in
+// SpiderMonkey.
+var QReturnValue;
+if (typeof ReturnValue !== "undefined") {
+ QReturnValue = ReturnValue;
+} else {
+ QReturnValue = function (value) {
+ this.value = value;
+ };
+}
+
+// long stack traces
+
+var STACK_JUMP_SEPARATOR = "From previous event:";
+
+function makeStackTraceLong(error, promise) {
+ // If possible, transform the error stack trace by removing Node and Q
+ // cruft, then concatenating with the stack trace of `promise`. See #57.
+ if (hasStacks &&
+ promise.stack &&
+ typeof error === "object" &&
+ error !== null &&
+ error.stack &&
+ error.stack.indexOf(STACK_JUMP_SEPARATOR) === -1
+ ) {
+ var stacks = [];
+ for (var p = promise; !!p; p = p.source) {
+ if (p.stack) {
+ stacks.unshift(p.stack);
+ }
+ }
+ stacks.unshift(error.stack);
+
+ var concatedStacks = stacks.join("\n" + STACK_JUMP_SEPARATOR + "\n");
+ error.stack = filterStackString(concatedStacks);
+ }
+}
+
+function filterStackString(stackString) {
+ var lines = stackString.split("\n");
+ var desiredLines = [];
+ for (var i = 0; i < lines.length; ++i) {
+ var line = lines[i];
+
+ if (!isInternalFrame(line) && !isNodeFrame(line) && line) {
+ desiredLines.push(line);
+ }
+ }
+ return desiredLines.join("\n");
+}
+
+function isNodeFrame(stackLine) {
+ return stackLine.indexOf("(module.js:") !== -1 ||
+ stackLine.indexOf("(node.js:") !== -1;
+}
+
+function getFileNameAndLineNumber(stackLine) {
+ // Named functions: "at functionName (filename:lineNumber:columnNumber)"
+ // In IE10 function name can have spaces ("Anonymous function") O_o
+ var attempt1 = /at .+ \((.+):(\d+):(?:\d+)\)$/.exec(stackLine);
+ if (attempt1) {
+ return [attempt1[1], Number(attempt1[2])];
+ }
+
+ // Anonymous functions: "at filename:lineNumber:columnNumber"
+ var attempt2 = /at ([^ ]+):(\d+):(?:\d+)$/.exec(stackLine);
+ if (attempt2) {
+ return [attempt2[1], Number(attempt2[2])];
+ }
+
+ // Firefox style: "function@filename:lineNumber or @filename:lineNumber"
+ var attempt3 = /.*@(.+):(\d+)$/.exec(stackLine);
+ if (attempt3) {
+ return [attempt3[1], Number(attempt3[2])];
+ }
+}
+
+function isInternalFrame(stackLine) {
+ var fileNameAndLineNumber = getFileNameAndLineNumber(stackLine);
+
+ if (!fileNameAndLineNumber) {
+ return false;
+ }
+
+ var fileName = fileNameAndLineNumber[0];
+ var lineNumber = fileNameAndLineNumber[1];
+
+ return fileName === qFileName &&
+ lineNumber >= qStartingLine &&
+ lineNumber <= qEndingLine;
+}
+
+// discover own file name and line number range for filtering stack
+// traces
+function captureLine() {
+ if (!hasStacks) {
+ return;
+ }
+
+ try {
+ throw new Error();
+ } catch (e) {
+ var lines = e.stack.split("\n");
+ var firstLine = lines[0].indexOf("@") > 0 ? lines[1] : lines[2];
+ var fileNameAndLineNumber = getFileNameAndLineNumber(firstLine);
+ if (!fileNameAndLineNumber) {
+ return;
+ }
+
+ qFileName = fileNameAndLineNumber[0];
+ return fileNameAndLineNumber[1];
+ }
+}
+
+function deprecate(callback, name, alternative) {
+ return function () {
+ if (typeof console !== "undefined" &&
+ typeof console.warn === "function") {
+ console.warn(name + " is deprecated, use " + alternative +
+ " instead.", new Error("").stack);
+ }
+ return callback.apply(callback, arguments);
+ };
+}
+
+// end of shims
+// beginning of real work
+
+/**
+ * Constructs a promise for an immediate reference, passes promises through, or
+ * coerces promises from different systems.
+ * @param value immediate reference or promise
+ */
+function Q(value) {
+ // If the object is already a Promise, return it directly. This enables
+ // the resolve function to both be used to created references from objects,
+ // but to tolerably coerce non-promises to promises.
+ if (value instanceof Promise) {
+ return value;
+ }
+
+ // assimilate thenables
+ if (isPromiseAlike(value)) {
+ return coerce(value);
+ } else {
+ return fulfill(value);
+ }
+}
+Q.resolve = Q;
+
+/**
+ * Performs a task in a future turn of the event loop.
+ * @param {Function} task
+ */
+Q.nextTick = nextTick;
+
+/**
+ * Controls whether or not long stack traces will be on
+ */
+Q.longStackSupport = false;
+
+// enable long stacks if Q_DEBUG is set
+if (typeof process === "object" && process && process.env && process.env.Q_DEBUG) {
+ Q.longStackSupport = true;
+}
+
+/**
+ * Constructs a {promise, resolve, reject} object.
+ *
+ * `resolve` is a callback to invoke with a more resolved value for the
+ * promise. To fulfill the promise, invoke `resolve` with any value that is
+ * not a thenable. To reject the promise, invoke `resolve` with a rejected
+ * thenable, or invoke `reject` with the reason directly. To resolve the
+ * promise to another thenable, thus putting it in the same state, invoke
+ * `resolve` with that other thenable.
+ */
+Q.defer = defer;
+function defer() {
+ // if "messages" is an "Array", that indicates that the promise has not yet
+ // been resolved. If it is "undefined", it has been resolved. Each
+ // element of the messages array is itself an array of complete arguments to
+ // forward to the resolved promise. We coerce the resolution value to a
+ // promise using the `resolve` function because it handles both fully
+ // non-thenable values and other thenables gracefully.
+ var messages = [], progressListeners = [], resolvedPromise;
+
+ var deferred = object_create(defer.prototype);
+ var promise = object_create(Promise.prototype);
+
+ promise.promiseDispatch = function (resolve, op, operands) {
+ var args = array_slice(arguments);
+ if (messages) {
+ messages.push(args);
+ if (op === "when" && operands[1]) { // progress operand
+ progressListeners.push(operands[1]);
+ }
+ } else {
+ Q.nextTick(function () {
+ resolvedPromise.promiseDispatch.apply(resolvedPromise, args);
+ });
+ }
+ };
+
+ // XXX deprecated
+ promise.valueOf = function () {
+ if (messages) {
+ return promise;
+ }
+ var nearerValue = nearer(resolvedPromise);
+ if (isPromise(nearerValue)) {
+ resolvedPromise = nearerValue; // shorten chain
+ }
+ return nearerValue;
+ };
+
+ promise.inspect = function () {
+ if (!resolvedPromise) {
+ return { state: "pending" };
+ }
+ return resolvedPromise.inspect();
+ };
+
+ if (Q.longStackSupport && hasStacks) {
+ try {
+ throw new Error();
+ } catch (e) {
+ // NOTE: don't try to use `Error.captureStackTrace` or transfer the
+ // accessor around; that causes memory leaks as per GH-111. Just
+ // reify the stack trace as a string ASAP.
+ //
+ // At the same time, cut off the first line; it's always just
+ // "[object Promise]\n", as per the `toString`.
+ promise.stack = e.stack.substring(e.stack.indexOf("\n") + 1);
+ }
+ }
+
+ // NOTE: we do the checks for `resolvedPromise` in each method, instead of
+ // consolidating them into `become`, since otherwise we'd create new
+ // promises with the lines `become(whatever(value))`. See e.g. GH-252.
+
+ function become(newPromise) {
+ resolvedPromise = newPromise;
+ promise.source = newPromise;
+
+ array_reduce(messages, function (undefined, message) {
+ Q.nextTick(function () {
+ newPromise.promiseDispatch.apply(newPromise, message);
+ });
+ }, void 0);
+
+ messages = void 0;
+ progressListeners = void 0;
+ }
+
+ deferred.promise = promise;
+ deferred.resolve = function (value) {
+ if (resolvedPromise) {
+ return;
+ }
+
+ become(Q(value));
+ };
+
+ deferred.fulfill = function (value) {
+ if (resolvedPromise) {
+ return;
+ }
+
+ become(fulfill(value));
+ };
+ deferred.reject = function (reason) {
+ if (resolvedPromise) {
+ return;
+ }
+
+ become(reject(reason));
+ };
+ deferred.notify = function (progress) {
+ if (resolvedPromise) {
+ return;
+ }
+
+ array_reduce(progressListeners, function (undefined, progressListener) {
+ Q.nextTick(function () {
+ progressListener(progress);
+ });
+ }, void 0);
+ };
+
+ return deferred;
+}
+
+/**
+ * Creates a Node-style callback that will resolve or reject the deferred
+ * promise.
+ * @returns a nodeback
+ */
+defer.prototype.makeNodeResolver = function () {
+ var self = this;
+ return function (error, value) {
+ if (error) {
+ self.reject(error);
+ } else if (arguments.length > 2) {
+ self.resolve(array_slice(arguments, 1));
+ } else {
+ self.resolve(value);
+ }
+ };
+};
+
+/**
+ * @param resolver {Function} a function that returns nothing and accepts
+ * the resolve, reject, and notify functions for a deferred.
+ * @returns a promise that may be resolved with the given resolve and reject
+ * functions, or rejected by a thrown exception in resolver
+ */
+Q.Promise = promise; // ES6
+Q.promise = promise;
+function promise(resolver) {
+ if (typeof resolver !== "function") {
+ throw new TypeError("resolver must be a function.");
+ }
+ var deferred = defer();
+ try {
+ resolver(deferred.resolve, deferred.reject, deferred.notify);
+ } catch (reason) {
+ deferred.reject(reason);
+ }
+ return deferred.promise;
+}
+
+promise.race = race; // ES6
+promise.all = all; // ES6
+promise.reject = reject; // ES6
+promise.resolve = Q; // ES6
+
+// XXX experimental. This method is a way to denote that a local value is
+// serializable and should be immediately dispatched to a remote upon request,
+// instead of passing a reference.
+Q.passByCopy = function (object) {
+ //freeze(object);
+ //passByCopies.set(object, true);
+ return object;
+};
+
+Promise.prototype.passByCopy = function () {
+ //freeze(object);
+ //passByCopies.set(object, true);
+ return this;
+};
+
+/**
+ * If two promises eventually fulfill to the same value, promises that value,
+ * but otherwise rejects.
+ * @param x {Any*}
+ * @param y {Any*}
+ * @returns {Any*} a promise for x and y if they are the same, but a rejection
+ * otherwise.
+ *
+ */
+Q.join = function (x, y) {
+ return Q(x).join(y);
+};
+
+Promise.prototype.join = function (that) {
+ return Q([this, that]).spread(function (x, y) {
+ if (x === y) {
+ // TODO: "===" should be Object.is or equiv
+ return x;
+ } else {
+ throw new Error("Can't join: not the same: " + x + " " + y);
+ }
+ });
+};
+
+/**
+ * Returns a promise for the first of an array of promises to become settled.
+ * @param answers {Array[Any*]} promises to race
+ * @returns {Any*} the first promise to be settled
+ */
+Q.race = race;
+function race(answerPs) {
+ return promise(function (resolve, reject) {
+ // Switch to this once we can assume at least ES5
+ // answerPs.forEach(function (answerP) {
+ // Q(answerP).then(resolve, reject);
+ // });
+ // Use this in the meantime
+ for (var i = 0, len = answerPs.length; i < len; i++) {
+ Q(answerPs[i]).then(resolve, reject);
+ }
+ });
+}
+
+Promise.prototype.race = function () {
+ return this.then(Q.race);
+};
+
+/**
+ * Constructs a Promise with a promise descriptor object and optional fallback
+ * function. The descriptor contains methods like when(rejected), get(name),
+ * set(name, value), post(name, args), and delete(name), which all
+ * return either a value, a promise for a value, or a rejection. The fallback
+ * accepts the operation name, a resolver, and any further arguments that would
+ * have been forwarded to the appropriate method above had a method been
+ * provided with the proper name. The API makes no guarantees about the nature
+ * of the returned object, apart from that it is usable whereever promises are
+ * bought and sold.
+ */
+Q.makePromise = Promise;
+function Promise(descriptor, fallback, inspect) {
+ if (fallback === void 0) {
+ fallback = function (op) {
+ return reject(new Error(
+ "Promise does not support operation: " + op
+ ));
+ };
+ }
+ if (inspect === void 0) {
+ inspect = function () {
+ return {state: "unknown"};
+ };
+ }
+
+ var promise = object_create(Promise.prototype);
+
+ promise.promiseDispatch = function (resolve, op, args) {
+ var result;
+ try {
+ if (descriptor[op]) {
+ result = descriptor[op].apply(promise, args);
+ } else {
+ result = fallback.call(promise, op, args);
+ }
+ } catch (exception) {
+ result = reject(exception);
+ }
+ if (resolve) {
+ resolve(result);
+ }
+ };
+
+ promise.inspect = inspect;
+
+ // XXX deprecated `valueOf` and `exception` support
+ if (inspect) {
+ var inspected = inspect();
+ if (inspected.state === "rejected") {
+ promise.exception = inspected.reason;
+ }
+
+ promise.valueOf = function () {
+ var inspected = inspect();
+ if (inspected.state === "pending" ||
+ inspected.state === "rejected") {
+ return promise;
+ }
+ return inspected.value;
+ };
+ }
+
+ return promise;
+}
+
+Promise.prototype.toString = function () {
+ return "[object Promise]";
+};
+
+Promise.prototype.then = function (fulfilled, rejected, progressed) {
+ var self = this;
+ var deferred = defer();
+ var done = false; // ensure the untrusted promise makes at most a
+ // single call to one of the callbacks
+
+ function _fulfilled(value) {
+ try {
+ return typeof fulfilled === "function" ? fulfilled(value) : value;
+ } catch (exception) {
+ return reject(exception);
+ }
+ }
+
+ function _rejected(exception) {
+ if (typeof rejected === "function") {
+ makeStackTraceLong(exception, self);
+ try {
+ return rejected(exception);
+ } catch (newException) {
+ return reject(newException);
+ }
+ }
+ return reject(exception);
+ }
+
+ function _progressed(value) {
+ return typeof progressed === "function" ? progressed(value) : value;
+ }
+
+ Q.nextTick(function () {
+ self.promiseDispatch(function (value) {
+ if (done) {
+ return;
+ }
+ done = true;
+
+ deferred.resolve(_fulfilled(value));
+ }, "when", [function (exception) {
+ if (done) {
+ return;
+ }
+ done = true;
+
+ deferred.resolve(_rejected(exception));
+ }]);
+ });
+
+ // Progress propagator need to be attached in the current tick.
+ self.promiseDispatch(void 0, "when", [void 0, function (value) {
+ var newValue;
+ var threw = false;
+ try {
+ newValue = _progressed(value);
+ } catch (e) {
+ threw = true;
+ if (Q.onerror) {
+ Q.onerror(e);
+ } else {
+ throw e;
+ }
+ }
+
+ if (!threw) {
+ deferred.notify(newValue);
+ }
+ }]);
+
+ return deferred.promise;
+};
+
+Q.tap = function (promise, callback) {
+ return Q(promise).tap(callback);
+};
+
+/**
+ * Works almost like "finally", but not called for rejections.
+ * Original resolution value is passed through callback unaffected.
+ * Callback may return a promise that will be awaited for.
+ * @param {Function} callback
+ * @returns {Q.Promise}
+ * @example
+ * doSomething()
+ * .then(...)
+ * .tap(console.log)
+ * .then(...);
+ */
+Promise.prototype.tap = function (callback) {
+ callback = Q(callback);
+
+ return this.then(function (value) {
+ return callback.fcall(value).thenResolve(value);
+ });
+};
+
+/**
+ * Registers an observer on a promise.
+ *
+ * Guarantees:
+ *
+ * 1. that fulfilled and rejected will be called only once.
+ * 2. that either the fulfilled callback or the rejected callback will be
+ * called, but not both.
+ * 3. that fulfilled and rejected will not be called in this turn.
+ *
+ * @param value promise or immediate reference to observe
+ * @param fulfilled function to be called with the fulfilled value
+ * @param rejected function to be called with the rejection exception
+ * @param progressed function to be called on any progress notifications
+ * @return promise for the return value from the invoked callback
+ */
+Q.when = when;
+function when(value, fulfilled, rejected, progressed) {
+ return Q(value).then(fulfilled, rejected, progressed);
+}
+
+Promise.prototype.thenResolve = function (value) {
+ return this.then(function () { return value; });
+};
+
+Q.thenResolve = function (promise, value) {
+ return Q(promise).thenResolve(value);
+};
+
+Promise.prototype.thenReject = function (reason) {
+ return this.then(function () { throw reason; });
+};
+
+Q.thenReject = function (promise, reason) {
+ return Q(promise).thenReject(reason);
+};
+
+/**
+ * If an object is not a promise, it is as "near" as possible.
+ * If a promise is rejected, it is as "near" as possible too.
+ * If it’s a fulfilled promise, the fulfillment value is nearer.
+ * If it’s a deferred promise and the deferred has been resolved, the
+ * resolution is "nearer".
+ * @param object
+ * @returns most resolved (nearest) form of the object
+ */
+
+// XXX should we re-do this?
+Q.nearer = nearer;
+function nearer(value) {
+ if (isPromise(value)) {
+ var inspected = value.inspect();
+ if (inspected.state === "fulfilled") {
+ return inspected.value;
+ }
+ }
+ return value;
+}
+
+/**
+ * @returns whether the given object is a promise.
+ * Otherwise it is a fulfilled value.
+ */
+Q.isPromise = isPromise;
+function isPromise(object) {
+ return object instanceof Promise;
+}
+
+Q.isPromiseAlike = isPromiseAlike;
+function isPromiseAlike(object) {
+ return isObject(object) && typeof object.then === "function";
+}
+
+/**
+ * @returns whether the given object is a pending promise, meaning not
+ * fulfilled or rejected.
+ */
+Q.isPending = isPending;
+function isPending(object) {
+ return isPromise(object) && object.inspect().state === "pending";
+}
+
+Promise.prototype.isPending = function () {
+ return this.inspect().state === "pending";
+};
+
+/**
+ * @returns whether the given object is a value or fulfilled
+ * promise.
+ */
+Q.isFulfilled = isFulfilled;
+function isFulfilled(object) {
+ return !isPromise(object) || object.inspect().state === "fulfilled";
+}
+
+Promise.prototype.isFulfilled = function () {
+ return this.inspect().state === "fulfilled";
+};
+
+/**
+ * @returns whether the given object is a rejected promise.
+ */
+Q.isRejected = isRejected;
+function isRejected(object) {
+ return isPromise(object) && object.inspect().state === "rejected";
+}
+
+Promise.prototype.isRejected = function () {
+ return this.inspect().state === "rejected";
+};
+
+//// BEGIN UNHANDLED REJECTION TRACKING
+
+// This promise library consumes exceptions thrown in handlers so they can be
+// handled by a subsequent promise. The exceptions get added to this array when
+// they are created, and removed when they are handled. Note that in ES6 or
+// shimmed environments, this would naturally be a `Set`.
+var unhandledReasons = [];
+var unhandledRejections = [];
+var reportedUnhandledRejections = [];
+var trackUnhandledRejections = true;
+
+function resetUnhandledRejections() {
+ unhandledReasons.length = 0;
+ unhandledRejections.length = 0;
+
+ if (!trackUnhandledRejections) {
+ trackUnhandledRejections = true;
+ }
+}
+
+function trackRejection(promise, reason) {
+ if (!trackUnhandledRejections) {
+ return;
+ }
+ if (typeof process === "object" && typeof process.emit === "function") {
+ Q.nextTick.runAfter(function () {
+ if (array_indexOf(unhandledRejections, promise) !== -1) {
+ process.emit("unhandledRejection", reason, promise);
+ reportedUnhandledRejections.push(promise);
+ }
+ });
+ }
+
+ unhandledRejections.push(promise);
+ if (reason && typeof reason.stack !== "undefined") {
+ unhandledReasons.push(reason.stack);
+ } else {
+ unhandledReasons.push("(no stack) " + reason);
+ }
+}
+
+function untrackRejection(promise) {
+ if (!trackUnhandledRejections) {
+ return;
+ }
+
+ var at = array_indexOf(unhandledRejections, promise);
+ if (at !== -1) {
+ if (typeof process === "object" && typeof process.emit === "function") {
+ Q.nextTick.runAfter(function () {
+ var atReport = array_indexOf(reportedUnhandledRejections, promise);
+ if (atReport !== -1) {
+ process.emit("rejectionHandled", unhandledReasons[at], promise);
+ reportedUnhandledRejections.splice(atReport, 1);
+ }
+ });
+ }
+ unhandledRejections.splice(at, 1);
+ unhandledReasons.splice(at, 1);
+ }
+}
+
+Q.resetUnhandledRejections = resetUnhandledRejections;
+
+Q.getUnhandledReasons = function () {
+ // Make a copy so that consumers can't interfere with our internal state.
+ return unhandledReasons.slice();
+};
+
+Q.stopUnhandledRejectionTracking = function () {
+ resetUnhandledRejections();
+ trackUnhandledRejections = false;
+};
+
+resetUnhandledRejections();
+
+//// END UNHANDLED REJECTION TRACKING
+
+/**
+ * Constructs a rejected promise.
+ * @param reason value describing the failure
+ */
+Q.reject = reject;
+function reject(reason) {
+ var rejection = Promise({
+ "when": function (rejected) {
+ // note that the error has been handled
+ if (rejected) {
+ untrackRejection(this);
+ }
+ return rejected ? rejected(reason) : this;
+ }
+ }, function fallback() {
+ return this;
+ }, function inspect() {
+ return { state: "rejected", reason: reason };
+ });
+
+ // Note that the reason has not been handled.
+ trackRejection(rejection, reason);
+
+ return rejection;
+}
+
+/**
+ * Constructs a fulfilled promise for an immediate reference.
+ * @param value immediate reference
+ */
+Q.fulfill = fulfill;
+function fulfill(value) {
+ return Promise({
+ "when": function () {
+ return value;
+ },
+ "get": function (name) {
+ return value[name];
+ },
+ "set": function (name, rhs) {
+ value[name] = rhs;
+ },
+ "delete": function (name) {
+ delete value[name];
+ },
+ "post": function (name, args) {
+ // Mark Miller proposes that post with no name should apply a
+ // promised function.
+ if (name === null || name === void 0) {
+ return value.apply(void 0, args);
+ } else {
+ return value[name].apply(value, args);
+ }
+ },
+ "apply": function (thisp, args) {
+ return value.apply(thisp, args);
+ },
+ "keys": function () {
+ return object_keys(value);
+ }
+ }, void 0, function inspect() {
+ return { state: "fulfilled", value: value };
+ });
+}
+
+/**
+ * Converts thenables to Q promises.
+ * @param promise thenable promise
+ * @returns a Q promise
+ */
+function coerce(promise) {
+ var deferred = defer();
+ Q.nextTick(function () {
+ try {
+ promise.then(deferred.resolve, deferred.reject, deferred.notify);
+ } catch (exception) {
+ deferred.reject(exception);
+ }
+ });
+ return deferred.promise;
+}
+
+/**
+ * Annotates an object such that it will never be
+ * transferred away from this process over any promise
+ * communication channel.
+ * @param object
+ * @returns promise a wrapping of that object that
+ * additionally responds to the "isDef" message
+ * without a rejection.
+ */
+Q.master = master;
+function master(object) {
+ return Promise({
+ "isDef": function () {}
+ }, function fallback(op, args) {
+ return dispatch(object, op, args);
+ }, function () {
+ return Q(object).inspect();
+ });
+}
+
+/**
+ * Spreads the values of a promised array of arguments into the
+ * fulfillment callback.
+ * @param fulfilled callback that receives variadic arguments from the
+ * promised array
+ * @param rejected callback that receives the exception if the promise
+ * is rejected.
+ * @returns a promise for the return value or thrown exception of
+ * either callback.
+ */
+Q.spread = spread;
+function spread(value, fulfilled, rejected) {
+ return Q(value).spread(fulfilled, rejected);
+}
+
+Promise.prototype.spread = function (fulfilled, rejected) {
+ return this.all().then(function (array) {
+ return fulfilled.apply(void 0, array);
+ }, rejected);
+};
+
+/**
+ * The async function is a decorator for generator functions, turning
+ * them into asynchronous generators. Although generators are only part
+ * of the newest ECMAScript 6 drafts, this code does not cause syntax
+ * errors in older engines. This code should continue to work and will
+ * in fact improve over time as the language improves.
+ *
+ * ES6 generators are currently part of V8 version 3.19 with the
+ * --harmony-generators runtime flag enabled. SpiderMonkey has had them
+ * for longer, but under an older Python-inspired form. This function
+ * works on both kinds of generators.
+ *
+ * Decorates a generator function such that:
+ * - it may yield promises
+ * - execution will continue when that promise is fulfilled
+ * - the value of the yield expression will be the fulfilled value
+ * - it returns a promise for the return value (when the generator
+ * stops iterating)
+ * - the decorated function returns a promise for the return value
+ * of the generator or the first rejected promise among those
+ * yielded.
+ * - if an error is thrown in the generator, it propagates through
+ * every following yield until it is caught, or until it escapes
+ * the generator function altogether, and is translated into a
+ * rejection for the promise returned by the decorated generator.
+ */
+Q.async = async;
+function async(makeGenerator) {
+ return function () {
+ // when verb is "send", arg is a value
+ // when verb is "throw", arg is an exception
+ function continuer(verb, arg) {
+ var result;
+
+ // Until V8 3.19 / Chromium 29 is released, SpiderMonkey is the only
+ // engine that has a deployed base of browsers that support generators.
+ // However, SM's generators use the Python-inspired semantics of
+ // outdated ES6 drafts. We would like to support ES6, but we'd also
+ // like to make it possible to use generators in deployed browsers, so
+ // we also support Python-style generators. At some point we can remove
+ // this block.
+
+ if (typeof StopIteration === "undefined") {
+ // ES6 Generators
+ try {
+ result = generator[verb](arg);
+ } catch (exception) {
+ return reject(exception);
+ }
+ if (result.done) {
+ return Q(result.value);
+ } else {
+ return when(result.value, callback, errback);
+ }
+ } else {
+ // SpiderMonkey Generators
+ // FIXME: Remove this case when SM does ES6 generators.
+ try {
+ result = generator[verb](arg);
+ } catch (exception) {
+ if (isStopIteration(exception)) {
+ return Q(exception.value);
+ } else {
+ return reject(exception);
+ }
+ }
+ return when(result, callback, errback);
+ }
+ }
+ var generator = makeGenerator.apply(this, arguments);
+ var callback = continuer.bind(continuer, "next");
+ var errback = continuer.bind(continuer, "throw");
+ return callback();
+ };
+}
+
+/**
+ * The spawn function is a small wrapper around async that immediately
+ * calls the generator and also ends the promise chain, so that any
+ * unhandled errors are thrown instead of forwarded to the error
+ * handler. This is useful because it's extremely common to run
+ * generators at the top-level to work with libraries.
+ */
+Q.spawn = spawn;
+function spawn(makeGenerator) {
+ Q.done(Q.async(makeGenerator)());
+}
+
+// FIXME: Remove this interface once ES6 generators are in SpiderMonkey.
+/**
+ * Throws a ReturnValue exception to stop an asynchronous generator.
+ *
+ * This interface is a stop-gap measure to support generator return
+ * values in older Firefox/SpiderMonkey. In browsers that support ES6
+ * generators like Chromium 29, just use "return" in your generator
+ * functions.
+ *
+ * @param value the return value for the surrounding generator
+ * @throws ReturnValue exception with the value.
+ * @example
+ * // ES6 style
+ * Q.async(function* () {
+ * var foo = yield getFooPromise();
+ * var bar = yield getBarPromise();
+ * return foo + bar;
+ * })
+ * // Older SpiderMonkey style
+ * Q.async(function () {
+ * var foo = yield getFooPromise();
+ * var bar = yield getBarPromise();
+ * Q.return(foo + bar);
+ * })
+ */
+Q["return"] = _return;
+function _return(value) {
+ throw new QReturnValue(value);
+}
+
+/**
+ * The promised function decorator ensures that any promise arguments
+ * are settled and passed as values (`this` is also settled and passed
+ * as a value). It will also ensure that the result of a function is
+ * always a promise.
+ *
+ * @example
+ * var add = Q.promised(function (a, b) {
+ * return a + b;
+ * });
+ * add(Q(a), Q(B));
+ *
+ * @param {function} callback The function to decorate
+ * @returns {function} a function that has been decorated.
+ */
+Q.promised = promised;
+function promised(callback) {
+ return function () {
+ return spread([this, all(arguments)], function (self, args) {
+ return callback.apply(self, args);
+ });
+ };
+}
+
+/**
+ * sends a message to a value in a future turn
+ * @param object* the recipient
+ * @param op the name of the message operation, e.g., "when",
+ * @param args further arguments to be forwarded to the operation
+ * @returns result {Promise} a promise for the result of the operation
+ */
+Q.dispatch = dispatch;
+function dispatch(object, op, args) {
+ return Q(object).dispatch(op, args);
+}
+
+Promise.prototype.dispatch = function (op, args) {
+ var self = this;
+ var deferred = defer();
+ Q.nextTick(function () {
+ self.promiseDispatch(deferred.resolve, op, args);
+ });
+ return deferred.promise;
+};
+
+/**
+ * Gets the value of a property in a future turn.
+ * @param object promise or immediate reference for target object
+ * @param name name of property to get
+ * @return promise for the property value
+ */
+Q.get = function (object, key) {
+ return Q(object).dispatch("get", [key]);
+};
+
+Promise.prototype.get = function (key) {
+ return this.dispatch("get", [key]);
+};
+
+/**
+ * Sets the value of a property in a future turn.
+ * @param object promise or immediate reference for object object
+ * @param name name of property to set
+ * @param value new value of property
+ * @return promise for the return value
+ */
+Q.set = function (object, key, value) {
+ return Q(object).dispatch("set", [key, value]);
+};
+
+Promise.prototype.set = function (key, value) {
+ return this.dispatch("set", [key, value]);
+};
+
+/**
+ * Deletes a property in a future turn.
+ * @param object promise or immediate reference for target object
+ * @param name name of property to delete
+ * @return promise for the return value
+ */
+Q.del = // XXX legacy
+Q["delete"] = function (object, key) {
+ return Q(object).dispatch("delete", [key]);
+};
+
+Promise.prototype.del = // XXX legacy
+Promise.prototype["delete"] = function (key) {
+ return this.dispatch("delete", [key]);
+};
+
+/**
+ * Invokes a method in a future turn.
+ * @param object promise or immediate reference for target object
+ * @param name name of method to invoke
+ * @param value a value to post, typically an array of
+ * invocation arguments for promises that
+ * are ultimately backed with `resolve` values,
+ * as opposed to those backed with URLs
+ * wherein the posted value can be any
+ * JSON serializable object.
+ * @return promise for the return value
+ */
+// bound locally because it is used by other methods
+Q.mapply = // XXX As proposed by "Redsandro"
+Q.post = function (object, name, args) {
+ return Q(object).dispatch("post", [name, args]);
+};
+
+Promise.prototype.mapply = // XXX As proposed by "Redsandro"
+Promise.prototype.post = function (name, args) {
+ return this.dispatch("post", [name, args]);
+};
+
+/**
+ * Invokes a method in a future turn.
+ * @param object promise or immediate reference for target object
+ * @param name name of method to invoke
+ * @param ...args array of invocation arguments
+ * @return promise for the return value
+ */
+Q.send = // XXX Mark Miller's proposed parlance
+Q.mcall = // XXX As proposed by "Redsandro"
+Q.invoke = function (object, name /*...args*/) {
+ return Q(object).dispatch("post", [name, array_slice(arguments, 2)]);
+};
+
+Promise.prototype.send = // XXX Mark Miller's proposed parlance
+Promise.prototype.mcall = // XXX As proposed by "Redsandro"
+Promise.prototype.invoke = function (name /*...args*/) {
+ return this.dispatch("post", [name, array_slice(arguments, 1)]);
+};
+
+/**
+ * Applies the promised function in a future turn.
+ * @param object promise or immediate reference for target function
+ * @param args array of application arguments
+ */
+Q.fapply = function (object, args) {
+ return Q(object).dispatch("apply", [void 0, args]);
+};
+
+Promise.prototype.fapply = function (args) {
+ return this.dispatch("apply", [void 0, args]);
+};
+
+/**
+ * Calls the promised function in a future turn.
+ * @param object promise or immediate reference for target function
+ * @param ...args array of application arguments
+ */
+Q["try"] =
+Q.fcall = function (object /* ...args*/) {
+ return Q(object).dispatch("apply", [void 0, array_slice(arguments, 1)]);
+};
+
+Promise.prototype.fcall = function (/*...args*/) {
+ return this.dispatch("apply", [void 0, array_slice(arguments)]);
+};
+
+/**
+ * Binds the promised function, transforming return values into a fulfilled
+ * promise and thrown errors into a rejected one.
+ * @param object promise or immediate reference for target function
+ * @param ...args array of application arguments
+ */
+Q.fbind = function (object /*...args*/) {
+ var promise = Q(object);
+ var args = array_slice(arguments, 1);
+ return function fbound() {
+ return promise.dispatch("apply", [
+ this,
+ args.concat(array_slice(arguments))
+ ]);
+ };
+};
+Promise.prototype.fbind = function (/*...args*/) {
+ var promise = this;
+ var args = array_slice(arguments);
+ return function fbound() {
+ return promise.dispatch("apply", [
+ this,
+ args.concat(array_slice(arguments))
+ ]);
+ };
+};
+
+/**
+ * Requests the names of the owned properties of a promised
+ * object in a future turn.
+ * @param object promise or immediate reference for target object
+ * @return promise for the keys of the eventually settled object
+ */
+Q.keys = function (object) {
+ return Q(object).dispatch("keys", []);
+};
+
+Promise.prototype.keys = function () {
+ return this.dispatch("keys", []);
+};
+
+/**
+ * Turns an array of promises into a promise for an array. If any of
+ * the promises gets rejected, the whole array is rejected immediately.
+ * @param {Array*} an array (or promise for an array) of values (or
+ * promises for values)
+ * @returns a promise for an array of the corresponding values
+ */
+// By Mark Miller
+// http://wiki.ecmascript.org/doku.php?id=strawman:concurrency&rev=1308776521#allfulfilled
+Q.all = all;
+function all(promises) {
+ return when(promises, function (promises) {
+ var pendingCount = 0;
+ var deferred = defer();
+ array_reduce(promises, function (undefined, promise, index) {
+ var snapshot;
+ if (
+ isPromise(promise) &&
+ (snapshot = promise.inspect()).state === "fulfilled"
+ ) {
+ promises[index] = snapshot.value;
+ } else {
+ ++pendingCount;
+ when(
+ promise,
+ function (value) {
+ promises[index] = value;
+ if (--pendingCount === 0) {
+ deferred.resolve(promises);
+ }
+ },
+ deferred.reject,
+ function (progress) {
+ deferred.notify({ index: index, value: progress });
+ }
+ );
+ }
+ }, void 0);
+ if (pendingCount === 0) {
+ deferred.resolve(promises);
+ }
+ return deferred.promise;
+ });
+}
+
+Promise.prototype.all = function () {
+ return all(this);
+};
+
+/**
+ * Returns the first resolved promise of an array. Prior rejected promises are
+ * ignored. Rejects only if all promises are rejected.
+ * @param {Array*} an array containing values or promises for values
+ * @returns a promise fulfilled with the value of the first resolved promise,
+ * or a rejected promise if all promises are rejected.
+ */
+Q.any = any;
+
+function any(promises) {
+ if (promises.length === 0) {
+ return Q.resolve();
+ }
+
+ var deferred = Q.defer();
+ var pendingCount = 0;
+ array_reduce(promises, function (prev, current, index) {
+ var promise = promises[index];
+
+ pendingCount++;
+
+ when(promise, onFulfilled, onRejected, onProgress);
+ function onFulfilled(result) {
+ deferred.resolve(result);
+ }
+ function onRejected() {
+ pendingCount--;
+ if (pendingCount === 0) {
+ deferred.reject(new Error(
+ "Can't get fulfillment value from any promise, all " +
+ "promises were rejected."
+ ));
+ }
+ }
+ function onProgress(progress) {
+ deferred.notify({
+ index: index,
+ value: progress
+ });
+ }
+ }, undefined);
+
+ return deferred.promise;
+}
+
+Promise.prototype.any = function () {
+ return any(this);
+};
+
+/**
+ * Waits for all promises to be settled, either fulfilled or
+ * rejected. This is distinct from `all` since that would stop
+ * waiting at the first rejection. The promise returned by
+ * `allResolved` will never be rejected.
+ * @param promises a promise for an array (or an array) of promises
+ * (or values)
+ * @return a promise for an array of promises
+ */
+Q.allResolved = deprecate(allResolved, "allResolved", "allSettled");
+function allResolved(promises) {
+ return when(promises, function (promises) {
+ promises = array_map(promises, Q);
+ return when(all(array_map(promises, function (promise) {
+ return when(promise, noop, noop);
+ })), function () {
+ return promises;
+ });
+ });
+}
+
+Promise.prototype.allResolved = function () {
+ return allResolved(this);
+};
+
+/**
+ * @see Promise#allSettled
+ */
+Q.allSettled = allSettled;
+function allSettled(promises) {
+ return Q(promises).allSettled();
+}
+
+/**
+ * Turns an array of promises into a promise for an array of their states (as
+ * returned by `inspect`) when they have all settled.
+ * @param {Array[Any*]} values an array (or promise for an array) of values (or
+ * promises for values)
+ * @returns {Array[State]} an array of states for the respective values.
+ */
+Promise.prototype.allSettled = function () {
+ return this.then(function (promises) {
+ return all(array_map(promises, function (promise) {
+ promise = Q(promise);
+ function regardless() {
+ return promise.inspect();
+ }
+ return promise.then(regardless, regardless);
+ }));
+ });
+};
+
+/**
+ * Captures the failure of a promise, giving an oportunity to recover
+ * with a callback. If the given promise is fulfilled, the returned
+ * promise is fulfilled.
+ * @param {Any*} promise for something
+ * @param {Function} callback to fulfill the returned promise if the
+ * given promise is rejected
+ * @returns a promise for the return value of the callback
+ */
+Q.fail = // XXX legacy
+Q["catch"] = function (object, rejected) {
+ return Q(object).then(void 0, rejected);
+};
+
+Promise.prototype.fail = // XXX legacy
+Promise.prototype["catch"] = function (rejected) {
+ return this.then(void 0, rejected);
+};
+
+/**
+ * Attaches a listener that can respond to progress notifications from a
+ * promise's originating deferred. This listener receives the exact arguments
+ * passed to ``deferred.notify``.
+ * @param {Any*} promise for something
+ * @param {Function} callback to receive any progress notifications
+ * @returns the given promise, unchanged
+ */
+Q.progress = progress;
+function progress(object, progressed) {
+ return Q(object).then(void 0, void 0, progressed);
+}
+
+Promise.prototype.progress = function (progressed) {
+ return this.then(void 0, void 0, progressed);
+};
+
+/**
+ * Provides an opportunity to observe the settling of a promise,
+ * regardless of whether the promise is fulfilled or rejected. Forwards
+ * the resolution to the returned promise when the callback is done.
+ * The callback can return a promise to defer completion.
+ * @param {Any*} promise
+ * @param {Function} callback to observe the resolution of the given
+ * promise, takes no arguments.
+ * @returns a promise for the resolution of the given promise when
+ * ``fin`` is done.
+ */
+Q.fin = // XXX legacy
+Q["finally"] = function (object, callback) {
+ return Q(object)["finally"](callback);
+};
+
+Promise.prototype.fin = // XXX legacy
+Promise.prototype["finally"] = function (callback) {
+ callback = Q(callback);
+ return this.then(function (value) {
+ return callback.fcall().then(function () {
+ return value;
+ });
+ }, function (reason) {
+ // TODO attempt to recycle the rejection with "this".
+ return callback.fcall().then(function () {
+ throw reason;
+ });
+ });
+};
+
+/**
+ * Terminates a chain of promises, forcing rejections to be
+ * thrown as exceptions.
+ * @param {Any*} promise at the end of a chain of promises
+ * @returns nothing
+ */
+Q.done = function (object, fulfilled, rejected, progress) {
+ return Q(object).done(fulfilled, rejected, progress);
+};
+
+Promise.prototype.done = function (fulfilled, rejected, progress) {
+ var onUnhandledError = function (error) {
+ // forward to a future turn so that ``when``
+ // does not catch it and turn it into a rejection.
+ Q.nextTick(function () {
+ makeStackTraceLong(error, promise);
+ if (Q.onerror) {
+ Q.onerror(error);
+ } else {
+ throw error;
+ }
+ });
+ };
+
+ // Avoid unnecessary `nextTick`ing via an unnecessary `when`.
+ var promise = fulfilled || rejected || progress ?
+ this.then(fulfilled, rejected, progress) :
+ this;
+
+ if (typeof process === "object" && process && process.domain) {
+ onUnhandledError = process.domain.bind(onUnhandledError);
+ }
+
+ promise.then(void 0, onUnhandledError);
+};
+
+/**
+ * Causes a promise to be rejected if it does not get fulfilled before
+ * some milliseconds time out.
+ * @param {Any*} promise
+ * @param {Number} milliseconds timeout
+ * @param {Any*} custom error message or Error object (optional)
+ * @returns a promise for the resolution of the given promise if it is
+ * fulfilled before the timeout, otherwise rejected.
+ */
+Q.timeout = function (object, ms, error) {
+ return Q(object).timeout(ms, error);
+};
+
+Promise.prototype.timeout = function (ms, error) {
+ var deferred = defer();
+ var timeoutId = setTimeout(function () {
+ if (!error || "string" === typeof error) {
+ error = new Error(error || "Timed out after " + ms + " ms");
+ error.code = "ETIMEDOUT";
+ }
+ deferred.reject(error);
+ }, ms);
+
+ this.then(function (value) {
+ clearTimeout(timeoutId);
+ deferred.resolve(value);
+ }, function (exception) {
+ clearTimeout(timeoutId);
+ deferred.reject(exception);
+ }, deferred.notify);
+
+ return deferred.promise;
+};
+
+/**
+ * Returns a promise for the given value (or promised value), some
+ * milliseconds after it resolved. Passes rejections immediately.
+ * @param {Any*} promise
+ * @param {Number} milliseconds
+ * @returns a promise for the resolution of the given promise after milliseconds
+ * time has elapsed since the resolution of the given promise.
+ * If the given promise rejects, that is passed immediately.
+ */
+Q.delay = function (object, timeout) {
+ if (timeout === void 0) {
+ timeout = object;
+ object = void 0;
+ }
+ return Q(object).delay(timeout);
+};
+
+Promise.prototype.delay = function (timeout) {
+ return this.then(function (value) {
+ var deferred = defer();
+ setTimeout(function () {
+ deferred.resolve(value);
+ }, timeout);
+ return deferred.promise;
+ });
+};
+
+/**
+ * Passes a continuation to a Node function, which is called with the given
+ * arguments provided as an array, and returns a promise.
+ *
+ * Q.nfapply(FS.readFile, [__filename])
+ * .then(function (content) {
+ * })
+ *
+ */
+Q.nfapply = function (callback, args) {
+ return Q(callback).nfapply(args);
+};
+
+Promise.prototype.nfapply = function (args) {
+ var deferred = defer();
+ var nodeArgs = array_slice(args);
+ nodeArgs.push(deferred.makeNodeResolver());
+ this.fapply(nodeArgs).fail(deferred.reject);
+ return deferred.promise;
+};
+
+/**
+ * Passes a continuation to a Node function, which is called with the given
+ * arguments provided individually, and returns a promise.
+ * @example
+ * Q.nfcall(FS.readFile, __filename)
+ * .then(function (content) {
+ * })
+ *
+ */
+Q.nfcall = function (callback /*...args*/) {
+ var args = array_slice(arguments, 1);
+ return Q(callback).nfapply(args);
+};
+
+Promise.prototype.nfcall = function (/*...args*/) {
+ var nodeArgs = array_slice(arguments);
+ var deferred = defer();
+ nodeArgs.push(deferred.makeNodeResolver());
+ this.fapply(nodeArgs).fail(deferred.reject);
+ return deferred.promise;
+};
+
+/**
+ * Wraps a NodeJS continuation passing function and returns an equivalent
+ * version that returns a promise.
+ * @example
+ * Q.nfbind(FS.readFile, __filename)("utf-8")
+ * .then(console.log)
+ * .done()
+ */
+Q.nfbind =
+Q.denodeify = function (callback /*...args*/) {
+ var baseArgs = array_slice(arguments, 1);
+ return function () {
+ var nodeArgs = baseArgs.concat(array_slice(arguments));
+ var deferred = defer();
+ nodeArgs.push(deferred.makeNodeResolver());
+ Q(callback).fapply(nodeArgs).fail(deferred.reject);
+ return deferred.promise;
+ };
+};
+
+Promise.prototype.nfbind =
+Promise.prototype.denodeify = function (/*...args*/) {
+ var args = array_slice(arguments);
+ args.unshift(this);
+ return Q.denodeify.apply(void 0, args);
+};
+
+Q.nbind = function (callback, thisp /*...args*/) {
+ var baseArgs = array_slice(arguments, 2);
+ return function () {
+ var nodeArgs = baseArgs.concat(array_slice(arguments));
+ var deferred = defer();
+ nodeArgs.push(deferred.makeNodeResolver());
+ function bound() {
+ return callback.apply(thisp, arguments);
+ }
+ Q(bound).fapply(nodeArgs).fail(deferred.reject);
+ return deferred.promise;
+ };
+};
+
+Promise.prototype.nbind = function (/*thisp, ...args*/) {
+ var args = array_slice(arguments, 0);
+ args.unshift(this);
+ return Q.nbind.apply(void 0, args);
+};
+
+/**
+ * Calls a method of a Node-style object that accepts a Node-style
+ * callback with a given array of arguments, plus a provided callback.
+ * @param object an object that has the named method
+ * @param {String} name name of the method of object
+ * @param {Array} args arguments to pass to the method; the callback
+ * will be provided by Q and appended to these arguments.
+ * @returns a promise for the value or error
+ */
+Q.nmapply = // XXX As proposed by "Redsandro"
+Q.npost = function (object, name, args) {
+ return Q(object).npost(name, args);
+};
+
+Promise.prototype.nmapply = // XXX As proposed by "Redsandro"
+Promise.prototype.npost = function (name, args) {
+ var nodeArgs = array_slice(args || []);
+ var deferred = defer();
+ nodeArgs.push(deferred.makeNodeResolver());
+ this.dispatch("post", [name, nodeArgs]).fail(deferred.reject);
+ return deferred.promise;
+};
+
+/**
+ * Calls a method of a Node-style object that accepts a Node-style
+ * callback, forwarding the given variadic arguments, plus a provided
+ * callback argument.
+ * @param object an object that has the named method
+ * @param {String} name name of the method of object
+ * @param ...args arguments to pass to the method; the callback will
+ * be provided by Q and appended to these arguments.
+ * @returns a promise for the value or error
+ */
+Q.nsend = // XXX Based on Mark Miller's proposed "send"
+Q.nmcall = // XXX Based on "Redsandro's" proposal
+Q.ninvoke = function (object, name /*...args*/) {
+ var nodeArgs = array_slice(arguments, 2);
+ var deferred = defer();
+ nodeArgs.push(deferred.makeNodeResolver());
+ Q(object).dispatch("post", [name, nodeArgs]).fail(deferred.reject);
+ return deferred.promise;
+};
+
+Promise.prototype.nsend = // XXX Based on Mark Miller's proposed "send"
+Promise.prototype.nmcall = // XXX Based on "Redsandro's" proposal
+Promise.prototype.ninvoke = function (name /*...args*/) {
+ var nodeArgs = array_slice(arguments, 1);
+ var deferred = defer();
+ nodeArgs.push(deferred.makeNodeResolver());
+ this.dispatch("post", [name, nodeArgs]).fail(deferred.reject);
+ return deferred.promise;
+};
+
+/**
+ * If a function would like to support both Node continuation-passing-style and
+ * promise-returning-style, it can end its internal promise chain with
+ * `nodeify(nodeback)`, forwarding the optional nodeback argument. If the user
+ * elects to use a nodeback, the result will be sent there. If they do not
+ * pass a nodeback, they will receive the result promise.
+ * @param object a result (or a promise for a result)
+ * @param {Function} nodeback a Node.js-style callback
+ * @returns either the promise or nothing
+ */
+Q.nodeify = nodeify;
+function nodeify(object, nodeback) {
+ return Q(object).nodeify(nodeback);
+}
+
+Promise.prototype.nodeify = function (nodeback) {
+ if (nodeback) {
+ this.then(function (value) {
+ Q.nextTick(function () {
+ nodeback(null, value);
+ });
+ }, function (error) {
+ Q.nextTick(function () {
+ nodeback(error);
+ });
+ });
+ } else {
+ return this;
+ }
+};
+
+Q.noConflict = function() {
+ throw new Error("Q.noConflict only works when Q is used as a global");
+};
+
+// All code before this point will be filtered from stack traces.
+var qEndingLine = captureLine();
+
+return Q;
+
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/q/queue.js
----------------------------------------------------------------------
diff --git a/node_modules/q/queue.js b/node_modules/q/queue.js
new file mode 100644
index 0000000..1505fd0
--- /dev/null
+++ b/node_modules/q/queue.js
@@ -0,0 +1,35 @@
+
+var Q = require("./q");
+
+module.exports = Queue;
+function Queue() {
+ var ends = Q.defer();
+ var closed = Q.defer();
+ return {
+ put: function (value) {
+ var next = Q.defer();
+ ends.resolve({
+ head: value,
+ tail: next.promise
+ });
+ ends.resolve = next.resolve;
+ },
+ get: function () {
+ var result = ends.promise.get("head");
+ ends.promise = ends.promise.get("tail");
+ return result.fail(function (error) {
+ closed.resolve(error);
+ throw error;
+ });
+ },
+ closed: closed.promise,
+ close: function (error) {
+ error = error || new Error("Can't get value from closed queue");
+ var end = {head: Q.reject(error)};
+ end.tail = end;
+ ends.resolve(end);
+ return closed.promise;
+ }
+ };
+}
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/AUTHORS
----------------------------------------------------------------------
diff --git a/node_modules/sax/AUTHORS b/node_modules/sax/AUTHORS
new file mode 100644
index 0000000..26d8659
--- /dev/null
+++ b/node_modules/sax/AUTHORS
@@ -0,0 +1,9 @@
+# contributors sorted by whether or not they're me.
+Isaac Z. Schlueter <i...@izs.me>
+Stein Martin Hustad <st...@hustad.com>
+Mikeal Rogers <mi...@gmail.com>
+Laurie Harper <la...@holoweb.net>
+Jann Horn <ja...@Jann-PC.fritz.box>
+Elijah Insua <tm...@gmail.com>
+Henry Rawas <he...@schakra.com>
+Justin Makeig <jm...@makeig.com>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/sax/LICENSE b/node_modules/sax/LICENSE
new file mode 100644
index 0000000..05a4010
--- /dev/null
+++ b/node_modules/sax/LICENSE
@@ -0,0 +1,23 @@
+Copyright 2009, 2010, 2011 Isaac Z. Schlueter.
+All rights reserved.
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/README.md
----------------------------------------------------------------------
diff --git a/node_modules/sax/README.md b/node_modules/sax/README.md
new file mode 100644
index 0000000..9c63dc4
--- /dev/null
+++ b/node_modules/sax/README.md
@@ -0,0 +1,213 @@
+# sax js
+
+A sax-style parser for XML and HTML.
+
+Designed with [node](http://nodejs.org/) in mind, but should work fine in
+the browser or other CommonJS implementations.
+
+## What This Is
+
+* A very simple tool to parse through an XML string.
+* A stepping stone to a streaming HTML parser.
+* A handy way to deal with RSS and other mostly-ok-but-kinda-broken XML
+ docs.
+
+## What This Is (probably) Not
+
+* An HTML Parser - That's a fine goal, but this isn't it. It's just
+ XML.
+* A DOM Builder - You can use it to build an object model out of XML,
+ but it doesn't do that out of the box.
+* XSLT - No DOM = no querying.
+* 100% Compliant with (some other SAX implementation) - Most SAX
+ implementations are in Java and do a lot more than this does.
+* An XML Validator - It does a little validation when in strict mode, but
+ not much.
+* A Schema-Aware XSD Thing - Schemas are an exercise in fetishistic
+ masochism.
+* A DTD-aware Thing - Fetching DTDs is a much bigger job.
+
+## Regarding `<!DOCTYPE`s and `<!ENTITY`s
+
+The parser will handle the basic XML entities in text nodes and attribute
+values: `& < > ' "`. It's possible to define additional
+entities in XML by putting them in the DTD. This parser doesn't do anything
+with that. If you want to listen to the `ondoctype` event, and then fetch
+the doctypes, and read the entities and add them to `parser.ENTITIES`, then
+be my guest.
+
+Unknown entities will fail in strict mode, and in loose mode, will pass
+through unmolested.
+
+## Usage
+
+ var sax = require("./lib/sax"),
+ strict = true, // set to false for html-mode
+ parser = sax.parser(strict);
+
+ parser.onerror = function (e) {
+ // an error happened.
+ };
+ parser.ontext = function (t) {
+ // got some text. t is the string of text.
+ };
+ parser.onopentag = function (node) {
+ // opened a tag. node has "name" and "attributes"
+ };
+ parser.onattribute = function (attr) {
+ // an attribute. attr has "name" and "value"
+ };
+ parser.onend = function () {
+ // parser stream is done, and ready to have more stuff written to it.
+ };
+
+ parser.write('<xml>Hello, <who name="world">world</who>!</xml>').close();
+
+ // stream usage
+ // takes the same options as the parser
+ var saxStream = require("sax").createStream(strict, options)
+ saxStream.on("error", function (e) {
+ // unhandled errors will throw, since this is a proper node
+ // event emitter.
+ console.error("error!", e)
+ // clear the error
+ this._parser.error = null
+ this._parser.resume()
+ })
+ saxStream.on("opentag", function (node) {
+ // same object as above
+ })
+ // pipe is supported, and it's readable/writable
+ // same chunks coming in also go out.
+ fs.createReadStream("file.xml")
+ .pipe(saxStream)
+ .pipe(fs.createReadStream("file-copy.xml"))
+
+
+
+## Arguments
+
+Pass the following arguments to the parser function. All are optional.
+
+`strict` - Boolean. Whether or not to be a jerk. Default: `false`.
+
+`opt` - Object bag of settings regarding string formatting. All default to `false`.
+
+Settings supported:
+
+* `trim` - Boolean. Whether or not to trim text and comment nodes.
+* `normalize` - Boolean. If true, then turn any whitespace into a single
+ space.
+* `lowercasetags` - Boolean. If true, then lowercase tags in loose mode,
+ rather than uppercasing them.
+* `xmlns` - Boolean. If true, then namespaces are supported.
+
+## Methods
+
+`write` - Write bytes onto the stream. You don't have to do this all at
+once. You can keep writing as much as you want.
+
+`close` - Close the stream. Once closed, no more data may be written until
+it is done processing the buffer, which is signaled by the `end` event.
+
+`resume` - To gracefully handle errors, assign a listener to the `error`
+event. Then, when the error is taken care of, you can call `resume` to
+continue parsing. Otherwise, the parser will not continue while in an error
+state.
+
+## Members
+
+At all times, the parser object will have the following members:
+
+`line`, `column`, `position` - Indications of the position in the XML
+document where the parser currently is looking.
+
+`startTagPosition` - Indicates the position where the current tag starts.
+
+`closed` - Boolean indicating whether or not the parser can be written to.
+If it's `true`, then wait for the `ready` event to write again.
+
+`strict` - Boolean indicating whether or not the parser is a jerk.
+
+`opt` - Any options passed into the constructor.
+
+`tag` - The current tag being dealt with.
+
+And a bunch of other stuff that you probably shouldn't touch.
+
+## Events
+
+All events emit with a single argument. To listen to an event, assign a
+function to `on<eventname>`. Functions get executed in the this-context of
+the parser object. The list of supported events are also in the exported
+`EVENTS` array.
+
+When using the stream interface, assign handlers using the EventEmitter
+`on` function in the normal fashion.
+
+`error` - Indication that something bad happened. The error will be hanging
+out on `parser.error`, and must be deleted before parsing can continue. By
+listening to this event, you can keep an eye on that kind of stuff. Note:
+this happens *much* more in strict mode. Argument: instance of `Error`.
+
+`text` - Text node. Argument: string of text.
+
+`doctype` - The `<!DOCTYPE` declaration. Argument: doctype string.
+
+`processinginstruction` - Stuff like `<?xml foo="blerg" ?>`. Argument:
+object with `name` and `body` members. Attributes are not parsed, as
+processing instructions have implementation dependent semantics.
+
+`sgmldeclaration` - Random SGML declarations. Stuff like `<!ENTITY p>`
+would trigger this kind of event. This is a weird thing to support, so it
+might go away at some point. SAX isn't intended to be used to parse SGML,
+after all.
+
+`opentag` - An opening tag. Argument: object with `name` and `attributes`.
+In non-strict mode, tag names are uppercased, unless the `lowercasetags`
+option is set. If the `xmlns` option is set, then it will contain
+namespace binding information on the `ns` member, and will have a
+`local`, `prefix`, and `uri` member.
+
+`closetag` - A closing tag. In loose mode, tags are auto-closed if their
+parent closes. In strict mode, well-formedness is enforced. Note that
+self-closing tags will have `closeTag` emitted immediately after `openTag`.
+Argument: tag name.
+
+`attribute` - An attribute node. Argument: object with `name` and `value`,
+and also namespace information if the `xmlns` option flag is set.
+
+`comment` - A comment node. Argument: the string of the comment.
+
+`opencdata` - The opening tag of a `<![CDATA[` block.
+
+`cdata` - The text of a `<![CDATA[` block. Since `<![CDATA[` blocks can get
+quite large, this event may fire multiple times for a single block, if it
+is broken up into multiple `write()`s. Argument: the string of random
+character data.
+
+`closecdata` - The closing tag (`]]>`) of a `<![CDATA[` block.
+
+`opennamespace` - If the `xmlns` option is set, then this event will
+signal the start of a new namespace binding.
+
+`closenamespace` - If the `xmlns` option is set, then this event will
+signal the end of a namespace binding.
+
+`end` - Indication that the closed stream has ended.
+
+`ready` - Indication that the stream has reset, and is ready to be written
+to.
+
+`noscript` - In non-strict mode, `<script>` tags trigger a `"script"`
+event, and their contents are not checked for special xml characters.
+If you pass `noscript: true`, then this behavior is suppressed.
+
+## Reporting Problems
+
+It's best to write a failing test if you find an issue. I will always
+accept pull requests with failing tests if they demonstrate intended
+behavior, but it is very hard to figure out what issue you're describing
+without a test. Writing a test is also the best way for you yourself
+to figure out if you really understand the issue you think you have with
+sax-js.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[31/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/escapeStringChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/escapeStringChar.js b/node_modules/lodash-node/underscore/internals/escapeStringChar.js
new file mode 100644
index 0000000..bd88060
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/escapeStringChar.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to escape characters for inclusion in compiled string literals */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\t': 't',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `template` to escape characters for inclusion in compiled
+ * string literals.
+ *
+ * @private
+ * @param {string} match The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeStringChar(match) {
+ return '\\' + stringEscapes[match];
+}
+
+module.exports = escapeStringChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/htmlEscapes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/htmlEscapes.js b/node_modules/lodash-node/underscore/internals/htmlEscapes.js
new file mode 100644
index 0000000..d221a5c
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/htmlEscapes.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used to convert characters to HTML entities:
+ *
+ * Though the `>` character is escaped for symmetry, characters like `>` and `/`
+ * don't require escaping in HTML and have no special meaning unless they're part
+ * of a tag or an unquoted attribute value.
+ * http://mathiasbynens.be/notes/ambiguous-ampersands (under "semi-related fun fact")
+ */
+var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+};
+
+module.exports = htmlEscapes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/htmlUnescapes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/htmlUnescapes.js b/node_modules/lodash-node/underscore/internals/htmlUnescapes.js
new file mode 100644
index 0000000..0f818c1
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/htmlUnescapes.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes'),
+ invert = require('../objects/invert');
+
+/** Used to convert HTML entities to characters */
+var htmlUnescapes = invert(htmlEscapes);
+
+module.exports = htmlUnescapes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/indicatorObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/indicatorObject.js b/node_modules/lodash-node/underscore/internals/indicatorObject.js
new file mode 100644
index 0000000..684ec9b
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/indicatorObject.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used internally to indicate various things */
+var indicatorObject = {};
+
+module.exports = indicatorObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/isNative.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/isNative.js b/node_modules/lodash-node/underscore/internals/isNative.js
new file mode 100644
index 0000000..5c1457e
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/isNative.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Used to detect if a method is native */
+var reNative = RegExp('^' +
+ String(toString)
+ .replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
+ .replace(/toString| for [^\]]+/g, '.*?') + '$'
+);
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a native function, else `false`.
+ */
+function isNative(value) {
+ return typeof value == 'function' && reNative.test(value);
+}
+
+module.exports = isNative;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/keyPrefix.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/keyPrefix.js b/node_modules/lodash-node/underscore/internals/keyPrefix.js
new file mode 100644
index 0000000..d4b4945
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/keyPrefix.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to prefix keys to avoid issues with `__proto__` and properties on `Object.prototype` */
+var keyPrefix = +new Date + '';
+
+module.exports = keyPrefix;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/lodashWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/lodashWrapper.js b/node_modules/lodash-node/underscore/internals/lodashWrapper.js
new file mode 100644
index 0000000..e8d38b5
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/lodashWrapper.js
@@ -0,0 +1,23 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * A fast path for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @param {boolean} chainAll A flag to enable chaining for all methods
+ * @returns {Object} Returns a `lodash` instance.
+ */
+function lodashWrapper(value, chainAll) {
+ this.__chain__ = !!chainAll;
+ this.__wrapped__ = value;
+}
+
+module.exports = lodashWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/objectTypes.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/objectTypes.js b/node_modules/lodash-node/underscore/internals/objectTypes.js
new file mode 100644
index 0000000..0ff2c28
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/objectTypes.js
@@ -0,0 +1,20 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to determine if values are of the language type Object */
+var objectTypes = {
+ 'boolean': false,
+ 'function': true,
+ 'object': true,
+ 'number': false,
+ 'string': false,
+ 'undefined': false
+};
+
+module.exports = objectTypes;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/reEscapedHtml.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/reEscapedHtml.js b/node_modules/lodash-node/underscore/internals/reEscapedHtml.js
new file mode 100644
index 0000000..07fe67c
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/reEscapedHtml.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlUnescapes = require('./htmlUnescapes'),
+ keys = require('../objects/keys');
+
+/** Used to match HTML entities and HTML characters */
+var reEscapedHtml = RegExp('(' + keys(htmlUnescapes).join('|') + ')', 'g');
+
+module.exports = reEscapedHtml;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/reInterpolate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/reInterpolate.js b/node_modules/lodash-node/underscore/internals/reInterpolate.js
new file mode 100644
index 0000000..07af4c8
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/reInterpolate.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to match "interpolate" template delimiters */
+var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+module.exports = reInterpolate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/reUnescapedHtml.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/reUnescapedHtml.js b/node_modules/lodash-node/underscore/internals/reUnescapedHtml.js
new file mode 100644
index 0000000..f9cdf92
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/reUnescapedHtml.js
@@ -0,0 +1,15 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlEscapes = require('./htmlEscapes'),
+ keys = require('../objects/keys');
+
+/** Used to match HTML entities and HTML characters */
+var reUnescapedHtml = RegExp('[' + keys(htmlEscapes).join('') + ']', 'g');
+
+module.exports = reUnescapedHtml;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/shimKeys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/shimKeys.js b/node_modules/lodash-node/underscore/internals/shimKeys.js
new file mode 100644
index 0000000..6471285
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/shimKeys.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('./objectTypes');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A fallback implementation of `Object.keys` which produces an array of the
+ * given object's own enumerable property names.
+ *
+ * @private
+ * @type Function
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names.
+ */
+var shimKeys = function(object) {
+ var index, iterable = object, result = [];
+ if (!iterable) return result;
+ if (!(objectTypes[typeof object])) return result;
+ for (index in iterable) {
+ if (hasOwnProperty.call(iterable, index)) {
+ result.push(index);
+ }
+ }
+ return result
+};
+
+module.exports = shimKeys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/slice.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/slice.js b/node_modules/lodash-node/underscore/internals/slice.js
new file mode 100644
index 0000000..7c19750
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/slice.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Slices the `collection` from the `start` index up to, but not including,
+ * the `end` index.
+ *
+ * Note: This function is used instead of `Array#slice` to support node lists
+ * in IE < 9 and to ensure dense arrays are returned.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to slice.
+ * @param {number} start The start index.
+ * @param {number} end The end index.
+ * @returns {Array} Returns the new array.
+ */
+function slice(array, start, end) {
+ start || (start = 0);
+ if (typeof end == 'undefined') {
+ end = array ? array.length : 0;
+ }
+ var index = -1,
+ length = end - start || 0,
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = array[start + index];
+ }
+ return result;
+}
+
+module.exports = slice;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/internals/unescapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/internals/unescapeHtmlChar.js b/node_modules/lodash-node/underscore/internals/unescapeHtmlChar.js
new file mode 100644
index 0000000..080bb93
--- /dev/null
+++ b/node_modules/lodash-node/underscore/internals/unescapeHtmlChar.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var htmlUnescapes = require('./htmlUnescapes');
+
+/**
+ * Used by `unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {string} match The matched character to unescape.
+ * @returns {string} Returns the unescaped character.
+ */
+function unescapeHtmlChar(match) {
+ return htmlUnescapes[match];
+}
+
+module.exports = unescapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects.js b/node_modules/lodash-node/underscore/objects.js
new file mode 100644
index 0000000..1f45ee6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'assign': require('./objects/assign'),
+ 'clone': require('./objects/clone'),
+ 'defaults': require('./objects/defaults'),
+ 'extend': require('./objects/assign'),
+ 'forIn': require('./objects/forIn'),
+ 'forOwn': require('./objects/forOwn'),
+ 'functions': require('./objects/functions'),
+ 'has': require('./objects/has'),
+ 'invert': require('./objects/invert'),
+ 'isArguments': require('./objects/isArguments'),
+ 'isArray': require('./objects/isArray'),
+ 'isBoolean': require('./objects/isBoolean'),
+ 'isDate': require('./objects/isDate'),
+ 'isElement': require('./objects/isElement'),
+ 'isEmpty': require('./objects/isEmpty'),
+ 'isEqual': require('./objects/isEqual'),
+ 'isFinite': require('./objects/isFinite'),
+ 'isFunction': require('./objects/isFunction'),
+ 'isNaN': require('./objects/isNaN'),
+ 'isNull': require('./objects/isNull'),
+ 'isNumber': require('./objects/isNumber'),
+ 'isObject': require('./objects/isObject'),
+ 'isRegExp': require('./objects/isRegExp'),
+ 'isString': require('./objects/isString'),
+ 'isUndefined': require('./objects/isUndefined'),
+ 'keys': require('./objects/keys'),
+ 'methods': require('./objects/functions'),
+ 'omit': require('./objects/omit'),
+ 'pairs': require('./objects/pairs'),
+ 'pick': require('./objects/pick'),
+ 'values': require('./objects/values')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/assign.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/assign.js b/node_modules/lodash-node/underscore/objects/assign.js
new file mode 100644
index 0000000..cf2ce8a
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/assign.js
@@ -0,0 +1,58 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ keys = require('./keys'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object. Subsequent sources will overwrite property assignments of previous
+ * sources. If a callback is provided it will be executed to produce the
+ * assigned values. The callback is bound to `thisArg` and invoked with two
+ * arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @alias extend
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param {Function} [callback] The function to customize assigning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * _.assign({ 'name': 'fred' }, { 'employer': 'slate' });
+ * // => { 'name': 'fred', 'employer': 'slate' }
+ *
+ * var defaults = _.partialRight(_.assign, function(a, b) {
+ * return typeof a == 'undefined' ? b : a;
+ * });
+ *
+ * var object = { 'name': 'barney' };
+ * defaults(object, { 'name': 'fred', 'employer': 'slate' });
+ * // => { 'name': 'barney', 'employer': 'slate' }
+ */
+function assign(object) {
+ if (!object) {
+ return object;
+ }
+ for (var argsIndex = 1, argsLength = arguments.length; argsIndex < argsLength; argsIndex++) {
+ var iterable = arguments[argsIndex];
+ if (iterable) {
+ for (var key in iterable) {
+ object[key] = iterable[key];
+ }
+ }
+ }
+ return object;
+}
+
+module.exports = assign;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/clone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/clone.js b/node_modules/lodash-node/underscore/objects/clone.js
new file mode 100644
index 0000000..f5735b2
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/clone.js
@@ -0,0 +1,61 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var assign = require('./assign'),
+ baseCreateCallback = require('../internals/baseCreateCallback'),
+ isArray = require('./isArray'),
+ isObject = require('./isObject'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a clone of `value`. If `isDeep` is `true` nested objects will also
+ * be cloned, otherwise they will be assigned by reference. If a callback
+ * is provided it will be executed to produce the cloned values. If the
+ * callback returns `undefined` cloning will be handled by the method instead.
+ * The callback is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep=false] Specify a deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the cloned value.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * var shallow = _.clone(characters);
+ * shallow[0] === characters[0];
+ * // => true
+ *
+ * var deep = _.clone(characters, true);
+ * deep[0] === characters[0];
+ * // => false
+ *
+ * _.mixin({
+ * 'clone': _.partialRight(_.clone, function(value) {
+ * return _.isElement(value) ? value.cloneNode(false) : undefined;
+ * })
+ * });
+ *
+ * var clone = _.clone(document.body);
+ * clone.childNodes.length;
+ * // => 0
+ */
+function clone(value) {
+ return isObject(value)
+ ? (isArray(value) ? slice(value) : assign({}, value))
+ : value;
+}
+
+module.exports = clone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/defaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/defaults.js b/node_modules/lodash-node/underscore/objects/defaults.js
new file mode 100644
index 0000000..a27605d
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/defaults.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object for all destination properties that resolve to `undefined`. Once a
+ * property is set, additional defaults of the same property will be ignored.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param- {Object} [guard] Allows working with `_.reduce` without using its
+ * `key` and `object` arguments as sources.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var object = { 'name': 'barney' };
+ * _.defaults(object, { 'name': 'fred', 'employer': 'slate' });
+ * // => { 'name': 'barney', 'employer': 'slate' }
+ */
+function defaults(object) {
+ if (!object) {
+ return object;
+ }
+ for (var argsIndex = 1, argsLength = arguments.length; argsIndex < argsLength; argsIndex++) {
+ var iterable = arguments[argsIndex];
+ if (iterable) {
+ for (var key in iterable) {
+ if (typeof object[key] == 'undefined') {
+ object[key] = iterable[key];
+ }
+ }
+ }
+ }
+ return object;
+}
+
+module.exports = defaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/forIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/forIn.js b/node_modules/lodash-node/underscore/objects/forIn.js
new file mode 100644
index 0000000..e8d29f9
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/forIn.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ indicatorObject = require('../internals/indicatorObject'),
+ objectTypes = require('../internals/objectTypes');
+
+/**
+ * Iterates over own and inherited enumerable properties of an object,
+ * executing the callback for each property. The callback is bound to `thisArg`
+ * and invoked with three arguments; (value, key, object). Callbacks may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * Shape.prototype.move = function(x, y) {
+ * this.x += x;
+ * this.y += y;
+ * };
+ *
+ * _.forIn(new Shape, function(value, key) {
+ * console.log(key);
+ * });
+ * // => logs 'x', 'y', and 'move' (property order is not guaranteed across environments)
+ */
+var forIn = function(collection, callback) {
+ var index, iterable = collection, result = iterable;
+ if (!iterable) return result;
+ if (!objectTypes[typeof iterable]) return result;
+ for (index in iterable) {
+ if (callback(iterable[index], index, collection) === indicatorObject) return result;
+ }
+ return result
+};
+
+module.exports = forIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/forOwn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/forOwn.js b/node_modules/lodash-node/underscore/objects/forOwn.js
new file mode 100644
index 0000000..b91a110
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/forOwn.js
@@ -0,0 +1,53 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ indicatorObject = require('../internals/indicatorObject'),
+ keys = require('./keys'),
+ objectTypes = require('../internals/objectTypes');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Iterates over own enumerable properties of an object, executing the callback
+ * for each property. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, key, object). Callbacks may exit iteration early by
+ * explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.forOwn({ '0': 'zero', '1': 'one', 'length': 2 }, function(num, key) {
+ * console.log(key);
+ * });
+ * // => logs '0', '1', and 'length' (property order is not guaranteed across environments)
+ */
+var forOwn = function(collection, callback) {
+ var index, iterable = collection, result = iterable;
+ if (!iterable) return result;
+ if (!objectTypes[typeof iterable]) return result;
+ for (index in iterable) {
+ if (hasOwnProperty.call(iterable, index)) {
+ if (callback(iterable[index], index, collection) === indicatorObject) return result;
+ }
+ }
+ return result
+};
+
+module.exports = forOwn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/functions.js b/node_modules/lodash-node/underscore/objects/functions.js
new file mode 100644
index 0000000..2ac5794
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/functions.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('./forIn'),
+ isFunction = require('./isFunction');
+
+/**
+ * Creates a sorted array of property names of all enumerable properties,
+ * own and inherited, of `object` that have function values.
+ *
+ * @static
+ * @memberOf _
+ * @alias methods
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names that have function values.
+ * @example
+ *
+ * _.functions(_);
+ * // => ['all', 'any', 'bind', 'bindAll', 'clone', 'compact', 'compose', ...]
+ */
+function functions(object) {
+ var result = [];
+ forIn(object, function(value, key) {
+ if (isFunction(value)) {
+ result.push(key);
+ }
+ });
+ return result.sort();
+}
+
+module.exports = functions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/has.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/has.js b/node_modules/lodash-node/underscore/objects/has.js
new file mode 100644
index 0000000..838a4d6
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/has.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if the specified property name exists as a direct property of `object`,
+ * instead of an inherited property.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @param {string} key The name of the property to check.
+ * @returns {boolean} Returns `true` if key is a direct property, else `false`.
+ * @example
+ *
+ * _.has({ 'a': 1, 'b': 2, 'c': 3 }, 'b');
+ * // => true
+ */
+function has(object, key) {
+ return object ? hasOwnProperty.call(object, key) : false;
+}
+
+module.exports = has;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/invert.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/invert.js b/node_modules/lodash-node/underscore/objects/invert.js
new file mode 100644
index 0000000..a9716ca
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/invert.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates an object composed of the inverted keys and values of the given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to invert.
+ * @returns {Object} Returns the created inverted object.
+ * @example
+ *
+ * _.invert({ 'first': 'fred', 'second': 'barney' });
+ * // => { 'fred': 'first', 'barney': 'second' }
+ */
+function invert(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ result[object[key]] = key;
+ }
+ return result;
+}
+
+module.exports = invert;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isArguments.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isArguments.js b/node_modules/lodash-node/underscore/objects/isArguments.js
new file mode 100644
index 0000000..74736fe
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isArguments.js
@@ -0,0 +1,51 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * Checks if `value` is an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an `arguments` object, else `false`.
+ * @example
+ *
+ * (function() { return _.isArguments(arguments); })(1, 2, 3);
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ toString.call(value) == argsClass || false;
+}
+// fallback for browsers that can't detect `arguments` objects by [[Class]]
+if (!isArguments(arguments)) {
+ isArguments = function(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ hasOwnProperty.call(value, 'callee') && !propertyIsEnumerable.call(value, 'callee') || false;
+ };
+}
+
+module.exports = isArguments;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isArray.js b/node_modules/lodash-node/underscore/objects/isArray.js
new file mode 100644
index 0000000..7ef5cd1
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isArray.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative');
+
+/** `Object#toString` result shortcuts */
+var arrayClass = '[object Array]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeIsArray = isNative(nativeIsArray = Array.isArray) && nativeIsArray;
+
+/**
+ * Checks if `value` is an array.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an array, else `false`.
+ * @example
+ *
+ * (function() { return _.isArray(arguments); })();
+ * // => false
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ */
+var isArray = nativeIsArray || function(value) {
+ return value && typeof value == 'object' && typeof value.length == 'number' &&
+ toString.call(value) == arrayClass || false;
+};
+
+module.exports = isArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isBoolean.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isBoolean.js b/node_modules/lodash-node/underscore/objects/isBoolean.js
new file mode 100644
index 0000000..ac09e8f
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isBoolean.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var boolClass = '[object Boolean]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a boolean value.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a boolean value, else `false`.
+ * @example
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+function isBoolean(value) {
+ return value === true || value === false ||
+ value && typeof value == 'object' && toString.call(value) == boolClass || false;
+}
+
+module.exports = isBoolean;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isDate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isDate.js b/node_modules/lodash-node/underscore/objects/isDate.js
new file mode 100644
index 0000000..2c9416a
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isDate.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var dateClass = '[object Date]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a date.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a date, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ */
+function isDate(value) {
+ return value && typeof value == 'object' && toString.call(value) == dateClass || false;
+}
+
+module.exports = isDate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isElement.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isElement.js b/node_modules/lodash-node/underscore/objects/isElement.js
new file mode 100644
index 0000000..86a26a0
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isElement.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ */
+function isElement(value) {
+ return value && value.nodeType === 1 || false;
+}
+
+module.exports = isElement;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isEmpty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isEmpty.js b/node_modules/lodash-node/underscore/objects/isEmpty.js
new file mode 100644
index 0000000..1b90fb5
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isEmpty.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArray = require('./isArray'),
+ isString = require('./isString');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Checks if `value` is empty. Arrays, strings, or `arguments` objects with a
+ * length of `0` and objects with no own enumerable properties are considered
+ * "empty".
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Array|Object|string} value The value to inspect.
+ * @returns {boolean} Returns `true` if the `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({});
+ * // => true
+ *
+ * _.isEmpty('');
+ * // => true
+ */
+function isEmpty(value) {
+ if (!value) {
+ return true;
+ }
+ if (isArray(value) || isString(value)) {
+ return !value.length;
+ }
+ for (var key in value) {
+ if (hasOwnProperty.call(value, key)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = isEmpty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isEqual.js b/node_modules/lodash-node/underscore/objects/isEqual.js
new file mode 100644
index 0000000..741f165
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isEqual.js
@@ -0,0 +1,53 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIsEqual = require('../internals/baseIsEqual');
+
+/**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent to each other. If a callback is provided it will be executed
+ * to compare values. If the callback returns `undefined` comparisons will
+ * be handled by the method instead. The callback is bound to `thisArg` and
+ * invoked with two arguments; (a, b).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} a The value to compare.
+ * @param {*} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * var copy = { 'name': 'fred' };
+ *
+ * object == copy;
+ * // => false
+ *
+ * _.isEqual(object, copy);
+ * // => true
+ *
+ * var words = ['hello', 'goodbye'];
+ * var otherWords = ['hi', 'goodbye'];
+ *
+ * _.isEqual(words, otherWords, function(a, b) {
+ * var reGreet = /^(?:hello|hi)$/i,
+ * aGreet = _.isString(a) && reGreet.test(a),
+ * bGreet = _.isString(b) && reGreet.test(b);
+ *
+ * return (aGreet || bGreet) ? (aGreet == bGreet) : undefined;
+ * });
+ * // => true
+ */
+function isEqual(a, b) {
+ return baseIsEqual(a, b);
+}
+
+module.exports = isEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isFinite.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isFinite.js b/node_modules/lodash-node/underscore/objects/isFinite.js
new file mode 100644
index 0000000..bc29c51
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isFinite.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeIsFinite = global.isFinite,
+ nativeIsNaN = global.isNaN;
+
+/**
+ * Checks if `value` is, or can be coerced to, a finite number.
+ *
+ * Note: This is not the same as native `isFinite` which will return true for
+ * booleans and empty strings. See http://es5.github.io/#x15.1.2.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is finite, else `false`.
+ * @example
+ *
+ * _.isFinite(-101);
+ * // => true
+ *
+ * _.isFinite('10');
+ * // => true
+ *
+ * _.isFinite(true);
+ * // => false
+ *
+ * _.isFinite('');
+ * // => false
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ */
+function isFinite(value) {
+ return nativeIsFinite(value) && !nativeIsNaN(parseFloat(value));
+}
+
+module.exports = isFinite;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isFunction.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isFunction.js b/node_modules/lodash-node/underscore/objects/isFunction.js
new file mode 100644
index 0000000..2ef33dc
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isFunction.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var funcClass = '[object Function]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a function.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ */
+function isFunction(value) {
+ return typeof value == 'function';
+}
+// fallback for older versions of Chrome and Safari
+if (isFunction(/x/)) {
+ isFunction = function(value) {
+ return typeof value == 'function' && toString.call(value) == funcClass;
+ };
+}
+
+module.exports = isFunction;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isNaN.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isNaN.js b/node_modules/lodash-node/underscore/objects/isNaN.js
new file mode 100644
index 0000000..cc807b7
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isNaN.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNumber = require('./isNumber');
+
+/**
+ * Checks if `value` is `NaN`.
+ *
+ * Note: This is not the same as native `isNaN` which will return `true` for
+ * `undefined` and other non-numeric values. See http://es5.github.io/#x15.1.2.4.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+function isNaN(value) {
+ // `NaN` as a primitive is the only value that is not equal to itself
+ // (perform the [[Class]] check first to avoid errors with some host objects in IE)
+ return isNumber(value) && value != +value;
+}
+
+module.exports = isNaN;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isNull.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isNull.js b/node_modules/lodash-node/underscore/objects/isNull.js
new file mode 100644
index 0000000..8dc0797
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isNull.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(undefined);
+ * // => false
+ */
+function isNull(value) {
+ return value === null;
+}
+
+module.exports = isNull;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isNumber.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isNumber.js b/node_modules/lodash-node/underscore/objects/isNumber.js
new file mode 100644
index 0000000..f9d88af
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isNumber.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var numberClass = '[object Number]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a number.
+ *
+ * Note: `NaN` is considered a number. See http://es5.github.io/#x8.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(8.4 * 5);
+ * // => true
+ */
+function isNumber(value) {
+ return typeof value == 'number' ||
+ value && typeof value == 'object' && toString.call(value) == numberClass || false;
+}
+
+module.exports = isNumber;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isObject.js b/node_modules/lodash-node/underscore/objects/isObject.js
new file mode 100644
index 0000000..53ceabb
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isObject.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('../internals/objectTypes');
+
+/**
+ * Checks if `value` is the language type of Object.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // check if the value is the ECMAScript language type of Object
+ // http://es5.github.io/#x8
+ // and avoid a V8 bug
+ // http://code.google.com/p/v8/issues/detail?id=2291
+ return !!(value && objectTypes[typeof value]);
+}
+
+module.exports = isObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isRegExp.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isRegExp.js b/node_modules/lodash-node/underscore/objects/isRegExp.js
new file mode 100644
index 0000000..48626e5
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isRegExp.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('../internals/objectTypes');
+
+/** `Object#toString` result shortcuts */
+var regexpClass = '[object RegExp]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a regular expression.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a regular expression, else `false`.
+ * @example
+ *
+ * _.isRegExp(/fred/);
+ * // => true
+ */
+function isRegExp(value) {
+ return value && objectTypes[typeof value] && toString.call(value) == regexpClass || false;
+}
+
+module.exports = isRegExp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isString.js b/node_modules/lodash-node/underscore/objects/isString.js
new file mode 100644
index 0000000..e8df7c4
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isString.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a string.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('fred');
+ * // => true
+ */
+function isString(value) {
+ return typeof value == 'string' ||
+ value && typeof value == 'object' && toString.call(value) == stringClass || false;
+}
+
+module.exports = isString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/isUndefined.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/isUndefined.js b/node_modules/lodash-node/underscore/objects/isUndefined.js
new file mode 100644
index 0000000..4d54db3
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/isUndefined.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ */
+function isUndefined(value) {
+ return typeof value == 'undefined';
+}
+
+module.exports = isUndefined;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/keys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/keys.js b/node_modules/lodash-node/underscore/objects/keys.js
new file mode 100644
index 0000000..ae7401d
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/keys.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative'),
+ isObject = require('./isObject'),
+ shimKeys = require('../internals/shimKeys');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeKeys = isNative(nativeKeys = Object.keys) && nativeKeys;
+
+/**
+ * Creates an array composed of the own enumerable property names of an object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names.
+ * @example
+ *
+ * _.keys({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => ['one', 'two', 'three'] (property order is not guaranteed across environments)
+ */
+var keys = !nativeKeys ? shimKeys : function(object) {
+ if (!isObject(object)) {
+ return [];
+ }
+ return nativeKeys(object);
+};
+
+module.exports = keys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/omit.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/omit.js b/node_modules/lodash-node/underscore/objects/omit.js
new file mode 100644
index 0000000..33c65ae
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/omit.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseFlatten = require('../internals/baseFlatten'),
+ forIn = require('./forIn');
+
+/**
+ * Creates a shallow clone of `object` excluding the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a callback is provided it will be executed for each
+ * property of `object` omitting the properties the callback returns truey
+ * for. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|...string|string[]} [callback] The properties to omit or the
+ * function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object without the omitted properties.
+ * @example
+ *
+ * _.omit({ 'name': 'fred', 'age': 40 }, 'age');
+ * // => { 'name': 'fred' }
+ *
+ * _.omit({ 'name': 'fred', 'age': 40 }, function(value) {
+ * return typeof value == 'number';
+ * });
+ * // => { 'name': 'fred' }
+ */
+function omit(object) {
+ var props = [];
+ forIn(object, function(value, key) {
+ props.push(key);
+ });
+ props = baseDifference(props, baseFlatten(arguments, true, false, 1));
+
+ var index = -1,
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ result[key] = object[key];
+ }
+ return result;
+}
+
+module.exports = omit;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/pairs.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/pairs.js b/node_modules/lodash-node/underscore/objects/pairs.js
new file mode 100644
index 0000000..ea8c7ae
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/pairs.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates a two dimensional array of an object's key-value pairs,
+ * i.e. `[[key1, value1], [key2, value2]]`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns new array of key-value pairs.
+ * @example
+ *
+ * _.pairs({ 'barney': 36, 'fred': 40 });
+ * // => [['barney', 36], ['fred', 40]] (property order is not guaranteed across environments)
+ */
+function pairs(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ var key = props[index];
+ result[index] = [key, object[key]];
+ }
+ return result;
+}
+
+module.exports = pairs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/pick.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/pick.js b/node_modules/lodash-node/underscore/objects/pick.js
new file mode 100644
index 0000000..d195fb5
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/pick.js
@@ -0,0 +1,53 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten');
+
+/**
+ * Creates a shallow clone of `object` composed of the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a callback is provided it will be executed for each
+ * property of `object` picking the properties the callback returns truey
+ * for. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|...string|string[]} [callback] The function called per
+ * iteration or property names to pick, specified as individual property
+ * names or arrays of property names.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object composed of the picked properties.
+ * @example
+ *
+ * _.pick({ 'name': 'fred', '_userid': 'fred1' }, 'name');
+ * // => { 'name': 'fred' }
+ *
+ * _.pick({ 'name': 'fred', '_userid': 'fred1' }, function(value, key) {
+ * return key.charAt(0) != '_';
+ * });
+ * // => { 'name': 'fred' }
+ */
+function pick(object) {
+ var index = -1,
+ props = baseFlatten(arguments, true, false, 1),
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ if (key in object) {
+ result[key] = object[key];
+ }
+ }
+ return result;
+}
+
+module.exports = pick;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/objects/values.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/objects/values.js b/node_modules/lodash-node/underscore/objects/values.js
new file mode 100644
index 0000000..d11055e
--- /dev/null
+++ b/node_modules/lodash-node/underscore/objects/values.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates an array composed of the own enumerable property values of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property values.
+ * @example
+ *
+ * _.values({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => [1, 2, 3] (property order is not guaranteed across environments)
+ */
+function values(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = object[props[index]];
+ }
+ return result;
+}
+
+module.exports = values;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/support.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/support.js b/node_modules/lodash-node/underscore/support.js
new file mode 100644
index 0000000..90fbe96
--- /dev/null
+++ b/node_modules/lodash-node/underscore/support.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./internals/isNative');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/**
+ * An object used to flag environments features.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var support = {};
+
+(function() {
+ var object = { '0': 1, 'length': 1 };
+
+ /**
+ * Detect if `Array#shift` and `Array#splice` augment array-like objects correctly.
+ *
+ * Firefox < 10, IE compatibility mode, and IE < 9 have buggy Array `shift()`
+ * and `splice()` functions that fail to remove the last element, `value[0]`,
+ * of array-like objects even though the `length` property is set to `0`.
+ * The `shift()` method is buggy in IE 8 compatibility mode, while `splice()`
+ * is buggy regardless of mode in IE < 9 and buggy in compatibility mode in IE 9.
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+ support.spliceObjects = (arrayRef.splice.call(object, 0, 1), !object[0]);
+}(1));
+
+module.exports = support;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities.js b/node_modules/lodash-node/underscore/utilities.js
new file mode 100644
index 0000000..cef5f20
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'createCallback': require('./functions/createCallback'),
+ 'escape': require('./utilities/escape'),
+ 'identity': require('./utilities/identity'),
+ 'mixin': require('./utilities/mixin'),
+ 'noConflict': require('./utilities/noConflict'),
+ 'noop': require('./utilities/noop'),
+ 'now': require('./utilities/now'),
+ 'property': require('./utilities/property'),
+ 'random': require('./utilities/random'),
+ 'result': require('./utilities/result'),
+ 'template': require('./utilities/template'),
+ 'templateSettings': require('./utilities/templateSettings'),
+ 'times': require('./utilities/times'),
+ 'unescape': require('./utilities/unescape'),
+ 'uniqueId': require('./utilities/uniqueId')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/escape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/escape.js b/node_modules/lodash-node/underscore/utilities/escape.js
new file mode 100644
index 0000000..2e1f5c0
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/escape.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var escapeHtmlChar = require('../internals/escapeHtmlChar'),
+ keys = require('../objects/keys'),
+ reUnescapedHtml = require('../internals/reUnescapedHtml');
+
+/**
+ * Converts the characters `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding HTML entities.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} string The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('Fred, Wilma, & Pebbles');
+ * // => 'Fred, Wilma, & Pebbles'
+ */
+function escape(string) {
+ return string == null ? '' : String(string).replace(reUnescapedHtml, escapeHtmlChar);
+}
+
+module.exports = escape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/identity.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/identity.js b/node_modules/lodash-node/underscore/utilities/identity.js
new file mode 100644
index 0000000..5734880
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/identity.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * This method returns the first argument provided to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * _.identity(object) === object;
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+module.exports = identity;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/mixin.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/mixin.js b/node_modules/lodash-node/underscore/utilities/mixin.js
new file mode 100644
index 0000000..9865704
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/mixin.js
@@ -0,0 +1,74 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ functions = require('../objects/functions'),
+ isFunction = require('../objects/isFunction');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * Adds function properties of a source object to the destination object.
+ * If `object` is a function methods will be added to its prototype as well.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Function|Object} [object=lodash] object The destination object.
+ * @param {Object} source The object of functions to add.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.chain=true] Specify whether the functions added are chainable.
+ * @example
+ *
+ * function capitalize(string) {
+ * return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase();
+ * }
+ *
+ * _.mixin({ 'capitalize': capitalize });
+ * _.capitalize('fred');
+ * // => 'Fred'
+ *
+ * _('fred').capitalize().value();
+ * // => 'Fred'
+ *
+ * _.mixin({ 'capitalize': capitalize }, { 'chain': false });
+ * _('fred').capitalize();
+ * // => 'Fred'
+ */
+function mixin(object, source) {
+ var ctor = object,
+ isFunc = isFunction(ctor);
+
+ forEach(functions(source), function(methodName) {
+ var func = object[methodName] = source[methodName];
+ if (isFunc) {
+ ctor.prototype[methodName] = function() {
+ var args = [this.__wrapped__];
+ push.apply(args, arguments);
+
+ var result = func.apply(object, args);
+ if (this.__chain__) {
+ result = new ctor(result);
+ result.__chain__ = true;
+ }
+ return result;
+ };
+ }
+ });
+}
+
+module.exports = mixin;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/noConflict.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/noConflict.js b/node_modules/lodash-node/underscore/utilities/noConflict.js
new file mode 100644
index 0000000..349a093
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/noConflict.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to restore the original `_` reference in `noConflict` */
+var oldDash = global._;
+
+/**
+ * Reverts the '_' variable to its previous value and returns a reference to
+ * the `lodash` function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @returns {Function} Returns the `lodash` function.
+ * @example
+ *
+ * var lodash = _.noConflict();
+ */
+function noConflict() {
+ global._ = oldDash;
+ return this;
+}
+
+module.exports = noConflict;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/noop.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/noop.js b/node_modules/lodash-node/underscore/utilities/noop.js
new file mode 100644
index 0000000..2756a24
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/noop.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * A no-operation function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * _.noop(object) === undefined;
+ * // => true
+ */
+function noop() {
+ // no operation performed
+}
+
+module.exports = noop;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/now.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/now.js b/node_modules/lodash-node/underscore/utilities/now.js
new file mode 100644
index 0000000..c340513
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/now.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative');
+
+/**
+ * Gets the number of milliseconds that have elapsed since the Unix epoch
+ * (1 January 1970 00:00:00 UTC).
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @example
+ *
+ * var stamp = _.now();
+ * _.defer(function() { console.log(_.now() - stamp); });
+ * // => logs the number of milliseconds it took for the deferred function to be called
+ */
+var now = isNative(now = Date.now) && now || function() {
+ return new Date().getTime();
+};
+
+module.exports = now;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/utilities/property.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/utilities/property.js b/node_modules/lodash-node/underscore/utilities/property.js
new file mode 100644
index 0000000..232d11d
--- /dev/null
+++ b/node_modules/lodash-node/underscore/utilities/property.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates a "_.pluck" style function, which returns the `key` value of a
+ * given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} key The name of the property to retrieve.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'barney', 'age': 36 }
+ * ];
+ *
+ * var getName = _.property('name');
+ *
+ * _.map(characters, getName);
+ * // => ['barney', 'fred']
+ *
+ * _.sortBy(characters, getName);
+ * // => [{ 'name': 'barney', 'age': 36 }, { 'name': 'fred', 'age': 40 }]
+ */
+function property(key) {
+ return function(object) {
+ return object[key];
+ };
+}
+
+module.exports = property;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[22/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/template.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/template.js b/node_modules/lodash/string/template.js
new file mode 100644
index 0000000..e75e992
--- /dev/null
+++ b/node_modules/lodash/string/template.js
@@ -0,0 +1,226 @@
+var assignOwnDefaults = require('../internal/assignOwnDefaults'),
+ assignWith = require('../internal/assignWith'),
+ attempt = require('../utility/attempt'),
+ baseAssign = require('../internal/baseAssign'),
+ baseToString = require('../internal/baseToString'),
+ baseValues = require('../internal/baseValues'),
+ escapeStringChar = require('../internal/escapeStringChar'),
+ isError = require('../lang/isError'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ keys = require('../object/keys'),
+ reInterpolate = require('../internal/reInterpolate'),
+ templateSettings = require('./templateSettings');
+
+/** Used to match empty string literals in compiled template source. */
+var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+/** Used to match [ES template delimiters](http://ecma-international.org/ecma-262/6.0/#sec-template-literal-lexical-components). */
+var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+/** Used to ensure capturing order of template delimiters. */
+var reNoMatch = /($^)/;
+
+/** Used to match unescaped characters in compiled string literals. */
+var reUnescapedString = /['\n\r\u2028\u2029\\]/g;
+
+/**
+ * Creates a compiled template function that can interpolate data properties
+ * in "interpolate" delimiters, HTML-escape interpolated data properties in
+ * "escape" delimiters, and execute JavaScript in "evaluate" delimiters. Data
+ * properties may be accessed as free variables in the template. If a setting
+ * object is provided it takes precedence over `_.templateSettings` values.
+ *
+ * **Note:** In the development build `_.template` utilizes
+ * [sourceURLs](http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl)
+ * for easier debugging.
+ *
+ * For more information on precompiling templates see
+ * [lodash's custom builds documentation](https://lodash.com/custom-builds).
+ *
+ * For more information on Chrome extension sandboxes see
+ * [Chrome's extensions documentation](https://developer.chrome.com/extensions/sandboxingEval).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The template string.
+ * @param {Object} [options] The options object.
+ * @param {RegExp} [options.escape] The HTML "escape" delimiter.
+ * @param {RegExp} [options.evaluate] The "evaluate" delimiter.
+ * @param {Object} [options.imports] An object to import into the template as free variables.
+ * @param {RegExp} [options.interpolate] The "interpolate" delimiter.
+ * @param {string} [options.sourceURL] The sourceURL of the template's compiled source.
+ * @param {string} [options.variable] The data object variable name.
+ * @param- {Object} [otherOptions] Enables the legacy `options` param signature.
+ * @returns {Function} Returns the compiled template function.
+ * @example
+ *
+ * // using the "interpolate" delimiter to create a compiled template
+ * var compiled = _.template('hello <%= user %>!');
+ * compiled({ 'user': 'fred' });
+ * // => 'hello fred!'
+ *
+ * // using the HTML "escape" delimiter to escape data property values
+ * var compiled = _.template('<b><%- value %></b>');
+ * compiled({ 'value': '<script>' });
+ * // => '<b><script></b>'
+ *
+ * // using the "evaluate" delimiter to execute JavaScript and generate HTML
+ * var compiled = _.template('<% _.forEach(users, function(user) { %><li><%- user %></li><% }); %>');
+ * compiled({ 'users': ['fred', 'barney'] });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the internal `print` function in "evaluate" delimiters
+ * var compiled = _.template('<% print("hello " + user); %>!');
+ * compiled({ 'user': 'barney' });
+ * // => 'hello barney!'
+ *
+ * // using the ES delimiter as an alternative to the default "interpolate" delimiter
+ * var compiled = _.template('hello ${ user }!');
+ * compiled({ 'user': 'pebbles' });
+ * // => 'hello pebbles!'
+ *
+ * // using custom template delimiters
+ * _.templateSettings.interpolate = /{{([\s\S]+?)}}/g;
+ * var compiled = _.template('hello {{ user }}!');
+ * compiled({ 'user': 'mustache' });
+ * // => 'hello mustache!'
+ *
+ * // using backslashes to treat delimiters as plain text
+ * var compiled = _.template('<%= "\\<%- value %\\>" %>');
+ * compiled({ 'value': 'ignored' });
+ * // => '<%- value %>'
+ *
+ * // using the `imports` option to import `jQuery` as `jq`
+ * var text = '<% jq.each(users, function(user) { %><li><%- user %></li><% }); %>';
+ * var compiled = _.template(text, { 'imports': { 'jq': jQuery } });
+ * compiled({ 'users': ['fred', 'barney'] });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the `sourceURL` option to specify a custom sourceURL for the template
+ * var compiled = _.template('hello <%= user %>!', { 'sourceURL': '/basic/greeting.jst' });
+ * compiled(data);
+ * // => find the source of "greeting.jst" under the Sources tab or Resources panel of the web inspector
+ *
+ * // using the `variable` option to ensure a with-statement isn't used in the compiled template
+ * var compiled = _.template('hi <%= data.user %>!', { 'variable': 'data' });
+ * compiled.source;
+ * // => function(data) {
+ * // var __t, __p = '';
+ * // __p += 'hi ' + ((__t = ( data.user )) == null ? '' : __t) + '!';
+ * // return __p;
+ * // }
+ *
+ * // using the `source` property to inline compiled templates for meaningful
+ * // line numbers in error messages and a stack trace
+ * fs.writeFileSync(path.join(cwd, 'jst.js'), '\
+ * var JST = {\
+ * "main": ' + _.template(mainText).source + '\
+ * };\
+ * ');
+ */
+function template(string, options, otherOptions) {
+ // Based on John Resig's `tmpl` implementation (http://ejohn.org/blog/javascript-micro-templating/)
+ // and Laura Doktorova's doT.js (https://github.com/olado/doT).
+ var settings = templateSettings.imports._.templateSettings || templateSettings;
+
+ if (otherOptions && isIterateeCall(string, options, otherOptions)) {
+ options = otherOptions = undefined;
+ }
+ string = baseToString(string);
+ options = assignWith(baseAssign({}, otherOptions || options), settings, assignOwnDefaults);
+
+ var imports = assignWith(baseAssign({}, options.imports), settings.imports, assignOwnDefaults),
+ importsKeys = keys(imports),
+ importsValues = baseValues(imports, importsKeys);
+
+ var isEscaping,
+ isEvaluating,
+ index = 0,
+ interpolate = options.interpolate || reNoMatch,
+ source = "__p += '";
+
+ // Compile the regexp to match each delimiter.
+ var reDelimiters = RegExp(
+ (options.escape || reNoMatch).source + '|' +
+ interpolate.source + '|' +
+ (interpolate === reInterpolate ? reEsTemplate : reNoMatch).source + '|' +
+ (options.evaluate || reNoMatch).source + '|$'
+ , 'g');
+
+ // Use a sourceURL for easier debugging.
+ var sourceURL = 'sourceURL' in options ? '//# sourceURL=' + options.sourceURL + '\n' : '';
+
+ string.replace(reDelimiters, function(match, escapeValue, interpolateValue, esTemplateValue, evaluateValue, offset) {
+ interpolateValue || (interpolateValue = esTemplateValue);
+
+ // Escape characters that can't be included in string literals.
+ source += string.slice(index, offset).replace(reUnescapedString, escapeStringChar);
+
+ // Replace delimiters with snippets.
+ if (escapeValue) {
+ isEscaping = true;
+ source += "' +\n__e(" + escapeValue + ") +\n'";
+ }
+ if (evaluateValue) {
+ isEvaluating = true;
+ source += "';\n" + evaluateValue + ";\n__p += '";
+ }
+ if (interpolateValue) {
+ source += "' +\n((__t = (" + interpolateValue + ")) == null ? '' : __t) +\n'";
+ }
+ index = offset + match.length;
+
+ // The JS engine embedded in Adobe products requires returning the `match`
+ // string in order to produce the correct `offset` value.
+ return match;
+ });
+
+ source += "';\n";
+
+ // If `variable` is not specified wrap a with-statement around the generated
+ // code to add the data object to the top of the scope chain.
+ var variable = options.variable;
+ if (!variable) {
+ source = 'with (obj) {\n' + source + '\n}\n';
+ }
+ // Cleanup code by stripping empty strings.
+ source = (isEvaluating ? source.replace(reEmptyStringLeading, '') : source)
+ .replace(reEmptyStringMiddle, '$1')
+ .replace(reEmptyStringTrailing, '$1;');
+
+ // Frame code as the function body.
+ source = 'function(' + (variable || 'obj') + ') {\n' +
+ (variable
+ ? ''
+ : 'obj || (obj = {});\n'
+ ) +
+ "var __t, __p = ''" +
+ (isEscaping
+ ? ', __e = _.escape'
+ : ''
+ ) +
+ (isEvaluating
+ ? ', __j = Array.prototype.join;\n' +
+ "function print() { __p += __j.call(arguments, '') }\n"
+ : ';\n'
+ ) +
+ source +
+ 'return __p\n}';
+
+ var result = attempt(function() {
+ return Function(importsKeys, sourceURL + 'return ' + source).apply(undefined, importsValues);
+ });
+
+ // Provide the compiled function's source by its `toString` method or
+ // the `source` property as a convenience for inlining compiled templates.
+ result.source = source;
+ if (isError(result)) {
+ throw result;
+ }
+ return result;
+}
+
+module.exports = template;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/templateSettings.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/templateSettings.js b/node_modules/lodash/string/templateSettings.js
new file mode 100644
index 0000000..cdcef9b
--- /dev/null
+++ b/node_modules/lodash/string/templateSettings.js
@@ -0,0 +1,67 @@
+var escape = require('./escape'),
+ reEscape = require('../internal/reEscape'),
+ reEvaluate = require('../internal/reEvaluate'),
+ reInterpolate = require('../internal/reInterpolate');
+
+/**
+ * By default, the template delimiters used by lodash are like those in
+ * embedded Ruby (ERB). Change the following template settings to use
+ * alternative delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'escape': reEscape,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'evaluate': reEvaluate,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type string
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type Object
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type Function
+ */
+ '_': { 'escape': escape }
+ }
+};
+
+module.exports = templateSettings;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/trim.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/trim.js b/node_modules/lodash/string/trim.js
new file mode 100644
index 0000000..22cd38a
--- /dev/null
+++ b/node_modules/lodash/string/trim.js
@@ -0,0 +1,42 @@
+var baseToString = require('../internal/baseToString'),
+ charsLeftIndex = require('../internal/charsLeftIndex'),
+ charsRightIndex = require('../internal/charsRightIndex'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ trimmedLeftIndex = require('../internal/trimmedLeftIndex'),
+ trimmedRightIndex = require('../internal/trimmedRightIndex');
+
+/**
+ * Removes leading and trailing whitespace or specified characters from `string`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to trim.
+ * @param {string} [chars=whitespace] The characters to trim.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {string} Returns the trimmed string.
+ * @example
+ *
+ * _.trim(' abc ');
+ * // => 'abc'
+ *
+ * _.trim('-_-abc-_-', '_-');
+ * // => 'abc'
+ *
+ * _.map([' foo ', ' bar '], _.trim);
+ * // => ['foo', 'bar']
+ */
+function trim(string, chars, guard) {
+ var value = string;
+ string = baseToString(string);
+ if (!string) {
+ return string;
+ }
+ if (guard ? isIterateeCall(value, chars, guard) : chars == null) {
+ return string.slice(trimmedLeftIndex(string), trimmedRightIndex(string) + 1);
+ }
+ chars = (chars + '');
+ return string.slice(charsLeftIndex(string, chars), charsRightIndex(string, chars) + 1);
+}
+
+module.exports = trim;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/trimLeft.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/trimLeft.js b/node_modules/lodash/string/trimLeft.js
new file mode 100644
index 0000000..2929967
--- /dev/null
+++ b/node_modules/lodash/string/trimLeft.js
@@ -0,0 +1,36 @@
+var baseToString = require('../internal/baseToString'),
+ charsLeftIndex = require('../internal/charsLeftIndex'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ trimmedLeftIndex = require('../internal/trimmedLeftIndex');
+
+/**
+ * Removes leading whitespace or specified characters from `string`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to trim.
+ * @param {string} [chars=whitespace] The characters to trim.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {string} Returns the trimmed string.
+ * @example
+ *
+ * _.trimLeft(' abc ');
+ * // => 'abc '
+ *
+ * _.trimLeft('-_-abc-_-', '_-');
+ * // => 'abc-_-'
+ */
+function trimLeft(string, chars, guard) {
+ var value = string;
+ string = baseToString(string);
+ if (!string) {
+ return string;
+ }
+ if (guard ? isIterateeCall(value, chars, guard) : chars == null) {
+ return string.slice(trimmedLeftIndex(string));
+ }
+ return string.slice(charsLeftIndex(string, (chars + '')));
+}
+
+module.exports = trimLeft;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/trimRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/trimRight.js b/node_modules/lodash/string/trimRight.js
new file mode 100644
index 0000000..0f9be71
--- /dev/null
+++ b/node_modules/lodash/string/trimRight.js
@@ -0,0 +1,36 @@
+var baseToString = require('../internal/baseToString'),
+ charsRightIndex = require('../internal/charsRightIndex'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ trimmedRightIndex = require('../internal/trimmedRightIndex');
+
+/**
+ * Removes trailing whitespace or specified characters from `string`.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to trim.
+ * @param {string} [chars=whitespace] The characters to trim.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {string} Returns the trimmed string.
+ * @example
+ *
+ * _.trimRight(' abc ');
+ * // => ' abc'
+ *
+ * _.trimRight('-_-abc-_-', '_-');
+ * // => '-_-abc'
+ */
+function trimRight(string, chars, guard) {
+ var value = string;
+ string = baseToString(string);
+ if (!string) {
+ return string;
+ }
+ if (guard ? isIterateeCall(value, chars, guard) : chars == null) {
+ return string.slice(0, trimmedRightIndex(string) + 1);
+ }
+ return string.slice(0, charsRightIndex(string, (chars + '')) + 1);
+}
+
+module.exports = trimRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/trunc.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/trunc.js b/node_modules/lodash/string/trunc.js
new file mode 100644
index 0000000..29e1939
--- /dev/null
+++ b/node_modules/lodash/string/trunc.js
@@ -0,0 +1,105 @@
+var baseToString = require('../internal/baseToString'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ isObject = require('../lang/isObject'),
+ isRegExp = require('../lang/isRegExp');
+
+/** Used as default options for `_.trunc`. */
+var DEFAULT_TRUNC_LENGTH = 30,
+ DEFAULT_TRUNC_OMISSION = '...';
+
+/** Used to match `RegExp` flags from their coerced string values. */
+var reFlags = /\w*$/;
+
+/**
+ * Truncates `string` if it's longer than the given maximum string length.
+ * The last characters of the truncated string are replaced with the omission
+ * string which defaults to "...".
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to truncate.
+ * @param {Object|number} [options] The options object or maximum string length.
+ * @param {number} [options.length=30] The maximum string length.
+ * @param {string} [options.omission='...'] The string to indicate text is omitted.
+ * @param {RegExp|string} [options.separator] The separator pattern to truncate to.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {string} Returns the truncated string.
+ * @example
+ *
+ * _.trunc('hi-diddly-ho there, neighborino');
+ * // => 'hi-diddly-ho there, neighbo...'
+ *
+ * _.trunc('hi-diddly-ho there, neighborino', 24);
+ * // => 'hi-diddly-ho there, n...'
+ *
+ * _.trunc('hi-diddly-ho there, neighborino', {
+ * 'length': 24,
+ * 'separator': ' '
+ * });
+ * // => 'hi-diddly-ho there,...'
+ *
+ * _.trunc('hi-diddly-ho there, neighborino', {
+ * 'length': 24,
+ * 'separator': /,? +/
+ * });
+ * // => 'hi-diddly-ho there...'
+ *
+ * _.trunc('hi-diddly-ho there, neighborino', {
+ * 'omission': ' [...]'
+ * });
+ * // => 'hi-diddly-ho there, neig [...]'
+ */
+function trunc(string, options, guard) {
+ if (guard && isIterateeCall(string, options, guard)) {
+ options = undefined;
+ }
+ var length = DEFAULT_TRUNC_LENGTH,
+ omission = DEFAULT_TRUNC_OMISSION;
+
+ if (options != null) {
+ if (isObject(options)) {
+ var separator = 'separator' in options ? options.separator : separator;
+ length = 'length' in options ? (+options.length || 0) : length;
+ omission = 'omission' in options ? baseToString(options.omission) : omission;
+ } else {
+ length = +options || 0;
+ }
+ }
+ string = baseToString(string);
+ if (length >= string.length) {
+ return string;
+ }
+ var end = length - omission.length;
+ if (end < 1) {
+ return omission;
+ }
+ var result = string.slice(0, end);
+ if (separator == null) {
+ return result + omission;
+ }
+ if (isRegExp(separator)) {
+ if (string.slice(end).search(separator)) {
+ var match,
+ newEnd,
+ substring = string.slice(0, end);
+
+ if (!separator.global) {
+ separator = RegExp(separator.source, (reFlags.exec(separator) || '') + 'g');
+ }
+ separator.lastIndex = 0;
+ while ((match = separator.exec(substring))) {
+ newEnd = match.index;
+ }
+ result = result.slice(0, newEnd == null ? end : newEnd);
+ }
+ } else if (string.indexOf(separator, end) != end) {
+ var index = result.lastIndexOf(separator);
+ if (index > -1) {
+ result = result.slice(0, index);
+ }
+ }
+ return result + omission;
+}
+
+module.exports = trunc;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/unescape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/unescape.js b/node_modules/lodash/string/unescape.js
new file mode 100644
index 0000000..b0266a7
--- /dev/null
+++ b/node_modules/lodash/string/unescape.js
@@ -0,0 +1,33 @@
+var baseToString = require('../internal/baseToString'),
+ unescapeHtmlChar = require('../internal/unescapeHtmlChar');
+
+/** Used to match HTML entities and HTML characters. */
+var reEscapedHtml = /&(?:amp|lt|gt|quot|#39|#96);/g,
+ reHasEscapedHtml = RegExp(reEscapedHtml.source);
+
+/**
+ * The inverse of `_.escape`; this method converts the HTML entities
+ * `&`, `<`, `>`, `"`, `'`, and ``` in `string` to their
+ * corresponding characters.
+ *
+ * **Note:** No other HTML entities are unescaped. To unescape additional HTML
+ * entities use a third-party library like [_he_](https://mths.be/he).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to unescape.
+ * @returns {string} Returns the unescaped string.
+ * @example
+ *
+ * _.unescape('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles'
+ */
+function unescape(string) {
+ string = baseToString(string);
+ return (string && reHasEscapedHtml.test(string))
+ ? string.replace(reEscapedHtml, unescapeHtmlChar)
+ : string;
+}
+
+module.exports = unescape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/string/words.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/string/words.js b/node_modules/lodash/string/words.js
new file mode 100644
index 0000000..3013ad6
--- /dev/null
+++ b/node_modules/lodash/string/words.js
@@ -0,0 +1,38 @@
+var baseToString = require('../internal/baseToString'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/** Used to match words to create compound words. */
+var reWords = (function() {
+ var upper = '[A-Z\\xc0-\\xd6\\xd8-\\xde]',
+ lower = '[a-z\\xdf-\\xf6\\xf8-\\xff]+';
+
+ return RegExp(upper + '+(?=' + upper + lower + ')|' + upper + '?' + lower + '|' + upper + '+|[0-9]+', 'g');
+}());
+
+/**
+ * Splits `string` into an array of its words.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to inspect.
+ * @param {RegExp|string} [pattern] The pattern to match words.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Array} Returns the words of `string`.
+ * @example
+ *
+ * _.words('fred, barney, & pebbles');
+ * // => ['fred', 'barney', 'pebbles']
+ *
+ * _.words('fred, barney, & pebbles', /[^, ]+/g);
+ * // => ['fred', 'barney', '&', 'pebbles']
+ */
+function words(string, pattern, guard) {
+ if (guard && isIterateeCall(string, pattern, guard)) {
+ pattern = undefined;
+ }
+ string = baseToString(string);
+ return string.match(pattern || reWords) || [];
+}
+
+module.exports = words;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/support.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/support.js b/node_modules/lodash/support.js
new file mode 100644
index 0000000..5009c2c
--- /dev/null
+++ b/node_modules/lodash/support.js
@@ -0,0 +1,10 @@
+/**
+ * An object environment feature flags.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var support = {};
+
+module.exports = support;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility.js b/node_modules/lodash/utility.js
new file mode 100644
index 0000000..25311fa
--- /dev/null
+++ b/node_modules/lodash/utility.js
@@ -0,0 +1,18 @@
+module.exports = {
+ 'attempt': require('./utility/attempt'),
+ 'callback': require('./utility/callback'),
+ 'constant': require('./utility/constant'),
+ 'identity': require('./utility/identity'),
+ 'iteratee': require('./utility/iteratee'),
+ 'matches': require('./utility/matches'),
+ 'matchesProperty': require('./utility/matchesProperty'),
+ 'method': require('./utility/method'),
+ 'methodOf': require('./utility/methodOf'),
+ 'mixin': require('./utility/mixin'),
+ 'noop': require('./utility/noop'),
+ 'property': require('./utility/property'),
+ 'propertyOf': require('./utility/propertyOf'),
+ 'range': require('./utility/range'),
+ 'times': require('./utility/times'),
+ 'uniqueId': require('./utility/uniqueId')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/attempt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/attempt.js b/node_modules/lodash/utility/attempt.js
new file mode 100644
index 0000000..8d8fb98
--- /dev/null
+++ b/node_modules/lodash/utility/attempt.js
@@ -0,0 +1,32 @@
+var isError = require('../lang/isError'),
+ restParam = require('../function/restParam');
+
+/**
+ * Attempts to invoke `func`, returning either the result or the caught error
+ * object. Any additional arguments are provided to `func` when it's invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Function} func The function to attempt.
+ * @returns {*} Returns the `func` result or error object.
+ * @example
+ *
+ * // avoid throwing errors for invalid selectors
+ * var elements = _.attempt(function(selector) {
+ * return document.querySelectorAll(selector);
+ * }, '>_>');
+ *
+ * if (_.isError(elements)) {
+ * elements = [];
+ * }
+ */
+var attempt = restParam(function(func, args) {
+ try {
+ return func.apply(undefined, args);
+ } catch(e) {
+ return isError(e) ? e : new Error(e);
+ }
+});
+
+module.exports = attempt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/callback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/callback.js b/node_modules/lodash/utility/callback.js
new file mode 100644
index 0000000..21223d0
--- /dev/null
+++ b/node_modules/lodash/utility/callback.js
@@ -0,0 +1,53 @@
+var baseCallback = require('../internal/baseCallback'),
+ isIterateeCall = require('../internal/isIterateeCall'),
+ isObjectLike = require('../internal/isObjectLike'),
+ matches = require('./matches');
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of `thisArg`
+ * and arguments of the created function. If `func` is a property name the
+ * created callback returns the property value for a given element. If `func`
+ * is an object the created callback returns `true` for elements that contain
+ * the equivalent object properties, otherwise it returns `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias iteratee
+ * @category Utility
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param- {Object} [guard] Enables use as a callback for functions like `_.map`.
+ * @returns {Function} Returns the callback.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36 },
+ * { 'user': 'fred', 'age': 40 }
+ * ];
+ *
+ * // wrap to create custom callback shorthands
+ * _.callback = _.wrap(_.callback, function(callback, func, thisArg) {
+ * var match = /^(.+?)__([gl]t)(.+)$/.exec(func);
+ * if (!match) {
+ * return callback(func, thisArg);
+ * }
+ * return function(object) {
+ * return match[2] == 'gt'
+ * ? object[match[1]] > match[3]
+ * : object[match[1]] < match[3];
+ * };
+ * });
+ *
+ * _.filter(users, 'age__gt36');
+ * // => [{ 'user': 'fred', 'age': 40 }]
+ */
+function callback(func, thisArg, guard) {
+ if (guard && isIterateeCall(func, thisArg, guard)) {
+ thisArg = undefined;
+ }
+ return isObjectLike(func)
+ ? matches(func)
+ : baseCallback(func, thisArg);
+}
+
+module.exports = callback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/constant.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/constant.js b/node_modules/lodash/utility/constant.js
new file mode 100644
index 0000000..6919b76
--- /dev/null
+++ b/node_modules/lodash/utility/constant.js
@@ -0,0 +1,23 @@
+/**
+ * Creates a function that returns `value`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {*} value The value to return from the new function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var object = { 'user': 'fred' };
+ * var getter = _.constant(object);
+ *
+ * getter() === object;
+ * // => true
+ */
+function constant(value) {
+ return function() {
+ return value;
+ };
+}
+
+module.exports = constant;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/identity.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/identity.js b/node_modules/lodash/utility/identity.js
new file mode 100644
index 0000000..3a1d1d4
--- /dev/null
+++ b/node_modules/lodash/utility/identity.js
@@ -0,0 +1,20 @@
+/**
+ * This method returns the first argument provided to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * _.identity(object) === object;
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+module.exports = identity;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/iteratee.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/iteratee.js b/node_modules/lodash/utility/iteratee.js
new file mode 100644
index 0000000..fcfa202
--- /dev/null
+++ b/node_modules/lodash/utility/iteratee.js
@@ -0,0 +1 @@
+module.exports = require('./callback');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/matches.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/matches.js b/node_modules/lodash/utility/matches.js
new file mode 100644
index 0000000..a182637
--- /dev/null
+++ b/node_modules/lodash/utility/matches.js
@@ -0,0 +1,33 @@
+var baseClone = require('../internal/baseClone'),
+ baseMatches = require('../internal/baseMatches');
+
+/**
+ * Creates a function that performs a deep comparison between a given object
+ * and `source`, returning `true` if the given object has equivalent property
+ * values, else `false`.
+ *
+ * **Note:** This method supports comparing arrays, booleans, `Date` objects,
+ * numbers, `Object` objects, regexes, and strings. Objects are compared by
+ * their own, not inherited, enumerable properties. For comparing a single
+ * own or inherited property value see `_.matchesProperty`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney', 'age': 36, 'active': true },
+ * { 'user': 'fred', 'age': 40, 'active': false }
+ * ];
+ *
+ * _.filter(users, _.matches({ 'age': 40, 'active': false }));
+ * // => [{ 'user': 'fred', 'age': 40, 'active': false }]
+ */
+function matches(source) {
+ return baseMatches(baseClone(source, true));
+}
+
+module.exports = matches;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/matchesProperty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/matchesProperty.js b/node_modules/lodash/utility/matchesProperty.js
new file mode 100644
index 0000000..91e51a5
--- /dev/null
+++ b/node_modules/lodash/utility/matchesProperty.js
@@ -0,0 +1,32 @@
+var baseClone = require('../internal/baseClone'),
+ baseMatchesProperty = require('../internal/baseMatchesProperty');
+
+/**
+ * Creates a function that compares the property value of `path` on a given
+ * object to `value`.
+ *
+ * **Note:** This method supports comparing arrays, booleans, `Date` objects,
+ * numbers, `Object` objects, regexes, and strings. Objects are compared by
+ * their own, not inherited, enumerable properties.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Array|string} path The path of the property to get.
+ * @param {*} srcValue The value to match.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var users = [
+ * { 'user': 'barney' },
+ * { 'user': 'fred' }
+ * ];
+ *
+ * _.find(users, _.matchesProperty('user', 'fred'));
+ * // => { 'user': 'fred' }
+ */
+function matchesProperty(path, srcValue) {
+ return baseMatchesProperty(path, baseClone(srcValue, true));
+}
+
+module.exports = matchesProperty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/method.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/method.js b/node_modules/lodash/utility/method.js
new file mode 100644
index 0000000..e315b7f
--- /dev/null
+++ b/node_modules/lodash/utility/method.js
@@ -0,0 +1,33 @@
+var invokePath = require('../internal/invokePath'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates a function that invokes the method at `path` on a given object.
+ * Any additional arguments are provided to the invoked method.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Array|string} path The path of the method to invoke.
+ * @param {...*} [args] The arguments to invoke the method with.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var objects = [
+ * { 'a': { 'b': { 'c': _.constant(2) } } },
+ * { 'a': { 'b': { 'c': _.constant(1) } } }
+ * ];
+ *
+ * _.map(objects, _.method('a.b.c'));
+ * // => [2, 1]
+ *
+ * _.invoke(_.sortBy(objects, _.method(['a', 'b', 'c'])), 'a.b.c');
+ * // => [1, 2]
+ */
+var method = restParam(function(path, args) {
+ return function(object) {
+ return invokePath(object, path, args);
+ };
+});
+
+module.exports = method;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/methodOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/methodOf.js b/node_modules/lodash/utility/methodOf.js
new file mode 100644
index 0000000..f61b782
--- /dev/null
+++ b/node_modules/lodash/utility/methodOf.js
@@ -0,0 +1,32 @@
+var invokePath = require('../internal/invokePath'),
+ restParam = require('../function/restParam');
+
+/**
+ * The opposite of `_.method`; this method creates a function that invokes
+ * the method at a given path on `object`. Any additional arguments are
+ * provided to the invoked method.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Object} object The object to query.
+ * @param {...*} [args] The arguments to invoke the method with.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var array = _.times(3, _.constant),
+ * object = { 'a': array, 'b': array, 'c': array };
+ *
+ * _.map(['a[2]', 'c[0]'], _.methodOf(object));
+ * // => [2, 0]
+ *
+ * _.map([['a', '2'], ['c', '0']], _.methodOf(object));
+ * // => [2, 0]
+ */
+var methodOf = restParam(function(object, args) {
+ return function(path) {
+ return invokePath(object, path, args);
+ };
+});
+
+module.exports = methodOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/mixin.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/mixin.js b/node_modules/lodash/utility/mixin.js
new file mode 100644
index 0000000..5c4a372
--- /dev/null
+++ b/node_modules/lodash/utility/mixin.js
@@ -0,0 +1,82 @@
+var arrayCopy = require('../internal/arrayCopy'),
+ arrayPush = require('../internal/arrayPush'),
+ baseFunctions = require('../internal/baseFunctions'),
+ isFunction = require('../lang/isFunction'),
+ isObject = require('../lang/isObject'),
+ keys = require('../object/keys');
+
+/**
+ * Adds all own enumerable function properties of a source object to the
+ * destination object. If `object` is a function then methods are added to
+ * its prototype as well.
+ *
+ * **Note:** Use `_.runInContext` to create a pristine `lodash` function to
+ * avoid conflicts caused by modifying the original.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Function|Object} [object=lodash] The destination object.
+ * @param {Object} source The object of functions to add.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.chain=true] Specify whether the functions added
+ * are chainable.
+ * @returns {Function|Object} Returns `object`.
+ * @example
+ *
+ * function vowels(string) {
+ * return _.filter(string, function(v) {
+ * return /[aeiou]/i.test(v);
+ * });
+ * }
+ *
+ * _.mixin({ 'vowels': vowels });
+ * _.vowels('fred');
+ * // => ['e']
+ *
+ * _('fred').vowels().value();
+ * // => ['e']
+ *
+ * _.mixin({ 'vowels': vowels }, { 'chain': false });
+ * _('fred').vowels();
+ * // => ['e']
+ */
+function mixin(object, source, options) {
+ var methodNames = baseFunctions(source, keys(source));
+
+ var chain = true,
+ index = -1,
+ isFunc = isFunction(object),
+ length = methodNames.length;
+
+ if (options === false) {
+ chain = false;
+ } else if (isObject(options) && 'chain' in options) {
+ chain = options.chain;
+ }
+ while (++index < length) {
+ var methodName = methodNames[index],
+ func = source[methodName];
+
+ object[methodName] = func;
+ if (isFunc) {
+ object.prototype[methodName] = (function(func) {
+ return function() {
+ var chainAll = this.__chain__;
+ if (chain || chainAll) {
+ var result = object(this.__wrapped__),
+ actions = result.__actions__ = arrayCopy(this.__actions__);
+
+ actions.push({ 'func': func, 'args': arguments, 'thisArg': object });
+ result.__chain__ = chainAll;
+ return result;
+ }
+ return func.apply(object, arrayPush([this.value()], arguments));
+ };
+ }(func));
+ }
+ }
+ return object;
+}
+
+module.exports = mixin;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/noop.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/noop.js b/node_modules/lodash/utility/noop.js
new file mode 100644
index 0000000..56d6513
--- /dev/null
+++ b/node_modules/lodash/utility/noop.js
@@ -0,0 +1,19 @@
+/**
+ * A no-operation function that returns `undefined` regardless of the
+ * arguments it receives.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @example
+ *
+ * var object = { 'user': 'fred' };
+ *
+ * _.noop(object) === undefined;
+ * // => true
+ */
+function noop() {
+ // No operation performed.
+}
+
+module.exports = noop;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/property.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/property.js b/node_modules/lodash/utility/property.js
new file mode 100644
index 0000000..ddfd597
--- /dev/null
+++ b/node_modules/lodash/utility/property.js
@@ -0,0 +1,31 @@
+var baseProperty = require('../internal/baseProperty'),
+ basePropertyDeep = require('../internal/basePropertyDeep'),
+ isKey = require('../internal/isKey');
+
+/**
+ * Creates a function that returns the property value at `path` on a
+ * given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var objects = [
+ * { 'a': { 'b': { 'c': 2 } } },
+ * { 'a': { 'b': { 'c': 1 } } }
+ * ];
+ *
+ * _.map(objects, _.property('a.b.c'));
+ * // => [2, 1]
+ *
+ * _.pluck(_.sortBy(objects, _.property(['a', 'b', 'c'])), 'a.b.c');
+ * // => [1, 2]
+ */
+function property(path) {
+ return isKey(path) ? baseProperty(path) : basePropertyDeep(path);
+}
+
+module.exports = property;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/propertyOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/propertyOf.js b/node_modules/lodash/utility/propertyOf.js
new file mode 100644
index 0000000..593a266
--- /dev/null
+++ b/node_modules/lodash/utility/propertyOf.js
@@ -0,0 +1,30 @@
+var baseGet = require('../internal/baseGet'),
+ toPath = require('../internal/toPath');
+
+/**
+ * The opposite of `_.property`; this method creates a function that returns
+ * the property value at a given path on `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {Object} object The object to query.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var array = [0, 1, 2],
+ * object = { 'a': array, 'b': array, 'c': array };
+ *
+ * _.map(['a[2]', 'c[0]'], _.propertyOf(object));
+ * // => [2, 0]
+ *
+ * _.map([['a', '2'], ['c', '0']], _.propertyOf(object));
+ * // => [2, 0]
+ */
+function propertyOf(object) {
+ return function(path) {
+ return baseGet(object, toPath(path), (path + ''));
+ };
+}
+
+module.exports = propertyOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/range.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/range.js b/node_modules/lodash/utility/range.js
new file mode 100644
index 0000000..671939a
--- /dev/null
+++ b/node_modules/lodash/utility/range.js
@@ -0,0 +1,66 @@
+var isIterateeCall = require('../internal/isIterateeCall');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil,
+ nativeMax = Math.max;
+
+/**
+ * Creates an array of numbers (positive and/or negative) progressing from
+ * `start` up to, but not including, `end`. If `end` is not specified it's
+ * set to `start` with `start` then set to `0`. If `end` is less than `start`
+ * a zero-length range is created unless a negative `step` is specified.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @param {number} [step=1] The value to increment or decrement by.
+ * @returns {Array} Returns the new array of numbers.
+ * @example
+ *
+ * _.range(4);
+ * // => [0, 1, 2, 3]
+ *
+ * _.range(1, 5);
+ * // => [1, 2, 3, 4]
+ *
+ * _.range(0, 20, 5);
+ * // => [0, 5, 10, 15]
+ *
+ * _.range(0, -4, -1);
+ * // => [0, -1, -2, -3]
+ *
+ * _.range(1, 4, 0);
+ * // => [1, 1, 1]
+ *
+ * _.range(0);
+ * // => []
+ */
+function range(start, end, step) {
+ if (step && isIterateeCall(start, end, step)) {
+ end = step = undefined;
+ }
+ start = +start || 0;
+ step = step == null ? 1 : (+step || 0);
+
+ if (end == null) {
+ end = start;
+ start = 0;
+ } else {
+ end = +end || 0;
+ }
+ // Use `Array(length)` so engines like Chakra and V8 avoid slower modes.
+ // See https://youtu.be/XAqIpGU8ZZk#t=17m25s for more details.
+ var index = -1,
+ length = nativeMax(nativeCeil((end - start) / (step || 1)), 0),
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = start;
+ start += step;
+ }
+ return result;
+}
+
+module.exports = range;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/times.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/times.js b/node_modules/lodash/utility/times.js
new file mode 100644
index 0000000..9a41e2f
--- /dev/null
+++ b/node_modules/lodash/utility/times.js
@@ -0,0 +1,60 @@
+var bindCallback = require('../internal/bindCallback');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor,
+ nativeIsFinite = global.isFinite,
+ nativeMin = Math.min;
+
+/** Used as references for the maximum length and index of an array. */
+var MAX_ARRAY_LENGTH = 4294967295;
+
+/**
+ * Invokes the iteratee function `n` times, returning an array of the results
+ * of each invocation. The `iteratee` is bound to `thisArg` and invoked with
+ * one argument; (index).
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {number} n The number of times to invoke `iteratee`.
+ * @param {Function} [iteratee=_.identity] The function invoked per iteration.
+ * @param {*} [thisArg] The `this` binding of `iteratee`.
+ * @returns {Array} Returns the array of results.
+ * @example
+ *
+ * var diceRolls = _.times(3, _.partial(_.random, 1, 6, false));
+ * // => [3, 6, 4]
+ *
+ * _.times(3, function(n) {
+ * mage.castSpell(n);
+ * });
+ * // => invokes `mage.castSpell(n)` three times with `n` of `0`, `1`, and `2`
+ *
+ * _.times(3, function(n) {
+ * this.cast(n);
+ * }, mage);
+ * // => also invokes `mage.castSpell(n)` three times
+ */
+function times(n, iteratee, thisArg) {
+ n = nativeFloor(n);
+
+ // Exit early to avoid a JSC JIT bug in Safari 8
+ // where `Array(0)` is treated as `Array(1)`.
+ if (n < 1 || !nativeIsFinite(n)) {
+ return [];
+ }
+ var index = -1,
+ result = Array(nativeMin(n, MAX_ARRAY_LENGTH));
+
+ iteratee = bindCallback(iteratee, thisArg, 1);
+ while (++index < n) {
+ if (index < MAX_ARRAY_LENGTH) {
+ result[index] = iteratee(index);
+ } else {
+ iteratee(index);
+ }
+ }
+ return result;
+}
+
+module.exports = times;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/utility/uniqueId.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/utility/uniqueId.js b/node_modules/lodash/utility/uniqueId.js
new file mode 100644
index 0000000..88e02bf
--- /dev/null
+++ b/node_modules/lodash/utility/uniqueId.js
@@ -0,0 +1,27 @@
+var baseToString = require('../internal/baseToString');
+
+/** Used to generate unique IDs. */
+var idCounter = 0;
+
+/**
+ * Generates a unique ID. If `prefix` is provided the ID is appended to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utility
+ * @param {string} [prefix] The value to prefix the ID with.
+ * @returns {string} Returns the unique ID.
+ * @example
+ *
+ * _.uniqueId('contact_');
+ * // => 'contact_104'
+ *
+ * _.uniqueId();
+ * // => '105'
+ */
+function uniqueId(prefix) {
+ var id = ++idCounter;
+ return baseToString(prefix) + id;
+}
+
+module.exports = uniqueId;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/minimatch/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/minimatch/LICENSE b/node_modules/minimatch/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/minimatch/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/minimatch/README.md
----------------------------------------------------------------------
diff --git a/node_modules/minimatch/README.md b/node_modules/minimatch/README.md
new file mode 100644
index 0000000..d458bc2
--- /dev/null
+++ b/node_modules/minimatch/README.md
@@ -0,0 +1,216 @@
+# minimatch
+
+A minimal matching utility.
+
+[](http://travis-ci.org/isaacs/minimatch)
+
+
+This is the matching library used internally by npm.
+
+It works by converting glob expressions into JavaScript `RegExp`
+objects.
+
+## Usage
+
+```javascript
+var minimatch = require("minimatch")
+
+minimatch("bar.foo", "*.foo") // true!
+minimatch("bar.foo", "*.bar") // false!
+minimatch("bar.foo", "*.+(bar|foo)", { debug: true }) // true, and noisy!
+```
+
+## Features
+
+Supports these glob features:
+
+* Brace Expansion
+* Extended glob matching
+* "Globstar" `**` matching
+
+See:
+
+* `man sh`
+* `man bash`
+* `man 3 fnmatch`
+* `man 5 gitignore`
+
+## Minimatch Class
+
+Create a minimatch object by instanting the `minimatch.Minimatch` class.
+
+```javascript
+var Minimatch = require("minimatch").Minimatch
+var mm = new Minimatch(pattern, options)
+```
+
+### Properties
+
+* `pattern` The original pattern the minimatch object represents.
+* `options` The options supplied to the constructor.
+* `set` A 2-dimensional array of regexp or string expressions.
+ Each row in the
+ array corresponds to a brace-expanded pattern. Each item in the row
+ corresponds to a single path-part. For example, the pattern
+ `{a,b/c}/d` would expand to a set of patterns like:
+
+ [ [ a, d ]
+ , [ b, c, d ] ]
+
+ If a portion of the pattern doesn't have any "magic" in it
+ (that is, it's something like `"foo"` rather than `fo*o?`), then it
+ will be left as a string rather than converted to a regular
+ expression.
+
+* `regexp` Created by the `makeRe` method. A single regular expression
+ expressing the entire pattern. This is useful in cases where you wish
+ to use the pattern somewhat like `fnmatch(3)` with `FNM_PATH` enabled.
+* `negate` True if the pattern is negated.
+* `comment` True if the pattern is a comment.
+* `empty` True if the pattern is `""`.
+
+### Methods
+
+* `makeRe` Generate the `regexp` member if necessary, and return it.
+ Will return `false` if the pattern is invalid.
+* `match(fname)` Return true if the filename matches the pattern, or
+ false otherwise.
+* `matchOne(fileArray, patternArray, partial)` Take a `/`-split
+ filename, and match it against a single row in the `regExpSet`. This
+ method is mainly for internal use, but is exposed so that it can be
+ used by a glob-walker that needs to avoid excessive filesystem calls.
+
+All other methods are internal, and will be called as necessary.
+
+## Functions
+
+The top-level exported function has a `cache` property, which is an LRU
+cache set to store 100 items. So, calling these methods repeatedly
+with the same pattern and options will use the same Minimatch object,
+saving the cost of parsing it multiple times.
+
+### minimatch(path, pattern, options)
+
+Main export. Tests a path against the pattern using the options.
+
+```javascript
+var isJS = minimatch(file, "*.js", { matchBase: true })
+```
+
+### minimatch.filter(pattern, options)
+
+Returns a function that tests its
+supplied argument, suitable for use with `Array.filter`. Example:
+
+```javascript
+var javascripts = fileList.filter(minimatch.filter("*.js", {matchBase: true}))
+```
+
+### minimatch.match(list, pattern, options)
+
+Match against the list of
+files, in the style of fnmatch or glob. If nothing is matched, and
+options.nonull is set, then return a list containing the pattern itself.
+
+```javascript
+var javascripts = minimatch.match(fileList, "*.js", {matchBase: true}))
+```
+
+### minimatch.makeRe(pattern, options)
+
+Make a regular expression object from the pattern.
+
+## Options
+
+All options are `false` by default.
+
+### debug
+
+Dump a ton of stuff to stderr.
+
+### nobrace
+
+Do not expand `{a,b}` and `{1..3}` brace sets.
+
+### noglobstar
+
+Disable `**` matching against multiple folder names.
+
+### dot
+
+Allow patterns to match filenames starting with a period, even if
+the pattern does not explicitly have a period in that spot.
+
+Note that by default, `a/**/b` will **not** match `a/.d/b`, unless `dot`
+is set.
+
+### noext
+
+Disable "extglob" style patterns like `+(a|b)`.
+
+### nocase
+
+Perform a case-insensitive match.
+
+### nonull
+
+When a match is not found by `minimatch.match`, return a list containing
+the pattern itself if this option is set. When not set, an empty list
+is returned if there are no matches.
+
+### matchBase
+
+If set, then patterns without slashes will be matched
+against the basename of the path if it contains slashes. For example,
+`a?b` would match the path `/xyz/123/acb`, but not `/xyz/acb/123`.
+
+### nocomment
+
+Suppress the behavior of treating `#` at the start of a pattern as a
+comment.
+
+### nonegate
+
+Suppress the behavior of treating a leading `!` character as negation.
+
+### flipNegate
+
+Returns from negate expressions the same as if they were not negated.
+(Ie, true on a hit, false on a miss.)
+
+
+## Comparisons to other fnmatch/glob implementations
+
+While strict compliance with the existing standards is a worthwhile
+goal, some discrepancies exist between minimatch and other
+implementations, and are intentional.
+
+If the pattern starts with a `!` character, then it is negated. Set the
+`nonegate` flag to suppress this behavior, and treat leading `!`
+characters normally. This is perhaps relevant if you wish to start the
+pattern with a negative extglob pattern like `!(a|B)`. Multiple `!`
+characters at the start of a pattern will negate the pattern multiple
+times.
+
+If a pattern starts with `#`, then it is treated as a comment, and
+will not match anything. Use `\#` to match a literal `#` at the
+start of a line, or set the `nocomment` flag to suppress this behavior.
+
+The double-star character `**` is supported by default, unless the
+`noglobstar` flag is set. This is supported in the manner of bsdglob
+and bash 4.1, where `**` only has special significance if it is the only
+thing in a path part. That is, `a/**/b` will match `a/x/y/b`, but
+`a/**b` will not.
+
+If an escaped pattern has no matches, and the `nonull` flag is set,
+then minimatch.match returns the pattern as-provided, rather than
+interpreting the character escapes. For example,
+`minimatch.match([], "\\*a\\?")` will return `"\\*a\\?"` rather than
+`"*a?"`. This is akin to setting the `nullglob` option in bash, except
+that it does not resolve escaped pattern characters.
+
+If brace expansion is not disabled, then it is performed before any
+other interpretation of the glob pattern. Thus, a pattern like
+`+(a|{b),c)}`, which would not be valid in bash or zsh, is expanded
+**first** into the set of `+(a|b)` and `+(a|c)`, and those patterns are
+checked for validity. Since those two are valid, matching proceeds.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/minimatch/minimatch.js
----------------------------------------------------------------------
diff --git a/node_modules/minimatch/minimatch.js b/node_modules/minimatch/minimatch.js
new file mode 100644
index 0000000..ec4c05c
--- /dev/null
+++ b/node_modules/minimatch/minimatch.js
@@ -0,0 +1,912 @@
+module.exports = minimatch
+minimatch.Minimatch = Minimatch
+
+var path = { sep: '/' }
+try {
+ path = require('path')
+} catch (er) {}
+
+var GLOBSTAR = minimatch.GLOBSTAR = Minimatch.GLOBSTAR = {}
+var expand = require('brace-expansion')
+
+// any single thing other than /
+// don't need to escape / when using new RegExp()
+var qmark = '[^/]'
+
+// * => any number of characters
+var star = qmark + '*?'
+
+// ** when dots are allowed. Anything goes, except .. and .
+// not (^ or / followed by one or two dots followed by $ or /),
+// followed by anything, any number of times.
+var twoStarDot = '(?:(?!(?:\\\/|^)(?:\\.{1,2})($|\\\/)).)*?'
+
+// not a ^ or / followed by a dot,
+// followed by anything, any number of times.
+var twoStarNoDot = '(?:(?!(?:\\\/|^)\\.).)*?'
+
+// characters that need to be escaped in RegExp.
+var reSpecials = charSet('().*{}+?[]^$\\!')
+
+// "abc" -> { a:true, b:true, c:true }
+function charSet (s) {
+ return s.split('').reduce(function (set, c) {
+ set[c] = true
+ return set
+ }, {})
+}
+
+// normalizes slashes.
+var slashSplit = /\/+/
+
+minimatch.filter = filter
+function filter (pattern, options) {
+ options = options || {}
+ return function (p, i, list) {
+ return minimatch(p, pattern, options)
+ }
+}
+
+function ext (a, b) {
+ a = a || {}
+ b = b || {}
+ var t = {}
+ Object.keys(b).forEach(function (k) {
+ t[k] = b[k]
+ })
+ Object.keys(a).forEach(function (k) {
+ t[k] = a[k]
+ })
+ return t
+}
+
+minimatch.defaults = function (def) {
+ if (!def || !Object.keys(def).length) return minimatch
+
+ var orig = minimatch
+
+ var m = function minimatch (p, pattern, options) {
+ return orig.minimatch(p, pattern, ext(def, options))
+ }
+
+ m.Minimatch = function Minimatch (pattern, options) {
+ return new orig.Minimatch(pattern, ext(def, options))
+ }
+
+ return m
+}
+
+Minimatch.defaults = function (def) {
+ if (!def || !Object.keys(def).length) return Minimatch
+ return minimatch.defaults(def).Minimatch
+}
+
+function minimatch (p, pattern, options) {
+ if (typeof pattern !== 'string') {
+ throw new TypeError('glob pattern string required')
+ }
+
+ if (!options) options = {}
+
+ // shortcut: comments match nothing.
+ if (!options.nocomment && pattern.charAt(0) === '#') {
+ return false
+ }
+
+ // "" only matches ""
+ if (pattern.trim() === '') return p === ''
+
+ return new Minimatch(pattern, options).match(p)
+}
+
+function Minimatch (pattern, options) {
+ if (!(this instanceof Minimatch)) {
+ return new Minimatch(pattern, options)
+ }
+
+ if (typeof pattern !== 'string') {
+ throw new TypeError('glob pattern string required')
+ }
+
+ if (!options) options = {}
+ pattern = pattern.trim()
+
+ // windows support: need to use /, not \
+ if (path.sep !== '/') {
+ pattern = pattern.split(path.sep).join('/')
+ }
+
+ this.options = options
+ this.set = []
+ this.pattern = pattern
+ this.regexp = null
+ this.negate = false
+ this.comment = false
+ this.empty = false
+
+ // make the set of regexps etc.
+ this.make()
+}
+
+Minimatch.prototype.debug = function () {}
+
+Minimatch.prototype.make = make
+function make () {
+ // don't do it more than once.
+ if (this._made) return
+
+ var pattern = this.pattern
+ var options = this.options
+
+ // empty patterns and comments match nothing.
+ if (!options.nocomment && pattern.charAt(0) === '#') {
+ this.comment = true
+ return
+ }
+ if (!pattern) {
+ this.empty = true
+ return
+ }
+
+ // step 1: figure out negation, etc.
+ this.parseNegate()
+
+ // step 2: expand braces
+ var set = this.globSet = this.braceExpand()
+
+ if (options.debug) this.debug = console.error
+
+ this.debug(this.pattern, set)
+
+ // step 3: now we have a set, so turn each one into a series of path-portion
+ // matching patterns.
+ // These will be regexps, except in the case of "**", which is
+ // set to the GLOBSTAR object for globstar behavior,
+ // and will not contain any / characters
+ set = this.globParts = set.map(function (s) {
+ return s.split(slashSplit)
+ })
+
+ this.debug(this.pattern, set)
+
+ // glob --> regexps
+ set = set.map(function (s, si, set) {
+ return s.map(this.parse, this)
+ }, this)
+
+ this.debug(this.pattern, set)
+
+ // filter out everything that didn't compile properly.
+ set = set.filter(function (s) {
+ return s.indexOf(false) === -1
+ })
+
+ this.debug(this.pattern, set)
+
+ this.set = set
+}
+
+Minimatch.prototype.parseNegate = parseNegate
+function parseNegate () {
+ var pattern = this.pattern
+ var negate = false
+ var options = this.options
+ var negateOffset = 0
+
+ if (options.nonegate) return
+
+ for (var i = 0, l = pattern.length
+ ; i < l && pattern.charAt(i) === '!'
+ ; i++) {
+ negate = !negate
+ negateOffset++
+ }
+
+ if (negateOffset) this.pattern = pattern.substr(negateOffset)
+ this.negate = negate
+}
+
+// Brace expansion:
+// a{b,c}d -> abd acd
+// a{b,}c -> abc ac
+// a{0..3}d -> a0d a1d a2d a3d
+// a{b,c{d,e}f}g -> abg acdfg acefg
+// a{b,c}d{e,f}g -> abdeg acdeg abdeg abdfg
+//
+// Invalid sets are not expanded.
+// a{2..}b -> a{2..}b
+// a{b}c -> a{b}c
+minimatch.braceExpand = function (pattern, options) {
+ return braceExpand(pattern, options)
+}
+
+Minimatch.prototype.braceExpand = braceExpand
+
+function braceExpand (pattern, options) {
+ if (!options) {
+ if (this instanceof Minimatch) {
+ options = this.options
+ } else {
+ options = {}
+ }
+ }
+
+ pattern = typeof pattern === 'undefined'
+ ? this.pattern : pattern
+
+ if (typeof pattern === 'undefined') {
+ throw new Error('undefined pattern')
+ }
+
+ if (options.nobrace ||
+ !pattern.match(/\{.*\}/)) {
+ // shortcut. no need to expand.
+ return [pattern]
+ }
+
+ return expand(pattern)
+}
+
+// parse a component of the expanded set.
+// At this point, no pattern may contain "/" in it
+// so we're going to return a 2d array, where each entry is the full
+// pattern, split on '/', and then turned into a regular expression.
+// A regexp is made at the end which joins each array with an
+// escaped /, and another full one which joins each regexp with |.
+//
+// Following the lead of Bash 4.1, note that "**" only has special meaning
+// when it is the *only* thing in a path portion. Otherwise, any series
+// of * is equivalent to a single *. Globstar behavior is enabled by
+// default, and can be disabled by setting options.noglobstar.
+Minimatch.prototype.parse = parse
+var SUBPARSE = {}
+function parse (pattern, isSub) {
+ var options = this.options
+
+ // shortcuts
+ if (!options.noglobstar && pattern === '**') return GLOBSTAR
+ if (pattern === '') return ''
+
+ var re = ''
+ var hasMagic = !!options.nocase
+ var escaping = false
+ // ? => one single character
+ var patternListStack = []
+ var negativeLists = []
+ var plType
+ var stateChar
+ var inClass = false
+ var reClassStart = -1
+ var classStart = -1
+ // . and .. never match anything that doesn't start with .,
+ // even when options.dot is set.
+ var patternStart = pattern.charAt(0) === '.' ? '' // anything
+ // not (start or / followed by . or .. followed by / or end)
+ : options.dot ? '(?!(?:^|\\\/)\\.{1,2}(?:$|\\\/))'
+ : '(?!\\.)'
+ var self = this
+
+ function clearStateChar () {
+ if (stateChar) {
+ // we had some state-tracking character
+ // that wasn't consumed by this pass.
+ switch (stateChar) {
+ case '*':
+ re += star
+ hasMagic = true
+ break
+ case '?':
+ re += qmark
+ hasMagic = true
+ break
+ default:
+ re += '\\' + stateChar
+ break
+ }
+ self.debug('clearStateChar %j %j', stateChar, re)
+ stateChar = false
+ }
+ }
+
+ for (var i = 0, len = pattern.length, c
+ ; (i < len) && (c = pattern.charAt(i))
+ ; i++) {
+ this.debug('%s\t%s %s %j', pattern, i, re, c)
+
+ // skip over any that are escaped.
+ if (escaping && reSpecials[c]) {
+ re += '\\' + c
+ escaping = false
+ continue
+ }
+
+ switch (c) {
+ case '/':
+ // completely not allowed, even escaped.
+ // Should already be path-split by now.
+ return false
+
+ case '\\':
+ clearStateChar()
+ escaping = true
+ continue
+
+ // the various stateChar values
+ // for the "extglob" stuff.
+ case '?':
+ case '*':
+ case '+':
+ case '@':
+ case '!':
+ this.debug('%s\t%s %s %j <-- stateChar', pattern, i, re, c)
+
+ // all of those are literals inside a class, except that
+ // the glob [!a] means [^a] in regexp
+ if (inClass) {
+ this.debug(' in class')
+ if (c === '!' && i === classStart + 1) c = '^'
+ re += c
+ continue
+ }
+
+ // if we already have a stateChar, then it means
+ // that there was something like ** or +? in there.
+ // Handle the stateChar, then proceed with this one.
+ self.debug('call clearStateChar %j', stateChar)
+ clearStateChar()
+ stateChar = c
+ // if extglob is disabled, then +(asdf|foo) isn't a thing.
+ // just clear the statechar *now*, rather than even diving into
+ // the patternList stuff.
+ if (options.noext) clearStateChar()
+ continue
+
+ case '(':
+ if (inClass) {
+ re += '('
+ continue
+ }
+
+ if (!stateChar) {
+ re += '\\('
+ continue
+ }
+
+ plType = stateChar
+ patternListStack.push({
+ type: plType,
+ start: i - 1,
+ reStart: re.length
+ })
+ // negation is (?:(?!js)[^/]*)
+ re += stateChar === '!' ? '(?:(?!(?:' : '(?:'
+ this.debug('plType %j %j', stateChar, re)
+ stateChar = false
+ continue
+
+ case ')':
+ if (inClass || !patternListStack.length) {
+ re += '\\)'
+ continue
+ }
+
+ clearStateChar()
+ hasMagic = true
+ re += ')'
+ var pl = patternListStack.pop()
+ plType = pl.type
+ // negation is (?:(?!js)[^/]*)
+ // The others are (?:<pattern>)<type>
+ switch (plType) {
+ case '!':
+ negativeLists.push(pl)
+ re += ')[^/]*?)'
+ pl.reEnd = re.length
+ break
+ case '?':
+ case '+':
+ case '*':
+ re += plType
+ break
+ case '@': break // the default anyway
+ }
+ continue
+
+ case '|':
+ if (inClass || !patternListStack.length || escaping) {
+ re += '\\|'
+ escaping = false
+ continue
+ }
+
+ clearStateChar()
+ re += '|'
+ continue
+
+ // these are mostly the same in regexp and glob
+ case '[':
+ // swallow any state-tracking char before the [
+ clearStateChar()
+
+ if (inClass) {
+ re += '\\' + c
+ continue
+ }
+
+ inClass = true
+ classStart = i
+ reClassStart = re.length
+ re += c
+ continue
+
+ case ']':
+ // a right bracket shall lose its special
+ // meaning and represent itself in
+ // a bracket expression if it occurs
+ // first in the list. -- POSIX.2 2.8.3.2
+ if (i === classStart + 1 || !inClass) {
+ re += '\\' + c
+ escaping = false
+ continue
+ }
+
+ // handle the case where we left a class open.
+ // "[z-a]" is valid, equivalent to "\[z-a\]"
+ if (inClass) {
+ // split where the last [ was, make sure we don't have
+ // an invalid re. if so, re-walk the contents of the
+ // would-be class to re-translate any characters that
+ // were passed through as-is
+ // TODO: It would probably be faster to determine this
+ // without a try/catch and a new RegExp, but it's tricky
+ // to do safely. For now, this is safe and works.
+ var cs = pattern.substring(classStart + 1, i)
+ try {
+ RegExp('[' + cs + ']')
+ } catch (er) {
+ // not a valid class!
+ var sp = this.parse(cs, SUBPARSE)
+ re = re.substr(0, reClassStart) + '\\[' + sp[0] + '\\]'
+ hasMagic = hasMagic || sp[1]
+ inClass = false
+ continue
+ }
+ }
+
+ // finish up the class.
+ hasMagic = true
+ inClass = false
+ re += c
+ continue
+
+ default:
+ // swallow any state char that wasn't consumed
+ clearStateChar()
+
+ if (escaping) {
+ // no need
+ escaping = false
+ } else if (reSpecials[c]
+ && !(c === '^' && inClass)) {
+ re += '\\'
+ }
+
+ re += c
+
+ } // switch
+ } // for
+
+ // handle the case where we left a class open.
+ // "[abc" is valid, equivalent to "\[abc"
+ if (inClass) {
+ // split where the last [ was, and escape it
+ // this is a huge pita. We now have to re-walk
+ // the contents of the would-be class to re-translate
+ // any characters that were passed through as-is
+ cs = pattern.substr(classStart + 1)
+ sp = this.parse(cs, SUBPARSE)
+ re = re.substr(0, reClassStart) + '\\[' + sp[0]
+ hasMagic = hasMagic || sp[1]
+ }
+
+ // handle the case where we had a +( thing at the *end*
+ // of the pattern.
+ // each pattern list stack adds 3 chars, and we need to go through
+ // and escape any | chars that were passed through as-is for the regexp.
+ // Go through and escape them, taking care not to double-escape any
+ // | chars that were already escaped.
+ for (pl = patternListStack.pop(); pl; pl = patternListStack.pop()) {
+ var tail = re.slice(pl.reStart + 3)
+ // maybe some even number of \, then maybe 1 \, followed by a |
+ tail = tail.replace(/((?:\\{2})*)(\\?)\|/g, function (_, $1, $2) {
+ if (!$2) {
+ // the | isn't already escaped, so escape it.
+ $2 = '\\'
+ }
+
+ // need to escape all those slashes *again*, without escaping the
+ // one that we need for escaping the | character. As it works out,
+ // escaping an even number of slashes can be done by simply repeating
+ // it exactly after itself. That's why this trick works.
+ //
+ // I am sorry that you have to see this.
+ return $1 + $1 + $2 + '|'
+ })
+
+ this.debug('tail=%j\n %s', tail, tail)
+ var t = pl.type === '*' ? star
+ : pl.type === '?' ? qmark
+ : '\\' + pl.type
+
+ hasMagic = true
+ re = re.slice(0, pl.reStart) + t + '\\(' + tail
+ }
+
+ // handle trailing things that only matter at the very end.
+ clearStateChar()
+ if (escaping) {
+ // trailing \\
+ re += '\\\\'
+ }
+
+ // only need to apply the nodot start if the re starts with
+ // something that could conceivably capture a dot
+ var addPatternStart = false
+ switch (re.charAt(0)) {
+ case '.':
+ case '[':
+ case '(': addPatternStart = true
+ }
+
+ // Hack to work around lack of negative lookbehind in JS
+ // A pattern like: *.!(x).!(y|z) needs to ensure that a name
+ // like 'a.xyz.yz' doesn't match. So, the first negative
+ // lookahead, has to look ALL the way ahead, to the end of
+ // the pattern.
+ for (var n = negativeLists.length - 1; n > -1; n--) {
+ var nl = negativeLists[n]
+
+ var nlBefore = re.slice(0, nl.reStart)
+ var nlFirst = re.slice(nl.reStart, nl.reEnd - 8)
+ var nlLast = re.slice(nl.reEnd - 8, nl.reEnd)
+ var nlAfter = re.slice(nl.reEnd)
+
+ nlLast += nlAfter
+
+ // Handle nested stuff like *(*.js|!(*.json)), where open parens
+ // mean that we should *not* include the ) in the bit that is considered
+ // "after" the negated section.
+ var openParensBefore = nlBefore.split('(').length - 1
+ var cleanAfter = nlAfter
+ for (i = 0; i < openParensBefore; i++) {
+ cleanAfter = cleanAfter.replace(/\)[+*?]?/, '')
+ }
+ nlAfter = cleanAfter
+
+ var dollar = ''
+ if (nlAfter === '' && isSub !== SUBPARSE) {
+ dollar = '$'
+ }
+ var newRe = nlBefore + nlFirst + nlAfter + dollar + nlLast
+ re = newRe
+ }
+
+ // if the re is not "" at this point, then we need to make sure
+ // it doesn't match against an empty path part.
+ // Otherwise a/* will match a/, which it should not.
+ if (re !== '' && hasMagic) {
+ re = '(?=.)' + re
+ }
+
+ if (addPatternStart) {
+ re = patternStart + re
+ }
+
+ // parsing just a piece of a larger pattern.
+ if (isSub === SUBPARSE) {
+ return [re, hasMagic]
+ }
+
+ // skip the regexp for non-magical patterns
+ // unescape anything in it, though, so that it'll be
+ // an exact match against a file etc.
+ if (!hasMagic) {
+ return globUnescape(pattern)
+ }
+
+ var flags = options.nocase ? 'i' : ''
+ var regExp = new RegExp('^' + re + '$', flags)
+
+ regExp._glob = pattern
+ regExp._src = re
+
+ return regExp
+}
+
+minimatch.makeRe = function (pattern, options) {
+ return new Minimatch(pattern, options || {}).makeRe()
+}
+
+Minimatch.prototype.makeRe = makeRe
+function makeRe () {
+ if (this.regexp || this.regexp === false) return this.regexp
+
+ // at this point, this.set is a 2d array of partial
+ // pattern strings, or "**".
+ //
+ // It's better to use .match(). This function shouldn't
+ // be used, really, but it's pretty convenient sometimes,
+ // when you just want to work with a regex.
+ var set = this.set
+
+ if (!set.length) {
+ this.regexp = false
+ return this.regexp
+ }
+ var options = this.options
+
+ var twoStar = options.noglobstar ? star
+ : options.dot ? twoStarDot
+ : twoStarNoDot
+ var flags = options.nocase ? 'i' : ''
+
+ var re = set.map(function (pattern) {
+ return pattern.map(function (p) {
+ return (p === GLOBSTAR) ? twoStar
+ : (typeof p === 'string') ? regExpEscape(p)
+ : p._src
+ }).join('\\\/')
+ }).join('|')
+
+ // must match entire pattern
+ // ending in a * or ** will make it less strict.
+ re = '^(?:' + re + ')$'
+
+ // can match anything, as long as it's not this.
+ if (this.negate) re = '^(?!' + re + ').*$'
+
+ try {
+ this.regexp = new RegExp(re, flags)
+ } catch (ex) {
+ this.regexp = false
+ }
+ return this.regexp
+}
+
+minimatch.match = function (list, pattern, options) {
+ options = options || {}
+ var mm = new Minimatch(pattern, options)
+ list = list.filter(function (f) {
+ return mm.match(f)
+ })
+ if (mm.options.nonull && !list.length) {
+ list.push(pattern)
+ }
+ return list
+}
+
+Minimatch.prototype.match = match
+function match (f, partial) {
+ this.debug('match', f, this.pattern)
+ // short-circuit in the case of busted things.
+ // comments, etc.
+ if (this.comment) return false
+ if (this.empty) return f === ''
+
+ if (f === '/' && partial) return true
+
+ var options = this.options
+
+ // windows: need to use /, not \
+ if (path.sep !== '/') {
+ f = f.split(path.sep).join('/')
+ }
+
+ // treat the test path as a set of pathparts.
+ f = f.split(slashSplit)
+ this.debug(this.pattern, 'split', f)
+
+ // just ONE of the pattern sets in this.set needs to match
+ // in order for it to be valid. If negating, then just one
+ // match means that we have failed.
+ // Either way, return on the first hit.
+
+ var set = this.set
+ this.debug(this.pattern, 'set', set)
+
+ // Find the basename of the path by looking for the last non-empty segment
+ var filename
+ var i
+ for (i = f.length - 1; i >= 0; i--) {
+ filename = f[i]
+ if (filename) break
+ }
+
+ for (i = 0; i < set.length; i++) {
+ var pattern = set[i]
+ var file = f
+ if (options.matchBase && pattern.length === 1) {
+ file = [filename]
+ }
+ var hit = this.matchOne(file, pattern, partial)
+ if (hit) {
+ if (options.flipNegate) return true
+ return !this.negate
+ }
+ }
+
+ // didn't get any hits. this is success if it's a negative
+ // pattern, failure otherwise.
+ if (options.flipNegate) return false
+ return this.negate
+}
+
+// set partial to true to test if, for example,
+// "/a/b" matches the start of "/*/b/*/d"
+// Partial means, if you run out of file before you run
+// out of pattern, then that's fine, as long as all
+// the parts match.
+Minimatch.prototype.matchOne = function (file, pattern, partial) {
+ var options = this.options
+
+ this.debug('matchOne',
+ { 'this': this, file: file, pattern: pattern })
+
+ this.debug('matchOne', file.length, pattern.length)
+
+ for (var fi = 0,
+ pi = 0,
+ fl = file.length,
+ pl = pattern.length
+ ; (fi < fl) && (pi < pl)
+ ; fi++, pi++) {
+ this.debug('matchOne loop')
+ var p = pattern[pi]
+ var f = file[fi]
+
+ this.debug(pattern, p, f)
+
+ // should be impossible.
+ // some invalid regexp stuff in the set.
+ if (p === false) return false
+
+ if (p === GLOBSTAR) {
+ this.debug('GLOBSTAR', [pattern, p, f])
+
+ // "**"
+ // a/**/b/**/c would match the following:
+ // a/b/x/y/z/c
+ // a/x/y/z/b/c
+ // a/b/x/b/x/c
+ // a/b/c
+ // To do this, take the rest of the pattern after
+ // the **, and see if it would match the file remainder.
+ // If so, return success.
+ // If not, the ** "swallows" a segment, and try again.
+ // This is recursively awful.
+ //
+ // a/**/b/**/c matching a/b/x/y/z/c
+ // - a matches a
+ // - doublestar
+ // - matchOne(b/x/y/z/c, b/**/c)
+ // - b matches b
+ // - doublestar
+ // - matchOne(x/y/z/c, c) -> no
+ // - matchOne(y/z/c, c) -> no
+ // - matchOne(z/c, c) -> no
+ // - matchOne(c, c) yes, hit
+ var fr = fi
+ var pr = pi + 1
+ if (pr === pl) {
+ this.debug('** at the end')
+ // a ** at the end will just swallow the rest.
+ // We have found a match.
+ // however, it will not swallow /.x, unless
+ // options.dot is set.
+ // . and .. are *never* matched by **, for explosively
+ // exponential reasons.
+ for (; fi < fl; fi++) {
+ if (file[fi] === '.' || file[fi] === '..' ||
+ (!options.dot && file[fi].charAt(0) === '.')) return false
+ }
+ return true
+ }
+
+ // ok, let's see if we can swallow whatever we can.
+ while (fr < fl) {
+ var swallowee = file[fr]
+
+ this.debug('\nglobstar while', file, fr, pattern, pr, swallowee)
+
+ // XXX remove this slice. Just pass the start index.
+ if (this.matchOne(file.slice(fr), pattern.slice(pr), partial)) {
+ this.debug('globstar found match!', fr, fl, swallowee)
+ // found a match.
+ return true
+ } else {
+ // can't swallow "." or ".." ever.
+ // can only swallow ".foo" when explicitly asked.
+ if (swallowee === '.' || swallowee === '..' ||
+ (!options.dot && swallowee.charAt(0) === '.')) {
+ this.debug('dot detected!', file, fr, pattern, pr)
+ break
+ }
+
+ // ** swallows a segment, and continue.
+ this.debug('globstar swallow a segment, and continue')
+ fr++
+ }
+ }
+
+ // no match was found.
+ // However, in partial mode, we can't say this is necessarily over.
+ // If there's more *pattern* left, then
+ if (partial) {
+ // ran out of file
+ this.debug('\n>>> no match, partial?', file, fr, pattern, pr)
+ if (fr === fl) return true
+ }
+ return false
+ }
+
+ // something other than **
+ // non-magic patterns just have to match exactly
+ // patterns with magic have been turned into regexps.
+ var hit
+ if (typeof p === 'string') {
+ if (options.nocase) {
+ hit = f.toLowerCase() === p.toLowerCase()
+ } else {
+ hit = f === p
+ }
+ this.debug('string match', p, f, hit)
+ } else {
+ hit = f.match(p)
+ this.debug('pattern match', p, f, hit)
+ }
+
+ if (!hit) return false
+ }
+
+ // Note: ending in / means that we'll get a final ""
+ // at the end of the pattern. This can only match a
+ // corresponding "" at the end of the file.
+ // If the file ends in /, then it can only match a
+ // a pattern that ends in /, unless the pattern just
+ // doesn't have any more for it. But, a/b/ should *not*
+ // match "a/b/*", even though "" matches against the
+ // [^/]*? pattern, except in partial mode, where it might
+ // simply not be reached yet.
+ // However, a/b/ should still satisfy a/*
+
+ // now either we fell off the end of the pattern, or we're done.
+ if (fi === fl && pi === pl) {
+ // ran out of pattern and filename at the same time.
+ // an exact hit!
+ return true
+ } else if (fi === fl) {
+ // ran out of file, but still had pattern left.
+ // this is ok if we're doing the match as part of
+ // a glob fs traversal.
+ return partial
+ } else if (pi === pl) {
+ // ran out of pattern, still have file left.
+ // this is only acceptable if we're on the very last
+ // empty segment of a file with a trailing slash.
+ // a/* should match a/b/
+ var emptyFileEnd = (fi === fl - 1) && (file[fi] === '')
+ return emptyFileEnd
+ }
+
+ // should be unreachable.
+ throw new Error('wtf?')
+}
+
+// replace stuff like \* with *
+function globUnescape (s) {
+ return s.replace(/\\(.)/g, '$1')
+}
+
+function regExpEscape (s) {
+ return s.replace(/[-[\]{}()*+?.,\\^$|#\s]/g, '\\$&')
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/minimatch/package.json
----------------------------------------------------------------------
diff --git a/node_modules/minimatch/package.json b/node_modules/minimatch/package.json
new file mode 100644
index 0000000..85c32dc
--- /dev/null
+++ b/node_modules/minimatch/package.json
@@ -0,0 +1,85 @@
+{
+ "_args": [
+ [
+ "minimatch@2 || 3",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob"
+ ]
+ ],
+ "_from": "minimatch@>=2.0.0 <3.0.0||>=3.0.0 <4.0.0",
+ "_id": "minimatch@3.0.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/minimatch",
+ "_nodeVersion": "4.0.0",
+ "_npmUser": {
+ "email": "isaacs@npmjs.com",
+ "name": "isaacs"
+ },
+ "_npmVersion": "3.3.2",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "minimatch",
+ "raw": "minimatch@2 || 3",
+ "rawSpec": "2 || 3",
+ "scope": null,
+ "spec": ">=2.0.0 <3.0.0||>=3.0.0 <4.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/glob"
+ ],
+ "_resolved": "http://registry.npmjs.org/minimatch/-/minimatch-3.0.0.tgz",
+ "_shasum": "5236157a51e4f004c177fb3c527ff7dd78f0ef83",
+ "_shrinkwrap": null,
+ "_spec": "minimatch@2 || 3",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/minimatch/issues"
+ },
+ "dependencies": {
+ "brace-expansion": "^1.0.0"
+ },
+ "description": "a glob matcher in javascript",
+ "devDependencies": {
+ "standard": "^3.7.2",
+ "tap": "^1.2.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "5236157a51e4f004c177fb3c527ff7dd78f0ef83",
+ "tarball": "http://registry.npmjs.org/minimatch/-/minimatch-3.0.0.tgz"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "files": [
+ "minimatch.js"
+ ],
+ "gitHead": "270dbea567f0af6918cb18103e98c612aa717a20",
+ "homepage": "https://github.com/isaacs/minimatch#readme",
+ "license": "ISC",
+ "main": "minimatch.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "name": "minimatch",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/minimatch.git"
+ },
+ "scripts": {
+ "posttest": "standard minimatch.js test/*.js",
+ "test": "tap test/*.js"
+ },
+ "version": "3.0.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/.npmignore b/node_modules/node-uuid/.npmignore
new file mode 100644
index 0000000..fd4f2b0
--- /dev/null
+++ b/node_modules/node-uuid/.npmignore
@@ -0,0 +1,2 @@
+node_modules
+.DS_Store
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/LICENSE.md
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/LICENSE.md b/node_modules/node-uuid/LICENSE.md
new file mode 100644
index 0000000..bcdddf9
--- /dev/null
+++ b/node_modules/node-uuid/LICENSE.md
@@ -0,0 +1,3 @@
+Copyright (c) 2010 Robert Kieffer
+
+Dual licensed under the [MIT](http://en.wikipedia.org/wiki/MIT_License) and [GPL](http://en.wikipedia.org/wiki/GNU_General_Public_License) licenses.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[58/59] [abbrv] mac commit: Update JS snapshot to version 4.0.1 (via
coho)
Posted by st...@apache.org.
Update JS snapshot to version 4.0.1 (via coho)
Project: http://git-wip-us.apache.org/repos/asf/cordova-osx/repo
Commit: http://git-wip-us.apache.org/repos/asf/cordova-osx/commit/199e1abe
Tree: http://git-wip-us.apache.org/repos/asf/cordova-osx/tree/199e1abe
Diff: http://git-wip-us.apache.org/repos/asf/cordova-osx/diff/199e1abe
Branch: refs/heads/4.0.x
Commit: 199e1abe7c16fa4d9efec749e8b05d1d6f7b68bc
Parents: 1ed8a4c
Author: Steve Gill <st...@gmail.com>
Authored: Thu Mar 3 15:45:28 2016 -0800
Committer: Steve Gill <st...@gmail.com>
Committed: Thu Mar 3 15:45:28 2016 -0800
----------------------------------------------------------------------
CordovaLib/cordova.js | 4 ++--
1 file changed, 2 insertions(+), 2 deletions(-)
----------------------------------------------------------------------
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/199e1abe/CordovaLib/cordova.js
----------------------------------------------------------------------
diff --git a/CordovaLib/cordova.js b/CordovaLib/cordova.js
index 135b74b..d6d8dd4 100644
--- a/CordovaLib/cordova.js
+++ b/CordovaLib/cordova.js
@@ -1,5 +1,5 @@
// Platform: osx
-// 1b111058631e118dc37da473e30b60214c211d76
+// c517ca811b4948b630e0b74dbae6c9637939da24
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
@@ -19,7 +19,7 @@
under the License.
*/
;(function() {
-var PLATFORM_VERSION_BUILD_LABEL = '4.0.0';
+var PLATFORM_VERSION_BUILD_LABEL = '4.0.1';
// file: src/scripts/require.js
/*jshint -W079 */
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[46/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/rm.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/rm.js b/node_modules/cordova-common/node_modules/shelljs/src/rm.js
new file mode 100644
index 0000000..bd608cb
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/rm.js
@@ -0,0 +1,163 @@
+var common = require('./common');
+var fs = require('fs');
+
+// Recursively removes 'dir'
+// Adapted from https://github.com/ryanmcgrath/wrench-js
+//
+// Copyright (c) 2010 Ryan McGrath
+// Copyright (c) 2012 Artur Adib
+//
+// Licensed under the MIT License
+// http://www.opensource.org/licenses/mit-license.php
+function rmdirSyncRecursive(dir, force) {
+ var files;
+
+ files = fs.readdirSync(dir);
+
+ // Loop through and delete everything in the sub-tree after checking it
+ for(var i = 0; i < files.length; i++) {
+ var file = dir + "/" + files[i],
+ currFile = fs.lstatSync(file);
+
+ if(currFile.isDirectory()) { // Recursive function back to the beginning
+ rmdirSyncRecursive(file, force);
+ }
+
+ else if(currFile.isSymbolicLink()) { // Unlink symlinks
+ if (force || isWriteable(file)) {
+ try {
+ common.unlinkSync(file);
+ } catch (e) {
+ common.error('could not remove file (code '+e.code+'): ' + file, true);
+ }
+ }
+ }
+
+ else // Assume it's a file - perhaps a try/catch belongs here?
+ if (force || isWriteable(file)) {
+ try {
+ common.unlinkSync(file);
+ } catch (e) {
+ common.error('could not remove file (code '+e.code+'): ' + file, true);
+ }
+ }
+ }
+
+ // Now that we know everything in the sub-tree has been deleted, we can delete the main directory.
+ // Huzzah for the shopkeep.
+
+ var result;
+ try {
+ // Retry on windows, sometimes it takes a little time before all the files in the directory are gone
+ var start = Date.now();
+ while (true) {
+ try {
+ result = fs.rmdirSync(dir);
+ if (fs.existsSync(dir)) throw { code: "EAGAIN" }
+ break;
+ } catch(er) {
+ // In addition to error codes, also check if the directory still exists and loop again if true
+ if (process.platform === "win32" && (er.code === "ENOTEMPTY" || er.code === "EBUSY" || er.code === "EPERM" || er.code === "EAGAIN")) {
+ if (Date.now() - start > 1000) throw er;
+ } else if (er.code === "ENOENT") {
+ // Directory did not exist, deletion was successful
+ break;
+ } else {
+ throw er;
+ }
+ }
+ }
+ } catch(e) {
+ common.error('could not remove directory (code '+e.code+'): ' + dir, true);
+ }
+
+ return result;
+} // rmdirSyncRecursive
+
+// Hack to determine if file has write permissions for current user
+// Avoids having to check user, group, etc, but it's probably slow
+function isWriteable(file) {
+ var writePermission = true;
+ try {
+ var __fd = fs.openSync(file, 'a');
+ fs.closeSync(__fd);
+ } catch(e) {
+ writePermission = false;
+ }
+
+ return writePermission;
+}
+
+//@
+//@ ### rm([options ,] file [, file ...])
+//@ ### rm([options ,] file_array)
+//@ Available options:
+//@
+//@ + `-f`: force
+//@ + `-r, -R`: recursive
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ rm('-rf', '/tmp/*');
+//@ rm('some_file.txt', 'another_file.txt');
+//@ rm(['some_file.txt', 'another_file.txt']); // same as above
+//@ ```
+//@
+//@ Removes files. The wildcard `*` is accepted.
+function _rm(options, files) {
+ options = common.parseOptions(options, {
+ 'f': 'force',
+ 'r': 'recursive',
+ 'R': 'recursive'
+ });
+ if (!files)
+ common.error('no paths given');
+
+ if (typeof files === 'string')
+ files = [].slice.call(arguments, 1);
+ // if it's array leave it as it is
+
+ files = common.expand(files);
+
+ files.forEach(function(file) {
+ if (!fs.existsSync(file)) {
+ // Path does not exist, no force flag given
+ if (!options.force)
+ common.error('no such file or directory: '+file, true);
+
+ return; // skip file
+ }
+
+ // If here, path exists
+
+ var stats = fs.lstatSync(file);
+ if (stats.isFile() || stats.isSymbolicLink()) {
+
+ // Do not check for file writing permissions
+ if (options.force) {
+ common.unlinkSync(file);
+ return;
+ }
+
+ if (isWriteable(file))
+ common.unlinkSync(file);
+ else
+ common.error('permission denied: '+file, true);
+
+ return;
+ } // simple file
+
+ // Path is an existing directory, but no -r flag given
+ if (stats.isDirectory() && !options.recursive) {
+ common.error('path is a directory', true);
+ return; // skip path
+ }
+
+ // Recursively remove existing directory
+ if (stats.isDirectory() && options.recursive) {
+ rmdirSyncRecursive(file, options.force);
+ }
+ }); // forEach(file)
+} // rm
+module.exports = _rm;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/sed.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/sed.js b/node_modules/cordova-common/node_modules/shelljs/src/sed.js
new file mode 100644
index 0000000..65f7cb4
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/sed.js
@@ -0,0 +1,43 @@
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### sed([options ,] search_regex, replacement, file)
+//@ Available options:
+//@
+//@ + `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
+//@ sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
+//@ ```
+//@
+//@ Reads an input string from `file` and performs a JavaScript `replace()` on the input
+//@ using the given search regex and replacement string or function. Returns the new string after replacement.
+function _sed(options, regex, replacement, file) {
+ options = common.parseOptions(options, {
+ 'i': 'inplace'
+ });
+
+ if (typeof replacement === 'string' || typeof replacement === 'function')
+ replacement = replacement; // no-op
+ else if (typeof replacement === 'number')
+ replacement = replacement.toString(); // fallback
+ else
+ common.error('invalid replacement string');
+
+ if (!file)
+ common.error('no file given');
+
+ if (!fs.existsSync(file))
+ common.error('no such file or directory: ' + file);
+
+ var result = fs.readFileSync(file, 'utf8').replace(regex, replacement);
+ if (options.inplace)
+ fs.writeFileSync(file, result, 'utf8');
+
+ return common.ShellString(result);
+}
+module.exports = _sed;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js b/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js
new file mode 100644
index 0000000..45953c2
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/tempdir.js
@@ -0,0 +1,56 @@
+var common = require('./common');
+var os = require('os');
+var fs = require('fs');
+
+// Returns false if 'dir' is not a writeable directory, 'dir' otherwise
+function writeableDir(dir) {
+ if (!dir || !fs.existsSync(dir))
+ return false;
+
+ if (!fs.statSync(dir).isDirectory())
+ return false;
+
+ var testFile = dir+'/'+common.randomFileName();
+ try {
+ fs.writeFileSync(testFile, ' ');
+ common.unlinkSync(testFile);
+ return dir;
+ } catch (e) {
+ return false;
+ }
+}
+
+
+//@
+//@ ### tempdir()
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var tmp = tempdir(); // "/tmp" for most *nix platforms
+//@ ```
+//@
+//@ Searches and returns string containing a writeable, platform-dependent temporary directory.
+//@ Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
+function _tempDir() {
+ var state = common.state;
+ if (state.tempDir)
+ return state.tempDir; // from cache
+
+ state.tempDir = writeableDir(os.tempDir && os.tempDir()) || // node 0.8+
+ writeableDir(process.env['TMPDIR']) ||
+ writeableDir(process.env['TEMP']) ||
+ writeableDir(process.env['TMP']) ||
+ writeableDir(process.env['Wimp$ScrapDir']) || // RiscOS
+ writeableDir('C:\\TEMP') || // Windows
+ writeableDir('C:\\TMP') || // Windows
+ writeableDir('\\TEMP') || // Windows
+ writeableDir('\\TMP') || // Windows
+ writeableDir('/tmp') ||
+ writeableDir('/var/tmp') ||
+ writeableDir('/usr/tmp') ||
+ writeableDir('.'); // last resort
+
+ return state.tempDir;
+}
+module.exports = _tempDir;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/test.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/test.js b/node_modules/cordova-common/node_modules/shelljs/src/test.js
new file mode 100644
index 0000000..8a4ac7d
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/test.js
@@ -0,0 +1,85 @@
+var common = require('./common');
+var fs = require('fs');
+
+//@
+//@ ### test(expression)
+//@ Available expression primaries:
+//@
+//@ + `'-b', 'path'`: true if path is a block device
+//@ + `'-c', 'path'`: true if path is a character device
+//@ + `'-d', 'path'`: true if path is a directory
+//@ + `'-e', 'path'`: true if path exists
+//@ + `'-f', 'path'`: true if path is a regular file
+//@ + `'-L', 'path'`: true if path is a symboilc link
+//@ + `'-p', 'path'`: true if path is a pipe (FIFO)
+//@ + `'-S', 'path'`: true if path is a socket
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ if (test('-d', path)) { /* do something with dir */ };
+//@ if (!test('-f', path)) continue; // skip if it's a regular file
+//@ ```
+//@
+//@ Evaluates expression using the available primaries and returns corresponding value.
+function _test(options, path) {
+ if (!path)
+ common.error('no path given');
+
+ // hack - only works with unary primaries
+ options = common.parseOptions(options, {
+ 'b': 'block',
+ 'c': 'character',
+ 'd': 'directory',
+ 'e': 'exists',
+ 'f': 'file',
+ 'L': 'link',
+ 'p': 'pipe',
+ 'S': 'socket'
+ });
+
+ var canInterpret = false;
+ for (var key in options)
+ if (options[key] === true) {
+ canInterpret = true;
+ break;
+ }
+
+ if (!canInterpret)
+ common.error('could not interpret expression');
+
+ if (options.link) {
+ try {
+ return fs.lstatSync(path).isSymbolicLink();
+ } catch(e) {
+ return false;
+ }
+ }
+
+ if (!fs.existsSync(path))
+ return false;
+
+ if (options.exists)
+ return true;
+
+ var stats = fs.statSync(path);
+
+ if (options.block)
+ return stats.isBlockDevice();
+
+ if (options.character)
+ return stats.isCharacterDevice();
+
+ if (options.directory)
+ return stats.isDirectory();
+
+ if (options.file)
+ return stats.isFile();
+
+ if (options.pipe)
+ return stats.isFIFO();
+
+ if (options.socket)
+ return stats.isSocket();
+} // test
+module.exports = _test;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/to.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/to.js b/node_modules/cordova-common/node_modules/shelljs/src/to.js
new file mode 100644
index 0000000..f029999
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/to.js
@@ -0,0 +1,29 @@
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+//@
+//@ ### 'string'.to(file)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ cat('input.txt').to('output.txt');
+//@ ```
+//@
+//@ Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
+//@ those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
+function _to(options, file) {
+ if (!file)
+ common.error('wrong arguments');
+
+ if (!fs.existsSync( path.dirname(file) ))
+ common.error('no such file or directory: ' + path.dirname(file));
+
+ try {
+ fs.writeFileSync(file, this.toString(), 'utf8');
+ } catch(e) {
+ common.error('could not write to file (code '+e.code+'): '+file, true);
+ }
+}
+module.exports = _to;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js b/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js
new file mode 100644
index 0000000..f6d099d
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/toEnd.js
@@ -0,0 +1,29 @@
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+//@
+//@ ### 'string'.toEnd(file)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ cat('input.txt').toEnd('output.txt');
+//@ ```
+//@
+//@ Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
+//@ those returned by `cat`, `grep`, etc).
+function _toEnd(options, file) {
+ if (!file)
+ common.error('wrong arguments');
+
+ if (!fs.existsSync( path.dirname(file) ))
+ common.error('no such file or directory: ' + path.dirname(file));
+
+ try {
+ fs.appendFileSync(file, this.toString(), 'utf8');
+ } catch(e) {
+ common.error('could not append to file (code '+e.code+'): '+file, true);
+ }
+}
+module.exports = _toEnd;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/node_modules/shelljs/src/which.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/which.js b/node_modules/cordova-common/node_modules/shelljs/src/which.js
new file mode 100644
index 0000000..2822ecf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/shelljs/src/which.js
@@ -0,0 +1,83 @@
+var common = require('./common');
+var fs = require('fs');
+var path = require('path');
+
+// Cross-platform method for splitting environment PATH variables
+function splitPath(p) {
+ for (i=1;i<2;i++) {}
+
+ if (!p)
+ return [];
+
+ if (common.platform === 'win')
+ return p.split(';');
+ else
+ return p.split(':');
+}
+
+function checkPath(path) {
+ return fs.existsSync(path) && fs.statSync(path).isDirectory() == false;
+}
+
+//@
+//@ ### which(command)
+//@
+//@ Examples:
+//@
+//@ ```javascript
+//@ var nodeExec = which('node');
+//@ ```
+//@
+//@ Searches for `command` in the system's PATH. On Windows looks for `.exe`, `.cmd`, and `.bat` extensions.
+//@ Returns string containing the absolute path to the command.
+function _which(options, cmd) {
+ if (!cmd)
+ common.error('must specify command');
+
+ var pathEnv = process.env.path || process.env.Path || process.env.PATH,
+ pathArray = splitPath(pathEnv),
+ where = null;
+
+ // No relative/absolute paths provided?
+ if (cmd.search(/\//) === -1) {
+ // Search for command in PATH
+ pathArray.forEach(function(dir) {
+ if (where)
+ return; // already found it
+
+ var attempt = path.resolve(dir + '/' + cmd);
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+
+ if (common.platform === 'win') {
+ var baseAttempt = attempt;
+ attempt = baseAttempt + '.exe';
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ attempt = baseAttempt + '.cmd';
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ attempt = baseAttempt + '.bat';
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ } // if 'win'
+ });
+ }
+
+ // Command not found anywhere?
+ if (!checkPath(cmd) && !where)
+ return null;
+
+ where = where || path.resolve(cmd);
+
+ return common.ShellString(where);
+}
+module.exports = _which;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/package.json b/node_modules/cordova-common/package.json
new file mode 100644
index 0000000..2ec1962
--- /dev/null
+++ b/node_modules/cordova-common/package.json
@@ -0,0 +1,119 @@
+{
+ "_args": [
+ [
+ "cordova-common@^1.0.0",
+ "/Users/steveng/repo/cordova/cordova-osx"
+ ]
+ ],
+ "_from": "cordova-common@>=1.0.0 <2.0.0",
+ "_id": "cordova-common@1.1.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/cordova-common",
+ "_nodeVersion": "4.2.3",
+ "_npmOperationalInternal": {
+ "host": "packages-5-east.internal.npmjs.com",
+ "tmp": "tmp/cordova-common-1.1.0.tgz_1455781889491_0.6937742941081524"
+ },
+ "_npmUser": {
+ "email": "kotikov.vladimir@gmail.com",
+ "name": "kotikov.vladimir"
+ },
+ "_npmVersion": "2.14.7",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "cordova-common",
+ "raw": "cordova-common@^1.0.0",
+ "rawSpec": "^1.0.0",
+ "scope": null,
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/"
+ ],
+ "_resolved": "http://registry.npmjs.org/cordova-common/-/cordova-common-1.1.0.tgz",
+ "_shasum": "8682721466ee354747ec6241f34f412b7e0ef636",
+ "_shrinkwrap": null,
+ "_spec": "cordova-common@^1.0.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx",
+ "author": {
+ "name": "Apache Software Foundation"
+ },
+ "bugs": {
+ "email": "dev@cordova.apache.org",
+ "url": "https://issues.apache.org/jira/browse/CB"
+ },
+ "contributors": [],
+ "dependencies": {
+ "ansi": "^0.3.1",
+ "bplist-parser": "^0.1.0",
+ "cordova-registry-mapper": "^1.1.8",
+ "elementtree": "^0.1.6",
+ "glob": "^5.0.13",
+ "osenv": "^0.1.3",
+ "plist": "^1.2.0",
+ "q": "^1.4.1",
+ "semver": "^5.0.1",
+ "shelljs": "^0.5.1",
+ "underscore": "^1.8.3",
+ "unorm": "^1.3.3"
+ },
+ "description": "Apache Cordova tools and platforms shared routines",
+ "devDependencies": {
+ "istanbul": "^0.3.17",
+ "jasmine-node": "^1.14.5",
+ "jshint": "^2.8.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "8682721466ee354747ec6241f34f412b7e0ef636",
+ "tarball": "http://registry.npmjs.org/cordova-common/-/cordova-common-1.1.0.tgz"
+ },
+ "engineStrict": true,
+ "engines": {
+ "node": ">=0.9.9"
+ },
+ "license": "Apache-2.0",
+ "main": "cordova-common.js",
+ "maintainers": [
+ {
+ "name": "bowserj",
+ "email": "bowserj@apache.org"
+ },
+ {
+ "name": "kotikov.vladimir",
+ "email": "kotikov.vladimir@gmail.com"
+ },
+ {
+ "name": "purplecabbage",
+ "email": "purplecabbage@gmail.com"
+ },
+ {
+ "name": "shazron",
+ "email": "shazron@gmail.com"
+ },
+ {
+ "name": "stevegill",
+ "email": "stevengill97@gmail.com"
+ },
+ {
+ "name": "timbarham",
+ "email": "npmjs@barhams.info"
+ }
+ ],
+ "name": "cordova-common",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://git-wip-us.apache.org/repos/asf/cordova-common.git"
+ },
+ "scripts": {
+ "cover": "node node_modules/istanbul/lib/cli.js cover --root src --print detail node_modules/jasmine-node/bin/jasmine-node -- spec",
+ "jasmine": "node node_modules/jasmine-node/bin/jasmine-node --captureExceptions --color spec",
+ "jshint": "node node_modules/jshint/bin/jshint src && node node_modules/jshint/bin/jshint spec",
+ "test": "npm run jshint && npm run jasmine"
+ },
+ "version": "1.1.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/.jshintrc b/node_modules/cordova-common/src/.jshintrc
new file mode 100644
index 0000000..89a121c
--- /dev/null
+++ b/node_modules/cordova-common/src/.jshintrc
@@ -0,0 +1,10 @@
+{
+ "node": true
+ , "bitwise": true
+ , "undef": true
+ , "trailing": true
+ , "quotmark": true
+ , "indent": 4
+ , "unused": "vars"
+ , "latedef": "nofunc"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ActionStack.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ActionStack.js b/node_modules/cordova-common/src/ActionStack.js
new file mode 100644
index 0000000..5ef6f84
--- /dev/null
+++ b/node_modules/cordova-common/src/ActionStack.js
@@ -0,0 +1,85 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+/* jshint quotmark:false */
+
+var events = require('./events'),
+ Q = require('q');
+
+function ActionStack() {
+ this.stack = [];
+ this.completed = [];
+}
+
+ActionStack.prototype = {
+ createAction:function(handler, action_params, reverter, revert_params) {
+ return {
+ handler:{
+ run:handler,
+ params:action_params
+ },
+ reverter:{
+ run:reverter,
+ params:revert_params
+ }
+ };
+ },
+ push:function(tx) {
+ this.stack.push(tx);
+ },
+ // Returns a promise.
+ process:function(platform) {
+ events.emit('verbose', 'Beginning processing of action stack for ' + platform + ' project...');
+
+ while (this.stack.length) {
+ var action = this.stack.shift();
+ var handler = action.handler.run;
+ var action_params = action.handler.params;
+
+ try {
+ handler.apply(null, action_params);
+ } catch(e) {
+ events.emit('warn', 'Error during processing of action! Attempting to revert...');
+ this.stack.unshift(action);
+ var issue = 'Uh oh!\n';
+ // revert completed tasks
+ while(this.completed.length) {
+ var undo = this.completed.shift();
+ var revert = undo.reverter.run;
+ var revert_params = undo.reverter.params;
+
+ try {
+ revert.apply(null, revert_params);
+ } catch(err) {
+ events.emit('warn', 'Error during reversion of action! We probably really messed up your project now, sorry! D:');
+ issue += 'A reversion action failed: ' + err.message + '\n';
+ }
+ }
+ e.message = issue + e.message;
+ return Q.reject(e);
+ }
+ this.completed.push(action);
+ }
+ events.emit('verbose', 'Action stack processing complete.');
+
+ return Q();
+ }
+};
+
+module.exports = ActionStack;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ConfigChanges/ConfigChanges.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ConfigChanges/ConfigChanges.js b/node_modules/cordova-common/src/ConfigChanges/ConfigChanges.js
new file mode 100644
index 0000000..a914fc8
--- /dev/null
+++ b/node_modules/cordova-common/src/ConfigChanges/ConfigChanges.js
@@ -0,0 +1,323 @@
+/*
+ *
+ * Copyright 2013 Anis Kadri
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+
+/*
+ * This module deals with shared configuration / dependency "stuff". That is:
+ * - XML configuration files such as config.xml, AndroidManifest.xml or WMAppManifest.xml.
+ * - plist files in iOS
+ * Essentially, any type of shared resources that we need to handle with awareness
+ * of how potentially multiple plugins depend on a single shared resource, should be
+ * handled in this module.
+ *
+ * The implementation uses an object as a hash table, with "leaves" of the table tracking
+ * reference counts.
+ */
+
+/* jshint sub:true */
+
+var fs = require('fs'),
+ path = require('path'),
+ et = require('elementtree'),
+ semver = require('semver'),
+ events = require('../events'),
+ ConfigKeeper = require('./ConfigKeeper');
+
+var mungeutil = require('./munge-util');
+
+exports.PlatformMunger = PlatformMunger;
+
+exports.process = function(plugins_dir, project_dir, platform, platformJson, pluginInfoProvider) {
+ var munger = new PlatformMunger(platform, project_dir, platformJson, pluginInfoProvider);
+ munger.process(plugins_dir);
+ munger.save_all();
+};
+
+/******************************************************************************
+* PlatformMunger class
+*
+* Can deal with config file of a single project.
+* Parsed config files are cached in a ConfigKeeper object.
+******************************************************************************/
+function PlatformMunger(platform, project_dir, platformJson, pluginInfoProvider) {
+ this.platform = platform;
+ this.project_dir = project_dir;
+ this.config_keeper = new ConfigKeeper(project_dir);
+ this.platformJson = platformJson;
+ this.pluginInfoProvider = pluginInfoProvider;
+}
+
+// Write out all unsaved files.
+PlatformMunger.prototype.save_all = PlatformMunger_save_all;
+function PlatformMunger_save_all() {
+ this.config_keeper.save_all();
+ this.platformJson.save();
+}
+
+// Apply a munge object to a single config file.
+// The remove parameter tells whether to add the change or remove it.
+PlatformMunger.prototype.apply_file_munge = PlatformMunger_apply_file_munge;
+function PlatformMunger_apply_file_munge(file, munge, remove) {
+ var self = this;
+
+ for (var selector in munge.parents) {
+ for (var xml_child in munge.parents[selector]) {
+ // this xml child is new, graft it (only if config file exists)
+ var config_file = self.config_keeper.get(self.project_dir, self.platform, file);
+ if (config_file.exists) {
+ if (remove) config_file.prune_child(selector, munge.parents[selector][xml_child]);
+ else config_file.graft_child(selector, munge.parents[selector][xml_child]);
+ }
+ }
+ }
+}
+
+
+PlatformMunger.prototype.remove_plugin_changes = remove_plugin_changes;
+function remove_plugin_changes(pluginInfo, is_top_level) {
+ var self = this;
+ var platform_config = self.platformJson.root;
+ var plugin_vars = is_top_level ?
+ platform_config.installed_plugins[pluginInfo.id] :
+ platform_config.dependent_plugins[pluginInfo.id];
+
+ // get config munge, aka how did this plugin change various config files
+ var config_munge = self.generate_plugin_config_munge(pluginInfo, plugin_vars);
+ // global munge looks at all plugins' changes to config files
+ var global_munge = platform_config.config_munge;
+ var munge = mungeutil.decrement_munge(global_munge, config_munge);
+
+ for (var file in munge.files) {
+ // CB-6976 Windows Universal Apps. Compatibility fix for existing plugins.
+ if (self.platform == 'windows' && file == 'package.appxmanifest' &&
+ !fs.existsSync(path.join(self.project_dir, 'package.appxmanifest'))) {
+ // New windows template separate manifest files for Windows10, Windows8.1 and WP8.1
+ var substs = ['package.phone.appxmanifest', 'package.windows.appxmanifest', 'package.windows10.appxmanifest'];
+ /* jshint loopfunc:true */
+ substs.forEach(function(subst) {
+ events.emit('verbose', 'Applying munge to ' + subst);
+ self.apply_file_munge(subst, munge.files[file], true);
+ });
+ /* jshint loopfunc:false */
+ }
+ self.apply_file_munge(file, munge.files[file], /* remove = */ true);
+ }
+
+ // Remove from installed_plugins
+ self.platformJson.removePlugin(pluginInfo.id, is_top_level);
+ return self;
+}
+
+
+PlatformMunger.prototype.add_plugin_changes = add_plugin_changes;
+function add_plugin_changes(pluginInfo, plugin_vars, is_top_level, should_increment) {
+ var self = this;
+ var platform_config = self.platformJson.root;
+
+ // get config munge, aka how should this plugin change various config files
+ var config_munge = self.generate_plugin_config_munge(pluginInfo, plugin_vars);
+ // global munge looks at all plugins' changes to config files
+
+ // TODO: The should_increment param is only used by cordova-cli and is going away soon.
+ // If should_increment is set to false, avoid modifying the global_munge (use clone)
+ // and apply the entire config_munge because it's already a proper subset of the global_munge.
+ var munge, global_munge;
+ if (should_increment) {
+ global_munge = platform_config.config_munge;
+ munge = mungeutil.increment_munge(global_munge, config_munge);
+ } else {
+ global_munge = mungeutil.clone_munge(platform_config.config_munge);
+ munge = config_munge;
+ }
+
+ for (var file in munge.files) {
+ // CB-6976 Windows Universal Apps. Compatibility fix for existing plugins.
+ if (self.platform == 'windows' && file == 'package.appxmanifest' &&
+ !fs.existsSync(path.join(self.project_dir, 'package.appxmanifest'))) {
+ var substs = ['package.phone.appxmanifest', 'package.windows.appxmanifest', 'package.windows10.appxmanifest'];
+ /* jshint loopfunc:true */
+ substs.forEach(function(subst) {
+ events.emit('verbose', 'Applying munge to ' + subst);
+ self.apply_file_munge(subst, munge.files[file]);
+ });
+ /* jshint loopfunc:false */
+ }
+ self.apply_file_munge(file, munge.files[file]);
+ }
+
+ // Move to installed/dependent_plugins
+ self.platformJson.addPlugin(pluginInfo.id, plugin_vars || {}, is_top_level);
+ return self;
+}
+
+
+// Load the global munge from platform json and apply all of it.
+// Used by cordova prepare to re-generate some config file from platform
+// defaults and the global munge.
+PlatformMunger.prototype.reapply_global_munge = reapply_global_munge ;
+function reapply_global_munge () {
+ var self = this;
+
+ var platform_config = self.platformJson.root;
+ var global_munge = platform_config.config_munge;
+ for (var file in global_munge.files) {
+ self.apply_file_munge(file, global_munge.files[file]);
+ }
+
+ return self;
+}
+
+
+// generate_plugin_config_munge
+// Generate the munge object from plugin.xml + vars
+PlatformMunger.prototype.generate_plugin_config_munge = generate_plugin_config_munge;
+function generate_plugin_config_munge(pluginInfo, vars) {
+ var self = this;
+
+ vars = vars || {};
+ var munge = { files: {} };
+ var changes = pluginInfo.getConfigFiles(self.platform);
+
+ // Demux 'package.appxmanifest' into relevant platform-specific appx manifests.
+ // Only spend the cycles if there are version-specific plugin settings
+ if (self.platform === 'windows' &&
+ changes.some(function(change) {
+ return ((typeof change.versions !== 'undefined') ||
+ (typeof change.deviceTarget !== 'undefined'));
+ }))
+ {
+ var manifests = {
+ 'windows': {
+ '8.1.0': 'package.windows.appxmanifest',
+ '10.0.0': 'package.windows10.appxmanifest'
+ },
+ 'phone': {
+ '8.1.0': 'package.phone.appxmanifest',
+ '10.0.0': 'package.windows10.appxmanifest'
+ },
+ 'all': {
+ '8.1.0': ['package.windows.appxmanifest', 'package.phone.appxmanifest'],
+ '10.0.0': 'package.windows10.appxmanifest'
+ }
+ };
+
+ var oldChanges = changes;
+ changes = [];
+
+ oldChanges.forEach(function(change, changeIndex) {
+ // Only support semver/device-target demux for package.appxmanifest
+ // Pass through in case something downstream wants to use it
+ if (change.target !== 'package.appxmanifest') {
+ changes.push(change);
+ return;
+ }
+
+ var hasVersion = (typeof change.versions !== 'undefined');
+ var hasTargets = (typeof change.deviceTarget !== 'undefined');
+
+ // No semver/device-target for this config-file, pass it through
+ if (!(hasVersion || hasTargets)) {
+ changes.push(change);
+ return;
+ }
+
+ var targetDeviceSet = hasTargets ? change.deviceTarget : 'all';
+ if (['windows', 'phone', 'all'].indexOf(targetDeviceSet) === -1) {
+ // target-device couldn't be resolved, fix it up here to a valid value
+ targetDeviceSet = 'all';
+ }
+ var knownWindowsVersionsForTargetDeviceSet = Object.keys(manifests[targetDeviceSet]);
+
+ // at this point, 'change' targets package.appxmanifest and has a version attribute
+ knownWindowsVersionsForTargetDeviceSet.forEach(function(winver) {
+ // This is a local function that creates the new replacement representing the
+ // mutation. Used to save code further down.
+ var createReplacement = function(manifestFile, originalChange) {
+ var replacement = {
+ target: manifestFile,
+ parent: originalChange.parent,
+ after: originalChange.after,
+ xmls: originalChange.xmls,
+ versions: originalChange.versions,
+ deviceTarget: originalChange.deviceTarget
+ };
+ return replacement;
+ };
+
+ // version doesn't satisfy, so skip
+ if (hasVersion && !semver.satisfies(winver, change.versions)) {
+ return;
+ }
+
+ var versionSpecificManifests = manifests[targetDeviceSet][winver];
+ if (versionSpecificManifests.constructor === Array) {
+ // e.g. all['8.1.0'] === ['pkg.windows.appxmanifest', 'pkg.phone.appxmanifest']
+ versionSpecificManifests.forEach(function(manifestFile) {
+ changes.push(createReplacement(manifestFile, change));
+ });
+ }
+ else {
+ // versionSpecificManifests is actually a single string
+ changes.push(createReplacement(versionSpecificManifests, change));
+ }
+ });
+ });
+ }
+
+ changes.forEach(function(change) {
+ change.xmls.forEach(function(xml) {
+ // 1. stringify each xml
+ var stringified = (new et.ElementTree(xml)).write({xml_declaration:false});
+ // interp vars
+ if (vars) {
+ Object.keys(vars).forEach(function(key) {
+ var regExp = new RegExp('\\$' + key, 'g');
+ stringified = stringified.replace(regExp, vars[key]);
+ });
+ }
+ // 2. add into munge
+ mungeutil.deep_add(munge, change.target, change.parent, { xml: stringified, count: 1, after: change.after });
+ });
+ });
+ return munge;
+}
+
+// Go over the prepare queue and apply the config munges for each plugin
+// that has been (un)installed.
+PlatformMunger.prototype.process = PlatformMunger_process;
+function PlatformMunger_process(plugins_dir) {
+ var self = this;
+ var platform_config = self.platformJson.root;
+
+ // Uninstallation first
+ platform_config.prepare_queue.uninstalled.forEach(function(u) {
+ var pluginInfo = self.pluginInfoProvider.get(path.join(plugins_dir, u.plugin));
+ self.remove_plugin_changes(pluginInfo, u.topLevel);
+ });
+
+ // Now handle installation
+ platform_config.prepare_queue.installed.forEach(function(u) {
+ var pluginInfo = self.pluginInfoProvider.get(path.join(plugins_dir, u.plugin));
+ self.add_plugin_changes(pluginInfo, u.vars, u.topLevel, true);
+ });
+
+ // Empty out installed/ uninstalled queues.
+ platform_config.prepare_queue.uninstalled = [];
+ platform_config.prepare_queue.installed = [];
+}
+/**** END of PlatformMunger ****/
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ConfigChanges/ConfigFile.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ConfigChanges/ConfigFile.js b/node_modules/cordova-common/src/ConfigChanges/ConfigFile.js
new file mode 100644
index 0000000..dd9ebbc
--- /dev/null
+++ b/node_modules/cordova-common/src/ConfigChanges/ConfigFile.js
@@ -0,0 +1,208 @@
+/*
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+
+var fs = require('fs');
+var path = require('path');
+
+var bplist = require('bplist-parser');
+var et = require('elementtree');
+var glob = require('glob');
+var plist = require('plist');
+
+var plist_helpers = require('../util/plist-helpers');
+var xml_helpers = require('../util/xml-helpers');
+
+/******************************************************************************
+* ConfigFile class
+*
+* Can load and keep various types of config files. Provides some functionality
+* specific to some file types such as grafting XML children. In most cases it
+* should be instantiated by ConfigKeeper.
+*
+* For plugin.xml files use as:
+* plugin_config = self.config_keeper.get(plugin_dir, '', 'plugin.xml');
+*
+* TODO: Consider moving it out to a separate file and maybe partially with
+* overrides in platform handlers.
+******************************************************************************/
+function ConfigFile(project_dir, platform, file_tag) {
+ this.project_dir = project_dir;
+ this.platform = platform;
+ this.file_tag = file_tag;
+ this.is_changed = false;
+
+ this.load();
+}
+
+// ConfigFile.load()
+ConfigFile.prototype.load = ConfigFile_load;
+function ConfigFile_load() {
+ var self = this;
+
+ // config file may be in a place not exactly specified in the target
+ var filepath = self.filepath = resolveConfigFilePath(self.project_dir, self.platform, self.file_tag);
+
+ if ( !filepath || !fs.existsSync(filepath) ) {
+ self.exists = false;
+ return;
+ }
+ self.exists = true;
+ self.mtime = fs.statSync(self.filepath).mtime;
+
+ var ext = path.extname(filepath);
+ // Windows8 uses an appxmanifest, and wp8 will likely use
+ // the same in a future release
+ if (ext == '.xml' || ext == '.appxmanifest') {
+ self.type = 'xml';
+ self.data = xml_helpers.parseElementtreeSync(filepath);
+ } else {
+ // plist file
+ self.type = 'plist';
+ // TODO: isBinaryPlist() reads the file and then parse re-reads it again.
+ // We always write out text plist, not binary.
+ // Do we still need to support binary plist?
+ // If yes, use plist.parseStringSync() and read the file once.
+ self.data = isBinaryPlist(filepath) ?
+ bplist.parseBuffer(fs.readFileSync(filepath)) :
+ plist.parse(fs.readFileSync(filepath, 'utf8'));
+ }
+}
+
+ConfigFile.prototype.save = function ConfigFile_save() {
+ var self = this;
+ if (self.type === 'xml') {
+ fs.writeFileSync(self.filepath, self.data.write({indent: 4}), 'utf-8');
+ } else {
+ // plist
+ var regExp = new RegExp('<string>[ \t\r\n]+?</string>', 'g');
+ fs.writeFileSync(self.filepath, plist.build(self.data).replace(regExp, '<string></string>'));
+ }
+ self.is_changed = false;
+};
+
+ConfigFile.prototype.graft_child = function ConfigFile_graft_child(selector, xml_child) {
+ var self = this;
+ var filepath = self.filepath;
+ var result;
+ if (self.type === 'xml') {
+ var xml_to_graft = [et.XML(xml_child.xml)];
+ result = xml_helpers.graftXML(self.data, xml_to_graft, selector, xml_child.after);
+ if ( !result) {
+ throw new Error('grafting xml at selector "' + selector + '" from "' + filepath + '" during config install went bad :(');
+ }
+ } else {
+ // plist file
+ result = plist_helpers.graftPLIST(self.data, xml_child.xml, selector);
+ if ( !result ) {
+ throw new Error('grafting to plist "' + filepath + '" during config install went bad :(');
+ }
+ }
+ self.is_changed = true;
+};
+
+ConfigFile.prototype.prune_child = function ConfigFile_prune_child(selector, xml_child) {
+ var self = this;
+ var filepath = self.filepath;
+ var result;
+ if (self.type === 'xml') {
+ var xml_to_graft = [et.XML(xml_child.xml)];
+ result = xml_helpers.pruneXML(self.data, xml_to_graft, selector);
+ } else {
+ // plist file
+ result = plist_helpers.prunePLIST(self.data, xml_child.xml, selector);
+ }
+ if (!result) {
+ var err_msg = 'Pruning at selector "' + selector + '" from "' + filepath + '" went bad.';
+ throw new Error(err_msg);
+ }
+ self.is_changed = true;
+};
+
+// Some config-file target attributes are not qualified with a full leading directory, or contain wildcards.
+// Resolve to a real path in this function.
+// TODO: getIOSProjectname is slow because of glob, try to avoid calling it several times per project.
+function resolveConfigFilePath(project_dir, platform, file) {
+ var filepath = path.join(project_dir, file);
+ var matches;
+
+ if (file.indexOf('*') > -1) {
+ // handle wildcards in targets using glob.
+ matches = glob.sync(path.join(project_dir, '**', file));
+ if (matches.length) filepath = matches[0];
+
+ // [CB-5989] multiple Info.plist files may exist. default to $PROJECT_NAME-Info.plist
+ if(matches.length > 1 && file.indexOf('-Info.plist')>-1){
+ var plistName = getIOSProjectname(project_dir)+'-Info.plist';
+ for (var i=0; i < matches.length; i++) {
+ if(matches[i].indexOf(plistName) > -1){
+ filepath = matches[i];
+ break;
+ }
+ }
+ }
+ return filepath;
+ }
+
+ // special-case config.xml target that is just "config.xml". This should be resolved to the real location of the file.
+ // TODO: move the logic that contains the locations of config.xml from cordova CLI into plugman.
+ if (file == 'config.xml') {
+ if (platform == 'ubuntu') {
+ filepath = path.join(project_dir, 'config.xml');
+ } else if (platform == 'ios') {
+ var iospath = getIOSProjectname(project_dir);
+ filepath = path.join(project_dir,iospath, 'config.xml');
+ } else if (platform == 'android') {
+ filepath = path.join(project_dir, 'res', 'xml', 'config.xml');
+ } else {
+ matches = glob.sync(path.join(project_dir, '**', 'config.xml'));
+ if (matches.length) filepath = matches[0];
+ }
+ return filepath;
+ }
+
+ // None of the special cases matched, returning project_dir/file.
+ return filepath;
+}
+
+// Find out the real name of an iOS project
+// TODO: glob is slow, need a better way or caching, or avoid using more than once.
+function getIOSProjectname(project_dir) {
+ var matches = glob.sync(path.join(project_dir, '*.xcodeproj'));
+ var iospath;
+ if (matches.length === 1) {
+ iospath = path.basename(matches[0],'.xcodeproj');
+ } else {
+ var msg;
+ if (matches.length === 0) {
+ msg = 'Does not appear to be an xcode project, no xcode project file in ' + project_dir;
+ } else {
+ msg = 'There are multiple *.xcodeproj dirs in ' + project_dir;
+ }
+ throw new Error(msg);
+ }
+ return iospath;
+}
+
+// determine if a plist file is binary
+function isBinaryPlist(filename) {
+ // I wish there was a synchronous way to read only the first 6 bytes of a
+ // file. This is wasteful :/
+ var buf = '' + fs.readFileSync(filename, 'utf8');
+ // binary plists start with a magic header, "bplist"
+ return buf.substring(0, 6) === 'bplist';
+}
+
+module.exports = ConfigFile;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ConfigChanges/ConfigKeeper.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ConfigChanges/ConfigKeeper.js b/node_modules/cordova-common/src/ConfigChanges/ConfigKeeper.js
new file mode 100644
index 0000000..894e922
--- /dev/null
+++ b/node_modules/cordova-common/src/ConfigChanges/ConfigKeeper.js
@@ -0,0 +1,65 @@
+/*
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+/* jshint sub:true */
+
+var path = require('path');
+var ConfigFile = require('./ConfigFile');
+
+/******************************************************************************
+* ConfigKeeper class
+*
+* Used to load and store config files to avoid re-parsing and writing them out
+* multiple times.
+*
+* The config files are referred to by a fake path constructed as
+* project_dir/platform/file
+* where file is the name used for the file in config munges.
+******************************************************************************/
+function ConfigKeeper(project_dir, plugins_dir) {
+ this.project_dir = project_dir;
+ this.plugins_dir = plugins_dir;
+ this._cached = {};
+}
+
+ConfigKeeper.prototype.get = function ConfigKeeper_get(project_dir, platform, file) {
+ var self = this;
+
+ // This fixes a bug with older plugins - when specifying config xml instead of res/xml/config.xml
+ // https://issues.apache.org/jira/browse/CB-6414
+ if(file == 'config.xml' && platform == 'android'){
+ file = 'res/xml/config.xml';
+ }
+ var fake_path = path.join(project_dir, platform, file);
+
+ if (self._cached[fake_path]) {
+ return self._cached[fake_path];
+ }
+ // File was not cached, need to load.
+ var config_file = new ConfigFile(project_dir, platform, file);
+ self._cached[fake_path] = config_file;
+ return config_file;
+};
+
+
+ConfigKeeper.prototype.save_all = function ConfigKeeper_save_all() {
+ var self = this;
+ Object.keys(self._cached).forEach(function (fake_path) {
+ var config_file = self._cached[fake_path];
+ if (config_file.is_changed) config_file.save();
+ });
+};
+
+module.exports = ConfigKeeper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ConfigChanges/munge-util.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ConfigChanges/munge-util.js b/node_modules/cordova-common/src/ConfigChanges/munge-util.js
new file mode 100644
index 0000000..307b3c1
--- /dev/null
+++ b/node_modules/cordova-common/src/ConfigChanges/munge-util.js
@@ -0,0 +1,160 @@
+/*
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+/* jshint sub:true */
+
+var _ = require('underscore');
+
+// add the count of [key1][key2]...[keyN] to obj
+// return true if it didn't exist before
+exports.deep_add = function deep_add(obj, keys /* or key1, key2 .... */ ) {
+ if ( !Array.isArray(keys) ) {
+ keys = Array.prototype.slice.call(arguments, 1);
+ }
+
+ return exports.process_munge(obj, true/*createParents*/, function (parentArray, k) {
+ var found = _.find(parentArray, function(element) {
+ return element.xml == k.xml;
+ });
+ if (found) {
+ found.after = found.after || k.after;
+ found.count += k.count;
+ } else {
+ parentArray.push(k);
+ }
+ return !found;
+ }, keys);
+};
+
+// decrement the count of [key1][key2]...[keyN] from obj and remove if it reaches 0
+// return true if it was removed or not found
+exports.deep_remove = function deep_remove(obj, keys /* or key1, key2 .... */ ) {
+ if ( !Array.isArray(keys) ) {
+ keys = Array.prototype.slice.call(arguments, 1);
+ }
+
+ var result = exports.process_munge(obj, false/*createParents*/, function (parentArray, k) {
+ var index = -1;
+ var found = _.find(parentArray, function (element) {
+ index++;
+ return element.xml == k.xml;
+ });
+ if (found) {
+ found.count -= k.count;
+ if (found.count > 0) {
+ return false;
+ }
+ else {
+ parentArray.splice(index, 1);
+ }
+ }
+ return undefined;
+ }, keys);
+
+ return typeof result === 'undefined' ? true : result;
+};
+
+// search for [key1][key2]...[keyN]
+// return the object or undefined if not found
+exports.deep_find = function deep_find(obj, keys /* or key1, key2 .... */ ) {
+ if ( !Array.isArray(keys) ) {
+ keys = Array.prototype.slice.call(arguments, 1);
+ }
+
+ return exports.process_munge(obj, false/*createParents?*/, function (parentArray, k) {
+ return _.find(parentArray, function (element) {
+ return element.xml == (k.xml || k);
+ });
+ }, keys);
+};
+
+// Execute func passing it the parent array and the xmlChild key.
+// When createParents is true, add the file and parent items they are missing
+// When createParents is false, stop and return undefined if the file and/or parent items are missing
+
+exports.process_munge = function process_munge(obj, createParents, func, keys /* or key1, key2 .... */ ) {
+ if ( !Array.isArray(keys) ) {
+ keys = Array.prototype.slice.call(arguments, 1);
+ }
+ var k = keys[0];
+ if (keys.length == 1) {
+ return func(obj, k);
+ } else if (keys.length == 2) {
+ if (!obj.parents[k] && !createParents) {
+ return undefined;
+ }
+ obj.parents[k] = obj.parents[k] || [];
+ return exports.process_munge(obj.parents[k], createParents, func, keys.slice(1));
+ } else if (keys.length == 3){
+ if (!obj.files[k] && !createParents) {
+ return undefined;
+ }
+ obj.files[k] = obj.files[k] || { parents: {} };
+ return exports.process_munge(obj.files[k], createParents, func, keys.slice(1));
+ } else {
+ throw new Error('Invalid key format. Must contain at most 3 elements (file, parent, xmlChild).');
+ }
+};
+
+// All values from munge are added to base as
+// base[file][selector][child] += munge[file][selector][child]
+// Returns a munge object containing values that exist in munge
+// but not in base.
+exports.increment_munge = function increment_munge(base, munge) {
+ var diff = { files: {} };
+
+ for (var file in munge.files) {
+ for (var selector in munge.files[file].parents) {
+ for (var xml_child in munge.files[file].parents[selector]) {
+ var val = munge.files[file].parents[selector][xml_child];
+ // if node not in base, add it to diff and base
+ // else increment it's value in base without adding to diff
+ var newlyAdded = exports.deep_add(base, [file, selector, val]);
+ if (newlyAdded) {
+ exports.deep_add(diff, file, selector, val);
+ }
+ }
+ }
+ }
+ return diff;
+};
+
+// Update the base munge object as
+// base[file][selector][child] -= munge[file][selector][child]
+// nodes that reached zero value are removed from base and added to the returned munge
+// object.
+exports.decrement_munge = function decrement_munge(base, munge) {
+ var zeroed = { files: {} };
+
+ for (var file in munge.files) {
+ for (var selector in munge.files[file].parents) {
+ for (var xml_child in munge.files[file].parents[selector]) {
+ var val = munge.files[file].parents[selector][xml_child];
+ // if node not in base, add it to diff and base
+ // else increment it's value in base without adding to diff
+ var removed = exports.deep_remove(base, [file, selector, val]);
+ if (removed) {
+ exports.deep_add(zeroed, file, selector, val);
+ }
+ }
+ }
+ }
+ return zeroed;
+};
+
+// For better readability where used
+exports.clone_munge = function clone_munge(munge) {
+ return exports.increment_munge({}, munge);
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ConfigParser/ConfigParser.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ConfigParser/ConfigParser.js b/node_modules/cordova-common/src/ConfigParser/ConfigParser.js
new file mode 100644
index 0000000..7abddf6
--- /dev/null
+++ b/node_modules/cordova-common/src/ConfigParser/ConfigParser.js
@@ -0,0 +1,499 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+/* jshint sub:true */
+
+var et = require('elementtree'),
+ xml= require('../util/xml-helpers'),
+ CordovaError = require('../CordovaError/CordovaError'),
+ fs = require('fs'),
+ events = require('../events');
+
+
+/** Wraps a config.xml file */
+function ConfigParser(path) {
+ this.path = path;
+ try {
+ this.doc = xml.parseElementtreeSync(path);
+ this.cdvNamespacePrefix = getCordovaNamespacePrefix(this.doc);
+ et.register_namespace(this.cdvNamespacePrefix, 'http://cordova.apache.org/ns/1.0');
+ } catch (e) {
+ console.error('Parsing '+path+' failed');
+ throw e;
+ }
+ var r = this.doc.getroot();
+ if (r.tag !== 'widget') {
+ throw new CordovaError(path + ' has incorrect root node name (expected "widget", was "' + r.tag + '")');
+ }
+}
+
+function getNodeTextSafe(el) {
+ return el && el.text && el.text.trim();
+}
+
+function findOrCreate(doc, name) {
+ var ret = doc.find(name);
+ if (!ret) {
+ ret = new et.Element(name);
+ doc.getroot().append(ret);
+ }
+ return ret;
+}
+
+function getCordovaNamespacePrefix(doc){
+ var rootAtribs = Object.getOwnPropertyNames(doc.getroot().attrib);
+ var prefix = 'cdv';
+ for (var j = 0; j < rootAtribs.length; j++ ) {
+ if(rootAtribs[j].indexOf('xmlns:') === 0 &&
+ doc.getroot().attrib[rootAtribs[j]] === 'http://cordova.apache.org/ns/1.0'){
+ var strings = rootAtribs[j].split(':');
+ prefix = strings[1];
+ break;
+ }
+ }
+ return prefix;
+}
+
+/**
+ * Finds the value of an element's attribute
+ * @param {String} attributeName Name of the attribute to search for
+ * @param {Array} elems An array of ElementTree nodes
+ * @return {String}
+ */
+function findElementAttributeValue(attributeName, elems) {
+
+ elems = Array.isArray(elems) ? elems : [ elems ];
+
+ var value = elems.filter(function (elem) {
+ return elem.attrib.name.toLowerCase() === attributeName.toLowerCase();
+ }).map(function (filteredElems) {
+ return filteredElems.attrib.value;
+ }).pop();
+
+ return value ? value : '';
+}
+
+ConfigParser.prototype = {
+ packageName: function(id) {
+ return this.doc.getroot().attrib['id'];
+ },
+ setPackageName: function(id) {
+ this.doc.getroot().attrib['id'] = id;
+ },
+ android_packageName: function() {
+ return this.doc.getroot().attrib['android-packageName'];
+ },
+ android_activityName: function() {
+ return this.doc.getroot().attrib['android-activityName'];
+ },
+ ios_CFBundleIdentifier: function() {
+ return this.doc.getroot().attrib['ios-CFBundleIdentifier'];
+ },
+ name: function() {
+ return getNodeTextSafe(this.doc.find('name'));
+ },
+ setName: function(name) {
+ var el = findOrCreate(this.doc, 'name');
+ el.text = name;
+ },
+ description: function() {
+ return getNodeTextSafe(this.doc.find('description'));
+ },
+ setDescription: function(text) {
+ var el = findOrCreate(this.doc, 'description');
+ el.text = text;
+ },
+ version: function() {
+ return this.doc.getroot().attrib['version'];
+ },
+ windows_packageVersion: function() {
+ return this.doc.getroot().attrib('windows-packageVersion');
+ },
+ android_versionCode: function() {
+ return this.doc.getroot().attrib['android-versionCode'];
+ },
+ ios_CFBundleVersion: function() {
+ return this.doc.getroot().attrib['ios-CFBundleVersion'];
+ },
+ setVersion: function(value) {
+ this.doc.getroot().attrib['version'] = value;
+ },
+ author: function() {
+ return getNodeTextSafe(this.doc.find('author'));
+ },
+ getGlobalPreference: function (name) {
+ return findElementAttributeValue(name, this.doc.findall('preference'));
+ },
+ setGlobalPreference: function (name, value) {
+ var pref = this.doc.find('preference[@name="' + name + '"]');
+ if (!pref) {
+ pref = new et.Element('preference');
+ pref.attrib.name = name;
+ this.doc.getroot().append(pref);
+ }
+ pref.attrib.value = value;
+ },
+ getPlatformPreference: function (name, platform) {
+ return findElementAttributeValue(name, this.doc.findall('platform[@name=\'' + platform + '\']/preference'));
+ },
+ getPreference: function(name, platform) {
+
+ var platformPreference = '';
+
+ if (platform) {
+ platformPreference = this.getPlatformPreference(name, platform);
+ }
+
+ return platformPreference ? platformPreference : this.getGlobalPreference(name);
+
+ },
+ /**
+ * Returns all resources for the platform specified.
+ * @param {String} platform The platform.
+ * @param {string} resourceName Type of static resources to return.
+ * "icon" and "splash" currently supported.
+ * @return {Array} Resources for the platform specified.
+ */
+ getStaticResources: function(platform, resourceName) {
+ var ret = [],
+ staticResources = [];
+ if (platform) { // platform specific icons
+ this.doc.findall('platform[@name=\'' + platform + '\']/' + resourceName).forEach(function(elt){
+ elt.platform = platform; // mark as platform specific resource
+ staticResources.push(elt);
+ });
+ }
+ // root level resources
+ staticResources = staticResources.concat(this.doc.findall(resourceName));
+ // parse resource elements
+ var that = this;
+ staticResources.forEach(function (elt) {
+ var res = {};
+ res.src = elt.attrib.src;
+ res.density = elt.attrib['density'] || elt.attrib[that.cdvNamespacePrefix+':density'] || elt.attrib['gap:density'];
+ res.platform = elt.platform || null; // null means icon represents default icon (shared between platforms)
+ res.width = +elt.attrib.width || undefined;
+ res.height = +elt.attrib.height || undefined;
+
+ // default icon
+ if (!res.width && !res.height && !res.density) {
+ ret.defaultResource = res;
+ }
+ ret.push(res);
+ });
+
+ /**
+ * Returns resource with specified width and/or height.
+ * @param {number} width Width of resource.
+ * @param {number} height Height of resource.
+ * @return {Resource} Resource object or null if not found.
+ */
+ ret.getBySize = function(width, height) {
+ return ret.filter(function(res) {
+ if (!res.width && !res.height) {
+ return false;
+ }
+ return ((!res.width || (width == res.width)) &&
+ (!res.height || (height == res.height)));
+ })[0] || null;
+ };
+
+ /**
+ * Returns resource with specified density.
+ * @param {string} density Density of resource.
+ * @return {Resource} Resource object or null if not found.
+ */
+ ret.getByDensity = function(density) {
+ return ret.filter(function(res) {
+ return res.density == density;
+ })[0] || null;
+ };
+
+ /** Returns default icons */
+ ret.getDefault = function() {
+ return ret.defaultResource;
+ };
+
+ return ret;
+ },
+
+ /**
+ * Returns all icons for specific platform.
+ * @param {string} platform Platform name
+ * @return {Resource[]} Array of icon objects.
+ */
+ getIcons: function(platform) {
+ return this.getStaticResources(platform, 'icon');
+ },
+
+ /**
+ * Returns all splash images for specific platform.
+ * @param {string} platform Platform name
+ * @return {Resource[]} Array of Splash objects.
+ */
+ getSplashScreens: function(platform) {
+ return this.getStaticResources(platform, 'splash');
+ },
+
+ /**
+ * Returns all hook scripts for the hook type specified.
+ * @param {String} hook The hook type.
+ * @param {Array} platforms Platforms to look for scripts into (root scripts will be included as well).
+ * @return {Array} Script elements.
+ */
+ getHookScripts: function(hook, platforms) {
+ var self = this;
+ var scriptElements = self.doc.findall('./hook');
+
+ if(platforms) {
+ platforms.forEach(function (platform) {
+ scriptElements = scriptElements.concat(self.doc.findall('./platform[@name="' + platform + '"]/hook'));
+ });
+ }
+
+ function filterScriptByHookType(el) {
+ return el.attrib.src && el.attrib.type && el.attrib.type.toLowerCase() === hook;
+ }
+
+ return scriptElements.filter(filterScriptByHookType);
+ },
+ /**
+ * Returns a list of plugin (IDs).
+ *
+ * This function also returns any plugin's that
+ * were defined using the legacy <feature> tags.
+ * @return {string[]} Array of plugin IDs
+ */
+ getPluginIdList: function () {
+ var plugins = this.doc.findall('plugin');
+ var result = plugins.map(function(plugin){
+ return plugin.attrib.name;
+ });
+ var features = this.doc.findall('feature');
+ features.forEach(function(element ){
+ var idTag = element.find('./param[@name="id"]');
+ if(idTag){
+ result.push(idTag.attrib.value);
+ }
+ });
+ return result;
+ },
+ getPlugins: function () {
+ return this.getPluginIdList().map(function (pluginId) {
+ return this.getPlugin(pluginId);
+ }, this);
+ },
+ /**
+ * Adds a plugin element. Does not check for duplicates.
+ * @name addPlugin
+ * @function
+ * @param {object} attributes name and spec are supported
+ * @param {Array|object} variables name, value or arbitary object
+ */
+ addPlugin: function (attributes, variables) {
+ if (!attributes && !attributes.name) return;
+ var el = new et.Element('plugin');
+ el.attrib.name = attributes.name;
+ if (attributes.spec) {
+ el.attrib.spec = attributes.spec;
+ }
+
+ // support arbitrary object as variables source
+ if (variables && typeof variables === 'object' && !Array.isArray(variables)) {
+ variables = Object.keys(variables)
+ .map(function (variableName) {
+ return {name: variableName, value: variables[variableName]};
+ });
+ }
+
+ if (variables) {
+ variables.forEach(function (variable) {
+ el.append(new et.Element('variable', { name: variable.name, value: variable.value }));
+ });
+ }
+ this.doc.getroot().append(el);
+ },
+ /**
+ * Retrives the plugin with the given id or null if not found.
+ *
+ * This function also returns any plugin's that
+ * were defined using the legacy <feature> tags.
+ * @name getPlugin
+ * @function
+ * @param {String} id
+ * @returns {object} plugin including any variables
+ */
+ getPlugin: function(id){
+ if(!id){
+ return undefined;
+ }
+ var pluginElement = this.doc.find('./plugin/[@name="' + id + '"]');
+ if (null === pluginElement) {
+ var legacyFeature = this.doc.find('./feature/param[@name="id"][@value="' + id + '"]/..');
+ if(legacyFeature){
+ events.emit('log', 'Found deprecated feature entry for ' + id +' in config.xml.');
+ return featureToPlugin(legacyFeature);
+ }
+ return undefined;
+ }
+ var plugin = {};
+
+ plugin.name = pluginElement.attrib.name;
+ plugin.spec = pluginElement.attrib.spec || pluginElement.attrib.src || pluginElement.attrib.version;
+ plugin.variables = {};
+ var variableElements = pluginElement.findall('variable');
+ variableElements.forEach(function(varElement){
+ var name = varElement.attrib.name;
+ var value = varElement.attrib.value;
+ if(name){
+ plugin.variables[name] = value;
+ }
+ });
+ return plugin;
+ },
+ /**
+ * Remove the plugin entry with give name (id).
+ *
+ * This function also operates on any plugin's that
+ * were defined using the legacy <feature> tags.
+ * @name removePlugin
+ * @function
+ * @param id name of the plugin
+ */
+ removePlugin: function(id){
+ if(id){
+ var plugins = this.doc.findall('./plugin/[@name="' + id + '"]')
+ .concat(this.doc.findall('./feature/param[@name="id"][@value="' + id + '"]/..'));
+ var children = this.doc.getroot().getchildren();
+ plugins.forEach(function (plugin) {
+ var idx = children.indexOf(plugin);
+ if (idx > -1) {
+ children.splice(idx, 1);
+ }
+ });
+ }
+ },
+
+ // Add any element to the root
+ addElement: function(name, attributes) {
+ var el = et.Element(name);
+ for (var a in attributes) {
+ el.attrib[a] = attributes[a];
+ }
+ this.doc.getroot().append(el);
+ },
+
+ /**
+ * Adds an engine. Does not check for duplicates.
+ * @param {String} name the engine name
+ * @param {String} spec engine source location or version (optional)
+ */
+ addEngine: function(name, spec){
+ if(!name) return;
+ var el = et.Element('engine');
+ el.attrib.name = name;
+ if(spec){
+ el.attrib.spec = spec;
+ }
+ this.doc.getroot().append(el);
+ },
+ /**
+ * Removes all the engines with given name
+ * @param {String} name the engine name.
+ */
+ removeEngine: function(name){
+ var engines = this.doc.findall('./engine/[@name="' +name+'"]');
+ for(var i=0; i < engines.length; i++){
+ var children = this.doc.getroot().getchildren();
+ var idx = children.indexOf(engines[i]);
+ if(idx > -1){
+ children.splice(idx,1);
+ }
+ }
+ },
+ getEngines: function(){
+ var engines = this.doc.findall('./engine');
+ return engines.map(function(engine){
+ var spec = engine.attrib.spec || engine.attrib.version;
+ return {
+ 'name': engine.attrib.name,
+ 'spec': spec ? spec : null
+ };
+ });
+ },
+ /* Get all the access tags */
+ getAccesses: function() {
+ var accesses = this.doc.findall('./access');
+ return accesses.map(function(access){
+ var minimum_tls_version = access.attrib['minimum-tls-version']; /* String */
+ var requires_forward_secrecy = access.attrib['requires-forward-secrecy']; /* Boolean */
+ return {
+ 'origin': access.attrib.origin,
+ 'minimum_tls_version': minimum_tls_version,
+ 'requires_forward_secrecy' : requires_forward_secrecy
+ };
+ });
+ },
+ /* Get all the allow-navigation tags */
+ getAllowNavigations: function() {
+ var allow_navigations = this.doc.findall('./allow-navigation');
+ return allow_navigations.map(function(allow_navigation){
+ var minimum_tls_version = allow_navigation.attrib['minimum-tls-version']; /* String */
+ var requires_forward_secrecy = allow_navigation.attrib['requires-forward-secrecy']; /* Boolean */
+ return {
+ 'href': allow_navigation.attrib.href,
+ 'minimum_tls_version': minimum_tls_version,
+ 'requires_forward_secrecy' : requires_forward_secrecy
+ };
+ });
+ },
+ write:function() {
+ fs.writeFileSync(this.path, this.doc.write({indent: 4}), 'utf-8');
+ }
+};
+
+function featureToPlugin(featureElement) {
+ var plugin = {};
+ plugin.variables = [];
+ var pluginVersion,
+ pluginSrc;
+
+ var nodes = featureElement.findall('param');
+ nodes.forEach(function (element) {
+ var n = element.attrib.name;
+ var v = element.attrib.value;
+ if (n === 'id') {
+ plugin.name = v;
+ } else if (n === 'version') {
+ pluginVersion = v;
+ } else if (n === 'url' || n === 'installPath') {
+ pluginSrc = v;
+ } else {
+ plugin.variables[n] = v;
+ }
+ });
+
+ var spec = pluginSrc || pluginVersion;
+ if (spec) {
+ plugin.spec = spec;
+ }
+
+ return plugin;
+}
+module.exports = ConfigParser;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/ConfigParser/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/ConfigParser/README.md b/node_modules/cordova-common/src/ConfigParser/README.md
new file mode 100644
index 0000000..e5cd1bf
--- /dev/null
+++ b/node_modules/cordova-common/src/ConfigParser/README.md
@@ -0,0 +1,86 @@
+<!--
+#
+# Licensed to the Apache Software Foundation (ASF) under one
+# or more contributor license agreements. See the NOTICE file
+# distributed with this work for additional information
+# regarding copyright ownership. The ASF licenses this file
+# to you under the Apache License, Version 2.0 (the
+# "License"); you may not use this file except in compliance
+# with the License. You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing,
+# software distributed under the License is distributed on an
+# "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+# KIND, either express or implied. See the License for the
+# specific language governing permissions and limitations
+# under the License.
+#
+-->
+
+# Cordova-Lib
+
+## ConfigParser
+
+wraps a valid cordova config.xml file
+
+### Usage
+
+### Include the ConfigParser module in a projet
+
+ var ConfigParser = require('cordova-lib').configparser;
+
+### Create a new ConfigParser
+
+ var config = new ConfigParser('path/to/config/xml/');
+
+### Utility Functions
+
+#### packageName(id)
+returns document root 'id' attribute value
+#### Usage
+
+ config.packageName: function(id)
+
+/*
+ * sets document root element 'id' attribute to @id
+ *
+ * @id - new id value
+ *
+ */
+#### setPackageName(id)
+set document root 'id' attribute to
+ function(id) {
+ this.doc.getroot().attrib['id'] = id;
+ },
+
+###
+ name: function() {
+ return getNodeTextSafe(this.doc.find('name'));
+ },
+ setName: function(name) {
+ var el = findOrCreate(this.doc, 'name');
+ el.text = name;
+ },
+
+### read the description element
+
+ config.description()
+
+ var text = "New and improved description of App"
+ setDescription(text)
+
+### version management
+ version()
+ android_versionCode()
+ ios_CFBundleVersion()
+ setVersion()
+
+### read author element
+
+ config.author();
+
+### read preference
+
+ config.getPreference(name);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/CordovaError/CordovaError.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/CordovaError/CordovaError.js b/node_modules/cordova-common/src/CordovaError/CordovaError.js
new file mode 100644
index 0000000..7262448
--- /dev/null
+++ b/node_modules/cordova-common/src/CordovaError/CordovaError.js
@@ -0,0 +1,91 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+/* jshint proto:true */
+
+var EOL = require('os').EOL;
+
+/**
+ * A derived exception class. See usage example in cli.js
+ * Based on:
+ * stackoverflow.com/questions/1382107/whats-a-good-way-to-extend-error-in-javascript/8460753#8460753
+ * @param {String} message Error message
+ * @param {Number} [code=0] Error code
+ * @param {CordovaExternalToolErrorContext} [context] External tool error context object
+ * @constructor
+ */
+function CordovaError(message, code, context) {
+ Error.captureStackTrace(this, this.constructor);
+ this.name = this.constructor.name;
+ this.message = message;
+ this.code = code || CordovaError.UNKNOWN_ERROR;
+ this.context = context;
+}
+CordovaError.prototype.__proto__ = Error.prototype;
+
+// TODO: Extend error codes according the projects specifics
+CordovaError.UNKNOWN_ERROR = 0;
+CordovaError.EXTERNAL_TOOL_ERROR = 1;
+
+/**
+ * Translates instance's error code number into error code name, e.g. 0 -> UNKNOWN_ERROR
+ * @returns {string} Error code string name
+ */
+CordovaError.prototype.getErrorCodeName = function() {
+ for(var key in CordovaError) {
+ if(CordovaError.hasOwnProperty(key)) {
+ if(CordovaError[key] === this.code) {
+ return key;
+ }
+ }
+ }
+};
+
+/**
+ * Converts CordovaError instance to string representation
+ * @param {Boolean} [isVerbose] Set up verbose mode. Used to provide more
+ * details including information about error code name and context
+ * @return {String} Stringified error representation
+ */
+CordovaError.prototype.toString = function(isVerbose) {
+ var message = '', codePrefix = '';
+
+ if(this.code !== CordovaError.UNKNOWN_ERROR) {
+ codePrefix = 'code: ' + this.code + (isVerbose ? (' (' + this.getErrorCodeName() + ')') : '') + ' ';
+ }
+
+ if(this.code === CordovaError.EXTERNAL_TOOL_ERROR) {
+ if(typeof this.context !== 'undefined') {
+ if(isVerbose) {
+ message = codePrefix + EOL + this.context.toString(isVerbose) + '\n failed with an error: ' +
+ this.message + EOL + 'Stack trace: ' + this.stack;
+ } else {
+ message = codePrefix + '\'' + this.context.toString(isVerbose) + '\' ' + this.message;
+ }
+ } else {
+ message = 'External tool failed with an error: ' + this.message;
+ }
+ } else {
+ message = isVerbose ? codePrefix + this.stack : codePrefix + this.message;
+ }
+
+ return message;
+};
+
+module.exports = CordovaError;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/CordovaError/CordovaExternalToolErrorContext.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/CordovaError/CordovaExternalToolErrorContext.js b/node_modules/cordova-common/src/CordovaError/CordovaExternalToolErrorContext.js
new file mode 100644
index 0000000..ca9a4aa
--- /dev/null
+++ b/node_modules/cordova-common/src/CordovaError/CordovaExternalToolErrorContext.js
@@ -0,0 +1,48 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+ */
+
+/* jshint proto:true */
+
+var path = require('path');
+
+/**
+ * @param {String} cmd Command full path
+ * @param {String[]} args Command args
+ * @param {String} [cwd] Command working directory
+ * @constructor
+ */
+function CordovaExternalToolErrorContext(cmd, args, cwd) {
+ this.cmd = cmd;
+ // Helper field for readability
+ this.cmdShortName = path.basename(cmd);
+ this.args = args;
+ this.cwd = cwd;
+}
+
+CordovaExternalToolErrorContext.prototype.toString = function(isVerbose) {
+ if(isVerbose) {
+ return 'External tool \'' + this.cmdShortName + '\'' +
+ '\nCommand full path: ' + this.cmd + '\nCommand args: ' + this.args +
+ (typeof this.cwd !== 'undefined' ? '\nCommand cwd: ' + this.cwd : '');
+ }
+
+ return this.cmdShortName;
+};
+
+module.exports = CordovaExternalToolErrorContext;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[41/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/shuffle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/shuffle.js b/node_modules/lodash-node/compat/collections/shuffle.js
new file mode 100644
index 0000000..d37777c
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/shuffle.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom'),
+ forEach = require('./forEach');
+
+/**
+ * Creates an array of shuffled values, using a version of the Fisher-Yates
+ * shuffle. See http://en.wikipedia.org/wiki/Fisher-Yates_shuffle.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to shuffle.
+ * @returns {Array} Returns a new shuffled collection.
+ * @example
+ *
+ * _.shuffle([1, 2, 3, 4, 5, 6]);
+ * // => [4, 1, 6, 3, 5, 2]
+ */
+function shuffle(collection) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ var rand = baseRandom(0, ++index);
+ result[index] = result[rand];
+ result[rand] = value;
+ });
+ return result;
+}
+
+module.exports = shuffle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/size.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/size.js b/node_modules/lodash-node/compat/collections/size.js
new file mode 100644
index 0000000..bfc821f
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/size.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys');
+
+/**
+ * Gets the size of the `collection` by returning `collection.length` for arrays
+ * and array-like objects or the number of own enumerable properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @returns {number} Returns `collection.length` or number of own enumerable properties.
+ * @example
+ *
+ * _.size([1, 2]);
+ * // => 2
+ *
+ * _.size({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => 3
+ *
+ * _.size('pebbles');
+ * // => 7
+ */
+function size(collection) {
+ var length = collection ? collection.length : 0;
+ return typeof length == 'number' ? length : keys(collection).length;
+}
+
+module.exports = size;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/some.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/some.js b/node_modules/lodash-node/compat/collections/some.js
new file mode 100644
index 0000000..c8d7ee5
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/some.js
@@ -0,0 +1,76 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Checks if the callback returns a truey value for **any** element of a
+ * collection. The function returns as soon as it finds a passing value and
+ * does not iterate over the entire collection. The callback is bound to
+ * `thisArg` and invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias any
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if any element passed the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.some(characters, 'blocked');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.some(characters, { 'age': 1 });
+ * // => false
+ */
+function some(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ if ((result = callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ baseEach(collection, function(value, index, collection) {
+ return !(result = callback(value, index, collection));
+ });
+ }
+ return !!result;
+}
+
+module.exports = some;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/sortBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/sortBy.js b/node_modules/lodash-node/compat/collections/sortBy.js
new file mode 100644
index 0000000..777b152
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/sortBy.js
@@ -0,0 +1,101 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var compareAscending = require('../internals/compareAscending'),
+ createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ getArray = require('../internals/getArray'),
+ getObject = require('../internals/getObject'),
+ isArray = require('../objects/isArray'),
+ map = require('./map'),
+ releaseArray = require('../internals/releaseArray'),
+ releaseObject = require('../internals/releaseObject');
+
+/**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in a collection through the callback. This method
+ * performs a stable sort, that is, it will preserve the original sort order
+ * of equal elements. The callback is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an array of property names is provided for `callback` the collection
+ * will be sorted by each property value.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Array|Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of sorted elements.
+ * @example
+ *
+ * _.sortBy([1, 2, 3], function(num) { return Math.sin(num); });
+ * // => [3, 1, 2]
+ *
+ * _.sortBy([1, 2, 3], function(num) { return this.sin(num); }, Math);
+ * // => [3, 1, 2]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'barney', 'age': 26 },
+ * { 'name': 'fred', 'age': 30 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(_.sortBy(characters, 'age'), _.values);
+ * // => [['barney', 26], ['fred', 30], ['barney', 36], ['fred', 40]]
+ *
+ * // sorting by multiple properties
+ * _.map(_.sortBy(characters, ['name', 'age']), _.values);
+ * // = > [['barney', 26], ['barney', 36], ['fred', 30], ['fred', 40]]
+ */
+function sortBy(collection, callback, thisArg) {
+ var index = -1,
+ isArr = isArray(callback),
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ if (!isArr) {
+ callback = createCallback(callback, thisArg, 3);
+ }
+ forEach(collection, function(value, key, collection) {
+ var object = result[++index] = getObject();
+ if (isArr) {
+ object.criteria = map(callback, function(key) { return value[key]; });
+ } else {
+ (object.criteria = getArray())[0] = callback(value, key, collection);
+ }
+ object.index = index;
+ object.value = value;
+ });
+
+ length = result.length;
+ result.sort(compareAscending);
+ while (length--) {
+ var object = result[length];
+ result[length] = object.value;
+ if (!isArr) {
+ releaseArray(object.criteria);
+ }
+ releaseObject(object);
+ }
+ return result;
+}
+
+module.exports = sortBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/toArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/toArray.js b/node_modules/lodash-node/compat/collections/toArray.js
new file mode 100644
index 0000000..223cf21
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/toArray.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isString = require('../objects/isString'),
+ slice = require('../internals/slice'),
+ support = require('../support'),
+ values = require('../objects/values');
+
+/**
+ * Converts the `collection` to an array.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to convert.
+ * @returns {Array} Returns the new converted array.
+ * @example
+ *
+ * (function() { return _.toArray(arguments).slice(1); })(1, 2, 3, 4);
+ * // => [2, 3, 4]
+ */
+function toArray(collection) {
+ if (collection && typeof collection.length == 'number') {
+ return (support.unindexedChars && isString(collection))
+ ? collection.split('')
+ : slice(collection);
+ }
+ return values(collection);
+}
+
+module.exports = toArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/where.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/where.js b/node_modules/lodash-node/compat/collections/where.js
new file mode 100644
index 0000000..c035b72
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/where.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var filter = require('./filter');
+
+/**
+ * Performs a deep comparison of each element in a `collection` to the given
+ * `properties` object, returning an array of all elements that have equivalent
+ * property values.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Object} props The object of property values to filter by.
+ * @returns {Array} Returns a new array of elements that have the given properties.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'pets': ['hoppy'] },
+ * { 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * _.where(characters, { 'age': 36 });
+ * // => [{ 'name': 'barney', 'age': 36, 'pets': ['hoppy'] }]
+ *
+ * _.where(characters, { 'pets': ['dino'] });
+ * // => [{ 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }]
+ */
+var where = filter;
+
+module.exports = where;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions.js b/node_modules/lodash-node/compat/functions.js
new file mode 100644
index 0000000..084bdd4
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'after': require('./functions/after'),
+ 'bind': require('./functions/bind'),
+ 'bindAll': require('./functions/bindAll'),
+ 'bindKey': require('./functions/bindKey'),
+ 'compose': require('./functions/compose'),
+ 'createCallback': require('./functions/createCallback'),
+ 'curry': require('./functions/curry'),
+ 'debounce': require('./functions/debounce'),
+ 'defer': require('./functions/defer'),
+ 'delay': require('./functions/delay'),
+ 'memoize': require('./functions/memoize'),
+ 'once': require('./functions/once'),
+ 'partial': require('./functions/partial'),
+ 'partialRight': require('./functions/partialRight'),
+ 'throttle': require('./functions/throttle'),
+ 'wrap': require('./functions/wrap')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/after.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/after.js b/node_modules/lodash-node/compat/functions/after.js
new file mode 100644
index 0000000..48ef88e
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/after.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that executes `func`, with the `this` binding and
+ * arguments of the created function, only after being called `n` times.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {number} n The number of times the function must be called before
+ * `func` is executed.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var saves = ['profile', 'settings'];
+ *
+ * var done = _.after(saves.length, function() {
+ * console.log('Done saving!');
+ * });
+ *
+ * _.forEach(saves, function(type) {
+ * asyncSave({ 'type': type, 'complete': done });
+ * });
+ * // => logs 'Done saving!', after all saves have completed
+ */
+function after(n, func) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+}
+
+module.exports = after;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/bind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/bind.js b/node_modules/lodash-node/compat/functions/bind.js
new file mode 100644
index 0000000..42284b3
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/bind.js
@@ -0,0 +1,41 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice'),
+ support = require('../support');
+
+/**
+ * Creates a function that, when called, invokes `func` with the `this`
+ * binding of `thisArg` and prepends any additional `bind` arguments to those
+ * provided to the bound function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to bind.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var func = function(greeting) {
+ * return greeting + ' ' + this.name;
+ * };
+ *
+ * func = _.bind(func, { 'name': 'fred' }, 'hi');
+ * func();
+ * // => 'hi fred'
+ */
+function bind(func, thisArg) {
+ return arguments.length > 2
+ ? createWrapper(func, 17, slice(arguments, 2), null, thisArg)
+ : createWrapper(func, 1, null, null, thisArg);
+}
+
+module.exports = bind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/bindAll.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/bindAll.js b/node_modules/lodash-node/compat/functions/bindAll.js
new file mode 100644
index 0000000..f62d906
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/bindAll.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ createWrapper = require('../internals/createWrapper'),
+ functions = require('../objects/functions');
+
+/**
+ * Binds methods of an object to the object itself, overwriting the existing
+ * method. Method names may be specified as individual arguments or as arrays
+ * of method names. If no method names are provided all the function properties
+ * of `object` will be bound.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object to bind and assign the bound methods to.
+ * @param {...string} [methodName] The object method names to
+ * bind, specified as individual method names or arrays of method names.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'onClick': function() { console.log('clicked ' + this.label); }
+ * };
+ *
+ * _.bindAll(view);
+ * jQuery('#docs').on('click', view.onClick);
+ * // => logs 'clicked docs', when the button is clicked
+ */
+function bindAll(object) {
+ var funcs = arguments.length > 1 ? baseFlatten(arguments, true, false, 1) : functions(object),
+ index = -1,
+ length = funcs.length;
+
+ while (++index < length) {
+ var key = funcs[index];
+ object[key] = createWrapper(object[key], 1, null, null, object);
+ }
+ return object;
+}
+
+module.exports = bindAll;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/bindKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/bindKey.js b/node_modules/lodash-node/compat/functions/bindKey.js
new file mode 100644
index 0000000..7e07139
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/bindKey.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes the method at `object[key]`
+ * and prepends any additional `bindKey` arguments to those provided to the bound
+ * function. This method differs from `_.bind` by allowing bound functions to
+ * reference methods that will be redefined or don't yet exist.
+ * See http://michaux.ca/articles/lazy-function-definition-pattern.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object the method belongs to.
+ * @param {string} key The key of the method.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var object = {
+ * 'name': 'fred',
+ * 'greet': function(greeting) {
+ * return greeting + ' ' + this.name;
+ * }
+ * };
+ *
+ * var func = _.bindKey(object, 'greet', 'hi');
+ * func();
+ * // => 'hi fred'
+ *
+ * object.greet = function(greeting) {
+ * return greeting + 'ya ' + this.name + '!';
+ * };
+ *
+ * func();
+ * // => 'hiya fred!'
+ */
+function bindKey(object, key) {
+ return arguments.length > 2
+ ? createWrapper(key, 19, slice(arguments, 2), null, object)
+ : createWrapper(key, 3, null, null, object);
+}
+
+module.exports = bindKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/compose.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/compose.js b/node_modules/lodash-node/compat/functions/compose.js
new file mode 100644
index 0000000..2a70d65
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/compose.js
@@ -0,0 +1,61 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that is the composition of the provided functions,
+ * where each function consumes the return value of the function that follows.
+ * For example, composing the functions `f()`, `g()`, and `h()` produces `f(g(h()))`.
+ * Each function is executed with the `this` binding of the composed function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {...Function} [func] Functions to compose.
+ * @returns {Function} Returns the new composed function.
+ * @example
+ *
+ * var realNameMap = {
+ * 'pebbles': 'penelope'
+ * };
+ *
+ * var format = function(name) {
+ * name = realNameMap[name.toLowerCase()] || name;
+ * return name.charAt(0).toUpperCase() + name.slice(1).toLowerCase();
+ * };
+ *
+ * var greet = function(formatted) {
+ * return 'Hiya ' + formatted + '!';
+ * };
+ *
+ * var welcome = _.compose(greet, format);
+ * welcome('pebbles');
+ * // => 'Hiya Penelope!'
+ */
+function compose() {
+ var funcs = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ if (!isFunction(funcs[length])) {
+ throw new TypeError;
+ }
+ }
+ return function() {
+ var args = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ args = [funcs[length].apply(this, args)];
+ }
+ return args[0];
+ };
+}
+
+module.exports = compose;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/createCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/createCallback.js b/node_modules/lodash-node/compat/functions/createCallback.js
new file mode 100644
index 0000000..460b80e
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/createCallback.js
@@ -0,0 +1,81 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseIsEqual = require('../internals/baseIsEqual'),
+ isObject = require('../objects/isObject'),
+ keys = require('../objects/keys'),
+ property = require('../utilities/property');
+
+/**
+ * Produces a callback bound to an optional `thisArg`. If `func` is a property
+ * name the created callback will return the property value for a given element.
+ * If `func` is an object the created callback will return `true` for elements
+ * that contain the equivalent object properties, otherwise it will return `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} [func=identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of the created callback.
+ * @param {number} [argCount] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // wrap to create custom callback shorthands
+ * _.createCallback = _.wrap(_.createCallback, function(func, callback, thisArg) {
+ * var match = /^(.+?)__([gl]t)(.+)$/.exec(callback);
+ * return !match ? func(callback, thisArg) : function(object) {
+ * return match[2] == 'gt' ? object[match[1]] > match[3] : object[match[1]] < match[3];
+ * };
+ * });
+ *
+ * _.filter(characters, 'age__gt38');
+ * // => [{ 'name': 'fred', 'age': 40 }]
+ */
+function createCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (func == null || type == 'function') {
+ return baseCreateCallback(func, thisArg, argCount);
+ }
+ // handle "_.pluck" style callback shorthands
+ if (type != 'object') {
+ return property(func);
+ }
+ var props = keys(func),
+ key = props[0],
+ a = func[key];
+
+ // handle "_.where" style callback shorthands
+ if (props.length == 1 && a === a && !isObject(a)) {
+ // fast path the common case of providing an object with a single
+ // property containing a primitive value
+ return function(object) {
+ var b = object[key];
+ return a === b && (a !== 0 || (1 / a == 1 / b));
+ };
+ }
+ return function(object) {
+ var length = props.length,
+ result = false;
+
+ while (length--) {
+ if (!(result = baseIsEqual(object[props[length]], func[props[length]], null, true))) {
+ break;
+ }
+ }
+ return result;
+ };
+}
+
+module.exports = createCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/curry.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/curry.js b/node_modules/lodash-node/compat/functions/curry.js
new file mode 100644
index 0000000..77fb780
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/curry.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper');
+
+/**
+ * Creates a function which accepts one or more arguments of `func` that when
+ * invoked either executes `func` returning its result, if all `func` arguments
+ * have been provided, or returns a function that accepts one or more of the
+ * remaining `func` arguments, and so on. The arity of `func` can be specified
+ * if `func.length` is not sufficient.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var curried = _.curry(function(a, b, c) {
+ * console.log(a + b + c);
+ * });
+ *
+ * curried(1)(2)(3);
+ * // => 6
+ *
+ * curried(1, 2)(3);
+ * // => 6
+ *
+ * curried(1, 2, 3);
+ * // => 6
+ */
+function curry(func, arity) {
+ arity = typeof arity == 'number' ? arity : (+arity || func.length);
+ return createWrapper(func, 4, null, null, null, arity);
+}
+
+module.exports = curry;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/debounce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/debounce.js b/node_modules/lodash-node/compat/functions/debounce.js
new file mode 100644
index 0000000..6bc27f4
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/debounce.js
@@ -0,0 +1,156 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject'),
+ now = require('../utilities/now');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that will delay the execution of `func` until after
+ * `wait` milliseconds have elapsed since the last time it was invoked.
+ * Provide an options object to indicate that `func` should be invoked on
+ * the leading and/or trailing edge of the `wait` timeout. Subsequent calls
+ * to the debounced function will return the result of the last `func` call.
+ *
+ * Note: If `leading` and `trailing` options are `true` `func` will be called
+ * on the trailing edge of the timeout only if the the debounced function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to debounce.
+ * @param {number} wait The number of milliseconds to delay.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=false] Specify execution on the leading edge of the timeout.
+ * @param {number} [options.maxWait] The maximum time `func` is allowed to be delayed before it's called.
+ * @param {boolean} [options.trailing=true] Specify execution on the trailing edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * // avoid costly calculations while the window size is in flux
+ * var lazyLayout = _.debounce(calculateLayout, 150);
+ * jQuery(window).on('resize', lazyLayout);
+ *
+ * // execute `sendMail` when the click event is fired, debouncing subsequent calls
+ * jQuery('#postbox').on('click', _.debounce(sendMail, 300, {
+ * 'leading': true,
+ * 'trailing': false
+ * });
+ *
+ * // ensure `batchLog` is executed once after 1 second of debounced calls
+ * var source = new EventSource('/stream');
+ * source.addEventListener('message', _.debounce(batchLog, 250, {
+ * 'maxWait': 1000
+ * }, false);
+ */
+function debounce(func, wait, options) {
+ var args,
+ maxTimeoutId,
+ result,
+ stamp,
+ thisArg,
+ timeoutId,
+ trailingCall,
+ lastCalled = 0,
+ maxWait = false,
+ trailing = true;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ wait = nativeMax(0, wait) || 0;
+ if (options === true) {
+ var leading = true;
+ trailing = false;
+ } else if (isObject(options)) {
+ leading = options.leading;
+ maxWait = 'maxWait' in options && (nativeMax(wait, options.maxWait) || 0);
+ trailing = 'trailing' in options ? options.trailing : trailing;
+ }
+ var delayed = function() {
+ var remaining = wait - (now() - stamp);
+ if (remaining <= 0) {
+ if (maxTimeoutId) {
+ clearTimeout(maxTimeoutId);
+ }
+ var isCalled = trailingCall;
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (isCalled) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ }
+ } else {
+ timeoutId = setTimeout(delayed, remaining);
+ }
+ };
+
+ var maxDelayed = function() {
+ if (timeoutId) {
+ clearTimeout(timeoutId);
+ }
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (trailing || (maxWait !== wait)) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ }
+ };
+
+ return function() {
+ args = arguments;
+ stamp = now();
+ thisArg = this;
+ trailingCall = trailing && (timeoutId || !leading);
+
+ if (maxWait === false) {
+ var leadingCall = leading && !timeoutId;
+ } else {
+ if (!maxTimeoutId && !leading) {
+ lastCalled = stamp;
+ }
+ var remaining = maxWait - (stamp - lastCalled),
+ isCalled = remaining <= 0;
+
+ if (isCalled) {
+ if (maxTimeoutId) {
+ maxTimeoutId = clearTimeout(maxTimeoutId);
+ }
+ lastCalled = stamp;
+ result = func.apply(thisArg, args);
+ }
+ else if (!maxTimeoutId) {
+ maxTimeoutId = setTimeout(maxDelayed, remaining);
+ }
+ }
+ if (isCalled && timeoutId) {
+ timeoutId = clearTimeout(timeoutId);
+ }
+ else if (!timeoutId && wait !== maxWait) {
+ timeoutId = setTimeout(delayed, wait);
+ }
+ if (leadingCall) {
+ isCalled = true;
+ result = func.apply(thisArg, args);
+ }
+ if (isCalled && !timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ return result;
+ };
+}
+
+module.exports = debounce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/defer.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/defer.js b/node_modules/lodash-node/compat/functions/defer.js
new file mode 100644
index 0000000..7a7bf32
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/defer.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ slice = require('../internals/slice');
+
+/**
+ * Defers executing the `func` function until the current call stack has cleared.
+ * Additional arguments will be provided to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to defer.
+ * @param {...*} [arg] Arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) { console.log(text); }, 'deferred');
+ * // logs 'deferred' after one or more milliseconds
+ */
+function defer(func) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var args = slice(arguments, 1);
+ return setTimeout(function() { func.apply(undefined, args); }, 1);
+}
+
+module.exports = defer;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/delay.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/delay.js b/node_modules/lodash-node/compat/functions/delay.js
new file mode 100644
index 0000000..5a466ae
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/delay.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ slice = require('../internals/slice');
+
+/**
+ * Executes the `func` function after `wait` milliseconds. Additional arguments
+ * will be provided to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay execution.
+ * @param {...*} [arg] Arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) { console.log(text); }, 1000, 'later');
+ * // => logs 'later' after one second
+ */
+function delay(func, wait) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var args = slice(arguments, 2);
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+}
+
+module.exports = delay;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/memoize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/memoize.js b/node_modules/lodash-node/compat/functions/memoize.js
new file mode 100644
index 0000000..4ba8192
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/memoize.js
@@ -0,0 +1,71 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ keyPrefix = require('../internals/keyPrefix');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates a function that memoizes the result of `func`. If `resolver` is
+ * provided it will be used to determine the cache key for storing the result
+ * based on the arguments provided to the memoized function. By default, the
+ * first argument provided to the memoized function is used as the cache key.
+ * The `func` is executed with the `this` binding of the memoized function.
+ * The result cache is exposed as the `cache` property on the memoized function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to have its output memoized.
+ * @param {Function} [resolver] A function used to resolve the cache key.
+ * @returns {Function} Returns the new memoizing function.
+ * @example
+ *
+ * var fibonacci = _.memoize(function(n) {
+ * return n < 2 ? n : fibonacci(n - 1) + fibonacci(n - 2);
+ * });
+ *
+ * fibonacci(9)
+ * // => 34
+ *
+ * var data = {
+ * 'fred': { 'name': 'fred', 'age': 40 },
+ * 'pebbles': { 'name': 'pebbles', 'age': 1 }
+ * };
+ *
+ * // modifying the result cache
+ * var get = _.memoize(function(name) { return data[name]; }, _.identity);
+ * get('pebbles');
+ * // => { 'name': 'pebbles', 'age': 1 }
+ *
+ * get.cache.pebbles.name = 'penelope';
+ * get('pebbles');
+ * // => { 'name': 'penelope', 'age': 1 }
+ */
+function memoize(func, resolver) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var memoized = function() {
+ var cache = memoized.cache,
+ key = resolver ? resolver.apply(this, arguments) : keyPrefix + arguments[0];
+
+ return hasOwnProperty.call(cache, key)
+ ? cache[key]
+ : (cache[key] = func.apply(this, arguments));
+ }
+ memoized.cache = {};
+ return memoized;
+}
+
+module.exports = memoize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/once.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/once.js b/node_modules/lodash-node/compat/functions/once.js
new file mode 100644
index 0000000..6409810
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/once.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that is restricted to execute `func` once. Repeat calls to
+ * the function will return the value of the first call. The `func` is executed
+ * with the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // `initialize` executes `createApplication` once
+ */
+function once(func) {
+ var ran,
+ result;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ return function() {
+ if (ran) {
+ return result;
+ }
+ ran = true;
+ result = func.apply(this, arguments);
+
+ // clear the `func` variable so the function may be garbage collected
+ func = null;
+ return result;
+ };
+}
+
+module.exports = once;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/partial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/partial.js b/node_modules/lodash-node/compat/functions/partial.js
new file mode 100644
index 0000000..64ff0ee
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/partial.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes `func` with any additional
+ * `partial` arguments prepended to those provided to the new function. This
+ * method is similar to `_.bind` except it does **not** alter the `this` binding.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var greet = function(greeting, name) { return greeting + ' ' + name; };
+ * var hi = _.partial(greet, 'hi');
+ * hi('fred');
+ * // => 'hi fred'
+ */
+function partial(func) {
+ return createWrapper(func, 16, slice(arguments, 1));
+}
+
+module.exports = partial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/partialRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/partialRight.js b/node_modules/lodash-node/compat/functions/partialRight.js
new file mode 100644
index 0000000..3028819
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/partialRight.js
@@ -0,0 +1,43 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * This method is like `_.partial` except that `partial` arguments are
+ * appended to those provided to the new function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var defaultsDeep = _.partialRight(_.merge, _.defaults);
+ *
+ * var options = {
+ * 'variable': 'data',
+ * 'imports': { 'jq': $ }
+ * };
+ *
+ * defaultsDeep(options, _.templateSettings);
+ *
+ * options.variable
+ * // => 'data'
+ *
+ * options.imports
+ * // => { '_': _, 'jq': $ }
+ */
+function partialRight(func) {
+ return createWrapper(func, 32, null, slice(arguments, 1));
+}
+
+module.exports = partialRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/throttle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/throttle.js b/node_modules/lodash-node/compat/functions/throttle.js
new file mode 100644
index 0000000..8300c65
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/throttle.js
@@ -0,0 +1,71 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var debounce = require('./debounce'),
+ isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject');
+
+/** Used as an internal `_.debounce` options object */
+var debounceOptions = {
+ 'leading': false,
+ 'maxWait': 0,
+ 'trailing': false
+};
+
+/**
+ * Creates a function that, when executed, will only call the `func` function
+ * at most once per every `wait` milliseconds. Provide an options object to
+ * indicate that `func` should be invoked on the leading and/or trailing edge
+ * of the `wait` timeout. Subsequent calls to the throttled function will
+ * return the result of the last `func` call.
+ *
+ * Note: If `leading` and `trailing` options are `true` `func` will be called
+ * on the trailing edge of the timeout only if the the throttled function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to throttle.
+ * @param {number} wait The number of milliseconds to throttle executions to.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=true] Specify execution on the leading edge of the timeout.
+ * @param {boolean} [options.trailing=true] Specify execution on the trailing edge of the timeout.
+ * @returns {Function} Returns the new throttled function.
+ * @example
+ *
+ * // avoid excessively updating the position while scrolling
+ * var throttled = _.throttle(updatePosition, 100);
+ * jQuery(window).on('scroll', throttled);
+ *
+ * // execute `renewToken` when the click event is fired, but not more than once every 5 minutes
+ * jQuery('.interactive').on('click', _.throttle(renewToken, 300000, {
+ * 'trailing': false
+ * }));
+ */
+function throttle(func, wait, options) {
+ var leading = true,
+ trailing = true;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ if (options === false) {
+ leading = false;
+ } else if (isObject(options)) {
+ leading = 'leading' in options ? options.leading : leading;
+ trailing = 'trailing' in options ? options.trailing : trailing;
+ }
+ debounceOptions.leading = leading;
+ debounceOptions.maxWait = wait;
+ debounceOptions.trailing = trailing;
+
+ return debounce(func, wait, debounceOptions);
+}
+
+module.exports = throttle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/functions/wrap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/functions/wrap.js b/node_modules/lodash-node/compat/functions/wrap.js
new file mode 100644
index 0000000..9168ffc
--- /dev/null
+++ b/node_modules/lodash-node/compat/functions/wrap.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper');
+
+/**
+ * Creates a function that provides `value` to the wrapper function as its
+ * first argument. Additional arguments provided to the function are appended
+ * to those provided to the wrapper function. The wrapper is executed with
+ * the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {*} value The value to wrap.
+ * @param {Function} wrapper The wrapper function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var p = _.wrap(_.escape, function(func, text) {
+ * return '<p>' + func(text) + '</p>';
+ * });
+ *
+ * p('Fred, Wilma, & Pebbles');
+ * // => '<p>Fred, Wilma, & Pebbles</p>'
+ */
+function wrap(value, wrapper) {
+ return createWrapper(wrapper, 16, [value]);
+}
+
+module.exports = wrap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/index.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/index.js b/node_modules/lodash-node/compat/index.js
new file mode 100644
index 0000000..cbea241
--- /dev/null
+++ b/node_modules/lodash-node/compat/index.js
@@ -0,0 +1,376 @@
+/**
+ * @license
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrays = require('./arrays'),
+ chaining = require('./chaining'),
+ collections = require('./collections'),
+ functions = require('./functions'),
+ objects = require('./objects'),
+ utilities = require('./utilities'),
+ baseEach = require('./internals/baseEach'),
+ forOwn = require('./objects/forOwn'),
+ isArray = require('./objects/isArray'),
+ lodashWrapper = require('./internals/lodashWrapper'),
+ mixin = require('./utilities/mixin'),
+ support = require('./support'),
+ templateSettings = require('./utilities/templateSettings');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates a `lodash` object which wraps the given value to enable intuitive
+ * method chaining.
+ *
+ * In addition to Lo-Dash methods, wrappers also have the following `Array` methods:
+ * `concat`, `join`, `pop`, `push`, `reverse`, `shift`, `slice`, `sort`, `splice`,
+ * and `unshift`
+ *
+ * Chaining is supported in custom builds as long as the `value` method is
+ * implicitly or explicitly included in the build.
+ *
+ * The chainable wrapper functions are:
+ * `after`, `assign`, `bind`, `bindAll`, `bindKey`, `chain`, `compact`,
+ * `compose`, `concat`, `countBy`, `create`, `createCallback`, `curry`,
+ * `debounce`, `defaults`, `defer`, `delay`, `difference`, `filter`, `flatten`,
+ * `forEach`, `forEachRight`, `forIn`, `forInRight`, `forOwn`, `forOwnRight`,
+ * `functions`, `groupBy`, `indexBy`, `initial`, `intersection`, `invert`,
+ * `invoke`, `keys`, `map`, `max`, `memoize`, `merge`, `min`, `object`, `omit`,
+ * `once`, `pairs`, `partial`, `partialRight`, `pick`, `pluck`, `pull`, `push`,
+ * `range`, `reject`, `remove`, `rest`, `reverse`, `shuffle`, `slice`, `sort`,
+ * `sortBy`, `splice`, `tap`, `throttle`, `times`, `toArray`, `transform`,
+ * `union`, `uniq`, `unshift`, `unzip`, `values`, `where`, `without`, `wrap`,
+ * and `zip`
+ *
+ * The non-chainable wrapper functions are:
+ * `clone`, `cloneDeep`, `contains`, `escape`, `every`, `find`, `findIndex`,
+ * `findKey`, `findLast`, `findLastIndex`, `findLastKey`, `has`, `identity`,
+ * `indexOf`, `isArguments`, `isArray`, `isBoolean`, `isDate`, `isElement`,
+ * `isEmpty`, `isEqual`, `isFinite`, `isFunction`, `isNaN`, `isNull`, `isNumber`,
+ * `isObject`, `isPlainObject`, `isRegExp`, `isString`, `isUndefined`, `join`,
+ * `lastIndexOf`, `mixin`, `noConflict`, `parseInt`, `pop`, `random`, `reduce`,
+ * `reduceRight`, `result`, `shift`, `size`, `some`, `sortedIndex`, `runInContext`,
+ * `template`, `unescape`, `uniqueId`, and `value`
+ *
+ * The wrapper functions `first` and `last` return wrapped values when `n` is
+ * provided, otherwise they return unwrapped values.
+ *
+ * Explicit chaining can be enabled by using the `_.chain` method.
+ *
+ * @name _
+ * @constructor
+ * @category Chaining
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns a `lodash` instance.
+ * @example
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // returns an unwrapped value
+ * wrapped.reduce(function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * // returns a wrapped value
+ * var squares = wrapped.map(function(num) {
+ * return num * num;
+ * });
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+function lodash(value) {
+ // don't wrap if already wrapped, even if wrapped by a different `lodash` constructor
+ return (value && typeof value == 'object' && !isArray(value) && hasOwnProperty.call(value, '__wrapped__'))
+ ? value
+ : new lodashWrapper(value);
+}
+// ensure `new lodashWrapper` is an instance of `lodash`
+lodashWrapper.prototype = lodash.prototype;
+
+// wrap `_.mixin` so it works when provided only one argument
+mixin = (function(fn) {
+ var functions = objects.functions;
+ return function(object, source, options) {
+ if (!source || (!options && !functions(source).length)) {
+ if (options == null) {
+ options = source;
+ }
+ source = object;
+ object = lodash;
+ }
+ return fn(object, source, options);
+ };
+}(mixin));
+
+// add functions that return wrapped values when chaining
+lodash.after = functions.after;
+lodash.assign = objects.assign;
+lodash.at = collections.at;
+lodash.bind = functions.bind;
+lodash.bindAll = functions.bindAll;
+lodash.bindKey = functions.bindKey;
+lodash.chain = chaining.chain;
+lodash.compact = arrays.compact;
+lodash.compose = functions.compose;
+lodash.constant = utilities.constant;
+lodash.countBy = collections.countBy;
+lodash.create = objects.create;
+lodash.createCallback = functions.createCallback;
+lodash.curry = functions.curry;
+lodash.debounce = functions.debounce;
+lodash.defaults = objects.defaults;
+lodash.defer = functions.defer;
+lodash.delay = functions.delay;
+lodash.difference = arrays.difference;
+lodash.filter = collections.filter;
+lodash.flatten = arrays.flatten;
+lodash.forEach = collections.forEach;
+lodash.forEachRight = collections.forEachRight;
+lodash.forIn = objects.forIn;
+lodash.forInRight = objects.forInRight;
+lodash.forOwn = forOwn;
+lodash.forOwnRight = objects.forOwnRight;
+lodash.functions = objects.functions;
+lodash.groupBy = collections.groupBy;
+lodash.indexBy = collections.indexBy;
+lodash.initial = arrays.initial;
+lodash.intersection = arrays.intersection;
+lodash.invert = objects.invert;
+lodash.invoke = collections.invoke;
+lodash.keys = objects.keys;
+lodash.map = collections.map;
+lodash.mapValues = objects.mapValues;
+lodash.max = collections.max;
+lodash.memoize = functions.memoize;
+lodash.merge = objects.merge;
+lodash.min = collections.min;
+lodash.omit = objects.omit;
+lodash.once = functions.once;
+lodash.pairs = objects.pairs;
+lodash.partial = functions.partial;
+lodash.partialRight = functions.partialRight;
+lodash.pick = objects.pick;
+lodash.pluck = collections.pluck;
+lodash.property = utilities.property;
+lodash.pull = arrays.pull;
+lodash.range = arrays.range;
+lodash.reject = collections.reject;
+lodash.remove = arrays.remove;
+lodash.rest = arrays.rest;
+lodash.shuffle = collections.shuffle;
+lodash.sortBy = collections.sortBy;
+lodash.tap = chaining.tap;
+lodash.throttle = functions.throttle;
+lodash.times = utilities.times;
+lodash.toArray = collections.toArray;
+lodash.transform = objects.transform;
+lodash.union = arrays.union;
+lodash.uniq = arrays.uniq;
+lodash.values = objects.values;
+lodash.where = collections.where;
+lodash.without = arrays.without;
+lodash.wrap = functions.wrap;
+lodash.xor = arrays.xor;
+lodash.zip = arrays.zip;
+lodash.zipObject = arrays.zipObject;
+
+// add aliases
+lodash.collect = collections.map;
+lodash.drop = arrays.rest;
+lodash.each = collections.forEach;
+lodash.eachRight = collections.forEachRight;
+lodash.extend = objects.assign;
+lodash.methods = objects.functions;
+lodash.object = arrays.zipObject;
+lodash.select = collections.filter;
+lodash.tail = arrays.rest;
+lodash.unique = arrays.uniq;
+lodash.unzip = arrays.zip;
+
+// add functions to `lodash.prototype`
+mixin(lodash);
+
+// add functions that return unwrapped values when chaining
+lodash.clone = objects.clone;
+lodash.cloneDeep = objects.cloneDeep;
+lodash.contains = collections.contains;
+lodash.escape = utilities.escape;
+lodash.every = collections.every;
+lodash.find = collections.find;
+lodash.findIndex = arrays.findIndex;
+lodash.findKey = objects.findKey;
+lodash.findLast = collections.findLast;
+lodash.findLastIndex = arrays.findLastIndex;
+lodash.findLastKey = objects.findLastKey;
+lodash.has = objects.has;
+lodash.identity = utilities.identity;
+lodash.indexOf = arrays.indexOf;
+lodash.isArguments = objects.isArguments;
+lodash.isArray = isArray;
+lodash.isBoolean = objects.isBoolean;
+lodash.isDate = objects.isDate;
+lodash.isElement = objects.isElement;
+lodash.isEmpty = objects.isEmpty;
+lodash.isEqual = objects.isEqual;
+lodash.isFinite = objects.isFinite;
+lodash.isFunction = objects.isFunction;
+lodash.isNaN = objects.isNaN;
+lodash.isNull = objects.isNull;
+lodash.isNumber = objects.isNumber;
+lodash.isObject = objects.isObject;
+lodash.isPlainObject = objects.isPlainObject;
+lodash.isRegExp = objects.isRegExp;
+lodash.isString = objects.isString;
+lodash.isUndefined = objects.isUndefined;
+lodash.lastIndexOf = arrays.lastIndexOf;
+lodash.mixin = mixin;
+lodash.noConflict = utilities.noConflict;
+lodash.noop = utilities.noop;
+lodash.now = utilities.now;
+lodash.parseInt = utilities.parseInt;
+lodash.random = utilities.random;
+lodash.reduce = collections.reduce;
+lodash.reduceRight = collections.reduceRight;
+lodash.result = utilities.result;
+lodash.size = collections.size;
+lodash.some = collections.some;
+lodash.sortedIndex = arrays.sortedIndex;
+lodash.template = utilities.template;
+lodash.unescape = utilities.unescape;
+lodash.uniqueId = utilities.uniqueId;
+
+// add aliases
+lodash.all = collections.every;
+lodash.any = collections.some;
+lodash.detect = collections.find;
+lodash.findWhere = collections.find;
+lodash.foldl = collections.reduce;
+lodash.foldr = collections.reduceRight;
+lodash.include = collections.contains;
+lodash.inject = collections.reduce;
+
+mixin(function() {
+ var source = {}
+ forOwn(lodash, function(func, methodName) {
+ if (!lodash.prototype[methodName]) {
+ source[methodName] = func;
+ }
+ });
+ return source;
+}(), false);
+
+// add functions capable of returning wrapped and unwrapped values when chaining
+lodash.first = arrays.first;
+lodash.last = arrays.last;
+lodash.sample = collections.sample;
+
+// add aliases
+lodash.take = arrays.first;
+lodash.head = arrays.first;
+
+forOwn(lodash, function(func, methodName) {
+ var callbackable = methodName !== 'sample';
+ if (!lodash.prototype[methodName]) {
+ lodash.prototype[methodName]= function(n, guard) {
+ var chainAll = this.__chain__,
+ result = func(this.__wrapped__, n, guard);
+
+ return !chainAll && (n == null || (guard && !(callbackable && typeof n == 'function')))
+ ? result
+ : new lodashWrapper(result, chainAll);
+ };
+ }
+});
+
+/**
+ * The semantic version number.
+ *
+ * @static
+ * @memberOf _
+ * @type string
+ */
+lodash.VERSION = '2.4.1';
+
+// add "Chaining" functions to the wrapper
+lodash.prototype.chain = chaining.wrapperChain;
+lodash.prototype.toString = chaining.wrapperToString;
+lodash.prototype.value = chaining.wrapperValueOf;
+lodash.prototype.valueOf = chaining.wrapperValueOf;
+
+// add `Array` functions that return unwrapped values
+baseEach(['join', 'pop', 'shift'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ var chainAll = this.__chain__,
+ result = func.apply(this.__wrapped__, arguments);
+
+ return chainAll
+ ? new lodashWrapper(result, chainAll)
+ : result;
+ };
+});
+
+// add `Array` functions that return the existing wrapped value
+baseEach(['push', 'reverse', 'sort', 'unshift'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ func.apply(this.__wrapped__, arguments);
+ return this;
+ };
+});
+
+// add `Array` functions that return new wrapped values
+baseEach(['concat', 'slice', 'splice'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ return new lodashWrapper(func.apply(this.__wrapped__, arguments), this.__chain__);
+ };
+});
+
+// avoid array-like object bugs with `Array#shift` and `Array#splice`
+// in IE < 9, Firefox < 10, Narwhal, and RingoJS
+if (!support.spliceObjects) {
+ baseEach(['pop', 'shift', 'splice'], function(methodName) {
+ var func = arrayRef[methodName],
+ isSplice = methodName == 'splice';
+
+ lodash.prototype[methodName] = function() {
+ var chainAll = this.__chain__,
+ value = this.__wrapped__,
+ result = func.apply(value, arguments);
+
+ if (value.length === 0) {
+ delete value[0];
+ }
+ return (chainAll || isSplice)
+ ? new lodashWrapper(result, chainAll)
+ : result;
+ };
+ });
+}
+
+lodash.support = support;
+(lodash.templateSettings = utilities.templateSettings).imports._ = lodash;
+module.exports = lodash;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/arrayPool.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/arrayPool.js b/node_modules/lodash-node/compat/internals/arrayPool.js
new file mode 100644
index 0000000..56e34e5
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/arrayPool.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to pool arrays and objects used internally */
+var arrayPool = [];
+
+module.exports = arrayPool;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseBind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseBind.js b/node_modules/lodash-node/compat/internals/baseBind.js
new file mode 100644
index 0000000..5e328f7
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseBind.js
@@ -0,0 +1,62 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('./baseCreate'),
+ isObject = require('../objects/isObject'),
+ setBindData = require('./setBindData'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * The base implementation of `_.bind` that creates the bound function and
+ * sets its meta data.
+ *
+ * @private
+ * @param {Array} bindData The bind data array.
+ * @returns {Function} Returns the new bound function.
+ */
+function baseBind(bindData) {
+ var func = bindData[0],
+ partialArgs = bindData[2],
+ thisArg = bindData[4];
+
+ function bound() {
+ // `Function#bind` spec
+ // http://es5.github.io/#x15.3.4.5
+ if (partialArgs) {
+ // avoid `arguments` object deoptimizations by using `slice` instead
+ // of `Array.prototype.slice.call` and not assigning `arguments` to a
+ // variable as a ternary expression
+ var args = slice(partialArgs);
+ push.apply(args, arguments);
+ }
+ // mimic the constructor's `return` behavior
+ // http://es5.github.io/#x13.2.2
+ if (this instanceof bound) {
+ // ensure `new bound` is an instance of `func`
+ var thisBinding = baseCreate(func.prototype),
+ result = func.apply(thisBinding, args || arguments);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisArg, args || arguments);
+ }
+ setBindData(bound, bindData);
+ return bound;
+}
+
+module.exports = baseBind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseClone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseClone.js b/node_modules/lodash-node/compat/internals/baseClone.js
new file mode 100644
index 0000000..fa262bf
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseClone.js
@@ -0,0 +1,154 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var assign = require('../objects/assign'),
+ baseEach = require('./baseEach'),
+ forOwn = require('../objects/forOwn'),
+ getArray = require('./getArray'),
+ isArray = require('../objects/isArray'),
+ isNode = require('./isNode'),
+ isObject = require('../objects/isObject'),
+ releaseArray = require('./releaseArray'),
+ slice = require('./slice'),
+ support = require('../support');
+
+/** Used to match regexp flags from their coerced string values */
+var reFlags = /\w*$/;
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ funcClass = '[object Function]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+/** Used to identify object classifications that `_.clone` supports */
+var cloneableClasses = {};
+cloneableClasses[funcClass] = false;
+cloneableClasses[argsClass] = cloneableClasses[arrayClass] =
+cloneableClasses[boolClass] = cloneableClasses[dateClass] =
+cloneableClasses[numberClass] = cloneableClasses[objectClass] =
+cloneableClasses[regexpClass] = cloneableClasses[stringClass] = true;
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to lookup a built-in constructor by [[Class]] */
+var ctorByClass = {};
+ctorByClass[arrayClass] = Array;
+ctorByClass[boolClass] = Boolean;
+ctorByClass[dateClass] = Date;
+ctorByClass[funcClass] = Function;
+ctorByClass[objectClass] = Object;
+ctorByClass[numberClass] = Number;
+ctorByClass[regexpClass] = RegExp;
+ctorByClass[stringClass] = String;
+
+/**
+ * The base implementation of `_.clone` without argument juggling or support
+ * for `thisArg` binding.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep=false] Specify a deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates clones with source counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+function baseClone(value, isDeep, callback, stackA, stackB) {
+ if (callback) {
+ var result = callback(value);
+ if (typeof result != 'undefined') {
+ return result;
+ }
+ }
+ // inspect [[Class]]
+ var isObj = isObject(value);
+ if (isObj) {
+ var className = toString.call(value);
+ if (!cloneableClasses[className] || (!support.nodeClass && isNode(value))) {
+ return value;
+ }
+ var ctor = ctorByClass[className];
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ return new ctor(+value);
+
+ case numberClass:
+ case stringClass:
+ return new ctor(value);
+
+ case regexpClass:
+ result = ctor(value.source, reFlags.exec(value));
+ result.lastIndex = value.lastIndex;
+ return result;
+ }
+ } else {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isDeep) {
+ // check for circular references and return corresponding clone
+ var initedStack = !stackA;
+ stackA || (stackA = getArray());
+ stackB || (stackB = getArray());
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == value) {
+ return stackB[length];
+ }
+ }
+ result = isArr ? ctor(value.length) : {};
+ }
+ else {
+ result = isArr ? slice(value) : assign({}, value);
+ }
+ // add array properties assigned by `RegExp#exec`
+ if (isArr) {
+ if (hasOwnProperty.call(value, 'index')) {
+ result.index = value.index;
+ }
+ if (hasOwnProperty.call(value, 'input')) {
+ result.input = value.input;
+ }
+ }
+ // exit for shallow clone
+ if (!isDeep) {
+ return result;
+ }
+ // add the source value to the stack of traversed objects
+ // and associate it with its clone
+ stackA.push(value);
+ stackB.push(result);
+
+ // recursively populate clone (susceptible to call stack limits)
+ (isArr ? baseEach : forOwn)(value, function(objValue, key) {
+ result[key] = baseClone(objValue, isDeep, callback, stackA, stackB);
+ });
+
+ if (initedStack) {
+ releaseArray(stackA);
+ releaseArray(stackB);
+ }
+ return result;
+}
+
+module.exports = baseClone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseCreate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseCreate.js b/node_modules/lodash-node/compat/internals/baseCreate.js
new file mode 100644
index 0000000..90fcd68
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseCreate.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./isNative'),
+ isObject = require('../objects/isObject'),
+ noop = require('../utilities/noop');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeCreate = isNative(nativeCreate = Object.create) && nativeCreate;
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+function baseCreate(prototype, properties) {
+ return isObject(prototype) ? nativeCreate(prototype) : {};
+}
+// fallback for browsers without `Object.create`
+if (!nativeCreate) {
+ baseCreate = (function() {
+ function Object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ Object.prototype = prototype;
+ var result = new Object;
+ Object.prototype = null;
+ }
+ return result || global.Object();
+ };
+ }());
+}
+
+module.exports = baseCreate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseCreateCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseCreateCallback.js b/node_modules/lodash-node/compat/internals/baseCreateCallback.js
new file mode 100644
index 0000000..cb9657b
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseCreateCallback.js
@@ -0,0 +1,80 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var bind = require('../functions/bind'),
+ identity = require('../utilities/identity'),
+ setBindData = require('./setBindData'),
+ support = require('../support');
+
+/** Used to detected named functions */
+var reFuncName = /^\s*function[ \n\r\t]+\w/;
+
+/** Used to detect functions containing a `this` reference */
+var reThis = /\bthis\b/;
+
+/** Native method shortcuts */
+var fnToString = Function.prototype.toString;
+
+/**
+ * The base implementation of `_.createCallback` without support for creating
+ * "_.pluck" or "_.where" style callbacks.
+ *
+ * @private
+ * @param {*} [func=identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of the created callback.
+ * @param {number} [argCount] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ */
+function baseCreateCallback(func, thisArg, argCount) {
+ if (typeof func != 'function') {
+ return identity;
+ }
+ // exit early for no `thisArg` or already bound by `Function#bind`
+ if (typeof thisArg == 'undefined' || !('prototype' in func)) {
+ return func;
+ }
+ var bindData = func.__bindData__;
+ if (typeof bindData == 'undefined') {
+ if (support.funcNames) {
+ bindData = !func.name;
+ }
+ bindData = bindData || !support.funcDecomp;
+ if (!bindData) {
+ var source = fnToString.call(func);
+ if (!support.funcNames) {
+ bindData = !reFuncName.test(source);
+ }
+ if (!bindData) {
+ // checks if `func` references the `this` keyword and stores the result
+ bindData = reThis.test(source);
+ setBindData(func, bindData);
+ }
+ }
+ }
+ // exit early if there are no `this` references or `func` is bound
+ if (bindData === false || (bindData !== true && bindData[1] & 1)) {
+ return func;
+ }
+ switch (argCount) {
+ case 1: return function(value) {
+ return func.call(thisArg, value);
+ };
+ case 2: return function(a, b) {
+ return func.call(thisArg, a, b);
+ };
+ case 3: return function(value, index, collection) {
+ return func.call(thisArg, value, index, collection);
+ };
+ case 4: return function(accumulator, value, index, collection) {
+ return func.call(thisArg, accumulator, value, index, collection);
+ };
+ }
+ return bind(func, thisArg);
+}
+
+module.exports = baseCreateCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseCreateWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseCreateWrapper.js b/node_modules/lodash-node/compat/internals/baseCreateWrapper.js
new file mode 100644
index 0000000..41bb35f
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseCreateWrapper.js
@@ -0,0 +1,78 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('./baseCreate'),
+ isObject = require('../objects/isObject'),
+ setBindData = require('./setBindData'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * The base implementation of `createWrapper` that creates the wrapper and
+ * sets its meta data.
+ *
+ * @private
+ * @param {Array} bindData The bind data array.
+ * @returns {Function} Returns the new function.
+ */
+function baseCreateWrapper(bindData) {
+ var func = bindData[0],
+ bitmask = bindData[1],
+ partialArgs = bindData[2],
+ partialRightArgs = bindData[3],
+ thisArg = bindData[4],
+ arity = bindData[5];
+
+ var isBind = bitmask & 1,
+ isBindKey = bitmask & 2,
+ isCurry = bitmask & 4,
+ isCurryBound = bitmask & 8,
+ key = func;
+
+ function bound() {
+ var thisBinding = isBind ? thisArg : this;
+ if (partialArgs) {
+ var args = slice(partialArgs);
+ push.apply(args, arguments);
+ }
+ if (partialRightArgs || isCurry) {
+ args || (args = slice(arguments));
+ if (partialRightArgs) {
+ push.apply(args, partialRightArgs);
+ }
+ if (isCurry && args.length < arity) {
+ bitmask |= 16 & ~32;
+ return baseCreateWrapper([func, (isCurryBound ? bitmask : bitmask & ~3), args, null, thisArg, arity]);
+ }
+ }
+ args || (args = arguments);
+ if (isBindKey) {
+ func = thisBinding[key];
+ }
+ if (this instanceof bound) {
+ thisBinding = baseCreate(func.prototype);
+ var result = func.apply(thisBinding, args);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisBinding, args);
+ }
+ setBindData(bound, bindData);
+ return bound;
+}
+
+module.exports = baseCreateWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseDifference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseDifference.js b/node_modules/lodash-node/compat/internals/baseDifference.js
new file mode 100644
index 0000000..cc32c28
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseDifference.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf'),
+ cacheIndexOf = require('./cacheIndexOf'),
+ createCache = require('./createCache'),
+ largeArraySize = require('./largeArraySize'),
+ releaseObject = require('./releaseObject');
+
+/**
+ * The base implementation of `_.difference` that accepts a single array
+ * of values to exclude.
+ *
+ * @private
+ * @param {Array} array The array to process.
+ * @param {Array} [values] The array of values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ */
+function baseDifference(array, values) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ isLarge = length >= largeArraySize,
+ result = [];
+
+ if (isLarge) {
+ var cache = createCache(values);
+ if (cache) {
+ indexOf = cacheIndexOf;
+ values = cache;
+ } else {
+ isLarge = false;
+ }
+ }
+ while (++index < length) {
+ var value = array[index];
+ if (indexOf(values, value) < 0) {
+ result.push(value);
+ }
+ }
+ if (isLarge) {
+ releaseObject(values);
+ }
+ return result;
+}
+
+module.exports = baseDifference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/internals/baseEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/internals/baseEach.js b/node_modules/lodash-node/compat/internals/baseEach.js
new file mode 100644
index 0000000..0c833bc
--- /dev/null
+++ b/node_modules/lodash-node/compat/internals/baseEach.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createIterator = require('./createIterator'),
+ eachIteratorOptions = require('./eachIteratorOptions');
+
+/**
+ * A function compiled to iterate `arguments` objects, arrays, objects, and
+ * strings consistenly across environments, executing the callback for each
+ * element in the collection. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index|key, collection). Callbacks may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @private
+ * @type Function
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+var baseEach = createIterator(eachIteratorOptions);
+
+module.exports = baseEach;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[55/59] [abbrv] mac commit: CB-10668 fixed failing tests,
reverted shelljs to 0.5.3
Posted by st...@apache.org.
CB-10668 fixed failing tests, reverted shelljs to 0.5.3
Project: http://git-wip-us.apache.org/repos/asf/cordova-osx/repo
Commit: http://git-wip-us.apache.org/repos/asf/cordova-osx/commit/562f4668
Tree: http://git-wip-us.apache.org/repos/asf/cordova-osx/tree/562f4668
Diff: http://git-wip-us.apache.org/repos/asf/cordova-osx/diff/562f4668
Branch: refs/heads/4.0.x
Commit: 562f46687deb19988a0291b38c2bd73489a2e653
Parents: 42cbe9f
Author: Steve Gill <st...@gmail.com>
Authored: Mon Feb 22 12:29:05 2016 -0800
Committer: Steve Gill <st...@gmail.com>
Committed: Thu Mar 3 15:23:22 2016 -0800
----------------------------------------------------------------------
bin/templates/scripts/cordova/lib/prepare.js | 4 +-
node_modules/abbrev/package.json | 1 +
node_modules/brace-expansion/package.json | 2 +
.../cordova-common/node_modules/.bin/shjs | 1 -
.../node_modules/shelljs/.documentup.json | 6 -
.../node_modules/shelljs/.jshintrc | 7 -
.../node_modules/shelljs/.npmignore | 2 -
.../node_modules/shelljs/.travis.yml | 6 -
.../cordova-common/node_modules/shelljs/LICENSE | 26 -
.../node_modules/shelljs/README.md | 579 -----
.../node_modules/shelljs/RELEASE.md | 9 -
.../node_modules/shelljs/bin/shjs | 51 -
.../node_modules/shelljs/global.js | 3 -
.../cordova-common/node_modules/shelljs/make.js | 56 -
.../node_modules/shelljs/package.json | 88 -
.../shelljs/scripts/generate-docs.js | 21 -
.../node_modules/shelljs/scripts/run-tests.js | 50 -
.../node_modules/shelljs/shell.js | 159 --
.../node_modules/shelljs/src/cat.js | 43 -
.../node_modules/shelljs/src/cd.js | 19 -
.../node_modules/shelljs/src/chmod.js | 208 --
.../node_modules/shelljs/src/common.js | 203 --
.../node_modules/shelljs/src/cp.js | 204 --
.../node_modules/shelljs/src/dirs.js | 191 --
.../node_modules/shelljs/src/echo.js | 20 -
.../node_modules/shelljs/src/error.js | 10 -
.../node_modules/shelljs/src/exec.js | 216 --
.../node_modules/shelljs/src/find.js | 51 -
.../node_modules/shelljs/src/grep.js | 52 -
.../node_modules/shelljs/src/ln.js | 53 -
.../node_modules/shelljs/src/ls.js | 126 -
.../node_modules/shelljs/src/mkdir.js | 68 -
.../node_modules/shelljs/src/mv.js | 80 -
.../node_modules/shelljs/src/popd.js | 1 -
.../node_modules/shelljs/src/pushd.js | 1 -
.../node_modules/shelljs/src/pwd.js | 11 -
.../node_modules/shelljs/src/rm.js | 163 --
.../node_modules/shelljs/src/sed.js | 43 -
.../node_modules/shelljs/src/tempdir.js | 56 -
.../node_modules/shelljs/src/test.js | 85 -
.../node_modules/shelljs/src/to.js | 29 -
.../node_modules/shelljs/src/toEnd.js | 29 -
.../node_modules/shelljs/src/which.js | 83 -
node_modules/glob/package.json | 3 +-
node_modules/inflight/package.json | 6 +-
node_modules/inherits/package.json | 15 +-
node_modules/lodash/package.json | 1 +
node_modules/minimatch/package.json | 6 +-
node_modules/nopt/package.json | 3 +-
node_modules/once/package.json | 7 +-
node_modules/os-homedir/package.json | 3 +-
node_modules/os-tmpdir/package.json | 3 +-
node_modules/path-is-absolute/package.json | 6 +-
node_modules/semver/package.json | 3 +-
node_modules/shelljs/.idea/.name | 1 -
node_modules/shelljs/.idea/encodings.xml | 6 -
.../inspectionProfiles/Project_Default.xml | 7 -
.../inspectionProfiles/profiles_settings.xml | 7 -
.../shelljs/.idea/jsLibraryMappings.xml | 6 -
.../.idea/libraries/shelljs_node_modules.xml | 14 -
node_modules/shelljs/.idea/misc.xml | 28 -
node_modules/shelljs/.idea/modules.xml | 8 -
node_modules/shelljs/.idea/shelljs.iml | 9 -
node_modules/shelljs/.idea/vcs.xml | 6 -
node_modules/shelljs/.idea/workspace.xml | 764 ------
node_modules/shelljs/.npmignore | 9 +-
node_modules/shelljs/LICENSE | 2 +-
node_modules/shelljs/MAINTAINERS | 3 -
node_modules/shelljs/README.md | 211 +-
node_modules/shelljs/bin/shjs | 8 +-
node_modules/shelljs/build/output.js | 2411 ------------------
node_modules/shelljs/make.js | 9 +-
node_modules/shelljs/package.json | 71 +-
node_modules/shelljs/scripts/generate-docs.js | 9 +-
node_modules/shelljs/scripts/run-tests.js | 13 +-
node_modules/shelljs/shell.js | 29 +-
node_modules/shelljs/src/cat.js | 5 +-
node_modules/shelljs/src/cd.js | 15 +-
node_modules/shelljs/src/chmod.js | 23 +-
node_modules/shelljs/src/common.js | 108 +-
node_modules/shelljs/src/cp.js | 24 +-
node_modules/shelljs/src/echo.js | 4 +-
node_modules/shelljs/src/error.js | 2 +-
node_modules/shelljs/src/exec.js | 141 +-
node_modules/shelljs/src/find.js | 2 +-
node_modules/shelljs/src/grep.js | 4 +-
node_modules/shelljs/src/ln.js | 40 +-
node_modules/shelljs/src/ls.js | 64 +-
node_modules/shelljs/src/mkdir.js | 6 +-
node_modules/shelljs/src/mv.js | 16 +-
node_modules/shelljs/src/pwd.js | 2 +-
node_modules/shelljs/src/rm.js | 6 +-
node_modules/shelljs/src/sed.js | 43 +-
node_modules/shelljs/src/set.js | 49 -
node_modules/shelljs/src/tempdir.js | 3 +-
node_modules/shelljs/src/test.js | 2 +-
node_modules/shelljs/src/to.js | 1 -
node_modules/shelljs/src/toEnd.js | 1 -
node_modules/shelljs/src/touch.js | 109 -
node_modules/shelljs/src/which.js | 55 +-
node_modules/underscore/package.json | 3 +-
node_modules/util-deprecate/package.json | 5 +-
package.json | 4 +-
103 files changed, 346 insertions(+), 7194 deletions(-)
----------------------------------------------------------------------
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/bin/templates/scripts/cordova/lib/prepare.js
----------------------------------------------------------------------
diff --git a/bin/templates/scripts/cordova/lib/prepare.js b/bin/templates/scripts/cordova/lib/prepare.js
index 3e6389d..b090e6a 100644
--- a/bin/templates/scripts/cordova/lib/prepare.js
+++ b/bin/templates/scripts/cordova/lib/prepare.js
@@ -69,7 +69,7 @@ module.exports.prepare = function (cordovaProject) {
* configuration is already dumped to appropriate config.xml file.
*/
function updateConfigFile(sourceConfig, configMunger, locations) {
- events.emit('verbose', 'Generating config.xml from defaults for platform "ios"');
+ events.emit('verbose', 'Generating config.xml from defaults for platform "osx"');
// First cleanup current config and merge project's one into own
// Overwrite platform config.xml with defaults.xml.
@@ -108,7 +108,7 @@ function updateWww(cordovaProject, destinations) {
// If project contains 'merges' for our platform, use them as another overrides
var merges_path = path.join(cordovaProject.root, 'merges', 'osx');
if (fs.existsSync(merges_path)) {
- events.emit('verbose', 'Found "merges" for ios platform. Copying over existing "www" files.');
+ events.emit('verbose', 'Found "merges" for osx platform. Copying over existing "www" files.');
var overrides = path.join(merges_path, '*');
shell.cp('-rf', overrides, destinations.www);
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/abbrev/package.json
----------------------------------------------------------------------
diff --git a/node_modules/abbrev/package.json b/node_modules/abbrev/package.json
index 3822a2d..cdfead8 100644
--- a/node_modules/abbrev/package.json
+++ b/node_modules/abbrev/package.json
@@ -26,6 +26,7 @@
"type": "range"
},
"_requiredBy": [
+ "/istanbul",
"/nopt"
],
"_resolved": "http://registry.npmjs.org/abbrev/-/abbrev-1.0.7.tgz",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/brace-expansion/package.json
----------------------------------------------------------------------
diff --git a/node_modules/brace-expansion/package.json b/node_modules/brace-expansion/package.json
index acbbaa3..0e85889 100644
--- a/node_modules/brace-expansion/package.json
+++ b/node_modules/brace-expansion/package.json
@@ -30,6 +30,8 @@
"type": "range"
},
"_requiredBy": [
+ "/istanbul/minimatch",
+ "/jshint/minimatch",
"/minimatch"
],
"_resolved": "http://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.3.tgz",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/.bin/shjs
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/.bin/shjs b/node_modules/cordova-common/node_modules/.bin/shjs
deleted file mode 120000
index a044997..0000000
--- a/node_modules/cordova-common/node_modules/.bin/shjs
+++ /dev/null
@@ -1 +0,0 @@
-../shelljs/bin/shjs
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/.documentup.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.documentup.json b/node_modules/cordova-common/node_modules/shelljs/.documentup.json
deleted file mode 100644
index 57fe301..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/.documentup.json
+++ /dev/null
@@ -1,6 +0,0 @@
-{
- "name": "ShellJS",
- "twitter": [
- "r2r"
- ]
-}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.jshintrc b/node_modules/cordova-common/node_modules/shelljs/.jshintrc
deleted file mode 100644
index a80c559..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/.jshintrc
+++ /dev/null
@@ -1,7 +0,0 @@
-{
- "loopfunc": true,
- "sub": true,
- "undef": true,
- "unused": true,
- "node": true
-}
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.npmignore b/node_modules/cordova-common/node_modules/shelljs/.npmignore
deleted file mode 100644
index 6b20c38..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/.npmignore
+++ /dev/null
@@ -1,2 +0,0 @@
-test/
-tmp/
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/.travis.yml b/node_modules/cordova-common/node_modules/shelljs/.travis.yml
deleted file mode 100644
index 1b3280a..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/.travis.yml
+++ /dev/null
@@ -1,6 +0,0 @@
-language: node_js
-node_js:
- - "0.10"
- - "0.11"
- - "0.12"
-
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/LICENSE b/node_modules/cordova-common/node_modules/shelljs/LICENSE
deleted file mode 100644
index 1b35ee9..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/LICENSE
+++ /dev/null
@@ -1,26 +0,0 @@
-Copyright (c) 2012, Artur Adib <aa...@mozilla.com>
-All rights reserved.
-
-You may use this project under the terms of the New BSD license as follows:
-
-Redistribution and use in source and binary forms, with or without
-modification, are permitted provided that the following conditions are met:
- * Redistributions of source code must retain the above copyright
- notice, this list of conditions and the following disclaimer.
- * Redistributions in binary form must reproduce the above copyright
- notice, this list of conditions and the following disclaimer in the
- documentation and/or other materials provided with the distribution.
- * Neither the name of Artur Adib nor the
- names of the contributors may be used to endorse or promote products
- derived from this software without specific prior written permission.
-
-THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
-ARE DISCLAIMED. IN NO EVENT SHALL ARTUR ADIB BE LIABLE FOR ANY
-DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
-(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
-LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
-ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
-(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
-THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/README.md b/node_modules/cordova-common/node_modules/shelljs/README.md
deleted file mode 100644
index d08d13e..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/README.md
+++ /dev/null
@@ -1,579 +0,0 @@
-# ShellJS - Unix shell commands for Node.js [](http://travis-ci.org/arturadib/shelljs)
-
-ShellJS is a portable **(Windows/Linux/OS X)** implementation of Unix shell commands on top of the Node.js API. You can use it to eliminate your shell script's dependency on Unix while still keeping its familiar and powerful commands. You can also install it globally so you can run it from outside Node projects - say goodbye to those gnarly Bash scripts!
-
-The project is [unit-tested](http://travis-ci.org/arturadib/shelljs) and battled-tested in projects like:
-
-+ [PDF.js](http://github.com/mozilla/pdf.js) - Firefox's next-gen PDF reader
-+ [Firebug](http://getfirebug.com/) - Firefox's infamous debugger
-+ [JSHint](http://jshint.com) - Most popular JavaScript linter
-+ [Zepto](http://zeptojs.com) - jQuery-compatible JavaScript library for modern browsers
-+ [Yeoman](http://yeoman.io/) - Web application stack and development tool
-+ [Deployd.com](http://deployd.com) - Open source PaaS for quick API backend generation
-
-and [many more](https://npmjs.org/browse/depended/shelljs).
-
-Connect with [@r2r](http://twitter.com/r2r) on Twitter for questions, suggestions, etc.
-
-## Installing
-
-Via npm:
-
-```bash
-$ npm install [-g] shelljs
-```
-
-If the global option `-g` is specified, the binary `shjs` will be installed. This makes it possible to
-run ShellJS scripts much like any shell script from the command line, i.e. without requiring a `node_modules` folder:
-
-```bash
-$ shjs my_script
-```
-
-You can also just copy `shell.js` into your project's directory, and `require()` accordingly.
-
-
-## Examples
-
-### JavaScript
-
-```javascript
-require('shelljs/global');
-
-if (!which('git')) {
- echo('Sorry, this script requires git');
- exit(1);
-}
-
-// Copy files to release dir
-mkdir('-p', 'out/Release');
-cp('-R', 'stuff/*', 'out/Release');
-
-// Replace macros in each .js file
-cd('lib');
-ls('*.js').forEach(function(file) {
- sed('-i', 'BUILD_VERSION', 'v0.1.2', file);
- sed('-i', /.*REMOVE_THIS_LINE.*\n/, '', file);
- sed('-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file);
-});
-cd('..');
-
-// Run external tool synchronously
-if (exec('git commit -am "Auto-commit"').code !== 0) {
- echo('Error: Git commit failed');
- exit(1);
-}
-```
-
-### CoffeeScript
-
-```coffeescript
-require 'shelljs/global'
-
-if not which 'git'
- echo 'Sorry, this script requires git'
- exit 1
-
-# Copy files to release dir
-mkdir '-p', 'out/Release'
-cp '-R', 'stuff/*', 'out/Release'
-
-# Replace macros in each .js file
-cd 'lib'
-for file in ls '*.js'
- sed '-i', 'BUILD_VERSION', 'v0.1.2', file
- sed '-i', /.*REMOVE_THIS_LINE.*\n/, '', file
- sed '-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat 'macro.js', file
-cd '..'
-
-# Run external tool synchronously
-if (exec 'git commit -am "Auto-commit"').code != 0
- echo 'Error: Git commit failed'
- exit 1
-```
-
-## Global vs. Local
-
-The example above uses the convenience script `shelljs/global` to reduce verbosity. If polluting your global namespace is not desirable, simply require `shelljs`.
-
-Example:
-
-```javascript
-var shell = require('shelljs');
-shell.echo('hello world');
-```
-
-## Make tool
-
-A convenience script `shelljs/make` is also provided to mimic the behavior of a Unix Makefile. In this case all shell objects are global, and command line arguments will cause the script to execute only the corresponding function in the global `target` object. To avoid redundant calls, target functions are executed only once per script.
-
-Example (CoffeeScript):
-
-```coffeescript
-require 'shelljs/make'
-
-target.all = ->
- target.bundle()
- target.docs()
-
-target.bundle = ->
- cd __dirname
- mkdir 'build'
- cd 'lib'
- (cat '*.js').to '../build/output.js'
-
-target.docs = ->
- cd __dirname
- mkdir 'docs'
- cd 'lib'
- for file in ls '*.js'
- text = grep '//@', file # extract special comments
- text.replace '//@', '' # remove comment tags
- text.to 'docs/my_docs.md'
-```
-
-To run the target `all`, call the above script without arguments: `$ node make`. To run the target `docs`: `$ node make docs`.
-
-You can also pass arguments to your targets by using the `--` separator. For example, to pass `arg1` and `arg2` to a target `bundle`, do `$ node make bundle -- arg1 arg2`:
-
-```javascript
-require('shelljs/make');
-
-target.bundle = function(argsArray) {
- // argsArray = ['arg1', 'arg2']
- /* ... */
-}
-```
-
-
-<!-- DO NOT MODIFY BEYOND THIS POINT - IT'S AUTOMATICALLY GENERATED -->
-
-
-## Command reference
-
-
-All commands run synchronously, unless otherwise stated.
-
-
-### cd('dir')
-Changes to directory `dir` for the duration of the script
-
-
-### pwd()
-Returns the current directory.
-
-
-### ls([options ,] path [,path ...])
-### ls([options ,] path_array)
-Available options:
-
-+ `-R`: recursive
-+ `-A`: all files (include files beginning with `.`, except for `.` and `..`)
-
-Examples:
-
-```javascript
-ls('projs/*.js');
-ls('-R', '/users/me', '/tmp');
-ls('-R', ['/users/me', '/tmp']); // same as above
-```
-
-Returns array of files in the given path, or in current directory if no path provided.
-
-
-### find(path [,path ...])
-### find(path_array)
-Examples:
-
-```javascript
-find('src', 'lib');
-find(['src', 'lib']); // same as above
-find('.').filter(function(file) { return file.match(/\.js$/); });
-```
-
-Returns array of all files (however deep) in the given paths.
-
-The main difference from `ls('-R', path)` is that the resulting file names
-include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
-
-
-### cp([options ,] source [,source ...], dest)
-### cp([options ,] source_array, dest)
-Available options:
-
-+ `-f`: force
-+ `-r, -R`: recursive
-
-Examples:
-
-```javascript
-cp('file1', 'dir1');
-cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
-cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
-```
-
-Copies files. The wildcard `*` is accepted.
-
-
-### rm([options ,] file [, file ...])
-### rm([options ,] file_array)
-Available options:
-
-+ `-f`: force
-+ `-r, -R`: recursive
-
-Examples:
-
-```javascript
-rm('-rf', '/tmp/*');
-rm('some_file.txt', 'another_file.txt');
-rm(['some_file.txt', 'another_file.txt']); // same as above
-```
-
-Removes files. The wildcard `*` is accepted.
-
-
-### mv(source [, source ...], dest')
-### mv(source_array, dest')
-Available options:
-
-+ `f`: force
-
-Examples:
-
-```javascript
-mv('-f', 'file', 'dir/');
-mv('file1', 'file2', 'dir/');
-mv(['file1', 'file2'], 'dir/'); // same as above
-```
-
-Moves files. The wildcard `*` is accepted.
-
-
-### mkdir([options ,] dir [, dir ...])
-### mkdir([options ,] dir_array)
-Available options:
-
-+ `p`: full path (will create intermediate dirs if necessary)
-
-Examples:
-
-```javascript
-mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
-mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
-```
-
-Creates directories.
-
-
-### test(expression)
-Available expression primaries:
-
-+ `'-b', 'path'`: true if path is a block device
-+ `'-c', 'path'`: true if path is a character device
-+ `'-d', 'path'`: true if path is a directory
-+ `'-e', 'path'`: true if path exists
-+ `'-f', 'path'`: true if path is a regular file
-+ `'-L', 'path'`: true if path is a symbolic link
-+ `'-p', 'path'`: true if path is a pipe (FIFO)
-+ `'-S', 'path'`: true if path is a socket
-
-Examples:
-
-```javascript
-if (test('-d', path)) { /* do something with dir */ };
-if (!test('-f', path)) continue; // skip if it's a regular file
-```
-
-Evaluates expression using the available primaries and returns corresponding value.
-
-
-### cat(file [, file ...])
-### cat(file_array)
-
-Examples:
-
-```javascript
-var str = cat('file*.txt');
-var str = cat('file1', 'file2');
-var str = cat(['file1', 'file2']); // same as above
-```
-
-Returns a string containing the given file, or a concatenated string
-containing the files if more than one file is given (a new line character is
-introduced between each file). Wildcard `*` accepted.
-
-
-### 'string'.to(file)
-
-Examples:
-
-```javascript
-cat('input.txt').to('output.txt');
-```
-
-Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
-those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
-
-
-### 'string'.toEnd(file)
-
-Examples:
-
-```javascript
-cat('input.txt').toEnd('output.txt');
-```
-
-Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
-those returned by `cat`, `grep`, etc).
-
-
-### sed([options ,] search_regex, replacement, file)
-Available options:
-
-+ `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
-
-Examples:
-
-```javascript
-sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
-sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
-```
-
-Reads an input string from `file` and performs a JavaScript `replace()` on the input
-using the given search regex and replacement string or function. Returns the new string after replacement.
-
-
-### grep([options ,] regex_filter, file [, file ...])
-### grep([options ,] regex_filter, file_array)
-Available options:
-
-+ `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
-
-Examples:
-
-```javascript
-grep('-v', 'GLOBAL_VARIABLE', '*.js');
-grep('GLOBAL_VARIABLE', '*.js');
-```
-
-Reads input string from given files and returns a string containing all lines of the
-file that match the given `regex_filter`. Wildcard `*` accepted.
-
-
-### which(command)
-
-Examples:
-
-```javascript
-var nodeExec = which('node');
-```
-
-Searches for `command` in the system's PATH. On Windows looks for `.exe`, `.cmd`, and `.bat` extensions.
-Returns string containing the absolute path to the command.
-
-
-### echo(string [,string ...])
-
-Examples:
-
-```javascript
-echo('hello world');
-var str = echo('hello world');
-```
-
-Prints string to stdout, and returns string with additional utility methods
-like `.to()`.
-
-
-### pushd([options,] [dir | '-N' | '+N'])
-
-Available options:
-
-+ `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
-
-Arguments:
-
-+ `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
-+ `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
-+ `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
-
-Examples:
-
-```javascript
-// process.cwd() === '/usr'
-pushd('/etc'); // Returns /etc /usr
-pushd('+1'); // Returns /usr /etc
-```
-
-Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
-
-### popd([options,] ['-N' | '+N'])
-
-Available options:
-
-+ `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
-
-Arguments:
-
-+ `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
-+ `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
-
-Examples:
-
-```javascript
-echo(process.cwd()); // '/usr'
-pushd('/etc'); // '/etc /usr'
-echo(process.cwd()); // '/etc'
-popd(); // '/usr'
-echo(process.cwd()); // '/usr'
-```
-
-When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
-
-### dirs([options | '+N' | '-N'])
-
-Available options:
-
-+ `-c`: Clears the directory stack by deleting all of the elements.
-
-Arguments:
-
-+ `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
-+ `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
-
-Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
-
-See also: pushd, popd
-
-
-### ln(options, source, dest)
-### ln(source, dest)
-Available options:
-
-+ `s`: symlink
-+ `f`: force
-
-Examples:
-
-```javascript
-ln('file', 'newlink');
-ln('-sf', 'file', 'existing');
-```
-
-Links source to dest. Use -f to force the link, should dest already exist.
-
-
-### exit(code)
-Exits the current process with the given exit code.
-
-### env['VAR_NAME']
-Object containing environment variables (both getter and setter). Shortcut to process.env.
-
-### exec(command [, options] [, callback])
-Available options (all `false` by default):
-
-+ `async`: Asynchronous execution. Defaults to true if a callback is provided.
-+ `silent`: Do not echo program output to console.
-
-Examples:
-
-```javascript
-var version = exec('node --version', {silent:true}).output;
-
-var child = exec('some_long_running_process', {async:true});
-child.stdout.on('data', function(data) {
- /* ... do something with data ... */
-});
-
-exec('some_long_running_process', function(code, output) {
- console.log('Exit code:', code);
- console.log('Program output:', output);
-});
-```
-
-Executes the given `command` _synchronously_, unless otherwise specified.
-When in synchronous mode returns the object `{ code:..., output:... }`, containing the program's
-`output` (stdout + stderr) and its exit `code`. Otherwise returns the child process object, and
-the `callback` gets the arguments `(code, output)`.
-
-**Note:** For long-lived processes, it's best to run `exec()` asynchronously as
-the current synchronous implementation uses a lot of CPU. This should be getting
-fixed soon.
-
-
-### chmod(octal_mode || octal_string, file)
-### chmod(symbolic_mode, file)
-
-Available options:
-
-+ `-v`: output a diagnostic for every file processed
-+ `-c`: like verbose but report only when a change is made
-+ `-R`: change files and directories recursively
-
-Examples:
-
-```javascript
-chmod(755, '/Users/brandon');
-chmod('755', '/Users/brandon'); // same as above
-chmod('u+x', '/Users/brandon');
-```
-
-Alters the permissions of a file or directory by either specifying the
-absolute permissions in octal form or expressing the changes in symbols.
-This command tries to mimic the POSIX behavior as much as possible.
-Notable exceptions:
-
-+ In symbolic modes, 'a-r' and '-r' are identical. No consideration is
- given to the umask.
-+ There is no "quiet" option since default behavior is to run silent.
-
-
-## Non-Unix commands
-
-
-### tempdir()
-
-Examples:
-
-```javascript
-var tmp = tempdir(); // "/tmp" for most *nix platforms
-```
-
-Searches and returns string containing a writeable, platform-dependent temporary directory.
-Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
-
-
-### error()
-Tests if error occurred in the last command. Returns `null` if no error occurred,
-otherwise returns string explaining the error
-
-
-## Configuration
-
-
-### config.silent
-Example:
-
-```javascript
-var sh = require('shelljs');
-var silentState = sh.config.silent; // save old silent state
-sh.config.silent = true;
-/* ... */
-sh.config.silent = silentState; // restore old silent state
-```
-
-Suppresses all command output if `true`, except for `echo()` calls.
-Default is `false`.
-
-### config.fatal
-Example:
-
-```javascript
-require('shelljs/global');
-config.fatal = true;
-cp('this_file_does_not_exist', '/dev/null'); // dies here
-/* more commands... */
-```
-
-If `true` the script will die on errors. Default is `false`.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/RELEASE.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/RELEASE.md b/node_modules/cordova-common/node_modules/shelljs/RELEASE.md
deleted file mode 100644
index 69ef3fb..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/RELEASE.md
+++ /dev/null
@@ -1,9 +0,0 @@
-# Release steps
-
-* Ensure master passes CI tests
-* Bump version in package.json. Any breaking change or new feature should bump minor (or even major). Non-breaking changes or fixes can just bump patch.
-* Update README manually if the changes are not documented in-code. If so, run `scripts/generate-docs.js`
-* Commit
-* `$ git tag <version>` (see `git tag -l` for latest)
-* `$ git push origin master --tags`
-* `$ npm publish .`
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/bin/shjs
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/bin/shjs b/node_modules/cordova-common/node_modules/shelljs/bin/shjs
deleted file mode 100755
index d239a7a..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/bin/shjs
+++ /dev/null
@@ -1,51 +0,0 @@
-#!/usr/bin/env node
-require('../global');
-
-if (process.argv.length < 3) {
- console.log('ShellJS: missing argument (script name)');
- console.log();
- process.exit(1);
-}
-
-var args,
- scriptName = process.argv[2];
-env['NODE_PATH'] = __dirname + '/../..';
-
-if (!scriptName.match(/\.js/) && !scriptName.match(/\.coffee/)) {
- if (test('-f', scriptName + '.js'))
- scriptName += '.js';
- if (test('-f', scriptName + '.coffee'))
- scriptName += '.coffee';
-}
-
-if (!test('-f', scriptName)) {
- console.log('ShellJS: script not found ('+scriptName+')');
- console.log();
- process.exit(1);
-}
-
-args = process.argv.slice(3);
-
-for (var i = 0, l = args.length; i < l; i++) {
- if (args[i][0] !== "-"){
- args[i] = '"' + args[i] + '"'; // fixes arguments with multiple words
- }
-}
-
-if (scriptName.match(/\.coffee$/)) {
- //
- // CoffeeScript
- //
- if (which('coffee')) {
- exec('coffee ' + scriptName + ' ' + args.join(' '), { async: true });
- } else {
- console.log('ShellJS: CoffeeScript interpreter not found');
- console.log();
- process.exit(1);
- }
-} else {
- //
- // JavaScript
- //
- exec('node ' + scriptName + ' ' + args.join(' '), { async: true });
-}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/global.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/global.js b/node_modules/cordova-common/node_modules/shelljs/global.js
deleted file mode 100644
index 97f0033..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/global.js
+++ /dev/null
@@ -1,3 +0,0 @@
-var shell = require('./shell.js');
-for (var cmd in shell)
- global[cmd] = shell[cmd];
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/make.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/make.js b/node_modules/cordova-common/node_modules/shelljs/make.js
deleted file mode 100644
index f78b4cf..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/make.js
+++ /dev/null
@@ -1,56 +0,0 @@
-require('./global');
-
-global.config.fatal = true;
-global.target = {};
-
-var args = process.argv.slice(2),
- targetArgs,
- dashesLoc = args.indexOf('--');
-
-// split args, everything after -- if only for targets
-if (dashesLoc > -1) {
- targetArgs = args.slice(dashesLoc + 1, args.length);
- args = args.slice(0, dashesLoc);
-}
-
-// This ensures we only execute the script targets after the entire script has
-// been evaluated
-setTimeout(function() {
- var t;
-
- if (args.length === 1 && args[0] === '--help') {
- console.log('Available targets:');
- for (t in global.target)
- console.log(' ' + t);
- return;
- }
-
- // Wrap targets to prevent duplicate execution
- for (t in global.target) {
- (function(t, oldTarget){
-
- // Wrap it
- global.target[t] = function() {
- if (oldTarget.done)
- return;
- oldTarget.done = true;
- return oldTarget.apply(oldTarget, arguments);
- };
-
- })(t, global.target[t]);
- }
-
- // Execute desired targets
- if (args.length > 0) {
- args.forEach(function(arg) {
- if (arg in global.target)
- global.target[arg](targetArgs);
- else {
- console.log('no such target: ' + arg);
- }
- });
- } else if ('all' in global.target) {
- global.target.all(targetArgs);
- }
-
-}, 0);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/package.json b/node_modules/cordova-common/node_modules/shelljs/package.json
deleted file mode 100644
index f6f61cc..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/package.json
+++ /dev/null
@@ -1,88 +0,0 @@
-{
- "_args": [
- [
- "shelljs@^0.5.1",
- "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
- ]
- ],
- "_from": "shelljs@>=0.5.1 <0.6.0",
- "_id": "shelljs@0.5.3",
- "_inCache": true,
- "_installable": true,
- "_location": "/cordova-common/shelljs",
- "_nodeVersion": "1.2.0",
- "_npmUser": {
- "email": "arturadib@gmail.com",
- "name": "artur"
- },
- "_npmVersion": "2.5.1",
- "_phantomChildren": {},
- "_requested": {
- "name": "shelljs",
- "raw": "shelljs@^0.5.1",
- "rawSpec": "^0.5.1",
- "scope": null,
- "spec": ">=0.5.1 <0.6.0",
- "type": "range"
- },
- "_requiredBy": [
- "/cordova-common"
- ],
- "_resolved": "http://registry.npmjs.org/shelljs/-/shelljs-0.5.3.tgz",
- "_shasum": "c54982b996c76ef0c1e6b59fbdc5825f5b713113",
- "_shrinkwrap": null,
- "_spec": "shelljs@^0.5.1",
- "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
- "author": {
- "email": "arturadib@gmail.com",
- "name": "Artur Adib"
- },
- "bin": {
- "shjs": "./bin/shjs"
- },
- "bugs": {
- "url": "https://github.com/arturadib/shelljs/issues"
- },
- "dependencies": {},
- "description": "Portable Unix shell commands for Node.js",
- "devDependencies": {
- "jshint": "~2.1.11"
- },
- "directories": {},
- "dist": {
- "shasum": "c54982b996c76ef0c1e6b59fbdc5825f5b713113",
- "tarball": "http://registry.npmjs.org/shelljs/-/shelljs-0.5.3.tgz"
- },
- "engines": {
- "node": ">=0.8.0"
- },
- "gitHead": "22d0975040b9b8234755dc6e692d6869436e8485",
- "homepage": "http://github.com/arturadib/shelljs",
- "keywords": [
- "jake",
- "make",
- "makefile",
- "shell",
- "synchronous",
- "unix"
- ],
- "license": "BSD*",
- "main": "./shell.js",
- "maintainers": [
- {
- "name": "artur",
- "email": "arturadib@gmail.com"
- }
- ],
- "name": "shelljs",
- "optionalDependencies": {},
- "readme": "ERROR: No README data found!",
- "repository": {
- "type": "git",
- "url": "git://github.com/arturadib/shelljs.git"
- },
- "scripts": {
- "test": "node scripts/run-tests"
- },
- "version": "0.5.3"
-}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js b/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js
deleted file mode 100755
index 532fed9..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/scripts/generate-docs.js
+++ /dev/null
@@ -1,21 +0,0 @@
-#!/usr/bin/env node
-require('../global');
-
-echo('Appending docs to README.md');
-
-cd(__dirname + '/..');
-
-// Extract docs from shell.js
-var docs = grep('//@', 'shell.js');
-
-docs = docs.replace(/\/\/\@include (.+)/g, function(match, path) {
- var file = path.match('.js$') ? path : path+'.js';
- return grep('//@', file);
-});
-
-// Remove '//@'
-docs = docs.replace(/\/\/\@ ?/g, '');
-// Append docs to README
-sed('-i', /## Command reference(.|\n)*/, '## Command reference\n\n' + docs, 'README.md');
-
-echo('All done.');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js b/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js
deleted file mode 100755
index f9d31e0..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/scripts/run-tests.js
+++ /dev/null
@@ -1,50 +0,0 @@
-#!/usr/bin/env node
-require('../global');
-
-var path = require('path');
-
-var failed = false;
-
-//
-// Lint
-//
-JSHINT_BIN = './node_modules/jshint/bin/jshint';
-cd(__dirname + '/..');
-
-if (!test('-f', JSHINT_BIN)) {
- echo('JSHint not found. Run `npm install` in the root dir first.');
- exit(1);
-}
-
-if (exec(JSHINT_BIN + ' *.js test/*.js').code !== 0) {
- failed = true;
- echo('*** JSHINT FAILED! (return code != 0)');
- echo();
-} else {
- echo('All JSHint tests passed');
- echo();
-}
-
-//
-// Unit tests
-//
-cd(__dirname + '/../test');
-ls('*.js').forEach(function(file) {
- echo('Running test:', file);
- if (exec('node ' + file).code !== 123) { // 123 avoids false positives (e.g. premature exit)
- failed = true;
- echo('*** TEST FAILED! (missing exit code "123")');
- echo();
- }
-});
-
-if (failed) {
- echo();
- echo('*******************************************************');
- echo('WARNING: Some tests did not pass!');
- echo('*******************************************************');
- exit(1);
-} else {
- echo();
- echo('All tests passed.');
-}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/shell.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/shell.js b/node_modules/cordova-common/node_modules/shelljs/shell.js
deleted file mode 100644
index bdeb559..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/shell.js
+++ /dev/null
@@ -1,159 +0,0 @@
-//
-// ShellJS
-// Unix shell commands on top of Node's API
-//
-// Copyright (c) 2012 Artur Adib
-// http://github.com/arturadib/shelljs
-//
-
-var common = require('./src/common');
-
-
-//@
-//@ All commands run synchronously, unless otherwise stated.
-//@
-
-//@include ./src/cd
-var _cd = require('./src/cd');
-exports.cd = common.wrap('cd', _cd);
-
-//@include ./src/pwd
-var _pwd = require('./src/pwd');
-exports.pwd = common.wrap('pwd', _pwd);
-
-//@include ./src/ls
-var _ls = require('./src/ls');
-exports.ls = common.wrap('ls', _ls);
-
-//@include ./src/find
-var _find = require('./src/find');
-exports.find = common.wrap('find', _find);
-
-//@include ./src/cp
-var _cp = require('./src/cp');
-exports.cp = common.wrap('cp', _cp);
-
-//@include ./src/rm
-var _rm = require('./src/rm');
-exports.rm = common.wrap('rm', _rm);
-
-//@include ./src/mv
-var _mv = require('./src/mv');
-exports.mv = common.wrap('mv', _mv);
-
-//@include ./src/mkdir
-var _mkdir = require('./src/mkdir');
-exports.mkdir = common.wrap('mkdir', _mkdir);
-
-//@include ./src/test
-var _test = require('./src/test');
-exports.test = common.wrap('test', _test);
-
-//@include ./src/cat
-var _cat = require('./src/cat');
-exports.cat = common.wrap('cat', _cat);
-
-//@include ./src/to
-var _to = require('./src/to');
-String.prototype.to = common.wrap('to', _to);
-
-//@include ./src/toEnd
-var _toEnd = require('./src/toEnd');
-String.prototype.toEnd = common.wrap('toEnd', _toEnd);
-
-//@include ./src/sed
-var _sed = require('./src/sed');
-exports.sed = common.wrap('sed', _sed);
-
-//@include ./src/grep
-var _grep = require('./src/grep');
-exports.grep = common.wrap('grep', _grep);
-
-//@include ./src/which
-var _which = require('./src/which');
-exports.which = common.wrap('which', _which);
-
-//@include ./src/echo
-var _echo = require('./src/echo');
-exports.echo = _echo; // don't common.wrap() as it could parse '-options'
-
-//@include ./src/dirs
-var _dirs = require('./src/dirs').dirs;
-exports.dirs = common.wrap("dirs", _dirs);
-var _pushd = require('./src/dirs').pushd;
-exports.pushd = common.wrap('pushd', _pushd);
-var _popd = require('./src/dirs').popd;
-exports.popd = common.wrap("popd", _popd);
-
-//@include ./src/ln
-var _ln = require('./src/ln');
-exports.ln = common.wrap('ln', _ln);
-
-//@
-//@ ### exit(code)
-//@ Exits the current process with the given exit code.
-exports.exit = process.exit;
-
-//@
-//@ ### env['VAR_NAME']
-//@ Object containing environment variables (both getter and setter). Shortcut to process.env.
-exports.env = process.env;
-
-//@include ./src/exec
-var _exec = require('./src/exec');
-exports.exec = common.wrap('exec', _exec, {notUnix:true});
-
-//@include ./src/chmod
-var _chmod = require('./src/chmod');
-exports.chmod = common.wrap('chmod', _chmod);
-
-
-
-//@
-//@ ## Non-Unix commands
-//@
-
-//@include ./src/tempdir
-var _tempDir = require('./src/tempdir');
-exports.tempdir = common.wrap('tempdir', _tempDir);
-
-
-//@include ./src/error
-var _error = require('./src/error');
-exports.error = _error;
-
-
-
-//@
-//@ ## Configuration
-//@
-
-exports.config = common.config;
-
-//@
-//@ ### config.silent
-//@ Example:
-//@
-//@ ```javascript
-//@ var sh = require('shelljs');
-//@ var silentState = sh.config.silent; // save old silent state
-//@ sh.config.silent = true;
-//@ /* ... */
-//@ sh.config.silent = silentState; // restore old silent state
-//@ ```
-//@
-//@ Suppresses all command output if `true`, except for `echo()` calls.
-//@ Default is `false`.
-
-//@
-//@ ### config.fatal
-//@ Example:
-//@
-//@ ```javascript
-//@ require('shelljs/global');
-//@ config.fatal = true;
-//@ cp('this_file_does_not_exist', '/dev/null'); // dies here
-//@ /* more commands... */
-//@ ```
-//@
-//@ If `true` the script will die on errors. Default is `false`.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/cat.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/cat.js b/node_modules/cordova-common/node_modules/shelljs/src/cat.js
deleted file mode 100644
index f6f4d25..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/cat.js
+++ /dev/null
@@ -1,43 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### cat(file [, file ...])
-//@ ### cat(file_array)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var str = cat('file*.txt');
-//@ var str = cat('file1', 'file2');
-//@ var str = cat(['file1', 'file2']); // same as above
-//@ ```
-//@
-//@ Returns a string containing the given file, or a concatenated string
-//@ containing the files if more than one file is given (a new line character is
-//@ introduced between each file). Wildcard `*` accepted.
-function _cat(options, files) {
- var cat = '';
-
- if (!files)
- common.error('no paths given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 1);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- files.forEach(function(file) {
- if (!fs.existsSync(file))
- common.error('no such file or directory: ' + file);
-
- cat += fs.readFileSync(file, 'utf8') + '\n';
- });
-
- if (cat[cat.length-1] === '\n')
- cat = cat.substring(0, cat.length-1);
-
- return common.ShellString(cat);
-}
-module.exports = _cat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/cd.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/cd.js b/node_modules/cordova-common/node_modules/shelljs/src/cd.js
deleted file mode 100644
index 230f432..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/cd.js
+++ /dev/null
@@ -1,19 +0,0 @@
-var fs = require('fs');
-var common = require('./common');
-
-//@
-//@ ### cd('dir')
-//@ Changes to directory `dir` for the duration of the script
-function _cd(options, dir) {
- if (!dir)
- common.error('directory not specified');
-
- if (!fs.existsSync(dir))
- common.error('no such file or directory: ' + dir);
-
- if (!fs.statSync(dir).isDirectory())
- common.error('not a directory: ' + dir);
-
- process.chdir(dir);
-}
-module.exports = _cd;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/chmod.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/chmod.js b/node_modules/cordova-common/node_modules/shelljs/src/chmod.js
deleted file mode 100644
index f288893..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/chmod.js
+++ /dev/null
@@ -1,208 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-var PERMS = (function (base) {
- return {
- OTHER_EXEC : base.EXEC,
- OTHER_WRITE : base.WRITE,
- OTHER_READ : base.READ,
-
- GROUP_EXEC : base.EXEC << 3,
- GROUP_WRITE : base.WRITE << 3,
- GROUP_READ : base.READ << 3,
-
- OWNER_EXEC : base.EXEC << 6,
- OWNER_WRITE : base.WRITE << 6,
- OWNER_READ : base.READ << 6,
-
- // Literal octal numbers are apparently not allowed in "strict" javascript. Using parseInt is
- // the preferred way, else a jshint warning is thrown.
- STICKY : parseInt('01000', 8),
- SETGID : parseInt('02000', 8),
- SETUID : parseInt('04000', 8),
-
- TYPE_MASK : parseInt('0770000', 8)
- };
-})({
- EXEC : 1,
- WRITE : 2,
- READ : 4
-});
-
-//@
-//@ ### chmod(octal_mode || octal_string, file)
-//@ ### chmod(symbolic_mode, file)
-//@
-//@ Available options:
-//@
-//@ + `-v`: output a diagnostic for every file processed//@
-//@ + `-c`: like verbose but report only when a change is made//@
-//@ + `-R`: change files and directories recursively//@
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ chmod(755, '/Users/brandon');
-//@ chmod('755', '/Users/brandon'); // same as above
-//@ chmod('u+x', '/Users/brandon');
-//@ ```
-//@
-//@ Alters the permissions of a file or directory by either specifying the
-//@ absolute permissions in octal form or expressing the changes in symbols.
-//@ This command tries to mimic the POSIX behavior as much as possible.
-//@ Notable exceptions:
-//@
-//@ + In symbolic modes, 'a-r' and '-r' are identical. No consideration is
-//@ given to the umask.
-//@ + There is no "quiet" option since default behavior is to run silent.
-function _chmod(options, mode, filePattern) {
- if (!filePattern) {
- if (options.length > 0 && options.charAt(0) === '-') {
- // Special case where the specified file permissions started with - to subtract perms, which
- // get picked up by the option parser as command flags.
- // If we are down by one argument and options starts with -, shift everything over.
- filePattern = mode;
- mode = options;
- options = '';
- }
- else {
- common.error('You must specify a file.');
- }
- }
-
- options = common.parseOptions(options, {
- 'R': 'recursive',
- 'c': 'changes',
- 'v': 'verbose'
- });
-
- if (typeof filePattern === 'string') {
- filePattern = [ filePattern ];
- }
-
- var files;
-
- if (options.recursive) {
- files = [];
- common.expand(filePattern).forEach(function addFile(expandedFile) {
- var stat = fs.lstatSync(expandedFile);
-
- if (!stat.isSymbolicLink()) {
- files.push(expandedFile);
-
- if (stat.isDirectory()) { // intentionally does not follow symlinks.
- fs.readdirSync(expandedFile).forEach(function (child) {
- addFile(expandedFile + '/' + child);
- });
- }
- }
- });
- }
- else {
- files = common.expand(filePattern);
- }
-
- files.forEach(function innerChmod(file) {
- file = path.resolve(file);
- if (!fs.existsSync(file)) {
- common.error('File not found: ' + file);
- }
-
- // When recursing, don't follow symlinks.
- if (options.recursive && fs.lstatSync(file).isSymbolicLink()) {
- return;
- }
-
- var perms = fs.statSync(file).mode;
- var type = perms & PERMS.TYPE_MASK;
-
- var newPerms = perms;
-
- if (isNaN(parseInt(mode, 8))) {
- // parse options
- mode.split(',').forEach(function (symbolicMode) {
- /*jshint regexdash:true */
- var pattern = /([ugoa]*)([=\+-])([rwxXst]*)/i;
- var matches = pattern.exec(symbolicMode);
-
- if (matches) {
- var applyTo = matches[1];
- var operator = matches[2];
- var change = matches[3];
-
- var changeOwner = applyTo.indexOf('u') != -1 || applyTo === 'a' || applyTo === '';
- var changeGroup = applyTo.indexOf('g') != -1 || applyTo === 'a' || applyTo === '';
- var changeOther = applyTo.indexOf('o') != -1 || applyTo === 'a' || applyTo === '';
-
- var changeRead = change.indexOf('r') != -1;
- var changeWrite = change.indexOf('w') != -1;
- var changeExec = change.indexOf('x') != -1;
- var changeSticky = change.indexOf('t') != -1;
- var changeSetuid = change.indexOf('s') != -1;
-
- var mask = 0;
- if (changeOwner) {
- mask |= (changeRead ? PERMS.OWNER_READ : 0) + (changeWrite ? PERMS.OWNER_WRITE : 0) + (changeExec ? PERMS.OWNER_EXEC : 0) + (changeSetuid ? PERMS.SETUID : 0);
- }
- if (changeGroup) {
- mask |= (changeRead ? PERMS.GROUP_READ : 0) + (changeWrite ? PERMS.GROUP_WRITE : 0) + (changeExec ? PERMS.GROUP_EXEC : 0) + (changeSetuid ? PERMS.SETGID : 0);
- }
- if (changeOther) {
- mask |= (changeRead ? PERMS.OTHER_READ : 0) + (changeWrite ? PERMS.OTHER_WRITE : 0) + (changeExec ? PERMS.OTHER_EXEC : 0);
- }
-
- // Sticky bit is special - it's not tied to user, group or other.
- if (changeSticky) {
- mask |= PERMS.STICKY;
- }
-
- switch (operator) {
- case '+':
- newPerms |= mask;
- break;
-
- case '-':
- newPerms &= ~mask;
- break;
-
- case '=':
- newPerms = type + mask;
-
- // According to POSIX, when using = to explicitly set the permissions, setuid and setgid can never be cleared.
- if (fs.statSync(file).isDirectory()) {
- newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
- }
- break;
- }
-
- if (options.verbose) {
- log(file + ' -> ' + newPerms.toString(8));
- }
-
- if (perms != newPerms) {
- if (!options.verbose && options.changes) {
- log(file + ' -> ' + newPerms.toString(8));
- }
- fs.chmodSync(file, newPerms);
- }
- }
- else {
- common.error('Invalid symbolic mode change: ' + symbolicMode);
- }
- });
- }
- else {
- // they gave us a full number
- newPerms = type + parseInt(mode, 8);
-
- // POSIX rules are that setuid and setgid can only be added using numeric form, but not cleared.
- if (fs.statSync(file).isDirectory()) {
- newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
- }
-
- fs.chmodSync(file, newPerms);
- }
- });
-}
-module.exports = _chmod;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/common.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/common.js b/node_modules/cordova-common/node_modules/shelljs/src/common.js
deleted file mode 100644
index d8c2312..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/common.js
+++ /dev/null
@@ -1,203 +0,0 @@
-var os = require('os');
-var fs = require('fs');
-var _ls = require('./ls');
-
-// Module globals
-var config = {
- silent: false,
- fatal: false
-};
-exports.config = config;
-
-var state = {
- error: null,
- currentCmd: 'shell.js',
- tempDir: null
-};
-exports.state = state;
-
-var platform = os.type().match(/^Win/) ? 'win' : 'unix';
-exports.platform = platform;
-
-function log() {
- if (!config.silent)
- console.log.apply(this, arguments);
-}
-exports.log = log;
-
-// Shows error message. Throws unless _continue or config.fatal are true
-function error(msg, _continue) {
- if (state.error === null)
- state.error = '';
- state.error += state.currentCmd + ': ' + msg + '\n';
-
- if (msg.length > 0)
- log(state.error);
-
- if (config.fatal)
- process.exit(1);
-
- if (!_continue)
- throw '';
-}
-exports.error = error;
-
-// In the future, when Proxies are default, we can add methods like `.to()` to primitive strings.
-// For now, this is a dummy function to bookmark places we need such strings
-function ShellString(str) {
- return str;
-}
-exports.ShellString = ShellString;
-
-// Returns {'alice': true, 'bob': false} when passed a dictionary, e.g.:
-// parseOptions('-a', {'a':'alice', 'b':'bob'});
-function parseOptions(str, map) {
- if (!map)
- error('parseOptions() internal error: no map given');
-
- // All options are false by default
- var options = {};
- for (var letter in map)
- options[map[letter]] = false;
-
- if (!str)
- return options; // defaults
-
- if (typeof str !== 'string')
- error('parseOptions() internal error: wrong str');
-
- // e.g. match[1] = 'Rf' for str = '-Rf'
- var match = str.match(/^\-(.+)/);
- if (!match)
- return options;
-
- // e.g. chars = ['R', 'f']
- var chars = match[1].split('');
-
- chars.forEach(function(c) {
- if (c in map)
- options[map[c]] = true;
- else
- error('option not recognized: '+c);
- });
-
- return options;
-}
-exports.parseOptions = parseOptions;
-
-// Expands wildcards with matching (ie. existing) file names.
-// For example:
-// expand(['file*.js']) = ['file1.js', 'file2.js', ...]
-// (if the files 'file1.js', 'file2.js', etc, exist in the current dir)
-function expand(list) {
- var expanded = [];
- list.forEach(function(listEl) {
- // Wildcard present on directory names ?
- if(listEl.search(/\*[^\/]*\//) > -1 || listEl.search(/\*\*[^\/]*\//) > -1) {
- var match = listEl.match(/^([^*]+\/|)(.*)/);
- var root = match[1];
- var rest = match[2];
- var restRegex = rest.replace(/\*\*/g, ".*").replace(/\*/g, "[^\\/]*");
- restRegex = new RegExp(restRegex);
-
- _ls('-R', root).filter(function (e) {
- return restRegex.test(e);
- }).forEach(function(file) {
- expanded.push(file);
- });
- }
- // Wildcard present on file names ?
- else if (listEl.search(/\*/) > -1) {
- _ls('', listEl).forEach(function(file) {
- expanded.push(file);
- });
- } else {
- expanded.push(listEl);
- }
- });
- return expanded;
-}
-exports.expand = expand;
-
-// Normalizes _unlinkSync() across platforms to match Unix behavior, i.e.
-// file can be unlinked even if it's read-only, see https://github.com/joyent/node/issues/3006
-function unlinkSync(file) {
- try {
- fs.unlinkSync(file);
- } catch(e) {
- // Try to override file permission
- if (e.code === 'EPERM') {
- fs.chmodSync(file, '0666');
- fs.unlinkSync(file);
- } else {
- throw e;
- }
- }
-}
-exports.unlinkSync = unlinkSync;
-
-// e.g. 'shelljs_a5f185d0443ca...'
-function randomFileName() {
- function randomHash(count) {
- if (count === 1)
- return parseInt(16*Math.random(), 10).toString(16);
- else {
- var hash = '';
- for (var i=0; i<count; i++)
- hash += randomHash(1);
- return hash;
- }
- }
-
- return 'shelljs_'+randomHash(20);
-}
-exports.randomFileName = randomFileName;
-
-// extend(target_obj, source_obj1 [, source_obj2 ...])
-// Shallow extend, e.g.:
-// extend({A:1}, {b:2}, {c:3}) returns {A:1, b:2, c:3}
-function extend(target) {
- var sources = [].slice.call(arguments, 1);
- sources.forEach(function(source) {
- for (var key in source)
- target[key] = source[key];
- });
-
- return target;
-}
-exports.extend = extend;
-
-// Common wrapper for all Unix-like commands
-function wrap(cmd, fn, options) {
- return function() {
- var retValue = null;
-
- state.currentCmd = cmd;
- state.error = null;
-
- try {
- var args = [].slice.call(arguments, 0);
-
- if (options && options.notUnix) {
- retValue = fn.apply(this, args);
- } else {
- if (args.length === 0 || typeof args[0] !== 'string' || args[0][0] !== '-')
- args.unshift(''); // only add dummy option if '-option' not already present
- retValue = fn.apply(this, args);
- }
- } catch (e) {
- if (!state.error) {
- // If state.error hasn't been set it's an error thrown by Node, not us - probably a bug...
- console.log('shell.js: internal error');
- console.log(e.stack || e);
- process.exit(1);
- }
- if (config.fatal)
- throw e;
- }
-
- state.currentCmd = 'shell.js';
- return retValue;
- };
-} // wrap
-exports.wrap = wrap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/cp.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/cp.js b/node_modules/cordova-common/node_modules/shelljs/src/cp.js
deleted file mode 100644
index ef19f96..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/cp.js
+++ /dev/null
@@ -1,204 +0,0 @@
-var fs = require('fs');
-var path = require('path');
-var common = require('./common');
-var os = require('os');
-
-// Buffered file copy, synchronous
-// (Using readFileSync() + writeFileSync() could easily cause a memory overflow
-// with large files)
-function copyFileSync(srcFile, destFile) {
- if (!fs.existsSync(srcFile))
- common.error('copyFileSync: no such file or directory: ' + srcFile);
-
- var BUF_LENGTH = 64*1024,
- buf = new Buffer(BUF_LENGTH),
- bytesRead = BUF_LENGTH,
- pos = 0,
- fdr = null,
- fdw = null;
-
- try {
- fdr = fs.openSync(srcFile, 'r');
- } catch(e) {
- common.error('copyFileSync: could not read src file ('+srcFile+')');
- }
-
- try {
- fdw = fs.openSync(destFile, 'w');
- } catch(e) {
- common.error('copyFileSync: could not write to dest file (code='+e.code+'):'+destFile);
- }
-
- while (bytesRead === BUF_LENGTH) {
- bytesRead = fs.readSync(fdr, buf, 0, BUF_LENGTH, pos);
- fs.writeSync(fdw, buf, 0, bytesRead);
- pos += bytesRead;
- }
-
- fs.closeSync(fdr);
- fs.closeSync(fdw);
-
- fs.chmodSync(destFile, fs.statSync(srcFile).mode);
-}
-
-// Recursively copies 'sourceDir' into 'destDir'
-// Adapted from https://github.com/ryanmcgrath/wrench-js
-//
-// Copyright (c) 2010 Ryan McGrath
-// Copyright (c) 2012 Artur Adib
-//
-// Licensed under the MIT License
-// http://www.opensource.org/licenses/mit-license.php
-function cpdirSyncRecursive(sourceDir, destDir, opts) {
- if (!opts) opts = {};
-
- /* Create the directory where all our junk is moving to; read the mode of the source directory and mirror it */
- var checkDir = fs.statSync(sourceDir);
- try {
- fs.mkdirSync(destDir, checkDir.mode);
- } catch (e) {
- //if the directory already exists, that's okay
- if (e.code !== 'EEXIST') throw e;
- }
-
- var files = fs.readdirSync(sourceDir);
-
- for (var i = 0; i < files.length; i++) {
- var srcFile = sourceDir + "/" + files[i];
- var destFile = destDir + "/" + files[i];
- var srcFileStat = fs.lstatSync(srcFile);
-
- if (srcFileStat.isDirectory()) {
- /* recursion this thing right on back. */
- cpdirSyncRecursive(srcFile, destFile, opts);
- } else if (srcFileStat.isSymbolicLink()) {
- var symlinkFull = fs.readlinkSync(srcFile);
- fs.symlinkSync(symlinkFull, destFile, os.platform() === "win32" ? "junction" : null);
- } else {
- /* At this point, we've hit a file actually worth copying... so copy it on over. */
- if (fs.existsSync(destFile) && !opts.force) {
- common.log('skipping existing file: ' + files[i]);
- } else {
- copyFileSync(srcFile, destFile);
- }
- }
-
- } // for files
-} // cpdirSyncRecursive
-
-
-//@
-//@ ### cp([options ,] source [,source ...], dest)
-//@ ### cp([options ,] source_array, dest)
-//@ Available options:
-//@
-//@ + `-f`: force
-//@ + `-r, -R`: recursive
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ cp('file1', 'dir1');
-//@ cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
-//@ cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
-//@ ```
-//@
-//@ Copies files. The wildcard `*` is accepted.
-function _cp(options, sources, dest) {
- options = common.parseOptions(options, {
- 'f': 'force',
- 'R': 'recursive',
- 'r': 'recursive'
- });
-
- // Get sources, dest
- if (arguments.length < 3) {
- common.error('missing <source> and/or <dest>');
- } else if (arguments.length > 3) {
- sources = [].slice.call(arguments, 1, arguments.length - 1);
- dest = arguments[arguments.length - 1];
- } else if (typeof sources === 'string') {
- sources = [sources];
- } else if ('length' in sources) {
- sources = sources; // no-op for array
- } else {
- common.error('invalid arguments');
- }
-
- var exists = fs.existsSync(dest),
- stats = exists && fs.statSync(dest);
-
- // Dest is not existing dir, but multiple sources given
- if ((!exists || !stats.isDirectory()) && sources.length > 1)
- common.error('dest is not a directory (too many sources)');
-
- // Dest is an existing file, but no -f given
- if (exists && stats.isFile() && !options.force)
- common.error('dest file already exists: ' + dest);
-
- if (options.recursive) {
- // Recursive allows the shortcut syntax "sourcedir/" for "sourcedir/*"
- // (see Github issue #15)
- sources.forEach(function(src, i) {
- if (src[src.length - 1] === '/')
- sources[i] += '*';
- });
-
- // Create dest
- try {
- fs.mkdirSync(dest, parseInt('0777', 8));
- } catch (e) {
- // like Unix's cp, keep going even if we can't create dest dir
- }
- }
-
- sources = common.expand(sources);
-
- sources.forEach(function(src) {
- if (!fs.existsSync(src)) {
- common.error('no such file or directory: '+src, true);
- return; // skip file
- }
-
- // If here, src exists
- if (fs.statSync(src).isDirectory()) {
- if (!options.recursive) {
- // Non-Recursive
- common.log(src + ' is a directory (not copied)');
- } else {
- // Recursive
- // 'cp /a/source dest' should create 'source' in 'dest'
- var newDest = path.join(dest, path.basename(src)),
- checkDir = fs.statSync(src);
- try {
- fs.mkdirSync(newDest, checkDir.mode);
- } catch (e) {
- //if the directory already exists, that's okay
- if (e.code !== 'EEXIST') {
- common.error('dest file no such file or directory: ' + newDest, true);
- throw e;
- }
- }
-
- cpdirSyncRecursive(src, newDest, {force: options.force});
- }
- return; // done with dir
- }
-
- // If here, src is a file
-
- // When copying to '/path/dir':
- // thisDest = '/path/dir/file1'
- var thisDest = dest;
- if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
- thisDest = path.normalize(dest + '/' + path.basename(src));
-
- if (fs.existsSync(thisDest) && !options.force) {
- common.error('dest file already exists: ' + thisDest, true);
- return; // skip file
- }
-
- copyFileSync(src, thisDest);
- }); // forEach(src)
-}
-module.exports = _cp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/dirs.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/dirs.js b/node_modules/cordova-common/node_modules/shelljs/src/dirs.js
deleted file mode 100644
index 58fae8b..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/dirs.js
+++ /dev/null
@@ -1,191 +0,0 @@
-var common = require('./common');
-var _cd = require('./cd');
-var path = require('path');
-
-// Pushd/popd/dirs internals
-var _dirStack = [];
-
-function _isStackIndex(index) {
- return (/^[\-+]\d+$/).test(index);
-}
-
-function _parseStackIndex(index) {
- if (_isStackIndex(index)) {
- if (Math.abs(index) < _dirStack.length + 1) { // +1 for pwd
- return (/^-/).test(index) ? Number(index) - 1 : Number(index);
- } else {
- common.error(index + ': directory stack index out of range');
- }
- } else {
- common.error(index + ': invalid number');
- }
-}
-
-function _actualDirStack() {
- return [process.cwd()].concat(_dirStack);
-}
-
-//@
-//@ ### pushd([options,] [dir | '-N' | '+N'])
-//@
-//@ Available options:
-//@
-//@ + `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
-//@
-//@ Arguments:
-//@
-//@ + `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
-//@ + `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
-//@ + `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ // process.cwd() === '/usr'
-//@ pushd('/etc'); // Returns /etc /usr
-//@ pushd('+1'); // Returns /usr /etc
-//@ ```
-//@
-//@ Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
-function _pushd(options, dir) {
- if (_isStackIndex(options)) {
- dir = options;
- options = '';
- }
-
- options = common.parseOptions(options, {
- 'n' : 'no-cd'
- });
-
- var dirs = _actualDirStack();
-
- if (dir === '+0') {
- return dirs; // +0 is a noop
- } else if (!dir) {
- if (dirs.length > 1) {
- dirs = dirs.splice(1, 1).concat(dirs);
- } else {
- return common.error('no other directory');
- }
- } else if (_isStackIndex(dir)) {
- var n = _parseStackIndex(dir);
- dirs = dirs.slice(n).concat(dirs.slice(0, n));
- } else {
- if (options['no-cd']) {
- dirs.splice(1, 0, dir);
- } else {
- dirs.unshift(dir);
- }
- }
-
- if (options['no-cd']) {
- dirs = dirs.slice(1);
- } else {
- dir = path.resolve(dirs.shift());
- _cd('', dir);
- }
-
- _dirStack = dirs;
- return _dirs('');
-}
-exports.pushd = _pushd;
-
-//@
-//@ ### popd([options,] ['-N' | '+N'])
-//@
-//@ Available options:
-//@
-//@ + `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
-//@
-//@ Arguments:
-//@
-//@ + `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
-//@ + `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ echo(process.cwd()); // '/usr'
-//@ pushd('/etc'); // '/etc /usr'
-//@ echo(process.cwd()); // '/etc'
-//@ popd(); // '/usr'
-//@ echo(process.cwd()); // '/usr'
-//@ ```
-//@
-//@ When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
-function _popd(options, index) {
- if (_isStackIndex(options)) {
- index = options;
- options = '';
- }
-
- options = common.parseOptions(options, {
- 'n' : 'no-cd'
- });
-
- if (!_dirStack.length) {
- return common.error('directory stack empty');
- }
-
- index = _parseStackIndex(index || '+0');
-
- if (options['no-cd'] || index > 0 || _dirStack.length + index === 0) {
- index = index > 0 ? index - 1 : index;
- _dirStack.splice(index, 1);
- } else {
- var dir = path.resolve(_dirStack.shift());
- _cd('', dir);
- }
-
- return _dirs('');
-}
-exports.popd = _popd;
-
-//@
-//@ ### dirs([options | '+N' | '-N'])
-//@
-//@ Available options:
-//@
-//@ + `-c`: Clears the directory stack by deleting all of the elements.
-//@
-//@ Arguments:
-//@
-//@ + `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
-//@ + `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
-//@
-//@ Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
-//@
-//@ See also: pushd, popd
-function _dirs(options, index) {
- if (_isStackIndex(options)) {
- index = options;
- options = '';
- }
-
- options = common.parseOptions(options, {
- 'c' : 'clear'
- });
-
- if (options['clear']) {
- _dirStack = [];
- return _dirStack;
- }
-
- var stack = _actualDirStack();
-
- if (index) {
- index = _parseStackIndex(index);
-
- if (index < 0) {
- index = stack.length + index;
- }
-
- common.log(stack[index]);
- return stack[index];
- }
-
- common.log(stack.join(' '));
-
- return stack;
-}
-exports.dirs = _dirs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/echo.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/echo.js b/node_modules/cordova-common/node_modules/shelljs/src/echo.js
deleted file mode 100644
index 760ea84..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/echo.js
+++ /dev/null
@@ -1,20 +0,0 @@
-var common = require('./common');
-
-//@
-//@ ### echo(string [,string ...])
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ echo('hello world');
-//@ var str = echo('hello world');
-//@ ```
-//@
-//@ Prints string to stdout, and returns string with additional utility methods
-//@ like `.to()`.
-function _echo() {
- var messages = [].slice.call(arguments, 0);
- console.log.apply(this, messages);
- return common.ShellString(messages.join(' '));
-}
-module.exports = _echo;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/error.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/error.js b/node_modules/cordova-common/node_modules/shelljs/src/error.js
deleted file mode 100644
index cca3efb..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/error.js
+++ /dev/null
@@ -1,10 +0,0 @@
-var common = require('./common');
-
-//@
-//@ ### error()
-//@ Tests if error occurred in the last command. Returns `null` if no error occurred,
-//@ otherwise returns string explaining the error
-function error() {
- return common.state.error;
-};
-module.exports = error;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/exec.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/exec.js b/node_modules/cordova-common/node_modules/shelljs/src/exec.js
deleted file mode 100644
index d259a9f..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/exec.js
+++ /dev/null
@@ -1,216 +0,0 @@
-var common = require('./common');
-var _tempDir = require('./tempdir');
-var _pwd = require('./pwd');
-var path = require('path');
-var fs = require('fs');
-var child = require('child_process');
-
-// Hack to run child_process.exec() synchronously (sync avoids callback hell)
-// Uses a custom wait loop that checks for a flag file, created when the child process is done.
-// (Can't do a wait loop that checks for internal Node variables/messages as
-// Node is single-threaded; callbacks and other internal state changes are done in the
-// event loop).
-function execSync(cmd, opts) {
- var tempDir = _tempDir();
- var stdoutFile = path.resolve(tempDir+'/'+common.randomFileName()),
- codeFile = path.resolve(tempDir+'/'+common.randomFileName()),
- scriptFile = path.resolve(tempDir+'/'+common.randomFileName()),
- sleepFile = path.resolve(tempDir+'/'+common.randomFileName());
-
- var options = common.extend({
- silent: common.config.silent
- }, opts);
-
- var previousStdoutContent = '';
- // Echoes stdout changes from running process, if not silent
- function updateStdout() {
- if (options.silent || !fs.existsSync(stdoutFile))
- return;
-
- var stdoutContent = fs.readFileSync(stdoutFile, 'utf8');
- // No changes since last time?
- if (stdoutContent.length <= previousStdoutContent.length)
- return;
-
- process.stdout.write(stdoutContent.substr(previousStdoutContent.length));
- previousStdoutContent = stdoutContent;
- }
-
- function escape(str) {
- return (str+'').replace(/([\\"'])/g, "\\$1").replace(/\0/g, "\\0");
- }
-
- if (fs.existsSync(scriptFile)) common.unlinkSync(scriptFile);
- if (fs.existsSync(stdoutFile)) common.unlinkSync(stdoutFile);
- if (fs.existsSync(codeFile)) common.unlinkSync(codeFile);
-
- var execCommand = '"'+process.execPath+'" '+scriptFile;
- var execOptions = {
- env: process.env,
- cwd: _pwd(),
- maxBuffer: 20*1024*1024
- };
-
- if (typeof child.execSync === 'function') {
- var script = [
- "var child = require('child_process')",
- " , fs = require('fs');",
- "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
- " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
- "});",
- "var stdoutStream = fs.createWriteStream('"+escape(stdoutFile)+"');",
- "childProcess.stdout.pipe(stdoutStream, {end: false});",
- "childProcess.stderr.pipe(stdoutStream, {end: false});",
- "childProcess.stdout.pipe(process.stdout);",
- "childProcess.stderr.pipe(process.stderr);",
- "var stdoutEnded = false, stderrEnded = false;",
- "function tryClosing(){ if(stdoutEnded && stderrEnded){ stdoutStream.end(); } }",
- "childProcess.stdout.on('end', function(){ stdoutEnded = true; tryClosing(); });",
- "childProcess.stderr.on('end', function(){ stderrEnded = true; tryClosing(); });"
- ].join('\n');
-
- fs.writeFileSync(scriptFile, script);
-
- if (options.silent) {
- execOptions.stdio = 'ignore';
- } else {
- execOptions.stdio = [0, 1, 2];
- }
-
- // Welcome to the future
- child.execSync(execCommand, execOptions);
- } else {
- cmd += ' > '+stdoutFile+' 2>&1'; // works on both win/unix
-
- var script = [
- "var child = require('child_process')",
- " , fs = require('fs');",
- "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
- " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
- "});"
- ].join('\n');
-
- fs.writeFileSync(scriptFile, script);
-
- child.exec(execCommand, execOptions);
-
- // The wait loop
- // sleepFile is used as a dummy I/O op to mitigate unnecessary CPU usage
- // (tried many I/O sync ops, writeFileSync() seems to be only one that is effective in reducing
- // CPU usage, though apparently not so much on Windows)
- while (!fs.existsSync(codeFile)) { updateStdout(); fs.writeFileSync(sleepFile, 'a'); }
- while (!fs.existsSync(stdoutFile)) { updateStdout(); fs.writeFileSync(sleepFile, 'a'); }
- }
-
- // At this point codeFile exists, but it's not necessarily flushed yet.
- // Keep reading it until it is.
- var code = parseInt('', 10);
- while (isNaN(code)) {
- code = parseInt(fs.readFileSync(codeFile, 'utf8'), 10);
- }
-
- var stdout = fs.readFileSync(stdoutFile, 'utf8');
-
- // No biggie if we can't erase the files now -- they're in a temp dir anyway
- try { common.unlinkSync(scriptFile); } catch(e) {}
- try { common.unlinkSync(stdoutFile); } catch(e) {}
- try { common.unlinkSync(codeFile); } catch(e) {}
- try { common.unlinkSync(sleepFile); } catch(e) {}
-
- // some shell return codes are defined as errors, per http://tldp.org/LDP/abs/html/exitcodes.html
- if (code === 1 || code === 2 || code >= 126) {
- common.error('', true); // unix/shell doesn't really give an error message after non-zero exit codes
- }
- // True if successful, false if not
- var obj = {
- code: code,
- output: stdout
- };
- return obj;
-} // execSync()
-
-// Wrapper around exec() to enable echoing output to console in real time
-function execAsync(cmd, opts, callback) {
- var output = '';
-
- var options = common.extend({
- silent: common.config.silent
- }, opts);
-
- var c = child.exec(cmd, {env: process.env, maxBuffer: 20*1024*1024}, function(err) {
- if (callback)
- callback(err ? err.code : 0, output);
- });
-
- c.stdout.on('data', function(data) {
- output += data;
- if (!options.silent)
- process.stdout.write(data);
- });
-
- c.stderr.on('data', function(data) {
- output += data;
- if (!options.silent)
- process.stdout.write(data);
- });
-
- return c;
-}
-
-//@
-//@ ### exec(command [, options] [, callback])
-//@ Available options (all `false` by default):
-//@
-//@ + `async`: Asynchronous execution. Defaults to true if a callback is provided.
-//@ + `silent`: Do not echo program output to console.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var version = exec('node --version', {silent:true}).output;
-//@
-//@ var child = exec('some_long_running_process', {async:true});
-//@ child.stdout.on('data', function(data) {
-//@ /* ... do something with data ... */
-//@ });
-//@
-//@ exec('some_long_running_process', function(code, output) {
-//@ console.log('Exit code:', code);
-//@ console.log('Program output:', output);
-//@ });
-//@ ```
-//@
-//@ Executes the given `command` _synchronously_, unless otherwise specified.
-//@ When in synchronous mode returns the object `{ code:..., output:... }`, containing the program's
-//@ `output` (stdout + stderr) and its exit `code`. Otherwise returns the child process object, and
-//@ the `callback` gets the arguments `(code, output)`.
-//@
-//@ **Note:** For long-lived processes, it's best to run `exec()` asynchronously as
-//@ the current synchronous implementation uses a lot of CPU. This should be getting
-//@ fixed soon.
-function _exec(command, options, callback) {
- if (!command)
- common.error('must specify command');
-
- // Callback is defined instead of options.
- if (typeof options === 'function') {
- callback = options;
- options = { async: true };
- }
-
- // Callback is defined with options.
- if (typeof options === 'object' && typeof callback === 'function') {
- options.async = true;
- }
-
- options = common.extend({
- silent: common.config.silent,
- async: false
- }, options);
-
- if (options.async)
- return execAsync(command, options, callback);
- else
- return execSync(command, options);
-}
-module.exports = _exec;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/find.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/find.js b/node_modules/cordova-common/node_modules/shelljs/src/find.js
deleted file mode 100644
index d9eeec2..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/find.js
+++ /dev/null
@@ -1,51 +0,0 @@
-var fs = require('fs');
-var common = require('./common');
-var _ls = require('./ls');
-
-//@
-//@ ### find(path [,path ...])
-//@ ### find(path_array)
-//@ Examples:
-//@
-//@ ```javascript
-//@ find('src', 'lib');
-//@ find(['src', 'lib']); // same as above
-//@ find('.').filter(function(file) { return file.match(/\.js$/); });
-//@ ```
-//@
-//@ Returns array of all files (however deep) in the given paths.
-//@
-//@ The main difference from `ls('-R', path)` is that the resulting file names
-//@ include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
-function _find(options, paths) {
- if (!paths)
- common.error('no path specified');
- else if (typeof paths === 'object')
- paths = paths; // assume array
- else if (typeof paths === 'string')
- paths = [].slice.call(arguments, 1);
-
- var list = [];
-
- function pushFile(file) {
- if (common.platform === 'win')
- file = file.replace(/\\/g, '/');
- list.push(file);
- }
-
- // why not simply do ls('-R', paths)? because the output wouldn't give the base dirs
- // to get the base dir in the output, we need instead ls('-R', 'dir/*') for every directory
-
- paths.forEach(function(file) {
- pushFile(file);
-
- if (fs.statSync(file).isDirectory()) {
- _ls('-RA', file+'/*').forEach(function(subfile) {
- pushFile(subfile);
- });
- }
- });
-
- return list;
-}
-module.exports = _find;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/grep.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/grep.js b/node_modules/cordova-common/node_modules/shelljs/src/grep.js
deleted file mode 100644
index 00c7d6a..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/grep.js
+++ /dev/null
@@ -1,52 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### grep([options ,] regex_filter, file [, file ...])
-//@ ### grep([options ,] regex_filter, file_array)
-//@ Available options:
-//@
-//@ + `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ grep('-v', 'GLOBAL_VARIABLE', '*.js');
-//@ grep('GLOBAL_VARIABLE', '*.js');
-//@ ```
-//@
-//@ Reads input string from given files and returns a string containing all lines of the
-//@ file that match the given `regex_filter`. Wildcard `*` accepted.
-function _grep(options, regex, files) {
- options = common.parseOptions(options, {
- 'v': 'inverse'
- });
-
- if (!files)
- common.error('no paths given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 2);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- var grep = '';
- files.forEach(function(file) {
- if (!fs.existsSync(file)) {
- common.error('no such file or directory: ' + file, true);
- return;
- }
-
- var contents = fs.readFileSync(file, 'utf8'),
- lines = contents.split(/\r*\n/);
- lines.forEach(function(line) {
- var matched = line.match(regex);
- if ((options.inverse && !matched) || (!options.inverse && matched))
- grep += line + '\n';
- });
- });
-
- return common.ShellString(grep);
-}
-module.exports = _grep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/cordova-common/node_modules/shelljs/src/ln.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/shelljs/src/ln.js b/node_modules/cordova-common/node_modules/shelljs/src/ln.js
deleted file mode 100644
index a7b9701..0000000
--- a/node_modules/cordova-common/node_modules/shelljs/src/ln.js
+++ /dev/null
@@ -1,53 +0,0 @@
-var fs = require('fs');
-var path = require('path');
-var common = require('./common');
-var os = require('os');
-
-//@
-//@ ### ln(options, source, dest)
-//@ ### ln(source, dest)
-//@ Available options:
-//@
-//@ + `s`: symlink
-//@ + `f`: force
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ ln('file', 'newlink');
-//@ ln('-sf', 'file', 'existing');
-//@ ```
-//@
-//@ Links source to dest. Use -f to force the link, should dest already exist.
-function _ln(options, source, dest) {
- options = common.parseOptions(options, {
- 's': 'symlink',
- 'f': 'force'
- });
-
- if (!source || !dest) {
- common.error('Missing <source> and/or <dest>');
- }
-
- source = path.resolve(process.cwd(), String(source));
- dest = path.resolve(process.cwd(), String(dest));
-
- if (!fs.existsSync(source)) {
- common.error('Source file does not exist', true);
- }
-
- if (fs.existsSync(dest)) {
- if (!options.force) {
- common.error('Destination file exists', true);
- }
-
- fs.unlinkSync(dest);
- }
-
- if (options.symlink) {
- fs.symlinkSync(source, dest, os.platform() === "win32" ? "junction" : null);
- } else {
- fs.linkSync(source, dest, os.platform() === "win32" ? "junction" : null);
- }
-}
-module.exports = _ln;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[42/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/range.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/range.js b/node_modules/lodash-node/compat/arrays/range.js
new file mode 100644
index 0000000..5ea7f49
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/range.js
@@ -0,0 +1,69 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Native method shortcuts */
+var ceil = Math.ceil;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Creates an array of numbers (positive and/or negative) progressing from
+ * `start` up to but not including `end`. If `start` is less than `stop` a
+ * zero-length range is created unless a negative `step` is specified.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {number} [start=0] The start of the range.
+ * @param {number} end The end of the range.
+ * @param {number} [step=1] The value to increment or decrement by.
+ * @returns {Array} Returns a new range array.
+ * @example
+ *
+ * _.range(4);
+ * // => [0, 1, 2, 3]
+ *
+ * _.range(1, 5);
+ * // => [1, 2, 3, 4]
+ *
+ * _.range(0, 20, 5);
+ * // => [0, 5, 10, 15]
+ *
+ * _.range(0, -4, -1);
+ * // => [0, -1, -2, -3]
+ *
+ * _.range(1, 4, 0);
+ * // => [1, 1, 1]
+ *
+ * _.range(0);
+ * // => []
+ */
+function range(start, end, step) {
+ start = +start || 0;
+ step = typeof step == 'number' ? step : (+step || 1);
+
+ if (end == null) {
+ end = start;
+ start = 0;
+ }
+ // use `Array(length)` so engines like Chakra and V8 avoid slower modes
+ // http://youtu.be/XAqIpGU8ZZk#t=17m25s
+ var index = -1,
+ length = nativeMax(0, ceil((end - start) / (step || 1))),
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = start;
+ start += step;
+ }
+ return result;
+}
+
+module.exports = range;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/remove.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/remove.js b/node_modules/lodash-node/compat/arrays/remove.js
new file mode 100644
index 0000000..7c0f8f0
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/remove.js
@@ -0,0 +1,71 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var splice = arrayRef.splice;
+
+/**
+ * Removes all elements from an array that the callback returns truey for
+ * and returns an array of removed elements. The callback is bound to `thisArg`
+ * and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to modify.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of removed elements.
+ * @example
+ *
+ * var array = [1, 2, 3, 4, 5, 6];
+ * var evens = _.remove(array, function(num) { return num % 2 == 0; });
+ *
+ * console.log(array);
+ * // => [1, 3, 5]
+ *
+ * console.log(evens);
+ * // => [2, 4, 6]
+ */
+function remove(array, callback, thisArg) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length) {
+ var value = array[index];
+ if (callback(value, index, array)) {
+ result.push(value);
+ splice.call(array, index--, 1);
+ length--;
+ }
+ }
+ return result;
+}
+
+module.exports = remove;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/rest.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/rest.js b/node_modules/lodash-node/compat/arrays/rest.js
new file mode 100644
index 0000000..d778fb6
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/rest.js
@@ -0,0 +1,83 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * The opposite of `_.initial` this method gets all but the first element or
+ * first `n` elements of an array. If a callback function is provided elements
+ * at the beginning of the array are excluded from the result as long as the
+ * callback returns truey. The callback is bound to `thisArg` and invoked
+ * with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias drop, tail
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.rest([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.rest([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.rest([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [3]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.pluck(_.rest(characters, 'blocked'), 'name');
+ * // => ['fred', 'pebbles']
+ *
+ * // using "_.where" callback shorthand
+ * _.rest(characters, { 'employer': 'slate' });
+ * // => [{ 'name': 'pebbles', 'blocked': true, 'employer': 'na' }]
+ */
+function rest(array, callback, thisArg) {
+ if (typeof callback != 'number' && callback != null) {
+ var n = 0,
+ index = -1,
+ length = array ? array.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : nativeMax(0, callback);
+ }
+ return slice(array, n);
+}
+
+module.exports = rest;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/sortedIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/sortedIndex.js b/node_modules/lodash-node/compat/arrays/sortedIndex.js
new file mode 100644
index 0000000..1bcb5b5
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/sortedIndex.js
@@ -0,0 +1,77 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ identity = require('../utilities/identity');
+
+/**
+ * Uses a binary search to determine the smallest index at which a value
+ * should be inserted into a given sorted array in order to maintain the sort
+ * order of the array. If a callback is provided it will be executed for
+ * `value` and each element of `array` to compute their sort ranking. The
+ * callback is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedIndex([20, 30, 50], 40);
+ * // => 2
+ *
+ * // using "_.pluck" callback shorthand
+ * _.sortedIndex([{ 'x': 20 }, { 'x': 30 }, { 'x': 50 }], { 'x': 40 }, 'x');
+ * // => 2
+ *
+ * var dict = {
+ * 'wordToNumber': { 'twenty': 20, 'thirty': 30, 'fourty': 40, 'fifty': 50 }
+ * };
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return dict.wordToNumber[word];
+ * });
+ * // => 2
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return this.wordToNumber[word];
+ * }, dict);
+ * // => 2
+ */
+function sortedIndex(array, value, callback, thisArg) {
+ var low = 0,
+ high = array ? array.length : low;
+
+ // explicitly reference `identity` for better inlining in Firefox
+ callback = callback ? createCallback(callback, thisArg, 1) : identity;
+ value = callback(value);
+
+ while (low < high) {
+ var mid = (low + high) >>> 1;
+ (callback(array[mid]) < value)
+ ? low = mid + 1
+ : high = mid;
+ }
+ return low;
+}
+
+module.exports = sortedIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/union.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/union.js b/node_modules/lodash-node/compat/arrays/union.js
new file mode 100644
index 0000000..854f7b1
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/union.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ baseUniq = require('../internals/baseUniq');
+
+/**
+ * Creates an array of unique values, in order, of the provided arrays using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of combined values.
+ * @example
+ *
+ * _.union([1, 2, 3], [5, 2, 1, 4], [2, 1]);
+ * // => [1, 2, 3, 5, 4]
+ */
+function union() {
+ return baseUniq(baseFlatten(arguments, true, true));
+}
+
+module.exports = union;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/uniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/uniq.js b/node_modules/lodash-node/compat/arrays/uniq.js
new file mode 100644
index 0000000..50df292
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/uniq.js
@@ -0,0 +1,69 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseUniq = require('../internals/baseUniq'),
+ createCallback = require('../functions/createCallback');
+
+/**
+ * Creates a duplicate-value-free version of an array using strict equality
+ * for comparisons, i.e. `===`. If the array is sorted, providing
+ * `true` for `isSorted` will use a faster algorithm. If a callback is provided
+ * each element of `array` is passed through the callback before uniqueness
+ * is computed. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias unique
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {boolean} [isSorted=false] A flag to indicate that `array` is sorted.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a duplicate-value-free array.
+ * @example
+ *
+ * _.uniq([1, 2, 1, 3, 1]);
+ * // => [1, 2, 3]
+ *
+ * _.uniq([1, 1, 2, 2, 3], true);
+ * // => [1, 2, 3]
+ *
+ * _.uniq(['A', 'b', 'C', 'a', 'B', 'c'], function(letter) { return letter.toLowerCase(); });
+ * // => ['A', 'b', 'C']
+ *
+ * _.uniq([1, 2.5, 3, 1.5, 2, 3.5], function(num) { return this.floor(num); }, Math);
+ * // => [1, 2.5, 3]
+ *
+ * // using "_.pluck" callback shorthand
+ * _.uniq([{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+function uniq(array, isSorted, callback, thisArg) {
+ // juggle arguments
+ if (typeof isSorted != 'boolean' && isSorted != null) {
+ thisArg = callback;
+ callback = (typeof isSorted != 'function' && thisArg && thisArg[isSorted] === array) ? null : isSorted;
+ isSorted = false;
+ }
+ if (callback != null) {
+ callback = createCallback(callback, thisArg, 3);
+ }
+ return baseUniq(array, isSorted, callback);
+}
+
+module.exports = uniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/without.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/without.js b/node_modules/lodash-node/compat/arrays/without.js
new file mode 100644
index 0000000..5fda56c
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/without.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates an array excluding all provided values using strict equality for
+ * comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to filter.
+ * @param {...*} [value] The values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.without([1, 2, 1, 0, 3, 1, 4], 0, 1);
+ * // => [2, 3, 4]
+ */
+function without(array) {
+ return baseDifference(array, slice(arguments, 1));
+}
+
+module.exports = without;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/xor.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/xor.js b/node_modules/lodash-node/compat/arrays/xor.js
new file mode 100644
index 0000000..1a444a1
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/xor.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseUniq = require('../internals/baseUniq'),
+ isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates an array that is the symmetric difference of the provided arrays.
+ * See http://en.wikipedia.org/wiki/Symmetric_difference.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {...Array} [array] The arrays to inspect.
+ * @returns {Array} Returns an array of values.
+ * @example
+ *
+ * _.xor([1, 2, 3], [5, 2, 1, 4]);
+ * // => [3, 5, 4]
+ *
+ * _.xor([1, 2, 5], [2, 3, 5], [3, 4, 5]);
+ * // => [1, 4, 5]
+ */
+function xor() {
+ var index = -1,
+ length = arguments.length;
+
+ while (++index < length) {
+ var array = arguments[index];
+ if (isArray(array) || isArguments(array)) {
+ var result = result
+ ? baseUniq(baseDifference(result, array).concat(baseDifference(array, result)))
+ : array;
+ }
+ }
+ return result || [];
+}
+
+module.exports = xor;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/zip.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/zip.js b/node_modules/lodash-node/compat/arrays/zip.js
new file mode 100644
index 0000000..7d3d6e6
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/zip.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var max = require('../collections/max'),
+ pluck = require('../collections/pluck');
+
+/**
+ * Creates an array of grouped elements, the first of which contains the first
+ * elements of the given arrays, the second of which contains the second
+ * elements of the given arrays, and so on.
+ *
+ * @static
+ * @memberOf _
+ * @alias unzip
+ * @category Arrays
+ * @param {...Array} [array] Arrays to process.
+ * @returns {Array} Returns a new array of grouped elements.
+ * @example
+ *
+ * _.zip(['fred', 'barney'], [30, 40], [true, false]);
+ * // => [['fred', 30, true], ['barney', 40, false]]
+ */
+function zip() {
+ var array = arguments.length > 1 ? arguments : arguments[0],
+ index = -1,
+ length = array ? max(pluck(array, 'length')) : 0,
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = pluck(array, index);
+ }
+ return result;
+}
+
+module.exports = zip;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/arrays/zipObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/arrays/zipObject.js b/node_modules/lodash-node/compat/arrays/zipObject.js
new file mode 100644
index 0000000..6f901ef
--- /dev/null
+++ b/node_modules/lodash-node/compat/arrays/zipObject.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArray = require('../objects/isArray');
+
+/**
+ * Creates an object composed from arrays of `keys` and `values`. Provide
+ * either a single two dimensional array, i.e. `[[key1, value1], [key2, value2]]`
+ * or two arrays, one of `keys` and one of corresponding `values`.
+ *
+ * @static
+ * @memberOf _
+ * @alias object
+ * @category Arrays
+ * @param {Array} keys The array of keys.
+ * @param {Array} [values=[]] The array of values.
+ * @returns {Object} Returns an object composed of the given keys and
+ * corresponding values.
+ * @example
+ *
+ * _.zipObject(['fred', 'barney'], [30, 40]);
+ * // => { 'fred': 30, 'barney': 40 }
+ */
+function zipObject(keys, values) {
+ var index = -1,
+ length = keys ? keys.length : 0,
+ result = {};
+
+ if (!values && length && !isArray(keys[0])) {
+ values = [];
+ }
+ while (++index < length) {
+ var key = keys[index];
+ if (values) {
+ result[key] = values[index];
+ } else if (key) {
+ result[key[0]] = key[1];
+ }
+ }
+ return result;
+}
+
+module.exports = zipObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/chaining.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/chaining.js b/node_modules/lodash-node/compat/chaining.js
new file mode 100644
index 0000000..6cde453
--- /dev/null
+++ b/node_modules/lodash-node/compat/chaining.js
@@ -0,0 +1,17 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'chain': require('./chaining/chain'),
+ 'tap': require('./chaining/tap'),
+ 'value': require('./chaining/wrapperValueOf'),
+ 'wrapperChain': require('./chaining/wrapperChain'),
+ 'wrapperToString': require('./chaining/wrapperToString'),
+ 'wrapperValueOf': require('./chaining/wrapperValueOf')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/chaining/chain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/chaining/chain.js b/node_modules/lodash-node/compat/chaining/chain.js
new file mode 100644
index 0000000..d613c55
--- /dev/null
+++ b/node_modules/lodash-node/compat/chaining/chain.js
@@ -0,0 +1,41 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var lodashWrapper = require('../internals/lodashWrapper');
+
+/**
+ * Creates a `lodash` object that wraps the given value with explicit
+ * method chaining enabled.
+ *
+ * @static
+ * @memberOf _
+ * @category Chaining
+ * @param {*} value The value to wrap.
+ * @returns {Object} Returns the wrapper object.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'pebbles', 'age': 1 }
+ * ];
+ *
+ * var youngest = _.chain(characters)
+ * .sortBy('age')
+ * .map(function(chr) { return chr.name + ' is ' + chr.age; })
+ * .first()
+ * .value();
+ * // => 'pebbles is 1'
+ */
+function chain(value) {
+ value = new lodashWrapper(value);
+ value.__chain__ = true;
+ return value;
+}
+
+module.exports = chain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/chaining/tap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/chaining/tap.js b/node_modules/lodash-node/compat/chaining/tap.js
new file mode 100644
index 0000000..0be66d3
--- /dev/null
+++ b/node_modules/lodash-node/compat/chaining/tap.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Invokes `interceptor` with the `value` as the first argument and then
+ * returns `value`. The purpose of this method is to "tap into" a method
+ * chain in order to perform operations on intermediate results within
+ * the chain.
+ *
+ * @static
+ * @memberOf _
+ * @category Chaining
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * _([1, 2, 3, 4])
+ * .tap(function(array) { array.pop(); })
+ * .reverse()
+ * .value();
+ * // => [3, 2, 1]
+ */
+function tap(value, interceptor) {
+ interceptor(value);
+ return value;
+}
+
+module.exports = tap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/chaining/wrapperChain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/chaining/wrapperChain.js b/node_modules/lodash-node/compat/chaining/wrapperChain.js
new file mode 100644
index 0000000..e6bf9a8
--- /dev/null
+++ b/node_modules/lodash-node/compat/chaining/wrapperChain.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Enables explicit method chaining on the wrapper object.
+ *
+ * @name chain
+ * @memberOf _
+ * @category Chaining
+ * @returns {*} Returns the wrapper object.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // without explicit chaining
+ * _(characters).first();
+ * // => { 'name': 'barney', 'age': 36 }
+ *
+ * // with explicit chaining
+ * _(characters).chain()
+ * .first()
+ * .pick('age')
+ * .value();
+ * // => { 'age': 36 }
+ */
+function wrapperChain() {
+ this.__chain__ = true;
+ return this;
+}
+
+module.exports = wrapperChain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/chaining/wrapperToString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/chaining/wrapperToString.js b/node_modules/lodash-node/compat/chaining/wrapperToString.js
new file mode 100644
index 0000000..393d86d
--- /dev/null
+++ b/node_modules/lodash-node/compat/chaining/wrapperToString.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Produces the `toString` result of the wrapped value.
+ *
+ * @name toString
+ * @memberOf _
+ * @category Chaining
+ * @returns {string} Returns the string result.
+ * @example
+ *
+ * _([1, 2, 3]).toString();
+ * // => '1,2,3'
+ */
+function wrapperToString() {
+ return String(this.__wrapped__);
+}
+
+module.exports = wrapperToString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/chaining/wrapperValueOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/chaining/wrapperValueOf.js b/node_modules/lodash-node/compat/chaining/wrapperValueOf.js
new file mode 100644
index 0000000..96bd2f4
--- /dev/null
+++ b/node_modules/lodash-node/compat/chaining/wrapperValueOf.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var support = require('../support');
+
+/**
+ * Extracts the wrapped value.
+ *
+ * @name valueOf
+ * @memberOf _
+ * @alias value
+ * @category Chaining
+ * @returns {*} Returns the wrapped value.
+ * @example
+ *
+ * _([1, 2, 3]).valueOf();
+ * // => [1, 2, 3]
+ */
+function wrapperValueOf() {
+ return this.__wrapped__;
+}
+
+module.exports = wrapperValueOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections.js b/node_modules/lodash-node/compat/collections.js
new file mode 100644
index 0000000..5d2fc99
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'all': require('./collections/every'),
+ 'any': require('./collections/some'),
+ 'at': require('./collections/at'),
+ 'collect': require('./collections/map'),
+ 'contains': require('./collections/contains'),
+ 'countBy': require('./collections/countBy'),
+ 'detect': require('./collections/find'),
+ 'each': require('./collections/forEach'),
+ 'eachRight': require('./collections/forEachRight'),
+ 'every': require('./collections/every'),
+ 'filter': require('./collections/filter'),
+ 'find': require('./collections/find'),
+ 'findLast': require('./collections/findLast'),
+ 'findWhere': require('./collections/find'),
+ 'foldl': require('./collections/reduce'),
+ 'foldr': require('./collections/reduceRight'),
+ 'forEach': require('./collections/forEach'),
+ 'forEachRight': require('./collections/forEachRight'),
+ 'groupBy': require('./collections/groupBy'),
+ 'include': require('./collections/contains'),
+ 'indexBy': require('./collections/indexBy'),
+ 'inject': require('./collections/reduce'),
+ 'invoke': require('./collections/invoke'),
+ 'map': require('./collections/map'),
+ 'max': require('./collections/max'),
+ 'min': require('./collections/min'),
+ 'pluck': require('./collections/pluck'),
+ 'reduce': require('./collections/reduce'),
+ 'reduceRight': require('./collections/reduceRight'),
+ 'reject': require('./collections/reject'),
+ 'sample': require('./collections/sample'),
+ 'select': require('./collections/filter'),
+ 'shuffle': require('./collections/shuffle'),
+ 'size': require('./collections/size'),
+ 'some': require('./collections/some'),
+ 'sortBy': require('./collections/sortBy'),
+ 'toArray': require('./collections/toArray'),
+ 'where': require('./collections/where')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/at.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/at.js b/node_modules/lodash-node/compat/collections/at.js
new file mode 100644
index 0000000..a994bb7
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/at.js
@@ -0,0 +1,50 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ isString = require('../objects/isString'),
+ support = require('../support');
+
+/**
+ * Creates an array of elements from the specified indexes, or keys, of the
+ * `collection`. Indexes may be specified as individual arguments or as arrays
+ * of indexes.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {...(number|number[]|string|string[])} [index] The indexes of `collection`
+ * to retrieve, specified as individual indexes or arrays of indexes.
+ * @returns {Array} Returns a new array of elements corresponding to the
+ * provided indexes.
+ * @example
+ *
+ * _.at(['a', 'b', 'c', 'd', 'e'], [0, 2, 4]);
+ * // => ['a', 'c', 'e']
+ *
+ * _.at(['fred', 'barney', 'pebbles'], 0, 2);
+ * // => ['fred', 'pebbles']
+ */
+function at(collection) {
+ var args = arguments,
+ index = -1,
+ props = baseFlatten(args, true, false, 1),
+ length = (args[2] && args[2][args[1]] === collection) ? 1 : props.length,
+ result = Array(length);
+
+ if (support.unindexedChars && isString(collection)) {
+ collection = collection.split('');
+ }
+ while(++index < length) {
+ result[index] = collection[props[index]];
+ }
+ return result;
+}
+
+module.exports = at;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/contains.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/contains.js b/node_modules/lodash-node/compat/collections/contains.js
new file mode 100644
index 0000000..69e552d
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/contains.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ baseIndexOf = require('../internals/baseIndexOf'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Checks if a given value is present in a collection using strict equality
+ * for comparisons, i.e. `===`. If `fromIndex` is negative, it is used as the
+ * offset from the end of the collection.
+ *
+ * @static
+ * @memberOf _
+ * @alias include
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {*} target The value to check for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {boolean} Returns `true` if the `target` element is found, else `false`.
+ * @example
+ *
+ * _.contains([1, 2, 3], 1);
+ * // => true
+ *
+ * _.contains([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.contains({ 'name': 'fred', 'age': 40 }, 'fred');
+ * // => true
+ *
+ * _.contains('pebbles', 'eb');
+ * // => true
+ */
+function contains(collection, target, fromIndex) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = collection ? collection.length : 0,
+ result = false;
+
+ fromIndex = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex) || 0;
+ if (isArray(collection)) {
+ result = indexOf(collection, target, fromIndex) > -1;
+ } else if (typeof length == 'number') {
+ result = (isString(collection) ? collection.indexOf(target, fromIndex) : indexOf(collection, target, fromIndex)) > -1;
+ } else {
+ baseEach(collection, function(value) {
+ if (++index >= fromIndex) {
+ return !(result = value === target);
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = contains;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/countBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/countBy.js b/node_modules/lodash-node/compat/collections/countBy.js
new file mode 100644
index 0000000..ece9ece
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/countBy.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` through the callback. The corresponding value
+ * of each key is the number of times the key was returned by the callback.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+var countBy = createAggregator(function(result, value, key) {
+ (hasOwnProperty.call(result, key) ? result[key]++ : result[key] = 1);
+});
+
+module.exports = countBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/every.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/every.js b/node_modules/lodash-node/compat/collections/every.js
new file mode 100644
index 0000000..633489c
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/every.js
@@ -0,0 +1,75 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Checks if the given callback returns truey value for **all** elements of
+ * a collection. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if all elements passed the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes']);
+ * // => false
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.every(characters, 'age');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.every(characters, { 'age': 36 });
+ * // => false
+ */
+function every(collection, callback, thisArg) {
+ var result = true;
+ callback = createCallback(callback, thisArg, 3);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ if (!(result = !!callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ baseEach(collection, function(value, index, collection) {
+ return (result = !!callback(value, index, collection));
+ });
+ }
+ return result;
+}
+
+module.exports = every;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/filter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/filter.js b/node_modules/lodash-node/compat/collections/filter.js
new file mode 100644
index 0000000..30c351e
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/filter.js
@@ -0,0 +1,77 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Iterates over elements of a collection, returning an array of all elements
+ * the callback returns truey for. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias select
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that passed the callback check.
+ * @example
+ *
+ * var evens = _.filter([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [2, 4, 6]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.filter(characters, 'blocked');
+ * // => [{ 'name': 'fred', 'age': 40, 'blocked': true }]
+ *
+ * // using "_.where" callback shorthand
+ * _.filter(characters, { 'age': 36 });
+ * // => [{ 'name': 'barney', 'age': 36, 'blocked': false }]
+ */
+function filter(collection, callback, thisArg) {
+ var result = [];
+ callback = createCallback(callback, thisArg, 3);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ }
+ } else {
+ baseEach(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = filter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/find.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/find.js b/node_modules/lodash-node/compat/collections/find.js
new file mode 100644
index 0000000..59f9853
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/find.js
@@ -0,0 +1,81 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Iterates over elements of a collection, returning the first element that
+ * the callback returns truey for. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias detect, findWhere
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the found element, else `undefined`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ * ];
+ *
+ * _.find(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => { 'name': 'barney', 'age': 36, 'blocked': false }
+ *
+ * // using "_.where" callback shorthand
+ * _.find(characters, { 'age': 1 });
+ * // => { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.find(characters, 'blocked');
+ * // => { 'name': 'fred', 'age': 40, 'blocked': true }
+ */
+function find(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg, 3);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ return value;
+ }
+ }
+ } else {
+ var result;
+ baseEach(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result = value;
+ return false;
+ }
+ });
+ return result;
+ }
+}
+
+module.exports = find;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/findLast.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/findLast.js b/node_modules/lodash-node/compat/collections/findLast.js
new file mode 100644
index 0000000..43d18e8
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/findLast.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEachRight = require('./forEachRight');
+
+/**
+ * This method is like `_.find` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the found element, else `undefined`.
+ * @example
+ *
+ * _.findLast([1, 2, 3, 4], function(num) {
+ * return num % 2 == 1;
+ * });
+ * // => 3
+ */
+function findLast(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+ forEachRight(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result = value;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = findLast;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/forEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/forEach.js b/node_modules/lodash-node/compat/collections/forEach.js
new file mode 100644
index 0000000..4f89582
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/forEach.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseEach = require('../internals/baseEach'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Iterates over elements of a collection, executing the callback for each
+ * element. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection). Callbacks may exit iteration early by
+ * explicitly returning `false`.
+ *
+ * Note: As with other "Collections" methods, objects with a `length` property
+ * are iterated like arrays. To avoid this behavior `_.forIn` or `_.forOwn`
+ * may be used for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @alias each
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEach(function(num) { console.log(num); }).join(',');
+ * // => logs each number and returns '1,2,3'
+ *
+ * _.forEach({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { console.log(num); });
+ * // => logs each number and returns the object (property order is not guaranteed across environments)
+ */
+function forEach(collection, callback, thisArg) {
+ if (callback && typeof thisArg == 'undefined' && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ if (callback(collection[index], index, collection) === false) {
+ break;
+ }
+ }
+ } else {
+ baseEach(collection, callback, thisArg);
+ }
+ return collection;
+}
+
+module.exports = forEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/forEachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/forEachRight.js b/node_modules/lodash-node/compat/collections/forEachRight.js
new file mode 100644
index 0000000..fc003a1
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/forEachRight.js
@@ -0,0 +1,59 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseEach = require('../internals/baseEach'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString'),
+ keys = require('../objects/keys'),
+ support = require('../support');
+
+/**
+ * This method is like `_.forEach` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias eachRight
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEachRight(function(num) { console.log(num); }).join(',');
+ * // => logs each number from right to left and returns '3,2,1'
+ */
+function forEachRight(collection, callback, thisArg) {
+ var iterable = collection,
+ length = collection ? collection.length : 0;
+
+ callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3);
+ if (isArray(collection)) {
+ while (length--) {
+ if (callback(collection[length], length, collection) === false) {
+ break;
+ }
+ }
+ } else {
+ if (typeof length != 'number') {
+ var props = keys(collection);
+ length = props.length;
+ } else if (support.unindexedChars && isString(collection)) {
+ iterable = collection.split('');
+ }
+ baseEach(collection, function(value, key, collection) {
+ key = props ? props[--length] : --length;
+ return callback(iterable[key], key, collection);
+ });
+ }
+ return collection;
+}
+
+module.exports = forEachRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/groupBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/groupBy.js b/node_modules/lodash-node/compat/collections/groupBy.js
new file mode 100644
index 0000000..90756c4
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/groupBy.js
@@ -0,0 +1,56 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of a collection through the callback. The corresponding value
+ * of each key is an array of the elements responsible for generating the key.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+var groupBy = createAggregator(function(result, value, key) {
+ (hasOwnProperty.call(result, key) ? result[key] : result[key] = []).push(value);
+});
+
+module.exports = groupBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/indexBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/indexBy.js b/node_modules/lodash-node/compat/collections/indexBy.js
new file mode 100644
index 0000000..b8c277e
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/indexBy.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of the collection through the given callback. The corresponding
+ * value of each key is the last element responsible for generating the key.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * var keys = [
+ * { 'dir': 'left', 'code': 97 },
+ * { 'dir': 'right', 'code': 100 }
+ * ];
+ *
+ * _.indexBy(keys, 'dir');
+ * // => { 'left': { 'dir': 'left', 'code': 97 }, 'right': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(keys, function(key) { return String.fromCharCode(key.code); });
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(characters, function(key) { this.fromCharCode(key.code); }, String);
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ */
+var indexBy = createAggregator(function(result, value, key) {
+ result[key] = value;
+});
+
+module.exports = indexBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/invoke.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/invoke.js b/node_modules/lodash-node/compat/collections/invoke.js
new file mode 100644
index 0000000..17de1ee
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/invoke.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('./forEach'),
+ slice = require('../internals/slice');
+
+/**
+ * Invokes the method named by `methodName` on each element in the `collection`
+ * returning an array of the results of each invoked method. Additional arguments
+ * will be provided to each invoked method. If `methodName` is a function it
+ * will be invoked for, and `this` bound to, each element in the `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|string} methodName The name of the method to invoke or
+ * the function invoked per iteration.
+ * @param {...*} [arg] Arguments to invoke the method with.
+ * @returns {Array} Returns a new array of the results of each invoked method.
+ * @example
+ *
+ * _.invoke([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invoke([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+function invoke(collection, methodName) {
+ var args = slice(arguments, 2),
+ index = -1,
+ isFunc = typeof methodName == 'function',
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ result[++index] = (isFunc ? methodName : value[methodName]).apply(value, args);
+ });
+ return result;
+}
+
+module.exports = invoke;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/map.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/map.js b/node_modules/lodash-node/compat/collections/map.js
new file mode 100644
index 0000000..cd371e8
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/map.js
@@ -0,0 +1,70 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates an array of values by running each element in the collection
+ * through the callback. The callback is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias collect
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of the results of each `callback` execution.
+ * @example
+ *
+ * _.map([1, 2, 3], function(num) { return num * 3; });
+ * // => [3, 6, 9]
+ *
+ * _.map({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { return num * 3; });
+ * // => [3, 6, 9] (property order is not guaranteed across environments)
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(characters, 'name');
+ * // => ['barney', 'fred']
+ */
+function map(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ callback = createCallback(callback, thisArg, 3);
+ if (isArray(collection)) {
+ while (++index < length) {
+ result[index] = callback(collection[index], index, collection);
+ }
+ } else {
+ baseEach(collection, function(value, key, collection) {
+ result[++index] = callback(value, key, collection);
+ });
+ }
+ return result;
+}
+
+module.exports = map;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/max.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/max.js b/node_modules/lodash-node/compat/collections/max.js
new file mode 100644
index 0000000..8547613
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/max.js
@@ -0,0 +1,90 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ charAtCallback = require('../internals/charAtCallback'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString');
+
+/**
+ * Retrieves the maximum value of a collection. If the collection is empty or
+ * falsey `-Infinity` is returned. If a callback is provided it will be executed
+ * for each value in the collection to generate the criterion by which the value
+ * is ranked. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the maximum value.
+ * @example
+ *
+ * _.max([4, 2, 8, 6]);
+ * // => 8
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.max(characters, function(chr) { return chr.age; });
+ * // => { 'name': 'fred', 'age': 40 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.max(characters, 'age');
+ * // => { 'name': 'fred', 'age': 40 };
+ */
+function max(collection, callback, thisArg) {
+ var computed = -Infinity,
+ result = computed;
+
+ // allows working with functions like `_.map` without using
+ // their `index` argument as a callback
+ if (typeof callback != 'function' && thisArg && thisArg[callback] === collection) {
+ callback = null;
+ }
+ if (callback == null && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (value > result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = (callback == null && isString(collection))
+ ? charAtCallback
+ : createCallback(callback, thisArg, 3);
+
+ baseEach(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current > computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = max;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/min.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/min.js b/node_modules/lodash-node/compat/collections/min.js
new file mode 100644
index 0000000..925f32c
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/min.js
@@ -0,0 +1,90 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ charAtCallback = require('../internals/charAtCallback'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString');
+
+/**
+ * Retrieves the minimum value of a collection. If the collection is empty or
+ * falsey `Infinity` is returned. If a callback is provided it will be executed
+ * for each value in the collection to generate the criterion by which the value
+ * is ranked. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the minimum value.
+ * @example
+ *
+ * _.min([4, 2, 8, 6]);
+ * // => 2
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.min(characters, function(chr) { return chr.age; });
+ * // => { 'name': 'barney', 'age': 36 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.min(characters, 'age');
+ * // => { 'name': 'barney', 'age': 36 };
+ */
+function min(collection, callback, thisArg) {
+ var computed = Infinity,
+ result = computed;
+
+ // allows working with functions like `_.map` without using
+ // their `index` argument as a callback
+ if (typeof callback != 'function' && thisArg && thisArg[callback] === collection) {
+ callback = null;
+ }
+ if (callback == null && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (value < result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = (callback == null && isString(collection))
+ ? charAtCallback
+ : createCallback(callback, thisArg, 3);
+
+ baseEach(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current < computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = min;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/pluck.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/pluck.js b/node_modules/lodash-node/compat/collections/pluck.js
new file mode 100644
index 0000000..8ef0be0
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/pluck.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var map = require('./map');
+
+/**
+ * Retrieves the value of a specified property from all elements in the collection.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {string} property The name of the property to pluck.
+ * @returns {Array} Returns a new array of property values.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.pluck(characters, 'name');
+ * // => ['barney', 'fred']
+ */
+var pluck = map;
+
+module.exports = pluck;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/reduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/reduce.js b/node_modules/lodash-node/compat/collections/reduce.js
new file mode 100644
index 0000000..fb7854b
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/reduce.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseEach = require('../internals/baseEach'),
+ createCallback = require('../functions/createCallback'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Reduces a collection to a value which is the accumulated result of running
+ * each element in the collection through the callback, where each successive
+ * callback execution consumes the return value of the previous execution. If
+ * `accumulator` is not provided the first element of the collection will be
+ * used as the initial `accumulator` value. The callback is bound to `thisArg`
+ * and invoked with four arguments; (accumulator, value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @alias foldl, inject
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] Initial value of the accumulator.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var sum = _.reduce([1, 2, 3], function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * var mapped = _.reduce({ 'a': 1, 'b': 2, 'c': 3 }, function(result, num, key) {
+ * result[key] = num * 3;
+ * return result;
+ * }, {});
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ */
+function reduce(collection, callback, accumulator, thisArg) {
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ if (noaccum) {
+ accumulator = collection[++index];
+ }
+ while (++index < length) {
+ accumulator = callback(accumulator, collection[index], index, collection);
+ }
+ } else {
+ baseEach(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection)
+ });
+ }
+ return accumulator;
+}
+
+module.exports = reduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/reduceRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/reduceRight.js b/node_modules/lodash-node/compat/collections/reduceRight.js
new file mode 100644
index 0000000..58625ea
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/reduceRight.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEachRight = require('./forEachRight');
+
+/**
+ * This method is like `_.reduce` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias foldr
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] Initial value of the accumulator.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var list = [[0, 1], [2, 3], [4, 5]];
+ * var flat = _.reduceRight(list, function(a, b) { return a.concat(b); }, []);
+ * // => [4, 5, 2, 3, 0, 1]
+ */
+function reduceRight(collection, callback, accumulator, thisArg) {
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+ forEachRight(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection);
+ });
+ return accumulator;
+}
+
+module.exports = reduceRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/reject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/reject.js b/node_modules/lodash-node/compat/collections/reject.js
new file mode 100644
index 0000000..f33239b
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/reject.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ filter = require('./filter');
+
+/**
+ * The opposite of `_.filter` this method returns the elements of a
+ * collection that the callback does **not** return truey for.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that failed the callback check.
+ * @example
+ *
+ * var odds = _.reject([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [1, 3, 5]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.reject(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'age': 36, 'blocked': false }]
+ *
+ * // using "_.where" callback shorthand
+ * _.reject(characters, { 'age': 36 });
+ * // => [{ 'name': 'fred', 'age': 40, 'blocked': true }]
+ */
+function reject(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg, 3);
+ return filter(collection, function(value, index, collection) {
+ return !callback(value, index, collection);
+ });
+}
+
+module.exports = reject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/compat/collections/sample.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/compat/collections/sample.js b/node_modules/lodash-node/compat/collections/sample.js
new file mode 100644
index 0000000..481c7e4
--- /dev/null
+++ b/node_modules/lodash-node/compat/collections/sample.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize exports="node" -o ./compat/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom'),
+ isString = require('../objects/isString'),
+ shuffle = require('./shuffle'),
+ support = require('../support'),
+ values = require('../objects/values');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Retrieves a random element or `n` random elements from a collection.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to sample.
+ * @param {number} [n] The number of elements to sample.
+ * @param- {Object} [guard] Allows working with functions like `_.map`
+ * without using their `index` arguments as `n`.
+ * @returns {Array} Returns the random sample(s) of `collection`.
+ * @example
+ *
+ * _.sample([1, 2, 3, 4]);
+ * // => 2
+ *
+ * _.sample([1, 2, 3, 4], 2);
+ * // => [3, 1]
+ */
+function sample(collection, n, guard) {
+ if (collection && typeof collection.length != 'number') {
+ collection = values(collection);
+ } else if (support.unindexedChars && isString(collection)) {
+ collection = collection.split('');
+ }
+ if (n == null || guard) {
+ return collection ? collection[baseRandom(0, collection.length - 1)] : undefined;
+ }
+ var result = shuffle(collection);
+ result.length = nativeMin(nativeMax(0, n), result.length);
+ return result;
+}
+
+module.exports = sample;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[21/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/README.md
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/README.md b/node_modules/node-uuid/README.md
new file mode 100644
index 0000000..a44d9a7
--- /dev/null
+++ b/node_modules/node-uuid/README.md
@@ -0,0 +1,199 @@
+# node-uuid
+
+Simple, fast generation of [RFC4122](http://www.ietf.org/rfc/rfc4122.txt) UUIDS.
+
+Features:
+
+* Generate RFC4122 version 1 or version 4 UUIDs
+* Runs in node.js and all browsers.
+* Cryptographically strong random # generation on supporting platforms
+* 1.1K minified and gzip'ed (Want something smaller? Check this [crazy shit](https://gist.github.com/982883) out! )
+* [Annotated source code](http://broofa.github.com/node-uuid/docs/uuid.html)
+
+## Getting Started
+
+Install it in your browser:
+
+```html
+<script src="uuid.js"></script>
+```
+
+Or in node.js:
+
+```
+npm install node-uuid
+```
+
+```javascript
+var uuid = require('node-uuid');
+```
+
+Then create some ids ...
+
+```javascript
+// Generate a v1 (time-based) id
+uuid.v1(); // -> '6c84fb90-12c4-11e1-840d-7b25c5ee775a'
+
+// Generate a v4 (random) id
+uuid.v4(); // -> '110ec58a-a0f2-4ac4-8393-c866d813b8d1'
+```
+
+## API
+
+### uuid.v1([`options` [, `buffer` [, `offset`]]])
+
+Generate and return a RFC4122 v1 (timestamp-based) UUID.
+
+* `options` - (Object) Optional uuid state to apply. Properties may include:
+
+ * `node` - (Array) Node id as Array of 6 bytes (per 4.1.6). Default: Randomnly generated ID. See note 1.
+ * `clockseq` - (Number between 0 - 0x3fff) RFC clock sequence. Default: An internally maintained clockseq is used.
+ * `msecs` - (Number | Date) Time in milliseconds since unix Epoch. Default: The current time is used.
+ * `nsecs` - (Number between 0-9999) additional time, in 100-nanosecond units. Ignored if `msecs` is unspecified. Default: internal uuid counter is used, as per 4.2.1.2.
+
+* `buffer` - (Array | Buffer) Array or buffer where UUID bytes are to be written.
+* `offset` - (Number) Starting index in `buffer` at which to begin writing.
+
+Returns `buffer`, if specified, otherwise the string form of the UUID
+
+Notes:
+
+1. The randomly generated node id is only guaranteed to stay constant for the lifetime of the current JS runtime. (Future versions of this module may use persistent storage mechanisms to extend this guarantee.)
+
+Example: Generate string UUID with fully-specified options
+
+```javascript
+uuid.v1({
+ node: [0x01, 0x23, 0x45, 0x67, 0x89, 0xab],
+ clockseq: 0x1234,
+ msecs: new Date('2011-11-01').getTime(),
+ nsecs: 5678
+}); // -> "710b962e-041c-11e1-9234-0123456789ab"
+```
+
+Example: In-place generation of two binary IDs
+
+```javascript
+// Generate two ids in an array
+var arr = new Array(32); // -> []
+uuid.v1(null, arr, 0); // -> [02 a2 ce 90 14 32 11 e1 85 58 0b 48 8e 4f c1 15]
+uuid.v1(null, arr, 16); // -> [02 a2 ce 90 14 32 11 e1 85 58 0b 48 8e 4f c1 15 02 a3 1c b0 14 32 11 e1 85 58 0b 48 8e 4f c1 15]
+
+// Optionally use uuid.unparse() to get stringify the ids
+uuid.unparse(buffer); // -> '02a2ce90-1432-11e1-8558-0b488e4fc115'
+uuid.unparse(buffer, 16) // -> '02a31cb0-1432-11e1-8558-0b488e4fc115'
+```
+
+### uuid.v4([`options` [, `buffer` [, `offset`]]])
+
+Generate and return a RFC4122 v4 UUID.
+
+* `options` - (Object) Optional uuid state to apply. Properties may include:
+
+ * `random` - (Number[16]) Array of 16 numbers (0-255) to use in place of randomly generated values
+ * `rng` - (Function) Random # generator to use. Set to one of the built-in generators - `uuid.mathRNG` (all platforms), `uuid.nodeRNG` (node.js only), `uuid.whatwgRNG` (WebKit only) - or a custom function that returns an array[16] of byte values.
+
+* `buffer` - (Array | Buffer) Array or buffer where UUID bytes are to be written.
+* `offset` - (Number) Starting index in `buffer` at which to begin writing.
+
+Returns `buffer`, if specified, otherwise the string form of the UUID
+
+Example: Generate string UUID with fully-specified options
+
+```javascript
+uuid.v4({
+ random: [
+ 0x10, 0x91, 0x56, 0xbe, 0xc4, 0xfb, 0xc1, 0xea,
+ 0x71, 0xb4, 0xef, 0xe1, 0x67, 0x1c, 0x58, 0x36
+ ]
+});
+// -> "109156be-c4fb-41ea-b1b4-efe1671c5836"
+```
+
+Example: Generate two IDs in a single buffer
+
+```javascript
+var buffer = new Array(32); // (or 'new Buffer' in node.js)
+uuid.v4(null, buffer, 0);
+uuid.v4(null, buffer, 16);
+```
+
+### uuid.parse(id[, buffer[, offset]])
+### uuid.unparse(buffer[, offset])
+
+Parse and unparse UUIDs
+
+ * `id` - (String) UUID(-like) string
+ * `buffer` - (Array | Buffer) Array or buffer where UUID bytes are to be written. Default: A new Array or Buffer is used
+ * `offset` - (Number) Starting index in `buffer` at which to begin writing. Default: 0
+
+Example parsing and unparsing a UUID string
+
+```javascript
+var bytes = uuid.parse('797ff043-11eb-11e1-80d6-510998755d10'); // -> <Buffer 79 7f f0 43 11 eb 11 e1 80 d6 51 09 98 75 5d 10>
+var string = uuid.unparse(bytes); // -> '797ff043-11eb-11e1-80d6-510998755d10'
+```
+
+### uuid.noConflict()
+
+(Browsers only) Set `uuid` property back to it's previous value.
+
+Returns the node-uuid object.
+
+Example:
+
+```javascript
+var myUuid = uuid.noConflict();
+myUuid.v1(); // -> '6c84fb90-12c4-11e1-840d-7b25c5ee775a'
+```
+
+## Deprecated APIs
+
+Support for the following v1.2 APIs is available in v1.3, but is deprecated and will be removed in the next major version.
+
+### uuid([format [, buffer [, offset]]])
+
+uuid() has become uuid.v4(), and the `format` argument is now implicit in the `buffer` argument. (i.e. if you specify a buffer, the format is assumed to be binary).
+
+### uuid.BufferClass
+
+The class of container created when generating binary uuid data if no buffer argument is specified. This is expected to go away, with no replacement API.
+
+## Testing
+
+In node.js
+
+```
+> cd test
+> node uuid.js
+```
+
+In Browser
+
+```
+open test/test.html
+```
+
+### Benchmarking
+
+Requires node.js
+
+```
+npm install uuid uuid-js
+node test/benchmark.js
+```
+
+For a more complete discussion of node-uuid performance, please see the `benchmark/README.md` file, and the [benchmark wiki](https://github.com/broofa/node-uuid/wiki/Benchmark)
+
+For browser performance [checkout the JSPerf tests](http://jsperf.com/node-uuid-performance).
+
+## Release notes
+
+v1.3.2:
+* Improve tests and handling of v1() options (Issue #24)
+* Expose RNG option to allow for perf testing with different generators
+
+v1.3:
+* Support for version 1 ids, thanks to [@ctavan](https://github.com/ctavan)!
+* Support for node.js crypto API
+* De-emphasizing performance in favor of a) cryptographic quality PRNGs where available and b) more manageable code
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/benchmark/README.md
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/benchmark/README.md b/node_modules/node-uuid/benchmark/README.md
new file mode 100644
index 0000000..aaeb2ea
--- /dev/null
+++ b/node_modules/node-uuid/benchmark/README.md
@@ -0,0 +1,53 @@
+# node-uuid Benchmarks
+
+### Results
+
+To see the results of our benchmarks visit https://github.com/broofa/node-uuid/wiki/Benchmark
+
+### Run them yourself
+
+node-uuid comes with some benchmarks to measure performance of generating UUIDs. These can be run using node.js. node-uuid is being benchmarked against some other uuid modules, that are available through npm namely `uuid` and `uuid-js`.
+
+To prepare and run the benchmark issue;
+
+```
+npm install uuid uuid-js
+node benchmark/benchmark.js
+```
+
+You'll see an output like this one:
+
+```
+# v4
+nodeuuid.v4(): 854700 uuids/second
+nodeuuid.v4('binary'): 788643 uuids/second
+nodeuuid.v4('binary', buffer): 1336898 uuids/second
+uuid(): 479386 uuids/second
+uuid('binary'): 582072 uuids/second
+uuidjs.create(4): 312304 uuids/second
+
+# v1
+nodeuuid.v1(): 938086 uuids/second
+nodeuuid.v1('binary'): 683060 uuids/second
+nodeuuid.v1('binary', buffer): 1644736 uuids/second
+uuidjs.create(1): 190621 uuids/second
+```
+
+* The `uuid()` entries are for Nikhil Marathe's [uuid module](https://bitbucket.org/nikhilm/uuidjs) which is a wrapper around the native libuuid library.
+* The `uuidjs()` entries are for Patrick Negri's [uuid-js module](https://github.com/pnegri/uuid-js) which is a pure javascript implementation based on [UUID.js](https://github.com/LiosK/UUID.js) by LiosK.
+
+If you want to get more reliable results you can run the benchmark multiple times and write the output into a log file:
+
+```
+for i in {0..9}; do node benchmark/benchmark.js >> benchmark/bench_0.4.12.log; done;
+```
+
+If you're interested in how performance varies between different node versions, you can issue the above command multiple times.
+
+You can then use the shell script `bench.sh` provided in this directory to calculate the averages over all benchmark runs and draw a nice plot:
+
+```
+(cd benchmark/ && ./bench.sh)
+```
+
+This assumes you have [gnuplot](http://www.gnuplot.info/) and [ImageMagick](http://www.imagemagick.org/) installed. You'll find a nice `bench.png` graph in the `benchmark/` directory then.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/benchmark/bench.gnu
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/benchmark/bench.gnu b/node_modules/node-uuid/benchmark/bench.gnu
new file mode 100644
index 0000000..a342fbb
--- /dev/null
+++ b/node_modules/node-uuid/benchmark/bench.gnu
@@ -0,0 +1,174 @@
+#!/opt/local/bin/gnuplot -persist
+#
+#
+# G N U P L O T
+# Version 4.4 patchlevel 3
+# last modified March 2011
+# System: Darwin 10.8.0
+#
+# Copyright (C) 1986-1993, 1998, 2004, 2007-2010
+# Thomas Williams, Colin Kelley and many others
+#
+# gnuplot home: http://www.gnuplot.info
+# faq, bugs, etc: type "help seeking-assistance"
+# immediate help: type "help"
+# plot window: hit 'h'
+set terminal postscript eps noenhanced defaultplex \
+ leveldefault color colortext \
+ solid linewidth 1.2 butt noclip \
+ palfuncparam 2000,0.003 \
+ "Helvetica" 14
+set output 'bench.eps'
+unset clip points
+set clip one
+unset clip two
+set bar 1.000000 front
+set border 31 front linetype -1 linewidth 1.000
+set xdata
+set ydata
+set zdata
+set x2data
+set y2data
+set timefmt x "%d/%m/%y,%H:%M"
+set timefmt y "%d/%m/%y,%H:%M"
+set timefmt z "%d/%m/%y,%H:%M"
+set timefmt x2 "%d/%m/%y,%H:%M"
+set timefmt y2 "%d/%m/%y,%H:%M"
+set timefmt cb "%d/%m/%y,%H:%M"
+set boxwidth
+set style fill empty border
+set style rectangle back fc lt -3 fillstyle solid 1.00 border lt -1
+set style circle radius graph 0.02, first 0, 0
+set dummy x,y
+set format x "% g"
+set format y "% g"
+set format x2 "% g"
+set format y2 "% g"
+set format z "% g"
+set format cb "% g"
+set angles radians
+unset grid
+set key title ""
+set key outside left top horizontal Right noreverse enhanced autotitles columnhead nobox
+set key noinvert samplen 4 spacing 1 width 0 height 0
+set key maxcolumns 2 maxrows 0
+unset label
+unset arrow
+set style increment default
+unset style line
+set style line 1 linetype 1 linewidth 2.000 pointtype 1 pointsize default pointinterval 0
+unset style arrow
+set style histogram clustered gap 2 title offset character 0, 0, 0
+unset logscale
+set offsets graph 0.05, 0.15, 0, 0
+set pointsize 1.5
+set pointintervalbox 1
+set encoding default
+unset polar
+unset parametric
+unset decimalsign
+set view 60, 30, 1, 1
+set samples 100, 100
+set isosamples 10, 10
+set surface
+unset contour
+set clabel '%8.3g'
+set mapping cartesian
+set datafile separator whitespace
+unset hidden3d
+set cntrparam order 4
+set cntrparam linear
+set cntrparam levels auto 5
+set cntrparam points 5
+set size ratio 0 1,1
+set origin 0,0
+set style data points
+set style function lines
+set xzeroaxis linetype -2 linewidth 1.000
+set yzeroaxis linetype -2 linewidth 1.000
+set zzeroaxis linetype -2 linewidth 1.000
+set x2zeroaxis linetype -2 linewidth 1.000
+set y2zeroaxis linetype -2 linewidth 1.000
+set ticslevel 0.5
+set mxtics default
+set mytics default
+set mztics default
+set mx2tics default
+set my2tics default
+set mcbtics default
+set xtics border in scale 1,0.5 mirror norotate offset character 0, 0, 0
+set xtics norangelimit
+set xtics ()
+set ytics border in scale 1,0.5 mirror norotate offset character 0, 0, 0
+set ytics autofreq norangelimit
+set ztics border in scale 1,0.5 nomirror norotate offset character 0, 0, 0
+set ztics autofreq norangelimit
+set nox2tics
+set noy2tics
+set cbtics border in scale 1,0.5 mirror norotate offset character 0, 0, 0
+set cbtics autofreq norangelimit
+set title ""
+set title offset character 0, 0, 0 font "" norotate
+set timestamp bottom
+set timestamp ""
+set timestamp offset character 0, 0, 0 font "" norotate
+set rrange [ * : * ] noreverse nowriteback # (currently [8.98847e+307:-8.98847e+307] )
+set autoscale rfixmin
+set autoscale rfixmax
+set trange [ * : * ] noreverse nowriteback # (currently [-5.00000:5.00000] )
+set autoscale tfixmin
+set autoscale tfixmax
+set urange [ * : * ] noreverse nowriteback # (currently [-10.0000:10.0000] )
+set autoscale ufixmin
+set autoscale ufixmax
+set vrange [ * : * ] noreverse nowriteback # (currently [-10.0000:10.0000] )
+set autoscale vfixmin
+set autoscale vfixmax
+set xlabel ""
+set xlabel offset character 0, 0, 0 font "" textcolor lt -1 norotate
+set x2label ""
+set x2label offset character 0, 0, 0 font "" textcolor lt -1 norotate
+set xrange [ * : * ] noreverse nowriteback # (currently [-0.150000:3.15000] )
+set autoscale xfixmin
+set autoscale xfixmax
+set x2range [ * : * ] noreverse nowriteback # (currently [0.00000:3.00000] )
+set autoscale x2fixmin
+set autoscale x2fixmax
+set ylabel ""
+set ylabel offset character 0, 0, 0 font "" textcolor lt -1 rotate by -270
+set y2label ""
+set y2label offset character 0, 0, 0 font "" textcolor lt -1 rotate by -270
+set yrange [ 0.00000 : 1.90000e+06 ] noreverse nowriteback # (currently [:] )
+set autoscale yfixmin
+set autoscale yfixmax
+set y2range [ * : * ] noreverse nowriteback # (currently [0.00000:1.90000e+06] )
+set autoscale y2fixmin
+set autoscale y2fixmax
+set zlabel ""
+set zlabel offset character 0, 0, 0 font "" textcolor lt -1 norotate
+set zrange [ * : * ] noreverse nowriteback # (currently [-10.0000:10.0000] )
+set autoscale zfixmin
+set autoscale zfixmax
+set cblabel ""
+set cblabel offset character 0, 0, 0 font "" textcolor lt -1 rotate by -270
+set cbrange [ * : * ] noreverse nowriteback # (currently [8.98847e+307:-8.98847e+307] )
+set autoscale cbfixmin
+set autoscale cbfixmax
+set zero 1e-08
+set lmargin -1
+set bmargin -1
+set rmargin -1
+set tmargin -1
+set pm3d explicit at s
+set pm3d scansautomatic
+set pm3d interpolate 1,1 flush begin noftriangles nohidden3d corners2color mean
+set palette positive nops_allcF maxcolors 0 gamma 1.5 color model RGB
+set palette rgbformulae 7, 5, 15
+set colorbox default
+set colorbox vertical origin screen 0.9, 0.2, 0 size screen 0.05, 0.6, 0 front bdefault
+set loadpath
+set fontpath
+set fit noerrorvariables
+GNUTERM = "aqua"
+plot 'bench_results.txt' using 2:xticlabel(1) w lp lw 2, '' using 3:xticlabel(1) w lp lw 2, '' using 4:xticlabel(1) w lp lw 2, '' using 5:xticlabel(1) w lp lw 2, '' using 6:xticlabel(1) w lp lw 2, '' using 7:xticlabel(1) w lp lw 2, '' using 8:xticlabel(1) w lp lw 2, '' using 9:xticlabel(1) w lp lw 2
+# EOF
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/benchmark/bench.sh
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/benchmark/bench.sh b/node_modules/node-uuid/benchmark/bench.sh
new file mode 100755
index 0000000..d870a0c
--- /dev/null
+++ b/node_modules/node-uuid/benchmark/bench.sh
@@ -0,0 +1,34 @@
+#!/bin/bash
+
+# for a given node version run:
+# for i in {0..9}; do node benchmark.js >> bench_0.6.2.log; done;
+
+PATTERNS=('nodeuuid.v1()' "nodeuuid.v1('binary'," 'nodeuuid.v4()' "nodeuuid.v4('binary'," "uuid()" "uuid('binary')" 'uuidjs.create(1)' 'uuidjs.create(4)' '140byte')
+FILES=(node_uuid_v1_string node_uuid_v1_buf node_uuid_v4_string node_uuid_v4_buf libuuid_v4_string libuuid_v4_binary uuidjs_v1_string uuidjs_v4_string 140byte_es)
+INDICES=(2 3 2 3 2 2 2 2 2)
+VERSIONS=$( ls bench_*.log | sed -e 's/^bench_\([0-9\.]*\)\.log/\1/' | tr "\\n" " " )
+TMPJOIN="tmp_join"
+OUTPUT="bench_results.txt"
+
+for I in ${!FILES[*]}; do
+ F=${FILES[$I]}
+ P=${PATTERNS[$I]}
+ INDEX=${INDICES[$I]}
+ echo "version $F" > $F
+ for V in $VERSIONS; do
+ (VAL=$( grep "$P" bench_$V.log | LC_ALL=en_US awk '{ sum += $'$INDEX' } END { print sum/NR }' ); echo $V $VAL) >> $F
+ done
+ if [ $I == 0 ]; then
+ cat $F > $TMPJOIN
+ else
+ join $TMPJOIN $F > $OUTPUT
+ cp $OUTPUT $TMPJOIN
+ fi
+ rm $F
+done
+
+rm $TMPJOIN
+
+gnuplot bench.gnu
+convert -density 200 -resize 800x560 -flatten bench.eps bench.png
+rm bench.eps
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/benchmark/benchmark-native.c
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/benchmark/benchmark-native.c b/node_modules/node-uuid/benchmark/benchmark-native.c
new file mode 100644
index 0000000..dbfc75f
--- /dev/null
+++ b/node_modules/node-uuid/benchmark/benchmark-native.c
@@ -0,0 +1,34 @@
+/*
+Test performance of native C UUID generation
+
+To Compile: cc -luuid benchmark-native.c -o benchmark-native
+*/
+
+#include <stdio.h>
+#include <unistd.h>
+#include <sys/time.h>
+#include <uuid/uuid.h>
+
+int main() {
+ uuid_t myid;
+ char buf[36+1];
+ int i;
+ struct timeval t;
+ double start, finish;
+
+ gettimeofday(&t, NULL);
+ start = t.tv_sec + t.tv_usec/1e6;
+
+ int n = 2e5;
+ for (i = 0; i < n; i++) {
+ uuid_generate(myid);
+ uuid_unparse(myid, buf);
+ }
+
+ gettimeofday(&t, NULL);
+ finish = t.tv_sec + t.tv_usec/1e6;
+ double dur = finish - start;
+
+ printf("%d uuids/sec", (int)(n/dur));
+ return 0;
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/benchmark/benchmark.js
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/benchmark/benchmark.js b/node_modules/node-uuid/benchmark/benchmark.js
new file mode 100644
index 0000000..40e6efb
--- /dev/null
+++ b/node_modules/node-uuid/benchmark/benchmark.js
@@ -0,0 +1,84 @@
+try {
+ var nodeuuid = require('../uuid');
+} catch (e) {
+ console.error('node-uuid require failed - skipping tests');
+}
+
+try {
+ var uuid = require('uuid');
+} catch (e) {
+ console.error('uuid require failed - skipping tests');
+}
+
+try {
+ var uuidjs = require('uuid-js');
+} catch (e) {
+ console.error('uuid-js require failed - skipping tests');
+}
+
+var N = 5e5;
+
+function rate(msg, t) {
+ console.log(msg + ': ' +
+ (N / (Date.now() - t) * 1e3 | 0) +
+ ' uuids/second');
+}
+
+console.log('# v4');
+
+// node-uuid - string form
+if (nodeuuid) {
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v4();
+ rate('nodeuuid.v4() - using node.js crypto RNG', t);
+
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v4({rng: nodeuuid.mathRNG});
+ rate('nodeuuid.v4() - using Math.random() RNG', t);
+
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v4('binary');
+ rate('nodeuuid.v4(\'binary\')', t);
+
+ var buffer = new nodeuuid.BufferClass(16);
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v4('binary', buffer);
+ rate('nodeuuid.v4(\'binary\', buffer)', t);
+}
+
+// libuuid - string form
+if (uuid) {
+ for (var i = 0, t = Date.now(); i < N; i++) uuid();
+ rate('uuid()', t);
+
+ for (var i = 0, t = Date.now(); i < N; i++) uuid('binary');
+ rate('uuid(\'binary\')', t);
+}
+
+// uuid-js - string form
+if (uuidjs) {
+ for (var i = 0, t = Date.now(); i < N; i++) uuidjs.create(4);
+ rate('uuidjs.create(4)', t);
+}
+
+// 140byte.es
+for (var i = 0, t = Date.now(); i < N; i++) 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g,function(s,r){r=Math.random()*16|0;return (s=='x'?r:r&0x3|0x8).toString(16)});
+rate('140byte.es_v4', t);
+
+console.log('');
+console.log('# v1');
+
+// node-uuid - v1 string form
+if (nodeuuid) {
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v1();
+ rate('nodeuuid.v1()', t);
+
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v1('binary');
+ rate('nodeuuid.v1(\'binary\')', t);
+
+ var buffer = new nodeuuid.BufferClass(16);
+ for (var i = 0, t = Date.now(); i < N; i++) nodeuuid.v1('binary', buffer);
+ rate('nodeuuid.v1(\'binary\', buffer)', t);
+}
+
+// uuid-js - v1 string form
+if (uuidjs) {
+ for (var i = 0, t = Date.now(); i < N; i++) uuidjs.create(1);
+ rate('uuidjs.create(1)', t);
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/package.json
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/package.json b/node_modules/node-uuid/package.json
new file mode 100644
index 0000000..9c2bef0
--- /dev/null
+++ b/node_modules/node-uuid/package.json
@@ -0,0 +1,85 @@
+{
+ "_args": [
+ [
+ "node-uuid@1.3.3",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/xcode"
+ ]
+ ],
+ "_defaultsLoaded": true,
+ "_engineSupported": true,
+ "_from": "node-uuid@1.3.3",
+ "_id": "node-uuid@1.3.3",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/node-uuid",
+ "_nodeVersion": "v0.4.6",
+ "_npmUser": {
+ "email": "robert@broofa.com",
+ "name": "broofa"
+ },
+ "_npmVersion": "1.0.106",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "node-uuid",
+ "raw": "node-uuid@1.3.3",
+ "rawSpec": "1.3.3",
+ "scope": null,
+ "spec": "1.3.3",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/xcode"
+ ],
+ "_resolved": "http://registry.npmjs.org/node-uuid/-/node-uuid-1.3.3.tgz",
+ "_shasum": "d3db4d7b56810d9e4032342766282af07391729b",
+ "_shrinkwrap": null,
+ "_spec": "node-uuid@1.3.3",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/xcode",
+ "author": {
+ "email": "robert@broofa.com",
+ "name": "Robert Kieffer"
+ },
+ "bugs": {
+ "url": "https://github.com/broofa/node-uuid/issues"
+ },
+ "contributors": [
+ {
+ "name": "Christoph Tavan",
+ "email": "dev@tavan.de"
+ }
+ ],
+ "dependencies": {},
+ "description": "Rigorous implementation of RFC4122 (v1 and v4) UUIDs.",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "d3db4d7b56810d9e4032342766282af07391729b",
+ "tarball": "http://registry.npmjs.org/node-uuid/-/node-uuid-1.3.3.tgz"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "homepage": "https://github.com/broofa/node-uuid#readme",
+ "keywords": [
+ "guid",
+ "rfc4122",
+ "uuid"
+ ],
+ "lib": ".",
+ "main": "./uuid.js",
+ "maintainers": [
+ {
+ "name": "broofa",
+ "email": "robert@broofa.com"
+ }
+ ],
+ "name": "node-uuid",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/broofa/node-uuid.git"
+ },
+ "url": "http://github.com/broofa/node-uuid",
+ "version": "1.3.3"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/test/compare_v1.js
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/test/compare_v1.js b/node_modules/node-uuid/test/compare_v1.js
new file mode 100644
index 0000000..05af822
--- /dev/null
+++ b/node_modules/node-uuid/test/compare_v1.js
@@ -0,0 +1,63 @@
+var assert = require('assert'),
+ nodeuuid = require('../uuid'),
+ uuidjs = require('uuid-js'),
+ libuuid = require('uuid').generate,
+ util = require('util'),
+ exec = require('child_process').exec,
+ os = require('os');
+
+// On Mac Os X / macports there's only the ossp-uuid package that provides uuid
+// On Linux there's uuid-runtime which provides uuidgen
+var uuidCmd = os.type() === 'Darwin' ? 'uuid -1' : 'uuidgen -t';
+
+function compare(ids) {
+ console.log(ids);
+ for (var i = 0; i < ids.length; i++) {
+ var id = ids[i].split('-');
+ id = [id[2], id[1], id[0]].join('');
+ ids[i] = id;
+ }
+ var sorted = ([].concat(ids)).sort();
+
+ if (sorted.toString() !== ids.toString()) {
+ console.log('Warning: sorted !== ids');
+ } else {
+ console.log('everything in order!');
+ }
+}
+
+// Test time order of v1 uuids
+var ids = [];
+while (ids.length < 10e3) ids.push(nodeuuid.v1());
+
+var max = 10;
+console.log('node-uuid:');
+ids = [];
+for (var i = 0; i < max; i++) ids.push(nodeuuid.v1());
+compare(ids);
+
+console.log('');
+console.log('uuidjs:');
+ids = [];
+for (var i = 0; i < max; i++) ids.push(uuidjs.create(1).toString());
+compare(ids);
+
+console.log('');
+console.log('libuuid:');
+ids = [];
+var count = 0;
+var last = function() {
+ compare(ids);
+}
+var cb = function(err, stdout, stderr) {
+ ids.push(stdout.substring(0, stdout.length-1));
+ count++;
+ if (count < max) {
+ return next();
+ }
+ last();
+};
+var next = function() {
+ exec(uuidCmd, cb);
+};
+next();
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/test/test.html
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/test/test.html b/node_modules/node-uuid/test/test.html
new file mode 100644
index 0000000..d80326e
--- /dev/null
+++ b/node_modules/node-uuid/test/test.html
@@ -0,0 +1,17 @@
+<html>
+ <head>
+ <style>
+ div {
+ font-family: monospace;
+ font-size: 8pt;
+ }
+ div.log {color: #444;}
+ div.warn {color: #550;}
+ div.error {color: #800; font-weight: bold;}
+ </style>
+ <script src="../uuid.js"></script>
+ </head>
+ <body>
+ <script src="./test.js"></script>
+ </body>
+</html>
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/test/test.js
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/test/test.js b/node_modules/node-uuid/test/test.js
new file mode 100644
index 0000000..be23919
--- /dev/null
+++ b/node_modules/node-uuid/test/test.js
@@ -0,0 +1,240 @@
+if (!this.uuid) {
+ // node.js
+ uuid = require('../uuid');
+}
+
+//
+// x-platform log/assert shims
+//
+
+function _log(msg, type) {
+ type = type || 'log';
+
+ if (typeof(document) != 'undefined') {
+ document.write('<div class="' + type + '">' + msg.replace(/\n/g, '<br />') + '</div>');
+ }
+ if (typeof(console) != 'undefined') {
+ var color = {
+ log: '\033[39m',
+ warn: '\033[33m',
+ error: '\033[31m'
+ }
+ console[type](color[type] + msg + color.log);
+ }
+}
+
+function log(msg) {_log(msg, 'log');}
+function warn(msg) {_log(msg, 'warn');}
+function error(msg) {_log(msg, 'error');}
+
+function assert(res, msg) {
+ if (!res) {
+ error('FAIL: ' + msg);
+ } else {
+ log('Pass: ' + msg);
+ }
+}
+
+//
+// Unit tests
+//
+
+// Verify ordering of v1 ids created with explicit times
+var TIME = 1321644961388; // 2011-11-18 11:36:01.388-08:00
+
+function compare(name, ids) {
+ ids = ids.map(function(id) {
+ return id.split('-').reverse().join('-');
+ }).sort();
+ var sorted = ([].concat(ids)).sort();
+
+ assert(sorted.toString() == ids.toString(), name + ' have expected order');
+}
+
+// Verify ordering of v1 ids created using default behavior
+compare('uuids with current time', [
+ uuid.v1(),
+ uuid.v1(),
+ uuid.v1(),
+ uuid.v1(),
+ uuid.v1()
+]);
+
+// Verify ordering of v1 ids created with explicit times
+compare('uuids with time option', [
+ uuid.v1({msecs: TIME - 10*3600*1000}),
+ uuid.v1({msecs: TIME - 1}),
+ uuid.v1({msecs: TIME}),
+ uuid.v1({msecs: TIME + 1}),
+ uuid.v1({msecs: TIME + 28*24*3600*1000}),
+]);
+
+assert(
+ uuid.v1({msecs: TIME}) != uuid.v1({msecs: TIME}),
+ 'IDs created at same msec are different'
+);
+
+// Verify throw if too many ids created
+var thrown = false;
+try {
+ uuid.v1({msecs: TIME, nsecs: 10000});
+} catch (e) {
+ thrown = true;
+}
+assert(thrown, 'Exception thrown when > 10K ids created in 1 ms');
+
+// Verify clock regression bumps clockseq
+var uidt = uuid.v1({msecs: TIME});
+var uidtb = uuid.v1({msecs: TIME - 1});
+assert(
+ parseInt(uidtb.split('-')[3], 16) - parseInt(uidt.split('-')[3], 16) === 1,
+ 'Clock regression by msec increments the clockseq'
+);
+
+// Verify clock regression bumps clockseq
+var uidtn = uuid.v1({msecs: TIME, nsecs: 10});
+var uidtnb = uuid.v1({msecs: TIME, nsecs: 9});
+assert(
+ parseInt(uidtnb.split('-')[3], 16) - parseInt(uidtn.split('-')[3], 16) === 1,
+ 'Clock regression by nsec increments the clockseq'
+);
+
+// Verify explicit options produce expected id
+var id = uuid.v1({
+ msecs: 1321651533573,
+ nsecs: 5432,
+ clockseq: 0x385c,
+ node: [ 0x61, 0xcd, 0x3c, 0xbb, 0x32, 0x10 ]
+});
+assert(id == 'd9428888-122b-11e1-b85c-61cd3cbb3210', 'Explicit options produce expected id');
+
+// Verify adjacent ids across a msec boundary are 1 time unit apart
+var u0 = uuid.v1({msecs: TIME, nsecs: 9999});
+var u1 = uuid.v1({msecs: TIME + 1, nsecs: 0});
+
+var before = u0.split('-')[0], after = u1.split('-')[0];
+var dt = parseInt(after, 16) - parseInt(before, 16);
+assert(dt === 1, 'Ids spanning 1ms boundary are 100ns apart');
+
+//
+// Test parse/unparse
+//
+
+id = '00112233445566778899aabbccddeeff';
+assert(uuid.unparse(uuid.parse(id.substr(0,10))) ==
+ '00112233-4400-0000-0000-000000000000', 'Short parse');
+assert(uuid.unparse(uuid.parse('(this is the uuid -> ' + id + id)) ==
+ '00112233-4455-6677-8899-aabbccddeeff', 'Dirty parse');
+
+//
+// Perf tests
+//
+
+var generators = {
+ v1: uuid.v1,
+ v4: uuid.v4
+};
+
+var UUID_FORMAT = {
+ v1: /[0-9a-f]{8}-[0-9a-f]{4}-1[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}/i,
+ v4: /[0-9a-f]{8}-[0-9a-f]{4}-4[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}/i
+};
+
+var N = 1e4;
+
+// Get %'age an actual value differs from the ideal value
+function divergence(actual, ideal) {
+ return Math.round(100*100*(actual - ideal)/ideal)/100;
+}
+
+function rate(msg, t) {
+ log(msg + ': ' + (N / (Date.now() - t) * 1e3 | 0) + ' uuids\/second');
+}
+
+for (var version in generators) {
+ var counts = {}, max = 0;
+ var generator = generators[version];
+ var format = UUID_FORMAT[version];
+
+ log('\nSanity check ' + N + ' ' + version + ' uuids');
+ for (var i = 0, ok = 0; i < N; i++) {
+ id = generator();
+ if (!format.test(id)) {
+ throw Error(id + ' is not a valid UUID string');
+ }
+
+ if (id != uuid.unparse(uuid.parse(id))) {
+ assert(fail, id + ' is not a valid id');
+ }
+
+ // Count digits for our randomness check
+ if (version == 'v4') {
+ var digits = id.replace(/-/g, '').split('');
+ for (var j = digits.length-1; j >= 0; j--) {
+ var c = digits[j];
+ max = Math.max(max, counts[c] = (counts[c] || 0) + 1);
+ }
+ }
+ }
+
+ // Check randomness for v4 UUIDs
+ if (version == 'v4') {
+ // Limit that we get worried about randomness. (Purely empirical choice, this!)
+ var limit = 2*100*Math.sqrt(1/N);
+
+ log('\nChecking v4 randomness. Distribution of Hex Digits (% deviation from ideal)');
+
+ for (var i = 0; i < 16; i++) {
+ var c = i.toString(16);
+ var bar = '', n = counts[c], p = Math.round(n/max*100|0);
+
+ // 1-3,5-8, and D-F: 1:16 odds over 30 digits
+ var ideal = N*30/16;
+ if (i == 4) {
+ // 4: 1:1 odds on 1 digit, plus 1:16 odds on 30 digits
+ ideal = N*(1 + 30/16);
+ } else if (i >= 8 && i <= 11) {
+ // 8-B: 1:4 odds on 1 digit, plus 1:16 odds on 30 digits
+ ideal = N*(1/4 + 30/16);
+ } else {
+ // Otherwise: 1:16 odds on 30 digits
+ ideal = N*30/16;
+ }
+ var d = divergence(n, ideal);
+
+ // Draw bar using UTF squares (just for grins)
+ var s = n/max*50 | 0;
+ while (s--) bar += '=';
+
+ assert(Math.abs(d) < limit, c + ' |' + bar + '| ' + counts[c] + ' (' + d + '% < ' + limit + '%)');
+ }
+ }
+}
+
+// Perf tests
+for (var version in generators) {
+ log('\nPerformance testing ' + version + ' UUIDs');
+ var generator = generators[version];
+ var buf = new uuid.BufferClass(16);
+
+ if (version == 'v4') {
+ ['mathRNG', 'whatwgRNG', 'nodeRNG'].forEach(function(rng) {
+ if (uuid[rng]) {
+ var options = {rng: uuid[rng]};
+ for (var i = 0, t = Date.now(); i < N; i++) generator(options);
+ rate('uuid.' + version + '() with ' + rng, t);
+ } else {
+ log('uuid.' + version + '() with ' + rng + ': not defined');
+ }
+ });
+ } else {
+ for (var i = 0, t = Date.now(); i < N; i++) generator();
+ rate('uuid.' + version + '()', t);
+ }
+
+ for (var i = 0, t = Date.now(); i < N; i++) generator('binary');
+ rate('uuid.' + version + '(\'binary\')', t);
+
+ for (var i = 0, t = Date.now(); i < N; i++) generator('binary', buf);
+ rate('uuid.' + version + '(\'binary\', buffer)', t);
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/node-uuid/uuid.js
----------------------------------------------------------------------
diff --git a/node_modules/node-uuid/uuid.js b/node_modules/node-uuid/uuid.js
new file mode 100644
index 0000000..27f1d12
--- /dev/null
+++ b/node_modules/node-uuid/uuid.js
@@ -0,0 +1,249 @@
+// node-uuid/uuid.js
+//
+// Copyright (c) 2010 Robert Kieffer
+// Dual licensed under the MIT and GPL licenses.
+// Documentation and details at https://github.com/broofa/node-uuid
+(function() {
+ var _global = this;
+
+ // Unique ID creation requires a high quality random # generator, but
+ // Math.random() does not guarantee "cryptographic quality". So we feature
+ // detect for more robust APIs, normalizing each method to return 128-bits
+ // (16 bytes) of random data.
+ var mathRNG, nodeRNG, whatwgRNG;
+
+ // Math.random()-based RNG. All platforms, very fast, unknown quality
+ var _rndBytes = new Array(16);
+ mathRNG = function() {
+ var r, b = _rndBytes, i = 0;
+
+ for (var i = 0, r; i < 16; i++) {
+ if ((i & 0x03) == 0) r = Math.random() * 0x100000000;
+ b[i] = r >>> ((i & 0x03) << 3) & 0xff;
+ }
+
+ return b;
+ }
+
+ // WHATWG crypto-based RNG - http://wiki.whatwg.org/wiki/Crypto
+ // WebKit only (currently), moderately fast, high quality
+ if (_global.crypto && crypto.getRandomValues) {
+ var _rnds = new Uint32Array(4);
+ whatwgRNG = function() {
+ crypto.getRandomValues(_rnds);
+
+ for (var c = 0 ; c < 16; c++) {
+ _rndBytes[c] = _rnds[c >> 2] >>> ((c & 0x03) * 8) & 0xff;
+ }
+ return _rndBytes;
+ }
+ }
+
+ // Node.js crypto-based RNG - http://nodejs.org/docs/v0.6.2/api/crypto.html
+ // Node.js only, moderately fast, high quality
+ try {
+ var _rb = require('crypto').randomBytes;
+ nodeRNG = _rb && function() {
+ return _rb(16);
+ };
+ } catch (e) {}
+
+ // Select RNG with best quality
+ var _rng = nodeRNG || whatwgRNG || mathRNG;
+
+ // Buffer class to use
+ var BufferClass = typeof(Buffer) == 'function' ? Buffer : Array;
+
+ // Maps for number <-> hex string conversion
+ var _byteToHex = [];
+ var _hexToByte = {};
+ for (var i = 0; i < 256; i++) {
+ _byteToHex[i] = (i + 0x100).toString(16).substr(1);
+ _hexToByte[_byteToHex[i]] = i;
+ }
+
+ // **`parse()` - Parse a UUID into it's component bytes**
+ function parse(s, buf, offset) {
+ var i = (buf && offset) || 0, ii = 0;
+
+ buf = buf || [];
+ s.toLowerCase().replace(/[0-9a-f]{2}/g, function(byte) {
+ if (ii < 16) { // Don't overflow!
+ buf[i + ii++] = _hexToByte[byte];
+ }
+ });
+
+ // Zero out remaining bytes if string was short
+ while (ii < 16) {
+ buf[i + ii++] = 0;
+ }
+
+ return buf;
+ }
+
+ // **`unparse()` - Convert UUID byte array (ala parse()) into a string**
+ function unparse(buf, offset) {
+ var i = offset || 0, bth = _byteToHex;
+ return bth[buf[i++]] + bth[buf[i++]] +
+ bth[buf[i++]] + bth[buf[i++]] + '-' +
+ bth[buf[i++]] + bth[buf[i++]] + '-' +
+ bth[buf[i++]] + bth[buf[i++]] + '-' +
+ bth[buf[i++]] + bth[buf[i++]] + '-' +
+ bth[buf[i++]] + bth[buf[i++]] +
+ bth[buf[i++]] + bth[buf[i++]] +
+ bth[buf[i++]] + bth[buf[i++]];
+ }
+
+ // **`v1()` - Generate time-based UUID**
+ //
+ // Inspired by https://github.com/LiosK/UUID.js
+ // and http://docs.python.org/library/uuid.html
+
+ // random #'s we need to init node and clockseq
+ var _seedBytes = _rng();
+
+ // Per 4.5, create and 48-bit node id, (47 random bits + multicast bit = 1)
+ var _nodeId = [
+ _seedBytes[0] | 0x01,
+ _seedBytes[1], _seedBytes[2], _seedBytes[3], _seedBytes[4], _seedBytes[5]
+ ];
+
+ // Per 4.2.2, randomize (14 bit) clockseq
+ var _clockseq = (_seedBytes[6] << 8 | _seedBytes[7]) & 0x3fff;
+
+ // Previous uuid creation time
+ var _lastMSecs = 0, _lastNSecs = 0;
+
+ // See https://github.com/broofa/node-uuid for API details
+ function v1(options, buf, offset) {
+ var i = buf && offset || 0;
+ var b = buf || [];
+
+ options = options || {};
+
+ var clockseq = options.clockseq != null ? options.clockseq : _clockseq;
+
+ // UUID timestamps are 100 nano-second units since the Gregorian epoch,
+ // (1582-10-15 00:00). JSNumbers aren't precise enough for this, so
+ // time is handled internally as 'msecs' (integer milliseconds) and 'nsecs'
+ // (100-nanoseconds offset from msecs) since unix epoch, 1970-01-01 00:00.
+ var msecs = options.msecs != null ? options.msecs : new Date().getTime();
+
+ // Per 4.2.1.2, use count of uuid's generated during the current clock
+ // cycle to simulate higher resolution clock
+ var nsecs = options.nsecs != null ? options.nsecs : _lastNSecs + 1;
+
+ // Time since last uuid creation (in msecs)
+ var dt = (msecs - _lastMSecs) + (nsecs - _lastNSecs)/10000;
+
+ // Per 4.2.1.2, Bump clockseq on clock regression
+ if (dt < 0 && options.clockseq == null) {
+ clockseq = clockseq + 1 & 0x3fff;
+ }
+
+ // Reset nsecs if clock regresses (new clockseq) or we've moved onto a new
+ // time interval
+ if ((dt < 0 || msecs > _lastMSecs) && options.nsecs == null) {
+ nsecs = 0;
+ }
+
+ // Per 4.2.1.2 Throw error if too many uuids are requested
+ if (nsecs >= 10000) {
+ throw new Error('uuid.v1(): Can\'t create more than 10M uuids/sec');
+ }
+
+ _lastMSecs = msecs;
+ _lastNSecs = nsecs;
+ _clockseq = clockseq;
+
+ // Per 4.1.4 - Convert from unix epoch to Gregorian epoch
+ msecs += 12219292800000;
+
+ // `time_low`
+ var tl = ((msecs & 0xfffffff) * 10000 + nsecs) % 0x100000000;
+ b[i++] = tl >>> 24 & 0xff;
+ b[i++] = tl >>> 16 & 0xff;
+ b[i++] = tl >>> 8 & 0xff;
+ b[i++] = tl & 0xff;
+
+ // `time_mid`
+ var tmh = (msecs / 0x100000000 * 10000) & 0xfffffff;
+ b[i++] = tmh >>> 8 & 0xff;
+ b[i++] = tmh & 0xff;
+
+ // `time_high_and_version`
+ b[i++] = tmh >>> 24 & 0xf | 0x10; // include version
+ b[i++] = tmh >>> 16 & 0xff;
+
+ // `clock_seq_hi_and_reserved` (Per 4.2.2 - include variant)
+ b[i++] = clockseq >>> 8 | 0x80;
+
+ // `clock_seq_low`
+ b[i++] = clockseq & 0xff;
+
+ // `node`
+ var node = options.node || _nodeId;
+ for (var n = 0; n < 6; n++) {
+ b[i + n] = node[n];
+ }
+
+ return buf ? buf : unparse(b);
+ }
+
+ // **`v4()` - Generate random UUID**
+
+ // See https://github.com/broofa/node-uuid for API details
+ function v4(options, buf, offset) {
+ // Deprecated - 'format' argument, as supported in v1.2
+ var i = buf && offset || 0;
+
+ if (typeof(options) == 'string') {
+ buf = options == 'binary' ? new BufferClass(16) : null;
+ options = null;
+ }
+ options = options || {};
+
+ var rnds = options.random || (options.rng || _rng)();
+
+ // Per 4.4, set bits for version and `clock_seq_hi_and_reserved`
+ rnds[6] = (rnds[6] & 0x0f) | 0x40;
+ rnds[8] = (rnds[8] & 0x3f) | 0x80;
+
+ // Copy bytes to buffer, if provided
+ if (buf) {
+ for (var ii = 0; ii < 16; ii++) {
+ buf[i + ii] = rnds[ii];
+ }
+ }
+
+ return buf || unparse(rnds);
+ }
+
+ // Export public API
+ var uuid = v4;
+ uuid.v1 = v1;
+ uuid.v4 = v4;
+ uuid.parse = parse;
+ uuid.unparse = unparse;
+ uuid.BufferClass = BufferClass;
+
+ // Export RNG options
+ uuid.mathRNG = mathRNG;
+ uuid.nodeRNG = nodeRNG;
+ uuid.whatwgRNG = whatwgRNG;
+
+ if (typeof(module) != 'undefined') {
+ // Play nice with node.js
+ module.exports = uuid;
+ } else {
+ // Play nice with browsers
+ var _previousRoot = _global.uuid;
+
+ // **`noConflict()` - (browser only) to reset global 'uuid' var**
+ uuid.noConflict = function() {
+ _global.uuid = _previousRoot;
+ return uuid;
+ }
+ _global.uuid = uuid;
+ }
+}());
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/nopt/.npmignore b/node_modules/nopt/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/nopt/.npmignore
@@ -0,0 +1 @@
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/nopt/.travis.yml b/node_modules/nopt/.travis.yml
new file mode 100644
index 0000000..99f2bbf
--- /dev/null
+++ b/node_modules/nopt/.travis.yml
@@ -0,0 +1,9 @@
+language: node_js
+language: node_js
+node_js:
+ - '0.8'
+ - '0.10'
+ - '0.12'
+ - 'iojs'
+before_install:
+ - npm install -g npm@latest
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/nopt/LICENSE b/node_modules/nopt/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/nopt/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/README.md
----------------------------------------------------------------------
diff --git a/node_modules/nopt/README.md b/node_modules/nopt/README.md
new file mode 100644
index 0000000..f21a4b3
--- /dev/null
+++ b/node_modules/nopt/README.md
@@ -0,0 +1,211 @@
+If you want to write an option parser, and have it be good, there are
+two ways to do it. The Right Way, and the Wrong Way.
+
+The Wrong Way is to sit down and write an option parser. We've all done
+that.
+
+The Right Way is to write some complex configurable program with so many
+options that you hit the limit of your frustration just trying to
+manage them all, and defer it with duct-tape solutions until you see
+exactly to the core of the problem, and finally snap and write an
+awesome option parser.
+
+If you want to write an option parser, don't write an option parser.
+Write a package manager, or a source control system, or a service
+restarter, or an operating system. You probably won't end up with a
+good one of those, but if you don't give up, and you are relentless and
+diligent enough in your procrastination, you may just end up with a very
+nice option parser.
+
+## USAGE
+
+ // my-program.js
+ var nopt = require("nopt")
+ , Stream = require("stream").Stream
+ , path = require("path")
+ , knownOpts = { "foo" : [String, null]
+ , "bar" : [Stream, Number]
+ , "baz" : path
+ , "bloo" : [ "big", "medium", "small" ]
+ , "flag" : Boolean
+ , "pick" : Boolean
+ , "many1" : [String, Array]
+ , "many2" : [path]
+ }
+ , shortHands = { "foofoo" : ["--foo", "Mr. Foo"]
+ , "b7" : ["--bar", "7"]
+ , "m" : ["--bloo", "medium"]
+ , "p" : ["--pick"]
+ , "f" : ["--flag"]
+ }
+ // everything is optional.
+ // knownOpts and shorthands default to {}
+ // arg list defaults to process.argv
+ // slice defaults to 2
+ , parsed = nopt(knownOpts, shortHands, process.argv, 2)
+ console.log(parsed)
+
+This would give you support for any of the following:
+
+```bash
+$ node my-program.js --foo "blerp" --no-flag
+{ "foo" : "blerp", "flag" : false }
+
+$ node my-program.js ---bar 7 --foo "Mr. Hand" --flag
+{ bar: 7, foo: "Mr. Hand", flag: true }
+
+$ node my-program.js --foo "blerp" -f -----p
+{ foo: "blerp", flag: true, pick: true }
+
+$ node my-program.js -fp --foofoo
+{ foo: "Mr. Foo", flag: true, pick: true }
+
+$ node my-program.js --foofoo -- -fp # -- stops the flag parsing.
+{ foo: "Mr. Foo", argv: { remain: ["-fp"] } }
+
+$ node my-program.js --blatzk -fp # unknown opts are ok.
+{ blatzk: true, flag: true, pick: true }
+
+$ node my-program.js --blatzk=1000 -fp # but you need to use = if they have a value
+{ blatzk: 1000, flag: true, pick: true }
+
+$ node my-program.js --no-blatzk -fp # unless they start with "no-"
+{ blatzk: false, flag: true, pick: true }
+
+$ node my-program.js --baz b/a/z # known paths are resolved.
+{ baz: "/Users/isaacs/b/a/z" }
+
+# if Array is one of the types, then it can take many
+# values, and will always be an array. The other types provided
+# specify what types are allowed in the list.
+
+$ node my-program.js --many1 5 --many1 null --many1 foo
+{ many1: ["5", "null", "foo"] }
+
+$ node my-program.js --many2 foo --many2 bar
+{ many2: ["/path/to/foo", "path/to/bar"] }
+```
+
+Read the tests at the bottom of `lib/nopt.js` for more examples of
+what this puppy can do.
+
+## Types
+
+The following types are supported, and defined on `nopt.typeDefs`
+
+* String: A normal string. No parsing is done.
+* path: A file system path. Gets resolved against cwd if not absolute.
+* url: A url. If it doesn't parse, it isn't accepted.
+* Number: Must be numeric.
+* Date: Must parse as a date. If it does, and `Date` is one of the options,
+ then it will return a Date object, not a string.
+* Boolean: Must be either `true` or `false`. If an option is a boolean,
+ then it does not need a value, and its presence will imply `true` as
+ the value. To negate boolean flags, do `--no-whatever` or `--whatever
+ false`
+* NaN: Means that the option is strictly not allowed. Any value will
+ fail.
+* Stream: An object matching the "Stream" class in node. Valuable
+ for use when validating programmatically. (npm uses this to let you
+ supply any WriteStream on the `outfd` and `logfd` config options.)
+* Array: If `Array` is specified as one of the types, then the value
+ will be parsed as a list of options. This means that multiple values
+ can be specified, and that the value will always be an array.
+
+If a type is an array of values not on this list, then those are
+considered valid values. For instance, in the example above, the
+`--bloo` option can only be one of `"big"`, `"medium"`, or `"small"`,
+and any other value will be rejected.
+
+When parsing unknown fields, `"true"`, `"false"`, and `"null"` will be
+interpreted as their JavaScript equivalents.
+
+You can also mix types and values, or multiple types, in a list. For
+instance `{ blah: [Number, null] }` would allow a value to be set to
+either a Number or null. When types are ordered, this implies a
+preference, and the first type that can be used to properly interpret
+the value will be used.
+
+To define a new type, add it to `nopt.typeDefs`. Each item in that
+hash is an object with a `type` member and a `validate` method. The
+`type` member is an object that matches what goes in the type list. The
+`validate` method is a function that gets called with `validate(data,
+key, val)`. Validate methods should assign `data[key]` to the valid
+value of `val` if it can be handled properly, or return boolean
+`false` if it cannot.
+
+You can also call `nopt.clean(data, types, typeDefs)` to clean up a
+config object and remove its invalid properties.
+
+## Error Handling
+
+By default, nopt outputs a warning to standard error when invalid values for
+known options are found. You can change this behavior by assigning a method
+to `nopt.invalidHandler`. This method will be called with
+the offending `nopt.invalidHandler(key, val, types)`.
+
+If no `nopt.invalidHandler` is assigned, then it will console.error
+its whining. If it is assigned to boolean `false` then the warning is
+suppressed.
+
+## Abbreviations
+
+Yes, they are supported. If you define options like this:
+
+```javascript
+{ "foolhardyelephants" : Boolean
+, "pileofmonkeys" : Boolean }
+```
+
+Then this will work:
+
+```bash
+node program.js --foolhar --pil
+node program.js --no-f --pileofmon
+# etc.
+```
+
+## Shorthands
+
+Shorthands are a hash of shorter option names to a snippet of args that
+they expand to.
+
+If multiple one-character shorthands are all combined, and the
+combination does not unambiguously match any other option or shorthand,
+then they will be broken up into their constituent parts. For example:
+
+```json
+{ "s" : ["--loglevel", "silent"]
+, "g" : "--global"
+, "f" : "--force"
+, "p" : "--parseable"
+, "l" : "--long"
+}
+```
+
+```bash
+npm ls -sgflp
+# just like doing this:
+npm ls --loglevel silent --global --force --long --parseable
+```
+
+## The Rest of the args
+
+The config object returned by nopt is given a special member called
+`argv`, which is an object with the following fields:
+
+* `remain`: The remaining args after all the parsing has occurred.
+* `original`: The args as they originally appeared.
+* `cooked`: The args after flags and shorthands are expanded.
+
+## Slicing
+
+Node programs are called with more or less the exact argv as it appears
+in C land, after the v8 and node-specific options have been plucked off.
+As such, `argv[0]` is always `node` and `argv[1]` is always the
+JavaScript program being run.
+
+That's usually not very useful to you. So they're sliced off by
+default. If you want them, then you can pass in `0` as the last
+argument, or any other number that you'd like to slice off the start of
+the list.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/bin/nopt.js
----------------------------------------------------------------------
diff --git a/node_modules/nopt/bin/nopt.js b/node_modules/nopt/bin/nopt.js
new file mode 100755
index 0000000..3232d4c
--- /dev/null
+++ b/node_modules/nopt/bin/nopt.js
@@ -0,0 +1,54 @@
+#!/usr/bin/env node
+var nopt = require("../lib/nopt")
+ , path = require("path")
+ , types = { num: Number
+ , bool: Boolean
+ , help: Boolean
+ , list: Array
+ , "num-list": [Number, Array]
+ , "str-list": [String, Array]
+ , "bool-list": [Boolean, Array]
+ , str: String
+ , clear: Boolean
+ , config: Boolean
+ , length: Number
+ , file: path
+ }
+ , shorthands = { s: [ "--str", "astring" ]
+ , b: [ "--bool" ]
+ , nb: [ "--no-bool" ]
+ , tft: [ "--bool-list", "--no-bool-list", "--bool-list", "true" ]
+ , "?": ["--help"]
+ , h: ["--help"]
+ , H: ["--help"]
+ , n: [ "--num", "125" ]
+ , c: ["--config"]
+ , l: ["--length"]
+ , f: ["--file"]
+ }
+ , parsed = nopt( types
+ , shorthands
+ , process.argv
+ , 2 )
+
+console.log("parsed", parsed)
+
+if (parsed.help) {
+ console.log("")
+ console.log("nopt cli tester")
+ console.log("")
+ console.log("types")
+ console.log(Object.keys(types).map(function M (t) {
+ var type = types[t]
+ if (Array.isArray(type)) {
+ return [t, type.map(function (type) { return type.name })]
+ }
+ return [t, type && type.name]
+ }).reduce(function (s, i) {
+ s[i[0]] = i[1]
+ return s
+ }, {}))
+ console.log("")
+ console.log("shorthands")
+ console.log(shorthands)
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/examples/my-program.js
----------------------------------------------------------------------
diff --git a/node_modules/nopt/examples/my-program.js b/node_modules/nopt/examples/my-program.js
new file mode 100755
index 0000000..142447e
--- /dev/null
+++ b/node_modules/nopt/examples/my-program.js
@@ -0,0 +1,30 @@
+#!/usr/bin/env node
+
+//process.env.DEBUG_NOPT = 1
+
+// my-program.js
+var nopt = require("../lib/nopt")
+ , Stream = require("stream").Stream
+ , path = require("path")
+ , knownOpts = { "foo" : [String, null]
+ , "bar" : [Stream, Number]
+ , "baz" : path
+ , "bloo" : [ "big", "medium", "small" ]
+ , "flag" : Boolean
+ , "pick" : Boolean
+ }
+ , shortHands = { "foofoo" : ["--foo", "Mr. Foo"]
+ , "b7" : ["--bar", "7"]
+ , "m" : ["--bloo", "medium"]
+ , "p" : ["--pick"]
+ , "f" : ["--flag", "true"]
+ , "g" : ["--flag"]
+ , "s" : "--flag"
+ }
+ // everything is optional.
+ // knownOpts and shorthands default to {}
+ // arg list defaults to process.argv
+ // slice defaults to 2
+ , parsed = nopt(knownOpts, shortHands, process.argv, 2)
+
+console.log("parsed =\n"+ require("util").inspect(parsed))
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/lib/nopt.js
----------------------------------------------------------------------
diff --git a/node_modules/nopt/lib/nopt.js b/node_modules/nopt/lib/nopt.js
new file mode 100644
index 0000000..97707e7
--- /dev/null
+++ b/node_modules/nopt/lib/nopt.js
@@ -0,0 +1,415 @@
+// info about each config option.
+
+var debug = process.env.DEBUG_NOPT || process.env.NOPT_DEBUG
+ ? function () { console.error.apply(console, arguments) }
+ : function () {}
+
+var url = require("url")
+ , path = require("path")
+ , Stream = require("stream").Stream
+ , abbrev = require("abbrev")
+
+module.exports = exports = nopt
+exports.clean = clean
+
+exports.typeDefs =
+ { String : { type: String, validate: validateString }
+ , Boolean : { type: Boolean, validate: validateBoolean }
+ , url : { type: url, validate: validateUrl }
+ , Number : { type: Number, validate: validateNumber }
+ , path : { type: path, validate: validatePath }
+ , Stream : { type: Stream, validate: validateStream }
+ , Date : { type: Date, validate: validateDate }
+ }
+
+function nopt (types, shorthands, args, slice) {
+ args = args || process.argv
+ types = types || {}
+ shorthands = shorthands || {}
+ if (typeof slice !== "number") slice = 2
+
+ debug(types, shorthands, args, slice)
+
+ args = args.slice(slice)
+ var data = {}
+ , key
+ , remain = []
+ , cooked = args
+ , original = args.slice(0)
+
+ parse(args, data, remain, types, shorthands)
+ // now data is full
+ clean(data, types, exports.typeDefs)
+ data.argv = {remain:remain,cooked:cooked,original:original}
+ Object.defineProperty(data.argv, 'toString', { value: function () {
+ return this.original.map(JSON.stringify).join(" ")
+ }, enumerable: false })
+ return data
+}
+
+function clean (data, types, typeDefs) {
+ typeDefs = typeDefs || exports.typeDefs
+ var remove = {}
+ , typeDefault = [false, true, null, String, Array]
+
+ Object.keys(data).forEach(function (k) {
+ if (k === "argv") return
+ var val = data[k]
+ , isArray = Array.isArray(val)
+ , type = types[k]
+ if (!isArray) val = [val]
+ if (!type) type = typeDefault
+ if (type === Array) type = typeDefault.concat(Array)
+ if (!Array.isArray(type)) type = [type]
+
+ debug("val=%j", val)
+ debug("types=", type)
+ val = val.map(function (val) {
+ // if it's an unknown value, then parse false/true/null/numbers/dates
+ if (typeof val === "string") {
+ debug("string %j", val)
+ val = val.trim()
+ if ((val === "null" && ~type.indexOf(null))
+ || (val === "true" &&
+ (~type.indexOf(true) || ~type.indexOf(Boolean)))
+ || (val === "false" &&
+ (~type.indexOf(false) || ~type.indexOf(Boolean)))) {
+ val = JSON.parse(val)
+ debug("jsonable %j", val)
+ } else if (~type.indexOf(Number) && !isNaN(val)) {
+ debug("convert to number", val)
+ val = +val
+ } else if (~type.indexOf(Date) && !isNaN(Date.parse(val))) {
+ debug("convert to date", val)
+ val = new Date(val)
+ }
+ }
+
+ if (!types.hasOwnProperty(k)) {
+ return val
+ }
+
+ // allow `--no-blah` to set 'blah' to null if null is allowed
+ if (val === false && ~type.indexOf(null) &&
+ !(~type.indexOf(false) || ~type.indexOf(Boolean))) {
+ val = null
+ }
+
+ var d = {}
+ d[k] = val
+ debug("prevalidated val", d, val, types[k])
+ if (!validate(d, k, val, types[k], typeDefs)) {
+ if (exports.invalidHandler) {
+ exports.invalidHandler(k, val, types[k], data)
+ } else if (exports.invalidHandler !== false) {
+ debug("invalid: "+k+"="+val, types[k])
+ }
+ return remove
+ }
+ debug("validated val", d, val, types[k])
+ return d[k]
+ }).filter(function (val) { return val !== remove })
+
+ if (!val.length) delete data[k]
+ else if (isArray) {
+ debug(isArray, data[k], val)
+ data[k] = val
+ } else data[k] = val[0]
+
+ debug("k=%s val=%j", k, val, data[k])
+ })
+}
+
+function validateString (data, k, val) {
+ data[k] = String(val)
+}
+
+function validatePath (data, k, val) {
+ if (val === true) return false
+ if (val === null) return true
+
+ val = String(val)
+ var homePattern = process.platform === 'win32' ? /^~(\/|\\)/ : /^~\//
+ if (val.match(homePattern) && process.env.HOME) {
+ val = path.resolve(process.env.HOME, val.substr(2))
+ }
+ data[k] = path.resolve(String(val))
+ return true
+}
+
+function validateNumber (data, k, val) {
+ debug("validate Number %j %j %j", k, val, isNaN(val))
+ if (isNaN(val)) return false
+ data[k] = +val
+}
+
+function validateDate (data, k, val) {
+ debug("validate Date %j %j %j", k, val, Date.parse(val))
+ var s = Date.parse(val)
+ if (isNaN(s)) return false
+ data[k] = new Date(val)
+}
+
+function validateBoolean (data, k, val) {
+ if (val instanceof Boolean) val = val.valueOf()
+ else if (typeof val === "string") {
+ if (!isNaN(val)) val = !!(+val)
+ else if (val === "null" || val === "false") val = false
+ else val = true
+ } else val = !!val
+ data[k] = val
+}
+
+function validateUrl (data, k, val) {
+ val = url.parse(String(val))
+ if (!val.host) return false
+ data[k] = val.href
+}
+
+function validateStream (data, k, val) {
+ if (!(val instanceof Stream)) return false
+ data[k] = val
+}
+
+function validate (data, k, val, type, typeDefs) {
+ // arrays are lists of types.
+ if (Array.isArray(type)) {
+ for (var i = 0, l = type.length; i < l; i ++) {
+ if (type[i] === Array) continue
+ if (validate(data, k, val, type[i], typeDefs)) return true
+ }
+ delete data[k]
+ return false
+ }
+
+ // an array of anything?
+ if (type === Array) return true
+
+ // NaN is poisonous. Means that something is not allowed.
+ if (type !== type) {
+ debug("Poison NaN", k, val, type)
+ delete data[k]
+ return false
+ }
+
+ // explicit list of values
+ if (val === type) {
+ debug("Explicitly allowed %j", val)
+ // if (isArray) (data[k] = data[k] || []).push(val)
+ // else data[k] = val
+ data[k] = val
+ return true
+ }
+
+ // now go through the list of typeDefs, validate against each one.
+ var ok = false
+ , types = Object.keys(typeDefs)
+ for (var i = 0, l = types.length; i < l; i ++) {
+ debug("test type %j %j %j", k, val, types[i])
+ var t = typeDefs[types[i]]
+ if (t &&
+ ((type && type.name && t.type && t.type.name) ? (type.name === t.type.name) : (type === t.type))) {
+ var d = {}
+ ok = false !== t.validate(d, k, val)
+ val = d[k]
+ if (ok) {
+ // if (isArray) (data[k] = data[k] || []).push(val)
+ // else data[k] = val
+ data[k] = val
+ break
+ }
+ }
+ }
+ debug("OK? %j (%j %j %j)", ok, k, val, types[i])
+
+ if (!ok) delete data[k]
+ return ok
+}
+
+function parse (args, data, remain, types, shorthands) {
+ debug("parse", args, data, remain)
+
+ var key = null
+ , abbrevs = abbrev(Object.keys(types))
+ , shortAbbr = abbrev(Object.keys(shorthands))
+
+ for (var i = 0; i < args.length; i ++) {
+ var arg = args[i]
+ debug("arg", arg)
+
+ if (arg.match(/^-{2,}$/)) {
+ // done with keys.
+ // the rest are args.
+ remain.push.apply(remain, args.slice(i + 1))
+ args[i] = "--"
+ break
+ }
+ var hadEq = false
+ if (arg.charAt(0) === "-" && arg.length > 1) {
+ if (arg.indexOf("=") !== -1) {
+ hadEq = true
+ var v = arg.split("=")
+ arg = v.shift()
+ v = v.join("=")
+ args.splice.apply(args, [i, 1].concat([arg, v]))
+ }
+
+ // see if it's a shorthand
+ // if so, splice and back up to re-parse it.
+ var shRes = resolveShort(arg, shorthands, shortAbbr, abbrevs)
+ debug("arg=%j shRes=%j", arg, shRes)
+ if (shRes) {
+ debug(arg, shRes)
+ args.splice.apply(args, [i, 1].concat(shRes))
+ if (arg !== shRes[0]) {
+ i --
+ continue
+ }
+ }
+ arg = arg.replace(/^-+/, "")
+ var no = null
+ while (arg.toLowerCase().indexOf("no-") === 0) {
+ no = !no
+ arg = arg.substr(3)
+ }
+
+ if (abbrevs[arg]) arg = abbrevs[arg]
+
+ var isArray = types[arg] === Array ||
+ Array.isArray(types[arg]) && types[arg].indexOf(Array) !== -1
+
+ // allow unknown things to be arrays if specified multiple times.
+ if (!types.hasOwnProperty(arg) && data.hasOwnProperty(arg)) {
+ if (!Array.isArray(data[arg]))
+ data[arg] = [data[arg]]
+ isArray = true
+ }
+
+ var val
+ , la = args[i + 1]
+
+ var isBool = typeof no === 'boolean' ||
+ types[arg] === Boolean ||
+ Array.isArray(types[arg]) && types[arg].indexOf(Boolean) !== -1 ||
+ (typeof types[arg] === 'undefined' && !hadEq) ||
+ (la === "false" &&
+ (types[arg] === null ||
+ Array.isArray(types[arg]) && ~types[arg].indexOf(null)))
+
+ if (isBool) {
+ // just set and move along
+ val = !no
+ // however, also support --bool true or --bool false
+ if (la === "true" || la === "false") {
+ val = JSON.parse(la)
+ la = null
+ if (no) val = !val
+ i ++
+ }
+
+ // also support "foo":[Boolean, "bar"] and "--foo bar"
+ if (Array.isArray(types[arg]) && la) {
+ if (~types[arg].indexOf(la)) {
+ // an explicit type
+ val = la
+ i ++
+ } else if ( la === "null" && ~types[arg].indexOf(null) ) {
+ // null allowed
+ val = null
+ i ++
+ } else if ( !la.match(/^-{2,}[^-]/) &&
+ !isNaN(la) &&
+ ~types[arg].indexOf(Number) ) {
+ // number
+ val = +la
+ i ++
+ } else if ( !la.match(/^-[^-]/) && ~types[arg].indexOf(String) ) {
+ // string
+ val = la
+ i ++
+ }
+ }
+
+ if (isArray) (data[arg] = data[arg] || []).push(val)
+ else data[arg] = val
+
+ continue
+ }
+
+ if (types[arg] === String && la === undefined)
+ la = ""
+
+ if (la && la.match(/^-{2,}$/)) {
+ la = undefined
+ i --
+ }
+
+ val = la === undefined ? true : la
+ if (isArray) (data[arg] = data[arg] || []).push(val)
+ else data[arg] = val
+
+ i ++
+ continue
+ }
+ remain.push(arg)
+ }
+}
+
+function resolveShort (arg, shorthands, shortAbbr, abbrevs) {
+ // handle single-char shorthands glommed together, like
+ // npm ls -glp, but only if there is one dash, and only if
+ // all of the chars are single-char shorthands, and it's
+ // not a match to some other abbrev.
+ arg = arg.replace(/^-+/, '')
+
+ // if it's an exact known option, then don't go any further
+ if (abbrevs[arg] === arg)
+ return null
+
+ // if it's an exact known shortopt, same deal
+ if (shorthands[arg]) {
+ // make it an array, if it's a list of words
+ if (shorthands[arg] && !Array.isArray(shorthands[arg]))
+ shorthands[arg] = shorthands[arg].split(/\s+/)
+
+ return shorthands[arg]
+ }
+
+ // first check to see if this arg is a set of single-char shorthands
+ var singles = shorthands.___singles
+ if (!singles) {
+ singles = Object.keys(shorthands).filter(function (s) {
+ return s.length === 1
+ }).reduce(function (l,r) {
+ l[r] = true
+ return l
+ }, {})
+ shorthands.___singles = singles
+ debug('shorthand singles', singles)
+ }
+
+ var chrs = arg.split("").filter(function (c) {
+ return singles[c]
+ })
+
+ if (chrs.join("") === arg) return chrs.map(function (c) {
+ return shorthands[c]
+ }).reduce(function (l, r) {
+ return l.concat(r)
+ }, [])
+
+
+ // if it's an arg abbrev, and not a literal shorthand, then prefer the arg
+ if (abbrevs[arg] && !shorthands[arg])
+ return null
+
+ // if it's an abbr for a shorthand, then use that
+ if (shortAbbr[arg])
+ arg = shortAbbr[arg]
+
+ // make it an array, if it's a list of words
+ if (shorthands[arg] && !Array.isArray(shorthands[arg]))
+ shorthands[arg] = shorthands[arg].split(/\s+/)
+
+ return shorthands[arg]
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/package.json
----------------------------------------------------------------------
diff --git a/node_modules/nopt/package.json b/node_modules/nopt/package.json
new file mode 100644
index 0000000..4628882
--- /dev/null
+++ b/node_modules/nopt/package.json
@@ -0,0 +1,88 @@
+{
+ "_args": [
+ [
+ "nopt@^3.0.6",
+ "/Users/steveng/repo/cordova/cordova-osx"
+ ]
+ ],
+ "_from": "nopt@>=3.0.6 <4.0.0",
+ "_id": "nopt@3.0.6",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/nopt",
+ "_nodeVersion": "4.2.1",
+ "_npmUser": {
+ "email": "ogd@aoaioxxysz.net",
+ "name": "othiym23"
+ },
+ "_npmVersion": "2.14.10",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "nopt",
+ "raw": "nopt@^3.0.6",
+ "rawSpec": "^3.0.6",
+ "scope": null,
+ "spec": ">=3.0.6 <4.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/"
+ ],
+ "_resolved": "http://registry.npmjs.org/nopt/-/nopt-3.0.6.tgz",
+ "_shasum": "c6465dbf08abcd4db359317f79ac68a646b28ff9",
+ "_shrinkwrap": null,
+ "_spec": "nopt@^3.0.6",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me/"
+ },
+ "bin": {
+ "nopt": "./bin/nopt.js"
+ },
+ "bugs": {
+ "url": "https://github.com/npm/nopt/issues"
+ },
+ "dependencies": {
+ "abbrev": "1"
+ },
+ "description": "Option parsing for Node, supporting types, shorthands, etc. Used by npm.",
+ "devDependencies": {
+ "tap": "^1.2.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "c6465dbf08abcd4db359317f79ac68a646b28ff9",
+ "tarball": "http://registry.npmjs.org/nopt/-/nopt-3.0.6.tgz"
+ },
+ "gitHead": "10a750c9bb99c1950160353459e733ac2aa18cb6",
+ "homepage": "https://github.com/npm/nopt#readme",
+ "license": "ISC",
+ "main": "lib/nopt.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ {
+ "name": "othiym23",
+ "email": "ogd@aoaioxxysz.net"
+ },
+ {
+ "name": "zkat",
+ "email": "kat@sykosomatic.org"
+ }
+ ],
+ "name": "nopt",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/npm/nopt.git"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "version": "3.0.6"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/nopt/test/basic.js
----------------------------------------------------------------------
diff --git a/node_modules/nopt/test/basic.js b/node_modules/nopt/test/basic.js
new file mode 100644
index 0000000..d399de9
--- /dev/null
+++ b/node_modules/nopt/test/basic.js
@@ -0,0 +1,273 @@
+var nopt = require("../")
+ , test = require('tap').test
+
+
+test("passing a string results in a string", function (t) {
+ var parsed = nopt({ key: String }, {}, ["--key", "myvalue"], 0)
+ t.same(parsed.key, "myvalue")
+ t.end()
+})
+
+// https://github.com/npm/nopt/issues/31
+test("Empty String results in empty string, not true", function (t) {
+ var parsed = nopt({ empty: String }, {}, ["--empty"], 0)
+ t.same(parsed.empty, "")
+ t.end()
+})
+
+test("~ path is resolved to $HOME", function (t) {
+ var path = require("path")
+ if (!process.env.HOME) process.env.HOME = "/tmp"
+ var parsed = nopt({key: path}, {}, ["--key=~/val"], 0)
+ t.same(parsed.key, path.resolve(process.env.HOME, "val"))
+ t.end()
+})
+
+// https://github.com/npm/nopt/issues/24
+test("Unknown options are not parsed as numbers", function (t) {
+ var parsed = nopt({"parse-me": Number}, null, ['--leave-as-is=1.20', '--parse-me=1.20'], 0)
+ t.equal(parsed['leave-as-is'], '1.20')
+ t.equal(parsed['parse-me'], 1.2)
+ t.end()
+});
+
+// https://github.com/npm/nopt/issues/48
+test("Check types based on name of type", function (t) {
+ var parsed = nopt({"parse-me": {name: "Number"}}, null, ['--parse-me=1.20'], 0)
+ t.equal(parsed['parse-me'], 1.2)
+ t.end()
+})
+
+
+test("Missing types are not parsed", function (t) {
+ var parsed = nopt({"parse-me": {}}, null, ['--parse-me=1.20'], 0)
+ //should only contain argv
+ t.equal(Object.keys(parsed).length, 1)
+ t.end()
+})
+
+test("Types passed without a name are not parsed", function (t) {
+ var parsed = nopt({"parse-me": {}}, {}, ['--parse-me=1.20'], 0)
+ //should only contain argv
+ t.equal(Object.keys(parsed).length, 1)
+ t.end()
+})
+
+test("other tests", function (t) {
+
+ var util = require("util")
+ , Stream = require("stream")
+ , path = require("path")
+ , url = require("url")
+
+ , shorthands =
+ { s : ["--loglevel", "silent"]
+ , d : ["--loglevel", "info"]
+ , dd : ["--loglevel", "verbose"]
+ , ddd : ["--loglevel", "silly"]
+ , noreg : ["--no-registry"]
+ , reg : ["--registry"]
+ , "no-reg" : ["--no-registry"]
+ , silent : ["--loglevel", "silent"]
+ , verbose : ["--loglevel", "verbose"]
+ , h : ["--usage"]
+ , H : ["--usage"]
+ , "?" : ["--usage"]
+ , help : ["--usage"]
+ , v : ["--version"]
+ , f : ["--force"]
+ , desc : ["--description"]
+ , "no-desc" : ["--no-description"]
+ , "local" : ["--no-global"]
+ , l : ["--long"]
+ , p : ["--parseable"]
+ , porcelain : ["--parseable"]
+ , g : ["--global"]
+ }
+
+ , types =
+ { aoa: Array
+ , nullstream: [null, Stream]
+ , date: Date
+ , str: String
+ , browser : String
+ , cache : path
+ , color : ["always", Boolean]
+ , depth : Number
+ , description : Boolean
+ , dev : Boolean
+ , editor : path
+ , force : Boolean
+ , global : Boolean
+ , globalconfig : path
+ , group : [String, Number]
+ , gzipbin : String
+ , logfd : [Number, Stream]
+ , loglevel : ["silent","win","error","warn","info","verbose","silly"]
+ , long : Boolean
+ , "node-version" : [false, String]
+ , npaturl : url
+ , npat : Boolean
+ , "onload-script" : [false, String]
+ , outfd : [Number, Stream]
+ , parseable : Boolean
+ , pre: Boolean
+ , prefix: path
+ , proxy : url
+ , "rebuild-bundle" : Boolean
+ , registry : url
+ , searchopts : String
+ , searchexclude: [null, String]
+ , shell : path
+ , t: [Array, String]
+ , tag : String
+ , tar : String
+ , tmp : path
+ , "unsafe-perm" : Boolean
+ , usage : Boolean
+ , user : String
+ , username : String
+ , userconfig : path
+ , version : Boolean
+ , viewer: path
+ , _exit : Boolean
+ , path: path
+ }
+
+ ; [["-v", {version:true}, []]
+ ,["---v", {version:true}, []]
+ ,["ls -s --no-reg connect -d",
+ {loglevel:"info",registry:null},["ls","connect"]]
+ ,["ls ---s foo",{loglevel:"silent"},["ls","foo"]]
+ ,["ls --registry blargle", {}, ["ls"]]
+ ,["--no-registry", {registry:null}, []]
+ ,["--no-color true", {color:false}, []]
+ ,["--no-color false", {color:true}, []]
+ ,["--no-color", {color:false}, []]
+ ,["--color false", {color:false}, []]
+ ,["--color --logfd 7", {logfd:7,color:true}, []]
+ ,["--color=true", {color:true}, []]
+ ,["--logfd=10", {logfd:10}, []]
+ ,["--tmp=/tmp -tar=gtar",{tmp:"/tmp",tar:"gtar"},[]]
+ ,["--tmp=tmp -tar=gtar",
+ {tmp:path.resolve(process.cwd(), "tmp"),tar:"gtar"},[]]
+ ,["--logfd x", {}, []]
+ ,["a -true -- -no-false", {true:true},["a","-no-false"]]
+ ,["a -no-false", {false:false},["a"]]
+ ,["a -no-no-true", {true:true}, ["a"]]
+ ,["a -no-no-no-false", {false:false}, ["a"]]
+ ,["---NO-no-No-no-no-no-nO-no-no"+
+ "-No-no-no-no-no-no-no-no-no"+
+ "-no-no-no-no-NO-NO-no-no-no-no-no-no"+
+ "-no-body-can-do-the-boogaloo-like-I-do"
+ ,{"body-can-do-the-boogaloo-like-I-do":false}, []]
+ ,["we are -no-strangers-to-love "+
+ "--you-know=the-rules --and=so-do-i "+
+ "---im-thinking-of=a-full-commitment "+
+ "--no-you-would-get-this-from-any-other-guy "+
+ "--no-gonna-give-you-up "+
+ "-no-gonna-let-you-down=true "+
+ "--no-no-gonna-run-around false "+
+ "--desert-you=false "+
+ "--make-you-cry false "+
+ "--no-tell-a-lie "+
+ "--no-no-and-hurt-you false"
+ ,{"strangers-to-love":false
+ ,"you-know":"the-rules"
+ ,"and":"so-do-i"
+ ,"you-would-get-this-from-any-other-guy":false
+ ,"gonna-give-you-up":false
+ ,"gonna-let-you-down":false
+ ,"gonna-run-around":false
+ ,"desert-you":false
+ ,"make-you-cry":false
+ ,"tell-a-lie":false
+ ,"and-hurt-you":false
+ },["we", "are"]]
+ ,["-t one -t two -t three"
+ ,{t: ["one", "two", "three"]}
+ ,[]]
+ ,["-t one -t null -t three four five null"
+ ,{t: ["one", "null", "three"]}
+ ,["four", "five", "null"]]
+ ,["-t foo"
+ ,{t:["foo"]}
+ ,[]]
+ ,["--no-t"
+ ,{t:["false"]}
+ ,[]]
+ ,["-no-no-t"
+ ,{t:["true"]}
+ ,[]]
+ ,["-aoa one -aoa null -aoa 100"
+ ,{aoa:["one", null, '100']}
+ ,[]]
+ ,["-str 100"
+ ,{str:"100"}
+ ,[]]
+ ,["--color always"
+ ,{color:"always"}
+ ,[]]
+ ,["--no-nullstream"
+ ,{nullstream:null}
+ ,[]]
+ ,["--nullstream false"
+ ,{nullstream:null}
+ ,[]]
+ ,["--notadate=2011-01-25"
+ ,{notadate: "2011-01-25"}
+ ,[]]
+ ,["--date 2011-01-25"
+ ,{date: new Date("2011-01-25")}
+ ,[]]
+ ,["-cl 1"
+ ,{config: true, length: 1}
+ ,[]
+ ,{config: Boolean, length: Number, clear: Boolean}
+ ,{c: "--config", l: "--length"}]
+ ,["--acount bla"
+ ,{"acount":true}
+ ,["bla"]
+ ,{account: Boolean, credentials: Boolean, options: String}
+ ,{a:"--account", c:"--credentials",o:"--options"}]
+ ,["--clear"
+ ,{clear:true}
+ ,[]
+ ,{clear:Boolean,con:Boolean,len:Boolean,exp:Boolean,add:Boolean,rep:Boolean}
+ ,{c:"--con",l:"--len",e:"--exp",a:"--add",r:"--rep"}]
+ ,["--file -"
+ ,{"file":"-"}
+ ,[]
+ ,{file:String}
+ ,{}]
+ ,["--file -"
+ ,{"file":true}
+ ,["-"]
+ ,{file:Boolean}
+ ,{}]
+ ,["--path"
+ ,{"path":null}
+ ,[]]
+ ,["--path ."
+ ,{"path":process.cwd()}
+ ,[]]
+ ].forEach(function (test) {
+ var argv = test[0].split(/\s+/)
+ , opts = test[1]
+ , rem = test[2]
+ , actual = nopt(test[3] || types, test[4] || shorthands, argv, 0)
+ , parsed = actual.argv
+ delete actual.argv
+ for (var i in opts) {
+ var e = JSON.stringify(opts[i])
+ , a = JSON.stringify(actual[i] === undefined ? null : actual[i])
+ if (e && typeof e === "object") {
+ t.deepEqual(e, a)
+ } else {
+ t.equal(e, a)
+ }
+ }
+ t.deepEqual(rem, parsed.remain)
+ })
+ t.end()
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/once/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/once/LICENSE b/node_modules/once/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/once/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/once/README.md
----------------------------------------------------------------------
diff --git a/node_modules/once/README.md b/node_modules/once/README.md
new file mode 100644
index 0000000..a2981ea
--- /dev/null
+++ b/node_modules/once/README.md
@@ -0,0 +1,51 @@
+# once
+
+Only call a function once.
+
+## usage
+
+```javascript
+var once = require('once')
+
+function load (file, cb) {
+ cb = once(cb)
+ loader.load('file')
+ loader.once('load', cb)
+ loader.once('error', cb)
+}
+```
+
+Or add to the Function.prototype in a responsible way:
+
+```javascript
+// only has to be done once
+require('once').proto()
+
+function load (file, cb) {
+ cb = cb.once()
+ loader.load('file')
+ loader.once('load', cb)
+ loader.once('error', cb)
+}
+```
+
+Ironically, the prototype feature makes this module twice as
+complicated as necessary.
+
+To check whether you function has been called, use `fn.called`. Once the
+function is called for the first time the return value of the original
+function is saved in `fn.value` and subsequent calls will continue to
+return this value.
+
+```javascript
+var once = require('once')
+
+function load (cb) {
+ cb = once(cb)
+ var stream = createStream()
+ stream.once('data', cb)
+ stream.once('end', function () {
+ if (!cb.called) cb(new Error('not found'))
+ })
+}
+```
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/once/once.js
----------------------------------------------------------------------
diff --git a/node_modules/once/once.js b/node_modules/once/once.js
new file mode 100644
index 0000000..2e1e721
--- /dev/null
+++ b/node_modules/once/once.js
@@ -0,0 +1,21 @@
+var wrappy = require('wrappy')
+module.exports = wrappy(once)
+
+once.proto = once(function () {
+ Object.defineProperty(Function.prototype, 'once', {
+ value: function () {
+ return once(this)
+ },
+ configurable: true
+ })
+})
+
+function once (fn) {
+ var f = function () {
+ if (f.called) return f.value
+ f.called = true
+ return f.value = fn.apply(this, arguments)
+ }
+ f.called = false
+ return f
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/once/package.json
----------------------------------------------------------------------
diff --git a/node_modules/once/package.json b/node_modules/once/package.json
new file mode 100644
index 0000000..15cb893
--- /dev/null
+++ b/node_modules/once/package.json
@@ -0,0 +1,89 @@
+{
+ "_args": [
+ [
+ "once@^1.3.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob"
+ ]
+ ],
+ "_from": "once@>=1.3.0 <2.0.0",
+ "_id": "once@1.3.3",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/once",
+ "_nodeVersion": "4.0.0",
+ "_npmUser": {
+ "email": "i@izs.me",
+ "name": "isaacs"
+ },
+ "_npmVersion": "3.3.2",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "once",
+ "raw": "once@^1.3.0",
+ "rawSpec": "^1.3.0",
+ "scope": null,
+ "spec": ">=1.3.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/glob",
+ "/inflight"
+ ],
+ "_resolved": "http://registry.npmjs.org/once/-/once-1.3.3.tgz",
+ "_shasum": "b2e261557ce4c314ec8304f3fa82663e4297ca20",
+ "_shrinkwrap": null,
+ "_spec": "once@^1.3.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/glob",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me/"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/once/issues"
+ },
+ "dependencies": {
+ "wrappy": "1"
+ },
+ "description": "Run a function exactly one time",
+ "devDependencies": {
+ "tap": "^1.2.0"
+ },
+ "directories": {
+ "test": "test"
+ },
+ "dist": {
+ "shasum": "b2e261557ce4c314ec8304f3fa82663e4297ca20",
+ "tarball": "http://registry.npmjs.org/once/-/once-1.3.3.tgz"
+ },
+ "files": [
+ "once.js"
+ ],
+ "gitHead": "2ad558657e17fafd24803217ba854762842e4178",
+ "homepage": "https://github.com/isaacs/once#readme",
+ "keywords": [
+ "function",
+ "once",
+ "one",
+ "single"
+ ],
+ "license": "ISC",
+ "main": "once.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "name": "once",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/once.git"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "version": "1.3.3"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-homedir/index.js
----------------------------------------------------------------------
diff --git a/node_modules/os-homedir/index.js b/node_modules/os-homedir/index.js
new file mode 100644
index 0000000..3306616
--- /dev/null
+++ b/node_modules/os-homedir/index.js
@@ -0,0 +1,24 @@
+'use strict';
+var os = require('os');
+
+function homedir() {
+ var env = process.env;
+ var home = env.HOME;
+ var user = env.LOGNAME || env.USER || env.LNAME || env.USERNAME;
+
+ if (process.platform === 'win32') {
+ return env.USERPROFILE || env.HOMEDRIVE + env.HOMEPATH || home || null;
+ }
+
+ if (process.platform === 'darwin') {
+ return home || (user ? '/Users/' + user : null);
+ }
+
+ if (process.platform === 'linux') {
+ return home || (process.getuid() === 0 ? '/root' : (user ? '/home/' + user : null));
+ }
+
+ return home || null;
+}
+
+module.exports = typeof os.homedir === 'function' ? os.homedir : homedir;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-homedir/license
----------------------------------------------------------------------
diff --git a/node_modules/os-homedir/license b/node_modules/os-homedir/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/os-homedir/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus <si...@gmail.com> (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/os-homedir/package.json
----------------------------------------------------------------------
diff --git a/node_modules/os-homedir/package.json b/node_modules/os-homedir/package.json
new file mode 100644
index 0000000..2c7e01f
--- /dev/null
+++ b/node_modules/os-homedir/package.json
@@ -0,0 +1,96 @@
+{
+ "_args": [
+ [
+ "os-homedir@^1.0.0",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/osenv"
+ ]
+ ],
+ "_from": "os-homedir@>=1.0.0 <2.0.0",
+ "_id": "os-homedir@1.0.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/os-homedir",
+ "_nodeVersion": "0.12.5",
+ "_npmUser": {
+ "email": "sindresorhus@gmail.com",
+ "name": "sindresorhus"
+ },
+ "_npmVersion": "2.11.2",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "os-homedir",
+ "raw": "os-homedir@^1.0.0",
+ "rawSpec": "^1.0.0",
+ "scope": null,
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/osenv"
+ ],
+ "_resolved": "http://registry.npmjs.org/os-homedir/-/os-homedir-1.0.1.tgz",
+ "_shasum": "0d62bdf44b916fd3bbdcf2cab191948fb094f007",
+ "_shrinkwrap": null,
+ "_spec": "os-homedir@^1.0.0",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/osenv",
+ "author": {
+ "email": "sindresorhus@gmail.com",
+ "name": "Sindre Sorhus",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/os-homedir/issues"
+ },
+ "dependencies": {},
+ "description": "io.js 2.3.0 os.homedir() ponyfill",
+ "devDependencies": {
+ "ava": "0.0.4",
+ "path-exists": "^1.0.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "0d62bdf44b916fd3bbdcf2cab191948fb094f007",
+ "tarball": "http://registry.npmjs.org/os-homedir/-/os-homedir-1.0.1.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "13ff83fbd13ebe286a6092286b2c634ab4534c5f",
+ "homepage": "https://github.com/sindresorhus/os-homedir",
+ "keywords": [
+ "built-in",
+ "core",
+ "dir",
+ "directory",
+ "folder",
+ "home",
+ "homedir",
+ "os",
+ "path",
+ "polyfill",
+ "ponyfill",
+ "shim",
+ "user"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "os-homedir",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/os-homedir.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "1.0.1"
+}
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[08/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/shopping.xml
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/shopping.xml b/node_modules/sax/examples/shopping.xml
new file mode 100644
index 0000000..223c6c6
--- /dev/null
+++ b/node_modules/sax/examples/shopping.xml
@@ -0,0 +1,2 @@
+
+<GeneralSearchResponse xmlns="urn:types.partner.api.shopping.com"><serverDetail><apiEnv>sandbox</apiEnv><apiVersion>3.1 r31.Kadu4DC.phase3</apiVersion><buildNumber>5778</buildNumber><buildTimestamp>2011.10.06 15:37:23 PST</buildTimestamp><requestId>p2.a121bc2aaf029435dce6</requestId><timestamp>2011-10-21T18:38:45.982-04:00</timestamp><responseTime>P0Y0M0DT0H0M0.169S</responseTime></serverDetail><exceptions exceptionCount="1"><exception type="warning"><code>1112</code><message>You are currently using the SDC API sandbox environment! No clicks to merchant URLs from this response will be paid. Please change the host of your API requests to 'publisher.api.shopping.com' when you have finished development and testing</message></exception></exceptions><clientTracking height="19" type="logo" width="106"><sourceURL>http://statTest.dealtime.com/pixel/noscript?PV_EvnTyp=APPV&APPV_APITSP=10%2F21%2F11_06%3A38%3A45_PM&APPV_DSPRQSID=p2.a121bc2aaf029435dce6&APPV_IMGURL=http://img.shop
ping.com/sc/glb/sdc_logo_106x19.gif&APPV_LI_LNKINID=7000610&APPV_LI_SBMKYW=nikon&APPV_MTCTYP=1000&APPV_PRTID=2002&APPV_BrnID=14804</sourceURL><hrefURL>http://www.shopping.com/digital-cameras/products</hrefURL><titleText>Digital Cameras</titleText><altText>Digital Cameras</altText></clientTracking><searchHistory><categorySelection id="3"><name>Electronics</name><categoryURL>http://www.shopping.com/xCH-electronics-nikon~linkin_id-7000610?oq=nikon</categoryURL></categorySelection><categorySelection id="449"><name>Cameras and Photography</name><categoryURL>http://www.shopping.com/xCH-cameras_and_photography-nikon~linkin_id-7000610?oq=nikon</categoryURL></categorySelection><categorySelection id="7185"><name>Digital Cameras</name><categoryURL>http://www.shopping.com/digital-cameras/nikon/products?oq=nikon&linkin_id=7000610</categoryURL></categorySelection><dynamicNavigationHistory><keywordSearch dropped="false" modified="false"><originalKeyword>nikon</originalKeywo
rd><resultKeyword>nikon</resultKeyword></keywordSearch></dynamicNavigationHistory></searchHistory><categories matchedCategoryCount="1" returnedCategoryCount="1"><category id="7185"><name>Digital Cameras</name><categoryURL>http://www.shopping.com/digital-cameras/nikon/products?oq=nikon&linkin_id=7000610</categoryURL><items matchedItemCount="322" pageNumber="1" returnedItemCount="5"><product id="101677489"><name>Nikon D3100 Digital Camera</name><shortDescription>14.2 Megapixel, SLR Camera, 3 in. LCD Screen, With High Definition Video, Weight: 1 lb.</shortDescription><fullDescription>The Nikon D3100 digital SLR camera speaks to the growing ranks of enthusiastic D-SLR users and aspiring photographers by providing an easy-to-use and affordable entrance to the world of Nikon D-SLR’s. The 14.2-megapixel D3100 has powerful features, such as the enhanced Guide Mode that makes it easy to unleash creative potential and capture memories with still images and full HD video. Like having a p
ersonal photo tutor at your fingertips, this unique feature provides a simple graphical interface on the camera’s LCD that guides users by suggesting and/or adjusting camera settings to achieve the desired end result images. The D3100 is also the world’s first D-SLR to introduce full time auto focus (AF) in Live View and D-Movie mode to effortlessly achieve the critical focus needed when shooting Full HD 1080p video.</fullDescription><images><image available="true" height="100" width="100"><sourceURL>http://di1.shopping.com/images/pi/93/bc/04/101677489-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di1.shopping.com/images/pi/93/bc/04/101677489-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di1.shopping.com/i
mages/pi/93/bc/04/101677489-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di1.shopping.com/images/pi/93/bc/04/101677489-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="500" width="606"><sourceURL>http://di1.shopping.com/images/pi/93/bc/04/101677489-606x500-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=1</sourceURL></image></images><rating><reviewCount>9</reviewCount><rating>4.56</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/pr/sdc_stars_sm_4.5.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/Nikon-D3100/reviews~linkin_id-7000610</reviewURL></rating><minPrice>429.00</minPrice><maxPrice>1360.00</maxPrice><productOffersURL>http://www.shopping.com/Nikon-D310
0/prices~linkin_id-7000610</productOffersURL><productSpecsURL>http://www.shopping.com/Nikon-D3100/info~linkin_id-7000610</productSpecsURL><offers matchedOfferCount="64" pageNumber="1" returnedOfferCount="5"><offer featured="false" id="-ZW6BMZqz6fbS-aULwga_g==" smartBuy="false" used="false"><name>Nikon D3100 Digital SLR Camera with 18-55mm NIKKOR VR Lens</name><description>The Nikon D3100 Digital SLR Camera is an affordable compact and lightweight photographic power-house. It features the all-purpose 18-55mm VR lens a high-resolution 14.2 MP CMOS sensor along with a feature set that's comprehensive yet easy to navigate - the intuitive onboard learn-as-you grow guide mode allows the photographer to understand what the 3100 can do quickly and easily. Capture beautiful pictures and amazing Full HD 1080p movies with sound and full-time autofocus. Availabilty: In Stock!</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" heigh
t="100" width="100"><sourceURL>http://di102.shopping.com/images/di/2d/5a/57/36424d5a717a366662532d61554c7767615f67-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di102.shopping.com/images/di/2d/5a/57/36424d5a717a366662532d61554c7767615f67-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di102.shopping.com/images/di/2d/5a/57/36424d5a717a366662532d61554c7767615f67-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" he
ight="350" width="350"><sourceURL>http://di102.shopping.com/images/di/2d/5a/57/36424d5a717a366662532d61554c7767615f67-350x350-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Free Shipping with Any Purchase!</storeNotes><basePrice currency="USD">529.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">799.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=647&BEFID=7185&aon=%5E1&MerchantID=475674&crawler_id=475674&dealId=-ZW6BMZqz6fbS-aULwga_g%3D%3D&url=http%3A%2F%2Fwww.fumfie.com%2Fproduct%2F343.5%2Fshopping-com%3F&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D3100+Digital+SLR+Camera+with+18-55mm+NIKKOR+VR+Lens&dlprc=529.0&crn=&istrsm
rc=1&isathrsl=0&AR=1&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=101677489&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=1&cid=&semid1=&semid2=&IsLps=0&CC=1&SL=1&FS=1&code=&acode=658&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="475674" trusted="true"><name>FumFie</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/475674.gif</sourceURL></logo><phoneNumber>866 666 9198</phoneNumber><ratingInfo><reviewCount>560</reviewCount><rating>4.27</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_45.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_fumfie~MRD-475674~S-1~linkin_id-7000610</reviewURL></ratingInfo><
countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>F343C5</sku></offer><offer featured="false" id="md1e9lD8vdOu4FHQfJqKng==" smartBuy="false" used="false"><name>Nikon Nikon D3100 14.2MP Digital SLR Camera with 18-55mm f/3.5-5.6 AF-S DX VR, Cameras</name><description>Nikon D3100 14.2MP Digital SLR Camera with 18-55mm f/3.5-5.6 AF-S DX VR</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di109.shopping.com/images/di/6d/64/31/65396c443876644f7534464851664a714b6e67-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di109.shopping.com/images/di/6d/64/31/65396c443876644f7534464851664a714b6e67-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&am
p;l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di109.shopping.com/images/di/6d/64/31/65396c443876644f7534464851664a714b6e67-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="352" width="385"><sourceURL>http://di109.shopping.com/images/di/6d/64/31/65396c443876644f7534464851664a714b6e67-385x352-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">549.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></tot
alPrice><originalPrice currency="USD">549.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=779&BEFID=7185&aon=%5E1&MerchantID=305814&crawler_id=305814&dealId=md1e9lD8vdOu4FHQfJqKng%3D%3D&url=http%3A%2F%2Fwww.electronics-expo.com%2Findex.php%3Fpage%3Ditem%26id%3DNIKD3100%26source%3DSideCar%26scpid%3D8%26scid%3Dscsho318727%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+Nikon+D3100+14.2MP+Digital+SLR+Camera+with+18-55mm+f%2F3.5-5.6+AF-S+DX+VR%2C+Cameras&dlprc=549.0&crn=&istrsmrc=1&isathrsl=0&AR=9&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=101677489&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=9&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=771&category=&HasLink=&frameId=&ND=
&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="305814" trusted="true"><name>Electronics Expo</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/305814.gif</sourceURL></logo><phoneNumber>1-888-707-EXPO</phoneNumber><ratingInfo><reviewCount>371</reviewCount><rating>3.90</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_4.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_electronics_expo~MRD-305814~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>NIKD3100</sku></offer><offer featured="false" id="yYuaXnDFtCY7rDUjkY2aaw==" smartBuy="false" used="false"><name>Nikon D3100 14.2-Megapixel Digital SLR Camera With 18-55mm Zoom-Nikkor Lens, Black</name><description>
Split-second shutter response captures shots other cameras may have missed Helps eliminate the frustration of shutter delay! 14.2-megapixels for enlargements worth framing and hanging. Takes breathtaking 1080p HD movies. ISO sensitivity from 100-1600 for bright or dimly lit settings. 3.0in. color LCD for beautiful, wide-angle framing and viewing. In-camera image editing lets you retouch with no PC. Automatic scene modes include Child, Sports, Night Portrait and more. Accepts SDHC memory cards. Nikon D3100 14.2-Megapixel Digital SLR Camera With 18-55mm Zoom-Nikkor Lens, Black is one of many Digital SLR Cameras available through Office Depot. Made by Nikon.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di109.shopping.com/images/di/79/59/75/61586e4446744359377244556a6b5932616177-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</so
urceURL></image><image available="true" height="200" width="200"><sourceURL>http://di109.shopping.com/images/di/79/59/75/61586e4446744359377244556a6b5932616177-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="250" width="250"><sourceURL>http://di109.shopping.com/images/di/79/59/75/61586e4446744359377244556a6b5932616177-250x250-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image></imageList><stockStatus>in-s
tock</stockStatus><basePrice currency="USD">549.99</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">699.99</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=698&BEFID=7185&aon=%5E1&MerchantID=467671&crawler_id=467671&dealId=yYuaXnDFtCY7rDUjkY2aaw%3D%3D&url=http%3A%2F%2Flink.mercent.com%2Fredirect.ashx%3Fmr%3AmerchantID%3DOfficeDepot%26mr%3AtrackingCode%3DCEC9669E-6ABC-E011-9F24-0019B9C043EB%26mr%3AtargetUrl%3Dhttp%3A%2F%2Fwww.officedepot.com%2Fa%2Fproducts%2F486292%2FNikon-D3100-142-Megapixel-Digital-SLR%2F%253fcm_mmc%253dMercent-_-Shopping-_-Cameras_and_Camcorders-_-486292&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D3100+14.2-Megapixel+Digital+SLR+Camera+With+18-55mm+Zoom-Nikkor+Lens%2C+Black&dlprc=549.99&crn=&istrsmrc=1&isathrsl=0&AR=10&N
G=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=101677489&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=10&cid=&semid1=&semid2=&IsLps=0&CC=1&SL=1&FS=1&code=&acode=690&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="467671" trusted="true"><name>Office Depot</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/467671.gif</sourceURL></logo><phoneNumber>1-800-GO-DEPOT</phoneNumber><ratingInfo><reviewCount>135</reviewCount><rating>2.37</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_office_depot_4158555~MRD-467671~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag
height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>486292</sku></offer><offer featured="false" id="Rl56U7CuiTYsH4MGZ02lxQ==" smartBuy="false" used="false"><name>Nikon® D3100™ 14.2MP Digital SLR with 18-55mm Lens</name><description>The Nikon D3100 DSLR will surprise you with its simplicity and impress you with superb results.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di103.shopping.com/images/di/52/6c/35/36553743756954597348344d475a30326c7851-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di103.shopping.com/images/di/52/6c/35/36553743756954597348344d475a30326c7851-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=11
1021183845&r=4</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="220" width="220"><sourceURL>http://di103.shopping.com/images/di/52/6c/35/36553743756954597348344d475a30326c7851-220x220-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">549.99</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">6.05</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">549.99</originalPrice><offerUR
L>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=504&BEFID=7185&aon=%5E1&MerchantID=332477&crawler_id=332477&dealId=Rl56U7CuiTYsH4MGZ02lxQ%3D%3D&url=http%3A%2F%2Ftracking.searchmarketing.com%2Fgsic.asp%3Faid%3D903483107%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon%C2%AE+D3100%E2%84%A2+14.2MP+Digital+SLR+with+18-55mm+Lens&dlprc=549.99&crn=&istrsmrc=0&isathrsl=0&AR=11&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=101677489&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=11&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=0&code=&acode=496&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="332477" trusted="false"><name>RadioShack</name><lo
go available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/332477.gif</sourceURL></logo><ratingInfo><reviewCount>24</reviewCount><rating>2.25</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_radioshack_9689~MRD-332477~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>9614867</sku></offer><offer featured="false" id="huS6xZKDKaKMTMP71eI6DA==" smartBuy="false" used="false"><name>Nikon D3100 SLR w/Nikon 18-55mm VR & 55-200mm VR Lenses</name><description>14.2 Megapixels3" LCDLive ViewHD 1080p Video w/ Sound & Autofocus11-point Autofocus3 Frames per Second ShootingISO 100 to 3200 (Expand to 12800-Hi2)Self Cleaning SensorEXPEED 2, Image Processing EngineScene Recognition S
ystem</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di105.shopping.com/images/di/68/75/53/36785a4b444b614b4d544d5037316549364441-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di105.shopping.com/images/di/68/75/53/36785a4b444b614b4d544d5037316549364441-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di105.shopping.com/images/di/68/75/53/36785a4b444b614b4d544d5037316549364441-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a
121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="345" width="345"><sourceURL>http://di105.shopping.com/images/di/68/75/53/36785a4b444b614b4d544d5037316549364441-345x345-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">695.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">695.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=371&BEFID=7185&aon=%5E1&MerchantID=487342&crawler_id=487342&dealId=huS6xZKDKaKMTMP71eI6DA%3D%3D&url=http%3A%2F%2Fwww.rythercamera.com%2Fcatalog%2Fproduct_info.php%3Fcsv%3Dsh%26products_id%3D32983%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&Dea
lName=Nikon+D3100+SLR+w%2FNikon+18-55mm+VR+%26+55-200mm+VR+Lenses&dlprc=695.0&crn=&istrsmrc=0&isathrsl=0&AR=15&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=101677489&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=15&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=379&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="487342" trusted="false"><name>RytherCamera.com</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/487342.gif</sourceURL></logo><phoneNumber>1-877-644-7593</phoneNumber><ratingInfo><reviewCount>0</reviewCount></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countr
yCode></countryFlag></store><sku>32983</sku></offer></offers></product><product id="95397883"><name>Nikon COOLPIX S203 Digital Camera</name><shortDescription>10 Megapixel, Ultra-Compact Camera, 2.5 in. LCD Screen, 3x Optical Zoom, With Video Capability, Weight: 0.23 lb.</shortDescription><fullDescription>With 10.34 mega pixel, electronic VR vibration reduction, 5-level brightness adjustment, 3x optical zoom, and TFT LCD, Nikon Coolpix s203 fulfills all the demands of any photographer. The digital camera has an inbuilt memory of 44MB and an external memory slot made for all kinds of SD (Secure Digital) cards.</fullDescription><images><image available="true" height="100" width="100"><sourceURL>http://di1.shopping.com/images/pi/c4/ef/1b/95397883-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di1.shopping.com/images/pi/c4/ef/1b/95397883-200x200-0-0.jp
g?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di1.shopping.com/images/pi/c4/ef/1b/95397883-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di1.shopping.com/images/pi/c4/ef/1b/95397883-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="499" width="500"><sourceURL>http://di1.shopping.com/images/pi/c4/ef/1b/95397883-500x499-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=2</sourceURL></image></images><rating><reviewCount>0</reviewCount></rating><minPrice>139.00</minPrice><maxPrice>139.00</maxPrice><productOffersURL>http://www.shopping.com/Nikon-Coolpix-S203/prices~linkin_id-7000610</productO
ffersURL><productSpecsURL>http://www.shopping.com/Nikon-Coolpix-S203/info~linkin_id-7000610</productSpecsURL><offers matchedOfferCount="1" pageNumber="1" returnedOfferCount="1"><offer featured="false" id="sBd2JnIEPM-A_lBAM1RZgQ==" smartBuy="false" used="false"><name>Nikon Coolpix S203 Digital Camera (Red)</name><description>With 10.34 mega pixel, electronic VR vibration reduction, 5-level brightness adjustment, 3x optical zoom, and TFT LCD, Nikon Coolpix s203 fulfills all the demands of any photographer. The digital camera has an inbuilt memory of 44MB and an external memory slot made for all kinds of SD (Secure Digital) cards.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di108.shopping.com/images/di/73/42/64/324a6e4945504d2d415f6c42414d31525a6751-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image avail
able="true" height="200" width="200"><sourceURL>http://di108.shopping.com/images/di/73/42/64/324a6e4945504d2d415f6c42414d31525a6751-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di108.shopping.com/images/di/73/42/64/324a6e4945504d2d415f6c42414d31525a6751-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di108.shopping.com/images/di/73/42/64/324a6e4945504d2d415f6c42414d31525a6751-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="500" width="500"><sourceURL>http://di108.shopping.com/images/di/73/42/64/324a6e4945504d2d415f6c42414d31525a6751-500x500-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=1
11021183845&r=1</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Fantastic prices with ease & comfort of Amazon.com!</storeNotes><basePrice currency="USD">139.00</basePrice><shippingCost currency="USD">9.50</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">139.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=566&BEFID=7185&aon=%5E1&MerchantID=301531&crawler_id=1903313&dealId=sBd2JnIEPM-A_lBAM1RZgQ%3D%3D&url=http%3A%2F%2Fwww.amazon.com%2Fdp%2FB002T964IM%2Fref%3Dasc_df_B002T964IM1751618%3Fsmid%3DA22UHVNXG98FAT%26tag%3Ddealtime-ce-mp01feed-20%26linkCode%3Dasn%26creative%3D395105%26creativeASIN%3DB002T964IM&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+Coolpix+S203+Digital+Camera+%28Red%29&dlprc=139.0&crn=&istrsmrc=0&isathrsl=0&AR=63&NG=20&NDP=200&PN=1&ST=7&DB=sdc
prod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=95397883&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=63&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=0&code=&acode=518&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="301531" trusted="false"><name>Amazon Marketplace</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/301531.gif</sourceURL></logo><ratingInfo><reviewCount>213</reviewCount><rating>2.73</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_amazon_marketplace_9689~MRD-301531~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif
</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>B002T964IM</sku></offer></offers></product><product id="106834268"><name>Nikon S3100 Digital Camera</name><shortDescription>14.5 Megapixel, Compact Camera, 2.7 in. LCD Screen, 5x Optical Zoom, With High Definition Video, Weight: 0.23 lb.</shortDescription><fullDescription>This digital camera features a wide-angle optical Zoom-NIKKOR glass lens that allows you to capture anything from landscapes to portraits to action shots. The high-definition movie mode with one-touch recording makes it easy to capture video clips.</fullDescription><images><image available="true" height="100" width="100"><sourceURL>http://di1.shopping.com/images/pi/66/2d/33/106834268-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di1.shopping.com/images/pi/66/2d/33/106834268-200x200-0-0.jpg?p=p2.a121bc2aaf029435d
ce6&a=2&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di1.shopping.com/images/pi/66/2d/33/106834268-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di1.shopping.com/images/pi/66/2d/33/106834268-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="387" width="507"><sourceURL>http://di1.shopping.com/images/pi/66/2d/33/106834268-507x387-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=3</sourceURL></image></images><rating><reviewCount>1</reviewCount><rating>2.00</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/pr/sdc_stars_sm_2.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/ni
kon-s3100/reviews~linkin_id-7000610</reviewURL></rating><minPrice>99.95</minPrice><maxPrice>134.95</maxPrice><productOffersURL>http://www.shopping.com/nikon-s3100/prices~linkin_id-7000610</productOffersURL><productSpecsURL>http://www.shopping.com/nikon-s3100/info~linkin_id-7000610</productSpecsURL><offers matchedOfferCount="67" pageNumber="1" returnedOfferCount="5"><offer featured="false" id="UUyGoqV8r0-xrkn-rnGNbg==" smartBuy="false" used="false"><name>CoolPix S3100 14 Megapixel Compact Digital Camera- Red</name><description>Nikon Coolpix S3100 - Digital camera - compact - 14.0 Mpix - optical zoom: 5 x - supported memory: SD, SDXC, SDHC - red</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di111.shopping.com/images/di/55/55/79/476f71563872302d78726b6e2d726e474e6267-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></im
age><image available="true" height="200" width="200"><sourceURL>http://di111.shopping.com/images/di/55/55/79/476f71563872302d78726b6e2d726e474e6267-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di111.shopping.com/images/di/55/55/79/476f71563872302d78726b6e2d726e474e6267-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di111.shopping.com/images/di/55/55/79/476f71563872302d78726b6e2d726e474e6267-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Get 30 days FREE SHIPPING w/ ShipVantage</storeNotes><basePrice currency="USD">119.95</basePrice><tax checkSite="true"></tax><shippingCost currency=
"USD">6.95</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">139.95</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=578&BEFID=7185&aon=%5E1&MerchantID=485615&crawler_id=485615&dealId=UUyGoqV8r0-xrkn-rnGNbg%3D%3D&url=http%3A%2F%2Fsears.rdr.channelintelligence.com%2Fgo.asp%3FfVhzOGNRAAQIASNiE1NbQBJpFHJ3Yx0CTAICI2BbH1lEFmgKP3QvUVpEREdlfUAUHAQPLVpFTVdtJzxAHUNYW3AhQBM0QhFvEXAbYh8EAAVmDAJeU1oyGG0GcBdhGwUGCAVqYF9SO0xSN1sZdmA7dmMdBQAJB24qX1NbQxI6AjA2ME5dVFULPDsGPFcQTTdaLTA6SR0OFlQvPAwMDxYcYlxIVkcoLTcCDA%3D%3D%26nAID%3D13736960%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=CoolPix+S3100+14+Megapixel+Compact+Digital+Camera-+Red&dlprc=119.95&crn=&istrsmrc=1&isathrsl=0&AR=28&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=106834268&brnId=14804&IsFtr=0&a
mp;IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=28&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=1&FS=0&code=&acode=583&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="485615" trusted="true"><name>Sears</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/485615.gif</sourceURL></logo><phoneNumber>1-800-349-4358</phoneNumber><ratingInfo><reviewCount>888</reviewCount><rating>2.85</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_3.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_sears_4189479~MRD-485615~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>00337013000</sku></offer>
<offer featured="false" id="X87AwXlW1dXoMXk4QQDToQ==" smartBuy="false" used="false"><name>COOLPIX S3100 Pink</name><description>Nikon Coolpix S3100 - Digital camera - compact - 14.0 Mpix - optical zoom: 5 x - supported memory: SD, SDXC, SDHC - pink</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di111.shopping.com/images/di/58/38/37/4177586c573164586f4d586b34515144546f51-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di111.shopping.com/images/di/58/38/37/4177586c573164586f4d586b34515144546f51-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di111.shopping.com/images/di/58/38/37/4177586c573164586f4d586b34515144
546f51-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di111.shopping.com/images/di/58/38/37/4177586c573164586f4d586b34515144546f51-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Get 30 days FREE SHIPPING w/ ShipVantage</storeNotes><basePrice currency="USD">119.95</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">6.95</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">139.95</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=578&BEFID=7185&aon=%5E1&MerchantID=485615&crawler_id=485615&dealId=X87AwXlW1dXoMXk4QQDToQ%3D%3D&url=http%3A%2F%2Fsears.rdr.channelintelligence.com%2Fgo.asp%3FfVhzOGNRAAQIASNiE1NbQBJpFHJxYx0CTAIC
I2BbH1lEFmgKP3QvUVpEREdlfUAUHAQPLVpFTVdtJzxAHUNYW3AhQBM0QhFvEXAbYh8EAAVmb2JcUFxDEGsPc3QDEkFZVQ0WFhdRW0MWbgYWDlxzdGMdAVQWRi0xDAwPFhw9TSobb05eWVVYKzsLTFVVQi5RICs3SA8MU1s2MQQKD1wf%26nAID%3D13736960%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=COOLPIX+S3100+Pink&dlprc=119.95&crn=&istrsmrc=1&isathrsl=0&AR=31&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=106834268&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=31&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=1&FS=0&code=&acode=583&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="485615" trusted="true"><name>Sears</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/485615.gif</
sourceURL></logo><phoneNumber>1-800-349-4358</phoneNumber><ratingInfo><reviewCount>888</reviewCount><rating>2.85</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_3.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_sears_4189479~MRD-485615~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>00337015000</sku></offer><offer featured="false" id="nvFwnpfA4rlA1Dbksdsa0w==" smartBuy="false" used="false"><name>Nikon Coolpix S3100 14.0 MP Digital Camera - Silver</name><description>Nikon Coolpix S3100 14.0 MP Digital Camera - Silver</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di109.shopping.com/images/di/6e/76/46/776e70664134726c413144626b736473613077-100x100-0-0.jpg
?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di109.shopping.com/images/di/6e/76/46/776e70664134726c413144626b736473613077-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="270" width="270"><sourceURL>http://di109.shopping.com/images/di/6e/76/46/776e70664134726c413144626b736473613077-270x270-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&am
p;l=7000610&t=111021183845&r=3</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">109.97</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">109.97</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=803&BEFID=7185&aon=%5E&MerchantID=475774&crawler_id=475774&dealId=nvFwnpfA4rlA1Dbksdsa0w%3D%3D&url=http%3A%2F%2Fwww.thewiz.com%2Fcatalog%2Fproduct.jsp%3FmodelNo%3DS3100SILVER%26gdftrk%3DgdfV2677_a_7c996_a_7c4049_a_7c26262&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+Coolpix+S3100+14.0+MP+Digital+Camera+-+Silver&dlprc=109.97&crn=&istrsmrc=0&isathrsl=0&AR=33&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=106834268&brnId=14804&Is
Ftr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=33&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=797&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="475774" trusted="false"><name>TheWiz.com</name><logo available="false" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/475774.gif</sourceURL></logo><phoneNumber>877-542-6988</phoneNumber><ratingInfo><reviewCount>0</reviewCount></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>26262</sku></offer><offer featured="false" id="5GtaN2NeryKwps-Se2l-4g==" smartBuy="false" used="false"><name>Nikon� COOLPIX� S3100 14MP Digital Camera (Silver)</name><description>The Nikon COOLPIX S3100 is the easy way to share your life and stay c
onnected.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di102.shopping.com/images/di/35/47/74/614e324e6572794b7770732d5365326c2d3467-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di102.shopping.com/images/di/35/47/74/614e324e6572794b7770732d5365326c2d3467-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=70
00610&t=111021183845&r=4</sourceURL></image><image available="true" height="220" width="220"><sourceURL>http://di102.shopping.com/images/di/35/47/74/614e324e6572794b7770732d5365326c2d3467-220x220-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">119.99</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">6.05</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">119.99</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=504&BEFID=7185&aon=%5E1&MerchantID=332477&crawler_id=332477&dealId=5GtaN2NeryKwps-Se2l-4g%3D%3D&url=http%3A%2F%2Ftracking.searchmarketing.com%2Fgsic.asp%3Faid%3D848064082%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon%C3%AF%C2%BF%C2%BD+COOLPIX%C3%AF%C2%BF%C2%BD+S3100+14MP+Di
gital+Camera+%28Silver%29&dlprc=119.99&crn=&istrsmrc=0&isathrsl=0&AR=37&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=106834268&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=37&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=0&code=&acode=509&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="332477" trusted="false"><name>RadioShack</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/332477.gif</sourceURL></logo><ratingInfo><reviewCount>24</reviewCount><rating>2.25</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_radioshack_9689~MRD-332477~S-1~linki
n_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>10101095</sku></offer><offer featured="false" id="a43m0RXulX38zCnQjU59jw==" smartBuy="false" used="false"><name>COOLPIX S3100 Yellow</name><description>Nikon Coolpix S3100 - Digital camera - compact - 14.0 Mpix - optical zoom: 5 x - supported memory: SD, SDXC, SDHC - yellow</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di107.shopping.com/images/di/61/34/33/6d305258756c5833387a436e516a5535396a77-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di107.shopping.com/images/di/61/34/33/6d305258756c5833387a436e516a5535396a77-200x200-0-0.jpg?p=p2.a121bc2aaf0294
35dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di107.shopping.com/images/di/61/34/33/6d305258756c5833387a436e516a5535396a77-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di107.shopping.com/images/di/61/34/33/6d305258756c5833387a436e516a5535396a77-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Get 30 days FREE SHIPPING w/ ShipVantage</storeNotes><basePrice currency="USD">119.95</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">6.95</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">139.95</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?b
m=578&BEFID=7185&aon=%5E1&MerchantID=485615&crawler_id=485615&dealId=a43m0RXulX38zCnQjU59jw%3D%3D&url=http%3A%2F%2Fsears.rdr.channelintelligence.com%2Fgo.asp%3FfVhzOGNRAAQIASNiE1NbQBJpFHJwYx0CTAICI2BbH1lEFmgKP3QvUVpEREdlfUAUHAQPLVpFTVdtJzxAHUNYW3AhQBM0QhFvEXAbYh8EAAVmb2JcUFxDEGoPc3QDEkFZVQ0WFhdRW0MWbgYWDlxzdGMdAVQWRi0xDAwPFhw9TSobb05eWVVYKzsLTFVVQi5RICs3SA8MU1s2MQQKD1wf%26nAID%3D13736960%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=COOLPIX+S3100+Yellow&dlprc=119.95&crn=&istrsmrc=1&isathrsl=0&AR=38&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=106834268&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=38&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=1&FS=0&code=&acode=583&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR
=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="485615" trusted="true"><name>Sears</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/485615.gif</sourceURL></logo><phoneNumber>1-800-349-4358</phoneNumber><ratingInfo><reviewCount>888</reviewCount><rating>2.85</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_3.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_sears_4189479~MRD-485615~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>00337014000</sku></offer></offers></product><product id="99671132"><name>Nikon D90 Digital Camera</name><shortDescription>12.3 Megapixel, Point and Shoot Camera, 3 in. LCD Screen, With Video Capability, Weight: 1.36 lb.</shortDescription><fullDescription>Untitle
d Document Nikon D90 SLR Digital Camera With 28-80mm 75-300mm Lens Kit The Nikon D90 SLR Digital Camera, with its 12.3-megapixel DX-format CMOS, 3" High resolution LCD display, Scene Recognition System, Picture Control, Active D-Lighting, and one-button Live View, provides photo enthusiasts with the image quality and performance they need to pursue their own vision while still being intuitive enough for use as an everyday camera.</fullDescription><images><image available="true" height="100" width="100"><sourceURL>http://di1.shopping.com/images/pi/52/fb/d3/99671132-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di1.shopping.com/images/pi/52/fb/d3/99671132-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di1.shoppin
g.com/images/pi/52/fb/d3/99671132-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di1.shopping.com/images/pi/52/fb/d3/99671132-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="255" width="499"><sourceURL>http://di1.shopping.com/images/pi/52/fb/d3/99671132-499x255-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=4</sourceURL></image></images><rating><reviewCount>7</reviewCount><rating>5.00</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/pr/sdc_stars_sm_5.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/Nikon-D90-with-18-270mm-Lens/reviews~linkin_id-7000610</reviewURL></rating><minPrice>689.00</minPrice><maxPrice>2299.00</maxPrice><productOffersURL>http://www.shop
ping.com/Nikon-D90-with-18-270mm-Lens/prices~linkin_id-7000610</productOffersURL><productSpecsURL>http://www.shopping.com/Nikon-D90-with-18-270mm-Lens/info~linkin_id-7000610</productSpecsURL><offers matchedOfferCount="43" pageNumber="1" returnedOfferCount="5"><offer featured="false" id="GU5JJkpUAxe5HujB7fkwAA==" smartBuy="false" used="false"><name>Nikon® D90 12.3MP Digital SLR Camera (Body Only)</name><description>The Nikon D90 will make you rethink what a digital SLR camera can achieve.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di106.shopping.com/images/di/47/55/35/4a4a6b70554178653548756a4237666b774141-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di106.shopping.com/images/di/47/55/35/4a4a6b70554178653548756a4237666b774141-200x200-0-
0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="220" width="220"><sourceURL>http://di106.shopping.com/images/di/47/55/35/4a4a6b70554178653548756a4237666b774141-220x220-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">1015.99</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">6.05</shippingCost><totalPrice checkSite="true"></
totalPrice><originalPrice currency="USD">1015.99</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=504&BEFID=7185&aon=%5E1&MerchantID=332477&crawler_id=332477&dealId=GU5JJkpUAxe5HujB7fkwAA%3D%3D&url=http%3A%2F%2Ftracking.searchmarketing.com%2Fgsic.asp%3Faid%3D851830266%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon%C2%AE+D90+12.3MP+Digital+SLR+Camera+%28Body+Only%29&dlprc=1015.99&crn=&istrsmrc=0&isathrsl=0&AR=14&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=99671132&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=14&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=0&code=&acode=496&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedResel
ler="false" id="332477" trusted="false"><name>RadioShack</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/332477.gif</sourceURL></logo><ratingInfo><reviewCount>24</reviewCount><rating>2.25</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_radioshack_9689~MRD-332477~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>10148659</sku></offer><offer featured="false" id="XhURuSC-spBbTIDfo4qfzQ==" smartBuy="false" used="false"><name>Nikon D90 SLR Digital Camera (Camera Body)</name><description>The Nikon D90 SLR Digital Camera with its 12.3-megapixel DX-format CCD 3" High resolution LCD display Scene Recognition System Picture Control Active D-Lighting and one
-button Live View provides photo enthusiasts with the image quality and performance they need to pursue their own vision while still being intuitive enough for use as an everyday camera. In addition the D90 introduces the D-Movie mode allowing for the first time an interchangeable lens SLR camera that is capable of recording 720p HD movie clips. Availabilty: In Stock</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di109.shopping.com/images/di/58/68/55/527553432d73704262544944666f3471667a51-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di109.shopping.com/images/di/58/68/55/527553432d73704262544944666f3471667a51-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image avai
lable="true" height="300" width="300"><sourceURL>http://di109.shopping.com/images/di/58/68/55/527553432d73704262544944666f3471667a51-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="350" width="350"><sourceURL>http://di109.shopping.com/images/di/58/68/55/527553432d73704262544944666f3471667a51-350x350-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Free Shipping with Any Purchase!</storeNotes><basePrice currency="USD">689.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><ori
ginalPrice currency="USD">900.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=647&BEFID=7185&aon=%5E1&MerchantID=475674&crawler_id=475674&dealId=XhURuSC-spBbTIDfo4qfzQ%3D%3D&url=http%3A%2F%2Fwww.fumfie.com%2Fproduct%2F169.5%2Fshopping-com%3F&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D90+SLR+Digital+Camera+%28Camera+Body%29&dlprc=689.0&crn=&istrsmrc=1&isathrsl=0&AR=16&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=99671132&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=16&cid=&semid1=&semid2=&IsLps=0&CC=1&SL=1&FS=1&code=&acode=658&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="475674" trusted="true">
<name>FumFie</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/475674.gif</sourceURL></logo><phoneNumber>866 666 9198</phoneNumber><ratingInfo><reviewCount>560</reviewCount><rating>4.27</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_45.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_fumfie~MRD-475674~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>F169C5</sku></offer><offer featured="false" id="o0Px_XLWDbrxAYRy3rCmyQ==" smartBuy="false" used="false"><name>Nikon D90 SLR w/Nikon 18-105mm VR & 55-200mm VR Lenses</name><description>12.3 MegapixelDX Format CMOS Sensor3" VGA LCD DisplayLive ViewSelf Cleaning SensorD-Movie ModeHigh Sensitivity (ISO 3200)4.5 fps BurstIn-Camera Image Editing</description><c
ategoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di101.shopping.com/images/di/6f/30/50/785f584c5744627278415952793372436d7951-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di101.shopping.com/images/di/6f/30/50/785f584c5744627278415952793372436d7951-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di101.shopping.com/images/di/6f/30/50/785f584c5744627278415952793372436d7951-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di101.shopping.com/images/di/6f/30/50/785f584c5744627278415952793372436d
7951-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="500" width="500"><sourceURL>http://di101.shopping.com/images/di/6f/30/50/785f584c5744627278415952793372436d7951-500x500-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">1189.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">1189.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=371&BEFID=7185&aon=%5E1&MerchantID=487342&crawler_id=487342&dealId=o0Px_XLWDbrxAYRy3rCmyQ%3D%3D&url=http%3A%2F%2Fwww.rythercamera.com%2Fcatalog%2Fproduct_info.php%3Fcsv%3Dsh%26products_id%3D30619%26&linkin_id=7000610&Issdt=111021183845&searchID=p2
.a121bc2aaf029435dce6&DealName=Nikon+D90+SLR+w%2FNikon+18-105mm+VR+%26+55-200mm+VR+Lenses&dlprc=1189.0&crn=&istrsmrc=0&isathrsl=0&AR=20&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=99671132&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=20&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=379&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="487342" trusted="false"><name>RytherCamera.com</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/487342.gif</sourceURL></logo><phoneNumber>1-877-644-7593</phoneNumber><ratingInfo><reviewCount>0</reviewCount></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourc
eURL><countryCode>US</countryCode></countryFlag></store><sku>30619</sku></offer><offer featured="false" id="4HgbWJSJ8ssgIf8B0MXIwA==" smartBuy="false" used="false"><name>Nikon D90 12.3 Megapixel Digital SLR Camera (Body Only)</name><description>Fusing 12.3 megapixel image quality and a cinematic 24fps D-Movie Mode, the Nikon D90 exceeds the demands of passionate photographers. Coupled with Nikon's EXPEED image processing technologies and NIKKOR optics, breathtaking image fidelity is assured. Combined with fast 0.15ms power-up and split-second 65ms shooting lag, dramatic action and decisive moments are captured easily. Effective 4-frequency, ultrasonic sensor cleaning frees image degrading dust particles from the sensor's optical low pass filter.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di110.shopping.com/images/di/34/48/67/62574a534a3873736749663842304d58497741-100x100-0-
0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di110.shopping.com/images/di/34/48/67/62574a534a3873736749663842304d58497741-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di110.shopping.com/images/di/34/48/67/62574a534a3873736749663842304d58497741-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>FREE FEDEX 2-3 DAY DELIVERY</storeNotes><basePrice currency="USD">899.95</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">899.95</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/Dea
lFrame.cmp?bm=269&BEFID=7185&aon=%5E&MerchantID=9296&crawler_id=811558&dealId=4HgbWJSJ8ssgIf8B0MXIwA%3D%3D&url=http%3A%2F%2Fwww.pcnation.com%2Foptics-gallery%2Fdetails.asp%3Faffid%3D305%26item%3D2N145P&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D90+12.3+Megapixel+Digital+SLR+Camera+%28Body+Only%29&dlprc=899.95&crn=&istrsmrc=1&isathrsl=0&AR=21&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=99671132&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=21&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=257&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="9296" trusted="true"><name>PCNation</name><logo available="true" height="31" width="8
8"><sourceURL>http://img.shopping.com/cctool/merch_logos/9296.gif</sourceURL></logo><phoneNumber>800-470-7079</phoneNumber><ratingInfo><reviewCount>1622</reviewCount><rating>4.43</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_45.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_pcnation_9689~MRD-9296~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>2N145P</sku></offer><offer featured="false" id="UNDa3uMDZXOnvD_7sTILYg==" smartBuy="false" used="false"><name>Nikon D90 12.3MP Digital SLR Camera (Body Only)</name><description>Fusing 12.3-megapixel image quality inherited from the award-winning D300 with groundbreaking features, the D90's breathtaking, low-noise image quality is further advanced with EXPEED image processing. Split-second shutter response and contin
uous shooting at up to 4.5 frames-per-second provide the power to capture fast action and precise moments perfectly, while Nikon's exclusive Scene Recognition System contributes to faster 11-area autofocus performance, finer white balance detection and more. The D90 delivers the control passionate photographers demand, utilizing comprehensive exposure functions and the intelligence of 3D Color Matrix Metering II. Stunning results come to life on a 3-inch 920,000-dot color LCD monitor, providing accurate image review, Live View composition and brilliant playback of the D90's cinematic-quality 24-fps HD D-Movie mode.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di102.shopping.com/images/di/55/4e/44/6133754d445a584f6e76445f377354494c5967-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" h
eight="200" width="200"><sourceURL>http://di102.shopping.com/images/di/55/4e/44/6133754d445a584f6e76445f377354494c5967-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di102.shopping.com/images/di/55/4e/44/6133754d445a584f6e76445f377354494c5967-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di102.shopping.com/images/di/55/4e/44/6133754d445a584f6e76445f377354494c5967-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="500" width="500"><sourceURL>http://di102.shopping.com/images/di/55/4e/44/6133754d445a584f6e76445f377354494c5967-500x500-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&a
mp;r=5</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Fantastic prices with ease & comfort of Amazon.com!</storeNotes><basePrice currency="USD">780.00</basePrice><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">780.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=566&BEFID=7185&aon=%5E1&MerchantID=301531&crawler_id=1903313&dealId=UNDa3uMDZXOnvD_7sTILYg%3D%3D&url=http%3A%2F%2Fwww.amazon.com%2Fdp%2FB001ET5U92%2Fref%3Dasc_df_B001ET5U921751618%3Fsmid%3DAHF4SYKP09WBH%26tag%3Ddealtime-ce-mp01feed-20%26linkCode%3Dasn%26creative%3D395105%26creativeASIN%3DB001ET5U92&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D90+12.3MP+Digital+SLR+Camera+%28Body+Only%29&dlprc=780.0&crn=&istrsmrc=0&isathrsl=0&AR=29&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&a
mp;MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=99671132&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=29&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=520&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="301531" trusted="false"><name>Amazon Marketplace</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/301531.gif</sourceURL></logo><ratingInfo><reviewCount>213</reviewCount><rating>2.73</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_amazon_marketplace_9689~MRD-301531~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sour
ceURL><countryCode>US</countryCode></countryFlag></store><sku>B001ET5U92</sku></offer></offers></product><product id="70621646"><name>Nikon D90 Digital Camera with 18-105mm lens</name><shortDescription>12.9 Megapixel, SLR Camera, 3 in. LCD Screen, 5.8x Optical Zoom, With Video Capability, Weight: 2.3 lb.</shortDescription><fullDescription>Its 12.3 megapixel DX-format CMOS image sensor and EXPEED image processing system offer outstanding image quality across a wide ISO light sensitivity range. Live View mode lets you compose and shoot via the high-resolution 3-inch LCD monitor, and an advanced Scene Recognition System and autofocus performance help capture images with astounding accuracy. Movies can be shot in Motion JPEG format using the D-Movie function. The camera’s large image sensor ensures exceptional movie image quality and you can create dramatic effects by shooting with a wide range of interchangeable NIKKOR lenses, from wide-angle to macro to fisheye, or by adjusting the
lens aperture and experimenting with depth-of-field. The D90 – designed to fuel your passion for photography.</fullDescription><images><image available="true" height="100" width="100"><sourceURL>http://di1.shopping.com/images/pi/57/6a/4f/70621646-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di1.shopping.com/images/pi/57/6a/4f/70621646-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di1.shopping.com/images/pi/57/6a/4f/70621646-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di1.shopping.com/images/pi/57/6a/4f/70621646-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&
amp;l=7000610&t=111021183845&r=5</sourceURL></image><image available="true" height="489" width="490"><sourceURL>http://di1.shopping.com/images/pi/57/6a/4f/70621646-490x489-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=2&c=1&l=7000610&t=111021183845&r=5</sourceURL></image></images><rating><reviewCount>32</reviewCount><rating>4.81</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/pr/sdc_stars_sm_5.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/Nikon-D90-with-18-105mm-lens/reviews~linkin_id-7000610</reviewURL></rating><minPrice>849.95</minPrice><maxPrice>1599.95</maxPrice><productOffersURL>http://www.shopping.com/Nikon-D90-with-18-105mm-lens/prices~linkin_id-7000610</productOffersURL><productSpecsURL>http://www.shopping.com/Nikon-D90-with-18-105mm-lens/info~linkin_id-7000610</productSpecsURL><offers matchedOfferCount="25" pageNumber="1" returnedOfferCount="5"><offer featured="false" id="3o5e1VghgJPfhLvT1JFKTA==" smartBu
y="false" used="false"><name>Nikon D90 18-105mm VR Lens</name><description>The Nikon D90 SLR Digital Camera with its 12.3-megapixel DX-format CMOS 3" High resolution LCD display Scene Recognition System Picture Control Active D-Lighting and one-button Live View prov</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di111.shopping.com/images/di/33/6f/35/6531566768674a5066684c7654314a464b5441-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di111.shopping.com/images/di/33/6f/35/6531566768674a5066684c7654314a464b5441-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?
p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image><image available="true" height="260" width="260"><sourceURL>http://di111.shopping.com/images/di/33/6f/35/6531566768674a5066684c7654314a464b5441-260x260-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=1</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">849.95</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">849.95</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=419&BEFID=7185&aon=%5E1&MerchantID=9390&crawler_id=1905054&dealId=3o5e1V
ghgJPfhLvT1JFKTA%3D%3D&url=http%3A%2F%2Fwww.ajrichard.com%2FNikon-D90-18-105mm-VR-Lens%2Fp-292%3Frefid%3DShopping%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D90+18-105mm+VR+Lens&dlprc=849.95&crn=&istrsmrc=0&isathrsl=0&AR=2&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=70621646&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=2&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=425&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="9390" trusted="false"><name>AJRichard</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/9390.gif</sourceURL></logo><phoneNumber>1-888-871-1256</phoneNumber><ratingInfo><
reviewCount>3124</reviewCount><rating>4.48</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_45.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_ajrichard~MRD-9390~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>292</sku></offer><offer featured="false" id="_lYWj_jbwfsSkfcwUcDuww==" smartBuy="false" used="false"><name>Nikon D90 SLR w/Nikon 18-105mm VR Lens</name><description>12.3 MegapixelDX Format CMOS Sensor3" VGA LCD DisplayLive ViewSelf Cleaning SensorD-Movie ModeHigh Sensitivity (ISO 3200)4.5 fps BurstIn-Camera Image Editing</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di108.shopping.com/images/di/5f/6c/59/576a5f6a62776673536b666377556344757777-100x1
00-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di108.shopping.com/images/di/5f/6c/59/576a5f6a62776673536b666377556344757777-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di108.shopping.com/images/di/5f/6c/59/576a5f6a62776673536b666377556344757777-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di108.shopping.com/images/di/5f/6c/59/576a5f6a62776673536b666377556344757777-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image><image available="true" height="500" width="500"><sourceURL>http://di108.shopping.com/images/di/5f
/6c/59/576a5f6a62776673536b666377556344757777-500x500-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=2</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">909.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">0.00</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">909.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=371&BEFID=7185&aon=%5E1&MerchantID=487342&crawler_id=487342&dealId=_lYWj_jbwfsSkfcwUcDuww%3D%3D&url=http%3A%2F%2Fwww.rythercamera.com%2Fcatalog%2Fproduct_info.php%3Fcsv%3Dsh%26products_id%3D30971%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon+D90+SLR+w%2FNikon+18-105mm+VR+Lens&dlprc=909.0&crn=&istrsmrc=0&isathrsl=0&AR=3&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=
DSP&NDS=&NMS=&MRS=&PD=70621646&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=3&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=1&code=&acode=379&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="487342" trusted="false"><name>RytherCamera.com</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/487342.gif</sourceURL></logo><phoneNumber>1-877-644-7593</phoneNumber><ratingInfo><reviewCount>0</reviewCount></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>30971</sku></offer><offer featured="false" id="1KCclCGuWvty2XKU9skadg==" smartBuy="false" used="false"><name>25448/D90 12.3 Megapixel Digital Camera 18-105mm Zoom Lens w/ 3" Screen -
Black</name><description>Nikon D90 - Digital camera - SLR - 12.3 Mpix - Nikon AF-S DX 18-105mm lens - optical zoom: 5.8 x - supported memory: SD, SDHC</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di110.shopping.com/images/di/31/4b/43/636c4347755776747932584b5539736b616467-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di110.shopping.com/images/di/31/4b/43/636c4347755776747932584b5539736b616467-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image><image available="true" height="300" width="300"><sourceURL>http://di110.shopping.com/images/di/31/4b/43/636c4347755776747932584b5539736b616467-300x300-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&
amp;r=3</sourceURL></image><image available="true" height="400" width="400"><sourceURL>http://di110.shopping.com/images/di/31/4b/43/636c4347755776747932584b5539736b616467-400x400-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=3</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><storeNotes>Get 30 days FREE SHIPPING w/ ShipVantage</storeNotes><basePrice currency="USD">1199.00</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">8.20</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">1199.00</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=578&BEFID=7185&aon=%5E1&MerchantID=485615&crawler_id=485615&dealId=1KCclCGuWvty2XKU9skadg%3D%3D&url=http%3A%2F%2Fsears.rdr.channelintelligence.com%2Fgo.asp%3FfVhzOGNRAAQIASNiE1NbQBRtFXpzYx0CTAICI2BbH1lEFmgKP3QvUVpEREdlfUAUHAQPLVpFTVdtJzxAHUNYW3AhQBM0QhFvEXAbYh8EAAVmb2JcVlhCGGkPc3QDEkFZVQ0W
FhdRW0MWbgYWDlxzdGMdAVQWRi0xDAwPFhw9TSobb05eWVVYKzsLTFVVQi5RICs3SA8MU1s2MQQKD1wf%26nAID%3D13736960%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=25448%2FD90+12.3+Megapixel+Digital+Camera+18-105mm+Zoom+Lens+w%2F+3%22+Screen+-+Black&dlprc=1199.0&crn=&istrsmrc=1&isathrsl=0&AR=4&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=70621646&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1&RR=4&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=0&code=&acode=586&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="485615" trusted="true"><name>Sears</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/485615.gif</sourceURL></logo><phoneNumber>1-
800-349-4358</phoneNumber><ratingInfo><reviewCount>888</reviewCount><rating>2.85</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_3.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_sears_4189479~MRD-485615~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>00353197000</sku></offer><offer featured="false" id="3-VOSfVV5Jo7HlA4kJtanA==" smartBuy="false" used="false"><name>Nikon® D90 12.3MP Digital SLR with 18-105mm Lens</name><description>The Nikon D90 will make you rethink what a digital SLR camera can achieve.</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di101.shopping.com/images/di/33/2d/56/4f53665656354a6f37486c41346b4a74616e41-100x100-0-0.jpg?p=p2.a121b
c2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="200" width="200"><sourceURL>http://di101.shopping.com/images/di/33/2d/56/4f53665656354a6f37486c41346b4a74616e41-200x200-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="false" height="300" width="300"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="false" height="400" width="400"><sourceURL>http://img.shopping.com/sc/ds/no_image_100X100.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610&t=111021183845&r=4</sourceURL></image><image available="true" height="220" width="220"><sourceURL>http://di101.shopping.com/images/di/33/2d/56/4f53665656354a6f37486c41346b4a74616e41-220x220-0-0.jpg?p=p2.a121bc2aaf029435dce6&a=1&c=1&l=7000610
&t=111021183845&r=4</sourceURL></image></imageList><stockStatus>in-stock</stockStatus><basePrice currency="USD">1350.99</basePrice><tax checkSite="true"></tax><shippingCost currency="USD">6.05</shippingCost><totalPrice checkSite="true"></totalPrice><originalPrice currency="USD">1350.99</originalPrice><offerURL>http://statTest.dealtime.com/DealFrame/DealFrame.cmp?bm=504&BEFID=7185&aon=%5E1&MerchantID=332477&crawler_id=332477&dealId=3-VOSfVV5Jo7HlA4kJtanA%3D%3D&url=http%3A%2F%2Ftracking.searchmarketing.com%2Fgsic.asp%3Faid%3D982673361%26&linkin_id=7000610&Issdt=111021183845&searchID=p2.a121bc2aaf029435dce6&DealName=Nikon%C2%AE+D90+12.3MP+Digital+SLR+with+18-105mm+Lens&dlprc=1350.99&crn=&istrsmrc=0&isathrsl=0&AR=5&NG=20&NDP=200&PN=1&ST=7&DB=sdcprod&MT=phx-pkadudc2&FPT=DSP&NDS=&NMS=&MRS=&PD=70621646&brnId=14804&IsFtr=0&IsSmart=0&DMT=&op=&CM=&DlLng=1
&RR=5&cid=&semid1=&semid2=&IsLps=0&CC=0&SL=0&FS=0&code=&acode=496&category=&HasLink=&frameId=&ND=&MN=&PT=&prjID=&GR=&lnkId=&VK=</offerURL><store authorizedReseller="false" id="332477" trusted="false"><name>RadioShack</name><logo available="true" height="31" width="88"><sourceURL>http://img.shopping.com/cctool/merch_logos/332477.gif</sourceURL></logo><ratingInfo><reviewCount>24</reviewCount><rating>2.25</rating><ratingImage height="18" width="91"><sourceURL>http://img.shopping.com/sc/mr/sdc_checks_25.gif</sourceURL></ratingImage><reviewURL>http://www.shopping.com/xMR-store_radioshack_9689~MRD-332477~S-1~linkin_id-7000610</reviewURL></ratingInfo><countryFlag height="11" width="18"><sourceURL>http://img.shopping.com/sc/glb/flag/US.gif</sourceURL><countryCode>US</countryCode></countryFlag></store><sku>11148905</sku></offer><offer featured="false" id="kQnB6rS4AjN5dx5h2_631g==" smartBuy="false" used="false"><n
ame>Nikon D90 Kit 12.3-megapixel Digital SLR with 18-105mm VR Lens</name><description>Photographers, take your passion further!Now is the time for new creativity, and to rethink what a digital SLR camera can achieve. It's time for the D90, a camera with everything you would expect from Nikon's next-generation D-SLRs, and some unexpected surprises, as well. The stunning image quality is inherited from the D300, Nikon's DX-format flagship. The D90 also has Nikon's unmatched ergonomics and high performance, and now takes high-quality movies with beautifully cinematic results. The world of photography has changed, and with the D90 in your hands, it's time to make your own rules.AF-S DX NIKKOR 18-105mm f/3.5-5.6G ED VR LensWide-ratio 5.8x zoom Compact, versatile and ideal for a broad range of shooting situations, ranging from interiors and landscapes to beautiful portraits� a perfect everyday zoom. Nikon VR (Vibration Reduction) image stabilization Vibration Reduction is engineered
specifically for each VR NIKKOR lens and enables handheld shooting at up to 3 shutter speeds slower than would</description><categoryId>7185</categoryId><manufacturer>Nikon</manufacturer><imageList><image available="true" height="100" width="100"><sourceURL>http://di110.shopping.com/images/di/6b/51/6e/4236725334416a4e3564783568325f36333167-100x100-0-0.jpg?p=p2.a121bc2aaf029435dce6&a
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[16/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/package.json
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/package.json b/node_modules/pegjs/package.json
new file mode 100644
index 0000000..4a997fc
--- /dev/null
+++ b/node_modules/pegjs/package.json
@@ -0,0 +1,82 @@
+{
+ "_args": [
+ [
+ "pegjs@0.6.2",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/xcode"
+ ]
+ ],
+ "_defaultsLoaded": true,
+ "_engineSupported": true,
+ "_from": "pegjs@0.6.2",
+ "_id": "pegjs@0.6.2",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/pegjs",
+ "_nodeVersion": "v0.4.8",
+ "_npmJsonOpts": {
+ "contributors": false,
+ "file": "/home/dmajda/.npm/pegjs/0.6.2/package/package.json",
+ "serverjs": false,
+ "wscript": false
+ },
+ "_npmVersion": "1.0.15",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "pegjs",
+ "raw": "pegjs@0.6.2",
+ "rawSpec": "0.6.2",
+ "scope": null,
+ "spec": "0.6.2",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/xcode"
+ ],
+ "_resolved": "http://registry.npmjs.org/pegjs/-/pegjs-0.6.2.tgz",
+ "_shasum": "74651f8a800e444db688e4eeae8edb65637a17a5",
+ "_shrinkwrap": null,
+ "_spec": "pegjs@0.6.2",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/xcode",
+ "author": {
+ "email": "david@majda.cz",
+ "name": "David Majda",
+ "url": "http://majda.cz/"
+ },
+ "bin": {
+ "pegjs": "bin/pegjs"
+ },
+ "bugs": {
+ "url": "https://github.com/dmajda/pegjs/issues"
+ },
+ "dependencies": {},
+ "description": "Parser generator for JavaScript",
+ "devDependencies": {
+ "jake": ">= 0.1.10",
+ "uglify-js": ">= 0.0.5"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "74651f8a800e444db688e4eeae8edb65637a17a5",
+ "tarball": "http://registry.npmjs.org/pegjs/-/pegjs-0.6.2.tgz"
+ },
+ "engines": {
+ "node": ">= 0.4.4"
+ },
+ "homepage": "http://pegjs.majda.cz/",
+ "main": "lib/peg",
+ "maintainers": [
+ {
+ "name": "dmajda",
+ "email": "david@majda.cz"
+ }
+ ],
+ "name": "pegjs",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/dmajda/pegjs.git"
+ },
+ "scripts": {},
+ "version": "0.6.2"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/plist/.jshintrc b/node_modules/plist/.jshintrc
new file mode 100644
index 0000000..3f42622
--- /dev/null
+++ b/node_modules/plist/.jshintrc
@@ -0,0 +1,4 @@
+{
+ "laxbreak": true,
+ "laxcomma": true
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/plist/.travis.yml b/node_modules/plist/.travis.yml
new file mode 100644
index 0000000..f82fbdc
--- /dev/null
+++ b/node_modules/plist/.travis.yml
@@ -0,0 +1,34 @@
+language: node_js
+node_js:
+- '0.10'
+- '0.11'
+- '4.0'
+- '4.1'
+env:
+ global:
+ - secure: xlLmWO7akYQjmDgrv6/b/ZMGILF8FReD+k6A/u8pYRD2JW29hhwvRwIQGcKp9+zmJdn4i5M4D1/qJkCeI3pdhAYBDHvzHOHSEwLJz1ESB2Crv6fa69CtpIufQkWvIxmZoU49tCaLpMBaIroGihJ4DAXdIVOIz6Ur9vXLDhGsE4c=
+ - secure: aQ46RdxL10xR5ZJJTMUKdH5k4tdrzgZ87nlwHC+pTr6bfRw3UKYC+6Rm7yQpg9wq0Io9O9dYCP007gQGSWstbjr1+jXNu/ubtNG+q5cpWBQZZZ013VHh9QJTf1MnetsZxbv8Yhrjg590s6vruT0oqesOnB2CizO/BsKxnY37Nos=
+matrix:
+ include:
+ - node_js: '0.10'
+ env: BROWSER_NAME=chrome BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=chrome BROWSER_VERSION=29
+ - node_js: '0.10'
+ env: BROWSER_NAME=firefox BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=opera BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=7
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=6
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=5
+ - node_js: '0.10'
+ env: BROWSER_NAME=ie BROWSER_VERSION=11
+ - node_js: '0.10'
+ env: BROWSER_NAME=ie BROWSER_VERSION=10
+ - node_js: '0.10'
+ env: BROWSER_NAME=ie BROWSER_VERSION=9
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/History.md
----------------------------------------------------------------------
diff --git a/node_modules/plist/History.md b/node_modules/plist/History.md
new file mode 100644
index 0000000..73f36ae
--- /dev/null
+++ b/node_modules/plist/History.md
@@ -0,0 +1,122 @@
+1.2.0 / 2015-11-10
+
+* package: update "browserify" to v12.0.1
+* package: update "zuul" to v3.7.2
+* package: update "xmlbuilder" to v4.0.0
+* package: update "util-deprecate" to v1.0.2
+* package: update "mocha" to v2.3.3
+* package: update "base64-js" to v0.0.8
+* build: omit undefined values
+* travis: add node 4.0 and 4.1 to test matrix
+
+1.1.0 / 2014-08-27
+==================
+
+ * package: update "browserify" to v5.10.1
+ * package: update "zuul" to v1.10.2
+ * README: add "Sauce Test Status" build badge
+ * travis: use new "plistjs" sauce credentials
+ * travis: set up zuul saucelabs automated testing
+
+1.0.1 / 2014-06-25
+==================
+
+ * add .zuul.yml file for browser testing
+ * remove Testling stuff
+ * build: fix global variable `val` leak
+ * package: use --check-leaks when running mocha tests
+ * README: update examples to use preferred API
+ * package: add "browser" keyword
+
+1.0.0 / 2014-05-20
+==================
+
+ * package: remove "android-browser"
+ * test: add <dict> build() test
+ * test: re-add the empty string build() test
+ * test: remove "fixtures" and legacy "tests" dir
+ * test: add some more build() tests
+ * test: add a parse() CDATA test
+ * test: starting on build() tests
+ * test: more parse() tests
+ * package: attempt to fix "android-browser" testling
+ * parse: better <data> with newline handling
+ * README: add Testling badge
+ * test: add <data> node tests
+ * test: add a <date> parse() test
+ * travis: don't test node v0.6 or v0.8
+ * test: some more parse() tests
+ * test: add simple <string> parsing test
+ * build: add support for an optional "opts" object
+ * package: test mobile devices
+ * test: use multiline to inline the XML
+ * package: beautify
+ * package: fix "mocha" harness
+ * package: more testling browsers
+ * build: add the "version=1.0" attribute
+ * beginnings of "mocha" tests
+ * build: more JSDocs
+ * tests: add test that ensures that empty string conversion works
+ * build: update "xmlbuilder" to v2.2.1
+ * parse: ignore comment and cdata nodes
+ * tests: make the "Newlines" test actually contain a newline
+ * parse: lint
+ * test travis
+ * README: add Travis CI badge
+ * add .travis.yml file
+ * build: updated DTD to reflect name change
+ * parse: return falsey values in an Array plist
+ * build: fix encoding a typed array in the browser
+ * build: add support for Typed Arrays and ArrayBuffers
+ * build: more lint
+ * build: slight cleanup and optimizations
+ * build: use .txt() for the "date" value
+ * parse: always return a Buffer for <data> nodes
+ * build: don't interpret Strings as base64
+ * dist: commit prebuilt plist*.js files
+ * parse: fix typo in deprecate message
+ * parse: fix parse() return value
+ * parse: add jsdoc comments for the deprecated APIs
+ * parse: add `parse()` function
+ * node, parse: use `util-deprecate` module
+ * re-implemented parseFile to be asynchronous
+ * node: fix jsdoc comment
+ * Makefile: fix "node" require stubbing
+ * examples: add "browser" example
+ * package: tweak "main"
+ * package: remove "engines" field
+ * Makefile: fix --exclude command for browserify
+ * package: update "description"
+ * lib: more styling
+ * Makefile: add -build.js and -parse.js dist files
+ * lib: separate out the parse and build logic into their own files
+ * Makefile: add makefile with browserify build rules
+ * package: add "browserify" as a dev dependency
+ * plist: tabs to spaces (again)
+ * add a .jshintrc file
+ * LICENSE: update
+ * node-webkit support
+ * Ignore tests/ in .npmignore file
+ * Remove duplicate devDependencies key
+ * Remove trailing whitespace
+ * adding recent contributors. Bumping npm package number (patch release)
+ * Fixed node.js string handling
+ * bumping version number
+ * Fixed global variable plist leak
+ * patch release 0.4.1
+ * removed temporary debug output file
+ * flipping the cases for writing data and string elements in build(). removed the 125 length check. Added validation of base64 encoding for data fields when parsing. added unit tests.
+ * fixed syntax errors in README examples (issue #20)
+ * added Sync versions of calls. added deprecation warnings for old method calls. updated documentation. If the resulting object from parseStringSync is an array with 1 element, return just the element. If a plist string or file doesnt have a <plist> tag as the document root element, fail noisily (issue #15)
+ * incrementing package version
+ * added cross platform base64 encode/decode for data elements (issue #17.) Comments and hygiene.
+ * refactored the code to use a DOM parser instead of SAX. closes issues #5 and #16
+ * rolling up package version
+ * updated base64 detection regexp. updated README. hygiene.
+ * refactored the build function. Fixes issue #14
+ * refactored tests. Modified tests from issue #9. thanks @sylvinus
+ * upgrade xmlbuilder package version. this is why .end() was needed in last commit; breaking change to xmlbuilder lib. :/
+ * bug fix in build function, forgot to call .end() Refactored tests to use nodeunit
+ * Implemented support for real, identity tests
+ * Refactored base64 detection - still sloppy, fixed date building. Passing tests OK.
+ * Implemented basic plist builder that turns an existing JS object into plist XML. date, real and data types still need to be implemented.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/plist/LICENSE b/node_modules/plist/LICENSE
new file mode 100644
index 0000000..04a9e91
--- /dev/null
+++ b/node_modules/plist/LICENSE
@@ -0,0 +1,24 @@
+(The MIT License)
+
+Copyright (c) 2010-2014 Nathan Rajlich <na...@tootallnate.net>
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/Makefile
----------------------------------------------------------------------
diff --git a/node_modules/plist/Makefile b/node_modules/plist/Makefile
new file mode 100644
index 0000000..62695e0
--- /dev/null
+++ b/node_modules/plist/Makefile
@@ -0,0 +1,76 @@
+
+# get Makefile directory name: http://stackoverflow.com/a/5982798/376773
+THIS_MAKEFILE_PATH:=$(word $(words $(MAKEFILE_LIST)),$(MAKEFILE_LIST))
+THIS_DIR:=$(shell cd $(dir $(THIS_MAKEFILE_PATH));pwd)
+
+# BIN directory
+BIN := $(THIS_DIR)/node_modules/.bin
+
+# applications
+NODE ?= node
+NPM ?= $(NODE) $(shell which npm)
+BROWSERIFY ?= $(NODE) $(BIN)/browserify
+MOCHA ?= $(NODE) $(BIN)/mocha
+ZUUL ?= $(NODE) $(BIN)/zuul
+
+REPORTER ?= spec
+
+all: dist/plist.js dist/plist-build.js dist/plist-parse.js
+
+install: node_modules
+
+clean:
+ @rm -rf node_modules dist
+
+dist:
+ @mkdir -p $@
+
+dist/plist-build.js: node_modules lib/build.js dist
+ @$(BROWSERIFY) \
+ --standalone plist \
+ lib/build.js > $@
+
+dist/plist-parse.js: node_modules lib/parse.js dist
+ @$(BROWSERIFY) \
+ --standalone plist \
+ lib/parse.js > $@
+
+dist/plist.js: node_modules lib/*.js dist
+ @$(BROWSERIFY) \
+ --standalone plist \
+ --ignore lib/node.js \
+ lib/plist.js > $@
+
+node_modules: package.json
+ @NODE_ENV= $(NPM) install
+ @touch node_modules
+
+test:
+ @if [ "x$(BROWSER_NAME)" = "x" ]; then \
+ $(MAKE) test-node; \
+ else \
+ $(MAKE) test-zuul; \
+ fi
+
+test-node:
+ @$(MOCHA) \
+ --reporter $(REPORTER) \
+ test/*.js
+
+test-zuul:
+ @if [ "x$(BROWSER_PLATFORM)" = "x" ]; then \
+ $(ZUUL) \
+ --ui mocha-bdd \
+ --browser-name $(BROWSER_NAME) \
+ --browser-version $(BROWSER_VERSION) \
+ test/*.js; \
+ else \
+ $(ZUUL) \
+ --ui mocha-bdd \
+ --browser-name $(BROWSER_NAME) \
+ --browser-version $(BROWSER_VERSION) \
+ --browser-platform "$(BROWSER_PLATFORM)" \
+ test/*.js; \
+ fi
+
+.PHONY: all install clean test test-node test-zuul
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/README.md
----------------------------------------------------------------------
diff --git a/node_modules/plist/README.md b/node_modules/plist/README.md
new file mode 100644
index 0000000..4d0310a
--- /dev/null
+++ b/node_modules/plist/README.md
@@ -0,0 +1,113 @@
+plist.js
+========
+### Mac OS X Plist parser/builder for Node.js and browsers
+
+[](https://saucelabs.com/u/plistjs)
+
+[](https://travis-ci.org/TooTallNate/plist.js)
+
+Provides facilities for reading and writing Mac OS X Plist (property list)
+files. These are often used in programming OS X and iOS applications, as
+well as the iTunes configuration XML file.
+
+Plist files represent stored programming "object"s. They are very similar
+to JSON. A valid Plist file is representable as a native JavaScript Object
+and vice-versa.
+
+
+## Usage
+
+### Node.js
+
+Install using `npm`:
+
+``` bash
+$ npm install --save plist
+```
+
+Then `require()` the _plist_ module in your file:
+
+``` js
+var plist = require('plist');
+
+// now use the `parse()` and `build()` functions
+var val = plist.parse('<plist><string>Hello World!</string></plist>');
+console.log(val); // "Hello World!"
+```
+
+
+### Browser
+
+Include the `dist/plist.js` in a `<script>` tag in your HTML file:
+
+``` html
+<script src="plist.js"></script>
+<script>
+ // now use the `parse()` and `build()` functions
+ var val = plist.parse('<plist><string>Hello World!</string></plist>');
+ console.log(val); // "Hello World!"
+</script>
+```
+
+
+## API
+
+### Parsing
+
+Parsing a plist from filename:
+
+``` javascript
+var fs = require('fs');
+var plist = require('plist');
+
+var obj = plist.parse(fs.readFileSync('myPlist.plist', 'utf8'));
+console.log(JSON.stringify(obj));
+```
+
+Parsing a plist from string payload:
+
+``` javascript
+var plist = require('plist');
+
+var obj = plist.parse('<plist><string>Hello World!</string></plist>');
+console.log(obj); // Hello World!
+```
+
+### Building
+
+Given an existing JavaScript Object, you can turn it into an XML document
+that complies with the plist DTD:
+
+``` javascript
+var plist = require('plist');
+
+console.log(plist.build({ foo: 'bar' }));
+```
+
+
+## License
+
+(The MIT License)
+
+Copyright (c) 2010-2014 Nathan Rajlich <na...@tootallnate.net>
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[25/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseWhile.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseWhile.js b/node_modules/lodash/internal/baseWhile.js
new file mode 100644
index 0000000..c24e9bd
--- /dev/null
+++ b/node_modules/lodash/internal/baseWhile.js
@@ -0,0 +1,24 @@
+var baseSlice = require('./baseSlice');
+
+/**
+ * The base implementation of `_.dropRightWhile`, `_.dropWhile`, `_.takeRightWhile`,
+ * and `_.takeWhile` without support for callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to query.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {boolean} [isDrop] Specify dropping elements instead of taking them.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function baseWhile(array, predicate, isDrop, fromRight) {
+ var length = array.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length) && predicate(array[index], index, array)) {}
+ return isDrop
+ ? baseSlice(array, (fromRight ? 0 : index), (fromRight ? index + 1 : length))
+ : baseSlice(array, (fromRight ? index + 1 : 0), (fromRight ? length : index));
+}
+
+module.exports = baseWhile;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseWrapperValue.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseWrapperValue.js b/node_modules/lodash/internal/baseWrapperValue.js
new file mode 100644
index 0000000..629c01f
--- /dev/null
+++ b/node_modules/lodash/internal/baseWrapperValue.js
@@ -0,0 +1,29 @@
+var LazyWrapper = require('./LazyWrapper'),
+ arrayPush = require('./arrayPush');
+
+/**
+ * The base implementation of `wrapperValue` which returns the result of
+ * performing a sequence of actions on the unwrapped `value`, where each
+ * successive action is supplied the return value of the previous.
+ *
+ * @private
+ * @param {*} value The unwrapped value.
+ * @param {Array} actions Actions to peform to resolve the unwrapped value.
+ * @returns {*} Returns the resolved value.
+ */
+function baseWrapperValue(value, actions) {
+ var result = value;
+ if (result instanceof LazyWrapper) {
+ result = result.value();
+ }
+ var index = -1,
+ length = actions.length;
+
+ while (++index < length) {
+ var action = actions[index];
+ result = action.func.apply(action.thisArg, arrayPush([result], action.args));
+ }
+ return result;
+}
+
+module.exports = baseWrapperValue;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/binaryIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/binaryIndex.js b/node_modules/lodash/internal/binaryIndex.js
new file mode 100644
index 0000000..af419a2
--- /dev/null
+++ b/node_modules/lodash/internal/binaryIndex.js
@@ -0,0 +1,39 @@
+var binaryIndexBy = require('./binaryIndexBy'),
+ identity = require('../utility/identity');
+
+/** Used as references for the maximum length and index of an array. */
+var MAX_ARRAY_LENGTH = 4294967295,
+ HALF_MAX_ARRAY_LENGTH = MAX_ARRAY_LENGTH >>> 1;
+
+/**
+ * Performs a binary search of `array` to determine the index at which `value`
+ * should be inserted into `array` in order to maintain its sort order.
+ *
+ * @private
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ */
+function binaryIndex(array, value, retHighest) {
+ var low = 0,
+ high = array ? array.length : low;
+
+ if (typeof value == 'number' && value === value && high <= HALF_MAX_ARRAY_LENGTH) {
+ while (low < high) {
+ var mid = (low + high) >>> 1,
+ computed = array[mid];
+
+ if ((retHighest ? (computed <= value) : (computed < value)) && computed !== null) {
+ low = mid + 1;
+ } else {
+ high = mid;
+ }
+ }
+ return high;
+ }
+ return binaryIndexBy(array, value, identity, retHighest);
+}
+
+module.exports = binaryIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/binaryIndexBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/binaryIndexBy.js b/node_modules/lodash/internal/binaryIndexBy.js
new file mode 100644
index 0000000..767cbd2
--- /dev/null
+++ b/node_modules/lodash/internal/binaryIndexBy.js
@@ -0,0 +1,57 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor,
+ nativeMin = Math.min;
+
+/** Used as references for the maximum length and index of an array. */
+var MAX_ARRAY_LENGTH = 4294967295,
+ MAX_ARRAY_INDEX = MAX_ARRAY_LENGTH - 1;
+
+/**
+ * This function is like `binaryIndex` except that it invokes `iteratee` for
+ * `value` and each element of `array` to compute their sort ranking. The
+ * iteratee is invoked with one argument; (value).
+ *
+ * @private
+ * @param {Array} array The sorted array to inspect.
+ * @param {*} value The value to evaluate.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {number} Returns the index at which `value` should be inserted
+ * into `array`.
+ */
+function binaryIndexBy(array, value, iteratee, retHighest) {
+ value = iteratee(value);
+
+ var low = 0,
+ high = array ? array.length : 0,
+ valIsNaN = value !== value,
+ valIsNull = value === null,
+ valIsUndef = value === undefined;
+
+ while (low < high) {
+ var mid = nativeFloor((low + high) / 2),
+ computed = iteratee(array[mid]),
+ isDef = computed !== undefined,
+ isReflexive = computed === computed;
+
+ if (valIsNaN) {
+ var setLow = isReflexive || retHighest;
+ } else if (valIsNull) {
+ setLow = isReflexive && isDef && (retHighest || computed != null);
+ } else if (valIsUndef) {
+ setLow = isReflexive && (retHighest || isDef);
+ } else if (computed == null) {
+ setLow = false;
+ } else {
+ setLow = retHighest ? (computed <= value) : (computed < value);
+ }
+ if (setLow) {
+ low = mid + 1;
+ } else {
+ high = mid;
+ }
+ }
+ return nativeMin(high, MAX_ARRAY_INDEX);
+}
+
+module.exports = binaryIndexBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/bindCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/bindCallback.js b/node_modules/lodash/internal/bindCallback.js
new file mode 100644
index 0000000..cdc7f49
--- /dev/null
+++ b/node_modules/lodash/internal/bindCallback.js
@@ -0,0 +1,39 @@
+var identity = require('../utility/identity');
+
+/**
+ * A specialized version of `baseCallback` which only supports `this` binding
+ * and specifying the number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Returns the callback.
+ */
+function bindCallback(func, thisArg, argCount) {
+ if (typeof func != 'function') {
+ return identity;
+ }
+ if (thisArg === undefined) {
+ return func;
+ }
+ switch (argCount) {
+ case 1: return function(value) {
+ return func.call(thisArg, value);
+ };
+ case 3: return function(value, index, collection) {
+ return func.call(thisArg, value, index, collection);
+ };
+ case 4: return function(accumulator, value, index, collection) {
+ return func.call(thisArg, accumulator, value, index, collection);
+ };
+ case 5: return function(value, other, key, object, source) {
+ return func.call(thisArg, value, other, key, object, source);
+ };
+ }
+ return function() {
+ return func.apply(thisArg, arguments);
+ };
+}
+
+module.exports = bindCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/bufferClone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/bufferClone.js b/node_modules/lodash/internal/bufferClone.js
new file mode 100644
index 0000000..f3c12b8
--- /dev/null
+++ b/node_modules/lodash/internal/bufferClone.js
@@ -0,0 +1,20 @@
+/** Native method references. */
+var ArrayBuffer = global.ArrayBuffer,
+ Uint8Array = global.Uint8Array;
+
+/**
+ * Creates a clone of the given array buffer.
+ *
+ * @private
+ * @param {ArrayBuffer} buffer The array buffer to clone.
+ * @returns {ArrayBuffer} Returns the cloned array buffer.
+ */
+function bufferClone(buffer) {
+ var result = new ArrayBuffer(buffer.byteLength),
+ view = new Uint8Array(result);
+
+ view.set(new Uint8Array(buffer));
+ return result;
+}
+
+module.exports = bufferClone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/cacheIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/cacheIndexOf.js b/node_modules/lodash/internal/cacheIndexOf.js
new file mode 100644
index 0000000..09f698a
--- /dev/null
+++ b/node_modules/lodash/internal/cacheIndexOf.js
@@ -0,0 +1,19 @@
+var isObject = require('../lang/isObject');
+
+/**
+ * Checks if `value` is in `cache` mimicking the return signature of
+ * `_.indexOf` by returning `0` if the value is found, else `-1`.
+ *
+ * @private
+ * @param {Object} cache The cache to search.
+ * @param {*} value The value to search for.
+ * @returns {number} Returns `0` if `value` is found, else `-1`.
+ */
+function cacheIndexOf(cache, value) {
+ var data = cache.data,
+ result = (typeof value == 'string' || isObject(value)) ? data.set.has(value) : data.hash[value];
+
+ return result ? 0 : -1;
+}
+
+module.exports = cacheIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/cachePush.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/cachePush.js b/node_modules/lodash/internal/cachePush.js
new file mode 100644
index 0000000..ba03a15
--- /dev/null
+++ b/node_modules/lodash/internal/cachePush.js
@@ -0,0 +1,20 @@
+var isObject = require('../lang/isObject');
+
+/**
+ * Adds `value` to the cache.
+ *
+ * @private
+ * @name push
+ * @memberOf SetCache
+ * @param {*} value The value to cache.
+ */
+function cachePush(value) {
+ var data = this.data;
+ if (typeof value == 'string' || isObject(value)) {
+ data.set.add(value);
+ } else {
+ data.hash[value] = true;
+ }
+}
+
+module.exports = cachePush;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/charsLeftIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/charsLeftIndex.js b/node_modules/lodash/internal/charsLeftIndex.js
new file mode 100644
index 0000000..a6d1d81
--- /dev/null
+++ b/node_modules/lodash/internal/charsLeftIndex.js
@@ -0,0 +1,18 @@
+/**
+ * Used by `_.trim` and `_.trimLeft` to get the index of the first character
+ * of `string` that is not found in `chars`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @param {string} chars The characters to find.
+ * @returns {number} Returns the index of the first character not found in `chars`.
+ */
+function charsLeftIndex(string, chars) {
+ var index = -1,
+ length = string.length;
+
+ while (++index < length && chars.indexOf(string.charAt(index)) > -1) {}
+ return index;
+}
+
+module.exports = charsLeftIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/charsRightIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/charsRightIndex.js b/node_modules/lodash/internal/charsRightIndex.js
new file mode 100644
index 0000000..1251dcb
--- /dev/null
+++ b/node_modules/lodash/internal/charsRightIndex.js
@@ -0,0 +1,17 @@
+/**
+ * Used by `_.trim` and `_.trimRight` to get the index of the last character
+ * of `string` that is not found in `chars`.
+ *
+ * @private
+ * @param {string} string The string to inspect.
+ * @param {string} chars The characters to find.
+ * @returns {number} Returns the index of the last character not found in `chars`.
+ */
+function charsRightIndex(string, chars) {
+ var index = string.length;
+
+ while (index-- && chars.indexOf(string.charAt(index)) > -1) {}
+ return index;
+}
+
+module.exports = charsRightIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/compareAscending.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/compareAscending.js b/node_modules/lodash/internal/compareAscending.js
new file mode 100644
index 0000000..f17b117
--- /dev/null
+++ b/node_modules/lodash/internal/compareAscending.js
@@ -0,0 +1,16 @@
+var baseCompareAscending = require('./baseCompareAscending');
+
+/**
+ * Used by `_.sortBy` to compare transformed elements of a collection and stable
+ * sort them in ascending order.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @returns {number} Returns the sort order indicator for `object`.
+ */
+function compareAscending(object, other) {
+ return baseCompareAscending(object.criteria, other.criteria) || (object.index - other.index);
+}
+
+module.exports = compareAscending;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/compareMultiple.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/compareMultiple.js b/node_modules/lodash/internal/compareMultiple.js
new file mode 100644
index 0000000..b2139f7
--- /dev/null
+++ b/node_modules/lodash/internal/compareMultiple.js
@@ -0,0 +1,44 @@
+var baseCompareAscending = require('./baseCompareAscending');
+
+/**
+ * Used by `_.sortByOrder` to compare multiple properties of a value to another
+ * and stable sort them.
+ *
+ * If `orders` is unspecified, all valuess are sorted in ascending order. Otherwise,
+ * a value is sorted in ascending order if its corresponding order is "asc", and
+ * descending if "desc".
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {boolean[]} orders The order to sort by for each property.
+ * @returns {number} Returns the sort order indicator for `object`.
+ */
+function compareMultiple(object, other, orders) {
+ var index = -1,
+ objCriteria = object.criteria,
+ othCriteria = other.criteria,
+ length = objCriteria.length,
+ ordersLength = orders.length;
+
+ while (++index < length) {
+ var result = baseCompareAscending(objCriteria[index], othCriteria[index]);
+ if (result) {
+ if (index >= ordersLength) {
+ return result;
+ }
+ var order = orders[index];
+ return result * ((order === 'asc' || order === true) ? 1 : -1);
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to provide the same value for
+ // `object` and `other`. See https://github.com/jashkenas/underscore/pull/1247
+ // for more details.
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See https://code.google.com/p/v8/issues/detail?id=90 for more details.
+ return object.index - other.index;
+}
+
+module.exports = compareMultiple;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/composeArgs.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/composeArgs.js b/node_modules/lodash/internal/composeArgs.js
new file mode 100644
index 0000000..cd5a2fe
--- /dev/null
+++ b/node_modules/lodash/internal/composeArgs.js
@@ -0,0 +1,34 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates an array that is the composition of partially applied arguments,
+ * placeholders, and provided arguments into a single array of arguments.
+ *
+ * @private
+ * @param {Array|Object} args The provided arguments.
+ * @param {Array} partials The arguments to prepend to those provided.
+ * @param {Array} holders The `partials` placeholder indexes.
+ * @returns {Array} Returns the new array of composed arguments.
+ */
+function composeArgs(args, partials, holders) {
+ var holdersLength = holders.length,
+ argsIndex = -1,
+ argsLength = nativeMax(args.length - holdersLength, 0),
+ leftIndex = -1,
+ leftLength = partials.length,
+ result = Array(leftLength + argsLength);
+
+ while (++leftIndex < leftLength) {
+ result[leftIndex] = partials[leftIndex];
+ }
+ while (++argsIndex < holdersLength) {
+ result[holders[argsIndex]] = args[argsIndex];
+ }
+ while (argsLength--) {
+ result[leftIndex++] = args[argsIndex++];
+ }
+ return result;
+}
+
+module.exports = composeArgs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/composeArgsRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/composeArgsRight.js b/node_modules/lodash/internal/composeArgsRight.js
new file mode 100644
index 0000000..38ab139
--- /dev/null
+++ b/node_modules/lodash/internal/composeArgsRight.js
@@ -0,0 +1,36 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * This function is like `composeArgs` except that the arguments composition
+ * is tailored for `_.partialRight`.
+ *
+ * @private
+ * @param {Array|Object} args The provided arguments.
+ * @param {Array} partials The arguments to append to those provided.
+ * @param {Array} holders The `partials` placeholder indexes.
+ * @returns {Array} Returns the new array of composed arguments.
+ */
+function composeArgsRight(args, partials, holders) {
+ var holdersIndex = -1,
+ holdersLength = holders.length,
+ argsIndex = -1,
+ argsLength = nativeMax(args.length - holdersLength, 0),
+ rightIndex = -1,
+ rightLength = partials.length,
+ result = Array(argsLength + rightLength);
+
+ while (++argsIndex < argsLength) {
+ result[argsIndex] = args[argsIndex];
+ }
+ var offset = argsIndex;
+ while (++rightIndex < rightLength) {
+ result[offset + rightIndex] = partials[rightIndex];
+ }
+ while (++holdersIndex < holdersLength) {
+ result[offset + holders[holdersIndex]] = args[argsIndex++];
+ }
+ return result;
+}
+
+module.exports = composeArgsRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createAggregator.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createAggregator.js b/node_modules/lodash/internal/createAggregator.js
new file mode 100644
index 0000000..c3d3cec
--- /dev/null
+++ b/node_modules/lodash/internal/createAggregator.js
@@ -0,0 +1,35 @@
+var baseCallback = require('./baseCallback'),
+ baseEach = require('./baseEach'),
+ isArray = require('../lang/isArray');
+
+/**
+ * Creates a `_.countBy`, `_.groupBy`, `_.indexBy`, or `_.partition` function.
+ *
+ * @private
+ * @param {Function} setter The function to set keys and values of the accumulator object.
+ * @param {Function} [initializer] The function to initialize the accumulator object.
+ * @returns {Function} Returns the new aggregator function.
+ */
+function createAggregator(setter, initializer) {
+ return function(collection, iteratee, thisArg) {
+ var result = initializer ? initializer() : {};
+ iteratee = baseCallback(iteratee, thisArg, 3);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ setter(result, value, iteratee(value, index, collection), collection);
+ }
+ } else {
+ baseEach(collection, function(value, key, collection) {
+ setter(result, value, iteratee(value, key, collection), collection);
+ });
+ }
+ return result;
+ };
+}
+
+module.exports = createAggregator;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createAssigner.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createAssigner.js b/node_modules/lodash/internal/createAssigner.js
new file mode 100644
index 0000000..ea5a5a4
--- /dev/null
+++ b/node_modules/lodash/internal/createAssigner.js
@@ -0,0 +1,41 @@
+var bindCallback = require('./bindCallback'),
+ isIterateeCall = require('./isIterateeCall'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates a `_.assign`, `_.defaults`, or `_.merge` function.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+function createAssigner(assigner) {
+ return restParam(function(object, sources) {
+ var index = -1,
+ length = object == null ? 0 : sources.length,
+ customizer = length > 2 ? sources[length - 2] : undefined,
+ guard = length > 2 ? sources[2] : undefined,
+ thisArg = length > 1 ? sources[length - 1] : undefined;
+
+ if (typeof customizer == 'function') {
+ customizer = bindCallback(customizer, thisArg, 5);
+ length -= 2;
+ } else {
+ customizer = typeof thisArg == 'function' ? thisArg : undefined;
+ length -= (customizer ? 1 : 0);
+ }
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ customizer = length < 3 ? undefined : customizer;
+ length = 1;
+ }
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, customizer);
+ }
+ }
+ return object;
+ });
+}
+
+module.exports = createAssigner;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createBaseEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createBaseEach.js b/node_modules/lodash/internal/createBaseEach.js
new file mode 100644
index 0000000..b55c39b
--- /dev/null
+++ b/node_modules/lodash/internal/createBaseEach.js
@@ -0,0 +1,31 @@
+var getLength = require('./getLength'),
+ isLength = require('./isLength'),
+ toObject = require('./toObject');
+
+/**
+ * Creates a `baseEach` or `baseEachRight` function.
+ *
+ * @private
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseEach(eachFunc, fromRight) {
+ return function(collection, iteratee) {
+ var length = collection ? getLength(collection) : 0;
+ if (!isLength(length)) {
+ return eachFunc(collection, iteratee);
+ }
+ var index = fromRight ? length : -1,
+ iterable = toObject(collection);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (iteratee(iterable[index], index, iterable) === false) {
+ break;
+ }
+ }
+ return collection;
+ };
+}
+
+module.exports = createBaseEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createBaseFor.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createBaseFor.js b/node_modules/lodash/internal/createBaseFor.js
new file mode 100644
index 0000000..3c2cac5
--- /dev/null
+++ b/node_modules/lodash/internal/createBaseFor.js
@@ -0,0 +1,27 @@
+var toObject = require('./toObject');
+
+/**
+ * Creates a base function for `_.forIn` or `_.forInRight`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseFor(fromRight) {
+ return function(object, iteratee, keysFunc) {
+ var iterable = toObject(object),
+ props = keysFunc(object),
+ length = props.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length)) {
+ var key = props[index];
+ if (iteratee(iterable[key], key, iterable) === false) {
+ break;
+ }
+ }
+ return object;
+ };
+}
+
+module.exports = createBaseFor;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createBindWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createBindWrapper.js b/node_modules/lodash/internal/createBindWrapper.js
new file mode 100644
index 0000000..54086ee
--- /dev/null
+++ b/node_modules/lodash/internal/createBindWrapper.js
@@ -0,0 +1,22 @@
+var createCtorWrapper = require('./createCtorWrapper');
+
+/**
+ * Creates a function that wraps `func` and invokes it with the `this`
+ * binding of `thisArg`.
+ *
+ * @private
+ * @param {Function} func The function to bind.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @returns {Function} Returns the new bound function.
+ */
+function createBindWrapper(func, thisArg) {
+ var Ctor = createCtorWrapper(func);
+
+ function wrapper() {
+ var fn = (this && this !== global && this instanceof wrapper) ? Ctor : func;
+ return fn.apply(thisArg, arguments);
+ }
+ return wrapper;
+}
+
+module.exports = createBindWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createCache.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createCache.js b/node_modules/lodash/internal/createCache.js
new file mode 100644
index 0000000..025e566
--- /dev/null
+++ b/node_modules/lodash/internal/createCache.js
@@ -0,0 +1,21 @@
+var SetCache = require('./SetCache'),
+ getNative = require('./getNative');
+
+/** Native method references. */
+var Set = getNative(global, 'Set');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeCreate = getNative(Object, 'create');
+
+/**
+ * Creates a `Set` cache object to optimize linear searches of large arrays.
+ *
+ * @private
+ * @param {Array} [values] The values to cache.
+ * @returns {null|Object} Returns the new cache object if `Set` is supported, else `null`.
+ */
+function createCache(values) {
+ return (nativeCreate && Set) ? new SetCache(values) : null;
+}
+
+module.exports = createCache;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createCompounder.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createCompounder.js b/node_modules/lodash/internal/createCompounder.js
new file mode 100644
index 0000000..4c75512
--- /dev/null
+++ b/node_modules/lodash/internal/createCompounder.js
@@ -0,0 +1,26 @@
+var deburr = require('../string/deburr'),
+ words = require('../string/words');
+
+/**
+ * Creates a function that produces compound words out of the words in a
+ * given string.
+ *
+ * @private
+ * @param {Function} callback The function to combine each word.
+ * @returns {Function} Returns the new compounder function.
+ */
+function createCompounder(callback) {
+ return function(string) {
+ var index = -1,
+ array = words(deburr(string)),
+ length = array.length,
+ result = '';
+
+ while (++index < length) {
+ result = callback(result, array[index], index);
+ }
+ return result;
+ };
+}
+
+module.exports = createCompounder;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createCtorWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createCtorWrapper.js b/node_modules/lodash/internal/createCtorWrapper.js
new file mode 100644
index 0000000..ffbee80
--- /dev/null
+++ b/node_modules/lodash/internal/createCtorWrapper.js
@@ -0,0 +1,37 @@
+var baseCreate = require('./baseCreate'),
+ isObject = require('../lang/isObject');
+
+/**
+ * Creates a function that produces an instance of `Ctor` regardless of
+ * whether it was invoked as part of a `new` expression or by `call` or `apply`.
+ *
+ * @private
+ * @param {Function} Ctor The constructor to wrap.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createCtorWrapper(Ctor) {
+ return function() {
+ // Use a `switch` statement to work with class constructors.
+ // See http://ecma-international.org/ecma-262/6.0/#sec-ecmascript-function-objects-call-thisargument-argumentslist
+ // for more details.
+ var args = arguments;
+ switch (args.length) {
+ case 0: return new Ctor;
+ case 1: return new Ctor(args[0]);
+ case 2: return new Ctor(args[0], args[1]);
+ case 3: return new Ctor(args[0], args[1], args[2]);
+ case 4: return new Ctor(args[0], args[1], args[2], args[3]);
+ case 5: return new Ctor(args[0], args[1], args[2], args[3], args[4]);
+ case 6: return new Ctor(args[0], args[1], args[2], args[3], args[4], args[5]);
+ case 7: return new Ctor(args[0], args[1], args[2], args[3], args[4], args[5], args[6]);
+ }
+ var thisBinding = baseCreate(Ctor.prototype),
+ result = Ctor.apply(thisBinding, args);
+
+ // Mimic the constructor's `return` behavior.
+ // See https://es5.github.io/#x13.2.2 for more details.
+ return isObject(result) ? result : thisBinding;
+ };
+}
+
+module.exports = createCtorWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createCurry.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createCurry.js b/node_modules/lodash/internal/createCurry.js
new file mode 100644
index 0000000..e5ced0e
--- /dev/null
+++ b/node_modules/lodash/internal/createCurry.js
@@ -0,0 +1,23 @@
+var createWrapper = require('./createWrapper'),
+ isIterateeCall = require('./isIterateeCall');
+
+/**
+ * Creates a `_.curry` or `_.curryRight` function.
+ *
+ * @private
+ * @param {boolean} flag The curry bit flag.
+ * @returns {Function} Returns the new curry function.
+ */
+function createCurry(flag) {
+ function curryFunc(func, arity, guard) {
+ if (guard && isIterateeCall(func, arity, guard)) {
+ arity = undefined;
+ }
+ var result = createWrapper(func, flag, undefined, undefined, undefined, undefined, undefined, arity);
+ result.placeholder = curryFunc.placeholder;
+ return result;
+ }
+ return curryFunc;
+}
+
+module.exports = createCurry;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createDefaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createDefaults.js b/node_modules/lodash/internal/createDefaults.js
new file mode 100644
index 0000000..5663bcb
--- /dev/null
+++ b/node_modules/lodash/internal/createDefaults.js
@@ -0,0 +1,22 @@
+var restParam = require('../function/restParam');
+
+/**
+ * Creates a `_.defaults` or `_.defaultsDeep` function.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Function} Returns the new defaults function.
+ */
+function createDefaults(assigner, customizer) {
+ return restParam(function(args) {
+ var object = args[0];
+ if (object == null) {
+ return object;
+ }
+ args.push(customizer);
+ return assigner.apply(undefined, args);
+ });
+}
+
+module.exports = createDefaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createExtremum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createExtremum.js b/node_modules/lodash/internal/createExtremum.js
new file mode 100644
index 0000000..5c4003e
--- /dev/null
+++ b/node_modules/lodash/internal/createExtremum.js
@@ -0,0 +1,33 @@
+var arrayExtremum = require('./arrayExtremum'),
+ baseCallback = require('./baseCallback'),
+ baseExtremum = require('./baseExtremum'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('./isIterateeCall'),
+ toIterable = require('./toIterable');
+
+/**
+ * Creates a `_.max` or `_.min` function.
+ *
+ * @private
+ * @param {Function} comparator The function used to compare values.
+ * @param {*} exValue The initial extremum value.
+ * @returns {Function} Returns the new extremum function.
+ */
+function createExtremum(comparator, exValue) {
+ return function(collection, iteratee, thisArg) {
+ if (thisArg && isIterateeCall(collection, iteratee, thisArg)) {
+ iteratee = undefined;
+ }
+ iteratee = baseCallback(iteratee, thisArg, 3);
+ if (iteratee.length == 1) {
+ collection = isArray(collection) ? collection : toIterable(collection);
+ var result = arrayExtremum(collection, iteratee, comparator, exValue);
+ if (!(collection.length && result === exValue)) {
+ return result;
+ }
+ }
+ return baseExtremum(collection, iteratee, comparator, exValue);
+ };
+}
+
+module.exports = createExtremum;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createFind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createFind.js b/node_modules/lodash/internal/createFind.js
new file mode 100644
index 0000000..29bf580
--- /dev/null
+++ b/node_modules/lodash/internal/createFind.js
@@ -0,0 +1,25 @@
+var baseCallback = require('./baseCallback'),
+ baseFind = require('./baseFind'),
+ baseFindIndex = require('./baseFindIndex'),
+ isArray = require('../lang/isArray');
+
+/**
+ * Creates a `_.find` or `_.findLast` function.
+ *
+ * @private
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new find function.
+ */
+function createFind(eachFunc, fromRight) {
+ return function(collection, predicate, thisArg) {
+ predicate = baseCallback(predicate, thisArg, 3);
+ if (isArray(collection)) {
+ var index = baseFindIndex(collection, predicate, fromRight);
+ return index > -1 ? collection[index] : undefined;
+ }
+ return baseFind(collection, predicate, eachFunc);
+ };
+}
+
+module.exports = createFind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createFindIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createFindIndex.js b/node_modules/lodash/internal/createFindIndex.js
new file mode 100644
index 0000000..3947bea
--- /dev/null
+++ b/node_modules/lodash/internal/createFindIndex.js
@@ -0,0 +1,21 @@
+var baseCallback = require('./baseCallback'),
+ baseFindIndex = require('./baseFindIndex');
+
+/**
+ * Creates a `_.findIndex` or `_.findLastIndex` function.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new find function.
+ */
+function createFindIndex(fromRight) {
+ return function(array, predicate, thisArg) {
+ if (!(array && array.length)) {
+ return -1;
+ }
+ predicate = baseCallback(predicate, thisArg, 3);
+ return baseFindIndex(array, predicate, fromRight);
+ };
+}
+
+module.exports = createFindIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createFindKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createFindKey.js b/node_modules/lodash/internal/createFindKey.js
new file mode 100644
index 0000000..0ce85e4
--- /dev/null
+++ b/node_modules/lodash/internal/createFindKey.js
@@ -0,0 +1,18 @@
+var baseCallback = require('./baseCallback'),
+ baseFind = require('./baseFind');
+
+/**
+ * Creates a `_.findKey` or `_.findLastKey` function.
+ *
+ * @private
+ * @param {Function} objectFunc The function to iterate over an object.
+ * @returns {Function} Returns the new find function.
+ */
+function createFindKey(objectFunc) {
+ return function(object, predicate, thisArg) {
+ predicate = baseCallback(predicate, thisArg, 3);
+ return baseFind(object, predicate, objectFunc, true);
+ };
+}
+
+module.exports = createFindKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createFlow.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createFlow.js b/node_modules/lodash/internal/createFlow.js
new file mode 100644
index 0000000..52ab388
--- /dev/null
+++ b/node_modules/lodash/internal/createFlow.js
@@ -0,0 +1,74 @@
+var LodashWrapper = require('./LodashWrapper'),
+ getData = require('./getData'),
+ getFuncName = require('./getFuncName'),
+ isArray = require('../lang/isArray'),
+ isLaziable = require('./isLaziable');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var CURRY_FLAG = 8,
+ PARTIAL_FLAG = 32,
+ ARY_FLAG = 128,
+ REARG_FLAG = 256;
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * Creates a `_.flow` or `_.flowRight` function.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new flow function.
+ */
+function createFlow(fromRight) {
+ return function() {
+ var wrapper,
+ length = arguments.length,
+ index = fromRight ? length : -1,
+ leftIndex = 0,
+ funcs = Array(length);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ var func = funcs[leftIndex++] = arguments[index];
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ if (!wrapper && LodashWrapper.prototype.thru && getFuncName(func) == 'wrapper') {
+ wrapper = new LodashWrapper([], true);
+ }
+ }
+ index = wrapper ? -1 : length;
+ while (++index < length) {
+ func = funcs[index];
+
+ var funcName = getFuncName(func),
+ data = funcName == 'wrapper' ? getData(func) : undefined;
+
+ if (data && isLaziable(data[0]) && data[1] == (ARY_FLAG | CURRY_FLAG | PARTIAL_FLAG | REARG_FLAG) && !data[4].length && data[9] == 1) {
+ wrapper = wrapper[getFuncName(data[0])].apply(wrapper, data[3]);
+ } else {
+ wrapper = (func.length == 1 && isLaziable(func)) ? wrapper[funcName]() : wrapper.thru(func);
+ }
+ }
+ return function() {
+ var args = arguments,
+ value = args[0];
+
+ if (wrapper && args.length == 1 && isArray(value) && value.length >= LARGE_ARRAY_SIZE) {
+ return wrapper.plant(value).value();
+ }
+ var index = 0,
+ result = length ? funcs[index].apply(this, args) : value;
+
+ while (++index < length) {
+ result = funcs[index].call(this, result);
+ }
+ return result;
+ };
+ };
+}
+
+module.exports = createFlow;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createForEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createForEach.js b/node_modules/lodash/internal/createForEach.js
new file mode 100644
index 0000000..2aad11c
--- /dev/null
+++ b/node_modules/lodash/internal/createForEach.js
@@ -0,0 +1,20 @@
+var bindCallback = require('./bindCallback'),
+ isArray = require('../lang/isArray');
+
+/**
+ * Creates a function for `_.forEach` or `_.forEachRight`.
+ *
+ * @private
+ * @param {Function} arrayFunc The function to iterate over an array.
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @returns {Function} Returns the new each function.
+ */
+function createForEach(arrayFunc, eachFunc) {
+ return function(collection, iteratee, thisArg) {
+ return (typeof iteratee == 'function' && thisArg === undefined && isArray(collection))
+ ? arrayFunc(collection, iteratee)
+ : eachFunc(collection, bindCallback(iteratee, thisArg, 3));
+ };
+}
+
+module.exports = createForEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createForIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createForIn.js b/node_modules/lodash/internal/createForIn.js
new file mode 100644
index 0000000..f63ffa0
--- /dev/null
+++ b/node_modules/lodash/internal/createForIn.js
@@ -0,0 +1,20 @@
+var bindCallback = require('./bindCallback'),
+ keysIn = require('../object/keysIn');
+
+/**
+ * Creates a function for `_.forIn` or `_.forInRight`.
+ *
+ * @private
+ * @param {Function} objectFunc The function to iterate over an object.
+ * @returns {Function} Returns the new each function.
+ */
+function createForIn(objectFunc) {
+ return function(object, iteratee, thisArg) {
+ if (typeof iteratee != 'function' || thisArg !== undefined) {
+ iteratee = bindCallback(iteratee, thisArg, 3);
+ }
+ return objectFunc(object, iteratee, keysIn);
+ };
+}
+
+module.exports = createForIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createForOwn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createForOwn.js b/node_modules/lodash/internal/createForOwn.js
new file mode 100644
index 0000000..b9a83c3
--- /dev/null
+++ b/node_modules/lodash/internal/createForOwn.js
@@ -0,0 +1,19 @@
+var bindCallback = require('./bindCallback');
+
+/**
+ * Creates a function for `_.forOwn` or `_.forOwnRight`.
+ *
+ * @private
+ * @param {Function} objectFunc The function to iterate over an object.
+ * @returns {Function} Returns the new each function.
+ */
+function createForOwn(objectFunc) {
+ return function(object, iteratee, thisArg) {
+ if (typeof iteratee != 'function' || thisArg !== undefined) {
+ iteratee = bindCallback(iteratee, thisArg, 3);
+ }
+ return objectFunc(object, iteratee);
+ };
+}
+
+module.exports = createForOwn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createHybridWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createHybridWrapper.js b/node_modules/lodash/internal/createHybridWrapper.js
new file mode 100644
index 0000000..5382fa0
--- /dev/null
+++ b/node_modules/lodash/internal/createHybridWrapper.js
@@ -0,0 +1,111 @@
+var arrayCopy = require('./arrayCopy'),
+ composeArgs = require('./composeArgs'),
+ composeArgsRight = require('./composeArgsRight'),
+ createCtorWrapper = require('./createCtorWrapper'),
+ isLaziable = require('./isLaziable'),
+ reorder = require('./reorder'),
+ replaceHolders = require('./replaceHolders'),
+ setData = require('./setData');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1,
+ BIND_KEY_FLAG = 2,
+ CURRY_BOUND_FLAG = 4,
+ CURRY_FLAG = 8,
+ CURRY_RIGHT_FLAG = 16,
+ PARTIAL_FLAG = 32,
+ PARTIAL_RIGHT_FLAG = 64,
+ ARY_FLAG = 128;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that wraps `func` and invokes it with optional `this`
+ * binding of, partial application, and currying.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to reference.
+ * @param {number} bitmask The bitmask of flags. See `createWrapper` for more details.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to prepend to those provided to the new function.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [partialsRight] The arguments to append to those provided to the new function.
+ * @param {Array} [holdersRight] The `partialsRight` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createHybridWrapper(func, bitmask, thisArg, partials, holders, partialsRight, holdersRight, argPos, ary, arity) {
+ var isAry = bitmask & ARY_FLAG,
+ isBind = bitmask & BIND_FLAG,
+ isBindKey = bitmask & BIND_KEY_FLAG,
+ isCurry = bitmask & CURRY_FLAG,
+ isCurryBound = bitmask & CURRY_BOUND_FLAG,
+ isCurryRight = bitmask & CURRY_RIGHT_FLAG,
+ Ctor = isBindKey ? undefined : createCtorWrapper(func);
+
+ function wrapper() {
+ // Avoid `arguments` object use disqualifying optimizations by
+ // converting it to an array before providing it to other functions.
+ var length = arguments.length,
+ index = length,
+ args = Array(length);
+
+ while (index--) {
+ args[index] = arguments[index];
+ }
+ if (partials) {
+ args = composeArgs(args, partials, holders);
+ }
+ if (partialsRight) {
+ args = composeArgsRight(args, partialsRight, holdersRight);
+ }
+ if (isCurry || isCurryRight) {
+ var placeholder = wrapper.placeholder,
+ argsHolders = replaceHolders(args, placeholder);
+
+ length -= argsHolders.length;
+ if (length < arity) {
+ var newArgPos = argPos ? arrayCopy(argPos) : undefined,
+ newArity = nativeMax(arity - length, 0),
+ newsHolders = isCurry ? argsHolders : undefined,
+ newHoldersRight = isCurry ? undefined : argsHolders,
+ newPartials = isCurry ? args : undefined,
+ newPartialsRight = isCurry ? undefined : args;
+
+ bitmask |= (isCurry ? PARTIAL_FLAG : PARTIAL_RIGHT_FLAG);
+ bitmask &= ~(isCurry ? PARTIAL_RIGHT_FLAG : PARTIAL_FLAG);
+
+ if (!isCurryBound) {
+ bitmask &= ~(BIND_FLAG | BIND_KEY_FLAG);
+ }
+ var newData = [func, bitmask, thisArg, newPartials, newsHolders, newPartialsRight, newHoldersRight, newArgPos, ary, newArity],
+ result = createHybridWrapper.apply(undefined, newData);
+
+ if (isLaziable(func)) {
+ setData(result, newData);
+ }
+ result.placeholder = placeholder;
+ return result;
+ }
+ }
+ var thisBinding = isBind ? thisArg : this,
+ fn = isBindKey ? thisBinding[func] : func;
+
+ if (argPos) {
+ args = reorder(args, argPos);
+ }
+ if (isAry && ary < args.length) {
+ args.length = ary;
+ }
+ if (this && this !== global && this instanceof wrapper) {
+ fn = Ctor || createCtorWrapper(func);
+ }
+ return fn.apply(thisBinding, args);
+ }
+ return wrapper;
+}
+
+module.exports = createHybridWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createObjectMapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createObjectMapper.js b/node_modules/lodash/internal/createObjectMapper.js
new file mode 100644
index 0000000..06d6a87
--- /dev/null
+++ b/node_modules/lodash/internal/createObjectMapper.js
@@ -0,0 +1,26 @@
+var baseCallback = require('./baseCallback'),
+ baseForOwn = require('./baseForOwn');
+
+/**
+ * Creates a function for `_.mapKeys` or `_.mapValues`.
+ *
+ * @private
+ * @param {boolean} [isMapKeys] Specify mapping keys instead of values.
+ * @returns {Function} Returns the new map function.
+ */
+function createObjectMapper(isMapKeys) {
+ return function(object, iteratee, thisArg) {
+ var result = {};
+ iteratee = baseCallback(iteratee, thisArg, 3);
+
+ baseForOwn(object, function(value, key, object) {
+ var mapped = iteratee(value, key, object);
+ key = isMapKeys ? mapped : key;
+ value = isMapKeys ? value : mapped;
+ result[key] = value;
+ });
+ return result;
+ };
+}
+
+module.exports = createObjectMapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createPadDir.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createPadDir.js b/node_modules/lodash/internal/createPadDir.js
new file mode 100644
index 0000000..da0ebf1
--- /dev/null
+++ b/node_modules/lodash/internal/createPadDir.js
@@ -0,0 +1,18 @@
+var baseToString = require('./baseToString'),
+ createPadding = require('./createPadding');
+
+/**
+ * Creates a function for `_.padLeft` or `_.padRight`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify padding from the right.
+ * @returns {Function} Returns the new pad function.
+ */
+function createPadDir(fromRight) {
+ return function(string, length, chars) {
+ string = baseToString(string);
+ return (fromRight ? string : '') + createPadding(string, length, chars) + (fromRight ? '' : string);
+ };
+}
+
+module.exports = createPadDir;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createPadding.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createPadding.js b/node_modules/lodash/internal/createPadding.js
new file mode 100644
index 0000000..810dc24
--- /dev/null
+++ b/node_modules/lodash/internal/createPadding.js
@@ -0,0 +1,29 @@
+var repeat = require('../string/repeat');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeCeil = Math.ceil,
+ nativeIsFinite = global.isFinite;
+
+/**
+ * Creates the padding required for `string` based on the given `length`.
+ * The `chars` string is truncated if the number of characters exceeds `length`.
+ *
+ * @private
+ * @param {string} string The string to create padding for.
+ * @param {number} [length=0] The padding length.
+ * @param {string} [chars=' '] The string used as padding.
+ * @returns {string} Returns the pad for `string`.
+ */
+function createPadding(string, length, chars) {
+ var strLength = string.length;
+ length = +length;
+
+ if (strLength >= length || !nativeIsFinite(length)) {
+ return '';
+ }
+ var padLength = length - strLength;
+ chars = chars == null ? ' ' : (chars + '');
+ return repeat(chars, nativeCeil(padLength / chars.length)).slice(0, padLength);
+}
+
+module.exports = createPadding;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createPartial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createPartial.js b/node_modules/lodash/internal/createPartial.js
new file mode 100644
index 0000000..7533275
--- /dev/null
+++ b/node_modules/lodash/internal/createPartial.js
@@ -0,0 +1,20 @@
+var createWrapper = require('./createWrapper'),
+ replaceHolders = require('./replaceHolders'),
+ restParam = require('../function/restParam');
+
+/**
+ * Creates a `_.partial` or `_.partialRight` function.
+ *
+ * @private
+ * @param {boolean} flag The partial bit flag.
+ * @returns {Function} Returns the new partial function.
+ */
+function createPartial(flag) {
+ var partialFunc = restParam(function(func, partials) {
+ var holders = replaceHolders(partials, partialFunc.placeholder);
+ return createWrapper(func, flag, undefined, partials, holders);
+ });
+ return partialFunc;
+}
+
+module.exports = createPartial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createPartialWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createPartialWrapper.js b/node_modules/lodash/internal/createPartialWrapper.js
new file mode 100644
index 0000000..b19f9f0
--- /dev/null
+++ b/node_modules/lodash/internal/createPartialWrapper.js
@@ -0,0 +1,43 @@
+var createCtorWrapper = require('./createCtorWrapper');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1;
+
+/**
+ * Creates a function that wraps `func` and invokes it with the optional `this`
+ * binding of `thisArg` and the `partials` prepended to those provided to
+ * the wrapper.
+ *
+ * @private
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {number} bitmask The bitmask of flags. See `createWrapper` for more details.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} partials The arguments to prepend to those provided to the new function.
+ * @returns {Function} Returns the new bound function.
+ */
+function createPartialWrapper(func, bitmask, thisArg, partials) {
+ var isBind = bitmask & BIND_FLAG,
+ Ctor = createCtorWrapper(func);
+
+ function wrapper() {
+ // Avoid `arguments` object use disqualifying optimizations by
+ // converting it to an array before providing it `func`.
+ var argsIndex = -1,
+ argsLength = arguments.length,
+ leftIndex = -1,
+ leftLength = partials.length,
+ args = Array(leftLength + argsLength);
+
+ while (++leftIndex < leftLength) {
+ args[leftIndex] = partials[leftIndex];
+ }
+ while (argsLength--) {
+ args[leftIndex++] = arguments[++argsIndex];
+ }
+ var fn = (this && this !== global && this instanceof wrapper) ? Ctor : func;
+ return fn.apply(isBind ? thisArg : this, args);
+ }
+ return wrapper;
+}
+
+module.exports = createPartialWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createReduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createReduce.js b/node_modules/lodash/internal/createReduce.js
new file mode 100644
index 0000000..816f4ce
--- /dev/null
+++ b/node_modules/lodash/internal/createReduce.js
@@ -0,0 +1,22 @@
+var baseCallback = require('./baseCallback'),
+ baseReduce = require('./baseReduce'),
+ isArray = require('../lang/isArray');
+
+/**
+ * Creates a function for `_.reduce` or `_.reduceRight`.
+ *
+ * @private
+ * @param {Function} arrayFunc The function to iterate over an array.
+ * @param {Function} eachFunc The function to iterate over a collection.
+ * @returns {Function} Returns the new each function.
+ */
+function createReduce(arrayFunc, eachFunc) {
+ return function(collection, iteratee, accumulator, thisArg) {
+ var initFromArray = arguments.length < 3;
+ return (typeof iteratee == 'function' && thisArg === undefined && isArray(collection))
+ ? arrayFunc(collection, iteratee, accumulator, initFromArray)
+ : baseReduce(collection, baseCallback(iteratee, thisArg, 4), accumulator, initFromArray, eachFunc);
+ };
+}
+
+module.exports = createReduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createRound.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createRound.js b/node_modules/lodash/internal/createRound.js
new file mode 100644
index 0000000..21240ef
--- /dev/null
+++ b/node_modules/lodash/internal/createRound.js
@@ -0,0 +1,23 @@
+/** Native method references. */
+var pow = Math.pow;
+
+/**
+ * Creates a `_.ceil`, `_.floor`, or `_.round` function.
+ *
+ * @private
+ * @param {string} methodName The name of the `Math` method to use when rounding.
+ * @returns {Function} Returns the new round function.
+ */
+function createRound(methodName) {
+ var func = Math[methodName];
+ return function(number, precision) {
+ precision = precision === undefined ? 0 : (+precision || 0);
+ if (precision) {
+ precision = pow(10, precision);
+ return func(number * precision) / precision;
+ }
+ return func(number);
+ };
+}
+
+module.exports = createRound;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createSortedIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createSortedIndex.js b/node_modules/lodash/internal/createSortedIndex.js
new file mode 100644
index 0000000..86c7852
--- /dev/null
+++ b/node_modules/lodash/internal/createSortedIndex.js
@@ -0,0 +1,20 @@
+var baseCallback = require('./baseCallback'),
+ binaryIndex = require('./binaryIndex'),
+ binaryIndexBy = require('./binaryIndexBy');
+
+/**
+ * Creates a `_.sortedIndex` or `_.sortedLastIndex` function.
+ *
+ * @private
+ * @param {boolean} [retHighest] Specify returning the highest qualified index.
+ * @returns {Function} Returns the new index function.
+ */
+function createSortedIndex(retHighest) {
+ return function(array, value, iteratee, thisArg) {
+ return iteratee == null
+ ? binaryIndex(array, value, retHighest)
+ : binaryIndexBy(array, value, baseCallback(iteratee, thisArg, 1), retHighest);
+ };
+}
+
+module.exports = createSortedIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/createWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/createWrapper.js b/node_modules/lodash/internal/createWrapper.js
new file mode 100644
index 0000000..ea7a9b1
--- /dev/null
+++ b/node_modules/lodash/internal/createWrapper.js
@@ -0,0 +1,86 @@
+var baseSetData = require('./baseSetData'),
+ createBindWrapper = require('./createBindWrapper'),
+ createHybridWrapper = require('./createHybridWrapper'),
+ createPartialWrapper = require('./createPartialWrapper'),
+ getData = require('./getData'),
+ mergeData = require('./mergeData'),
+ setData = require('./setData');
+
+/** Used to compose bitmasks for wrapper metadata. */
+var BIND_FLAG = 1,
+ BIND_KEY_FLAG = 2,
+ PARTIAL_FLAG = 32,
+ PARTIAL_RIGHT_FLAG = 64;
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that either curries or invokes `func` with optional
+ * `this` binding and partially applied arguments.
+ *
+ * @private
+ * @param {Function|string} func The function or method name to reference.
+ * @param {number} bitmask The bitmask of flags.
+ * The bitmask may be composed of the following flags:
+ * 1 - `_.bind`
+ * 2 - `_.bindKey`
+ * 4 - `_.curry` or `_.curryRight` of a bound function
+ * 8 - `_.curry`
+ * 16 - `_.curryRight`
+ * 32 - `_.partial`
+ * 64 - `_.partialRight`
+ * 128 - `_.rearg`
+ * 256 - `_.ary`
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {Array} [partials] The arguments to be partially applied.
+ * @param {Array} [holders] The `partials` placeholder indexes.
+ * @param {Array} [argPos] The argument positions of the new function.
+ * @param {number} [ary] The arity cap of `func`.
+ * @param {number} [arity] The arity of `func`.
+ * @returns {Function} Returns the new wrapped function.
+ */
+function createWrapper(func, bitmask, thisArg, partials, holders, argPos, ary, arity) {
+ var isBindKey = bitmask & BIND_KEY_FLAG;
+ if (!isBindKey && typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ var length = partials ? partials.length : 0;
+ if (!length) {
+ bitmask &= ~(PARTIAL_FLAG | PARTIAL_RIGHT_FLAG);
+ partials = holders = undefined;
+ }
+ length -= (holders ? holders.length : 0);
+ if (bitmask & PARTIAL_RIGHT_FLAG) {
+ var partialsRight = partials,
+ holdersRight = holders;
+
+ partials = holders = undefined;
+ }
+ var data = isBindKey ? undefined : getData(func),
+ newData = [func, bitmask, thisArg, partials, holders, partialsRight, holdersRight, argPos, ary, arity];
+
+ if (data) {
+ mergeData(newData, data);
+ bitmask = newData[1];
+ arity = newData[9];
+ }
+ newData[9] = arity == null
+ ? (isBindKey ? 0 : func.length)
+ : (nativeMax(arity - length, 0) || 0);
+
+ if (bitmask == BIND_FLAG) {
+ var result = createBindWrapper(newData[0], newData[2]);
+ } else if ((bitmask == PARTIAL_FLAG || bitmask == (BIND_FLAG | PARTIAL_FLAG)) && !newData[4].length) {
+ result = createPartialWrapper.apply(undefined, newData);
+ } else {
+ result = createHybridWrapper.apply(undefined, newData);
+ }
+ var setter = data ? baseSetData : setData;
+ return setter(result, newData);
+}
+
+module.exports = createWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/deburrLetter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/deburrLetter.js b/node_modules/lodash/internal/deburrLetter.js
new file mode 100644
index 0000000..e559dbe
--- /dev/null
+++ b/node_modules/lodash/internal/deburrLetter.js
@@ -0,0 +1,33 @@
+/** Used to map latin-1 supplementary letters to basic latin letters. */
+var deburredLetters = {
+ '\xc0': 'A', '\xc1': 'A', '\xc2': 'A', '\xc3': 'A', '\xc4': 'A', '\xc5': 'A',
+ '\xe0': 'a', '\xe1': 'a', '\xe2': 'a', '\xe3': 'a', '\xe4': 'a', '\xe5': 'a',
+ '\xc7': 'C', '\xe7': 'c',
+ '\xd0': 'D', '\xf0': 'd',
+ '\xc8': 'E', '\xc9': 'E', '\xca': 'E', '\xcb': 'E',
+ '\xe8': 'e', '\xe9': 'e', '\xea': 'e', '\xeb': 'e',
+ '\xcC': 'I', '\xcd': 'I', '\xce': 'I', '\xcf': 'I',
+ '\xeC': 'i', '\xed': 'i', '\xee': 'i', '\xef': 'i',
+ '\xd1': 'N', '\xf1': 'n',
+ '\xd2': 'O', '\xd3': 'O', '\xd4': 'O', '\xd5': 'O', '\xd6': 'O', '\xd8': 'O',
+ '\xf2': 'o', '\xf3': 'o', '\xf4': 'o', '\xf5': 'o', '\xf6': 'o', '\xf8': 'o',
+ '\xd9': 'U', '\xda': 'U', '\xdb': 'U', '\xdc': 'U',
+ '\xf9': 'u', '\xfa': 'u', '\xfb': 'u', '\xfc': 'u',
+ '\xdd': 'Y', '\xfd': 'y', '\xff': 'y',
+ '\xc6': 'Ae', '\xe6': 'ae',
+ '\xde': 'Th', '\xfe': 'th',
+ '\xdf': 'ss'
+};
+
+/**
+ * Used by `_.deburr` to convert latin-1 supplementary letters to basic latin letters.
+ *
+ * @private
+ * @param {string} letter The matched letter to deburr.
+ * @returns {string} Returns the deburred letter.
+ */
+function deburrLetter(letter) {
+ return deburredLetters[letter];
+}
+
+module.exports = deburrLetter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/equalArrays.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/equalArrays.js b/node_modules/lodash/internal/equalArrays.js
new file mode 100644
index 0000000..e0bb2d3
--- /dev/null
+++ b/node_modules/lodash/internal/equalArrays.js
@@ -0,0 +1,51 @@
+var arraySome = require('./arraySome');
+
+/**
+ * A specialized version of `baseIsEqualDeep` for arrays with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Array} array The array to compare.
+ * @param {Array} other The other array to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing arrays.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the arrays are equivalent, else `false`.
+ */
+function equalArrays(array, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var index = -1,
+ arrLength = array.length,
+ othLength = other.length;
+
+ if (arrLength != othLength && !(isLoose && othLength > arrLength)) {
+ return false;
+ }
+ // Ignore non-index properties.
+ while (++index < arrLength) {
+ var arrValue = array[index],
+ othValue = other[index],
+ result = customizer ? customizer(isLoose ? othValue : arrValue, isLoose ? arrValue : othValue, index) : undefined;
+
+ if (result !== undefined) {
+ if (result) {
+ continue;
+ }
+ return false;
+ }
+ // Recursively compare arrays (susceptible to call stack limits).
+ if (isLoose) {
+ if (!arraySome(other, function(othValue) {
+ return arrValue === othValue || equalFunc(arrValue, othValue, customizer, isLoose, stackA, stackB);
+ })) {
+ return false;
+ }
+ } else if (!(arrValue === othValue || equalFunc(arrValue, othValue, customizer, isLoose, stackA, stackB))) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = equalArrays;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/equalByTag.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/equalByTag.js b/node_modules/lodash/internal/equalByTag.js
new file mode 100644
index 0000000..d25c8e1
--- /dev/null
+++ b/node_modules/lodash/internal/equalByTag.js
@@ -0,0 +1,48 @@
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ numberTag = '[object Number]',
+ regexpTag = '[object RegExp]',
+ stringTag = '[object String]';
+
+/**
+ * A specialized version of `baseIsEqualDeep` for comparing objects of
+ * the same `toStringTag`.
+ *
+ * **Note:** This function only supports comparing values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {string} tag The `toStringTag` of the objects to compare.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function equalByTag(object, other, tag) {
+ switch (tag) {
+ case boolTag:
+ case dateTag:
+ // Coerce dates and booleans to numbers, dates to milliseconds and booleans
+ // to `1` or `0` treating invalid dates coerced to `NaN` as not equal.
+ return +object == +other;
+
+ case errorTag:
+ return object.name == other.name && object.message == other.message;
+
+ case numberTag:
+ // Treat `NaN` vs. `NaN` as equal.
+ return (object != +object)
+ ? other != +other
+ : object == +other;
+
+ case regexpTag:
+ case stringTag:
+ // Coerce regexes to strings and treat strings primitives and string
+ // objects as equal. See https://es5.github.io/#x15.10.6.4 for more details.
+ return object == (other + '');
+ }
+ return false;
+}
+
+module.exports = equalByTag;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/equalObjects.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/equalObjects.js b/node_modules/lodash/internal/equalObjects.js
new file mode 100644
index 0000000..1297a3b
--- /dev/null
+++ b/node_modules/lodash/internal/equalObjects.js
@@ -0,0 +1,67 @@
+var keys = require('../object/keys');
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * A specialized version of `baseIsEqualDeep` for objects with support for
+ * partial deep comparisons.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing values.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function equalObjects(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var objProps = keys(object),
+ objLength = objProps.length,
+ othProps = keys(other),
+ othLength = othProps.length;
+
+ if (objLength != othLength && !isLoose) {
+ return false;
+ }
+ var index = objLength;
+ while (index--) {
+ var key = objProps[index];
+ if (!(isLoose ? key in other : hasOwnProperty.call(other, key))) {
+ return false;
+ }
+ }
+ var skipCtor = isLoose;
+ while (++index < objLength) {
+ key = objProps[index];
+ var objValue = object[key],
+ othValue = other[key],
+ result = customizer ? customizer(isLoose ? othValue : objValue, isLoose? objValue : othValue, key) : undefined;
+
+ // Recursively compare objects (susceptible to call stack limits).
+ if (!(result === undefined ? equalFunc(objValue, othValue, customizer, isLoose, stackA, stackB) : result)) {
+ return false;
+ }
+ skipCtor || (skipCtor = key == 'constructor');
+ }
+ if (!skipCtor) {
+ var objCtor = object.constructor,
+ othCtor = other.constructor;
+
+ // Non `Object` object instances with different constructors are not equal.
+ if (objCtor != othCtor &&
+ ('constructor' in object && 'constructor' in other) &&
+ !(typeof objCtor == 'function' && objCtor instanceof objCtor &&
+ typeof othCtor == 'function' && othCtor instanceof othCtor)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = equalObjects;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/escapeHtmlChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/escapeHtmlChar.js b/node_modules/lodash/internal/escapeHtmlChar.js
new file mode 100644
index 0000000..b21e452
--- /dev/null
+++ b/node_modules/lodash/internal/escapeHtmlChar.js
@@ -0,0 +1,22 @@
+/** Used to map characters to HTML entities. */
+var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": ''',
+ '`': '`'
+};
+
+/**
+ * Used by `_.escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeHtmlChar(chr) {
+ return htmlEscapes[chr];
+}
+
+module.exports = escapeHtmlChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/escapeRegExpChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/escapeRegExpChar.js b/node_modules/lodash/internal/escapeRegExpChar.js
new file mode 100644
index 0000000..8427de0
--- /dev/null
+++ b/node_modules/lodash/internal/escapeRegExpChar.js
@@ -0,0 +1,38 @@
+/** Used to escape characters for inclusion in compiled regexes. */
+var regexpEscapes = {
+ '0': 'x30', '1': 'x31', '2': 'x32', '3': 'x33', '4': 'x34',
+ '5': 'x35', '6': 'x36', '7': 'x37', '8': 'x38', '9': 'x39',
+ 'A': 'x41', 'B': 'x42', 'C': 'x43', 'D': 'x44', 'E': 'x45', 'F': 'x46',
+ 'a': 'x61', 'b': 'x62', 'c': 'x63', 'd': 'x64', 'e': 'x65', 'f': 'x66',
+ 'n': 'x6e', 'r': 'x72', 't': 'x74', 'u': 'x75', 'v': 'x76', 'x': 'x78'
+};
+
+/** Used to escape characters for inclusion in compiled string literals. */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `_.escapeRegExp` to escape characters for inclusion in compiled regexes.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @param {string} leadingChar The capture group for a leading character.
+ * @param {string} whitespaceChar The capture group for a whitespace character.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeRegExpChar(chr, leadingChar, whitespaceChar) {
+ if (leadingChar) {
+ chr = regexpEscapes[chr];
+ } else if (whitespaceChar) {
+ chr = stringEscapes[chr];
+ }
+ return '\\' + chr;
+}
+
+module.exports = escapeRegExpChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/escapeStringChar.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/escapeStringChar.js b/node_modules/lodash/internal/escapeStringChar.js
new file mode 100644
index 0000000..44eca96
--- /dev/null
+++ b/node_modules/lodash/internal/escapeStringChar.js
@@ -0,0 +1,22 @@
+/** Used to escape characters for inclusion in compiled string literals. */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `_.template` to escape characters for inclusion in compiled string literals.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeStringChar(chr) {
+ return '\\' + stringEscapes[chr];
+}
+
+module.exports = escapeStringChar;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/getData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/getData.js b/node_modules/lodash/internal/getData.js
new file mode 100644
index 0000000..5bb4f46
--- /dev/null
+++ b/node_modules/lodash/internal/getData.js
@@ -0,0 +1,15 @@
+var metaMap = require('./metaMap'),
+ noop = require('../utility/noop');
+
+/**
+ * Gets metadata for `func`.
+ *
+ * @private
+ * @param {Function} func The function to query.
+ * @returns {*} Returns the metadata for `func`.
+ */
+var getData = !metaMap ? noop : function(func) {
+ return metaMap.get(func);
+};
+
+module.exports = getData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/getFuncName.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/getFuncName.js b/node_modules/lodash/internal/getFuncName.js
new file mode 100644
index 0000000..ed92867
--- /dev/null
+++ b/node_modules/lodash/internal/getFuncName.js
@@ -0,0 +1,25 @@
+var realNames = require('./realNames');
+
+/**
+ * Gets the name of `func`.
+ *
+ * @private
+ * @param {Function} func The function to query.
+ * @returns {string} Returns the function name.
+ */
+function getFuncName(func) {
+ var result = (func.name + ''),
+ array = realNames[result],
+ length = array ? array.length : 0;
+
+ while (length--) {
+ var data = array[length],
+ otherFunc = data.func;
+ if (otherFunc == null || otherFunc == func) {
+ return data.name;
+ }
+ }
+ return result;
+}
+
+module.exports = getFuncName;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/getLength.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/getLength.js b/node_modules/lodash/internal/getLength.js
new file mode 100644
index 0000000..48d75ae
--- /dev/null
+++ b/node_modules/lodash/internal/getLength.js
@@ -0,0 +1,15 @@
+var baseProperty = require('./baseProperty');
+
+/**
+ * Gets the "length" property value of `object`.
+ *
+ * **Note:** This function is used to avoid a [JIT bug](https://bugs.webkit.org/show_bug.cgi?id=142792)
+ * that affects Safari on at least iOS 8.1-8.3 ARM64.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {*} Returns the "length" value.
+ */
+var getLength = baseProperty('length');
+
+module.exports = getLength;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/getMatchData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/getMatchData.js b/node_modules/lodash/internal/getMatchData.js
new file mode 100644
index 0000000..6d235b9
--- /dev/null
+++ b/node_modules/lodash/internal/getMatchData.js
@@ -0,0 +1,21 @@
+var isStrictComparable = require('./isStrictComparable'),
+ pairs = require('../object/pairs');
+
+/**
+ * Gets the propery names, values, and compare flags of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the match data of `object`.
+ */
+function getMatchData(object) {
+ var result = pairs(object),
+ length = result.length;
+
+ while (length--) {
+ result[length][2] = isStrictComparable(result[length][1]);
+ }
+ return result;
+}
+
+module.exports = getMatchData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/getNative.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/getNative.js b/node_modules/lodash/internal/getNative.js
new file mode 100644
index 0000000..bceb317
--- /dev/null
+++ b/node_modules/lodash/internal/getNative.js
@@ -0,0 +1,16 @@
+var isNative = require('../lang/isNative');
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = object == null ? undefined : object[key];
+ return isNative(value) ? value : undefined;
+}
+
+module.exports = getNative;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/getView.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/getView.js b/node_modules/lodash/internal/getView.js
new file mode 100644
index 0000000..f49ec6d
--- /dev/null
+++ b/node_modules/lodash/internal/getView.js
@@ -0,0 +1,33 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the view, applying any `transforms` to the `start` and `end` positions.
+ *
+ * @private
+ * @param {number} start The start of the view.
+ * @param {number} end The end of the view.
+ * @param {Array} transforms The transformations to apply to the view.
+ * @returns {Object} Returns an object containing the `start` and `end`
+ * positions of the view.
+ */
+function getView(start, end, transforms) {
+ var index = -1,
+ length = transforms.length;
+
+ while (++index < length) {
+ var data = transforms[index],
+ size = data.size;
+
+ switch (data.type) {
+ case 'drop': start += size; break;
+ case 'dropRight': end -= size; break;
+ case 'take': end = nativeMin(end, start + size); break;
+ case 'takeRight': start = nativeMax(start, end - size); break;
+ }
+ }
+ return { 'start': start, 'end': end };
+}
+
+module.exports = getView;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/indexOfNaN.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/indexOfNaN.js b/node_modules/lodash/internal/indexOfNaN.js
new file mode 100644
index 0000000..05b8207
--- /dev/null
+++ b/node_modules/lodash/internal/indexOfNaN.js
@@ -0,0 +1,23 @@
+/**
+ * Gets the index at which the first occurrence of `NaN` is found in `array`.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {number} fromIndex The index to search from.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched `NaN`, else `-1`.
+ */
+function indexOfNaN(array, fromIndex, fromRight) {
+ var length = array.length,
+ index = fromIndex + (fromRight ? 0 : -1);
+
+ while ((fromRight ? index-- : ++index < length)) {
+ var other = array[index];
+ if (other !== other) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = indexOfNaN;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/initCloneArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/initCloneArray.js b/node_modules/lodash/internal/initCloneArray.js
new file mode 100644
index 0000000..c92dfa2
--- /dev/null
+++ b/node_modules/lodash/internal/initCloneArray.js
@@ -0,0 +1,26 @@
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Initializes an array clone.
+ *
+ * @private
+ * @param {Array} array The array to clone.
+ * @returns {Array} Returns the initialized clone.
+ */
+function initCloneArray(array) {
+ var length = array.length,
+ result = new array.constructor(length);
+
+ // Add array properties assigned by `RegExp#exec`.
+ if (length && typeof array[0] == 'string' && hasOwnProperty.call(array, 'index')) {
+ result.index = array.index;
+ result.input = array.input;
+ }
+ return result;
+}
+
+module.exports = initCloneArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/initCloneByTag.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/initCloneByTag.js b/node_modules/lodash/internal/initCloneByTag.js
new file mode 100644
index 0000000..8e3afc6
--- /dev/null
+++ b/node_modules/lodash/internal/initCloneByTag.js
@@ -0,0 +1,63 @@
+var bufferClone = require('./bufferClone');
+
+/** `Object#toString` result references. */
+var boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ numberTag = '[object Number]',
+ regexpTag = '[object RegExp]',
+ stringTag = '[object String]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/** Used to match `RegExp` flags from their coerced string values. */
+var reFlags = /\w*$/;
+
+/**
+ * Initializes an object clone based on its `toStringTag`.
+ *
+ * **Note:** This function only supports cloning values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @param {string} tag The `toStringTag` of the object to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneByTag(object, tag, isDeep) {
+ var Ctor = object.constructor;
+ switch (tag) {
+ case arrayBufferTag:
+ return bufferClone(object);
+
+ case boolTag:
+ case dateTag:
+ return new Ctor(+object);
+
+ case float32Tag: case float64Tag:
+ case int8Tag: case int16Tag: case int32Tag:
+ case uint8Tag: case uint8ClampedTag: case uint16Tag: case uint32Tag:
+ var buffer = object.buffer;
+ return new Ctor(isDeep ? bufferClone(buffer) : buffer, object.byteOffset, object.length);
+
+ case numberTag:
+ case stringTag:
+ return new Ctor(object);
+
+ case regexpTag:
+ var result = new Ctor(object.source, reFlags.exec(object));
+ result.lastIndex = object.lastIndex;
+ }
+ return result;
+}
+
+module.exports = initCloneByTag;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/initCloneObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/initCloneObject.js b/node_modules/lodash/internal/initCloneObject.js
new file mode 100644
index 0000000..48c4a23
--- /dev/null
+++ b/node_modules/lodash/internal/initCloneObject.js
@@ -0,0 +1,16 @@
+/**
+ * Initializes an object clone.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneObject(object) {
+ var Ctor = object.constructor;
+ if (!(typeof Ctor == 'function' && Ctor instanceof Ctor)) {
+ Ctor = Object;
+ }
+ return new Ctor;
+}
+
+module.exports = initCloneObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/invokePath.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/invokePath.js b/node_modules/lodash/internal/invokePath.js
new file mode 100644
index 0000000..935110f
--- /dev/null
+++ b/node_modules/lodash/internal/invokePath.js
@@ -0,0 +1,26 @@
+var baseGet = require('./baseGet'),
+ baseSlice = require('./baseSlice'),
+ isKey = require('./isKey'),
+ last = require('../array/last'),
+ toPath = require('./toPath');
+
+/**
+ * Invokes the method at `path` on `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array|string} path The path of the method to invoke.
+ * @param {Array} args The arguments to invoke the method with.
+ * @returns {*} Returns the result of the invoked method.
+ */
+function invokePath(object, path, args) {
+ if (object != null && !isKey(path, object)) {
+ path = toPath(path);
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ path = last(path);
+ }
+ var func = object == null ? object : object[path];
+ return func == null ? undefined : func.apply(object, args);
+}
+
+module.exports = invokePath;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isArrayLike.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isArrayLike.js b/node_modules/lodash/internal/isArrayLike.js
new file mode 100644
index 0000000..72443cd
--- /dev/null
+++ b/node_modules/lodash/internal/isArrayLike.js
@@ -0,0 +1,15 @@
+var getLength = require('./getLength'),
+ isLength = require('./isLength');
+
+/**
+ * Checks if `value` is array-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ */
+function isArrayLike(value) {
+ return value != null && isLength(getLength(value));
+}
+
+module.exports = isArrayLike;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/isIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/isIndex.js b/node_modules/lodash/internal/isIndex.js
new file mode 100644
index 0000000..469164b
--- /dev/null
+++ b/node_modules/lodash/internal/isIndex.js
@@ -0,0 +1,24 @@
+/** Used to detect unsigned integer values. */
+var reIsUint = /^\d+$/;
+
+/**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ value = (typeof value == 'number' || reIsUint.test(value)) ? +value : -1;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+ return value > -1 && value % 1 == 0 && value < length;
+}
+
+module.exports = isIndex;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[06/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/examples/test.xml
----------------------------------------------------------------------
diff --git a/node_modules/sax/examples/test.xml b/node_modules/sax/examples/test.xml
new file mode 100644
index 0000000..801292d
--- /dev/null
+++ b/node_modules/sax/examples/test.xml
@@ -0,0 +1,1254 @@
+<?xml version="1.0" encoding="UTF-8"?>
+<!DOCTYPE RootElement [
+ <!ENTITY e SYSTEM "001.ent">
+]>
+<RootElement param="value">
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+ <FirstElement>
+ Some Text
+ </FirstElement>
+ <?some_pi some_attr="some_value"?>
+ <!-- this is a comment -- but this isnt part of the comment -->
+ <!-- this is a comment == and this is a part of the comment -->
+ <!-- this is a comment > and this is also part of the thing -->
+ <!invalid comment>
+ <![CDATA[ this is random stuff. & and < and > are ok in here. ]]>
+ <SecondElement param2="something">
+ Pre-Text & <Inline>Inlined text</Inline> Post-text.
+ 
+ </SecondElement>
+</RootElement>
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/lib/sax.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/lib/sax.js b/node_modules/sax/lib/sax.js
new file mode 100644
index 0000000..17fb08e
--- /dev/null
+++ b/node_modules/sax/lib/sax.js
@@ -0,0 +1,1006 @@
+// wrapper for non-node envs
+;(function (sax) {
+
+sax.parser = function (strict, opt) { return new SAXParser(strict, opt) }
+sax.SAXParser = SAXParser
+sax.SAXStream = SAXStream
+sax.createStream = createStream
+
+// When we pass the MAX_BUFFER_LENGTH position, start checking for buffer overruns.
+// When we check, schedule the next check for MAX_BUFFER_LENGTH - (max(buffer lengths)),
+// since that's the earliest that a buffer overrun could occur. This way, checks are
+// as rare as required, but as often as necessary to ensure never crossing this bound.
+// Furthermore, buffers are only tested at most once per write(), so passing a very
+// large string into write() might have undesirable effects, but this is manageable by
+// the caller, so it is assumed to be safe. Thus, a call to write() may, in the extreme
+// edge case, result in creating at most one complete copy of the string passed in.
+// Set to Infinity to have unlimited buffers.
+sax.MAX_BUFFER_LENGTH = 64 * 1024
+
+var buffers = [
+ "comment", "sgmlDecl", "textNode", "tagName", "doctype",
+ "procInstName", "procInstBody", "entity", "attribName",
+ "attribValue", "cdata", "script"
+]
+
+sax.EVENTS = // for discoverability.
+ [ "text"
+ , "processinginstruction"
+ , "sgmldeclaration"
+ , "doctype"
+ , "comment"
+ , "attribute"
+ , "opentag"
+ , "closetag"
+ , "opencdata"
+ , "cdata"
+ , "closecdata"
+ , "error"
+ , "end"
+ , "ready"
+ , "script"
+ , "opennamespace"
+ , "closenamespace"
+ ]
+
+function SAXParser (strict, opt) {
+ if (!(this instanceof SAXParser)) return new SAXParser(strict, opt)
+
+ var parser = this
+ clearBuffers(parser)
+ parser.q = parser.c = ""
+ parser.bufferCheckPosition = sax.MAX_BUFFER_LENGTH
+ parser.opt = opt || {}
+ parser.tagCase = parser.opt.lowercasetags ? "toLowerCase" : "toUpperCase"
+ parser.tags = []
+ parser.closed = parser.closedRoot = parser.sawRoot = false
+ parser.tag = parser.error = null
+ parser.strict = !!strict
+ parser.noscript = !!(strict || parser.opt.noscript)
+ parser.state = S.BEGIN
+ parser.ENTITIES = Object.create(sax.ENTITIES)
+ parser.attribList = []
+
+ // namespaces form a prototype chain.
+ // it always points at the current tag,
+ // which protos to its parent tag.
+ if (parser.opt.xmlns) parser.ns = Object.create(rootNS)
+
+ // mostly just for error reporting
+ parser.position = parser.line = parser.column = 0
+ emit(parser, "onready")
+}
+
+if (!Object.create) Object.create = function (o) {
+ function f () { this.__proto__ = o }
+ f.prototype = o
+ return new f
+}
+
+if (!Object.getPrototypeOf) Object.getPrototypeOf = function (o) {
+ return o.__proto__
+}
+
+if (!Object.keys) Object.keys = function (o) {
+ var a = []
+ for (var i in o) if (o.hasOwnProperty(i)) a.push(i)
+ return a
+}
+
+function checkBufferLength (parser) {
+ var maxAllowed = Math.max(sax.MAX_BUFFER_LENGTH, 10)
+ , maxActual = 0
+ for (var i = 0, l = buffers.length; i < l; i ++) {
+ var len = parser[buffers[i]].length
+ if (len > maxAllowed) {
+ // Text/cdata nodes can get big, and since they're buffered,
+ // we can get here under normal conditions.
+ // Avoid issues by emitting the text node now,
+ // so at least it won't get any bigger.
+ switch (buffers[i]) {
+ case "textNode":
+ closeText(parser)
+ break
+
+ case "cdata":
+ emitNode(parser, "oncdata", parser.cdata)
+ parser.cdata = ""
+ break
+
+ case "script":
+ emitNode(parser, "onscript", parser.script)
+ parser.script = ""
+ break
+
+ default:
+ error(parser, "Max buffer length exceeded: "+buffers[i])
+ }
+ }
+ maxActual = Math.max(maxActual, len)
+ }
+ // schedule the next check for the earliest possible buffer overrun.
+ parser.bufferCheckPosition = (sax.MAX_BUFFER_LENGTH - maxActual)
+ + parser.position
+}
+
+function clearBuffers (parser) {
+ for (var i = 0, l = buffers.length; i < l; i ++) {
+ parser[buffers[i]] = ""
+ }
+}
+
+SAXParser.prototype =
+ { end: function () { end(this) }
+ , write: write
+ , resume: function () { this.error = null; return this }
+ , close: function () { return this.write(null) }
+ , end: function () { return this.write(null) }
+ }
+
+try {
+ var Stream = require("stream").Stream
+} catch (ex) {
+ var Stream = function () {}
+}
+
+
+var streamWraps = sax.EVENTS.filter(function (ev) {
+ return ev !== "error" && ev !== "end"
+})
+
+function createStream (strict, opt) {
+ return new SAXStream(strict, opt)
+}
+
+function SAXStream (strict, opt) {
+ if (!(this instanceof SAXStream)) return new SAXStream(strict, opt)
+
+ Stream.apply(me)
+
+ this._parser = new SAXParser(strict, opt)
+ this.writable = true
+ this.readable = true
+
+
+ var me = this
+
+ this._parser.onend = function () {
+ me.emit("end")
+ }
+
+ this._parser.onerror = function (er) {
+ me.emit("error", er)
+
+ // if didn't throw, then means error was handled.
+ // go ahead and clear error, so we can write again.
+ me._parser.error = null
+ }
+
+ streamWraps.forEach(function (ev) {
+ Object.defineProperty(me, "on" + ev, {
+ get: function () { return me._parser["on" + ev] },
+ set: function (h) {
+ if (!h) {
+ me.removeAllListeners(ev)
+ return me._parser["on"+ev] = h
+ }
+ me.on(ev, h)
+ },
+ enumerable: true,
+ configurable: false
+ })
+ })
+}
+
+SAXStream.prototype = Object.create(Stream.prototype,
+ { constructor: { value: SAXStream } })
+
+SAXStream.prototype.write = function (data) {
+ this._parser.write(data.toString())
+ this.emit("data", data)
+ return true
+}
+
+SAXStream.prototype.end = function (chunk) {
+ if (chunk && chunk.length) this._parser.write(chunk.toString())
+ this._parser.end()
+ return true
+}
+
+SAXStream.prototype.on = function (ev, handler) {
+ var me = this
+ if (!me._parser["on"+ev] && streamWraps.indexOf(ev) !== -1) {
+ me._parser["on"+ev] = function () {
+ var args = arguments.length === 1 ? [arguments[0]]
+ : Array.apply(null, arguments)
+ args.splice(0, 0, ev)
+ me.emit.apply(me, args)
+ }
+ }
+
+ return Stream.prototype.on.call(me, ev, handler)
+}
+
+
+
+// character classes and tokens
+var whitespace = "\r\n\t "
+ // this really needs to be replaced with character classes.
+ // XML allows all manner of ridiculous numbers and digits.
+ , number = "0124356789"
+ , letter = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"
+ // (Letter | "_" | ":")
+ , nameStart = letter+"_:"
+ , nameBody = nameStart+number+"-."
+ , quote = "'\""
+ , entity = number+letter+"#"
+ , attribEnd = whitespace + ">"
+ , CDATA = "[CDATA["
+ , DOCTYPE = "DOCTYPE"
+ , XML_NAMESPACE = "http://www.w3.org/XML/1998/namespace"
+ , XMLNS_NAMESPACE = "http://www.w3.org/2000/xmlns/"
+ , rootNS = { xml: XML_NAMESPACE, xmlns: XMLNS_NAMESPACE }
+
+// turn all the string character sets into character class objects.
+whitespace = charClass(whitespace)
+number = charClass(number)
+letter = charClass(letter)
+nameStart = charClass(nameStart)
+nameBody = charClass(nameBody)
+quote = charClass(quote)
+entity = charClass(entity)
+attribEnd = charClass(attribEnd)
+
+function charClass (str) {
+ return str.split("").reduce(function (s, c) {
+ s[c] = true
+ return s
+ }, {})
+}
+
+function is (charclass, c) {
+ return charclass[c]
+}
+
+function not (charclass, c) {
+ return !charclass[c]
+}
+
+var S = 0
+sax.STATE =
+{ BEGIN : S++
+, TEXT : S++ // general stuff
+, TEXT_ENTITY : S++ // & and such.
+, OPEN_WAKA : S++ // <
+, SGML_DECL : S++ // <!BLARG
+, SGML_DECL_QUOTED : S++ // <!BLARG foo "bar
+, DOCTYPE : S++ // <!DOCTYPE
+, DOCTYPE_QUOTED : S++ // <!DOCTYPE "//blah
+, DOCTYPE_DTD : S++ // <!DOCTYPE "//blah" [ ...
+, DOCTYPE_DTD_QUOTED : S++ // <!DOCTYPE "//blah" [ "foo
+, COMMENT_STARTING : S++ // <!-
+, COMMENT : S++ // <!--
+, COMMENT_ENDING : S++ // <!-- blah -
+, COMMENT_ENDED : S++ // <!-- blah --
+, CDATA : S++ // <![CDATA[ something
+, CDATA_ENDING : S++ // ]
+, CDATA_ENDING_2 : S++ // ]]
+, PROC_INST : S++ // <?hi
+, PROC_INST_BODY : S++ // <?hi there
+, PROC_INST_QUOTED : S++ // <?hi "there
+, PROC_INST_ENDING : S++ // <?hi "there" ?
+, OPEN_TAG : S++ // <strong
+, OPEN_TAG_SLASH : S++ // <strong /
+, ATTRIB : S++ // <a
+, ATTRIB_NAME : S++ // <a foo
+, ATTRIB_NAME_SAW_WHITE : S++ // <a foo _
+, ATTRIB_VALUE : S++ // <a foo=
+, ATTRIB_VALUE_QUOTED : S++ // <a foo="bar
+, ATTRIB_VALUE_UNQUOTED : S++ // <a foo=bar
+, ATTRIB_VALUE_ENTITY_Q : S++ // <foo bar="""
+, ATTRIB_VALUE_ENTITY_U : S++ // <foo bar="
+, CLOSE_TAG : S++ // </a
+, CLOSE_TAG_SAW_WHITE : S++ // </a >
+, SCRIPT : S++ // <script> ...
+, SCRIPT_ENDING : S++ // <script> ... <
+}
+
+sax.ENTITIES =
+{ "apos" : "'"
+, "quot" : "\""
+, "amp" : "&"
+, "gt" : ">"
+, "lt" : "<"
+}
+
+for (var S in sax.STATE) sax.STATE[sax.STATE[S]] = S
+
+// shorthand
+S = sax.STATE
+
+function emit (parser, event, data) {
+ parser[event] && parser[event](data)
+}
+
+function emitNode (parser, nodeType, data) {
+ if (parser.textNode) closeText(parser)
+ emit(parser, nodeType, data)
+}
+
+function closeText (parser) {
+ parser.textNode = textopts(parser.opt, parser.textNode)
+ if (parser.textNode) emit(parser, "ontext", parser.textNode)
+ parser.textNode = ""
+}
+
+function textopts (opt, text) {
+ if (opt.trim) text = text.trim()
+ if (opt.normalize) text = text.replace(/\s+/g, " ")
+ return text
+}
+
+function error (parser, er) {
+ closeText(parser)
+ er += "\nLine: "+parser.line+
+ "\nColumn: "+parser.column+
+ "\nChar: "+parser.c
+ er = new Error(er)
+ parser.error = er
+ emit(parser, "onerror", er)
+ return parser
+}
+
+function end (parser) {
+ if (parser.state !== S.TEXT) error(parser, "Unexpected end")
+ closeText(parser)
+ parser.c = ""
+ parser.closed = true
+ emit(parser, "onend")
+ SAXParser.call(parser, parser.strict, parser.opt)
+ return parser
+}
+
+function strictFail (parser, message) {
+ if (parser.strict) error(parser, message)
+}
+
+function newTag (parser) {
+ if (!parser.strict) parser.tagName = parser.tagName[parser.tagCase]()
+ var parent = parser.tags[parser.tags.length - 1] || parser
+ , tag = parser.tag = { name : parser.tagName, attributes : {} }
+
+ // will be overridden if tag contails an xmlns="foo" or xmlns:foo="bar"
+ if (parser.opt.xmlns) tag.ns = parent.ns
+ parser.attribList.length = 0
+}
+
+function qname (name) {
+ var i = name.indexOf(":")
+ , qualName = i < 0 ? [ "", name ] : name.split(":")
+ , prefix = qualName[0]
+ , local = qualName[1]
+
+ // <x "xmlns"="http://foo">
+ if (name === "xmlns") {
+ prefix = "xmlns"
+ local = ""
+ }
+
+ return { prefix: prefix, local: local }
+}
+
+function attrib (parser) {
+ if (parser.opt.xmlns) {
+ var qn = qname(parser.attribName)
+ , prefix = qn.prefix
+ , local = qn.local
+
+ if (prefix === "xmlns") {
+ // namespace binding attribute; push the binding into scope
+ if (local === "xml" && parser.attribValue !== XML_NAMESPACE) {
+ strictFail( parser
+ , "xml: prefix must be bound to " + XML_NAMESPACE + "\n"
+ + "Actual: " + parser.attribValue )
+ } else if (local === "xmlns" && parser.attribValue !== XMLNS_NAMESPACE) {
+ strictFail( parser
+ , "xmlns: prefix must be bound to " + XMLNS_NAMESPACE + "\n"
+ + "Actual: " + parser.attribValue )
+ } else {
+ var tag = parser.tag
+ , parent = parser.tags[parser.tags.length - 1] || parser
+ if (tag.ns === parent.ns) {
+ tag.ns = Object.create(parent.ns)
+ }
+ tag.ns[local] = parser.attribValue
+ }
+ }
+
+ // defer onattribute events until all attributes have been seen
+ // so any new bindings can take effect; preserve attribute order
+ // so deferred events can be emitted in document order
+ parser.attribList.push([parser.attribName, parser.attribValue])
+ } else {
+ // in non-xmlns mode, we can emit the event right away
+ parser.tag.attributes[parser.attribName] = parser.attribValue
+ emitNode( parser
+ , "onattribute"
+ , { name: parser.attribName
+ , value: parser.attribValue } )
+ }
+
+ parser.attribName = parser.attribValue = ""
+}
+
+function openTag (parser, selfClosing) {
+ if (parser.opt.xmlns) {
+ // emit namespace binding events
+ var tag = parser.tag
+
+ // add namespace info to tag
+ var qn = qname(parser.tagName)
+ tag.prefix = qn.prefix
+ tag.local = qn.local
+ tag.uri = tag.ns[qn.prefix] || qn.prefix
+
+ if (tag.prefix && !tag.uri) {
+ strictFail(parser, "Unbound namespace prefix: "
+ + JSON.stringify(parser.tagName))
+ }
+
+ var parent = parser.tags[parser.tags.length - 1] || parser
+ if (tag.ns && parent.ns !== tag.ns) {
+ Object.keys(tag.ns).forEach(function (p) {
+ emitNode( parser
+ , "onopennamespace"
+ , { prefix: p , uri: tag.ns[p] } )
+ })
+ }
+
+ // handle deferred onattribute events
+ for (var i = 0, l = parser.attribList.length; i < l; i ++) {
+ var nv = parser.attribList[i]
+ var name = nv[0]
+ , value = nv[1]
+ , qualName = qname(name)
+ , prefix = qualName.prefix
+ , local = qualName.local
+ , uri = tag.ns[prefix] || ""
+ , a = { name: name
+ , value: value
+ , prefix: prefix
+ , local: local
+ , uri: uri
+ }
+
+ // if there's any attributes with an undefined namespace,
+ // then fail on them now.
+ if (prefix && prefix != "xmlns" && !uri) {
+ strictFail(parser, "Unbound namespace prefix: "
+ + JSON.stringify(prefix))
+ a.uri = prefix
+ }
+ parser.tag.attributes[name] = a
+ emitNode(parser, "onattribute", a)
+ }
+ parser.attribList.length = 0
+ }
+
+ // process the tag
+ parser.sawRoot = true
+ parser.tags.push(parser.tag)
+ emitNode(parser, "onopentag", parser.tag)
+ if (!selfClosing) {
+ // special case for <script> in non-strict mode.
+ if (!parser.noscript && parser.tagName.toLowerCase() === "script") {
+ parser.state = S.SCRIPT
+ } else {
+ parser.state = S.TEXT
+ }
+ parser.tag = null
+ parser.tagName = ""
+ }
+ parser.attribName = parser.attribValue = ""
+ parser.attribList.length = 0
+}
+
+function closeTag (parser) {
+ if (!parser.tagName) {
+ strictFail(parser, "Weird empty close tag.")
+ parser.textNode += "</>"
+ parser.state = S.TEXT
+ return
+ }
+ // first make sure that the closing tag actually exists.
+ // <a><b></c></b></a> will close everything, otherwise.
+ var t = parser.tags.length
+ var tagName = parser.tagName
+ if (!parser.strict) tagName = tagName[parser.tagCase]()
+ var closeTo = tagName
+ while (t --) {
+ var close = parser.tags[t]
+ if (close.name !== closeTo) {
+ // fail the first time in strict mode
+ strictFail(parser, "Unexpected close tag")
+ } else break
+ }
+
+ // didn't find it. we already failed for strict, so just abort.
+ if (t < 0) {
+ strictFail(parser, "Unmatched closing tag: "+parser.tagName)
+ parser.textNode += "</" + parser.tagName + ">"
+ parser.state = S.TEXT
+ return
+ }
+ parser.tagName = tagName
+ var s = parser.tags.length
+ while (s --> t) {
+ var tag = parser.tag = parser.tags.pop()
+ parser.tagName = parser.tag.name
+ emitNode(parser, "onclosetag", parser.tagName)
+
+ var x = {}
+ for (var i in tag.ns) x[i] = tag.ns[i]
+
+ var parent = parser.tags[parser.tags.length - 1] || parser
+ if (parser.opt.xmlns && tag.ns !== parent.ns) {
+ // remove namespace bindings introduced by tag
+ Object.keys(tag.ns).forEach(function (p) {
+ var n = tag.ns[p]
+ emitNode(parser, "onclosenamespace", { prefix: p, uri: n })
+ })
+ }
+ }
+ if (t === 0) parser.closedRoot = true
+ parser.tagName = parser.attribValue = parser.attribName = ""
+ parser.attribList.length = 0
+ parser.state = S.TEXT
+}
+
+function parseEntity (parser) {
+ var entity = parser.entity.toLowerCase()
+ , num
+ , numStr = ""
+ if (parser.ENTITIES[entity]) return parser.ENTITIES[entity]
+ if (entity.charAt(0) === "#") {
+ if (entity.charAt(1) === "x") {
+ entity = entity.slice(2)
+ num = parseInt(entity, 16)
+ numStr = num.toString(16)
+ } else {
+ entity = entity.slice(1)
+ num = parseInt(entity, 10)
+ numStr = num.toString(10)
+ }
+ }
+ entity = entity.replace(/^0+/, "")
+ if (numStr.toLowerCase() !== entity) {
+ strictFail(parser, "Invalid character entity")
+ return "&"+parser.entity + ";"
+ }
+ return String.fromCharCode(num)
+}
+
+function write (chunk) {
+ var parser = this
+ if (this.error) throw this.error
+ if (parser.closed) return error(parser,
+ "Cannot write after close. Assign an onready handler.")
+ if (chunk === null) return end(parser)
+ var i = 0, c = ""
+ while (parser.c = c = chunk.charAt(i++)) {
+ parser.position ++
+ if (c === "\n") {
+ parser.line ++
+ parser.column = 0
+ } else parser.column ++
+ switch (parser.state) {
+
+ case S.BEGIN:
+ if (c === "<") parser.state = S.OPEN_WAKA
+ else if (not(whitespace,c)) {
+ // have to process this as a text node.
+ // weird, but happens.
+ strictFail(parser, "Non-whitespace before first tag.")
+ parser.textNode = c
+ parser.state = S.TEXT
+ }
+ continue
+
+ case S.TEXT:
+ if (parser.sawRoot && !parser.closedRoot) {
+ var starti = i-1
+ while (c && c!=="<" && c!=="&") {
+ c = chunk.charAt(i++)
+ if (c) {
+ parser.position ++
+ if (c === "\n") {
+ parser.line ++
+ parser.column = 0
+ } else parser.column ++
+ }
+ }
+ parser.textNode += chunk.substring(starti, i-1)
+ }
+ if (c === "<") parser.state = S.OPEN_WAKA
+ else {
+ if (not(whitespace, c) && (!parser.sawRoot || parser.closedRoot))
+ strictFail("Text data outside of root node.")
+ if (c === "&") parser.state = S.TEXT_ENTITY
+ else parser.textNode += c
+ }
+ continue
+
+ case S.SCRIPT:
+ // only non-strict
+ if (c === "<") {
+ parser.state = S.SCRIPT_ENDING
+ } else parser.script += c
+ continue
+
+ case S.SCRIPT_ENDING:
+ if (c === "/") {
+ emitNode(parser, "onscript", parser.script)
+ parser.state = S.CLOSE_TAG
+ parser.script = ""
+ parser.tagName = ""
+ } else {
+ parser.script += "<" + c
+ parser.state = S.SCRIPT
+ }
+ continue
+
+ case S.OPEN_WAKA:
+ // either a /, ?, !, or text is coming next.
+ if (c === "!") {
+ parser.state = S.SGML_DECL
+ parser.sgmlDecl = ""
+ } else if (is(whitespace, c)) {
+ // wait for it...
+ } else if (is(nameStart,c)) {
+ parser.startTagPosition = parser.position - 1
+ parser.state = S.OPEN_TAG
+ parser.tagName = c
+ } else if (c === "/") {
+ parser.startTagPosition = parser.position - 1
+ parser.state = S.CLOSE_TAG
+ parser.tagName = ""
+ } else if (c === "?") {
+ parser.state = S.PROC_INST
+ parser.procInstName = parser.procInstBody = ""
+ } else {
+ strictFail(parser, "Unencoded <")
+ parser.textNode += "<" + c
+ parser.state = S.TEXT
+ }
+ continue
+
+ case S.SGML_DECL:
+ if ((parser.sgmlDecl+c).toUpperCase() === CDATA) {
+ emitNode(parser, "onopencdata")
+ parser.state = S.CDATA
+ parser.sgmlDecl = ""
+ parser.cdata = ""
+ } else if (parser.sgmlDecl+c === "--") {
+ parser.state = S.COMMENT
+ parser.comment = ""
+ parser.sgmlDecl = ""
+ } else if ((parser.sgmlDecl+c).toUpperCase() === DOCTYPE) {
+ parser.state = S.DOCTYPE
+ if (parser.doctype || parser.sawRoot) strictFail(parser,
+ "Inappropriately located doctype declaration")
+ parser.doctype = ""
+ parser.sgmlDecl = ""
+ } else if (c === ">") {
+ emitNode(parser, "onsgmldeclaration", parser.sgmlDecl)
+ parser.sgmlDecl = ""
+ parser.state = S.TEXT
+ } else if (is(quote, c)) {
+ parser.state = S.SGML_DECL_QUOTED
+ parser.sgmlDecl += c
+ } else parser.sgmlDecl += c
+ continue
+
+ case S.SGML_DECL_QUOTED:
+ if (c === parser.q) {
+ parser.state = S.SGML_DECL
+ parser.q = ""
+ }
+ parser.sgmlDecl += c
+ continue
+
+ case S.DOCTYPE:
+ if (c === ">") {
+ parser.state = S.TEXT
+ emitNode(parser, "ondoctype", parser.doctype)
+ parser.doctype = true // just remember that we saw it.
+ } else {
+ parser.doctype += c
+ if (c === "[") parser.state = S.DOCTYPE_DTD
+ else if (is(quote, c)) {
+ parser.state = S.DOCTYPE_QUOTED
+ parser.q = c
+ }
+ }
+ continue
+
+ case S.DOCTYPE_QUOTED:
+ parser.doctype += c
+ if (c === parser.q) {
+ parser.q = ""
+ parser.state = S.DOCTYPE
+ }
+ continue
+
+ case S.DOCTYPE_DTD:
+ parser.doctype += c
+ if (c === "]") parser.state = S.DOCTYPE
+ else if (is(quote,c)) {
+ parser.state = S.DOCTYPE_DTD_QUOTED
+ parser.q = c
+ }
+ continue
+
+ case S.DOCTYPE_DTD_QUOTED:
+ parser.doctype += c
+ if (c === parser.q) {
+ parser.state = S.DOCTYPE_DTD
+ parser.q = ""
+ }
+ continue
+
+ case S.COMMENT:
+ if (c === "-") parser.state = S.COMMENT_ENDING
+ else parser.comment += c
+ continue
+
+ case S.COMMENT_ENDING:
+ if (c === "-") {
+ parser.state = S.COMMENT_ENDED
+ parser.comment = textopts(parser.opt, parser.comment)
+ if (parser.comment) emitNode(parser, "oncomment", parser.comment)
+ parser.comment = ""
+ } else {
+ parser.comment += "-" + c
+ parser.state = S.COMMENT
+ }
+ continue
+
+ case S.COMMENT_ENDED:
+ if (c !== ">") {
+ strictFail(parser, "Malformed comment")
+ // allow <!-- blah -- bloo --> in non-strict mode,
+ // which is a comment of " blah -- bloo "
+ parser.comment += "--" + c
+ parser.state = S.COMMENT
+ } else parser.state = S.TEXT
+ continue
+
+ case S.CDATA:
+ if (c === "]") parser.state = S.CDATA_ENDING
+ else parser.cdata += c
+ continue
+
+ case S.CDATA_ENDING:
+ if (c === "]") parser.state = S.CDATA_ENDING_2
+ else {
+ parser.cdata += "]" + c
+ parser.state = S.CDATA
+ }
+ continue
+
+ case S.CDATA_ENDING_2:
+ if (c === ">") {
+ if (parser.cdata) emitNode(parser, "oncdata", parser.cdata)
+ emitNode(parser, "onclosecdata")
+ parser.cdata = ""
+ parser.state = S.TEXT
+ } else if (c === "]") {
+ parser.cdata += "]"
+ } else {
+ parser.cdata += "]]" + c
+ parser.state = S.CDATA
+ }
+ continue
+
+ case S.PROC_INST:
+ if (c === "?") parser.state = S.PROC_INST_ENDING
+ else if (is(whitespace, c)) parser.state = S.PROC_INST_BODY
+ else parser.procInstName += c
+ continue
+
+ case S.PROC_INST_BODY:
+ if (!parser.procInstBody && is(whitespace, c)) continue
+ else if (c === "?") parser.state = S.PROC_INST_ENDING
+ else if (is(quote, c)) {
+ parser.state = S.PROC_INST_QUOTED
+ parser.q = c
+ parser.procInstBody += c
+ } else parser.procInstBody += c
+ continue
+
+ case S.PROC_INST_ENDING:
+ if (c === ">") {
+ emitNode(parser, "onprocessinginstruction", {
+ name : parser.procInstName,
+ body : parser.procInstBody
+ })
+ parser.procInstName = parser.procInstBody = ""
+ parser.state = S.TEXT
+ } else {
+ parser.procInstBody += "?" + c
+ parser.state = S.PROC_INST_BODY
+ }
+ continue
+
+ case S.PROC_INST_QUOTED:
+ parser.procInstBody += c
+ if (c === parser.q) {
+ parser.state = S.PROC_INST_BODY
+ parser.q = ""
+ }
+ continue
+
+ case S.OPEN_TAG:
+ if (is(nameBody, c)) parser.tagName += c
+ else {
+ newTag(parser)
+ if (c === ">") openTag(parser)
+ else if (c === "/") parser.state = S.OPEN_TAG_SLASH
+ else {
+ if (not(whitespace, c)) strictFail(
+ parser, "Invalid character in tag name")
+ parser.state = S.ATTRIB
+ }
+ }
+ continue
+
+ case S.OPEN_TAG_SLASH:
+ if (c === ">") {
+ openTag(parser, true)
+ closeTag(parser)
+ } else {
+ strictFail(parser, "Forward-slash in opening tag not followed by >")
+ parser.state = S.ATTRIB
+ }
+ continue
+
+ case S.ATTRIB:
+ // haven't read the attribute name yet.
+ if (is(whitespace, c)) continue
+ else if (c === ">") openTag(parser)
+ else if (c === "/") parser.state = S.OPEN_TAG_SLASH
+ else if (is(nameStart, c)) {
+ parser.attribName = c
+ parser.attribValue = ""
+ parser.state = S.ATTRIB_NAME
+ } else strictFail(parser, "Invalid attribute name")
+ continue
+
+ case S.ATTRIB_NAME:
+ if (c === "=") parser.state = S.ATTRIB_VALUE
+ else if (is(whitespace, c)) parser.state = S.ATTRIB_NAME_SAW_WHITE
+ else if (is(nameBody, c)) parser.attribName += c
+ else strictFail(parser, "Invalid attribute name")
+ continue
+
+ case S.ATTRIB_NAME_SAW_WHITE:
+ if (c === "=") parser.state = S.ATTRIB_VALUE
+ else if (is(whitespace, c)) continue
+ else {
+ strictFail(parser, "Attribute without value")
+ parser.tag.attributes[parser.attribName] = ""
+ parser.attribValue = ""
+ emitNode(parser, "onattribute",
+ { name : parser.attribName, value : "" })
+ parser.attribName = ""
+ if (c === ">") openTag(parser)
+ else if (is(nameStart, c)) {
+ parser.attribName = c
+ parser.state = S.ATTRIB_NAME
+ } else {
+ strictFail(parser, "Invalid attribute name")
+ parser.state = S.ATTRIB
+ }
+ }
+ continue
+
+ case S.ATTRIB_VALUE:
+ if (is(whitespace, c)) continue
+ else if (is(quote, c)) {
+ parser.q = c
+ parser.state = S.ATTRIB_VALUE_QUOTED
+ } else {
+ strictFail(parser, "Unquoted attribute value")
+ parser.state = S.ATTRIB_VALUE_UNQUOTED
+ parser.attribValue = c
+ }
+ continue
+
+ case S.ATTRIB_VALUE_QUOTED:
+ if (c !== parser.q) {
+ if (c === "&") parser.state = S.ATTRIB_VALUE_ENTITY_Q
+ else parser.attribValue += c
+ continue
+ }
+ attrib(parser)
+ parser.q = ""
+ parser.state = S.ATTRIB
+ continue
+
+ case S.ATTRIB_VALUE_UNQUOTED:
+ if (not(attribEnd,c)) {
+ if (c === "&") parser.state = S.ATTRIB_VALUE_ENTITY_U
+ else parser.attribValue += c
+ continue
+ }
+ attrib(parser)
+ if (c === ">") openTag(parser)
+ else parser.state = S.ATTRIB
+ continue
+
+ case S.CLOSE_TAG:
+ if (!parser.tagName) {
+ if (is(whitespace, c)) continue
+ else if (not(nameStart, c)) strictFail(parser,
+ "Invalid tagname in closing tag.")
+ else parser.tagName = c
+ }
+ else if (c === ">") closeTag(parser)
+ else if (is(nameBody, c)) parser.tagName += c
+ else {
+ if (not(whitespace, c)) strictFail(parser,
+ "Invalid tagname in closing tag")
+ parser.state = S.CLOSE_TAG_SAW_WHITE
+ }
+ continue
+
+ case S.CLOSE_TAG_SAW_WHITE:
+ if (is(whitespace, c)) continue
+ if (c === ">") closeTag(parser)
+ else strictFail("Invalid characters in closing tag")
+ continue
+
+ case S.TEXT_ENTITY:
+ case S.ATTRIB_VALUE_ENTITY_Q:
+ case S.ATTRIB_VALUE_ENTITY_U:
+ switch(parser.state) {
+ case S.TEXT_ENTITY:
+ var returnState = S.TEXT, buffer = "textNode"
+ break
+
+ case S.ATTRIB_VALUE_ENTITY_Q:
+ var returnState = S.ATTRIB_VALUE_QUOTED, buffer = "attribValue"
+ break
+
+ case S.ATTRIB_VALUE_ENTITY_U:
+ var returnState = S.ATTRIB_VALUE_UNQUOTED, buffer = "attribValue"
+ break
+ }
+ if (c === ";") {
+ parser[buffer] += parseEntity(parser)
+ parser.entity = ""
+ parser.state = returnState
+ }
+ else if (is(entity, c)) parser.entity += c
+ else {
+ strictFail("Invalid character entity")
+ parser[buffer] += "&" + parser.entity + c
+ parser.entity = ""
+ parser.state = returnState
+ }
+ continue
+
+ default:
+ throw new Error(parser, "Unknown state: " + parser.state)
+ }
+ } // while
+ // cdata blocks can get very big under normal conditions. emit and move on.
+ // if (parser.state === S.CDATA && parser.cdata) {
+ // emitNode(parser, "oncdata", parser.cdata)
+ // parser.cdata = ""
+ // }
+ if (parser.position >= parser.bufferCheckPosition) checkBufferLength(parser)
+ return parser
+}
+
+})(typeof exports === "undefined" ? sax = {} : exports)
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/package.json
----------------------------------------------------------------------
diff --git a/node_modules/sax/package.json b/node_modules/sax/package.json
new file mode 100644
index 0000000..d0b601c
--- /dev/null
+++ b/node_modules/sax/package.json
@@ -0,0 +1,114 @@
+{
+ "_args": [
+ [
+ "sax@0.3.5",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/elementtree"
+ ]
+ ],
+ "_defaultsLoaded": true,
+ "_engineSupported": true,
+ "_from": "sax@0.3.5",
+ "_id": "sax@0.3.5",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/sax",
+ "_nodeVersion": "v0.6.7-pre",
+ "_npmUser": {
+ "email": "i@izs.me",
+ "name": "isaacs"
+ },
+ "_npmVersion": "1.1.0-beta-7",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "sax",
+ "raw": "sax@0.3.5",
+ "rawSpec": "0.3.5",
+ "scope": null,
+ "spec": "0.3.5",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/elementtree"
+ ],
+ "_resolved": "http://registry.npmjs.org/sax/-/sax-0.3.5.tgz",
+ "_shasum": "88fcfc1f73c0c8bbd5b7c776b6d3f3501eed073d",
+ "_shrinkwrap": null,
+ "_spec": "sax@0.3.5",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/elementtree",
+ "author": {
+ "email": "i@izs.me",
+ "name": "Isaac Z. Schlueter",
+ "url": "http://blog.izs.me/"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/sax-js/issues"
+ },
+ "contributors": [
+ {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me"
+ },
+ {
+ "name": "Stein Martin Hustad",
+ "email": "stein@hustad.com"
+ },
+ {
+ "name": "Mikeal Rogers",
+ "email": "mikeal.rogers@gmail.com"
+ },
+ {
+ "name": "Laurie Harper",
+ "email": "laurie@holoweb.net"
+ },
+ {
+ "name": "Jann Horn",
+ "email": "jann@Jann-PC.fritz.box"
+ },
+ {
+ "name": "Elijah Insua",
+ "email": "tmpvar@gmail.com"
+ },
+ {
+ "name": "Henry Rawas",
+ "email": "henryr@schakra.com"
+ },
+ {
+ "name": "Justin Makeig",
+ "email": "jmpublic@makeig.com"
+ }
+ ],
+ "dependencies": {},
+ "description": "An evented streaming XML parser in JavaScript",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "88fcfc1f73c0c8bbd5b7c776b6d3f3501eed073d",
+ "tarball": "http://registry.npmjs.org/sax/-/sax-0.3.5.tgz"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "homepage": "https://github.com/isaacs/sax-js#readme",
+ "license": {
+ "type": "MIT",
+ "url": "https://raw.github.com/isaacs/sax-js/master/LICENSE"
+ },
+ "main": "lib/sax.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "name": "sax",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/sax-js.git"
+ },
+ "scripts": {
+ "test": "node test/index.js"
+ },
+ "version": "0.3.5"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/buffer-overrun.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/buffer-overrun.js b/node_modules/sax/test/buffer-overrun.js
new file mode 100644
index 0000000..8d12fac
--- /dev/null
+++ b/node_modules/sax/test/buffer-overrun.js
@@ -0,0 +1,25 @@
+// set this really low so that I don't have to put 64 MB of xml in here.
+var sax = require("../lib/sax")
+var bl = sax.MAX_BUFFER_LENGTH
+sax.MAX_BUFFER_LENGTH = 5;
+
+require(__dirname).test({
+ expect : [
+ ["error", "Max buffer length exceeded: tagName\nLine: 0\nColumn: 15\nChar: "],
+ ["error", "Max buffer length exceeded: tagName\nLine: 0\nColumn: 30\nChar: "],
+ ["error", "Max buffer length exceeded: tagName\nLine: 0\nColumn: 45\nChar: "],
+ ["opentag", {
+ "name": "ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZ",
+ "attributes": {}
+ }],
+ ["text", "yo"],
+ ["closetag", "ABCDEFGHIJKLMNOPQRSTUVWXYZABCDEFGHIJKLMNOPQRSTUVWXYZ"]
+ ]
+}).write("<abcdefghijklmn")
+ .write("opqrstuvwxyzABC")
+ .write("DEFGHIJKLMNOPQR")
+ .write("STUVWXYZ>")
+ .write("yo")
+ .write("</abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ>")
+ .close();
+sax.MAX_BUFFER_LENGTH = bl
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/cdata-chunked.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/cdata-chunked.js b/node_modules/sax/test/cdata-chunked.js
new file mode 100644
index 0000000..ccd5ee6
--- /dev/null
+++ b/node_modules/sax/test/cdata-chunked.js
@@ -0,0 +1,11 @@
+
+require(__dirname).test({
+ expect : [
+ ["opentag", {"name": "R","attributes": {}}],
+ ["opencdata", undefined],
+ ["cdata", " this is character data "],
+ ["closecdata", undefined],
+ ["closetag", "R"]
+ ]
+}).write("<r><![CDATA[ this is ").write("character data ").write("]]></r>").close();
+
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[19/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/examples/javascript.pegjs
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/examples/javascript.pegjs b/node_modules/pegjs/examples/javascript.pegjs
new file mode 100644
index 0000000..229a582
--- /dev/null
+++ b/node_modules/pegjs/examples/javascript.pegjs
@@ -0,0 +1,1530 @@
+/*
+ * JavaScript parser based on the grammar described in ECMA-262, 5th ed.
+ * (http://www.ecma-international.org/publications/standards/Ecma-262.htm)
+ *
+ * The parser builds a tree representing the parsed JavaScript, composed of
+ * basic JavaScript values, arrays and objects (basically JSON). It can be
+ * easily used by various JavaScript processors, transformers, etc.
+ *
+ * Intentional deviations from ECMA-262, 5th ed.:
+ *
+ * * The specification does not consider |FunctionDeclaration| and
+ * |FunctionExpression| as statements, but JavaScript implementations do and
+ * so are we. This syntax is actually used in the wild (e.g. by jQuery).
+ *
+ * Limitations:
+ *
+ * * Non-BMP characters are completely ignored to avoid surrogate
+ * pair handling (JavaScript strings in most implementations are AFAIK
+ * encoded in UTF-16, though this is not required by the specification --
+ * see ECMA-262, 5th ed., 4.3.16).
+ *
+ * * One can create identifiers containing illegal characters using Unicode
+ * escape sequences. For example, "abcd\u0020efgh" is not a valid
+ * identifier, but it is accepted by the parser.
+ *
+ * * Strict mode is not recognized. That means that within strict mode code,
+ * "implements", "interface", "let", "package", "private", "protected",
+ * "public", "static" and "yield" can be used as names. Many other
+ * restrictions and exceptions from ECMA-262, 5th ed., Annex C are also not
+ * applied.
+ *
+ * * The parser does not handle regular expression literal syntax (basically,
+ * it treats anything between "/"'s as an opaque character sequence and also
+ * does not recognize invalid flags properly).
+ *
+ * * The parser doesn't report any early errors except syntax errors (see
+ * ECMA-262, 5th ed., 16).
+ *
+ * At least some of these limitations should be fixed sometimes.
+ *
+ * Many thanks to inimino (http://inimino.org/~inimino/blog/) for his ES5 PEG
+ * (http://boshi.inimino.org/3box/asof/1270029991384/PEG/ECMAScript_unified.peg),
+ * which helped me to solve some problems (such as automatic semicolon
+ * insertion) and also served to double check that I converted the original
+ * grammar correctly.
+ */
+
+start
+ = __ program:Program __ { return program; }
+
+/* ===== A.1 Lexical Grammar ===== */
+
+SourceCharacter
+ = .
+
+WhiteSpace "whitespace"
+ = [\t\v\f \u00A0\uFEFF]
+ / Zs
+
+LineTerminator
+ = [\n\r\u2028\u2029]
+
+LineTerminatorSequence "end of line"
+ = "\n"
+ / "\r\n"
+ / "\r"
+ / "\u2028" // line spearator
+ / "\u2029" // paragraph separator
+
+Comment "comment"
+ = MultiLineComment
+ / SingleLineComment
+
+MultiLineComment
+ = "/*" (!"*/" SourceCharacter)* "*/"
+
+MultiLineCommentNoLineTerminator
+ = "/*" (!("*/" / LineTerminator) SourceCharacter)* "*/"
+
+SingleLineComment
+ = "//" (!LineTerminator SourceCharacter)*
+
+Identifier "identifier"
+ = !ReservedWord name:IdentifierName { return name; }
+
+IdentifierName "identifier"
+ = start:IdentifierStart parts:IdentifierPart* {
+ return start + parts.join("");
+ }
+
+IdentifierStart
+ = UnicodeLetter
+ / "$"
+ / "_"
+ / "\\" sequence:UnicodeEscapeSequence { return sequence; }
+
+IdentifierPart
+ = IdentifierStart
+ / UnicodeCombiningMark
+ / UnicodeDigit
+ / UnicodeConnectorPunctuation
+ / "\u200C" { return "\u200C"; } // zero-width non-joiner
+ / "\u200D" { return "\u200D"; } // zero-width joiner
+
+UnicodeLetter
+ = Lu
+ / Ll
+ / Lt
+ / Lm
+ / Lo
+ / Nl
+
+UnicodeCombiningMark
+ = Mn
+ / Mc
+
+UnicodeDigit
+ = Nd
+
+UnicodeConnectorPunctuation
+ = Pc
+
+ReservedWord
+ = Keyword
+ / FutureReservedWord
+ / NullLiteral
+ / BooleanLiteral
+
+Keyword
+ = (
+ "break"
+ / "case"
+ / "catch"
+ / "continue"
+ / "debugger"
+ / "default"
+ / "delete"
+ / "do"
+ / "else"
+ / "finally"
+ / "for"
+ / "function"
+ / "if"
+ / "instanceof"
+ / "in"
+ / "new"
+ / "return"
+ / "switch"
+ / "this"
+ / "throw"
+ / "try"
+ / "typeof"
+ / "var"
+ / "void"
+ / "while"
+ / "with"
+ )
+ !IdentifierPart
+
+FutureReservedWord
+ = (
+ "class"
+ / "const"
+ / "enum"
+ / "export"
+ / "extends"
+ / "import"
+ / "super"
+ )
+ !IdentifierPart
+
+/*
+ * This rule contains an error in the specification: |RegularExpressionLiteral|
+ * is missing.
+ */
+Literal
+ = NullLiteral
+ / BooleanLiteral
+ / value:NumericLiteral {
+ return {
+ type: "NumericLiteral",
+ value: value
+ };
+ }
+ / value:StringLiteral {
+ return {
+ type: "StringLiteral",
+ value: value
+ };
+ }
+ / RegularExpressionLiteral
+
+NullLiteral
+ = NullToken { return { type: "NullLiteral" }; }
+
+BooleanLiteral
+ = TrueToken { return { type: "BooleanLiteral", value: true }; }
+ / FalseToken { return { type: "BooleanLiteral", value: false }; }
+
+NumericLiteral "number"
+ = literal:(HexIntegerLiteral / DecimalLiteral) !IdentifierStart {
+ return literal;
+ }
+
+DecimalLiteral
+ = before:DecimalIntegerLiteral
+ "."
+ after:DecimalDigits?
+ exponent:ExponentPart? {
+ return parseFloat(before + "." + after + exponent);
+ }
+ / "." after:DecimalDigits exponent:ExponentPart? {
+ return parseFloat("." + after + exponent);
+ }
+ / before:DecimalIntegerLiteral exponent:ExponentPart? {
+ return parseFloat(before + exponent);
+ }
+
+DecimalIntegerLiteral
+ = "0" / digit:NonZeroDigit digits:DecimalDigits? { return digit + digits; }
+
+DecimalDigits
+ = digits:DecimalDigit+ { return digits.join(""); }
+
+DecimalDigit
+ = [0-9]
+
+NonZeroDigit
+ = [1-9]
+
+ExponentPart
+ = indicator:ExponentIndicator integer:SignedInteger {
+ return indicator + integer;
+ }
+
+ExponentIndicator
+ = [eE]
+
+SignedInteger
+ = sign:[-+]? digits:DecimalDigits { return sign + digits; }
+
+HexIntegerLiteral
+ = "0" [xX] digits:HexDigit+ { return parseInt("0x" + digits.join("")); }
+
+HexDigit
+ = [0-9a-fA-F]
+
+StringLiteral "string"
+ = parts:('"' DoubleStringCharacters? '"' / "'" SingleStringCharacters? "'") {
+ return parts[1];
+ }
+
+DoubleStringCharacters
+ = chars:DoubleStringCharacter+ { return chars.join(""); }
+
+SingleStringCharacters
+ = chars:SingleStringCharacter+ { return chars.join(""); }
+
+DoubleStringCharacter
+ = !('"' / "\\" / LineTerminator) char_:SourceCharacter { return char_; }
+ / "\\" sequence:EscapeSequence { return sequence; }
+ / LineContinuation
+
+SingleStringCharacter
+ = !("'" / "\\" / LineTerminator) char_:SourceCharacter { return char_; }
+ / "\\" sequence:EscapeSequence { return sequence; }
+ / LineContinuation
+
+LineContinuation
+ = "\\" sequence:LineTerminatorSequence { return sequence; }
+
+EscapeSequence
+ = CharacterEscapeSequence
+ / "0" !DecimalDigit { return "\0"; }
+ / HexEscapeSequence
+ / UnicodeEscapeSequence
+
+CharacterEscapeSequence
+ = SingleEscapeCharacter
+ / NonEscapeCharacter
+
+SingleEscapeCharacter
+ = char_:['"\\bfnrtv] {
+ return char_
+ .replace("b", "\b")
+ .replace("f", "\f")
+ .replace("n", "\n")
+ .replace("r", "\r")
+ .replace("t", "\t")
+ .replace("v", "\x0B") // IE does not recognize "\v".
+ }
+
+NonEscapeCharacter
+ = (!EscapeCharacter / LineTerminator) char_:SourceCharacter { return char_; }
+
+EscapeCharacter
+ = SingleEscapeCharacter
+ / DecimalDigit
+ / "x"
+ / "u"
+
+HexEscapeSequence
+ = "x" h1:HexDigit h2:HexDigit {
+ return String.fromCharCode(parseInt("0x" + h1 + h2));
+ }
+
+UnicodeEscapeSequence
+ = "u" h1:HexDigit h2:HexDigit h3:HexDigit h4:HexDigit {
+ return String.fromCharCode(parseInt("0x" + h1 + h2 + h3 + h4));
+ }
+
+RegularExpressionLiteral "regular expression"
+ = "/" body:RegularExpressionBody "/" flags:RegularExpressionFlags {
+ return {
+ type: "RegularExpressionLiteral",
+ body: body,
+ flags: flags
+ };
+ }
+
+RegularExpressionBody
+ = char_:RegularExpressionFirstChar chars:RegularExpressionChars {
+ return char_ + chars;
+ }
+
+RegularExpressionChars
+ = chars:RegularExpressionChar* { return chars.join(""); }
+
+RegularExpressionFirstChar
+ = ![*\\/[] char_:RegularExpressionNonTerminator { return char_; }
+ / RegularExpressionBackslashSequence
+ / RegularExpressionClass
+
+RegularExpressionChar
+ = ![\\/[] char_:RegularExpressionNonTerminator { return char_; }
+ / RegularExpressionBackslashSequence
+ / RegularExpressionClass
+
+/*
+ * This rule contains an error in the specification: "NonTerminator" instead of
+ * "RegularExpressionNonTerminator".
+ */
+RegularExpressionBackslashSequence
+ = "\\" char_:RegularExpressionNonTerminator { return "\\" + char_; }
+
+RegularExpressionNonTerminator
+ = !LineTerminator char_:SourceCharacter { return char_; }
+
+RegularExpressionClass
+ = "[" chars:RegularExpressionClassChars "]" { return "[" + chars + "]"; }
+
+RegularExpressionClassChars
+ = chars:RegularExpressionClassChar* { return chars.join(""); }
+
+RegularExpressionClassChar
+ = ![\]\\] char_:RegularExpressionNonTerminator { return char_; }
+ / RegularExpressionBackslashSequence
+
+RegularExpressionFlags
+ = parts:IdentifierPart* { return parts.join(""); }
+
+/* Tokens */
+
+BreakToken = "break" !IdentifierPart
+CaseToken = "case" !IdentifierPart
+CatchToken = "catch" !IdentifierPart
+ContinueToken = "continue" !IdentifierPart
+DebuggerToken = "debugger" !IdentifierPart
+DefaultToken = "default" !IdentifierPart
+DeleteToken = "delete" !IdentifierPart { return "delete"; }
+DoToken = "do" !IdentifierPart
+ElseToken = "else" !IdentifierPart
+FalseToken = "false" !IdentifierPart
+FinallyToken = "finally" !IdentifierPart
+ForToken = "for" !IdentifierPart
+FunctionToken = "function" !IdentifierPart
+GetToken = "get" !IdentifierPart
+IfToken = "if" !IdentifierPart
+InstanceofToken = "instanceof" !IdentifierPart { return "instanceof"; }
+InToken = "in" !IdentifierPart { return "in"; }
+NewToken = "new" !IdentifierPart
+NullToken = "null" !IdentifierPart
+ReturnToken = "return" !IdentifierPart
+SetToken = "set" !IdentifierPart
+SwitchToken = "switch" !IdentifierPart
+ThisToken = "this" !IdentifierPart
+ThrowToken = "throw" !IdentifierPart
+TrueToken = "true" !IdentifierPart
+TryToken = "try" !IdentifierPart
+TypeofToken = "typeof" !IdentifierPart { return "typeof"; }
+VarToken = "var" !IdentifierPart
+VoidToken = "void" !IdentifierPart { return "void"; }
+WhileToken = "while" !IdentifierPart
+WithToken = "with" !IdentifierPart
+
+/*
+ * Unicode Character Categories
+ *
+ * Source: http://www.fileformat.info/info/unicode/category/index.htm
+ */
+
+/*
+ * Non-BMP characters are completely ignored to avoid surrogate pair handling
+ * (JavaScript strings in most implementations are encoded in UTF-16, though
+ * this is not required by the specification -- see ECMA-262, 5th ed., 4.3.16).
+ *
+ * If you ever need to correctly recognize all the characters, please feel free
+ * to implement that and send a patch.
+ */
+
+// Letter, Lowercase
+Ll = [\u0061\u0062\u0063\u0064\u0065\u0066\u0067\u0068\u0069\u006A\u006B\u006C\u006D\u006E\u006F\u0070\u0071\u0072\u0073\u0074\u0075\u0076\u0077\u0078\u0079\u007A\u00AA\u00B5\u00BA\u00DF\u00E0\u00E1\u00E2\u00E3\u00E4\u00E5\u00E6\u00E7\u00E8\u00E9\u00EA\u00EB\u00EC\u00ED\u00EE\u00EF\u00F0\u00F1\u00F2\u00F3\u00F4\u00F5\u00F6\u00F8\u00F9\u00FA\u00FB\u00FC\u00FD\u00FE\u00FF\u0101\u0103\u0105\u0107\u0109\u010B\u010D\u010F\u0111\u0113\u0115\u0117\u0119\u011B\u011D\u011F\u0121\u0123\u0125\u0127\u0129\u012B\u012D\u012F\u0131\u0133\u0135\u0137\u0138\u013A\u013C\u013E\u0140\u0142\u0144\u0146\u0148\u0149\u014B\u014D\u014F\u0151\u0153\u0155\u0157\u0159\u015B\u015D\u015F\u0161\u0163\u0165\u0167\u0169\u016B\u016D\u016F\u0171\u0173\u0175\u0177\u017A\u017C\u017E\u017F\u0180\u0183\u0185\u0188\u018C\u018D\u0192\u0195\u0199\u019A\u019B\u019E\u01A1\u01A3\u01A5\u01A8\u01AA\u01AB\u01AD\u01B0\u01B4\u01B6\u01B9\u01BA\u01BD\u01BE\u01BF\u01C6\u01C9\u01CC\u01CE\u01D0\u01D2\u01D4\u01D6\u01D8\u01DA\u01DC\u01DD\
u01DF\u01E1\u01E3\u01E5\u01E7\u01E9\u01EB\u01ED\u01EF\u01F0\u01F3\u01F5\u01F9\u01FB\u01FD\u01FF\u0201\u0203\u0205\u0207\u0209\u020B\u020D\u020F\u0211\u0213\u0215\u0217\u0219\u021B\u021D\u021F\u0221\u0223\u0225\u0227\u0229\u022B\u022D\u022F\u0231\u0233\u0234\u0235\u0236\u0237\u0238\u0239\u023C\u023F\u0240\u0242\u0247\u0249\u024B\u024D\u024F\u0250\u0251\u0252\u0253\u0254\u0255\u0256\u0257\u0258\u0259\u025A\u025B\u025C\u025D\u025E\u025F\u0260\u0261\u0262\u0263\u0264\u0265\u0266\u0267\u0268\u0269\u026A\u026B\u026C\u026D\u026E\u026F\u0270\u0271\u0272\u0273\u0274\u0275\u0276\u0277\u0278\u0279\u027A\u027B\u027C\u027D\u027E\u027F\u0280\u0281\u0282\u0283\u0284\u0285\u0286\u0287\u0288\u0289\u028A\u028B\u028C\u028D\u028E\u028F\u0290\u0291\u0292\u0293\u0295\u0296\u0297\u0298\u0299\u029A\u029B\u029C\u029D\u029E\u029F\u02A0\u02A1\u02A2\u02A3\u02A4\u02A5\u02A6\u02A7\u02A8\u02A9\u02AA\u02AB\u02AC\u02AD\u02AE\u02AF\u0371\u0373\u0377\u037B\u037C\u037D\u0390\u03AC\u03AD\u03AE\u03AF\u03B0\u03B1\u03B2\u
03B3\u03B4\u03B5\u03B6\u03B7\u03B8\u03B9\u03BA\u03BB\u03BC\u03BD\u03BE\u03BF\u03C0\u03C1\u03C2\u03C3\u03C4\u03C5\u03C6\u03C7\u03C8\u03C9\u03CA\u03CB\u03CC\u03CD\u03CE\u03D0\u03D1\u03D5\u03D6\u03D7\u03D9\u03DB\u03DD\u03DF\u03E1\u03E3\u03E5\u03E7\u03E9\u03EB\u03ED\u03EF\u03F0\u03F1\u03F2\u03F3\u03F5\u03F8\u03FB\u03FC\u0430\u0431\u0432\u0433\u0434\u0435\u0436\u0437\u0438\u0439\u043A\u043B\u043C\u043D\u043E\u043F\u0440\u0441\u0442\u0443\u0444\u0445\u0446\u0447\u0448\u0449\u044A\u044B\u044C\u044D\u044E\u044F\u0450\u0451\u0452\u0453\u0454\u0455\u0456\u0457\u0458\u0459\u045A\u045B\u045C\u045D\u045E\u045F\u0461\u0463\u0465\u0467\u0469\u046B\u046D\u046F\u0471\u0473\u0475\u0477\u0479\u047B\u047D\u047F\u0481\u048B\u048D\u048F\u0491\u0493\u0495\u0497\u0499\u049B\u049D\u049F\u04A1\u04A3\u04A5\u04A7\u04A9\u04AB\u04AD\u04AF\u04B1\u04B3\u04B5\u04B7\u04B9\u04BB\u04BD\u04BF\u04C2\u04C4\u04C6\u04C8\u04CA\u04CC\u04CE\u04CF\u04D1\u04D3\u04D5\u04D7\u04D9\u04DB\u04DD\u04DF\u04E1\u04E3\u04E5\u04E7\u04E9\u0
4EB\u04ED\u04EF\u04F1\u04F3\u04F5\u04F7\u04F9\u04FB\u04FD\u04FF\u0501\u0503\u0505\u0507\u0509\u050B\u050D\u050F\u0511\u0513\u0515\u0517\u0519\u051B\u051D\u051F\u0521\u0523\u0561\u0562\u0563\u0564\u0565\u0566\u0567\u0568\u0569\u056A\u056B\u056C\u056D\u056E\u056F\u0570\u0571\u0572\u0573\u0574\u0575\u0576\u0577\u0578\u0579\u057A\u057B\u057C\u057D\u057E\u057F\u0580\u0581\u0582\u0583\u0584\u0585\u0586\u0587\u1D00\u1D01\u1D02\u1D03\u1D04\u1D05\u1D06\u1D07\u1D08\u1D09\u1D0A\u1D0B\u1D0C\u1D0D\u1D0E\u1D0F\u1D10\u1D11\u1D12\u1D13\u1D14\u1D15\u1D16\u1D17\u1D18\u1D19\u1D1A\u1D1B\u1D1C\u1D1D\u1D1E\u1D1F\u1D20\u1D21\u1D22\u1D23\u1D24\u1D25\u1D26\u1D27\u1D28\u1D29\u1D2A\u1D2B\u1D62\u1D63\u1D64\u1D65\u1D66\u1D67\u1D68\u1D69\u1D6A\u1D6B\u1D6C\u1D6D\u1D6E\u1D6F\u1D70\u1D71\u1D72\u1D73\u1D74\u1D75\u1D76\u1D77\u1D79\u1D7A\u1D7B\u1D7C\u1D7D\u1D7E\u1D7F\u1D80\u1D81\u1D82\u1D83\u1D84\u1D85\u1D86\u1D87\u1D88\u1D89\u1D8A\u1D8B\u1D8C\u1D8D\u1D8E\u1D8F\u1D90\u1D91\u1D92\u1D93\u1D94\u1D95\u1D96\u1D97\u1D98\u1D
99\u1D9A\u1E01\u1E03\u1E05\u1E07\u1E09\u1E0B\u1E0D\u1E0F\u1E11\u1E13\u1E15\u1E17\u1E19\u1E1B\u1E1D\u1E1F\u1E21\u1E23\u1E25\u1E27\u1E29\u1E2B\u1E2D\u1E2F\u1E31\u1E33\u1E35\u1E37\u1E39\u1E3B\u1E3D\u1E3F\u1E41\u1E43\u1E45\u1E47\u1E49\u1E4B\u1E4D\u1E4F\u1E51\u1E53\u1E55\u1E57\u1E59\u1E5B\u1E5D\u1E5F\u1E61\u1E63\u1E65\u1E67\u1E69\u1E6B\u1E6D\u1E6F\u1E71\u1E73\u1E75\u1E77\u1E79\u1E7B\u1E7D\u1E7F\u1E81\u1E83\u1E85\u1E87\u1E89\u1E8B\u1E8D\u1E8F\u1E91\u1E93\u1E95\u1E96\u1E97\u1E98\u1E99\u1E9A\u1E9B\u1E9C\u1E9D\u1E9F\u1EA1\u1EA3\u1EA5\u1EA7\u1EA9\u1EAB\u1EAD\u1EAF\u1EB1\u1EB3\u1EB5\u1EB7\u1EB9\u1EBB\u1EBD\u1EBF\u1EC1\u1EC3\u1EC5\u1EC7\u1EC9\u1ECB\u1ECD\u1ECF\u1ED1\u1ED3\u1ED5\u1ED7\u1ED9\u1EDB\u1EDD\u1EDF\u1EE1\u1EE3\u1EE5\u1EE7\u1EE9\u1EEB\u1EED\u1EEF\u1EF1\u1EF3\u1EF5\u1EF7\u1EF9\u1EFB\u1EFD\u1EFF\u1F00\u1F01\u1F02\u1F03\u1F04\u1F05\u1F06\u1F07\u1F10\u1F11\u1F12\u1F13\u1F14\u1F15\u1F20\u1F21\u1F22\u1F23\u1F24\u1F25\u1F26\u1F27\u1F30\u1F31\u1F32\u1F33\u1F34\u1F35\u1F36\u1F37\u1F40\u1F41\u1F4
2\u1F43\u1F44\u1F45\u1F50\u1F51\u1F52\u1F53\u1F54\u1F55\u1F56\u1F57\u1F60\u1F61\u1F62\u1F63\u1F64\u1F65\u1F66\u1F67\u1F70\u1F71\u1F72\u1F73\u1F74\u1F75\u1F76\u1F77\u1F78\u1F79\u1F7A\u1F7B\u1F7C\u1F7D\u1F80\u1F81\u1F82\u1F83\u1F84\u1F85\u1F86\u1F87\u1F90\u1F91\u1F92\u1F93\u1F94\u1F95\u1F96\u1F97\u1FA0\u1FA1\u1FA2\u1FA3\u1FA4\u1FA5\u1FA6\u1FA7\u1FB0\u1FB1\u1FB2\u1FB3\u1FB4\u1FB6\u1FB7\u1FBE\u1FC2\u1FC3\u1FC4\u1FC6\u1FC7\u1FD0\u1FD1\u1FD2\u1FD3\u1FD6\u1FD7\u1FE0\u1FE1\u1FE2\u1FE3\u1FE4\u1FE5\u1FE6\u1FE7\u1FF2\u1FF3\u1FF4\u1FF6\u1FF7\u2071\u207F\u210A\u210E\u210F\u2113\u212F\u2134\u2139\u213C\u213D\u2146\u2147\u2148\u2149\u214E\u2184\u2C30\u2C31\u2C32\u2C33\u2C34\u2C35\u2C36\u2C37\u2C38\u2C39\u2C3A\u2C3B\u2C3C\u2C3D\u2C3E\u2C3F\u2C40\u2C41\u2C42\u2C43\u2C44\u2C45\u2C46\u2C47\u2C48\u2C49\u2C4A\u2C4B\u2C4C\u2C4D\u2C4E\u2C4F\u2C50\u2C51\u2C52\u2C53\u2C54\u2C55\u2C56\u2C57\u2C58\u2C59\u2C5A\u2C5B\u2C5C\u2C5D\u2C5E\u2C61\u2C65\u2C66\u2C68\u2C6A\u2C6C\u2C71\u2C73\u2C74\u2C76\u2C77\u2C78\u2C79
\u2C7A\u2C7B\u2C7C\u2C81\u2C83\u2C85\u2C87\u2C89\u2C8B\u2C8D\u2C8F\u2C91\u2C93\u2C95\u2C97\u2C99\u2C9B\u2C9D\u2C9F\u2CA1\u2CA3\u2CA5\u2CA7\u2CA9\u2CAB\u2CAD\u2CAF\u2CB1\u2CB3\u2CB5\u2CB7\u2CB9\u2CBB\u2CBD\u2CBF\u2CC1\u2CC3\u2CC5\u2CC7\u2CC9\u2CCB\u2CCD\u2CCF\u2CD1\u2CD3\u2CD5\u2CD7\u2CD9\u2CDB\u2CDD\u2CDF\u2CE1\u2CE3\u2CE4\u2D00\u2D01\u2D02\u2D03\u2D04\u2D05\u2D06\u2D07\u2D08\u2D09\u2D0A\u2D0B\u2D0C\u2D0D\u2D0E\u2D0F\u2D10\u2D11\u2D12\u2D13\u2D14\u2D15\u2D16\u2D17\u2D18\u2D19\u2D1A\u2D1B\u2D1C\u2D1D\u2D1E\u2D1F\u2D20\u2D21\u2D22\u2D23\u2D24\u2D25\uA641\uA643\uA645\uA647\uA649\uA64B\uA64D\uA64F\uA651\uA653\uA655\uA657\uA659\uA65B\uA65D\uA65F\uA663\uA665\uA667\uA669\uA66B\uA66D\uA681\uA683\uA685\uA687\uA689\uA68B\uA68D\uA68F\uA691\uA693\uA695\uA697\uA723\uA725\uA727\uA729\uA72B\uA72D\uA72F\uA730\uA731\uA733\uA735\uA737\uA739\uA73B\uA73D\uA73F\uA741\uA743\uA745\uA747\uA749\uA74B\uA74D\uA74F\uA751\uA753\uA755\uA757\uA759\uA75B\uA75D\uA75F\uA761\uA763\uA765\uA767\uA769\uA76B\uA76D\uA76F\
uA771\uA772\uA773\uA774\uA775\uA776\uA777\uA778\uA77A\uA77C\uA77F\uA781\uA783\uA785\uA787\uA78C\uFB00\uFB01\uFB02\uFB03\uFB04\uFB05\uFB06\uFB13\uFB14\uFB15\uFB16\uFB17\uFF41\uFF42\uFF43\uFF44\uFF45\uFF46\uFF47\uFF48\uFF49\uFF4A\uFF4B\uFF4C\uFF4D\uFF4E\uFF4F\uFF50\uFF51\uFF52\uFF53\uFF54\uFF55\uFF56\uFF57\uFF58\uFF59\uFF5A]
+
+// Letter, Modifier
+Lm = [\u02B0\u02B1\u02B2\u02B3\u02B4\u02B5\u02B6\u02B7\u02B8\u02B9\u02BA\u02BB\u02BC\u02BD\u02BE\u02BF\u02C0\u02C1\u02C6\u02C7\u02C8\u02C9\u02CA\u02CB\u02CC\u02CD\u02CE\u02CF\u02D0\u02D1\u02E0\u02E1\u02E2\u02E3\u02E4\u02EC\u02EE\u0374\u037A\u0559\u0640\u06E5\u06E6\u07F4\u07F5\u07FA\u0971\u0E46\u0EC6\u10FC\u17D7\u1843\u1C78\u1C79\u1C7A\u1C7B\u1C7C\u1C7D\u1D2C\u1D2D\u1D2E\u1D2F\u1D30\u1D31\u1D32\u1D33\u1D34\u1D35\u1D36\u1D37\u1D38\u1D39\u1D3A\u1D3B\u1D3C\u1D3D\u1D3E\u1D3F\u1D40\u1D41\u1D42\u1D43\u1D44\u1D45\u1D46\u1D47\u1D48\u1D49\u1D4A\u1D4B\u1D4C\u1D4D\u1D4E\u1D4F\u1D50\u1D51\u1D52\u1D53\u1D54\u1D55\u1D56\u1D57\u1D58\u1D59\u1D5A\u1D5B\u1D5C\u1D5D\u1D5E\u1D5F\u1D60\u1D61\u1D78\u1D9B\u1D9C\u1D9D\u1D9E\u1D9F\u1DA0\u1DA1\u1DA2\u1DA3\u1DA4\u1DA5\u1DA6\u1DA7\u1DA8\u1DA9\u1DAA\u1DAB\u1DAC\u1DAD\u1DAE\u1DAF\u1DB0\u1DB1\u1DB2\u1DB3\u1DB4\u1DB5\u1DB6\u1DB7\u1DB8\u1DB9\u1DBA\u1DBB\u1DBC\u1DBD\u1DBE\u1DBF\u2090\u2091\u2092\u2093\u2094\u2C7D\u2D6F\u2E2F\u3005\u3031\u3032\u3033\u3034\u3035\u303B\
u309D\u309E\u30FC\u30FD\u30FE\uA015\uA60C\uA67F\uA717\uA718\uA719\uA71A\uA71B\uA71C\uA71D\uA71E\uA71F\uA770\uA788\uFF70\uFF9E\uFF9F]
+
+// Letter, Other
+Lo = [\u01BB\u01C0\u01C1\u01C2\u01C3\u0294\u05D0\u05D1\u05D2\u05D3\u05D4\u05D5\u05D6\u05D7\u05D8\u05D9\u05DA\u05DB\u05DC\u05DD\u05DE\u05DF\u05E0\u05E1\u05E2\u05E3\u05E4\u05E5\u05E6\u05E7\u05E8\u05E9\u05EA\u05F0\u05F1\u05F2\u0621\u0622\u0623\u0624\u0625\u0626\u0627\u0628\u0629\u062A\u062B\u062C\u062D\u062E\u062F\u0630\u0631\u0632\u0633\u0634\u0635\u0636\u0637\u0638\u0639\u063A\u063B\u063C\u063D\u063E\u063F\u0641\u0642\u0643\u0644\u0645\u0646\u0647\u0648\u0649\u064A\u066E\u066F\u0671\u0672\u0673\u0674\u0675\u0676\u0677\u0678\u0679\u067A\u067B\u067C\u067D\u067E\u067F\u0680\u0681\u0682\u0683\u0684\u0685\u0686\u0687\u0688\u0689\u068A\u068B\u068C\u068D\u068E\u068F\u0690\u0691\u0692\u0693\u0694\u0695\u0696\u0697\u0698\u0699\u069A\u069B\u069C\u069D\u069E\u069F\u06A0\u06A1\u06A2\u06A3\u06A4\u06A5\u06A6\u06A7\u06A8\u06A9\u06AA\u06AB\u06AC\u06AD\u06AE\u06AF\u06B0\u06B1\u06B2\u06B3\u06B4\u06B5\u06B6\u06B7\u06B8\u06B9\u06BA\u06BB\u06BC\u06BD\u06BE\u06BF\u06C0\u06C1\u06C2\u06C3\u06C4\u06C5\u06C6\
u06C7\u06C8\u06C9\u06CA\u06CB\u06CC\u06CD\u06CE\u06CF\u06D0\u06D1\u06D2\u06D3\u06D5\u06EE\u06EF\u06FA\u06FB\u06FC\u06FF\u0710\u0712\u0713\u0714\u0715\u0716\u0717\u0718\u0719\u071A\u071B\u071C\u071D\u071E\u071F\u0720\u0721\u0722\u0723\u0724\u0725\u0726\u0727\u0728\u0729\u072A\u072B\u072C\u072D\u072E\u072F\u074D\u074E\u074F\u0750\u0751\u0752\u0753\u0754\u0755\u0756\u0757\u0758\u0759\u075A\u075B\u075C\u075D\u075E\u075F\u0760\u0761\u0762\u0763\u0764\u0765\u0766\u0767\u0768\u0769\u076A\u076B\u076C\u076D\u076E\u076F\u0770\u0771\u0772\u0773\u0774\u0775\u0776\u0777\u0778\u0779\u077A\u077B\u077C\u077D\u077E\u077F\u0780\u0781\u0782\u0783\u0784\u0785\u0786\u0787\u0788\u0789\u078A\u078B\u078C\u078D\u078E\u078F\u0790\u0791\u0792\u0793\u0794\u0795\u0796\u0797\u0798\u0799\u079A\u079B\u079C\u079D\u079E\u079F\u07A0\u07A1\u07A2\u07A3\u07A4\u07A5\u07B1\u07CA\u07CB\u07CC\u07CD\u07CE\u07CF\u07D0\u07D1\u07D2\u07D3\u07D4\u07D5\u07D6\u07D7\u07D8\u07D9\u07DA\u07DB\u07DC\u07DD\u07DE\u07DF\u07E0\u07E1\u07E2\u
07E3\u07E4\u07E5\u07E6\u07E7\u07E8\u07E9\u07EA\u0904\u0905\u0906\u0907\u0908\u0909\u090A\u090B\u090C\u090D\u090E\u090F\u0910\u0911\u0912\u0913\u0914\u0915\u0916\u0917\u0918\u0919\u091A\u091B\u091C\u091D\u091E\u091F\u0920\u0921\u0922\u0923\u0924\u0925\u0926\u0927\u0928\u0929\u092A\u092B\u092C\u092D\u092E\u092F\u0930\u0931\u0932\u0933\u0934\u0935\u0936\u0937\u0938\u0939\u093D\u0950\u0958\u0959\u095A\u095B\u095C\u095D\u095E\u095F\u0960\u0961\u0972\u097B\u097C\u097D\u097E\u097F\u0985\u0986\u0987\u0988\u0989\u098A\u098B\u098C\u098F\u0990\u0993\u0994\u0995\u0996\u0997\u0998\u0999\u099A\u099B\u099C\u099D\u099E\u099F\u09A0\u09A1\u09A2\u09A3\u09A4\u09A5\u09A6\u09A7\u09A8\u09AA\u09AB\u09AC\u09AD\u09AE\u09AF\u09B0\u09B2\u09B6\u09B7\u09B8\u09B9\u09BD\u09CE\u09DC\u09DD\u09DF\u09E0\u09E1\u09F0\u09F1\u0A05\u0A06\u0A07\u0A08\u0A09\u0A0A\u0A0F\u0A10\u0A13\u0A14\u0A15\u0A16\u0A17\u0A18\u0A19\u0A1A\u0A1B\u0A1C\u0A1D\u0A1E\u0A1F\u0A20\u0A21\u0A22\u0A23\u0A24\u0A25\u0A26\u0A27\u0A28\u0A2A\u0A2B\u0A2C\u0
A2D\u0A2E\u0A2F\u0A30\u0A32\u0A33\u0A35\u0A36\u0A38\u0A39\u0A59\u0A5A\u0A5B\u0A5C\u0A5E\u0A72\u0A73\u0A74\u0A85\u0A86\u0A87\u0A88\u0A89\u0A8A\u0A8B\u0A8C\u0A8D\u0A8F\u0A90\u0A91\u0A93\u0A94\u0A95\u0A96\u0A97\u0A98\u0A99\u0A9A\u0A9B\u0A9C\u0A9D\u0A9E\u0A9F\u0AA0\u0AA1\u0AA2\u0AA3\u0AA4\u0AA5\u0AA6\u0AA7\u0AA8\u0AAA\u0AAB\u0AAC\u0AAD\u0AAE\u0AAF\u0AB0\u0AB2\u0AB3\u0AB5\u0AB6\u0AB7\u0AB8\u0AB9\u0ABD\u0AD0\u0AE0\u0AE1\u0B05\u0B06\u0B07\u0B08\u0B09\u0B0A\u0B0B\u0B0C\u0B0F\u0B10\u0B13\u0B14\u0B15\u0B16\u0B17\u0B18\u0B19\u0B1A\u0B1B\u0B1C\u0B1D\u0B1E\u0B1F\u0B20\u0B21\u0B22\u0B23\u0B24\u0B25\u0B26\u0B27\u0B28\u0B2A\u0B2B\u0B2C\u0B2D\u0B2E\u0B2F\u0B30\u0B32\u0B33\u0B35\u0B36\u0B37\u0B38\u0B39\u0B3D\u0B5C\u0B5D\u0B5F\u0B60\u0B61\u0B71\u0B83\u0B85\u0B86\u0B87\u0B88\u0B89\u0B8A\u0B8E\u0B8F\u0B90\u0B92\u0B93\u0B94\u0B95\u0B99\u0B9A\u0B9C\u0B9E\u0B9F\u0BA3\u0BA4\u0BA8\u0BA9\u0BAA\u0BAE\u0BAF\u0BB0\u0BB1\u0BB2\u0BB3\u0BB4\u0BB5\u0BB6\u0BB7\u0BB8\u0BB9\u0BD0\u0C05\u0C06\u0C07\u0C08\u0C09\u0C0A\u0C
0B\u0C0C\u0C0E\u0C0F\u0C10\u0C12\u0C13\u0C14\u0C15\u0C16\u0C17\u0C18\u0C19\u0C1A\u0C1B\u0C1C\u0C1D\u0C1E\u0C1F\u0C20\u0C21\u0C22\u0C23\u0C24\u0C25\u0C26\u0C27\u0C28\u0C2A\u0C2B\u0C2C\u0C2D\u0C2E\u0C2F\u0C30\u0C31\u0C32\u0C33\u0C35\u0C36\u0C37\u0C38\u0C39\u0C3D\u0C58\u0C59\u0C60\u0C61\u0C85\u0C86\u0C87\u0C88\u0C89\u0C8A\u0C8B\u0C8C\u0C8E\u0C8F\u0C90\u0C92\u0C93\u0C94\u0C95\u0C96\u0C97\u0C98\u0C99\u0C9A\u0C9B\u0C9C\u0C9D\u0C9E\u0C9F\u0CA0\u0CA1\u0CA2\u0CA3\u0CA4\u0CA5\u0CA6\u0CA7\u0CA8\u0CAA\u0CAB\u0CAC\u0CAD\u0CAE\u0CAF\u0CB0\u0CB1\u0CB2\u0CB3\u0CB5\u0CB6\u0CB7\u0CB8\u0CB9\u0CBD\u0CDE\u0CE0\u0CE1\u0D05\u0D06\u0D07\u0D08\u0D09\u0D0A\u0D0B\u0D0C\u0D0E\u0D0F\u0D10\u0D12\u0D13\u0D14\u0D15\u0D16\u0D17\u0D18\u0D19\u0D1A\u0D1B\u0D1C\u0D1D\u0D1E\u0D1F\u0D20\u0D21\u0D22\u0D23\u0D24\u0D25\u0D26\u0D27\u0D28\u0D2A\u0D2B\u0D2C\u0D2D\u0D2E\u0D2F\u0D30\u0D31\u0D32\u0D33\u0D34\u0D35\u0D36\u0D37\u0D38\u0D39\u0D3D\u0D60\u0D61\u0D7A\u0D7B\u0D7C\u0D7D\u0D7E\u0D7F\u0D85\u0D86\u0D87\u0D88\u0D89\u0D8A\u0D8
B\u0D8C\u0D8D\u0D8E\u0D8F\u0D90\u0D91\u0D92\u0D93\u0D94\u0D95\u0D96\u0D9A\u0D9B\u0D9C\u0D9D\u0D9E\u0D9F\u0DA0\u0DA1\u0DA2\u0DA3\u0DA4\u0DA5\u0DA6\u0DA7\u0DA8\u0DA9\u0DAA\u0DAB\u0DAC\u0DAD\u0DAE\u0DAF\u0DB0\u0DB1\u0DB3\u0DB4\u0DB5\u0DB6\u0DB7\u0DB8\u0DB9\u0DBA\u0DBB\u0DBD\u0DC0\u0DC1\u0DC2\u0DC3\u0DC4\u0DC5\u0DC6\u0E01\u0E02\u0E03\u0E04\u0E05\u0E06\u0E07\u0E08\u0E09\u0E0A\u0E0B\u0E0C\u0E0D\u0E0E\u0E0F\u0E10\u0E11\u0E12\u0E13\u0E14\u0E15\u0E16\u0E17\u0E18\u0E19\u0E1A\u0E1B\u0E1C\u0E1D\u0E1E\u0E1F\u0E20\u0E21\u0E22\u0E23\u0E24\u0E25\u0E26\u0E27\u0E28\u0E29\u0E2A\u0E2B\u0E2C\u0E2D\u0E2E\u0E2F\u0E30\u0E32\u0E33\u0E40\u0E41\u0E42\u0E43\u0E44\u0E45\u0E81\u0E82\u0E84\u0E87\u0E88\u0E8A\u0E8D\u0E94\u0E95\u0E96\u0E97\u0E99\u0E9A\u0E9B\u0E9C\u0E9D\u0E9E\u0E9F\u0EA1\u0EA2\u0EA3\u0EA5\u0EA7\u0EAA\u0EAB\u0EAD\u0EAE\u0EAF\u0EB0\u0EB2\u0EB3\u0EBD\u0EC0\u0EC1\u0EC2\u0EC3\u0EC4\u0EDC\u0EDD\u0F00\u0F40\u0F41\u0F42\u0F43\u0F44\u0F45\u0F46\u0F47\u0F49\u0F4A\u0F4B\u0F4C\u0F4D\u0F4E\u0F4F\u0F50\u0F51\u0F52
\u0F53\u0F54\u0F55\u0F56\u0F57\u0F58\u0F59\u0F5A\u0F5B\u0F5C\u0F5D\u0F5E\u0F5F\u0F60\u0F61\u0F62\u0F63\u0F64\u0F65\u0F66\u0F67\u0F68\u0F69\u0F6A\u0F6B\u0F6C\u0F88\u0F89\u0F8A\u0F8B\u1000\u1001\u1002\u1003\u1004\u1005\u1006\u1007\u1008\u1009\u100A\u100B\u100C\u100D\u100E\u100F\u1010\u1011\u1012\u1013\u1014\u1015\u1016\u1017\u1018\u1019\u101A\u101B\u101C\u101D\u101E\u101F\u1020\u1021\u1022\u1023\u1024\u1025\u1026\u1027\u1028\u1029\u102A\u103F\u1050\u1051\u1052\u1053\u1054\u1055\u105A\u105B\u105C\u105D\u1061\u1065\u1066\u106E\u106F\u1070\u1075\u1076\u1077\u1078\u1079\u107A\u107B\u107C\u107D\u107E\u107F\u1080\u1081\u108E\u10D0\u10D1\u10D2\u10D3\u10D4\u10D5\u10D6\u10D7\u10D8\u10D9\u10DA\u10DB\u10DC\u10DD\u10DE\u10DF\u10E0\u10E1\u10E2\u10E3\u10E4\u10E5\u10E6\u10E7\u10E8\u10E9\u10EA\u10EB\u10EC\u10ED\u10EE\u10EF\u10F0\u10F1\u10F2\u10F3\u10F4\u10F5\u10F6\u10F7\u10F8\u10F9\u10FA\u1100\u1101\u1102\u1103\u1104\u1105\u1106\u1107\u1108\u1109\u110A\u110B\u110C\u110D\u110E\u110F\u1110\u1111\u1112\
u1113\u1114\u1115\u1116\u1117\u1118\u1119\u111A\u111B\u111C\u111D\u111E\u111F\u1120\u1121\u1122\u1123\u1124\u1125\u1126\u1127\u1128\u1129\u112A\u112B\u112C\u112D\u112E\u112F\u1130\u1131\u1132\u1133\u1134\u1135\u1136\u1137\u1138\u1139\u113A\u113B\u113C\u113D\u113E\u113F\u1140\u1141\u1142\u1143\u1144\u1145\u1146\u1147\u1148\u1149\u114A\u114B\u114C\u114D\u114E\u114F\u1150\u1151\u1152\u1153\u1154\u1155\u1156\u1157\u1158\u1159\u115F\u1160\u1161\u1162\u1163\u1164\u1165\u1166\u1167\u1168\u1169\u116A\u116B\u116C\u116D\u116E\u116F\u1170\u1171\u1172\u1173\u1174\u1175\u1176\u1177\u1178\u1179\u117A\u117B\u117C\u117D\u117E\u117F\u1180\u1181\u1182\u1183\u1184\u1185\u1186\u1187\u1188\u1189\u118A\u118B\u118C\u118D\u118E\u118F\u1190\u1191\u1192\u1193\u1194\u1195\u1196\u1197\u1198\u1199\u119A\u119B\u119C\u119D\u119E\u119F\u11A0\u11A1\u11A2\u11A8\u11A9\u11AA\u11AB\u11AC\u11AD\u11AE\u11AF\u11B0\u11B1\u11B2\u11B3\u11B4\u11B5\u11B6\u11B7\u11B8\u11B9\u11BA\u11BB\u11BC\u11BD\u11BE\u11BF\u11C0\u11C1\u11C2\u
11C3\u11C4\u11C5\u11C6\u11C7\u11C8\u11C9\u11CA\u11CB\u11CC\u11CD\u11CE\u11CF\u11D0\u11D1\u11D2\u11D3\u11D4\u11D5\u11D6\u11D7\u11D8\u11D9\u11DA\u11DB\u11DC\u11DD\u11DE\u11DF\u11E0\u11E1\u11E2\u11E3\u11E4\u11E5\u11E6\u11E7\u11E8\u11E9\u11EA\u11EB\u11EC\u11ED\u11EE\u11EF\u11F0\u11F1\u11F2\u11F3\u11F4\u11F5\u11F6\u11F7\u11F8\u11F9\u1200\u1201\u1202\u1203\u1204\u1205\u1206\u1207\u1208\u1209\u120A\u120B\u120C\u120D\u120E\u120F\u1210\u1211\u1212\u1213\u1214\u1215\u1216\u1217\u1218\u1219\u121A\u121B\u121C\u121D\u121E\u121F\u1220\u1221\u1222\u1223\u1224\u1225\u1226\u1227\u1228\u1229\u122A\u122B\u122C\u122D\u122E\u122F\u1230\u1231\u1232\u1233\u1234\u1235\u1236\u1237\u1238\u1239\u123A\u123B\u123C\u123D\u123E\u123F\u1240\u1241\u1242\u1243\u1244\u1245\u1246\u1247\u1248\u124A\u124B\u124C\u124D\u1250\u1251\u1252\u1253\u1254\u1255\u1256\u1258\u125A\u125B\u125C\u125D\u1260\u1261\u1262\u1263\u1264\u1265\u1266\u1267\u1268\u1269\u126A\u126B\u126C\u126D\u126E\u126F\u1270\u1271\u1272\u1273\u1274\u1275\u1
276\u1277\u1278\u1279\u127A\u127B\u127C\u127D\u127E\u127F\u1280\u1281\u1282\u1283\u1284\u1285\u1286\u1287\u1288\u128A\u128B\u128C\u128D\u1290\u1291\u1292\u1293\u1294\u1295\u1296\u1297\u1298\u1299\u129A\u129B\u129C\u129D\u129E\u129F\u12A0\u12A1\u12A2\u12A3\u12A4\u12A5\u12A6\u12A7\u12A8\u12A9\u12AA\u12AB\u12AC\u12AD\u12AE\u12AF\u12B0\u12B2\u12B3\u12B4\u12B5\u12B8\u12B9\u12BA\u12BB\u12BC\u12BD\u12BE\u12C0\u12C2\u12C3\u12C4\u12C5\u12C8\u12C9\u12CA\u12CB\u12CC\u12CD\u12CE\u12CF\u12D0\u12D1\u12D2\u12D3\u12D4\u12D5\u12D6\u12D8\u12D9\u12DA\u12DB\u12DC\u12DD\u12DE\u12DF\u12E0\u12E1\u12E2\u12E3\u12E4\u12E5\u12E6\u12E7\u12E8\u12E9\u12EA\u12EB\u12EC\u12ED\u12EE\u12EF\u12F0\u12F1\u12F2\u12F3\u12F4\u12F5\u12F6\u12F7\u12F8\u12F9\u12FA\u12FB\u12FC\u12FD\u12FE\u12FF\u1300\u1301\u1302\u1303\u1304\u1305\u1306\u1307\u1308\u1309\u130A\u130B\u130C\u130D\u130E\u130F\u1310\u1312\u1313\u1314\u1315\u1318\u1319\u131A\u131B\u131C\u131D\u131E\u131F\u1320\u1321\u1322\u1323\u1324\u1325\u1326\u1327\u1328\u1329\u13
2A\u132B\u132C\u132D\u132E\u132F\u1330\u1331\u1332\u1333\u1334\u1335\u1336\u1337\u1338\u1339\u133A\u133B\u133C\u133D\u133E\u133F\u1340\u1341\u1342\u1343\u1344\u1345\u1346\u1347\u1348\u1349\u134A\u134B\u134C\u134D\u134E\u134F\u1350\u1351\u1352\u1353\u1354\u1355\u1356\u1357\u1358\u1359\u135A\u1380\u1381\u1382\u1383\u1384\u1385\u1386\u1387\u1388\u1389\u138A\u138B\u138C\u138D\u138E\u138F\u13A0\u13A1\u13A2\u13A3\u13A4\u13A5\u13A6\u13A7\u13A8\u13A9\u13AA\u13AB\u13AC\u13AD\u13AE\u13AF\u13B0\u13B1\u13B2\u13B3\u13B4\u13B5\u13B6\u13B7\u13B8\u13B9\u13BA\u13BB\u13BC\u13BD\u13BE\u13BF\u13C0\u13C1\u13C2\u13C3\u13C4\u13C5\u13C6\u13C7\u13C8\u13C9\u13CA\u13CB\u13CC\u13CD\u13CE\u13CF\u13D0\u13D1\u13D2\u13D3\u13D4\u13D5\u13D6\u13D7\u13D8\u13D9\u13DA\u13DB\u13DC\u13DD\u13DE\u13DF\u13E0\u13E1\u13E2\u13E3\u13E4\u13E5\u13E6\u13E7\u13E8\u13E9\u13EA\u13EB\u13EC\u13ED\u13EE\u13EF\u13F0\u13F1\u13F2\u13F3\u13F4\u1401\u1402\u1403\u1404\u1405\u1406\u1407\u1408\u1409\u140A\u140B\u140C\u140D\u140E\u140F\u1410\u141
1\u1412\u1413\u1414\u1415\u1416\u1417\u1418\u1419\u141A\u141B\u141C\u141D\u141E\u141F\u1420\u1421\u1422\u1423\u1424\u1425\u1426\u1427\u1428\u1429\u142A\u142B\u142C\u142D\u142E\u142F\u1430\u1431\u1432\u1433\u1434\u1435\u1436\u1437\u1438\u1439\u143A\u143B\u143C\u143D\u143E\u143F\u1440\u1441\u1442\u1443\u1444\u1445\u1446\u1447\u1448\u1449\u144A\u144B\u144C\u144D\u144E\u144F\u1450\u1451\u1452\u1453\u1454\u1455\u1456\u1457\u1458\u1459\u145A\u145B\u145C\u145D\u145E\u145F\u1460\u1461\u1462\u1463\u1464\u1465\u1466\u1467\u1468\u1469\u146A\u146B\u146C\u146D\u146E\u146F\u1470\u1471\u1472\u1473\u1474\u1475\u1476\u1477\u1478\u1479\u147A\u147B\u147C\u147D\u147E\u147F\u1480\u1481\u1482\u1483\u1484\u1485\u1486\u1487\u1488\u1489\u148A\u148B\u148C\u148D\u148E\u148F\u1490\u1491\u1492\u1493\u1494\u1495\u1496\u1497\u1498\u1499\u149A\u149B\u149C\u149D\u149E\u149F\u14A0\u14A1\u14A2\u14A3\u14A4\u14A5\u14A6\u14A7\u14A8\u14A9\u14AA\u14AB\u14AC\u14AD\u14AE\u14AF\u14B0\u14B1\u14B2\u14B3\u14B4\u14B5\u14B6\u14B7
\u14B8\u14B9\u14BA\u14BB\u14BC\u14BD\u14BE\u14BF\u14C0\u14C1\u14C2\u14C3\u14C4\u14C5\u14C6\u14C7\u14C8\u14C9\u14CA\u14CB\u14CC\u14CD\u14CE\u14CF\u14D0\u14D1\u14D2\u14D3\u14D4\u14D5\u14D6\u14D7\u14D8\u14D9\u14DA\u14DB\u14DC\u14DD\u14DE\u14DF\u14E0\u14E1\u14E2\u14E3\u14E4\u14E5\u14E6\u14E7\u14E8\u14E9\u14EA\u14EB\u14EC\u14ED\u14EE\u14EF\u14F0\u14F1\u14F2\u14F3\u14F4\u14F5\u14F6\u14F7\u14F8\u14F9\u14FA\u14FB\u14FC\u14FD\u14FE\u14FF\u1500\u1501\u1502\u1503\u1504\u1505\u1506\u1507\u1508\u1509\u150A\u150B\u150C\u150D\u150E\u150F\u1510\u1511\u1512\u1513\u1514\u1515\u1516\u1517\u1518\u1519\u151A\u151B\u151C\u151D\u151E\u151F\u1520\u1521\u1522\u1523\u1524\u1525\u1526\u1527\u1528\u1529\u152A\u152B\u152C\u152D\u152E\u152F\u1530\u1531\u1532\u1533\u1534\u1535\u1536\u1537\u1538\u1539\u153A\u153B\u153C\u153D\u153E\u153F\u1540\u1541\u1542\u1543\u1544\u1545\u1546\u1547\u1548\u1549\u154A\u154B\u154C\u154D\u154E\u154F\u1550\u1551\u1552\u1553\u1554\u1555\u1556\u1557\u1558\u1559\u155A\u155B\u155C\u155D\
u155E\u155F\u1560\u1561\u1562\u1563\u1564\u1565\u1566\u1567\u1568\u1569\u156A\u156B\u156C\u156D\u156E\u156F\u1570\u1571\u1572\u1573\u1574\u1575\u1576\u1577\u1578\u1579\u157A\u157B\u157C\u157D\u157E\u157F\u1580\u1581\u1582\u1583\u1584\u1585\u1586\u1587\u1588\u1589\u158A\u158B\u158C\u158D\u158E\u158F\u1590\u1591\u1592\u1593\u1594\u1595\u1596\u1597\u1598\u1599\u159A\u159B\u159C\u159D\u159E\u159F\u15A0\u15A1\u15A2\u15A3\u15A4\u15A5\u15A6\u15A7\u15A8\u15A9\u15AA\u15AB\u15AC\u15AD\u15AE\u15AF\u15B0\u15B1\u15B2\u15B3\u15B4\u15B5\u15B6\u15B7\u15B8\u15B9\u15BA\u15BB\u15BC\u15BD\u15BE\u15BF\u15C0\u15C1\u15C2\u15C3\u15C4\u15C5\u15C6\u15C7\u15C8\u15C9\u15CA\u15CB\u15CC\u15CD\u15CE\u15CF\u15D0\u15D1\u15D2\u15D3\u15D4\u15D5\u15D6\u15D7\u15D8\u15D9\u15DA\u15DB\u15DC\u15DD\u15DE\u15DF\u15E0\u15E1\u15E2\u15E3\u15E4\u15E5\u15E6\u15E7\u15E8\u15E9\u15EA\u15EB\u15EC\u15ED\u15EE\u15EF\u15F0\u15F1\u15F2\u15F3\u15F4\u15F5\u15F6\u15F7\u15F8\u15F9\u15FA\u15FB\u15FC\u15FD\u15FE\u15FF\u1600\u1601\u1602\u1603\u
1604\u1605\u1606\u1607\u1608\u1609\u160A\u160B\u160C\u160D\u160E\u160F\u1610\u1611\u1612\u1613\u1614\u1615\u1616\u1617\u1618\u1619\u161A\u161B\u161C\u161D\u161E\u161F\u1620\u1621\u1622\u1623\u1624\u1625\u1626\u1627\u1628\u1629\u162A\u162B\u162C\u162D\u162E\u162F\u1630\u1631\u1632\u1633\u1634\u1635\u1636\u1637\u1638\u1639\u163A\u163B\u163C\u163D\u163E\u163F\u1640\u1641\u1642\u1643\u1644\u1645\u1646\u1647\u1648\u1649\u164A\u164B\u164C\u164D\u164E\u164F\u1650\u1651\u1652\u1653\u1654\u1655\u1656\u1657\u1658\u1659\u165A\u165B\u165C\u165D\u165E\u165F\u1660\u1661\u1662\u1663\u1664\u1665\u1666\u1667\u1668\u1669\u166A\u166B\u166C\u166F\u1670\u1671\u1672\u1673\u1674\u1675\u1676\u1681\u1682\u1683\u1684\u1685\u1686\u1687\u1688\u1689\u168A\u168B\u168C\u168D\u168E\u168F\u1690\u1691\u1692\u1693\u1694\u1695\u1696\u1697\u1698\u1699\u169A\u16A0\u16A1\u16A2\u16A3\u16A4\u16A5\u16A6\u16A7\u16A8\u16A9\u16AA\u16AB\u16AC\u16AD\u16AE\u16AF\u16B0\u16B1\u16B2\u16B3\u16B4\u16B5\u16B6\u16B7\u16B8\u16B9\u16BA\u1
6BB\u16BC\u16BD\u16BE\u16BF\u16C0\u16C1\u16C2\u16C3\u16C4\u16C5\u16C6\u16C7\u16C8\u16C9\u16CA\u16CB\u16CC\u16CD\u16CE\u16CF\u16D0\u16D1\u16D2\u16D3\u16D4\u16D5\u16D6\u16D7\u16D8\u16D9\u16DA\u16DB\u16DC\u16DD\u16DE\u16DF\u16E0\u16E1\u16E2\u16E3\u16E4\u16E5\u16E6\u16E7\u16E8\u16E9\u16EA\u1700\u1701\u1702\u1703\u1704\u1705\u1706\u1707\u1708\u1709\u170A\u170B\u170C\u170E\u170F\u1710\u1711\u1720\u1721\u1722\u1723\u1724\u1725\u1726\u1727\u1728\u1729\u172A\u172B\u172C\u172D\u172E\u172F\u1730\u1731\u1740\u1741\u1742\u1743\u1744\u1745\u1746\u1747\u1748\u1749\u174A\u174B\u174C\u174D\u174E\u174F\u1750\u1751\u1760\u1761\u1762\u1763\u1764\u1765\u1766\u1767\u1768\u1769\u176A\u176B\u176C\u176E\u176F\u1770\u1780\u1781\u1782\u1783\u1784\u1785\u1786\u1787\u1788\u1789\u178A\u178B\u178C\u178D\u178E\u178F\u1790\u1791\u1792\u1793\u1794\u1795\u1796\u1797\u1798\u1799\u179A\u179B\u179C\u179D\u179E\u179F\u17A0\u17A1\u17A2\u17A3\u17A4\u17A5\u17A6\u17A7\u17A8\u17A9\u17AA\u17AB\u17AC\u17AD\u17AE\u17AF\u17B0\u17
B1\u17B2\u17B3\u17DC\u1820\u1821\u1822\u1823\u1824\u1825\u1826\u1827\u1828\u1829\u182A\u182B\u182C\u182D\u182E\u182F\u1830\u1831\u1832\u1833\u1834\u1835\u1836\u1837\u1838\u1839\u183A\u183B\u183C\u183D\u183E\u183F\u1840\u1841\u1842\u1844\u1845\u1846\u1847\u1848\u1849\u184A\u184B\u184C\u184D\u184E\u184F\u1850\u1851\u1852\u1853\u1854\u1855\u1856\u1857\u1858\u1859\u185A\u185B\u185C\u185D\u185E\u185F\u1860\u1861\u1862\u1863\u1864\u1865\u1866\u1867\u1868\u1869\u186A\u186B\u186C\u186D\u186E\u186F\u1870\u1871\u1872\u1873\u1874\u1875\u1876\u1877\u1880\u1881\u1882\u1883\u1884\u1885\u1886\u1887\u1888\u1889\u188A\u188B\u188C\u188D\u188E\u188F\u1890\u1891\u1892\u1893\u1894\u1895\u1896\u1897\u1898\u1899\u189A\u189B\u189C\u189D\u189E\u189F\u18A0\u18A1\u18A2\u18A3\u18A4\u18A5\u18A6\u18A7\u18A8\u18AA\u1900\u1901\u1902\u1903\u1904\u1905\u1906\u1907\u1908\u1909\u190A\u190B\u190C\u190D\u190E\u190F\u1910\u1911\u1912\u1913\u1914\u1915\u1916\u1917\u1918\u1919\u191A\u191B\u191C\u1950\u1951\u1952\u1953\u195
4\u1955\u1956\u1957\u1958\u1959\u195A\u195B\u195C\u195D\u195E\u195F\u1960\u1961\u1962\u1963\u1964\u1965\u1966\u1967\u1968\u1969\u196A\u196B\u196C\u196D\u1970\u1971\u1972\u1973\u1974\u1980\u1981\u1982\u1983\u1984\u1985\u1986\u1987\u1988\u1989\u198A\u198B\u198C\u198D\u198E\u198F\u1990\u1991\u1992\u1993\u1994\u1995\u1996\u1997\u1998\u1999\u199A\u199B\u199C\u199D\u199E\u199F\u19A0\u19A1\u19A2\u19A3\u19A4\u19A5\u19A6\u19A7\u19A8\u19A9\u19C1\u19C2\u19C3\u19C4\u19C5\u19C6\u19C7\u1A00\u1A01\u1A02\u1A03\u1A04\u1A05\u1A06\u1A07\u1A08\u1A09\u1A0A\u1A0B\u1A0C\u1A0D\u1A0E\u1A0F\u1A10\u1A11\u1A12\u1A13\u1A14\u1A15\u1A16\u1B05\u1B06\u1B07\u1B08\u1B09\u1B0A\u1B0B\u1B0C\u1B0D\u1B0E\u1B0F\u1B10\u1B11\u1B12\u1B13\u1B14\u1B15\u1B16\u1B17\u1B18\u1B19\u1B1A\u1B1B\u1B1C\u1B1D\u1B1E\u1B1F\u1B20\u1B21\u1B22\u1B23\u1B24\u1B25\u1B26\u1B27\u1B28\u1B29\u1B2A\u1B2B\u1B2C\u1B2D\u1B2E\u1B2F\u1B30\u1B31\u1B32\u1B33\u1B45\u1B46\u1B47\u1B48\u1B49\u1B4A\u1B4B\u1B83\u1B84\u1B85\u1B86\u1B87\u1B88\u1B89\u1B8A\u1B8B\u1B8C
\u1B8D\u1B8E\u1B8F\u1B90\u1B91\u1B92\u1B93\u1B94\u1B95\u1B96\u1B97\u1B98\u1B99\u1B9A\u1B9B\u1B9C\u1B9D\u1B9E\u1B9F\u1BA0\u1BAE\u1BAF\u1C00\u1C01\u1C02\u1C03\u1C04\u1C05\u1C06\u1C07\u1C08\u1C09\u1C0A\u1C0B\u1C0C\u1C0D\u1C0E\u1C0F\u1C10\u1C11\u1C12\u1C13\u1C14\u1C15\u1C16\u1C17\u1C18\u1C19\u1C1A\u1C1B\u1C1C\u1C1D\u1C1E\u1C1F\u1C20\u1C21\u1C22\u1C23\u1C4D\u1C4E\u1C4F\u1C5A\u1C5B\u1C5C\u1C5D\u1C5E\u1C5F\u1C60\u1C61\u1C62\u1C63\u1C64\u1C65\u1C66\u1C67\u1C68\u1C69\u1C6A\u1C6B\u1C6C\u1C6D\u1C6E\u1C6F\u1C70\u1C71\u1C72\u1C73\u1C74\u1C75\u1C76\u1C77\u2135\u2136\u2137\u2138\u2D30\u2D31\u2D32\u2D33\u2D34\u2D35\u2D36\u2D37\u2D38\u2D39\u2D3A\u2D3B\u2D3C\u2D3D\u2D3E\u2D3F\u2D40\u2D41\u2D42\u2D43\u2D44\u2D45\u2D46\u2D47\u2D48\u2D49\u2D4A\u2D4B\u2D4C\u2D4D\u2D4E\u2D4F\u2D50\u2D51\u2D52\u2D53\u2D54\u2D55\u2D56\u2D57\u2D58\u2D59\u2D5A\u2D5B\u2D5C\u2D5D\u2D5E\u2D5F\u2D60\u2D61\u2D62\u2D63\u2D64\u2D65\u2D80\u2D81\u2D82\u2D83\u2D84\u2D85\u2D86\u2D87\u2D88\u2D89\u2D8A\u2D8B\u2D8C\u2D8D\u2D8E\u2D8F\u2D90\
u2D91\u2D92\u2D93\u2D94\u2D95\u2D96\u2DA0\u2DA1\u2DA2\u2DA3\u2DA4\u2DA5\u2DA6\u2DA8\u2DA9\u2DAA\u2DAB\u2DAC\u2DAD\u2DAE\u2DB0\u2DB1\u2DB2\u2DB3\u2DB4\u2DB5\u2DB6\u2DB8\u2DB9\u2DBA\u2DBB\u2DBC\u2DBD\u2DBE\u2DC0\u2DC1\u2DC2\u2DC3\u2DC4\u2DC5\u2DC6\u2DC8\u2DC9\u2DCA\u2DCB\u2DCC\u2DCD\u2DCE\u2DD0\u2DD1\u2DD2\u2DD3\u2DD4\u2DD5\u2DD6\u2DD8\u2DD9\u2DDA\u2DDB\u2DDC\u2DDD\u2DDE\u3006\u303C\u3041\u3042\u3043\u3044\u3045\u3046\u3047\u3048\u3049\u304A\u304B\u304C\u304D\u304E\u304F\u3050\u3051\u3052\u3053\u3054\u3055\u3056\u3057\u3058\u3059\u305A\u305B\u305C\u305D\u305E\u305F\u3060\u3061\u3062\u3063\u3064\u3065\u3066\u3067\u3068\u3069\u306A\u306B\u306C\u306D\u306E\u306F\u3070\u3071\u3072\u3073\u3074\u3075\u3076\u3077\u3078\u3079\u307A\u307B\u307C\u307D\u307E\u307F\u3080\u3081\u3082\u3083\u3084\u3085\u3086\u3087\u3088\u3089\u308A\u308B\u308C\u308D\u308E\u308F\u3090\u3091\u3092\u3093\u3094\u3095\u3096\u309F\u30A1\u30A2\u30A3\u30A4\u30A5\u30A6\u30A7\u30A8\u30A9\u30AA\u30AB\u30AC\u30AD\u30AE\u30AF\u
30B0\u30B1\u30B2\u30B3\u30B4\u30B5\u30B6\u30B7\u30B8\u30B9\u30BA\u30BB\u30BC\u30BD\u30BE\u30BF\u30C0\u30C1\u30C2\u30C3\u30C4\u30C5\u30C6\u30C7\u30C8\u30C9\u30CA\u30CB\u30CC\u30CD\u30CE\u30CF\u30D0\u30D1\u30D2\u30D3\u30D4\u30D5\u30D6\u30D7\u30D8\u30D9\u30DA\u30DB\u30DC\u30DD\u30DE\u30DF\u30E0\u30E1\u30E2\u30E3\u30E4\u30E5\u30E6\u30E7\u30E8\u30E9\u30EA\u30EB\u30EC\u30ED\u30EE\u30EF\u30F0\u30F1\u30F2\u30F3\u30F4\u30F5\u30F6\u30F7\u30F8\u30F9\u30FA\u30FF\u3105\u3106\u3107\u3108\u3109\u310A\u310B\u310C\u310D\u310E\u310F\u3110\u3111\u3112\u3113\u3114\u3115\u3116\u3117\u3118\u3119\u311A\u311B\u311C\u311D\u311E\u311F\u3120\u3121\u3122\u3123\u3124\u3125\u3126\u3127\u3128\u3129\u312A\u312B\u312C\u312D\u3131\u3132\u3133\u3134\u3135\u3136\u3137\u3138\u3139\u313A\u313B\u313C\u313D\u313E\u313F\u3140\u3141\u3142\u3143\u3144\u3145\u3146\u3147\u3148\u3149\u314A\u314B\u314C\u314D\u314E\u314F\u3150\u3151\u3152\u3153\u3154\u3155\u3156\u3157\u3158\u3159\u315A\u315B\u315C\u315D\u315E\u315F\u3160\u3161\u3
162\u3163\u3164\u3165\u3166\u3167\u3168\u3169\u316A\u316B\u316C\u316D\u316E\u316F\u3170\u3171\u3172\u3173\u3174\u3175\u3176\u3177\u3178\u3179\u317A\u317B\u317C\u317D\u317E\u317F\u3180\u3181\u3182\u3183\u3184\u3185\u3186\u3187\u3188\u3189\u318A\u318B\u318C\u318D\u318E\u31A0\u31A1\u31A2\u31A3\u31A4\u31A5\u31A6\u31A7\u31A8\u31A9\u31AA\u31AB\u31AC\u31AD\u31AE\u31AF\u31B0\u31B1\u31B2\u31B3\u31B4\u31B5\u31B6\u31B7\u31F0\u31F1\u31F2\u31F3\u31F4\u31F5\u31F6\u31F7\u31F8\u31F9\u31FA\u31FB\u31FC\u31FD\u31FE\u31FF\u3400\u4DB5\u4E00\u9FC3\uA000\uA001\uA002\uA003\uA004\uA005\uA006\uA007\uA008\uA009\uA00A\uA00B\uA00C\uA00D\uA00E\uA00F\uA010\uA011\uA012\uA013\uA014\uA016\uA017\uA018\uA019\uA01A\uA01B\uA01C\uA01D\uA01E\uA01F\uA020\uA021\uA022\uA023\uA024\uA025\uA026\uA027\uA028\uA029\uA02A\uA02B\uA02C\uA02D\uA02E\uA02F\uA030\uA031\uA032\uA033\uA034\uA035\uA036\uA037\uA038\uA039\uA03A\uA03B\uA03C\uA03D\uA03E\uA03F\uA040\uA041\uA042\uA043\uA044\uA045\uA046\uA047\uA048\uA049\uA04A\uA04B\uA04C\uA04D\uA0
4E\uA04F\uA050\uA051\uA052\uA053\uA054\uA055\uA056\uA057\uA058\uA059\uA05A\uA05B\uA05C\uA05D\uA05E\uA05F\uA060\uA061\uA062\uA063\uA064\uA065\uA066\uA067\uA068\uA069\uA06A\uA06B\uA06C\uA06D\uA06E\uA06F\uA070\uA071\uA072\uA073\uA074\uA075\uA076\uA077\uA078\uA079\uA07A\uA07B\uA07C\uA07D\uA07E\uA07F\uA080\uA081\uA082\uA083\uA084\uA085\uA086\uA087\uA088\uA089\uA08A\uA08B\uA08C\uA08D\uA08E\uA08F\uA090\uA091\uA092\uA093\uA094\uA095\uA096\uA097\uA098\uA099\uA09A\uA09B\uA09C\uA09D\uA09E\uA09F\uA0A0\uA0A1\uA0A2\uA0A3\uA0A4\uA0A5\uA0A6\uA0A7\uA0A8\uA0A9\uA0AA\uA0AB\uA0AC\uA0AD\uA0AE\uA0AF\uA0B0\uA0B1\uA0B2\uA0B3\uA0B4\uA0B5\uA0B6\uA0B7\uA0B8\uA0B9\uA0BA\uA0BB\uA0BC\uA0BD\uA0BE\uA0BF\uA0C0\uA0C1\uA0C2\uA0C3\uA0C4\uA0C5\uA0C6\uA0C7\uA0C8\uA0C9\uA0CA\uA0CB\uA0CC\uA0CD\uA0CE\uA0CF\uA0D0\uA0D1\uA0D2\uA0D3\uA0D4\uA0D5\uA0D6\uA0D7\uA0D8\uA0D9\uA0DA\uA0DB\uA0DC\uA0DD\uA0DE\uA0DF\uA0E0\uA0E1\uA0E2\uA0E3\uA0E4\uA0E5\uA0E6\uA0E7\uA0E8\uA0E9\uA0EA\uA0EB\uA0EC\uA0ED\uA0EE\uA0EF\uA0F0\uA0F1\uA0F2\uA0F3\uA0F
4\uA0F5\uA0F6\uA0F7\uA0F8\uA0F9\uA0FA\uA0FB\uA0FC\uA0FD\uA0FE\uA0FF\uA100\uA101\uA102\uA103\uA104\uA105\uA106\uA107\uA108\uA109\uA10A\uA10B\uA10C\uA10D\uA10E\uA10F\uA110\uA111\uA112\uA113\uA114\uA115\uA116\uA117\uA118\uA119\uA11A\uA11B\uA11C\uA11D\uA11E\uA11F\uA120\uA121\uA122\uA123\uA124\uA125\uA126\uA127\uA128\uA129\uA12A\uA12B\uA12C\uA12D\uA12E\uA12F\uA130\uA131\uA132\uA133\uA134\uA135\uA136\uA137\uA138\uA139\uA13A\uA13B\uA13C\uA13D\uA13E\uA13F\uA140\uA141\uA142\uA143\uA144\uA145\uA146\uA147\uA148\uA149\uA14A\uA14B\uA14C\uA14D\uA14E\uA14F\uA150\uA151\uA152\uA153\uA154\uA155\uA156\uA157\uA158\uA159\uA15A\uA15B\uA15C\uA15D\uA15E\uA15F\uA160\uA161\uA162\uA163\uA164\uA165\uA166\uA167\uA168\uA169\uA16A\uA16B\uA16C\uA16D\uA16E\uA16F\uA170\uA171\uA172\uA173\uA174\uA175\uA176\uA177\uA178\uA179\uA17A\uA17B\uA17C\uA17D\uA17E\uA17F\uA180\uA181\uA182\uA183\uA184\uA185\uA186\uA187\uA188\uA189\uA18A\uA18B\uA18C\uA18D\uA18E\uA18F\uA190\uA191\uA192\uA193\uA194\uA195\uA196\uA197\uA198\uA199\uA19A
\uA19B\uA19C\uA19D\uA19E\uA19F\uA1A0\uA1A1\uA1A2\uA1A3\uA1A4\uA1A5\uA1A6\uA1A7\uA1A8\uA1A9\uA1AA\uA1AB\uA1AC\uA1AD\uA1AE\uA1AF\uA1B0\uA1B1\uA1B2\uA1B3\uA1B4\uA1B5\uA1B6\uA1B7\uA1B8\uA1B9\uA1BA\uA1BB\uA1BC\uA1BD\uA1BE\uA1BF\uA1C0\uA1C1\uA1C2\uA1C3\uA1C4\uA1C5\uA1C6\uA1C7\uA1C8\uA1C9\uA1CA\uA1CB\uA1CC\uA1CD\uA1CE\uA1CF\uA1D0\uA1D1\uA1D2\uA1D3\uA1D4\uA1D5\uA1D6\uA1D7\uA1D8\uA1D9\uA1DA\uA1DB\uA1DC\uA1DD\uA1DE\uA1DF\uA1E0\uA1E1\uA1E2\uA1E3\uA1E4\uA1E5\uA1E6\uA1E7\uA1E8\uA1E9\uA1EA\uA1EB\uA1EC\uA1ED\uA1EE\uA1EF\uA1F0\uA1F1\uA1F2\uA1F3\uA1F4\uA1F5\uA1F6\uA1F7\uA1F8\uA1F9\uA1FA\uA1FB\uA1FC\uA1FD\uA1FE\uA1FF\uA200\uA201\uA202\uA203\uA204\uA205\uA206\uA207\uA208\uA209\uA20A\uA20B\uA20C\uA20D\uA20E\uA20F\uA210\uA211\uA212\uA213\uA214\uA215\uA216\uA217\uA218\uA219\uA21A\uA21B\uA21C\uA21D\uA21E\uA21F\uA220\uA221\uA222\uA223\uA224\uA225\uA226\uA227\uA228\uA229\uA22A\uA22B\uA22C\uA22D\uA22E\uA22F\uA230\uA231\uA232\uA233\uA234\uA235\uA236\uA237\uA238\uA239\uA23A\uA23B\uA23C\uA23D\uA23E\uA23F\uA240\
uA241\uA242\uA243\uA244\uA245\uA246\uA247\uA248\uA249\uA24A\uA24B\uA24C\uA24D\uA24E\uA24F\uA250\uA251\uA252\uA253\uA254\uA255\uA256\uA257\uA258\uA259\uA25A\uA25B\uA25C\uA25D\uA25E\uA25F\uA260\uA261\uA262\uA263\uA264\uA265\uA266\uA267\uA268\uA269\uA26A\uA26B\uA26C\uA26D\uA26E\uA26F\uA270\uA271\uA272\uA273\uA274\uA275\uA276\uA277\uA278\uA279\uA27A\uA27B\uA27C\uA27D\uA27E\uA27F\uA280\uA281\uA282\uA283\uA284\uA285\uA286\uA287\uA288\uA289\uA28A\uA28B\uA28C\uA28D\uA28E\uA28F\uA290\uA291\uA292\uA293\uA294\uA295\uA296\uA297\uA298\uA299\uA29A\uA29B\uA29C\uA29D\uA29E\uA29F\uA2A0\uA2A1\uA2A2\uA2A3\uA2A4\uA2A5\uA2A6\uA2A7\uA2A8\uA2A9\uA2AA\uA2AB\uA2AC\uA2AD\uA2AE\uA2AF\uA2B0\uA2B1\uA2B2\uA2B3\uA2B4\uA2B5\uA2B6\uA2B7\uA2B8\uA2B9\uA2BA\uA2BB\uA2BC\uA2BD\uA2BE\uA2BF\uA2C0\uA2C1\uA2C2\uA2C3\uA2C4\uA2C5\uA2C6\uA2C7\uA2C8\uA2C9\uA2CA\uA2CB\uA2CC\uA2CD\uA2CE\uA2CF\uA2D0\uA2D1\uA2D2\uA2D3\uA2D4\uA2D5\uA2D6\uA2D7\uA2D8\uA2D9\uA2DA\uA2DB\uA2DC\uA2DD\uA2DE\uA2DF\uA2E0\uA2E1\uA2E2\uA2E3\uA2E4\uA2E5\uA2E6\u
A2E7\uA2E8\uA2E9\uA2EA\uA2EB\uA2EC\uA2ED\uA2EE\uA2EF\uA2F0\uA2F1\uA2F2\uA2F3\uA2F4\uA2F5\uA2F6\uA2F7\uA2F8\uA2F9\uA2FA\uA2FB\uA2FC\uA2FD\uA2FE\uA2FF\uA300\uA301\uA302\uA303\uA304\uA305\uA306\uA307\uA308\uA309\uA30A\uA30B\uA30C\uA30D\uA30E\uA30F\uA310\uA311\uA312\uA313\uA314\uA315\uA316\uA317\uA318\uA319\uA31A\uA31B\uA31C\uA31D\uA31E\uA31F\uA320\uA321\uA322\uA323\uA324\uA325\uA326\uA327\uA328\uA329\uA32A\uA32B\uA32C\uA32D\uA32E\uA32F\uA330\uA331\uA332\uA333\uA334\uA335\uA336\uA337\uA338\uA339\uA33A\uA33B\uA33C\uA33D\uA33E\uA33F\uA340\uA341\uA342\uA343\uA344\uA345\uA346\uA347\uA348\uA349\uA34A\uA34B\uA34C\uA34D\uA34E\uA34F\uA350\uA351\uA352\uA353\uA354\uA355\uA356\uA357\uA358\uA359\uA35A\uA35B\uA35C\uA35D\uA35E\uA35F\uA360\uA361\uA362\uA363\uA364\uA365\uA366\uA367\uA368\uA369\uA36A\uA36B\uA36C\uA36D\uA36E\uA36F\uA370\uA371\uA372\uA373\uA374\uA375\uA376\uA377\uA378\uA379\uA37A\uA37B\uA37C\uA37D\uA37E\uA37F\uA380\uA381\uA382\uA383\uA384\uA385\uA386\uA387\uA388\uA389\uA38A\uA38B\uA38C\uA
38D\uA38E\uA38F\uA390\uA391\uA392\uA393\uA394\uA395\uA396\uA397\uA398\uA399\uA39A\uA39B\uA39C\uA39D\uA39E\uA39F\uA3A0\uA3A1\uA3A2\uA3A3\uA3A4\uA3A5\uA3A6\uA3A7\uA3A8\uA3A9\uA3AA\uA3AB\uA3AC\uA3AD\uA3AE\uA3AF\uA3B0\uA3B1\uA3B2\uA3B3\uA3B4\uA3B5\uA3B6\uA3B7\uA3B8\uA3B9\uA3BA\uA3BB\uA3BC\uA3BD\uA3BE\uA3BF\uA3C0\uA3C1\uA3C2\uA3C3\uA3C4\uA3C5\uA3C6\uA3C7\uA3C8\uA3C9\uA3CA\uA3CB\uA3CC\uA3CD\uA3CE\uA3CF\uA3D0\uA3D1\uA3D2\uA3D3\uA3D4\uA3D5\uA3D6\uA3D7\uA3D8\uA3D9\uA3DA\uA3DB\uA3DC\uA3DD\uA3DE\uA3DF\uA3E0\uA3E1\uA3E2\uA3E3\uA3E4\uA3E5\uA3E6\uA3E7\uA3E8\uA3E9\uA3EA\uA3EB\uA3EC\uA3ED\uA3EE\uA3EF\uA3F0\uA3F1\uA3F2\uA3F3\uA3F4\uA3F5\uA3F6\uA3F7\uA3F8\uA3F9\uA3FA\uA3FB\uA3FC\uA3FD\uA3FE\uA3FF\uA400\uA401\uA402\uA403\uA404\uA405\uA406\uA407\uA408\uA409\uA40A\uA40B\uA40C\uA40D\uA40E\uA40F\uA410\uA411\uA412\uA413\uA414\uA415\uA416\uA417\uA418\uA419\uA41A\uA41B\uA41C\uA41D\uA41E\uA41F\uA420\uA421\uA422\uA423\uA424\uA425\uA426\uA427\uA428\uA429\uA42A\uA42B\uA42C\uA42D\uA42E\uA42F\uA430\uA431\uA432\uA4
33\uA434\uA435\uA436\uA437\uA438\uA439\uA43A\uA43B\uA43C\uA43D\uA43E\uA43F\uA440\uA441\uA442\uA443\uA444\uA445\uA446\uA447\uA448\uA449\uA44A\uA44B\uA44C\uA44D\uA44E\uA44F\uA450\uA451\uA452\uA453\uA454\uA455\uA456\uA457\uA458\uA459\uA45A\uA45B\uA45C\uA45D\uA45E\uA45F\uA460\uA461\uA462\uA463\uA464\uA465\uA466\uA467\uA468\uA469\uA46A\uA46B\uA46C\uA46D\uA46E\uA46F\uA470\uA471\uA472\uA473\uA474\uA475\uA476\uA477\uA478\uA479\uA47A\uA47B\uA47C\uA47D\uA47E\uA47F\uA480\uA481\uA482\uA483\uA484\uA485\uA486\uA487\uA488\uA489\uA48A\uA48B\uA48C\uA500\uA501\uA502\uA503\uA504\uA505\uA506\uA507\uA508\uA509\uA50A\uA50B\uA50C\uA50D\uA50E\uA50F\uA510\uA511\uA512\uA513\uA514\uA515\uA516\uA517\uA518\uA519\uA51A\uA51B\uA51C\uA51D\uA51E\uA51F\uA520\uA521\uA522\uA523\uA524\uA525\uA526\uA527\uA528\uA529\uA52A\uA52B\uA52C\uA52D\uA52E\uA52F\uA530\uA531\uA532\uA533\uA534\uA535\uA536\uA537\uA538\uA539\uA53A\uA53B\uA53C\uA53D\uA53E\uA53F\uA540\uA541\uA542\uA543\uA544\uA545\uA546\uA547\uA548\uA549\uA54A\uA54B\uA54
C\uA54D\uA54E\uA54F\uA550\uA551\uA552\uA553\uA554\uA555\uA556\uA557\uA558\uA559\uA55A\uA55B\uA55C\uA55D\uA55E\uA55F\uA560\uA561\uA562\uA563\uA564\uA565\uA566\uA567\uA568\uA569\uA56A\uA56B\uA56C\uA56D\uA56E\uA56F\uA570\uA571\uA572\uA573\uA574\uA575\uA576\uA577\uA578\uA579\uA57A\uA57B\uA57C\uA57D\uA57E\uA57F\uA580\uA581\uA582\uA583\uA584\uA585\uA586\uA587\uA588\uA589\uA58A\uA58B\uA58C\uA58D\uA58E\uA58F\uA590\uA591\uA592\uA593\uA594\uA595\uA596\uA597\uA598\uA599\uA59A\uA59B\uA59C\uA59D\uA59E\uA59F\uA5A0\uA5A1\uA5A2\uA5A3\uA5A4\uA5A5\uA5A6\uA5A7\uA5A8\uA5A9\uA5AA\uA5AB\uA5AC\uA5AD\uA5AE\uA5AF\uA5B0\uA5B1\uA5B2\uA5B3\uA5B4\uA5B5\uA5B6\uA5B7\uA5B8\uA5B9\uA5BA\uA5BB\uA5BC\uA5BD\uA5BE\uA5BF\uA5C0\uA5C1\uA5C2\uA5C3\uA5C4\uA5C5\uA5C6\uA5C7\uA5C8\uA5C9\uA5CA\uA5CB\uA5CC\uA5CD\uA5CE\uA5CF\uA5D0\uA5D1\uA5D2\uA5D3\uA5D4\uA5D5\uA5D6\uA5D7\uA5D8\uA5D9\uA5DA\uA5DB\uA5DC\uA5DD\uA5DE\uA5DF\uA5E0\uA5E1\uA5E2\uA5E3\uA5E4\uA5E5\uA5E6\uA5E7\uA5E8\uA5E9\uA5EA\uA5EB\uA5EC\uA5ED\uA5EE\uA5EF\uA5F0\uA5F1\uA5F2
\uA5F3\uA5F4\uA5F5\uA5F6\uA5F7\uA5F8\uA5F9\uA5FA\uA5FB\uA5FC\uA5FD\uA5FE\uA5FF\uA600\uA601\uA602\uA603\uA604\uA605\uA606\uA607\uA608\uA609\uA60A\uA60B\uA610\uA611\uA612\uA613\uA614\uA615\uA616\uA617\uA618\uA619\uA61A\uA61B\uA61C\uA61D\uA61E\uA61F\uA62A\uA62B\uA66E\uA7FB\uA7FC\uA7FD\uA7FE\uA7FF\uA800\uA801\uA803\uA804\uA805\uA807\uA808\uA809\uA80A\uA80C\uA80D\uA80E\uA80F\uA810\uA811\uA812\uA813\uA814\uA815\uA816\uA817\uA818\uA819\uA81A\uA81B\uA81C\uA81D\uA81E\uA81F\uA820\uA821\uA822\uA840\uA841\uA842\uA843\uA844\uA845\uA846\uA847\uA848\uA849\uA84A\uA84B\uA84C\uA84D\uA84E\uA84F\uA850\uA851\uA852\uA853\uA854\uA855\uA856\uA857\uA858\uA859\uA85A\uA85B\uA85C\uA85D\uA85E\uA85F\uA860\uA861\uA862\uA863\uA864\uA865\uA866\uA867\uA868\uA869\uA86A\uA86B\uA86C\uA86D\uA86E\uA86F\uA870\uA871\uA872\uA873\uA882\uA883\uA884\uA885\uA886\uA887\uA888\uA889\uA88A\uA88B\uA88C\uA88D\uA88E\uA88F\uA890\uA891\uA892\uA893\uA894\uA895\uA896\uA897\uA898\uA899\uA89A\uA89B\uA89C\uA89D\uA89E\uA89F\uA8A0\uA8A1\uA8A2\
uA8A3\uA8A4\uA8A5\uA8A6\uA8A7\uA8A8\uA8A9\uA8AA\uA8AB\uA8AC\uA8AD\uA8AE\uA8AF\uA8B0\uA8B1\uA8B2\uA8B3\uA90A\uA90B\uA90C\uA90D\uA90E\uA90F\uA910\uA911\uA912\uA913\uA914\uA915\uA916\uA917\uA918\uA919\uA91A\uA91B\uA91C\uA91D\uA91E\uA91F\uA920\uA921\uA922\uA923\uA924\uA925\uA930\uA931\uA932\uA933\uA934\uA935\uA936\uA937\uA938\uA939\uA93A\uA93B\uA93C\uA93D\uA93E\uA93F\uA940\uA941\uA942\uA943\uA944\uA945\uA946\uAA00\uAA01\uAA02\uAA03\uAA04\uAA05\uAA06\uAA07\uAA08\uAA09\uAA0A\uAA0B\uAA0C\uAA0D\uAA0E\uAA0F\uAA10\uAA11\uAA12\uAA13\uAA14\uAA15\uAA16\uAA17\uAA18\uAA19\uAA1A\uAA1B\uAA1C\uAA1D\uAA1E\uAA1F\uAA20\uAA21\uAA22\uAA23\uAA24\uAA25\uAA26\uAA27\uAA28\uAA40\uAA41\uAA42\uAA44\uAA45\uAA46\uAA47\uAA48\uAA49\uAA4A\uAA4B\uAC00\uD7A3\uF900\uF901\uF902\uF903\uF904\uF905\uF906\uF907\uF908\uF909\uF90A\uF90B\uF90C\uF90D\uF90E\uF90F\uF910\uF911\uF912\uF913\uF914\uF915\uF916\uF917\uF918\uF919\uF91A\uF91B\uF91C\uF91D\uF91E\uF91F\uF920\uF921\uF922\uF923\uF924\uF925\uF926\uF927\uF928\uF929\uF92A\uF92B\u
F92C\uF92D\uF92E\uF92F\uF930\uF931\uF932\uF933\uF934\uF935\uF936\uF937\uF938\uF939\uF93A\uF93B\uF93C\uF93D\uF93E\uF93F\uF940\uF941\uF942\uF943\uF944\uF945\uF946\uF947\uF948\uF949\uF94A\uF94B\uF94C\uF94D\uF94E\uF94F\uF950\uF951\uF952\uF953\uF954\uF955\uF956\uF957\uF958\uF959\uF95A\uF95B\uF95C\uF95D\uF95E\uF95F\uF960\uF961\uF962\uF963\uF964\uF965\uF966\uF967\uF968\uF969\uF96A\uF96B\uF96C\uF96D\uF96E\uF96F\uF970\uF971\uF972\uF973\uF974\uF975\uF976\uF977\uF978\uF979\uF97A\uF97B\uF97C\uF97D\uF97E\uF97F\uF980\uF981\uF982\uF983\uF984\uF985\uF986\uF987\uF988\uF989\uF98A\uF98B\uF98C\uF98D\uF98E\uF98F\uF990\uF991\uF992\uF993\uF994\uF995\uF996\uF997\uF998\uF999\uF99A\uF99B\uF99C\uF99D\uF99E\uF99F\uF9A0\uF9A1\uF9A2\uF9A3\uF9A4\uF9A5\uF9A6\uF9A7\uF9A8\uF9A9\uF9AA\uF9AB\uF9AC\uF9AD\uF9AE\uF9AF\uF9B0\uF9B1\uF9B2\uF9B3\uF9B4\uF9B5\uF9B6\uF9B7\uF9B8\uF9B9\uF9BA\uF9BB\uF9BC\uF9BD\uF9BE\uF9BF\uF9C0\uF9C1\uF9C2\uF9C3\uF9C4\uF9C5\uF9C6\uF9C7\uF9C8\uF9C9\uF9CA\uF9CB\uF9CC\uF9CD\uF9CE\uF9CF\uF9D0\uF9D1\uF
9D2\uF9D3\uF9D4\uF9D5\uF9D6\uF9D7\uF9D8\uF9D9\uF9DA\uF9DB\uF9DC\uF9DD\uF9DE\uF9DF\uF9E0\uF9E1\uF9E2\uF9E3\uF9E4\uF9E5\uF9E6\uF9E7\uF9E8\uF9E9\uF9EA\uF9EB\uF9EC\uF9ED\uF9EE\uF9EF\uF9F0\uF9F1\uF9F2\uF9F3\uF9F4\uF9F5\uF9F6\uF9F7\uF9F8\uF9F9\uF9FA\uF9FB\uF9FC\uF9FD\uF9FE\uF9FF\uFA00\uFA01\uFA02\uFA03\uFA04\uFA05\uFA06\uFA07\uFA08\uFA09\uFA0A\uFA0B\uFA0C\uFA0D\uFA0E\uFA0F\uFA10\uFA11\uFA12\uFA13\uFA14\uFA15\uFA16\uFA17\uFA18\uFA19\uFA1A\uFA1B\uFA1C\uFA1D\uFA1E\uFA1F\uFA20\uFA21\uFA22\uFA23\uFA24\uFA25\uFA26\uFA27\uFA28\uFA29\uFA2A\uFA2B\uFA2C\uFA2D\uFA30\uFA31\uFA32\uFA33\uFA34\uFA35\uFA36\uFA37\uFA38\uFA39\uFA3A\uFA3B\uFA3C\uFA3D\uFA3E\uFA3F\uFA40\uFA41\uFA42\uFA43\uFA44\uFA45\uFA46\uFA47\uFA48\uFA49\uFA4A\uFA4B\uFA4C\uFA4D\uFA4E\uFA4F\uFA50\uFA51\uFA52\uFA53\uFA54\uFA55\uFA56\uFA57\uFA58\uFA59\uFA5A\uFA5B\uFA5C\uFA5D\uFA5E\uFA5F\uFA60\uFA61\uFA62\uFA63\uFA64\uFA65\uFA66\uFA67\uFA68\uFA69\uFA6A\uFA70\uFA71\uFA72\uFA73\uFA74\uFA75\uFA76\uFA77\uFA78\uFA79\uFA7A\uFA7B\uFA7C\uFA7D\uFA7E\uFA
7F\uFA80\uFA81\uFA82\uFA83\uFA84\uFA85\uFA86\uFA87\uFA88\uFA89\uFA8A\uFA8B\uFA8C\uFA8D\uFA8E\uFA8F\uFA90\uFA91\uFA92\uFA93\uFA94\uFA95\uFA96\uFA97\uFA98\uFA99\uFA9A\uFA9B\uFA9C\uFA9D\uFA9E\uFA9F\uFAA0\uFAA1\uFAA2\uFAA3\uFAA4\uFAA5\uFAA6\uFAA7\uFAA8\uFAA9\uFAAA\uFAAB\uFAAC\uFAAD\uFAAE\uFAAF\uFAB0\uFAB1\uFAB2\uFAB3\uFAB4\uFAB5\uFAB6\uFAB7\uFAB8\uFAB9\uFABA\uFABB\uFABC\uFABD\uFABE\uFABF\uFAC0\uFAC1\uFAC2\uFAC3\uFAC4\uFAC5\uFAC6\uFAC7\uFAC8\uFAC9\uFACA\uFACB\uFACC\uFACD\uFACE\uFACF\uFAD0\uFAD1\uFAD2\uFAD3\uFAD4\uFAD5\uFAD6\uFAD7\uFAD8\uFAD9\uFB1D\uFB1F\uFB20\uFB21\uFB22\uFB23\uFB24\uFB25\uFB26\uFB27\uFB28\uFB2A\uFB2B\uFB2C\uFB2D\uFB2E\uFB2F\uFB30\uFB31\uFB32\uFB33\uFB34\uFB35\uFB36\uFB38\uFB39\uFB3A\uFB3B\uFB3C\uFB3E\uFB40\uFB41\uFB43\uFB44\uFB46\uFB47\uFB48\uFB49\uFB4A\uFB4B\uFB4C\uFB4D\uFB4E\uFB4F\uFB50\uFB51\uFB52\uFB53\uFB54\uFB55\uFB56\uFB57\uFB58\uFB59\uFB5A\uFB5B\uFB5C\uFB5D\uFB5E\uFB5F\uFB60\uFB61\uFB62\uFB63\uFB64\uFB65\uFB66\uFB67\uFB68\uFB69\uFB6A\uFB6B\uFB6C\uFB6D\uFB6E\uFB6
F\uFB70\uFB71\uFB72\uFB73\uFB74\uFB75\uFB76\uFB77\uFB78\uFB79\uFB7A\uFB7B\uFB7C\uFB7D\uFB7E\uFB7F\uFB80\uFB81\uFB82\uFB83\uFB84\uFB85\uFB86\uFB87\uFB88\uFB89\uFB8A\uFB8B\uFB8C\uFB8D\uFB8E\uFB8F\uFB90\uFB91\uFB92\uFB93\uFB94\uFB95\uFB96\uFB97\uFB98\uFB99\uFB9A\uFB9B\uFB9C\uFB9D\uFB9E\uFB9F\uFBA0\uFBA1\uFBA2\uFBA3\uFBA4\uFBA5\uFBA6\uFBA7\uFBA8\uFBA9\uFBAA\uFBAB\uFBAC\uFBAD\uFBAE\uFBAF\uFBB0\uFBB1\uFBD3\uFBD4\uFBD5\uFBD6\uFBD7\uFBD8\uFBD9\uFBDA\uFBDB\uFBDC\uFBDD\uFBDE\uFBDF\uFBE0\uFBE1\uFBE2\uFBE3\uFBE4\uFBE5\uFBE6\uFBE7\uFBE8\uFBE9\uFBEA\uFBEB\uFBEC\uFBED\uFBEE\uFBEF\uFBF0\uFBF1\uFBF2\uFBF3\uFBF4\uFBF5\uFBF6\uFBF7\uFBF8\uFBF9\uFBFA\uFBFB\uFBFC\uFBFD\uFBFE\uFBFF\uFC00\uFC01\uFC02\uFC03\uFC04\uFC05\uFC06\uFC07\uFC08\uFC09\uFC0A\uFC0B\uFC0C\uFC0D\uFC0E\uFC0F\uFC10\uFC11\uFC12\uFC13\uFC14\uFC15\uFC16\uFC17\uFC18\uFC19\uFC1A\uFC1B\uFC1C\uFC1D\uFC1E\uFC1F\uFC20\uFC21\uFC22\uFC23\uFC24\uFC25\uFC26\uFC27\uFC28\uFC29\uFC2A\uFC2B\uFC2C\uFC2D\uFC2E\uFC2F\uFC30\uFC31\uFC32\uFC33\uFC34\uFC35\uFC36
\uFC37\uFC38\uFC39\uFC3A\uFC3B\uFC3C\uFC3D\uFC3E\uFC3F\uFC40\uFC41\uFC42\uFC43\uFC44\uFC45\uFC46\uFC47\uFC48\uFC49\uFC4A\uFC4B\uFC4C\uFC4D\uFC4E\uFC4F\uFC50\uFC51\uFC52\uFC53\uFC54\uFC55\uFC56\uFC57\uFC58\uFC59\uFC5A\uFC5B\uFC5C\uFC5D\uFC5E\uFC5F\uFC60\uFC61\uFC62\uFC63\uFC64\uFC65\uFC66\uFC67\uFC68\uFC69\uFC6A\uFC6B\uFC6C\uFC6D\uFC6E\uFC6F\uFC70\uFC71\uFC72\uFC73\uFC74\uFC75\uFC76\uFC77\uFC78\uFC79\uFC7A\uFC7B\uFC7C\uFC7D\uFC7E\uFC7F\uFC80\uFC81\uFC82\uFC83\uFC84\uFC85\uFC86\uFC87\uFC88\uFC89\uFC8A\uFC8B\uFC8C\uFC8D\uFC8E\uFC8F\uFC90\uFC91\uFC92\uFC93\uFC94\uFC95\uFC96\uFC97\uFC98\uFC99\uFC9A\uFC9B\uFC9C\uFC9D\uFC9E\uFC9F\uFCA0\uFCA1\uFCA2\uFCA3\uFCA4\uFCA5\uFCA6\uFCA7\uFCA8\uFCA9\uFCAA\uFCAB\uFCAC\uFCAD\uFCAE\uFCAF\uFCB0\uFCB1\uFCB2\uFCB3\uFCB4\uFCB5\uFCB6\uFCB7\uFCB8\uFCB9\uFCBA\uFCBB\uFCBC\uFCBD\uFCBE\uFCBF\uFCC0\uFCC1\uFCC2\uFCC3\uFCC4\uFCC5\uFCC6\uFCC7\uFCC8\uFCC9\uFCCA\uFCCB\uFCCC\uFCCD\uFCCE\uFCCF\uFCD0\uFCD1\uFCD2\uFCD3\uFCD4\uFCD5\uFCD6\uFCD7\uFCD8\uFCD9\uFCDA\uFCDB\uFCDC\
uFCDD\uFCDE\uFCDF\uFCE0\uFCE1\uFCE2\uFCE3\uFCE4\uFCE5\uFCE6\uFCE7\uFCE8\uFCE9\uFCEA\uFCEB\uFCEC\uFCED\uFCEE\uFCEF\uFCF0\uFCF1\uFCF2\uFCF3\uFCF4\uFCF5\uFCF6\uFCF7\uFCF8\uFCF9\uFCFA\uFCFB\uFCFC\uFCFD\uFCFE\uFCFF\uFD00\uFD01\uFD02\uFD03\uFD04\uFD05\uFD06\uFD07\uFD08\uFD09\uFD0A\uFD0B\uFD0C\uFD0D\uFD0E\uFD0F\uFD10\uFD11\uFD12\uFD13\uFD14\uFD15\uFD16\uFD17\uFD18\uFD19\uFD1A\uFD1B\uFD1C\uFD1D\uFD1E\uFD1F\uFD20\uFD21\uFD22\uFD23\uFD24\uFD25\uFD26\uFD27\uFD28\uFD29\uFD2A\uFD2B\uFD2C\uFD2D\uFD2E\uFD2F\uFD30\uFD31\uFD32\uFD33\uFD34\uFD35\uFD36\uFD37\uFD38\uFD39\uFD3A\uFD3B\uFD3C\uFD3D\uFD50\uFD51\uFD52\uFD53\uFD54\uFD55\uFD56\uFD57\uFD58\uFD59\uFD5A\uFD5B\uFD5C\uFD5D\uFD5E\uFD5F\uFD60\uFD61\uFD62\uFD63\uFD64\uFD65\uFD66\uFD67\uFD68\uFD69\uFD6A\uFD6B\uFD6C\uFD6D\uFD6E\uFD6F\uFD70\uFD71\uFD72\uFD73\uFD74\uFD75\uFD76\uFD77\uFD78\uFD79\uFD7A\uFD7B\uFD7C\uFD7D\uFD7E\uFD7F\uFD80\uFD81\uFD82\uFD83\uFD84\uFD85\uFD86\uFD87\uFD88\uFD89\uFD8A\uFD8B\uFD8C\uFD8D\uFD8E\uFD8F\uFD92\uFD93\uFD94\uFD95\uFD96\u
FD97\uFD98\uFD99\uFD9A\uFD9B\uFD9C\uFD9D\uFD9E\uFD9F\uFDA0\uFDA1\uFDA2\uFDA3\uFDA4\uFDA5\uFDA6\uFDA7\uFDA8\uFDA9\uFDAA\uFDAB\uFDAC\uFDAD\uFDAE\uFDAF\uFDB0\uFDB1\uFDB2\uFDB3\uFDB4\uFDB5\uFDB6\uFDB7\uFDB8\uFDB9\uFDBA\uFDBB\uFDBC\uFDBD\uFDBE\uFDBF\uFDC0\uFDC1\uFDC2\uFDC3\uFDC4\uFDC5\uFDC6\uFDC7\uFDF0\uFDF1\uFDF2\uFDF3\uFDF4\uFDF5\uFDF6\uFDF7\uFDF8\uFDF9\uFDFA\uFDFB\uFE70\uFE71\uFE72\uFE73\uFE74\uFE76\uFE77\uFE78\uFE79\uFE7A\uFE7B\uFE7C\uFE7D\uFE7E\uFE7F\uFE80\uFE81\uFE82\uFE83\uFE84\uFE85\uFE86\uFE87\uFE88\uFE89\uFE8A\uFE8B\uFE8C\uFE8D\uFE8E\uFE8F\uFE90\uFE91\uFE92\uFE93\uFE94\uFE95\uFE96\uFE97\uFE98\uFE99\uFE9A\uFE9B\uFE9C\uFE9D\uFE9E\uFE9F\uFEA0\uFEA1\uFEA2\uFEA3\uFEA4\uFEA5\uFEA6\uFEA7\uFEA8\uFEA9\uFEAA\uFEAB\uFEAC\uFEAD\uFEAE\uFEAF\uFEB0\uFEB1\uFEB2\uFEB3\uFEB4\uFEB5\uFEB6\uFEB7\uFEB8\uFEB9\uFEBA\uFEBB\uFEBC\uFEBD\uFEBE\uFEBF\uFEC0\uFEC1\uFEC2\uFEC3\uFEC4\uFEC5\uFEC6\uFEC7\uFEC8\uFEC9\uFECA\uFECB\uFECC\uFECD\uFECE\uFECF\uFED0\uFED1\uFED2\uFED3\uFED4\uFED5\uFED6\uFED7\uFED8\uFED9\uF
EDA\uFEDB\uFEDC\uFEDD\uFEDE\uFEDF\uFEE0\uFEE1\uFEE2\uFEE3\uFEE4\uFEE5\uFEE6\uFEE7\uFEE8\uFEE9\uFEEA\uFEEB\uFEEC\uFEED\uFEEE\uFEEF\uFEF0\uFEF1\uFEF2\uFEF3\uFEF4\uFEF5\uFEF6\uFEF7\uFEF8\uFEF9\uFEFA\uFEFB\uFEFC\uFF66\uFF67\uFF68\uFF69\uFF6A\uFF6B\uFF6C\uFF6D\uFF6E\uFF6F\uFF71\uFF72\uFF73\uFF74\uFF75\uFF76\uFF77\uFF78\uFF79\uFF7A\uFF7B\uFF7C\uFF7D\uFF7E\uFF7F\uFF80\uFF81\uFF82\uFF83\uFF84\uFF85\uFF86\uFF87\uFF88\uFF89\uFF8A\uFF8B\uFF8C\uFF8D\uFF8E\uFF8F\uFF90\uFF91\uFF92\uFF93\uFF94\uFF95\uFF96\uFF97\uFF98\uFF99\uFF9A\uFF9B\uFF9C\uFF9D\uFFA0\uFFA1\uFFA2\uFFA3\uFFA4\uFFA5\uFFA6\uFFA7\uFFA8\uFFA9\uFFAA\uFFAB\uFFAC\uFFAD\uFFAE\uFFAF\uFFB0\uFFB1\uFFB2\uFFB3\uFFB4\uFFB5\uFFB6\uFFB7\uFFB8\uFFB9\uFFBA\uFFBB\uFFBC\uFFBD\uFFBE\uFFC2\uFFC3\uFFC4\uFFC5\uFFC6\uFFC7\uFFCA\uFFCB\uFFCC\uFFCD\uFFCE\uFFCF\uFFD2\uFFD3\uFFD4\uFFD5\uFFD6\uFFD7\uFFDA\uFFDB\uFFDC]
+
+// Letter, Titlecase
+Lt = [\u01C5\u01C8\u01CB\u01F2\u1F88\u1F89\u1F8A\u1F8B\u1F8C\u1F8D\u1F8E\u1F8F\u1F98\u1F99\u1F9A\u1F9B\u1F9C\u1F9D\u1F9E\u1F9F\u1FA8\u1FA9\u1FAA\u1FAB\u1FAC\u1FAD\u1FAE\u1FAF\u1FBC\u1FCC\u1FFC]
+
+// Letter, Uppercase
+Lu = [\u0041\u0042\u0043\u0044\u0045\u0046\u0047\u0048\u0049\u004A\u004B\u004C\u004D\u004E\u004F\u0050\u0051\u0052\u0053\u0054\u0055\u0056\u0057\u0058\u0059\u005A\u00C0\u00C1\u00C2\u00C3\u00C4\u00C5\u00C6\u00C7\u00C8\u00C9\u00CA\u00CB\u00CC\u00CD\u00CE\u00CF\u00D0\u00D1\u00D2\u00D3\u00D4\u00D5\u00D6\u00D8\u00D9\u00DA\u00DB\u00DC\u00DD\u00DE\u0100\u0102\u0104\u0106\u0108\u010A\u010C\u010E\u0110\u0112\u0114\u0116\u0118\u011A\u011C\u011E\u0120\u0122\u0124\u0126\u0128\u012A\u012C\u012E\u0130\u0132\u0134\u0136\u0139\u013B\u013D\u013F\u0141\u0143\u0145\u0147\u014A\u014C\u014E\u0150\u0152\u0154\u0156\u0158\u015A\u015C\u015E\u0160\u0162\u0164\u0166\u0168\u016A\u016C\u016E\u0170\u0172\u0174\u0176\u0178\u0179\u017B\u017D\u0181\u0182\u0184\u0186\u0187\u0189\u018A\u018B\u018E\u018F\u0190\u0191\u0193\u0194\u0196\u0197\u0198\u019C\u019D\u019F\u01A0\u01A2\u01A4\u01A6\u01A7\u01A9\u01AC\u01AE\u01AF\u01B1\u01B2\u01B3\u01B5\u01B7\u01B8\u01BC\u01C4\u01C7\u01CA\u01CD\u01CF\u01D1\u01D3\u01D5\u01D7\u01D9\
u01DB\u01DE\u01E0\u01E2\u01E4\u01E6\u01E8\u01EA\u01EC\u01EE\u01F1\u01F4\u01F6\u01F7\u01F8\u01FA\u01FC\u01FE\u0200\u0202\u0204\u0206\u0208\u020A\u020C\u020E\u0210\u0212\u0214\u0216\u0218\u021A\u021C\u021E\u0220\u0222\u0224\u0226\u0228\u022A\u022C\u022E\u0230\u0232\u023A\u023B\u023D\u023E\u0241\u0243\u0244\u0245\u0246\u0248\u024A\u024C\u024E\u0370\u0372\u0376\u0386\u0388\u0389\u038A\u038C\u038E\u038F\u0391\u0392\u0393\u0394\u0395\u0396\u0397\u0398\u0399\u039A\u039B\u039C\u039D\u039E\u039F\u03A0\u03A1\u03A3\u03A4\u03A5\u03A6\u03A7\u03A8\u03A9\u03AA\u03AB\u03CF\u03D2\u03D3\u03D4\u03D8\u03DA\u03DC\u03DE\u03E0\u03E2\u03E4\u03E6\u03E8\u03EA\u03EC\u03EE\u03F4\u03F7\u03F9\u03FA\u03FD\u03FE\u03FF\u0400\u0401\u0402\u0403\u0404\u0405\u0406\u0407\u0408\u0409\u040A\u040B\u040C\u040D\u040E\u040F\u0410\u0411\u0412\u0413\u0414\u0415\u0416\u0417\u0418\u0419\u041A\u041B\u041C\u041D\u041E\u041F\u0420\u0421\u0422\u0423\u0424\u0425\u0426\u0427\u0428\u0429\u042A\u042B\u042C\u042D\u042E\u042F\u0460\u0462\u
0464\u0466\u0468\u046A\u046C\u046E\u0470\u0472\u0474\u0476\u0478\u047A\u047C\u047E\u0480\u048A\u048C\u048E\u0490\u0492\u0494\u0496\u0498\u049A\u049C\u049E\u04A0\u04A2\u04A4\u04A6\u04A8\u04AA\u04AC\u04AE\u04B0\u04B2\u04B4\u04B6\u04B8\u04BA\u04BC\u04BE\u04C0\u04C1\u04C3\u04C5\u04C7\u04C9\u04CB\u04CD\u04D0\u04D2\u04D4\u04D6\u04D8\u04DA\u04DC\u04DE\u04E0\u04E2\u04E4\u04E6\u04E8\u04EA\u04EC\u04EE\u04F0\u04F2\u04F4\u04F6\u04F8\u04FA\u04FC\u04FE\u0500\u0502\u0504\u0506\u0508\u050A\u050C\u050E\u0510\u0512\u0514\u0516\u0518\u051A\u051C\u051E\u0520\u0522\u0531\u0532\u0533\u0534\u0535\u0536\u0537\u0538\u0539\u053A\u053B\u053C\u053D\u053E\u053F\u0540\u0541\u0542\u0543\u0544\u0545\u0546\u0547\u0548\u0549\u054A\u054B\u054C\u054D\u054E\u054F\u0550\u0551\u0552\u0553\u0554\u0555\u0556\u10A0\u10A1\u10A2\u10A3\u10A4\u10A5\u10A6\u10A7\u10A8\u10A9\u10AA\u10AB\u10AC\u10AD\u10AE\u10AF\u10B0\u10B1\u10B2\u10B3\u10B4\u10B5\u10B6\u10B7\u10B8\u10B9\u10BA\u10BB\u10BC\u10BD\u10BE\u10BF\u10C0\u10C1\u10C2\u10C3\u1
0C4\u10C5\u1E00\u1E02\u1E04\u1E06\u1E08\u1E0A\u1E0C\u1E0E\u1E10\u1E12\u1E14\u1E16\u1E18\u1E1A\u1E1C\u1E1E\u1E20\u1E22\u1E24\u1E26\u1E28\u1E2A\u1E2C\u1E2E\u1E30\u1E32\u1E34\u1E36\u1E38\u1E3A\u1E3C\u1E3E\u1E40\u1E42\u1E44\u1E46\u1E48\u1E4A\u1E4C\u1E4E\u1E50\u1E52\u1E54\u1E56\u1E58\u1E5A\u1E5C\u1E5E\u1E60\u1E62\u1E64\u1E66\u1E68\u1E6A\u1E6C\u1E6E\u1E70\u1E72\u1E74\u1E76\u1E78\u1E7A\u1E7C\u1E7E\u1E80\u1E82\u1E84\u1E86\u1E88\u1E8A\u1E8C\u1E8E\u1E90\u1E92\u1E94\u1E9E\u1EA0\u1EA2\u1EA4\u1EA6\u1EA8\u1EAA\u1EAC\u1EAE\u1EB0\u1EB2\u1EB4\u1EB6\u1EB8\u1EBA\u1EBC\u1EBE\u1EC0\u1EC2\u1EC4\u1EC6\u1EC8\u1ECA\u1ECC\u1ECE\u1ED0\u1ED2\u1ED4\u1ED6\u1ED8\u1EDA\u1EDC\u1EDE\u1EE0\u1EE2\u1EE4\u1EE6\u1EE8\u1EEA\u1EEC\u1EEE\u1EF0\u1EF2\u1EF4\u1EF6\u1EF8\u1EFA\u1EFC\u1EFE\u1F08\u1F09\u1F0A\u1F0B\u1F0C\u1F0D\u1F0E\u1F0F\u1F18\u1F19\u1F1A\u1F1B\u1F1C\u1F1D\u1F28\u1F29\u1F2A\u1F2B\u1F2C\u1F2D\u1F2E\u1F2F\u1F38\u1F39\u1F3A\u1F3B\u1F3C\u1F3D\u1F3E\u1F3F\u1F48\u1F49\u1F4A\u1F4B\u1F4C\u1F4D\u1F59\u1F5B\u1F5D\u1F5F\u1F
68\u1F69\u1F6A\u1F6B\u1F6C\u1F6D\u1F6E\u1F6F\u1FB8\u1FB9\u1FBA\u1FBB\u1FC8\u1FC9\u1FCA\u1FCB\u1FD8\u1FD9\u1FDA\u1FDB\u1FE8\u1FE9\u1FEA\u1FEB\u1FEC\u1FF8\u1FF9\u1FFA\u1FFB\u2102\u2107\u210B\u210C\u210D\u2110\u2111\u2112\u2115\u2119\u211A\u211B\u211C\u211D\u2124\u2126\u2128\u212A\u212B\u212C\u212D\u2130\u2131\u2132\u2133\u213E\u213F\u2145\u2183\u2C00\u2C01\u2C02\u2C03\u2C04\u2C05\u2C06\u2C07\u2C08\u2C09\u2C0A\u2C0B\u2C0C\u2C0D\u2C0E\u2C0F\u2C10\u2C11\u2C12\u2C13\u2C14\u2C15\u2C16\u2C17\u2C18\u2C19\u2C1A\u2C1B\u2C1C\u2C1D\u2C1E\u2C1F\u2C20\u2C21\u2C22\u2C23\u2C24\u2C25\u2C26\u2C27\u2C28\u2C29\u2C2A\u2C2B\u2C2C\u2C2D\u2C2E\u2C60\u2C62\u2C63\u2C64\u2C67\u2C69\u2C6B\u2C6D\u2C6E\u2C6F\u2C72\u2C75\u2C80\u2C82\u2C84\u2C86\u2C88\u2C8A\u2C8C\u2C8E\u2C90\u2C92\u2C94\u2C96\u2C98\u2C9A\u2C9C\u2C9E\u2CA0\u2CA2\u2CA4\u2CA6\u2CA8\u2CAA\u2CAC\u2CAE\u2CB0\u2CB2\u2CB4\u2CB6\u2CB8\u2CBA\u2CBC\u2CBE\u2CC0\u2CC2\u2CC4\u2CC6\u2CC8\u2CCA\u2CCC\u2CCE\u2CD0\u2CD2\u2CD4\u2CD6\u2CD8\u2CDA\u2CDC\u2CDE\u2CE0\u2CE
2\uA640\uA642\uA644\uA646\uA648\uA64A\uA64C\uA64E\uA650\uA652\uA654\uA656\uA658\uA65A\uA65C\uA65E\uA662\uA664\uA666\uA668\uA66A\uA66C\uA680\uA682\uA684\uA686\uA688\uA68A\uA68C\uA68E\uA690\uA692\uA694\uA696\uA722\uA724\uA726\uA728\uA72A\uA72C\uA72E\uA732\uA734\uA736\uA738\uA73A\uA73C\uA73E\uA740\uA742\uA744\uA746\uA748\uA74A\uA74C\uA74E\uA750\uA752\uA754\uA756\uA758\uA75A\uA75C\uA75E\uA760\uA762\uA764\uA766\uA768\uA76A\uA76C\uA76E\uA779\uA77B\uA77D\uA77E\uA780\uA782\uA784\uA786\uA78B\uFF21\uFF22\uFF23\uFF24\uFF25\uFF26\uFF27\uFF28\uFF29\uFF2A\uFF2B\uFF2C\uFF2D\uFF2E\uFF2F\uFF30\uFF31\uFF32\uFF33\uFF34\uFF35\uFF36\uFF37\uFF38\uFF39\uFF3A]
+
+// Mark, Spacing Combining
+Mc = [\u0903\u093E\u093F\u0940\u0949\u094A\u094B\u094C\u0982\u0983\u09BE\u09BF\u09C0\u09C7\u09C8\u09CB\u09CC\u09D7\u0A03\u0A3E\u0A3F\u0A40\u0A83\u0ABE\u0ABF\u0AC0\u0AC9\u0ACB\u0ACC\u0B02\u0B03\u0B3E\u0B40\u0B47\u0B48\u0B4B\u0B4C\u0B57\u0BBE\u0BBF\u0BC1\u0BC2\u0BC6\u0BC7\u0BC8\u0BCA\u0BCB\u0BCC\u0BD7\u0C01\u0C02\u0C03\u0C41\u0C42\u0C43\u0C44\u0C82\u0C83\u0CBE\u0CC0\u0CC1\u0CC2\u0CC3\u0CC4\u0CC7\u0CC8\u0CCA\u0CCB\u0CD5\u0CD6\u0D02\u0D03\u0D3E\u0D3F\u0D40\u0D46\u0D47\u0D48\u0D4A\u0D4B\u0D4C\u0D57\u0D82\u0D83\u0DCF\u0DD0\u0DD1\u0DD8\u0DD9\u0DDA\u0DDB\u0DDC\u0DDD\u0DDE\u0DDF\u0DF2\u0DF3\u0F3E\u0F3F\u0F7F\u102B\u102C\u1031\u1038\u103B\u103C\u1056\u1057\u1062\u1063\u1064\u1067\u1068\u1069\u106A\u106B\u106C\u106D\u1083\u1084\u1087\u1088\u1089\u108A\u108B\u108C\u108F\u17B6\u17BE\u17BF\u17C0\u17C1\u17C2\u17C3\u17C4\u17C5\u17C7\u17C8\u1923\u1924\u1925\u1926\u1929\u192A\u192B\u1930\u1931\u1933\u1934\u1935\u1936\u1937\u1938\u19B0\u19B1\u19B2\u19B3\u19B4\u19B5\u19B6\u19B7\u19B8\u19B9\u19BA\u19BB\
u19BC\u19BD\u19BE\u19BF\u19C0\u19C8\u19C9\u1A19\u1A1A\u1A1B\u1B04\u1B35\u1B3B\u1B3D\u1B3E\u1B3F\u1B40\u1B41\u1B43\u1B44\u1B82\u1BA1\u1BA6\u1BA7\u1BAA\u1C24\u1C25\u1C26\u1C27\u1C28\u1C29\u1C2A\u1C2B\u1C34\u1C35\uA823\uA824\uA827\uA880\uA881\uA8B4\uA8B5\uA8B6\uA8B7\uA8B8\uA8B9\uA8BA\uA8BB\uA8BC\uA8BD\uA8BE\uA8BF\uA8C0\uA8C1\uA8C2\uA8C3\uA952\uA953\uAA2F\uAA30\uAA33\uAA34\uAA4D]
+
+// Mark, Nonspacing
+Mn = [\u0300\u0301\u0302\u0303\u0304\u0305\u0306\u0307\u0308\u0309\u030A\u030B\u030C\u030D\u030E\u030F\u0310\u0311\u0312\u0313\u0314\u0315\u0316\u0317\u0318\u0319\u031A\u031B\u031C\u031D\u031E\u031F\u0320\u0321\u0322\u0323\u0324\u0325\u0326\u0327\u0328\u0329\u032A\u032B\u032C\u032D\u032E\u032F\u0330\u0331\u0332\u0333\u0334\u0335\u0336\u0337\u0338\u0339\u033A\u033B\u033C\u033D\u033E\u033F\u0340\u0341\u0342\u0343\u0344\u0345\u0346\u0347\u0348\u0349\u034A\u034B\u034C\u034D\u034E\u034F\u0350\u0351\u0352\u0353\u0354\u0355\u0356\u0357\u0358\u0359\u035A\u035B\u035C\u035D\u035E\u035F\u0360\u0361\u0362\u0363\u0364\u0365\u0366\u0367\u0368\u0369\u036A\u036B\u036C\u036D\u036E\u036F\u0483\u0484\u0485\u0486\u0487\u0591\u0592\u0593\u0594\u0595\u0596\u0597\u0598\u0599\u059A\u059B\u059C\u059D\u059E\u059F\u05A0\u05A1\u05A2\u05A3\u05A4\u05A5\u05A6\u05A7\u05A8\u05A9\u05AA\u05AB\u05AC\u05AD\u05AE\u05AF\u05B0\u05B1\u05B2\u05B3\u05B4\u05B5\u05B6\u05B7\u05B8\u05B9\u05BA\u05BB\u05BC\u05BD\u05BF\u05C1\u05C2\
u05C4\u05C5\u05C7\u0610\u0611\u0612\u0613\u0614\u0615\u0616\u0617\u0618\u0619\u061A\u064B\u064C\u064D\u064E\u064F\u0650\u0651\u0652\u0653\u0654\u0655\u0656\u0657\u0658\u0659\u065A\u065B\u065C\u065D\u065E\u0670\u06D6\u06D7\u06D8\u06D9\u06DA\u06DB\u06DC\u06DF\u06E0\u06E1\u06E2\u06E3\u06E4\u06E7\u06E8\u06EA\u06EB\u06EC\u06ED\u0711\u0730\u0731\u0732\u0733\u0734\u0735\u0736\u0737\u0738\u0739\u073A\u073B\u073C\u073D\u073E\u073F\u0740\u0741\u0742\u0743\u0744\u0745\u0746\u0747\u0748\u0749\u074A\u07A6\u07A7\u07A8\u07A9\u07AA\u07AB\u07AC\u07AD\u07AE\u07AF\u07B0\u07EB\u07EC\u07ED\u07EE\u07EF\u07F0\u07F1\u07F2\u07F3\u0901\u0902\u093C\u0941\u0942\u0943\u0944\u0945\u0946\u0947\u0948\u094D\u0951\u0952\u0953\u0954\u0962\u0963\u0981\u09BC\u09C1\u09C2\u09C3\u09C4\u09CD\u09E2\u09E3\u0A01\u0A02\u0A3C\u0A41\u0A42\u0A47\u0A48\u0A4B\u0A4C\u0A4D\u0A51\u0A70\u0A71\u0A75\u0A81\u0A82\u0ABC\u0AC1\u0AC2\u0AC3\u0AC4\u0AC5\u0AC7\u0AC8\u0ACD\u0AE2\u0AE3\u0B01\u0B3C\u0B3F\u0B41\u0B42\u0B43\u0B44\u0B4D\u0B56\u0B62\u
0B63\u0B82\u0BC0\u0BCD\u0C3E\u0C3F\u0C40\u0C46\u0C47\u0C48\u0C4A\u0C4B\u0C4C\u0C4D\u0C55\u0C56\u0C62\u0C63\u0CBC\u0CBF\u0CC6\u0CCC\u0CCD\u0CE2\u0CE3\u0D41\u0D42\u0D43\u0D44\u0D4D\u0D62\u0D63\u0DCA\u0DD2\u0DD3\u0DD4\u0DD6\u0E31\u0E34\u0E35\u0E36\u0E37\u0E38\u0E39\u0E3A\u0E47\u0E48\u0E49\u0E4A\u0E4B\u0E4C\u0E4D\u0E4E\u0EB1\u0EB4\u0EB5\u0EB6\u0EB7\u0EB8\u0EB9\u0EBB\u0EBC\u0EC8\u0EC9\u0ECA\u0ECB\u0ECC\u0ECD\u0F18\u0F19\u0F35\u0F37\u0F39\u0F71\u0F72\u0F73\u0F74\u0F75\u0F76\u0F77\u0F78\u0F79\u0F7A\u0F7B\u0F7C\u0F7D\u0F7E\u0F80\u0F81\u0F82\u0F83\u0F84\u0F86\u0F87\u0F90\u0F91\u0F92\u0F93\u0F94\u0F95\u0F96\u0F97\u0F99\u0F9A\u0F9B\u0F9C\u0F9D\u0F9E\u0F9F\u0FA0\u0FA1\u0FA2\u0FA3\u0FA4\u0FA5\u0FA6\u0FA7\u0FA8\u0FA9\u0FAA\u0FAB\u0FAC\u0FAD\u0FAE\u0FAF\u0FB0\u0FB1\u0FB2\u0FB3\u0FB4\u0FB5\u0FB6\u0FB7\u0FB8\u0FB9\u0FBA\u0FBB\u0FBC\u0FC6\u102D\u102E\u102F\u1030\u1032\u1033\u1034\u1035\u1036\u1037\u1039\u103A\u103D\u103E\u1058\u1059\u105E\u105F\u1060\u1071\u1072\u1073\u1074\u1082\u1085\u1086\u108D\u1
35F\u1712\u1713\u1714\u1732\u1733\u1734\u1752\u1753\u1772\u1773\u17B7\u17B8\u17B9\u17BA\u17BB\u17BC\u17BD\u17C6\u17C9\u17CA\u17CB\u17CC\u17CD\u17CE\u17CF\u17D0\u17D1\u17D2\u17D3\u17DD\u180B\u180C\u180D\u18A9\u1920\u1921\u1922\u1927\u1928\u1932\u1939\u193A\u193B\u1A17\u1A18\u1B00\u1B01\u1B02\u1B03\u1B34\u1B36\u1B37\u1B38\u1B39\u1B3A\u1B3C\u1B42\u1B6B\u1B6C\u1B6D\u1B6E\u1B6F\u1B70\u1B71\u1B72\u1B73\u1B80\u1B81\u1BA2\u1BA3\u1BA4\u1BA5\u1BA8\u1BA9\u1C2C\u1C2D\u1C2E\u1C2F\u1C30\u1C31\u1C32\u1C33\u1C36\u1C37\u1DC0\u1DC1\u1DC2\u1DC3\u1DC4\u1DC5\u1DC6\u1DC7\u1DC8\u1DC9\u1DCA\u1DCB\u1DCC\u1DCD\u1DCE\u1DCF\u1DD0\u1DD1\u1DD2\u1DD3\u1DD4\u1DD5\u1DD6\u1DD7\u1DD8\u1DD9\u1DDA\u1DDB\u1DDC\u1DDD\u1DDE\u1DDF\u1DE0\u1DE1\u1DE2\u1DE3\u1DE4\u1DE5\u1DE6\u1DFE\u1DFF\u20D0\u20D1\u20D2\u20D3\u20D4\u20D5\u20D6\u20D7\u20D8\u20D9\u20DA\u20DB\u20DC\u20E1\u20E5\u20E6\u20E7\u20E8\u20E9\u20EA\u20EB\u20EC\u20ED\u20EE\u20EF\u20F0\u2DE0\u2DE1\u2DE2\u2DE3\u2DE4\u2DE5\u2DE6\u2DE7\u2DE8\u2DE9\u2DEA\u2DEB\u2DEC\u2DED\u2D
EE\u2DEF\u2DF0\u2DF1\u2DF2\u2DF3\u2DF4\u2DF5\u2DF6\u2DF7\u2DF8\u2DF9\u2DFA\u2DFB\u2DFC\u2DFD\u2DFE\u2DFF\u302A\u302B\u302C\u302D\u302E\u302F\u3099\u309A\uA66F\uA67C\uA67D\uA802\uA806\uA80B\uA825\uA826\uA8C4\uA926\uA927\uA928\uA929\uA92A\uA92B\uA92C\uA92D\uA947\uA948\uA949\uA94A\uA94B\uA94C\uA94D\uA94E\uA94F\uA950\uA951\uAA29\uAA2A\uAA2B\uAA2C\uAA2D\uAA2E\uAA31\uAA32\uAA35\uAA36\uAA43\uAA4C\uFB1E\uFE00\uFE01\uFE02\uFE03\uFE04\uFE05\uFE06\uFE07\uFE08\uFE09\uFE0A\uFE0B\uFE0C\uFE0D\uFE0E\uFE0F\uFE20\uFE21\uFE22\uFE23\uFE24\uFE25\uFE26]
+
+// Number, Decimal Digit
+Nd = [\u0030\u0031\u0032\u0033\u0034\u0035\u0036\u0037\u0038\u0039\u0660\u0661\u0662\u0663\u0664\u0665\u0666\u0667\u0668\u0669\u06F0\u06F1\u06F2\u06F3\u06F4\u06F5\u06F6\u06F7\u06F8\u06F9\u07C0\u07C1\u07C2\u07C3\u07C4\u07C5\u07C6\u07C7\u07C8\u07C9\u0966\u0967\u0968\u0969\u096A\u096B\u096C\u096D\u096E\u096F\u09E6\u09E7\u09E8\u09E9\u09EA\u09EB\u09EC\u09ED\u09EE\u09EF\u0A66\u0A67\u0A68\u0A69\u0A6A\u0A6B\u0A6C\u0A6D\u0A6E\u0A6F\u0AE6\u0AE7\u0AE8\u0AE9\u0AEA\u0AEB\u0AEC\u0AED\u0AEE\u0AEF\u0B66\u0B67\u0B68\u0B69\u0B6A\u0B6B\u0B6C\u0B6D\u0B6E\u0B6F\u0BE6\u0BE7\u0BE8\u0BE9\u0BEA\u0BEB\u0BEC\u0BED\u0BEE\u0BEF\u0C66\u0C67\u0C68\u0C69\u0C6A\u0C6B\u0C6C\u0C6D\u0C6E\u0C6F\u0CE6\u0CE7\u0CE8\u0CE9\u0CEA\u0CEB\u0CEC\u0CED\u0CEE\u0CEF\u0D66\u0D67\u0D68\u0D69\u0D6A\u0D6B\u0D6C\u0D6D\u0D6E\u0D6F\u0E50\u0E51\u0E52\u0E53\u0E54\u0E55\u0E56\u0E57\u0E58\u0E59\u0ED0\u0ED1\u0ED2\u0ED3\u0ED4\u0ED5\u0ED6\u0ED7\u0ED8\u0ED9\u0F20\u0F21\u0F22\u0F23\u0F24\u0F25\u0F26\u0F27\u0F28\u0F29\u1040\u1041\u1042\u1043\u1044\
u1045\u1046\u1047\u1048\u1049\u1090\u1091\u1092\u1093\u1094\u1095\u1096\u1097\u1098\u1099\u17E0\u17E1\u17E2\u17E3\u17E4\u17E5\u17E6\u17E7\u17E8\u17E9\u1810\u1811\u1812\u1813\u1814\u1815\u1816\u1817\u1818\u1819\u1946\u1947\u1948\u1949\u194A\u194B\u194C\u194D\u194E\u194F\u19D0\u19D1\u19D2\u19D3\u19D4\u19D5\u19D6\u19D7\u19D8\u19D9\u1B50\u1B51\u1B52\u1B53\u1B54\u1B55\u1B56\u1B57\u1B58\u1B59\u1BB0\u1BB1\u1BB2\u1BB3\u1BB4\u1BB5\u1BB6\u1BB7\u1BB8\u1BB9\u1C40\u1C41\u1C42\u1C43\u1C44\u1C45\u1C46\u1C47\u1C48\u1C49\u1C50\u1C51\u1C52\u1C53\u1C54\u1C55\u1C56\u1C57\u1C58\u1C59\uA620\uA621\uA622\uA623\uA624\uA625\uA626\uA627\uA628\uA629\uA8D0\uA8D1\uA8D2\uA8D3\uA8D4\uA8D5\uA8D6\uA8D7\uA8D8\uA8D9\uA900\uA901\uA902\uA903\uA904\uA905\uA906\uA907\uA908\uA909\uAA50\uAA51\uAA52\uAA53\uAA54\uAA55\uAA56\uAA57\uAA58\uAA59\uFF10\uFF11\uFF12\uFF13\uFF14\uFF15\uFF16\uFF17\uFF18\uFF19]
+
+// Number, Letter
+Nl = [\u16EE\u16EF\u16F0\u2160\u2161\u2162\u2163\u2164\u2165\u2166\u2167\u2168\u2169\u216A\u216B\u216C\u216D\u216E\u216F\u2170\u2171\u2172\u2173\u2174\u2175\u2176\u2177\u2178\u2179\u217A\u217B\u217C\u217D\u217E\u217F\u2180\u2181\u2182\u2185\u2186\u2187\u2188\u3007\u3021\u3022\u3023\u3024\u3025\u3026\u3027\u3028\u3029\u3038\u3039\u303A]
+
+// Punctuation, Connector
+Pc = [\u005F\u203F\u2040\u2054\uFE33\uFE34\uFE4D\uFE4E\uFE4F\uFF3F]
+
+// Separator, Space
+Zs = [\u0020\u00A0\u1680\u180E\u2000\u2001\u2002\u2003\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000]
+
+/* Automatic Semicolon Insertion */
+
+EOS
+ = __ ";"
+ / _ LineTerminatorSequence
+ / _ &"}"
+ / __ EOF
+
+EOSNoLineTerminator
+ = _ ";"
+ / _ LineTerminatorSequence
+ / _ &"}"
+ / _ EOF
+
+EOF
+ = !.
+
+/* Whitespace */
+
+_
+ = (WhiteSpace / MultiLineCommentNoLineTerminator / SingleLineComment)*
+
+__
+ = (WhiteSpace / LineTerminatorSequence / Comment)*
+
+/* ===== A.2 Number Conversions ===== */
+
+/*
+ * Rules from this section are either unused or merged into previous section of
+ * the grammar.
+ */
+
+/* ===== A.3 Expressions ===== */
+
+PrimaryExpression
+ = ThisToken { return { type: "This" }; }
+ / name:Identifier { return { type: "Variable", name: name }; }
+ / Literal
+ / ArrayLiteral
+ / ObjectLiteral
+ / "(" __ expression:Expression __ ")" { return expression; }
+
+ArrayLiteral
+ = "[" __ elements:ElementList? __ (Elision __)? "]" {
+ return {
+ type: "ArrayLiteral",
+ elements: elements !== "" ? elements : []
+ };
+ }
+
+ElementList
+ = (Elision __)?
+ head:AssignmentExpression
+ tail:(__ "," __ Elision? __ AssignmentExpression)* {
+ var result = [head];
+ for (var i = 0; i < tail.length; i++) {
+ result.push(tail[i][5]);
+ }
+ return result;
+ }
+
+Elision
+ = "," (__ ",")*
+
+ObjectLiteral
+ = "{" __ properties:(PropertyNameAndValueList __ ("," __)?)? "}" {
+ return {
+ type: "ObjectLiteral",
+ properties: properties !== "" ? properties[0] : []
+ };
+ }
+
+PropertyNameAndValueList
+ = head:PropertyAssignment tail:(__ "," __ PropertyAssignment)* {
+ var result = [head];
+ for (var i = 0; i < tail.length; i++) {
+ result.push(tail[i][3]);
+ }
+ return result;
+ }
+
+PropertyAssignment
+ = name:PropertyName __ ":" __ value:AssignmentExpression {
+ return {
+ type: "PropertyAssignment",
+ name: name,
+ value: value
+ };
+ }
+ / GetToken __ name:PropertyName __
+ "(" __ ")" __
+ "{" __ body:FunctionBody __ "}" {
+ return {
+ type: "GetterDefinition",
+ name: name,
+ body: body
+ };
+ }
+ / SetToken __ name:PropertyName __
+ "(" __ param:PropertySetParameterList __ ")" __
+ "{" __ body:FunctionBody __ "}" {
+ return {
+ type: "SetterDefinition",
+ name: name,
+ param: param,
+ body: body
+ };
+ }
+
+PropertyName
+ = IdentifierName
+ / StringLiteral
+ / NumericLiteral
+
+PropertySetParameterList
+ = Identifier
+
+MemberExpression
+ = base:(
+ PrimaryExpression
+ / FunctionExpression
+ / NewToken __ constructor:MemberExpression __ arguments:Arguments {
+ return {
+ type: "NewOperator",
+ constructor: constructor,
+ arguments: arguments
+ };
+ }
+ )
+ accessors:(
+ __ "[" __ name:Expression __ "]" { return name; }
+ / __ "." __ name:IdentifierName { return name; }
+ )* {
+ var result = base;
+ for (var i = 0; i < accessors.length; i++) {
+ result = {
+ type: "PropertyAccess",
+ base: result,
+ name: accessors[i]
+ };
+ }
+ return result;
+ }
+
+NewExpression
+ = MemberExpression
+ / NewToken __ constructor:NewExpression {
+ return {
+ type: "NewOperator",
+ constructor: constructor,
+ arguments: []
+ };
+ }
+
+CallExpression
+ = base:(
+ name:MemberExpression __ arguments:Arguments {
+ return {
+ type: "FunctionCall",
+ name: name,
+ arguments: arguments
+ };
+ }
+ )
+ argumentsOrAccessors:(
+ __ arguments:Arguments {
+ return {
+ type: "FunctionCallArguments",
+ arguments: arguments
+ };
+ }
+ / __ "[" __ name:Expression __ "]" {
+ return {
+ type: "PropertyAccessProperty",
+ name: name
+ };
+ }
+ / __ "." __ name:IdentifierName {
+ return {
+ type: "PropertyAccessProperty",
+ name: name
+ };
+ }
+ )* {
+ var result = base;
+ for (var i = 0; i < argumentsOrAccessors.length; i++) {
+ switch (argumentsOrAccessors[i].type) {
+ case "FunctionCallArguments":
+ result = {
+ type: "FuctionCall",
+ name: result,
+ arguments: argumentsOrAccessors[i].arguments
+ };
+ break;
+ case "PropertyAccessProperty":
+ result = {
+ type: "PropertyAccess",
+ base: result,
+ name: argumentsOrAccessors[i].name
+ };
+ break;
+ default:
+ throw new Error(
+ "Invalid expression type: " + argumentsOrAccessors[i].type
+ );
+ }
+ }
+ return result;
+ }
+
+Arguments
+ = "(" __ arguments:ArgumentList? __ ")" {
+ return arguments !== "" ? arguments : [];
+ }
+
+ArgumentList
+ = head:AssignmentExpression tail:(__ "," __ AssignmentExpression)* {
+ var result = [head];
+ for (var i = 0; i < tail.length; i++) {
+ result.push(tail[i][3]);
+ }
+ return result;
+ }
+
+LeftHandSideExpression
+ = CallExpression
+ / NewExpression
+
+PostfixExpression
+ = expression:LeftHandSideExpression _ operator:PostfixOperator {
+ return {
+ type: "PostfixExpression",
+ operator: operator,
+ expression: expression
+ };
+ }
+ / LeftHandSideExpression
+
+PostfixOperator
+ = "++"
+ / "--"
+
+UnaryExpression
+ = PostfixExpression
+ / operator:UnaryOperator __ expression:UnaryExpression {
+ return {
+ type: "UnaryExpression",
+ operator: operator,
+ expression: expression
+ };
+ }
+
+UnaryOperator
+ = DeleteToken
+ / VoidToken
+ / TypeofToken
+ / "++"
+ / "--"
+ / "+"
+ / "-"
+ / "~"
+ / "!"
+
+MultiplicativeExpression
+ = head:UnaryExpression
+ tail:(__ MultiplicativeOperator __ UnaryExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+MultiplicativeOperator
+ = operator:("*" / "/" / "%") !"=" { return operator; }
+
+AdditiveExpression
+ = head:MultiplicativeExpression
+ tail:(__ AdditiveOperator __ MultiplicativeExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+AdditiveOperator
+ = "+" !("+" / "=") { return "+"; }
+ / "-" !("-" / "=") { return "-"; }
+
+ShiftExpression
+ = head:AdditiveExpression
+ tail:(__ ShiftOperator __ AdditiveExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+ShiftOperator
+ = "<<"
+ / ">>>"
+ / ">>"
+
+RelationalExpression
+ = head:ShiftExpression
+ tail:(__ RelationalOperator __ ShiftExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+RelationalOperator
+ = "<="
+ / ">="
+ / "<"
+ / ">"
+ / InstanceofToken
+ / InToken
+
+RelationalExpressionNoIn
+ = head:ShiftExpression
+ tail:(__ RelationalOperatorNoIn __ ShiftExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+RelationalOperatorNoIn
+ = "<="
+ / ">="
+ / "<"
+ / ">"
+ / InstanceofToken
+
+EqualityExpression
+ = head:RelationalExpression
+ tail:(__ EqualityOperator __ RelationalExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+EqualityExpressionNoIn
+ = head:RelationalExpressionNoIn
+ tail:(__ EqualityOperator __ RelationalExpressionNoIn)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+EqualityOperator
+ = "==="
+ / "!=="
+ / "=="
+ / "!="
+
+BitwiseANDExpression
+ = head:EqualityExpression
+ tail:(__ BitwiseANDOperator __ EqualityExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+BitwiseANDExpressionNoIn
+ = head:EqualityExpressionNoIn
+ tail:(__ BitwiseANDOperator __ EqualityExpressionNoIn)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+BitwiseANDOperator
+ = "&" !("&" / "=") { return "&"; }
+
+BitwiseXORExpression
+ = head:BitwiseANDExpression
+ tail:(__ BitwiseXOROperator __ BitwiseANDExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+BitwiseXORExpressionNoIn
+ = head:BitwiseANDExpressionNoIn
+ tail:(__ BitwiseXOROperator __ BitwiseANDExpressionNoIn)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+BitwiseXOROperator
+ = "^" !("^" / "=") { return "^"; }
+
+BitwiseORExpression
+ = head:BitwiseXORExpression
+ tail:(__ BitwiseOROperator __ BitwiseXORExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+BitwiseORExpressionNoIn
+ = head:BitwiseXORExpressionNoIn
+ tail:(__ BitwiseOROperator __ BitwiseXORExpressionNoIn)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+BitwiseOROperator
+ = "|" !("|" / "=") { return "|"; }
+
+LogicalANDExpression
+ = head:BitwiseORExpression
+ tail:(__ LogicalANDOperator __ BitwiseORExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+LogicalANDExpressionNoIn
+ = head:BitwiseORExpressionNoIn
+ tail:(__ LogicalANDOperator __ BitwiseORExpressionNoIn)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+LogicalANDOperator
+ = "&&" !"=" { return "&&"; }
+
+LogicalORExpression
+ = head:LogicalANDExpression
+ tail:(__ LogicalOROperator __ LogicalANDExpression)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+LogicalORExpressionNoIn
+ = head:LogicalANDExpressionNoIn
+ tail:(__ LogicalOROperator __ LogicalANDExpressionNoIn)* {
+ var result = head;
+ for (var i = 0; i < tail.length; i++) {
+ result = {
+ type: "BinaryExpression",
+ operator: tail[i][1],
+ left: result,
+ right: tail[i][3]
+ };
+ }
+ return result;
+ }
+
+LogicalOROperator
+ = "||" !"=" { return "||"; }
+
+ConditionalExpression
+ = condition:LogicalORExpression __
+ "?" __ trueExpression:AssignmentExpression __
+ ":" __ falseExpression:AssignmentExpression {
+ return {
+ type: "ConditionalExpression",
+ condition: condition,
+ trueExpression: trueExpression,
+ falseExpression: falseExpression
+ };
+ }
+ / LogicalORExpression
+
+ConditionalExpressionNoIn
+ = condition:LogicalORExpressionNoIn __
+ "?" __ trueExpression:AssignmentExpressionNoIn __
+ ":" __ falseExpression:AssignmentExpressionNoIn {
+ return {
+ type: "ConditionalExpression",
+ condition: condition,
+ trueExpression: trueExpression,
+ falseExpression: falseExpression
+ };
+ }
+ / LogicalORExpressionNoIn
+
+AssignmentExpression
+ = left:LeftHandSideExpression __
+ operator:AssignmentOperator __
+ right:AssignmentExpression {
+ return {
+ type: "AssignmentExpression",
+ operator: operator,
+ left: left,
+ right: right
+ };
+ }
+ / ConditionalExpression
+
+AssignmentExpressionNoIn
+ = left:LeftHandSideExpression __
+ operator:AssignmentOperator __
+ right:Assignme
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[52/59] [abbrv] mac commit: CB-10668 fixed failing tests,
reverted shelljs to 0.5.3
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/scripts/generate-docs.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/scripts/generate-docs.js b/node_modules/shelljs/scripts/generate-docs.js
index 3a31a91..532fed9 100755
--- a/node_modules/shelljs/scripts/generate-docs.js
+++ b/node_modules/shelljs/scripts/generate-docs.js
@@ -1,5 +1,4 @@
#!/usr/bin/env node
-/* globals cat, cd, echo, grep, sed */
require('../global');
echo('Appending docs to README.md');
@@ -16,11 +15,7 @@ docs = docs.replace(/\/\/\@include (.+)/g, function(match, path) {
// Remove '//@'
docs = docs.replace(/\/\/\@ ?/g, '');
-
-// Wipe out the old docs
-cat('README.md').replace(/## Command reference(.|\n)*/, '## Command reference').to('README.md');
-
-// Append new docs to README
-sed('-i', /## Command reference/, '## Command reference\n\n' + docs, 'README.md');
+// Append docs to README
+sed('-i', /## Command reference(.|\n)*/, '## Command reference\n\n' + docs, 'README.md');
echo('All done.');
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/scripts/run-tests.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/scripts/run-tests.js b/node_modules/shelljs/scripts/run-tests.js
index e8e7ff2..f9d31e0 100755
--- a/node_modules/shelljs/scripts/run-tests.js
+++ b/node_modules/shelljs/scripts/run-tests.js
@@ -1,14 +1,14 @@
#!/usr/bin/env node
-/* globals cd, echo, exec, exit, ls, pwd, test */
require('../global');
-var common = require('../src/common');
+
+var path = require('path');
var failed = false;
//
// Lint
//
-var JSHINT_BIN = 'node_modules/jshint/bin/jshint';
+JSHINT_BIN = './node_modules/jshint/bin/jshint';
cd(__dirname + '/..');
if (!test('-f', JSHINT_BIN)) {
@@ -16,12 +16,7 @@ if (!test('-f', JSHINT_BIN)) {
exit(1);
}
-var jsfiles = common.expand([pwd() + '/*.js',
- pwd() + '/scripts/*.js',
- pwd() + '/src/*.js',
- pwd() + '/test/*.js'
- ]).join(' ');
-if (exec('node ' + pwd() + '/' + JSHINT_BIN + ' ' + jsfiles).code !== 0) {
+if (exec(JSHINT_BIN + ' *.js test/*.js').code !== 0) {
failed = true;
echo('*** JSHINT FAILED! (return code != 0)');
echo();
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/shell.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/shell.js b/node_modules/shelljs/shell.js
index 93aff70..bdeb559 100644
--- a/node_modules/shelljs/shell.js
+++ b/node_modules/shelljs/shell.js
@@ -107,13 +107,6 @@ exports.exec = common.wrap('exec', _exec, {notUnix:true});
var _chmod = require('./src/chmod');
exports.chmod = common.wrap('chmod', _chmod);
-//@include ./src/touch
-var _touch = require('./src/touch');
-exports.touch = common.wrap('touch', _touch);
-
-//@include ./src/set
-var _set = require('./src/set');
-exports.set = common.wrap('set', _set);
//@
@@ -158,27 +151,9 @@ exports.config = common.config;
//@
//@ ```javascript
//@ require('shelljs/global');
-//@ config.fatal = true; // or set('-e');
+//@ config.fatal = true;
//@ cp('this_file_does_not_exist', '/dev/null'); // dies here
//@ /* more commands... */
//@ ```
//@
-//@ If `true` the script will die on errors. Default is `false`. This is
-//@ analogous to Bash's `set -e`
-
-//@
-//@ ### config.verbose
-//@ Example:
-//@
-//@ ```javascript
-//@ config.verbose = true; // or set('-v');
-//@ cd('dir/');
-//@ ls('subdir/');
-//@ ```
-//@
-//@ Will print each command as follows:
-//@
-//@ ```
-//@ cd dir/
-//@ ls subdir/
-//@ ```
+//@ If `true` the script will die on errors. Default is `false`.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/cat.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/cat.js b/node_modules/shelljs/src/cat.js
index 5840b4e..f6f4d25 100644
--- a/node_modules/shelljs/src/cat.js
+++ b/node_modules/shelljs/src/cat.js
@@ -32,9 +32,12 @@ function _cat(options, files) {
if (!fs.existsSync(file))
common.error('no such file or directory: ' + file);
- cat += fs.readFileSync(file, 'utf8');
+ cat += fs.readFileSync(file, 'utf8') + '\n';
});
+ if (cat[cat.length-1] === '\n')
+ cat = cat.substring(0, cat.length-1);
+
return common.ShellString(cat);
}
module.exports = _cat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/cd.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/cd.js b/node_modules/shelljs/src/cd.js
index b7b9931..230f432 100644
--- a/node_modules/shelljs/src/cd.js
+++ b/node_modules/shelljs/src/cd.js
@@ -2,19 +2,11 @@ var fs = require('fs');
var common = require('./common');
//@
-//@ ### cd([dir])
-//@ Changes to directory `dir` for the duration of the script. Changes to home
-//@ directory if no argument is supplied.
+//@ ### cd('dir')
+//@ Changes to directory `dir` for the duration of the script
function _cd(options, dir) {
if (!dir)
- dir = common.getUserHome();
-
- if (dir === '-') {
- if (!common.state.previousDir)
- common.error('could not find previous directory');
- else
- dir = common.state.previousDir;
- }
+ common.error('directory not specified');
if (!fs.existsSync(dir))
common.error('no such file or directory: ' + dir);
@@ -22,7 +14,6 @@ function _cd(options, dir) {
if (!fs.statSync(dir).isDirectory())
common.error('not a directory: ' + dir);
- common.state.previousDir = process.cwd();
process.chdir(dir);
}
module.exports = _cd;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/chmod.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/chmod.js b/node_modules/shelljs/src/chmod.js
index 6c6de10..f288893 100644
--- a/node_modules/shelljs/src/chmod.js
+++ b/node_modules/shelljs/src/chmod.js
@@ -114,9 +114,7 @@ function _chmod(options, mode, filePattern) {
return;
}
- var stat = fs.statSync(file);
- var isDir = stat.isDirectory();
- var perms = stat.mode;
+ var perms = fs.statSync(file).mode;
var type = perms & PERMS.TYPE_MASK;
var newPerms = perms;
@@ -137,15 +135,11 @@ function _chmod(options, mode, filePattern) {
var changeGroup = applyTo.indexOf('g') != -1 || applyTo === 'a' || applyTo === '';
var changeOther = applyTo.indexOf('o') != -1 || applyTo === 'a' || applyTo === '';
- var changeRead = change.indexOf('r') != -1;
- var changeWrite = change.indexOf('w') != -1;
- var changeExec = change.indexOf('x') != -1;
- var changeExecDir = change.indexOf('X') != -1;
- var changeSticky = change.indexOf('t') != -1;
- var changeSetuid = change.indexOf('s') != -1;
-
- if (changeExecDir && isDir)
- changeExec = true;
+ var changeRead = change.indexOf('r') != -1;
+ var changeWrite = change.indexOf('w') != -1;
+ var changeExec = change.indexOf('x') != -1;
+ var changeSticky = change.indexOf('t') != -1;
+ var changeSetuid = change.indexOf('s') != -1;
var mask = 0;
if (changeOwner) {
@@ -183,15 +177,14 @@ function _chmod(options, mode, filePattern) {
}
if (options.verbose) {
- console.log(file + ' -> ' + newPerms.toString(8));
+ log(file + ' -> ' + newPerms.toString(8));
}
if (perms != newPerms) {
if (!options.verbose && options.changes) {
- console.log(file + ' -> ' + newPerms.toString(8));
+ log(file + ' -> ' + newPerms.toString(8));
}
fs.chmodSync(file, newPerms);
- perms = newPerms; // for the next round of changes!
}
}
else {
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/common.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/common.js b/node_modules/shelljs/src/common.js
index 33198bd..d8c2312 100644
--- a/node_modules/shelljs/src/common.js
+++ b/node_modules/shelljs/src/common.js
@@ -5,15 +5,13 @@ var _ls = require('./ls');
// Module globals
var config = {
silent: false,
- fatal: false,
- verbose: false,
+ fatal: false
};
exports.config = config;
var state = {
error: null,
currentCmd: 'shell.js',
- previousDir: null,
tempDir: null
};
exports.state = state;
@@ -23,7 +21,7 @@ exports.platform = platform;
function log() {
if (!config.silent)
- console.error.apply(console, arguments);
+ console.log.apply(this, arguments);
}
exports.log = log;
@@ -31,14 +29,10 @@ exports.log = log;
function error(msg, _continue) {
if (state.error === null)
state.error = '';
- var log_entry = state.currentCmd + ': ' + msg;
- if (state.error === '')
- state.error = log_entry;
- else
- state.error += '\n' + log_entry;
+ state.error += state.currentCmd + ': ' + msg + '\n';
if (msg.length > 0)
- log(log_entry);
+ log(state.error);
if (config.fatal)
process.exit(1);
@@ -55,69 +49,38 @@ function ShellString(str) {
}
exports.ShellString = ShellString;
-// Return the home directory in a platform-agnostic way, with consideration for
-// older versions of node
-function getUserHome() {
- var result;
- if (os.homedir)
- result = os.homedir(); // node 3+
- else
- result = process.env[(process.platform === 'win32') ? 'USERPROFILE' : 'HOME'];
- return result;
-}
-exports.getUserHome = getUserHome;
-
-// Returns {'alice': true, 'bob': false} when passed a string and dictionary as follows:
+// Returns {'alice': true, 'bob': false} when passed a dictionary, e.g.:
// parseOptions('-a', {'a':'alice', 'b':'bob'});
-// Returns {'reference': 'string-value', 'bob': false} when passed two dictionaries of the form:
-// parseOptions({'-r': 'string-value'}, {'r':'reference', 'b':'bob'});
-function parseOptions(opt, map) {
+function parseOptions(str, map) {
if (!map)
error('parseOptions() internal error: no map given');
// All options are false by default
var options = {};
- for (var letter in map) {
- if (map[letter][0] !== '!')
- options[map[letter]] = false;
- }
+ for (var letter in map)
+ options[map[letter]] = false;
- if (!opt)
+ if (!str)
return options; // defaults
- var optionName;
- if (typeof opt === 'string') {
- if (opt[0] !== '-')
- return options;
+ if (typeof str !== 'string')
+ error('parseOptions() internal error: wrong str');
- // e.g. chars = ['R', 'f']
- var chars = opt.slice(1).split('');
+ // e.g. match[1] = 'Rf' for str = '-Rf'
+ var match = str.match(/^\-(.+)/);
+ if (!match)
+ return options;
+
+ // e.g. chars = ['R', 'f']
+ var chars = match[1].split('');
+
+ chars.forEach(function(c) {
+ if (c in map)
+ options[map[c]] = true;
+ else
+ error('option not recognized: '+c);
+ });
- chars.forEach(function(c) {
- if (c in map) {
- optionName = map[c];
- if (optionName[0] === '!')
- options[optionName.slice(1, optionName.length-1)] = false;
- else
- options[optionName] = true;
- } else {
- error('option not recognized: '+c);
- }
- });
- } else if (typeof opt === 'object') {
- for (var key in opt) {
- // key is a string of the form '-r', '-d', etc.
- var c = key[1];
- if (c in map) {
- optionName = map[c];
- options[optionName] = opt[key]; // assign the given value
- } else {
- error('option not recognized: '+c);
- }
- }
- } else {
- error('options must be strings or key-value pairs');
- }
return options;
}
exports.parseOptions = parseOptions;
@@ -136,7 +99,7 @@ function expand(list) {
var rest = match[2];
var restRegex = rest.replace(/\*\*/g, ".*").replace(/\*/g, "[^\\/]*");
restRegex = new RegExp(restRegex);
-
+
_ls('-R', root).filter(function (e) {
return restRegex.test(e);
}).forEach(function(file) {
@@ -215,28 +178,11 @@ function wrap(cmd, fn, options) {
try {
var args = [].slice.call(arguments, 0);
- if (config.verbose) {
- args.unshift(cmd);
- console.log.apply(console, args);
- args.shift();
- }
-
if (options && options.notUnix) {
retValue = fn.apply(this, args);
} else {
- if (typeof args[0] === 'object' && args[0].constructor.name === 'Object') {
- args = args; // object count as options
- } else if (args.length === 0 || typeof args[0] !== 'string' || args[0].length <= 1 || args[0][0] !== '-') {
+ if (args.length === 0 || typeof args[0] !== 'string' || args[0][0] !== '-')
args.unshift(''); // only add dummy option if '-option' not already present
- }
- // Expand the '~' if appropriate
- var homeDir = getUserHome();
- args = args.map(function(arg) {
- if (typeof arg === 'string' && arg.slice(0, 2) === '~/' || arg === '~')
- return arg.replace(/^~/, homeDir);
- else
- return arg;
- });
retValue = fn.apply(this, args);
}
} catch (e) {
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/cp.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/cp.js b/node_modules/shelljs/src/cp.js
index 54404ef..ef19f96 100644
--- a/node_modules/shelljs/src/cp.js
+++ b/node_modules/shelljs/src/cp.js
@@ -76,7 +76,7 @@ function cpdirSyncRecursive(sourceDir, destDir, opts) {
fs.symlinkSync(symlinkFull, destFile, os.platform() === "win32" ? "junction" : null);
} else {
/* At this point, we've hit a file actually worth copying... so copy it on over. */
- if (fs.existsSync(destFile) && opts.no_force) {
+ if (fs.existsSync(destFile) && !opts.force) {
common.log('skipping existing file: ' + files[i]);
} else {
copyFileSync(srcFile, destFile);
@@ -88,12 +88,11 @@ function cpdirSyncRecursive(sourceDir, destDir, opts) {
//@
-//@ ### cp([options,] source [, source ...], dest)
-//@ ### cp([options,] source_array, dest)
+//@ ### cp([options ,] source [,source ...], dest)
+//@ ### cp([options ,] source_array, dest)
//@ Available options:
//@
-//@ + `-f`: force (default behavior)
-//@ + `-n`: no-clobber
+//@ + `-f`: force
//@ + `-r, -R`: recursive
//@
//@ Examples:
@@ -107,8 +106,7 @@ function cpdirSyncRecursive(sourceDir, destDir, opts) {
//@ Copies files. The wildcard `*` is accepted.
function _cp(options, sources, dest) {
options = common.parseOptions(options, {
- 'f': '!no_force',
- 'n': 'no_force',
+ 'f': 'force',
'R': 'recursive',
'r': 'recursive'
});
@@ -135,19 +133,15 @@ function _cp(options, sources, dest) {
common.error('dest is not a directory (too many sources)');
// Dest is an existing file, but no -f given
- if (exists && stats.isFile() && options.no_force)
+ if (exists && stats.isFile() && !options.force)
common.error('dest file already exists: ' + dest);
if (options.recursive) {
// Recursive allows the shortcut syntax "sourcedir/" for "sourcedir/*"
// (see Github issue #15)
sources.forEach(function(src, i) {
- if (src[src.length - 1] === '/') {
+ if (src[src.length - 1] === '/')
sources[i] += '*';
- // If src is a directory and dest doesn't exist, 'cp -r src dest' should copy src/* into dest
- } else if (fs.statSync(src).isDirectory() && !exists) {
- sources[i] += '/*';
- }
});
// Create dest
@@ -186,7 +180,7 @@ function _cp(options, sources, dest) {
}
}
- cpdirSyncRecursive(src, newDest, {no_force: options.no_force});
+ cpdirSyncRecursive(src, newDest, {force: options.force});
}
return; // done with dir
}
@@ -199,7 +193,7 @@ function _cp(options, sources, dest) {
if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
thisDest = path.normalize(dest + '/' + path.basename(src));
- if (fs.existsSync(thisDest) && options.no_force) {
+ if (fs.existsSync(thisDest) && !options.force) {
common.error('dest file already exists: ' + thisDest, true);
return; // skip file
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/echo.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/echo.js b/node_modules/shelljs/src/echo.js
index b574adc..760ea84 100644
--- a/node_modules/shelljs/src/echo.js
+++ b/node_modules/shelljs/src/echo.js
@@ -1,7 +1,7 @@
var common = require('./common');
//@
-//@ ### echo(string [, string ...])
+//@ ### echo(string [,string ...])
//@
//@ Examples:
//@
@@ -14,7 +14,7 @@ var common = require('./common');
//@ like `.to()`.
function _echo() {
var messages = [].slice.call(arguments, 0);
- console.log.apply(console, messages);
+ console.log.apply(this, messages);
return common.ShellString(messages.join(' '));
}
module.exports = _echo;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/error.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/error.js b/node_modules/shelljs/src/error.js
index 112563d..cca3efb 100644
--- a/node_modules/shelljs/src/error.js
+++ b/node_modules/shelljs/src/error.js
@@ -6,5 +6,5 @@ var common = require('./common');
//@ otherwise returns string explaining the error
function error() {
return common.state.error;
-}
+};
module.exports = error;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/exec.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/exec.js b/node_modules/shelljs/src/exec.js
index 4174adb..d259a9f 100644
--- a/node_modules/shelljs/src/exec.js
+++ b/node_modules/shelljs/src/exec.js
@@ -5,8 +5,6 @@ var path = require('path');
var fs = require('fs');
var child = require('child_process');
-var DEFAULT_MAXBUFFER_SIZE = 20*1024*1024;
-
// Hack to run child_process.exec() synchronously (sync avoids callback hell)
// Uses a custom wait loop that checks for a flag file, created when the child process is done.
// (Can't do a wait loop that checks for internal Node variables/messages as
@@ -15,42 +13,27 @@ var DEFAULT_MAXBUFFER_SIZE = 20*1024*1024;
function execSync(cmd, opts) {
var tempDir = _tempDir();
var stdoutFile = path.resolve(tempDir+'/'+common.randomFileName()),
- stderrFile = path.resolve(tempDir+'/'+common.randomFileName()),
codeFile = path.resolve(tempDir+'/'+common.randomFileName()),
scriptFile = path.resolve(tempDir+'/'+common.randomFileName()),
sleepFile = path.resolve(tempDir+'/'+common.randomFileName());
- opts = common.extend({
- silent: common.config.silent,
- cwd: _pwd(),
- env: process.env,
- maxBuffer: DEFAULT_MAXBUFFER_SIZE
+ var options = common.extend({
+ silent: common.config.silent
}, opts);
- var previousStdoutContent = '',
- previousStderrContent = '';
- // Echoes stdout and stderr changes from running process, if not silent
- function updateStream(streamFile) {
- if (opts.silent || !fs.existsSync(streamFile))
+ var previousStdoutContent = '';
+ // Echoes stdout changes from running process, if not silent
+ function updateStdout() {
+ if (options.silent || !fs.existsSync(stdoutFile))
return;
- var previousStreamContent,
- proc_stream;
- if (streamFile === stdoutFile) {
- previousStreamContent = previousStdoutContent;
- proc_stream = process.stdout;
- } else { // assume stderr
- previousStreamContent = previousStderrContent;
- proc_stream = process.stderr;
- }
-
- var streamContent = fs.readFileSync(streamFile, 'utf8');
+ var stdoutContent = fs.readFileSync(stdoutFile, 'utf8');
// No changes since last time?
- if (streamContent.length <= previousStreamContent.length)
+ if (stdoutContent.length <= previousStdoutContent.length)
return;
- proc_stream.write(streamContent.substr(previousStreamContent.length));
- previousStreamContent = streamContent;
+ process.stdout.write(stdoutContent.substr(previousStdoutContent.length));
+ previousStdoutContent = stdoutContent;
}
function escape(str) {
@@ -59,64 +42,64 @@ function execSync(cmd, opts) {
if (fs.existsSync(scriptFile)) common.unlinkSync(scriptFile);
if (fs.existsSync(stdoutFile)) common.unlinkSync(stdoutFile);
- if (fs.existsSync(stderrFile)) common.unlinkSync(stderrFile);
if (fs.existsSync(codeFile)) common.unlinkSync(codeFile);
var execCommand = '"'+process.execPath+'" '+scriptFile;
- var script;
+ var execOptions = {
+ env: process.env,
+ cwd: _pwd(),
+ maxBuffer: 20*1024*1024
+ };
if (typeof child.execSync === 'function') {
- script = [
+ var script = [
"var child = require('child_process')",
" , fs = require('fs');",
- "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: "+opts.maxBuffer+"}, function(err) {",
+ "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
" fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
"});",
"var stdoutStream = fs.createWriteStream('"+escape(stdoutFile)+"');",
- "var stderrStream = fs.createWriteStream('"+escape(stderrFile)+"');",
"childProcess.stdout.pipe(stdoutStream, {end: false});",
- "childProcess.stderr.pipe(stderrStream, {end: false});",
+ "childProcess.stderr.pipe(stdoutStream, {end: false});",
"childProcess.stdout.pipe(process.stdout);",
"childProcess.stderr.pipe(process.stderr);",
"var stdoutEnded = false, stderrEnded = false;",
- "function tryClosingStdout(){ if(stdoutEnded){ stdoutStream.end(); } }",
- "function tryClosingStderr(){ if(stderrEnded){ stderrStream.end(); } }",
- "childProcess.stdout.on('end', function(){ stdoutEnded = true; tryClosingStdout(); });",
- "childProcess.stderr.on('end', function(){ stderrEnded = true; tryClosingStderr(); });"
+ "function tryClosing(){ if(stdoutEnded && stderrEnded){ stdoutStream.end(); } }",
+ "childProcess.stdout.on('end', function(){ stdoutEnded = true; tryClosing(); });",
+ "childProcess.stderr.on('end', function(){ stderrEnded = true; tryClosing(); });"
].join('\n');
fs.writeFileSync(scriptFile, script);
- if (opts.silent) {
- opts.stdio = 'ignore';
+ if (options.silent) {
+ execOptions.stdio = 'ignore';
} else {
- opts.stdio = [0, 1, 2];
+ execOptions.stdio = [0, 1, 2];
}
// Welcome to the future
- child.execSync(execCommand, opts);
+ child.execSync(execCommand, execOptions);
} else {
- cmd += ' > '+stdoutFile+' 2> '+stderrFile; // works on both win/unix
+ cmd += ' > '+stdoutFile+' 2>&1'; // works on both win/unix
- script = [
+ var script = [
"var child = require('child_process')",
" , fs = require('fs');",
- "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: "+opts.maxBuffer+"}, function(err) {",
+ "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
" fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
"});"
].join('\n');
fs.writeFileSync(scriptFile, script);
- child.exec(execCommand, opts);
+ child.exec(execCommand, execOptions);
// The wait loop
// sleepFile is used as a dummy I/O op to mitigate unnecessary CPU usage
// (tried many I/O sync ops, writeFileSync() seems to be only one that is effective in reducing
// CPU usage, though apparently not so much on Windows)
- while (!fs.existsSync(codeFile)) { updateStream(stdoutFile); fs.writeFileSync(sleepFile, 'a'); }
- while (!fs.existsSync(stdoutFile)) { updateStream(stdoutFile); fs.writeFileSync(sleepFile, 'a'); }
- while (!fs.existsSync(stderrFile)) { updateStream(stderrFile); fs.writeFileSync(sleepFile, 'a'); }
+ while (!fs.existsSync(codeFile)) { updateStdout(); fs.writeFileSync(sleepFile, 'a'); }
+ while (!fs.existsSync(stdoutFile)) { updateStdout(); fs.writeFileSync(sleepFile, 'a'); }
}
// At this point codeFile exists, but it's not necessarily flushed yet.
@@ -127,12 +110,10 @@ function execSync(cmd, opts) {
}
var stdout = fs.readFileSync(stdoutFile, 'utf8');
- var stderr = fs.readFileSync(stderrFile, 'utf8');
// No biggie if we can't erase the files now -- they're in a temp dir anyway
try { common.unlinkSync(scriptFile); } catch(e) {}
try { common.unlinkSync(stdoutFile); } catch(e) {}
- try { common.unlinkSync(stderrFile); } catch(e) {}
try { common.unlinkSync(codeFile); } catch(e) {}
try { common.unlinkSync(sleepFile); } catch(e) {}
@@ -143,40 +124,34 @@ function execSync(cmd, opts) {
// True if successful, false if not
var obj = {
code: code,
- output: stdout, // deprecated
- stdout: stdout,
- stderr: stderr
+ output: stdout
};
return obj;
} // execSync()
// Wrapper around exec() to enable echoing output to console in real time
function execAsync(cmd, opts, callback) {
- var stdout = '';
- var stderr = '';
+ var output = '';
- opts = common.extend({
- silent: common.config.silent,
- cwd: _pwd(),
- env: process.env,
- maxBuffer: DEFAULT_MAXBUFFER_SIZE
+ var options = common.extend({
+ silent: common.config.silent
}, opts);
- var c = child.exec(cmd, opts, function(err) {
+ var c = child.exec(cmd, {env: process.env, maxBuffer: 20*1024*1024}, function(err) {
if (callback)
- callback(err ? err.code : 0, stdout, stderr);
+ callback(err ? err.code : 0, output);
});
c.stdout.on('data', function(data) {
- stdout += data;
- if (!opts.silent)
+ output += data;
+ if (!options.silent)
process.stdout.write(data);
});
c.stderr.on('data', function(data) {
- stderr += data;
- if (!opts.silent)
- process.stderr.write(data);
+ output += data;
+ if (!options.silent)
+ process.stdout.write(data);
});
return c;
@@ -186,33 +161,29 @@ function execAsync(cmd, opts, callback) {
//@ ### exec(command [, options] [, callback])
//@ Available options (all `false` by default):
//@
-//@ + `async`: Asynchronous execution. If a callback is provided, it will be set to
-//@ `true`, regardless of the passed value.
+//@ + `async`: Asynchronous execution. Defaults to true if a callback is provided.
//@ + `silent`: Do not echo program output to console.
-//@ + and any option available to NodeJS's
-//@ [child_process.exec()](https://nodejs.org/api/child_process.html#child_process_child_process_exec_command_options_callback)
//@
//@ Examples:
//@
//@ ```javascript
-//@ var version = exec('node --version', {silent:true}).stdout;
+//@ var version = exec('node --version', {silent:true}).output;
//@
//@ var child = exec('some_long_running_process', {async:true});
//@ child.stdout.on('data', function(data) {
//@ /* ... do something with data ... */
//@ });
//@
-//@ exec('some_long_running_process', function(code, stdout, stderr) {
+//@ exec('some_long_running_process', function(code, output) {
//@ console.log('Exit code:', code);
-//@ console.log('Program output:', stdout);
-//@ console.log('Program stderr:', stderr);
+//@ console.log('Program output:', output);
//@ });
//@ ```
//@
-//@ Executes the given `command` _synchronously_, unless otherwise specified. When in synchronous
-//@ mode returns the object `{ code:..., stdout:... , stderr:... }`, containing the program's
-//@ `stdout`, `stderr`, and its exit `code`. Otherwise returns the child process object,
-//@ and the `callback` gets the arguments `(code, stdout, stderr)`.
+//@ Executes the given `command` _synchronously_, unless otherwise specified.
+//@ When in synchronous mode returns the object `{ code:..., output:... }`, containing the program's
+//@ `output` (stdout + stderr) and its exit `code`. Otherwise returns the child process object, and
+//@ the `callback` gets the arguments `(code, output)`.
//@
//@ **Note:** For long-lived processes, it's best to run `exec()` asynchronously as
//@ the current synchronous implementation uses a lot of CPU. This should be getting
@@ -237,13 +208,9 @@ function _exec(command, options, callback) {
async: false
}, options);
- try {
- if (options.async)
- return execAsync(command, options, callback);
- else
- return execSync(command, options);
- } catch (e) {
- common.error('internal error');
- }
+ if (options.async)
+ return execAsync(command, options, callback);
+ else
+ return execSync(command, options);
}
module.exports = _exec;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/find.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/find.js b/node_modules/shelljs/src/find.js
index c96fb2f..d9eeec2 100644
--- a/node_modules/shelljs/src/find.js
+++ b/node_modules/shelljs/src/find.js
@@ -3,7 +3,7 @@ var common = require('./common');
var _ls = require('./ls');
//@
-//@ ### find(path [, path ...])
+//@ ### find(path [,path ...])
//@ ### find(path_array)
//@ Examples:
//@
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/grep.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/grep.js b/node_modules/shelljs/src/grep.js
index 78008ce..00c7d6a 100644
--- a/node_modules/shelljs/src/grep.js
+++ b/node_modules/shelljs/src/grep.js
@@ -2,8 +2,8 @@ var common = require('./common');
var fs = require('fs');
//@
-//@ ### grep([options,] regex_filter, file [, file ...])
-//@ ### grep([options,] regex_filter, file_array)
+//@ ### grep([options ,] regex_filter, file [, file ...])
+//@ ### grep([options ,] regex_filter, file_array)
//@ Available options:
//@
//@ + `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/ln.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/ln.js b/node_modules/shelljs/src/ln.js
index 878fda1..a7b9701 100644
--- a/node_modules/shelljs/src/ln.js
+++ b/node_modules/shelljs/src/ln.js
@@ -1,13 +1,15 @@
var fs = require('fs');
var path = require('path');
var common = require('./common');
+var os = require('os');
//@
-//@ ### ln([options,] source, dest)
+//@ ### ln(options, source, dest)
+//@ ### ln(source, dest)
//@ Available options:
//@
-//@ + `-s`: symlink
-//@ + `-f`: force
+//@ + `s`: symlink
+//@ + `f`: force
//@
//@ Examples:
//@
@@ -27,11 +29,13 @@ function _ln(options, source, dest) {
common.error('Missing <source> and/or <dest>');
}
- source = String(source);
- var sourcePath = path.normalize(source).replace(RegExp(path.sep + '$'), '');
- var isAbsolute = (path.resolve(source) === sourcePath);
+ source = path.resolve(process.cwd(), String(source));
dest = path.resolve(process.cwd(), String(dest));
+ if (!fs.existsSync(source)) {
+ common.error('Source file does not exist', true);
+ }
+
if (fs.existsSync(dest)) {
if (!options.force) {
common.error('Destination file exists', true);
@@ -41,29 +45,9 @@ function _ln(options, source, dest) {
}
if (options.symlink) {
- var isWindows = common.platform === 'win';
- var linkType = isWindows ? 'file' : null;
- var resolvedSourcePath = isAbsolute ? sourcePath : path.resolve(process.cwd(), path.dirname(dest), source);
- if (!fs.existsSync(resolvedSourcePath)) {
- common.error('Source file does not exist', true);
- } else if (isWindows && fs.statSync(resolvedSourcePath).isDirectory()) {
- linkType = 'junction';
- }
-
- try {
- fs.symlinkSync(linkType === 'junction' ? resolvedSourcePath: source, dest, linkType);
- } catch (err) {
- common.error(err.message);
- }
+ fs.symlinkSync(source, dest, os.platform() === "win32" ? "junction" : null);
} else {
- if (!fs.existsSync(source)) {
- common.error('Source file does not exist', true);
- }
- try {
- fs.linkSync(source, dest);
- } catch (err) {
- common.error(err.message);
- }
+ fs.linkSync(source, dest, os.platform() === "win32" ? "junction" : null);
}
}
module.exports = _ln;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/ls.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/ls.js b/node_modules/shelljs/src/ls.js
index 6a54b3a..3345db4 100644
--- a/node_modules/shelljs/src/ls.js
+++ b/node_modules/shelljs/src/ls.js
@@ -5,17 +5,12 @@ var _cd = require('./cd');
var _pwd = require('./pwd');
//@
-//@ ### ls([options,] [path, ...])
-//@ ### ls([options,] path_array)
+//@ ### ls([options ,] path [,path ...])
+//@ ### ls([options ,] path_array)
//@ Available options:
//@
//@ + `-R`: recursive
//@ + `-A`: all files (include files beginning with `.`, except for `.` and `..`)
-//@ + `-d`: list directories themselves, not their contents
-//@ + `-l`: list objects representing each file, each with fields containing `ls
-//@ -l` output fields. See
-//@ [fs.Stats](https://nodejs.org/api/fs.html#fs_class_fs_stats)
-//@ for more info
//@
//@ Examples:
//@
@@ -23,7 +18,6 @@ var _pwd = require('./pwd');
//@ ls('projs/*.js');
//@ ls('-R', '/users/me', '/tmp');
//@ ls('-R', ['/users/me', '/tmp']); // same as above
-//@ ls('-l', 'file.txt'); // { name: 'file.txt', mode: 33188, nlink: 1, ...}
//@ ```
//@
//@ Returns array of files in the given path, or in current directory if no path provided.
@@ -31,9 +25,7 @@ function _ls(options, paths) {
options = common.parseOptions(options, {
'R': 'recursive',
'A': 'all',
- 'a': 'all_deprecated',
- 'd': 'directory',
- 'l': 'long'
+ 'a': 'all_deprecated'
});
if (options.all_deprecated) {
@@ -56,49 +48,33 @@ function _ls(options, paths) {
// Conditionally pushes file to list - returns true if pushed, false otherwise
// (e.g. prevents hidden files to be included unless explicitly told so)
function pushFile(file, query) {
- var name = file.name || file;
// hidden file?
- if (path.basename(name)[0] === '.') {
+ if (path.basename(file)[0] === '.') {
// not explicitly asking for hidden files?
if (!options.all && !(path.basename(query)[0] === '.' && path.basename(query).length > 1))
return false;
}
if (common.platform === 'win')
- name = name.replace(/\\/g, '/');
+ file = file.replace(/\\/g, '/');
- if (file.name) {
- file.name = name;
- } else {
- file = name;
- }
list.push(file);
return true;
}
paths.forEach(function(p) {
if (fs.existsSync(p)) {
- var stats = ls_stat(p);
+ var stats = fs.statSync(p);
// Simple file?
if (stats.isFile()) {
- if (options.long) {
- pushFile(stats, p);
- } else {
- pushFile(p, p);
- }
+ pushFile(p, p);
return; // continue
}
// Simple dir?
- if (options.directory) {
- pushFile(p, p);
- return;
- } else if (stats.isDirectory()) {
+ if (stats.isDirectory()) {
// Iterate over p contents
fs.readdirSync(p).forEach(function(file) {
- var orig_file = file;
- if (options.long)
- file = ls_stat(path.join(p, file));
if (!pushFile(file, p))
return;
@@ -106,8 +82,8 @@ function _ls(options, paths) {
if (options.recursive) {
var oldDir = _pwd();
_cd('', p);
- if (fs.statSync(orig_file).isDirectory())
- list = list.concat(_ls('-R'+(options.all?'A':''), orig_file+'/*'));
+ if (fs.statSync(file).isDirectory())
+ list = list.concat(_ls('-R'+(options.all?'A':''), file+'/*'));
_cd('', oldDir);
}
});
@@ -128,13 +104,7 @@ function _ls(options, paths) {
// Iterate over directory contents
fs.readdirSync(dirname).forEach(function(file) {
if (file.match(new RegExp(regexp))) {
- var file_path = path.join(dirname, file);
- file_path = options.long ? ls_stat(file_path) : file_path;
- if (file_path.name)
- file_path.name = path.normalize(file_path.name);
- else
- file_path = path.normalize(file_path);
- if (!pushFile(file_path, basename))
+ if (!pushFile(path.normalize(dirname+'/'+file), basename))
return;
// Recursive?
@@ -154,15 +124,3 @@ function _ls(options, paths) {
return list;
}
module.exports = _ls;
-
-
-function ls_stat(path) {
- var stats = fs.statSync(path);
- // Note: this object will contain more information than .toString() returns
- stats.name = path;
- stats.toString = function() {
- // Return a string resembling unix's `ls -l` format
- return [this.mode, this.nlink, this.uid, this.gid, this.size, this.mtime, this.name].join(' ');
- };
- return stats;
-}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/mkdir.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/mkdir.js b/node_modules/shelljs/src/mkdir.js
index 8b4fd99..5a7088f 100644
--- a/node_modules/shelljs/src/mkdir.js
+++ b/node_modules/shelljs/src/mkdir.js
@@ -20,11 +20,11 @@ function mkdirSyncRecursive(dir) {
}
//@
-//@ ### mkdir([options,] dir [, dir ...])
-//@ ### mkdir([options,] dir_array)
+//@ ### mkdir([options ,] dir [, dir ...])
+//@ ### mkdir([options ,] dir_array)
//@ Available options:
//@
-//@ + `-p`: full path (will create intermediate dirs if necessary)
+//@ + `p`: full path (will create intermediate dirs if necessary)
//@
//@ Examples:
//@
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/mv.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/mv.js b/node_modules/shelljs/src/mv.js
index 69cc03f..11f9607 100644
--- a/node_modules/shelljs/src/mv.js
+++ b/node_modules/shelljs/src/mv.js
@@ -3,17 +3,16 @@ var path = require('path');
var common = require('./common');
//@
-//@ ### mv([options ,] source [, source ...], dest')
-//@ ### mv([options ,] source_array, dest')
+//@ ### mv(source [, source ...], dest')
+//@ ### mv(source_array, dest')
//@ Available options:
//@
-//@ + `-f`: force (default behavior)
-//@ + `-n`: no-clobber
+//@ + `f`: force
//@
//@ Examples:
//@
//@ ```javascript
-//@ mv('-n', 'file', 'dir/');
+//@ mv('-f', 'file', 'dir/');
//@ mv('file1', 'file2', 'dir/');
//@ mv(['file1', 'file2'], 'dir/'); // same as above
//@ ```
@@ -21,8 +20,7 @@ var common = require('./common');
//@ Moves files. The wildcard `*` is accepted.
function _mv(options, sources, dest) {
options = common.parseOptions(options, {
- 'f': '!no_force',
- 'n': 'no_force'
+ 'f': 'force'
});
// Get sources, dest
@@ -49,7 +47,7 @@ function _mv(options, sources, dest) {
common.error('dest is not a directory (too many sources)');
// Dest is an existing file, but no -f given
- if (exists && stats.isFile() && options.no_force)
+ if (exists && stats.isFile() && !options.force)
common.error('dest file already exists: ' + dest);
sources.forEach(function(src) {
@@ -66,7 +64,7 @@ function _mv(options, sources, dest) {
if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
thisDest = path.normalize(dest + '/' + path.basename(src));
- if (fs.existsSync(thisDest) && options.no_force) {
+ if (fs.existsSync(thisDest) && !options.force) {
common.error('dest file already exists: ' + thisDest, true);
return; // skip file
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/pwd.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/pwd.js b/node_modules/shelljs/src/pwd.js
index 26cefe0..41727bb 100644
--- a/node_modules/shelljs/src/pwd.js
+++ b/node_modules/shelljs/src/pwd.js
@@ -4,7 +4,7 @@ var common = require('./common');
//@
//@ ### pwd()
//@ Returns the current directory.
-function _pwd() {
+function _pwd(options) {
var pwd = path.resolve(process.cwd());
return common.ShellString(pwd);
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/rm.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/rm.js b/node_modules/shelljs/src/rm.js
index cf2e95b..bd608cb 100644
--- a/node_modules/shelljs/src/rm.js
+++ b/node_modules/shelljs/src/rm.js
@@ -53,7 +53,7 @@ function rmdirSyncRecursive(dir, force) {
while (true) {
try {
result = fs.rmdirSync(dir);
- if (fs.existsSync(dir)) throw { code: "EAGAIN" };
+ if (fs.existsSync(dir)) throw { code: "EAGAIN" }
break;
} catch(er) {
// In addition to error codes, also check if the directory still exists and loop again if true
@@ -89,8 +89,8 @@ function isWriteable(file) {
}
//@
-//@ ### rm([options,] file [, file ...])
-//@ ### rm([options,] file_array)
+//@ ### rm([options ,] file [, file ...])
+//@ ### rm([options ,] file_array)
//@ Available options:
//@
//@ + `-f`: force
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/sed.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/sed.js b/node_modules/shelljs/src/sed.js
index baa385b..65f7cb4 100644
--- a/node_modules/shelljs/src/sed.js
+++ b/node_modules/shelljs/src/sed.js
@@ -2,8 +2,7 @@ var common = require('./common');
var fs = require('fs');
//@
-//@ ### sed([options,] search_regex, replacement, file [, file ...])
-//@ ### sed([options,] search_regex, replacement, file_array)
+//@ ### sed([options ,] search_regex, replacement, file)
//@ Available options:
//@
//@ + `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
@@ -15,9 +14,9 @@ var fs = require('fs');
//@ sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
//@ ```
//@
-//@ Reads an input string from `files` and performs a JavaScript `replace()` on the input
+//@ Reads an input string from `file` and performs a JavaScript `replace()` on the input
//@ using the given search regex and replacement string or function. Returns the new string after replacement.
-function _sed(options, regex, replacement, files) {
+function _sed(options, regex, replacement, file) {
options = common.parseOptions(options, {
'i': 'inplace'
});
@@ -29,36 +28,16 @@ function _sed(options, regex, replacement, files) {
else
common.error('invalid replacement string');
- // Convert all search strings to RegExp
- if (typeof regex === 'string')
- regex = RegExp(regex);
+ if (!file)
+ common.error('no file given');
- if (!files)
- common.error('no files given');
+ if (!fs.existsSync(file))
+ common.error('no such file or directory: ' + file);
- if (typeof files === 'string')
- files = [].slice.call(arguments, 3);
- // if it's array leave it as it is
+ var result = fs.readFileSync(file, 'utf8').replace(regex, replacement);
+ if (options.inplace)
+ fs.writeFileSync(file, result, 'utf8');
- files = common.expand(files);
-
- var sed = [];
- files.forEach(function(file) {
- if (!fs.existsSync(file)) {
- common.error('no such file or directory: ' + file, true);
- return;
- }
-
- var result = fs.readFileSync(file, 'utf8').split('\n').map(function (line) {
- return line.replace(regex, replacement);
- }).join('\n');
-
- sed.push(result);
-
- if (options.inplace)
- fs.writeFileSync(file, result, 'utf8');
- });
-
- return common.ShellString(sed.join('\n'));
+ return common.ShellString(result);
}
module.exports = _sed;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/set.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/set.js b/node_modules/shelljs/src/set.js
deleted file mode 100644
index 19e26d9..0000000
--- a/node_modules/shelljs/src/set.js
+++ /dev/null
@@ -1,49 +0,0 @@
-var common = require('./common');
-
-//@
-//@ ### set(options)
-//@ Available options:
-//@
-//@ + `+/-e`: exit upon error (`config.fatal`)
-//@ + `+/-v`: verbose: show all commands (`config.verbose`)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ set('-e'); // exit upon first error
-//@ set('+e'); // this undoes a "set('-e')"
-//@ ```
-//@
-//@ Sets global configuration variables
-function _set(options) {
- if (!options) {
- var args = [].slice.call(arguments, 0);
- if (args.length < 2)
- common.error('must provide an argument');
- options = args[1];
- }
- var negate = (options[0] === '+');
- if (negate) {
- options = '-' + options.slice(1); // parseOptions needs a '-' prefix
- }
- options = common.parseOptions(options, {
- 'e': 'fatal',
- 'v': 'verbose'
- });
-
- var key;
- if (negate) {
- for (key in options)
- options[key] = !options[key];
- }
-
- for (key in options) {
- // Only change the global config if `negate` is false and the option is true
- // or if `negate` is true and the option is false (aka negate !== option)
- if (negate !== options[key]) {
- common.config[key] = options[key];
- }
- }
- return;
-}
-module.exports = _set;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/tempdir.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/tempdir.js b/node_modules/shelljs/src/tempdir.js
index 79b949f..45953c2 100644
--- a/node_modules/shelljs/src/tempdir.js
+++ b/node_modules/shelljs/src/tempdir.js
@@ -37,8 +37,7 @@ function _tempDir() {
if (state.tempDir)
return state.tempDir; // from cache
- state.tempDir = writeableDir(os.tmpdir && os.tmpdir()) || // node 0.10+
- writeableDir(os.tmpDir && os.tmpDir()) || // node 0.8+
+ state.tempDir = writeableDir(os.tempDir && os.tempDir()) || // node 0.8+
writeableDir(process.env['TMPDIR']) ||
writeableDir(process.env['TEMP']) ||
writeableDir(process.env['TMP']) ||
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/test.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/test.js b/node_modules/shelljs/src/test.js
index 068a1ce..8a4ac7d 100644
--- a/node_modules/shelljs/src/test.js
+++ b/node_modules/shelljs/src/test.js
@@ -10,7 +10,7 @@ var fs = require('fs');
//@ + `'-d', 'path'`: true if path is a directory
//@ + `'-e', 'path'`: true if path exists
//@ + `'-f', 'path'`: true if path is a regular file
-//@ + `'-L', 'path'`: true if path is a symbolic link
+//@ + `'-L', 'path'`: true if path is a symboilc link
//@ + `'-p', 'path'`: true if path is a pipe (FIFO)
//@ + `'-S', 'path'`: true if path is a socket
//@
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/to.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/to.js b/node_modules/shelljs/src/to.js
index 65d6d54..f029999 100644
--- a/node_modules/shelljs/src/to.js
+++ b/node_modules/shelljs/src/to.js
@@ -22,7 +22,6 @@ function _to(options, file) {
try {
fs.writeFileSync(file, this.toString(), 'utf8');
- return this;
} catch(e) {
common.error('could not write to file (code '+e.code+'): '+file, true);
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/toEnd.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/toEnd.js b/node_modules/shelljs/src/toEnd.js
index bf29a65..f6d099d 100644
--- a/node_modules/shelljs/src/toEnd.js
+++ b/node_modules/shelljs/src/toEnd.js
@@ -22,7 +22,6 @@ function _toEnd(options, file) {
try {
fs.appendFileSync(file, this.toString(), 'utf8');
- return this;
} catch(e) {
common.error('could not append to file (code '+e.code+'): '+file, true);
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/touch.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/touch.js b/node_modules/shelljs/src/touch.js
deleted file mode 100644
index bbc2c19..0000000
--- a/node_modules/shelljs/src/touch.js
+++ /dev/null
@@ -1,109 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### touch([options,] file)
-//@ Available options:
-//@
-//@ + `-a`: Change only the access time
-//@ + `-c`: Do not create any files
-//@ + `-m`: Change only the modification time
-//@ + `-d DATE`: Parse DATE and use it instead of current time
-//@ + `-r FILE`: Use FILE's times instead of current time
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ touch('source.js');
-//@ touch('-c', '/path/to/some/dir/source.js');
-//@ touch({ '-r': FILE }, '/path/to/some/dir/source.js');
-//@ ```
-//@
-//@ Update the access and modification times of each FILE to the current time.
-//@ A FILE argument that does not exist is created empty, unless -c is supplied.
-//@ This is a partial implementation of *[touch(1)](http://linux.die.net/man/1/touch)*.
-function _touch(opts, files) {
- opts = common.parseOptions(opts, {
- 'a': 'atime_only',
- 'c': 'no_create',
- 'd': 'date',
- 'm': 'mtime_only',
- 'r': 'reference',
- });
-
- if (!files) {
- common.error('no paths given');
- }
-
- if (Array.isArray(files)) {
- files.forEach(function(f) {
- touchFile(opts, f);
- });
- } else if (typeof files === 'string') {
- touchFile(opts, files);
- } else {
- common.error('file arg should be a string file path or an Array of string file paths');
- }
-
-}
-
-function touchFile(opts, file) {
- var stat = tryStatFile(file);
-
- if (stat && stat.isDirectory()) {
- // don't error just exit
- return;
- }
-
- // if the file doesn't already exist and the user has specified --no-create then
- // this script is finished
- if (!stat && opts.no_create) {
- return;
- }
-
- // open the file and then close it. this will create it if it doesn't exist but will
- // not truncate the file
- fs.closeSync(fs.openSync(file, 'a'));
-
- //
- // Set timestamps
- //
-
- // setup some defaults
- var now = new Date();
- var mtime = opts.date || now;
- var atime = opts.date || now;
-
- // use reference file
- if (opts.reference) {
- var refStat = tryStatFile(opts.reference);
- if (!refStat) {
- common.error('failed to get attributess of ' + opts.reference);
- }
- mtime = refStat.mtime;
- atime = refStat.atime;
- } else if (opts.date) {
- mtime = opts.date;
- atime = opts.date;
- }
-
- if (opts.atime_only && opts.mtime_only) {
- // keep the new values of mtime and atime like GNU
- } else if (opts.atime_only) {
- mtime = stat.mtime;
- } else if (opts.mtime_only) {
- atime = stat.atime;
- }
-
- fs.utimesSync(file, atime, mtime);
-}
-
-module.exports = _touch;
-
-function tryStatFile(filePath) {
- try {
- return fs.statSync(filePath);
- } catch (e) {
- return null;
- }
-}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/src/which.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/src/which.js b/node_modules/shelljs/src/which.js
index d17634e..2822ecf 100644
--- a/node_modules/shelljs/src/which.js
+++ b/node_modules/shelljs/src/which.js
@@ -2,12 +2,10 @@ var common = require('./common');
var fs = require('fs');
var path = require('path');
-// XP's system default value for PATHEXT system variable, just in case it's not
-// set on Windows.
-var XP_DEFAULT_PATHEXT = '.com;.exe;.bat;.cmd;.vbs;.vbe;.js;.jse;.wsf;.wsh';
-
// Cross-platform method for splitting environment PATH variables
function splitPath(p) {
+ for (i=1;i<2;i++) {}
+
if (!p)
return [];
@@ -18,7 +16,7 @@ function splitPath(p) {
}
function checkPath(path) {
- return fs.existsSync(path) && !fs.statSync(path).isDirectory();
+ return fs.existsSync(path) && fs.statSync(path).isDirectory() == false;
}
//@
@@ -30,8 +28,7 @@ function checkPath(path) {
//@ var nodeExec = which('node');
//@ ```
//@
-//@ Searches for `command` in the system's PATH. On Windows, this uses the
-//@ `PATHEXT` variable to append the extension if it's not already executable.
+//@ Searches for `command` in the system's PATH. On Windows looks for `.exe`, `.cmd`, and `.bat` extensions.
//@ Returns string containing the absolute path to the command.
function _which(options, cmd) {
if (!cmd)
@@ -48,42 +45,30 @@ function _which(options, cmd) {
if (where)
return; // already found it
- var attempt = path.resolve(dir, cmd);
+ var attempt = path.resolve(dir + '/' + cmd);
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
if (common.platform === 'win') {
- attempt = attempt.toUpperCase();
-
- // In case the PATHEXT variable is somehow not set (e.g.
- // child_process.spawn with an empty environment), use the XP default.
- var pathExtEnv = process.env.PATHEXT || XP_DEFAULT_PATHEXT;
- var pathExtArray = splitPath(pathExtEnv.toUpperCase());
- var i;
-
- // If the extension is already in PATHEXT, just return that.
- for (i = 0; i < pathExtArray.length; i++) {
- var ext = pathExtArray[i];
- if (attempt.slice(-ext.length) === ext && checkPath(attempt)) {
- where = attempt;
- return;
- }
- }
-
- // Cycle through the PATHEXT variable
var baseAttempt = attempt;
- for (i = 0; i < pathExtArray.length; i++) {
- attempt = baseAttempt + pathExtArray[i];
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
+ attempt = baseAttempt + '.exe';
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
}
- } else {
- // Assume it's Unix-like
+ attempt = baseAttempt + '.cmd';
if (checkPath(attempt)) {
where = attempt;
return;
}
- }
+ attempt = baseAttempt + '.bat';
+ if (checkPath(attempt)) {
+ where = attempt;
+ return;
+ }
+ } // if 'win'
});
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/underscore/package.json
----------------------------------------------------------------------
diff --git a/node_modules/underscore/package.json b/node_modules/underscore/package.json
index 101d9c3..da48a67 100644
--- a/node_modules/underscore/package.json
+++ b/node_modules/underscore/package.json
@@ -26,7 +26,8 @@
},
"_requiredBy": [
"/",
- "/cordova-common"
+ "/cordova-common",
+ "/jasmine-node"
],
"_resolved": "http://registry.npmjs.org/underscore/-/underscore-1.8.3.tgz",
"_shasum": "4f3fb53b106e6097fcf9cb4109f2a5e9bdfa5022",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/util-deprecate/package.json
----------------------------------------------------------------------
diff --git a/node_modules/util-deprecate/package.json b/node_modules/util-deprecate/package.json
index 0990ecc..1d299c9 100644
--- a/node_modules/util-deprecate/package.json
+++ b/node_modules/util-deprecate/package.json
@@ -26,7 +26,10 @@
"type": "version"
},
"_requiredBy": [
- "/plist"
+ "/coveralls/readable-stream",
+ "/plist",
+ "/tap-parser/readable-stream",
+ "/tap/readable-stream"
],
"_resolved": "http://registry.npmjs.org/util-deprecate/-/util-deprecate-1.0.2.tgz",
"_shasum": "450d4dc9fa70de732762fbd2d4a28981419a0ccf",
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/package.json
----------------------------------------------------------------------
diff --git a/package.json b/package.json
index d686e51..b619150 100644
--- a/package.json
+++ b/package.json
@@ -18,7 +18,7 @@
"test": "npm run jshint && npm run jasmine",
"jasmine": "npm run objc-tests && npm run jasmine-tests",
"objc-tests": "jasmine-node --captureExceptions --color tests/spec/cordovalib.spec.js",
- "jasmine-tests": "jasmine-node --captureExceptions --color tests/spec/create.spec.js",
+ "jasmine-tests": "jasmine-node --captureExceptions --color tests/spec/create.spec.js tests/spec/platform.spec.js",
"jshint": "node node_modules/jshint/bin/jshint bin && node node_modules/jshint/bin/jshint tests"
},
"author": "Apache Software Foundation",
@@ -27,7 +27,7 @@
"cordova-common": "^1.0.0",
"nopt": "^3.0.6",
"q": "^1.4.1",
- "shelljs": "^0.6.0",
+ "shelljs": "^0.5.3",
"underscore": "^1.8.3",
"unorm": "^1.4.1",
"xcode": "^0.8.3"
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[34/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isNumber.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isNumber.js b/node_modules/lodash-node/modern/objects/isNumber.js
new file mode 100644
index 0000000..7188d0d
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isNumber.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var numberClass = '[object Number]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a number.
+ *
+ * Note: `NaN` is considered a number. See http://es5.github.io/#x8.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(8.4 * 5);
+ * // => true
+ */
+function isNumber(value) {
+ return typeof value == 'number' ||
+ value && typeof value == 'object' && toString.call(value) == numberClass || false;
+}
+
+module.exports = isNumber;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isObject.js b/node_modules/lodash-node/modern/objects/isObject.js
new file mode 100644
index 0000000..4a140a6
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isObject.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var objectTypes = require('../internals/objectTypes');
+
+/**
+ * Checks if `value` is the language type of Object.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // check if the value is the ECMAScript language type of Object
+ // http://es5.github.io/#x8
+ // and avoid a V8 bug
+ // http://code.google.com/p/v8/issues/detail?id=2291
+ return !!(value && objectTypes[typeof value]);
+}
+
+module.exports = isObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isPlainObject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isPlainObject.js b/node_modules/lodash-node/modern/objects/isPlainObject.js
new file mode 100644
index 0000000..629a958
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isPlainObject.js
@@ -0,0 +1,60 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative'),
+ shimIsPlainObject = require('../internals/shimIsPlainObject');
+
+/** `Object#toString` result shortcuts */
+var objectClass = '[object Object]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var getPrototypeOf = isNative(getPrototypeOf = Object.getPrototypeOf) && getPrototypeOf;
+
+/**
+ * Checks if `value` is an object created by the `Object` constructor.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Shape() {
+ * this.x = 0;
+ * this.y = 0;
+ * }
+ *
+ * _.isPlainObject(new Shape);
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'x': 0, 'y': 0 });
+ * // => true
+ */
+var isPlainObject = !getPrototypeOf ? shimIsPlainObject : function(value) {
+ if (!(value && toString.call(value) == objectClass)) {
+ return false;
+ }
+ var valueOf = value.valueOf,
+ objProto = isNative(valueOf) && (objProto = getPrototypeOf(valueOf)) && getPrototypeOf(objProto);
+
+ return objProto
+ ? (value == objProto || getPrototypeOf(value) == objProto)
+ : shimIsPlainObject(value);
+};
+
+module.exports = isPlainObject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isRegExp.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isRegExp.js b/node_modules/lodash-node/modern/objects/isRegExp.js
new file mode 100644
index 0000000..6e50c7a
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isRegExp.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var regexpClass = '[object RegExp]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a regular expression.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a regular expression, else `false`.
+ * @example
+ *
+ * _.isRegExp(/fred/);
+ * // => true
+ */
+function isRegExp(value) {
+ return value && typeof value == 'object' && toString.call(value) == regexpClass || false;
+}
+
+module.exports = isRegExp;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isString.js b/node_modules/lodash-node/modern/objects/isString.js
new file mode 100644
index 0000000..2c1527a
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isString.js
@@ -0,0 +1,37 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** `Object#toString` result shortcuts */
+var stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/**
+ * Checks if `value` is a string.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('fred');
+ * // => true
+ */
+function isString(value) {
+ return typeof value == 'string' ||
+ value && typeof value == 'object' && toString.call(value) == stringClass || false;
+}
+
+module.exports = isString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/isUndefined.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/isUndefined.js b/node_modules/lodash-node/modern/objects/isUndefined.js
new file mode 100644
index 0000000..4e75280
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/isUndefined.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if the `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ */
+function isUndefined(value) {
+ return typeof value == 'undefined';
+}
+
+module.exports = isUndefined;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/keys.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/keys.js b/node_modules/lodash-node/modern/objects/keys.js
new file mode 100644
index 0000000..163e03d
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/keys.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative'),
+ isObject = require('./isObject'),
+ shimKeys = require('../internals/shimKeys');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeKeys = isNative(nativeKeys = Object.keys) && nativeKeys;
+
+/**
+ * Creates an array composed of the own enumerable property names of an object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property names.
+ * @example
+ *
+ * _.keys({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => ['one', 'two', 'three'] (property order is not guaranteed across environments)
+ */
+var keys = !nativeKeys ? shimKeys : function(object) {
+ if (!isObject(object)) {
+ return [];
+ }
+ return nativeKeys(object);
+};
+
+module.exports = keys;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/mapValues.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/mapValues.js b/node_modules/lodash-node/modern/objects/mapValues.js
new file mode 100644
index 0000000..9e797da
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/mapValues.js
@@ -0,0 +1,58 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('./forOwn');
+
+/**
+ * Creates an object with the same keys as `object` and values generated by
+ * running each own enumerable property of `object` through the callback.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new object with values of the results of each `callback` execution.
+ * @example
+ *
+ * _.mapValues({ 'a': 1, 'b': 2, 'c': 3} , function(num) { return num * 3; });
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ *
+ * var characters = {
+ * 'fred': { 'name': 'fred', 'age': 40 },
+ * 'pebbles': { 'name': 'pebbles', 'age': 1 }
+ * };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.mapValues(characters, 'age');
+ * // => { 'fred': 40, 'pebbles': 1 }
+ */
+function mapValues(object, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg, 3);
+
+ forOwn(object, function(value, key, object) {
+ result[key] = callback(value, key, object);
+ });
+ return result;
+}
+
+module.exports = mapValues;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/merge.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/merge.js b/node_modules/lodash-node/modern/objects/merge.js
new file mode 100644
index 0000000..d4bddc3
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/merge.js
@@ -0,0 +1,97 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseMerge = require('../internals/baseMerge'),
+ getArray = require('../internals/getArray'),
+ isObject = require('./isObject'),
+ releaseArray = require('../internals/releaseArray'),
+ slice = require('../internals/slice');
+
+/**
+ * Recursively merges own enumerable properties of the source object(s), that
+ * don't resolve to `undefined` into the destination object. Subsequent sources
+ * will overwrite property assignments of previous sources. If a callback is
+ * provided it will be executed to produce the merged values of the destination
+ * and source properties. If the callback returns `undefined` merging will
+ * be handled by the method instead. The callback is bound to `thisArg` and
+ * invoked with two arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {...Object} [source] The source objects.
+ * @param {Function} [callback] The function to customize merging properties.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var names = {
+ * 'characters': [
+ * { 'name': 'barney' },
+ * { 'name': 'fred' }
+ * ]
+ * };
+ *
+ * var ages = {
+ * 'characters': [
+ * { 'age': 36 },
+ * { 'age': 40 }
+ * ]
+ * };
+ *
+ * _.merge(names, ages);
+ * // => { 'characters': [{ 'name': 'barney', 'age': 36 }, { 'name': 'fred', 'age': 40 }] }
+ *
+ * var food = {
+ * 'fruits': ['apple'],
+ * 'vegetables': ['beet']
+ * };
+ *
+ * var otherFood = {
+ * 'fruits': ['banana'],
+ * 'vegetables': ['carrot']
+ * };
+ *
+ * _.merge(food, otherFood, function(a, b) {
+ * return _.isArray(a) ? a.concat(b) : undefined;
+ * });
+ * // => { 'fruits': ['apple', 'banana'], 'vegetables': ['beet', 'carrot] }
+ */
+function merge(object) {
+ var args = arguments,
+ length = 2;
+
+ if (!isObject(object)) {
+ return object;
+ }
+ // allows working with `_.reduce` and `_.reduceRight` without using
+ // their `index` and `collection` arguments
+ if (typeof args[2] != 'number') {
+ length = args.length;
+ }
+ if (length > 3 && typeof args[length - 2] == 'function') {
+ var callback = baseCreateCallback(args[--length - 1], args[length--], 2);
+ } else if (length > 2 && typeof args[length - 1] == 'function') {
+ callback = args[--length];
+ }
+ var sources = slice(arguments, 1, length),
+ index = -1,
+ stackA = getArray(),
+ stackB = getArray();
+
+ while (++index < length) {
+ baseMerge(object, sources[index], callback, stackA, stackB);
+ }
+ releaseArray(stackA);
+ releaseArray(stackB);
+ return object;
+}
+
+module.exports = merge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/omit.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/omit.js b/node_modules/lodash-node/modern/objects/omit.js
new file mode 100644
index 0000000..759798d
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/omit.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseFlatten = require('../internals/baseFlatten'),
+ createCallback = require('../functions/createCallback'),
+ forIn = require('./forIn');
+
+/**
+ * Creates a shallow clone of `object` excluding the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a callback is provided it will be executed for each
+ * property of `object` omitting the properties the callback returns truey
+ * for. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|...string|string[]} [callback] The properties to omit or the
+ * function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object without the omitted properties.
+ * @example
+ *
+ * _.omit({ 'name': 'fred', 'age': 40 }, 'age');
+ * // => { 'name': 'fred' }
+ *
+ * _.omit({ 'name': 'fred', 'age': 40 }, function(value) {
+ * return typeof value == 'number';
+ * });
+ * // => { 'name': 'fred' }
+ */
+function omit(object, callback, thisArg) {
+ var result = {};
+ if (typeof callback != 'function') {
+ var props = [];
+ forIn(object, function(value, key) {
+ props.push(key);
+ });
+ props = baseDifference(props, baseFlatten(arguments, true, false, 1));
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ result[key] = object[key];
+ }
+ } else {
+ callback = createCallback(callback, thisArg, 3);
+ forIn(object, function(value, key, object) {
+ if (!callback(value, key, object)) {
+ result[key] = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = omit;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/pairs.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/pairs.js b/node_modules/lodash-node/modern/objects/pairs.js
new file mode 100644
index 0000000..e9e06a6
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/pairs.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates a two dimensional array of an object's key-value pairs,
+ * i.e. `[[key1, value1], [key2, value2]]`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns new array of key-value pairs.
+ * @example
+ *
+ * _.pairs({ 'barney': 36, 'fred': 40 });
+ * // => [['barney', 36], ['fred', 40]] (property order is not guaranteed across environments)
+ */
+function pairs(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ var key = props[index];
+ result[index] = [key, object[key]];
+ }
+ return result;
+}
+
+module.exports = pairs;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/pick.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/pick.js b/node_modules/lodash-node/modern/objects/pick.js
new file mode 100644
index 0000000..718a4b5
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/pick.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ createCallback = require('../functions/createCallback'),
+ forIn = require('./forIn'),
+ isObject = require('./isObject');
+
+/**
+ * Creates a shallow clone of `object` composed of the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a callback is provided it will be executed for each
+ * property of `object` picking the properties the callback returns truey
+ * for. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|...string|string[]} [callback] The function called per
+ * iteration or property names to pick, specified as individual property
+ * names or arrays of property names.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object composed of the picked properties.
+ * @example
+ *
+ * _.pick({ 'name': 'fred', '_userid': 'fred1' }, 'name');
+ * // => { 'name': 'fred' }
+ *
+ * _.pick({ 'name': 'fred', '_userid': 'fred1' }, function(value, key) {
+ * return key.charAt(0) != '_';
+ * });
+ * // => { 'name': 'fred' }
+ */
+function pick(object, callback, thisArg) {
+ var result = {};
+ if (typeof callback != 'function') {
+ var index = -1,
+ props = baseFlatten(arguments, true, false, 1),
+ length = isObject(object) ? props.length : 0;
+
+ while (++index < length) {
+ var key = props[index];
+ if (key in object) {
+ result[key] = object[key];
+ }
+ }
+ } else {
+ callback = createCallback(callback, thisArg, 3);
+ forIn(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result[key] = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = pick;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/transform.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/transform.js b/node_modules/lodash-node/modern/objects/transform.js
new file mode 100644
index 0000000..7c59ded
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/transform.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('../internals/baseCreate'),
+ createCallback = require('../functions/createCallback'),
+ forEach = require('../collections/forEach'),
+ forOwn = require('./forOwn'),
+ isArray = require('./isArray');
+
+/**
+ * An alternative to `_.reduce` this method transforms `object` to a new
+ * `accumulator` object which is the result of running each of its own
+ * enumerable properties through a callback, with each callback execution
+ * potentially mutating the `accumulator` object. The callback is bound to
+ * `thisArg` and invoked with four arguments; (accumulator, value, key, object).
+ * Callbacks may exit iteration early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Array|Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] The custom accumulator value.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var squares = _.transform([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], function(result, num) {
+ * num *= num;
+ * if (num % 2) {
+ * return result.push(num) < 3;
+ * }
+ * });
+ * // => [1, 9, 25]
+ *
+ * var mapped = _.transform({ 'a': 1, 'b': 2, 'c': 3 }, function(result, num, key) {
+ * result[key] = num * 3;
+ * });
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ */
+function transform(object, callback, accumulator, thisArg) {
+ var isArr = isArray(object);
+ if (accumulator == null) {
+ if (isArr) {
+ accumulator = [];
+ } else {
+ var ctor = object && object.constructor,
+ proto = ctor && ctor.prototype;
+
+ accumulator = baseCreate(proto);
+ }
+ }
+ if (callback) {
+ callback = createCallback(callback, thisArg, 4);
+ (isArr ? forEach : forOwn)(object, function(value, index, object) {
+ return callback(accumulator, value, index, object);
+ });
+ }
+ return accumulator;
+}
+
+module.exports = transform;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/objects/values.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/objects/values.js b/node_modules/lodash-node/modern/objects/values.js
new file mode 100644
index 0000000..ac9c3c1
--- /dev/null
+++ b/node_modules/lodash-node/modern/objects/values.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('./keys');
+
+/**
+ * Creates an array composed of the own enumerable property values of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns an array of property values.
+ * @example
+ *
+ * _.values({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => [1, 2, 3] (property order is not guaranteed across environments)
+ */
+function values(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = object[props[index]];
+ }
+ return result;
+}
+
+module.exports = values;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/support.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/support.js b/node_modules/lodash-node/modern/support.js
new file mode 100644
index 0000000..646a9ab
--- /dev/null
+++ b/node_modules/lodash-node/modern/support.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./internals/isNative');
+
+/** Used to detect functions containing a `this` reference */
+var reThis = /\bthis\b/;
+
+/**
+ * An object used to flag environments features.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var support = {};
+
+/**
+ * Detect if functions can be decompiled by `Function#toString`
+ * (all but PS3 and older Opera mobile browsers & avoided in Windows 8 apps).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+support.funcDecomp = !isNative(global.WinRTError) && reThis.test(function() { return this; });
+
+/**
+ * Detect if `Function#name` is supported (all but IE).
+ *
+ * @memberOf _.support
+ * @type boolean
+ */
+support.funcNames = typeof Function.name == 'string';
+
+module.exports = support;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities.js b/node_modules/lodash-node/modern/utilities.js
new file mode 100644
index 0000000..b686eee
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'constant': require('./utilities/constant'),
+ 'createCallback': require('./functions/createCallback'),
+ 'escape': require('./utilities/escape'),
+ 'identity': require('./utilities/identity'),
+ 'mixin': require('./utilities/mixin'),
+ 'noConflict': require('./utilities/noConflict'),
+ 'noop': require('./utilities/noop'),
+ 'now': require('./utilities/now'),
+ 'parseInt': require('./utilities/parseInt'),
+ 'property': require('./utilities/property'),
+ 'random': require('./utilities/random'),
+ 'result': require('./utilities/result'),
+ 'template': require('./utilities/template'),
+ 'templateSettings': require('./utilities/templateSettings'),
+ 'times': require('./utilities/times'),
+ 'unescape': require('./utilities/unescape'),
+ 'uniqueId': require('./utilities/uniqueId')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/constant.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/constant.js b/node_modules/lodash-node/modern/utilities/constant.js
new file mode 100644
index 0000000..8e45e85
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/constant.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates a function that returns `value`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} value The value to return from the new function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * var getter = _.constant(object);
+ * getter() === object;
+ * // => true
+ */
+function constant(value) {
+ return function() {
+ return value;
+ };
+}
+
+module.exports = constant;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/escape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/escape.js b/node_modules/lodash-node/modern/utilities/escape.js
new file mode 100644
index 0000000..7f76531
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/escape.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var escapeHtmlChar = require('../internals/escapeHtmlChar'),
+ keys = require('../objects/keys'),
+ reUnescapedHtml = require('../internals/reUnescapedHtml');
+
+/**
+ * Converts the characters `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding HTML entities.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} string The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('Fred, Wilma, & Pebbles');
+ * // => 'Fred, Wilma, & Pebbles'
+ */
+function escape(string) {
+ return string == null ? '' : String(string).replace(reUnescapedHtml, escapeHtmlChar);
+}
+
+module.exports = escape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/identity.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/identity.js b/node_modules/lodash-node/modern/utilities/identity.js
new file mode 100644
index 0000000..0af8ec0
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/identity.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * This method returns the first argument provided to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * _.identity(object) === object;
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+module.exports = identity;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/mixin.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/mixin.js b/node_modules/lodash-node/modern/utilities/mixin.js
new file mode 100644
index 0000000..dd08b7d
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/mixin.js
@@ -0,0 +1,88 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ functions = require('../objects/functions'),
+ isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * Adds function properties of a source object to the destination object.
+ * If `object` is a function methods will be added to its prototype as well.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Function|Object} [object=lodash] object The destination object.
+ * @param {Object} source The object of functions to add.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.chain=true] Specify whether the functions added are chainable.
+ * @example
+ *
+ * function capitalize(string) {
+ * return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase();
+ * }
+ *
+ * _.mixin({ 'capitalize': capitalize });
+ * _.capitalize('fred');
+ * // => 'Fred'
+ *
+ * _('fred').capitalize().value();
+ * // => 'Fred'
+ *
+ * _.mixin({ 'capitalize': capitalize }, { 'chain': false });
+ * _('fred').capitalize();
+ * // => 'Fred'
+ */
+function mixin(object, source, options) {
+ var chain = true,
+ methodNames = source && functions(source);
+
+ if (options === false) {
+ chain = false;
+ } else if (isObject(options) && 'chain' in options) {
+ chain = options.chain;
+ }
+ var ctor = object,
+ isFunc = isFunction(ctor);
+
+ forEach(methodNames, function(methodName) {
+ var func = object[methodName] = source[methodName];
+ if (isFunc) {
+ ctor.prototype[methodName] = function() {
+ var chainAll = this.__chain__,
+ value = this.__wrapped__,
+ args = [value];
+
+ push.apply(args, arguments);
+ var result = func.apply(object, args);
+ if (chain || chainAll) {
+ if (value === result && isObject(result)) {
+ return this;
+ }
+ result = new ctor(result);
+ result.__chain__ = chainAll;
+ }
+ return result;
+ };
+ }
+ });
+}
+
+module.exports = mixin;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/noConflict.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/noConflict.js b/node_modules/lodash-node/modern/utilities/noConflict.js
new file mode 100644
index 0000000..306ee81
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/noConflict.js
@@ -0,0 +1,30 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to restore the original `_` reference in `noConflict` */
+var oldDash = global._;
+
+/**
+ * Reverts the '_' variable to its previous value and returns a reference to
+ * the `lodash` function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @returns {Function} Returns the `lodash` function.
+ * @example
+ *
+ * var lodash = _.noConflict();
+ */
+function noConflict() {
+ global._ = oldDash;
+ return this;
+}
+
+module.exports = noConflict;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/noop.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/noop.js b/node_modules/lodash-node/modern/utilities/noop.js
new file mode 100644
index 0000000..f6a7a7a
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/noop.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * A no-operation function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @example
+ *
+ * var object = { 'name': 'fred' };
+ * _.noop(object) === undefined;
+ * // => true
+ */
+function noop() {
+ // no operation performed
+}
+
+module.exports = noop;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/now.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/now.js b/node_modules/lodash-node/modern/utilities/now.js
new file mode 100644
index 0000000..09a9928
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/now.js
@@ -0,0 +1,28 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('../internals/isNative');
+
+/**
+ * Gets the number of milliseconds that have elapsed since the Unix epoch
+ * (1 January 1970 00:00:00 UTC).
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @example
+ *
+ * var stamp = _.now();
+ * _.defer(function() { console.log(_.now() - stamp); });
+ * // => logs the number of milliseconds it took for the deferred function to be called
+ */
+var now = isNative(now = Date.now) && now || function() {
+ return new Date().getTime();
+};
+
+module.exports = now;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/parseInt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/parseInt.js b/node_modules/lodash-node/modern/utilities/parseInt.js
new file mode 100644
index 0000000..6462865
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/parseInt.js
@@ -0,0 +1,53 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isString = require('../objects/isString');
+
+/** Used to detect and test whitespace */
+var whitespace = (
+ // whitespace
+ ' \t\x0B\f\xA0\ufeff' +
+
+ // line terminators
+ '\n\r\u2028\u2029' +
+
+ // unicode category "Zs" space separators
+ '\u1680\u180e\u2000\u2001\u2002\u2003\u2004\u2005\u2006\u2007\u2008\u2009\u200a\u202f\u205f\u3000'
+);
+
+/** Used to match leading whitespace and zeros to be removed */
+var reLeadingSpacesAndZeros = RegExp('^[' + whitespace + ']*0+(?=.$)');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeParseInt = global.parseInt;
+
+/**
+ * Converts the given value into an integer of the specified radix.
+ * If `radix` is `undefined` or `0` a `radix` of `10` is used unless the
+ * `value` is a hexadecimal, in which case a `radix` of `16` is used.
+ *
+ * Note: This method avoids differences in native ES3 and ES5 `parseInt`
+ * implementations. See http://es5.github.io/#E.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} value The value to parse.
+ * @param {number} [radix] The radix used to interpret the value to parse.
+ * @returns {number} Returns the new integer value.
+ * @example
+ *
+ * _.parseInt('08');
+ * // => 8
+ */
+var parseInt = nativeParseInt(whitespace + '08') == 8 ? nativeParseInt : function(value, radix) {
+ // Firefox < 21 and Opera < 15 follow the ES3 specified implementation of `parseInt`
+ return nativeParseInt(isString(value) ? value.replace(reLeadingSpacesAndZeros, '') : value, radix || 0);
+};
+
+module.exports = parseInt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/property.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/property.js b/node_modules/lodash-node/modern/utilities/property.js
new file mode 100644
index 0000000..c9a735c
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/property.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates a "_.pluck" style function, which returns the `key` value of a
+ * given object.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} key The name of the property to retrieve.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'barney', 'age': 36 }
+ * ];
+ *
+ * var getName = _.property('name');
+ *
+ * _.map(characters, getName);
+ * // => ['barney', 'fred']
+ *
+ * _.sortBy(characters, getName);
+ * // => [{ 'name': 'barney', 'age': 36 }, { 'name': 'fred', 'age': 40 }]
+ */
+function property(key) {
+ return function(object) {
+ return object[key];
+ };
+}
+
+module.exports = property;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/random.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/random.js b/node_modules/lodash-node/modern/utilities/random.js
new file mode 100644
index 0000000..cbf8ef2
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/random.js
@@ -0,0 +1,73 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMin = Math.min,
+ nativeRandom = Math.random;
+
+/**
+ * Produces a random number between `min` and `max` (inclusive). If only one
+ * argument is provided a number between `0` and the given number will be
+ * returned. If `floating` is truey or either `min` or `max` are floats a
+ * floating-point number will be returned instead of an integer.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {number} [min=0] The minimum possible value.
+ * @param {number} [max=1] The maximum possible value.
+ * @param {boolean} [floating=false] Specify returning a floating-point number.
+ * @returns {number} Returns a random number.
+ * @example
+ *
+ * _.random(0, 5);
+ * // => an integer between 0 and 5
+ *
+ * _.random(5);
+ * // => also an integer between 0 and 5
+ *
+ * _.random(5, true);
+ * // => a floating-point number between 0 and 5
+ *
+ * _.random(1.2, 5.2);
+ * // => a floating-point number between 1.2 and 5.2
+ */
+function random(min, max, floating) {
+ var noMin = min == null,
+ noMax = max == null;
+
+ if (floating == null) {
+ if (typeof min == 'boolean' && noMax) {
+ floating = min;
+ min = 1;
+ }
+ else if (!noMax && typeof max == 'boolean') {
+ floating = max;
+ noMax = true;
+ }
+ }
+ if (noMin && noMax) {
+ max = 1;
+ }
+ min = +min || 0;
+ if (noMax) {
+ max = min;
+ min = 0;
+ } else {
+ max = +max || 0;
+ }
+ if (floating || min % 1 || max % 1) {
+ var rand = nativeRandom();
+ return nativeMin(min + (rand * (max - min + parseFloat('1e-' + ((rand +'').length - 1)))), max);
+ }
+ return baseRandom(min, max);
+}
+
+module.exports = random;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/result.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/result.js b/node_modules/lodash-node/modern/utilities/result.js
new file mode 100644
index 0000000..cffcb50
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/result.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Resolves the value of property `key` on `object`. If `key` is a function
+ * it will be invoked with the `this` binding of `object` and its result returned,
+ * else the property value is returned. If `object` is falsey then `undefined`
+ * is returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Object} object The object to inspect.
+ * @param {string} key The name of the property to resolve.
+ * @returns {*} Returns the resolved value.
+ * @example
+ *
+ * var object = {
+ * 'cheese': 'crumpets',
+ * 'stuff': function() {
+ * return 'nonsense';
+ * }
+ * };
+ *
+ * _.result(object, 'cheese');
+ * // => 'crumpets'
+ *
+ * _.result(object, 'stuff');
+ * // => 'nonsense'
+ */
+function result(object, key) {
+ if (object) {
+ var value = object[key];
+ return isFunction(value) ? object[key]() : value;
+ }
+}
+
+module.exports = result;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/template.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/template.js b/node_modules/lodash-node/modern/utilities/template.js
new file mode 100644
index 0000000..85ab79d
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/template.js
@@ -0,0 +1,216 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var defaults = require('../objects/defaults'),
+ escape = require('./escape'),
+ escapeStringChar = require('../internals/escapeStringChar'),
+ keys = require('../objects/keys'),
+ reInterpolate = require('../internals/reInterpolate'),
+ templateSettings = require('./templateSettings'),
+ values = require('../objects/values');
+
+/** Used to match empty string literals in compiled template source */
+var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+/**
+ * Used to match ES6 template delimiters
+ * http://people.mozilla.org/~jorendorff/es6-draft.html#sec-literals-string-literals
+ */
+var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+/** Used to ensure capturing order of template delimiters */
+var reNoMatch = /($^)/;
+
+/** Used to match unescaped characters in compiled string literals */
+var reUnescapedString = /['\n\r\t\u2028\u2029\\]/g;
+
+/**
+ * A micro-templating method that handles arbitrary delimiters, preserves
+ * whitespace, and correctly escapes quotes within interpolated code.
+ *
+ * Note: In the development build, `_.template` utilizes sourceURLs for easier
+ * debugging. See http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl
+ *
+ * For more information on precompiling templates see:
+ * http://lodash.com/custom-builds
+ *
+ * For more information on Chrome extension sandboxes see:
+ * http://developer.chrome.com/stable/extensions/sandboxingEval.html
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} text The template text.
+ * @param {Object} data The data object used to populate the text.
+ * @param {Object} [options] The options object.
+ * @param {RegExp} [options.escape] The "escape" delimiter.
+ * @param {RegExp} [options.evaluate] The "evaluate" delimiter.
+ * @param {Object} [options.imports] An object to import into the template as local variables.
+ * @param {RegExp} [options.interpolate] The "interpolate" delimiter.
+ * @param {string} [sourceURL] The sourceURL of the template's compiled source.
+ * @param {string} [variable] The data object variable name.
+ * @returns {Function|string} Returns a compiled function when no `data` object
+ * is given, else it returns the interpolated text.
+ * @example
+ *
+ * // using the "interpolate" delimiter to create a compiled template
+ * var compiled = _.template('hello <%= name %>');
+ * compiled({ 'name': 'fred' });
+ * // => 'hello fred'
+ *
+ * // using the "escape" delimiter to escape HTML in data property values
+ * _.template('<b><%- value %></b>', { 'value': '<script>' });
+ * // => '<b><script></b>'
+ *
+ * // using the "evaluate" delimiter to generate HTML
+ * var list = '<% _.forEach(people, function(name) { %><li><%- name %></li><% }); %>';
+ * _.template(list, { 'people': ['fred', 'barney'] });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the ES6 delimiter as an alternative to the default "interpolate" delimiter
+ * _.template('hello ${ name }', { 'name': 'pebbles' });
+ * // => 'hello pebbles'
+ *
+ * // using the internal `print` function in "evaluate" delimiters
+ * _.template('<% print("hello " + name); %>!', { 'name': 'barney' });
+ * // => 'hello barney!'
+ *
+ * // using a custom template delimiters
+ * _.templateSettings = {
+ * 'interpolate': /{{([\s\S]+?)}}/g
+ * };
+ *
+ * _.template('hello {{ name }}!', { 'name': 'mustache' });
+ * // => 'hello mustache!'
+ *
+ * // using the `imports` option to import jQuery
+ * var list = '<% jq.each(people, function(name) { %><li><%- name %></li><% }); %>';
+ * _.template(list, { 'people': ['fred', 'barney'] }, { 'imports': { 'jq': jQuery } });
+ * // => '<li>fred</li><li>barney</li>'
+ *
+ * // using the `sourceURL` option to specify a custom sourceURL for the template
+ * var compiled = _.template('hello <%= name %>', null, { 'sourceURL': '/basic/greeting.jst' });
+ * compiled(data);
+ * // => find the source of "greeting.jst" under the Sources tab or Resources panel of the web inspector
+ *
+ * // using the `variable` option to ensure a with-statement isn't used in the compiled template
+ * var compiled = _.template('hi <%= data.name %>!', null, { 'variable': 'data' });
+ * compiled.source;
+ * // => function(data) {
+ * var __t, __p = '', __e = _.escape;
+ * __p += 'hi ' + ((__t = ( data.name )) == null ? '' : __t) + '!';
+ * return __p;
+ * }
+ *
+ * // using the `source` property to inline compiled templates for meaningful
+ * // line numbers in error messages and a stack trace
+ * fs.writeFileSync(path.join(cwd, 'jst.js'), '\
+ * var JST = {\
+ * "main": ' + _.template(mainText).source + '\
+ * };\
+ * ');
+ */
+function template(text, data, options) {
+ // based on John Resig's `tmpl` implementation
+ // http://ejohn.org/blog/javascript-micro-templating/
+ // and Laura Doktorova's doT.js
+ // https://github.com/olado/doT
+ var settings = templateSettings.imports._.templateSettings || templateSettings;
+ text = String(text || '');
+
+ // avoid missing dependencies when `iteratorTemplate` is not defined
+ options = defaults({}, options, settings);
+
+ var imports = defaults({}, options.imports, settings.imports),
+ importsKeys = keys(imports),
+ importsValues = values(imports);
+
+ var isEvaluating,
+ index = 0,
+ interpolate = options.interpolate || reNoMatch,
+ source = "__p += '";
+
+ // compile the regexp to match each delimiter
+ var reDelimiters = RegExp(
+ (options.escape || reNoMatch).source + '|' +
+ interpolate.source + '|' +
+ (interpolate === reInterpolate ? reEsTemplate : reNoMatch).source + '|' +
+ (options.evaluate || reNoMatch).source + '|$'
+ , 'g');
+
+ text.replace(reDelimiters, function(match, escapeValue, interpolateValue, esTemplateValue, evaluateValue, offset) {
+ interpolateValue || (interpolateValue = esTemplateValue);
+
+ // escape characters that cannot be included in string literals
+ source += text.slice(index, offset).replace(reUnescapedString, escapeStringChar);
+
+ // replace delimiters with snippets
+ if (escapeValue) {
+ source += "' +\n__e(" + escapeValue + ") +\n'";
+ }
+ if (evaluateValue) {
+ isEvaluating = true;
+ source += "';\n" + evaluateValue + ";\n__p += '";
+ }
+ if (interpolateValue) {
+ source += "' +\n((__t = (" + interpolateValue + ")) == null ? '' : __t) +\n'";
+ }
+ index = offset + match.length;
+
+ // the JS engine embedded in Adobe products requires returning the `match`
+ // string in order to produce the correct `offset` value
+ return match;
+ });
+
+ source += "';\n";
+
+ // if `variable` is not specified, wrap a with-statement around the generated
+ // code to add the data object to the top of the scope chain
+ var variable = options.variable,
+ hasVariable = variable;
+
+ if (!hasVariable) {
+ variable = 'obj';
+ source = 'with (' + variable + ') {\n' + source + '\n}\n';
+ }
+ // cleanup code by stripping empty strings
+ source = (isEvaluating ? source.replace(reEmptyStringLeading, '') : source)
+ .replace(reEmptyStringMiddle, '$1')
+ .replace(reEmptyStringTrailing, '$1;');
+
+ // frame code as the function body
+ source = 'function(' + variable + ') {\n' +
+ (hasVariable ? '' : variable + ' || (' + variable + ' = {});\n') +
+ "var __t, __p = '', __e = _.escape" +
+ (isEvaluating
+ ? ', __j = Array.prototype.join;\n' +
+ "function print() { __p += __j.call(arguments, '') }\n"
+ : ';\n'
+ ) +
+ source +
+ 'return __p\n}';
+
+ try {
+ var result = Function(importsKeys, 'return ' + source ).apply(undefined, importsValues);
+ } catch(e) {
+ e.source = source;
+ throw e;
+ }
+ if (data) {
+ return result(data);
+ }
+ // provide the compiled function's source by its `toString` method, in
+ // supported environments, or the `source` property as a convenience for
+ // inlining compiled templates during the build process
+ result.source = source;
+ return result;
+}
+
+module.exports = template;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/templateSettings.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/templateSettings.js b/node_modules/lodash-node/modern/utilities/templateSettings.js
new file mode 100644
index 0000000..c4bbb05
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/templateSettings.js
@@ -0,0 +1,73 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var escape = require('./escape'),
+ reInterpolate = require('../internals/reInterpolate');
+
+/**
+ * By default, the template delimiters used by Lo-Dash are similar to those in
+ * embedded Ruby (ERB). Change the following template settings to use alternative
+ * delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+var templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'escape': /<%-([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'evaluate': /<%([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type string
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type Object
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type Function
+ */
+ '_': { 'escape': escape }
+ }
+};
+
+module.exports = templateSettings;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/times.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/times.js b/node_modules/lodash-node/modern/utilities/times.js
new file mode 100644
index 0000000..8ac64ff
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/times.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback');
+
+/**
+ * Executes the callback `n` times, returning an array of the results
+ * of each callback execution. The callback is bound to `thisArg` and invoked
+ * with one argument; (index).
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {number} n The number of times to execute the callback.
+ * @param {Function} callback The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns an array of the results of each `callback` execution.
+ * @example
+ *
+ * var diceRolls = _.times(3, _.partial(_.random, 1, 6));
+ * // => [3, 6, 4]
+ *
+ * _.times(3, function(n) { mage.castSpell(n); });
+ * // => calls `mage.castSpell(n)` three times, passing `n` of `0`, `1`, and `2` respectively
+ *
+ * _.times(3, function(n) { this.cast(n); }, mage);
+ * // => also calls `mage.castSpell(n)` three times
+ */
+function times(n, callback, thisArg) {
+ n = (n = +n) > -1 ? n : 0;
+ var index = -1,
+ result = Array(n);
+
+ callback = baseCreateCallback(callback, thisArg, 1);
+ while (++index < n) {
+ result[index] = callback(index);
+ }
+ return result;
+}
+
+module.exports = times;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/unescape.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/unescape.js b/node_modules/lodash-node/modern/utilities/unescape.js
new file mode 100644
index 0000000..57403f4
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/unescape.js
@@ -0,0 +1,32 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys'),
+ reEscapedHtml = require('../internals/reEscapedHtml'),
+ unescapeHtmlChar = require('../internals/unescapeHtmlChar');
+
+/**
+ * The inverse of `_.escape` this method converts the HTML entities
+ * `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding characters.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} string The string to unescape.
+ * @returns {string} Returns the unescaped string.
+ * @example
+ *
+ * _.unescape('Fred, Barney & Pebbles');
+ * // => 'Fred, Barney & Pebbles'
+ */
+function unescape(string) {
+ return string == null ? '' : String(string).replace(reEscapedHtml, unescapeHtmlChar);
+}
+
+module.exports = unescape;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/utilities/uniqueId.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/utilities/uniqueId.js b/node_modules/lodash-node/modern/utilities/uniqueId.js
new file mode 100644
index 0000000..cb4fd02
--- /dev/null
+++ b/node_modules/lodash-node/modern/utilities/uniqueId.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to generate unique IDs */
+var idCounter = 0;
+
+/**
+ * Generates a unique ID. If `prefix` is provided the ID will be appended to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {string} [prefix] The value to prefix the ID with.
+ * @returns {string} Returns the unique ID.
+ * @example
+ *
+ * _.uniqueId('contact_');
+ * // => 'contact_104'
+ *
+ * _.uniqueId();
+ * // => '105'
+ */
+function uniqueId(prefix) {
+ var id = ++idCounter;
+ return String(prefix == null ? '' : prefix) + id;
+}
+
+module.exports = uniqueId;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/package.json
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/package.json b/node_modules/lodash-node/package.json
new file mode 100644
index 0000000..6492b60
--- /dev/null
+++ b/node_modules/lodash-node/package.json
@@ -0,0 +1,111 @@
+{
+ "_args": [
+ [
+ "lodash-node@~2.4.1",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/simple-plist/node_modules/xmlbuilder"
+ ]
+ ],
+ "_from": "lodash-node@>=2.4.1 <2.5.0",
+ "_id": "lodash-node@2.4.1",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/lodash-node",
+ "_npmUser": {
+ "email": "john.david.dalton@gmail.com",
+ "name": "jdalton"
+ },
+ "_npmVersion": "1.3.14",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "lodash-node",
+ "raw": "lodash-node@~2.4.1",
+ "rawSpec": "~2.4.1",
+ "scope": null,
+ "spec": ">=2.4.1 <2.5.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/simple-plist/xmlbuilder"
+ ],
+ "_resolved": "http://registry.npmjs.org/lodash-node/-/lodash-node-2.4.1.tgz",
+ "_shasum": "ea82f7b100c733d1a42af76801e506105e2a80ec",
+ "_shrinkwrap": null,
+ "_spec": "lodash-node@~2.4.1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/simple-plist/node_modules/xmlbuilder",
+ "author": {
+ "email": "john.david.dalton@gmail.com",
+ "name": "John-David Dalton",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash-cli/issues"
+ },
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "http://mathiasbynens.be/"
+ }
+ ],
+ "dependencies": {},
+ "deprecated": "This package has been discontinued in favor of lodash@^4.0.0.",
+ "description": "A collection of Lo-Dash methods as Node.js modules generated by lodash-cli.",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "ea82f7b100c733d1a42af76801e506105e2a80ec",
+ "tarball": "http://registry.npmjs.org/lodash-node/-/lodash-node-2.4.1.tgz"
+ },
+ "homepage": "http://lodash.com/custom-builds",
+ "keywords": [
+ "customize",
+ "functional",
+ "lodash",
+ "modules",
+ "server",
+ "util"
+ ],
+ "license": "MIT",
+ "main": "modern/index.js",
+ "maintainers": [
+ {
+ "name": "jdalton",
+ "email": "john.david.dalton@gmail.com"
+ },
+ {
+ "name": "kitcambridge",
+ "email": "github@kitcambridge.be"
+ },
+ {
+ "name": "mathias",
+ "email": "mathias@qiwi.be"
+ },
+ {
+ "name": "phated",
+ "email": "blaine@iceddev.com"
+ }
+ ],
+ "name": "lodash-node",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash-node.git"
+ },
+ "version": "2.4.1"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays.js b/node_modules/lodash-node/underscore/arrays.js
new file mode 100644
index 0000000..4290b26
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'compact': require('./arrays/compact'),
+ 'difference': require('./arrays/difference'),
+ 'drop': require('./arrays/rest'),
+ 'first': require('./arrays/first'),
+ 'flatten': require('./arrays/flatten'),
+ 'head': require('./arrays/first'),
+ 'indexOf': require('./arrays/indexOf'),
+ 'initial': require('./arrays/initial'),
+ 'intersection': require('./arrays/intersection'),
+ 'last': require('./arrays/last'),
+ 'lastIndexOf': require('./arrays/lastIndexOf'),
+ 'object': require('./arrays/zipObject'),
+ 'range': require('./arrays/range'),
+ 'rest': require('./arrays/rest'),
+ 'sortedIndex': require('./arrays/sortedIndex'),
+ 'tail': require('./arrays/rest'),
+ 'take': require('./arrays/first'),
+ 'union': require('./arrays/union'),
+ 'uniq': require('./arrays/uniq'),
+ 'unique': require('./arrays/uniq'),
+ 'unzip': require('./arrays/zip'),
+ 'without': require('./arrays/without'),
+ 'zip': require('./arrays/zip'),
+ 'zipObject': require('./arrays/zipObject')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/compact.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/compact.js b/node_modules/lodash-node/underscore/arrays/compact.js
new file mode 100644
index 0000000..f82622b
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/compact.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Creates an array with all falsey values removed. The values `false`, `null`,
+ * `0`, `""`, `undefined`, and `NaN` are all falsey.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+function compact(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = compact;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/difference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/difference.js b/node_modules/lodash-node/underscore/arrays/difference.js
new file mode 100644
index 0000000..c5d16e4
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/difference.js
@@ -0,0 +1,31 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseDifference = require('../internals/baseDifference'),
+ baseFlatten = require('../internals/baseFlatten');
+
+/**
+ * Creates an array excluding all values of the provided arrays using strict
+ * equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {...Array} [values] The arrays of values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ * @example
+ *
+ * _.difference([1, 2, 3, 4, 5], [5, 2, 10]);
+ * // => [1, 3, 4]
+ */
+function difference(array) {
+ return baseDifference(array, baseFlatten(arguments, true, true, 1));
+}
+
+module.exports = difference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/first.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/first.js b/node_modules/lodash-node/underscore/arrays/first.js
new file mode 100644
index 0000000..1afd4fd
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/first.js
@@ -0,0 +1,86 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets the first element or first `n` elements of an array. If a callback
+ * is provided elements at the beginning of the array are returned as long
+ * as the callback returns truey. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias head, take
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the first element(s) of `array`.
+ * @example
+ *
+ * _.first([1, 2, 3]);
+ * // => 1
+ *
+ * _.first([1, 2, 3], 2);
+ * // => [1, 2]
+ *
+ * _.first([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [1, 2]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.first(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'blocked': true, 'employer': 'slate' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.pluck(_.first(characters, { 'employer': 'slate' }), 'name');
+ * // => ['barney', 'fred']
+ */
+function first(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = -1;
+ callback = createCallback(callback, thisArg, 3);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array ? array[0] : undefined;
+ }
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, n), length));
+}
+
+module.exports = first;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/flatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/flatten.js b/node_modules/lodash-node/underscore/arrays/flatten.js
new file mode 100644
index 0000000..03108f0
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/flatten.js
@@ -0,0 +1,56 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten');
+
+/**
+ * Flattens a nested array (the nesting can be to any depth). If `isShallow`
+ * is truey, the array will only be flattened a single level. If a callback
+ * is provided each element of the array is passed through the callback before
+ * flattening. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isShallow=false] A flag to restrict flattening to a single level.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2], [3, [[4]]]]);
+ * // => [1, 2, 3, 4];
+ *
+ * _.flatten([1, [2], [3, [[4]]]], true);
+ * // => [1, 2, 3, [[4]]];
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 30, 'pets': ['hoppy'] },
+ * { 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.flatten(characters, 'pets');
+ * // => ['hoppy', 'baby puss', 'dino']
+ */
+function flatten(array, isShallow) {
+ return baseFlatten(array, isShallow);
+}
+
+module.exports = flatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/indexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/indexOf.js b/node_modules/lodash-node/underscore/arrays/indexOf.js
new file mode 100644
index 0000000..8a8948d
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/indexOf.js
@@ -0,0 +1,50 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ sortedIndex = require('./sortedIndex');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Gets the index at which the first occurrence of `value` is found using
+ * strict equality for comparisons, i.e. `===`. If the array is already sorted
+ * providing `true` for `fromIndex` will run a faster binary search.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {boolean|number} [fromIndex=0] The index to search from or `true`
+ * to perform a binary search on a sorted array.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 1
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 4
+ *
+ * _.indexOf([1, 1, 2, 2, 3, 3], 2, true);
+ * // => 2
+ */
+function indexOf(array, value, fromIndex) {
+ if (typeof fromIndex == 'number') {
+ var length = array ? array.length : 0;
+ fromIndex = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex || 0);
+ } else if (fromIndex) {
+ var index = sortedIndex(array, value);
+ return array[index] === value ? index : -1;
+ }
+ return baseIndexOf(array, value, fromIndex);
+}
+
+module.exports = indexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/underscore/arrays/initial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/underscore/arrays/initial.js b/node_modules/lodash-node/underscore/arrays/initial.js
new file mode 100644
index 0000000..f36f4c1
--- /dev/null
+++ b/node_modules/lodash-node/underscore/arrays/initial.js
@@ -0,0 +1,82 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize underscore exports="node" -o ./underscore/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ slice = require('../internals/slice');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Gets all but the last element or last `n` elements of an array. If a
+ * callback is provided elements at the end of the array are excluded from
+ * the result as long as the callback returns truey. The callback is bound
+ * to `thisArg` and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|number|string} [callback=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is provided it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.initial([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.initial([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [1]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'blocked': false, 'employer': 'slate' },
+ * { 'name': 'fred', 'blocked': true, 'employer': 'slate' },
+ * { 'name': 'pebbles', 'blocked': true, 'employer': 'na' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.initial(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'blocked': false, 'employer': 'slate' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.pluck(_.initial(characters, { 'employer': 'na' }), 'name');
+ * // => ['barney', 'fred']
+ */
+function initial(array, callback, thisArg) {
+ var n = 0,
+ length = array ? array.length : 0;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg, 3);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : callback || n;
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, length - n), length));
+}
+
+module.exports = initial;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[05/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/cdata-end-split.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/cdata-end-split.js b/node_modules/sax/test/cdata-end-split.js
new file mode 100644
index 0000000..b41bd00
--- /dev/null
+++ b/node_modules/sax/test/cdata-end-split.js
@@ -0,0 +1,15 @@
+
+require(__dirname).test({
+ expect : [
+ ["opentag", {"name": "R","attributes": {}}],
+ ["opencdata", undefined],
+ ["cdata", " this is "],
+ ["closecdata", undefined],
+ ["closetag", "R"]
+ ]
+})
+ .write("<r><![CDATA[ this is ]")
+ .write("]>")
+ .write("</r>")
+ .close();
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/cdata-fake-end.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/cdata-fake-end.js b/node_modules/sax/test/cdata-fake-end.js
new file mode 100644
index 0000000..07aeac4
--- /dev/null
+++ b/node_modules/sax/test/cdata-fake-end.js
@@ -0,0 +1,28 @@
+
+var p = require(__dirname).test({
+ expect : [
+ ["opentag", {"name": "R","attributes": {}}],
+ ["opencdata", undefined],
+ ["cdata", "[[[[[[[[]]]]]]]]"],
+ ["closecdata", undefined],
+ ["closetag", "R"]
+ ]
+})
+var x = "<r><![CDATA[[[[[[[[[]]]]]]]]]]></r>"
+for (var i = 0; i < x.length ; i ++) {
+ p.write(x.charAt(i))
+}
+p.close();
+
+
+var p2 = require(__dirname).test({
+ expect : [
+ ["opentag", {"name": "R","attributes": {}}],
+ ["opencdata", undefined],
+ ["cdata", "[[[[[[[[]]]]]]]]"],
+ ["closecdata", undefined],
+ ["closetag", "R"]
+ ]
+})
+var x = "<r><![CDATA[[[[[[[[[]]]]]]]]]]></r>"
+p2.write(x).close();
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/cdata-multiple.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/cdata-multiple.js b/node_modules/sax/test/cdata-multiple.js
new file mode 100644
index 0000000..dab2015
--- /dev/null
+++ b/node_modules/sax/test/cdata-multiple.js
@@ -0,0 +1,15 @@
+
+require(__dirname).test({
+ expect : [
+ ["opentag", {"name": "R","attributes": {}}],
+ ["opencdata", undefined],
+ ["cdata", " this is "],
+ ["closecdata", undefined],
+ ["opencdata", undefined],
+ ["cdata", "character data "],
+ ["closecdata", undefined],
+ ["closetag", "R"]
+ ]
+}).write("<r><![CDATA[ this is ]]>").write("<![CDA").write("T").write("A[")
+ .write("character data ").write("]]></r>").close();
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/cdata.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/cdata.js b/node_modules/sax/test/cdata.js
new file mode 100644
index 0000000..0f09cce
--- /dev/null
+++ b/node_modules/sax/test/cdata.js
@@ -0,0 +1,10 @@
+require(__dirname).test({
+ xml : "<r><![CDATA[ this is character data ]]></r>",
+ expect : [
+ ["opentag", {"name": "R","attributes": {}}],
+ ["opencdata", undefined],
+ ["cdata", " this is character data "],
+ ["closecdata", undefined],
+ ["closetag", "R"]
+ ]
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/index.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/index.js b/node_modules/sax/test/index.js
new file mode 100644
index 0000000..d4e1ef4
--- /dev/null
+++ b/node_modules/sax/test/index.js
@@ -0,0 +1,86 @@
+var globalsBefore = JSON.stringify(Object.keys(global))
+ , util = require("util")
+ , assert = require("assert")
+ , fs = require("fs")
+ , path = require("path")
+ , sax = require("../lib/sax")
+
+exports.sax = sax
+
+// handy way to do simple unit tests
+// if the options contains an xml string, it'll be written and the parser closed.
+// otherwise, it's assumed that the test will write and close.
+exports.test = function test (options) {
+ var xml = options.xml
+ , parser = sax.parser(options.strict, options.opt)
+ , expect = options.expect
+ , e = 0
+ sax.EVENTS.forEach(function (ev) {
+ parser["on" + ev] = function (n) {
+ if (process.env.DEBUG) {
+ console.error({ expect: expect[e]
+ , actual: [ev, n] })
+ }
+ if (e >= expect.length && (ev === "end" || ev === "ready")) return
+ assert.ok( e < expect.length,
+ "expectation #"+e+" "+util.inspect(expect[e])+"\n"+
+ "Unexpected event: "+ev+" "+(n ? util.inspect(n) : ""))
+ var inspected = n instanceof Error ? "\n"+ n.message : util.inspect(n)
+ assert.equal(ev, expect[e][0],
+ "expectation #"+e+"\n"+
+ "Didn't get expected event\n"+
+ "expect: "+expect[e][0] + " " +util.inspect(expect[e][1])+"\n"+
+ "actual: "+ev+" "+inspected+"\n")
+ if (ev === "error") assert.equal(n.message, expect[e][1])
+ else assert.deepEqual(n, expect[e][1],
+ "expectation #"+e+"\n"+
+ "Didn't get expected argument\n"+
+ "expect: "+expect[e][0] + " " +util.inspect(expect[e][1])+"\n"+
+ "actual: "+ev+" "+inspected+"\n")
+ e++
+ if (ev === "error") parser.resume()
+ }
+ })
+ if (xml) parser.write(xml).close()
+ return parser
+}
+
+if (module === require.main) {
+ var running = true
+ , failures = 0
+
+ function fail (file, er) {
+ util.error("Failed: "+file)
+ util.error(er.stack || er.message)
+ failures ++
+ }
+
+ fs.readdir(__dirname, function (error, files) {
+ files = files.filter(function (file) {
+ return (/\.js$/.exec(file) && file !== 'index.js')
+ })
+ var n = files.length
+ , i = 0
+ console.log("0.." + n)
+ files.forEach(function (file) {
+ // run this test.
+ try {
+ require(path.resolve(__dirname, file))
+ var globalsAfter = JSON.stringify(Object.keys(global))
+ if (globalsAfter !== globalsBefore) {
+ var er = new Error("new globals introduced\n"+
+ "expected: "+globalsBefore+"\n"+
+ "actual: "+globalsAfter)
+ globalsBefore = globalsAfter
+ throw er
+ }
+ console.log("ok " + (++i) + " - " + file)
+ } catch (er) {
+ console.log("not ok "+ (++i) + " - " + file)
+ fail(file, er)
+ }
+ })
+ if (!failures) return console.log("#all pass")
+ else return console.error(failures + " failure" + (failures > 1 ? "s" : ""))
+ })
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/issue-23.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/issue-23.js b/node_modules/sax/test/issue-23.js
new file mode 100644
index 0000000..e7991b2
--- /dev/null
+++ b/node_modules/sax/test/issue-23.js
@@ -0,0 +1,43 @@
+
+require(__dirname).test
+ ( { xml :
+ "<compileClassesResponse>"+
+ "<result>"+
+ "<bodyCrc>653724009</bodyCrc>"+
+ "<column>-1</column>"+
+ "<id>01pG0000002KoSUIA0</id>"+
+ "<line>-1</line>"+
+ "<name>CalendarController</name>"+
+ "<success>true</success>"+
+ "</result>"+
+ "</compileClassesResponse>"
+
+ , expect :
+ [ [ "opentag", { name: "COMPILECLASSESRESPONSE", attributes: {} } ]
+ , [ "opentag", { name : "RESULT", attributes: {} } ]
+ , [ "opentag", { name: "BODYCRC", attributes: {} } ]
+ , [ "text", "653724009" ]
+ , [ "closetag", "BODYCRC" ]
+ , [ "opentag", { name: "COLUMN", attributes: {} } ]
+ , [ "text", "-1" ]
+ , [ "closetag", "COLUMN" ]
+ , [ "opentag", { name: "ID", attributes: {} } ]
+ , [ "text", "01pG0000002KoSUIA0" ]
+ , [ "closetag", "ID" ]
+ , [ "opentag", {name: "LINE", attributes: {} } ]
+ , [ "text", "-1" ]
+ , [ "closetag", "LINE" ]
+ , [ "opentag", {name: "NAME", attributes: {} } ]
+ , [ "text", "CalendarController" ]
+ , [ "closetag", "NAME" ]
+ , [ "opentag", {name: "SUCCESS", attributes: {} } ]
+ , [ "text", "true" ]
+ , [ "closetag", "SUCCESS" ]
+ , [ "closetag", "RESULT" ]
+ , [ "closetag", "COMPILECLASSESRESPONSE" ]
+ ]
+ , strict : false
+ , opt : {}
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/issue-30.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/issue-30.js b/node_modules/sax/test/issue-30.js
new file mode 100644
index 0000000..c2cc809
--- /dev/null
+++ b/node_modules/sax/test/issue-30.js
@@ -0,0 +1,24 @@
+// https://github.com/isaacs/sax-js/issues/33
+require(__dirname).test
+ ( { xml : "<xml>\n"+
+ "<!-- \n"+
+ " comment with a single dash- in it\n"+
+ "-->\n"+
+ "<data/>\n"+
+ "</xml>"
+
+ , expect :
+ [ [ "opentag", { name: "xml", attributes: {} } ]
+ , [ "text", "\n" ]
+ , [ "comment", " \n comment with a single dash- in it\n" ]
+ , [ "text", "\n" ]
+ , [ "opentag", { name: "data", attributes: {} } ]
+ , [ "closetag", "data" ]
+ , [ "text", "\n" ]
+ , [ "closetag", "xml" ]
+ ]
+ , strict : true
+ , opt : {}
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/issue-35.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/issue-35.js b/node_modules/sax/test/issue-35.js
new file mode 100644
index 0000000..7c521c5
--- /dev/null
+++ b/node_modules/sax/test/issue-35.js
@@ -0,0 +1,15 @@
+// https://github.com/isaacs/sax-js/issues/35
+require(__dirname).test
+ ( { xml : "<xml>

\n"+
+ "</xml>"
+
+ , expect :
+ [ [ "opentag", { name: "xml", attributes: {} } ]
+ , [ "text", "\r\r\n" ]
+ , [ "closetag", "xml" ]
+ ]
+ , strict : true
+ , opt : {}
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/issue-47.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/issue-47.js b/node_modules/sax/test/issue-47.js
new file mode 100644
index 0000000..911c7d0
--- /dev/null
+++ b/node_modules/sax/test/issue-47.js
@@ -0,0 +1,13 @@
+// https://github.com/isaacs/sax-js/issues/47
+require(__dirname).test
+ ( { xml : '<a href="query.svc?x=1&y=2&z=3"/>'
+ , expect : [
+ [ "attribute", { name:'href', value:"query.svc?x=1&y=2&z=3"} ],
+ [ "opentag", { name: "a", attributes: { href:"query.svc?x=1&y=2&z=3"} } ],
+ [ "closetag", "a" ]
+ ]
+ , strict : true
+ , opt : {}
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/issue-49.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/issue-49.js b/node_modules/sax/test/issue-49.js
new file mode 100644
index 0000000..2964325
--- /dev/null
+++ b/node_modules/sax/test/issue-49.js
@@ -0,0 +1,31 @@
+// https://github.com/isaacs/sax-js/issues/49
+require(__dirname).test
+ ( { xml : "<xml><script>hello world</script></xml>"
+ , expect :
+ [ [ "opentag", { name: "xml", attributes: {} } ]
+ , [ "opentag", { name: "script", attributes: {} } ]
+ , [ "text", "hello world" ]
+ , [ "closetag", "script" ]
+ , [ "closetag", "xml" ]
+ ]
+ , strict : false
+ , opt : { lowercasetags: true, noscript: true }
+ }
+ )
+
+require(__dirname).test
+ ( { xml : "<xml><script><![CDATA[hello world]]></script></xml>"
+ , expect :
+ [ [ "opentag", { name: "xml", attributes: {} } ]
+ , [ "opentag", { name: "script", attributes: {} } ]
+ , [ "opencdata", undefined ]
+ , [ "cdata", "hello world" ]
+ , [ "closecdata", undefined ]
+ , [ "closetag", "script" ]
+ , [ "closetag", "xml" ]
+ ]
+ , strict : false
+ , opt : { lowercasetags: true, noscript: true }
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/parser-position.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/parser-position.js b/node_modules/sax/test/parser-position.js
new file mode 100644
index 0000000..e4a68b1
--- /dev/null
+++ b/node_modules/sax/test/parser-position.js
@@ -0,0 +1,28 @@
+var sax = require("../lib/sax"),
+ assert = require("assert")
+
+function testPosition(chunks, expectedEvents) {
+ var parser = sax.parser();
+ expectedEvents.forEach(function(expectation) {
+ parser['on' + expectation[0]] = function() {
+ for (var prop in expectation[1]) {
+ assert.equal(parser[prop], expectation[1][prop]);
+ }
+ }
+ });
+ chunks.forEach(function(chunk) {
+ parser.write(chunk);
+ });
+};
+
+testPosition(['<div>abcdefgh</div>'],
+ [ ['opentag', { position: 5, startTagPosition: 1 }]
+ , ['text', { position: 19, startTagPosition: 14 }]
+ , ['closetag', { position: 19, startTagPosition: 14 }]
+ ]);
+
+testPosition(['<div>abcde','fgh</div>'],
+ [ ['opentag', { position: 5, startTagPosition: 1 }]
+ , ['text', { position: 19, startTagPosition: 14 }]
+ , ['closetag', { position: 19, startTagPosition: 14 }]
+ ]);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/script.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/script.js b/node_modules/sax/test/script.js
new file mode 100644
index 0000000..464c051
--- /dev/null
+++ b/node_modules/sax/test/script.js
@@ -0,0 +1,12 @@
+require(__dirname).test({
+ xml : "<html><head><script>if (1 < 0) { console.log('elo there'); }</script></head></html>",
+ expect : [
+ ["opentag", {"name": "HTML","attributes": {}}],
+ ["opentag", {"name": "HEAD","attributes": {}}],
+ ["opentag", {"name": "SCRIPT","attributes": {}}],
+ ["script", "if (1 < 0) { console.log('elo there'); }"],
+ ["closetag", "SCRIPT"],
+ ["closetag", "HEAD"],
+ ["closetag", "HTML"]
+ ]
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/self-closing-child-strict.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/self-closing-child-strict.js b/node_modules/sax/test/self-closing-child-strict.js
new file mode 100644
index 0000000..ce9c045
--- /dev/null
+++ b/node_modules/sax/test/self-closing-child-strict.js
@@ -0,0 +1,40 @@
+
+require(__dirname).test({
+ xml :
+ "<root>"+
+ "<child>" +
+ "<haha />" +
+ "</child>" +
+ "<monkey>" +
+ "=(|)" +
+ "</monkey>" +
+ "</root>",
+ expect : [
+ ["opentag", {
+ "name": "root",
+ "attributes": {}
+ }],
+ ["opentag", {
+ "name": "child",
+ "attributes": {}
+ }],
+ ["opentag", {
+ "name": "haha",
+ "attributes": {}
+ }],
+ ["closetag", "haha"],
+ ["closetag", "child"],
+ ["opentag", {
+ "name": "monkey",
+ "attributes": {}
+ }],
+ ["text", "=(|)"],
+ ["closetag", "monkey"],
+ ["closetag", "root"],
+ ["end"],
+ ["ready"]
+ ],
+ strict : true,
+ opt : {}
+});
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/self-closing-child.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/self-closing-child.js b/node_modules/sax/test/self-closing-child.js
new file mode 100644
index 0000000..bc6b52b
--- /dev/null
+++ b/node_modules/sax/test/self-closing-child.js
@@ -0,0 +1,40 @@
+
+require(__dirname).test({
+ xml :
+ "<root>"+
+ "<child>" +
+ "<haha />" +
+ "</child>" +
+ "<monkey>" +
+ "=(|)" +
+ "</monkey>" +
+ "</root>",
+ expect : [
+ ["opentag", {
+ "name": "ROOT",
+ "attributes": {}
+ }],
+ ["opentag", {
+ "name": "CHILD",
+ "attributes": {}
+ }],
+ ["opentag", {
+ "name": "HAHA",
+ "attributes": {}
+ }],
+ ["closetag", "HAHA"],
+ ["closetag", "CHILD"],
+ ["opentag", {
+ "name": "MONKEY",
+ "attributes": {}
+ }],
+ ["text", "=(|)"],
+ ["closetag", "MONKEY"],
+ ["closetag", "ROOT"],
+ ["end"],
+ ["ready"]
+ ],
+ strict : false,
+ opt : {}
+});
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/self-closing-tag.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/self-closing-tag.js b/node_modules/sax/test/self-closing-tag.js
new file mode 100644
index 0000000..b2c5736
--- /dev/null
+++ b/node_modules/sax/test/self-closing-tag.js
@@ -0,0 +1,25 @@
+
+require(__dirname).test({
+ xml :
+ "<root> "+
+ "<haha /> "+
+ "<haha/> "+
+ "<monkey> "+
+ "=(|) "+
+ "</monkey>"+
+ "</root> ",
+ expect : [
+ ["opentag", {name:"ROOT", attributes:{}}],
+ ["opentag", {name:"HAHA", attributes:{}}],
+ ["closetag", "HAHA"],
+ ["opentag", {name:"HAHA", attributes:{}}],
+ ["closetag", "HAHA"],
+ // ["opentag", {name:"HAHA", attributes:{}}],
+ // ["closetag", "HAHA"],
+ ["opentag", {name:"MONKEY", attributes:{}}],
+ ["text", "=(|)"],
+ ["closetag", "MONKEY"],
+ ["closetag", "ROOT"]
+ ],
+ opt : { trim : true }
+});
\ No newline at end of file
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/stray-ending.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/stray-ending.js b/node_modules/sax/test/stray-ending.js
new file mode 100644
index 0000000..6b0aa7f
--- /dev/null
+++ b/node_modules/sax/test/stray-ending.js
@@ -0,0 +1,17 @@
+// stray ending tags should just be ignored in non-strict mode.
+// https://github.com/isaacs/sax-js/issues/32
+require(__dirname).test
+ ( { xml :
+ "<a><b></c></b></a>"
+ , expect :
+ [ [ "opentag", { name: "A", attributes: {} } ]
+ , [ "opentag", { name: "B", attributes: {} } ]
+ , [ "text", "</c>" ]
+ , [ "closetag", "B" ]
+ , [ "closetag", "A" ]
+ ]
+ , strict : false
+ , opt : {}
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/trailing-non-whitespace.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/trailing-non-whitespace.js b/node_modules/sax/test/trailing-non-whitespace.js
new file mode 100644
index 0000000..3e1fb2e
--- /dev/null
+++ b/node_modules/sax/test/trailing-non-whitespace.js
@@ -0,0 +1,17 @@
+
+require(__dirname).test({
+ xml : "<span>Welcome,</span> to monkey land",
+ expect : [
+ ["opentag", {
+ "name": "SPAN",
+ "attributes": {}
+ }],
+ ["text", "Welcome,"],
+ ["closetag", "SPAN"],
+ ["text", " to monkey land"],
+ ["end"],
+ ["ready"]
+ ],
+ strict : false,
+ opt : {}
+});
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/unquoted.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/unquoted.js b/node_modules/sax/test/unquoted.js
new file mode 100644
index 0000000..79f1d0b
--- /dev/null
+++ b/node_modules/sax/test/unquoted.js
@@ -0,0 +1,17 @@
+// unquoted attributes should be ok in non-strict mode
+// https://github.com/isaacs/sax-js/issues/31
+require(__dirname).test
+ ( { xml :
+ "<span class=test hello=world></span>"
+ , expect :
+ [ [ "attribute", { name: "class", value: "test" } ]
+ , [ "attribute", { name: "hello", value: "world" } ]
+ , [ "opentag", { name: "SPAN",
+ attributes: { class: "test", hello: "world" } } ]
+ , [ "closetag", "SPAN" ]
+ ]
+ , strict : false
+ , opt : {}
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-issue-41.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-issue-41.js b/node_modules/sax/test/xmlns-issue-41.js
new file mode 100644
index 0000000..596d82b
--- /dev/null
+++ b/node_modules/sax/test/xmlns-issue-41.js
@@ -0,0 +1,67 @@
+var t = require(__dirname)
+
+ , xmls = // should be the same both ways.
+ [ "<parent xmlns:a='http://ATTRIBUTE' a:attr='value' />"
+ , "<parent a:attr='value' xmlns:a='http://ATTRIBUTE' />" ]
+
+ , ex1 =
+ [ [ "opennamespace"
+ , { prefix: "a"
+ , uri: "http://ATTRIBUTE"
+ }
+ ]
+ , [ "attribute"
+ , { name: "xmlns:a"
+ , value: "http://ATTRIBUTE"
+ , prefix: "xmlns"
+ , local: "a"
+ , uri: "http://www.w3.org/2000/xmlns/"
+ }
+ ]
+ , [ "attribute"
+ , { name: "a:attr"
+ , local: "attr"
+ , prefix: "a"
+ , uri: "http://ATTRIBUTE"
+ , value: "value"
+ }
+ ]
+ , [ "opentag"
+ , { name: "parent"
+ , uri: ""
+ , prefix: ""
+ , local: "parent"
+ , attributes:
+ { "a:attr":
+ { name: "a:attr"
+ , local: "attr"
+ , prefix: "a"
+ , uri: "http://ATTRIBUTE"
+ , value: "value"
+ }
+ , "xmlns:a":
+ { name: "xmlns:a"
+ , local: "a"
+ , prefix: "xmlns"
+ , uri: "http://www.w3.org/2000/xmlns/"
+ , value: "http://ATTRIBUTE"
+ }
+ }
+ , ns: {"a": "http://ATTRIBUTE"}
+ }
+ ]
+ , ["closetag", "parent"]
+ , ["closenamespace", { prefix: "a", uri: "http://ATTRIBUTE" }]
+ ]
+
+ // swap the order of elements 2 and 1
+ , ex2 = [ex1[0], ex1[2], ex1[1]].concat(ex1.slice(3))
+ , expected = [ex1, ex2]
+
+xmls.forEach(function (x, i) {
+ t.test({ xml: x
+ , expect: expected[i]
+ , strict: true
+ , opt: { xmlns: true }
+ })
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-rebinding.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-rebinding.js b/node_modules/sax/test/xmlns-rebinding.js
new file mode 100644
index 0000000..f464876
--- /dev/null
+++ b/node_modules/sax/test/xmlns-rebinding.js
@@ -0,0 +1,59 @@
+
+require(__dirname).test
+ ( { xml :
+ "<root xmlns:x='x1' xmlns:y='y1' x:a='x1' y:a='y1'>"+
+ "<rebind xmlns:x='x2'>"+
+ "<check x:a='x2' y:a='y1'/>"+
+ "</rebind>"+
+ "<check x:a='x1' y:a='y1'/>"+
+ "</root>"
+
+ , expect :
+ [ [ "opennamespace", { prefix: "x", uri: "x1" } ]
+ , [ "opennamespace", { prefix: "y", uri: "y1" } ]
+ , [ "attribute", { name: "xmlns:x", value: "x1", uri: "http://www.w3.org/2000/xmlns/", prefix: "xmlns", local: "x" } ]
+ , [ "attribute", { name: "xmlns:y", value: "y1", uri: "http://www.w3.org/2000/xmlns/", prefix: "xmlns", local: "y" } ]
+ , [ "attribute", { name: "x:a", value: "x1", uri: "x1", prefix: "x", local: "a" } ]
+ , [ "attribute", { name: "y:a", value: "y1", uri: "y1", prefix: "y", local: "a" } ]
+ , [ "opentag", { name: "root", uri: "", prefix: "", local: "root",
+ attributes: { "xmlns:x": { name: "xmlns:x", value: "x1", uri: "http://www.w3.org/2000/xmlns/", prefix: "xmlns", local: "x" }
+ , "xmlns:y": { name: "xmlns:y", value: "y1", uri: "http://www.w3.org/2000/xmlns/", prefix: "xmlns", local: "y" }
+ , "x:a": { name: "x:a", value: "x1", uri: "x1", prefix: "x", local: "a" }
+ , "y:a": { name: "y:a", value: "y1", uri: "y1", prefix: "y", local: "a" } },
+ ns: { x: 'x1', y: 'y1' } } ]
+
+ , [ "opennamespace", { prefix: "x", uri: "x2" } ]
+ , [ "attribute", { name: "xmlns:x", value: "x2", uri: "http://www.w3.org/2000/xmlns/", prefix: "xmlns", local: "x" } ]
+ , [ "opentag", { name: "rebind", uri: "", prefix: "", local: "rebind",
+ attributes: { "xmlns:x": { name: "xmlns:x", value: "x2", uri: "http://www.w3.org/2000/xmlns/", prefix: "xmlns", local: "x" } },
+ ns: { x: 'x2' } } ]
+
+ , [ "attribute", { name: "x:a", value: "x2", uri: "x2", prefix: "x", local: "a" } ]
+ , [ "attribute", { name: "y:a", value: "y1", uri: "y1", prefix: "y", local: "a" } ]
+ , [ "opentag", { name: "check", uri: "", prefix: "", local: "check",
+ attributes: { "x:a": { name: "x:a", value: "x2", uri: "x2", prefix: "x", local: "a" }
+ , "y:a": { name: "y:a", value: "y1", uri: "y1", prefix: "y", local: "a" } },
+ ns: { x: 'x2' } } ]
+
+ , [ "closetag", "check" ]
+
+ , [ "closetag", "rebind" ]
+ , [ "closenamespace", { prefix: "x", uri: "x2" } ]
+
+ , [ "attribute", { name: "x:a", value: "x1", uri: "x1", prefix: "x", local: "a" } ]
+ , [ "attribute", { name: "y:a", value: "y1", uri: "y1", prefix: "y", local: "a" } ]
+ , [ "opentag", { name: "check", uri: "", prefix: "", local: "check",
+ attributes: { "x:a": { name: "x:a", value: "x1", uri: "x1", prefix: "x", local: "a" }
+ , "y:a": { name: "y:a", value: "y1", uri: "y1", prefix: "y", local: "a" } },
+ ns: { x: 'x1', y: 'y1' } } ]
+ , [ "closetag", "check" ]
+
+ , [ "closetag", "root" ]
+ , [ "closenamespace", { prefix: "x", uri: "x1" } ]
+ , [ "closenamespace", { prefix: "y", uri: "y1" } ]
+ ]
+ , strict : true
+ , opt : { xmlns: true }
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-strict.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-strict.js b/node_modules/sax/test/xmlns-strict.js
new file mode 100644
index 0000000..4ad615b
--- /dev/null
+++ b/node_modules/sax/test/xmlns-strict.js
@@ -0,0 +1,71 @@
+
+require(__dirname).test
+ ( { xml :
+ "<root>"+
+ "<plain attr='normal'/>"+
+ "<ns1 xmlns='uri:default'>"+
+ "<plain attr='normal'/>"+
+ "</ns1>"+
+ "<ns2 xmlns:a='uri:nsa'>"+
+ "<plain attr='normal'/>"+
+ "<a:ns a:attr='namespaced'/>"+
+ "</ns2>"+
+ "</root>"
+
+ , expect :
+ [ [ "opentag", { name: "root", prefix: "", local: "root", uri: "",
+ attributes: {}, ns: {} } ]
+
+ , [ "attribute", { name: "attr", value: "normal", prefix: "", local: "attr", uri: "" } ]
+ , [ "opentag", { name: "plain", prefix: "", local: "plain", uri: "",
+ attributes: { "attr": { name: "attr", value: "normal", uri: "", prefix: "", local: "attr", uri: "" } },
+ ns: {} } ]
+ , [ "closetag", "plain" ]
+
+ , [ "opennamespace", { prefix: "", uri: "uri:default" } ]
+
+ , [ "attribute", { name: "xmlns", value: "uri:default", prefix: "xmlns", local: "", uri: "http://www.w3.org/2000/xmlns/" } ]
+ , [ "opentag", { name: "ns1", prefix: "", local: "ns1", uri: "uri:default",
+ attributes: { "xmlns": { name: "xmlns", value: "uri:default", prefix: "xmlns", local: "", uri: "http://www.w3.org/2000/xmlns/" } },
+ ns: { "": "uri:default" } } ]
+
+ , [ "attribute", { name: "attr", value: "normal", prefix: "", local: "attr", uri: "uri:default" } ]
+ , [ "opentag", { name: "plain", prefix: "", local: "plain", uri: "uri:default", ns: { '': 'uri:default' },
+ attributes: { "attr": { name: "attr", value: "normal", prefix: "", local: "attr", uri: "uri:default" } } } ]
+ , [ "closetag", "plain" ]
+
+ , [ "closetag", "ns1" ]
+
+ , [ "closenamespace", { prefix: "", uri: "uri:default" } ]
+
+ , [ "opennamespace", { prefix: "a", uri: "uri:nsa" } ]
+
+ , [ "attribute", { name: "xmlns:a", value: "uri:nsa", prefix: "xmlns", local: "a", uri: "http://www.w3.org/2000/xmlns/" } ]
+
+ , [ "opentag", { name: "ns2", prefix: "", local: "ns2", uri: "",
+ attributes: { "xmlns:a": { name: "xmlns:a", value: "uri:nsa", prefix: "xmlns", local: "a", uri: "http://www.w3.org/2000/xmlns/" } },
+ ns: { a: "uri:nsa" } } ]
+
+ , [ "attribute", { name: "attr", value: "normal", prefix: "", local: "attr", uri: "" } ]
+ , [ "opentag", { name: "plain", prefix: "", local: "plain", uri: "",
+ attributes: { "attr": { name: "attr", value: "normal", prefix: "", local: "attr", uri: "" } },
+ ns: { a: 'uri:nsa' } } ]
+ , [ "closetag", "plain" ]
+
+ , [ "attribute", { name: "a:attr", value: "namespaced", prefix: "a", local: "attr", uri: "uri:nsa" } ]
+ , [ "opentag", { name: "a:ns", prefix: "a", local: "ns", uri: "uri:nsa",
+ attributes: { "a:attr": { name: "a:attr", value: "namespaced", prefix: "a", local: "attr", uri: "uri:nsa" } },
+ ns: { a: 'uri:nsa' } } ]
+ , [ "closetag", "a:ns" ]
+
+ , [ "closetag", "ns2" ]
+
+ , [ "closenamespace", { prefix: "a", uri: "uri:nsa" } ]
+
+ , [ "closetag", "root" ]
+ ]
+ , strict : true
+ , opt : { xmlns: true }
+ }
+ )
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-unbound.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-unbound.js b/node_modules/sax/test/xmlns-unbound.js
new file mode 100644
index 0000000..2944b87
--- /dev/null
+++ b/node_modules/sax/test/xmlns-unbound.js
@@ -0,0 +1,15 @@
+
+require(__dirname).test(
+ { strict : true
+ , opt : { xmlns: true }
+ , expect :
+ [ ["error", "Unbound namespace prefix: \"unbound\"\nLine: 0\nColumn: 28\nChar: >"]
+
+ , [ "attribute", { name: "unbound:attr", value: "value", uri: "unbound", prefix: "unbound", local: "attr" } ]
+ , [ "opentag", { name: "root", uri: "", prefix: "", local: "root",
+ attributes: { "unbound:attr": { name: "unbound:attr", value: "value", uri: "unbound", prefix: "unbound", local: "attr" } },
+ ns: {} } ]
+ , [ "closetag", "root" ]
+ ]
+ }
+).write("<root unbound:attr='value'/>")
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-xml-default-prefix-attribute.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-xml-default-prefix-attribute.js b/node_modules/sax/test/xmlns-xml-default-prefix-attribute.js
new file mode 100644
index 0000000..16da771
--- /dev/null
+++ b/node_modules/sax/test/xmlns-xml-default-prefix-attribute.js
@@ -0,0 +1,35 @@
+require(__dirname).test(
+ { xml : "<root xml:lang='en'/>"
+ , expect :
+ [ [ "attribute"
+ , { name: "xml:lang"
+ , local: "lang"
+ , prefix: "xml"
+ , uri: "http://www.w3.org/XML/1998/namespace"
+ , value: "en"
+ }
+ ]
+ , [ "opentag"
+ , { name: "root"
+ , uri: ""
+ , prefix: ""
+ , local: "root"
+ , attributes:
+ { "xml:lang":
+ { name: "xml:lang"
+ , local: "lang"
+ , prefix: "xml"
+ , uri: "http://www.w3.org/XML/1998/namespace"
+ , value: "en"
+ }
+ }
+ , ns: {}
+ }
+ ]
+ , ["closetag", "root"]
+ ]
+ , strict : true
+ , opt : { xmlns: true }
+ }
+)
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-xml-default-prefix.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-xml-default-prefix.js b/node_modules/sax/test/xmlns-xml-default-prefix.js
new file mode 100644
index 0000000..9a1ce1b
--- /dev/null
+++ b/node_modules/sax/test/xmlns-xml-default-prefix.js
@@ -0,0 +1,20 @@
+require(__dirname).test(
+ { xml : "<xml:root/>"
+ , expect :
+ [
+ [ "opentag"
+ , { name: "xml:root"
+ , uri: "http://www.w3.org/XML/1998/namespace"
+ , prefix: "xml"
+ , local: "root"
+ , attributes: {}
+ , ns: {}
+ }
+ ]
+ , ["closetag", "xml:root"]
+ ]
+ , strict : true
+ , opt : { xmlns: true }
+ }
+)
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/sax/test/xmlns-xml-default-redefine.js
----------------------------------------------------------------------
diff --git a/node_modules/sax/test/xmlns-xml-default-redefine.js b/node_modules/sax/test/xmlns-xml-default-redefine.js
new file mode 100644
index 0000000..1eba9c7
--- /dev/null
+++ b/node_modules/sax/test/xmlns-xml-default-redefine.js
@@ -0,0 +1,40 @@
+require(__dirname).test(
+ { xml : "<xml:root xmlns:xml='ERROR'/>"
+ , expect :
+ [ ["error"
+ , "xml: prefix must be bound to http://www.w3.org/XML/1998/namespace\n"
+ + "Actual: ERROR\n"
+ + "Line: 0\nColumn: 27\nChar: '"
+ ]
+ , [ "attribute"
+ , { name: "xmlns:xml"
+ , local: "xml"
+ , prefix: "xmlns"
+ , uri: "http://www.w3.org/2000/xmlns/"
+ , value: "ERROR"
+ }
+ ]
+ , [ "opentag"
+ , { name: "xml:root"
+ , uri: "http://www.w3.org/XML/1998/namespace"
+ , prefix: "xml"
+ , local: "root"
+ , attributes:
+ { "xmlns:xml":
+ { name: "xmlns:xml"
+ , local: "xml"
+ , prefix: "xmlns"
+ , uri: "http://www.w3.org/2000/xmlns/"
+ , value: "ERROR"
+ }
+ }
+ , ns: {}
+ }
+ ]
+ , ["closetag", "xml:root"]
+ ]
+ , strict : true
+ , opt : { xmlns: true }
+ }
+)
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/semver/.npmignore b/node_modules/semver/.npmignore
new file mode 100644
index 0000000..534108e
--- /dev/null
+++ b/node_modules/semver/.npmignore
@@ -0,0 +1,4 @@
+node_modules/
+coverage/
+.nyc_output/
+nyc_output/
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/semver/.travis.yml b/node_modules/semver/.travis.yml
new file mode 100644
index 0000000..991d04b
--- /dev/null
+++ b/node_modules/semver/.travis.yml
@@ -0,0 +1,5 @@
+language: node_js
+node_js:
+ - '0.10'
+ - '0.12'
+ - 'iojs'
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/semver/LICENSE b/node_modules/semver/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/semver/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/README.md
----------------------------------------------------------------------
diff --git a/node_modules/semver/README.md b/node_modules/semver/README.md
new file mode 100644
index 0000000..0b14a7e
--- /dev/null
+++ b/node_modules/semver/README.md
@@ -0,0 +1,327 @@
+semver(1) -- The semantic versioner for npm
+===========================================
+
+## Usage
+
+ $ npm install semver
+
+ semver.valid('1.2.3') // '1.2.3'
+ semver.valid('a.b.c') // null
+ semver.clean(' =v1.2.3 ') // '1.2.3'
+ semver.satisfies('1.2.3', '1.x || >=2.5.0 || 5.0.0 - 7.2.3') // true
+ semver.gt('1.2.3', '9.8.7') // false
+ semver.lt('1.2.3', '9.8.7') // true
+
+As a command-line utility:
+
+ $ semver -h
+
+ Usage: semver <version> [<version> [...]] [-r <range> | -i <inc> | --preid <identifier> | -l | -rv]
+ Test if version(s) satisfy the supplied range(s), and sort them.
+
+ Multiple versions or ranges may be supplied, unless increment
+ option is specified. In that case, only a single version may
+ be used, and it is incremented by the specified level
+
+ Program exits successfully if any valid version satisfies
+ all supplied ranges, and prints all satisfying versions.
+
+ If no versions are valid, or ranges are not satisfied,
+ then exits failure.
+
+ Versions are printed in ascending order, so supplying
+ multiple versions to the utility will just sort them.
+
+## Versions
+
+A "version" is described by the `v2.0.0` specification found at
+<http://semver.org/>.
+
+A leading `"="` or `"v"` character is stripped off and ignored.
+
+## Ranges
+
+A `version range` is a set of `comparators` which specify versions
+that satisfy the range.
+
+A `comparator` is composed of an `operator` and a `version`. The set
+of primitive `operators` is:
+
+* `<` Less than
+* `<=` Less than or equal to
+* `>` Greater than
+* `>=` Greater than or equal to
+* `=` Equal. If no operator is specified, then equality is assumed,
+ so this operator is optional, but MAY be included.
+
+For example, the comparator `>=1.2.7` would match the versions
+`1.2.7`, `1.2.8`, `2.5.3`, and `1.3.9`, but not the versions `1.2.6`
+or `1.1.0`.
+
+Comparators can be joined by whitespace to form a `comparator set`,
+which is satisfied by the **intersection** of all of the comparators
+it includes.
+
+A range is composed of one or more comparator sets, joined by `||`. A
+version matches a range if and only if every comparator in at least
+one of the `||`-separated comparator sets is satisfied by the version.
+
+For example, the range `>=1.2.7 <1.3.0` would match the versions
+`1.2.7`, `1.2.8`, and `1.2.99`, but not the versions `1.2.6`, `1.3.0`,
+or `1.1.0`.
+
+The range `1.2.7 || >=1.2.9 <2.0.0` would match the versions `1.2.7`,
+`1.2.9`, and `1.4.6`, but not the versions `1.2.8` or `2.0.0`.
+
+### Prerelease Tags
+
+If a version has a prerelease tag (for example, `1.2.3-alpha.3`) then
+it will only be allowed to satisfy comparator sets if at least one
+comparator with the same `[major, minor, patch]` tuple also has a
+prerelease tag.
+
+For example, the range `>1.2.3-alpha.3` would be allowed to match the
+version `1.2.3-alpha.7`, but it would *not* be satisfied by
+`3.4.5-alpha.9`, even though `3.4.5-alpha.9` is technically "greater
+than" `1.2.3-alpha.3` according to the SemVer sort rules. The version
+range only accepts prerelease tags on the `1.2.3` version. The
+version `3.4.5` *would* satisfy the range, because it does not have a
+prerelease flag, and `3.4.5` is greater than `1.2.3-alpha.7`.
+
+The purpose for this behavior is twofold. First, prerelease versions
+frequently are updated very quickly, and contain many breaking changes
+that are (by the author's design) not yet fit for public consumption.
+Therefore, by default, they are excluded from range matching
+semantics.
+
+Second, a user who has opted into using a prerelease version has
+clearly indicated the intent to use *that specific* set of
+alpha/beta/rc versions. By including a prerelease tag in the range,
+the user is indicating that they are aware of the risk. However, it
+is still not appropriate to assume that they have opted into taking a
+similar risk on the *next* set of prerelease versions.
+
+#### Prerelease Identifiers
+
+The method `.inc` takes an additional `identifier` string argument that
+will append the value of the string as a prerelease identifier:
+
+```javascript
+> semver.inc('1.2.3', 'prerelease', 'beta')
+'1.2.4-beta.0'
+```
+
+command-line example:
+
+```shell
+$ semver 1.2.3 -i prerelease --preid beta
+1.2.4-beta.0
+```
+
+Which then can be used to increment further:
+
+```shell
+$ semver 1.2.4-beta.0 -i prerelease
+1.2.4-beta.1
+```
+
+### Advanced Range Syntax
+
+Advanced range syntax desugars to primitive comparators in
+deterministic ways.
+
+Advanced ranges may be combined in the same way as primitive
+comparators using white space or `||`.
+
+#### Hyphen Ranges `X.Y.Z - A.B.C`
+
+Specifies an inclusive set.
+
+* `1.2.3 - 2.3.4` := `>=1.2.3 <=2.3.4`
+
+If a partial version is provided as the first version in the inclusive
+range, then the missing pieces are replaced with zeroes.
+
+* `1.2 - 2.3.4` := `>=1.2.0 <=2.3.4`
+
+If a partial version is provided as the second version in the
+inclusive range, then all versions that start with the supplied parts
+of the tuple are accepted, but nothing that would be greater than the
+provided tuple parts.
+
+* `1.2.3 - 2.3` := `>=1.2.3 <2.4.0`
+* `1.2.3 - 2` := `>=1.2.3 <3.0.0`
+
+#### X-Ranges `1.2.x` `1.X` `1.2.*` `*`
+
+Any of `X`, `x`, or `*` may be used to "stand in" for one of the
+numeric values in the `[major, minor, patch]` tuple.
+
+* `*` := `>=0.0.0` (Any version satisfies)
+* `1.x` := `>=1.0.0 <2.0.0` (Matching major version)
+* `1.2.x` := `>=1.2.0 <1.3.0` (Matching major and minor versions)
+
+A partial version range is treated as an X-Range, so the special
+character is in fact optional.
+
+* `""` (empty string) := `*` := `>=0.0.0`
+* `1` := `1.x.x` := `>=1.0.0 <2.0.0`
+* `1.2` := `1.2.x` := `>=1.2.0 <1.3.0`
+
+#### Tilde Ranges `~1.2.3` `~1.2` `~1`
+
+Allows patch-level changes if a minor version is specified on the
+comparator. Allows minor-level changes if not.
+
+* `~1.2.3` := `>=1.2.3 <1.(2+1).0` := `>=1.2.3 <1.3.0`
+* `~1.2` := `>=1.2.0 <1.(2+1).0` := `>=1.2.0 <1.3.0` (Same as `1.2.x`)
+* `~1` := `>=1.0.0 <(1+1).0.0` := `>=1.0.0 <2.0.0` (Same as `1.x`)
+* `~0.2.3` := `>=0.2.3 <0.(2+1).0` := `>=0.2.3 <0.3.0`
+* `~0.2` := `>=0.2.0 <0.(2+1).0` := `>=0.2.0 <0.3.0` (Same as `0.2.x`)
+* `~0` := `>=0.0.0 <(0+1).0.0` := `>=0.0.0 <1.0.0` (Same as `0.x`)
+* `~1.2.3-beta.2` := `>=1.2.3-beta.2 <1.3.0` Note that prereleases in
+ the `1.2.3` version will be allowed, if they are greater than or
+ equal to `beta.2`. So, `1.2.3-beta.4` would be allowed, but
+ `1.2.4-beta.2` would not, because it is a prerelease of a
+ different `[major, minor, patch]` tuple.
+
+#### Caret Ranges `^1.2.3` `^0.2.5` `^0.0.4`
+
+Allows changes that do not modify the left-most non-zero digit in the
+`[major, minor, patch]` tuple. In other words, this allows patch and
+minor updates for versions `1.0.0` and above, patch updates for
+versions `0.X >=0.1.0`, and *no* updates for versions `0.0.X`.
+
+Many authors treat a `0.x` version as if the `x` were the major
+"breaking-change" indicator.
+
+Caret ranges are ideal when an author may make breaking changes
+between `0.2.4` and `0.3.0` releases, which is a common practice.
+However, it presumes that there will *not* be breaking changes between
+`0.2.4` and `0.2.5`. It allows for changes that are presumed to be
+additive (but non-breaking), according to commonly observed practices.
+
+* `^1.2.3` := `>=1.2.3 <2.0.0`
+* `^0.2.3` := `>=0.2.3 <0.3.0`
+* `^0.0.3` := `>=0.0.3 <0.0.4`
+* `^1.2.3-beta.2` := `>=1.2.3-beta.2 <2.0.0` Note that prereleases in
+ the `1.2.3` version will be allowed, if they are greater than or
+ equal to `beta.2`. So, `1.2.3-beta.4` would be allowed, but
+ `1.2.4-beta.2` would not, because it is a prerelease of a
+ different `[major, minor, patch]` tuple.
+* `^0.0.3-beta` := `>=0.0.3-beta <0.0.4` Note that prereleases in the
+ `0.0.3` version *only* will be allowed, if they are greater than or
+ equal to `beta`. So, `0.0.3-pr.2` would be allowed.
+
+When parsing caret ranges, a missing `patch` value desugars to the
+number `0`, but will allow flexibility within that value, even if the
+major and minor versions are both `0`.
+
+* `^1.2.x` := `>=1.2.0 <2.0.0`
+* `^0.0.x` := `>=0.0.0 <0.1.0`
+* `^0.0` := `>=0.0.0 <0.1.0`
+
+A missing `minor` and `patch` values will desugar to zero, but also
+allow flexibility within those values, even if the major version is
+zero.
+
+* `^1.x` := `>=1.0.0 <2.0.0`
+* `^0.x` := `>=0.0.0 <1.0.0`
+
+### Range Grammar
+
+Putting all this together, here is a Backus-Naur grammar for ranges,
+for the benefit of parser authors:
+
+```bnf
+range-set ::= range ( logical-or range ) *
+logical-or ::= ( ' ' ) * '||' ( ' ' ) *
+range ::= hyphen | simple ( ' ' simple ) * | ''
+hyphen ::= partial ' - ' partial
+simple ::= primitive | partial | tilde | caret
+primitive ::= ( '<' | '>' | '>=' | '<=' | '=' | ) partial
+partial ::= xr ( '.' xr ( '.' xr qualifier ? )? )?
+xr ::= 'x' | 'X' | '*' | nr
+nr ::= '0' | ['1'-'9']['0'-'9']+
+tilde ::= '~' partial
+caret ::= '^' partial
+qualifier ::= ( '-' pre )? ( '+' build )?
+pre ::= parts
+build ::= parts
+parts ::= part ( '.' part ) *
+part ::= nr | [-0-9A-Za-z]+
+```
+
+## Functions
+
+All methods and classes take a final `loose` boolean argument that, if
+true, will be more forgiving about not-quite-valid semver strings.
+The resulting output will always be 100% strict, of course.
+
+Strict-mode Comparators and Ranges will be strict about the SemVer
+strings that they parse.
+
+* `valid(v)`: Return the parsed version, or null if it's not valid.
+* `inc(v, release)`: Return the version incremented by the release
+ type (`major`, `premajor`, `minor`, `preminor`, `patch`,
+ `prepatch`, or `prerelease`), or null if it's not valid
+ * `premajor` in one call will bump the version up to the next major
+ version and down to a prerelease of that major version.
+ `preminor`, and `prepatch` work the same way.
+ * If called from a non-prerelease version, the `prerelease` will work the
+ same as `prepatch`. It increments the patch version, then makes a
+ prerelease. If the input version is already a prerelease it simply
+ increments it.
+* `major(v)`: Return the major version number.
+* `minor(v)`: Return the minor version number.
+* `patch(v)`: Return the patch version number.
+
+### Comparison
+
+* `gt(v1, v2)`: `v1 > v2`
+* `gte(v1, v2)`: `v1 >= v2`
+* `lt(v1, v2)`: `v1 < v2`
+* `lte(v1, v2)`: `v1 <= v2`
+* `eq(v1, v2)`: `v1 == v2` This is true if they're logically equivalent,
+ even if they're not the exact same string. You already know how to
+ compare strings.
+* `neq(v1, v2)`: `v1 != v2` The opposite of `eq`.
+* `cmp(v1, comparator, v2)`: Pass in a comparison string, and it'll call
+ the corresponding function above. `"==="` and `"!=="` do simple
+ string comparison, but are included for completeness. Throws if an
+ invalid comparison string is provided.
+* `compare(v1, v2)`: Return `0` if `v1 == v2`, or `1` if `v1` is greater, or `-1` if
+ `v2` is greater. Sorts in ascending order if passed to `Array.sort()`.
+* `rcompare(v1, v2)`: The reverse of compare. Sorts an array of versions
+ in descending order when passed to `Array.sort()`.
+* `diff(v1, v2)`: Returns difference between two versions by the release type
+ (`major`, `premajor`, `minor`, `preminor`, `patch`, `prepatch`, or `prerelease`),
+ or null if the versions are the same.
+
+
+### Ranges
+
+* `validRange(range)`: Return the valid range or null if it's not valid
+* `satisfies(version, range)`: Return true if the version satisfies the
+ range.
+* `maxSatisfying(versions, range)`: Return the highest version in the list
+ that satisfies the range, or `null` if none of them do.
+* `gtr(version, range)`: Return `true` if version is greater than all the
+ versions possible in the range.
+* `ltr(version, range)`: Return `true` if version is less than all the
+ versions possible in the range.
+* `outside(version, range, hilo)`: Return true if the version is outside
+ the bounds of the range in either the high or low direction. The
+ `hilo` argument must be either the string `'>'` or `'<'`. (This is
+ the function called by `gtr` and `ltr`.)
+
+Note that, since ranges may be non-contiguous, a version might not be
+greater than a range, less than a range, *or* satisfy a range! For
+example, the range `1.2 <1.2.9 || >2.0.0` would have a hole from `1.2.9`
+until `2.0.0`, so the version `1.2.10` would not be greater than the
+range (because `2.0.1` satisfies, which is higher), nor less than the
+range (since `1.2.8` satisfies, which is lower), and it also does not
+satisfy the range.
+
+If you want to know if a version satisfies or does not satisfy a
+range, use the `satisfies(version, range)` function.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/bin/semver
----------------------------------------------------------------------
diff --git a/node_modules/semver/bin/semver b/node_modules/semver/bin/semver
new file mode 100755
index 0000000..c5f2e85
--- /dev/null
+++ b/node_modules/semver/bin/semver
@@ -0,0 +1,133 @@
+#!/usr/bin/env node
+// Standalone semver comparison program.
+// Exits successfully and prints matching version(s) if
+// any supplied version is valid and passes all tests.
+
+var argv = process.argv.slice(2)
+ , versions = []
+ , range = []
+ , gt = []
+ , lt = []
+ , eq = []
+ , inc = null
+ , version = require("../package.json").version
+ , loose = false
+ , identifier = undefined
+ , semver = require("../semver")
+ , reverse = false
+
+main()
+
+function main () {
+ if (!argv.length) return help()
+ while (argv.length) {
+ var a = argv.shift()
+ var i = a.indexOf('=')
+ if (i !== -1) {
+ a = a.slice(0, i)
+ argv.unshift(a.slice(i + 1))
+ }
+ switch (a) {
+ case "-rv": case "-rev": case "--rev": case "--reverse":
+ reverse = true
+ break
+ case "-l": case "--loose":
+ loose = true
+ break
+ case "-v": case "--version":
+ versions.push(argv.shift())
+ break
+ case "-i": case "--inc": case "--increment":
+ switch (argv[0]) {
+ case "major": case "minor": case "patch": case "prerelease":
+ case "premajor": case "preminor": case "prepatch":
+ inc = argv.shift()
+ break
+ default:
+ inc = "patch"
+ break
+ }
+ break
+ case "--preid":
+ identifier = argv.shift()
+ break
+ case "-r": case "--range":
+ range.push(argv.shift())
+ break
+ case "-h": case "--help": case "-?":
+ return help()
+ default:
+ versions.push(a)
+ break
+ }
+ }
+
+ versions = versions.filter(function (v) {
+ return semver.valid(v, loose)
+ })
+ if (!versions.length) return fail()
+ if (inc && (versions.length !== 1 || range.length))
+ return failInc()
+
+ for (var i = 0, l = range.length; i < l ; i ++) {
+ versions = versions.filter(function (v) {
+ return semver.satisfies(v, range[i], loose)
+ })
+ if (!versions.length) return fail()
+ }
+ return success(versions)
+}
+
+function failInc () {
+ console.error("--inc can only be used on a single version with no range")
+ fail()
+}
+
+function fail () { process.exit(1) }
+
+function success () {
+ var compare = reverse ? "rcompare" : "compare"
+ versions.sort(function (a, b) {
+ return semver[compare](a, b, loose)
+ }).map(function (v) {
+ return semver.clean(v, loose)
+ }).map(function (v) {
+ return inc ? semver.inc(v, inc, loose, identifier) : v
+ }).forEach(function (v,i,_) { console.log(v) })
+}
+
+function help () {
+ console.log(["SemVer " + version
+ ,""
+ ,"A JavaScript implementation of the http://semver.org/ specification"
+ ,"Copyright Isaac Z. Schlueter"
+ ,""
+ ,"Usage: semver [options] <version> [<version> [...]]"
+ ,"Prints valid versions sorted by SemVer precedence"
+ ,""
+ ,"Options:"
+ ,"-r --range <range>"
+ ," Print versions that match the specified range."
+ ,""
+ ,"-i --increment [<level>]"
+ ," Increment a version by the specified level. Level can"
+ ," be one of: major, minor, patch, premajor, preminor,"
+ ," prepatch, or prerelease. Default level is 'patch'."
+ ," Only one version may be specified."
+ ,""
+ ,"--preid <identifier>"
+ ," Identifier to be used to prefix premajor, preminor,"
+ ," prepatch or prerelease version increments."
+ ,""
+ ,"-l --loose"
+ ," Interpret versions and ranges loosely"
+ ,""
+ ,"Program exits successfully if any valid version satisfies"
+ ,"all supplied ranges, and prints all satisfying versions."
+ ,""
+ ,"If no satisfying versions are found, then exits failure."
+ ,""
+ ,"Versions are printed in ascending order, so supplying"
+ ,"multiple versions to the utility will just sort them."
+ ].join("\n"))
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/package.json
----------------------------------------------------------------------
diff --git a/node_modules/semver/package.json b/node_modules/semver/package.json
new file mode 100644
index 0000000..cbd09b4
--- /dev/null
+++ b/node_modules/semver/package.json
@@ -0,0 +1,77 @@
+{
+ "_args": [
+ [
+ "semver@^5.0.1",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "semver@>=5.0.1 <6.0.0",
+ "_id": "semver@5.1.0",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/semver",
+ "_nodeVersion": "4.0.0",
+ "_npmUser": {
+ "email": "i@izs.me",
+ "name": "isaacs"
+ },
+ "_npmVersion": "3.3.2",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "semver",
+ "raw": "semver@^5.0.1",
+ "rawSpec": "^5.0.1",
+ "scope": null,
+ "spec": ">=5.0.1 <6.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/semver/-/semver-5.1.0.tgz",
+ "_shasum": "85f2cf8550465c4df000cf7d86f6b054106ab9e5",
+ "_shrinkwrap": null,
+ "_spec": "semver@^5.0.1",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "bin": {
+ "semver": "./bin/semver"
+ },
+ "bugs": {
+ "url": "https://github.com/npm/node-semver/issues"
+ },
+ "dependencies": {},
+ "description": "The semantic version parser used by npm.",
+ "devDependencies": {
+ "tap": "^2.0.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "85f2cf8550465c4df000cf7d86f6b054106ab9e5",
+ "tarball": "http://registry.npmjs.org/semver/-/semver-5.1.0.tgz"
+ },
+ "gitHead": "8e33a30e62e40e4983d1c5f55e794331b861aadc",
+ "homepage": "https://github.com/npm/node-semver#readme",
+ "license": "ISC",
+ "main": "semver.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ },
+ {
+ "name": "othiym23",
+ "email": "ogd@aoaioxxysz.net"
+ }
+ ],
+ "name": "semver",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/npm/node-semver.git"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "version": "5.1.0"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/semver/range.bnf
----------------------------------------------------------------------
diff --git a/node_modules/semver/range.bnf b/node_modules/semver/range.bnf
new file mode 100644
index 0000000..000df92
--- /dev/null
+++ b/node_modules/semver/range.bnf
@@ -0,0 +1,16 @@
+range-set ::= range ( logical-or range ) *
+logical-or ::= ( ' ' ) * '||' ( ' ' ) *
+range ::= hyphen | simple ( ' ' simple ) * | ''
+hyphen ::= partial ' - ' partial
+simple ::= primitive | partial | tilde | caret
+primitive ::= ( '<' | '>' | '>=' | '<=' | '=' | ) partial
+partial ::= xr ( '.' xr ( '.' xr qualifier ? )? )?
+xr ::= 'x' | 'X' | '*' | nr
+nr ::= '0' | ['1'-'9']['0'-'9']+
+tilde ::= '~' partial
+caret ::= '^' partial
+qualifier ::= ( '-' pre )? ( '+' build )?
+pre ::= parts
+build ::= parts
+parts ::= part ( '.' part ) *
+part ::= nr | [-0-9A-Za-z]+
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[37/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/chaining/tap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/chaining/tap.js b/node_modules/lodash-node/modern/chaining/tap.js
new file mode 100644
index 0000000..6249412
--- /dev/null
+++ b/node_modules/lodash-node/modern/chaining/tap.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Invokes `interceptor` with the `value` as the first argument and then
+ * returns `value`. The purpose of this method is to "tap into" a method
+ * chain in order to perform operations on intermediate results within
+ * the chain.
+ *
+ * @static
+ * @memberOf _
+ * @category Chaining
+ * @param {*} value The value to provide to `interceptor`.
+ * @param {Function} interceptor The function to invoke.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * _([1, 2, 3, 4])
+ * .tap(function(array) { array.pop(); })
+ * .reverse()
+ * .value();
+ * // => [3, 2, 1]
+ */
+function tap(value, interceptor) {
+ interceptor(value);
+ return value;
+}
+
+module.exports = tap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/chaining/wrapperChain.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/chaining/wrapperChain.js b/node_modules/lodash-node/modern/chaining/wrapperChain.js
new file mode 100644
index 0000000..72f13a4
--- /dev/null
+++ b/node_modules/lodash-node/modern/chaining/wrapperChain.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Enables explicit method chaining on the wrapper object.
+ *
+ * @name chain
+ * @memberOf _
+ * @category Chaining
+ * @returns {*} Returns the wrapper object.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // without explicit chaining
+ * _(characters).first();
+ * // => { 'name': 'barney', 'age': 36 }
+ *
+ * // with explicit chaining
+ * _(characters).chain()
+ * .first()
+ * .pick('age')
+ * .value();
+ * // => { 'age': 36 }
+ */
+function wrapperChain() {
+ this.__chain__ = true;
+ return this;
+}
+
+module.exports = wrapperChain;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/chaining/wrapperToString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/chaining/wrapperToString.js b/node_modules/lodash-node/modern/chaining/wrapperToString.js
new file mode 100644
index 0000000..29abeec
--- /dev/null
+++ b/node_modules/lodash-node/modern/chaining/wrapperToString.js
@@ -0,0 +1,26 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Produces the `toString` result of the wrapped value.
+ *
+ * @name toString
+ * @memberOf _
+ * @category Chaining
+ * @returns {string} Returns the string result.
+ * @example
+ *
+ * _([1, 2, 3]).toString();
+ * // => '1,2,3'
+ */
+function wrapperToString() {
+ return String(this.__wrapped__);
+}
+
+module.exports = wrapperToString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/chaining/wrapperValueOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/chaining/wrapperValueOf.js b/node_modules/lodash-node/modern/chaining/wrapperValueOf.js
new file mode 100644
index 0000000..a76aa8b
--- /dev/null
+++ b/node_modules/lodash-node/modern/chaining/wrapperValueOf.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ support = require('../support');
+
+/**
+ * Extracts the wrapped value.
+ *
+ * @name valueOf
+ * @memberOf _
+ * @alias value
+ * @category Chaining
+ * @returns {*} Returns the wrapped value.
+ * @example
+ *
+ * _([1, 2, 3]).valueOf();
+ * // => [1, 2, 3]
+ */
+function wrapperValueOf() {
+ return this.__wrapped__;
+}
+
+module.exports = wrapperValueOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections.js b/node_modules/lodash-node/modern/collections.js
new file mode 100644
index 0000000..58cc42e
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'all': require('./collections/every'),
+ 'any': require('./collections/some'),
+ 'at': require('./collections/at'),
+ 'collect': require('./collections/map'),
+ 'contains': require('./collections/contains'),
+ 'countBy': require('./collections/countBy'),
+ 'detect': require('./collections/find'),
+ 'each': require('./collections/forEach'),
+ 'eachRight': require('./collections/forEachRight'),
+ 'every': require('./collections/every'),
+ 'filter': require('./collections/filter'),
+ 'find': require('./collections/find'),
+ 'findLast': require('./collections/findLast'),
+ 'findWhere': require('./collections/find'),
+ 'foldl': require('./collections/reduce'),
+ 'foldr': require('./collections/reduceRight'),
+ 'forEach': require('./collections/forEach'),
+ 'forEachRight': require('./collections/forEachRight'),
+ 'groupBy': require('./collections/groupBy'),
+ 'include': require('./collections/contains'),
+ 'indexBy': require('./collections/indexBy'),
+ 'inject': require('./collections/reduce'),
+ 'invoke': require('./collections/invoke'),
+ 'map': require('./collections/map'),
+ 'max': require('./collections/max'),
+ 'min': require('./collections/min'),
+ 'pluck': require('./collections/pluck'),
+ 'reduce': require('./collections/reduce'),
+ 'reduceRight': require('./collections/reduceRight'),
+ 'reject': require('./collections/reject'),
+ 'sample': require('./collections/sample'),
+ 'select': require('./collections/filter'),
+ 'shuffle': require('./collections/shuffle'),
+ 'size': require('./collections/size'),
+ 'some': require('./collections/some'),
+ 'sortBy': require('./collections/sortBy'),
+ 'toArray': require('./collections/toArray'),
+ 'where': require('./collections/where')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/at.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/at.js b/node_modules/lodash-node/modern/collections/at.js
new file mode 100644
index 0000000..7f48511
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/at.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ isString = require('../objects/isString');
+
+/**
+ * Creates an array of elements from the specified indexes, or keys, of the
+ * `collection`. Indexes may be specified as individual arguments or as arrays
+ * of indexes.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {...(number|number[]|string|string[])} [index] The indexes of `collection`
+ * to retrieve, specified as individual indexes or arrays of indexes.
+ * @returns {Array} Returns a new array of elements corresponding to the
+ * provided indexes.
+ * @example
+ *
+ * _.at(['a', 'b', 'c', 'd', 'e'], [0, 2, 4]);
+ * // => ['a', 'c', 'e']
+ *
+ * _.at(['fred', 'barney', 'pebbles'], 0, 2);
+ * // => ['fred', 'pebbles']
+ */
+function at(collection) {
+ var args = arguments,
+ index = -1,
+ props = baseFlatten(args, true, false, 1),
+ length = (args[2] && args[2][args[1]] === collection) ? 1 : props.length,
+ result = Array(length);
+
+ while(++index < length) {
+ result[index] = collection[props[index]];
+ }
+ return result;
+}
+
+module.exports = at;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/contains.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/contains.js b/node_modules/lodash-node/modern/collections/contains.js
new file mode 100644
index 0000000..6ca66a6
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/contains.js
@@ -0,0 +1,65 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('../internals/baseIndexOf'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Checks if a given value is present in a collection using strict equality
+ * for comparisons, i.e. `===`. If `fromIndex` is negative, it is used as the
+ * offset from the end of the collection.
+ *
+ * @static
+ * @memberOf _
+ * @alias include
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {*} target The value to check for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {boolean} Returns `true` if the `target` element is found, else `false`.
+ * @example
+ *
+ * _.contains([1, 2, 3], 1);
+ * // => true
+ *
+ * _.contains([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.contains({ 'name': 'fred', 'age': 40 }, 'fred');
+ * // => true
+ *
+ * _.contains('pebbles', 'eb');
+ * // => true
+ */
+function contains(collection, target, fromIndex) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = collection ? collection.length : 0,
+ result = false;
+
+ fromIndex = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex) || 0;
+ if (isArray(collection)) {
+ result = indexOf(collection, target, fromIndex) > -1;
+ } else if (typeof length == 'number') {
+ result = (isString(collection) ? collection.indexOf(target, fromIndex) : indexOf(collection, target, fromIndex)) > -1;
+ } else {
+ forOwn(collection, function(value) {
+ if (++index >= fromIndex) {
+ return !(result = value === target);
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = contains;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/countBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/countBy.js b/node_modules/lodash-node/modern/collections/countBy.js
new file mode 100644
index 0000000..a11a038
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/countBy.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of `collection` through the callback. The corresponding value
+ * of each key is the number of times the key was returned by the callback.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+var countBy = createAggregator(function(result, value, key) {
+ (hasOwnProperty.call(result, key) ? result[key]++ : result[key] = 1);
+});
+
+module.exports = countBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/every.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/every.js b/node_modules/lodash-node/modern/collections/every.js
new file mode 100644
index 0000000..2b9c2c6
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/every.js
@@ -0,0 +1,74 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Checks if the given callback returns truey value for **all** elements of
+ * a collection. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if all elements passed the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes']);
+ * // => false
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.every(characters, 'age');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.every(characters, { 'age': 36 });
+ * // => false
+ */
+function every(collection, callback, thisArg) {
+ var result = true;
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ if (!(result = !!callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ return (result = !!callback(value, index, collection));
+ });
+ }
+ return result;
+}
+
+module.exports = every;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/filter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/filter.js b/node_modules/lodash-node/modern/collections/filter.js
new file mode 100644
index 0000000..b6df332
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/filter.js
@@ -0,0 +1,76 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Iterates over elements of a collection, returning an array of all elements
+ * the callback returns truey for. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias select
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that passed the callback check.
+ * @example
+ *
+ * var evens = _.filter([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [2, 4, 6]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.filter(characters, 'blocked');
+ * // => [{ 'name': 'fred', 'age': 40, 'blocked': true }]
+ *
+ * // using "_.where" callback shorthand
+ * _.filter(characters, { 'age': 36 });
+ * // => [{ 'name': 'barney', 'age': 36, 'blocked': false }]
+ */
+function filter(collection, callback, thisArg) {
+ var result = [];
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = filter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/find.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/find.js b/node_modules/lodash-node/modern/collections/find.js
new file mode 100644
index 0000000..0c75700
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/find.js
@@ -0,0 +1,80 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Iterates over elements of a collection, returning the first element that
+ * the callback returns truey for. The callback is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias detect, findWhere
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the found element, else `undefined`.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true },
+ * { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ * ];
+ *
+ * _.find(characters, function(chr) {
+ * return chr.age < 40;
+ * });
+ * // => { 'name': 'barney', 'age': 36, 'blocked': false }
+ *
+ * // using "_.where" callback shorthand
+ * _.find(characters, { 'age': 1 });
+ * // => { 'name': 'pebbles', 'age': 1, 'blocked': false }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.find(characters, 'blocked');
+ * // => { 'name': 'fred', 'age': 40, 'blocked': true }
+ */
+function find(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ return value;
+ }
+ }
+ } else {
+ var result;
+ forOwn(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result = value;
+ return false;
+ }
+ });
+ return result;
+ }
+}
+
+module.exports = find;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/findLast.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/findLast.js b/node_modules/lodash-node/modern/collections/findLast.js
new file mode 100644
index 0000000..64e05d6
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/findLast.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEachRight = require('./forEachRight');
+
+/**
+ * This method is like `_.find` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the found element, else `undefined`.
+ * @example
+ *
+ * _.findLast([1, 2, 3, 4], function(num) {
+ * return num % 2 == 1;
+ * });
+ * // => 3
+ */
+function findLast(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+ forEachRight(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result = value;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = findLast;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/forEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/forEach.js b/node_modules/lodash-node/modern/collections/forEach.js
new file mode 100644
index 0000000..65511e3
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/forEach.js
@@ -0,0 +1,55 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Iterates over elements of a collection, executing the callback for each
+ * element. The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection). Callbacks may exit iteration early by
+ * explicitly returning `false`.
+ *
+ * Note: As with other "Collections" methods, objects with a `length` property
+ * are iterated like arrays. To avoid this behavior `_.forIn` or `_.forOwn`
+ * may be used for object iteration.
+ *
+ * @static
+ * @memberOf _
+ * @alias each
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEach(function(num) { console.log(num); }).join(',');
+ * // => logs each number and returns '1,2,3'
+ *
+ * _.forEach({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { console.log(num); });
+ * // => logs each number and returns the object (property order is not guaranteed across environments)
+ */
+function forEach(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3);
+ if (typeof length == 'number') {
+ while (++index < length) {
+ if (callback(collection[index], index, collection) === false) {
+ break;
+ }
+ }
+ } else {
+ forOwn(collection, callback);
+ }
+ return collection;
+}
+
+module.exports = forEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/forEachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/forEachRight.js b/node_modules/lodash-node/modern/collections/forEachRight.js
new file mode 100644
index 0000000..8817169
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/forEachRight.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString'),
+ keys = require('../objects/keys');
+
+/**
+ * This method is like `_.forEach` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias eachRight
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|string} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEachRight(function(num) { console.log(num); }).join(',');
+ * // => logs each number from right to left and returns '3,2,1'
+ */
+function forEachRight(collection, callback, thisArg) {
+ var length = collection ? collection.length : 0;
+ callback = callback && typeof thisArg == 'undefined' ? callback : baseCreateCallback(callback, thisArg, 3);
+ if (typeof length == 'number') {
+ while (length--) {
+ if (callback(collection[length], length, collection) === false) {
+ break;
+ }
+ }
+ } else {
+ var props = keys(collection);
+ length = props.length;
+ forOwn(collection, function(value, key, collection) {
+ key = props ? props[--length] : --length;
+ return callback(collection[key], key, collection);
+ });
+ }
+ return collection;
+}
+
+module.exports = forEachRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/groupBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/groupBy.js b/node_modules/lodash-node/modern/collections/groupBy.js
new file mode 100644
index 0000000..f2df3ba
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/groupBy.js
@@ -0,0 +1,56 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of a collection through the callback. The corresponding value
+ * of each key is an array of the elements responsible for generating the key.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+var groupBy = createAggregator(function(result, value, key) {
+ (hasOwnProperty.call(result, key) ? result[key] : result[key] = []).push(value);
+});
+
+module.exports = groupBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/indexBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/indexBy.js b/node_modules/lodash-node/modern/collections/indexBy.js
new file mode 100644
index 0000000..34312c1
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/indexBy.js
@@ -0,0 +1,54 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createAggregator = require('../internals/createAggregator');
+
+/**
+ * Creates an object composed of keys generated from the results of running
+ * each element of the collection through the given callback. The corresponding
+ * value of each key is the last element responsible for generating the key.
+ * The callback is bound to `thisArg` and invoked with three arguments;
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * var keys = [
+ * { 'dir': 'left', 'code': 97 },
+ * { 'dir': 'right', 'code': 100 }
+ * ];
+ *
+ * _.indexBy(keys, 'dir');
+ * // => { 'left': { 'dir': 'left', 'code': 97 }, 'right': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(keys, function(key) { return String.fromCharCode(key.code); });
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ *
+ * _.indexBy(characters, function(key) { this.fromCharCode(key.code); }, String);
+ * // => { 'a': { 'dir': 'left', 'code': 97 }, 'd': { 'dir': 'right', 'code': 100 } }
+ */
+var indexBy = createAggregator(function(result, value, key) {
+ result[key] = value;
+});
+
+module.exports = indexBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/invoke.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/invoke.js b/node_modules/lodash-node/modern/collections/invoke.js
new file mode 100644
index 0000000..3a2f0f7
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/invoke.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('./forEach'),
+ slice = require('../internals/slice');
+
+/**
+ * Invokes the method named by `methodName` on each element in the `collection`
+ * returning an array of the results of each invoked method. Additional arguments
+ * will be provided to each invoked method. If `methodName` is a function it
+ * will be invoked for, and `this` bound to, each element in the `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|string} methodName The name of the method to invoke or
+ * the function invoked per iteration.
+ * @param {...*} [arg] Arguments to invoke the method with.
+ * @returns {Array} Returns a new array of the results of each invoked method.
+ * @example
+ *
+ * _.invoke([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invoke([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+function invoke(collection, methodName) {
+ var args = slice(arguments, 2),
+ index = -1,
+ isFunc = typeof methodName == 'function',
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ result[++index] = (isFunc ? methodName : value[methodName]).apply(value, args);
+ });
+ return result;
+}
+
+module.exports = invoke;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/map.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/map.js b/node_modules/lodash-node/modern/collections/map.js
new file mode 100644
index 0000000..5d1935c
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/map.js
@@ -0,0 +1,70 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Creates an array of values by running each element in the collection
+ * through the callback. The callback is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias collect
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of the results of each `callback` execution.
+ * @example
+ *
+ * _.map([1, 2, 3], function(num) { return num * 3; });
+ * // => [3, 6, 9]
+ *
+ * _.map({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { return num * 3; });
+ * // => [3, 6, 9] (property order is not guaranteed across environments)
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(characters, 'name');
+ * // => ['barney', 'fred']
+ */
+function map(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ callback = createCallback(callback, thisArg, 3);
+ if (typeof length == 'number') {
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = callback(collection[index], index, collection);
+ }
+ } else {
+ result = [];
+ forOwn(collection, function(value, key, collection) {
+ result[++index] = callback(value, key, collection);
+ });
+ }
+ return result;
+}
+
+module.exports = map;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/max.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/max.js b/node_modules/lodash-node/modern/collections/max.js
new file mode 100644
index 0000000..9a8f789
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/max.js
@@ -0,0 +1,91 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var charAtCallback = require('../internals/charAtCallback'),
+ createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString');
+
+/**
+ * Retrieves the maximum value of a collection. If the collection is empty or
+ * falsey `-Infinity` is returned. If a callback is provided it will be executed
+ * for each value in the collection to generate the criterion by which the value
+ * is ranked. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the maximum value.
+ * @example
+ *
+ * _.max([4, 2, 8, 6]);
+ * // => 8
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.max(characters, function(chr) { return chr.age; });
+ * // => { 'name': 'fred', 'age': 40 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.max(characters, 'age');
+ * // => { 'name': 'fred', 'age': 40 };
+ */
+function max(collection, callback, thisArg) {
+ var computed = -Infinity,
+ result = computed;
+
+ // allows working with functions like `_.map` without using
+ // their `index` argument as a callback
+ if (typeof callback != 'function' && thisArg && thisArg[callback] === collection) {
+ callback = null;
+ }
+ if (callback == null && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (value > result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = (callback == null && isString(collection))
+ ? charAtCallback
+ : createCallback(callback, thisArg, 3);
+
+ forEach(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current > computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = max;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/min.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/min.js b/node_modules/lodash-node/modern/collections/min.js
new file mode 100644
index 0000000..22a7c54
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/min.js
@@ -0,0 +1,91 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var charAtCallback = require('../internals/charAtCallback'),
+ createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray'),
+ isString = require('../objects/isString');
+
+/**
+ * Retrieves the minimum value of a collection. If the collection is empty or
+ * falsey `Infinity` is returned. If a callback is provided it will be executed
+ * for each value in the collection to generate the criterion by which the value
+ * is ranked. The callback is bound to `thisArg` and invoked with three
+ * arguments; (value, index, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the minimum value.
+ * @example
+ *
+ * _.min([4, 2, 8, 6]);
+ * // => 2
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.min(characters, function(chr) { return chr.age; });
+ * // => { 'name': 'barney', 'age': 36 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.min(characters, 'age');
+ * // => { 'name': 'barney', 'age': 36 };
+ */
+function min(collection, callback, thisArg) {
+ var computed = Infinity,
+ result = computed;
+
+ // allows working with functions like `_.map` without using
+ // their `index` argument as a callback
+ if (typeof callback != 'function' && thisArg && thisArg[callback] === collection) {
+ callback = null;
+ }
+ if (callback == null && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (value < result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = (callback == null && isString(collection))
+ ? charAtCallback
+ : createCallback(callback, thisArg, 3);
+
+ forEach(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current < computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+}
+
+module.exports = min;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/pluck.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/pluck.js b/node_modules/lodash-node/modern/collections/pluck.js
new file mode 100644
index 0000000..b83a638
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/pluck.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var map = require('./map');
+
+/**
+ * Retrieves the value of a specified property from all elements in the collection.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {string} property The name of the property to pluck.
+ * @returns {Array} Returns a new array of property values.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * _.pluck(characters, 'name');
+ * // => ['barney', 'fred']
+ */
+var pluck = map;
+
+module.exports = pluck;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/reduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/reduce.js b/node_modules/lodash-node/modern/collections/reduce.js
new file mode 100644
index 0000000..6d6c0ea
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/reduce.js
@@ -0,0 +1,67 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn');
+
+/**
+ * Reduces a collection to a value which is the accumulated result of running
+ * each element in the collection through the callback, where each successive
+ * callback execution consumes the return value of the previous execution. If
+ * `accumulator` is not provided the first element of the collection will be
+ * used as the initial `accumulator` value. The callback is bound to `thisArg`
+ * and invoked with four arguments; (accumulator, value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @alias foldl, inject
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] Initial value of the accumulator.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var sum = _.reduce([1, 2, 3], function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * var mapped = _.reduce({ 'a': 1, 'b': 2, 'c': 3 }, function(result, num, key) {
+ * result[key] = num * 3;
+ * return result;
+ * }, {});
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ */
+function reduce(collection, callback, accumulator, thisArg) {
+ if (!collection) return accumulator;
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+
+ var index = -1,
+ length = collection.length;
+
+ if (typeof length == 'number') {
+ if (noaccum) {
+ accumulator = collection[++index];
+ }
+ while (++index < length) {
+ accumulator = callback(accumulator, collection[index], index, collection);
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection)
+ });
+ }
+ return accumulator;
+}
+
+module.exports = reduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/reduceRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/reduceRight.js b/node_modules/lodash-node/modern/collections/reduceRight.js
new file mode 100644
index 0000000..8ac5e24
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/reduceRight.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forEachRight = require('./forEachRight');
+
+/**
+ * This method is like `_.reduce` except that it iterates over elements
+ * of a `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias foldr
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {*} [accumulator] Initial value of the accumulator.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {*} Returns the accumulated value.
+ * @example
+ *
+ * var list = [[0, 1], [2, 3], [4, 5]];
+ * var flat = _.reduceRight(list, function(a, b) { return a.concat(b); }, []);
+ * // => [4, 5, 2, 3, 0, 1]
+ */
+function reduceRight(collection, callback, accumulator, thisArg) {
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+ forEachRight(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection);
+ });
+ return accumulator;
+}
+
+module.exports = reduceRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/reject.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/reject.js b/node_modules/lodash-node/modern/collections/reject.js
new file mode 100644
index 0000000..3abf516
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/reject.js
@@ -0,0 +1,57 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ filter = require('./filter');
+
+/**
+ * The opposite of `_.filter` this method returns the elements of a
+ * collection that the callback does **not** return truey for.
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that failed the callback check.
+ * @example
+ *
+ * var odds = _.reject([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [1, 3, 5]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.reject(characters, 'blocked');
+ * // => [{ 'name': 'barney', 'age': 36, 'blocked': false }]
+ *
+ * // using "_.where" callback shorthand
+ * _.reject(characters, { 'age': 36 });
+ * // => [{ 'name': 'fred', 'age': 40, 'blocked': true }]
+ */
+function reject(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg, 3);
+ return filter(collection, function(value, index, collection) {
+ return !callback(value, index, collection);
+ });
+}
+
+module.exports = reject;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/sample.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/sample.js b/node_modules/lodash-node/modern/collections/sample.js
new file mode 100644
index 0000000..5f0bedc
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/sample.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom'),
+ isString = require('../objects/isString'),
+ shuffle = require('./shuffle'),
+ values = require('../objects/values');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max,
+ nativeMin = Math.min;
+
+/**
+ * Retrieves a random element or `n` random elements from a collection.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to sample.
+ * @param {number} [n] The number of elements to sample.
+ * @param- {Object} [guard] Allows working with functions like `_.map`
+ * without using their `index` arguments as `n`.
+ * @returns {Array} Returns the random sample(s) of `collection`.
+ * @example
+ *
+ * _.sample([1, 2, 3, 4]);
+ * // => 2
+ *
+ * _.sample([1, 2, 3, 4], 2);
+ * // => [3, 1]
+ */
+function sample(collection, n, guard) {
+ if (collection && typeof collection.length != 'number') {
+ collection = values(collection);
+ }
+ if (n == null || guard) {
+ return collection ? collection[baseRandom(0, collection.length - 1)] : undefined;
+ }
+ var result = shuffle(collection);
+ result.length = nativeMin(nativeMax(0, n), result.length);
+ return result;
+}
+
+module.exports = sample;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/shuffle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/shuffle.js b/node_modules/lodash-node/modern/collections/shuffle.js
new file mode 100644
index 0000000..fc3d3c9
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/shuffle.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseRandom = require('../internals/baseRandom'),
+ forEach = require('./forEach');
+
+/**
+ * Creates an array of shuffled values, using a version of the Fisher-Yates
+ * shuffle. See http://en.wikipedia.org/wiki/Fisher-Yates_shuffle.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to shuffle.
+ * @returns {Array} Returns a new shuffled collection.
+ * @example
+ *
+ * _.shuffle([1, 2, 3, 4, 5, 6]);
+ * // => [4, 1, 6, 3, 5, 2]
+ */
+function shuffle(collection) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ var rand = baseRandom(0, ++index);
+ result[index] = result[rand];
+ result[rand] = value;
+ });
+ return result;
+}
+
+module.exports = shuffle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/size.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/size.js b/node_modules/lodash-node/modern/collections/size.js
new file mode 100644
index 0000000..b4b3cf3
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/size.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keys = require('../objects/keys');
+
+/**
+ * Gets the size of the `collection` by returning `collection.length` for arrays
+ * and array-like objects or the number of own enumerable properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to inspect.
+ * @returns {number} Returns `collection.length` or number of own enumerable properties.
+ * @example
+ *
+ * _.size([1, 2]);
+ * // => 2
+ *
+ * _.size({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => 3
+ *
+ * _.size('pebbles');
+ * // => 7
+ */
+function size(collection) {
+ var length = collection ? collection.length : 0;
+ return typeof length == 'number' ? length : keys(collection).length;
+}
+
+module.exports = size;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/some.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/some.js b/node_modules/lodash-node/modern/collections/some.js
new file mode 100644
index 0000000..e635563
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/some.js
@@ -0,0 +1,76 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Checks if the callback returns a truey value for **any** element of a
+ * collection. The function returns as soon as it finds a passing value and
+ * does not iterate over the entire collection. The callback is bound to
+ * `thisArg` and invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias any
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {boolean} Returns `true` if any element passed the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'blocked': false },
+ * { 'name': 'fred', 'age': 40, 'blocked': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.some(characters, 'blocked');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.some(characters, { 'age': 1 });
+ * // => false
+ */
+function some(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ if ((result = callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ forOwn(collection, function(value, index, collection) {
+ return !(result = callback(value, index, collection));
+ });
+ }
+ return !!result;
+}
+
+module.exports = some;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/sortBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/sortBy.js b/node_modules/lodash-node/modern/collections/sortBy.js
new file mode 100644
index 0000000..4e765d3
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/sortBy.js
@@ -0,0 +1,101 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var compareAscending = require('../internals/compareAscending'),
+ createCallback = require('../functions/createCallback'),
+ forEach = require('./forEach'),
+ getArray = require('../internals/getArray'),
+ getObject = require('../internals/getObject'),
+ isArray = require('../objects/isArray'),
+ map = require('./map'),
+ releaseArray = require('../internals/releaseArray'),
+ releaseObject = require('../internals/releaseObject');
+
+/**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in a collection through the callback. This method
+ * performs a stable sort, that is, it will preserve the original sort order
+ * of equal elements. The callback is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is provided for `callback` the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an array of property names is provided for `callback` the collection
+ * will be sorted by each property value.
+ *
+ * If an object is provided for `callback` the created "_.where" style callback
+ * will return `true` for elements that have the properties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Array|Function|Object|string} [callback=identity] The function called
+ * per iteration. If a property name or object is provided it will be used
+ * to create a "_.pluck" or "_.where" style callback, respectively.
+ * @param {*} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of sorted elements.
+ * @example
+ *
+ * _.sortBy([1, 2, 3], function(num) { return Math.sin(num); });
+ * // => [3, 1, 2]
+ *
+ * _.sortBy([1, 2, 3], function(num) { return this.sin(num); }, Math);
+ * // => [3, 1, 2]
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 },
+ * { 'name': 'barney', 'age': 26 },
+ * { 'name': 'fred', 'age': 30 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(_.sortBy(characters, 'age'), _.values);
+ * // => [['barney', 26], ['fred', 30], ['barney', 36], ['fred', 40]]
+ *
+ * // sorting by multiple properties
+ * _.map(_.sortBy(characters, ['name', 'age']), _.values);
+ * // = > [['barney', 26], ['barney', 36], ['fred', 30], ['fred', 40]]
+ */
+function sortBy(collection, callback, thisArg) {
+ var index = -1,
+ isArr = isArray(callback),
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ if (!isArr) {
+ callback = createCallback(callback, thisArg, 3);
+ }
+ forEach(collection, function(value, key, collection) {
+ var object = result[++index] = getObject();
+ if (isArr) {
+ object.criteria = map(callback, function(key) { return value[key]; });
+ } else {
+ (object.criteria = getArray())[0] = callback(value, key, collection);
+ }
+ object.index = index;
+ object.value = value;
+ });
+
+ length = result.length;
+ result.sort(compareAscending);
+ while (length--) {
+ var object = result[length];
+ result[length] = object.value;
+ if (!isArr) {
+ releaseArray(object.criteria);
+ }
+ releaseObject(object);
+ }
+ return result;
+}
+
+module.exports = sortBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/toArray.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/toArray.js b/node_modules/lodash-node/modern/collections/toArray.js
new file mode 100644
index 0000000..24c1f52
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/toArray.js
@@ -0,0 +1,33 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isString = require('../objects/isString'),
+ slice = require('../internals/slice'),
+ values = require('../objects/values');
+
+/**
+ * Converts the `collection` to an array.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to convert.
+ * @returns {Array} Returns the new converted array.
+ * @example
+ *
+ * (function() { return _.toArray(arguments).slice(1); })(1, 2, 3, 4);
+ * // => [2, 3, 4]
+ */
+function toArray(collection) {
+ if (collection && typeof collection.length == 'number') {
+ return slice(collection);
+ }
+ return values(collection);
+}
+
+module.exports = toArray;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/collections/where.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/collections/where.js b/node_modules/lodash-node/modern/collections/where.js
new file mode 100644
index 0000000..6f8fb6f
--- /dev/null
+++ b/node_modules/lodash-node/modern/collections/where.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var filter = require('./filter');
+
+/**
+ * Performs a deep comparison of each element in a `collection` to the given
+ * `properties` object, returning an array of all elements that have equivalent
+ * property values.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Object} props The object of property values to filter by.
+ * @returns {Array} Returns a new array of elements that have the given properties.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36, 'pets': ['hoppy'] },
+ * { 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }
+ * ];
+ *
+ * _.where(characters, { 'age': 36 });
+ * // => [{ 'name': 'barney', 'age': 36, 'pets': ['hoppy'] }]
+ *
+ * _.where(characters, { 'pets': ['dino'] });
+ * // => [{ 'name': 'fred', 'age': 40, 'pets': ['baby puss', 'dino'] }]
+ */
+var where = filter;
+
+module.exports = where;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions.js b/node_modules/lodash-node/modern/functions.js
new file mode 100644
index 0000000..f50894d
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions.js
@@ -0,0 +1,27 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+module.exports = {
+ 'after': require('./functions/after'),
+ 'bind': require('./functions/bind'),
+ 'bindAll': require('./functions/bindAll'),
+ 'bindKey': require('./functions/bindKey'),
+ 'compose': require('./functions/compose'),
+ 'createCallback': require('./functions/createCallback'),
+ 'curry': require('./functions/curry'),
+ 'debounce': require('./functions/debounce'),
+ 'defer': require('./functions/defer'),
+ 'delay': require('./functions/delay'),
+ 'memoize': require('./functions/memoize'),
+ 'once': require('./functions/once'),
+ 'partial': require('./functions/partial'),
+ 'partialRight': require('./functions/partialRight'),
+ 'throttle': require('./functions/throttle'),
+ 'wrap': require('./functions/wrap')
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/after.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/after.js b/node_modules/lodash-node/modern/functions/after.js
new file mode 100644
index 0000000..cbff273
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/after.js
@@ -0,0 +1,46 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that executes `func`, with the `this` binding and
+ * arguments of the created function, only after being called `n` times.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {number} n The number of times the function must be called before
+ * `func` is executed.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var saves = ['profile', 'settings'];
+ *
+ * var done = _.after(saves.length, function() {
+ * console.log('Done saving!');
+ * });
+ *
+ * _.forEach(saves, function(type) {
+ * asyncSave({ 'type': type, 'complete': done });
+ * });
+ * // => logs 'Done saving!', after all saves have completed
+ */
+function after(n, func) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+}
+
+module.exports = after;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/bind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/bind.js b/node_modules/lodash-node/modern/functions/bind.js
new file mode 100644
index 0000000..f150cbc
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/bind.js
@@ -0,0 +1,40 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes `func` with the `this`
+ * binding of `thisArg` and prepends any additional `bind` arguments to those
+ * provided to the bound function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to bind.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var func = function(greeting) {
+ * return greeting + ' ' + this.name;
+ * };
+ *
+ * func = _.bind(func, { 'name': 'fred' }, 'hi');
+ * func();
+ * // => 'hi fred'
+ */
+function bind(func, thisArg) {
+ return arguments.length > 2
+ ? createWrapper(func, 17, slice(arguments, 2), null, thisArg)
+ : createWrapper(func, 1, null, null, thisArg);
+}
+
+module.exports = bind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/bindAll.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/bindAll.js b/node_modules/lodash-node/modern/functions/bindAll.js
new file mode 100644
index 0000000..8d20be5
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/bindAll.js
@@ -0,0 +1,49 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseFlatten = require('../internals/baseFlatten'),
+ createWrapper = require('../internals/createWrapper'),
+ functions = require('../objects/functions');
+
+/**
+ * Binds methods of an object to the object itself, overwriting the existing
+ * method. Method names may be specified as individual arguments or as arrays
+ * of method names. If no method names are provided all the function properties
+ * of `object` will be bound.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object to bind and assign the bound methods to.
+ * @param {...string} [methodName] The object method names to
+ * bind, specified as individual method names or arrays of method names.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'onClick': function() { console.log('clicked ' + this.label); }
+ * };
+ *
+ * _.bindAll(view);
+ * jQuery('#docs').on('click', view.onClick);
+ * // => logs 'clicked docs', when the button is clicked
+ */
+function bindAll(object) {
+ var funcs = arguments.length > 1 ? baseFlatten(arguments, true, false, 1) : functions(object),
+ index = -1,
+ length = funcs.length;
+
+ while (++index < length) {
+ var key = funcs[index];
+ object[key] = createWrapper(object[key], 1, null, null, object);
+ }
+ return object;
+}
+
+module.exports = bindAll;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/bindKey.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/bindKey.js b/node_modules/lodash-node/modern/functions/bindKey.js
new file mode 100644
index 0000000..eb31c2f
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/bindKey.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes the method at `object[key]`
+ * and prepends any additional `bindKey` arguments to those provided to the bound
+ * function. This method differs from `_.bind` by allowing bound functions to
+ * reference methods that will be redefined or don't yet exist.
+ * See http://michaux.ca/articles/lazy-function-definition-pattern.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object the method belongs to.
+ * @param {string} key The key of the method.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var object = {
+ * 'name': 'fred',
+ * 'greet': function(greeting) {
+ * return greeting + ' ' + this.name;
+ * }
+ * };
+ *
+ * var func = _.bindKey(object, 'greet', 'hi');
+ * func();
+ * // => 'hi fred'
+ *
+ * object.greet = function(greeting) {
+ * return greeting + 'ya ' + this.name + '!';
+ * };
+ *
+ * func();
+ * // => 'hiya fred!'
+ */
+function bindKey(object, key) {
+ return arguments.length > 2
+ ? createWrapper(key, 19, slice(arguments, 2), null, object)
+ : createWrapper(key, 3, null, null, object);
+}
+
+module.exports = bindKey;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/compose.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/compose.js b/node_modules/lodash-node/modern/functions/compose.js
new file mode 100644
index 0000000..d0af883
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/compose.js
@@ -0,0 +1,61 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that is the composition of the provided functions,
+ * where each function consumes the return value of the function that follows.
+ * For example, composing the functions `f()`, `g()`, and `h()` produces `f(g(h()))`.
+ * Each function is executed with the `this` binding of the composed function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {...Function} [func] Functions to compose.
+ * @returns {Function} Returns the new composed function.
+ * @example
+ *
+ * var realNameMap = {
+ * 'pebbles': 'penelope'
+ * };
+ *
+ * var format = function(name) {
+ * name = realNameMap[name.toLowerCase()] || name;
+ * return name.charAt(0).toUpperCase() + name.slice(1).toLowerCase();
+ * };
+ *
+ * var greet = function(formatted) {
+ * return 'Hiya ' + formatted + '!';
+ * };
+ *
+ * var welcome = _.compose(greet, format);
+ * welcome('pebbles');
+ * // => 'Hiya Penelope!'
+ */
+function compose() {
+ var funcs = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ if (!isFunction(funcs[length])) {
+ throw new TypeError;
+ }
+ }
+ return function() {
+ var args = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ args = [funcs[length].apply(this, args)];
+ }
+ return args[0];
+ };
+}
+
+module.exports = compose;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[17/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/pegjs/lib/peg.js
----------------------------------------------------------------------
diff --git a/node_modules/pegjs/lib/peg.js b/node_modules/pegjs/lib/peg.js
new file mode 100644
index 0000000..94c9423
--- /dev/null
+++ b/node_modules/pegjs/lib/peg.js
@@ -0,0 +1,5141 @@
+/* PEG.js 0.6.2 (http://pegjs.majda.cz/) */
+
+(function() {
+
+var undefined;
+
+var PEG = {
+ /* PEG.js version. */
+ VERSION: "0.6.2",
+
+ /*
+ * Generates a parser from a specified grammar and returns it.
+ *
+ * The grammar must be a string in the format described by the metagramar in
+ * the parser.pegjs file.
+ *
+ * Throws |PEG.parser.SyntaxError| if the grammar contains a syntax error or
+ * |PEG.GrammarError| if it contains a semantic error. Note that not all
+ * errors are detected during the generation and some may protrude to the
+ * generated parser and cause its malfunction.
+ */
+ buildParser: function(grammar) {
+ return PEG.compiler.compile(PEG.parser.parse(grammar));
+ }
+};
+
+/* Thrown when the grammar contains an error. */
+
+PEG.GrammarError = function(message) {
+ this.name = "PEG.GrammarError";
+ this.message = message;
+};
+
+PEG.GrammarError.prototype = Error.prototype;
+
+function contains(array, value) {
+ /*
+ * Stupid IE does not have Array.prototype.indexOf, otherwise this function
+ * would be a one-liner.
+ */
+ var length = array.length;
+ for (var i = 0; i < length; i++) {
+ if (array[i] === value) {
+ return true;
+ }
+ }
+ return false;
+}
+
+function each(array, callback) {
+ var length = array.length;
+ for (var i = 0; i < length; i++) {
+ callback(array[i]);
+ }
+}
+
+function map(array, callback) {
+ var result = [];
+ var length = array.length;
+ for (var i = 0; i < length; i++) {
+ result[i] = callback(array[i]);
+ }
+ return result;
+}
+
+/*
+ * Returns a string padded on the left to a desired length with a character.
+ *
+ * The code needs to be in sync with th code template in the compilation
+ * function for "action" nodes.
+ */
+function padLeft(input, padding, length) {
+ var result = input;
+
+ var padLength = length - input.length;
+ for (var i = 0; i < padLength; i++) {
+ result = padding + result;
+ }
+
+ return result;
+}
+
+/*
+ * Returns an escape sequence for given character. Uses \x for characters <=
+ * 0xFF to save space, \u for the rest.
+ *
+ * The code needs to be in sync with th code template in the compilation
+ * function for "action" nodes.
+ */
+function escape(ch) {
+ var charCode = ch.charCodeAt(0);
+
+ if (charCode <= 0xFF) {
+ var escapeChar = 'x';
+ var length = 2;
+ } else {
+ var escapeChar = 'u';
+ var length = 4;
+ }
+
+ return '\\' + escapeChar + padLeft(charCode.toString(16).toUpperCase(), '0', length);
+}
+
+/*
+ * Surrounds the string with quotes and escapes characters inside so that the
+ * result is a valid JavaScript string.
+ *
+ * The code needs to be in sync with th code template in the compilation
+ * function for "action" nodes.
+ */
+function quote(s) {
+ /*
+ * ECMA-262, 5th ed., 7.8.4: All characters may appear literally in a string
+ * literal except for the closing quote character, backslash, carriage return,
+ * line separator, paragraph separator, and line feed. Any character may
+ * appear in the form of an escape sequence.
+ *
+ * For portability, we also escape escape all non-ASCII characters.
+ */
+ return '"' + s
+ .replace(/\\/g, '\\\\') // backslash
+ .replace(/"/g, '\\"') // closing quote character
+ .replace(/\r/g, '\\r') // carriage return
+ .replace(/\n/g, '\\n') // line feed
+ .replace(/[\x80-\uFFFF]/g, escape) // non-ASCII characters
+ + '"';
+};
+
+/*
+ * Escapes characters inside the string so that it can be used as a list of
+ * characters in a character class of a regular expression.
+ */
+function quoteForRegexpClass(s) {
+ /*
+ * Based on ECMA-262, 5th ed., 7.8.5 & 15.10.1.
+ *
+ * For portability, we also escape escape all non-ASCII characters.
+ */
+ return s
+ .replace(/\\/g, '\\\\') // backslash
+ .replace(/\0/g, '\\0') // null, IE needs this
+ .replace(/\//g, '\\/') // closing slash
+ .replace(/]/g, '\\]') // closing bracket
+ .replace(/-/g, '\\-') // dash
+ .replace(/\r/g, '\\r') // carriage return
+ .replace(/\n/g, '\\n') // line feed
+ .replace(/[\x80-\uFFFF]/g, escape) // non-ASCII characters
+}
+
+/*
+ * Builds a node visitor -- a function which takes a node and any number of
+ * other parameters, calls an appropriate function according to the node type,
+ * passes it all its parameters and returns its value. The functions for various
+ * node types are passed in a parameter to |buildNodeVisitor| as a hash.
+ */
+function buildNodeVisitor(functions) {
+ return function(node) {
+ return functions[node.type].apply(null, arguments);
+ }
+}
+PEG.parser = (function(){
+ /* Generated by PEG.js 0.6.2 (http://pegjs.majda.cz/). */
+
+ var result = {
+ /*
+ * Parses the input with a generated parser. If the parsing is successfull,
+ * returns a value explicitly or implicitly specified by the grammar from
+ * which the parser was generated (see |PEG.buildParser|). If the parsing is
+ * unsuccessful, throws |PEG.parser.SyntaxError| describing the error.
+ */
+ parse: function(input, startRule) {
+ var parseFunctions = {
+ "__": parse___,
+ "action": parse_action,
+ "and": parse_and,
+ "braced": parse_braced,
+ "bracketDelimitedCharacter": parse_bracketDelimitedCharacter,
+ "choice": parse_choice,
+ "class": parse_class,
+ "classCharacter": parse_classCharacter,
+ "classCharacterRange": parse_classCharacterRange,
+ "colon": parse_colon,
+ "comment": parse_comment,
+ "digit": parse_digit,
+ "dot": parse_dot,
+ "doubleQuotedCharacter": parse_doubleQuotedCharacter,
+ "doubleQuotedLiteral": parse_doubleQuotedLiteral,
+ "eol": parse_eol,
+ "eolChar": parse_eolChar,
+ "eolEscapeSequence": parse_eolEscapeSequence,
+ "equals": parse_equals,
+ "grammar": parse_grammar,
+ "hexDigit": parse_hexDigit,
+ "hexEscapeSequence": parse_hexEscapeSequence,
+ "identifier": parse_identifier,
+ "initializer": parse_initializer,
+ "labeled": parse_labeled,
+ "letter": parse_letter,
+ "literal": parse_literal,
+ "lowerCaseLetter": parse_lowerCaseLetter,
+ "lparen": parse_lparen,
+ "multiLineComment": parse_multiLineComment,
+ "nonBraceCharacter": parse_nonBraceCharacter,
+ "nonBraceCharacters": parse_nonBraceCharacters,
+ "not": parse_not,
+ "plus": parse_plus,
+ "prefixed": parse_prefixed,
+ "primary": parse_primary,
+ "question": parse_question,
+ "rparen": parse_rparen,
+ "rule": parse_rule,
+ "semicolon": parse_semicolon,
+ "sequence": parse_sequence,
+ "simpleBracketDelimitedCharacter": parse_simpleBracketDelimitedCharacter,
+ "simpleDoubleQuotedCharacter": parse_simpleDoubleQuotedCharacter,
+ "simpleEscapeSequence": parse_simpleEscapeSequence,
+ "simpleSingleQuotedCharacter": parse_simpleSingleQuotedCharacter,
+ "singleLineComment": parse_singleLineComment,
+ "singleQuotedCharacter": parse_singleQuotedCharacter,
+ "singleQuotedLiteral": parse_singleQuotedLiteral,
+ "slash": parse_slash,
+ "star": parse_star,
+ "suffixed": parse_suffixed,
+ "unicodeEscapeSequence": parse_unicodeEscapeSequence,
+ "upperCaseLetter": parse_upperCaseLetter,
+ "whitespace": parse_whitespace,
+ "zeroEscapeSequence": parse_zeroEscapeSequence
+ };
+
+ if (startRule !== undefined) {
+ if (parseFunctions[startRule] === undefined) {
+ throw new Error("Invalid rule name: " + quote(startRule) + ".");
+ }
+ } else {
+ startRule = "grammar";
+ }
+
+ var pos = 0;
+ var reportMatchFailures = true;
+ var rightmostMatchFailuresPos = 0;
+ var rightmostMatchFailuresExpected = [];
+ var cache = {};
+
+ function padLeft(input, padding, length) {
+ var result = input;
+
+ var padLength = length - input.length;
+ for (var i = 0; i < padLength; i++) {
+ result = padding + result;
+ }
+
+ return result;
+ }
+
+ function escape(ch) {
+ var charCode = ch.charCodeAt(0);
+
+ if (charCode <= 0xFF) {
+ var escapeChar = 'x';
+ var length = 2;
+ } else {
+ var escapeChar = 'u';
+ var length = 4;
+ }
+
+ return '\\' + escapeChar + padLeft(charCode.toString(16).toUpperCase(), '0', length);
+ }
+
+ function quote(s) {
+ /*
+ * ECMA-262, 5th ed., 7.8.4: All characters may appear literally in a
+ * string literal except for the closing quote character, backslash,
+ * carriage return, line separator, paragraph separator, and line feed.
+ * Any character may appear in the form of an escape sequence.
+ */
+ return '"' + s
+ .replace(/\\/g, '\\\\') // backslash
+ .replace(/"/g, '\\"') // closing quote character
+ .replace(/\r/g, '\\r') // carriage return
+ .replace(/\n/g, '\\n') // line feed
+ .replace(/[\x80-\uFFFF]/g, escape) // non-ASCII characters
+ + '"';
+ }
+
+ function matchFailed(failure) {
+ if (pos < rightmostMatchFailuresPos) {
+ return;
+ }
+
+ if (pos > rightmostMatchFailuresPos) {
+ rightmostMatchFailuresPos = pos;
+ rightmostMatchFailuresExpected = [];
+ }
+
+ rightmostMatchFailuresExpected.push(failure);
+ }
+
+ function parse_grammar() {
+ var cacheKey = 'grammar@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result3 = parse___();
+ if (result3 !== null) {
+ var result7 = parse_initializer();
+ var result4 = result7 !== null ? result7 : '';
+ if (result4 !== null) {
+ var result6 = parse_rule();
+ if (result6 !== null) {
+ var result5 = [];
+ while (result6 !== null) {
+ result5.push(result6);
+ var result6 = parse_rule();
+ }
+ } else {
+ var result5 = null;
+ }
+ if (result5 !== null) {
+ var result1 = [result3, result4, result5];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(initializer, rules) {
+ var rulesConverted = {};
+ each(rules, function(rule) { rulesConverted[rule.name] = rule; });
+
+ return {
+ type: "grammar",
+ initializer: initializer !== "" ? initializer : null,
+ rules: rulesConverted,
+ startRule: rules[0].name
+ }
+ })(result1[1], result1[2])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_initializer() {
+ var cacheKey = 'initializer@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result3 = parse_action();
+ if (result3 !== null) {
+ var result5 = parse_semicolon();
+ var result4 = result5 !== null ? result5 : '';
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(code) {
+ return {
+ type: "initializer",
+ code: code
+ };
+ })(result1[0])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_rule() {
+ var cacheKey = 'rule@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result3 = parse_identifier();
+ if (result3 !== null) {
+ var result10 = parse_literal();
+ if (result10 !== null) {
+ var result4 = result10;
+ } else {
+ if (input.substr(pos, 0) === "") {
+ var result9 = "";
+ pos += 0;
+ } else {
+ var result9 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\"");
+ }
+ }
+ if (result9 !== null) {
+ var result4 = result9;
+ } else {
+ var result4 = null;;
+ };
+ }
+ if (result4 !== null) {
+ var result5 = parse_equals();
+ if (result5 !== null) {
+ var result6 = parse_choice();
+ if (result6 !== null) {
+ var result8 = parse_semicolon();
+ var result7 = result8 !== null ? result8 : '';
+ if (result7 !== null) {
+ var result1 = [result3, result4, result5, result6, result7];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(name, displayName, expression) {
+ return {
+ type: "rule",
+ name: name,
+ displayName: displayName !== "" ? displayName : null,
+ expression: expression
+ };
+ })(result1[0], result1[1], result1[3])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_choice() {
+ var cacheKey = 'choice@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result3 = parse_sequence();
+ if (result3 !== null) {
+ var result4 = [];
+ var savedPos2 = pos;
+ var result6 = parse_slash();
+ if (result6 !== null) {
+ var result7 = parse_sequence();
+ if (result7 !== null) {
+ var result5 = [result6, result7];
+ } else {
+ var result5 = null;
+ pos = savedPos2;
+ }
+ } else {
+ var result5 = null;
+ pos = savedPos2;
+ }
+ while (result5 !== null) {
+ result4.push(result5);
+ var savedPos2 = pos;
+ var result6 = parse_slash();
+ if (result6 !== null) {
+ var result7 = parse_sequence();
+ if (result7 !== null) {
+ var result5 = [result6, result7];
+ } else {
+ var result5 = null;
+ pos = savedPos2;
+ }
+ } else {
+ var result5 = null;
+ pos = savedPos2;
+ }
+ }
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(head, tail) {
+ if (tail.length > 0) {
+ var alternatives = [head].concat(map(
+ tail,
+ function(element) { return element[1]; }
+ ));
+ return {
+ type: "choice",
+ alternatives: alternatives
+ }
+ } else {
+ return head;
+ }
+ })(result1[0], result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_sequence() {
+ var cacheKey = 'sequence@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos1 = pos;
+ var savedPos2 = pos;
+ var result8 = [];
+ var result10 = parse_labeled();
+ while (result10 !== null) {
+ result8.push(result10);
+ var result10 = parse_labeled();
+ }
+ if (result8 !== null) {
+ var result9 = parse_action();
+ if (result9 !== null) {
+ var result6 = [result8, result9];
+ } else {
+ var result6 = null;
+ pos = savedPos2;
+ }
+ } else {
+ var result6 = null;
+ pos = savedPos2;
+ }
+ var result7 = result6 !== null
+ ? (function(elements, code) {
+ var expression = elements.length != 1
+ ? {
+ type: "sequence",
+ elements: elements
+ }
+ : elements[0];
+ return {
+ type: "action",
+ expression: expression,
+ code: code
+ };
+ })(result6[0], result6[1])
+ : null;
+ if (result7 !== null) {
+ var result5 = result7;
+ } else {
+ var result5 = null;
+ pos = savedPos1;
+ }
+ if (result5 !== null) {
+ var result0 = result5;
+ } else {
+ var savedPos0 = pos;
+ var result2 = [];
+ var result4 = parse_labeled();
+ while (result4 !== null) {
+ result2.push(result4);
+ var result4 = parse_labeled();
+ }
+ var result3 = result2 !== null
+ ? (function(elements) {
+ return elements.length != 1
+ ? {
+ type: "sequence",
+ elements: elements
+ }
+ : elements[0];
+ })(result2)
+ : null;
+ if (result3 !== null) {
+ var result1 = result3;
+ } else {
+ var result1 = null;
+ pos = savedPos0;
+ }
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_labeled() {
+ var cacheKey = 'labeled@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result5 = parse_identifier();
+ if (result5 !== null) {
+ var result6 = parse_colon();
+ if (result6 !== null) {
+ var result7 = parse_prefixed();
+ if (result7 !== null) {
+ var result3 = [result5, result6, result7];
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ var result4 = result3 !== null
+ ? (function(label, expression) {
+ return {
+ type: "labeled",
+ label: label,
+ expression: expression
+ };
+ })(result3[0], result3[2])
+ : null;
+ if (result4 !== null) {
+ var result2 = result4;
+ } else {
+ var result2 = null;
+ pos = savedPos0;
+ }
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result1 = parse_prefixed();
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_prefixed() {
+ var cacheKey = 'prefixed@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos6 = pos;
+ var savedPos7 = pos;
+ var result20 = parse_and();
+ if (result20 !== null) {
+ var result21 = parse_action();
+ if (result21 !== null) {
+ var result18 = [result20, result21];
+ } else {
+ var result18 = null;
+ pos = savedPos7;
+ }
+ } else {
+ var result18 = null;
+ pos = savedPos7;
+ }
+ var result19 = result18 !== null
+ ? (function(code) {
+ return {
+ type: "semantic_and",
+ code: code
+ };
+ })(result18[1])
+ : null;
+ if (result19 !== null) {
+ var result17 = result19;
+ } else {
+ var result17 = null;
+ pos = savedPos6;
+ }
+ if (result17 !== null) {
+ var result0 = result17;
+ } else {
+ var savedPos4 = pos;
+ var savedPos5 = pos;
+ var result15 = parse_and();
+ if (result15 !== null) {
+ var result16 = parse_suffixed();
+ if (result16 !== null) {
+ var result13 = [result15, result16];
+ } else {
+ var result13 = null;
+ pos = savedPos5;
+ }
+ } else {
+ var result13 = null;
+ pos = savedPos5;
+ }
+ var result14 = result13 !== null
+ ? (function(expression) {
+ return {
+ type: "simple_and",
+ expression: expression
+ };
+ })(result13[1])
+ : null;
+ if (result14 !== null) {
+ var result12 = result14;
+ } else {
+ var result12 = null;
+ pos = savedPos4;
+ }
+ if (result12 !== null) {
+ var result0 = result12;
+ } else {
+ var savedPos2 = pos;
+ var savedPos3 = pos;
+ var result10 = parse_not();
+ if (result10 !== null) {
+ var result11 = parse_action();
+ if (result11 !== null) {
+ var result8 = [result10, result11];
+ } else {
+ var result8 = null;
+ pos = savedPos3;
+ }
+ } else {
+ var result8 = null;
+ pos = savedPos3;
+ }
+ var result9 = result8 !== null
+ ? (function(code) {
+ return {
+ type: "semantic_not",
+ code: code
+ };
+ })(result8[1])
+ : null;
+ if (result9 !== null) {
+ var result7 = result9;
+ } else {
+ var result7 = null;
+ pos = savedPos2;
+ }
+ if (result7 !== null) {
+ var result0 = result7;
+ } else {
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result5 = parse_not();
+ if (result5 !== null) {
+ var result6 = parse_suffixed();
+ if (result6 !== null) {
+ var result3 = [result5, result6];
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ var result4 = result3 !== null
+ ? (function(expression) {
+ return {
+ type: "simple_not",
+ expression: expression
+ };
+ })(result3[1])
+ : null;
+ if (result4 !== null) {
+ var result2 = result4;
+ } else {
+ var result2 = null;
+ pos = savedPos0;
+ }
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result1 = parse_suffixed();
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ };
+ };
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_suffixed() {
+ var cacheKey = 'suffixed@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos4 = pos;
+ var savedPos5 = pos;
+ var result15 = parse_primary();
+ if (result15 !== null) {
+ var result16 = parse_question();
+ if (result16 !== null) {
+ var result13 = [result15, result16];
+ } else {
+ var result13 = null;
+ pos = savedPos5;
+ }
+ } else {
+ var result13 = null;
+ pos = savedPos5;
+ }
+ var result14 = result13 !== null
+ ? (function(expression) {
+ return {
+ type: "optional",
+ expression: expression
+ };
+ })(result13[0])
+ : null;
+ if (result14 !== null) {
+ var result12 = result14;
+ } else {
+ var result12 = null;
+ pos = savedPos4;
+ }
+ if (result12 !== null) {
+ var result0 = result12;
+ } else {
+ var savedPos2 = pos;
+ var savedPos3 = pos;
+ var result10 = parse_primary();
+ if (result10 !== null) {
+ var result11 = parse_star();
+ if (result11 !== null) {
+ var result8 = [result10, result11];
+ } else {
+ var result8 = null;
+ pos = savedPos3;
+ }
+ } else {
+ var result8 = null;
+ pos = savedPos3;
+ }
+ var result9 = result8 !== null
+ ? (function(expression) {
+ return {
+ type: "zero_or_more",
+ expression: expression
+ };
+ })(result8[0])
+ : null;
+ if (result9 !== null) {
+ var result7 = result9;
+ } else {
+ var result7 = null;
+ pos = savedPos2;
+ }
+ if (result7 !== null) {
+ var result0 = result7;
+ } else {
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result5 = parse_primary();
+ if (result5 !== null) {
+ var result6 = parse_plus();
+ if (result6 !== null) {
+ var result3 = [result5, result6];
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result3 = null;
+ pos = savedPos1;
+ }
+ var result4 = result3 !== null
+ ? (function(expression) {
+ return {
+ type: "one_or_more",
+ expression: expression
+ };
+ })(result3[0])
+ : null;
+ if (result4 !== null) {
+ var result2 = result4;
+ } else {
+ var result2 = null;
+ pos = savedPos0;
+ }
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result1 = parse_primary();
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ };
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_primary() {
+ var cacheKey = 'primary@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos4 = pos;
+ var savedPos5 = pos;
+ var result17 = parse_identifier();
+ if (result17 !== null) {
+ var savedPos6 = pos;
+ var savedReportMatchFailuresVar0 = reportMatchFailures;
+ reportMatchFailures = false;
+ var savedPos7 = pos;
+ var result23 = parse_literal();
+ if (result23 !== null) {
+ var result20 = result23;
+ } else {
+ if (input.substr(pos, 0) === "") {
+ var result22 = "";
+ pos += 0;
+ } else {
+ var result22 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\"");
+ }
+ }
+ if (result22 !== null) {
+ var result20 = result22;
+ } else {
+ var result20 = null;;
+ };
+ }
+ if (result20 !== null) {
+ var result21 = parse_equals();
+ if (result21 !== null) {
+ var result19 = [result20, result21];
+ } else {
+ var result19 = null;
+ pos = savedPos7;
+ }
+ } else {
+ var result19 = null;
+ pos = savedPos7;
+ }
+ reportMatchFailures = savedReportMatchFailuresVar0;
+ if (result19 === null) {
+ var result18 = '';
+ } else {
+ var result18 = null;
+ pos = savedPos6;
+ }
+ if (result18 !== null) {
+ var result15 = [result17, result18];
+ } else {
+ var result15 = null;
+ pos = savedPos5;
+ }
+ } else {
+ var result15 = null;
+ pos = savedPos5;
+ }
+ var result16 = result15 !== null
+ ? (function(name) {
+ return {
+ type: "rule_ref",
+ name: name
+ };
+ })(result15[0])
+ : null;
+ if (result16 !== null) {
+ var result14 = result16;
+ } else {
+ var result14 = null;
+ pos = savedPos4;
+ }
+ if (result14 !== null) {
+ var result0 = result14;
+ } else {
+ var savedPos3 = pos;
+ var result12 = parse_literal();
+ var result13 = result12 !== null
+ ? (function(value) {
+ return {
+ type: "literal",
+ value: value
+ };
+ })(result12)
+ : null;
+ if (result13 !== null) {
+ var result11 = result13;
+ } else {
+ var result11 = null;
+ pos = savedPos3;
+ }
+ if (result11 !== null) {
+ var result0 = result11;
+ } else {
+ var savedPos2 = pos;
+ var result9 = parse_dot();
+ var result10 = result9 !== null
+ ? (function() { return { type: "any" }; })()
+ : null;
+ if (result10 !== null) {
+ var result8 = result10;
+ } else {
+ var result8 = null;
+ pos = savedPos2;
+ }
+ if (result8 !== null) {
+ var result0 = result8;
+ } else {
+ var result7 = parse_class();
+ if (result7 !== null) {
+ var result0 = result7;
+ } else {
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result4 = parse_lparen();
+ if (result4 !== null) {
+ var result5 = parse_choice();
+ if (result5 !== null) {
+ var result6 = parse_rparen();
+ if (result6 !== null) {
+ var result2 = [result4, result5, result6];
+ } else {
+ var result2 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result2 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result2 = null;
+ pos = savedPos1;
+ }
+ var result3 = result2 !== null
+ ? (function(expression) { return expression; })(result2[1])
+ : null;
+ if (result3 !== null) {
+ var result1 = result3;
+ } else {
+ var result1 = null;
+ pos = savedPos0;
+ }
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ };
+ };
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_action() {
+ var cacheKey = 'action@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+ var savedReportMatchFailures = reportMatchFailures;
+ reportMatchFailures = false;
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result3 = parse_braced();
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(braced) { return braced.substr(1, braced.length - 2); })(result1[0])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+ reportMatchFailures = savedReportMatchFailures;
+ if (reportMatchFailures && result0 === null) {
+ matchFailed("action");
+ }
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_braced() {
+ var cacheKey = 'braced@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "{") {
+ var result3 = "{";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"{\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = [];
+ var result8 = parse_braced();
+ if (result8 !== null) {
+ var result6 = result8;
+ } else {
+ var result7 = parse_nonBraceCharacter();
+ if (result7 !== null) {
+ var result6 = result7;
+ } else {
+ var result6 = null;;
+ };
+ }
+ while (result6 !== null) {
+ result4.push(result6);
+ var result8 = parse_braced();
+ if (result8 !== null) {
+ var result6 = result8;
+ } else {
+ var result7 = parse_nonBraceCharacter();
+ if (result7 !== null) {
+ var result6 = result7;
+ } else {
+ var result6 = null;;
+ };
+ }
+ }
+ if (result4 !== null) {
+ if (input.substr(pos, 1) === "}") {
+ var result5 = "}";
+ pos += 1;
+ } else {
+ var result5 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"}\"");
+ }
+ }
+ if (result5 !== null) {
+ var result1 = [result3, result4, result5];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(parts) {
+ return "{" + parts.join("") + "}";
+ })(result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_nonBraceCharacters() {
+ var cacheKey = 'nonBraceCharacters@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var result3 = parse_nonBraceCharacter();
+ if (result3 !== null) {
+ var result1 = [];
+ while (result3 !== null) {
+ result1.push(result3);
+ var result3 = parse_nonBraceCharacter();
+ }
+ } else {
+ var result1 = null;
+ }
+ var result2 = result1 !== null
+ ? (function(chars) { return chars.join(""); })(result1)
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_nonBraceCharacter() {
+ var cacheKey = 'nonBraceCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ if (input.substr(pos).match(/^[^{}]/) !== null) {
+ var result0 = input.charAt(pos);
+ pos++;
+ } else {
+ var result0 = null;
+ if (reportMatchFailures) {
+ matchFailed("[^{}]");
+ }
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_equals() {
+ var cacheKey = 'equals@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "=") {
+ var result3 = "=";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"=\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "="; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_colon() {
+ var cacheKey = 'colon@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === ":") {
+ var result3 = ":";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\":\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return ":"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_semicolon() {
+ var cacheKey = 'semicolon@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === ";") {
+ var result3 = ";";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\";\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return ";"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_slash() {
+ var cacheKey = 'slash@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "/") {
+ var result3 = "/";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"/\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "/"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_and() {
+ var cacheKey = 'and@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "&") {
+ var result3 = "&";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"&\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "&"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_not() {
+ var cacheKey = 'not@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "!") {
+ var result3 = "!";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"!\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "!"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_question() {
+ var cacheKey = 'question@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "?") {
+ var result3 = "?";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"?\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "?"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_star() {
+ var cacheKey = 'star@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "*") {
+ var result3 = "*";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"*\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "*"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_plus() {
+ var cacheKey = 'plus@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "+") {
+ var result3 = "+";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"+\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "+"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_lparen() {
+ var cacheKey = 'lparen@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "(") {
+ var result3 = "(";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"(\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "("; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_rparen() {
+ var cacheKey = 'rparen@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === ")") {
+ var result3 = ")";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\")\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return ")"; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_dot() {
+ var cacheKey = 'dot@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === ".") {
+ var result3 = ".";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\".\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function() { return "."; })()
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_identifier() {
+ var cacheKey = 'identifier@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+ var savedReportMatchFailures = reportMatchFailures;
+ reportMatchFailures = false;
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result13 = parse_letter();
+ if (result13 !== null) {
+ var result3 = result13;
+ } else {
+ if (input.substr(pos, 1) === "_") {
+ var result12 = "_";
+ pos += 1;
+ } else {
+ var result12 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"_\"");
+ }
+ }
+ if (result12 !== null) {
+ var result3 = result12;
+ } else {
+ if (input.substr(pos, 1) === "$") {
+ var result11 = "$";
+ pos += 1;
+ } else {
+ var result11 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"$\"");
+ }
+ }
+ if (result11 !== null) {
+ var result3 = result11;
+ } else {
+ var result3 = null;;
+ };
+ };
+ }
+ if (result3 !== null) {
+ var result4 = [];
+ var result10 = parse_letter();
+ if (result10 !== null) {
+ var result6 = result10;
+ } else {
+ var result9 = parse_digit();
+ if (result9 !== null) {
+ var result6 = result9;
+ } else {
+ if (input.substr(pos, 1) === "_") {
+ var result8 = "_";
+ pos += 1;
+ } else {
+ var result8 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"_\"");
+ }
+ }
+ if (result8 !== null) {
+ var result6 = result8;
+ } else {
+ if (input.substr(pos, 1) === "$") {
+ var result7 = "$";
+ pos += 1;
+ } else {
+ var result7 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"$\"");
+ }
+ }
+ if (result7 !== null) {
+ var result6 = result7;
+ } else {
+ var result6 = null;;
+ };
+ };
+ };
+ }
+ while (result6 !== null) {
+ result4.push(result6);
+ var result10 = parse_letter();
+ if (result10 !== null) {
+ var result6 = result10;
+ } else {
+ var result9 = parse_digit();
+ if (result9 !== null) {
+ var result6 = result9;
+ } else {
+ if (input.substr(pos, 1) === "_") {
+ var result8 = "_";
+ pos += 1;
+ } else {
+ var result8 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"_\"");
+ }
+ }
+ if (result8 !== null) {
+ var result6 = result8;
+ } else {
+ if (input.substr(pos, 1) === "$") {
+ var result7 = "$";
+ pos += 1;
+ } else {
+ var result7 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"$\"");
+ }
+ }
+ if (result7 !== null) {
+ var result6 = result7;
+ } else {
+ var result6 = null;;
+ };
+ };
+ };
+ }
+ }
+ if (result4 !== null) {
+ var result5 = parse___();
+ if (result5 !== null) {
+ var result1 = [result3, result4, result5];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(head, tail) {
+ return head + tail.join("");
+ })(result1[0], result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+ reportMatchFailures = savedReportMatchFailures;
+ if (reportMatchFailures && result0 === null) {
+ matchFailed("identifier");
+ }
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_literal() {
+ var cacheKey = 'literal@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+ var savedReportMatchFailures = reportMatchFailures;
+ reportMatchFailures = false;
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result6 = parse_doubleQuotedLiteral();
+ if (result6 !== null) {
+ var result3 = result6;
+ } else {
+ var result5 = parse_singleQuotedLiteral();
+ if (result5 !== null) {
+ var result3 = result5;
+ } else {
+ var result3 = null;;
+ };
+ }
+ if (result3 !== null) {
+ var result4 = parse___();
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(literal) { return literal; })(result1[0])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+ reportMatchFailures = savedReportMatchFailures;
+ if (reportMatchFailures && result0 === null) {
+ matchFailed("literal");
+ }
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_doubleQuotedLiteral() {
+ var cacheKey = 'doubleQuotedLiteral@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "\"") {
+ var result3 = "\"";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\\\"\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = [];
+ var result6 = parse_doubleQuotedCharacter();
+ while (result6 !== null) {
+ result4.push(result6);
+ var result6 = parse_doubleQuotedCharacter();
+ }
+ if (result4 !== null) {
+ if (input.substr(pos, 1) === "\"") {
+ var result5 = "\"";
+ pos += 1;
+ } else {
+ var result5 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\\\"\"");
+ }
+ }
+ if (result5 !== null) {
+ var result1 = [result3, result4, result5];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(chars) { return chars.join(""); })(result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_doubleQuotedCharacter() {
+ var cacheKey = 'doubleQuotedCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var result6 = parse_simpleDoubleQuotedCharacter();
+ if (result6 !== null) {
+ var result0 = result6;
+ } else {
+ var result5 = parse_simpleEscapeSequence();
+ if (result5 !== null) {
+ var result0 = result5;
+ } else {
+ var result4 = parse_zeroEscapeSequence();
+ if (result4 !== null) {
+ var result0 = result4;
+ } else {
+ var result3 = parse_hexEscapeSequence();
+ if (result3 !== null) {
+ var result0 = result3;
+ } else {
+ var result2 = parse_unicodeEscapeSequence();
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result1 = parse_eolEscapeSequence();
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ };
+ };
+ };
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_simpleDoubleQuotedCharacter() {
+ var cacheKey = 'simpleDoubleQuotedCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var savedPos2 = pos;
+ var savedReportMatchFailuresVar0 = reportMatchFailures;
+ reportMatchFailures = false;
+ if (input.substr(pos, 1) === "\"") {
+ var result8 = "\"";
+ pos += 1;
+ } else {
+ var result8 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\\\"\"");
+ }
+ }
+ if (result8 !== null) {
+ var result5 = result8;
+ } else {
+ if (input.substr(pos, 1) === "\\") {
+ var result7 = "\\";
+ pos += 1;
+ } else {
+ var result7 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\\\\\"");
+ }
+ }
+ if (result7 !== null) {
+ var result5 = result7;
+ } else {
+ var result6 = parse_eolChar();
+ if (result6 !== null) {
+ var result5 = result6;
+ } else {
+ var result5 = null;;
+ };
+ };
+ }
+ reportMatchFailures = savedReportMatchFailuresVar0;
+ if (result5 === null) {
+ var result3 = '';
+ } else {
+ var result3 = null;
+ pos = savedPos2;
+ }
+ if (result3 !== null) {
+ if (input.length > pos) {
+ var result4 = input.charAt(pos);
+ pos++;
+ } else {
+ var result4 = null;
+ if (reportMatchFailures) {
+ matchFailed('any character');
+ }
+ }
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(char_) { return char_; })(result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_singleQuotedLiteral() {
+ var cacheKey = 'singleQuotedLiteral@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "'") {
+ var result3 = "'";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"'\"");
+ }
+ }
+ if (result3 !== null) {
+ var result4 = [];
+ var result6 = parse_singleQuotedCharacter();
+ while (result6 !== null) {
+ result4.push(result6);
+ var result6 = parse_singleQuotedCharacter();
+ }
+ if (result4 !== null) {
+ if (input.substr(pos, 1) === "'") {
+ var result5 = "'";
+ pos += 1;
+ } else {
+ var result5 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"'\"");
+ }
+ }
+ if (result5 !== null) {
+ var result1 = [result3, result4, result5];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(chars) { return chars.join(""); })(result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_singleQuotedCharacter() {
+ var cacheKey = 'singleQuotedCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var result6 = parse_simpleSingleQuotedCharacter();
+ if (result6 !== null) {
+ var result0 = result6;
+ } else {
+ var result5 = parse_simpleEscapeSequence();
+ if (result5 !== null) {
+ var result0 = result5;
+ } else {
+ var result4 = parse_zeroEscapeSequence();
+ if (result4 !== null) {
+ var result0 = result4;
+ } else {
+ var result3 = parse_hexEscapeSequence();
+ if (result3 !== null) {
+ var result0 = result3;
+ } else {
+ var result2 = parse_unicodeEscapeSequence();
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result1 = parse_eolEscapeSequence();
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ };
+ };
+ };
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_simpleSingleQuotedCharacter() {
+ var cacheKey = 'simpleSingleQuotedCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var savedPos2 = pos;
+ var savedReportMatchFailuresVar0 = reportMatchFailures;
+ reportMatchFailures = false;
+ if (input.substr(pos, 1) === "'") {
+ var result8 = "'";
+ pos += 1;
+ } else {
+ var result8 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"'\"");
+ }
+ }
+ if (result8 !== null) {
+ var result5 = result8;
+ } else {
+ if (input.substr(pos, 1) === "\\") {
+ var result7 = "\\";
+ pos += 1;
+ } else {
+ var result7 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\\\\\"");
+ }
+ }
+ if (result7 !== null) {
+ var result5 = result7;
+ } else {
+ var result6 = parse_eolChar();
+ if (result6 !== null) {
+ var result5 = result6;
+ } else {
+ var result5 = null;;
+ };
+ };
+ }
+ reportMatchFailures = savedReportMatchFailuresVar0;
+ if (result5 === null) {
+ var result3 = '';
+ } else {
+ var result3 = null;
+ pos = savedPos2;
+ }
+ if (result3 !== null) {
+ if (input.length > pos) {
+ var result4 = input.charAt(pos);
+ pos++;
+ } else {
+ var result4 = null;
+ if (reportMatchFailures) {
+ matchFailed('any character');
+ }
+ }
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(char_) { return char_; })(result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_class() {
+ var cacheKey = 'class@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+ var savedReportMatchFailures = reportMatchFailures;
+ reportMatchFailures = false;
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ if (input.substr(pos, 1) === "[") {
+ var result3 = "[";
+ pos += 1;
+ } else {
+ var result3 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"[\"");
+ }
+ }
+ if (result3 !== null) {
+ if (input.substr(pos, 1) === "^") {
+ var result11 = "^";
+ pos += 1;
+ } else {
+ var result11 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"^\"");
+ }
+ }
+ var result4 = result11 !== null ? result11 : '';
+ if (result4 !== null) {
+ var result5 = [];
+ var result10 = parse_classCharacterRange();
+ if (result10 !== null) {
+ var result8 = result10;
+ } else {
+ var result9 = parse_classCharacter();
+ if (result9 !== null) {
+ var result8 = result9;
+ } else {
+ var result8 = null;;
+ };
+ }
+ while (result8 !== null) {
+ result5.push(result8);
+ var result10 = parse_classCharacterRange();
+ if (result10 !== null) {
+ var result8 = result10;
+ } else {
+ var result9 = parse_classCharacter();
+ if (result9 !== null) {
+ var result8 = result9;
+ } else {
+ var result8 = null;;
+ };
+ }
+ }
+ if (result5 !== null) {
+ if (input.substr(pos, 1) === "]") {
+ var result6 = "]";
+ pos += 1;
+ } else {
+ var result6 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"]\"");
+ }
+ }
+ if (result6 !== null) {
+ var result7 = parse___();
+ if (result7 !== null) {
+ var result1 = [result3, result4, result5, result6, result7];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(inverted, parts) {
+ var partsConverted = map(parts, function(part) { return part.data; });
+ var rawText = "["
+ + inverted
+ + map(parts, function(part) { return part.rawText; }).join("")
+ + "]";
+
+ return {
+ type: "class",
+ inverted: inverted === "^",
+ parts: partsConverted,
+ // FIXME: Get the raw text from the input directly.
+ rawText: rawText
+ };
+ })(result1[1], result1[2])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+ reportMatchFailures = savedReportMatchFailures;
+ if (reportMatchFailures && result0 === null) {
+ matchFailed("character class");
+ }
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_classCharacterRange() {
+ var cacheKey = 'classCharacterRange@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var result3 = parse_classCharacter();
+ if (result3 !== null) {
+ if (input.substr(pos, 1) === "-") {
+ var result4 = "-";
+ pos += 1;
+ } else {
+ var result4 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"-\"");
+ }
+ }
+ if (result4 !== null) {
+ var result5 = parse_classCharacter();
+ if (result5 !== null) {
+ var result1 = [result3, result4, result5];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(begin, end) {
+ if (begin.data.charCodeAt(0) > end.data.charCodeAt(0)) {
+ throw new this.SyntaxError(
+ "Invalid character range: " + begin.rawText + "-" + end.rawText + "."
+ );
+ }
+
+ return {
+ data: [begin.data, end.data],
+ // FIXME: Get the raw text from the input directly.
+ rawText: begin.rawText + "-" + end.rawText
+ }
+ })(result1[0], result1[2])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_classCharacter() {
+ var cacheKey = 'classCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var result1 = parse_bracketDelimitedCharacter();
+ var result2 = result1 !== null
+ ? (function(char_) {
+ return {
+ data: char_,
+ // FIXME: Get the raw text from the input directly.
+ rawText: quoteForRegexpClass(char_)
+ };
+ })(result1)
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_bracketDelimitedCharacter() {
+ var cacheKey = 'bracketDelimitedCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var result6 = parse_simpleBracketDelimitedCharacter();
+ if (result6 !== null) {
+ var result0 = result6;
+ } else {
+ var result5 = parse_simpleEscapeSequence();
+ if (result5 !== null) {
+ var result0 = result5;
+ } else {
+ var result4 = parse_zeroEscapeSequence();
+ if (result4 !== null) {
+ var result0 = result4;
+ } else {
+ var result3 = parse_hexEscapeSequence();
+ if (result3 !== null) {
+ var result0 = result3;
+ } else {
+ var result2 = parse_unicodeEscapeSequence();
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result1 = parse_eolEscapeSequence();
+ if (result1 !== null) {
+ var result0 = result1;
+ } else {
+ var result0 = null;;
+ };
+ };
+ };
+ };
+ };
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_simpleBracketDelimitedCharacter() {
+ var cacheKey = 'simpleBracketDelimitedCharacter@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+ pos = cachedResult.nextPos;
+ return cachedResult.result;
+ }
+
+
+ var savedPos0 = pos;
+ var savedPos1 = pos;
+ var savedPos2 = pos;
+ var savedReportMatchFailuresVar0 = reportMatchFailures;
+ reportMatchFailures = false;
+ if (input.substr(pos, 1) === "]") {
+ var result8 = "]";
+ pos += 1;
+ } else {
+ var result8 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"]\"");
+ }
+ }
+ if (result8 !== null) {
+ var result5 = result8;
+ } else {
+ if (input.substr(pos, 1) === "\\") {
+ var result7 = "\\";
+ pos += 1;
+ } else {
+ var result7 = null;
+ if (reportMatchFailures) {
+ matchFailed("\"\\\\\"");
+ }
+ }
+ if (result7 !== null) {
+ var result5 = result7;
+ } else {
+ var result6 = parse_eolChar();
+ if (result6 !== null) {
+ var result5 = result6;
+ } else {
+ var result5 = null;;
+ };
+ };
+ }
+ reportMatchFailures = savedReportMatchFailuresVar0;
+ if (result5 === null) {
+ var result3 = '';
+ } else {
+ var result3 = null;
+ pos = savedPos2;
+ }
+ if (result3 !== null) {
+ if (input.length > pos) {
+ var result4 = input.charAt(pos);
+ pos++;
+ } else {
+ var result4 = null;
+ if (reportMatchFailures) {
+ matchFailed('any character');
+ }
+ }
+ if (result4 !== null) {
+ var result1 = [result3, result4];
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ } else {
+ var result1 = null;
+ pos = savedPos1;
+ }
+ var result2 = result1 !== null
+ ? (function(char_) { return char_; })(result1[1])
+ : null;
+ if (result2 !== null) {
+ var result0 = result2;
+ } else {
+ var result0 = null;
+ pos = savedPos0;
+ }
+
+
+
+ cache[cacheKey] = {
+ nextPos: pos,
+ result: result0
+ };
+ return result0;
+ }
+
+ function parse_simpleEscapeSequence() {
+ var cacheKey = 'simpleEscapeSequence@' + pos;
+ var cachedResult = cache[cacheKey];
+ if (cachedResult) {
+
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[13/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/plist/dist/plist.js
----------------------------------------------------------------------
diff --git a/node_modules/plist/dist/plist.js b/node_modules/plist/dist/plist.js
new file mode 100644
index 0000000..96828ea
--- /dev/null
+++ b/node_modules/plist/dist/plist.js
@@ -0,0 +1,7987 @@
+(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.plist = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){
+(function (Buffer){
+
+/**
+ * Module dependencies.
+ */
+
+var base64 = require('base64-js');
+var xmlbuilder = require('xmlbuilder');
+
+/**
+ * Module exports.
+ */
+
+exports.build = build;
+
+/**
+ * Accepts a `Date` instance and returns an ISO date string.
+ *
+ * @param {Date} d - Date instance to serialize
+ * @returns {String} ISO date string representation of `d`
+ * @api private
+ */
+
+function ISODateString(d){
+ function pad(n){
+ return n < 10 ? '0' + n : n;
+ }
+ return d.getUTCFullYear()+'-'
+ + pad(d.getUTCMonth()+1)+'-'
+ + pad(d.getUTCDate())+'T'
+ + pad(d.getUTCHours())+':'
+ + pad(d.getUTCMinutes())+':'
+ + pad(d.getUTCSeconds())+'Z';
+}
+
+/**
+ * Returns the internal "type" of `obj` via the
+ * `Object.prototype.toString()` trick.
+ *
+ * @param {Mixed} obj - any value
+ * @returns {String} the internal "type" name
+ * @api private
+ */
+
+var toString = Object.prototype.toString;
+function type (obj) {
+ var m = toString.call(obj).match(/\[object (.*)\]/);
+ return m ? m[1] : m;
+}
+
+/**
+ * Generate an XML plist string from the input object `obj`.
+ *
+ * @param {Object} obj - the object to convert
+ * @param {Object} [opts] - optional options object
+ * @returns {String} converted plist XML string
+ * @api public
+ */
+
+function build (obj, opts) {
+ var XMLHDR = {
+ version: '1.0',
+ encoding: 'UTF-8'
+ };
+
+ var XMLDTD = {
+ pubid: '-//Apple//DTD PLIST 1.0//EN',
+ sysid: 'http://www.apple.com/DTDs/PropertyList-1.0.dtd'
+ };
+
+ var doc = xmlbuilder.create('plist');
+
+ doc.dec(XMLHDR.version, XMLHDR.encoding, XMLHDR.standalone);
+ doc.dtd(XMLDTD.pubid, XMLDTD.sysid);
+ doc.att('version', '1.0');
+
+ walk_obj(obj, doc);
+
+ if (!opts) opts = {};
+ // default `pretty` to `true`
+ opts.pretty = opts.pretty !== false;
+ return doc.end(opts);
+}
+
+/**
+ * depth first, recursive traversal of a javascript object. when complete,
+ * next_child contains a reference to the build XML object.
+ *
+ * @api private
+ */
+
+function walk_obj(next, next_child) {
+ var tag_type, i, prop;
+ var name = type(next);
+
+ if ('Undefined' == name) {
+ return;
+ } else if (Array.isArray(next)) {
+ next_child = next_child.ele('array');
+ for (i = 0; i < next.length; i++) {
+ walk_obj(next[i], next_child);
+ }
+
+ } else if (Buffer.isBuffer(next)) {
+ next_child.ele('data').raw(next.toString('base64'));
+
+ } else if ('Object' == name) {
+ next_child = next_child.ele('dict');
+ for (prop in next) {
+ if (next.hasOwnProperty(prop)) {
+ next_child.ele('key').txt(prop);
+ walk_obj(next[prop], next_child);
+ }
+ }
+
+ } else if ('Number' == name) {
+ // detect if this is an integer or real
+ // TODO: add an ability to force one way or another via a "cast"
+ tag_type = (next % 1 === 0) ? 'integer' : 'real';
+ next_child.ele(tag_type).txt(next.toString());
+
+ } else if ('Date' == name) {
+ next_child.ele('date').txt(ISODateString(new Date(next)));
+
+ } else if ('Boolean' == name) {
+ next_child.ele(next ? 'true' : 'false');
+
+ } else if ('String' == name) {
+ next_child.ele('string').txt(next);
+
+ } else if ('ArrayBuffer' == name) {
+ next_child.ele('data').raw(base64.fromByteArray(next));
+
+ } else if (next && next.buffer && 'ArrayBuffer' == type(next.buffer)) {
+ // a typed array
+ next_child.ele('data').raw(base64.fromByteArray(new Uint8Array(next.buffer), next_child));
+
+ }
+}
+
+}).call(this,{"isBuffer":require("../node_modules/is-buffer/index.js")})
+},{"../node_modules/is-buffer/index.js":10,"base64-js":5,"xmlbuilder":87}],2:[function(require,module,exports){
+/**
+ * Module dependencies.
+ */
+
+var fs = require('fs');
+var parse = require('./parse');
+var deprecate = require('util-deprecate');
+
+/**
+ * Module exports.
+ */
+
+exports.parseFile = deprecate(parseFile, '`parseFile()` is deprecated. ' +
+ 'Use `parseString()` instead.');
+exports.parseFileSync = deprecate(parseFileSync, '`parseFileSync()` is deprecated. ' +
+ 'Use `parseStringSync()` instead.');
+
+/**
+ * Parses file `filename` as a .plist file.
+ * Invokes `fn` callback function when done.
+ *
+ * @param {String} filename - name of the file to read
+ * @param {Function} fn - callback function
+ * @api public
+ * @deprecated use parseString() instead
+ */
+
+function parseFile (filename, fn) {
+ fs.readFile(filename, { encoding: 'utf8' }, onread);
+ function onread (err, inxml) {
+ if (err) return fn(err);
+ parse.parseString(inxml, fn);
+ }
+}
+
+/**
+ * Parses file `filename` as a .plist file.
+ * Returns a when done.
+ *
+ * @param {String} filename - name of the file to read
+ * @param {Function} fn - callback function
+ * @api public
+ * @deprecated use parseStringSync() instead
+ */
+
+function parseFileSync (filename) {
+ var inxml = fs.readFileSync(filename, 'utf8');
+ return parse.parseStringSync(inxml);
+}
+
+},{"./parse":3,"fs":6,"util-deprecate":70}],3:[function(require,module,exports){
+(function (Buffer){
+
+/**
+ * Module dependencies.
+ */
+
+var deprecate = require('util-deprecate');
+var DOMParser = require('xmldom').DOMParser;
+
+/**
+ * Module exports.
+ */
+
+exports.parse = parse;
+exports.parseString = deprecate(parseString, '`parseString()` is deprecated. ' +
+ 'It\'s not actually async. Use `parse()` instead.');
+exports.parseStringSync = deprecate(parseStringSync, '`parseStringSync()` is ' +
+ 'deprecated. Use `parse()` instead.');
+
+/**
+ * We ignore raw text (usually whitespace), <!-- xml comments -->,
+ * and raw CDATA nodes.
+ *
+ * @param {Element} node
+ * @returns {Boolean}
+ * @api private
+ */
+
+function shouldIgnoreNode (node) {
+ return node.nodeType === 3 // text
+ || node.nodeType === 8 // comment
+ || node.nodeType === 4; // cdata
+}
+
+
+/**
+ * Parses a Plist XML string. Returns an Object.
+ *
+ * @param {String} xml - the XML String to decode
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ */
+
+function parse (xml) {
+ var doc = new DOMParser().parseFromString(xml);
+ if (doc.documentElement.nodeName !== 'plist') {
+ throw new Error('malformed document. First element should be <plist>');
+ }
+ var plist = parsePlistXML(doc.documentElement);
+
+ // the root <plist> node gets interpreted as an Array,
+ // so pull out the inner data first
+ if (plist.length == 1) plist = plist[0];
+
+ return plist;
+}
+
+/**
+ * Parses a Plist XML string. Returns an Object. Takes a `callback` function.
+ *
+ * @param {String} xml - the XML String to decode
+ * @param {Function} callback - callback function
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ * @deprecated not actually async. use parse() instead
+ */
+
+function parseString (xml, callback) {
+ var doc, error, plist;
+ try {
+ doc = new DOMParser().parseFromString(xml);
+ plist = parsePlistXML(doc.documentElement);
+ } catch(e) {
+ error = e;
+ }
+ callback(error, plist);
+}
+
+/**
+ * Parses a Plist XML string. Returns an Object.
+ *
+ * @param {String} xml - the XML String to decode
+ * @param {Function} callback - callback function
+ * @returns {Mixed} the decoded value from the Plist XML
+ * @api public
+ * @deprecated use parse() instead
+ */
+
+function parseStringSync (xml) {
+ var doc = new DOMParser().parseFromString(xml);
+ var plist;
+ if (doc.documentElement.nodeName !== 'plist') {
+ throw new Error('malformed document. First element should be <plist>');
+ }
+ plist = parsePlistXML(doc.documentElement);
+
+ // if the plist is an array with 1 element, pull it out of the array
+ if (plist.length == 1) {
+ plist = plist[0];
+ }
+ return plist;
+}
+
+/**
+ * Convert an XML based plist document into a JSON representation.
+ *
+ * @param {Object} xml_node - current XML node in the plist
+ * @returns {Mixed} built up JSON object
+ * @api private
+ */
+
+function parsePlistXML (node) {
+ var i, new_obj, key, val, new_arr, res, d;
+
+ if (!node)
+ return null;
+
+ if (node.nodeName === 'plist') {
+ new_arr = [];
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ new_arr.push( parsePlistXML(node.childNodes[i]));
+ }
+ }
+ return new_arr;
+
+ } else if (node.nodeName === 'dict') {
+ new_obj = {};
+ key = null;
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ if (key === null) {
+ key = parsePlistXML(node.childNodes[i]);
+ } else {
+ new_obj[key] = parsePlistXML(node.childNodes[i]);
+ key = null;
+ }
+ }
+ }
+ return new_obj;
+
+ } else if (node.nodeName === 'array') {
+ new_arr = [];
+ for (i=0; i < node.childNodes.length; i++) {
+ // ignore comment nodes (text)
+ if (!shouldIgnoreNode(node.childNodes[i])) {
+ res = parsePlistXML(node.childNodes[i]);
+ if (null != res) new_arr.push(res);
+ }
+ }
+ return new_arr;
+
+ } else if (node.nodeName === '#text') {
+ // TODO: what should we do with text types? (CDATA sections)
+
+ } else if (node.nodeName === 'key') {
+ return node.childNodes[0].nodeValue;
+
+ } else if (node.nodeName === 'string') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ res += node.childNodes[d].nodeValue;
+ }
+ return res;
+
+ } else if (node.nodeName === 'integer') {
+ // parse as base 10 integer
+ return parseInt(node.childNodes[0].nodeValue, 10);
+
+ } else if (node.nodeName === 'real') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ if (node.childNodes[d].nodeType === 3) {
+ res += node.childNodes[d].nodeValue;
+ }
+ }
+ return parseFloat(res);
+
+ } else if (node.nodeName === 'data') {
+ res = '';
+ for (d=0; d < node.childNodes.length; d++) {
+ if (node.childNodes[d].nodeType === 3) {
+ res += node.childNodes[d].nodeValue.replace(/\s+/g, '');
+ }
+ }
+
+ // decode base64 data to a Buffer instance
+ return new Buffer(res, 'base64');
+
+ } else if (node.nodeName === 'date') {
+ return new Date(node.childNodes[0].nodeValue);
+
+ } else if (node.nodeName === 'true') {
+ return true;
+
+ } else if (node.nodeName === 'false') {
+ return false;
+ }
+}
+
+}).call(this,require("buffer").Buffer)
+},{"buffer":7,"util-deprecate":70,"xmldom":88}],4:[function(require,module,exports){
+
+var i;
+
+/**
+ * Parser functions.
+ */
+
+var parserFunctions = require('./parse');
+for (i in parserFunctions) exports[i] = parserFunctions[i];
+
+/**
+ * Builder functions.
+ */
+
+var builderFunctions = require('./build');
+for (i in builderFunctions) exports[i] = builderFunctions[i];
+
+/**
+ * Add Node.js-specific functions (they're deprecated…).
+ */
+
+var nodeFunctions = require('./node');
+for (i in nodeFunctions) exports[i] = nodeFunctions[i];
+
+},{"./build":1,"./node":2,"./parse":3}],5:[function(require,module,exports){
+var lookup = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/';
+
+;(function (exports) {
+ 'use strict';
+
+ var Arr = (typeof Uint8Array !== 'undefined')
+ ? Uint8Array
+ : Array
+
+ var PLUS = '+'.charCodeAt(0)
+ var SLASH = '/'.charCodeAt(0)
+ var NUMBER = '0'.charCodeAt(0)
+ var LOWER = 'a'.charCodeAt(0)
+ var UPPER = 'A'.charCodeAt(0)
+ var PLUS_URL_SAFE = '-'.charCodeAt(0)
+ var SLASH_URL_SAFE = '_'.charCodeAt(0)
+
+ function decode (elt) {
+ var code = elt.charCodeAt(0)
+ if (code === PLUS ||
+ code === PLUS_URL_SAFE)
+ return 62 // '+'
+ if (code === SLASH ||
+ code === SLASH_URL_SAFE)
+ return 63 // '/'
+ if (code < NUMBER)
+ return -1 //no match
+ if (code < NUMBER + 10)
+ return code - NUMBER + 26 + 26
+ if (code < UPPER + 26)
+ return code - UPPER
+ if (code < LOWER + 26)
+ return code - LOWER + 26
+ }
+
+ function b64ToByteArray (b64) {
+ var i, j, l, tmp, placeHolders, arr
+
+ if (b64.length % 4 > 0) {
+ throw new Error('Invalid string. Length must be a multiple of 4')
+ }
+
+ // the number of equal signs (place holders)
+ // if there are two placeholders, than the two characters before it
+ // represent one byte
+ // if there is only one, then the three characters before it represent 2 bytes
+ // this is just a cheap hack to not do indexOf twice
+ var len = b64.length
+ placeHolders = '=' === b64.charAt(len - 2) ? 2 : '=' === b64.charAt(len - 1) ? 1 : 0
+
+ // base64 is 4/3 + up to two characters of the original data
+ arr = new Arr(b64.length * 3 / 4 - placeHolders)
+
+ // if there are placeholders, only get up to the last complete 4 chars
+ l = placeHolders > 0 ? b64.length - 4 : b64.length
+
+ var L = 0
+
+ function push (v) {
+ arr[L++] = v
+ }
+
+ for (i = 0, j = 0; i < l; i += 4, j += 3) {
+ tmp = (decode(b64.charAt(i)) << 18) | (decode(b64.charAt(i + 1)) << 12) | (decode(b64.charAt(i + 2)) << 6) | decode(b64.charAt(i + 3))
+ push((tmp & 0xFF0000) >> 16)
+ push((tmp & 0xFF00) >> 8)
+ push(tmp & 0xFF)
+ }
+
+ if (placeHolders === 2) {
+ tmp = (decode(b64.charAt(i)) << 2) | (decode(b64.charAt(i + 1)) >> 4)
+ push(tmp & 0xFF)
+ } else if (placeHolders === 1) {
+ tmp = (decode(b64.charAt(i)) << 10) | (decode(b64.charAt(i + 1)) << 4) | (decode(b64.charAt(i + 2)) >> 2)
+ push((tmp >> 8) & 0xFF)
+ push(tmp & 0xFF)
+ }
+
+ return arr
+ }
+
+ function uint8ToBase64 (uint8) {
+ var i,
+ extraBytes = uint8.length % 3, // if we have 1 byte left, pad 2 bytes
+ output = "",
+ temp, length
+
+ function encode (num) {
+ return lookup.charAt(num)
+ }
+
+ function tripletToBase64 (num) {
+ return encode(num >> 18 & 0x3F) + encode(num >> 12 & 0x3F) + encode(num >> 6 & 0x3F) + encode(num & 0x3F)
+ }
+
+ // go through the array every three bytes, we'll deal with trailing stuff later
+ for (i = 0, length = uint8.length - extraBytes; i < length; i += 3) {
+ temp = (uint8[i] << 16) + (uint8[i + 1] << 8) + (uint8[i + 2])
+ output += tripletToBase64(temp)
+ }
+
+ // pad the end with zeros, but make sure to not forget the extra bytes
+ switch (extraBytes) {
+ case 1:
+ temp = uint8[uint8.length - 1]
+ output += encode(temp >> 2)
+ output += encode((temp << 4) & 0x3F)
+ output += '=='
+ break
+ case 2:
+ temp = (uint8[uint8.length - 2] << 8) + (uint8[uint8.length - 1])
+ output += encode(temp >> 10)
+ output += encode((temp >> 4) & 0x3F)
+ output += encode((temp << 2) & 0x3F)
+ output += '='
+ break
+ }
+
+ return output
+ }
+
+ exports.toByteArray = b64ToByteArray
+ exports.fromByteArray = uint8ToBase64
+}(typeof exports === 'undefined' ? (this.base64js = {}) : exports))
+
+},{}],6:[function(require,module,exports){
+
+},{}],7:[function(require,module,exports){
+(function (global){
+/*!
+ * The buffer module from node.js, for the browser.
+ *
+ * @author Feross Aboukhadijeh <fe...@feross.org> <http://feross.org>
+ * @license MIT
+ */
+/* eslint-disable no-proto */
+
+var base64 = require('base64-js')
+var ieee754 = require('ieee754')
+var isArray = require('is-array')
+
+exports.Buffer = Buffer
+exports.SlowBuffer = SlowBuffer
+exports.INSPECT_MAX_BYTES = 50
+Buffer.poolSize = 8192 // not used by this implementation
+
+var rootParent = {}
+
+/**
+ * If `Buffer.TYPED_ARRAY_SUPPORT`:
+ * === true Use Uint8Array implementation (fastest)
+ * === false Use Object implementation (most compatible, even IE6)
+ *
+ * Browsers that support typed arrays are IE 10+, Firefox 4+, Chrome 7+, Safari 5.1+,
+ * Opera 11.6+, iOS 4.2+.
+ *
+ * Due to various browser bugs, sometimes the Object implementation will be used even
+ * when the browser supports typed arrays.
+ *
+ * Note:
+ *
+ * - Firefox 4-29 lacks support for adding new properties to `Uint8Array` instances,
+ * See: https://bugzilla.mozilla.org/show_bug.cgi?id=695438.
+ *
+ * - Safari 5-7 lacks support for changing the `Object.prototype.constructor` property
+ * on objects.
+ *
+ * - Chrome 9-10 is missing the `TypedArray.prototype.subarray` function.
+ *
+ * - IE10 has a broken `TypedArray.prototype.subarray` function which returns arrays of
+ * incorrect length in some situations.
+
+ * We detect these buggy browsers and set `Buffer.TYPED_ARRAY_SUPPORT` to `false` so they
+ * get the Object implementation, which is slower but behaves correctly.
+ */
+Buffer.TYPED_ARRAY_SUPPORT = global.TYPED_ARRAY_SUPPORT !== undefined
+ ? global.TYPED_ARRAY_SUPPORT
+ : typedArraySupport()
+
+function typedArraySupport () {
+ function Bar () {}
+ try {
+ var arr = new Uint8Array(1)
+ arr.foo = function () { return 42 }
+ arr.constructor = Bar
+ return arr.foo() === 42 && // typed array instances can be augmented
+ arr.constructor === Bar && // constructor can be set
+ typeof arr.subarray === 'function' && // chrome 9-10 lack `subarray`
+ arr.subarray(1, 1).byteLength === 0 // ie10 has broken `subarray`
+ } catch (e) {
+ return false
+ }
+}
+
+function kMaxLength () {
+ return Buffer.TYPED_ARRAY_SUPPORT
+ ? 0x7fffffff
+ : 0x3fffffff
+}
+
+/**
+ * Class: Buffer
+ * =============
+ *
+ * The Buffer constructor returns instances of `Uint8Array` that are augmented
+ * with function properties for all the node `Buffer` API functions. We use
+ * `Uint8Array` so that square bracket notation works as expected -- it returns
+ * a single octet.
+ *
+ * By augmenting the instances, we can avoid modifying the `Uint8Array`
+ * prototype.
+ */
+function Buffer (arg) {
+ if (!(this instanceof Buffer)) {
+ // Avoid going through an ArgumentsAdaptorTrampoline in the common case.
+ if (arguments.length > 1) return new Buffer(arg, arguments[1])
+ return new Buffer(arg)
+ }
+
+ this.length = 0
+ this.parent = undefined
+
+ // Common case.
+ if (typeof arg === 'number') {
+ return fromNumber(this, arg)
+ }
+
+ // Slightly less common case.
+ if (typeof arg === 'string') {
+ return fromString(this, arg, arguments.length > 1 ? arguments[1] : 'utf8')
+ }
+
+ // Unusual.
+ return fromObject(this, arg)
+}
+
+function fromNumber (that, length) {
+ that = allocate(that, length < 0 ? 0 : checked(length) | 0)
+ if (!Buffer.TYPED_ARRAY_SUPPORT) {
+ for (var i = 0; i < length; i++) {
+ that[i] = 0
+ }
+ }
+ return that
+}
+
+function fromString (that, string, encoding) {
+ if (typeof encoding !== 'string' || encoding === '') encoding = 'utf8'
+
+ // Assumption: byteLength() return value is always < kMaxLength.
+ var length = byteLength(string, encoding) | 0
+ that = allocate(that, length)
+
+ that.write(string, encoding)
+ return that
+}
+
+function fromObject (that, object) {
+ if (Buffer.isBuffer(object)) return fromBuffer(that, object)
+
+ if (isArray(object)) return fromArray(that, object)
+
+ if (object == null) {
+ throw new TypeError('must start with number, buffer, array or string')
+ }
+
+ if (typeof ArrayBuffer !== 'undefined') {
+ if (object.buffer instanceof ArrayBuffer) {
+ return fromTypedArray(that, object)
+ }
+ if (object instanceof ArrayBuffer) {
+ return fromArrayBuffer(that, object)
+ }
+ }
+
+ if (object.length) return fromArrayLike(that, object)
+
+ return fromJsonObject(that, object)
+}
+
+function fromBuffer (that, buffer) {
+ var length = checked(buffer.length) | 0
+ that = allocate(that, length)
+ buffer.copy(that, 0, 0, length)
+ return that
+}
+
+function fromArray (that, array) {
+ var length = checked(array.length) | 0
+ that = allocate(that, length)
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+// Duplicate of fromArray() to keep fromArray() monomorphic.
+function fromTypedArray (that, array) {
+ var length = checked(array.length) | 0
+ that = allocate(that, length)
+ // Truncating the elements is probably not what people expect from typed
+ // arrays with BYTES_PER_ELEMENT > 1 but it's compatible with the behavior
+ // of the old Buffer constructor.
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+function fromArrayBuffer (that, array) {
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ // Return an augmented `Uint8Array` instance, for best performance
+ array.byteLength
+ that = Buffer._augment(new Uint8Array(array))
+ } else {
+ // Fallback: Return an object instance of the Buffer class
+ that = fromTypedArray(that, new Uint8Array(array))
+ }
+ return that
+}
+
+function fromArrayLike (that, array) {
+ var length = checked(array.length) | 0
+ that = allocate(that, length)
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+// Deserialize { type: 'Buffer', data: [1,2,3,...] } into a Buffer object.
+// Returns a zero-length buffer for inputs that don't conform to the spec.
+function fromJsonObject (that, object) {
+ var array
+ var length = 0
+
+ if (object.type === 'Buffer' && isArray(object.data)) {
+ array = object.data
+ length = checked(array.length) | 0
+ }
+ that = allocate(that, length)
+
+ for (var i = 0; i < length; i += 1) {
+ that[i] = array[i] & 255
+ }
+ return that
+}
+
+if (Buffer.TYPED_ARRAY_SUPPORT) {
+ Buffer.prototype.__proto__ = Uint8Array.prototype
+ Buffer.__proto__ = Uint8Array
+}
+
+function allocate (that, length) {
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ // Return an augmented `Uint8Array` instance, for best performance
+ that = Buffer._augment(new Uint8Array(length))
+ that.__proto__ = Buffer.prototype
+ } else {
+ // Fallback: Return an object instance of the Buffer class
+ that.length = length
+ that._isBuffer = true
+ }
+
+ var fromPool = length !== 0 && length <= Buffer.poolSize >>> 1
+ if (fromPool) that.parent = rootParent
+
+ return that
+}
+
+function checked (length) {
+ // Note: cannot use `length < kMaxLength` here because that fails when
+ // length is NaN (which is otherwise coerced to zero.)
+ if (length >= kMaxLength()) {
+ throw new RangeError('Attempt to allocate Buffer larger than maximum ' +
+ 'size: 0x' + kMaxLength().toString(16) + ' bytes')
+ }
+ return length | 0
+}
+
+function SlowBuffer (subject, encoding) {
+ if (!(this instanceof SlowBuffer)) return new SlowBuffer(subject, encoding)
+
+ var buf = new Buffer(subject, encoding)
+ delete buf.parent
+ return buf
+}
+
+Buffer.isBuffer = function isBuffer (b) {
+ return !!(b != null && b._isBuffer)
+}
+
+Buffer.compare = function compare (a, b) {
+ if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) {
+ throw new TypeError('Arguments must be Buffers')
+ }
+
+ if (a === b) return 0
+
+ var x = a.length
+ var y = b.length
+
+ var i = 0
+ var len = Math.min(x, y)
+ while (i < len) {
+ if (a[i] !== b[i]) break
+
+ ++i
+ }
+
+ if (i !== len) {
+ x = a[i]
+ y = b[i]
+ }
+
+ if (x < y) return -1
+ if (y < x) return 1
+ return 0
+}
+
+Buffer.isEncoding = function isEncoding (encoding) {
+ switch (String(encoding).toLowerCase()) {
+ case 'hex':
+ case 'utf8':
+ case 'utf-8':
+ case 'ascii':
+ case 'binary':
+ case 'base64':
+ case 'raw':
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return true
+ default:
+ return false
+ }
+}
+
+Buffer.concat = function concat (list, length) {
+ if (!isArray(list)) throw new TypeError('list argument must be an Array of Buffers.')
+
+ if (list.length === 0) {
+ return new Buffer(0)
+ }
+
+ var i
+ if (length === undefined) {
+ length = 0
+ for (i = 0; i < list.length; i++) {
+ length += list[i].length
+ }
+ }
+
+ var buf = new Buffer(length)
+ var pos = 0
+ for (i = 0; i < list.length; i++) {
+ var item = list[i]
+ item.copy(buf, pos)
+ pos += item.length
+ }
+ return buf
+}
+
+function byteLength (string, encoding) {
+ if (typeof string !== 'string') string = '' + string
+
+ var len = string.length
+ if (len === 0) return 0
+
+ // Use a for loop to avoid recursion
+ var loweredCase = false
+ for (;;) {
+ switch (encoding) {
+ case 'ascii':
+ case 'binary':
+ // Deprecated
+ case 'raw':
+ case 'raws':
+ return len
+ case 'utf8':
+ case 'utf-8':
+ return utf8ToBytes(string).length
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return len * 2
+ case 'hex':
+ return len >>> 1
+ case 'base64':
+ return base64ToBytes(string).length
+ default:
+ if (loweredCase) return utf8ToBytes(string).length // assume utf8
+ encoding = ('' + encoding).toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+Buffer.byteLength = byteLength
+
+// pre-set for values that may exist in the future
+Buffer.prototype.length = undefined
+Buffer.prototype.parent = undefined
+
+function slowToString (encoding, start, end) {
+ var loweredCase = false
+
+ start = start | 0
+ end = end === undefined || end === Infinity ? this.length : end | 0
+
+ if (!encoding) encoding = 'utf8'
+ if (start < 0) start = 0
+ if (end > this.length) end = this.length
+ if (end <= start) return ''
+
+ while (true) {
+ switch (encoding) {
+ case 'hex':
+ return hexSlice(this, start, end)
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Slice(this, start, end)
+
+ case 'ascii':
+ return asciiSlice(this, start, end)
+
+ case 'binary':
+ return binarySlice(this, start, end)
+
+ case 'base64':
+ return base64Slice(this, start, end)
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return utf16leSlice(this, start, end)
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
+ encoding = (encoding + '').toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+
+Buffer.prototype.toString = function toString () {
+ var length = this.length | 0
+ if (length === 0) return ''
+ if (arguments.length === 0) return utf8Slice(this, 0, length)
+ return slowToString.apply(this, arguments)
+}
+
+Buffer.prototype.equals = function equals (b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
+ if (this === b) return true
+ return Buffer.compare(this, b) === 0
+}
+
+Buffer.prototype.inspect = function inspect () {
+ var str = ''
+ var max = exports.INSPECT_MAX_BYTES
+ if (this.length > 0) {
+ str = this.toString('hex', 0, max).match(/.{2}/g).join(' ')
+ if (this.length > max) str += ' ... '
+ }
+ return '<Buffer ' + str + '>'
+}
+
+Buffer.prototype.compare = function compare (b) {
+ if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer')
+ if (this === b) return 0
+ return Buffer.compare(this, b)
+}
+
+Buffer.prototype.indexOf = function indexOf (val, byteOffset) {
+ if (byteOffset > 0x7fffffff) byteOffset = 0x7fffffff
+ else if (byteOffset < -0x80000000) byteOffset = -0x80000000
+ byteOffset >>= 0
+
+ if (this.length === 0) return -1
+ if (byteOffset >= this.length) return -1
+
+ // Negative offsets start from the end of the buffer
+ if (byteOffset < 0) byteOffset = Math.max(this.length + byteOffset, 0)
+
+ if (typeof val === 'string') {
+ if (val.length === 0) return -1 // special case: looking for empty string always fails
+ return String.prototype.indexOf.call(this, val, byteOffset)
+ }
+ if (Buffer.isBuffer(val)) {
+ return arrayIndexOf(this, val, byteOffset)
+ }
+ if (typeof val === 'number') {
+ if (Buffer.TYPED_ARRAY_SUPPORT && Uint8Array.prototype.indexOf === 'function') {
+ return Uint8Array.prototype.indexOf.call(this, val, byteOffset)
+ }
+ return arrayIndexOf(this, [ val ], byteOffset)
+ }
+
+ function arrayIndexOf (arr, val, byteOffset) {
+ var foundIndex = -1
+ for (var i = 0; byteOffset + i < arr.length; i++) {
+ if (arr[byteOffset + i] === val[foundIndex === -1 ? 0 : i - foundIndex]) {
+ if (foundIndex === -1) foundIndex = i
+ if (i - foundIndex + 1 === val.length) return byteOffset + foundIndex
+ } else {
+ foundIndex = -1
+ }
+ }
+ return -1
+ }
+
+ throw new TypeError('val must be string, number or Buffer')
+}
+
+// `get` is deprecated
+Buffer.prototype.get = function get (offset) {
+ console.log('.get() is deprecated. Access using array indexes instead.')
+ return this.readUInt8(offset)
+}
+
+// `set` is deprecated
+Buffer.prototype.set = function set (v, offset) {
+ console.log('.set() is deprecated. Access using array indexes instead.')
+ return this.writeUInt8(v, offset)
+}
+
+function hexWrite (buf, string, offset, length) {
+ offset = Number(offset) || 0
+ var remaining = buf.length - offset
+ if (!length) {
+ length = remaining
+ } else {
+ length = Number(length)
+ if (length > remaining) {
+ length = remaining
+ }
+ }
+
+ // must be an even number of digits
+ var strLen = string.length
+ if (strLen % 2 !== 0) throw new Error('Invalid hex string')
+
+ if (length > strLen / 2) {
+ length = strLen / 2
+ }
+ for (var i = 0; i < length; i++) {
+ var parsed = parseInt(string.substr(i * 2, 2), 16)
+ if (isNaN(parsed)) throw new Error('Invalid hex string')
+ buf[offset + i] = parsed
+ }
+ return i
+}
+
+function utf8Write (buf, string, offset, length) {
+ return blitBuffer(utf8ToBytes(string, buf.length - offset), buf, offset, length)
+}
+
+function asciiWrite (buf, string, offset, length) {
+ return blitBuffer(asciiToBytes(string), buf, offset, length)
+}
+
+function binaryWrite (buf, string, offset, length) {
+ return asciiWrite(buf, string, offset, length)
+}
+
+function base64Write (buf, string, offset, length) {
+ return blitBuffer(base64ToBytes(string), buf, offset, length)
+}
+
+function ucs2Write (buf, string, offset, length) {
+ return blitBuffer(utf16leToBytes(string, buf.length - offset), buf, offset, length)
+}
+
+Buffer.prototype.write = function write (string, offset, length, encoding) {
+ // Buffer#write(string)
+ if (offset === undefined) {
+ encoding = 'utf8'
+ length = this.length
+ offset = 0
+ // Buffer#write(string, encoding)
+ } else if (length === undefined && typeof offset === 'string') {
+ encoding = offset
+ length = this.length
+ offset = 0
+ // Buffer#write(string, offset[, length][, encoding])
+ } else if (isFinite(offset)) {
+ offset = offset | 0
+ if (isFinite(length)) {
+ length = length | 0
+ if (encoding === undefined) encoding = 'utf8'
+ } else {
+ encoding = length
+ length = undefined
+ }
+ // legacy write(string, encoding, offset, length) - remove in v0.13
+ } else {
+ var swap = encoding
+ encoding = offset
+ offset = length | 0
+ length = swap
+ }
+
+ var remaining = this.length - offset
+ if (length === undefined || length > remaining) length = remaining
+
+ if ((string.length > 0 && (length < 0 || offset < 0)) || offset > this.length) {
+ throw new RangeError('attempt to write outside buffer bounds')
+ }
+
+ if (!encoding) encoding = 'utf8'
+
+ var loweredCase = false
+ for (;;) {
+ switch (encoding) {
+ case 'hex':
+ return hexWrite(this, string, offset, length)
+
+ case 'utf8':
+ case 'utf-8':
+ return utf8Write(this, string, offset, length)
+
+ case 'ascii':
+ return asciiWrite(this, string, offset, length)
+
+ case 'binary':
+ return binaryWrite(this, string, offset, length)
+
+ case 'base64':
+ // Warning: maxLength not taken into account in base64Write
+ return base64Write(this, string, offset, length)
+
+ case 'ucs2':
+ case 'ucs-2':
+ case 'utf16le':
+ case 'utf-16le':
+ return ucs2Write(this, string, offset, length)
+
+ default:
+ if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding)
+ encoding = ('' + encoding).toLowerCase()
+ loweredCase = true
+ }
+ }
+}
+
+Buffer.prototype.toJSON = function toJSON () {
+ return {
+ type: 'Buffer',
+ data: Array.prototype.slice.call(this._arr || this, 0)
+ }
+}
+
+function base64Slice (buf, start, end) {
+ if (start === 0 && end === buf.length) {
+ return base64.fromByteArray(buf)
+ } else {
+ return base64.fromByteArray(buf.slice(start, end))
+ }
+}
+
+function utf8Slice (buf, start, end) {
+ end = Math.min(buf.length, end)
+ var res = []
+
+ var i = start
+ while (i < end) {
+ var firstByte = buf[i]
+ var codePoint = null
+ var bytesPerSequence = (firstByte > 0xEF) ? 4
+ : (firstByte > 0xDF) ? 3
+ : (firstByte > 0xBF) ? 2
+ : 1
+
+ if (i + bytesPerSequence <= end) {
+ var secondByte, thirdByte, fourthByte, tempCodePoint
+
+ switch (bytesPerSequence) {
+ case 1:
+ if (firstByte < 0x80) {
+ codePoint = firstByte
+ }
+ break
+ case 2:
+ secondByte = buf[i + 1]
+ if ((secondByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0x1F) << 0x6 | (secondByte & 0x3F)
+ if (tempCodePoint > 0x7F) {
+ codePoint = tempCodePoint
+ }
+ }
+ break
+ case 3:
+ secondByte = buf[i + 1]
+ thirdByte = buf[i + 2]
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0xC | (secondByte & 0x3F) << 0x6 | (thirdByte & 0x3F)
+ if (tempCodePoint > 0x7FF && (tempCodePoint < 0xD800 || tempCodePoint > 0xDFFF)) {
+ codePoint = tempCodePoint
+ }
+ }
+ break
+ case 4:
+ secondByte = buf[i + 1]
+ thirdByte = buf[i + 2]
+ fourthByte = buf[i + 3]
+ if ((secondByte & 0xC0) === 0x80 && (thirdByte & 0xC0) === 0x80 && (fourthByte & 0xC0) === 0x80) {
+ tempCodePoint = (firstByte & 0xF) << 0x12 | (secondByte & 0x3F) << 0xC | (thirdByte & 0x3F) << 0x6 | (fourthByte & 0x3F)
+ if (tempCodePoint > 0xFFFF && tempCodePoint < 0x110000) {
+ codePoint = tempCodePoint
+ }
+ }
+ }
+ }
+
+ if (codePoint === null) {
+ // we did not generate a valid codePoint so insert a
+ // replacement char (U+FFFD) and advance only 1 byte
+ codePoint = 0xFFFD
+ bytesPerSequence = 1
+ } else if (codePoint > 0xFFFF) {
+ // encode to utf16 (surrogate pair dance)
+ codePoint -= 0x10000
+ res.push(codePoint >>> 10 & 0x3FF | 0xD800)
+ codePoint = 0xDC00 | codePoint & 0x3FF
+ }
+
+ res.push(codePoint)
+ i += bytesPerSequence
+ }
+
+ return decodeCodePointsArray(res)
+}
+
+// Based on http://stackoverflow.com/a/22747272/680742, the browser with
+// the lowest limit is Chrome, with 0x10000 args.
+// We go 1 magnitude less, for safety
+var MAX_ARGUMENTS_LENGTH = 0x1000
+
+function decodeCodePointsArray (codePoints) {
+ var len = codePoints.length
+ if (len <= MAX_ARGUMENTS_LENGTH) {
+ return String.fromCharCode.apply(String, codePoints) // avoid extra slice()
+ }
+
+ // Decode in chunks to avoid "call stack size exceeded".
+ var res = ''
+ var i = 0
+ while (i < len) {
+ res += String.fromCharCode.apply(
+ String,
+ codePoints.slice(i, i += MAX_ARGUMENTS_LENGTH)
+ )
+ }
+ return res
+}
+
+function asciiSlice (buf, start, end) {
+ var ret = ''
+ end = Math.min(buf.length, end)
+
+ for (var i = start; i < end; i++) {
+ ret += String.fromCharCode(buf[i] & 0x7F)
+ }
+ return ret
+}
+
+function binarySlice (buf, start, end) {
+ var ret = ''
+ end = Math.min(buf.length, end)
+
+ for (var i = start; i < end; i++) {
+ ret += String.fromCharCode(buf[i])
+ }
+ return ret
+}
+
+function hexSlice (buf, start, end) {
+ var len = buf.length
+
+ if (!start || start < 0) start = 0
+ if (!end || end < 0 || end > len) end = len
+
+ var out = ''
+ for (var i = start; i < end; i++) {
+ out += toHex(buf[i])
+ }
+ return out
+}
+
+function utf16leSlice (buf, start, end) {
+ var bytes = buf.slice(start, end)
+ var res = ''
+ for (var i = 0; i < bytes.length; i += 2) {
+ res += String.fromCharCode(bytes[i] + bytes[i + 1] * 256)
+ }
+ return res
+}
+
+Buffer.prototype.slice = function slice (start, end) {
+ var len = this.length
+ start = ~~start
+ end = end === undefined ? len : ~~end
+
+ if (start < 0) {
+ start += len
+ if (start < 0) start = 0
+ } else if (start > len) {
+ start = len
+ }
+
+ if (end < 0) {
+ end += len
+ if (end < 0) end = 0
+ } else if (end > len) {
+ end = len
+ }
+
+ if (end < start) end = start
+
+ var newBuf
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ newBuf = Buffer._augment(this.subarray(start, end))
+ } else {
+ var sliceLen = end - start
+ newBuf = new Buffer(sliceLen, undefined)
+ for (var i = 0; i < sliceLen; i++) {
+ newBuf[i] = this[i + start]
+ }
+ }
+
+ if (newBuf.length) newBuf.parent = this.parent || this
+
+ return newBuf
+}
+
+/*
+ * Need to make sure that buffer isn't trying to write out of bounds.
+ */
+function checkOffset (offset, ext, length) {
+ if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint')
+ if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length')
+}
+
+Buffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var val = this[offset]
+ var mul = 1
+ var i = 0
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul
+ }
+
+ return val
+}
+
+Buffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) {
+ checkOffset(offset, byteLength, this.length)
+ }
+
+ var val = this[offset + --byteLength]
+ var mul = 1
+ while (byteLength > 0 && (mul *= 0x100)) {
+ val += this[offset + --byteLength] * mul
+ }
+
+ return val
+}
+
+Buffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 1, this.length)
+ return this[offset]
+}
+
+Buffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ return this[offset] | (this[offset + 1] << 8)
+}
+
+Buffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ return (this[offset] << 8) | this[offset + 1]
+}
+
+Buffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return ((this[offset]) |
+ (this[offset + 1] << 8) |
+ (this[offset + 2] << 16)) +
+ (this[offset + 3] * 0x1000000)
+}
+
+Buffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset] * 0x1000000) +
+ ((this[offset + 1] << 16) |
+ (this[offset + 2] << 8) |
+ this[offset + 3])
+}
+
+Buffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var val = this[offset]
+ var mul = 1
+ var i = 0
+ while (++i < byteLength && (mul *= 0x100)) {
+ val += this[offset + i] * mul
+ }
+ mul *= 0x80
+
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength)
+
+ return val
+}
+
+Buffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) {
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkOffset(offset, byteLength, this.length)
+
+ var i = byteLength
+ var mul = 1
+ var val = this[offset + --i]
+ while (i > 0 && (mul *= 0x100)) {
+ val += this[offset + --i] * mul
+ }
+ mul *= 0x80
+
+ if (val >= mul) val -= Math.pow(2, 8 * byteLength)
+
+ return val
+}
+
+Buffer.prototype.readInt8 = function readInt8 (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 1, this.length)
+ if (!(this[offset] & 0x80)) return (this[offset])
+ return ((0xff - this[offset] + 1) * -1)
+}
+
+Buffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ var val = this[offset] | (this[offset + 1] << 8)
+ return (val & 0x8000) ? val | 0xFFFF0000 : val
+}
+
+Buffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 2, this.length)
+ var val = this[offset + 1] | (this[offset] << 8)
+ return (val & 0x8000) ? val | 0xFFFF0000 : val
+}
+
+Buffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset]) |
+ (this[offset + 1] << 8) |
+ (this[offset + 2] << 16) |
+ (this[offset + 3] << 24)
+}
+
+Buffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+
+ return (this[offset] << 24) |
+ (this[offset + 1] << 16) |
+ (this[offset + 2] << 8) |
+ (this[offset + 3])
+}
+
+Buffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+ return ieee754.read(this, offset, true, 23, 4)
+}
+
+Buffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 4, this.length)
+ return ieee754.read(this, offset, false, 23, 4)
+}
+
+Buffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 8, this.length)
+ return ieee754.read(this, offset, true, 52, 8)
+}
+
+Buffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) {
+ if (!noAssert) checkOffset(offset, 8, this.length)
+ return ieee754.read(this, offset, false, 52, 8)
+}
+
+function checkInt (buf, value, offset, ext, max, min) {
+ if (!Buffer.isBuffer(buf)) throw new TypeError('buffer must be a Buffer instance')
+ if (value > max || value < min) throw new RangeError('value is out of bounds')
+ if (offset + ext > buf.length) throw new RangeError('index out of range')
+}
+
+Buffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0)
+
+ var mul = 1
+ var i = 0
+ this[offset] = value & 0xFF
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = (value / mul) & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ byteLength = byteLength | 0
+ if (!noAssert) checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0)
+
+ var i = byteLength - 1
+ var mul = 1
+ this[offset + i] = value & 0xFF
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = (value / mul) & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0)
+ if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value)
+ this[offset] = (value & 0xff)
+ return offset + 1
+}
+
+function objectWriteUInt16 (buf, value, offset, littleEndian) {
+ if (value < 0) value = 0xffff + value + 1
+ for (var i = 0, j = Math.min(buf.length - offset, 2); i < j; i++) {
+ buf[offset + i] = (value & (0xff << (8 * (littleEndian ? i : 1 - i)))) >>>
+ (littleEndian ? i : 1 - i) * 8
+ }
+}
+
+Buffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ } else {
+ objectWriteUInt16(this, value, offset, true)
+ }
+ return offset + 2
+}
+
+Buffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 8)
+ this[offset + 1] = (value & 0xff)
+ } else {
+ objectWriteUInt16(this, value, offset, false)
+ }
+ return offset + 2
+}
+
+function objectWriteUInt32 (buf, value, offset, littleEndian) {
+ if (value < 0) value = 0xffffffff + value + 1
+ for (var i = 0, j = Math.min(buf.length - offset, 4); i < j; i++) {
+ buf[offset + i] = (value >>> (littleEndian ? i : 3 - i) * 8) & 0xff
+ }
+}
+
+Buffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset + 3] = (value >>> 24)
+ this[offset + 2] = (value >>> 16)
+ this[offset + 1] = (value >>> 8)
+ this[offset] = (value & 0xff)
+ } else {
+ objectWriteUInt32(this, value, offset, true)
+ }
+ return offset + 4
+}
+
+Buffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 24)
+ this[offset + 1] = (value >>> 16)
+ this[offset + 2] = (value >>> 8)
+ this[offset + 3] = (value & 0xff)
+ } else {
+ objectWriteUInt32(this, value, offset, false)
+ }
+ return offset + 4
+}
+
+Buffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1)
+
+ checkInt(this, value, offset, byteLength, limit - 1, -limit)
+ }
+
+ var i = 0
+ var mul = 1
+ var sub = value < 0 ? 1 : 0
+ this[offset] = value & 0xFF
+ while (++i < byteLength && (mul *= 0x100)) {
+ this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) {
+ var limit = Math.pow(2, 8 * byteLength - 1)
+
+ checkInt(this, value, offset, byteLength, limit - 1, -limit)
+ }
+
+ var i = byteLength - 1
+ var mul = 1
+ var sub = value < 0 ? 1 : 0
+ this[offset + i] = value & 0xFF
+ while (--i >= 0 && (mul *= 0x100)) {
+ this[offset + i] = ((value / mul) >> 0) - sub & 0xFF
+ }
+
+ return offset + byteLength
+}
+
+Buffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80)
+ if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value)
+ if (value < 0) value = 0xff + value + 1
+ this[offset] = (value & 0xff)
+ return offset + 1
+}
+
+Buffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ } else {
+ objectWriteUInt16(this, value, offset, true)
+ }
+ return offset + 2
+}
+
+Buffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 8)
+ this[offset + 1] = (value & 0xff)
+ } else {
+ objectWriteUInt16(this, value, offset, false)
+ }
+ return offset + 2
+}
+
+Buffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value & 0xff)
+ this[offset + 1] = (value >>> 8)
+ this[offset + 2] = (value >>> 16)
+ this[offset + 3] = (value >>> 24)
+ } else {
+ objectWriteUInt32(this, value, offset, true)
+ }
+ return offset + 4
+}
+
+Buffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) {
+ value = +value
+ offset = offset | 0
+ if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000)
+ if (value < 0) value = 0xffffffff + value + 1
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ this[offset] = (value >>> 24)
+ this[offset + 1] = (value >>> 16)
+ this[offset + 2] = (value >>> 8)
+ this[offset + 3] = (value & 0xff)
+ } else {
+ objectWriteUInt32(this, value, offset, false)
+ }
+ return offset + 4
+}
+
+function checkIEEE754 (buf, value, offset, ext, max, min) {
+ if (value > max || value < min) throw new RangeError('value is out of bounds')
+ if (offset + ext > buf.length) throw new RangeError('index out of range')
+ if (offset < 0) throw new RangeError('index out of range')
+}
+
+function writeFloat (buf, value, offset, littleEndian, noAssert) {
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38)
+ }
+ ieee754.write(buf, value, offset, littleEndian, 23, 4)
+ return offset + 4
+}
+
+Buffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) {
+ return writeFloat(this, value, offset, true, noAssert)
+}
+
+Buffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) {
+ return writeFloat(this, value, offset, false, noAssert)
+}
+
+function writeDouble (buf, value, offset, littleEndian, noAssert) {
+ if (!noAssert) {
+ checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308)
+ }
+ ieee754.write(buf, value, offset, littleEndian, 52, 8)
+ return offset + 8
+}
+
+Buffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) {
+ return writeDouble(this, value, offset, true, noAssert)
+}
+
+Buffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) {
+ return writeDouble(this, value, offset, false, noAssert)
+}
+
+// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length)
+Buffer.prototype.copy = function copy (target, targetStart, start, end) {
+ if (!start) start = 0
+ if (!end && end !== 0) end = this.length
+ if (targetStart >= target.length) targetStart = target.length
+ if (!targetStart) targetStart = 0
+ if (end > 0 && end < start) end = start
+
+ // Copy 0 bytes; we're done
+ if (end === start) return 0
+ if (target.length === 0 || this.length === 0) return 0
+
+ // Fatal error conditions
+ if (targetStart < 0) {
+ throw new RangeError('targetStart out of bounds')
+ }
+ if (start < 0 || start >= this.length) throw new RangeError('sourceStart out of bounds')
+ if (end < 0) throw new RangeError('sourceEnd out of bounds')
+
+ // Are we oob?
+ if (end > this.length) end = this.length
+ if (target.length - targetStart < end - start) {
+ end = target.length - targetStart + start
+ }
+
+ var len = end - start
+ var i
+
+ if (this === target && start < targetStart && targetStart < end) {
+ // descending copy from end
+ for (i = len - 1; i >= 0; i--) {
+ target[i + targetStart] = this[i + start]
+ }
+ } else if (len < 1000 || !Buffer.TYPED_ARRAY_SUPPORT) {
+ // ascending copy from start
+ for (i = 0; i < len; i++) {
+ target[i + targetStart] = this[i + start]
+ }
+ } else {
+ target._set(this.subarray(start, start + len), targetStart)
+ }
+
+ return len
+}
+
+// fill(value, start=0, end=buffer.length)
+Buffer.prototype.fill = function fill (value, start, end) {
+ if (!value) value = 0
+ if (!start) start = 0
+ if (!end) end = this.length
+
+ if (end < start) throw new RangeError('end < start')
+
+ // Fill 0 bytes; we're done
+ if (end === start) return
+ if (this.length === 0) return
+
+ if (start < 0 || start >= this.length) throw new RangeError('start out of bounds')
+ if (end < 0 || end > this.length) throw new RangeError('end out of bounds')
+
+ var i
+ if (typeof value === 'number') {
+ for (i = start; i < end; i++) {
+ this[i] = value
+ }
+ } else {
+ var bytes = utf8ToBytes(value.toString())
+ var len = bytes.length
+ for (i = start; i < end; i++) {
+ this[i] = bytes[i % len]
+ }
+ }
+
+ return this
+}
+
+/**
+ * Creates a new `ArrayBuffer` with the *copied* memory of the buffer instance.
+ * Added in Node 0.12. Only available in browsers that support ArrayBuffer.
+ */
+Buffer.prototype.toArrayBuffer = function toArrayBuffer () {
+ if (typeof Uint8Array !== 'undefined') {
+ if (Buffer.TYPED_ARRAY_SUPPORT) {
+ return (new Buffer(this)).buffer
+ } else {
+ var buf = new Uint8Array(this.length)
+ for (var i = 0, len = buf.length; i < len; i += 1) {
+ buf[i] = this[i]
+ }
+ return buf.buffer
+ }
+ } else {
+ throw new TypeError('Buffer.toArrayBuffer not supported in this browser')
+ }
+}
+
+// HELPER FUNCTIONS
+// ================
+
+var BP = Buffer.prototype
+
+/**
+ * Augment a Uint8Array *instance* (not the Uint8Array class!) with Buffer methods
+ */
+Buffer._augment = function _augment (arr) {
+ arr.constructor = Buffer
+ arr._isBuffer = true
+
+ // save reference to original Uint8Array set method before overwriting
+ arr._set = arr.set
+
+ // deprecated
+ arr.get = BP.get
+ arr.set = BP.set
+
+ arr.write = BP.write
+ arr.toString = BP.toString
+ arr.toLocaleString = BP.toString
+ arr.toJSON = BP.toJSON
+ arr.equals = BP.equals
+ arr.compare = BP.compare
+ arr.indexOf = BP.indexOf
+ arr.copy = BP.copy
+ arr.slice = BP.slice
+ arr.readUIntLE = BP.readUIntLE
+ arr.readUIntBE = BP.readUIntBE
+ arr.readUInt8 = BP.readUInt8
+ arr.readUInt16LE = BP.readUInt16LE
+ arr.readUInt16BE = BP.readUInt16BE
+ arr.readUInt32LE = BP.readUInt32LE
+ arr.readUInt32BE = BP.readUInt32BE
+ arr.readIntLE = BP.readIntLE
+ arr.readIntBE = BP.readIntBE
+ arr.readInt8 = BP.readInt8
+ arr.readInt16LE = BP.readInt16LE
+ arr.readInt16BE = BP.readInt16BE
+ arr.readInt32LE = BP.readInt32LE
+ arr.readInt32BE = BP.readInt32BE
+ arr.readFloatLE = BP.readFloatLE
+ arr.readFloatBE = BP.readFloatBE
+ arr.readDoubleLE = BP.readDoubleLE
+ arr.readDoubleBE = BP.readDoubleBE
+ arr.writeUInt8 = BP.writeUInt8
+ arr.writeUIntLE = BP.writeUIntLE
+ arr.writeUIntBE = BP.writeUIntBE
+ arr.writeUInt16LE = BP.writeUInt16LE
+ arr.writeUInt16BE = BP.writeUInt16BE
+ arr.writeUInt32LE = BP.writeUInt32LE
+ arr.writeUInt32BE = BP.writeUInt32BE
+ arr.writeIntLE = BP.writeIntLE
+ arr.writeIntBE = BP.writeIntBE
+ arr.writeInt8 = BP.writeInt8
+ arr.writeInt16LE = BP.writeInt16LE
+ arr.writeInt16BE = BP.writeInt16BE
+ arr.writeInt32LE = BP.writeInt32LE
+ arr.writeInt32BE = BP.writeInt32BE
+ arr.writeFloatLE = BP.writeFloatLE
+ arr.writeFloatBE = BP.writeFloatBE
+ arr.writeDoubleLE = BP.writeDoubleLE
+ arr.writeDoubleBE = BP.writeDoubleBE
+ arr.fill = BP.fill
+ arr.inspect = BP.inspect
+ arr.toArrayBuffer = BP.toArrayBuffer
+
+ return arr
+}
+
+var INVALID_BASE64_RE = /[^+\/0-9A-Za-z-_]/g
+
+function base64clean (str) {
+ // Node strips out invalid characters like \n and \t from the string, base64-js does not
+ str = stringtrim(str).replace(INVALID_BASE64_RE, '')
+ // Node converts strings with length < 2 to ''
+ if (str.length < 2) return ''
+ // Node allows for non-padded base64 strings (missing trailing ===), base64-js does not
+ while (str.length % 4 !== 0) {
+ str = str + '='
+ }
+ return str
+}
+
+function stringtrim (str) {
+ if (str.trim) return str.trim()
+ return str.replace(/^\s+|\s+$/g, '')
+}
+
+function toHex (n) {
+ if (n < 16) return '0' + n.toString(16)
+ return n.toString(16)
+}
+
+function utf8ToBytes (string, units) {
+ units = units || Infinity
+ var codePoint
+ var length = string.length
+ var leadSurrogate = null
+ var bytes = []
+
+ for (var i = 0; i < length; i++) {
+ codePoint = string.charCodeAt(i)
+
+ // is surrogate component
+ if (codePoint > 0xD7FF && codePoint < 0xE000) {
+ // last char was a lead
+ if (!leadSurrogate) {
+ // no lead yet
+ if (codePoint > 0xDBFF) {
+ // unexpected trail
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ continue
+ } else if (i + 1 === length) {
+ // unpaired lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ continue
+ }
+
+ // valid lead
+ leadSurrogate = codePoint
+
+ continue
+ }
+
+ // 2 leads in a row
+ if (codePoint < 0xDC00) {
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ leadSurrogate = codePoint
+ continue
+ }
+
+ // valid surrogate pair
+ codePoint = leadSurrogate - 0xD800 << 10 | codePoint - 0xDC00 | 0x10000
+ } else if (leadSurrogate) {
+ // valid bmp char, but last char was a lead
+ if ((units -= 3) > -1) bytes.push(0xEF, 0xBF, 0xBD)
+ }
+
+ leadSurrogate = null
+
+ // encode utf8
+ if (codePoint < 0x80) {
+ if ((units -= 1) < 0) break
+ bytes.push(codePoint)
+ } else if (codePoint < 0x800) {
+ if ((units -= 2) < 0) break
+ bytes.push(
+ codePoint >> 0x6 | 0xC0,
+ codePoint & 0x3F | 0x80
+ )
+ } else if (codePoint < 0x10000) {
+ if ((units -= 3) < 0) break
+ bytes.push(
+ codePoint >> 0xC | 0xE0,
+ codePoint >> 0x6 & 0x3F | 0x80,
+ codePoint & 0x3F | 0x80
+ )
+ } else if (codePoint < 0x110000) {
+ if ((units -= 4) < 0) break
+ bytes.push(
+ codePoint >> 0x12 | 0xF0,
+ codePoint >> 0xC & 0x3F | 0x80,
+ codePoint >> 0x6 & 0x3F | 0x80,
+ codePoint & 0x3F | 0x80
+ )
+ } else {
+ throw new Error('Invalid code point')
+ }
+ }
+
+ return bytes
+}
+
+function asciiToBytes (str) {
+ var byteArray = []
+ for (var i = 0; i < str.length; i++) {
+ // Node's code seems to be doing this and not & 0x7F..
+ byteArray.push(str.charCodeAt(i) & 0xFF)
+ }
+ return byteArray
+}
+
+function utf16leToBytes (str, units) {
+ var c, hi, lo
+ var byteArray = []
+ for (var i = 0; i < str.length; i++) {
+ if ((units -= 2) < 0) break
+
+ c = str.charCodeAt(i)
+ hi = c >> 8
+ lo = c % 256
+ byteArray.push(lo)
+ byteArray.push(hi)
+ }
+
+ return byteArray
+}
+
+function base64ToBytes (str) {
+ return base64.toByteArray(base64clean(str))
+}
+
+function blitBuffer (src, dst, offset, length) {
+ for (var i = 0; i < length; i++) {
+ if ((i + offset >= dst.length) || (i >= src.length)) break
+ dst[i + offset] = src[i]
+ }
+ return i
+}
+
+}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {})
+},{"base64-js":5,"ieee754":8,"is-array":9}],8:[function(require,module,exports){
+exports.read = function (buffer, offset, isLE, mLen, nBytes) {
+ var e, m
+ var eLen = nBytes * 8 - mLen - 1
+ var eMax = (1 << eLen) - 1
+ var eBias = eMax >> 1
+ var nBits = -7
+ var i = isLE ? (nBytes - 1) : 0
+ var d = isLE ? -1 : 1
+ var s = buffer[offset + i]
+
+ i += d
+
+ e = s & ((1 << (-nBits)) - 1)
+ s >>= (-nBits)
+ nBits += eLen
+ for (; nBits > 0; e = e * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ m = e & ((1 << (-nBits)) - 1)
+ e >>= (-nBits)
+ nBits += mLen
+ for (; nBits > 0; m = m * 256 + buffer[offset + i], i += d, nBits -= 8) {}
+
+ if (e === 0) {
+ e = 1 - eBias
+ } else if (e === eMax) {
+ return m ? NaN : ((s ? -1 : 1) * Infinity)
+ } else {
+ m = m + Math.pow(2, mLen)
+ e = e - eBias
+ }
+ return (s ? -1 : 1) * m * Math.pow(2, e - mLen)
+}
+
+exports.write = function (buffer, value, offset, isLE, mLen, nBytes) {
+ var e, m, c
+ var eLen = nBytes * 8 - mLen - 1
+ var eMax = (1 << eLen) - 1
+ var eBias = eMax >> 1
+ var rt = (mLen === 23 ? Math.pow(2, -24) - Math.pow(2, -77) : 0)
+ var i = isLE ? 0 : (nBytes - 1)
+ var d = isLE ? 1 : -1
+ var s = value < 0 || (value === 0 && 1 / value < 0) ? 1 : 0
+
+ value = Math.abs(value)
+
+ if (isNaN(value) || value === Infinity) {
+ m = isNaN(value) ? 1 : 0
+ e = eMax
+ } else {
+ e = Math.floor(Math.log(value) / Math.LN2)
+ if (value * (c = Math.pow(2, -e)) < 1) {
+ e--
+ c *= 2
+ }
+ if (e + eBias >= 1) {
+ value += rt / c
+ } else {
+ value += rt * Math.pow(2, 1 - eBias)
+ }
+ if (value * c >= 2) {
+ e++
+ c /= 2
+ }
+
+ if (e + eBias >= eMax) {
+ m = 0
+ e = eMax
+ } else if (e + eBias >= 1) {
+ m = (value * c - 1) * Math.pow(2, mLen)
+ e = e + eBias
+ } else {
+ m = value * Math.pow(2, eBias - 1) * Math.pow(2, mLen)
+ e = 0
+ }
+ }
+
+ for (; mLen >= 8; buffer[offset + i] = m & 0xff, i += d, m /= 256, mLen -= 8) {}
+
+ e = (e << mLen) | m
+ eLen += mLen
+ for (; eLen > 0; buffer[offset + i] = e & 0xff, i += d, e /= 256, eLen -= 8) {}
+
+ buffer[offset + i - d] |= s * 128
+}
+
+},{}],9:[function(require,module,exports){
+
+/**
+ * isArray
+ */
+
+var isArray = Array.isArray;
+
+/**
+ * toString
+ */
+
+var str = Object.prototype.toString;
+
+/**
+ * Whether or not the given `val`
+ * is an array.
+ *
+ * example:
+ *
+ * isArray([]);
+ * // > true
+ * isArray(arguments);
+ * // > false
+ * isArray('');
+ * // > false
+ *
+ * @param {mixed} val
+ * @return {bool}
+ */
+
+module.exports = isArray || function (val) {
+ return !! val && '[object Array]' == str.call(val);
+};
+
+},{}],10:[function(require,module,exports){
+/**
+ * Determine if an object is Buffer
+ *
+ * Author: Feross Aboukhadijeh <fe...@feross.org> <http://feross.org>
+ * License: MIT
+ *
+ * `npm install is-buffer`
+ */
+
+module.exports = function (obj) {
+ return !!(obj != null &&
+ (obj._isBuffer || // For Safari 5-7 (missing Object.prototype.constructor)
+ (obj.constructor &&
+ typeof obj.constructor.isBuffer === 'function' &&
+ obj.constructor.isBuffer(obj))
+ ))
+}
+
+},{}],11:[function(require,module,exports){
+/**
+ * Gets the last element of `array`.
+ *
+ * @static
+ * @memberOf _
+ * @category Array
+ * @param {Array} array The array to query.
+ * @returns {*} Returns the last element of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ */
+function last(array) {
+ var length = array ? array.length : 0;
+ return length ? array[length - 1] : undefined;
+}
+
+module.exports = last;
+
+},{}],12:[function(require,module,exports){
+var arrayEvery = require('../internal/arrayEvery'),
+ baseCallback = require('../internal/baseCallback'),
+ baseEvery = require('../internal/baseEvery'),
+ isArray = require('../lang/isArray'),
+ isIterateeCall = require('../internal/isIterateeCall');
+
+/**
+ * Checks if `predicate` returns truthy for **all** elements of `collection`.
+ * The predicate is bound to `thisArg` and invoked with three arguments:
+ * (value, index|key, collection).
+ *
+ * If a property name is provided for `predicate` the created `_.property`
+ * style callback returns the property value of the given element.
+ *
+ * If a value is also provided for `thisArg` the created `_.matchesProperty`
+ * style callback returns `true` for elements that have a matching property
+ * value, else `false`.
+ *
+ * If an object is provided for `predicate` the created `_.matches` style
+ * callback returns `true` for elements that have the properties of the given
+ * object, else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collection
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function|Object|string} [predicate=_.identity] The function invoked
+ * per iteration.
+ * @param {*} [thisArg] The `this` binding of `predicate`.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var users = [
+ * { 'user': 'barney', 'active': false },
+ * { 'user': 'fred', 'active': false }
+ * ];
+ *
+ * // using the `_.matches` callback shorthand
+ * _.every(users, { 'user': 'barney', 'active': false });
+ * // => false
+ *
+ * // using the `_.matchesProperty` callback shorthand
+ * _.every(users, 'active', false);
+ * // => true
+ *
+ * // using the `_.property` callback shorthand
+ * _.every(users, 'active');
+ * // => false
+ */
+function every(collection, predicate, thisArg) {
+ var func = isArray(collection) ? arrayEvery : baseEvery;
+ if (thisArg && isIterateeCall(collection, predicate, thisArg)) {
+ predicate = undefined;
+ }
+ if (typeof predicate != 'function' || thisArg !== undefined) {
+ predicate = baseCallback(predicate, thisArg, 3);
+ }
+ return func(collection, predicate);
+}
+
+module.exports = every;
+
+},{"../internal/arrayEvery":14,"../internal/baseCallback":18,"../internal/baseEvery":22,"../internal/isIterateeCall":47,"../lang/isArray":56}],13:[function(require,module,exports){
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of the
+ * created function and arguments from `start` and beyond provided as an array.
+ *
+ * **Note:** This method is based on the [rest parameter](https://developer.mozilla.org/Web/JavaScript/Reference/Functions/rest_parameters).
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.restParam(function(what, names) {
+ * return what + ' ' + _.initial(names).join(', ') +
+ * (_.size(names) > 1 ? ', & ' : '') + _.last(names);
+ * });
+ *
+ * say('hello', 'fred', 'barney', 'pebbles');
+ * // => 'hello fred, barney, & pebbles'
+ */
+function restParam(func, start) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ start = nativeMax(start === undefined ? (func.length - 1) : (+start || 0), 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ rest = Array(length);
+
+ while (++index < length) {
+ rest[index] = args[start + index];
+ }
+ switch (start) {
+ case 0: return func.call(this, rest);
+ case 1: return func.call(this, args[0], rest);
+ case 2: return func.call(this, args[0], args[1], rest);
+ }
+ var otherArgs = Array(start + 1);
+ index = -1;
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = rest;
+ return func.apply(this, otherArgs);
+ };
+}
+
+module.exports = restParam;
+
+},{}],14:[function(require,module,exports){
+/**
+ * A specialized version of `_.every` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ */
+function arrayEvery(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (!predicate(array[index], index, array)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = arrayEvery;
+
+},{}],15:[function(require,module,exports){
+/**
+ * A specialized version of `_.some` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+function arraySome(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (predicate(array[index], index, array)) {
+ return true;
+ }
+ }
+ return false;
+}
+
+module.exports = arraySome;
+
+},{}],16:[function(require,module,exports){
+var keys = require('../object/keys');
+
+/**
+ * A specialized version of `_.assign` for customizing assigned values without
+ * support for argument juggling, multiple sources, and `this` binding `customizer`
+ * functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ */
+function assignWith(object, source, customizer) {
+ var index = -1,
+ props = keys(source),
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index],
+ value = object[key],
+ result = customizer(value, source[key], key, object, source);
+
+ if ((result === result ? (result !== value) : (value === value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = result;
+ }
+ }
+ return object;
+}
+
+module.exports = assignWith;
+
+},{"../object/keys":65}],17:[function(require,module,exports){
+var baseCopy = require('./baseCopy'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.assign` without support for argument juggling,
+ * multiple sources, and `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssign(object, source) {
+ return source == null
+ ? object
+ : baseCopy(source, keys(source), object);
+}
+
+module.exports = baseAssign;
+
+},{"../object/keys":65,"./baseCopy":19}],18:[function(require,module,exports){
+var baseMatches = require('./baseMatches'),
+ baseMatchesProperty = require('./baseMatchesProperty'),
+ bindCallback = require('./bindCallback'),
+ identity = require('../utility/identity'),
+ property = require('../utility/property');
+
+/**
+ * The base implementation of `_.callback` which supports specifying the
+ * number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Returns the callback.
+ */
+function baseCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (type == 'function') {
+ return thisArg === undefined
+ ? func
+ : bindCallback(func, thisArg, argCount);
+ }
+ if (func == null) {
+ return identity;
+ }
+ if (type == 'object') {
+ return baseMatches(func);
+ }
+ return thisArg === undefined
+ ? property(func)
+ : baseMatchesProperty(func, thisArg);
+}
+
+module.exports = baseCallback;
+
+},{"../utility/identity":68,"../utility/property":69,"./baseMatches":29,"./baseMatchesProperty":30,"./bindCallback":35}],19:[function(require,module,exports){
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property names to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @returns {Object} Returns `object`.
+ */
+function baseCopy(source, props, object) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ object[key] = source[key];
+ }
+ return object;
+}
+
+module.exports = baseCopy;
+
+},{}],20:[function(require,module,exports){
+var isObject = require('../lang/isObject');
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+var baseCreate = (function() {
+ function object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ object.prototype = prototype;
+ var result = new object;
+ object.prototype = undefined;
+ }
+ return result || {};
+ };
+}());
+
+module.exports = baseCreate;
+
+},{"../lang/isObject":60}],21:[function(require,module,exports){
+var baseForOwn = require('./baseForOwn'),
+ createBaseEach = require('./createBaseEach');
+
+/**
+ * The base implementation of `_.forEach` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+var baseEach = createBaseEach(baseForOwn);
+
+module.exports = baseEach;
+
+},{"./baseForOwn":24,"./createBaseEach":37}],22:[function(require,module,exports){
+var baseEach = require('./baseEach');
+
+/**
+ * The base implementation of `_.every` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+}
+
+module.exports = baseEvery;
+
+},{"./baseEach":21}],23:[function(require,module,exports){
+var createBaseFor = require('./createBaseFor');
+
+/**
+ * The base implementation of `baseForIn` and `baseForOwn` which iterates
+ * over `object` properties returned by `keysFunc` invoking `iteratee` for
+ * each property. Iteratee functions may exit iteration early by explicitly
+ * returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseFor = createBaseFor();
+
+module.exports = baseFor;
+
+},{"./createBaseFor":38}],24:[function(require,module,exports){
+var baseFor = require('./baseFor'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.forOwn` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForOwn(object, iteratee) {
+ return baseFor(object, iteratee, keys);
+}
+
+module.exports = baseForOwn;
+
+},{"../object/keys":65,"./baseFor":23}],25:[function(require,module,exports){
+var toObject = require('./toObject');
+
+/**
+ * The base implementation of `get` without support for string paths
+ * and default values.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} path The path of the property to get.
+ * @param {string} [pathKey] The key representation of path.
+ * @returns {*} Returns the resolved value.
+ */
+function baseGet(object, path, pathKey) {
+ if (object == null) {
+ return;
+ }
+ if (pathKey !== undefined && pathKey in toObject(object)) {
+ path = [pathKey];
+ }
+ var index = 0,
+ length = path.length;
+
+ while (object != null && index < length) {
+ object = object[path[index++]];
+ }
+ return (index && index == length) ? object : undefined;
+}
+
+module.exports = baseGet;
+
+},{"./toObject":53}],26:[function(require,module,exports){
+var baseIsEqualDeep = require('./baseIsEqualDeep'),
+ isObject = require('../lang/isObject'),
+ isObjectLike = require('./isObjectLike');
+
+/**
+ * The base implementation of `_.isEqual` without support for `this` binding
+ * `customizer` functions.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize comparing values.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(value, other, customizer, isLoose, stackA, stackB) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, baseIsEqual, customizer, isLoose, stackA, stackB);
+}
+
+module.exports = baseIsEqual;
+
+},{"../lang/isObject":60,"./baseIsEqualDeep":27,"./isObjectLike":50}],27:[function(require,module,exports){
+var equalArrays = require('./equalArrays'),
+ equalByTag = require('./equalByTag'),
+ equalObjects = require('./equalObjects'),
+ isArray = require('../lang/isArray'),
+ isTypedArray = require('../lang/isTypedArray');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ objectTag = '[object Object]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `value` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function baseIsEqualDeep(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = arrayTag,
+ othTag = arrayTag;
+
+ if (!objIsArr) {
+ objTag = objToString.call(object);
+ if (objTag == argsTag) {
+ objTag = objectTag;
+ } else if (objTag != objectTag) {
+ objIsArr = isTypedArray(object);
+ }
+ }
+ if (!othIsArr) {
+ othTag = objToString.call(other);
+ if (othTag == argsTag) {
+ othTag = objectTag;
+ } else if (othTag != objectTag) {
+ othIsArr = isTypedArray(other);
+ }
+ }
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ if (isSameTag && !(objIsArr || objIsObj)) {
+ return equalByTag(object, other, objTag);
+ }
+ if (!isLoose) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ return equalFunc(objIsWrapped ? object.value() : object, othIsWrapped ? other.value() : other, customizer, isLoose, stackA, stackB);
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ // Assume cyclic values are equal.
+ // For more information on detecting circular references see https://es5.github.io/#JO.
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == object) {
+ return stackB[length] == other;
+ }
+ }
+ // Add `object` and `other` to the stack of traversed objects.
+ stackA.push(object);
+ stackB.push(other);
+
+ var result = (objIsArr ? equalArrays : equalObjects)(object, other, equalFunc, customizer, isLoose, stackA, stackB);
+
+ stackA.pop();
+ stackB.pop();
+
+ return result;
+}
+
+module.exports = baseIsEqualDeep;
+
+},{"../lang/isArray":56,"../lang/isTypedArray":62,"./equalArrays":39,"./equalByTag":40,"./equalObjects":41}],28:[function(require,module,exports){
+var baseIsEqual = require('./baseIsEqual'),
+ toObject = require('./toObject');
+
+/**
+ * The base implementation of `_.isMatch` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} matchData The propery names, values, and compare flags to match.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ */
+function baseIsMatch(object, matchData, customizer) {
+ var index = matchData.length,
+ length = index,
+ noCustomizer = !customizer;
+
+ if (object == null) {
+ return !length;
+ }
+ object = toObject(object);
+ while (index--) {
+ var data = matchData[index];
+ if ((noCustomizer && data[2])
+ ? data[1] !== object[data[0]]
+ : !(data[0] in object)
+ ) {
+ return false;
+ }
+ }
+ while (++index < length) {
+ data = matchData[index];
+ var key = data[0],
+ objValue = object[key],
+ srcValue = data[1];
+
+ if (noCustomizer && data[2]) {
+ if (objValue === undefined && !(key in object)) {
+ return false;
+ }
+ } else {
+ var result = customizer ? customizer(objValue, srcValue, key) : undefined;
+ if (!(result === undefined ? baseIsEqual(srcValue, objValue, customizer, true) : result)) {
+ return false;
+ }
+ }
+ }
+ return true;
+}
+
+module.exports = baseIsMatch;
+
+},{"./baseIsEqual":26,"./toObject":53}],29:[function(require,module,exports){
+var baseIsMatch = require('./baseIsMatch'),
+ getMatchData = require('./getMatchData'),
+ toObject = require('./toObject');
+
+/**
+ * The base implementation of `_.matches` which does not clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new function.
+ */
+function baseMatches(source) {
+ var matchData = getMatchData(source);
+ if (matchData.length == 1 && matchData[0][2]) {
+ var key = matchData[0][0],
+ value = matchData[0][1];
+
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ return object[key] === value && (value !== undefined || (key in toObject(object)));
+ };
+ }
+ return function(object) {
+ return baseIsMatch(object, matchData);
+ };
+}
+
+module.exports = baseMatches;
+
+},{"./baseIsMatch":28,"./getMatchData":43,"./toObject":53}],30:[function(require,module,exports){
+var baseGet = require('./baseGet'),
+ baseIsEqual = require('./baseIsEqual'),
+ baseSlice = require('./baseSlice'),
+ isArray = require('../lang/isArray'),
+ isKey = require('./isKey'),
+ isStrictComparable = require('./isStrictComparable'),
+ last = require('../array/last'),
+ toObject = require('./toObject'),
+ toPath = require('./toPath');
+
+/**
+ * The base implementation of `_.matchesProperty` which does not clone `srcValue`.
+ *
+ * @private
+ * @param {string} path The path of the property to get.
+ * @param {*} srcValue The value to compare.
+ * @returns {Function} Returns the new function.
+ */
+function baseMatchesProperty(path, srcValue) {
+ var isArr = isArray(path),
+ isCommon = isKey(path) && isStrictComparable(srcValue),
+ pathKey = (path + '');
+
+ path = toPath(path);
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ var key = pathKey;
+ object = toObject(object);
+ if ((isArr || !isCommon) && !(key in object)) {
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ if (object == null) {
+ return false;
+ }
+ key = last(path);
+ object = toObject(object);
+ }
+ return object[key] === srcValue
+ ? (srcValue !== undefined || (key in object))
+ : baseIsEqual(srcValue, object[key], undefined, true);
+ };
+}
+
+module.exports = baseMatchesProperty;
+
+},{"../array/last":11,"../lang/isArray":56,"./baseGet":25,"./baseIsEqual":26,"./baseSlice":33,"./isKey":48,"./isStrictComparable":51,"./toObject":53,"./toPath":54}],31:[function(require,module,exports){
+/**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+module.exports = baseProperty;
+
+},{}],32:[function(require,module,exports){
+var baseGet = require('./baseGet'),
+ toPath = require('./toPath');
+
+/**
+ * A specialized version of `baseProperty` which supports deep paths.
+ *
+ * @private
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function basePropertyDeep(path) {
+ var pathKey = (path + '');
+ path = toPath(path);
+ return function(object) {
+ return baseGet(object, path, pathKey);
+ };
+}
+
+module.exports = basePropertyDeep;
+
+},{"./baseGet":25,"./toPath":54}],33:[function(require,module,exports){
+/**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ start = start == null ? 0 : (+start || 0);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : (+end || 0);
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+}
+
+module.exports = baseSlice;
+
+},{}],34:[function(require,module,exports){
+/**
+ * Converts `value` to a string if it's not one. An empty string is returned
+ * for `null` or `undefined` values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+function baseToString(value) {
+ return value == null ? '' : (value + '');
+}
+
+module.exports = baseToString;
+
+},{}],35:[function(require,module,exports){
+var identity = require('../utility/identity');
+
+/**
+ * A specialized version of `baseCallback` which only supports `this` binding
+ * and specifying the number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to bind.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Retur
<TRUNCATED>
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[53/59] [abbrv] mac commit: CB-10668 fixed failing tests,
reverted shelljs to 0.5.3
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/README.md
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/README.md b/node_modules/shelljs/README.md
index d6dcb63..d08d13e 100644
--- a/node_modules/shelljs/README.md
+++ b/node_modules/shelljs/README.md
@@ -1,12 +1,8 @@
-# ShellJS - Unix shell commands for Node.js
-
-[](https://gitter.im/shelljs/shelljs?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
-[](http://travis-ci.org/shelljs/shelljs)
-[](https://ci.appveyor.com/project/shelljs/shelljs)
+# ShellJS - Unix shell commands for Node.js [](http://travis-ci.org/arturadib/shelljs)
ShellJS is a portable **(Windows/Linux/OS X)** implementation of Unix shell commands on top of the Node.js API. You can use it to eliminate your shell script's dependency on Unix while still keeping its familiar and powerful commands. You can also install it globally so you can run it from outside Node projects - say goodbye to those gnarly Bash scripts!
-The project is [unit-tested](http://travis-ci.org/shelljs/shelljs) and battled-tested in projects like:
+The project is [unit-tested](http://travis-ci.org/arturadib/shelljs) and battled-tested in projects like:
+ [PDF.js](http://github.com/mozilla/pdf.js) - Firefox's next-gen PDF reader
+ [Firebug](http://getfirebug.com/) - Firefox's infamous debugger
@@ -17,7 +13,7 @@ The project is [unit-tested](http://travis-ci.org/shelljs/shelljs) and battled-t
and [many more](https://npmjs.org/browse/depended/shelljs).
-If you have feedback, suggestions, or need help, feel free to post in our [issue tracker](https://github.com/shelljs/shelljs/issues).
+Connect with [@r2r](http://twitter.com/r2r) on Twitter for questions, suggestions, etc.
## Installing
@@ -34,6 +30,9 @@ run ShellJS scripts much like any shell script from the command line, i.e. witho
$ shjs my_script
```
+You can also just copy `shell.js` into your project's directory, and `require()` accordingly.
+
+
## Examples
### JavaScript
@@ -68,8 +67,6 @@ if (exec('git commit -am "Auto-commit"').code !== 0) {
### CoffeeScript
-CoffeeScript is also supported automatically:
-
```coffeescript
require 'shelljs/global'
@@ -86,7 +83,7 @@ cd 'lib'
for file in ls '*.js'
sed '-i', 'BUILD_VERSION', 'v0.1.2', file
sed '-i', /.*REMOVE_THIS_LINE.*\n/, '', file
- sed '-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat('macro.js'), file
+ sed '-i', /.*REPLACE_LINE_WITH_MACRO.*\n/, cat 'macro.js', file
cd '..'
# Run external tool synchronously
@@ -108,38 +105,31 @@ shell.echo('hello world');
## Make tool
-A convenience script `shelljs/make` is also provided to mimic the behavior of a Unix Makefile.
-In this case all shell objects are global, and command line arguments will cause the script to
-execute only the corresponding function in the global `target` object. To avoid redundant calls,
-target functions are executed only once per script.
+A convenience script `shelljs/make` is also provided to mimic the behavior of a Unix Makefile. In this case all shell objects are global, and command line arguments will cause the script to execute only the corresponding function in the global `target` object. To avoid redundant calls, target functions are executed only once per script.
-Example:
+Example (CoffeeScript):
-```javascript
-require('shelljs/make');
+```coffeescript
+require 'shelljs/make'
+
+target.all = ->
+ target.bundle()
+ target.docs()
-target.all = function() {
- target.bundle();
- target.docs();
-};
-
-target.bundle = function() {
- cd(__dirname);
- mkdir('-p', 'build');
- cd('src');
- cat('*.js').to('../build/output.js');
-};
-
-target.docs = function() {
- cd(__dirname);
- mkdir('-p', 'docs');
- var files = ls('src/*.js');
- for(var i = 0; i < files.length; i++) {
- var text = grep('//@', files[i]); // extract special comments
- text = text.replace(/\/\/@/g, ''); // remove comment tags
- text.toEnd('docs/my_docs.md');
- }
-};
+target.bundle = ->
+ cd __dirname
+ mkdir 'build'
+ cd 'lib'
+ (cat '*.js').to '../build/output.js'
+
+target.docs = ->
+ cd __dirname
+ mkdir 'docs'
+ cd 'lib'
+ for file in ls '*.js'
+ text = grep '//@', file # extract special comments
+ text.replace '//@', '' # remove comment tags
+ text.to 'docs/my_docs.md'
```
To run the target `all`, call the above script without arguments: `$ node make`. To run the target `docs`: `$ node make docs`.
@@ -165,26 +155,20 @@ target.bundle = function(argsArray) {
All commands run synchronously, unless otherwise stated.
-### cd([dir])
-Changes to directory `dir` for the duration of the script. Changes to home
-directory if no argument is supplied.
+### cd('dir')
+Changes to directory `dir` for the duration of the script
### pwd()
Returns the current directory.
-### ls([options,] [path, ...])
-### ls([options,] path_array)
+### ls([options ,] path [,path ...])
+### ls([options ,] path_array)
Available options:
+ `-R`: recursive
+ `-A`: all files (include files beginning with `.`, except for `.` and `..`)
-+ `-d`: list directories themselves, not their contents
-+ `-l`: list objects representing each file, each with fields containing `ls
- -l` output fields. See
- [fs.Stats](https://nodejs.org/api/fs.html#fs_class_fs_stats)
- for more info
Examples:
@@ -192,13 +176,12 @@ Examples:
ls('projs/*.js');
ls('-R', '/users/me', '/tmp');
ls('-R', ['/users/me', '/tmp']); // same as above
-ls('-l', 'file.txt'); // { name: 'file.txt', mode: 33188, nlink: 1, ...}
```
Returns array of files in the given path, or in current directory if no path provided.
-### find(path [, path ...])
+### find(path [,path ...])
### find(path_array)
Examples:
@@ -214,12 +197,11 @@ The main difference from `ls('-R', path)` is that the resulting file names
include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
-### cp([options,] source [, source ...], dest)
-### cp([options,] source_array, dest)
+### cp([options ,] source [,source ...], dest)
+### cp([options ,] source_array, dest)
Available options:
-+ `-f`: force (default behavior)
-+ `-n`: no-clobber
++ `-f`: force
+ `-r, -R`: recursive
Examples:
@@ -233,8 +215,8 @@ cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
Copies files. The wildcard `*` is accepted.
-### rm([options,] file [, file ...])
-### rm([options,] file_array)
+### rm([options ,] file [, file ...])
+### rm([options ,] file_array)
Available options:
+ `-f`: force
@@ -251,17 +233,16 @@ rm(['some_file.txt', 'another_file.txt']); // same as above
Removes files. The wildcard `*` is accepted.
-### mv([options ,] source [, source ...], dest')
-### mv([options ,] source_array, dest')
+### mv(source [, source ...], dest')
+### mv(source_array, dest')
Available options:
-+ `-f`: force (default behavior)
-+ `-n`: no-clobber
++ `f`: force
Examples:
```javascript
-mv('-n', 'file', 'dir/');
+mv('-f', 'file', 'dir/');
mv('file1', 'file2', 'dir/');
mv(['file1', 'file2'], 'dir/'); // same as above
```
@@ -269,11 +250,11 @@ mv(['file1', 'file2'], 'dir/'); // same as above
Moves files. The wildcard `*` is accepted.
-### mkdir([options,] dir [, dir ...])
-### mkdir([options,] dir_array)
+### mkdir([options ,] dir [, dir ...])
+### mkdir([options ,] dir_array)
Available options:
-+ `-p`: full path (will create intermediate dirs if necessary)
++ `p`: full path (will create intermediate dirs if necessary)
Examples:
@@ -347,8 +328,7 @@ Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaS
those returned by `cat`, `grep`, etc).
-### sed([options,] search_regex, replacement, file [, file ...])
-### sed([options,] search_regex, replacement, file_array)
+### sed([options ,] search_regex, replacement, file)
Available options:
+ `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
@@ -360,12 +340,12 @@ sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
```
-Reads an input string from `files` and performs a JavaScript `replace()` on the input
+Reads an input string from `file` and performs a JavaScript `replace()` on the input
using the given search regex and replacement string or function. Returns the new string after replacement.
-### grep([options,] regex_filter, file [, file ...])
-### grep([options,] regex_filter, file_array)
+### grep([options ,] regex_filter, file [, file ...])
+### grep([options ,] regex_filter, file_array)
Available options:
+ `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
@@ -389,12 +369,11 @@ Examples:
var nodeExec = which('node');
```
-Searches for `command` in the system's PATH. On Windows, this uses the
-`PATHEXT` variable to append the extension if it's not already executable.
+Searches for `command` in the system's PATH. On Windows looks for `.exe`, `.cmd`, and `.bat` extensions.
Returns string containing the absolute path to the command.
-### echo(string [, string ...])
+### echo(string [,string ...])
Examples:
@@ -468,11 +447,12 @@ Display the list of currently remembered directories. Returns an array of paths
See also: pushd, popd
-### ln([options,] source, dest)
+### ln(options, source, dest)
+### ln(source, dest)
Available options:
-+ `-s`: symlink
-+ `-f`: force
++ `s`: symlink
++ `f`: force
Examples:
@@ -493,33 +473,29 @@ Object containing environment variables (both getter and setter). Shortcut to pr
### exec(command [, options] [, callback])
Available options (all `false` by default):
-+ `async`: Asynchronous execution. If a callback is provided, it will be set to
- `true`, regardless of the passed value.
++ `async`: Asynchronous execution. Defaults to true if a callback is provided.
+ `silent`: Do not echo program output to console.
-+ and any option available to NodeJS's
- [child_process.exec()](https://nodejs.org/api/child_process.html#child_process_child_process_exec_command_options_callback)
Examples:
```javascript
-var version = exec('node --version', {silent:true}).stdout;
+var version = exec('node --version', {silent:true}).output;
var child = exec('some_long_running_process', {async:true});
child.stdout.on('data', function(data) {
/* ... do something with data ... */
});
-exec('some_long_running_process', function(code, stdout, stderr) {
+exec('some_long_running_process', function(code, output) {
console.log('Exit code:', code);
- console.log('Program output:', stdout);
- console.log('Program stderr:', stderr);
+ console.log('Program output:', output);
});
```
-Executes the given `command` _synchronously_, unless otherwise specified. When in synchronous
-mode returns the object `{ code:..., stdout:... , stderr:... }`, containing the program's
-`stdout`, `stderr`, and its exit `code`. Otherwise returns the child process object,
-and the `callback` gets the arguments `(code, stdout, stderr)`.
+Executes the given `command` _synchronously_, unless otherwise specified.
+When in synchronous mode returns the object `{ code:..., output:... }`, containing the program's
+`output` (stdout + stderr) and its exit `code`. Otherwise returns the child process object, and
+the `callback` gets the arguments `(code, output)`.
**Note:** For long-lived processes, it's best to run `exec()` asynchronously as
the current synchronous implementation uses a lot of CPU. This should be getting
@@ -553,44 +529,6 @@ Notable exceptions:
+ There is no "quiet" option since default behavior is to run silent.
-### touch([options,] file)
-Available options:
-
-+ `-a`: Change only the access time
-+ `-c`: Do not create any files
-+ `-m`: Change only the modification time
-+ `-d DATE`: Parse DATE and use it instead of current time
-+ `-r FILE`: Use FILE's times instead of current time
-
-Examples:
-
-```javascript
-touch('source.js');
-touch('-c', '/path/to/some/dir/source.js');
-touch({ '-r': FILE }, '/path/to/some/dir/source.js');
-```
-
-Update the access and modification times of each FILE to the current time.
-A FILE argument that does not exist is created empty, unless -c is supplied.
-This is a partial implementation of *[touch(1)](http://linux.die.net/man/1/touch)*.
-
-
-### set(options)
-Available options:
-
-+ `+/-e`: exit upon error (`config.fatal`)
-+ `+/-v`: verbose: show all commands (`config.verbose`)
-
-Examples:
-
-```javascript
-set('-e'); // exit upon first error
-set('+e'); // this undoes a "set('-e')"
-```
-
-Sets global configuration variables
-
-
## Non-Unix commands
@@ -633,26 +571,9 @@ Example:
```javascript
require('shelljs/global');
-config.fatal = true; // or set('-e');
+config.fatal = true;
cp('this_file_does_not_exist', '/dev/null'); // dies here
/* more commands... */
```
-If `true` the script will die on errors. Default is `false`. This is
-analogous to Bash's `set -e`
-
-### config.verbose
-Example:
-
-```javascript
-config.verbose = true; // or set('-v');
-cd('dir/');
-ls('subdir/');
-```
-
-Will print each command as follows:
-
-```
-cd dir/
-ls subdir/
-```
+If `true` the script will die on errors. Default is `false`.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/bin/shjs
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/bin/shjs b/node_modules/shelljs/bin/shjs
index aae3bc6..d239a7a 100755
--- a/node_modules/shelljs/bin/shjs
+++ b/node_modules/shelljs/bin/shjs
@@ -37,9 +37,7 @@ if (scriptName.match(/\.coffee$/)) {
// CoffeeScript
//
if (which('coffee')) {
- exec('coffee "' + scriptName + '" ' + args.join(' '), function(code) {
- process.exit(code);
- });
+ exec('coffee ' + scriptName + ' ' + args.join(' '), { async: true });
} else {
console.log('ShellJS: CoffeeScript interpreter not found');
console.log();
@@ -49,7 +47,5 @@ if (scriptName.match(/\.coffee$/)) {
//
// JavaScript
//
- exec('node "' + scriptName + '" ' + args.join(' '), function(code) {
- process.exit(code);
- });
+ exec('node ' + scriptName + ' ' + args.join(' '), { async: true });
}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/build/output.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/build/output.js b/node_modules/shelljs/build/output.js
deleted file mode 100644
index 1b778b9..0000000
--- a/node_modules/shelljs/build/output.js
+++ /dev/null
@@ -1,2411 +0,0 @@
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### cat(file [, file ...])
-//@ ### cat(file_array)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var str = cat('file*.txt');
-//@ var str = cat('file1', 'file2');
-//@ var str = cat(['file1', 'file2']); // same as above
-//@ ```
-//@
-//@ Returns a string containing the given file, or a concatenated string
-//@ containing the files if more than one file is given (a new line character is
-//@ introduced between each file). Wildcard `*` accepted.
-function _cat(options, files) {
- var cat = '';
-
- if (!files)
- common.error('no paths given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 1);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- files.forEach(function(file) {
- if (!fs.existsSync(file))
- common.error('no such file or directory: ' + file);
-
- cat += fs.readFileSync(file, 'utf8');
- });
-
- return common.ShellString(cat);
-}
-module.exports = _cat;
-
-var fs = require('fs');
-var common = require('./common');
-
-//@
-//@ ### cd([dir])
-//@ Changes to directory `dir` for the duration of the script. Changes to home
-//@ directory if no argument is supplied.
-function _cd(options, dir) {
- if (!dir)
- dir = common.getUserHome();
-
- if (dir === '-') {
- if (!common.state.previousDir)
- common.error('could not find previous directory');
- else
- dir = common.state.previousDir;
- }
-
- if (!fs.existsSync(dir))
- common.error('no such file or directory: ' + dir);
-
- if (!fs.statSync(dir).isDirectory())
- common.error('not a directory: ' + dir);
-
- common.state.previousDir = process.cwd();
- process.chdir(dir);
-}
-module.exports = _cd;
-
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-var PERMS = (function (base) {
- return {
- OTHER_EXEC : base.EXEC,
- OTHER_WRITE : base.WRITE,
- OTHER_READ : base.READ,
-
- GROUP_EXEC : base.EXEC << 3,
- GROUP_WRITE : base.WRITE << 3,
- GROUP_READ : base.READ << 3,
-
- OWNER_EXEC : base.EXEC << 6,
- OWNER_WRITE : base.WRITE << 6,
- OWNER_READ : base.READ << 6,
-
- // Literal octal numbers are apparently not allowed in "strict" javascript. Using parseInt is
- // the preferred way, else a jshint warning is thrown.
- STICKY : parseInt('01000', 8),
- SETGID : parseInt('02000', 8),
- SETUID : parseInt('04000', 8),
-
- TYPE_MASK : parseInt('0770000', 8)
- };
-})({
- EXEC : 1,
- WRITE : 2,
- READ : 4
-});
-
-//@
-//@ ### chmod(octal_mode || octal_string, file)
-//@ ### chmod(symbolic_mode, file)
-//@
-//@ Available options:
-//@
-//@ + `-v`: output a diagnostic for every file processed//@
-//@ + `-c`: like verbose but report only when a change is made//@
-//@ + `-R`: change files and directories recursively//@
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ chmod(755, '/Users/brandon');
-//@ chmod('755', '/Users/brandon'); // same as above
-//@ chmod('u+x', '/Users/brandon');
-//@ ```
-//@
-//@ Alters the permissions of a file or directory by either specifying the
-//@ absolute permissions in octal form or expressing the changes in symbols.
-//@ This command tries to mimic the POSIX behavior as much as possible.
-//@ Notable exceptions:
-//@
-//@ + In symbolic modes, 'a-r' and '-r' are identical. No consideration is
-//@ given to the umask.
-//@ + There is no "quiet" option since default behavior is to run silent.
-function _chmod(options, mode, filePattern) {
- if (!filePattern) {
- if (options.length > 0 && options.charAt(0) === '-') {
- // Special case where the specified file permissions started with - to subtract perms, which
- // get picked up by the option parser as command flags.
- // If we are down by one argument and options starts with -, shift everything over.
- filePattern = mode;
- mode = options;
- options = '';
- }
- else {
- common.error('You must specify a file.');
- }
- }
-
- options = common.parseOptions(options, {
- 'R': 'recursive',
- 'c': 'changes',
- 'v': 'verbose'
- });
-
- if (typeof filePattern === 'string') {
- filePattern = [ filePattern ];
- }
-
- var files;
-
- if (options.recursive) {
- files = [];
- common.expand(filePattern).forEach(function addFile(expandedFile) {
- var stat = fs.lstatSync(expandedFile);
-
- if (!stat.isSymbolicLink()) {
- files.push(expandedFile);
-
- if (stat.isDirectory()) { // intentionally does not follow symlinks.
- fs.readdirSync(expandedFile).forEach(function (child) {
- addFile(expandedFile + '/' + child);
- });
- }
- }
- });
- }
- else {
- files = common.expand(filePattern);
- }
-
- files.forEach(function innerChmod(file) {
- file = path.resolve(file);
- if (!fs.existsSync(file)) {
- common.error('File not found: ' + file);
- }
-
- // When recursing, don't follow symlinks.
- if (options.recursive && fs.lstatSync(file).isSymbolicLink()) {
- return;
- }
-
- var stat = fs.statSync(file);
- var isDir = stat.isDirectory();
- var perms = stat.mode;
- var type = perms & PERMS.TYPE_MASK;
-
- var newPerms = perms;
-
- if (isNaN(parseInt(mode, 8))) {
- // parse options
- mode.split(',').forEach(function (symbolicMode) {
- /*jshint regexdash:true */
- var pattern = /([ugoa]*)([=\+-])([rwxXst]*)/i;
- var matches = pattern.exec(symbolicMode);
-
- if (matches) {
- var applyTo = matches[1];
- var operator = matches[2];
- var change = matches[3];
-
- var changeOwner = applyTo.indexOf('u') != -1 || applyTo === 'a' || applyTo === '';
- var changeGroup = applyTo.indexOf('g') != -1 || applyTo === 'a' || applyTo === '';
- var changeOther = applyTo.indexOf('o') != -1 || applyTo === 'a' || applyTo === '';
-
- var changeRead = change.indexOf('r') != -1;
- var changeWrite = change.indexOf('w') != -1;
- var changeExec = change.indexOf('x') != -1;
- var changeExecDir = change.indexOf('X') != -1;
- var changeSticky = change.indexOf('t') != -1;
- var changeSetuid = change.indexOf('s') != -1;
-
- if (changeExecDir && isDir)
- changeExec = true;
-
- var mask = 0;
- if (changeOwner) {
- mask |= (changeRead ? PERMS.OWNER_READ : 0) + (changeWrite ? PERMS.OWNER_WRITE : 0) + (changeExec ? PERMS.OWNER_EXEC : 0) + (changeSetuid ? PERMS.SETUID : 0);
- }
- if (changeGroup) {
- mask |= (changeRead ? PERMS.GROUP_READ : 0) + (changeWrite ? PERMS.GROUP_WRITE : 0) + (changeExec ? PERMS.GROUP_EXEC : 0) + (changeSetuid ? PERMS.SETGID : 0);
- }
- if (changeOther) {
- mask |= (changeRead ? PERMS.OTHER_READ : 0) + (changeWrite ? PERMS.OTHER_WRITE : 0) + (changeExec ? PERMS.OTHER_EXEC : 0);
- }
-
- // Sticky bit is special - it's not tied to user, group or other.
- if (changeSticky) {
- mask |= PERMS.STICKY;
- }
-
- switch (operator) {
- case '+':
- newPerms |= mask;
- break;
-
- case '-':
- newPerms &= ~mask;
- break;
-
- case '=':
- newPerms = type + mask;
-
- // According to POSIX, when using = to explicitly set the permissions, setuid and setgid can never be cleared.
- if (fs.statSync(file).isDirectory()) {
- newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
- }
- break;
- }
-
- if (options.verbose) {
- console.log(file + ' -> ' + newPerms.toString(8));
- }
-
- if (perms != newPerms) {
- if (!options.verbose && options.changes) {
- console.log(file + ' -> ' + newPerms.toString(8));
- }
- fs.chmodSync(file, newPerms);
- perms = newPerms; // for the next round of changes!
- }
- }
- else {
- common.error('Invalid symbolic mode change: ' + symbolicMode);
- }
- });
- }
- else {
- // they gave us a full number
- newPerms = type + parseInt(mode, 8);
-
- // POSIX rules are that setuid and setgid can only be added using numeric form, but not cleared.
- if (fs.statSync(file).isDirectory()) {
- newPerms |= (PERMS.SETUID + PERMS.SETGID) & perms;
- }
-
- fs.chmodSync(file, newPerms);
- }
- });
-}
-module.exports = _chmod;
-
-var os = require('os');
-var fs = require('fs');
-var _ls = require('./ls');
-
-// Module globals
-var config = {
- silent: false,
- fatal: false,
- verbose: false,
-};
-exports.config = config;
-
-var state = {
- error: null,
- currentCmd: 'shell.js',
- previousDir: null,
- tempDir: null
-};
-exports.state = state;
-
-var platform = os.type().match(/^Win/) ? 'win' : 'unix';
-exports.platform = platform;
-
-function log() {
- if (!config.silent)
- console.error.apply(console, arguments);
-}
-exports.log = log;
-
-// Shows error message. Throws unless _continue or config.fatal are true
-function error(msg, _continue) {
- if (state.error === null)
- state.error = '';
- var log_entry = state.currentCmd + ': ' + msg;
- if (state.error === '')
- state.error = log_entry;
- else
- state.error += '\n' + log_entry;
-
- if (msg.length > 0)
- log(log_entry);
-
- if (config.fatal)
- process.exit(1);
-
- if (!_continue)
- throw '';
-}
-exports.error = error;
-
-// In the future, when Proxies are default, we can add methods like `.to()` to primitive strings.
-// For now, this is a dummy function to bookmark places we need such strings
-function ShellString(str) {
- return str;
-}
-exports.ShellString = ShellString;
-
-// Return the home directory in a platform-agnostic way, with consideration for
-// older versions of node
-function getUserHome() {
- var result;
- if (os.homedir)
- result = os.homedir(); // node 3+
- else
- result = process.env[(process.platform === 'win32') ? 'USERPROFILE' : 'HOME'];
- return result;
-}
-exports.getUserHome = getUserHome;
-
-// Returns {'alice': true, 'bob': false} when passed a dictionary, e.g.:
-// parseOptions('-a', {'a':'alice', 'b':'bob'});
-function parseOptions(str, map) {
- if (!map)
- error('parseOptions() internal error: no map given');
-
- // All options are false by default
- var options = {};
- for (var letter in map) {
- if (!map[letter].match('^!'))
- options[map[letter]] = false;
- }
-
- if (!str)
- return options; // defaults
-
- if (typeof str !== 'string')
- error('parseOptions() internal error: wrong str');
-
- // e.g. match[1] = 'Rf' for str = '-Rf'
- var match = str.match(/^\-(.+)/);
- if (!match)
- return options;
-
- // e.g. chars = ['R', 'f']
- var chars = match[1].split('');
-
- var opt;
- chars.forEach(function(c) {
- if (c in map) {
- opt = map[c];
- if (opt.match('^!'))
- options[opt.slice(1, opt.length-1)] = false;
- else
- options[opt] = true;
- } else {
- error('option not recognized: '+c);
- }
- });
-
- return options;
-}
-exports.parseOptions = parseOptions;
-
-// Expands wildcards with matching (ie. existing) file names.
-// For example:
-// expand(['file*.js']) = ['file1.js', 'file2.js', ...]
-// (if the files 'file1.js', 'file2.js', etc, exist in the current dir)
-function expand(list) {
- var expanded = [];
- list.forEach(function(listEl) {
- // Wildcard present on directory names ?
- if(listEl.search(/\*[^\/]*\//) > -1 || listEl.search(/\*\*[^\/]*\//) > -1) {
- var match = listEl.match(/^([^*]+\/|)(.*)/);
- var root = match[1];
- var rest = match[2];
- var restRegex = rest.replace(/\*\*/g, ".*").replace(/\*/g, "[^\\/]*");
- restRegex = new RegExp(restRegex);
-
- _ls('-R', root).filter(function (e) {
- return restRegex.test(e);
- }).forEach(function(file) {
- expanded.push(file);
- });
- }
- // Wildcard present on file names ?
- else if (listEl.search(/\*/) > -1) {
- _ls('', listEl).forEach(function(file) {
- expanded.push(file);
- });
- } else {
- expanded.push(listEl);
- }
- });
- return expanded;
-}
-exports.expand = expand;
-
-// Normalizes _unlinkSync() across platforms to match Unix behavior, i.e.
-// file can be unlinked even if it's read-only, see https://github.com/joyent/node/issues/3006
-function unlinkSync(file) {
- try {
- fs.unlinkSync(file);
- } catch(e) {
- // Try to override file permission
- if (e.code === 'EPERM') {
- fs.chmodSync(file, '0666');
- fs.unlinkSync(file);
- } else {
- throw e;
- }
- }
-}
-exports.unlinkSync = unlinkSync;
-
-// e.g. 'shelljs_a5f185d0443ca...'
-function randomFileName() {
- function randomHash(count) {
- if (count === 1)
- return parseInt(16*Math.random(), 10).toString(16);
- else {
- var hash = '';
- for (var i=0; i<count; i++)
- hash += randomHash(1);
- return hash;
- }
- }
-
- return 'shelljs_'+randomHash(20);
-}
-exports.randomFileName = randomFileName;
-
-// extend(target_obj, source_obj1 [, source_obj2 ...])
-// Shallow extend, e.g.:
-// extend({A:1}, {b:2}, {c:3}) returns {A:1, b:2, c:3}
-function extend(target) {
- var sources = [].slice.call(arguments, 1);
- sources.forEach(function(source) {
- for (var key in source)
- target[key] = source[key];
- });
-
- return target;
-}
-exports.extend = extend;
-
-// Common wrapper for all Unix-like commands
-function wrap(cmd, fn, options) {
- return function() {
- var retValue = null;
-
- state.currentCmd = cmd;
- state.error = null;
-
- try {
- var args = [].slice.call(arguments, 0);
-
- if (config.verbose) {
- args.unshift(cmd);
- console.log.apply(console, args);
- args.shift();
- }
-
- if (options && options.notUnix) {
- retValue = fn.apply(this, args);
- } else {
- if (args.length === 0 || typeof args[0] !== 'string' || args[0].length <= 1 || args[0][0] !== '-')
- args.unshift(''); // only add dummy option if '-option' not already present
- // Expand the '~' if appropriate
- var homeDir = getUserHome();
- args = args.map(function(arg) {
- if (typeof arg === 'string' && arg.slice(0, 2) === '~/' || arg === '~')
- return arg.replace(/^~/, homeDir);
- else
- return arg;
- });
- retValue = fn.apply(this, args);
- }
- } catch (e) {
- if (!state.error) {
- // If state.error hasn't been set it's an error thrown by Node, not us - probably a bug...
- console.log('shell.js: internal error');
- console.log(e.stack || e);
- process.exit(1);
- }
- if (config.fatal)
- throw e;
- }
-
- state.currentCmd = 'shell.js';
- return retValue;
- };
-} // wrap
-exports.wrap = wrap;
-
-var fs = require('fs');
-var path = require('path');
-var common = require('./common');
-var os = require('os');
-
-// Buffered file copy, synchronous
-// (Using readFileSync() + writeFileSync() could easily cause a memory overflow
-// with large files)
-function copyFileSync(srcFile, destFile) {
- if (!fs.existsSync(srcFile))
- common.error('copyFileSync: no such file or directory: ' + srcFile);
-
- var BUF_LENGTH = 64*1024,
- buf = new Buffer(BUF_LENGTH),
- bytesRead = BUF_LENGTH,
- pos = 0,
- fdr = null,
- fdw = null;
-
- try {
- fdr = fs.openSync(srcFile, 'r');
- } catch(e) {
- common.error('copyFileSync: could not read src file ('+srcFile+')');
- }
-
- try {
- fdw = fs.openSync(destFile, 'w');
- } catch(e) {
- common.error('copyFileSync: could not write to dest file (code='+e.code+'):'+destFile);
- }
-
- while (bytesRead === BUF_LENGTH) {
- bytesRead = fs.readSync(fdr, buf, 0, BUF_LENGTH, pos);
- fs.writeSync(fdw, buf, 0, bytesRead);
- pos += bytesRead;
- }
-
- fs.closeSync(fdr);
- fs.closeSync(fdw);
-
- fs.chmodSync(destFile, fs.statSync(srcFile).mode);
-}
-
-// Recursively copies 'sourceDir' into 'destDir'
-// Adapted from https://github.com/ryanmcgrath/wrench-js
-//
-// Copyright (c) 2010 Ryan McGrath
-// Copyright (c) 2012 Artur Adib
-//
-// Licensed under the MIT License
-// http://www.opensource.org/licenses/mit-license.php
-function cpdirSyncRecursive(sourceDir, destDir, opts) {
- if (!opts) opts = {};
-
- /* Create the directory where all our junk is moving to; read the mode of the source directory and mirror it */
- var checkDir = fs.statSync(sourceDir);
- try {
- fs.mkdirSync(destDir, checkDir.mode);
- } catch (e) {
- //if the directory already exists, that's okay
- if (e.code !== 'EEXIST') throw e;
- }
-
- var files = fs.readdirSync(sourceDir);
-
- for (var i = 0; i < files.length; i++) {
- var srcFile = sourceDir + "/" + files[i];
- var destFile = destDir + "/" + files[i];
- var srcFileStat = fs.lstatSync(srcFile);
-
- if (srcFileStat.isDirectory()) {
- /* recursion this thing right on back. */
- cpdirSyncRecursive(srcFile, destFile, opts);
- } else if (srcFileStat.isSymbolicLink()) {
- var symlinkFull = fs.readlinkSync(srcFile);
- fs.symlinkSync(symlinkFull, destFile, os.platform() === "win32" ? "junction" : null);
- } else {
- /* At this point, we've hit a file actually worth copying... so copy it on over. */
- if (fs.existsSync(destFile) && opts.no_force) {
- common.log('skipping existing file: ' + files[i]);
- } else {
- copyFileSync(srcFile, destFile);
- }
- }
-
- } // for files
-} // cpdirSyncRecursive
-
-
-//@
-//@ ### cp([options,] source [, source ...], dest)
-//@ ### cp([options,] source_array, dest)
-//@ Available options:
-//@
-//@ + `-f`: force (default behavior)
-//@ + `-n`: no-clobber
-//@ + `-r, -R`: recursive
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ cp('file1', 'dir1');
-//@ cp('-Rf', '/tmp/*', '/usr/local/*', '/home/tmp');
-//@ cp('-Rf', ['/tmp/*', '/usr/local/*'], '/home/tmp'); // same as above
-//@ ```
-//@
-//@ Copies files. The wildcard `*` is accepted.
-function _cp(options, sources, dest) {
- options = common.parseOptions(options, {
- 'f': '!no_force',
- 'n': 'no_force',
- 'R': 'recursive',
- 'r': 'recursive'
- });
-
- // Get sources, dest
- if (arguments.length < 3) {
- common.error('missing <source> and/or <dest>');
- } else if (arguments.length > 3) {
- sources = [].slice.call(arguments, 1, arguments.length - 1);
- dest = arguments[arguments.length - 1];
- } else if (typeof sources === 'string') {
- sources = [sources];
- } else if ('length' in sources) {
- sources = sources; // no-op for array
- } else {
- common.error('invalid arguments');
- }
-
- var exists = fs.existsSync(dest),
- stats = exists && fs.statSync(dest);
-
- // Dest is not existing dir, but multiple sources given
- if ((!exists || !stats.isDirectory()) && sources.length > 1)
- common.error('dest is not a directory (too many sources)');
-
- // Dest is an existing file, but no -f given
- if (exists && stats.isFile() && options.no_force)
- common.error('dest file already exists: ' + dest);
-
- if (options.recursive) {
- // Recursive allows the shortcut syntax "sourcedir/" for "sourcedir/*"
- // (see Github issue #15)
- sources.forEach(function(src, i) {
- if (src[src.length - 1] === '/') {
- sources[i] += '*';
- // If src is a directory and dest doesn't exist, 'cp -r src dest' should copy src/* into dest
- } else if (fs.statSync(src).isDirectory() && !exists) {
- sources[i] += '/*';
- }
- });
-
- // Create dest
- try {
- fs.mkdirSync(dest, parseInt('0777', 8));
- } catch (e) {
- // like Unix's cp, keep going even if we can't create dest dir
- }
- }
-
- sources = common.expand(sources);
-
- sources.forEach(function(src) {
- if (!fs.existsSync(src)) {
- common.error('no such file or directory: '+src, true);
- return; // skip file
- }
-
- // If here, src exists
- if (fs.statSync(src).isDirectory()) {
- if (!options.recursive) {
- // Non-Recursive
- common.log(src + ' is a directory (not copied)');
- } else {
- // Recursive
- // 'cp /a/source dest' should create 'source' in 'dest'
- var newDest = path.join(dest, path.basename(src)),
- checkDir = fs.statSync(src);
- try {
- fs.mkdirSync(newDest, checkDir.mode);
- } catch (e) {
- //if the directory already exists, that's okay
- if (e.code !== 'EEXIST') {
- common.error('dest file no such file or directory: ' + newDest, true);
- throw e;
- }
- }
-
- cpdirSyncRecursive(src, newDest, {no_force: options.no_force});
- }
- return; // done with dir
- }
-
- // If here, src is a file
-
- // When copying to '/path/dir':
- // thisDest = '/path/dir/file1'
- var thisDest = dest;
- if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
- thisDest = path.normalize(dest + '/' + path.basename(src));
-
- if (fs.existsSync(thisDest) && options.no_force) {
- common.error('dest file already exists: ' + thisDest, true);
- return; // skip file
- }
-
- copyFileSync(src, thisDest);
- }); // forEach(src)
-}
-module.exports = _cp;
-
-var common = require('./common');
-var _cd = require('./cd');
-var path = require('path');
-
-// Pushd/popd/dirs internals
-var _dirStack = [];
-
-function _isStackIndex(index) {
- return (/^[\-+]\d+$/).test(index);
-}
-
-function _parseStackIndex(index) {
- if (_isStackIndex(index)) {
- if (Math.abs(index) < _dirStack.length + 1) { // +1 for pwd
- return (/^-/).test(index) ? Number(index) - 1 : Number(index);
- } else {
- common.error(index + ': directory stack index out of range');
- }
- } else {
- common.error(index + ': invalid number');
- }
-}
-
-function _actualDirStack() {
- return [process.cwd()].concat(_dirStack);
-}
-
-//@
-//@ ### pushd([options,] [dir | '-N' | '+N'])
-//@
-//@ Available options:
-//@
-//@ + `-n`: Suppresses the normal change of directory when adding directories to the stack, so that only the stack is manipulated.
-//@
-//@ Arguments:
-//@
-//@ + `dir`: Makes the current working directory be the top of the stack, and then executes the equivalent of `cd dir`.
-//@ + `+N`: Brings the Nth directory (counting from the left of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
-//@ + `-N`: Brings the Nth directory (counting from the right of the list printed by dirs, starting with zero) to the top of the list by rotating the stack.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ // process.cwd() === '/usr'
-//@ pushd('/etc'); // Returns /etc /usr
-//@ pushd('+1'); // Returns /usr /etc
-//@ ```
-//@
-//@ Save the current directory on the top of the directory stack and then cd to `dir`. With no arguments, pushd exchanges the top two directories. Returns an array of paths in the stack.
-function _pushd(options, dir) {
- if (_isStackIndex(options)) {
- dir = options;
- options = '';
- }
-
- options = common.parseOptions(options, {
- 'n' : 'no-cd'
- });
-
- var dirs = _actualDirStack();
-
- if (dir === '+0') {
- return dirs; // +0 is a noop
- } else if (!dir) {
- if (dirs.length > 1) {
- dirs = dirs.splice(1, 1).concat(dirs);
- } else {
- return common.error('no other directory');
- }
- } else if (_isStackIndex(dir)) {
- var n = _parseStackIndex(dir);
- dirs = dirs.slice(n).concat(dirs.slice(0, n));
- } else {
- if (options['no-cd']) {
- dirs.splice(1, 0, dir);
- } else {
- dirs.unshift(dir);
- }
- }
-
- if (options['no-cd']) {
- dirs = dirs.slice(1);
- } else {
- dir = path.resolve(dirs.shift());
- _cd('', dir);
- }
-
- _dirStack = dirs;
- return _dirs('');
-}
-exports.pushd = _pushd;
-
-//@
-//@ ### popd([options,] ['-N' | '+N'])
-//@
-//@ Available options:
-//@
-//@ + `-n`: Suppresses the normal change of directory when removing directories from the stack, so that only the stack is manipulated.
-//@
-//@ Arguments:
-//@
-//@ + `+N`: Removes the Nth directory (counting from the left of the list printed by dirs), starting with zero.
-//@ + `-N`: Removes the Nth directory (counting from the right of the list printed by dirs), starting with zero.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ echo(process.cwd()); // '/usr'
-//@ pushd('/etc'); // '/etc /usr'
-//@ echo(process.cwd()); // '/etc'
-//@ popd(); // '/usr'
-//@ echo(process.cwd()); // '/usr'
-//@ ```
-//@
-//@ When no arguments are given, popd removes the top directory from the stack and performs a cd to the new top directory. The elements are numbered from 0 starting at the first directory listed with dirs; i.e., popd is equivalent to popd +0. Returns an array of paths in the stack.
-function _popd(options, index) {
- if (_isStackIndex(options)) {
- index = options;
- options = '';
- }
-
- options = common.parseOptions(options, {
- 'n' : 'no-cd'
- });
-
- if (!_dirStack.length) {
- return common.error('directory stack empty');
- }
-
- index = _parseStackIndex(index || '+0');
-
- if (options['no-cd'] || index > 0 || _dirStack.length + index === 0) {
- index = index > 0 ? index - 1 : index;
- _dirStack.splice(index, 1);
- } else {
- var dir = path.resolve(_dirStack.shift());
- _cd('', dir);
- }
-
- return _dirs('');
-}
-exports.popd = _popd;
-
-//@
-//@ ### dirs([options | '+N' | '-N'])
-//@
-//@ Available options:
-//@
-//@ + `-c`: Clears the directory stack by deleting all of the elements.
-//@
-//@ Arguments:
-//@
-//@ + `+N`: Displays the Nth directory (counting from the left of the list printed by dirs when invoked without options), starting with zero.
-//@ + `-N`: Displays the Nth directory (counting from the right of the list printed by dirs when invoked without options), starting with zero.
-//@
-//@ Display the list of currently remembered directories. Returns an array of paths in the stack, or a single path if +N or -N was specified.
-//@
-//@ See also: pushd, popd
-function _dirs(options, index) {
- if (_isStackIndex(options)) {
- index = options;
- options = '';
- }
-
- options = common.parseOptions(options, {
- 'c' : 'clear'
- });
-
- if (options['clear']) {
- _dirStack = [];
- return _dirStack;
- }
-
- var stack = _actualDirStack();
-
- if (index) {
- index = _parseStackIndex(index);
-
- if (index < 0) {
- index = stack.length + index;
- }
-
- common.log(stack[index]);
- return stack[index];
- }
-
- common.log(stack.join(' '));
-
- return stack;
-}
-exports.dirs = _dirs;
-
-var common = require('./common');
-
-//@
-//@ ### echo(string [, string ...])
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ echo('hello world');
-//@ var str = echo('hello world');
-//@ ```
-//@
-//@ Prints string to stdout, and returns string with additional utility methods
-//@ like `.to()`.
-function _echo() {
- var messages = [].slice.call(arguments, 0);
- console.log.apply(console, messages);
- return common.ShellString(messages.join(' '));
-}
-module.exports = _echo;
-
-var common = require('./common');
-
-//@
-//@ ### error()
-//@ Tests if error occurred in the last command. Returns `null` if no error occurred,
-//@ otherwise returns string explaining the error
-function error() {
- return common.state.error;
-}
-module.exports = error;
-
-var common = require('./common');
-var _tempDir = require('./tempdir');
-var _pwd = require('./pwd');
-var path = require('path');
-var fs = require('fs');
-var child = require('child_process');
-
-// Hack to run child_process.exec() synchronously (sync avoids callback hell)
-// Uses a custom wait loop that checks for a flag file, created when the child process is done.
-// (Can't do a wait loop that checks for internal Node variables/messages as
-// Node is single-threaded; callbacks and other internal state changes are done in the
-// event loop).
-function execSync(cmd, opts) {
- var tempDir = _tempDir();
- var stdoutFile = path.resolve(tempDir+'/'+common.randomFileName()),
- stderrFile = path.resolve(tempDir+'/'+common.randomFileName()),
- codeFile = path.resolve(tempDir+'/'+common.randomFileName()),
- scriptFile = path.resolve(tempDir+'/'+common.randomFileName()),
- sleepFile = path.resolve(tempDir+'/'+common.randomFileName());
-
- var options = common.extend({
- silent: common.config.silent
- }, opts);
-
- var previousStdoutContent = '',
- previousStderrContent = '';
- // Echoes stdout and stderr changes from running process, if not silent
- function updateStream(streamFile) {
- if (options.silent || !fs.existsSync(streamFile))
- return;
-
- var previousStreamContent,
- proc_stream;
- if (streamFile === stdoutFile) {
- previousStreamContent = previousStdoutContent;
- proc_stream = process.stdout;
- } else { // assume stderr
- previousStreamContent = previousStderrContent;
- proc_stream = process.stderr;
- }
-
- var streamContent = fs.readFileSync(streamFile, 'utf8');
- // No changes since last time?
- if (streamContent.length <= previousStreamContent.length)
- return;
-
- proc_stream.write(streamContent.substr(previousStreamContent.length));
- previousStreamContent = streamContent;
- }
-
- function escape(str) {
- return (str+'').replace(/([\\"'])/g, "\\$1").replace(/\0/g, "\\0");
- }
-
- if (fs.existsSync(scriptFile)) common.unlinkSync(scriptFile);
- if (fs.existsSync(stdoutFile)) common.unlinkSync(stdoutFile);
- if (fs.existsSync(stderrFile)) common.unlinkSync(stderrFile);
- if (fs.existsSync(codeFile)) common.unlinkSync(codeFile);
-
- var execCommand = '"'+process.execPath+'" '+scriptFile;
- var execOptions = {
- env: process.env,
- cwd: _pwd(),
- maxBuffer: 20*1024*1024
- };
-
- var script;
-
- if (typeof child.execSync === 'function') {
- script = [
- "var child = require('child_process')",
- " , fs = require('fs');",
- "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
- " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
- "});",
- "var stdoutStream = fs.createWriteStream('"+escape(stdoutFile)+"');",
- "var stderrStream = fs.createWriteStream('"+escape(stderrFile)+"');",
- "childProcess.stdout.pipe(stdoutStream, {end: false});",
- "childProcess.stderr.pipe(stderrStream, {end: false});",
- "childProcess.stdout.pipe(process.stdout);",
- "childProcess.stderr.pipe(process.stderr);",
- "var stdoutEnded = false, stderrEnded = false;",
- "function tryClosingStdout(){ if(stdoutEnded){ stdoutStream.end(); } }",
- "function tryClosingStderr(){ if(stderrEnded){ stderrStream.end(); } }",
- "childProcess.stdout.on('end', function(){ stdoutEnded = true; tryClosingStdout(); });",
- "childProcess.stderr.on('end', function(){ stderrEnded = true; tryClosingStderr(); });"
- ].join('\n');
-
- fs.writeFileSync(scriptFile, script);
-
- if (options.silent) {
- execOptions.stdio = 'ignore';
- } else {
- execOptions.stdio = [0, 1, 2];
- }
-
- // Welcome to the future
- child.execSync(execCommand, execOptions);
- } else {
- cmd += ' > '+stdoutFile+' 2> '+stderrFile; // works on both win/unix
-
- script = [
- "var child = require('child_process')",
- " , fs = require('fs');",
- "var childProcess = child.exec('"+escape(cmd)+"', {env: process.env, maxBuffer: 20*1024*1024}, function(err) {",
- " fs.writeFileSync('"+escape(codeFile)+"', err ? err.code.toString() : '0');",
- "});"
- ].join('\n');
-
- fs.writeFileSync(scriptFile, script);
-
- child.exec(execCommand, execOptions);
-
- // The wait loop
- // sleepFile is used as a dummy I/O op to mitigate unnecessary CPU usage
- // (tried many I/O sync ops, writeFileSync() seems to be only one that is effective in reducing
- // CPU usage, though apparently not so much on Windows)
- while (!fs.existsSync(codeFile)) { updateStream(stdoutFile); fs.writeFileSync(sleepFile, 'a'); }
- while (!fs.existsSync(stdoutFile)) { updateStream(stdoutFile); fs.writeFileSync(sleepFile, 'a'); }
- while (!fs.existsSync(stderrFile)) { updateStream(stderrFile); fs.writeFileSync(sleepFile, 'a'); }
- }
-
- // At this point codeFile exists, but it's not necessarily flushed yet.
- // Keep reading it until it is.
- var code = parseInt('', 10);
- while (isNaN(code)) {
- code = parseInt(fs.readFileSync(codeFile, 'utf8'), 10);
- }
-
- var stdout = fs.readFileSync(stdoutFile, 'utf8');
- var stderr = fs.readFileSync(stderrFile, 'utf8');
-
- // No biggie if we can't erase the files now -- they're in a temp dir anyway
- try { common.unlinkSync(scriptFile); } catch(e) {}
- try { common.unlinkSync(stdoutFile); } catch(e) {}
- try { common.unlinkSync(stderrFile); } catch(e) {}
- try { common.unlinkSync(codeFile); } catch(e) {}
- try { common.unlinkSync(sleepFile); } catch(e) {}
-
- // some shell return codes are defined as errors, per http://tldp.org/LDP/abs/html/exitcodes.html
- if (code === 1 || code === 2 || code >= 126) {
- common.error('', true); // unix/shell doesn't really give an error message after non-zero exit codes
- }
- // True if successful, false if not
- var obj = {
- code: code,
- output: stdout, // deprecated
- stdout: stdout,
- stderr: stderr
- };
- return obj;
-} // execSync()
-
-// Wrapper around exec() to enable echoing output to console in real time
-function execAsync(cmd, opts, callback) {
- var stdout = '';
- var stderr = '';
-
- var options = common.extend({
- silent: common.config.silent
- }, opts);
-
- var c = child.exec(cmd, {env: process.env, maxBuffer: 20*1024*1024}, function(err) {
- if (callback)
- callback(err ? err.code : 0, stdout, stderr);
- });
-
- c.stdout.on('data', function(data) {
- stdout += data;
- if (!options.silent)
- process.stdout.write(data);
- });
-
- c.stderr.on('data', function(data) {
- stderr += data;
- if (!options.silent)
- process.stderr.write(data);
- });
-
- return c;
-}
-
-//@
-//@ ### exec(command [, options] [, callback])
-//@ Available options (all `false` by default):
-//@
-//@ + `async`: Asynchronous execution. If a callback is provided, it will be set to
-//@ `true`, regardless of the passed value.
-//@ + `silent`: Do not echo program output to console.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var version = exec('node --version', {silent:true}).stdout;
-//@
-//@ var child = exec('some_long_running_process', {async:true});
-//@ child.stdout.on('data', function(data) {
-//@ /* ... do something with data ... */
-//@ });
-//@
-//@ exec('some_long_running_process', function(code, stdout, stderr) {
-//@ console.log('Exit code:', code);
-//@ console.log('Program output:', stdout);
-//@ console.log('Program stderr:', stderr);
-//@ });
-//@ ```
-//@
-//@ Executes the given `command` _synchronously_, unless otherwise specified. When in synchronous
-//@ mode returns the object `{ code:..., stdout:... , stderr:... }`, containing the program's
-//@ `stdout`, `stderr`, and its exit `code`. Otherwise returns the child process object,
-//@ and the `callback` gets the arguments `(code, stdout, stderr)`.
-//@
-//@ **Note:** For long-lived processes, it's best to run `exec()` asynchronously as
-//@ the current synchronous implementation uses a lot of CPU. This should be getting
-//@ fixed soon.
-function _exec(command, options, callback) {
- if (!command)
- common.error('must specify command');
-
- // Callback is defined instead of options.
- if (typeof options === 'function') {
- callback = options;
- options = { async: true };
- }
-
- // Callback is defined with options.
- if (typeof options === 'object' && typeof callback === 'function') {
- options.async = true;
- }
-
- options = common.extend({
- silent: common.config.silent,
- async: false
- }, options);
-
- if (options.async)
- return execAsync(command, options, callback);
- else
- return execSync(command, options);
-}
-module.exports = _exec;
-
-var fs = require('fs');
-var common = require('./common');
-var _ls = require('./ls');
-
-//@
-//@ ### find(path [, path ...])
-//@ ### find(path_array)
-//@ Examples:
-//@
-//@ ```javascript
-//@ find('src', 'lib');
-//@ find(['src', 'lib']); // same as above
-//@ find('.').filter(function(file) { return file.match(/\.js$/); });
-//@ ```
-//@
-//@ Returns array of all files (however deep) in the given paths.
-//@
-//@ The main difference from `ls('-R', path)` is that the resulting file names
-//@ include the base directories, e.g. `lib/resources/file1` instead of just `file1`.
-function _find(options, paths) {
- if (!paths)
- common.error('no path specified');
- else if (typeof paths === 'object')
- paths = paths; // assume array
- else if (typeof paths === 'string')
- paths = [].slice.call(arguments, 1);
-
- var list = [];
-
- function pushFile(file) {
- if (common.platform === 'win')
- file = file.replace(/\\/g, '/');
- list.push(file);
- }
-
- // why not simply do ls('-R', paths)? because the output wouldn't give the base dirs
- // to get the base dir in the output, we need instead ls('-R', 'dir/*') for every directory
-
- paths.forEach(function(file) {
- pushFile(file);
-
- if (fs.statSync(file).isDirectory()) {
- _ls('-RA', file+'/*').forEach(function(subfile) {
- pushFile(subfile);
- });
- }
- });
-
- return list;
-}
-module.exports = _find;
-
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### grep([options,] regex_filter, file [, file ...])
-//@ ### grep([options,] regex_filter, file_array)
-//@ Available options:
-//@
-//@ + `-v`: Inverse the sense of the regex and print the lines not matching the criteria.
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ grep('-v', 'GLOBAL_VARIABLE', '*.js');
-//@ grep('GLOBAL_VARIABLE', '*.js');
-//@ ```
-//@
-//@ Reads input string from given files and returns a string containing all lines of the
-//@ file that match the given `regex_filter`. Wildcard `*` accepted.
-function _grep(options, regex, files) {
- options = common.parseOptions(options, {
- 'v': 'inverse'
- });
-
- if (!files)
- common.error('no paths given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 2);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- var grep = '';
- files.forEach(function(file) {
- if (!fs.existsSync(file)) {
- common.error('no such file or directory: ' + file, true);
- return;
- }
-
- var contents = fs.readFileSync(file, 'utf8'),
- lines = contents.split(/\r*\n/);
- lines.forEach(function(line) {
- var matched = line.match(regex);
- if ((options.inverse && !matched) || (!options.inverse && matched))
- grep += line + '\n';
- });
- });
-
- return common.ShellString(grep);
-}
-module.exports = _grep;
-
-var fs = require('fs');
-var path = require('path');
-var common = require('./common');
-
-//@
-//@ ### ln([options,] source, dest)
-//@ Available options:
-//@
-//@ + `-s`: symlink
-//@ + `-f`: force
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ ln('file', 'newlink');
-//@ ln('-sf', 'file', 'existing');
-//@ ```
-//@
-//@ Links source to dest. Use -f to force the link, should dest already exist.
-function _ln(options, source, dest) {
- options = common.parseOptions(options, {
- 's': 'symlink',
- 'f': 'force'
- });
-
- if (!source || !dest) {
- common.error('Missing <source> and/or <dest>');
- }
-
- source = String(source);
- var sourcePath = path.normalize(source).replace(RegExp(path.sep + '$'), '');
- var isAbsolute = (path.resolve(source) === sourcePath);
- dest = path.resolve(process.cwd(), String(dest));
-
- if (fs.existsSync(dest)) {
- if (!options.force) {
- common.error('Destination file exists', true);
- }
-
- fs.unlinkSync(dest);
- }
-
- if (options.symlink) {
- var isWindows = common.platform === 'win';
- var linkType = isWindows ? 'file' : null;
- var resolvedSourcePath = isAbsolute ? sourcePath : path.resolve(process.cwd(), path.dirname(dest), source);
- if (!fs.existsSync(resolvedSourcePath)) {
- common.error('Source file does not exist', true);
- } else if (isWindows && fs.statSync(resolvedSourcePath).isDirectory()) {
- linkType = 'junction';
- }
-
- try {
- fs.symlinkSync(linkType === 'junction' ? resolvedSourcePath: source, dest, linkType);
- } catch (err) {
- common.error(err.message);
- }
- } else {
- if (!fs.existsSync(source)) {
- common.error('Source file does not exist', true);
- }
- try {
- fs.linkSync(source, dest);
- } catch (err) {
- common.error(err.message);
- }
- }
-}
-module.exports = _ln;
-
-var path = require('path');
-var fs = require('fs');
-var common = require('./common');
-var _cd = require('./cd');
-var _pwd = require('./pwd');
-
-//@
-//@ ### ls([options,] [path, ...])
-//@ ### ls([options,] path_array)
-//@ Available options:
-//@
-//@ + `-R`: recursive
-//@ + `-A`: all files (include files beginning with `.`, except for `.` and `..`)
-//@ + `-d`: list directories themselves, not their contents
-//@ + `-l`: list objects representing each file, each with fields containing `ls
-//@ -l` output fields. See
-//@ [fs.Stats](https://nodejs.org/api/fs.html#fs_class_fs_stats)
-//@ for more info
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ ls('projs/*.js');
-//@ ls('-R', '/users/me', '/tmp');
-//@ ls('-R', ['/users/me', '/tmp']); // same as above
-//@ ls('-l', 'file.txt'); // { name: 'file.txt', mode: 33188, nlink: 1, ...}
-//@ ```
-//@
-//@ Returns array of files in the given path, or in current directory if no path provided.
-function _ls(options, paths) {
- options = common.parseOptions(options, {
- 'R': 'recursive',
- 'A': 'all',
- 'a': 'all_deprecated',
- 'd': 'directory',
- 'l': 'long'
- });
-
- if (options.all_deprecated) {
- // We won't support the -a option as it's hard to image why it's useful
- // (it includes '.' and '..' in addition to '.*' files)
- // For backwards compatibility we'll dump a deprecated message and proceed as before
- common.log('ls: Option -a is deprecated. Use -A instead');
- options.all = true;
- }
-
- if (!paths)
- paths = ['.'];
- else if (typeof paths === 'object')
- paths = paths; // assume array
- else if (typeof paths === 'string')
- paths = [].slice.call(arguments, 1);
-
- var list = [];
-
- // Conditionally pushes file to list - returns true if pushed, false otherwise
- // (e.g. prevents hidden files to be included unless explicitly told so)
- function pushFile(file, query) {
- var name = file.name || file;
- // hidden file?
- if (path.basename(name)[0] === '.') {
- // not explicitly asking for hidden files?
- if (!options.all && !(path.basename(query)[0] === '.' && path.basename(query).length > 1))
- return false;
- }
-
- if (common.platform === 'win')
- name = name.replace(/\\/g, '/');
-
- if (file.name) {
- file.name = name;
- } else {
- file = name;
- }
- list.push(file);
- return true;
- }
-
- paths.forEach(function(p) {
- if (fs.existsSync(p)) {
- var stats = ls_stat(p);
- // Simple file?
- if (stats.isFile()) {
- if (options.long) {
- pushFile(stats, p);
- } else {
- pushFile(p, p);
- }
- return; // continue
- }
-
- // Simple dir?
- if (options.directory) {
- pushFile(p, p);
- return;
- } else if (stats.isDirectory()) {
- // Iterate over p contents
- fs.readdirSync(p).forEach(function(file) {
- var orig_file = file;
- if (options.long)
- file = ls_stat(path.join(p, file));
- if (!pushFile(file, p))
- return;
-
- // Recursive?
- if (options.recursive) {
- var oldDir = _pwd();
- _cd('', p);
- if (fs.statSync(orig_file).isDirectory())
- list = list.concat(_ls('-R'+(options.all?'A':''), orig_file+'/*'));
- _cd('', oldDir);
- }
- });
- return; // continue
- }
- }
-
- // p does not exist - possible wildcard present
-
- var basename = path.basename(p);
- var dirname = path.dirname(p);
- // Wildcard present on an existing dir? (e.g. '/tmp/*.js')
- if (basename.search(/\*/) > -1 && fs.existsSync(dirname) && fs.statSync(dirname).isDirectory) {
- // Escape special regular expression chars
- var regexp = basename.replace(/(\^|\$|\(|\)|<|>|\[|\]|\{|\}|\.|\+|\?)/g, '\\$1');
- // Translates wildcard into regex
- regexp = '^' + regexp.replace(/\*/g, '.*') + '$';
- // Iterate over directory contents
- fs.readdirSync(dirname).forEach(function(file) {
- if (file.match(new RegExp(regexp))) {
- var file_path = path.join(dirname, file);
- file_path = options.long ? ls_stat(file_path) : file_path;
- if (file_path.name)
- file_path.name = path.normalize(file_path.name);
- else
- file_path = path.normalize(file_path);
- if (!pushFile(file_path, basename))
- return;
-
- // Recursive?
- if (options.recursive) {
- var pp = dirname + '/' + file;
- if (fs.lstatSync(pp).isDirectory())
- list = list.concat(_ls('-R'+(options.all?'A':''), pp+'/*'));
- } // recursive
- } // if file matches
- }); // forEach
- return;
- }
-
- common.error('no such file or directory: ' + p, true);
- });
-
- return list;
-}
-module.exports = _ls;
-
-
-function ls_stat(path) {
- var stats = fs.statSync(path);
- // Note: this object will contain more information than .toString() returns
- stats.name = path;
- stats.toString = function() {
- // Return a string resembling unix's `ls -l` format
- return [this.mode, this.nlink, this.uid, this.gid, this.size, this.mtime, this.name].join(' ');
- };
- return stats;
-}
-
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-// Recursively creates 'dir'
-function mkdirSyncRecursive(dir) {
- var baseDir = path.dirname(dir);
-
- // Base dir exists, no recursion necessary
- if (fs.existsSync(baseDir)) {
- fs.mkdirSync(dir, parseInt('0777', 8));
- return;
- }
-
- // Base dir does not exist, go recursive
- mkdirSyncRecursive(baseDir);
-
- // Base dir created, can create dir
- fs.mkdirSync(dir, parseInt('0777', 8));
-}
-
-//@
-//@ ### mkdir([options,] dir [, dir ...])
-//@ ### mkdir([options,] dir_array)
-//@ Available options:
-//@
-//@ + `-p`: full path (will create intermediate dirs if necessary)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ mkdir('-p', '/tmp/a/b/c/d', '/tmp/e/f/g');
-//@ mkdir('-p', ['/tmp/a/b/c/d', '/tmp/e/f/g']); // same as above
-//@ ```
-//@
-//@ Creates directories.
-function _mkdir(options, dirs) {
- options = common.parseOptions(options, {
- 'p': 'fullpath'
- });
- if (!dirs)
- common.error('no paths given');
-
- if (typeof dirs === 'string')
- dirs = [].slice.call(arguments, 1);
- // if it's array leave it as it is
-
- dirs.forEach(function(dir) {
- if (fs.existsSync(dir)) {
- if (!options.fullpath)
- common.error('path already exists: ' + dir, true);
- return; // skip dir
- }
-
- // Base dir does not exist, and no -p option given
- var baseDir = path.dirname(dir);
- if (!fs.existsSync(baseDir) && !options.fullpath) {
- common.error('no such file or directory: ' + baseDir, true);
- return; // skip dir
- }
-
- if (options.fullpath)
- mkdirSyncRecursive(dir);
- else
- fs.mkdirSync(dir, parseInt('0777', 8));
- });
-} // mkdir
-module.exports = _mkdir;
-
-var fs = require('fs');
-var path = require('path');
-var common = require('./common');
-
-//@
-//@ ### mv([options ,] source [, source ...], dest')
-//@ ### mv([options ,] source_array, dest')
-//@ Available options:
-//@
-//@ + `-f`: force (default behavior)
-//@ + `-n`: no-clobber
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ mv('-n', 'file', 'dir/');
-//@ mv('file1', 'file2', 'dir/');
-//@ mv(['file1', 'file2'], 'dir/'); // same as above
-//@ ```
-//@
-//@ Moves files. The wildcard `*` is accepted.
-function _mv(options, sources, dest) {
- options = common.parseOptions(options, {
- 'f': '!no_force',
- 'n': 'no_force'
- });
-
- // Get sources, dest
- if (arguments.length < 3) {
- common.error('missing <source> and/or <dest>');
- } else if (arguments.length > 3) {
- sources = [].slice.call(arguments, 1, arguments.length - 1);
- dest = arguments[arguments.length - 1];
- } else if (typeof sources === 'string') {
- sources = [sources];
- } else if ('length' in sources) {
- sources = sources; // no-op for array
- } else {
- common.error('invalid arguments');
- }
-
- sources = common.expand(sources);
-
- var exists = fs.existsSync(dest),
- stats = exists && fs.statSync(dest);
-
- // Dest is not existing dir, but multiple sources given
- if ((!exists || !stats.isDirectory()) && sources.length > 1)
- common.error('dest is not a directory (too many sources)');
-
- // Dest is an existing file, but no -f given
- if (exists && stats.isFile() && options.no_force)
- common.error('dest file already exists: ' + dest);
-
- sources.forEach(function(src) {
- if (!fs.existsSync(src)) {
- common.error('no such file or directory: '+src, true);
- return; // skip file
- }
-
- // If here, src exists
-
- // When copying to '/path/dir':
- // thisDest = '/path/dir/file1'
- var thisDest = dest;
- if (fs.existsSync(dest) && fs.statSync(dest).isDirectory())
- thisDest = path.normalize(dest + '/' + path.basename(src));
-
- if (fs.existsSync(thisDest) && options.no_force) {
- common.error('dest file already exists: ' + thisDest, true);
- return; // skip file
- }
-
- if (path.resolve(src) === path.dirname(path.resolve(thisDest))) {
- common.error('cannot move to self: '+src, true);
- return; // skip file
- }
-
- fs.renameSync(src, thisDest);
- }); // forEach(src)
-} // mv
-module.exports = _mv;
-
-// see dirs.js
-// see dirs.js
-var path = require('path');
-var common = require('./common');
-
-//@
-//@ ### pwd()
-//@ Returns the current directory.
-function _pwd() {
- var pwd = path.resolve(process.cwd());
- return common.ShellString(pwd);
-}
-module.exports = _pwd;
-
-var common = require('./common');
-var fs = require('fs');
-
-// Recursively removes 'dir'
-// Adapted from https://github.com/ryanmcgrath/wrench-js
-//
-// Copyright (c) 2010 Ryan McGrath
-// Copyright (c) 2012 Artur Adib
-//
-// Licensed under the MIT License
-// http://www.opensource.org/licenses/mit-license.php
-function rmdirSyncRecursive(dir, force) {
- var files;
-
- files = fs.readdirSync(dir);
-
- // Loop through and delete everything in the sub-tree after checking it
- for(var i = 0; i < files.length; i++) {
- var file = dir + "/" + files[i],
- currFile = fs.lstatSync(file);
-
- if(currFile.isDirectory()) { // Recursive function back to the beginning
- rmdirSyncRecursive(file, force);
- }
-
- else if(currFile.isSymbolicLink()) { // Unlink symlinks
- if (force || isWriteable(file)) {
- try {
- common.unlinkSync(file);
- } catch (e) {
- common.error('could not remove file (code '+e.code+'): ' + file, true);
- }
- }
- }
-
- else // Assume it's a file - perhaps a try/catch belongs here?
- if (force || isWriteable(file)) {
- try {
- common.unlinkSync(file);
- } catch (e) {
- common.error('could not remove file (code '+e.code+'): ' + file, true);
- }
- }
- }
-
- // Now that we know everything in the sub-tree has been deleted, we can delete the main directory.
- // Huzzah for the shopkeep.
-
- var result;
- try {
- // Retry on windows, sometimes it takes a little time before all the files in the directory are gone
- var start = Date.now();
- while (true) {
- try {
- result = fs.rmdirSync(dir);
- if (fs.existsSync(dir)) throw { code: "EAGAIN" };
- break;
- } catch(er) {
- // In addition to error codes, also check if the directory still exists and loop again if true
- if (process.platform === "win32" && (er.code === "ENOTEMPTY" || er.code === "EBUSY" || er.code === "EPERM" || er.code === "EAGAIN")) {
- if (Date.now() - start > 1000) throw er;
- } else if (er.code === "ENOENT") {
- // Directory did not exist, deletion was successful
- break;
- } else {
- throw er;
- }
- }
- }
- } catch(e) {
- common.error('could not remove directory (code '+e.code+'): ' + dir, true);
- }
-
- return result;
-} // rmdirSyncRecursive
-
-// Hack to determine if file has write permissions for current user
-// Avoids having to check user, group, etc, but it's probably slow
-function isWriteable(file) {
- var writePermission = true;
- try {
- var __fd = fs.openSync(file, 'a');
- fs.closeSync(__fd);
- } catch(e) {
- writePermission = false;
- }
-
- return writePermission;
-}
-
-//@
-//@ ### rm([options,] file [, file ...])
-//@ ### rm([options,] file_array)
-//@ Available options:
-//@
-//@ + `-f`: force
-//@ + `-r, -R`: recursive
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ rm('-rf', '/tmp/*');
-//@ rm('some_file.txt', 'another_file.txt');
-//@ rm(['some_file.txt', 'another_file.txt']); // same as above
-//@ ```
-//@
-//@ Removes files. The wildcard `*` is accepted.
-function _rm(options, files) {
- options = common.parseOptions(options, {
- 'f': 'force',
- 'r': 'recursive',
- 'R': 'recursive'
- });
- if (!files)
- common.error('no paths given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 1);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- files.forEach(function(file) {
- if (!fs.existsSync(file)) {
- // Path does not exist, no force flag given
- if (!options.force)
- common.error('no such file or directory: '+file, true);
-
- return; // skip file
- }
-
- // If here, path exists
-
- var stats = fs.lstatSync(file);
- if (stats.isFile() || stats.isSymbolicLink()) {
-
- // Do not check for file writing permissions
- if (options.force) {
- common.unlinkSync(file);
- return;
- }
-
- if (isWriteable(file))
- common.unlinkSync(file);
- else
- common.error('permission denied: '+file, true);
-
- return;
- } // simple file
-
- // Path is an existing directory, but no -r flag given
- if (stats.isDirectory() && !options.recursive) {
- common.error('path is a directory', true);
- return; // skip path
- }
-
- // Recursively remove existing directory
- if (stats.isDirectory() && options.recursive) {
- rmdirSyncRecursive(file, options.force);
- }
- }); // forEach(file)
-} // rm
-module.exports = _rm;
-
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### sed([options,] search_regex, replacement, file [, file ...])
-//@ ### sed([options,] search_regex, replacement, file_array)
-//@ Available options:
-//@
-//@ + `-i`: Replace contents of 'file' in-place. _Note that no backups will be created!_
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ sed('-i', 'PROGRAM_VERSION', 'v0.1.3', 'source.js');
-//@ sed(/.*DELETE_THIS_LINE.*\n/, '', 'source.js');
-//@ ```
-//@
-//@ Reads an input string from `files` and performs a JavaScript `replace()` on the input
-//@ using the given search regex and replacement string or function. Returns the new string after replacement.
-function _sed(options, regex, replacement, files) {
- options = common.parseOptions(options, {
- 'i': 'inplace'
- });
-
- if (typeof replacement === 'string' || typeof replacement === 'function')
- replacement = replacement; // no-op
- else if (typeof replacement === 'number')
- replacement = replacement.toString(); // fallback
- else
- common.error('invalid replacement string');
-
- // Convert all search strings to RegExp
- if (typeof regex === 'string')
- regex = RegExp(regex);
-
- if (!files)
- common.error('no files given');
-
- if (typeof files === 'string')
- files = [].slice.call(arguments, 3);
- // if it's array leave it as it is
-
- files = common.expand(files);
-
- var sed = [];
- files.forEach(function(file) {
- if (!fs.existsSync(file)) {
- common.error('no such file or directory: ' + file, true);
- return;
- }
-
- var result = fs.readFileSync(file, 'utf8').split('\n').map(function (line) {
- return line.replace(regex, replacement);
- }).join('\n');
-
- sed.push(result);
-
- if (options.inplace)
- fs.writeFileSync(file, result, 'utf8');
- });
-
- return common.ShellString(sed.join('\n'));
-}
-module.exports = _sed;
-
-var common = require('./common');
-
-//@
-//@ ### set(options)
-//@ Available options:
-//@
-//@ + `+/-e`: exit upon error (`config.fatal`)
-//@ + `+/-v`: verbose: show all commands (`config.verbose`)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ set('-e'); // exit upon first error
-//@ set('+e'); // this undoes a "set('-e')"
-//@ ```
-//@
-//@ Sets global configuration variables
-function _set(options) {
- if (!options) {
- var args = [].slice.call(arguments, 0);
- if (args.length < 2)
- common.error('must provide an argument');
- options = args[1];
- }
- var negate = (options[0] === '+');
- if (negate) {
- options = '-' + options.slice(1); // parseOptions needs a '-' prefix
- }
- options = common.parseOptions(options, {
- 'e': 'fatal',
- 'v': 'verbose'
- });
-
- var key;
- if (negate) {
- for (key in options)
- options[key] = !options[key];
- }
-
- for (key in options) {
- // Only change the global config if `negate` is false and the option is true
- // or if `negate` is true and the option is false (aka negate !== option)
- if (negate !== options[key]) {
- common.config[key] = options[key];
- }
- }
- return;
-}
-module.exports = _set;
-
-var common = require('./common');
-var os = require('os');
-var fs = require('fs');
-
-// Returns false if 'dir' is not a writeable directory, 'dir' otherwise
-function writeableDir(dir) {
- if (!dir || !fs.existsSync(dir))
- return false;
-
- if (!fs.statSync(dir).isDirectory())
- return false;
-
- var testFile = dir+'/'+common.randomFileName();
- try {
- fs.writeFileSync(testFile, ' ');
- common.unlinkSync(testFile);
- return dir;
- } catch (e) {
- return false;
- }
-}
-
-
-//@
-//@ ### tempdir()
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var tmp = tempdir(); // "/tmp" for most *nix platforms
-//@ ```
-//@
-//@ Searches and returns string containing a writeable, platform-dependent temporary directory.
-//@ Follows Python's [tempfile algorithm](http://docs.python.org/library/tempfile.html#tempfile.tempdir).
-function _tempDir() {
- var state = common.state;
- if (state.tempDir)
- return state.tempDir; // from cache
-
- state.tempDir = writeableDir(os.tmpdir && os.tmpdir()) || // node 0.10+
- writeableDir(os.tmpDir && os.tmpDir()) || // node 0.8+
- writeableDir(process.env['TMPDIR']) ||
- writeableDir(process.env['TEMP']) ||
- writeableDir(process.env['TMP']) ||
- writeableDir(process.env['Wimp$ScrapDir']) || // RiscOS
- writeableDir('C:\\TEMP') || // Windows
- writeableDir('C:\\TMP') || // Windows
- writeableDir('\\TEMP') || // Windows
- writeableDir('\\TMP') || // Windows
- writeableDir('/tmp') ||
- writeableDir('/var/tmp') ||
- writeableDir('/usr/tmp') ||
- writeableDir('.'); // last resort
-
- return state.tempDir;
-}
-module.exports = _tempDir;
-
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### test(expression)
-//@ Available expression primaries:
-//@
-//@ + `'-b', 'path'`: true if path is a block device
-//@ + `'-c', 'path'`: true if path is a character device
-//@ + `'-d', 'path'`: true if path is a directory
-//@ + `'-e', 'path'`: true if path exists
-//@ + `'-f', 'path'`: true if path is a regular file
-//@ + `'-L', 'path'`: true if path is a symbolic link
-//@ + `'-p', 'path'`: true if path is a pipe (FIFO)
-//@ + `'-S', 'path'`: true if path is a socket
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ if (test('-d', path)) { /* do something with dir */ };
-//@ if (!test('-f', path)) continue; // skip if it's a regular file
-//@ ```
-//@
-//@ Evaluates expression using the available primaries and returns corresponding value.
-function _test(options, path) {
- if (!path)
- common.error('no path given');
-
- // hack - only works with unary primaries
- options = common.parseOptions(options, {
- 'b': 'block',
- 'c': 'character',
- 'd': 'directory',
- 'e': 'exists',
- 'f': 'file',
- 'L': 'link',
- 'p': 'pipe',
- 'S': 'socket'
- });
-
- var canInterpret = false;
- for (var key in options)
- if (options[key] === true) {
- canInterpret = true;
- break;
- }
-
- if (!canInterpret)
- common.error('could not interpret expression');
-
- if (options.link) {
- try {
- return fs.lstatSync(path).isSymbolicLink();
- } catch(e) {
- return false;
- }
- }
-
- if (!fs.existsSync(path))
- return false;
-
- if (options.exists)
- return true;
-
- var stats = fs.statSync(path);
-
- if (options.block)
- return stats.isBlockDevice();
-
- if (options.character)
- return stats.isCharacterDevice();
-
- if (options.directory)
- return stats.isDirectory();
-
- if (options.file)
- return stats.isFile();
-
- if (options.pipe)
- return stats.isFIFO();
-
- if (options.socket)
- return stats.isSocket();
-} // test
-module.exports = _test;
-
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-//@
-//@ ### 'string'.to(file)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ cat('input.txt').to('output.txt');
-//@ ```
-//@
-//@ Analogous to the redirection operator `>` in Unix, but works with JavaScript strings (such as
-//@ those returned by `cat`, `grep`, etc). _Like Unix redirections, `to()` will overwrite any existing file!_
-function _to(options, file) {
- if (!file)
- common.error('wrong arguments');
-
- if (!fs.existsSync( path.dirname(file) ))
- common.error('no such file or directory: ' + path.dirname(file));
-
- try {
- fs.writeFileSync(file, this.toString(), 'utf8');
- return this;
- } catch(e) {
- common.error('could not write to file (code '+e.code+'): '+file, true);
- }
-}
-module.exports = _to;
-
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-//@
-//@ ### 'string'.toEnd(file)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ cat('input.txt').toEnd('output.txt');
-//@ ```
-//@
-//@ Analogous to the redirect-and-append operator `>>` in Unix, but works with JavaScript strings (such as
-//@ those returned by `cat`, `grep`, etc).
-function _toEnd(options, file) {
- if (!file)
- common.error('wrong arguments');
-
- if (!fs.existsSync( path.dirname(file) ))
- common.error('no such file or directory: ' + path.dirname(file));
-
- try {
- fs.appendFileSync(file, this.toString(), 'utf8');
- return this;
- } catch(e) {
- common.error('could not append to file (code '+e.code+'): '+file, true);
- }
-}
-module.exports = _toEnd;
-
-var common = require('./common');
-var fs = require('fs');
-
-//@
-//@ ### touch([options,] file)
-//@ Available options:
-//@
-//@ + `-a`: Change only the access time
-//@ + `-c`: Do not create any files
-//@ + `-m`: Change only the modification time
-//@ + `-d DATE`: Parse DATE and use it instead of current time
-//@ + `-r FILE`: Use FILE's times instead of current time
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ touch('source.js');
-//@ touch('-c', '/path/to/some/dir/source.js');
-//@ touch({ '-r': FILE }, '/path/to/some/dir/source.js');
-//@ ```
-//@
-//@ Update the access and modification times of each FILE to the current time.
-//@ A FILE argument that does not exist is created empty, unless -c is supplied.
-//@ This is a partial implementation of *[touch(1)](http://linux.die.net/man/1/touch)*.
-function _touch(opts, files) {
- opts = common.parseOptions(opts, {
- 'a': 'atime_only',
- 'c': 'no_create',
- 'd': 'date',
- 'm': 'mtime_only',
- 'r': 'reference',
- });
-
- if (!files) {
- common.error('no paths given');
- }
-
-
- if (Array.isArray(files)) {
- files.forEach(function(f) {
- touchFile(opts, f);
- });
- } else if (typeof files === 'string') {
- touchFile(opts, files);
- } else {
- common.error('file arg should be a string file path or an Array of string file paths');
- }
-
-}
-
-function touchFile(opts, file) {
- var stat = tryStatFile(file);
-
- if (stat && stat.isDirectory()) {
- // don't error just exit
- return;
- }
-
- // if the file doesn't already exist and the user has specified --no-create then
- // this script is finished
- if (!stat && opts.no_create) {
- return;
- }
-
- // open the file and then close it. this will create it if it doesn't exist but will
- // not truncate the file
- fs.closeSync(fs.openSync(file, 'a'));
-
- //
- // Set timestamps
- //
-
- // setup some defaults
- var now = new Date();
- var mtime = opts.date || now;
- var atime = opts.date || now;
-
- // use reference file
- if (opts.reference) {
- var refStat = tryStatFile(opts.reference);
- if (!refStat) {
- common.error('failed to get attributess of ' + opts.reference);
- }
- mtime = refStat.mtime;
- atime = refStat.atime;
- } else if (opts.date) {
- mtime = opts.date;
- atime = opts.date;
- }
-
- if (opts.atime_only && opts.mtime_only) {
- // keep the new values of mtime and atime like GNU
- } else if (opts.atime_only) {
- mtime = stat.mtime;
- } else if (opts.mtime_only) {
- atime = stat.atime;
- }
-
- fs.utimesSync(file, atime, mtime);
-}
-
-module.exports = _touch;
-
-function tryStatFile(filePath) {
- try {
- return fs.statSync(filePath);
- } catch (e) {
- return null;
- }
-}
-
-var common = require('./common');
-var fs = require('fs');
-var path = require('path');
-
-// XP's system default value for PATHEXT system variable, just in case it's not
-// set on Windows.
-var XP_DEFAULT_PATHEXT = '.com;.exe;.bat;.cmd;.vbs;.vbe;.js;.jse;.wsf;.wsh';
-
-// Cross-platform method for splitting environment PATH variables
-function splitPath(p) {
- if (!p)
- return [];
-
- if (common.platform === 'win')
- return p.split(';');
- else
- return p.split(':');
-}
-
-function checkPath(path) {
- return fs.existsSync(path) && !fs.statSync(path).isDirectory();
-}
-
-//@
-//@ ### which(command)
-//@
-//@ Examples:
-//@
-//@ ```javascript
-//@ var nodeExec = which('node');
-//@ ```
-//@
-//@ Searches for `command` in the system's PATH. On Windows, this uses the
-//@ `PATHEXT` variable to append the extension if it's not already executable.
-//@ Returns string containing the absolute path to the command.
-function _which(options, cmd) {
- if (!cmd)
- common.error('must specify command');
-
- var pathEnv = process.env.path || process.env.Path || process.env.PATH,
- pathArray = splitPath(pathEnv),
- where = null;
-
- // No relative/absolute paths provided?
- if (cmd.search(/\//) === -1) {
- // Search for command in PATH
- pathArray.forEach(function(dir) {
- if (where)
- return; // already found it
-
- var attempt = path.resolve(dir, cmd);
-
- if (common.platform === 'win') {
- attempt = attempt.toUpperCase();
-
- // In case the PATHEXT variable is somehow not set (e.g.
- // child_process.spawn with an empty environment), use the XP default.
- var pathExtEnv = process.env.PATHEXT || XP_DEFAULT_PATHEXT;
- var pathExtArray = splitPath(pathExtEnv.toUpperCase());
- var i;
-
- // If the extension is already in PATHEXT, just return that.
- for (i = 0; i < pathExtArray.length; i++) {
- var ext = pathExtArray[i];
- if (attempt.slice(-ext.length) === ext && checkPath(attempt)) {
- where = attempt;
- return;
- }
- }
-
- // Cycle through the PATHEXT variable
- var baseAttempt = attempt;
- for (i = 0; i < pathExtArray.length; i++) {
- attempt = baseAttempt + pathExtArray[i];
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
- }
- } else {
- // Assume it's Unix-like
- if (checkPath(attempt)) {
- where = attempt;
- return;
- }
- }
- });
- }
-
- // Command not found anywhere?
- if (!checkPath(cmd) && !where)
- return null;
-
- where = where || path.resolve(cmd);
-
- return common.ShellString(where);
-}
-module.exports = _which;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/make.js
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/make.js b/node_modules/shelljs/make.js
index a8438c8..f78b4cf 100644
--- a/node_modules/shelljs/make.js
+++ b/node_modules/shelljs/make.js
@@ -31,11 +31,10 @@ setTimeout(function() {
// Wrap it
global.target[t] = function() {
- if (!oldTarget.done){
- oldTarget.done = true;
- oldTarget.result = oldTarget.apply(oldTarget, arguments);
- }
- return oldTarget.result;
+ if (oldTarget.done)
+ return;
+ oldTarget.done = true;
+ return oldTarget.apply(oldTarget, arguments);
};
})(t, global.target[t]);
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/562f4668/node_modules/shelljs/package.json
----------------------------------------------------------------------
diff --git a/node_modules/shelljs/package.json b/node_modules/shelljs/package.json
index 2d0a809..6dfa17a 100644
--- a/node_modules/shelljs/package.json
+++ b/node_modules/shelljs/package.json
@@ -1,41 +1,38 @@
{
"_args": [
[
- "shelljs@^0.6.0",
+ "shelljs@^0.5.3",
"/Users/steveng/repo/cordova/cordova-osx"
]
],
- "_from": "shelljs@>=0.6.0 <0.7.0",
- "_id": "shelljs@0.6.0",
+ "_from": "shelljs@>=0.5.3 <0.6.0",
+ "_id": "shelljs@0.5.3",
"_inCache": true,
"_installable": true,
"_location": "/shelljs",
- "_nodeVersion": "5.4.0",
- "_npmOperationalInternal": {
- "host": "packages-6-west.internal.npmjs.com",
- "tmp": "tmp/shelljs-0.6.0.tgz_1454632811074_0.5800695188809186"
- },
+ "_nodeVersion": "1.2.0",
"_npmUser": {
- "email": "ari@ariporad.com",
- "name": "ariporad"
+ "email": "arturadib@gmail.com",
+ "name": "artur"
},
- "_npmVersion": "3.6.0",
+ "_npmVersion": "2.5.1",
"_phantomChildren": {},
"_requested": {
"name": "shelljs",
- "raw": "shelljs@^0.6.0",
- "rawSpec": "^0.6.0",
+ "raw": "shelljs@^0.5.3",
+ "rawSpec": "^0.5.3",
"scope": null,
- "spec": ">=0.6.0 <0.7.0",
+ "spec": ">=0.5.3 <0.6.0",
"type": "range"
},
"_requiredBy": [
- "/"
+ "/",
+ "/cordova-common"
],
- "_resolved": "http://registry.npmjs.org/shelljs/-/shelljs-0.6.0.tgz",
- "_shasum": "ce1ed837b4b0e55b5ec3dab84251ab9dbdc0c7ec",
+ "_resolved": "http://registry.npmjs.org/shelljs/-/shelljs-0.5.3.tgz",
+ "_shasum": "c54982b996c76ef0c1e6b59fbdc5825f5b713113",
"_shrinkwrap": null,
- "_spec": "shelljs@^0.6.0",
+ "_spec": "shelljs@^0.5.3",
"_where": "/Users/steveng/repo/cordova/cordova-osx",
"author": {
"email": "arturadib@gmail.com",
@@ -45,35 +42,23 @@
"shjs": "./bin/shjs"
},
"bugs": {
- "url": "https://github.com/shelljs/shelljs/issues"
+ "url": "https://github.com/arturadib/shelljs/issues"
},
- "contributors": [
- {
- "name": "Ari Porad",
- "email": "ari@ariporad.com",
- "url": "http://ariporad.com/"
- },
- {
- "name": "Nate Fischer",
- "email": "ntfschr@gmail.com"
- }
- ],
"dependencies": {},
"description": "Portable Unix shell commands for Node.js",
"devDependencies": {
- "coffee-script": "^1.10.0",
"jshint": "~2.1.11"
},
"directories": {},
"dist": {
- "shasum": "ce1ed837b4b0e55b5ec3dab84251ab9dbdc0c7ec",
- "tarball": "http://registry.npmjs.org/shelljs/-/shelljs-0.6.0.tgz"
+ "shasum": "c54982b996c76ef0c1e6b59fbdc5825f5b713113",
+ "tarball": "http://registry.npmjs.org/shelljs/-/shelljs-0.5.3.tgz"
},
"engines": {
- "node": ">=0.10.0"
+ "node": ">=0.8.0"
},
- "gitHead": "fe06baf1173ec6f0a70cd58ddb7d373f4c6446f5",
- "homepage": "http://github.com/shelljs/shelljs",
+ "gitHead": "22d0975040b9b8234755dc6e692d6869436e8485",
+ "homepage": "http://github.com/arturadib/shelljs",
"keywords": [
"jake",
"make",
@@ -82,20 +67,12 @@
"synchronous",
"unix"
],
- "license": "BSD-3-Clause",
+ "license": "BSD*",
"main": "./shell.js",
"maintainers": [
{
- "name": "ariporad",
- "email": "ari@ariporad.com"
- },
- {
"name": "artur",
"email": "arturadib@gmail.com"
- },
- {
- "name": "nfischer",
- "email": "ntfschr@gmail.com"
}
],
"name": "shelljs",
@@ -103,10 +80,10 @@
"readme": "ERROR: No README data found!",
"repository": {
"type": "git",
- "url": "git://github.com/shelljs/shelljs.git"
+ "url": "git://github.com/arturadib/shelljs.git"
},
"scripts": {
"test": "node scripts/run-tests"
},
- "version": "0.6.0"
+ "version": "0.5.3"
}
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[26/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/LazyWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/LazyWrapper.js b/node_modules/lodash/internal/LazyWrapper.js
new file mode 100644
index 0000000..d9c8080
--- /dev/null
+++ b/node_modules/lodash/internal/LazyWrapper.js
@@ -0,0 +1,26 @@
+var baseCreate = require('./baseCreate'),
+ baseLodash = require('./baseLodash');
+
+/** Used as references for `-Infinity` and `Infinity`. */
+var POSITIVE_INFINITY = Number.POSITIVE_INFINITY;
+
+/**
+ * Creates a lazy wrapper object which wraps `value` to enable lazy evaluation.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ */
+function LazyWrapper(value) {
+ this.__wrapped__ = value;
+ this.__actions__ = [];
+ this.__dir__ = 1;
+ this.__filtered__ = false;
+ this.__iteratees__ = [];
+ this.__takeCount__ = POSITIVE_INFINITY;
+ this.__views__ = [];
+}
+
+LazyWrapper.prototype = baseCreate(baseLodash.prototype);
+LazyWrapper.prototype.constructor = LazyWrapper;
+
+module.exports = LazyWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/LodashWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/LodashWrapper.js b/node_modules/lodash/internal/LodashWrapper.js
new file mode 100644
index 0000000..ab06bc7
--- /dev/null
+++ b/node_modules/lodash/internal/LodashWrapper.js
@@ -0,0 +1,21 @@
+var baseCreate = require('./baseCreate'),
+ baseLodash = require('./baseLodash');
+
+/**
+ * The base constructor for creating `lodash` wrapper objects.
+ *
+ * @private
+ * @param {*} value The value to wrap.
+ * @param {boolean} [chainAll] Enable chaining for all wrapper methods.
+ * @param {Array} [actions=[]] Actions to peform to resolve the unwrapped value.
+ */
+function LodashWrapper(value, chainAll, actions) {
+ this.__wrapped__ = value;
+ this.__actions__ = actions || [];
+ this.__chain__ = !!chainAll;
+}
+
+LodashWrapper.prototype = baseCreate(baseLodash.prototype);
+LodashWrapper.prototype.constructor = LodashWrapper;
+
+module.exports = LodashWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/MapCache.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/MapCache.js b/node_modules/lodash/internal/MapCache.js
new file mode 100644
index 0000000..1d7ab98
--- /dev/null
+++ b/node_modules/lodash/internal/MapCache.js
@@ -0,0 +1,24 @@
+var mapDelete = require('./mapDelete'),
+ mapGet = require('./mapGet'),
+ mapHas = require('./mapHas'),
+ mapSet = require('./mapSet');
+
+/**
+ * Creates a cache object to store key/value pairs.
+ *
+ * @private
+ * @static
+ * @name Cache
+ * @memberOf _.memoize
+ */
+function MapCache() {
+ this.__data__ = {};
+}
+
+// Add functions to the `Map` cache.
+MapCache.prototype['delete'] = mapDelete;
+MapCache.prototype.get = mapGet;
+MapCache.prototype.has = mapHas;
+MapCache.prototype.set = mapSet;
+
+module.exports = MapCache;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/SetCache.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/SetCache.js b/node_modules/lodash/internal/SetCache.js
new file mode 100644
index 0000000..ae29c55
--- /dev/null
+++ b/node_modules/lodash/internal/SetCache.js
@@ -0,0 +1,29 @@
+var cachePush = require('./cachePush'),
+ getNative = require('./getNative');
+
+/** Native method references. */
+var Set = getNative(global, 'Set');
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeCreate = getNative(Object, 'create');
+
+/**
+ *
+ * Creates a cache object to store unique values.
+ *
+ * @private
+ * @param {Array} [values] The values to cache.
+ */
+function SetCache(values) {
+ var length = values ? values.length : 0;
+
+ this.data = { 'hash': nativeCreate(null), 'set': new Set };
+ while (length--) {
+ this.push(values[length]);
+ }
+}
+
+// Add functions to the `Set` cache.
+SetCache.prototype.push = cachePush;
+
+module.exports = SetCache;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayConcat.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayConcat.js b/node_modules/lodash/internal/arrayConcat.js
new file mode 100644
index 0000000..0d131e3
--- /dev/null
+++ b/node_modules/lodash/internal/arrayConcat.js
@@ -0,0 +1,25 @@
+/**
+ * Creates a new array joining `array` with `other`.
+ *
+ * @private
+ * @param {Array} array The array to join.
+ * @param {Array} other The other array to join.
+ * @returns {Array} Returns the new concatenated array.
+ */
+function arrayConcat(array, other) {
+ var index = -1,
+ length = array.length,
+ othIndex = -1,
+ othLength = other.length,
+ result = Array(length + othLength);
+
+ while (++index < length) {
+ result[index] = array[index];
+ }
+ while (++othIndex < othLength) {
+ result[index++] = other[othIndex];
+ }
+ return result;
+}
+
+module.exports = arrayConcat;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayCopy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayCopy.js b/node_modules/lodash/internal/arrayCopy.js
new file mode 100644
index 0000000..fa7067f
--- /dev/null
+++ b/node_modules/lodash/internal/arrayCopy.js
@@ -0,0 +1,20 @@
+/**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+function arrayCopy(source, array) {
+ var index = -1,
+ length = source.length;
+
+ array || (array = Array(length));
+ while (++index < length) {
+ array[index] = source[index];
+ }
+ return array;
+}
+
+module.exports = arrayCopy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayEach.js b/node_modules/lodash/internal/arrayEach.js
new file mode 100644
index 0000000..0f51382
--- /dev/null
+++ b/node_modules/lodash/internal/arrayEach.js
@@ -0,0 +1,22 @@
+/**
+ * A specialized version of `_.forEach` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+function arrayEach(array, iteratee) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (iteratee(array[index], index, array) === false) {
+ break;
+ }
+ }
+ return array;
+}
+
+module.exports = arrayEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayEachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayEachRight.js b/node_modules/lodash/internal/arrayEachRight.js
new file mode 100644
index 0000000..367e066
--- /dev/null
+++ b/node_modules/lodash/internal/arrayEachRight.js
@@ -0,0 +1,21 @@
+/**
+ * A specialized version of `_.forEachRight` for arrays without support for
+ * callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+function arrayEachRight(array, iteratee) {
+ var length = array.length;
+
+ while (length--) {
+ if (iteratee(array[length], length, array) === false) {
+ break;
+ }
+ }
+ return array;
+}
+
+module.exports = arrayEachRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayEvery.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayEvery.js b/node_modules/lodash/internal/arrayEvery.js
new file mode 100644
index 0000000..3fe6ed2
--- /dev/null
+++ b/node_modules/lodash/internal/arrayEvery.js
@@ -0,0 +1,23 @@
+/**
+ * A specialized version of `_.every` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`.
+ */
+function arrayEvery(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (!predicate(array[index], index, array)) {
+ return false;
+ }
+ }
+ return true;
+}
+
+module.exports = arrayEvery;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayExtremum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayExtremum.js b/node_modules/lodash/internal/arrayExtremum.js
new file mode 100644
index 0000000..e45badb
--- /dev/null
+++ b/node_modules/lodash/internal/arrayExtremum.js
@@ -0,0 +1,30 @@
+/**
+ * A specialized version of `baseExtremum` for arrays which invokes `iteratee`
+ * with one argument: (value).
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} comparator The function used to compare values.
+ * @param {*} exValue The initial extremum value.
+ * @returns {*} Returns the extremum value.
+ */
+function arrayExtremum(array, iteratee, comparator, exValue) {
+ var index = -1,
+ length = array.length,
+ computed = exValue,
+ result = computed;
+
+ while (++index < length) {
+ var value = array[index],
+ current = +iteratee(value);
+
+ if (comparator(current, computed)) {
+ computed = current;
+ result = value;
+ }
+ }
+ return result;
+}
+
+module.exports = arrayExtremum;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayFilter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayFilter.js b/node_modules/lodash/internal/arrayFilter.js
new file mode 100644
index 0000000..e14fe06
--- /dev/null
+++ b/node_modules/lodash/internal/arrayFilter.js
@@ -0,0 +1,25 @@
+/**
+ * A specialized version of `_.filter` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+function arrayFilter(array, predicate) {
+ var index = -1,
+ length = array.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (predicate(value, index, array)) {
+ result[++resIndex] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = arrayFilter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayMap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayMap.js b/node_modules/lodash/internal/arrayMap.js
new file mode 100644
index 0000000..777c7c9
--- /dev/null
+++ b/node_modules/lodash/internal/arrayMap.js
@@ -0,0 +1,21 @@
+/**
+ * A specialized version of `_.map` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+function arrayMap(array, iteratee) {
+ var index = -1,
+ length = array.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = iteratee(array[index], index, array);
+ }
+ return result;
+}
+
+module.exports = arrayMap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayPush.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayPush.js b/node_modules/lodash/internal/arrayPush.js
new file mode 100644
index 0000000..7d742b3
--- /dev/null
+++ b/node_modules/lodash/internal/arrayPush.js
@@ -0,0 +1,20 @@
+/**
+ * Appends the elements of `values` to `array`.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to append.
+ * @returns {Array} Returns `array`.
+ */
+function arrayPush(array, values) {
+ var index = -1,
+ length = values.length,
+ offset = array.length;
+
+ while (++index < length) {
+ array[offset + index] = values[index];
+ }
+ return array;
+}
+
+module.exports = arrayPush;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayReduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayReduce.js b/node_modules/lodash/internal/arrayReduce.js
new file mode 100644
index 0000000..f948c8e
--- /dev/null
+++ b/node_modules/lodash/internal/arrayReduce.js
@@ -0,0 +1,26 @@
+/**
+ * A specialized version of `_.reduce` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initFromArray] Specify using the first element of `array`
+ * as the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+function arrayReduce(array, iteratee, accumulator, initFromArray) {
+ var index = -1,
+ length = array.length;
+
+ if (initFromArray && length) {
+ accumulator = array[++index];
+ }
+ while (++index < length) {
+ accumulator = iteratee(accumulator, array[index], index, array);
+ }
+ return accumulator;
+}
+
+module.exports = arrayReduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arrayReduceRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arrayReduceRight.js b/node_modules/lodash/internal/arrayReduceRight.js
new file mode 100644
index 0000000..d4d68df
--- /dev/null
+++ b/node_modules/lodash/internal/arrayReduceRight.js
@@ -0,0 +1,24 @@
+/**
+ * A specialized version of `_.reduceRight` for arrays without support for
+ * callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initFromArray] Specify using the last element of `array`
+ * as the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+function arrayReduceRight(array, iteratee, accumulator, initFromArray) {
+ var length = array.length;
+ if (initFromArray && length) {
+ accumulator = array[--length];
+ }
+ while (length--) {
+ accumulator = iteratee(accumulator, array[length], length, array);
+ }
+ return accumulator;
+}
+
+module.exports = arrayReduceRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arraySome.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arraySome.js b/node_modules/lodash/internal/arraySome.js
new file mode 100644
index 0000000..f7a0bb5
--- /dev/null
+++ b/node_modules/lodash/internal/arraySome.js
@@ -0,0 +1,23 @@
+/**
+ * A specialized version of `_.some` for arrays without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+function arraySome(array, predicate) {
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ if (predicate(array[index], index, array)) {
+ return true;
+ }
+ }
+ return false;
+}
+
+module.exports = arraySome;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/arraySum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/arraySum.js b/node_modules/lodash/internal/arraySum.js
new file mode 100644
index 0000000..0e40c91
--- /dev/null
+++ b/node_modules/lodash/internal/arraySum.js
@@ -0,0 +1,20 @@
+/**
+ * A specialized version of `_.sum` for arrays without support for callback
+ * shorthands and `this` binding..
+ *
+ * @private
+ * @param {Array} array The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the sum.
+ */
+function arraySum(array, iteratee) {
+ var length = array.length,
+ result = 0;
+
+ while (length--) {
+ result += +iteratee(array[length]) || 0;
+ }
+ return result;
+}
+
+module.exports = arraySum;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/assignDefaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/assignDefaults.js b/node_modules/lodash/internal/assignDefaults.js
new file mode 100644
index 0000000..affd993
--- /dev/null
+++ b/node_modules/lodash/internal/assignDefaults.js
@@ -0,0 +1,13 @@
+/**
+ * Used by `_.defaults` to customize its `_.assign` use.
+ *
+ * @private
+ * @param {*} objectValue The destination object property value.
+ * @param {*} sourceValue The source object property value.
+ * @returns {*} Returns the value to assign to the destination object.
+ */
+function assignDefaults(objectValue, sourceValue) {
+ return objectValue === undefined ? sourceValue : objectValue;
+}
+
+module.exports = assignDefaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/assignOwnDefaults.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/assignOwnDefaults.js b/node_modules/lodash/internal/assignOwnDefaults.js
new file mode 100644
index 0000000..682c460
--- /dev/null
+++ b/node_modules/lodash/internal/assignOwnDefaults.js
@@ -0,0 +1,26 @@
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used by `_.template` to customize its `_.assign` use.
+ *
+ * **Note:** This function is like `assignDefaults` except that it ignores
+ * inherited property values when checking if a property is `undefined`.
+ *
+ * @private
+ * @param {*} objectValue The destination object property value.
+ * @param {*} sourceValue The source object property value.
+ * @param {string} key The key associated with the object and source values.
+ * @param {Object} object The destination object.
+ * @returns {*} Returns the value to assign to the destination object.
+ */
+function assignOwnDefaults(objectValue, sourceValue, key, object) {
+ return (objectValue === undefined || !hasOwnProperty.call(object, key))
+ ? sourceValue
+ : objectValue;
+}
+
+module.exports = assignOwnDefaults;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/assignWith.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/assignWith.js b/node_modules/lodash/internal/assignWith.js
new file mode 100644
index 0000000..d2b261a
--- /dev/null
+++ b/node_modules/lodash/internal/assignWith.js
@@ -0,0 +1,32 @@
+var keys = require('../object/keys');
+
+/**
+ * A specialized version of `_.assign` for customizing assigned values without
+ * support for argument juggling, multiple sources, and `this` binding `customizer`
+ * functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ */
+function assignWith(object, source, customizer) {
+ var index = -1,
+ props = keys(source),
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index],
+ value = object[key],
+ result = customizer(value, source[key], key, object, source);
+
+ if ((result === result ? (result !== value) : (value === value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = result;
+ }
+ }
+ return object;
+}
+
+module.exports = assignWith;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseAssign.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseAssign.js b/node_modules/lodash/internal/baseAssign.js
new file mode 100644
index 0000000..cfad6e0
--- /dev/null
+++ b/node_modules/lodash/internal/baseAssign.js
@@ -0,0 +1,19 @@
+var baseCopy = require('./baseCopy'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.assign` without support for argument juggling,
+ * multiple sources, and `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssign(object, source) {
+ return source == null
+ ? object
+ : baseCopy(source, keys(source), object);
+}
+
+module.exports = baseAssign;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseAt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseAt.js b/node_modules/lodash/internal/baseAt.js
new file mode 100644
index 0000000..bbafd1d
--- /dev/null
+++ b/node_modules/lodash/internal/baseAt.js
@@ -0,0 +1,32 @@
+var isArrayLike = require('./isArrayLike'),
+ isIndex = require('./isIndex');
+
+/**
+ * The base implementation of `_.at` without support for string collections
+ * and individual key arguments.
+ *
+ * @private
+ * @param {Array|Object} collection The collection to iterate over.
+ * @param {number[]|string[]} props The property names or indexes of elements to pick.
+ * @returns {Array} Returns the new array of picked elements.
+ */
+function baseAt(collection, props) {
+ var index = -1,
+ isNil = collection == null,
+ isArr = !isNil && isArrayLike(collection),
+ length = isArr ? collection.length : 0,
+ propsLength = props.length,
+ result = Array(propsLength);
+
+ while(++index < propsLength) {
+ var key = props[index];
+ if (isArr) {
+ result[index] = isIndex(key, length) ? collection[key] : undefined;
+ } else {
+ result[index] = isNil ? undefined : collection[key];
+ }
+ }
+ return result;
+}
+
+module.exports = baseAt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseCallback.js b/node_modules/lodash/internal/baseCallback.js
new file mode 100644
index 0000000..67fe087
--- /dev/null
+++ b/node_modules/lodash/internal/baseCallback.js
@@ -0,0 +1,35 @@
+var baseMatches = require('./baseMatches'),
+ baseMatchesProperty = require('./baseMatchesProperty'),
+ bindCallback = require('./bindCallback'),
+ identity = require('../utility/identity'),
+ property = require('../utility/property');
+
+/**
+ * The base implementation of `_.callback` which supports specifying the
+ * number of arguments to provide to `func`.
+ *
+ * @private
+ * @param {*} [func=_.identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of `func`.
+ * @param {number} [argCount] The number of arguments to provide to `func`.
+ * @returns {Function} Returns the callback.
+ */
+function baseCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (type == 'function') {
+ return thisArg === undefined
+ ? func
+ : bindCallback(func, thisArg, argCount);
+ }
+ if (func == null) {
+ return identity;
+ }
+ if (type == 'object') {
+ return baseMatches(func);
+ }
+ return thisArg === undefined
+ ? property(func)
+ : baseMatchesProperty(func, thisArg);
+}
+
+module.exports = baseCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseClone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseClone.js b/node_modules/lodash/internal/baseClone.js
new file mode 100644
index 0000000..ebd6649
--- /dev/null
+++ b/node_modules/lodash/internal/baseClone.js
@@ -0,0 +1,128 @@
+var arrayCopy = require('./arrayCopy'),
+ arrayEach = require('./arrayEach'),
+ baseAssign = require('./baseAssign'),
+ baseForOwn = require('./baseForOwn'),
+ initCloneArray = require('./initCloneArray'),
+ initCloneByTag = require('./initCloneByTag'),
+ initCloneObject = require('./initCloneObject'),
+ isArray = require('../lang/isArray'),
+ isObject = require('../lang/isObject');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/** Used to identify `toStringTag` values supported by `_.clone`. */
+var cloneableTags = {};
+cloneableTags[argsTag] = cloneableTags[arrayTag] =
+cloneableTags[arrayBufferTag] = cloneableTags[boolTag] =
+cloneableTags[dateTag] = cloneableTags[float32Tag] =
+cloneableTags[float64Tag] = cloneableTags[int8Tag] =
+cloneableTags[int16Tag] = cloneableTags[int32Tag] =
+cloneableTags[numberTag] = cloneableTags[objectTag] =
+cloneableTags[regexpTag] = cloneableTags[stringTag] =
+cloneableTags[uint8Tag] = cloneableTags[uint8ClampedTag] =
+cloneableTags[uint16Tag] = cloneableTags[uint32Tag] = true;
+cloneableTags[errorTag] = cloneableTags[funcTag] =
+cloneableTags[mapTag] = cloneableTags[setTag] =
+cloneableTags[weakMapTag] = false;
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * The base implementation of `_.clone` without support for argument juggling
+ * and `this` binding `customizer` functions.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @param {Function} [customizer] The function to customize cloning values.
+ * @param {string} [key] The key of `value`.
+ * @param {Object} [object] The object `value` belongs to.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates clones with source counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+function baseClone(value, isDeep, customizer, key, object, stackA, stackB) {
+ var result;
+ if (customizer) {
+ result = object ? customizer(value, key, object) : customizer(value);
+ }
+ if (result !== undefined) {
+ return result;
+ }
+ if (!isObject(value)) {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isArr) {
+ result = initCloneArray(value);
+ if (!isDeep) {
+ return arrayCopy(value, result);
+ }
+ } else {
+ var tag = objToString.call(value),
+ isFunc = tag == funcTag;
+
+ if (tag == objectTag || tag == argsTag || (isFunc && !object)) {
+ result = initCloneObject(isFunc ? {} : value);
+ if (!isDeep) {
+ return baseAssign(result, value);
+ }
+ } else {
+ return cloneableTags[tag]
+ ? initCloneByTag(value, tag, isDeep)
+ : (object ? value : {});
+ }
+ }
+ // Check for circular references and return its corresponding clone.
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == value) {
+ return stackB[length];
+ }
+ }
+ // Add the source value to the stack of traversed objects and associate it with its clone.
+ stackA.push(value);
+ stackB.push(result);
+
+ // Recursively populate clone (susceptible to call stack limits).
+ (isArr ? arrayEach : baseForOwn)(value, function(subValue, key) {
+ result[key] = baseClone(subValue, isDeep, customizer, key, value, stackA, stackB);
+ });
+ return result;
+}
+
+module.exports = baseClone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseCompareAscending.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseCompareAscending.js b/node_modules/lodash/internal/baseCompareAscending.js
new file mode 100644
index 0000000..c8259c7
--- /dev/null
+++ b/node_modules/lodash/internal/baseCompareAscending.js
@@ -0,0 +1,34 @@
+/**
+ * The base implementation of `compareAscending` which compares values and
+ * sorts them in ascending order without guaranteeing a stable sort.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {number} Returns the sort order indicator for `value`.
+ */
+function baseCompareAscending(value, other) {
+ if (value !== other) {
+ var valIsNull = value === null,
+ valIsUndef = value === undefined,
+ valIsReflexive = value === value;
+
+ var othIsNull = other === null,
+ othIsUndef = other === undefined,
+ othIsReflexive = other === other;
+
+ if ((value > other && !othIsNull) || !valIsReflexive ||
+ (valIsNull && !othIsUndef && othIsReflexive) ||
+ (valIsUndef && othIsReflexive)) {
+ return 1;
+ }
+ if ((value < other && !valIsNull) || !othIsReflexive ||
+ (othIsNull && !valIsUndef && valIsReflexive) ||
+ (othIsUndef && valIsReflexive)) {
+ return -1;
+ }
+ }
+ return 0;
+}
+
+module.exports = baseCompareAscending;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseCopy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseCopy.js b/node_modules/lodash/internal/baseCopy.js
new file mode 100644
index 0000000..15059f3
--- /dev/null
+++ b/node_modules/lodash/internal/baseCopy.js
@@ -0,0 +1,23 @@
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property names to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @returns {Object} Returns `object`.
+ */
+function baseCopy(source, props, object) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ object[key] = source[key];
+ }
+ return object;
+}
+
+module.exports = baseCopy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseCreate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseCreate.js b/node_modules/lodash/internal/baseCreate.js
new file mode 100644
index 0000000..be5e1d9
--- /dev/null
+++ b/node_modules/lodash/internal/baseCreate.js
@@ -0,0 +1,23 @@
+var isObject = require('../lang/isObject');
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+var baseCreate = (function() {
+ function object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ object.prototype = prototype;
+ var result = new object;
+ object.prototype = undefined;
+ }
+ return result || {};
+ };
+}());
+
+module.exports = baseCreate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseDelay.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseDelay.js b/node_modules/lodash/internal/baseDelay.js
new file mode 100644
index 0000000..c405c37
--- /dev/null
+++ b/node_modules/lodash/internal/baseDelay.js
@@ -0,0 +1,21 @@
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/**
+ * The base implementation of `_.delay` and `_.defer` which accepts an index
+ * of where to slice the arguments to provide to `func`.
+ *
+ * @private
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay invocation.
+ * @param {Object} args The arguments provide to `func`.
+ * @returns {number} Returns the timer id.
+ */
+function baseDelay(func, wait, args) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+}
+
+module.exports = baseDelay;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseDifference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseDifference.js b/node_modules/lodash/internal/baseDifference.js
new file mode 100644
index 0000000..40da1b6
--- /dev/null
+++ b/node_modules/lodash/internal/baseDifference.js
@@ -0,0 +1,55 @@
+var baseIndexOf = require('./baseIndexOf'),
+ cacheIndexOf = require('./cacheIndexOf'),
+ createCache = require('./createCache');
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/**
+ * The base implementation of `_.difference` which accepts a single array
+ * of values to exclude.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Array} values The values to exclude.
+ * @returns {Array} Returns the new array of filtered values.
+ */
+function baseDifference(array, values) {
+ var length = array ? array.length : 0,
+ result = [];
+
+ if (!length) {
+ return result;
+ }
+ var index = -1,
+ indexOf = baseIndexOf,
+ isCommon = true,
+ cache = (isCommon && values.length >= LARGE_ARRAY_SIZE) ? createCache(values) : null,
+ valuesLength = values.length;
+
+ if (cache) {
+ indexOf = cacheIndexOf;
+ isCommon = false;
+ values = cache;
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index];
+
+ if (isCommon && value === value) {
+ var valuesIndex = valuesLength;
+ while (valuesIndex--) {
+ if (values[valuesIndex] === value) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ else if (indexOf(values, value, 0) < 0) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseDifference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseEach.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseEach.js b/node_modules/lodash/internal/baseEach.js
new file mode 100644
index 0000000..09ef5a3
--- /dev/null
+++ b/node_modules/lodash/internal/baseEach.js
@@ -0,0 +1,15 @@
+var baseForOwn = require('./baseForOwn'),
+ createBaseEach = require('./createBaseEach');
+
+/**
+ * The base implementation of `_.forEach` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+var baseEach = createBaseEach(baseForOwn);
+
+module.exports = baseEach;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseEachRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseEachRight.js b/node_modules/lodash/internal/baseEachRight.js
new file mode 100644
index 0000000..f0520a8
--- /dev/null
+++ b/node_modules/lodash/internal/baseEachRight.js
@@ -0,0 +1,15 @@
+var baseForOwnRight = require('./baseForOwnRight'),
+ createBaseEach = require('./createBaseEach');
+
+/**
+ * The base implementation of `_.forEachRight` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array|Object|string} Returns `collection`.
+ */
+var baseEachRight = createBaseEach(baseForOwnRight, true);
+
+module.exports = baseEachRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseEvery.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseEvery.js b/node_modules/lodash/internal/baseEvery.js
new file mode 100644
index 0000000..a1fc1f3
--- /dev/null
+++ b/node_modules/lodash/internal/baseEvery.js
@@ -0,0 +1,22 @@
+var baseEach = require('./baseEach');
+
+/**
+ * The base implementation of `_.every` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if all elements pass the predicate check,
+ * else `false`
+ */
+function baseEvery(collection, predicate) {
+ var result = true;
+ baseEach(collection, function(value, index, collection) {
+ result = !!predicate(value, index, collection);
+ return result;
+ });
+ return result;
+}
+
+module.exports = baseEvery;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseExtremum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseExtremum.js b/node_modules/lodash/internal/baseExtremum.js
new file mode 100644
index 0000000..b0efff6
--- /dev/null
+++ b/node_modules/lodash/internal/baseExtremum.js
@@ -0,0 +1,29 @@
+var baseEach = require('./baseEach');
+
+/**
+ * Gets the extremum value of `collection` invoking `iteratee` for each value
+ * in `collection` to generate the criterion by which the value is ranked.
+ * The `iteratee` is invoked with three arguments: (value, index|key, collection).
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} comparator The function used to compare values.
+ * @param {*} exValue The initial extremum value.
+ * @returns {*} Returns the extremum value.
+ */
+function baseExtremum(collection, iteratee, comparator, exValue) {
+ var computed = exValue,
+ result = computed;
+
+ baseEach(collection, function(value, index, collection) {
+ var current = +iteratee(value, index, collection);
+ if (comparator(current, computed) || (current === exValue && current === result)) {
+ computed = current;
+ result = value;
+ }
+ });
+ return result;
+}
+
+module.exports = baseExtremum;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFill.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFill.js b/node_modules/lodash/internal/baseFill.js
new file mode 100644
index 0000000..ef1a2fa
--- /dev/null
+++ b/node_modules/lodash/internal/baseFill.js
@@ -0,0 +1,31 @@
+/**
+ * The base implementation of `_.fill` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to fill.
+ * @param {*} value The value to fill `array` with.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns `array`.
+ */
+function baseFill(array, value, start, end) {
+ var length = array.length;
+
+ start = start == null ? 0 : (+start || 0);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : (+end || 0);
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : (end >>> 0);
+ start >>>= 0;
+
+ while (start < length) {
+ array[start++] = value;
+ }
+ return array;
+}
+
+module.exports = baseFill;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFilter.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFilter.js b/node_modules/lodash/internal/baseFilter.js
new file mode 100644
index 0000000..27773a4
--- /dev/null
+++ b/node_modules/lodash/internal/baseFilter.js
@@ -0,0 +1,22 @@
+var baseEach = require('./baseEach');
+
+/**
+ * The base implementation of `_.filter` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {Array} Returns the new filtered array.
+ */
+function baseFilter(collection, predicate) {
+ var result = [];
+ baseEach(collection, function(value, index, collection) {
+ if (predicate(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ return result;
+}
+
+module.exports = baseFilter;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFind.js b/node_modules/lodash/internal/baseFind.js
new file mode 100644
index 0000000..be5848f
--- /dev/null
+++ b/node_modules/lodash/internal/baseFind.js
@@ -0,0 +1,25 @@
+/**
+ * The base implementation of `_.find`, `_.findLast`, `_.findKey`, and `_.findLastKey`,
+ * without support for callback shorthands and `this` binding, which iterates
+ * over `collection` using the provided `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to search.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @param {boolean} [retKey] Specify returning the key of the found element
+ * instead of the element itself.
+ * @returns {*} Returns the found element or its key, else `undefined`.
+ */
+function baseFind(collection, predicate, eachFunc, retKey) {
+ var result;
+ eachFunc(collection, function(value, key, collection) {
+ if (predicate(value, key, collection)) {
+ result = retKey ? key : value;
+ return false;
+ }
+ });
+ return result;
+}
+
+module.exports = baseFind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFindIndex.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFindIndex.js b/node_modules/lodash/internal/baseFindIndex.js
new file mode 100644
index 0000000..7d4b502
--- /dev/null
+++ b/node_modules/lodash/internal/baseFindIndex.js
@@ -0,0 +1,23 @@
+/**
+ * The base implementation of `_.findIndex` and `_.findLastIndex` without
+ * support for callback shorthands and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {Function} predicate The function invoked per iteration.
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function baseFindIndex(array, predicate, fromRight) {
+ var length = array.length,
+ index = fromRight ? length : -1;
+
+ while ((fromRight ? index-- : ++index < length)) {
+ if (predicate(array[index], index, array)) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseFindIndex;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFlatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFlatten.js b/node_modules/lodash/internal/baseFlatten.js
new file mode 100644
index 0000000..7443233
--- /dev/null
+++ b/node_modules/lodash/internal/baseFlatten.js
@@ -0,0 +1,41 @@
+var arrayPush = require('./arrayPush'),
+ isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isArrayLike = require('./isArrayLike'),
+ isObjectLike = require('./isObjectLike');
+
+/**
+ * The base implementation of `_.flatten` with added support for restricting
+ * flattening and specifying the start index.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isDeep] Specify a deep flatten.
+ * @param {boolean} [isStrict] Restrict flattening to arrays-like objects.
+ * @param {Array} [result=[]] The initial result value.
+ * @returns {Array} Returns the new flattened array.
+ */
+function baseFlatten(array, isDeep, isStrict, result) {
+ result || (result = []);
+
+ var index = -1,
+ length = array.length;
+
+ while (++index < length) {
+ var value = array[index];
+ if (isObjectLike(value) && isArrayLike(value) &&
+ (isStrict || isArray(value) || isArguments(value))) {
+ if (isDeep) {
+ // Recursively flatten arrays (susceptible to call stack limits).
+ baseFlatten(value, isDeep, isStrict, result);
+ } else {
+ arrayPush(result, value);
+ }
+ } else if (!isStrict) {
+ result[result.length] = value;
+ }
+ }
+ return result;
+}
+
+module.exports = baseFlatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFor.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFor.js b/node_modules/lodash/internal/baseFor.js
new file mode 100644
index 0000000..94ee03f
--- /dev/null
+++ b/node_modules/lodash/internal/baseFor.js
@@ -0,0 +1,17 @@
+var createBaseFor = require('./createBaseFor');
+
+/**
+ * The base implementation of `baseForIn` and `baseForOwn` which iterates
+ * over `object` properties returned by `keysFunc` invoking `iteratee` for
+ * each property. Iteratee functions may exit iteration early by explicitly
+ * returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseFor = createBaseFor();
+
+module.exports = baseFor;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseForIn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseForIn.js b/node_modules/lodash/internal/baseForIn.js
new file mode 100644
index 0000000..47d622c
--- /dev/null
+++ b/node_modules/lodash/internal/baseForIn.js
@@ -0,0 +1,17 @@
+var baseFor = require('./baseFor'),
+ keysIn = require('../object/keysIn');
+
+/**
+ * The base implementation of `_.forIn` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForIn(object, iteratee) {
+ return baseFor(object, iteratee, keysIn);
+}
+
+module.exports = baseForIn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseForOwn.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseForOwn.js b/node_modules/lodash/internal/baseForOwn.js
new file mode 100644
index 0000000..bef4d4c
--- /dev/null
+++ b/node_modules/lodash/internal/baseForOwn.js
@@ -0,0 +1,17 @@
+var baseFor = require('./baseFor'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.forOwn` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForOwn(object, iteratee) {
+ return baseFor(object, iteratee, keys);
+}
+
+module.exports = baseForOwn;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseForOwnRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseForOwnRight.js b/node_modules/lodash/internal/baseForOwnRight.js
new file mode 100644
index 0000000..bb916bc
--- /dev/null
+++ b/node_modules/lodash/internal/baseForOwnRight.js
@@ -0,0 +1,17 @@
+var baseForRight = require('./baseForRight'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.forOwnRight` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Object} Returns `object`.
+ */
+function baseForOwnRight(object, iteratee) {
+ return baseForRight(object, iteratee, keys);
+}
+
+module.exports = baseForOwnRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseForRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseForRight.js b/node_modules/lodash/internal/baseForRight.js
new file mode 100644
index 0000000..5ddd191
--- /dev/null
+++ b/node_modules/lodash/internal/baseForRight.js
@@ -0,0 +1,15 @@
+var createBaseFor = require('./createBaseFor');
+
+/**
+ * This function is like `baseFor` except that it iterates over properties
+ * in the opposite order.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseForRight = createBaseFor(true);
+
+module.exports = baseForRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseFunctions.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseFunctions.js b/node_modules/lodash/internal/baseFunctions.js
new file mode 100644
index 0000000..d56ea9c
--- /dev/null
+++ b/node_modules/lodash/internal/baseFunctions.js
@@ -0,0 +1,27 @@
+var isFunction = require('../lang/isFunction');
+
+/**
+ * The base implementation of `_.functions` which creates an array of
+ * `object` function property names filtered from those provided.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} props The property names to filter.
+ * @returns {Array} Returns the new array of filtered property names.
+ */
+function baseFunctions(object, props) {
+ var index = -1,
+ length = props.length,
+ resIndex = -1,
+ result = [];
+
+ while (++index < length) {
+ var key = props[index];
+ if (isFunction(object[key])) {
+ result[++resIndex] = key;
+ }
+ }
+ return result;
+}
+
+module.exports = baseFunctions;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseGet.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseGet.js b/node_modules/lodash/internal/baseGet.js
new file mode 100644
index 0000000..ad9b1ee
--- /dev/null
+++ b/node_modules/lodash/internal/baseGet.js
@@ -0,0 +1,29 @@
+var toObject = require('./toObject');
+
+/**
+ * The base implementation of `get` without support for string paths
+ * and default values.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} path The path of the property to get.
+ * @param {string} [pathKey] The key representation of path.
+ * @returns {*} Returns the resolved value.
+ */
+function baseGet(object, path, pathKey) {
+ if (object == null) {
+ return;
+ }
+ if (pathKey !== undefined && pathKey in toObject(object)) {
+ path = [pathKey];
+ }
+ var index = 0,
+ length = path.length;
+
+ while (object != null && index < length) {
+ object = object[path[index++]];
+ }
+ return (index && index == length) ? object : undefined;
+}
+
+module.exports = baseGet;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseIndexOf.js b/node_modules/lodash/internal/baseIndexOf.js
new file mode 100644
index 0000000..6b479bc
--- /dev/null
+++ b/node_modules/lodash/internal/baseIndexOf.js
@@ -0,0 +1,27 @@
+var indexOfNaN = require('./indexOfNaN');
+
+/**
+ * The base implementation of `_.indexOf` without support for binary searches.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} fromIndex The index to search from.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function baseIndexOf(array, value, fromIndex) {
+ if (value !== value) {
+ return indexOfNaN(array, fromIndex);
+ }
+ var index = fromIndex - 1,
+ length = array.length;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseIsEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseIsEqual.js b/node_modules/lodash/internal/baseIsEqual.js
new file mode 100644
index 0000000..87e14ac
--- /dev/null
+++ b/node_modules/lodash/internal/baseIsEqual.js
@@ -0,0 +1,28 @@
+var baseIsEqualDeep = require('./baseIsEqualDeep'),
+ isObject = require('../lang/isObject'),
+ isObjectLike = require('./isObjectLike');
+
+/**
+ * The base implementation of `_.isEqual` without support for `this` binding
+ * `customizer` functions.
+ *
+ * @private
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @param {Function} [customizer] The function to customize comparing values.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA] Tracks traversed `value` objects.
+ * @param {Array} [stackB] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(value, other, customizer, isLoose, stackA, stackB) {
+ if (value === other) {
+ return true;
+ }
+ if (value == null || other == null || (!isObject(value) && !isObjectLike(other))) {
+ return value !== value && other !== other;
+ }
+ return baseIsEqualDeep(value, other, baseIsEqual, customizer, isLoose, stackA, stackB);
+}
+
+module.exports = baseIsEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseIsEqualDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseIsEqualDeep.js b/node_modules/lodash/internal/baseIsEqualDeep.js
new file mode 100644
index 0000000..f1dbffe
--- /dev/null
+++ b/node_modules/lodash/internal/baseIsEqualDeep.js
@@ -0,0 +1,102 @@
+var equalArrays = require('./equalArrays'),
+ equalByTag = require('./equalByTag'),
+ equalObjects = require('./equalObjects'),
+ isArray = require('../lang/isArray'),
+ isTypedArray = require('../lang/isTypedArray');
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ objectTag = '[object Object]';
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * A specialized version of `baseIsEqual` for arrays and objects which performs
+ * deep comparisons and tracks traversed objects enabling objects with circular
+ * references to be compared.
+ *
+ * @private
+ * @param {Object} object The object to compare.
+ * @param {Object} other The other object to compare.
+ * @param {Function} equalFunc The function to determine equivalents of values.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @param {boolean} [isLoose] Specify performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `value` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `other` objects.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function baseIsEqualDeep(object, other, equalFunc, customizer, isLoose, stackA, stackB) {
+ var objIsArr = isArray(object),
+ othIsArr = isArray(other),
+ objTag = arrayTag,
+ othTag = arrayTag;
+
+ if (!objIsArr) {
+ objTag = objToString.call(object);
+ if (objTag == argsTag) {
+ objTag = objectTag;
+ } else if (objTag != objectTag) {
+ objIsArr = isTypedArray(object);
+ }
+ }
+ if (!othIsArr) {
+ othTag = objToString.call(other);
+ if (othTag == argsTag) {
+ othTag = objectTag;
+ } else if (othTag != objectTag) {
+ othIsArr = isTypedArray(other);
+ }
+ }
+ var objIsObj = objTag == objectTag,
+ othIsObj = othTag == objectTag,
+ isSameTag = objTag == othTag;
+
+ if (isSameTag && !(objIsArr || objIsObj)) {
+ return equalByTag(object, other, objTag);
+ }
+ if (!isLoose) {
+ var objIsWrapped = objIsObj && hasOwnProperty.call(object, '__wrapped__'),
+ othIsWrapped = othIsObj && hasOwnProperty.call(other, '__wrapped__');
+
+ if (objIsWrapped || othIsWrapped) {
+ return equalFunc(objIsWrapped ? object.value() : object, othIsWrapped ? other.value() : other, customizer, isLoose, stackA, stackB);
+ }
+ }
+ if (!isSameTag) {
+ return false;
+ }
+ // Assume cyclic values are equal.
+ // For more information on detecting circular references see https://es5.github.io/#JO.
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == object) {
+ return stackB[length] == other;
+ }
+ }
+ // Add `object` and `other` to the stack of traversed objects.
+ stackA.push(object);
+ stackB.push(other);
+
+ var result = (objIsArr ? equalArrays : equalObjects)(object, other, equalFunc, customizer, isLoose, stackA, stackB);
+
+ stackA.pop();
+ stackB.pop();
+
+ return result;
+}
+
+module.exports = baseIsEqualDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseIsFunction.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseIsFunction.js b/node_modules/lodash/internal/baseIsFunction.js
new file mode 100644
index 0000000..cd92db3
--- /dev/null
+++ b/node_modules/lodash/internal/baseIsFunction.js
@@ -0,0 +1,15 @@
+/**
+ * The base implementation of `_.isFunction` without support for environments
+ * with incorrect `typeof` results.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ */
+function baseIsFunction(value) {
+ // Avoid a Chakra JIT bug in compatibility modes of IE 11.
+ // See https://github.com/jashkenas/underscore/issues/1621 for more details.
+ return typeof value == 'function' || false;
+}
+
+module.exports = baseIsFunction;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseIsMatch.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseIsMatch.js b/node_modules/lodash/internal/baseIsMatch.js
new file mode 100644
index 0000000..ea48bb6
--- /dev/null
+++ b/node_modules/lodash/internal/baseIsMatch.js
@@ -0,0 +1,52 @@
+var baseIsEqual = require('./baseIsEqual'),
+ toObject = require('./toObject');
+
+/**
+ * The base implementation of `_.isMatch` without support for callback
+ * shorthands and `this` binding.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @param {Array} matchData The propery names, values, and compare flags to match.
+ * @param {Function} [customizer] The function to customize comparing objects.
+ * @returns {boolean} Returns `true` if `object` is a match, else `false`.
+ */
+function baseIsMatch(object, matchData, customizer) {
+ var index = matchData.length,
+ length = index,
+ noCustomizer = !customizer;
+
+ if (object == null) {
+ return !length;
+ }
+ object = toObject(object);
+ while (index--) {
+ var data = matchData[index];
+ if ((noCustomizer && data[2])
+ ? data[1] !== object[data[0]]
+ : !(data[0] in object)
+ ) {
+ return false;
+ }
+ }
+ while (++index < length) {
+ data = matchData[index];
+ var key = data[0],
+ objValue = object[key],
+ srcValue = data[1];
+
+ if (noCustomizer && data[2]) {
+ if (objValue === undefined && !(key in object)) {
+ return false;
+ }
+ } else {
+ var result = customizer ? customizer(objValue, srcValue, key) : undefined;
+ if (!(result === undefined ? baseIsEqual(srcValue, objValue, customizer, true) : result)) {
+ return false;
+ }
+ }
+ }
+ return true;
+}
+
+module.exports = baseIsMatch;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseLodash.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseLodash.js b/node_modules/lodash/internal/baseLodash.js
new file mode 100644
index 0000000..15b79d3
--- /dev/null
+++ b/node_modules/lodash/internal/baseLodash.js
@@ -0,0 +1,10 @@
+/**
+ * The function whose prototype all chaining wrappers inherit from.
+ *
+ * @private
+ */
+function baseLodash() {
+ // No operation performed.
+}
+
+module.exports = baseLodash;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseMap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseMap.js b/node_modules/lodash/internal/baseMap.js
new file mode 100644
index 0000000..2906b51
--- /dev/null
+++ b/node_modules/lodash/internal/baseMap.js
@@ -0,0 +1,23 @@
+var baseEach = require('./baseEach'),
+ isArrayLike = require('./isArrayLike');
+
+/**
+ * The base implementation of `_.map` without support for callback shorthands
+ * and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the new mapped array.
+ */
+function baseMap(collection, iteratee) {
+ var index = -1,
+ result = isArrayLike(collection) ? Array(collection.length) : [];
+
+ baseEach(collection, function(value, key, collection) {
+ result[++index] = iteratee(value, key, collection);
+ });
+ return result;
+}
+
+module.exports = baseMap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseMatches.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseMatches.js b/node_modules/lodash/internal/baseMatches.js
new file mode 100644
index 0000000..5f76c67
--- /dev/null
+++ b/node_modules/lodash/internal/baseMatches.js
@@ -0,0 +1,30 @@
+var baseIsMatch = require('./baseIsMatch'),
+ getMatchData = require('./getMatchData'),
+ toObject = require('./toObject');
+
+/**
+ * The base implementation of `_.matches` which does not clone `source`.
+ *
+ * @private
+ * @param {Object} source The object of property values to match.
+ * @returns {Function} Returns the new function.
+ */
+function baseMatches(source) {
+ var matchData = getMatchData(source);
+ if (matchData.length == 1 && matchData[0][2]) {
+ var key = matchData[0][0],
+ value = matchData[0][1];
+
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ return object[key] === value && (value !== undefined || (key in toObject(object)));
+ };
+ }
+ return function(object) {
+ return baseIsMatch(object, matchData);
+ };
+}
+
+module.exports = baseMatches;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseMatchesProperty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseMatchesProperty.js b/node_modules/lodash/internal/baseMatchesProperty.js
new file mode 100644
index 0000000..8f9005c
--- /dev/null
+++ b/node_modules/lodash/internal/baseMatchesProperty.js
@@ -0,0 +1,45 @@
+var baseGet = require('./baseGet'),
+ baseIsEqual = require('./baseIsEqual'),
+ baseSlice = require('./baseSlice'),
+ isArray = require('../lang/isArray'),
+ isKey = require('./isKey'),
+ isStrictComparable = require('./isStrictComparable'),
+ last = require('../array/last'),
+ toObject = require('./toObject'),
+ toPath = require('./toPath');
+
+/**
+ * The base implementation of `_.matchesProperty` which does not clone `srcValue`.
+ *
+ * @private
+ * @param {string} path The path of the property to get.
+ * @param {*} srcValue The value to compare.
+ * @returns {Function} Returns the new function.
+ */
+function baseMatchesProperty(path, srcValue) {
+ var isArr = isArray(path),
+ isCommon = isKey(path) && isStrictComparable(srcValue),
+ pathKey = (path + '');
+
+ path = toPath(path);
+ return function(object) {
+ if (object == null) {
+ return false;
+ }
+ var key = pathKey;
+ object = toObject(object);
+ if ((isArr || !isCommon) && !(key in object)) {
+ object = path.length == 1 ? object : baseGet(object, baseSlice(path, 0, -1));
+ if (object == null) {
+ return false;
+ }
+ key = last(path);
+ object = toObject(object);
+ }
+ return object[key] === srcValue
+ ? (srcValue !== undefined || (key in object))
+ : baseIsEqual(srcValue, object[key], undefined, true);
+ };
+}
+
+module.exports = baseMatchesProperty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseMerge.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseMerge.js b/node_modules/lodash/internal/baseMerge.js
new file mode 100644
index 0000000..ab81900
--- /dev/null
+++ b/node_modules/lodash/internal/baseMerge.js
@@ -0,0 +1,56 @@
+var arrayEach = require('./arrayEach'),
+ baseMergeDeep = require('./baseMergeDeep'),
+ isArray = require('../lang/isArray'),
+ isArrayLike = require('./isArrayLike'),
+ isObject = require('../lang/isObject'),
+ isObjectLike = require('./isObjectLike'),
+ isTypedArray = require('../lang/isTypedArray'),
+ keys = require('../object/keys');
+
+/**
+ * The base implementation of `_.merge` without support for argument juggling,
+ * multiple sources, and `this` binding `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates values with source counterparts.
+ * @returns {Object} Returns `object`.
+ */
+function baseMerge(object, source, customizer, stackA, stackB) {
+ if (!isObject(object)) {
+ return object;
+ }
+ var isSrcArr = isArrayLike(source) && (isArray(source) || isTypedArray(source)),
+ props = isSrcArr ? undefined : keys(source);
+
+ arrayEach(props || source, function(srcValue, key) {
+ if (props) {
+ key = srcValue;
+ srcValue = source[key];
+ }
+ if (isObjectLike(srcValue)) {
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+ baseMergeDeep(object, source, key, baseMerge, customizer, stackA, stackB);
+ }
+ else {
+ var value = object[key],
+ result = customizer ? customizer(value, srcValue, key, object, source) : undefined,
+ isCommon = result === undefined;
+
+ if (isCommon) {
+ result = srcValue;
+ }
+ if ((result !== undefined || (isSrcArr && !(key in object))) &&
+ (isCommon || (result === result ? (result !== value) : (value === value)))) {
+ object[key] = result;
+ }
+ }
+ });
+ return object;
+}
+
+module.exports = baseMerge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseMergeDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseMergeDeep.js b/node_modules/lodash/internal/baseMergeDeep.js
new file mode 100644
index 0000000..f8aeac5
--- /dev/null
+++ b/node_modules/lodash/internal/baseMergeDeep.js
@@ -0,0 +1,67 @@
+var arrayCopy = require('./arrayCopy'),
+ isArguments = require('../lang/isArguments'),
+ isArray = require('../lang/isArray'),
+ isArrayLike = require('./isArrayLike'),
+ isPlainObject = require('../lang/isPlainObject'),
+ isTypedArray = require('../lang/isTypedArray'),
+ toPlainObject = require('../lang/toPlainObject');
+
+/**
+ * A specialized version of `baseMerge` for arrays and objects which performs
+ * deep merges and tracks traversed objects enabling objects with circular
+ * references to be merged.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {string} key The key of the value to merge.
+ * @param {Function} mergeFunc The function to merge values.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates values with source counterparts.
+ * @returns {boolean} Returns `true` if the objects are equivalent, else `false`.
+ */
+function baseMergeDeep(object, source, key, mergeFunc, customizer, stackA, stackB) {
+ var length = stackA.length,
+ srcValue = source[key];
+
+ while (length--) {
+ if (stackA[length] == srcValue) {
+ object[key] = stackB[length];
+ return;
+ }
+ }
+ var value = object[key],
+ result = customizer ? customizer(value, srcValue, key, object, source) : undefined,
+ isCommon = result === undefined;
+
+ if (isCommon) {
+ result = srcValue;
+ if (isArrayLike(srcValue) && (isArray(srcValue) || isTypedArray(srcValue))) {
+ result = isArray(value)
+ ? value
+ : (isArrayLike(value) ? arrayCopy(value) : []);
+ }
+ else if (isPlainObject(srcValue) || isArguments(srcValue)) {
+ result = isArguments(value)
+ ? toPlainObject(value)
+ : (isPlainObject(value) ? value : {});
+ }
+ else {
+ isCommon = false;
+ }
+ }
+ // Add the source value to the stack of traversed objects and associate
+ // it with its merged value.
+ stackA.push(srcValue);
+ stackB.push(result);
+
+ if (isCommon) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ object[key] = mergeFunc(result, srcValue, customizer, stackA, stackB);
+ } else if (result === result ? (result !== value) : (value === value)) {
+ object[key] = result;
+ }
+}
+
+module.exports = baseMergeDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseProperty.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseProperty.js b/node_modules/lodash/internal/baseProperty.js
new file mode 100644
index 0000000..e515941
--- /dev/null
+++ b/node_modules/lodash/internal/baseProperty.js
@@ -0,0 +1,14 @@
+/**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+module.exports = baseProperty;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/basePropertyDeep.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/basePropertyDeep.js b/node_modules/lodash/internal/basePropertyDeep.js
new file mode 100644
index 0000000..1b6ce40
--- /dev/null
+++ b/node_modules/lodash/internal/basePropertyDeep.js
@@ -0,0 +1,19 @@
+var baseGet = require('./baseGet'),
+ toPath = require('./toPath');
+
+/**
+ * A specialized version of `baseProperty` which supports deep paths.
+ *
+ * @private
+ * @param {Array|string} path The path of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function basePropertyDeep(path) {
+ var pathKey = (path + '');
+ path = toPath(path);
+ return function(object) {
+ return baseGet(object, path, pathKey);
+ };
+}
+
+module.exports = basePropertyDeep;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/basePullAt.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/basePullAt.js b/node_modules/lodash/internal/basePullAt.js
new file mode 100644
index 0000000..6c4ff84
--- /dev/null
+++ b/node_modules/lodash/internal/basePullAt.js
@@ -0,0 +1,30 @@
+var isIndex = require('./isIndex');
+
+/** Used for native method references. */
+var arrayProto = Array.prototype;
+
+/** Native method references. */
+var splice = arrayProto.splice;
+
+/**
+ * The base implementation of `_.pullAt` without support for individual
+ * index arguments and capturing the removed elements.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {number[]} indexes The indexes of elements to remove.
+ * @returns {Array} Returns `array`.
+ */
+function basePullAt(array, indexes) {
+ var length = array ? indexes.length : 0;
+ while (length--) {
+ var index = indexes[length];
+ if (index != previous && isIndex(index)) {
+ var previous = index;
+ splice.call(array, index, 1);
+ }
+ }
+ return array;
+}
+
+module.exports = basePullAt;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseRandom.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseRandom.js b/node_modules/lodash/internal/baseRandom.js
new file mode 100644
index 0000000..fa3326c
--- /dev/null
+++ b/node_modules/lodash/internal/baseRandom.js
@@ -0,0 +1,18 @@
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeFloor = Math.floor,
+ nativeRandom = Math.random;
+
+/**
+ * The base implementation of `_.random` without support for argument juggling
+ * and returning floating-point numbers.
+ *
+ * @private
+ * @param {number} min The minimum possible value.
+ * @param {number} max The maximum possible value.
+ * @returns {number} Returns the random number.
+ */
+function baseRandom(min, max) {
+ return min + nativeFloor(nativeRandom() * (max - min + 1));
+}
+
+module.exports = baseRandom;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseReduce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseReduce.js b/node_modules/lodash/internal/baseReduce.js
new file mode 100644
index 0000000..5e6ae55
--- /dev/null
+++ b/node_modules/lodash/internal/baseReduce.js
@@ -0,0 +1,24 @@
+/**
+ * The base implementation of `_.reduce` and `_.reduceRight` without support
+ * for callback shorthands and `this` binding, which iterates over `collection`
+ * using the provided `eachFunc`.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} accumulator The initial value.
+ * @param {boolean} initFromCollection Specify using the first or last element
+ * of `collection` as the initial value.
+ * @param {Function} eachFunc The function to iterate over `collection`.
+ * @returns {*} Returns the accumulated value.
+ */
+function baseReduce(collection, iteratee, accumulator, initFromCollection, eachFunc) {
+ eachFunc(collection, function(value, index, collection) {
+ accumulator = initFromCollection
+ ? (initFromCollection = false, value)
+ : iteratee(accumulator, value, index, collection);
+ });
+ return accumulator;
+}
+
+module.exports = baseReduce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseSetData.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseSetData.js b/node_modules/lodash/internal/baseSetData.js
new file mode 100644
index 0000000..5c98622
--- /dev/null
+++ b/node_modules/lodash/internal/baseSetData.js
@@ -0,0 +1,17 @@
+var identity = require('../utility/identity'),
+ metaMap = require('./metaMap');
+
+/**
+ * The base implementation of `setData` without support for hot loop detection.
+ *
+ * @private
+ * @param {Function} func The function to associate metadata with.
+ * @param {*} data The metadata.
+ * @returns {Function} Returns `func`.
+ */
+var baseSetData = !metaMap ? identity : function(func, data) {
+ metaMap.set(func, data);
+ return func;
+};
+
+module.exports = baseSetData;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseSlice.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseSlice.js b/node_modules/lodash/internal/baseSlice.js
new file mode 100644
index 0000000..9d1012e
--- /dev/null
+++ b/node_modules/lodash/internal/baseSlice.js
@@ -0,0 +1,32 @@
+/**
+ * The base implementation of `_.slice` without an iteratee call guard.
+ *
+ * @private
+ * @param {Array} array The array to slice.
+ * @param {number} [start=0] The start position.
+ * @param {number} [end=array.length] The end position.
+ * @returns {Array} Returns the slice of `array`.
+ */
+function baseSlice(array, start, end) {
+ var index = -1,
+ length = array.length;
+
+ start = start == null ? 0 : (+start || 0);
+ if (start < 0) {
+ start = -start > length ? 0 : (length + start);
+ }
+ end = (end === undefined || end > length) ? length : (+end || 0);
+ if (end < 0) {
+ end += length;
+ }
+ length = start > end ? 0 : ((end - start) >>> 0);
+ start >>>= 0;
+
+ var result = Array(length);
+ while (++index < length) {
+ result[index] = array[index + start];
+ }
+ return result;
+}
+
+module.exports = baseSlice;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseSome.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseSome.js b/node_modules/lodash/internal/baseSome.js
new file mode 100644
index 0000000..39a0058
--- /dev/null
+++ b/node_modules/lodash/internal/baseSome.js
@@ -0,0 +1,23 @@
+var baseEach = require('./baseEach');
+
+/**
+ * The base implementation of `_.some` without support for callback shorthands
+ * and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} predicate The function invoked per iteration.
+ * @returns {boolean} Returns `true` if any element passes the predicate check,
+ * else `false`.
+ */
+function baseSome(collection, predicate) {
+ var result;
+
+ baseEach(collection, function(value, index, collection) {
+ result = predicate(value, index, collection);
+ return !result;
+ });
+ return !!result;
+}
+
+module.exports = baseSome;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseSortBy.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseSortBy.js b/node_modules/lodash/internal/baseSortBy.js
new file mode 100644
index 0000000..fec0afe
--- /dev/null
+++ b/node_modules/lodash/internal/baseSortBy.js
@@ -0,0 +1,21 @@
+/**
+ * The base implementation of `_.sortBy` which uses `comparer` to define
+ * the sort order of `array` and replaces criteria objects with their
+ * corresponding values.
+ *
+ * @private
+ * @param {Array} array The array to sort.
+ * @param {Function} comparer The function to define sort order.
+ * @returns {Array} Returns `array`.
+ */
+function baseSortBy(array, comparer) {
+ var length = array.length;
+
+ array.sort(comparer);
+ while (length--) {
+ array[length] = array[length].value;
+ }
+ return array;
+}
+
+module.exports = baseSortBy;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseSortByOrder.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseSortByOrder.js b/node_modules/lodash/internal/baseSortByOrder.js
new file mode 100644
index 0000000..0a9ef20
--- /dev/null
+++ b/node_modules/lodash/internal/baseSortByOrder.js
@@ -0,0 +1,31 @@
+var arrayMap = require('./arrayMap'),
+ baseCallback = require('./baseCallback'),
+ baseMap = require('./baseMap'),
+ baseSortBy = require('./baseSortBy'),
+ compareMultiple = require('./compareMultiple');
+
+/**
+ * The base implementation of `_.sortByOrder` without param guards.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function[]|Object[]|string[]} iteratees The iteratees to sort by.
+ * @param {boolean[]} orders The sort orders of `iteratees`.
+ * @returns {Array} Returns the new sorted array.
+ */
+function baseSortByOrder(collection, iteratees, orders) {
+ var index = -1;
+
+ iteratees = arrayMap(iteratees, function(iteratee) { return baseCallback(iteratee); });
+
+ var result = baseMap(collection, function(value) {
+ var criteria = arrayMap(iteratees, function(iteratee) { return iteratee(value); });
+ return { 'criteria': criteria, 'index': ++index, 'value': value };
+ });
+
+ return baseSortBy(result, function(object, other) {
+ return compareMultiple(object, other, orders);
+ });
+}
+
+module.exports = baseSortByOrder;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseSum.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseSum.js b/node_modules/lodash/internal/baseSum.js
new file mode 100644
index 0000000..019e5ae
--- /dev/null
+++ b/node_modules/lodash/internal/baseSum.js
@@ -0,0 +1,20 @@
+var baseEach = require('./baseEach');
+
+/**
+ * The base implementation of `_.sum` without support for callback shorthands
+ * and `this` binding.
+ *
+ * @private
+ * @param {Array|Object|string} collection The collection to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {number} Returns the sum.
+ */
+function baseSum(collection, iteratee) {
+ var result = 0;
+ baseEach(collection, function(value, index, collection) {
+ result += +iteratee(value, index, collection) || 0;
+ });
+ return result;
+}
+
+module.exports = baseSum;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseToString.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseToString.js b/node_modules/lodash/internal/baseToString.js
new file mode 100644
index 0000000..b802640
--- /dev/null
+++ b/node_modules/lodash/internal/baseToString.js
@@ -0,0 +1,13 @@
+/**
+ * Converts `value` to a string if it's not one. An empty string is returned
+ * for `null` or `undefined` values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+function baseToString(value) {
+ return value == null ? '' : (value + '');
+}
+
+module.exports = baseToString;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseUniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseUniq.js b/node_modules/lodash/internal/baseUniq.js
new file mode 100644
index 0000000..a043443
--- /dev/null
+++ b/node_modules/lodash/internal/baseUniq.js
@@ -0,0 +1,60 @@
+var baseIndexOf = require('./baseIndexOf'),
+ cacheIndexOf = require('./cacheIndexOf'),
+ createCache = require('./createCache');
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/**
+ * The base implementation of `_.uniq` without support for callback shorthands
+ * and `this` binding.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {Function} [iteratee] The function invoked per iteration.
+ * @returns {Array} Returns the new duplicate free array.
+ */
+function baseUniq(array, iteratee) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array.length,
+ isCommon = true,
+ isLarge = isCommon && length >= LARGE_ARRAY_SIZE,
+ seen = isLarge ? createCache() : null,
+ result = [];
+
+ if (seen) {
+ indexOf = cacheIndexOf;
+ isCommon = false;
+ } else {
+ isLarge = false;
+ seen = iteratee ? [] : result;
+ }
+ outer:
+ while (++index < length) {
+ var value = array[index],
+ computed = iteratee ? iteratee(value, index, array) : value;
+
+ if (isCommon && value === value) {
+ var seenIndex = seen.length;
+ while (seenIndex--) {
+ if (seen[seenIndex] === computed) {
+ continue outer;
+ }
+ }
+ if (iteratee) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ else if (indexOf(seen, computed, 0) < 0) {
+ if (iteratee || isLarge) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseUniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash/internal/baseValues.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash/internal/baseValues.js b/node_modules/lodash/internal/baseValues.js
new file mode 100644
index 0000000..e8d3ac7
--- /dev/null
+++ b/node_modules/lodash/internal/baseValues.js
@@ -0,0 +1,22 @@
+/**
+ * The base implementation of `_.values` and `_.valuesIn` which creates an
+ * array of `object` property values corresponding to the property names
+ * of `props`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the array of property values.
+ */
+function baseValues(object, props) {
+ var index = -1,
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = object[props[index]];
+ }
+ return result;
+}
+
+module.exports = baseValues;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[59/59] [abbrv] mac commit: Set VERSION to 4.0.1 (via coho)
Posted by st...@apache.org.
Set VERSION to 4.0.1 (via coho)
Project: http://git-wip-us.apache.org/repos/asf/cordova-osx/repo
Commit: http://git-wip-us.apache.org/repos/asf/cordova-osx/commit/a8e7d75a
Tree: http://git-wip-us.apache.org/repos/asf/cordova-osx/tree/a8e7d75a
Diff: http://git-wip-us.apache.org/repos/asf/cordova-osx/diff/a8e7d75a
Branch: refs/heads/4.0.x
Commit: a8e7d75aaad9850ffe111effe7925b45ec0b674b
Parents: 199e1ab
Author: Steve Gill <st...@gmail.com>
Authored: Thu Mar 3 15:45:28 2016 -0800
Committer: Steve Gill <st...@gmail.com>
Committed: Thu Mar 3 15:45:28 2016 -0800
----------------------------------------------------------------------
CordovaLib/VERSION | 2 +-
package.json | 4 ++--
2 files changed, 3 insertions(+), 3 deletions(-)
----------------------------------------------------------------------
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/a8e7d75a/CordovaLib/VERSION
----------------------------------------------------------------------
diff --git a/CordovaLib/VERSION b/CordovaLib/VERSION
index fcdb2e1..1454f6e 100644
--- a/CordovaLib/VERSION
+++ b/CordovaLib/VERSION
@@ -1 +1 @@
-4.0.0
+4.0.1
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/a8e7d75a/package.json
----------------------------------------------------------------------
diff --git a/package.json b/package.json
index b619150..9a9d596 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
"name": "cordova-osx",
- "version": "4.0.0",
+ "version": "4.0.1",
"description": "cordova-osx release",
"main": "bin/templates/scripts/cordova/Api.js",
"repository": {
@@ -49,4 +49,4 @@
"coffee-script": "^1.7.1",
"nodeunit": "^0.8.7"
}
-}
+}
\ No newline at end of file
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[36/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/createCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/createCallback.js b/node_modules/lodash-node/modern/functions/createCallback.js
new file mode 100644
index 0000000..dcf255b
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/createCallback.js
@@ -0,0 +1,81 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreateCallback = require('../internals/baseCreateCallback'),
+ baseIsEqual = require('../internals/baseIsEqual'),
+ isObject = require('../objects/isObject'),
+ keys = require('../objects/keys'),
+ property = require('../utilities/property');
+
+/**
+ * Produces a callback bound to an optional `thisArg`. If `func` is a property
+ * name the created callback will return the property value for a given element.
+ * If `func` is an object the created callback will return `true` for elements
+ * that contain the equivalent object properties, otherwise it will return `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {*} [func=identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of the created callback.
+ * @param {number} [argCount] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ * @example
+ *
+ * var characters = [
+ * { 'name': 'barney', 'age': 36 },
+ * { 'name': 'fred', 'age': 40 }
+ * ];
+ *
+ * // wrap to create custom callback shorthands
+ * _.createCallback = _.wrap(_.createCallback, function(func, callback, thisArg) {
+ * var match = /^(.+?)__([gl]t)(.+)$/.exec(callback);
+ * return !match ? func(callback, thisArg) : function(object) {
+ * return match[2] == 'gt' ? object[match[1]] > match[3] : object[match[1]] < match[3];
+ * };
+ * });
+ *
+ * _.filter(characters, 'age__gt38');
+ * // => [{ 'name': 'fred', 'age': 40 }]
+ */
+function createCallback(func, thisArg, argCount) {
+ var type = typeof func;
+ if (func == null || type == 'function') {
+ return baseCreateCallback(func, thisArg, argCount);
+ }
+ // handle "_.pluck" style callback shorthands
+ if (type != 'object') {
+ return property(func);
+ }
+ var props = keys(func),
+ key = props[0],
+ a = func[key];
+
+ // handle "_.where" style callback shorthands
+ if (props.length == 1 && a === a && !isObject(a)) {
+ // fast path the common case of providing an object with a single
+ // property containing a primitive value
+ return function(object) {
+ var b = object[key];
+ return a === b && (a !== 0 || (1 / a == 1 / b));
+ };
+ }
+ return function(object) {
+ var length = props.length,
+ result = false;
+
+ while (length--) {
+ if (!(result = baseIsEqual(object[props[length]], func[props[length]], null, true))) {
+ break;
+ }
+ }
+ return result;
+ };
+}
+
+module.exports = createCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/curry.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/curry.js b/node_modules/lodash-node/modern/functions/curry.js
new file mode 100644
index 0000000..bf6b12d
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/curry.js
@@ -0,0 +1,44 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper');
+
+/**
+ * Creates a function which accepts one or more arguments of `func` that when
+ * invoked either executes `func` returning its result, if all `func` arguments
+ * have been provided, or returns a function that accepts one or more of the
+ * remaining `func` arguments, and so on. The arity of `func` can be specified
+ * if `func.length` is not sufficient.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to curry.
+ * @param {number} [arity=func.length] The arity of `func`.
+ * @returns {Function} Returns the new curried function.
+ * @example
+ *
+ * var curried = _.curry(function(a, b, c) {
+ * console.log(a + b + c);
+ * });
+ *
+ * curried(1)(2)(3);
+ * // => 6
+ *
+ * curried(1, 2)(3);
+ * // => 6
+ *
+ * curried(1, 2, 3);
+ * // => 6
+ */
+function curry(func, arity) {
+ arity = typeof arity == 'number' ? arity : (+arity || func.length);
+ return createWrapper(func, 4, null, null, null, arity);
+}
+
+module.exports = curry;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/debounce.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/debounce.js b/node_modules/lodash-node/modern/functions/debounce.js
new file mode 100644
index 0000000..a904202
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/debounce.js
@@ -0,0 +1,156 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject'),
+ now = require('../utilities/now');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that will delay the execution of `func` until after
+ * `wait` milliseconds have elapsed since the last time it was invoked.
+ * Provide an options object to indicate that `func` should be invoked on
+ * the leading and/or trailing edge of the `wait` timeout. Subsequent calls
+ * to the debounced function will return the result of the last `func` call.
+ *
+ * Note: If `leading` and `trailing` options are `true` `func` will be called
+ * on the trailing edge of the timeout only if the the debounced function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to debounce.
+ * @param {number} wait The number of milliseconds to delay.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=false] Specify execution on the leading edge of the timeout.
+ * @param {number} [options.maxWait] The maximum time `func` is allowed to be delayed before it's called.
+ * @param {boolean} [options.trailing=true] Specify execution on the trailing edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * // avoid costly calculations while the window size is in flux
+ * var lazyLayout = _.debounce(calculateLayout, 150);
+ * jQuery(window).on('resize', lazyLayout);
+ *
+ * // execute `sendMail` when the click event is fired, debouncing subsequent calls
+ * jQuery('#postbox').on('click', _.debounce(sendMail, 300, {
+ * 'leading': true,
+ * 'trailing': false
+ * });
+ *
+ * // ensure `batchLog` is executed once after 1 second of debounced calls
+ * var source = new EventSource('/stream');
+ * source.addEventListener('message', _.debounce(batchLog, 250, {
+ * 'maxWait': 1000
+ * }, false);
+ */
+function debounce(func, wait, options) {
+ var args,
+ maxTimeoutId,
+ result,
+ stamp,
+ thisArg,
+ timeoutId,
+ trailingCall,
+ lastCalled = 0,
+ maxWait = false,
+ trailing = true;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ wait = nativeMax(0, wait) || 0;
+ if (options === true) {
+ var leading = true;
+ trailing = false;
+ } else if (isObject(options)) {
+ leading = options.leading;
+ maxWait = 'maxWait' in options && (nativeMax(wait, options.maxWait) || 0);
+ trailing = 'trailing' in options ? options.trailing : trailing;
+ }
+ var delayed = function() {
+ var remaining = wait - (now() - stamp);
+ if (remaining <= 0) {
+ if (maxTimeoutId) {
+ clearTimeout(maxTimeoutId);
+ }
+ var isCalled = trailingCall;
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (isCalled) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ }
+ } else {
+ timeoutId = setTimeout(delayed, remaining);
+ }
+ };
+
+ var maxDelayed = function() {
+ if (timeoutId) {
+ clearTimeout(timeoutId);
+ }
+ maxTimeoutId = timeoutId = trailingCall = undefined;
+ if (trailing || (maxWait !== wait)) {
+ lastCalled = now();
+ result = func.apply(thisArg, args);
+ if (!timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ }
+ };
+
+ return function() {
+ args = arguments;
+ stamp = now();
+ thisArg = this;
+ trailingCall = trailing && (timeoutId || !leading);
+
+ if (maxWait === false) {
+ var leadingCall = leading && !timeoutId;
+ } else {
+ if (!maxTimeoutId && !leading) {
+ lastCalled = stamp;
+ }
+ var remaining = maxWait - (stamp - lastCalled),
+ isCalled = remaining <= 0;
+
+ if (isCalled) {
+ if (maxTimeoutId) {
+ maxTimeoutId = clearTimeout(maxTimeoutId);
+ }
+ lastCalled = stamp;
+ result = func.apply(thisArg, args);
+ }
+ else if (!maxTimeoutId) {
+ maxTimeoutId = setTimeout(maxDelayed, remaining);
+ }
+ }
+ if (isCalled && timeoutId) {
+ timeoutId = clearTimeout(timeoutId);
+ }
+ else if (!timeoutId && wait !== maxWait) {
+ timeoutId = setTimeout(delayed, wait);
+ }
+ if (leadingCall) {
+ isCalled = true;
+ result = func.apply(thisArg, args);
+ }
+ if (isCalled && !timeoutId && !maxTimeoutId) {
+ args = thisArg = null;
+ }
+ return result;
+ };
+}
+
+module.exports = debounce;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/defer.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/defer.js b/node_modules/lodash-node/modern/functions/defer.js
new file mode 100644
index 0000000..45271be
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/defer.js
@@ -0,0 +1,35 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ slice = require('../internals/slice');
+
+/**
+ * Defers executing the `func` function until the current call stack has cleared.
+ * Additional arguments will be provided to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to defer.
+ * @param {...*} [arg] Arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.defer(function(text) { console.log(text); }, 'deferred');
+ * // logs 'deferred' after one or more milliseconds
+ */
+function defer(func) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var args = slice(arguments, 1);
+ return setTimeout(function() { func.apply(undefined, args); }, 1);
+}
+
+module.exports = defer;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/delay.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/delay.js b/node_modules/lodash-node/modern/functions/delay.js
new file mode 100644
index 0000000..50b23af
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/delay.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ slice = require('../internals/slice');
+
+/**
+ * Executes the `func` function after `wait` milliseconds. Additional arguments
+ * will be provided to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to delay.
+ * @param {number} wait The number of milliseconds to delay execution.
+ * @param {...*} [arg] Arguments to invoke the function with.
+ * @returns {number} Returns the timer id.
+ * @example
+ *
+ * _.delay(function(text) { console.log(text); }, 1000, 'later');
+ * // => logs 'later' after one second
+ */
+function delay(func, wait) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var args = slice(arguments, 2);
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+}
+
+module.exports = delay;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/memoize.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/memoize.js b/node_modules/lodash-node/modern/functions/memoize.js
new file mode 100644
index 0000000..e02aecb
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/memoize.js
@@ -0,0 +1,71 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction'),
+ keyPrefix = require('../internals/keyPrefix');
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates a function that memoizes the result of `func`. If `resolver` is
+ * provided it will be used to determine the cache key for storing the result
+ * based on the arguments provided to the memoized function. By default, the
+ * first argument provided to the memoized function is used as the cache key.
+ * The `func` is executed with the `this` binding of the memoized function.
+ * The result cache is exposed as the `cache` property on the memoized function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to have its output memoized.
+ * @param {Function} [resolver] A function used to resolve the cache key.
+ * @returns {Function} Returns the new memoizing function.
+ * @example
+ *
+ * var fibonacci = _.memoize(function(n) {
+ * return n < 2 ? n : fibonacci(n - 1) + fibonacci(n - 2);
+ * });
+ *
+ * fibonacci(9)
+ * // => 34
+ *
+ * var data = {
+ * 'fred': { 'name': 'fred', 'age': 40 },
+ * 'pebbles': { 'name': 'pebbles', 'age': 1 }
+ * };
+ *
+ * // modifying the result cache
+ * var get = _.memoize(function(name) { return data[name]; }, _.identity);
+ * get('pebbles');
+ * // => { 'name': 'pebbles', 'age': 1 }
+ *
+ * get.cache.pebbles.name = 'penelope';
+ * get('pebbles');
+ * // => { 'name': 'penelope', 'age': 1 }
+ */
+function memoize(func, resolver) {
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ var memoized = function() {
+ var cache = memoized.cache,
+ key = resolver ? resolver.apply(this, arguments) : keyPrefix + arguments[0];
+
+ return hasOwnProperty.call(cache, key)
+ ? cache[key]
+ : (cache[key] = func.apply(this, arguments));
+ }
+ memoized.cache = {};
+ return memoized;
+}
+
+module.exports = memoize;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/once.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/once.js b/node_modules/lodash-node/modern/functions/once.js
new file mode 100644
index 0000000..8cc4d54
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/once.js
@@ -0,0 +1,48 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isFunction = require('../objects/isFunction');
+
+/**
+ * Creates a function that is restricted to execute `func` once. Repeat calls to
+ * the function will return the value of the first call. The `func` is executed
+ * with the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // `initialize` executes `createApplication` once
+ */
+function once(func) {
+ var ran,
+ result;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ return function() {
+ if (ran) {
+ return result;
+ }
+ ran = true;
+ result = func.apply(this, arguments);
+
+ // clear the `func` variable so the function may be garbage collected
+ func = null;
+ return result;
+ };
+}
+
+module.exports = once;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/partial.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/partial.js b/node_modules/lodash-node/modern/functions/partial.js
new file mode 100644
index 0000000..87854f5
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/partial.js
@@ -0,0 +1,34 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * Creates a function that, when called, invokes `func` with any additional
+ * `partial` arguments prepended to those provided to the new function. This
+ * method is similar to `_.bind` except it does **not** alter the `this` binding.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var greet = function(greeting, name) { return greeting + ' ' + name; };
+ * var hi = _.partial(greet, 'hi');
+ * hi('fred');
+ * // => 'hi fred'
+ */
+function partial(func) {
+ return createWrapper(func, 16, slice(arguments, 1));
+}
+
+module.exports = partial;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/partialRight.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/partialRight.js b/node_modules/lodash-node/modern/functions/partialRight.js
new file mode 100644
index 0000000..3958f6b
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/partialRight.js
@@ -0,0 +1,43 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper'),
+ slice = require('../internals/slice');
+
+/**
+ * This method is like `_.partial` except that `partial` arguments are
+ * appended to those provided to the new function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {...*} [arg] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var defaultsDeep = _.partialRight(_.merge, _.defaults);
+ *
+ * var options = {
+ * 'variable': 'data',
+ * 'imports': { 'jq': $ }
+ * };
+ *
+ * defaultsDeep(options, _.templateSettings);
+ *
+ * options.variable
+ * // => 'data'
+ *
+ * options.imports
+ * // => { '_': _, 'jq': $ }
+ */
+function partialRight(func) {
+ return createWrapper(func, 32, null, slice(arguments, 1));
+}
+
+module.exports = partialRight;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/throttle.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/throttle.js b/node_modules/lodash-node/modern/functions/throttle.js
new file mode 100644
index 0000000..5b5daf5
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/throttle.js
@@ -0,0 +1,71 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var debounce = require('./debounce'),
+ isFunction = require('../objects/isFunction'),
+ isObject = require('../objects/isObject');
+
+/** Used as an internal `_.debounce` options object */
+var debounceOptions = {
+ 'leading': false,
+ 'maxWait': 0,
+ 'trailing': false
+};
+
+/**
+ * Creates a function that, when executed, will only call the `func` function
+ * at most once per every `wait` milliseconds. Provide an options object to
+ * indicate that `func` should be invoked on the leading and/or trailing edge
+ * of the `wait` timeout. Subsequent calls to the throttled function will
+ * return the result of the last `func` call.
+ *
+ * Note: If `leading` and `trailing` options are `true` `func` will be called
+ * on the trailing edge of the timeout only if the the throttled function is
+ * invoked more than once during the `wait` timeout.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to throttle.
+ * @param {number} wait The number of milliseconds to throttle executions to.
+ * @param {Object} [options] The options object.
+ * @param {boolean} [options.leading=true] Specify execution on the leading edge of the timeout.
+ * @param {boolean} [options.trailing=true] Specify execution on the trailing edge of the timeout.
+ * @returns {Function} Returns the new throttled function.
+ * @example
+ *
+ * // avoid excessively updating the position while scrolling
+ * var throttled = _.throttle(updatePosition, 100);
+ * jQuery(window).on('scroll', throttled);
+ *
+ * // execute `renewToken` when the click event is fired, but not more than once every 5 minutes
+ * jQuery('.interactive').on('click', _.throttle(renewToken, 300000, {
+ * 'trailing': false
+ * }));
+ */
+function throttle(func, wait, options) {
+ var leading = true,
+ trailing = true;
+
+ if (!isFunction(func)) {
+ throw new TypeError;
+ }
+ if (options === false) {
+ leading = false;
+ } else if (isObject(options)) {
+ leading = 'leading' in options ? options.leading : leading;
+ trailing = 'trailing' in options ? options.trailing : trailing;
+ }
+ debounceOptions.leading = leading;
+ debounceOptions.maxWait = wait;
+ debounceOptions.trailing = trailing;
+
+ return debounce(func, wait, debounceOptions);
+}
+
+module.exports = throttle;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/functions/wrap.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/functions/wrap.js b/node_modules/lodash-node/modern/functions/wrap.js
new file mode 100644
index 0000000..e547b1d
--- /dev/null
+++ b/node_modules/lodash-node/modern/functions/wrap.js
@@ -0,0 +1,36 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createWrapper = require('../internals/createWrapper');
+
+/**
+ * Creates a function that provides `value` to the wrapper function as its
+ * first argument. Additional arguments provided to the function are appended
+ * to those provided to the wrapper function. The wrapper is executed with
+ * the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {*} value The value to wrap.
+ * @param {Function} wrapper The wrapper function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var p = _.wrap(_.escape, function(func, text) {
+ * return '<p>' + func(text) + '</p>';
+ * });
+ *
+ * p('Fred, Wilma, & Pebbles');
+ * // => '<p>Fred, Wilma, & Pebbles</p>'
+ */
+function wrap(value, wrapper) {
+ return createWrapper(wrapper, 16, [value]);
+}
+
+module.exports = wrap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/index.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/index.js b/node_modules/lodash-node/modern/index.js
new file mode 100644
index 0000000..e98868e
--- /dev/null
+++ b/node_modules/lodash-node/modern/index.js
@@ -0,0 +1,354 @@
+/**
+ * @license
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var arrays = require('./arrays'),
+ chaining = require('./chaining'),
+ collections = require('./collections'),
+ functions = require('./functions'),
+ objects = require('./objects'),
+ utilities = require('./utilities'),
+ forEach = require('./collections/forEach'),
+ forOwn = require('./objects/forOwn'),
+ isArray = require('./objects/isArray'),
+ lodashWrapper = require('./internals/lodashWrapper'),
+ mixin = require('./utilities/mixin'),
+ support = require('./support'),
+ templateSettings = require('./utilities/templateSettings');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Creates a `lodash` object which wraps the given value to enable intuitive
+ * method chaining.
+ *
+ * In addition to Lo-Dash methods, wrappers also have the following `Array` methods:
+ * `concat`, `join`, `pop`, `push`, `reverse`, `shift`, `slice`, `sort`, `splice`,
+ * and `unshift`
+ *
+ * Chaining is supported in custom builds as long as the `value` method is
+ * implicitly or explicitly included in the build.
+ *
+ * The chainable wrapper functions are:
+ * `after`, `assign`, `bind`, `bindAll`, `bindKey`, `chain`, `compact`,
+ * `compose`, `concat`, `countBy`, `create`, `createCallback`, `curry`,
+ * `debounce`, `defaults`, `defer`, `delay`, `difference`, `filter`, `flatten`,
+ * `forEach`, `forEachRight`, `forIn`, `forInRight`, `forOwn`, `forOwnRight`,
+ * `functions`, `groupBy`, `indexBy`, `initial`, `intersection`, `invert`,
+ * `invoke`, `keys`, `map`, `max`, `memoize`, `merge`, `min`, `object`, `omit`,
+ * `once`, `pairs`, `partial`, `partialRight`, `pick`, `pluck`, `pull`, `push`,
+ * `range`, `reject`, `remove`, `rest`, `reverse`, `shuffle`, `slice`, `sort`,
+ * `sortBy`, `splice`, `tap`, `throttle`, `times`, `toArray`, `transform`,
+ * `union`, `uniq`, `unshift`, `unzip`, `values`, `where`, `without`, `wrap`,
+ * and `zip`
+ *
+ * The non-chainable wrapper functions are:
+ * `clone`, `cloneDeep`, `contains`, `escape`, `every`, `find`, `findIndex`,
+ * `findKey`, `findLast`, `findLastIndex`, `findLastKey`, `has`, `identity`,
+ * `indexOf`, `isArguments`, `isArray`, `isBoolean`, `isDate`, `isElement`,
+ * `isEmpty`, `isEqual`, `isFinite`, `isFunction`, `isNaN`, `isNull`, `isNumber`,
+ * `isObject`, `isPlainObject`, `isRegExp`, `isString`, `isUndefined`, `join`,
+ * `lastIndexOf`, `mixin`, `noConflict`, `parseInt`, `pop`, `random`, `reduce`,
+ * `reduceRight`, `result`, `shift`, `size`, `some`, `sortedIndex`, `runInContext`,
+ * `template`, `unescape`, `uniqueId`, and `value`
+ *
+ * The wrapper functions `first` and `last` return wrapped values when `n` is
+ * provided, otherwise they return unwrapped values.
+ *
+ * Explicit chaining can be enabled by using the `_.chain` method.
+ *
+ * @name _
+ * @constructor
+ * @category Chaining
+ * @param {*} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns a `lodash` instance.
+ * @example
+ *
+ * var wrapped = _([1, 2, 3]);
+ *
+ * // returns an unwrapped value
+ * wrapped.reduce(function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * // returns a wrapped value
+ * var squares = wrapped.map(function(num) {
+ * return num * num;
+ * });
+ *
+ * _.isArray(squares);
+ * // => false
+ *
+ * _.isArray(squares.value());
+ * // => true
+ */
+function lodash(value) {
+ // don't wrap if already wrapped, even if wrapped by a different `lodash` constructor
+ return (value && typeof value == 'object' && !isArray(value) && hasOwnProperty.call(value, '__wrapped__'))
+ ? value
+ : new lodashWrapper(value);
+}
+// ensure `new lodashWrapper` is an instance of `lodash`
+lodashWrapper.prototype = lodash.prototype;
+
+// wrap `_.mixin` so it works when provided only one argument
+mixin = (function(fn) {
+ var functions = objects.functions;
+ return function(object, source, options) {
+ if (!source || (!options && !functions(source).length)) {
+ if (options == null) {
+ options = source;
+ }
+ source = object;
+ object = lodash;
+ }
+ return fn(object, source, options);
+ };
+}(mixin));
+
+// add functions that return wrapped values when chaining
+lodash.after = functions.after;
+lodash.assign = objects.assign;
+lodash.at = collections.at;
+lodash.bind = functions.bind;
+lodash.bindAll = functions.bindAll;
+lodash.bindKey = functions.bindKey;
+lodash.chain = chaining.chain;
+lodash.compact = arrays.compact;
+lodash.compose = functions.compose;
+lodash.constant = utilities.constant;
+lodash.countBy = collections.countBy;
+lodash.create = objects.create;
+lodash.createCallback = functions.createCallback;
+lodash.curry = functions.curry;
+lodash.debounce = functions.debounce;
+lodash.defaults = objects.defaults;
+lodash.defer = functions.defer;
+lodash.delay = functions.delay;
+lodash.difference = arrays.difference;
+lodash.filter = collections.filter;
+lodash.flatten = arrays.flatten;
+lodash.forEach = forEach;
+lodash.forEachRight = collections.forEachRight;
+lodash.forIn = objects.forIn;
+lodash.forInRight = objects.forInRight;
+lodash.forOwn = forOwn;
+lodash.forOwnRight = objects.forOwnRight;
+lodash.functions = objects.functions;
+lodash.groupBy = collections.groupBy;
+lodash.indexBy = collections.indexBy;
+lodash.initial = arrays.initial;
+lodash.intersection = arrays.intersection;
+lodash.invert = objects.invert;
+lodash.invoke = collections.invoke;
+lodash.keys = objects.keys;
+lodash.map = collections.map;
+lodash.mapValues = objects.mapValues;
+lodash.max = collections.max;
+lodash.memoize = functions.memoize;
+lodash.merge = objects.merge;
+lodash.min = collections.min;
+lodash.omit = objects.omit;
+lodash.once = functions.once;
+lodash.pairs = objects.pairs;
+lodash.partial = functions.partial;
+lodash.partialRight = functions.partialRight;
+lodash.pick = objects.pick;
+lodash.pluck = collections.pluck;
+lodash.property = utilities.property;
+lodash.pull = arrays.pull;
+lodash.range = arrays.range;
+lodash.reject = collections.reject;
+lodash.remove = arrays.remove;
+lodash.rest = arrays.rest;
+lodash.shuffle = collections.shuffle;
+lodash.sortBy = collections.sortBy;
+lodash.tap = chaining.tap;
+lodash.throttle = functions.throttle;
+lodash.times = utilities.times;
+lodash.toArray = collections.toArray;
+lodash.transform = objects.transform;
+lodash.union = arrays.union;
+lodash.uniq = arrays.uniq;
+lodash.values = objects.values;
+lodash.where = collections.where;
+lodash.without = arrays.without;
+lodash.wrap = functions.wrap;
+lodash.xor = arrays.xor;
+lodash.zip = arrays.zip;
+lodash.zipObject = arrays.zipObject;
+
+// add aliases
+lodash.collect = collections.map;
+lodash.drop = arrays.rest;
+lodash.each = forEach;
+lodash.eachRight = collections.forEachRight;
+lodash.extend = objects.assign;
+lodash.methods = objects.functions;
+lodash.object = arrays.zipObject;
+lodash.select = collections.filter;
+lodash.tail = arrays.rest;
+lodash.unique = arrays.uniq;
+lodash.unzip = arrays.zip;
+
+// add functions to `lodash.prototype`
+mixin(lodash);
+
+// add functions that return unwrapped values when chaining
+lodash.clone = objects.clone;
+lodash.cloneDeep = objects.cloneDeep;
+lodash.contains = collections.contains;
+lodash.escape = utilities.escape;
+lodash.every = collections.every;
+lodash.find = collections.find;
+lodash.findIndex = arrays.findIndex;
+lodash.findKey = objects.findKey;
+lodash.findLast = collections.findLast;
+lodash.findLastIndex = arrays.findLastIndex;
+lodash.findLastKey = objects.findLastKey;
+lodash.has = objects.has;
+lodash.identity = utilities.identity;
+lodash.indexOf = arrays.indexOf;
+lodash.isArguments = objects.isArguments;
+lodash.isArray = isArray;
+lodash.isBoolean = objects.isBoolean;
+lodash.isDate = objects.isDate;
+lodash.isElement = objects.isElement;
+lodash.isEmpty = objects.isEmpty;
+lodash.isEqual = objects.isEqual;
+lodash.isFinite = objects.isFinite;
+lodash.isFunction = objects.isFunction;
+lodash.isNaN = objects.isNaN;
+lodash.isNull = objects.isNull;
+lodash.isNumber = objects.isNumber;
+lodash.isObject = objects.isObject;
+lodash.isPlainObject = objects.isPlainObject;
+lodash.isRegExp = objects.isRegExp;
+lodash.isString = objects.isString;
+lodash.isUndefined = objects.isUndefined;
+lodash.lastIndexOf = arrays.lastIndexOf;
+lodash.mixin = mixin;
+lodash.noConflict = utilities.noConflict;
+lodash.noop = utilities.noop;
+lodash.now = utilities.now;
+lodash.parseInt = utilities.parseInt;
+lodash.random = utilities.random;
+lodash.reduce = collections.reduce;
+lodash.reduceRight = collections.reduceRight;
+lodash.result = utilities.result;
+lodash.size = collections.size;
+lodash.some = collections.some;
+lodash.sortedIndex = arrays.sortedIndex;
+lodash.template = utilities.template;
+lodash.unescape = utilities.unescape;
+lodash.uniqueId = utilities.uniqueId;
+
+// add aliases
+lodash.all = collections.every;
+lodash.any = collections.some;
+lodash.detect = collections.find;
+lodash.findWhere = collections.find;
+lodash.foldl = collections.reduce;
+lodash.foldr = collections.reduceRight;
+lodash.include = collections.contains;
+lodash.inject = collections.reduce;
+
+mixin(function() {
+ var source = {}
+ forOwn(lodash, function(func, methodName) {
+ if (!lodash.prototype[methodName]) {
+ source[methodName] = func;
+ }
+ });
+ return source;
+}(), false);
+
+// add functions capable of returning wrapped and unwrapped values when chaining
+lodash.first = arrays.first;
+lodash.last = arrays.last;
+lodash.sample = collections.sample;
+
+// add aliases
+lodash.take = arrays.first;
+lodash.head = arrays.first;
+
+forOwn(lodash, function(func, methodName) {
+ var callbackable = methodName !== 'sample';
+ if (!lodash.prototype[methodName]) {
+ lodash.prototype[methodName]= function(n, guard) {
+ var chainAll = this.__chain__,
+ result = func(this.__wrapped__, n, guard);
+
+ return !chainAll && (n == null || (guard && !(callbackable && typeof n == 'function')))
+ ? result
+ : new lodashWrapper(result, chainAll);
+ };
+ }
+});
+
+/**
+ * The semantic version number.
+ *
+ * @static
+ * @memberOf _
+ * @type string
+ */
+lodash.VERSION = '2.4.1';
+
+// add "Chaining" functions to the wrapper
+lodash.prototype.chain = chaining.wrapperChain;
+lodash.prototype.toString = chaining.wrapperToString;
+lodash.prototype.value = chaining.wrapperValueOf;
+lodash.prototype.valueOf = chaining.wrapperValueOf;
+
+// add `Array` functions that return unwrapped values
+forEach(['join', 'pop', 'shift'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ var chainAll = this.__chain__,
+ result = func.apply(this.__wrapped__, arguments);
+
+ return chainAll
+ ? new lodashWrapper(result, chainAll)
+ : result;
+ };
+});
+
+// add `Array` functions that return the existing wrapped value
+forEach(['push', 'reverse', 'sort', 'unshift'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ func.apply(this.__wrapped__, arguments);
+ return this;
+ };
+});
+
+// add `Array` functions that return new wrapped values
+forEach(['concat', 'slice', 'splice'], function(methodName) {
+ var func = arrayRef[methodName];
+ lodash.prototype[methodName] = function() {
+ return new lodashWrapper(func.apply(this.__wrapped__, arguments), this.__chain__);
+ };
+});
+
+lodash.support = support;
+(lodash.templateSettings = utilities.templateSettings).imports._ = lodash;
+module.exports = lodash;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/arrayPool.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/arrayPool.js b/node_modules/lodash-node/modern/internals/arrayPool.js
new file mode 100644
index 0000000..bc41f7f
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/arrayPool.js
@@ -0,0 +1,13 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Used to pool arrays and objects used internally */
+var arrayPool = [];
+
+module.exports = arrayPool;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseBind.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseBind.js b/node_modules/lodash-node/modern/internals/baseBind.js
new file mode 100644
index 0000000..ecf04c5
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseBind.js
@@ -0,0 +1,62 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('./baseCreate'),
+ isObject = require('../objects/isObject'),
+ setBindData = require('./setBindData'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * The base implementation of `_.bind` that creates the bound function and
+ * sets its meta data.
+ *
+ * @private
+ * @param {Array} bindData The bind data array.
+ * @returns {Function} Returns the new bound function.
+ */
+function baseBind(bindData) {
+ var func = bindData[0],
+ partialArgs = bindData[2],
+ thisArg = bindData[4];
+
+ function bound() {
+ // `Function#bind` spec
+ // http://es5.github.io/#x15.3.4.5
+ if (partialArgs) {
+ // avoid `arguments` object deoptimizations by using `slice` instead
+ // of `Array.prototype.slice.call` and not assigning `arguments` to a
+ // variable as a ternary expression
+ var args = slice(partialArgs);
+ push.apply(args, arguments);
+ }
+ // mimic the constructor's `return` behavior
+ // http://es5.github.io/#x13.2.2
+ if (this instanceof bound) {
+ // ensure `new bound` is an instance of `func`
+ var thisBinding = baseCreate(func.prototype),
+ result = func.apply(thisBinding, args || arguments);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisArg, args || arguments);
+ }
+ setBindData(bound, bindData);
+ return bound;
+}
+
+module.exports = baseBind;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseClone.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseClone.js b/node_modules/lodash-node/modern/internals/baseClone.js
new file mode 100644
index 0000000..13c8354
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseClone.js
@@ -0,0 +1,152 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var assign = require('../objects/assign'),
+ forEach = require('../collections/forEach'),
+ forOwn = require('../objects/forOwn'),
+ getArray = require('./getArray'),
+ isArray = require('../objects/isArray'),
+ isObject = require('../objects/isObject'),
+ releaseArray = require('./releaseArray'),
+ slice = require('./slice');
+
+/** Used to match regexp flags from their coerced string values */
+var reFlags = /\w*$/;
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ funcClass = '[object Function]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+/** Used to identify object classifications that `_.clone` supports */
+var cloneableClasses = {};
+cloneableClasses[funcClass] = false;
+cloneableClasses[argsClass] = cloneableClasses[arrayClass] =
+cloneableClasses[boolClass] = cloneableClasses[dateClass] =
+cloneableClasses[numberClass] = cloneableClasses[objectClass] =
+cloneableClasses[regexpClass] = cloneableClasses[stringClass] = true;
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to lookup a built-in constructor by [[Class]] */
+var ctorByClass = {};
+ctorByClass[arrayClass] = Array;
+ctorByClass[boolClass] = Boolean;
+ctorByClass[dateClass] = Date;
+ctorByClass[funcClass] = Function;
+ctorByClass[objectClass] = Object;
+ctorByClass[numberClass] = Number;
+ctorByClass[regexpClass] = RegExp;
+ctorByClass[stringClass] = String;
+
+/**
+ * The base implementation of `_.clone` without argument juggling or support
+ * for `thisArg` binding.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep=false] Specify a deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates clones with source counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+function baseClone(value, isDeep, callback, stackA, stackB) {
+ if (callback) {
+ var result = callback(value);
+ if (typeof result != 'undefined') {
+ return result;
+ }
+ }
+ // inspect [[Class]]
+ var isObj = isObject(value);
+ if (isObj) {
+ var className = toString.call(value);
+ if (!cloneableClasses[className]) {
+ return value;
+ }
+ var ctor = ctorByClass[className];
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ return new ctor(+value);
+
+ case numberClass:
+ case stringClass:
+ return new ctor(value);
+
+ case regexpClass:
+ result = ctor(value.source, reFlags.exec(value));
+ result.lastIndex = value.lastIndex;
+ return result;
+ }
+ } else {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isDeep) {
+ // check for circular references and return corresponding clone
+ var initedStack = !stackA;
+ stackA || (stackA = getArray());
+ stackB || (stackB = getArray());
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == value) {
+ return stackB[length];
+ }
+ }
+ result = isArr ? ctor(value.length) : {};
+ }
+ else {
+ result = isArr ? slice(value) : assign({}, value);
+ }
+ // add array properties assigned by `RegExp#exec`
+ if (isArr) {
+ if (hasOwnProperty.call(value, 'index')) {
+ result.index = value.index;
+ }
+ if (hasOwnProperty.call(value, 'input')) {
+ result.input = value.input;
+ }
+ }
+ // exit for shallow clone
+ if (!isDeep) {
+ return result;
+ }
+ // add the source value to the stack of traversed objects
+ // and associate it with its clone
+ stackA.push(value);
+ stackB.push(result);
+
+ // recursively populate clone (susceptible to call stack limits)
+ (isArr ? forEach : forOwn)(value, function(objValue, key) {
+ result[key] = baseClone(objValue, isDeep, callback, stackA, stackB);
+ });
+
+ if (initedStack) {
+ releaseArray(stackA);
+ releaseArray(stackB);
+ }
+ return result;
+}
+
+module.exports = baseClone;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseCreate.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseCreate.js b/node_modules/lodash-node/modern/internals/baseCreate.js
new file mode 100644
index 0000000..32d80e5
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseCreate.js
@@ -0,0 +1,42 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isNative = require('./isNative'),
+ isObject = require('../objects/isObject'),
+ noop = require('../utilities/noop');
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeCreate = isNative(nativeCreate = Object.create) && nativeCreate;
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+function baseCreate(prototype, properties) {
+ return isObject(prototype) ? nativeCreate(prototype) : {};
+}
+// fallback for browsers without `Object.create`
+if (!nativeCreate) {
+ baseCreate = (function() {
+ function Object() {}
+ return function(prototype) {
+ if (isObject(prototype)) {
+ Object.prototype = prototype;
+ var result = new Object;
+ Object.prototype = null;
+ }
+ return result || global.Object();
+ };
+ }());
+}
+
+module.exports = baseCreate;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseCreateCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseCreateCallback.js b/node_modules/lodash-node/modern/internals/baseCreateCallback.js
new file mode 100644
index 0000000..7f90303
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseCreateCallback.js
@@ -0,0 +1,80 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var bind = require('../functions/bind'),
+ identity = require('../utilities/identity'),
+ setBindData = require('./setBindData'),
+ support = require('../support');
+
+/** Used to detected named functions */
+var reFuncName = /^\s*function[ \n\r\t]+\w/;
+
+/** Used to detect functions containing a `this` reference */
+var reThis = /\bthis\b/;
+
+/** Native method shortcuts */
+var fnToString = Function.prototype.toString;
+
+/**
+ * The base implementation of `_.createCallback` without support for creating
+ * "_.pluck" or "_.where" style callbacks.
+ *
+ * @private
+ * @param {*} [func=identity] The value to convert to a callback.
+ * @param {*} [thisArg] The `this` binding of the created callback.
+ * @param {number} [argCount] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ */
+function baseCreateCallback(func, thisArg, argCount) {
+ if (typeof func != 'function') {
+ return identity;
+ }
+ // exit early for no `thisArg` or already bound by `Function#bind`
+ if (typeof thisArg == 'undefined' || !('prototype' in func)) {
+ return func;
+ }
+ var bindData = func.__bindData__;
+ if (typeof bindData == 'undefined') {
+ if (support.funcNames) {
+ bindData = !func.name;
+ }
+ bindData = bindData || !support.funcDecomp;
+ if (!bindData) {
+ var source = fnToString.call(func);
+ if (!support.funcNames) {
+ bindData = !reFuncName.test(source);
+ }
+ if (!bindData) {
+ // checks if `func` references the `this` keyword and stores the result
+ bindData = reThis.test(source);
+ setBindData(func, bindData);
+ }
+ }
+ }
+ // exit early if there are no `this` references or `func` is bound
+ if (bindData === false || (bindData !== true && bindData[1] & 1)) {
+ return func;
+ }
+ switch (argCount) {
+ case 1: return function(value) {
+ return func.call(thisArg, value);
+ };
+ case 2: return function(a, b) {
+ return func.call(thisArg, a, b);
+ };
+ case 3: return function(value, index, collection) {
+ return func.call(thisArg, value, index, collection);
+ };
+ case 4: return function(accumulator, value, index, collection) {
+ return func.call(thisArg, accumulator, value, index, collection);
+ };
+ }
+ return bind(func, thisArg);
+}
+
+module.exports = baseCreateCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseCreateWrapper.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseCreateWrapper.js b/node_modules/lodash-node/modern/internals/baseCreateWrapper.js
new file mode 100644
index 0000000..3ce5a77
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseCreateWrapper.js
@@ -0,0 +1,78 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseCreate = require('./baseCreate'),
+ isObject = require('../objects/isObject'),
+ setBindData = require('./setBindData'),
+ slice = require('./slice');
+
+/**
+ * Used for `Array` method references.
+ *
+ * Normally `Array.prototype` would suffice, however, using an array literal
+ * avoids issues in Narwhal.
+ */
+var arrayRef = [];
+
+/** Native method shortcuts */
+var push = arrayRef.push;
+
+/**
+ * The base implementation of `createWrapper` that creates the wrapper and
+ * sets its meta data.
+ *
+ * @private
+ * @param {Array} bindData The bind data array.
+ * @returns {Function} Returns the new function.
+ */
+function baseCreateWrapper(bindData) {
+ var func = bindData[0],
+ bitmask = bindData[1],
+ partialArgs = bindData[2],
+ partialRightArgs = bindData[3],
+ thisArg = bindData[4],
+ arity = bindData[5];
+
+ var isBind = bitmask & 1,
+ isBindKey = bitmask & 2,
+ isCurry = bitmask & 4,
+ isCurryBound = bitmask & 8,
+ key = func;
+
+ function bound() {
+ var thisBinding = isBind ? thisArg : this;
+ if (partialArgs) {
+ var args = slice(partialArgs);
+ push.apply(args, arguments);
+ }
+ if (partialRightArgs || isCurry) {
+ args || (args = slice(arguments));
+ if (partialRightArgs) {
+ push.apply(args, partialRightArgs);
+ }
+ if (isCurry && args.length < arity) {
+ bitmask |= 16 & ~32;
+ return baseCreateWrapper([func, (isCurryBound ? bitmask : bitmask & ~3), args, null, thisArg, arity]);
+ }
+ }
+ args || (args = arguments);
+ if (isBindKey) {
+ func = thisBinding[key];
+ }
+ if (this instanceof bound) {
+ thisBinding = baseCreate(func.prototype);
+ var result = func.apply(thisBinding, args);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisBinding, args);
+ }
+ setBindData(bound, bindData);
+ return bound;
+}
+
+module.exports = baseCreateWrapper;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseDifference.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseDifference.js b/node_modules/lodash-node/modern/internals/baseDifference.js
new file mode 100644
index 0000000..6cd38a5
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseDifference.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf'),
+ cacheIndexOf = require('./cacheIndexOf'),
+ createCache = require('./createCache'),
+ largeArraySize = require('./largeArraySize'),
+ releaseObject = require('./releaseObject');
+
+/**
+ * The base implementation of `_.difference` that accepts a single array
+ * of values to exclude.
+ *
+ * @private
+ * @param {Array} array The array to process.
+ * @param {Array} [values] The array of values to exclude.
+ * @returns {Array} Returns a new array of filtered values.
+ */
+function baseDifference(array, values) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ isLarge = length >= largeArraySize,
+ result = [];
+
+ if (isLarge) {
+ var cache = createCache(values);
+ if (cache) {
+ indexOf = cacheIndexOf;
+ values = cache;
+ } else {
+ isLarge = false;
+ }
+ }
+ while (++index < length) {
+ var value = array[index];
+ if (indexOf(values, value) < 0) {
+ result.push(value);
+ }
+ }
+ if (isLarge) {
+ releaseObject(values);
+ }
+ return result;
+}
+
+module.exports = baseDifference;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseFlatten.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseFlatten.js b/node_modules/lodash-node/modern/internals/baseFlatten.js
new file mode 100644
index 0000000..5da8ba0
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseFlatten.js
@@ -0,0 +1,52 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var isArguments = require('../objects/isArguments'),
+ isArray = require('../objects/isArray');
+
+/**
+ * The base implementation of `_.flatten` without support for callback
+ * shorthands or `thisArg` binding.
+ *
+ * @private
+ * @param {Array} array The array to flatten.
+ * @param {boolean} [isShallow=false] A flag to restrict flattening to a single level.
+ * @param {boolean} [isStrict=false] A flag to restrict flattening to arrays and `arguments` objects.
+ * @param {number} [fromIndex=0] The index to start from.
+ * @returns {Array} Returns a new flattened array.
+ */
+function baseFlatten(array, isShallow, isStrict, fromIndex) {
+ var index = (fromIndex || 0) - 1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+
+ if (value && typeof value == 'object' && typeof value.length == 'number'
+ && (isArray(value) || isArguments(value))) {
+ // recursively flatten arrays (susceptible to call stack limits)
+ if (!isShallow) {
+ value = baseFlatten(value, isShallow, isStrict);
+ }
+ var valIndex = -1,
+ valLength = value.length,
+ resIndex = result.length;
+
+ result.length += valLength;
+ while (++valIndex < valLength) {
+ result[resIndex++] = value[valIndex];
+ }
+ } else if (!isStrict) {
+ result.push(value);
+ }
+ }
+ return result;
+}
+
+module.exports = baseFlatten;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseIndexOf.js b/node_modules/lodash-node/modern/internals/baseIndexOf.js
new file mode 100644
index 0000000..f1f7fef
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseIndexOf.js
@@ -0,0 +1,32 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * The base implementation of `_.indexOf` without support for binary searches
+ * or `fromIndex` constraints.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {*} value The value to search for.
+ * @param {number} [fromIndex=0] The index to search from.
+ * @returns {number} Returns the index of the matched value or `-1`.
+ */
+function baseIndexOf(array, value, fromIndex) {
+ var index = (fromIndex || 0) - 1,
+ length = array ? array.length : 0;
+
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+}
+
+module.exports = baseIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseIsEqual.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseIsEqual.js b/node_modules/lodash-node/modern/internals/baseIsEqual.js
new file mode 100644
index 0000000..19504c7
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseIsEqual.js
@@ -0,0 +1,209 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forIn = require('../objects/forIn'),
+ getArray = require('./getArray'),
+ isFunction = require('../objects/isFunction'),
+ objectTypes = require('./objectTypes'),
+ releaseArray = require('./releaseArray');
+
+/** `Object#toString` result shortcuts */
+var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+/** Used for native method references */
+var objectProto = Object.prototype;
+
+/** Used to resolve the internal [[Class]] of values */
+var toString = objectProto.toString;
+
+/** Native method shortcuts */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * The base implementation of `_.isEqual`, without support for `thisArg` binding,
+ * that allows partial "_.where" style comparisons.
+ *
+ * @private
+ * @param {*} a The value to compare.
+ * @param {*} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {Function} [isWhere=false] A flag to indicate performing partial comparisons.
+ * @param {Array} [stackA=[]] Tracks traversed `a` objects.
+ * @param {Array} [stackB=[]] Tracks traversed `b` objects.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ */
+function baseIsEqual(a, b, callback, isWhere, stackA, stackB) {
+ // used to indicate that when comparing objects, `a` has at least the properties of `b`
+ if (callback) {
+ var result = callback(a, b);
+ if (typeof result != 'undefined') {
+ return !!result;
+ }
+ }
+ // exit early for identical values
+ if (a === b) {
+ // treat `+0` vs. `-0` as not equal
+ return a !== 0 || (1 / a == 1 / b);
+ }
+ var type = typeof a,
+ otherType = typeof b;
+
+ // exit early for unlike primitive values
+ if (a === a &&
+ !(a && objectTypes[type]) &&
+ !(b && objectTypes[otherType])) {
+ return false;
+ }
+ // exit early for `null` and `undefined` avoiding ES3's Function#call behavior
+ // http://es5.github.io/#x15.3.4.4
+ if (a == null || b == null) {
+ return a === b;
+ }
+ // compare [[Class]] names
+ var className = toString.call(a),
+ otherClass = toString.call(b);
+
+ if (className == argsClass) {
+ className = objectClass;
+ }
+ if (otherClass == argsClass) {
+ otherClass = objectClass;
+ }
+ if (className != otherClass) {
+ return false;
+ }
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ // coerce dates and booleans to numbers, dates to milliseconds and booleans
+ // to `1` or `0` treating invalid dates coerced to `NaN` as not equal
+ return +a == +b;
+
+ case numberClass:
+ // treat `NaN` vs. `NaN` as equal
+ return (a != +a)
+ ? b != +b
+ // but treat `+0` vs. `-0` as not equal
+ : (a == 0 ? (1 / a == 1 / b) : a == +b);
+
+ case regexpClass:
+ case stringClass:
+ // coerce regexes to strings (http://es5.github.io/#x15.10.6.4)
+ // treat string primitives and their corresponding object instances as equal
+ return a == String(b);
+ }
+ var isArr = className == arrayClass;
+ if (!isArr) {
+ // unwrap any `lodash` wrapped values
+ var aWrapped = hasOwnProperty.call(a, '__wrapped__'),
+ bWrapped = hasOwnProperty.call(b, '__wrapped__');
+
+ if (aWrapped || bWrapped) {
+ return baseIsEqual(aWrapped ? a.__wrapped__ : a, bWrapped ? b.__wrapped__ : b, callback, isWhere, stackA, stackB);
+ }
+ // exit for functions and DOM nodes
+ if (className != objectClass) {
+ return false;
+ }
+ // in older versions of Opera, `arguments` objects have `Array` constructors
+ var ctorA = a.constructor,
+ ctorB = b.constructor;
+
+ // non `Object` object instances with different constructors are not equal
+ if (ctorA != ctorB &&
+ !(isFunction(ctorA) && ctorA instanceof ctorA && isFunction(ctorB) && ctorB instanceof ctorB) &&
+ ('constructor' in a && 'constructor' in b)
+ ) {
+ return false;
+ }
+ }
+ // assume cyclic structures are equal
+ // the algorithm for detecting cyclic structures is adapted from ES 5.1
+ // section 15.12.3, abstract operation `JO` (http://es5.github.io/#x15.12.3)
+ var initedStack = !stackA;
+ stackA || (stackA = getArray());
+ stackB || (stackB = getArray());
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == a) {
+ return stackB[length] == b;
+ }
+ }
+ var size = 0;
+ result = true;
+
+ // add `a` and `b` to the stack of traversed objects
+ stackA.push(a);
+ stackB.push(b);
+
+ // recursively compare objects and arrays (susceptible to call stack limits)
+ if (isArr) {
+ // compare lengths to determine if a deep comparison is necessary
+ length = a.length;
+ size = b.length;
+ result = size == length;
+
+ if (result || isWhere) {
+ // deep compare the contents, ignoring non-numeric properties
+ while (size--) {
+ var index = length,
+ value = b[size];
+
+ if (isWhere) {
+ while (index--) {
+ if ((result = baseIsEqual(a[index], value, callback, isWhere, stackA, stackB))) {
+ break;
+ }
+ }
+ } else if (!(result = baseIsEqual(a[size], value, callback, isWhere, stackA, stackB))) {
+ break;
+ }
+ }
+ }
+ }
+ else {
+ // deep compare objects using `forIn`, instead of `forOwn`, to avoid `Object.keys`
+ // which, in this case, is more costly
+ forIn(b, function(value, key, b) {
+ if (hasOwnProperty.call(b, key)) {
+ // count the number of properties.
+ size++;
+ // deep compare each property value.
+ return (result = hasOwnProperty.call(a, key) && baseIsEqual(a[key], value, callback, isWhere, stackA, stackB));
+ }
+ });
+
+ if (result && !isWhere) {
+ // ensure both objects have the same number of properties
+ forIn(a, function(value, key, a) {
+ if (hasOwnProperty.call(a, key)) {
+ // `size` will be `-1` if `a` has more properties than `b`
+ return (result = --size > -1);
+ }
+ });
+ }
+ }
+ stackA.pop();
+ stackB.pop();
+
+ if (initedStack) {
+ releaseArray(stackA);
+ releaseArray(stackB);
+ }
+ return result;
+}
+
+module.exports = baseIsEqual;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseMerge.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseMerge.js b/node_modules/lodash-node/modern/internals/baseMerge.js
new file mode 100644
index 0000000..ae13bad
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseMerge.js
@@ -0,0 +1,79 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var forEach = require('../collections/forEach'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray'),
+ isPlainObject = require('../objects/isPlainObject');
+
+/**
+ * The base implementation of `_.merge` without argument juggling or support
+ * for `thisArg` binding.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} [callback] The function to customize merging properties.
+ * @param {Array} [stackA=[]] Tracks traversed source objects.
+ * @param {Array} [stackB=[]] Associates values with source counterparts.
+ */
+function baseMerge(object, source, callback, stackA, stackB) {
+ (isArray(source) ? forEach : forOwn)(source, function(source, key) {
+ var found,
+ isArr,
+ result = source,
+ value = object[key];
+
+ if (source && ((isArr = isArray(source)) || isPlainObject(source))) {
+ // avoid merging previously merged cyclic sources
+ var stackLength = stackA.length;
+ while (stackLength--) {
+ if ((found = stackA[stackLength] == source)) {
+ value = stackB[stackLength];
+ break;
+ }
+ }
+ if (!found) {
+ var isShallow;
+ if (callback) {
+ result = callback(value, source);
+ if ((isShallow = typeof result != 'undefined')) {
+ value = result;
+ }
+ }
+ if (!isShallow) {
+ value = isArr
+ ? (isArray(value) ? value : [])
+ : (isPlainObject(value) ? value : {});
+ }
+ // add `source` and associated `value` to the stack of traversed objects
+ stackA.push(source);
+ stackB.push(value);
+
+ // recursively merge objects and arrays (susceptible to call stack limits)
+ if (!isShallow) {
+ baseMerge(value, source, callback, stackA, stackB);
+ }
+ }
+ }
+ else {
+ if (callback) {
+ result = callback(value, source);
+ if (typeof result == 'undefined') {
+ result = source;
+ }
+ }
+ if (typeof result != 'undefined') {
+ value = result;
+ }
+ }
+ object[key] = value;
+ });
+}
+
+module.exports = baseMerge;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseRandom.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseRandom.js b/node_modules/lodash-node/modern/internals/baseRandom.js
new file mode 100644
index 0000000..3bce8f8
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseRandom.js
@@ -0,0 +1,29 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/** Native method shortcuts */
+var floor = Math.floor;
+
+/* Native method shortcuts for methods with the same name as other `lodash` methods */
+var nativeRandom = Math.random;
+
+/**
+ * The base implementation of `_.random` without argument juggling or support
+ * for returning floating-point numbers.
+ *
+ * @private
+ * @param {number} min The minimum possible value.
+ * @param {number} max The maximum possible value.
+ * @returns {number} Returns a random number.
+ */
+function baseRandom(min, max) {
+ return min + floor(nativeRandom() * (max - min + 1));
+}
+
+module.exports = baseRandom;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/baseUniq.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/baseUniq.js b/node_modules/lodash-node/modern/internals/baseUniq.js
new file mode 100644
index 0000000..32353cc
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/baseUniq.js
@@ -0,0 +1,64 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf'),
+ cacheIndexOf = require('./cacheIndexOf'),
+ createCache = require('./createCache'),
+ getArray = require('./getArray'),
+ largeArraySize = require('./largeArraySize'),
+ releaseArray = require('./releaseArray'),
+ releaseObject = require('./releaseObject');
+
+/**
+ * The base implementation of `_.uniq` without support for callback shorthands
+ * or `thisArg` binding.
+ *
+ * @private
+ * @param {Array} array The array to process.
+ * @param {boolean} [isSorted=false] A flag to indicate that `array` is sorted.
+ * @param {Function} [callback] The function called per iteration.
+ * @returns {Array} Returns a duplicate-value-free array.
+ */
+function baseUniq(array, isSorted, callback) {
+ var index = -1,
+ indexOf = baseIndexOf,
+ length = array ? array.length : 0,
+ result = [];
+
+ var isLarge = !isSorted && length >= largeArraySize,
+ seen = (callback || isLarge) ? getArray() : result;
+
+ if (isLarge) {
+ var cache = createCache(seen);
+ indexOf = cacheIndexOf;
+ seen = cache;
+ }
+ while (++index < length) {
+ var value = array[index],
+ computed = callback ? callback(value, index, array) : value;
+
+ if (isSorted
+ ? !index || seen[seen.length - 1] !== computed
+ : indexOf(seen, computed) < 0
+ ) {
+ if (callback || isLarge) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ if (isLarge) {
+ releaseArray(seen.array);
+ releaseObject(seen);
+ } else if (callback) {
+ releaseArray(seen);
+ }
+ return result;
+}
+
+module.exports = baseUniq;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/cacheIndexOf.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/cacheIndexOf.js b/node_modules/lodash-node/modern/internals/cacheIndexOf.js
new file mode 100644
index 0000000..2d8849c
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/cacheIndexOf.js
@@ -0,0 +1,39 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var baseIndexOf = require('./baseIndexOf'),
+ keyPrefix = require('./keyPrefix');
+
+/**
+ * An implementation of `_.contains` for cache objects that mimics the return
+ * signature of `_.indexOf` by returning `0` if the value is found, else `-1`.
+ *
+ * @private
+ * @param {Object} cache The cache object to inspect.
+ * @param {*} value The value to search for.
+ * @returns {number} Returns `0` if `value` is found, else `-1`.
+ */
+function cacheIndexOf(cache, value) {
+ var type = typeof value;
+ cache = cache.cache;
+
+ if (type == 'boolean' || value == null) {
+ return cache[value] ? 0 : -1;
+ }
+ if (type != 'number' && type != 'string') {
+ type = 'object';
+ }
+ var key = type == 'number' ? value : keyPrefix + value;
+ cache = (cache = cache[type]) && cache[key];
+
+ return type == 'object'
+ ? (cache && baseIndexOf(cache, value) > -1 ? 0 : -1)
+ : (cache ? 0 : -1);
+}
+
+module.exports = cacheIndexOf;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/cachePush.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/cachePush.js b/node_modules/lodash-node/modern/internals/cachePush.js
new file mode 100644
index 0000000..494ccb8
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/cachePush.js
@@ -0,0 +1,38 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var keyPrefix = require('./keyPrefix');
+
+/**
+ * Adds a given value to the corresponding cache object.
+ *
+ * @private
+ * @param {*} value The value to add to the cache.
+ */
+function cachePush(value) {
+ var cache = this.cache,
+ type = typeof value;
+
+ if (type == 'boolean' || value == null) {
+ cache[value] = true;
+ } else {
+ if (type != 'number' && type != 'string') {
+ type = 'object';
+ }
+ var key = type == 'number' ? value : keyPrefix + value,
+ typeCache = cache[type] || (cache[type] = {});
+
+ if (type == 'object') {
+ (typeCache[key] || (typeCache[key] = [])).push(value);
+ } else {
+ typeCache[key] = true;
+ }
+ }
+}
+
+module.exports = cachePush;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/charAtCallback.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/charAtCallback.js b/node_modules/lodash-node/modern/internals/charAtCallback.js
new file mode 100644
index 0000000..b2ee65a
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/charAtCallback.js
@@ -0,0 +1,22 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used by `_.max` and `_.min` as the default callback when a given
+ * collection is a string value.
+ *
+ * @private
+ * @param {string} value The character to inspect.
+ * @returns {number} Returns the code unit of given character.
+ */
+function charAtCallback(value) {
+ return value.charCodeAt(0);
+}
+
+module.exports = charAtCallback;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/compareAscending.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/compareAscending.js b/node_modules/lodash-node/modern/internals/compareAscending.js
new file mode 100644
index 0000000..4dd1a8f
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/compareAscending.js
@@ -0,0 +1,47 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+
+/**
+ * Used by `sortBy` to compare transformed `collection` elements, stable sorting
+ * them in ascending order.
+ *
+ * @private
+ * @param {Object} a The object to compare to `b`.
+ * @param {Object} b The object to compare to `a`.
+ * @returns {number} Returns the sort order indicator of `1` or `-1`.
+ */
+function compareAscending(a, b) {
+ var ac = a.criteria,
+ bc = b.criteria,
+ index = -1,
+ length = ac.length;
+
+ while (++index < length) {
+ var value = ac[index],
+ other = bc[index];
+
+ if (value !== other) {
+ if (value > other || typeof value == 'undefined') {
+ return 1;
+ }
+ if (value < other || typeof other == 'undefined') {
+ return -1;
+ }
+ }
+ }
+ // Fixes an `Array#sort` bug in the JS engine embedded in Adobe applications
+ // that causes it, under certain circumstances, to return the same value for
+ // `a` and `b`. See https://github.com/jashkenas/underscore/pull/1247
+ //
+ // This also ensures a stable sort in V8 and other engines.
+ // See http://code.google.com/p/v8/issues/detail?id=90
+ return a.index - b.index;
+}
+
+module.exports = compareAscending;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/createAggregator.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/createAggregator.js b/node_modules/lodash-node/modern/internals/createAggregator.js
new file mode 100644
index 0000000..3c99dba
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/createAggregator.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var createCallback = require('../functions/createCallback'),
+ forOwn = require('../objects/forOwn'),
+ isArray = require('../objects/isArray');
+
+/**
+ * Creates a function that aggregates a collection, creating an object composed
+ * of keys generated from the results of running each element of the collection
+ * through a callback. The given `setter` function sets the keys and values
+ * of the composed object.
+ *
+ * @private
+ * @param {Function} setter The setter function.
+ * @returns {Function} Returns the new aggregator function.
+ */
+function createAggregator(setter) {
+ return function(collection, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg, 3);
+
+ var index = -1,
+ length = collection ? collection.length : 0;
+
+ if (typeof length == 'number') {
+ while (++index < length) {
+ var value = collection[index];
+ setter(result, value, callback(value, index, collection), collection);
+ }
+ } else {
+ forOwn(collection, function(value, key, collection) {
+ setter(result, value, callback(value, key, collection), collection);
+ });
+ }
+ return result;
+ };
+}
+
+module.exports = createAggregator;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/lodash-node/modern/internals/createCache.js
----------------------------------------------------------------------
diff --git a/node_modules/lodash-node/modern/internals/createCache.js b/node_modules/lodash-node/modern/internals/createCache.js
new file mode 100644
index 0000000..e210516
--- /dev/null
+++ b/node_modules/lodash-node/modern/internals/createCache.js
@@ -0,0 +1,45 @@
+/**
+ * Lo-Dash 2.4.1 (Custom Build) <http://lodash.com/>
+ * Build: `lodash modularize modern exports="node" -o ./modern/`
+ * Copyright 2012-2013 The Dojo Foundation <http://dojofoundation.org/>
+ * Based on Underscore.js 1.5.2 <http://underscorejs.org/LICENSE>
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license <http://lodash.com/license>
+ */
+var cachePush = require('./cachePush'),
+ getObject = require('./getObject'),
+ releaseObject = require('./releaseObject');
+
+/**
+ * Creates a cache object to optimize linear searches of large arrays.
+ *
+ * @private
+ * @param {Array} [array=[]] The array to search.
+ * @returns {null|Object} Returns the cache object or `null` if caching should not be used.
+ */
+function createCache(array) {
+ var index = -1,
+ length = array.length,
+ first = array[0],
+ mid = array[(length / 2) | 0],
+ last = array[length - 1];
+
+ if (first && typeof first == 'object' &&
+ mid && typeof mid == 'object' && last && typeof last == 'object') {
+ return false;
+ }
+ var cache = getObject();
+ cache['false'] = cache['null'] = cache['true'] = cache['undefined'] = false;
+
+ var result = getObject();
+ result.array = array;
+ result.cache = cache;
+ result.push = cachePush;
+
+ while (++index < length) {
+ result.push(array[index]);
+ }
+ return result;
+}
+
+module.exports = createCache;
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org
[45/59] [abbrv] [partial] mac commit: CB-10668 added node_modules
directory
Posted by st...@apache.org.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/CordovaLogger.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/CordovaLogger.js b/node_modules/cordova-common/src/CordovaLogger.js
new file mode 100644
index 0000000..38bfe69
--- /dev/null
+++ b/node_modules/cordova-common/src/CordovaLogger.js
@@ -0,0 +1,203 @@
+/*
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+ */
+
+var ansi = require('ansi');
+var EventEmitter = require('events').EventEmitter;
+var CordovaError = require('./CordovaError/CordovaError');
+var EOL = require('os').EOL;
+
+var INSTANCE;
+
+/**
+ * @class CordovaLogger
+ *
+ * Implements logging facility that anybody could use. Should not be
+ * instantiated directly, `CordovaLogger.get()` method should be used instead
+ * to acquire logger instance
+ */
+function CordovaLogger () {
+ this.levels = {};
+ this.colors = {};
+ this.stdout = process.stdout;
+ this.stderr = process.stderr;
+
+ this.stdoutCursor = ansi(this.stdout);
+ this.stderrCursor = ansi(this.stderr);
+
+ this.addLevel('verbose', 1000, 'grey');
+ this.addLevel('normal' , 2000);
+ this.addLevel('warn' , 2000, 'yellow');
+ this.addLevel('info' , 3000, 'blue');
+ this.addLevel('error' , 5000, 'red');
+ this.addLevel('results' , 10000);
+
+ this.setLevel('normal');
+}
+
+/**
+ * Static method to create new or acquire existing instance.
+ *
+ * @return {CordovaLogger} Logger instance
+ */
+CordovaLogger.get = function () {
+ return INSTANCE || (INSTANCE = new CordovaLogger());
+};
+
+CordovaLogger.VERBOSE = 'verbose';
+CordovaLogger.NORMAL = 'normal';
+CordovaLogger.WARN = 'warn';
+CordovaLogger.INFO = 'info';
+CordovaLogger.ERROR = 'error';
+CordovaLogger.RESULTS = 'results';
+
+/**
+ * Emits log message to process' stdout/stderr depending on message's severity
+ * and current log level. If severity is less than current logger's level,
+ * then the message is ignored.
+ *
+ * @param {String} logLevel The message's log level. The logger should have
+ * corresponding level added (via logger.addLevel), otherwise
+ * `CordovaLogger.NORMAL` level will be used.
+ * @param {String} message The message, that should be logged to process'
+ * stdio
+ *
+ * @return {CordovaLogger} Current instance, to allow calls chaining.
+ */
+CordovaLogger.prototype.log = function (logLevel, message) {
+ // if there is no such logLevel defined, or provided level has
+ // less severity than active level, then just ignore this call and return
+ if (!this.levels[logLevel] || this.levels[logLevel] < this.levels[this.logLevel])
+ // return instance to allow to chain calls
+ return this;
+
+ var isVerbose = this.logLevel === 'verbose';
+ var cursor = this.stdoutCursor;
+
+ if(message instanceof Error || logLevel === CordovaLogger.ERROR) {
+ message = formatError(message, isVerbose);
+ cursor = this.stderrCursor;
+ }
+
+ var color = this.colors[logLevel];
+ if (color) {
+ cursor.bold().fg[color]();
+ }
+
+ cursor.write(message).reset().write(EOL);
+
+ return this;
+};
+
+/**
+ * Adds a new level to logger instance. This method also creates a shortcut
+ * method to log events with the level provided (i.e. after adding new level
+ * 'debug', the method `debug(message)`, equal to logger.log('debug', message),
+ * will be added to logger instance)
+ *
+ * @param {String} level A log level name. The levels with the following
+ * names added by default to every instance: 'verbose', 'normal', 'warn',
+ * 'info', 'error', 'results'
+ * @param {Number} severity A number that represents level's severity.
+ * @param {String} color A valid color name, that will be used to log
+ * messages with this level. Any CSS color code or RGB value is allowed
+ * (according to ansi documentation:
+ * https://github.com/TooTallNate/ansi.js#features)
+ *
+ * @return {CordovaLogger} Current instance, to allow calls chaining.
+ */
+CordovaLogger.prototype.addLevel = function (level, severity, color) {
+
+ this.levels[level] = severity;
+
+ if (color) {
+ this.colors[level] = color;
+ }
+
+ // Define own method with corresponding name
+ if (!this[level]) {
+ this[level] = this.log.bind(this, level);
+ }
+
+ return this;
+};
+
+/**
+ * Sets the current logger level to provided value. If logger doesn't have level
+ * with this name, `CordovaLogger.NORMAL` will be used.
+ *
+ * @param {String} logLevel Level name. The level with this name should be
+ * added to logger before.
+ *
+ * @return {CordovaLogger} Current instance, to allow calls chaining.
+ */
+CordovaLogger.prototype.setLevel = function (logLevel) {
+ this.logLevel = this.levels[logLevel] ? logLevel : CordovaLogger.NORMAL;
+
+ return this;
+};
+
+/**
+ * Attaches logger to EventEmitter instance provided.
+ *
+ * @param {EventEmitter} eventEmitter An EventEmitter instance to attach
+ * logger to.
+ *
+ * @return {CordovaLogger} Current instance, to allow calls chaining.
+ */
+CordovaLogger.prototype.subscribe = function (eventEmitter) {
+
+ if (!(eventEmitter instanceof EventEmitter))
+ throw new Error('Subscribe method only accepts an EventEmitter instance as argument');
+
+ eventEmitter.on('verbose', this.verbose)
+ .on('log', this.normal)
+ .on('info', this.info)
+ .on('warn', this.warn)
+ .on('warning', this.warn)
+ // Set up event handlers for logging and results emitted as events.
+ .on('results', this.results);
+
+ return this;
+};
+
+function formatError(error, isVerbose) {
+ var message = '';
+
+ if(error instanceof CordovaError) {
+ message = error.toString(isVerbose);
+ } else if(error instanceof Error) {
+ if(isVerbose) {
+ message = error.stack;
+ } else {
+ message = error.message;
+ }
+ } else {
+ // Plain text error message
+ message = error;
+ }
+
+ if(message.toUpperCase().indexOf('ERROR:') !== 0) {
+ // Needed for backward compatibility with external tools
+ message = 'Error: ' + message;
+ }
+
+ return message;
+}
+
+module.exports = CordovaLogger;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/PlatformJson.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/PlatformJson.js b/node_modules/cordova-common/src/PlatformJson.js
new file mode 100644
index 0000000..793e976
--- /dev/null
+++ b/node_modules/cordova-common/src/PlatformJson.js
@@ -0,0 +1,155 @@
+/*
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+/* jshint sub:true */
+
+var fs = require('fs');
+var path = require('path');
+var shelljs = require('shelljs');
+var mungeutil = require('./ConfigChanges/munge-util');
+var pluginMappernto = require('cordova-registry-mapper').newToOld;
+var pluginMapperotn = require('cordova-registry-mapper').oldToNew;
+
+function PlatformJson(filePath, platform, root) {
+ this.filePath = filePath;
+ this.platform = platform;
+ this.root = fix_munge(root || {});
+}
+
+PlatformJson.load = function(plugins_dir, platform) {
+ var filePath = path.join(plugins_dir, platform + '.json');
+ var root = null;
+ if (fs.existsSync(filePath)) {
+ root = JSON.parse(fs.readFileSync(filePath, 'utf-8'));
+ }
+ return new PlatformJson(filePath, platform, root);
+};
+
+PlatformJson.prototype.save = function() {
+ shelljs.mkdir('-p', path.dirname(this.filePath));
+ fs.writeFileSync(this.filePath, JSON.stringify(this.root, null, 4), 'utf-8');
+};
+
+/**
+ * Indicates whether the specified plugin is installed as a top-level (not as
+ * dependency to others)
+ * @method function
+ * @param {String} pluginId A plugin id to check for.
+ * @return {Boolean} true if plugin installed as top-level, otherwise false.
+ */
+PlatformJson.prototype.isPluginTopLevel = function(pluginId) {
+ var installedPlugins = this.root.installed_plugins;
+ return installedPlugins[pluginId] ||
+ installedPlugins[pluginMappernto[pluginId]] ||
+ installedPlugins[pluginMapperotn[pluginId]];
+};
+
+/**
+ * Indicates whether the specified plugin is installed as a dependency to other
+ * plugin.
+ * @method function
+ * @param {String} pluginId A plugin id to check for.
+ * @return {Boolean} true if plugin installed as a dependency, otherwise false.
+ */
+PlatformJson.prototype.isPluginDependent = function(pluginId) {
+ var dependentPlugins = this.root.dependent_plugins;
+ return dependentPlugins[pluginId] ||
+ dependentPlugins[pluginMappernto[pluginId]] ||
+ dependentPlugins[pluginMapperotn[pluginId]];
+};
+
+/**
+ * Indicates whether plugin is installed either as top-level or as dependency.
+ * @method function
+ * @param {String} pluginId A plugin id to check for.
+ * @return {Boolean} true if plugin installed, otherwise false.
+ */
+PlatformJson.prototype.isPluginInstalled = function(pluginId) {
+ return this.isPluginTopLevel(pluginId) ||
+ this.isPluginDependent(pluginId);
+};
+
+PlatformJson.prototype.addPlugin = function(pluginId, variables, isTopLevel) {
+ var pluginsList = isTopLevel ?
+ this.root.installed_plugins :
+ this.root.dependent_plugins;
+
+ pluginsList[pluginId] = variables;
+
+ return this;
+};
+
+PlatformJson.prototype.removePlugin = function(pluginId, isTopLevel) {
+ var pluginsList = isTopLevel ?
+ this.root.installed_plugins :
+ this.root.dependent_plugins;
+
+ delete pluginsList[pluginId];
+
+ return this;
+};
+
+PlatformJson.prototype.addInstalledPluginToPrepareQueue = function(pluginDirName, vars, is_top_level) {
+ this.root.prepare_queue.installed.push({'plugin':pluginDirName, 'vars':vars, 'topLevel':is_top_level});
+};
+
+PlatformJson.prototype.addUninstalledPluginToPrepareQueue = function(pluginId, is_top_level) {
+ this.root.prepare_queue.uninstalled.push({'plugin':pluginId, 'id':pluginId, 'topLevel':is_top_level});
+};
+
+/**
+ * Moves plugin, specified by id to top-level plugins. If plugin is top-level
+ * already, then does nothing.
+ * @method function
+ * @param {String} pluginId A plugin id to make top-level.
+ * @return {PlatformJson} PlatformJson instance.
+ */
+PlatformJson.prototype.makeTopLevel = function(pluginId) {
+ var plugin = this.root.dependent_plugins[pluginId];
+ if (plugin) {
+ delete this.root.dependent_plugins[pluginId];
+ this.root.installed_plugins[pluginId] = plugin;
+ }
+ return this;
+};
+
+// convert a munge from the old format ([file][parent][xml] = count) to the current one
+function fix_munge(root) {
+ root.prepare_queue = root.prepare_queue || {installed:[], uninstalled:[]};
+ root.config_munge = root.config_munge || {files: {}};
+ root.installed_plugins = root.installed_plugins || {};
+ root.dependent_plugins = root.dependent_plugins || {};
+
+ var munge = root.config_munge;
+ if (!munge.files) {
+ var new_munge = { files: {} };
+ for (var file in munge) {
+ for (var selector in munge[file]) {
+ for (var xml_child in munge[file][selector]) {
+ var val = parseInt(munge[file][selector][xml_child]);
+ for (var i = 0; i < val; i++) {
+ mungeutil.deep_add(new_munge, [file, selector, { xml: xml_child, count: val }]);
+ }
+ }
+ }
+ }
+ root.config_munge = new_munge;
+ }
+
+ return root;
+}
+
+module.exports = PlatformJson;
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/PluginInfo/PluginInfo.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/PluginInfo/PluginInfo.js b/node_modules/cordova-common/src/PluginInfo/PluginInfo.js
new file mode 100644
index 0000000..2554a3c
--- /dev/null
+++ b/node_modules/cordova-common/src/PluginInfo/PluginInfo.js
@@ -0,0 +1,410 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+/* jshint sub:true, laxcomma:true, laxbreak:true */
+
+/*
+A class for holidng the information currently stored in plugin.xml
+It should also be able to answer questions like whether the plugin
+is compatible with a given engine version.
+
+TODO (kamrik): refactor this to not use sync functions and return promises.
+*/
+
+
+var path = require('path')
+ , fs = require('fs')
+ , xml_helpers = require('../util/xml-helpers')
+ , CordovaError = require('../CordovaError/CordovaError')
+ ;
+
+function PluginInfo(dirname) {
+ var self = this;
+
+ // METHODS
+ // Defined inside the constructor to avoid the "this" binding problems.
+
+ // <preference> tag
+ // Example: <preference name="API_KEY" />
+ // Used to require a variable to be specified via --variable when installing the plugin.
+ self.getPreferences = getPreferences;
+ function getPreferences(platform) {
+ var arprefs = _getTags(self._et, 'preference', platform, _parsePreference);
+
+ var prefs= {};
+ for(var i in arprefs)
+ {
+ var pref=arprefs[i];
+ prefs[pref.preference]=pref.default;
+ }
+ // returns { key : default | null}
+ return prefs;
+ }
+
+ function _parsePreference(prefTag) {
+ var name = prefTag.attrib.name.toUpperCase();
+ var def = prefTag.attrib.default || null;
+ return {preference: name, default: def};
+ }
+
+ // <asset>
+ self.getAssets = getAssets;
+ function getAssets(platform) {
+ var assets = _getTags(self._et, 'asset', platform, _parseAsset);
+ return assets;
+ }
+
+ function _parseAsset(tag) {
+ var src = tag.attrib.src;
+ var target = tag.attrib.target;
+
+ if ( !src || !target) {
+ var msg =
+ 'Malformed <asset> tag. Both "src" and "target" attributes'
+ + 'must be specified in\n'
+ + self.filepath
+ ;
+ throw new Error(msg);
+ }
+
+ var asset = {
+ itemType: 'asset',
+ src: src,
+ target: target
+ };
+ return asset;
+ }
+
+
+ // <dependency>
+ // Example:
+ // <dependency id="com.plugin.id"
+ // url="https://github.com/myuser/someplugin"
+ // commit="428931ada3891801"
+ // subdir="some/path/here" />
+ self.getDependencies = getDependencies;
+ function getDependencies(platform) {
+ var deps = _getTags(
+ self._et,
+ 'dependency',
+ platform,
+ _parseDependency
+ );
+ return deps;
+ }
+
+ function _parseDependency(tag) {
+ var dep =
+ { id : tag.attrib.id
+ , url : tag.attrib.url || ''
+ , subdir : tag.attrib.subdir || ''
+ , commit : tag.attrib.commit
+ };
+
+ dep.git_ref = dep.commit;
+
+ if ( !dep.id ) {
+ var msg =
+ '<dependency> tag is missing id attribute in '
+ + self.filepath
+ ;
+ throw new CordovaError(msg);
+ }
+ return dep;
+ }
+
+
+ // <config-file> tag
+ self.getConfigFiles = getConfigFiles;
+ function getConfigFiles(platform) {
+ var configFiles = _getTags(self._et, 'config-file', platform, _parseConfigFile);
+ return configFiles;
+ }
+
+ function _parseConfigFile(tag) {
+ var configFile =
+ { target : tag.attrib['target']
+ , parent : tag.attrib['parent']
+ , after : tag.attrib['after']
+ , xmls : tag.getchildren()
+ // To support demuxing via versions
+ , versions : tag.attrib['versions']
+ , deviceTarget: tag.attrib['device-target']
+ };
+ return configFile;
+ }
+
+ // <info> tags, both global and within a <platform>
+ // TODO (kamrik): Do we ever use <info> under <platform>? Example wanted.
+ self.getInfo = getInfo;
+ function getInfo(platform) {
+ var infos = _getTags(
+ self._et,
+ 'info',
+ platform,
+ function(elem) { return elem.text; }
+ );
+ // Filter out any undefined or empty strings.
+ infos = infos.filter(Boolean);
+ return infos;
+ }
+
+ // <source-file>
+ // Examples:
+ // <source-file src="src/ios/someLib.a" framework="true" />
+ // <source-file src="src/ios/someLib.a" compiler-flags="-fno-objc-arc" />
+ self.getSourceFiles = getSourceFiles;
+ function getSourceFiles(platform) {
+ var sourceFiles = _getTagsInPlatform(self._et, 'source-file', platform, _parseSourceFile);
+ return sourceFiles;
+ }
+
+ function _parseSourceFile(tag) {
+ return {
+ itemType: 'source-file',
+ src: tag.attrib.src,
+ framework: isStrTrue(tag.attrib.framework),
+ weak: isStrTrue(tag.attrib.weak),
+ compilerFlags: tag.attrib['compiler-flags'],
+ targetDir: tag.attrib['target-dir']
+ };
+ }
+
+ // <header-file>
+ // Example:
+ // <header-file src="CDVFoo.h" />
+ self.getHeaderFiles = getHeaderFiles;
+ function getHeaderFiles(platform) {
+ var headerFiles = _getTagsInPlatform(self._et, 'header-file', platform, function(tag) {
+ return {
+ itemType: 'header-file',
+ src: tag.attrib.src,
+ targetDir: tag.attrib['target-dir']
+ };
+ });
+ return headerFiles;
+ }
+
+ // <resource-file>
+ // Example:
+ // <resource-file src="FooPluginStrings.xml" target="res/values/FooPluginStrings.xml" device-target="win" arch="x86" versions=">=8.1" />
+ self.getResourceFiles = getResourceFiles;
+ function getResourceFiles(platform) {
+ var resourceFiles = _getTagsInPlatform(self._et, 'resource-file', platform, function(tag) {
+ return {
+ itemType: 'resource-file',
+ src: tag.attrib.src,
+ target: tag.attrib.target,
+ versions: tag.attrib.versions,
+ deviceTarget: tag.attrib['device-target'],
+ arch: tag.attrib.arch
+ };
+ });
+ return resourceFiles;
+ }
+
+ // <lib-file>
+ // Example:
+ // <lib-file src="src/BlackBerry10/native/device/libfoo.so" arch="device" />
+ self.getLibFiles = getLibFiles;
+ function getLibFiles(platform) {
+ var libFiles = _getTagsInPlatform(self._et, 'lib-file', platform, function(tag) {
+ return {
+ itemType: 'lib-file',
+ src: tag.attrib.src,
+ arch: tag.attrib.arch,
+ Include: tag.attrib.Include,
+ versions: tag.attrib.versions,
+ deviceTarget: tag.attrib['device-target'] || tag.attrib.target
+ };
+ });
+ return libFiles;
+ }
+
+ // <hook>
+ // Example:
+ // <hook type="before_build" src="scripts/beforeBuild.js" />
+ self.getHookScripts = getHookScripts;
+ function getHookScripts(hook, platforms) {
+ var scriptElements = self._et.findall('./hook');
+
+ if(platforms) {
+ platforms.forEach(function (platform) {
+ scriptElements = scriptElements.concat(self._et.findall('./platform[@name="' + platform + '"]/hook'));
+ });
+ }
+
+ function filterScriptByHookType(el) {
+ return el.attrib.src && el.attrib.type && el.attrib.type.toLowerCase() === hook;
+ }
+
+ return scriptElements.filter(filterScriptByHookType);
+ }
+
+ self.getJsModules = getJsModules;
+ function getJsModules(platform) {
+ var modules = _getTags(self._et, 'js-module', platform, _parseJsModule);
+ return modules;
+ }
+
+ function _parseJsModule(tag) {
+ var ret = {
+ itemType: 'js-module',
+ name: tag.attrib.name,
+ src: tag.attrib.src,
+ clobbers: tag.findall('clobbers').map(function(tag) { return { target: tag.attrib.target }; }),
+ merges: tag.findall('merges').map(function(tag) { return { target: tag.attrib.target }; }),
+ runs: tag.findall('runs').length > 0
+ };
+
+ return ret;
+ }
+
+ self.getEngines = function() {
+ return self._et.findall('engines/engine').map(function(n) {
+ return {
+ name: n.attrib.name,
+ version: n.attrib.version,
+ platform: n.attrib.platform,
+ scriptSrc: n.attrib.scriptSrc
+ };
+ });
+ };
+
+ self.getPlatforms = function() {
+ return self._et.findall('platform').map(function(n) {
+ return { name: n.attrib.name };
+ });
+ };
+
+ self.getPlatformsArray = function() {
+ return self._et.findall('platform').map(function(n) {
+ return n.attrib.name;
+ });
+ };
+ self.getFrameworks = function(platform) {
+ return _getTags(self._et, 'framework', platform, function(el) {
+ var ret = {
+ itemType: 'framework',
+ type: el.attrib.type,
+ parent: el.attrib.parent,
+ custom: isStrTrue(el.attrib.custom),
+ src: el.attrib.src,
+ weak: isStrTrue(el.attrib.weak),
+ versions: el.attrib.versions,
+ targetDir: el.attrib['target-dir'],
+ deviceTarget: el.attrib['device-target'] || el.attrib.target,
+ arch: el.attrib.arch
+ };
+ return ret;
+ });
+ };
+
+ self.getFilesAndFrameworks = getFilesAndFrameworks;
+ function getFilesAndFrameworks(platform) {
+ // Please avoid changing the order of the calls below, files will be
+ // installed in this order.
+ var items = [].concat(
+ self.getSourceFiles(platform),
+ self.getHeaderFiles(platform),
+ self.getResourceFiles(platform),
+ self.getFrameworks(platform),
+ self.getLibFiles(platform)
+ );
+ return items;
+ }
+ ///// End of PluginInfo methods /////
+
+
+ ///// PluginInfo Constructor logic /////
+ self.filepath = path.join(dirname, 'plugin.xml');
+ if (!fs.existsSync(self.filepath)) {
+ throw new CordovaError('Cannot find plugin.xml for plugin \'' + path.basename(dirname) + '\'. Please try adding it again.');
+ }
+
+ self.dir = dirname;
+ var et = self._et = xml_helpers.parseElementtreeSync(self.filepath);
+ var pelem = et.getroot();
+ self.id = pelem.attrib.id;
+ self.version = pelem.attrib.version;
+
+ // Optional fields
+ self.name = pelem.findtext('name');
+ self.description = pelem.findtext('description');
+ self.license = pelem.findtext('license');
+ self.repo = pelem.findtext('repo');
+ self.issue = pelem.findtext('issue');
+ self.keywords = pelem.findtext('keywords');
+ self.info = pelem.findtext('info');
+ if (self.keywords) {
+ self.keywords = self.keywords.split(',').map( function(s) { return s.trim(); } );
+ }
+ self.getKeywordsAndPlatforms = function () {
+ var ret = self.keywords || [];
+ return ret.concat('ecosystem:cordova').concat(addCordova(self.getPlatformsArray()));
+ };
+} // End of PluginInfo constructor.
+
+// Helper function used to prefix every element of an array with cordova-
+// Useful when we want to modify platforms to be cordova-platform
+function addCordova(someArray) {
+ var newArray = someArray.map(function(element) {
+ return 'cordova-' + element;
+ });
+ return newArray;
+}
+
+// Helper function used by most of the getSomething methods of PluginInfo.
+// Get all elements of a given name. Both in root and in platform sections
+// for the given platform. If transform is given and is a function, it is
+// applied to each element.
+function _getTags(pelem, tag, platform, transform) {
+ var platformTag = pelem.find('./platform[@name="' + platform + '"]');
+ var tagsInRoot = pelem.findall(tag);
+ tagsInRoot = tagsInRoot || [];
+ var tagsInPlatform = platformTag ? platformTag.findall(tag) : [];
+ var tags = tagsInRoot.concat(tagsInPlatform);
+ if ( typeof transform === 'function' ) {
+ tags = tags.map(transform);
+ }
+ return tags;
+}
+
+// Same as _getTags() but only looks inside a platfrom section.
+function _getTagsInPlatform(pelem, tag, platform, transform) {
+ var platformTag = pelem.find('./platform[@name="' + platform + '"]');
+ var tags = platformTag ? platformTag.findall(tag) : [];
+ if ( typeof transform === 'function' ) {
+ tags = tags.map(transform);
+ }
+ return tags;
+}
+
+// Check if x is a string 'true'.
+function isStrTrue(x) {
+ return String(x).toLowerCase() == 'true';
+}
+
+module.exports = PluginInfo;
+// Backwards compat:
+PluginInfo.PluginInfo = PluginInfo;
+PluginInfo.loadPluginsDir = function(dir) {
+ var PluginInfoProvider = require('./PluginInfoProvider');
+ return new PluginInfoProvider().getAllWithinSearchPath(dir);
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/PluginInfo/PluginInfoProvider.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/PluginInfo/PluginInfoProvider.js b/node_modules/cordova-common/src/PluginInfo/PluginInfoProvider.js
new file mode 100644
index 0000000..6240119
--- /dev/null
+++ b/node_modules/cordova-common/src/PluginInfo/PluginInfoProvider.js
@@ -0,0 +1,82 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+/* jshint sub:true, laxcomma:true, laxbreak:true */
+
+var fs = require('fs');
+var path = require('path');
+var PluginInfo = require('./PluginInfo');
+var events = require('../events');
+
+function PluginInfoProvider() {
+ this._cache = {};
+ this._getAllCache = {};
+}
+
+PluginInfoProvider.prototype.get = function(dirName) {
+ var absPath = path.resolve(dirName);
+ if (!this._cache[absPath]) {
+ this._cache[absPath] = new PluginInfo(dirName);
+ }
+ return this._cache[absPath];
+};
+
+// Normally you don't need to put() entries, but it's used
+// when copying plugins, and in unit tests.
+PluginInfoProvider.prototype.put = function(pluginInfo) {
+ var absPath = path.resolve(pluginInfo.dir);
+ this._cache[absPath] = pluginInfo;
+};
+
+// Used for plugin search path processing.
+// Given a dir containing multiple plugins, create a PluginInfo object for
+// each of them and return as array.
+// Should load them all in parallel and return a promise, but not yet.
+PluginInfoProvider.prototype.getAllWithinSearchPath = function(dirName) {
+ var absPath = path.resolve(dirName);
+ if (!this._getAllCache[absPath]) {
+ this._getAllCache[absPath] = getAllHelper(absPath, this);
+ }
+ return this._getAllCache[absPath];
+};
+
+function getAllHelper(absPath, provider) {
+ if (!fs.existsSync(absPath)){
+ return [];
+ }
+ // If dir itself is a plugin, return it in an array with one element.
+ if (fs.existsSync(path.join(absPath, 'plugin.xml'))) {
+ return [provider.get(absPath)];
+ }
+ var subdirs = fs.readdirSync(absPath);
+ var plugins = [];
+ subdirs.forEach(function(subdir) {
+ var d = path.join(absPath, subdir);
+ if (fs.existsSync(path.join(d, 'plugin.xml'))) {
+ try {
+ plugins.push(provider.get(d));
+ } catch (e) {
+ events.emit('warn', 'Error parsing ' + path.join(d, 'plugin.xml.\n' + e.stack));
+ }
+ }
+ });
+ return plugins;
+}
+
+module.exports = PluginInfoProvider;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/events.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/events.js b/node_modules/cordova-common/src/events.js
new file mode 100644
index 0000000..868d363
--- /dev/null
+++ b/node_modules/cordova-common/src/events.js
@@ -0,0 +1,65 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+var EventEmitter = require('events').EventEmitter;
+
+var INSTANCE = new EventEmitter();
+var EVENTS_RECEIVER;
+
+module.exports = INSTANCE;
+
+/**
+ * Sets up current instance to forward emitted events to another EventEmitter
+ * instance.
+ *
+ * @param {EventEmitter} [eventEmitter] The emitter instance to forward
+ * events to. Falsy value, when passed, disables forwarding.
+ */
+module.exports.forwardEventsTo = function (eventEmitter) {
+
+ // If no argument is specified disable events forwarding
+ if (!eventEmitter) {
+ EVENTS_RECEIVER = undefined;
+ return;
+ }
+
+ if (!(eventEmitter instanceof EventEmitter))
+ throw new Error('Cordova events could be redirected to another EventEmitter instance only');
+
+ EVENTS_RECEIVER = eventEmitter;
+};
+
+var emit = INSTANCE.emit;
+
+/**
+ * This method replaces original 'emit' method to allow events forwarding.
+ *
+ * @return {eventEmitter} Current instance to allow calls chaining, as
+ * original 'emit' does
+ */
+module.exports.emit = function () {
+
+ var args = Array.prototype.slice.call(arguments);
+
+ if (EVENTS_RECEIVER) {
+ EVENTS_RECEIVER.emit.apply(EVENTS_RECEIVER, args);
+ }
+
+ return emit.apply(this, args);
+};
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/superspawn.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/superspawn.js b/node_modules/cordova-common/src/superspawn.js
new file mode 100644
index 0000000..a3f1431
--- /dev/null
+++ b/node_modules/cordova-common/src/superspawn.js
@@ -0,0 +1,184 @@
+/**
+ Licensed to the Apache Software Foundation (ASF) under one
+ or more contributor license agreements. See the NOTICE file
+ distributed with this work for additional information
+ regarding copyright ownership. The ASF licenses this file
+ to you under the Apache License, Version 2.0 (the
+ "License"); you may not use this file except in compliance
+ with the License. You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing,
+ software distributed under the License is distributed on an
+ "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ KIND, either express or implied. See the License for the
+ specific language governing permissions and limitations
+ under the License.
+*/
+
+var child_process = require('child_process');
+var fs = require('fs');
+var path = require('path');
+var _ = require('underscore');
+var Q = require('q');
+var shell = require('shelljs');
+var events = require('./events');
+var iswin32 = process.platform == 'win32';
+
+// On Windows, spawn() for batch files requires absolute path & having the extension.
+function resolveWindowsExe(cmd) {
+ var winExtensions = ['.exe', '.bat', '.cmd', '.js', '.vbs'];
+ function isValidExe(c) {
+ return winExtensions.indexOf(path.extname(c)) !== -1 && fs.existsSync(c);
+ }
+ if (isValidExe(cmd)) {
+ return cmd;
+ }
+ cmd = shell.which(cmd) || cmd;
+ if (!isValidExe(cmd)) {
+ winExtensions.some(function(ext) {
+ if (fs.existsSync(cmd + ext)) {
+ cmd = cmd + ext;
+ return true;
+ }
+ });
+ }
+ return cmd;
+}
+
+function maybeQuote(a) {
+ if (/^[^"].*[ &].*[^"]/.test(a)) return '"' + a + '"';
+ return a;
+}
+
+/**
+ * A special implementation for child_process.spawn that handles
+ * Windows-specific issues with batch files and spaces in paths. Returns a
+ * promise that succeeds only for return code 0. It is also possible to
+ * subscribe on spawned process' stdout and stderr streams using progress
+ * handler for resultant promise.
+ *
+ * @example spawn('mycommand', [], {stdio: 'pipe'}) .progress(function (stdio){
+ * if (stdio.stderr) { console.error(stdio.stderr); } })
+ * .then(function(result){ // do other stuff })
+ *
+ * @param {String} cmd A command to spawn
+ * @param {String[]} [args=[]] An array of arguments, passed to spawned
+ * process
+ * @param {Object} [opts={}] A configuration object
+ * @param {String|String[]|Object} opts.stdio Property that configures how
+ * spawned process' stdio will behave. Has the same meaning and possible
+ * values as 'stdio' options for child_process.spawn method
+ * (https://nodejs.org/api/child_process.html#child_process_options_stdio).
+ * @param {Object} [env={}] A map of extra environment variables
+ * @param {String} [cwd=process.cwd()] Working directory for the command
+ * @param {Boolean} [chmod=false] If truthy, will attempt to set the execute
+ * bit before executing on non-Windows platforms
+ *
+ * @return {Promise} A promise that is either fulfilled if the spawned
+ * process is exited with zero error code or rejected otherwise. If the
+ * 'stdio' option set to 'default' or 'pipe', the promise also emits progress
+ * messages with the following contents:
+ * {
+ * 'stdout': ...,
+ * 'stderr': ...
+ * }
+ */
+exports.spawn = function(cmd, args, opts) {
+ args = args || [];
+ opts = opts || {};
+ var spawnOpts = {};
+ var d = Q.defer();
+
+ if (iswin32) {
+ cmd = resolveWindowsExe(cmd);
+ // If we couldn't find the file, likely we'll end up failing,
+ // but for things like "del", cmd will do the trick.
+ if (path.extname(cmd) != '.exe') {
+ var cmdArgs = '"' + [cmd].concat(args).map(maybeQuote).join(' ') + '"';
+ // We need to use /s to ensure that spaces are parsed properly with cmd spawned content
+ args = [['/s', '/c', cmdArgs].join(' ')];
+ cmd = 'cmd';
+ spawnOpts.windowsVerbatimArguments = true;
+ } else if (!fs.existsSync(cmd)) {
+ // We need to use /s to ensure that spaces are parsed properly with cmd spawned content
+ args = ['/s', '/c', cmd].concat(args).map(maybeQuote);
+ }
+ }
+
+ if (opts.stdio !== 'default') {
+ // Ignore 'default' value for stdio because it corresponds to child_process's default 'pipe' option
+ spawnOpts.stdio = opts.stdio;
+ }
+
+ if (opts.cwd) {
+ spawnOpts.cwd = opts.cwd;
+ }
+
+ if (opts.env) {
+ spawnOpts.env = _.extend(_.extend({}, process.env), opts.env);
+ }
+
+ if (opts.chmod && !iswin32) {
+ try {
+ // This fails when module is installed in a system directory (e.g. via sudo npm install)
+ fs.chmodSync(cmd, '755');
+ } catch (e) {
+ // If the perms weren't set right, then this will come as an error upon execution.
+ }
+ }
+
+ events.emit(opts.printCommand ? 'log' : 'verbose', 'Running command: ' + maybeQuote(cmd) + ' ' + args.map(maybeQuote).join(' '));
+
+ var child = child_process.spawn(cmd, args, spawnOpts);
+ var capturedOut = '';
+ var capturedErr = '';
+
+ if (child.stdout) {
+ child.stdout.setEncoding('utf8');
+ child.stdout.on('data', function(data) {
+ capturedOut += data;
+ d.notify({'stdout': data});
+ });
+ }
+
+ if (child.stderr) {
+ child.stderr.setEncoding('utf8');
+ child.stderr.on('data', function(data) {
+ capturedErr += data;
+ d.notify({'stderr': data});
+ });
+ }
+
+ child.on('close', whenDone);
+ child.on('error', whenDone);
+ function whenDone(arg) {
+ child.removeListener('close', whenDone);
+ child.removeListener('error', whenDone);
+ var code = typeof arg == 'number' ? arg : arg && arg.code;
+
+ events.emit('verbose', 'Command finished with error code ' + code + ': ' + cmd + ' ' + args);
+ if (code === 0) {
+ d.resolve(capturedOut.trim());
+ } else {
+ var errMsg = cmd + ': Command failed with exit code ' + code;
+ if (capturedErr) {
+ errMsg += ' Error output:\n' + capturedErr.trim();
+ }
+ var err = new Error(errMsg);
+ err.code = code;
+ d.reject(err);
+ }
+ }
+
+ return d.promise;
+};
+
+exports.maybeSpawn = function(cmd, args, opts) {
+ if (fs.existsSync(cmd)) {
+ return exports.spawn(cmd, args, opts);
+ }
+ return Q(null);
+};
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/util/plist-helpers.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/util/plist-helpers.js b/node_modules/cordova-common/src/util/plist-helpers.js
new file mode 100644
index 0000000..9dee5c6
--- /dev/null
+++ b/node_modules/cordova-common/src/util/plist-helpers.js
@@ -0,0 +1,101 @@
+/*
+ *
+ * Copyright 2013 Brett Rudd
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+
+// contains PLIST utility functions
+var __ = require('underscore');
+var plist = require('plist');
+
+// adds node to doc at selector
+module.exports.graftPLIST = graftPLIST;
+function graftPLIST(doc, xml, selector) {
+ var obj = plist.parse('<plist>'+xml+'</plist>');
+
+ var node = doc[selector];
+ if (node && Array.isArray(node) && Array.isArray(obj)){
+ node = node.concat(obj);
+ for (var i =0;i<node.length; i++){
+ for (var j=i+1; j<node.length; ++j) {
+ if (nodeEqual(node[i], node[j]))
+ node.splice(j--,1);
+ }
+ }
+ doc[selector] = node;
+ } else {
+ //plist uses objects for <dict>. If we have two dicts we merge them instead of
+ // overriding the old one. See CB-6472
+ if (node && __.isObject(node) && __.isObject(obj) && !__.isDate(node) && !__.isDate(obj)){//arrays checked above
+ __.extend(obj,node);
+ }
+ doc[selector] = obj;
+ }
+
+ return true;
+}
+
+// removes node from doc at selector
+module.exports.prunePLIST = prunePLIST;
+function prunePLIST(doc, xml, selector) {
+ var obj = plist.parse('<plist>'+xml+'</plist>');
+
+ pruneOBJECT(doc, selector, obj);
+
+ return true;
+}
+
+function pruneOBJECT(doc, selector, fragment) {
+ if (Array.isArray(fragment) && Array.isArray(doc[selector])) {
+ var empty = true;
+ for (var i in fragment) {
+ for (var j in doc[selector]) {
+ empty = pruneOBJECT(doc[selector], j, fragment[i]) && empty;
+ }
+ }
+ if (empty)
+ {
+ delete doc[selector];
+ return true;
+ }
+ }
+ else if (nodeEqual(doc[selector], fragment)) {
+ delete doc[selector];
+ return true;
+ }
+
+ return false;
+}
+
+function nodeEqual(node1, node2) {
+ if (typeof node1 != typeof node2)
+ return false;
+ else if (typeof node1 == 'string') {
+ node2 = escapeRE(node2).replace(new RegExp('\\$[a-zA-Z0-9-_]+','gm'),'(.*?)');
+ return new RegExp('^' + node2 + '$').test(node1);
+ }
+ else {
+ for (var key in node2) {
+ if (!nodeEqual(node1[key], node2[key])) return false;
+ }
+ return true;
+ }
+}
+
+// escape string for use in regex
+function escapeRE(str) {
+ return str.replace(/[\-\[\]\/\{\}\(\)\*\+\?\.\\\^\$\|]/g, '$&');
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-common/src/util/xml-helpers.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/src/util/xml-helpers.js b/node_modules/cordova-common/src/util/xml-helpers.js
new file mode 100644
index 0000000..8b02989
--- /dev/null
+++ b/node_modules/cordova-common/src/util/xml-helpers.js
@@ -0,0 +1,266 @@
+/*
+ *
+ * Copyright 2013 Anis Kadri
+ *
+ * Licensed under the Apache License, Version 2.0 (the "License");
+ * you may not use this file except in compliance with the License.
+ * You may obtain a copy of the License at
+ *
+ * http://www.apache.org/licenses/LICENSE-2.0
+ *
+ * Unless required by applicable law or agreed to in writing,
+ * software distributed under the License is distributed on an
+ * "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
+ * KIND, either express or implied. See the License for the
+ * specific language governing permissions and limitations
+ * under the License.
+ *
+*/
+
+/* jshint sub:true, laxcomma:true */
+
+/**
+ * contains XML utility functions, some of which are specific to elementtree
+ */
+
+var fs = require('fs')
+ , path = require('path')
+ , _ = require('underscore')
+ , et = require('elementtree')
+ ;
+
+module.exports = {
+ // compare two et.XML nodes, see if they match
+ // compares tagName, text, attributes and children (recursively)
+ equalNodes: function(one, two) {
+ if (one.tag != two.tag) {
+ return false;
+ } else if (one.text.trim() != two.text.trim()) {
+ return false;
+ } else if (one._children.length != two._children.length) {
+ return false;
+ }
+
+ var oneAttribKeys = Object.keys(one.attrib),
+ twoAttribKeys = Object.keys(two.attrib),
+ i = 0, attribName;
+
+ if (oneAttribKeys.length != twoAttribKeys.length) {
+ return false;
+ }
+
+ for (i; i < oneAttribKeys.length; i++) {
+ attribName = oneAttribKeys[i];
+
+ if (one.attrib[attribName] != two.attrib[attribName]) {
+ return false;
+ }
+ }
+
+ for (i; i < one._children.length; i++) {
+ if (!module.exports.equalNodes(one._children[i], two._children[i])) {
+ return false;
+ }
+ }
+
+ return true;
+ },
+
+ // adds node to doc at selector, creating parent if it doesn't exist
+ graftXML: function(doc, nodes, selector, after) {
+ var parent = resolveParent(doc, selector);
+ if (!parent) {
+ //Try to create the parent recursively if necessary
+ try {
+ var parentToCreate = et.XML('<' + path.basename(selector) + '>'),
+ parentSelector = path.dirname(selector);
+
+ this.graftXML(doc, [parentToCreate], parentSelector);
+ } catch (e) {
+ return false;
+ }
+ parent = resolveParent(doc, selector);
+ if (!parent) return false;
+ }
+
+ nodes.forEach(function (node) {
+ // check if child is unique first
+ if (uniqueChild(node, parent)) {
+ var children = parent.getchildren();
+ var insertIdx = after ? findInsertIdx(children, after) : children.length;
+
+ //TODO: replace with parent.insert after the bug in ElementTree is fixed
+ parent.getchildren().splice(insertIdx, 0, node);
+ }
+ });
+
+ return true;
+ },
+
+ // removes node from doc at selector
+ pruneXML: function(doc, nodes, selector) {
+ var parent = resolveParent(doc, selector);
+ if (!parent) return false;
+
+ nodes.forEach(function (node) {
+ var matchingKid = null;
+ if ((matchingKid = findChild(node, parent)) !== null) {
+ // stupid elementtree takes an index argument it doesn't use
+ // and does not conform to the python lib
+ parent.remove(matchingKid);
+ }
+ });
+
+ return true;
+ },
+
+ parseElementtreeSync: function (filename) {
+ var contents = fs.readFileSync(filename, 'utf-8');
+ if(contents) {
+ //Windows is the BOM. Skip the Byte Order Mark.
+ contents = contents.substring(contents.indexOf('<'));
+ }
+ return new et.ElementTree(et.XML(contents));
+ }
+};
+
+function findChild(node, parent) {
+ var matchingKids = parent.findall(node.tag)
+ , i, j;
+
+ for (i = 0, j = matchingKids.length ; i < j ; i++) {
+ if (module.exports.equalNodes(node, matchingKids[i])) {
+ return matchingKids[i];
+ }
+ }
+ return null;
+}
+
+function uniqueChild(node, parent) {
+ var matchingKids = parent.findall(node.tag)
+ , i = 0;
+
+ if (matchingKids.length === 0) {
+ return true;
+ } else {
+ for (i; i < matchingKids.length; i++) {
+ if (module.exports.equalNodes(node, matchingKids[i])) {
+ return false;
+ }
+ }
+ return true;
+ }
+}
+
+var ROOT = /^\/([^\/]*)/,
+ ABSOLUTE = /^\/([^\/]*)\/(.*)/;
+
+function resolveParent(doc, selector) {
+ var parent, tagName, subSelector;
+
+ // handle absolute selector (which elementtree doesn't like)
+ if (ROOT.test(selector)) {
+ tagName = selector.match(ROOT)[1];
+ // test for wildcard "any-tag" root selector
+ if (tagName == '*' || tagName === doc._root.tag) {
+ parent = doc._root;
+
+ // could be an absolute path, but not selecting the root
+ if (ABSOLUTE.test(selector)) {
+ subSelector = selector.match(ABSOLUTE)[2];
+ parent = parent.find(subSelector);
+ }
+ } else {
+ return false;
+ }
+ } else {
+ parent = doc.find(selector);
+ }
+ return parent;
+}
+
+// Find the index at which to insert an entry. After is a ;-separated priority list
+// of tags after which the insertion should be made. E.g. If we need to
+// insert an element C, and the rule is that the order of children has to be
+// As, Bs, Cs. After will be equal to "C;B;A".
+function findInsertIdx(children, after) {
+ var childrenTags = children.map(function(child) { return child.tag; });
+ var afters = after.split(';');
+ var afterIndexes = afters.map(function(current) { return childrenTags.lastIndexOf(current); });
+ var foundIndex = _.find(afterIndexes, function(index) { return index != -1; });
+
+ //add to the beginning if no matching nodes are found
+ return typeof foundIndex === 'undefined' ? 0 : foundIndex+1;
+}
+
+var BLACKLIST = ['platform', 'feature','plugin','engine'];
+var SINGLETONS = ['content', 'author'];
+function mergeXml(src, dest, platform, clobber) {
+ // Do nothing for blacklisted tags.
+ if (BLACKLIST.indexOf(src.tag) != -1) return;
+
+ //Handle attributes
+ Object.getOwnPropertyNames(src.attrib).forEach(function (attribute) {
+ if (clobber || !dest.attrib[attribute]) {
+ dest.attrib[attribute] = src.attrib[attribute];
+ }
+ });
+ //Handle text
+ if (src.text && (clobber || !dest.text)) {
+ dest.text = src.text;
+ }
+ //Handle platform
+ if (platform) {
+ src.findall('platform[@name="' + platform + '"]').forEach(function (platformElement) {
+ platformElement.getchildren().forEach(mergeChild);
+ });
+ }
+
+ //Handle children
+ src.getchildren().forEach(mergeChild);
+
+ function mergeChild (srcChild) {
+ var srcTag = srcChild.tag,
+ destChild = new et.Element(srcTag),
+ foundChild,
+ query = srcTag + '',
+ shouldMerge = true;
+
+ if (BLACKLIST.indexOf(srcTag) === -1) {
+ if (SINGLETONS.indexOf(srcTag) !== -1) {
+ foundChild = dest.find(query);
+ if (foundChild) {
+ destChild = foundChild;
+ dest.remove(destChild);
+ }
+ } else {
+ //Check for an exact match and if you find one don't add
+ Object.getOwnPropertyNames(srcChild.attrib).forEach(function (attribute) {
+ query += '[@' + attribute + '="' + srcChild.attrib[attribute] + '"]';
+ });
+ var foundChildren = dest.findall(query);
+ for(var i = 0; i < foundChildren.length; i++) {
+ foundChild = foundChildren[i];
+ if (foundChild && textMatch(srcChild, foundChild) && (Object.keys(srcChild.attrib).length==Object.keys(foundChild.attrib).length)) {
+ destChild = foundChild;
+ dest.remove(destChild);
+ shouldMerge = false;
+ break;
+ }
+ }
+ }
+
+ mergeXml(srcChild, destChild, platform, clobber && shouldMerge);
+ dest.append(destChild);
+ }
+ }
+}
+
+// Expose for testing.
+module.exports.mergeXml = mergeXml;
+
+function textMatch(elm1, elm2) {
+ var text1 = elm1.text ? elm1.text.replace(/\s+/, '') : '',
+ text2 = elm2.text ? elm2.text.replace(/\s+/, '') : '';
+ return (text1 === '' || text1 === text2);
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-registry-mapper/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-registry-mapper/.npmignore b/node_modules/cordova-registry-mapper/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/cordova-registry-mapper/.npmignore
@@ -0,0 +1 @@
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-registry-mapper/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/cordova-registry-mapper/.travis.yml b/node_modules/cordova-registry-mapper/.travis.yml
new file mode 100644
index 0000000..ae381fc
--- /dev/null
+++ b/node_modules/cordova-registry-mapper/.travis.yml
@@ -0,0 +1,7 @@
+language: node_js
+sudo: false
+node_js:
+ - "0.10"
+install: npm install
+script:
+ - npm test
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-registry-mapper/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-registry-mapper/README.md b/node_modules/cordova-registry-mapper/README.md
new file mode 100644
index 0000000..3b93e5f
--- /dev/null
+++ b/node_modules/cordova-registry-mapper/README.md
@@ -0,0 +1,14 @@
+[](https://travis-ci.org/stevengill/cordova-registry-mapper)
+
+#Cordova Registry Mapper
+
+This module is used to map Cordova plugin ids to package names and vice versa.
+
+When Cordova users add plugins to their projects using ids
+(e.g. `cordova plugin add org.apache.cordova.device`),
+this module will map that id to the corresponding package name so `cordova-lib` knows what to fetch from **npm**.
+
+This module was created so the Apache Cordova project could migrate its plugins from
+the [Cordova Registry](http://registry.cordova.io/)
+to [npm](https://registry.npmjs.com/)
+instead of having to maintain a registry.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-registry-mapper/index.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-registry-mapper/index.js b/node_modules/cordova-registry-mapper/index.js
new file mode 100644
index 0000000..4550774
--- /dev/null
+++ b/node_modules/cordova-registry-mapper/index.js
@@ -0,0 +1,204 @@
+var map = {
+ 'org.apache.cordova.battery-status':'cordova-plugin-battery-status',
+ 'org.apache.cordova.camera':'cordova-plugin-camera',
+ 'org.apache.cordova.console':'cordova-plugin-console',
+ 'org.apache.cordova.contacts':'cordova-plugin-contacts',
+ 'org.apache.cordova.device':'cordova-plugin-device',
+ 'org.apache.cordova.device-motion':'cordova-plugin-device-motion',
+ 'org.apache.cordova.device-orientation':'cordova-plugin-device-orientation',
+ 'org.apache.cordova.dialogs':'cordova-plugin-dialogs',
+ 'org.apache.cordova.file':'cordova-plugin-file',
+ 'org.apache.cordova.file-transfer':'cordova-plugin-file-transfer',
+ 'org.apache.cordova.geolocation':'cordova-plugin-geolocation',
+ 'org.apache.cordova.globalization':'cordova-plugin-globalization',
+ 'org.apache.cordova.inappbrowser':'cordova-plugin-inappbrowser',
+ 'org.apache.cordova.media':'cordova-plugin-media',
+ 'org.apache.cordova.media-capture':'cordova-plugin-media-capture',
+ 'org.apache.cordova.network-information':'cordova-plugin-network-information',
+ 'org.apache.cordova.splashscreen':'cordova-plugin-splashscreen',
+ 'org.apache.cordova.statusbar':'cordova-plugin-statusbar',
+ 'org.apache.cordova.vibration':'cordova-plugin-vibration',
+ 'org.apache.cordova.test-framework':'cordova-plugin-test-framework',
+ 'com.msopentech.websql' : 'cordova-plugin-websql',
+ 'com.msopentech.indexeddb' : 'cordova-plugin-indexeddb',
+ 'com.microsoft.aad.adal' : 'cordova-plugin-ms-adal',
+ 'com.microsoft.capptain' : 'capptain-cordova',
+ 'com.microsoft.services.aadgraph' : 'cordova-plugin-ms-aad-graph',
+ 'com.microsoft.services.files' : 'cordova-plugin-ms-files',
+ 'om.microsoft.services.outlook' : 'cordova-plugin-ms-outlook',
+ 'com.pbakondy.sim' : 'cordova-plugin-sim',
+ 'android.support.v4' : 'cordova-plugin-android-support-v4',
+ 'android.support.v7-appcompat' : 'cordova-plugin-android-support-v7-appcompat',
+ 'com.google.playservices' : 'cordova-plugin-googleplayservices',
+ 'com.google.cordova.admob' : 'cordova-plugin-admobpro',
+ 'com.rjfun.cordova.extension' : 'cordova-plugin-extension',
+ 'com.rjfun.cordova.plugin.admob' : 'cordova-plugin-admob',
+ 'com.rjfun.cordova.flurryads' : 'cordova-plugin-flurry',
+ 'com.rjfun.cordova.facebookads' : 'cordova-plugin-facebookads',
+ 'com.rjfun.cordova.httpd' : 'cordova-plugin-httpd',
+ 'com.rjfun.cordova.iad' : 'cordova-plugin-iad',
+ 'com.rjfun.cordova.iflyspeech' : 'cordova-plugin-iflyspeech',
+ 'com.rjfun.cordova.lianlianpay' : 'cordova-plugin-lianlianpay',
+ 'com.rjfun.cordova.mobfox' : 'cordova-plugin-mobfox',
+ 'com.rjfun.cordova.mopub' : 'cordova-plugin-mopub',
+ 'com.rjfun.cordova.mmedia' : 'cordova-plugin-mmedia',
+ 'com.rjfun.cordova.nativeaudio' : 'cordova-plugin-nativeaudio',
+ 'com.rjfun.cordova.plugin.paypalmpl' : 'cordova-plugin-paypalmpl',
+ 'com.rjfun.cordova.smartadserver' : 'cordova-plugin-smartadserver',
+ 'com.rjfun.cordova.sms' : 'cordova-plugin-sms',
+ 'com.rjfun.cordova.wifi' : 'cordova-plugin-wifi',
+ 'com.ohh2ahh.plugins.appavailability' : 'cordova-plugin-appavailability',
+ 'org.adapt-it.cordova.fonts' : 'cordova-plugin-fonts',
+ 'de.martinreinhardt.cordova.plugins.barcodeScanner' : 'cordova-plugin-barcodescanner',
+ 'de.martinreinhardt.cordova.plugins.urlhandler' : 'cordova-plugin-urlhandler',
+ 'de.martinreinhardt.cordova.plugins.email' : 'cordova-plugin-email',
+ 'de.martinreinhardt.cordova.plugins.certificates' : 'cordova-plugin-certificates',
+ 'de.martinreinhardt.cordova.plugins.sqlite' : 'cordova-plugin-sqlite',
+ 'fr.smile.cordova.fileopener' : 'cordova-plugin-fileopener',
+ 'org.smile.websqldatabase.initializer' : 'cordova-plugin-websqldatabase-initializer',
+ 'org.smile.websqldatabase.wpdb' : 'cordova-plugin-websqldatabase',
+ 'org.jboss.aerogear.cordova.push' : 'aerogear-cordova-push',
+ 'org.jboss.aerogear.cordova.oauth2' : 'aerogear-cordova-oauth2',
+ 'org.jboss.aerogear.cordova.geo' : 'aerogear-cordova-geo',
+ 'org.jboss.aerogear.cordova.crypto' : 'aerogear-cordova-crypto',
+ 'org.jboss.aerogaer.cordova.otp' : 'aerogear-cordova-otp',
+ 'uk.co.ilee.applewatch' : 'cordova-plugin-apple-watch',
+ 'uk.co.ilee.directions' : 'cordova-plugin-directions',
+ 'uk.co.ilee.gamecenter' : 'cordova-plugin-game-center',
+ 'uk.co.ilee.jailbreakdetection' : 'cordova-plugin-jailbreak-detection',
+ 'uk.co.ilee.nativetransitions' : 'cordova-plugin-native-transitions',
+ 'uk.co.ilee.pedometer' : 'cordova-plugin-pedometer',
+ 'uk.co.ilee.shake' : 'cordova-plugin-shake',
+ 'uk.co.ilee.touchid' : 'cordova-plugin-touchid',
+ 'com.knowledgecode.cordova.websocket' : 'cordova-plugin-websocket',
+ 'com.elixel.plugins.settings' : 'cordova-plugin-settings',
+ 'com.cowbell.cordova.geofence' : 'cordova-plugin-geofence',
+ 'com.blackberry.community.preventsleep' : 'cordova-plugin-preventsleep',
+ 'com.blackberry.community.gamepad' : 'cordova-plugin-gamepad',
+ 'com.blackberry.community.led' : 'cordova-plugin-led',
+ 'com.blackberry.community.thumbnail' : 'cordova-plugin-thumbnail',
+ 'com.blackberry.community.mediakeys' : 'cordova-plugin-mediakeys',
+ 'com.blackberry.community.simplebtlehrplugin' : 'cordova-plugin-bluetoothheartmonitor',
+ 'com.blackberry.community.simplebeaconplugin' : 'cordova-plugin-bluetoothibeacon',
+ 'com.blackberry.community.simplebtsppplugin' : 'cordova-plugin-bluetoothspp',
+ 'com.blackberry.community.clipboard' : 'cordova-plugin-clipboard',
+ 'com.blackberry.community.curl' : 'cordova-plugin-curl',
+ 'com.blackberry.community.qt' : 'cordova-plugin-qtbridge',
+ 'com.blackberry.community.upnp' : 'cordova-plugin-upnp',
+ 'com.blackberry.community.PasswordCrypto' : 'cordova-plugin-password-crypto',
+ 'com.blackberry.community.deviceinfoplugin' : 'cordova-plugin-deviceinfo',
+ 'com.blackberry.community.gsecrypto' : 'cordova-plugin-bb-crypto',
+ 'com.blackberry.community.mongoose' : 'cordova-plugin-mongoose',
+ 'com.blackberry.community.sysdialog' : 'cordova-plugin-bb-sysdialog',
+ 'com.blackberry.community.screendisplay' : 'cordova-plugin-screendisplay',
+ 'com.blackberry.community.messageplugin' : 'cordova-plugin-bb-messageretrieve',
+ 'com.blackberry.community.emailsenderplugin' : 'cordova-plugin-emailsender',
+ 'com.blackberry.community.audiometadata' : 'cordova-plugin-audiometadata',
+ 'com.blackberry.community.deviceemails' : 'cordova-plugin-deviceemails',
+ 'com.blackberry.community.audiorecorder' : 'cordova-plugin-audiorecorder',
+ 'com.blackberry.community.vibration' : 'cordova-plugin-vibrate-intense',
+ 'com.blackberry.community.SMSPlugin' : 'cordova-plugin-bb-sms',
+ 'com.blackberry.community.extractZipFile' : 'cordova-plugin-bb-zip',
+ 'com.blackberry.community.lowlatencyaudio' : 'cordova-plugin-bb-nativeaudio',
+ 'com.blackberry.community.barcodescanner' : 'phonegap-plugin-barcodescanner',
+ 'com.blackberry.app' : 'cordova-plugin-bb-app',
+ 'com.blackberry.bbm.platform' : 'cordova-plugin-bbm',
+ 'com.blackberry.connection' : 'cordova-plugin-bb-connection',
+ 'com.blackberry.identity' : 'cordova-plugin-bb-identity',
+ 'com.blackberry.invoke.card' : 'cordova-plugin-bb-card',
+ 'com.blackberry.invoke' : 'cordova-plugin-bb-invoke',
+ 'com.blackberry.invoked' : 'cordova-plugin-bb-invoked',
+ 'com.blackberry.io.filetransfer' : 'cordova-plugin-bb-filetransfer',
+ 'com.blackberry.io' : 'cordova-plugin-bb-io',
+ 'com.blackberry.notification' : 'cordova-plugin-bb-notification',
+ 'com.blackberry.payment' : 'cordova-plugin-bb-payment',
+ 'com.blackberry.pim.calendar' : 'cordova-plugin-bb-calendar',
+ 'com.blackberry.pim.contacts' : 'cordova-plugin-bb-contacts',
+ 'com.blackberry.pim.lib' : 'cordova-plugin-bb-pimlib',
+ 'com.blackberry.push' : 'cordova-plugin-bb-push',
+ 'com.blackberry.screenshot' : 'cordova-plugin-screenshot',
+ 'com.blackberry.sensors' : 'cordova-plugin-bb-sensors',
+ 'com.blackberry.system' : 'cordova-plugin-bb-system',
+ 'com.blackberry.ui.contextmenu' : 'cordova-plugin-bb-ctxmenu',
+ 'com.blackberry.ui.cover' : 'cordova-plugin-bb-cover',
+ 'com.blackberry.ui.dialog' : 'cordova-plugin-bb-dialog',
+ 'com.blackberry.ui.input' : 'cordova-plugin-touch-keyboard',
+ 'com.blackberry.ui.toast' : 'cordova-plugin-toast',
+ 'com.blackberry.user.identity' : 'cordova-plugin-bb-idservice',
+ 'com.blackberry.utils' : 'cordova-plugin-bb-utils',
+ 'net.yoik.cordova.plugins.screenorientation' : 'cordova-plugin-screen-orientation',
+ 'com.phonegap.plugins.barcodescanner' : 'phonegap-plugin-barcodescanner',
+ 'com.manifoldjs.hostedwebapp' : 'cordova-plugin-hostedwebapp',
+ 'com.initialxy.cordova.themeablebrowser' : 'cordova-plugin-themeablebrowser',
+ 'gr.denton.photosphere' : 'cordova-plugin-panoramaviewer',
+ 'nl.x-services.plugins.actionsheet' : 'cordova-plugin-actionsheet',
+ 'nl.x-services.plugins.socialsharing' : 'cordova-plugin-x-socialsharing',
+ 'nl.x-services.plugins.googleplus' : 'cordova-plugin-googleplus',
+ 'nl.x-services.plugins.insomnia' : 'cordova-plugin-insomnia',
+ 'nl.x-services.plugins.toast' : 'cordova-plugin-x-toast',
+ 'nl.x-services.plugins.calendar' : 'cordova-plugin-calendar',
+ 'nl.x-services.plugins.launchmyapp' : 'cordova-plugin-customurlscheme',
+ 'nl.x-services.plugins.flashlight' : 'cordova-plugin-flashlight',
+ 'nl.x-services.plugins.sslcertificatechecker' : 'cordova-plugin-sslcertificatechecker',
+ 'com.bridge.open' : 'cordova-open',
+ 'com.bridge.safe' : 'cordova-safe',
+ 'com.disusered.open' : 'cordova-open',
+ 'com.disusered.safe' : 'cordova-safe',
+ 'me.apla.cordova.app-preferences' : 'cordova-plugin-app-preferences',
+ 'com.konotor.cordova' : 'cordova-plugin-konotor',
+ 'io.intercom.cordova' : 'cordova-plugin-intercom',
+ 'com.onesignal.plugins.onesignal' : 'onesignal-cordova-plugin',
+ 'com.danjarvis.document-contract': 'cordova-plugin-document-contract',
+ 'com.eface2face.iosrtc' : 'cordova-plugin-iosrtc',
+ 'com.mobileapptracking.matplugin' : 'cordova-plugin-tune',
+ 'com.marianhello.cordova.background-geolocation' : 'cordova-plugin-mauron85-background-geolocation',
+ 'fr.louisbl.cordova.locationservices' : 'cordova-plugin-locationservices',
+ 'fr.louisbl.cordova.gpslocation' : 'cordova-plugin-gpslocation',
+ 'com.hiliaox.weibo' : 'cordova-plugin-weibo',
+ 'com.uxcam.cordova.plugin' : 'cordova-uxcam',
+ 'de.fastr.phonegap.plugins.downloader' : 'cordova-plugin-fastrde-downloader',
+ 'de.fastr.phonegap.plugins.injectView' : 'cordova-plugin-fastrde-injectview',
+ 'de.fastr.phonegap.plugins.CheckGPS' : 'cordova-plugin-fastrde-checkgps',
+ 'de.fastr.phonegap.plugins.md5chksum' : 'cordova-plugin-fastrde-md5',
+ 'io.repro.cordova' : 'cordova-plugin-repro',
+ 're.notifica.cordova': 'cordova-plugin-notificare-push',
+ 'com.megster.cordova.ble': 'cordova-plugin-ble-central',
+ 'com.megster.cordova.bluetoothserial': 'cordova-plugin-bluetooth-serial',
+ 'com.megster.cordova.rfduino': 'cordova-plugin-rfduino',
+ 'cz.velda.cordova.plugin.devicefeedback': 'cordova-plugin-velda-devicefeedback',
+ 'cz.Velda.cordova.plugin.devicefeedback': 'cordova-plugin-velda-devicefeedback',
+ 'org.scriptotek.appinfo': 'cordova-plugin-appinfo',
+ 'com.yezhiming.cordova.appinfo': 'cordova-plugin-appinfo',
+ 'pl.makingwaves.estimotebeacons': 'cordova-plugin-estimote',
+ 'com.evothings.ble': 'cordova-plugin-ble',
+ 'com.appsee.plugin' : 'cordova-plugin-appsee',
+ 'am.armsoft.plugins.listpicker': 'cordova-plugin-listpicker',
+ 'com.pushbots.push': 'pushbots-cordova-plugin',
+ 'com.admob.google': 'cordova-admob',
+ 'admob.ads.google': 'cordova-admob-ads',
+ 'admob.google.plugin': 'admob-google',
+ 'com.admob.admobads': 'admob-ads',
+ 'com.connectivity.monitor': 'cordova-connectivity-monitor',
+ 'com.ios.libgoogleadmobads': 'cordova-libgoogleadmobads',
+ 'com.google.play.services': 'cordova-google-play-services',
+ 'android.support.v13': 'cordova-android-support-v13',
+ 'android.support.v4': 'cordova-android-support-v4', // Duplicated key ;)
+ 'com.analytics.google': 'cordova-plugin-analytics',
+ 'com.analytics.adid.google': 'cordova-plugin-analytics-adid',
+ 'com.chariotsolutions.nfc.plugin': 'phonegap-nfc',
+ 'com.samz.mixpanel': 'cordova-plugin-mixpanel',
+ 'de.appplant.cordova.common.RegisterUserNotificationSettings': 'cordova-plugin-registerusernotificationsettings',
+ 'plugin.google.maps': 'cordova-plugin-googlemaps',
+ 'xu.li.cordova.wechat': 'cordova-plugin-wechat',
+ 'es.keensoft.fullscreenimage': 'cordova-plugin-fullscreenimage',
+ 'com.arcoirislabs.plugin.mqtt' : 'cordova-plugin-mqtt'
+};
+
+module.exports.oldToNew = map;
+
+var reverseMap = {};
+Object.keys(map).forEach(function(elem){
+ reverseMap[map[elem]] = elem;
+});
+
+module.exports.newToOld = reverseMap;
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-registry-mapper/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-registry-mapper/package.json b/node_modules/cordova-registry-mapper/package.json
new file mode 100644
index 0000000..e27b292
--- /dev/null
+++ b/node_modules/cordova-registry-mapper/package.json
@@ -0,0 +1,77 @@
+{
+ "_args": [
+ [
+ "cordova-registry-mapper@^1.1.8",
+ "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common"
+ ]
+ ],
+ "_from": "cordova-registry-mapper@>=1.1.8 <2.0.0",
+ "_id": "cordova-registry-mapper@1.1.15",
+ "_inCache": true,
+ "_installable": true,
+ "_location": "/cordova-registry-mapper",
+ "_nodeVersion": "5.4.1",
+ "_npmUser": {
+ "email": "stevengill97@gmail.com",
+ "name": "stevegill"
+ },
+ "_npmVersion": "3.5.3",
+ "_phantomChildren": {},
+ "_requested": {
+ "name": "cordova-registry-mapper",
+ "raw": "cordova-registry-mapper@^1.1.8",
+ "rawSpec": "^1.1.8",
+ "scope": null,
+ "spec": ">=1.1.8 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/cordova-common"
+ ],
+ "_resolved": "http://registry.npmjs.org/cordova-registry-mapper/-/cordova-registry-mapper-1.1.15.tgz",
+ "_shasum": "e244b9185b8175473bff6079324905115f83dc7c",
+ "_shrinkwrap": null,
+ "_spec": "cordova-registry-mapper@^1.1.8",
+ "_where": "/Users/steveng/repo/cordova/cordova-osx/node_modules/cordova-common",
+ "author": {
+ "name": "Steve Gill"
+ },
+ "bugs": {
+ "url": "https://github.com/stevengill/cordova-registry-mapper/issues"
+ },
+ "dependencies": {},
+ "description": "Maps old plugin ids to new plugin names for fetching from npm",
+ "devDependencies": {
+ "tape": "^3.5.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "e244b9185b8175473bff6079324905115f83dc7c",
+ "tarball": "http://registry.npmjs.org/cordova-registry-mapper/-/cordova-registry-mapper-1.1.15.tgz"
+ },
+ "gitHead": "00af0f028ec94154a364eeabe38b8e22320647bd",
+ "homepage": "https://github.com/stevengill/cordova-registry-mapper#readme",
+ "keywords": [
+ "cordova",
+ "plugins"
+ ],
+ "license": "Apache version 2.0",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "stevegill",
+ "email": "stevengill97@gmail.com"
+ }
+ ],
+ "name": "cordova-registry-mapper",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/stevengill/cordova-registry-mapper.git"
+ },
+ "scripts": {
+ "test": "node tests/test.js"
+ },
+ "version": "1.1.15"
+}
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/cordova-registry-mapper/tests/test.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-registry-mapper/tests/test.js b/node_modules/cordova-registry-mapper/tests/test.js
new file mode 100644
index 0000000..35343be
--- /dev/null
+++ b/node_modules/cordova-registry-mapper/tests/test.js
@@ -0,0 +1,11 @@
+var test = require('tape');
+var oldToNew = require('../index').oldToNew;
+var newToOld = require('../index').newToOld;
+
+test('plugin mappings exist', function(t) {
+ t.plan(2);
+
+ t.equal('cordova-plugin-device', oldToNew['org.apache.cordova.device']);
+
+ t.equal('org.apache.cordova.device', newToOld['cordova-plugin-device']);
+})
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/.npmignore b/node_modules/elementtree/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/elementtree/.npmignore
@@ -0,0 +1 @@
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/.travis.yml b/node_modules/elementtree/.travis.yml
new file mode 100644
index 0000000..6f27c96
--- /dev/null
+++ b/node_modules/elementtree/.travis.yml
@@ -0,0 +1,10 @@
+language: node_js
+
+node_js:
+ - 0.6
+
+script: make test
+
+notifications:
+ email:
+ - tomaz+travisci@tomaz.me
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/CHANGES.md
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/CHANGES.md b/node_modules/elementtree/CHANGES.md
new file mode 100644
index 0000000..50d415d
--- /dev/null
+++ b/node_modules/elementtree/CHANGES.md
@@ -0,0 +1,39 @@
+elementtree v0.1.6 (in development)
+
+* Add support for CData elements. (#14)
+ [hermannpencole]
+
+elementtree v0.1.5 - 2012-11-14
+
+* Fix a bug in the find() and findtext() method which could manifest itself
+ under some conditions.
+ [metagriffin]
+
+elementtree v0.1.4 - 2012-10-15
+
+* Allow user to use namespaced attributes when using find* functions.
+ [Andrew Lunny]
+
+elementtree v0.1.3 - 2012-09-21
+
+* Improve the output of text content in the tags (strip unnecessary line break
+ characters).
+
+[Darryl Pogue]
+
+elementtree v0.1.2 - 2012-09-04
+
+ * Allow user to pass 'indent' option to ElementTree.write method. If this
+ option is specified (e.g. {'indent': 4}). XML will be pretty printed.
+ [Darryl Pogue, Tomaz Muraus]
+
+ * Bump sax dependency version.
+
+elementtree v0.1.1 - 2011-09-23
+
+ * Improve special character escaping.
+ [Ryan Phillips]
+
+elementtree v0.1.0 - 2011-09-05
+
+ * Initial release.
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/LICENSE.txt
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/LICENSE.txt b/node_modules/elementtree/LICENSE.txt
new file mode 100644
index 0000000..6b0b127
--- /dev/null
+++ b/node_modules/elementtree/LICENSE.txt
@@ -0,0 +1,203 @@
+
+ Apache License
+ Version 2.0, January 2004
+ http://www.apache.org/licenses/
+
+ TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
+
+ 1. Definitions.
+
+ "License" shall mean the terms and conditions for use, reproduction,
+ and distribution as defined by Sections 1 through 9 of this document.
+
+ "Licensor" shall mean the copyright owner or entity authorized by
+ the copyright owner that is granting the License.
+
+ "Legal Entity" shall mean the union of the acting entity and all
+ other entities that control, are controlled by, or are under common
+ control with that entity. For the purposes of this definition,
+ "control" means (i) the power, direct or indirect, to cause the
+ direction or management of such entity, whether by contract or
+ otherwise, or (ii) ownership of fifty percent (50%) or more of the
+ outstanding shares, or (iii) beneficial ownership of such entity.
+
+ "You" (or "Your") shall mean an individual or Legal Entity
+ exercising permissions granted by this License.
+
+ "Source" form shall mean the preferred form for making modifications,
+ including but not limited to software source code, documentation
+ source, and configuration files.
+
+ "Object" form shall mean any form resulting from mechanical
+ transformation or translation of a Source form, including but
+ not limited to compiled object code, generated documentation,
+ and conversions to other media types.
+
+ "Work" shall mean the work of authorship, whether in Source or
+ Object form, made available under the License, as indicated by a
+ copyright notice that is included in or attached to the work
+ (an example is provided in the Appendix below).
+
+ "Derivative Works" shall mean any work, whether in Source or Object
+ form, that is based on (or derived from) the Work and for which the
+ editorial revisions, annotations, elaborations, or other modifications
+ represent, as a whole, an original work of authorship. For the purposes
+ of this License, Derivative Works shall not include works that remain
+ separable from, or merely link (or bind by name) to the interfaces of,
+ the Work and Derivative Works thereof.
+
+ "Contribution" shall mean any work of authorship, including
+ the original version of the Work and any modifications or additions
+ to that Work or Derivative Works thereof, that is intentionally
+ submitted to Licensor for inclusion in the Work by the copyright owner
+ or by an individual or Legal Entity authorized to submit on behalf of
+ the copyright owner. For the purposes of this definition, "submitted"
+ means any form of electronic, verbal, or written communication sent
+ to the Licensor or its representatives, including but not limited to
+ communication on electronic mailing lists, source code control systems,
+ and issue tracking systems that are managed by, or on behalf of, the
+ Licensor for the purpose of discussing and improving the Work, but
+ excluding communication that is conspicuously marked or otherwise
+ designated in writing by the copyright owner as "Not a Contribution."
+
+ "Contributor" shall mean Licensor and any individual or Legal Entity
+ on behalf of whom a Contribution has been received by Licensor and
+ subsequently incorporated within the Work.
+
+ 2. Grant of Copyright License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ copyright license to reproduce, prepare Derivative Works of,
+ publicly display, publicly perform, sublicense, and distribute the
+ Work and such Derivative Works in Source or Object form.
+
+ 3. Grant of Patent License. Subject to the terms and conditions of
+ this License, each Contributor hereby grants to You a perpetual,
+ worldwide, non-exclusive, no-charge, royalty-free, irrevocable
+ (except as stated in this section) patent license to make, have made,
+ use, offer to sell, sell, import, and otherwise transfer the Work,
+ where such license applies only to those patent claims licensable
+ by such Contributor that are necessarily infringed by their
+ Contribution(s) alone or by combination of their Contribution(s)
+ with the Work to which such Contribution(s) was submitted. If You
+ institute patent litigation against any entity (including a
+ cross-claim or counterclaim in a lawsuit) alleging that the Work
+ or a Contribution incorporated within the Work constitutes direct
+ or contributory patent infringement, then any patent licenses
+ granted to You under this License for that Work shall terminate
+ as of the date such litigation is filed.
+
+ 4. Redistribution. You may reproduce and distribute copies of the
+ Work or Derivative Works thereof in any medium, with or without
+ modifications, and in Source or Object form, provided that You
+ meet the following conditions:
+
+ (a) You must give any other recipients of the Work or
+ Derivative Works a copy of this License; and
+
+ (b) You must cause any modified files to carry prominent notices
+ stating that You changed the files; and
+
+ (c) You must retain, in the Source form of any Derivative Works
+ that You distribute, all copyright, patent, trademark, and
+ attribution notices from the Source form of the Work,
+ excluding those notices that do not pertain to any part of
+ the Derivative Works; and
+
+ (d) If the Work includes a "NOTICE" text file as part of its
+ distribution, then any Derivative Works that You distribute must
+ include a readable copy of the attribution notices contained
+ within such NOTICE file, excluding those notices that do not
+ pertain to any part of the Derivative Works, in at least one
+ of the following places: within a NOTICE text file distributed
+ as part of the Derivative Works; within the Source form or
+ documentation, if provided along with the Derivative Works; or,
+ within a display generated by the Derivative Works, if and
+ wherever such third-party notices normally appear. The contents
+ of the NOTICE file are for informational purposes only and
+ do not modify the License. You may add Your own attribution
+ notices within Derivative Works that You distribute, alongside
+ or as an addendum to the NOTICE text from the Work, provided
+ that such additional attribution notices cannot be construed
+ as modifying the License.
+
+ You may add Your own copyright statement to Your modifications and
+ may provide additional or different license terms and conditions
+ for use, reproduction, or distribution of Your modifications, or
+ for any such Derivative Works as a whole, provided Your use,
+ reproduction, and distribution of the Work otherwise complies with
+ the conditions stated in this License.
+
+ 5. Submission of Contributions. Unless You explicitly state otherwise,
+ any Contribution intentionally submitted for inclusion in the Work
+ by You to the Licensor shall be under the terms and conditions of
+ this License, without any additional terms or conditions.
+ Notwithstanding the above, nothing herein shall supersede or modify
+ the terms of any separate license agreement you may have executed
+ with Licensor regarding such Contributions.
+
+ 6. Trademarks. This License does not grant permission to use the trade
+ names, trademarks, service marks, or product names of the Licensor,
+ except as required for reasonable and customary use in describing the
+ origin of the Work and reproducing the content of the NOTICE file.
+
+ 7. Disclaimer of Warranty. Unless required by applicable law or
+ agreed to in writing, Licensor provides the Work (and each
+ Contributor provides its Contributions) on an "AS IS" BASIS,
+ WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
+ implied, including, without limitation, any warranties or conditions
+ of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
+ PARTICULAR PURPOSE. You are solely responsible for determining the
+ appropriateness of using or redistributing the Work and assume any
+ risks associated with Your exercise of permissions under this License.
+
+ 8. Limitation of Liability. In no event and under no legal theory,
+ whether in tort (including negligence), contract, or otherwise,
+ unless required by applicable law (such as deliberate and grossly
+ negligent acts) or agreed to in writing, shall any Contributor be
+ liable to You for damages, including any direct, indirect, special,
+ incidental, or consequential damages of any character arising as a
+ result of this License or out of the use or inability to use the
+ Work (including but not limited to damages for loss of goodwill,
+ work stoppage, computer failure or malfunction, or any and all
+ other commercial damages or losses), even if such Contributor
+ has been advised of the possibility of such damages.
+
+ 9. Accepting Warranty or Additional Liability. While redistributing
+ the Work or Derivative Works thereof, You may choose to offer,
+ and charge a fee for, acceptance of support, warranty, indemnity,
+ or other liability obligations and/or rights consistent with this
+ License. However, in accepting such obligations, You may act only
+ on Your own behalf and on Your sole responsibility, not on behalf
+ of any other Contributor, and only if You agree to indemnify,
+ defend, and hold each Contributor harmless for any liability
+ incurred by, or claims asserted against, such Contributor by reason
+ of your accepting any such warranty or additional liability.
+
+ END OF TERMS AND CONDITIONS
+
+ APPENDIX: How to apply the Apache License to your work.
+
+ To apply the Apache License to your work, attach the following
+ boilerplate notice, with the fields enclosed by brackets "[]"
+ replaced with your own identifying information. (Don't include
+ the brackets!) The text should be enclosed in the appropriate
+ comment syntax for the file format. We also recommend that a
+ file or class name and description of purpose be included on the
+ same "printed page" as the copyright notice for easier
+ identification within third-party archives.
+
+ Copyright [yyyy] [name of copyright owner]
+
+ Licensed under the Apache License, Version 2.0 (the "License");
+ you may not use this file except in compliance with the License.
+ You may obtain a copy of the License at
+
+ http://www.apache.org/licenses/LICENSE-2.0
+
+ Unless required by applicable law or agreed to in writing, software
+ distributed under the License is distributed on an "AS IS" BASIS,
+ WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+ See the License for the specific language governing permissions and
+ limitations under the License.
+
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/Makefile
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/Makefile b/node_modules/elementtree/Makefile
new file mode 100755
index 0000000..ab7c4e0
--- /dev/null
+++ b/node_modules/elementtree/Makefile
@@ -0,0 +1,21 @@
+TESTS := \
+ tests/test-simple.js
+
+
+
+PATH := ./node_modules/.bin:$(PATH)
+
+WHISKEY := $(shell bash -c 'PATH=$(PATH) type -p whiskey')
+
+default: test
+
+test:
+ NODE_PATH=`pwd`/lib/ ${WHISKEY} --scope-leaks --sequential --real-time --tests "${TESTS}"
+
+tap:
+ NODE_PATH=`pwd`/lib/ ${WHISKEY} --test-reporter tap --sequential --real-time --tests "${TESTS}"
+
+coverage:
+ NODE_PATH=`pwd`/lib/ ${WHISKEY} --sequential --coverage --coverage-reporter html --coverage-dir coverage_html --tests "${TESTS}"
+
+.PHONY: default test coverage tap scope
http://git-wip-us.apache.org/repos/asf/cordova-osx/blob/42cbe9fd/node_modules/elementtree/NOTICE
----------------------------------------------------------------------
diff --git a/node_modules/elementtree/NOTICE b/node_modules/elementtree/NOTICE
new file mode 100644
index 0000000..28ad70a
--- /dev/null
+++ b/node_modules/elementtree/NOTICE
@@ -0,0 +1,5 @@
+node-elementtree
+Copyright (c) 2011, Rackspace, Inc.
+
+The ElementTree toolkit is Copyright (c) 1999-2007 by Fredrik Lundh
+
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org