You are viewing a plain text version of this content. The canonical link for it is here.
Posted to commits@cordova.apache.org by an...@apache.org on 2015/10/22 21:45:45 UTC
[27/32] android commit: CB-9782 Check in cordova-common dependency
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/README.md b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/README.md
new file mode 100644
index 0000000..2aff0eb
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/README.md
@@ -0,0 +1,80 @@
+# balanced-match
+
+Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`.
+
+[](http://travis-ci.org/juliangruber/balanced-match)
+[](https://www.npmjs.org/package/balanced-match)
+
+[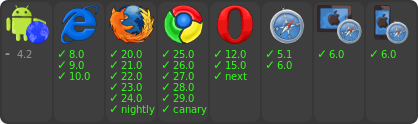](https://ci.testling.com/juliangruber/balanced-match)
+
+## Example
+
+Get the first matching pair of braces:
+
+```js
+var balanced = require('balanced-match');
+
+console.log(balanced('{', '}', 'pre{in{nested}}post'));
+console.log(balanced('{', '}', 'pre{first}between{second}post'));
+```
+
+The matches are:
+
+```bash
+$ node example.js
+{ start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' }
+{ start: 3,
+ end: 9,
+ pre: 'pre',
+ body: 'first',
+ post: 'between{second}post' }
+```
+
+## API
+
+### var m = balanced(a, b, str)
+
+For the first non-nested matching pair of `a` and `b` in `str`, return an
+object with those keys:
+
+* **start** the index of the first match of `a`
+* **end** the index of the matching `b`
+* **pre** the preamble, `a` and `b` not included
+* **body** the match, `a` and `b` not included
+* **post** the postscript, `a` and `b` not included
+
+If there's no match, `undefined` will be returned.
+
+If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']`.
+
+## Installation
+
+With [npm](https://npmjs.org) do:
+
+```bash
+npm install balanced-match
+```
+
+## License
+
+(MIT)
+
+Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
+of the Software, and to permit persons to whom the Software is furnished to do
+so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/example.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/example.js b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/example.js
new file mode 100644
index 0000000..c02ad34
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/example.js
@@ -0,0 +1,5 @@
+var balanced = require('./');
+
+console.log(balanced('{', '}', 'pre{in{nested}}post'));
+console.log(balanced('{', '}', 'pre{first}between{second}post'));
+
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/index.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/index.js b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/index.js
new file mode 100644
index 0000000..d165ae8
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/index.js
@@ -0,0 +1,38 @@
+module.exports = balanced;
+function balanced(a, b, str) {
+ var bal = 0;
+ var m = {};
+ var ended = false;
+
+ for (var i = 0; i < str.length; i++) {
+ if (a == str.substr(i, a.length)) {
+ if (!('start' in m)) m.start = i;
+ bal++;
+ }
+ else if (b == str.substr(i, b.length) && 'start' in m) {
+ ended = true;
+ bal--;
+ if (!bal) {
+ m.end = i;
+ m.pre = str.substr(0, m.start);
+ m.body = (m.end - m.start > 1)
+ ? str.substring(m.start + a.length, m.end)
+ : '';
+ m.post = str.slice(m.end + b.length);
+ return m;
+ }
+ }
+ }
+
+ // if we opened more than we closed, find the one we closed
+ if (bal && ended) {
+ var start = m.start + a.length;
+ m = balanced(a, b, str.substr(start));
+ if (m) {
+ m.start += start;
+ m.end += start;
+ m.pre = str.slice(0, start) + m.pre;
+ }
+ return m;
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/package.json
new file mode 100644
index 0000000..ede6efe
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/balanced-match/package.json
@@ -0,0 +1,73 @@
+{
+ "name": "balanced-match",
+ "description": "Match balanced character pairs, like \"{\" and \"}\"",
+ "version": "0.2.0",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/juliangruber/balanced-match.git"
+ },
+ "homepage": "https://github.com/juliangruber/balanced-match",
+ "main": "index.js",
+ "scripts": {
+ "test": "make test"
+ },
+ "dependencies": {},
+ "devDependencies": {
+ "tape": "~1.1.1"
+ },
+ "keywords": [
+ "match",
+ "regexp",
+ "test",
+ "balanced",
+ "parse"
+ ],
+ "author": {
+ "name": "Julian Gruber",
+ "email": "mail@juliangruber.com",
+ "url": "http://juliangruber.com"
+ },
+ "license": "MIT",
+ "testling": {
+ "files": "test/*.js",
+ "browsers": [
+ "ie/8..latest",
+ "firefox/20..latest",
+ "firefox/nightly",
+ "chrome/25..latest",
+ "chrome/canary",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest",
+ "android-browser/4.2..latest"
+ ]
+ },
+ "gitHead": "ba40ed78e7114a4a67c51da768a100184dead39c",
+ "bugs": {
+ "url": "https://github.com/juliangruber/balanced-match/issues"
+ },
+ "_id": "balanced-match@0.2.0",
+ "_shasum": "38f6730c03aab6d5edbb52bd934885e756d71674",
+ "_from": "balanced-match@>=0.2.0 <0.3.0",
+ "_npmVersion": "2.1.8",
+ "_nodeVersion": "0.10.32",
+ "_npmUser": {
+ "name": "juliangruber",
+ "email": "julian@juliangruber.com"
+ },
+ "maintainers": [
+ {
+ "name": "juliangruber",
+ "email": "julian@juliangruber.com"
+ }
+ ],
+ "dist": {
+ "shasum": "38f6730c03aab6d5edbb52bd934885e756d71674",
+ "tarball": "http://registry.npmjs.org/balanced-match/-/balanced-match-0.2.0.tgz"
+ },
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/balanced-match/-/balanced-match-0.2.0.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/.travis.yml b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/.travis.yml
new file mode 100644
index 0000000..f1d0f13
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/.travis.yml
@@ -0,0 +1,4 @@
+language: node_js
+node_js:
+ - 0.4
+ - 0.6
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/LICENSE b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/LICENSE
new file mode 100644
index 0000000..ee27ba4
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/LICENSE
@@ -0,0 +1,18 @@
+This software is released under the MIT license:
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
+the Software, and to permit persons to whom the Software is furnished to do so,
+subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
+FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
+COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
+IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
+CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/README.markdown
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/README.markdown b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/README.markdown
new file mode 100644
index 0000000..408f70a
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/README.markdown
@@ -0,0 +1,62 @@
+concat-map
+==========
+
+Concatenative mapdashery.
+
+[](http://ci.testling.com/substack/node-concat-map)
+
+[](http://travis-ci.org/substack/node-concat-map)
+
+example
+=======
+
+``` js
+var concatMap = require('concat-map');
+var xs = [ 1, 2, 3, 4, 5, 6 ];
+var ys = concatMap(xs, function (x) {
+ return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
+});
+console.dir(ys);
+```
+
+***
+
+```
+[ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]
+```
+
+methods
+=======
+
+``` js
+var concatMap = require('concat-map')
+```
+
+concatMap(xs, fn)
+-----------------
+
+Return an array of concatenated elements by calling `fn(x, i)` for each element
+`x` and each index `i` in the array `xs`.
+
+When `fn(x, i)` returns an array, its result will be concatenated with the
+result array. If `fn(x, i)` returns anything else, that value will be pushed
+onto the end of the result array.
+
+install
+=======
+
+With [npm](http://npmjs.org) do:
+
+```
+npm install concat-map
+```
+
+license
+=======
+
+MIT
+
+notes
+=====
+
+This module was written while sitting high above the ground in a tree.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/index.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/index.js b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/index.js
new file mode 100644
index 0000000..b29a781
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/index.js
@@ -0,0 +1,13 @@
+module.exports = function (xs, fn) {
+ var res = [];
+ for (var i = 0; i < xs.length; i++) {
+ var x = fn(xs[i], i);
+ if (isArray(x)) res.push.apply(res, x);
+ else res.push(x);
+ }
+ return res;
+};
+
+var isArray = Array.isArray || function (xs) {
+ return Object.prototype.toString.call(xs) === '[object Array]';
+};
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/package.json
new file mode 100644
index 0000000..b516138
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/node_modules/concat-map/package.json
@@ -0,0 +1,83 @@
+{
+ "name": "concat-map",
+ "description": "concatenative mapdashery",
+ "version": "0.0.1",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/substack/node-concat-map.git"
+ },
+ "main": "index.js",
+ "keywords": [
+ "concat",
+ "concatMap",
+ "map",
+ "functional",
+ "higher-order"
+ ],
+ "directories": {
+ "example": "example",
+ "test": "test"
+ },
+ "scripts": {
+ "test": "tape test/*.js"
+ },
+ "devDependencies": {
+ "tape": "~2.4.0"
+ },
+ "license": "MIT",
+ "author": {
+ "name": "James Halliday",
+ "email": "mail@substack.net",
+ "url": "http://substack.net"
+ },
+ "testling": {
+ "files": "test/*.js",
+ "browsers": {
+ "ie": [
+ 6,
+ 7,
+ 8,
+ 9
+ ],
+ "ff": [
+ 3.5,
+ 10,
+ 15
+ ],
+ "chrome": [
+ 10,
+ 22
+ ],
+ "safari": [
+ 5.1
+ ],
+ "opera": [
+ 12
+ ]
+ }
+ },
+ "bugs": {
+ "url": "https://github.com/substack/node-concat-map/issues"
+ },
+ "homepage": "https://github.com/substack/node-concat-map",
+ "_id": "concat-map@0.0.1",
+ "dist": {
+ "shasum": "d8a96bd77fd68df7793a73036a3ba0d5405d477b",
+ "tarball": "http://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz"
+ },
+ "_from": "concat-map@0.0.1",
+ "_npmVersion": "1.3.21",
+ "_npmUser": {
+ "name": "substack",
+ "email": "mail@substack.net"
+ },
+ "maintainers": [
+ {
+ "name": "substack",
+ "email": "mail@substack.net"
+ }
+ ],
+ "_shasum": "d8a96bd77fd68df7793a73036a3ba0d5405d477b",
+ "_resolved": "https://registry.npmjs.org/concat-map/-/concat-map-0.0.1.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/package.json
new file mode 100644
index 0000000..4cb3e05
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/node_modules/brace-expansion/package.json
@@ -0,0 +1,75 @@
+{
+ "name": "brace-expansion",
+ "description": "Brace expansion as known from sh/bash",
+ "version": "1.1.1",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/juliangruber/brace-expansion.git"
+ },
+ "homepage": "https://github.com/juliangruber/brace-expansion",
+ "main": "index.js",
+ "scripts": {
+ "test": "tape test/*.js",
+ "gentest": "bash test/generate.sh"
+ },
+ "dependencies": {
+ "balanced-match": "^0.2.0",
+ "concat-map": "0.0.1"
+ },
+ "devDependencies": {
+ "tape": "^3.0.3"
+ },
+ "keywords": [],
+ "author": {
+ "name": "Julian Gruber",
+ "email": "mail@juliangruber.com",
+ "url": "http://juliangruber.com"
+ },
+ "license": "MIT",
+ "testling": {
+ "files": "test/*.js",
+ "browsers": [
+ "ie/8..latest",
+ "firefox/20..latest",
+ "firefox/nightly",
+ "chrome/25..latest",
+ "chrome/canary",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest",
+ "android-browser/4.2..latest"
+ ]
+ },
+ "gitHead": "f50da498166d76ea570cf3b30179f01f0f119612",
+ "bugs": {
+ "url": "https://github.com/juliangruber/brace-expansion/issues"
+ },
+ "_id": "brace-expansion@1.1.1",
+ "_shasum": "da5fb78aef4c44c9e4acf525064fb3208ebab045",
+ "_from": "brace-expansion@>=1.0.0 <2.0.0",
+ "_npmVersion": "2.6.1",
+ "_nodeVersion": "0.10.36",
+ "_npmUser": {
+ "name": "juliangruber",
+ "email": "julian@juliangruber.com"
+ },
+ "maintainers": [
+ {
+ "name": "juliangruber",
+ "email": "julian@juliangruber.com"
+ },
+ {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ }
+ ],
+ "dist": {
+ "shasum": "da5fb78aef4c44c9e4acf525064fb3208ebab045",
+ "tarball": "http://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.1.tgz"
+ },
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-1.1.1.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/package.json
new file mode 100644
index 0000000..4944eb0
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/minimatch/package.json
@@ -0,0 +1,60 @@
+{
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me"
+ },
+ "name": "minimatch",
+ "description": "a glob matcher in javascript",
+ "version": "3.0.0",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/minimatch.git"
+ },
+ "main": "minimatch.js",
+ "scripts": {
+ "posttest": "standard minimatch.js test/*.js",
+ "test": "tap test/*.js"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "dependencies": {
+ "brace-expansion": "^1.0.0"
+ },
+ "devDependencies": {
+ "standard": "^3.7.2",
+ "tap": "^1.2.0"
+ },
+ "license": "ISC",
+ "files": [
+ "minimatch.js"
+ ],
+ "gitHead": "270dbea567f0af6918cb18103e98c612aa717a20",
+ "bugs": {
+ "url": "https://github.com/isaacs/minimatch/issues"
+ },
+ "homepage": "https://github.com/isaacs/minimatch#readme",
+ "_id": "minimatch@3.0.0",
+ "_shasum": "5236157a51e4f004c177fb3c527ff7dd78f0ef83",
+ "_from": "minimatch@>=2.0.0 <3.0.0||>=3.0.0 <4.0.0",
+ "_npmVersion": "3.3.2",
+ "_nodeVersion": "4.0.0",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ },
+ "dist": {
+ "shasum": "5236157a51e4f004c177fb3c527ff7dd78f0ef83",
+ "tarball": "http://registry.npmjs.org/minimatch/-/minimatch-3.0.0.tgz"
+ },
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/minimatch/-/minimatch-3.0.0.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/once/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/once/LICENSE b/node_modules/cordova-common/node_modules/glob/node_modules/once/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/once/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/once/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/once/README.md b/node_modules/cordova-common/node_modules/glob/node_modules/once/README.md
new file mode 100644
index 0000000..a2981ea
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/once/README.md
@@ -0,0 +1,51 @@
+# once
+
+Only call a function once.
+
+## usage
+
+```javascript
+var once = require('once')
+
+function load (file, cb) {
+ cb = once(cb)
+ loader.load('file')
+ loader.once('load', cb)
+ loader.once('error', cb)
+}
+```
+
+Or add to the Function.prototype in a responsible way:
+
+```javascript
+// only has to be done once
+require('once').proto()
+
+function load (file, cb) {
+ cb = cb.once()
+ loader.load('file')
+ loader.once('load', cb)
+ loader.once('error', cb)
+}
+```
+
+Ironically, the prototype feature makes this module twice as
+complicated as necessary.
+
+To check whether you function has been called, use `fn.called`. Once the
+function is called for the first time the return value of the original
+function is saved in `fn.value` and subsequent calls will continue to
+return this value.
+
+```javascript
+var once = require('once')
+
+function load (cb) {
+ cb = once(cb)
+ var stream = createStream()
+ stream.once('data', cb)
+ stream.once('end', function () {
+ if (!cb.called) cb(new Error('not found'))
+ })
+}
+```
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/once/once.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/once/once.js b/node_modules/cordova-common/node_modules/glob/node_modules/once/once.js
new file mode 100644
index 0000000..2e1e721
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/once/once.js
@@ -0,0 +1,21 @@
+var wrappy = require('wrappy')
+module.exports = wrappy(once)
+
+once.proto = once(function () {
+ Object.defineProperty(Function.prototype, 'once', {
+ value: function () {
+ return once(this)
+ },
+ configurable: true
+ })
+})
+
+function once (fn) {
+ var f = function () {
+ if (f.called) return f.value
+ f.called = true
+ return f.value = fn.apply(this, arguments)
+ }
+ f.called = false
+ return f
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/once/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/once/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/once/package.json
new file mode 100644
index 0000000..8f46e50
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/once/package.json
@@ -0,0 +1,60 @@
+{
+ "name": "once",
+ "version": "1.3.2",
+ "description": "Run a function exactly one time",
+ "main": "once.js",
+ "directories": {
+ "test": "test"
+ },
+ "dependencies": {
+ "wrappy": "1"
+ },
+ "devDependencies": {
+ "tap": "~0.3.0"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/once.git"
+ },
+ "keywords": [
+ "once",
+ "function",
+ "one",
+ "single"
+ ],
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me/"
+ },
+ "license": "ISC",
+ "gitHead": "e35eed5a7867574e2bf2260a1ba23970958b22f2",
+ "bugs": {
+ "url": "https://github.com/isaacs/once/issues"
+ },
+ "homepage": "https://github.com/isaacs/once#readme",
+ "_id": "once@1.3.2",
+ "_shasum": "d8feeca93b039ec1dcdee7741c92bdac5e28081b",
+ "_from": "once@>=1.3.0 <2.0.0",
+ "_npmVersion": "2.9.1",
+ "_nodeVersion": "2.0.0",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ },
+ "dist": {
+ "shasum": "d8feeca93b039ec1dcdee7741c92bdac5e28081b",
+ "tarball": "http://registry.npmjs.org/once/-/once-1.3.2.tgz"
+ },
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "_resolved": "https://registry.npmjs.org/once/-/once-1.3.2.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/index.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/index.js b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/index.js
new file mode 100644
index 0000000..19f103f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/index.js
@@ -0,0 +1,20 @@
+'use strict';
+
+function posix(path) {
+ return path.charAt(0) === '/';
+};
+
+function win32(path) {
+ // https://github.com/joyent/node/blob/b3fcc245fb25539909ef1d5eaa01dbf92e168633/lib/path.js#L56
+ var splitDeviceRe = /^([a-zA-Z]:|[\\\/]{2}[^\\\/]+[\\\/]+[^\\\/]+)?([\\\/])?([\s\S]*?)$/;
+ var result = splitDeviceRe.exec(path);
+ var device = result[1] || '';
+ var isUnc = !!device && device.charAt(1) !== ':';
+
+ // UNC paths are always absolute
+ return !!result[2] || isUnc;
+};
+
+module.exports = process.platform === 'win32' ? win32 : posix;
+module.exports.posix = posix;
+module.exports.win32 = win32;
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/license
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/license b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus <si...@gmail.com> (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/package.json
new file mode 100644
index 0000000..3937263
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/package.json
@@ -0,0 +1,70 @@
+{
+ "name": "path-is-absolute",
+ "version": "1.0.0",
+ "description": "Node.js 0.12 path.isAbsolute() ponyfill",
+ "license": "MIT",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/path-is-absolute.git"
+ },
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "files": [
+ "index.js"
+ ],
+ "keywords": [
+ "path",
+ "paths",
+ "file",
+ "dir",
+ "absolute",
+ "isabsolute",
+ "is-absolute",
+ "built-in",
+ "util",
+ "utils",
+ "core",
+ "ponyfill",
+ "polyfill",
+ "shim",
+ "is",
+ "detect",
+ "check"
+ ],
+ "gitHead": "7a76a0c9f2263192beedbe0a820e4d0baee5b7a1",
+ "bugs": {
+ "url": "https://github.com/sindresorhus/path-is-absolute/issues"
+ },
+ "homepage": "https://github.com/sindresorhus/path-is-absolute",
+ "_id": "path-is-absolute@1.0.0",
+ "_shasum": "263dada66ab3f2fb10bf7f9d24dd8f3e570ef912",
+ "_from": "path-is-absolute@>=1.0.0 <2.0.0",
+ "_npmVersion": "2.5.1",
+ "_nodeVersion": "0.12.0",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "dist": {
+ "shasum": "263dada66ab3f2fb10bf7f9d24dd8f3e570ef912",
+ "tarball": "http://registry.npmjs.org/path-is-absolute/-/path-is-absolute-1.0.0.tgz"
+ },
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/path-is-absolute/-/path-is-absolute-1.0.0.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/readme.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/readme.md b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/readme.md
new file mode 100644
index 0000000..cdf94f4
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/path-is-absolute/readme.md
@@ -0,0 +1,51 @@
+# path-is-absolute [](https://travis-ci.org/sindresorhus/path-is-absolute)
+
+> Node.js 0.12 [`path.isAbsolute()`](http://nodejs.org/api/path.html#path_path_isabsolute_path) ponyfill
+
+> Ponyfill: A polyfill that doesn't overwrite the native method
+
+
+## Install
+
+```
+$ npm install --save path-is-absolute
+```
+
+
+## Usage
+
+```js
+var pathIsAbsolute = require('path-is-absolute');
+
+// Linux
+pathIsAbsolute('/home/foo');
+//=> true
+
+// Windows
+pathIsAbsolute('C:/Users/');
+//=> true
+
+// Any OS
+pathIsAbsolute.posix('/home/foo');
+//=> true
+```
+
+
+## API
+
+See the [`path.isAbsolute()` docs](http://nodejs.org/api/path.html#path_path_isabsolute_path).
+
+### pathIsAbsolute(path)
+
+### pathIsAbsolute.posix(path)
+
+The Posix specific version.
+
+### pathIsAbsolute.win32(path)
+
+The Windows specific version.
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/LICENSE b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/README.md b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/README.md
new file mode 100644
index 0000000..98eab25
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/README.md
@@ -0,0 +1,36 @@
+# wrappy
+
+Callback wrapping utility
+
+## USAGE
+
+```javascript
+var wrappy = require("wrappy")
+
+// var wrapper = wrappy(wrapperFunction)
+
+// make sure a cb is called only once
+// See also: http://npm.im/once for this specific use case
+var once = wrappy(function (cb) {
+ var called = false
+ return function () {
+ if (called) return
+ called = true
+ return cb.apply(this, arguments)
+ }
+})
+
+function printBoo () {
+ console.log('boo')
+}
+// has some rando property
+printBoo.iAmBooPrinter = true
+
+var onlyPrintOnce = once(printBoo)
+
+onlyPrintOnce() // prints 'boo'
+onlyPrintOnce() // does nothing
+
+// random property is retained!
+assert.equal(onlyPrintOnce.iAmBooPrinter, true)
+```
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/package.json b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/package.json
new file mode 100644
index 0000000..5411286
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/package.json
@@ -0,0 +1,52 @@
+{
+ "name": "wrappy",
+ "version": "1.0.1",
+ "description": "Callback wrapping utility",
+ "main": "wrappy.js",
+ "directories": {
+ "test": "test"
+ },
+ "dependencies": {},
+ "devDependencies": {
+ "tap": "^0.4.12"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/npm/wrappy.git"
+ },
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me/"
+ },
+ "license": "ISC",
+ "bugs": {
+ "url": "https://github.com/npm/wrappy/issues"
+ },
+ "homepage": "https://github.com/npm/wrappy",
+ "gitHead": "006a8cbac6b99988315834c207896eed71fd069a",
+ "_id": "wrappy@1.0.1",
+ "_shasum": "1e65969965ccbc2db4548c6b84a6f2c5aedd4739",
+ "_from": "wrappy@1.0.1",
+ "_npmVersion": "2.0.0",
+ "_nodeVersion": "0.10.31",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "dist": {
+ "shasum": "1e65969965ccbc2db4548c6b84a6f2c5aedd4739",
+ "tarball": "http://registry.npmjs.org/wrappy/-/wrappy-1.0.1.tgz"
+ },
+ "_resolved": "https://registry.npmjs.org/wrappy/-/wrappy-1.0.1.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/wrappy.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/wrappy.js b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/wrappy.js
new file mode 100644
index 0000000..bb7e7d6
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/node_modules/wrappy/wrappy.js
@@ -0,0 +1,33 @@
+// Returns a wrapper function that returns a wrapped callback
+// The wrapper function should do some stuff, and return a
+// presumably different callback function.
+// This makes sure that own properties are retained, so that
+// decorations and such are not lost along the way.
+module.exports = wrappy
+function wrappy (fn, cb) {
+ if (fn && cb) return wrappy(fn)(cb)
+
+ if (typeof fn !== 'function')
+ throw new TypeError('need wrapper function')
+
+ Object.keys(fn).forEach(function (k) {
+ wrapper[k] = fn[k]
+ })
+
+ return wrapper
+
+ function wrapper() {
+ var args = new Array(arguments.length)
+ for (var i = 0; i < args.length; i++) {
+ args[i] = arguments[i]
+ }
+ var ret = fn.apply(this, args)
+ var cb = args[args.length-1]
+ if (typeof ret === 'function' && ret !== cb) {
+ Object.keys(cb).forEach(function (k) {
+ ret[k] = cb[k]
+ })
+ }
+ return ret
+ }
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/package.json b/node_modules/cordova-common/node_modules/glob/package.json
new file mode 100644
index 0000000..680006c
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/package.json
@@ -0,0 +1,73 @@
+{
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me/"
+ },
+ "name": "glob",
+ "description": "a little globber",
+ "version": "5.0.15",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/node-glob.git"
+ },
+ "main": "glob.js",
+ "files": [
+ "glob.js",
+ "sync.js",
+ "common.js"
+ ],
+ "engines": {
+ "node": "*"
+ },
+ "dependencies": {
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "2 || 3",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "devDependencies": {
+ "mkdirp": "0",
+ "rimraf": "^2.2.8",
+ "tap": "^1.1.4",
+ "tick": "0.0.6"
+ },
+ "scripts": {
+ "prepublish": "npm run benchclean",
+ "profclean": "rm -f v8.log profile.txt",
+ "test": "tap test/*.js --cov",
+ "test-regen": "npm run profclean && TEST_REGEN=1 node test/00-setup.js",
+ "bench": "bash benchmark.sh",
+ "prof": "bash prof.sh && cat profile.txt",
+ "benchclean": "node benchclean.js"
+ },
+ "license": "ISC",
+ "gitHead": "3a7e71d453dd80e75b196fd262dd23ed54beeceb",
+ "bugs": {
+ "url": "https://github.com/isaacs/node-glob/issues"
+ },
+ "homepage": "https://github.com/isaacs/node-glob#readme",
+ "_id": "glob@5.0.15",
+ "_shasum": "1bc936b9e02f4a603fcc222ecf7633d30b8b93b1",
+ "_from": "glob@>=5.0.13 <6.0.0",
+ "_npmVersion": "3.3.2",
+ "_nodeVersion": "4.0.0",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ },
+ "dist": {
+ "shasum": "1bc936b9e02f4a603fcc222ecf7633d30b8b93b1",
+ "tarball": "http://registry.npmjs.org/glob/-/glob-5.0.15.tgz"
+ },
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ }
+ ],
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/glob/-/glob-5.0.15.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/glob/sync.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/glob/sync.js b/node_modules/cordova-common/node_modules/glob/sync.js
new file mode 100644
index 0000000..09883d2
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/glob/sync.js
@@ -0,0 +1,460 @@
+module.exports = globSync
+globSync.GlobSync = GlobSync
+
+var fs = require('fs')
+var minimatch = require('minimatch')
+var Minimatch = minimatch.Minimatch
+var Glob = require('./glob.js').Glob
+var util = require('util')
+var path = require('path')
+var assert = require('assert')
+var isAbsolute = require('path-is-absolute')
+var common = require('./common.js')
+var alphasort = common.alphasort
+var alphasorti = common.alphasorti
+var setopts = common.setopts
+var ownProp = common.ownProp
+var childrenIgnored = common.childrenIgnored
+
+function globSync (pattern, options) {
+ if (typeof options === 'function' || arguments.length === 3)
+ throw new TypeError('callback provided to sync glob\n'+
+ 'See: https://github.com/isaacs/node-glob/issues/167')
+
+ return new GlobSync(pattern, options).found
+}
+
+function GlobSync (pattern, options) {
+ if (!pattern)
+ throw new Error('must provide pattern')
+
+ if (typeof options === 'function' || arguments.length === 3)
+ throw new TypeError('callback provided to sync glob\n'+
+ 'See: https://github.com/isaacs/node-glob/issues/167')
+
+ if (!(this instanceof GlobSync))
+ return new GlobSync(pattern, options)
+
+ setopts(this, pattern, options)
+
+ if (this.noprocess)
+ return this
+
+ var n = this.minimatch.set.length
+ this.matches = new Array(n)
+ for (var i = 0; i < n; i ++) {
+ this._process(this.minimatch.set[i], i, false)
+ }
+ this._finish()
+}
+
+GlobSync.prototype._finish = function () {
+ assert(this instanceof GlobSync)
+ if (this.realpath) {
+ var self = this
+ this.matches.forEach(function (matchset, index) {
+ var set = self.matches[index] = Object.create(null)
+ for (var p in matchset) {
+ try {
+ p = self._makeAbs(p)
+ var real = fs.realpathSync(p, self.realpathCache)
+ set[real] = true
+ } catch (er) {
+ if (er.syscall === 'stat')
+ set[self._makeAbs(p)] = true
+ else
+ throw er
+ }
+ }
+ })
+ }
+ common.finish(this)
+}
+
+
+GlobSync.prototype._process = function (pattern, index, inGlobStar) {
+ assert(this instanceof GlobSync)
+
+ // Get the first [n] parts of pattern that are all strings.
+ var n = 0
+ while (typeof pattern[n] === 'string') {
+ n ++
+ }
+ // now n is the index of the first one that is *not* a string.
+
+ // See if there's anything else
+ var prefix
+ switch (n) {
+ // if not, then this is rather simple
+ case pattern.length:
+ this._processSimple(pattern.join('/'), index)
+ return
+
+ case 0:
+ // pattern *starts* with some non-trivial item.
+ // going to readdir(cwd), but not include the prefix in matches.
+ prefix = null
+ break
+
+ default:
+ // pattern has some string bits in the front.
+ // whatever it starts with, whether that's 'absolute' like /foo/bar,
+ // or 'relative' like '../baz'
+ prefix = pattern.slice(0, n).join('/')
+ break
+ }
+
+ var remain = pattern.slice(n)
+
+ // get the list of entries.
+ var read
+ if (prefix === null)
+ read = '.'
+ else if (isAbsolute(prefix) || isAbsolute(pattern.join('/'))) {
+ if (!prefix || !isAbsolute(prefix))
+ prefix = '/' + prefix
+ read = prefix
+ } else
+ read = prefix
+
+ var abs = this._makeAbs(read)
+
+ //if ignored, skip processing
+ if (childrenIgnored(this, read))
+ return
+
+ var isGlobStar = remain[0] === minimatch.GLOBSTAR
+ if (isGlobStar)
+ this._processGlobStar(prefix, read, abs, remain, index, inGlobStar)
+ else
+ this._processReaddir(prefix, read, abs, remain, index, inGlobStar)
+}
+
+
+GlobSync.prototype._processReaddir = function (prefix, read, abs, remain, index, inGlobStar) {
+ var entries = this._readdir(abs, inGlobStar)
+
+ // if the abs isn't a dir, then nothing can match!
+ if (!entries)
+ return
+
+ // It will only match dot entries if it starts with a dot, or if
+ // dot is set. Stuff like @(.foo|.bar) isn't allowed.
+ var pn = remain[0]
+ var negate = !!this.minimatch.negate
+ var rawGlob = pn._glob
+ var dotOk = this.dot || rawGlob.charAt(0) === '.'
+
+ var matchedEntries = []
+ for (var i = 0; i < entries.length; i++) {
+ var e = entries[i]
+ if (e.charAt(0) !== '.' || dotOk) {
+ var m
+ if (negate && !prefix) {
+ m = !e.match(pn)
+ } else {
+ m = e.match(pn)
+ }
+ if (m)
+ matchedEntries.push(e)
+ }
+ }
+
+ var len = matchedEntries.length
+ // If there are no matched entries, then nothing matches.
+ if (len === 0)
+ return
+
+ // if this is the last remaining pattern bit, then no need for
+ // an additional stat *unless* the user has specified mark or
+ // stat explicitly. We know they exist, since readdir returned
+ // them.
+
+ if (remain.length === 1 && !this.mark && !this.stat) {
+ if (!this.matches[index])
+ this.matches[index] = Object.create(null)
+
+ for (var i = 0; i < len; i ++) {
+ var e = matchedEntries[i]
+ if (prefix) {
+ if (prefix.slice(-1) !== '/')
+ e = prefix + '/' + e
+ else
+ e = prefix + e
+ }
+
+ if (e.charAt(0) === '/' && !this.nomount) {
+ e = path.join(this.root, e)
+ }
+ this.matches[index][e] = true
+ }
+ // This was the last one, and no stats were needed
+ return
+ }
+
+ // now test all matched entries as stand-ins for that part
+ // of the pattern.
+ remain.shift()
+ for (var i = 0; i < len; i ++) {
+ var e = matchedEntries[i]
+ var newPattern
+ if (prefix)
+ newPattern = [prefix, e]
+ else
+ newPattern = [e]
+ this._process(newPattern.concat(remain), index, inGlobStar)
+ }
+}
+
+
+GlobSync.prototype._emitMatch = function (index, e) {
+ var abs = this._makeAbs(e)
+ if (this.mark)
+ e = this._mark(e)
+
+ if (this.matches[index][e])
+ return
+
+ if (this.nodir) {
+ var c = this.cache[this._makeAbs(e)]
+ if (c === 'DIR' || Array.isArray(c))
+ return
+ }
+
+ this.matches[index][e] = true
+ if (this.stat)
+ this._stat(e)
+}
+
+
+GlobSync.prototype._readdirInGlobStar = function (abs) {
+ // follow all symlinked directories forever
+ // just proceed as if this is a non-globstar situation
+ if (this.follow)
+ return this._readdir(abs, false)
+
+ var entries
+ var lstat
+ var stat
+ try {
+ lstat = fs.lstatSync(abs)
+ } catch (er) {
+ // lstat failed, doesn't exist
+ return null
+ }
+
+ var isSym = lstat.isSymbolicLink()
+ this.symlinks[abs] = isSym
+
+ // If it's not a symlink or a dir, then it's definitely a regular file.
+ // don't bother doing a readdir in that case.
+ if (!isSym && !lstat.isDirectory())
+ this.cache[abs] = 'FILE'
+ else
+ entries = this._readdir(abs, false)
+
+ return entries
+}
+
+GlobSync.prototype._readdir = function (abs, inGlobStar) {
+ var entries
+
+ if (inGlobStar && !ownProp(this.symlinks, abs))
+ return this._readdirInGlobStar(abs)
+
+ if (ownProp(this.cache, abs)) {
+ var c = this.cache[abs]
+ if (!c || c === 'FILE')
+ return null
+
+ if (Array.isArray(c))
+ return c
+ }
+
+ try {
+ return this._readdirEntries(abs, fs.readdirSync(abs))
+ } catch (er) {
+ this._readdirError(abs, er)
+ return null
+ }
+}
+
+GlobSync.prototype._readdirEntries = function (abs, entries) {
+ // if we haven't asked to stat everything, then just
+ // assume that everything in there exists, so we can avoid
+ // having to stat it a second time.
+ if (!this.mark && !this.stat) {
+ for (var i = 0; i < entries.length; i ++) {
+ var e = entries[i]
+ if (abs === '/')
+ e = abs + e
+ else
+ e = abs + '/' + e
+ this.cache[e] = true
+ }
+ }
+
+ this.cache[abs] = entries
+
+ // mark and cache dir-ness
+ return entries
+}
+
+GlobSync.prototype._readdirError = function (f, er) {
+ // handle errors, and cache the information
+ switch (er.code) {
+ case 'ENOTSUP': // https://github.com/isaacs/node-glob/issues/205
+ case 'ENOTDIR': // totally normal. means it *does* exist.
+ this.cache[this._makeAbs(f)] = 'FILE'
+ break
+
+ case 'ENOENT': // not terribly unusual
+ case 'ELOOP':
+ case 'ENAMETOOLONG':
+ case 'UNKNOWN':
+ this.cache[this._makeAbs(f)] = false
+ break
+
+ default: // some unusual error. Treat as failure.
+ this.cache[this._makeAbs(f)] = false
+ if (this.strict)
+ throw er
+ if (!this.silent)
+ console.error('glob error', er)
+ break
+ }
+}
+
+GlobSync.prototype._processGlobStar = function (prefix, read, abs, remain, index, inGlobStar) {
+
+ var entries = this._readdir(abs, inGlobStar)
+
+ // no entries means not a dir, so it can never have matches
+ // foo.txt/** doesn't match foo.txt
+ if (!entries)
+ return
+
+ // test without the globstar, and with every child both below
+ // and replacing the globstar.
+ var remainWithoutGlobStar = remain.slice(1)
+ var gspref = prefix ? [ prefix ] : []
+ var noGlobStar = gspref.concat(remainWithoutGlobStar)
+
+ // the noGlobStar pattern exits the inGlobStar state
+ this._process(noGlobStar, index, false)
+
+ var len = entries.length
+ var isSym = this.symlinks[abs]
+
+ // If it's a symlink, and we're in a globstar, then stop
+ if (isSym && inGlobStar)
+ return
+
+ for (var i = 0; i < len; i++) {
+ var e = entries[i]
+ if (e.charAt(0) === '.' && !this.dot)
+ continue
+
+ // these two cases enter the inGlobStar state
+ var instead = gspref.concat(entries[i], remainWithoutGlobStar)
+ this._process(instead, index, true)
+
+ var below = gspref.concat(entries[i], remain)
+ this._process(below, index, true)
+ }
+}
+
+GlobSync.prototype._processSimple = function (prefix, index) {
+ // XXX review this. Shouldn't it be doing the mounting etc
+ // before doing stat? kinda weird?
+ var exists = this._stat(prefix)
+
+ if (!this.matches[index])
+ this.matches[index] = Object.create(null)
+
+ // If it doesn't exist, then just mark the lack of results
+ if (!exists)
+ return
+
+ if (prefix && isAbsolute(prefix) && !this.nomount) {
+ var trail = /[\/\\]$/.test(prefix)
+ if (prefix.charAt(0) === '/') {
+ prefix = path.join(this.root, prefix)
+ } else {
+ prefix = path.resolve(this.root, prefix)
+ if (trail)
+ prefix += '/'
+ }
+ }
+
+ if (process.platform === 'win32')
+ prefix = prefix.replace(/\\/g, '/')
+
+ // Mark this as a match
+ this.matches[index][prefix] = true
+}
+
+// Returns either 'DIR', 'FILE', or false
+GlobSync.prototype._stat = function (f) {
+ var abs = this._makeAbs(f)
+ var needDir = f.slice(-1) === '/'
+
+ if (f.length > this.maxLength)
+ return false
+
+ if (!this.stat && ownProp(this.cache, abs)) {
+ var c = this.cache[abs]
+
+ if (Array.isArray(c))
+ c = 'DIR'
+
+ // It exists, but maybe not how we need it
+ if (!needDir || c === 'DIR')
+ return c
+
+ if (needDir && c === 'FILE')
+ return false
+
+ // otherwise we have to stat, because maybe c=true
+ // if we know it exists, but not what it is.
+ }
+
+ var exists
+ var stat = this.statCache[abs]
+ if (!stat) {
+ var lstat
+ try {
+ lstat = fs.lstatSync(abs)
+ } catch (er) {
+ return false
+ }
+
+ if (lstat.isSymbolicLink()) {
+ try {
+ stat = fs.statSync(abs)
+ } catch (er) {
+ stat = lstat
+ }
+ } else {
+ stat = lstat
+ }
+ }
+
+ this.statCache[abs] = stat
+
+ var c = stat.isDirectory() ? 'DIR' : 'FILE'
+ this.cache[abs] = this.cache[abs] || c
+
+ if (needDir && c !== 'DIR')
+ return false
+
+ return c
+}
+
+GlobSync.prototype._mark = function (p) {
+ return common.mark(this, p)
+}
+
+GlobSync.prototype._makeAbs = function (f) {
+ return common.makeAbs(this, f)
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/.npmignore
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/.npmignore b/node_modules/cordova-common/node_modules/osenv/.npmignore
new file mode 100644
index 0000000..8c23dee
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/.npmignore
@@ -0,0 +1,13 @@
+*.swp
+.*.swp
+
+.DS_Store
+*~
+.project
+.settings
+npm-debug.log
+coverage.html
+.idea
+lib-cov
+
+node_modules
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/.travis.yml b/node_modules/cordova-common/node_modules/osenv/.travis.yml
new file mode 100644
index 0000000..99f2bbf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/.travis.yml
@@ -0,0 +1,9 @@
+language: node_js
+language: node_js
+node_js:
+ - '0.8'
+ - '0.10'
+ - '0.12'
+ - 'iojs'
+before_install:
+ - npm install -g npm@latest
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/LICENSE b/node_modules/cordova-common/node_modules/osenv/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/README.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/README.md b/node_modules/cordova-common/node_modules/osenv/README.md
new file mode 100644
index 0000000..08fd900
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/README.md
@@ -0,0 +1,63 @@
+# osenv
+
+Look up environment settings specific to different operating systems.
+
+## Usage
+
+```javascript
+var osenv = require('osenv')
+var path = osenv.path()
+var user = osenv.user()
+// etc.
+
+// Some things are not reliably in the env, and have a fallback command:
+var h = osenv.hostname(function (er, hostname) {
+ h = hostname
+})
+// This will still cause it to be memoized, so calling osenv.hostname()
+// is now an immediate operation.
+
+// You can always send a cb, which will get called in the nextTick
+// if it's been memoized, or wait for the fallback data if it wasn't
+// found in the environment.
+osenv.hostname(function (er, hostname) {
+ if (er) console.error('error looking up hostname')
+ else console.log('this machine calls itself %s', hostname)
+})
+```
+
+## osenv.hostname()
+
+The machine name. Calls `hostname` if not found.
+
+## osenv.user()
+
+The currently logged-in user. Calls `whoami` if not found.
+
+## osenv.prompt()
+
+Either PS1 on unix, or PROMPT on Windows.
+
+## osenv.tmpdir()
+
+The place where temporary files should be created.
+
+## osenv.home()
+
+No place like it.
+
+## osenv.path()
+
+An array of the places that the operating system will search for
+executables.
+
+## osenv.editor()
+
+Return the executable name of the editor program. This uses the EDITOR
+and VISUAL environment variables, and falls back to `vi` on Unix, or
+`notepad.exe` on Windows.
+
+## osenv.shell()
+
+The SHELL on Unix, which Windows calls the ComSpec. Defaults to 'bash'
+or 'cmd'.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/index.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/index.js b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/index.js
new file mode 100644
index 0000000..3306616
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/index.js
@@ -0,0 +1,24 @@
+'use strict';
+var os = require('os');
+
+function homedir() {
+ var env = process.env;
+ var home = env.HOME;
+ var user = env.LOGNAME || env.USER || env.LNAME || env.USERNAME;
+
+ if (process.platform === 'win32') {
+ return env.USERPROFILE || env.HOMEDRIVE + env.HOMEPATH || home || null;
+ }
+
+ if (process.platform === 'darwin') {
+ return home || (user ? '/Users/' + user : null);
+ }
+
+ if (process.platform === 'linux') {
+ return home || (process.getuid() === 0 ? '/root' : (user ? '/home/' + user : null));
+ }
+
+ return home || null;
+}
+
+module.exports = typeof os.homedir === 'function' ? os.homedir : homedir;
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/license
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/license b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus <si...@gmail.com> (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/package.json b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/package.json
new file mode 100644
index 0000000..37698c4
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/package.json
@@ -0,0 +1,70 @@
+{
+ "name": "os-homedir",
+ "version": "1.0.1",
+ "description": "io.js 2.3.0 os.homedir() ponyfill",
+ "license": "MIT",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/os-homedir.git"
+ },
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "files": [
+ "index.js"
+ ],
+ "keywords": [
+ "built-in",
+ "core",
+ "ponyfill",
+ "polyfill",
+ "shim",
+ "os",
+ "homedir",
+ "home",
+ "dir",
+ "directory",
+ "folder",
+ "user",
+ "path"
+ ],
+ "devDependencies": {
+ "ava": "0.0.4",
+ "path-exists": "^1.0.0"
+ },
+ "gitHead": "13ff83fbd13ebe286a6092286b2c634ab4534c5f",
+ "bugs": {
+ "url": "https://github.com/sindresorhus/os-homedir/issues"
+ },
+ "homepage": "https://github.com/sindresorhus/os-homedir",
+ "_id": "os-homedir@1.0.1",
+ "_shasum": "0d62bdf44b916fd3bbdcf2cab191948fb094f007",
+ "_from": "os-homedir@>=1.0.0 <2.0.0",
+ "_npmVersion": "2.11.2",
+ "_nodeVersion": "0.12.5",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "dist": {
+ "shasum": "0d62bdf44b916fd3bbdcf2cab191948fb094f007",
+ "tarball": "http://registry.npmjs.org/os-homedir/-/os-homedir-1.0.1.tgz"
+ },
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/os-homedir/-/os-homedir-1.0.1.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/readme.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/readme.md b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/readme.md
new file mode 100644
index 0000000..4851f10
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-homedir/readme.md
@@ -0,0 +1,33 @@
+# os-homedir [](https://travis-ci.org/sindresorhus/os-homedir)
+
+> io.js 2.3.0 [`os.homedir()`](https://iojs.org/api/os.html#os_os_homedir) ponyfill
+
+> Ponyfill: A polyfill that doesn't overwrite the native method
+
+
+## Install
+
+```
+$ npm install --save os-homedir
+```
+
+
+## Usage
+
+```js
+var osHomedir = require('os-homedir');
+
+console.log(osHomedir());
+//=> /Users/sindresorhus
+```
+
+
+## Related
+
+- [user-home](https://github.com/sindresorhus/user-home) - Same as this module but caches the result
+- [home-or-tmp](https://github.com/sindresorhus/home-or-tmp) - Get the user home directory with fallback to the system temp directory
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/index.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/index.js b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/index.js
new file mode 100644
index 0000000..52d90bf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/index.js
@@ -0,0 +1,25 @@
+'use strict';
+var isWindows = process.platform === 'win32';
+var trailingSlashRe = isWindows ? /[^:]\\$/ : /.\/$/;
+
+// https://github.com/nodejs/io.js/blob/3e7a14381497a3b73dda68d05b5130563cdab420/lib/os.js#L25-L43
+module.exports = function () {
+ var path;
+
+ if (isWindows) {
+ path = process.env.TEMP ||
+ process.env.TMP ||
+ (process.env.SystemRoot || process.env.windir) + '\\temp';
+ } else {
+ path = process.env.TMPDIR ||
+ process.env.TMP ||
+ process.env.TEMP ||
+ '/tmp';
+ }
+
+ if (trailingSlashRe.test(path)) {
+ path = path.slice(0, -1);
+ }
+
+ return path;
+};
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/license
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/license b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus <si...@gmail.com> (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/package.json b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/package.json
new file mode 100644
index 0000000..1857f8f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/package.json
@@ -0,0 +1,70 @@
+{
+ "name": "os-tmpdir",
+ "version": "1.0.1",
+ "description": "Node.js os.tmpdir() ponyfill",
+ "license": "MIT",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/os-tmpdir.git"
+ },
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "files": [
+ "index.js"
+ ],
+ "keywords": [
+ "built-in",
+ "core",
+ "ponyfill",
+ "polyfill",
+ "shim",
+ "os",
+ "tmpdir",
+ "tempdir",
+ "tmp",
+ "temp",
+ "dir",
+ "directory",
+ "env",
+ "environment"
+ ],
+ "devDependencies": {
+ "ava": "0.0.4"
+ },
+ "gitHead": "5c5d355f81378980db629d60128ad03e02b1c1e5",
+ "bugs": {
+ "url": "https://github.com/sindresorhus/os-tmpdir/issues"
+ },
+ "homepage": "https://github.com/sindresorhus/os-tmpdir",
+ "_id": "os-tmpdir@1.0.1",
+ "_shasum": "e9b423a1edaf479882562e92ed71d7743a071b6e",
+ "_from": "os-tmpdir@>=1.0.0 <2.0.0",
+ "_npmVersion": "2.9.1",
+ "_nodeVersion": "0.12.3",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "dist": {
+ "shasum": "e9b423a1edaf479882562e92ed71d7743a071b6e",
+ "tarball": "http://registry.npmjs.org/os-tmpdir/-/os-tmpdir-1.0.1.tgz"
+ },
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "directories": {},
+ "_resolved": "https://registry.npmjs.org/os-tmpdir/-/os-tmpdir-1.0.1.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/readme.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/readme.md b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/readme.md
new file mode 100644
index 0000000..54d4c6e
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/node_modules/os-tmpdir/readme.md
@@ -0,0 +1,36 @@
+# os-tmpdir [](https://travis-ci.org/sindresorhus/os-tmpdir)
+
+> Node.js [`os.tmpdir()`](https://nodejs.org/api/os.html#os_os_tmpdir) ponyfill
+
+> Ponyfill: A polyfill that doesn't overwrite the native method
+
+Use this instead of `require('os').tmpdir()` to get a consistent behaviour on different Node.js versions (even 0.8).
+
+*This is actually taken from io.js 2.0.2 as it contains some fixes that haven't bubbled up to Node.js yet.*
+
+
+## Install
+
+```
+$ npm install --save os-tmpdir
+```
+
+
+## Usage
+
+```js
+var osTmpdir = require('os-tmpdir');
+
+osTmpdir();
+//=> /var/folders/m3/5574nnhn0yj488ccryqr7tc80000gn/T
+```
+
+
+## API
+
+See the [`os.tmpdir()` docs](https://nodejs.org/api/os.html#os_os_tmpdir).
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/osenv.js
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/osenv.js b/node_modules/cordova-common/node_modules/osenv/osenv.js
new file mode 100644
index 0000000..702a95b
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/osenv.js
@@ -0,0 +1,72 @@
+var isWindows = process.platform === 'win32'
+var path = require('path')
+var exec = require('child_process').exec
+var osTmpdir = require('os-tmpdir')
+var osHomedir = require('os-homedir')
+
+// looking up envs is a bit costly.
+// Also, sometimes we want to have a fallback
+// Pass in a callback to wait for the fallback on failures
+// After the first lookup, always returns the same thing.
+function memo (key, lookup, fallback) {
+ var fell = false
+ var falling = false
+ exports[key] = function (cb) {
+ var val = lookup()
+ if (!val && !fell && !falling && fallback) {
+ fell = true
+ falling = true
+ exec(fallback, function (er, output, stderr) {
+ falling = false
+ if (er) return // oh well, we tried
+ val = output.trim()
+ })
+ }
+ exports[key] = function (cb) {
+ if (cb) process.nextTick(cb.bind(null, null, val))
+ return val
+ }
+ if (cb && !falling) process.nextTick(cb.bind(null, null, val))
+ return val
+ }
+}
+
+memo('user', function () {
+ return ( isWindows
+ ? process.env.USERDOMAIN + '\\' + process.env.USERNAME
+ : process.env.USER
+ )
+}, 'whoami')
+
+memo('prompt', function () {
+ return isWindows ? process.env.PROMPT : process.env.PS1
+})
+
+memo('hostname', function () {
+ return isWindows ? process.env.COMPUTERNAME : process.env.HOSTNAME
+}, 'hostname')
+
+memo('tmpdir', function () {
+ return osTmpdir()
+})
+
+memo('home', function () {
+ return osHomedir()
+})
+
+memo('path', function () {
+ return (process.env.PATH ||
+ process.env.Path ||
+ process.env.path).split(isWindows ? ';' : ':')
+})
+
+memo('editor', function () {
+ return process.env.EDITOR ||
+ process.env.VISUAL ||
+ (isWindows ? 'notepad.exe' : 'vi')
+})
+
+memo('shell', function () {
+ return isWindows ? process.env.ComSpec || 'cmd'
+ : process.env.SHELL || 'bash'
+})
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/package.json
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/package.json b/node_modules/cordova-common/node_modules/osenv/package.json
new file mode 100644
index 0000000..089fe1f
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/package.json
@@ -0,0 +1,76 @@
+{
+ "name": "osenv",
+ "version": "0.1.3",
+ "main": "osenv.js",
+ "directories": {
+ "test": "test"
+ },
+ "dependencies": {
+ "os-homedir": "^1.0.0",
+ "os-tmpdir": "^1.0.0"
+ },
+ "devDependencies": {
+ "tap": "^1.2.0"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/npm/osenv.git"
+ },
+ "keywords": [
+ "environment",
+ "variable",
+ "home",
+ "tmpdir",
+ "path",
+ "prompt",
+ "ps1"
+ ],
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me/"
+ },
+ "license": "ISC",
+ "description": "Look up environment settings specific to different operating systems",
+ "gitHead": "f746b3405d8f9e28054d11b97e1436f6a15016c4",
+ "bugs": {
+ "url": "https://github.com/npm/osenv/issues"
+ },
+ "homepage": "https://github.com/npm/osenv#readme",
+ "_id": "osenv@0.1.3",
+ "_shasum": "83cf05c6d6458fc4d5ac6362ea325d92f2754217",
+ "_from": "osenv@>=0.1.3 <0.2.0",
+ "_npmVersion": "3.0.0",
+ "_nodeVersion": "2.2.1",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ },
+ "dist": {
+ "shasum": "83cf05c6d6458fc4d5ac6362ea325d92f2754217",
+ "tarball": "http://registry.npmjs.org/osenv/-/osenv-0.1.3.tgz"
+ },
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ {
+ "name": "robertkowalski",
+ "email": "rok@kowalski.gd"
+ },
+ {
+ "name": "othiym23",
+ "email": "ogd@aoaioxxysz.net"
+ },
+ {
+ "name": "iarna",
+ "email": "me@re-becca.org"
+ }
+ ],
+ "_resolved": "https://registry.npmjs.org/osenv/-/osenv-0.1.3.tgz",
+ "readme": "ERROR: No README data found!"
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/osenv/x.tap
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/osenv/x.tap b/node_modules/cordova-common/node_modules/osenv/x.tap
new file mode 100644
index 0000000..90d8472
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/osenv/x.tap
@@ -0,0 +1,39 @@
+TAP version 13
+ # Subtest: test/unix.js
+ TAP version 13
+ # Subtest: basic unix sanity test
+ ok 1 - should be equal
+ ok 2 - should be equal
+ ok 3 - should be equal
+ ok 4 - should be equivalent
+ ok 5 - should be equal
+ ok 6 - should be equal
+ ok 7 - should be equal
+ ok 8 - should be equal
+ ok 9 - should be equal
+ ok 10 - should be equal
+ ok 11 - should be equal
+ ok 12 - should be equal
+ ok 13 - should be equal
+ ok 14 - should be equal
+ 1..14
+ ok 1 - basic unix sanity test # time=10.712ms
+
+ 1..1
+ # time=18.422ms
+ok 1 - test/unix.js # time=169.827ms
+
+ # Subtest: test/windows.js
+ TAP version 13
+ 1..0 # Skip windows tests, this is not windows
+
+ok 2 - test/windows.js # SKIP Skip windows tests, this is not windows
+
+ # Subtest: test/nada.js
+ TAP version 13
+ 1..0
+
+ok 2 - test/nada.js
+
+1..3
+# time=274.247ms
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/plist/.jshintrc
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/plist/.jshintrc b/node_modules/cordova-common/node_modules/plist/.jshintrc
new file mode 100644
index 0000000..3f42622
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/plist/.jshintrc
@@ -0,0 +1,4 @@
+{
+ "laxbreak": true,
+ "laxcomma": true
+}
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/plist/.travis.yml
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/plist/.travis.yml b/node_modules/cordova-common/node_modules/plist/.travis.yml
new file mode 100644
index 0000000..4ed5002
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/plist/.travis.yml
@@ -0,0 +1,32 @@
+language: node_js
+node_js:
+- '0.10'
+- '0.11'
+env:
+ global:
+ - secure: xlLmWO7akYQjmDgrv6/b/ZMGILF8FReD+k6A/u8pYRD2JW29hhwvRwIQGcKp9+zmJdn4i5M4D1/qJkCeI3pdhAYBDHvzHOHSEwLJz1ESB2Crv6fa69CtpIufQkWvIxmZoU49tCaLpMBaIroGihJ4DAXdIVOIz6Ur9vXLDhGsE4c=
+ - secure: aQ46RdxL10xR5ZJJTMUKdH5k4tdrzgZ87nlwHC+pTr6bfRw3UKYC+6Rm7yQpg9wq0Io9O9dYCP007gQGSWstbjr1+jXNu/ubtNG+q5cpWBQZZZ013VHh9QJTf1MnetsZxbv8Yhrjg590s6vruT0oqesOnB2CizO/BsKxnY37Nos=
+matrix:
+ include:
+ - node_js: '0.10'
+ env: BROWSER_NAME=chrome BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=chrome BROWSER_VERSION=29
+ - node_js: '0.10'
+ env: BROWSER_NAME=firefox BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=opera BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=latest
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=7
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=6
+ - node_js: '0.10'
+ env: BROWSER_NAME=safari BROWSER_VERSION=5
+ - node_js: '0.10'
+ env: BROWSER_NAME=ie BROWSER_VERSION=11
+ - node_js: '0.10'
+ env: BROWSER_NAME=ie BROWSER_VERSION=10
+ - node_js: '0.10'
+ env: BROWSER_NAME=ie BROWSER_VERSION=9
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/plist/History.md
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/plist/History.md b/node_modules/cordova-common/node_modules/plist/History.md
new file mode 100644
index 0000000..dbcf0d6
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/plist/History.md
@@ -0,0 +1,112 @@
+
+1.1.0 / 2014-08-27
+==================
+
+ * package: update "browserify" to v5.10.1
+ * package: update "zuul" to v1.10.2
+ * README: add "Sauce Test Status" build badge
+ * travis: use new "plistjs" sauce credentials
+ * travis: set up zuul saucelabs automated testing
+
+1.0.1 / 2014-06-25
+==================
+
+ * add .zuul.yml file for browser testing
+ * remove Testling stuff
+ * build: fix global variable `val` leak
+ * package: use --check-leaks when running mocha tests
+ * README: update examples to use preferred API
+ * package: add "browser" keyword
+
+1.0.0 / 2014-05-20
+==================
+
+ * package: remove "android-browser"
+ * test: add <dict> build() test
+ * test: re-add the empty string build() test
+ * test: remove "fixtures" and legacy "tests" dir
+ * test: add some more build() tests
+ * test: add a parse() CDATA test
+ * test: starting on build() tests
+ * test: more parse() tests
+ * package: attempt to fix "android-browser" testling
+ * parse: better <data> with newline handling
+ * README: add Testling badge
+ * test: add <data> node tests
+ * test: add a <date> parse() test
+ * travis: don't test node v0.6 or v0.8
+ * test: some more parse() tests
+ * test: add simple <string> parsing test
+ * build: add support for an optional "opts" object
+ * package: test mobile devices
+ * test: use multiline to inline the XML
+ * package: beautify
+ * package: fix "mocha" harness
+ * package: more testling browsers
+ * build: add the "version=1.0" attribute
+ * beginnings of "mocha" tests
+ * build: more JSDocs
+ * tests: add test that ensures that empty string conversion works
+ * build: update "xmlbuilder" to v2.2.1
+ * parse: ignore comment and cdata nodes
+ * tests: make the "Newlines" test actually contain a newline
+ * parse: lint
+ * test travis
+ * README: add Travis CI badge
+ * add .travis.yml file
+ * build: updated DTD to reflect name change
+ * parse: return falsey values in an Array plist
+ * build: fix encoding a typed array in the browser
+ * build: add support for Typed Arrays and ArrayBuffers
+ * build: more lint
+ * build: slight cleanup and optimizations
+ * build: use .txt() for the "date" value
+ * parse: always return a Buffer for <data> nodes
+ * build: don't interpret Strings as base64
+ * dist: commit prebuilt plist*.js files
+ * parse: fix typo in deprecate message
+ * parse: fix parse() return value
+ * parse: add jsdoc comments for the deprecated APIs
+ * parse: add `parse()` function
+ * node, parse: use `util-deprecate` module
+ * re-implemented parseFile to be asynchronous
+ * node: fix jsdoc comment
+ * Makefile: fix "node" require stubbing
+ * examples: add "browser" example
+ * package: tweak "main"
+ * package: remove "engines" field
+ * Makefile: fix --exclude command for browserify
+ * package: update "description"
+ * lib: more styling
+ * Makefile: add -build.js and -parse.js dist files
+ * lib: separate out the parse and build logic into their own files
+ * Makefile: add makefile with browserify build rules
+ * package: add "browserify" as a dev dependency
+ * plist: tabs to spaces (again)
+ * add a .jshintrc file
+ * LICENSE: update
+ * node-webkit support
+ * Ignore tests/ in .npmignore file
+ * Remove duplicate devDependencies key
+ * Remove trailing whitespace
+ * adding recent contributors. Bumping npm package number (patch release)
+ * Fixed node.js string handling
+ * bumping version number
+ * Fixed global variable plist leak
+ * patch release 0.4.1
+ * removed temporary debug output file
+ * flipping the cases for writing data and string elements in build(). removed the 125 length check. Added validation of base64 encoding for data fields when parsing. added unit tests.
+ * fixed syntax errors in README examples (issue #20)
+ * added Sync versions of calls. added deprecation warnings for old method calls. updated documentation. If the resulting object from parseStringSync is an array with 1 element, return just the element. If a plist string or file doesnt have a <plist> tag as the document root element, fail noisily (issue #15)
+ * incrementing package version
+ * added cross platform base64 encode/decode for data elements (issue #17.) Comments and hygiene.
+ * refactored the code to use a DOM parser instead of SAX. closes issues #5 and #16
+ * rolling up package version
+ * updated base64 detection regexp. updated README. hygiene.
+ * refactored the build function. Fixes issue #14
+ * refactored tests. Modified tests from issue #9. thanks @sylvinus
+ * upgrade xmlbuilder package version. this is why .end() was needed in last commit; breaking change to xmlbuilder lib. :/
+ * bug fix in build function, forgot to call .end() Refactored tests to use nodeunit
+ * Implemented support for real, identity tests
+ * Refactored base64 detection - still sloppy, fixed date building. Passing tests OK.
+ * Implemented basic plist builder that turns an existing JS object into plist XML. date, real and data types still need to be implemented.
http://git-wip-us.apache.org/repos/asf/cordova-android/blob/ab72e484/node_modules/cordova-common/node_modules/plist/LICENSE
----------------------------------------------------------------------
diff --git a/node_modules/cordova-common/node_modules/plist/LICENSE b/node_modules/cordova-common/node_modules/plist/LICENSE
new file mode 100644
index 0000000..04a9e91
--- /dev/null
+++ b/node_modules/cordova-common/node_modules/plist/LICENSE
@@ -0,0 +1,24 @@
+(The MIT License)
+
+Copyright (c) 2010-2014 Nathan Rajlich <na...@tootallnate.net>
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
---------------------------------------------------------------------
To unsubscribe, e-mail: commits-unsubscribe@cordova.apache.org
For additional commands, e-mail: commits-help@cordova.apache.org