You are viewing a plain text version of this content. The canonical link for it is here.
Posted to notifications@apisix.apache.org by GitBox <gi...@apache.org> on 2020/11/10 03:43:51 UTC
[GitHub] [apisix] tokers opened a new pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
tokers opened a new pull request #2685:
URL: https://github.com/apache/apisix/pull/2685
Signed-off-by: Alex Zhang <zc...@gmail.com>
### What this PR does / why we need it:
<!--- Why is this change required? What problem does it solve? -->
<!--- If it fixes an open issue, please link to the issue here. -->
Move the etcd initialization into `apisix/cli/etcd.lua`, some auxiliary functions were moved to `apisix/cli/etcd.lua` and the yaml configuration file parsing function was moved to `apisix/cli/file.lua`.
### Pre-submission checklist:
* [x] Did you explain what problem does this PR solve? Or what new features have been added?
* [ ] Have you added corresponding test cases?
* [ ] Have you modified the corresponding document?
* [x] Is this PR backward compatible?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#issuecomment-732794784
@spacewander Resolved.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r527480414
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
Review comment:
@membphis Fixed.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525649569
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ --convert old single etcd config to multiple etcd config
Review comment:
Nice capture.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] spacewander merged pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
spacewander merged pull request #2685:
URL: https://github.com/apache/apisix/pull/2685
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#issuecomment-729346485
@membphis Rebased.
@spacewander Changes about configuration vars was moved to `apisix/cli/file.lua`, please help me to check whether any faults brought in due to this rebasing.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] Yiyiyimu commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
Yiyiyimu commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525773360
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
+ local cmd = "curl " .. uri .. " -X POST -d '" .. post_json
+ .. "' --connect-timeout " .. timeout
+ .. " --max-time " .. timeout * 2 .. " --retry 1 2>&1"
+
+ local res = util.execute_cmd(cmd)
+ if res:find("OK", 1, true) then
Review comment:
I can't reproduce why I did so. The original code before upgrading to etcd v3 for implementing this is
https://github.com/apache/apisix/blob/c54aec8f6cec2bd31c7c8717bf5220327584be03/bin/apisix#L899-L907
Maybe that could be of help
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525753350
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
+ local cmd = "curl " .. uri .. " -X POST -d '" .. post_json
+ .. "' --connect-timeout " .. timeout
+ .. " --max-time " .. timeout * 2 .. " --retry 1 2>&1"
+
+ local res = util.execute_cmd(cmd)
+ if res:find("OK", 1, true) then
Review comment:
Me too... @Yiyiyimu
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#issuecomment-731061041
@membphis Need your review again since more changes are introduced. :)
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525927117
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
+ local cmd = "curl " .. uri .. " -X POST -d '" .. post_json
+ .. "' --connect-timeout " .. timeout
+ .. " --max-time " .. timeout * 2 .. " --retry 1 2>&1"
+
+ local res = util.execute_cmd(cmd)
+ if res:find("OK", 1, true) then
Review comment:
Let me just adjust it.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] membphis commented on pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
membphis commented on pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#issuecomment-728946893
haha, @tokers you need to rebase your branch
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525753976
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
+ local cmd = "curl " .. uri .. " -X POST -d '" .. post_json
+ .. "' --connect-timeout " .. timeout
+ .. " --max-time " .. timeout * 2 .. " --retry 1 2>&1"
+
+ local res = util.execute_cmd(cmd)
+ if res:find("OK", 1, true) then
Review comment:
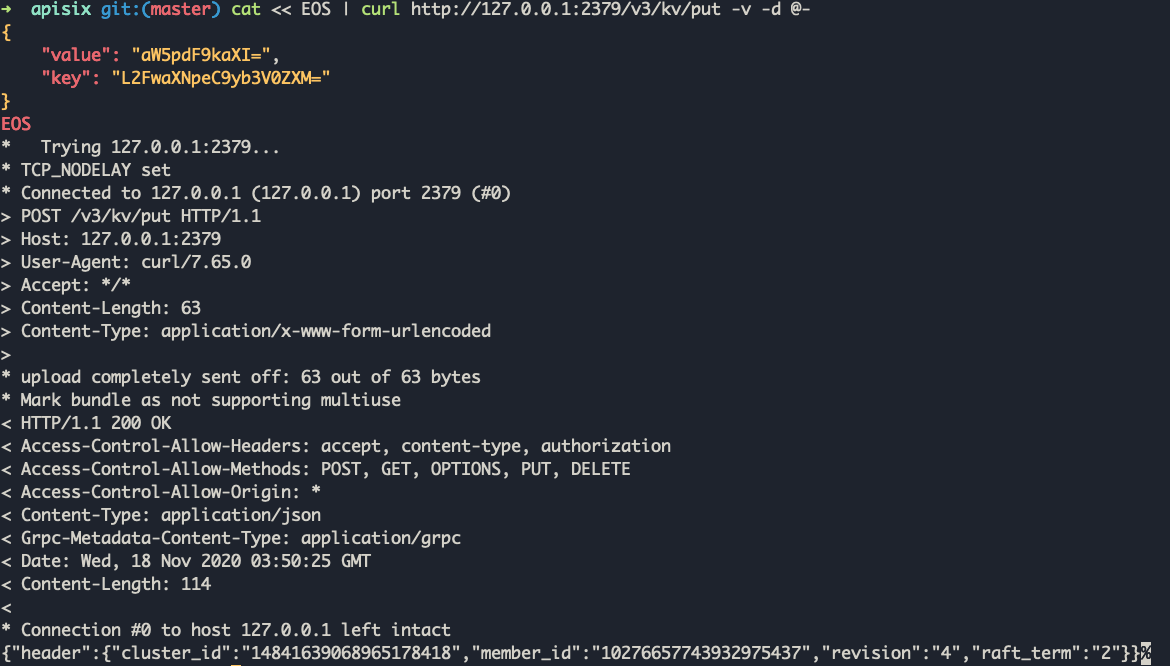
The output should like this.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525940135
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
+ local cmd = "curl " .. uri .. " -X POST -d '" .. post_json
+ .. "' --connect-timeout " .. timeout
+ .. " --max-time " .. timeout * 2 .. " --retry 1 2>&1"
+
+ local res = util.execute_cmd(cmd)
+ if res:find("OK", 1, true) then
Review comment:
Fixed
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525696266
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ --convert old single etcd config to multiple etcd config
Review comment:
Updated.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] Firstsawyou commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
Firstsawyou commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525646617
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ --convert old single etcd config to multiple etcd config
Review comment:
It would be better to add a space between the comment symbol and the description. ^-^
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] spacewander commented on pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
spacewander commented on pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#issuecomment-732751288
@tokers
Need to solve the conflict.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] membphis commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
membphis commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r526212475
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
Review comment:
style: add a space after `..`
##########
File path: apisix/cli/file.lua
##########
@@ -0,0 +1,144 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local yaml = require("tinyyaml")
+local profile = require("apisix.core.profile")
+local util = require("apisix.cli.util")
+
+local pairs = pairs
+local type = type
+local tonumber = tonumber
+local getenv = os.getenv
+local str_gmatch = string.gmatch
+local str_find = string.find
+
+local _M = {}
+
+
+local function is_empty_yaml_line(line)
+ return line == '' or str_find(line, '^%s*$') or str_find(line, '^%s*#')
+end
+
+
+local function tab_is_array(t)
+ local count = 0
+ for k,v in pairs(t) do
Review comment:
style: `k,v` -> `k, v`
##########
File path: apisix/cli/file.lua
##########
@@ -0,0 +1,144 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local yaml = require("tinyyaml")
+local profile = require("apisix.core.profile")
+local util = require("apisix.cli.util")
+
+local pairs = pairs
+local type = type
+local tonumber = tonumber
+local getenv = os.getenv
+local str_gmatch = string.gmatch
+local str_find = string.find
+
+local _M = {}
+
+
+local function is_empty_yaml_line(line)
+ return line == '' or str_find(line, '^%s*$') or str_find(line, '^%s*#')
+end
+
+
+local function tab_is_array(t)
+ local count = 0
+ for k,v in pairs(t) do
+ count = count + 1
+ end
+
+ return #t == count
+end
+
+
+local function resolve_conf_var(conf)
+ for key, val in pairs(conf) do
+ if type(val) == "table" then
+ resolve_conf_var(val)
+ elseif type(val) == "string" then
Review comment:
style: add a new blank line before this line
##########
File path: apisix/cli/file.lua
##########
@@ -0,0 +1,144 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local yaml = require("tinyyaml")
+local profile = require("apisix.core.profile")
+local util = require("apisix.cli.util")
+
+local pairs = pairs
+local type = type
+local tonumber = tonumber
+local getenv = os.getenv
+local str_gmatch = string.gmatch
+local str_find = string.find
+
+local _M = {}
+
+
+local function is_empty_yaml_line(line)
+ return line == '' or str_find(line, '^%s*$') or str_find(line, '^%s*#')
+end
+
+
+local function tab_is_array(t)
+ local count = 0
+ for k,v in pairs(t) do
+ count = count + 1
+ end
+
+ return #t == count
+end
+
+
+local function resolve_conf_var(conf)
Review comment:
I think `resolve_conf_env_var` is a better name. what do you think @spacewander ?
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] tokers commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
tokers commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525755333
##########
File path: apisix/cli/file.lua
##########
@@ -0,0 +1,146 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local yaml = require("tinyyaml")
+local profile = require("apisix.core.profile")
+local util = require("apisix.cli.util")
+
+local pairs = pairs
+local type = type
+local tonumber = tonumber
+local getenv = os.getenv
+local str_gmatch = string.gmatch
+local str_find = string.find
+
+local _M = {}
+
+
+local function is_empty_yaml_line(line)
+ return line == '' or str_find(line, '^%s*$') or str_find(line, '^%s*#')
+end
+
+
+local function tab_is_array(t)
+ local count = 0
+ for k,v in pairs(t) do
+ count = count + 1
+ end
+
+ return #t == count
+end
+
+
+local function resolve_conf_var(conf)
+ for key, val in pairs(conf) do
+ if type(val) == "table" then
+ resolve_conf_var(val)
+ elseif type(val) == "string" then
+ local var_used = false
+ -- we use '${{var}}' because '$var' and '${var}' are taken
+ -- by Nginx
+ local new_val = val:gsub("%$%{%{([%w_]+)%}%}", function(var)
+ local v = getenv(var)
+ if v then
+ var_used = true
+ return v
+ end
+
+ util.die("failed to handle configuration: ",
+ "can't find environment variable ",
+ var, "\n")
+ end)
+
+ if var_used then
+ if tonumber(new_val) ~= nil then
+ new_val = tonumber(new_val)
+ elseif new_val == "true" then
+ new_val = true
+ elseif new_val == "false" then
+ new_val = false
+ end
+ end
+
+ conf[key] = new_val
+ end
+ end
+end
+
+
+local function merge_conf(base, new_tab)
+ for key, val in pairs(new_tab) do
+ if type(val) == "table" then
+ if tab_is_array(val) then
+ base[key] = val
+ elseif base[key] == nil then
+ base[key] = val
+ else
+ merge_conf(base[key], val)
+ end
+
+ else
+ base[key] = val
+ end
+ end
+
+ return base
+end
+
+
+function _M.read_yaml_conf(apisix_home)
+ profile.apisix_home = apisix_home .. "/"
+ local local_conf_path = profile:yaml_path("config-default")
+ local default_conf_yaml, err = util.read_file(local_conf_path)
+ if not default_conf_yaml then
+ return nil, err
+ end
+
+ local default_conf = yaml.parse(default_conf_yaml)
+ if not default_conf then
+ return nil, "invalid config-default.yaml file"
+ end
+
+ local_conf_path = profile:yaml_path("config")
+ local user_conf_yaml, err = util.read_file(local_conf_path)
+ if not user_conf_yaml then
+ return nil, err
+ end
+
+ local is_empty_file = true
+ for line in str_gmatch(user_conf_yaml .. '\n', '(.-)\r?\n') do
+ if not is_empty_yaml_line(line) then
+ is_empty_file = false
+ break
+ end
+ end
+
+ if not is_empty_file then
+ local user_conf = yaml.parse(user_conf_yaml)
+ if not user_conf then
+ return nil, "invalid config.yaml file"
+ end
+
+ resolve_conf_var(user_conf)
+ merge_conf(default_conf, user_conf)
+ end
+
+ return default_conf
+end
+
+
+return _M
+
Review comment:
@spacewander Fixed.
##########
File path: apisix/cli/util.lua
##########
@@ -46,4 +50,36 @@ function _M.trim(s)
end
+function _M.split(self, sep)
+ local sep, fields = sep or ":", {}
+ local pattern = str_format("([^%s]+)", sep)
+
+ self:gsub(pattern, function(c) fields[#fields + 1] = c end)
+
+ return fields
+end
+
+
+function _M.read_file(file_path)
+ local file, err = open(file_path, "rb")
+ if not file then
+ return false, "failed to open file: " .. file_path .. ", error info:" .. err
+ end
+
+ local data, err = file:read("*all")
+ if err ~= nil then
Review comment:
@spacewander Fixed.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] spacewander commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
spacewander commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r525733685
##########
File path: apisix/cli/etcd.lua
##########
@@ -0,0 +1,205 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local base64_encode = require("base64").encode
+local dkjson = require("dkjson")
+local util = require("apisix.cli.util")
+local file = require("apisix.cli.file")
+
+local type = type
+local ipairs = ipairs
+local print = print
+local tonumber = tonumber
+local str_format = string.format
+
+local _M = {}
+
+
+local function parse_semantic_version(ver)
+ local errmsg = "invalid semantic version: " .. ver
+
+ local parts = util.split(ver, "-")
+ if #parts > 2 then
+ return nil, errmsg
+ end
+
+ if #parts == 2 then
+ ver = parts[1]
+ end
+
+ local fields = util.split(ver, ".")
+ if #fields ~= 3 then
+ return nil, errmsg
+ end
+
+ local major = tonumber(fields[1])
+ local minor = tonumber(fields[2])
+ local patch = tonumber(fields[3])
+
+ if not (major and minor and patch) then
+ return nil, errmsg
+ end
+
+ return {
+ major = major,
+ minor = minor,
+ patch = patch,
+ }
+end
+
+
+local function compare_semantic_version(v1, v2)
+ local ver1, err = parse_semantic_version(v1)
+ if not ver1 then
+ return nil, err
+ end
+
+ local ver2, err = parse_semantic_version(v2)
+ if not ver2 then
+ return nil, err
+ end
+
+ if ver1.major ~= ver2.major then
+ return ver1.major < ver2.major
+ end
+
+ if ver1.minor ~= ver2.minor then
+ return ver1.minor < ver2.minor
+ end
+
+ return ver1.patch < ver2.patch
+end
+
+
+function _M.init(env, show_output)
+ -- read_yaml_conf
+ local yaml_conf, err = file.read_yaml_conf(env.apisix_home)
+ if not yaml_conf then
+ util.die("failed to read local yaml config of apisix: ", err)
+ end
+
+ if not yaml_conf.apisix then
+ util.die("failed to read `apisix` field from yaml file when init etcd")
+ end
+
+ if yaml_conf.apisix.config_center ~= "etcd" then
+ return true
+ end
+
+ if not yaml_conf.etcd then
+ util.die("failed to read `etcd` field from yaml file when init etcd")
+ end
+
+ local etcd_conf = yaml_conf.etcd
+
+ local timeout = etcd_conf.timeout or 3
+ local uri
+
+ -- convert old single etcd config to multiple etcd config
+ if type(yaml_conf.etcd.host) == "string" then
+ yaml_conf.etcd.host = {yaml_conf.etcd.host}
+ end
+
+ local host_count = #(yaml_conf.etcd.host)
+ local scheme
+ for i = 1, host_count do
+ local host = yaml_conf.etcd.host[i]
+ local fields = util.split(host, "://")
+ if not fields then
+ util.die("malformed etcd endpoint: ", host, "\n")
+ end
+
+ if not scheme then
+ scheme = fields[1]
+ elseif scheme ~= fields[1] then
+ print([[WARNING: mixed protocols among etcd endpoints]])
+ end
+ end
+
+ -- check the etcd cluster version
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ uri = host .. "/version"
+ local cmd = str_format("curl -s -m %d %s", timeout * 2, uri)
+ local res = util.execute_cmd(cmd)
+ local errmsg = str_format("got malformed version message: \"%s\" from etcd\n",
+ res)
+
+ local body, _, err = dkjson.decode(res)
+ if err then
+ util.die(errmsg)
+ end
+
+ local cluster_version = body["etcdcluster"]
+ if not cluster_version then
+ util.die(errmsg)
+ end
+
+ if compare_semantic_version(cluster_version, env.min_etcd_version) then
+ util.die("etcd cluster version ", cluster_version,
+ " is less than the required version ",
+ env.min_etcd_version,
+ ", please upgrade your etcd cluster\n")
+ end
+ end
+
+ local etcd_ok = false
+ for index, host in ipairs(yaml_conf.etcd.host) do
+ local is_success = true
+
+ for _, dir_name in ipairs({"/routes", "/upstreams", "/services",
+ "/plugins", "/consumers", "/node_status",
+ "/ssl", "/global_rules", "/stream_routes",
+ "/proto", "/plugin_metadata"}) do
+
+ local key = (etcd_conf.prefix or "") .. dir_name .. "/"
+
+ local uri = host .. "/v3/kv/put"
+ local post_json = '{"value":"' .. base64_encode("init_dir")
+ ..'", "key":"' .. base64_encode(key) .. '"}'
+ local cmd = "curl " .. uri .. " -X POST -d '" .. post_json
+ .. "' --connect-timeout " .. timeout
+ .. " --max-time " .. timeout * 2 .. " --retry 1 2>&1"
+
+ local res = util.execute_cmd(cmd)
+ if res:find("OK", 1, true) then
Review comment:
The error check is confusing to me... Why "OK" means "is_success" is false?
##########
File path: apisix/cli/file.lua
##########
@@ -0,0 +1,146 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local yaml = require("tinyyaml")
+local profile = require("apisix.core.profile")
+local util = require("apisix.cli.util")
+
+local pairs = pairs
+local type = type
+local tonumber = tonumber
+local getenv = os.getenv
+local str_gmatch = string.gmatch
+local str_find = string.find
+
+local _M = {}
+
+
+local function is_empty_yaml_line(line)
+ return line == '' or str_find(line, '^%s*$') or str_find(line, '^%s*#')
+end
+
+
+local function tab_is_array(t)
+ local count = 0
+ for k,v in pairs(t) do
+ count = count + 1
+ end
+
+ return #t == count
+end
+
+
+local function resolve_conf_var(conf)
+ for key, val in pairs(conf) do
+ if type(val) == "table" then
+ resolve_conf_var(val)
+ elseif type(val) == "string" then
+ local var_used = false
+ -- we use '${{var}}' because '$var' and '${var}' are taken
+ -- by Nginx
+ local new_val = val:gsub("%$%{%{([%w_]+)%}%}", function(var)
+ local v = getenv(var)
+ if v then
+ var_used = true
+ return v
+ end
+
+ util.die("failed to handle configuration: ",
+ "can't find environment variable ",
+ var, "\n")
+ end)
+
+ if var_used then
+ if tonumber(new_val) ~= nil then
+ new_val = tonumber(new_val)
+ elseif new_val == "true" then
+ new_val = true
+ elseif new_val == "false" then
+ new_val = false
+ end
+ end
+
+ conf[key] = new_val
+ end
+ end
+end
+
+
+local function merge_conf(base, new_tab)
+ for key, val in pairs(new_tab) do
+ if type(val) == "table" then
+ if tab_is_array(val) then
+ base[key] = val
+ elseif base[key] == nil then
+ base[key] = val
+ else
+ merge_conf(base[key], val)
+ end
+
+ else
+ base[key] = val
+ end
+ end
+
+ return base
+end
+
+
+function _M.read_yaml_conf(apisix_home)
+ profile.apisix_home = apisix_home .. "/"
+ local local_conf_path = profile:yaml_path("config-default")
+ local default_conf_yaml, err = util.read_file(local_conf_path)
+ if not default_conf_yaml then
+ return nil, err
+ end
+
+ local default_conf = yaml.parse(default_conf_yaml)
+ if not default_conf then
+ return nil, "invalid config-default.yaml file"
+ end
+
+ local_conf_path = profile:yaml_path("config")
+ local user_conf_yaml, err = util.read_file(local_conf_path)
+ if not user_conf_yaml then
+ return nil, err
+ end
+
+ local is_empty_file = true
+ for line in str_gmatch(user_conf_yaml .. '\n', '(.-)\r?\n') do
+ if not is_empty_yaml_line(line) then
+ is_empty_file = false
+ break
+ end
+ end
+
+ if not is_empty_file then
+ local user_conf = yaml.parse(user_conf_yaml)
+ if not user_conf then
+ return nil, "invalid config.yaml file"
+ end
+
+ resolve_conf_var(user_conf)
+ merge_conf(default_conf, user_conf)
+ end
+
+ return default_conf
+end
+
+
+return _M
+
Review comment:
Why leave trailing newlines here?
##########
File path: apisix/cli/util.lua
##########
@@ -46,4 +50,36 @@ function _M.trim(s)
end
+function _M.split(self, sep)
+ local sep, fields = sep or ":", {}
+ local pattern = str_format("([^%s]+)", sep)
+
+ self:gsub(pattern, function(c) fields[#fields + 1] = c end)
+
+ return fields
+end
+
+
+function _M.read_file(file_path)
+ local file, err = open(file_path, "rb")
+ if not file then
+ return false, "failed to open file: " .. file_path .. ", error info:" .. err
+ end
+
+ local data, err = file:read("*all")
+ if err ~= nil then
Review comment:
Should close file when failed.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org
[GitHub] [apisix] spacewander commented on a change in pull request #2685: refactor: moved etcd initialiation to apisix/cli/etcd.lua
Posted by GitBox <gi...@apache.org>.
spacewander commented on a change in pull request #2685:
URL: https://github.com/apache/apisix/pull/2685#discussion_r526518746
##########
File path: apisix/cli/file.lua
##########
@@ -0,0 +1,144 @@
+--
+-- Licensed to the Apache Software Foundation (ASF) under one or more
+-- contributor license agreements. See the NOTICE file distributed with
+-- this work for additional information regarding copyright ownership.
+-- The ASF licenses this file to You under the Apache License, Version 2.0
+-- (the "License"); you may not use this file except in compliance with
+-- the License. You may obtain a copy of the License at
+--
+-- http://www.apache.org/licenses/LICENSE-2.0
+--
+-- Unless required by applicable law or agreed to in writing, software
+-- distributed under the License is distributed on an "AS IS" BASIS,
+-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+-- See the License for the specific language governing permissions and
+-- limitations under the License.
+--
+
+local yaml = require("tinyyaml")
+local profile = require("apisix.core.profile")
+local util = require("apisix.cli.util")
+
+local pairs = pairs
+local type = type
+local tonumber = tonumber
+local getenv = os.getenv
+local str_gmatch = string.gmatch
+local str_find = string.find
+
+local _M = {}
+
+
+local function is_empty_yaml_line(line)
+ return line == '' or str_find(line, '^%s*$') or str_find(line, '^%s*#')
+end
+
+
+local function tab_is_array(t)
+ local count = 0
+ for k,v in pairs(t) do
+ count = count + 1
+ end
+
+ return #t == count
+end
+
+
+local function resolve_conf_var(conf)
Review comment:
@membphis
We may resolve other variables in the future. So it would be better to keep the name.
----------------------------------------------------------------
This is an automated message from the Apache Git Service.
To respond to the message, please log on to GitHub and use the
URL above to go to the specific comment.
For queries about this service, please contact Infrastructure at:
users@infra.apache.org